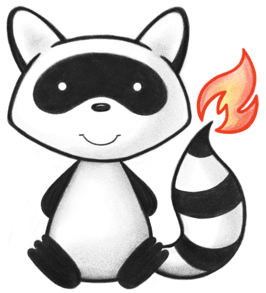
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 052 */ 053@ResourceDef(name="MedicationRequest", profile="http://hl7.org/fhir/StructureDefinition/MedicationRequest") 054public class MedicationRequest extends DomainResource { 055 056 public enum MedicationRequestIntent { 057 /** 058 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 059 */ 060 PROPOSAL, 061 /** 062 * The request represents an intention to ensure something occurs without providing an authorization for others to act. 063 */ 064 PLAN, 065 /** 066 * The request represents a request/demand and authorization for action 067 */ 068 ORDER, 069 /** 070 * The request represents the original authorization for the medication request. 071 */ 072 ORIGINALORDER, 073 /** 074 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization.. 075 */ 076 REFLEXORDER, 077 /** 078 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order. 079 */ 080 FILLERORDER, 081 /** 082 * The request represents an instance for the particular order and is used to generate a schedule of requests on a medication administration record (MAR). 083 */ 084 INSTANCEORDER, 085 /** 086 * The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. 087 */ 088 OPTION, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 public static MedicationRequestIntent fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("proposal".equals(codeString)) 097 return PROPOSAL; 098 if ("plan".equals(codeString)) 099 return PLAN; 100 if ("order".equals(codeString)) 101 return ORDER; 102 if ("original-order".equals(codeString)) 103 return ORIGINALORDER; 104 if ("reflex-order".equals(codeString)) 105 return REFLEXORDER; 106 if ("filler-order".equals(codeString)) 107 return FILLERORDER; 108 if ("instance-order".equals(codeString)) 109 return INSTANCEORDER; 110 if ("option".equals(codeString)) 111 return OPTION; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown MedicationRequestIntent code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case PROPOSAL: return "proposal"; 120 case PLAN: return "plan"; 121 case ORDER: return "order"; 122 case ORIGINALORDER: return "original-order"; 123 case REFLEXORDER: return "reflex-order"; 124 case FILLERORDER: return "filler-order"; 125 case INSTANCEORDER: return "instance-order"; 126 case OPTION: return "option"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getSystem() { 132 switch (this) { 133 case PROPOSAL: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 134 case PLAN: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 135 case ORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 136 case ORIGINALORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 137 case REFLEXORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 138 case FILLERORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 139 case INSTANCEORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 140 case OPTION: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getDefinition() { 146 switch (this) { 147 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 148 case PLAN: return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 149 case ORDER: return "The request represents a request/demand and authorization for action"; 150 case ORIGINALORDER: return "The request represents the original authorization for the medication request."; 151 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization.."; 152 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 153 case INSTANCEORDER: return "The request represents an instance for the particular order and is used to generate a schedule of requests on a medication administration record (MAR)."; 154 case OPTION: return "The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests."; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 public String getDisplay() { 160 switch (this) { 161 case PROPOSAL: return "Proposal"; 162 case PLAN: return "Plan"; 163 case ORDER: return "Order"; 164 case ORIGINALORDER: return "Original Order"; 165 case REFLEXORDER: return "Reflex Order"; 166 case FILLERORDER: return "Filler Order"; 167 case INSTANCEORDER: return "Instance Order"; 168 case OPTION: return "Option"; 169 case NULL: return null; 170 default: return "?"; 171 } 172 } 173 } 174 175 public static class MedicationRequestIntentEnumFactory implements EnumFactory<MedicationRequestIntent> { 176 public MedicationRequestIntent fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("proposal".equals(codeString)) 181 return MedicationRequestIntent.PROPOSAL; 182 if ("plan".equals(codeString)) 183 return MedicationRequestIntent.PLAN; 184 if ("order".equals(codeString)) 185 return MedicationRequestIntent.ORDER; 186 if ("original-order".equals(codeString)) 187 return MedicationRequestIntent.ORIGINALORDER; 188 if ("reflex-order".equals(codeString)) 189 return MedicationRequestIntent.REFLEXORDER; 190 if ("filler-order".equals(codeString)) 191 return MedicationRequestIntent.FILLERORDER; 192 if ("instance-order".equals(codeString)) 193 return MedicationRequestIntent.INSTANCEORDER; 194 if ("option".equals(codeString)) 195 return MedicationRequestIntent.OPTION; 196 throw new IllegalArgumentException("Unknown MedicationRequestIntent code '"+codeString+"'"); 197 } 198 public Enumeration<MedicationRequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.NULL, code); 203 String codeString = ((PrimitiveType) code).asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.NULL, code); 206 if ("proposal".equals(codeString)) 207 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PROPOSAL, code); 208 if ("plan".equals(codeString)) 209 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PLAN, code); 210 if ("order".equals(codeString)) 211 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.ORDER, code); 212 if ("original-order".equals(codeString)) 213 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.ORIGINALORDER, code); 214 if ("reflex-order".equals(codeString)) 215 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.REFLEXORDER, code); 216 if ("filler-order".equals(codeString)) 217 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.FILLERORDER, code); 218 if ("instance-order".equals(codeString)) 219 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.INSTANCEORDER, code); 220 if ("option".equals(codeString)) 221 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.OPTION, code); 222 throw new FHIRException("Unknown MedicationRequestIntent code '"+codeString+"'"); 223 } 224 public String toCode(MedicationRequestIntent code) { 225 if (code == MedicationRequestIntent.PROPOSAL) 226 return "proposal"; 227 if (code == MedicationRequestIntent.PLAN) 228 return "plan"; 229 if (code == MedicationRequestIntent.ORDER) 230 return "order"; 231 if (code == MedicationRequestIntent.ORIGINALORDER) 232 return "original-order"; 233 if (code == MedicationRequestIntent.REFLEXORDER) 234 return "reflex-order"; 235 if (code == MedicationRequestIntent.FILLERORDER) 236 return "filler-order"; 237 if (code == MedicationRequestIntent.INSTANCEORDER) 238 return "instance-order"; 239 if (code == MedicationRequestIntent.OPTION) 240 return "option"; 241 return "?"; 242 } 243 public String toSystem(MedicationRequestIntent code) { 244 return code.getSystem(); 245 } 246 } 247 248 public enum MedicationrequestStatus { 249 /** 250 * The request is 'actionable', but not all actions that are implied by it have occurred yet. 251 */ 252 ACTIVE, 253 /** 254 * Actions implied by the request are to be temporarily halted. The request might or might not be resumed. May also be called 'suspended'. 255 */ 256 ONHOLD, 257 /** 258 * The request is no longer active and the subject should no longer be taking the medication. 259 */ 260 ENDED, 261 /** 262 * Actions implied by the request are to be permanently halted, before all of the administrations occurred. This should not be used if the original order was entered in error 263 */ 264 STOPPED, 265 /** 266 * All actions that are implied by the request have occurred. 267 */ 268 COMPLETED, 269 /** 270 * The request has been withdrawn before any administrations have occurred 271 */ 272 CANCELLED, 273 /** 274 * The request was recorded against the wrong patient or for some reason should not have been recorded (e.g. wrong medication, wrong dose, etc.). Some of the actions that are implied by the medication request may have occurred. For example, the medication may have been dispensed and the patient may have taken some of the medication. 275 */ 276 ENTEREDINERROR, 277 /** 278 * The request is not yet 'actionable', e.g. it is a work in progress, requires sign-off, verification or needs to be run through decision support process. 279 */ 280 DRAFT, 281 /** 282 * The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 283 */ 284 UNKNOWN, 285 /** 286 * added to help the parsers with the generic types 287 */ 288 NULL; 289 public static MedicationrequestStatus fromCode(String codeString) throws FHIRException { 290 if (codeString == null || "".equals(codeString)) 291 return null; 292 if ("active".equals(codeString)) 293 return ACTIVE; 294 if ("on-hold".equals(codeString)) 295 return ONHOLD; 296 if ("ended".equals(codeString)) 297 return ENDED; 298 if ("stopped".equals(codeString)) 299 return STOPPED; 300 if ("completed".equals(codeString)) 301 return COMPLETED; 302 if ("cancelled".equals(codeString)) 303 return CANCELLED; 304 if ("entered-in-error".equals(codeString)) 305 return ENTEREDINERROR; 306 if ("draft".equals(codeString)) 307 return DRAFT; 308 if ("unknown".equals(codeString)) 309 return UNKNOWN; 310 if (Configuration.isAcceptInvalidEnums()) 311 return null; 312 else 313 throw new FHIRException("Unknown MedicationrequestStatus code '"+codeString+"'"); 314 } 315 public String toCode() { 316 switch (this) { 317 case ACTIVE: return "active"; 318 case ONHOLD: return "on-hold"; 319 case ENDED: return "ended"; 320 case STOPPED: return "stopped"; 321 case COMPLETED: return "completed"; 322 case CANCELLED: return "cancelled"; 323 case ENTEREDINERROR: return "entered-in-error"; 324 case DRAFT: return "draft"; 325 case UNKNOWN: return "unknown"; 326 case NULL: return null; 327 default: return "?"; 328 } 329 } 330 public String getSystem() { 331 switch (this) { 332 case ACTIVE: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 333 case ONHOLD: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 334 case ENDED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 335 case STOPPED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 336 case COMPLETED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 337 case CANCELLED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 338 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 339 case DRAFT: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 340 case UNKNOWN: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 341 case NULL: return null; 342 default: return "?"; 343 } 344 } 345 public String getDefinition() { 346 switch (this) { 347 case ACTIVE: return "The request is 'actionable', but not all actions that are implied by it have occurred yet."; 348 case ONHOLD: return "Actions implied by the request are to be temporarily halted. The request might or might not be resumed. May also be called 'suspended'."; 349 case ENDED: return "The request is no longer active and the subject should no longer be taking the medication."; 350 case STOPPED: return "Actions implied by the request are to be permanently halted, before all of the administrations occurred. This should not be used if the original order was entered in error"; 351 case COMPLETED: return "All actions that are implied by the request have occurred."; 352 case CANCELLED: return "The request has been withdrawn before any administrations have occurred"; 353 case ENTEREDINERROR: return "The request was recorded against the wrong patient or for some reason should not have been recorded (e.g. wrong medication, wrong dose, etc.). Some of the actions that are implied by the medication request may have occurred. For example, the medication may have been dispensed and the patient may have taken some of the medication."; 354 case DRAFT: return "The request is not yet 'actionable', e.g. it is a work in progress, requires sign-off, verification or needs to be run through decision support process."; 355 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 356 case NULL: return null; 357 default: return "?"; 358 } 359 } 360 public String getDisplay() { 361 switch (this) { 362 case ACTIVE: return "Active"; 363 case ONHOLD: return "On Hold"; 364 case ENDED: return "Ended"; 365 case STOPPED: return "Stopped"; 366 case COMPLETED: return "Completed"; 367 case CANCELLED: return "Cancelled"; 368 case ENTEREDINERROR: return "Entered in Error"; 369 case DRAFT: return "Draft"; 370 case UNKNOWN: return "Unknown"; 371 case NULL: return null; 372 default: return "?"; 373 } 374 } 375 } 376 377 public static class MedicationrequestStatusEnumFactory implements EnumFactory<MedicationrequestStatus> { 378 public MedicationrequestStatus fromCode(String codeString) throws IllegalArgumentException { 379 if (codeString == null || "".equals(codeString)) 380 if (codeString == null || "".equals(codeString)) 381 return null; 382 if ("active".equals(codeString)) 383 return MedicationrequestStatus.ACTIVE; 384 if ("on-hold".equals(codeString)) 385 return MedicationrequestStatus.ONHOLD; 386 if ("ended".equals(codeString)) 387 return MedicationrequestStatus.ENDED; 388 if ("stopped".equals(codeString)) 389 return MedicationrequestStatus.STOPPED; 390 if ("completed".equals(codeString)) 391 return MedicationrequestStatus.COMPLETED; 392 if ("cancelled".equals(codeString)) 393 return MedicationrequestStatus.CANCELLED; 394 if ("entered-in-error".equals(codeString)) 395 return MedicationrequestStatus.ENTEREDINERROR; 396 if ("draft".equals(codeString)) 397 return MedicationrequestStatus.DRAFT; 398 if ("unknown".equals(codeString)) 399 return MedicationrequestStatus.UNKNOWN; 400 throw new IllegalArgumentException("Unknown MedicationrequestStatus code '"+codeString+"'"); 401 } 402 public Enumeration<MedicationrequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 403 if (code == null) 404 return null; 405 if (code.isEmpty()) 406 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.NULL, code); 407 String codeString = ((PrimitiveType) code).asStringValue(); 408 if (codeString == null || "".equals(codeString)) 409 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.NULL, code); 410 if ("active".equals(codeString)) 411 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ACTIVE, code); 412 if ("on-hold".equals(codeString)) 413 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ONHOLD, code); 414 if ("ended".equals(codeString)) 415 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ENDED, code); 416 if ("stopped".equals(codeString)) 417 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.STOPPED, code); 418 if ("completed".equals(codeString)) 419 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.COMPLETED, code); 420 if ("cancelled".equals(codeString)) 421 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.CANCELLED, code); 422 if ("entered-in-error".equals(codeString)) 423 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ENTEREDINERROR, code); 424 if ("draft".equals(codeString)) 425 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.DRAFT, code); 426 if ("unknown".equals(codeString)) 427 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.UNKNOWN, code); 428 throw new FHIRException("Unknown MedicationrequestStatus code '"+codeString+"'"); 429 } 430 public String toCode(MedicationrequestStatus code) { 431 if (code == MedicationrequestStatus.ACTIVE) 432 return "active"; 433 if (code == MedicationrequestStatus.ONHOLD) 434 return "on-hold"; 435 if (code == MedicationrequestStatus.ENDED) 436 return "ended"; 437 if (code == MedicationrequestStatus.STOPPED) 438 return "stopped"; 439 if (code == MedicationrequestStatus.COMPLETED) 440 return "completed"; 441 if (code == MedicationrequestStatus.CANCELLED) 442 return "cancelled"; 443 if (code == MedicationrequestStatus.ENTEREDINERROR) 444 return "entered-in-error"; 445 if (code == MedicationrequestStatus.DRAFT) 446 return "draft"; 447 if (code == MedicationrequestStatus.UNKNOWN) 448 return "unknown"; 449 return "?"; 450 } 451 public String toSystem(MedicationrequestStatus code) { 452 return code.getSystem(); 453 } 454 } 455 456 @Block() 457 public static class MedicationRequestDispenseRequestComponent extends BackboneElement implements IBaseBackboneElement { 458 /** 459 * Indicates the quantity or duration for the first dispense of the medication. 460 */ 461 @Child(name = "initialFill", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 462 @Description(shortDefinition="First fill details", formalDefinition="Indicates the quantity or duration for the first dispense of the medication." ) 463 protected MedicationRequestDispenseRequestInitialFillComponent initialFill; 464 465 /** 466 * The minimum period of time that must occur between dispenses of the medication. 467 */ 468 @Child(name = "dispenseInterval", type = {Duration.class}, order=2, min=0, max=1, modifier=false, summary=false) 469 @Description(shortDefinition="Minimum period of time between dispenses", formalDefinition="The minimum period of time that must occur between dispenses of the medication." ) 470 protected Duration dispenseInterval; 471 472 /** 473 * This indicates the validity period of a prescription (stale dating the Prescription). 474 */ 475 @Child(name = "validityPeriod", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 476 @Description(shortDefinition="Time period supply is authorized for", formalDefinition="This indicates the validity period of a prescription (stale dating the Prescription)." ) 477 protected Period validityPeriod; 478 479 /** 480 * An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense. 481 */ 482 @Child(name = "numberOfRepeatsAllowed", type = {UnsignedIntType.class}, order=4, min=0, max=1, modifier=false, summary=false) 483 @Description(shortDefinition="Number of refills authorized", formalDefinition="An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense." ) 484 protected UnsignedIntType numberOfRepeatsAllowed; 485 486 /** 487 * The amount that is to be dispensed for one fill. 488 */ 489 @Child(name = "quantity", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 490 @Description(shortDefinition="Amount of medication to supply per dispense", formalDefinition="The amount that is to be dispensed for one fill." ) 491 protected Quantity quantity; 492 493 /** 494 * Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last. 495 */ 496 @Child(name = "expectedSupplyDuration", type = {Duration.class}, order=6, min=0, max=1, modifier=false, summary=false) 497 @Description(shortDefinition="Number of days supply per dispense", formalDefinition="Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last." ) 498 protected Duration expectedSupplyDuration; 499 500 /** 501 * Indicates the intended performing Organization that will dispense the medication as specified by the prescriber. 502 */ 503 @Child(name = "dispenser", type = {Organization.class}, order=7, min=0, max=1, modifier=false, summary=false) 504 @Description(shortDefinition="Intended performer of dispense", formalDefinition="Indicates the intended performing Organization that will dispense the medication as specified by the prescriber." ) 505 protected Reference dispenser; 506 507 /** 508 * Provides additional information to the dispenser, for example, counselling to be provided to the patient. 509 */ 510 @Child(name = "dispenserInstruction", type = {Annotation.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 511 @Description(shortDefinition="Additional information for the dispenser", formalDefinition="Provides additional information to the dispenser, for example, counselling to be provided to the patient." ) 512 protected List<Annotation> dispenserInstruction; 513 514 /** 515 * Provides information about the type of adherence packaging to be supplied for the medication dispense. 516 */ 517 @Child(name = "doseAdministrationAid", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 518 @Description(shortDefinition="Type of adherence packaging to use for the dispense", formalDefinition="Provides information about the type of adherence packaging to be supplied for the medication dispense." ) 519 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-dose-aid") 520 protected CodeableConcept doseAdministrationAid; 521 522 private static final long serialVersionUID = -916083616L; 523 524 /** 525 * Constructor 526 */ 527 public MedicationRequestDispenseRequestComponent() { 528 super(); 529 } 530 531 /** 532 * @return {@link #initialFill} (Indicates the quantity or duration for the first dispense of the medication.) 533 */ 534 public MedicationRequestDispenseRequestInitialFillComponent getInitialFill() { 535 if (this.initialFill == null) 536 if (Configuration.errorOnAutoCreate()) 537 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.initialFill"); 538 else if (Configuration.doAutoCreate()) 539 this.initialFill = new MedicationRequestDispenseRequestInitialFillComponent(); // cc 540 return this.initialFill; 541 } 542 543 public boolean hasInitialFill() { 544 return this.initialFill != null && !this.initialFill.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #initialFill} (Indicates the quantity or duration for the first dispense of the medication.) 549 */ 550 public MedicationRequestDispenseRequestComponent setInitialFill(MedicationRequestDispenseRequestInitialFillComponent value) { 551 this.initialFill = value; 552 return this; 553 } 554 555 /** 556 * @return {@link #dispenseInterval} (The minimum period of time that must occur between dispenses of the medication.) 557 */ 558 public Duration getDispenseInterval() { 559 if (this.dispenseInterval == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.dispenseInterval"); 562 else if (Configuration.doAutoCreate()) 563 this.dispenseInterval = new Duration(); // cc 564 return this.dispenseInterval; 565 } 566 567 public boolean hasDispenseInterval() { 568 return this.dispenseInterval != null && !this.dispenseInterval.isEmpty(); 569 } 570 571 /** 572 * @param value {@link #dispenseInterval} (The minimum period of time that must occur between dispenses of the medication.) 573 */ 574 public MedicationRequestDispenseRequestComponent setDispenseInterval(Duration value) { 575 this.dispenseInterval = value; 576 return this; 577 } 578 579 /** 580 * @return {@link #validityPeriod} (This indicates the validity period of a prescription (stale dating the Prescription).) 581 */ 582 public Period getValidityPeriod() { 583 if (this.validityPeriod == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.validityPeriod"); 586 else if (Configuration.doAutoCreate()) 587 this.validityPeriod = new Period(); // cc 588 return this.validityPeriod; 589 } 590 591 public boolean hasValidityPeriod() { 592 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #validityPeriod} (This indicates the validity period of a prescription (stale dating the Prescription).) 597 */ 598 public MedicationRequestDispenseRequestComponent setValidityPeriod(Period value) { 599 this.validityPeriod = value; 600 return this; 601 } 602 603 /** 604 * @return {@link #numberOfRepeatsAllowed} (An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.). This is the underlying object with id, value and extensions. The accessor "getNumberOfRepeatsAllowed" gives direct access to the value 605 */ 606 public UnsignedIntType getNumberOfRepeatsAllowedElement() { 607 if (this.numberOfRepeatsAllowed == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.numberOfRepeatsAllowed"); 610 else if (Configuration.doAutoCreate()) 611 this.numberOfRepeatsAllowed = new UnsignedIntType(); // bb 612 return this.numberOfRepeatsAllowed; 613 } 614 615 public boolean hasNumberOfRepeatsAllowedElement() { 616 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 617 } 618 619 public boolean hasNumberOfRepeatsAllowed() { 620 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #numberOfRepeatsAllowed} (An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.). This is the underlying object with id, value and extensions. The accessor "getNumberOfRepeatsAllowed" gives direct access to the value 625 */ 626 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowedElement(UnsignedIntType value) { 627 this.numberOfRepeatsAllowed = value; 628 return this; 629 } 630 631 /** 632 * @return An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense. 633 */ 634 public int getNumberOfRepeatsAllowed() { 635 return this.numberOfRepeatsAllowed == null || this.numberOfRepeatsAllowed.isEmpty() ? 0 : this.numberOfRepeatsAllowed.getValue(); 636 } 637 638 /** 639 * @param value An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense. 640 */ 641 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowed(int value) { 642 if (this.numberOfRepeatsAllowed == null) 643 this.numberOfRepeatsAllowed = new UnsignedIntType(); 644 this.numberOfRepeatsAllowed.setValue(value); 645 return this; 646 } 647 648 /** 649 * @return {@link #quantity} (The amount that is to be dispensed for one fill.) 650 */ 651 public Quantity getQuantity() { 652 if (this.quantity == null) 653 if (Configuration.errorOnAutoCreate()) 654 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.quantity"); 655 else if (Configuration.doAutoCreate()) 656 this.quantity = new Quantity(); // cc 657 return this.quantity; 658 } 659 660 public boolean hasQuantity() { 661 return this.quantity != null && !this.quantity.isEmpty(); 662 } 663 664 /** 665 * @param value {@link #quantity} (The amount that is to be dispensed for one fill.) 666 */ 667 public MedicationRequestDispenseRequestComponent setQuantity(Quantity value) { 668 this.quantity = value; 669 return this; 670 } 671 672 /** 673 * @return {@link #expectedSupplyDuration} (Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.) 674 */ 675 public Duration getExpectedSupplyDuration() { 676 if (this.expectedSupplyDuration == null) 677 if (Configuration.errorOnAutoCreate()) 678 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.expectedSupplyDuration"); 679 else if (Configuration.doAutoCreate()) 680 this.expectedSupplyDuration = new Duration(); // cc 681 return this.expectedSupplyDuration; 682 } 683 684 public boolean hasExpectedSupplyDuration() { 685 return this.expectedSupplyDuration != null && !this.expectedSupplyDuration.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #expectedSupplyDuration} (Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.) 690 */ 691 public MedicationRequestDispenseRequestComponent setExpectedSupplyDuration(Duration value) { 692 this.expectedSupplyDuration = value; 693 return this; 694 } 695 696 /** 697 * @return {@link #dispenser} (Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.) 698 */ 699 public Reference getDispenser() { 700 if (this.dispenser == null) 701 if (Configuration.errorOnAutoCreate()) 702 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.dispenser"); 703 else if (Configuration.doAutoCreate()) 704 this.dispenser = new Reference(); // cc 705 return this.dispenser; 706 } 707 708 public boolean hasDispenser() { 709 return this.dispenser != null && !this.dispenser.isEmpty(); 710 } 711 712 /** 713 * @param value {@link #dispenser} (Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.) 714 */ 715 public MedicationRequestDispenseRequestComponent setDispenser(Reference value) { 716 this.dispenser = value; 717 return this; 718 } 719 720 /** 721 * @return {@link #dispenserInstruction} (Provides additional information to the dispenser, for example, counselling to be provided to the patient.) 722 */ 723 public List<Annotation> getDispenserInstruction() { 724 if (this.dispenserInstruction == null) 725 this.dispenserInstruction = new ArrayList<Annotation>(); 726 return this.dispenserInstruction; 727 } 728 729 /** 730 * @return Returns a reference to <code>this</code> for easy method chaining 731 */ 732 public MedicationRequestDispenseRequestComponent setDispenserInstruction(List<Annotation> theDispenserInstruction) { 733 this.dispenserInstruction = theDispenserInstruction; 734 return this; 735 } 736 737 public boolean hasDispenserInstruction() { 738 if (this.dispenserInstruction == null) 739 return false; 740 for (Annotation item : this.dispenserInstruction) 741 if (!item.isEmpty()) 742 return true; 743 return false; 744 } 745 746 public Annotation addDispenserInstruction() { //3 747 Annotation t = new Annotation(); 748 if (this.dispenserInstruction == null) 749 this.dispenserInstruction = new ArrayList<Annotation>(); 750 this.dispenserInstruction.add(t); 751 return t; 752 } 753 754 public MedicationRequestDispenseRequestComponent addDispenserInstruction(Annotation t) { //3 755 if (t == null) 756 return this; 757 if (this.dispenserInstruction == null) 758 this.dispenserInstruction = new ArrayList<Annotation>(); 759 this.dispenserInstruction.add(t); 760 return this; 761 } 762 763 /** 764 * @return The first repetition of repeating field {@link #dispenserInstruction}, creating it if it does not already exist {3} 765 */ 766 public Annotation getDispenserInstructionFirstRep() { 767 if (getDispenserInstruction().isEmpty()) { 768 addDispenserInstruction(); 769 } 770 return getDispenserInstruction().get(0); 771 } 772 773 /** 774 * @return {@link #doseAdministrationAid} (Provides information about the type of adherence packaging to be supplied for the medication dispense.) 775 */ 776 public CodeableConcept getDoseAdministrationAid() { 777 if (this.doseAdministrationAid == null) 778 if (Configuration.errorOnAutoCreate()) 779 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.doseAdministrationAid"); 780 else if (Configuration.doAutoCreate()) 781 this.doseAdministrationAid = new CodeableConcept(); // cc 782 return this.doseAdministrationAid; 783 } 784 785 public boolean hasDoseAdministrationAid() { 786 return this.doseAdministrationAid != null && !this.doseAdministrationAid.isEmpty(); 787 } 788 789 /** 790 * @param value {@link #doseAdministrationAid} (Provides information about the type of adherence packaging to be supplied for the medication dispense.) 791 */ 792 public MedicationRequestDispenseRequestComponent setDoseAdministrationAid(CodeableConcept value) { 793 this.doseAdministrationAid = value; 794 return this; 795 } 796 797 protected void listChildren(List<Property> children) { 798 super.listChildren(children); 799 children.add(new Property("initialFill", "", "Indicates the quantity or duration for the first dispense of the medication.", 0, 1, initialFill)); 800 children.add(new Property("dispenseInterval", "Duration", "The minimum period of time that must occur between dispenses of the medication.", 0, 1, dispenseInterval)); 801 children.add(new Property("validityPeriod", "Period", "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, validityPeriod)); 802 children.add(new Property("numberOfRepeatsAllowed", "unsignedInt", "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.", 0, 1, numberOfRepeatsAllowed)); 803 children.add(new Property("quantity", "Quantity", "The amount that is to be dispensed for one fill.", 0, 1, quantity)); 804 children.add(new Property("expectedSupplyDuration", "Duration", "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 0, 1, expectedSupplyDuration)); 805 children.add(new Property("dispenser", "Reference(Organization)", "Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.", 0, 1, dispenser)); 806 children.add(new Property("dispenserInstruction", "Annotation", "Provides additional information to the dispenser, for example, counselling to be provided to the patient.", 0, java.lang.Integer.MAX_VALUE, dispenserInstruction)); 807 children.add(new Property("doseAdministrationAid", "CodeableConcept", "Provides information about the type of adherence packaging to be supplied for the medication dispense.", 0, 1, doseAdministrationAid)); 808 } 809 810 @Override 811 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 812 switch (_hash) { 813 case 1232961255: /*initialFill*/ return new Property("initialFill", "", "Indicates the quantity or duration for the first dispense of the medication.", 0, 1, initialFill); 814 case 757112130: /*dispenseInterval*/ return new Property("dispenseInterval", "Duration", "The minimum period of time that must occur between dispenses of the medication.", 0, 1, dispenseInterval); 815 case -1434195053: /*validityPeriod*/ return new Property("validityPeriod", "Period", "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, validityPeriod); 816 case -239736976: /*numberOfRepeatsAllowed*/ return new Property("numberOfRepeatsAllowed", "unsignedInt", "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.", 0, 1, numberOfRepeatsAllowed); 817 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount that is to be dispensed for one fill.", 0, 1, quantity); 818 case -1910182789: /*expectedSupplyDuration*/ return new Property("expectedSupplyDuration", "Duration", "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 0, 1, expectedSupplyDuration); 819 case 241511093: /*dispenser*/ return new Property("dispenser", "Reference(Organization)", "Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.", 0, 1, dispenser); 820 case 2073630361: /*dispenserInstruction*/ return new Property("dispenserInstruction", "Annotation", "Provides additional information to the dispenser, for example, counselling to be provided to the patient.", 0, java.lang.Integer.MAX_VALUE, dispenserInstruction); 821 case 390821217: /*doseAdministrationAid*/ return new Property("doseAdministrationAid", "CodeableConcept", "Provides information about the type of adherence packaging to be supplied for the medication dispense.", 0, 1, doseAdministrationAid); 822 default: return super.getNamedProperty(_hash, _name, _checkValid); 823 } 824 825 } 826 827 @Override 828 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 829 switch (hash) { 830 case 1232961255: /*initialFill*/ return this.initialFill == null ? new Base[0] : new Base[] {this.initialFill}; // MedicationRequestDispenseRequestInitialFillComponent 831 case 757112130: /*dispenseInterval*/ return this.dispenseInterval == null ? new Base[0] : new Base[] {this.dispenseInterval}; // Duration 832 case -1434195053: /*validityPeriod*/ return this.validityPeriod == null ? new Base[0] : new Base[] {this.validityPeriod}; // Period 833 case -239736976: /*numberOfRepeatsAllowed*/ return this.numberOfRepeatsAllowed == null ? new Base[0] : new Base[] {this.numberOfRepeatsAllowed}; // UnsignedIntType 834 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 835 case -1910182789: /*expectedSupplyDuration*/ return this.expectedSupplyDuration == null ? new Base[0] : new Base[] {this.expectedSupplyDuration}; // Duration 836 case 241511093: /*dispenser*/ return this.dispenser == null ? new Base[0] : new Base[] {this.dispenser}; // Reference 837 case 2073630361: /*dispenserInstruction*/ return this.dispenserInstruction == null ? new Base[0] : this.dispenserInstruction.toArray(new Base[this.dispenserInstruction.size()]); // Annotation 838 case 390821217: /*doseAdministrationAid*/ return this.doseAdministrationAid == null ? new Base[0] : new Base[] {this.doseAdministrationAid}; // CodeableConcept 839 default: return super.getProperty(hash, name, checkValid); 840 } 841 842 } 843 844 @Override 845 public Base setProperty(int hash, String name, Base value) throws FHIRException { 846 switch (hash) { 847 case 1232961255: // initialFill 848 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 849 return value; 850 case 757112130: // dispenseInterval 851 this.dispenseInterval = TypeConvertor.castToDuration(value); // Duration 852 return value; 853 case -1434195053: // validityPeriod 854 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 855 return value; 856 case -239736976: // numberOfRepeatsAllowed 857 this.numberOfRepeatsAllowed = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 858 return value; 859 case -1285004149: // quantity 860 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 861 return value; 862 case -1910182789: // expectedSupplyDuration 863 this.expectedSupplyDuration = TypeConvertor.castToDuration(value); // Duration 864 return value; 865 case 241511093: // dispenser 866 this.dispenser = TypeConvertor.castToReference(value); // Reference 867 return value; 868 case 2073630361: // dispenserInstruction 869 this.getDispenserInstruction().add(TypeConvertor.castToAnnotation(value)); // Annotation 870 return value; 871 case 390821217: // doseAdministrationAid 872 this.doseAdministrationAid = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 873 return value; 874 default: return super.setProperty(hash, name, value); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(String name, Base value) throws FHIRException { 881 if (name.equals("initialFill")) { 882 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 883 } else if (name.equals("dispenseInterval")) { 884 this.dispenseInterval = TypeConvertor.castToDuration(value); // Duration 885 } else if (name.equals("validityPeriod")) { 886 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 887 } else if (name.equals("numberOfRepeatsAllowed")) { 888 this.numberOfRepeatsAllowed = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 889 } else if (name.equals("quantity")) { 890 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 891 } else if (name.equals("expectedSupplyDuration")) { 892 this.expectedSupplyDuration = TypeConvertor.castToDuration(value); // Duration 893 } else if (name.equals("dispenser")) { 894 this.dispenser = TypeConvertor.castToReference(value); // Reference 895 } else if (name.equals("dispenserInstruction")) { 896 this.getDispenserInstruction().add(TypeConvertor.castToAnnotation(value)); 897 } else if (name.equals("doseAdministrationAid")) { 898 this.doseAdministrationAid = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 899 } else 900 return super.setProperty(name, value); 901 return value; 902 } 903 904 @Override 905 public void removeChild(String name, Base value) throws FHIRException { 906 if (name.equals("initialFill")) { 907 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 908 } else if (name.equals("dispenseInterval")) { 909 this.dispenseInterval = null; 910 } else if (name.equals("validityPeriod")) { 911 this.validityPeriod = null; 912 } else if (name.equals("numberOfRepeatsAllowed")) { 913 this.numberOfRepeatsAllowed = null; 914 } else if (name.equals("quantity")) { 915 this.quantity = null; 916 } else if (name.equals("expectedSupplyDuration")) { 917 this.expectedSupplyDuration = null; 918 } else if (name.equals("dispenser")) { 919 this.dispenser = null; 920 } else if (name.equals("dispenserInstruction")) { 921 this.getDispenserInstruction().remove(value); 922 } else if (name.equals("doseAdministrationAid")) { 923 this.doseAdministrationAid = null; 924 } else 925 super.removeChild(name, value); 926 927 } 928 929 @Override 930 public Base makeProperty(int hash, String name) throws FHIRException { 931 switch (hash) { 932 case 1232961255: return getInitialFill(); 933 case 757112130: return getDispenseInterval(); 934 case -1434195053: return getValidityPeriod(); 935 case -239736976: return getNumberOfRepeatsAllowedElement(); 936 case -1285004149: return getQuantity(); 937 case -1910182789: return getExpectedSupplyDuration(); 938 case 241511093: return getDispenser(); 939 case 2073630361: return addDispenserInstruction(); 940 case 390821217: return getDoseAdministrationAid(); 941 default: return super.makeProperty(hash, name); 942 } 943 944 } 945 946 @Override 947 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 948 switch (hash) { 949 case 1232961255: /*initialFill*/ return new String[] {}; 950 case 757112130: /*dispenseInterval*/ return new String[] {"Duration"}; 951 case -1434195053: /*validityPeriod*/ return new String[] {"Period"}; 952 case -239736976: /*numberOfRepeatsAllowed*/ return new String[] {"unsignedInt"}; 953 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 954 case -1910182789: /*expectedSupplyDuration*/ return new String[] {"Duration"}; 955 case 241511093: /*dispenser*/ return new String[] {"Reference"}; 956 case 2073630361: /*dispenserInstruction*/ return new String[] {"Annotation"}; 957 case 390821217: /*doseAdministrationAid*/ return new String[] {"CodeableConcept"}; 958 default: return super.getTypesForProperty(hash, name); 959 } 960 961 } 962 963 @Override 964 public Base addChild(String name) throws FHIRException { 965 if (name.equals("initialFill")) { 966 this.initialFill = new MedicationRequestDispenseRequestInitialFillComponent(); 967 return this.initialFill; 968 } 969 else if (name.equals("dispenseInterval")) { 970 this.dispenseInterval = new Duration(); 971 return this.dispenseInterval; 972 } 973 else if (name.equals("validityPeriod")) { 974 this.validityPeriod = new Period(); 975 return this.validityPeriod; 976 } 977 else if (name.equals("numberOfRepeatsAllowed")) { 978 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.dispenseRequest.numberOfRepeatsAllowed"); 979 } 980 else if (name.equals("quantity")) { 981 this.quantity = new Quantity(); 982 return this.quantity; 983 } 984 else if (name.equals("expectedSupplyDuration")) { 985 this.expectedSupplyDuration = new Duration(); 986 return this.expectedSupplyDuration; 987 } 988 else if (name.equals("dispenser")) { 989 this.dispenser = new Reference(); 990 return this.dispenser; 991 } 992 else if (name.equals("dispenserInstruction")) { 993 return addDispenserInstruction(); 994 } 995 else if (name.equals("doseAdministrationAid")) { 996 this.doseAdministrationAid = new CodeableConcept(); 997 return this.doseAdministrationAid; 998 } 999 else 1000 return super.addChild(name); 1001 } 1002 1003 public MedicationRequestDispenseRequestComponent copy() { 1004 MedicationRequestDispenseRequestComponent dst = new MedicationRequestDispenseRequestComponent(); 1005 copyValues(dst); 1006 return dst; 1007 } 1008 1009 public void copyValues(MedicationRequestDispenseRequestComponent dst) { 1010 super.copyValues(dst); 1011 dst.initialFill = initialFill == null ? null : initialFill.copy(); 1012 dst.dispenseInterval = dispenseInterval == null ? null : dispenseInterval.copy(); 1013 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1014 dst.numberOfRepeatsAllowed = numberOfRepeatsAllowed == null ? null : numberOfRepeatsAllowed.copy(); 1015 dst.quantity = quantity == null ? null : quantity.copy(); 1016 dst.expectedSupplyDuration = expectedSupplyDuration == null ? null : expectedSupplyDuration.copy(); 1017 dst.dispenser = dispenser == null ? null : dispenser.copy(); 1018 if (dispenserInstruction != null) { 1019 dst.dispenserInstruction = new ArrayList<Annotation>(); 1020 for (Annotation i : dispenserInstruction) 1021 dst.dispenserInstruction.add(i.copy()); 1022 }; 1023 dst.doseAdministrationAid = doseAdministrationAid == null ? null : doseAdministrationAid.copy(); 1024 } 1025 1026 @Override 1027 public boolean equalsDeep(Base other_) { 1028 if (!super.equalsDeep(other_)) 1029 return false; 1030 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1031 return false; 1032 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1033 return compareDeep(initialFill, o.initialFill, true) && compareDeep(dispenseInterval, o.dispenseInterval, true) 1034 && compareDeep(validityPeriod, o.validityPeriod, true) && compareDeep(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true) 1035 && compareDeep(quantity, o.quantity, true) && compareDeep(expectedSupplyDuration, o.expectedSupplyDuration, true) 1036 && compareDeep(dispenser, o.dispenser, true) && compareDeep(dispenserInstruction, o.dispenserInstruction, true) 1037 && compareDeep(doseAdministrationAid, o.doseAdministrationAid, true); 1038 } 1039 1040 @Override 1041 public boolean equalsShallow(Base other_) { 1042 if (!super.equalsShallow(other_)) 1043 return false; 1044 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1045 return false; 1046 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1047 return compareValues(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true); 1048 } 1049 1050 public boolean isEmpty() { 1051 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(initialFill, dispenseInterval 1052 , validityPeriod, numberOfRepeatsAllowed, quantity, expectedSupplyDuration, dispenser 1053 , dispenserInstruction, doseAdministrationAid); 1054 } 1055 1056 public String fhirType() { 1057 return "MedicationRequest.dispenseRequest"; 1058 1059 } 1060 1061 } 1062 1063 @Block() 1064 public static class MedicationRequestDispenseRequestInitialFillComponent extends BackboneElement implements IBaseBackboneElement { 1065 /** 1066 * The amount or quantity to provide as part of the first dispense. 1067 */ 1068 @Child(name = "quantity", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 1069 @Description(shortDefinition="First fill quantity", formalDefinition="The amount or quantity to provide as part of the first dispense." ) 1070 protected Quantity quantity; 1071 1072 /** 1073 * The length of time that the first dispense is expected to last. 1074 */ 1075 @Child(name = "duration", type = {Duration.class}, order=2, min=0, max=1, modifier=false, summary=false) 1076 @Description(shortDefinition="First fill duration", formalDefinition="The length of time that the first dispense is expected to last." ) 1077 protected Duration duration; 1078 1079 private static final long serialVersionUID = 1223227956L; 1080 1081 /** 1082 * Constructor 1083 */ 1084 public MedicationRequestDispenseRequestInitialFillComponent() { 1085 super(); 1086 } 1087 1088 /** 1089 * @return {@link #quantity} (The amount or quantity to provide as part of the first dispense.) 1090 */ 1091 public Quantity getQuantity() { 1092 if (this.quantity == null) 1093 if (Configuration.errorOnAutoCreate()) 1094 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestInitialFillComponent.quantity"); 1095 else if (Configuration.doAutoCreate()) 1096 this.quantity = new Quantity(); // cc 1097 return this.quantity; 1098 } 1099 1100 public boolean hasQuantity() { 1101 return this.quantity != null && !this.quantity.isEmpty(); 1102 } 1103 1104 /** 1105 * @param value {@link #quantity} (The amount or quantity to provide as part of the first dispense.) 1106 */ 1107 public MedicationRequestDispenseRequestInitialFillComponent setQuantity(Quantity value) { 1108 this.quantity = value; 1109 return this; 1110 } 1111 1112 /** 1113 * @return {@link #duration} (The length of time that the first dispense is expected to last.) 1114 */ 1115 public Duration getDuration() { 1116 if (this.duration == null) 1117 if (Configuration.errorOnAutoCreate()) 1118 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestInitialFillComponent.duration"); 1119 else if (Configuration.doAutoCreate()) 1120 this.duration = new Duration(); // cc 1121 return this.duration; 1122 } 1123 1124 public boolean hasDuration() { 1125 return this.duration != null && !this.duration.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #duration} (The length of time that the first dispense is expected to last.) 1130 */ 1131 public MedicationRequestDispenseRequestInitialFillComponent setDuration(Duration value) { 1132 this.duration = value; 1133 return this; 1134 } 1135 1136 protected void listChildren(List<Property> children) { 1137 super.listChildren(children); 1138 children.add(new Property("quantity", "Quantity", "The amount or quantity to provide as part of the first dispense.", 0, 1, quantity)); 1139 children.add(new Property("duration", "Duration", "The length of time that the first dispense is expected to last.", 0, 1, duration)); 1140 } 1141 1142 @Override 1143 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1144 switch (_hash) { 1145 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount or quantity to provide as part of the first dispense.", 0, 1, quantity); 1146 case -1992012396: /*duration*/ return new Property("duration", "Duration", "The length of time that the first dispense is expected to last.", 0, 1, duration); 1147 default: return super.getNamedProperty(_hash, _name, _checkValid); 1148 } 1149 1150 } 1151 1152 @Override 1153 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1154 switch (hash) { 1155 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1156 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // Duration 1157 default: return super.getProperty(hash, name, checkValid); 1158 } 1159 1160 } 1161 1162 @Override 1163 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1164 switch (hash) { 1165 case -1285004149: // quantity 1166 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1167 return value; 1168 case -1992012396: // duration 1169 this.duration = TypeConvertor.castToDuration(value); // Duration 1170 return value; 1171 default: return super.setProperty(hash, name, value); 1172 } 1173 1174 } 1175 1176 @Override 1177 public Base setProperty(String name, Base value) throws FHIRException { 1178 if (name.equals("quantity")) { 1179 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1180 } else if (name.equals("duration")) { 1181 this.duration = TypeConvertor.castToDuration(value); // Duration 1182 } else 1183 return super.setProperty(name, value); 1184 return value; 1185 } 1186 1187 @Override 1188 public void removeChild(String name, Base value) throws FHIRException { 1189 if (name.equals("quantity")) { 1190 this.quantity = null; 1191 } else if (name.equals("duration")) { 1192 this.duration = null; 1193 } else 1194 super.removeChild(name, value); 1195 1196 } 1197 1198 @Override 1199 public Base makeProperty(int hash, String name) throws FHIRException { 1200 switch (hash) { 1201 case -1285004149: return getQuantity(); 1202 case -1992012396: return getDuration(); 1203 default: return super.makeProperty(hash, name); 1204 } 1205 1206 } 1207 1208 @Override 1209 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1210 switch (hash) { 1211 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1212 case -1992012396: /*duration*/ return new String[] {"Duration"}; 1213 default: return super.getTypesForProperty(hash, name); 1214 } 1215 1216 } 1217 1218 @Override 1219 public Base addChild(String name) throws FHIRException { 1220 if (name.equals("quantity")) { 1221 this.quantity = new Quantity(); 1222 return this.quantity; 1223 } 1224 else if (name.equals("duration")) { 1225 this.duration = new Duration(); 1226 return this.duration; 1227 } 1228 else 1229 return super.addChild(name); 1230 } 1231 1232 public MedicationRequestDispenseRequestInitialFillComponent copy() { 1233 MedicationRequestDispenseRequestInitialFillComponent dst = new MedicationRequestDispenseRequestInitialFillComponent(); 1234 copyValues(dst); 1235 return dst; 1236 } 1237 1238 public void copyValues(MedicationRequestDispenseRequestInitialFillComponent dst) { 1239 super.copyValues(dst); 1240 dst.quantity = quantity == null ? null : quantity.copy(); 1241 dst.duration = duration == null ? null : duration.copy(); 1242 } 1243 1244 @Override 1245 public boolean equalsDeep(Base other_) { 1246 if (!super.equalsDeep(other_)) 1247 return false; 1248 if (!(other_ instanceof MedicationRequestDispenseRequestInitialFillComponent)) 1249 return false; 1250 MedicationRequestDispenseRequestInitialFillComponent o = (MedicationRequestDispenseRequestInitialFillComponent) other_; 1251 return compareDeep(quantity, o.quantity, true) && compareDeep(duration, o.duration, true); 1252 } 1253 1254 @Override 1255 public boolean equalsShallow(Base other_) { 1256 if (!super.equalsShallow(other_)) 1257 return false; 1258 if (!(other_ instanceof MedicationRequestDispenseRequestInitialFillComponent)) 1259 return false; 1260 MedicationRequestDispenseRequestInitialFillComponent o = (MedicationRequestDispenseRequestInitialFillComponent) other_; 1261 return true; 1262 } 1263 1264 public boolean isEmpty() { 1265 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, duration); 1266 } 1267 1268 public String fhirType() { 1269 return "MedicationRequest.dispenseRequest.initialFill"; 1270 1271 } 1272 1273 } 1274 1275 @Block() 1276 public static class MedicationRequestSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 1277 /** 1278 * True if the prescriber allows a different drug to be dispensed from what was prescribed. 1279 */ 1280 @Child(name = "allowed", type = {BooleanType.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1281 @Description(shortDefinition="Whether substitution is allowed or not", formalDefinition="True if the prescriber allows a different drug to be dispensed from what was prescribed." ) 1282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActSubstanceAdminSubstitutionCode") 1283 protected DataType allowed; 1284 1285 /** 1286 * Indicates the reason for the substitution, or why substitution must or must not be performed. 1287 */ 1288 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1289 @Description(shortDefinition="Why should (not) substitution be made", formalDefinition="Indicates the reason for the substitution, or why substitution must or must not be performed." ) 1290 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-SubstanceAdminSubstitutionReason") 1291 protected CodeableConcept reason; 1292 1293 private static final long serialVersionUID = -855005751L; 1294 1295 /** 1296 * Constructor 1297 */ 1298 public MedicationRequestSubstitutionComponent() { 1299 super(); 1300 } 1301 1302 /** 1303 * Constructor 1304 */ 1305 public MedicationRequestSubstitutionComponent(DataType allowed) { 1306 super(); 1307 this.setAllowed(allowed); 1308 } 1309 1310 /** 1311 * @return {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1312 */ 1313 public DataType getAllowed() { 1314 return this.allowed; 1315 } 1316 1317 /** 1318 * @return {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1319 */ 1320 public BooleanType getAllowedBooleanType() throws FHIRException { 1321 if (this.allowed == null) 1322 this.allowed = new BooleanType(); 1323 if (!(this.allowed instanceof BooleanType)) 1324 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 1325 return (BooleanType) this.allowed; 1326 } 1327 1328 public boolean hasAllowedBooleanType() { 1329 return this != null && this.allowed instanceof BooleanType; 1330 } 1331 1332 /** 1333 * @return {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1334 */ 1335 public CodeableConcept getAllowedCodeableConcept() throws FHIRException { 1336 if (this.allowed == null) 1337 this.allowed = new CodeableConcept(); 1338 if (!(this.allowed instanceof CodeableConcept)) 1339 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.allowed.getClass().getName()+" was encountered"); 1340 return (CodeableConcept) this.allowed; 1341 } 1342 1343 public boolean hasAllowedCodeableConcept() { 1344 return this != null && this.allowed instanceof CodeableConcept; 1345 } 1346 1347 public boolean hasAllowed() { 1348 return this.allowed != null && !this.allowed.isEmpty(); 1349 } 1350 1351 /** 1352 * @param value {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1353 */ 1354 public MedicationRequestSubstitutionComponent setAllowed(DataType value) { 1355 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 1356 throw new FHIRException("Not the right type for MedicationRequest.substitution.allowed[x]: "+value.fhirType()); 1357 this.allowed = value; 1358 return this; 1359 } 1360 1361 /** 1362 * @return {@link #reason} (Indicates the reason for the substitution, or why substitution must or must not be performed.) 1363 */ 1364 public CodeableConcept getReason() { 1365 if (this.reason == null) 1366 if (Configuration.errorOnAutoCreate()) 1367 throw new Error("Attempt to auto-create MedicationRequestSubstitutionComponent.reason"); 1368 else if (Configuration.doAutoCreate()) 1369 this.reason = new CodeableConcept(); // cc 1370 return this.reason; 1371 } 1372 1373 public boolean hasReason() { 1374 return this.reason != null && !this.reason.isEmpty(); 1375 } 1376 1377 /** 1378 * @param value {@link #reason} (Indicates the reason for the substitution, or why substitution must or must not be performed.) 1379 */ 1380 public MedicationRequestSubstitutionComponent setReason(CodeableConcept value) { 1381 this.reason = value; 1382 return this; 1383 } 1384 1385 protected void listChildren(List<Property> children) { 1386 super.listChildren(children); 1387 children.add(new Property("allowed[x]", "boolean|CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed)); 1388 children.add(new Property("reason", "CodeableConcept", "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, reason)); 1389 } 1390 1391 @Override 1392 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1393 switch (_hash) { 1394 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "boolean|CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1395 case -911343192: /*allowed*/ return new Property("allowed[x]", "boolean|CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1396 case 177755488: /*allowedBoolean*/ return new Property("allowed[x]", "boolean", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1397 case 963125465: /*allowedCodeableConcept*/ return new Property("allowed[x]", "CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1398 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, reason); 1399 default: return super.getNamedProperty(_hash, _name, _checkValid); 1400 } 1401 1402 } 1403 1404 @Override 1405 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1406 switch (hash) { 1407 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // DataType 1408 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1409 default: return super.getProperty(hash, name, checkValid); 1410 } 1411 1412 } 1413 1414 @Override 1415 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1416 switch (hash) { 1417 case -911343192: // allowed 1418 this.allowed = TypeConvertor.castToType(value); // DataType 1419 return value; 1420 case -934964668: // reason 1421 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1422 return value; 1423 default: return super.setProperty(hash, name, value); 1424 } 1425 1426 } 1427 1428 @Override 1429 public Base setProperty(String name, Base value) throws FHIRException { 1430 if (name.equals("allowed[x]")) { 1431 this.allowed = TypeConvertor.castToType(value); // DataType 1432 } else if (name.equals("reason")) { 1433 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1434 } else 1435 return super.setProperty(name, value); 1436 return value; 1437 } 1438 1439 @Override 1440 public void removeChild(String name, Base value) throws FHIRException { 1441 if (name.equals("allowed[x]")) { 1442 this.allowed = null; 1443 } else if (name.equals("reason")) { 1444 this.reason = null; 1445 } else 1446 super.removeChild(name, value); 1447 1448 } 1449 1450 @Override 1451 public Base makeProperty(int hash, String name) throws FHIRException { 1452 switch (hash) { 1453 case -1336663592: return getAllowed(); 1454 case -911343192: return getAllowed(); 1455 case -934964668: return getReason(); 1456 default: return super.makeProperty(hash, name); 1457 } 1458 1459 } 1460 1461 @Override 1462 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1463 switch (hash) { 1464 case -911343192: /*allowed*/ return new String[] {"boolean", "CodeableConcept"}; 1465 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1466 default: return super.getTypesForProperty(hash, name); 1467 } 1468 1469 } 1470 1471 @Override 1472 public Base addChild(String name) throws FHIRException { 1473 if (name.equals("allowedBoolean")) { 1474 this.allowed = new BooleanType(); 1475 return this.allowed; 1476 } 1477 else if (name.equals("allowedCodeableConcept")) { 1478 this.allowed = new CodeableConcept(); 1479 return this.allowed; 1480 } 1481 else if (name.equals("reason")) { 1482 this.reason = new CodeableConcept(); 1483 return this.reason; 1484 } 1485 else 1486 return super.addChild(name); 1487 } 1488 1489 public MedicationRequestSubstitutionComponent copy() { 1490 MedicationRequestSubstitutionComponent dst = new MedicationRequestSubstitutionComponent(); 1491 copyValues(dst); 1492 return dst; 1493 } 1494 1495 public void copyValues(MedicationRequestSubstitutionComponent dst) { 1496 super.copyValues(dst); 1497 dst.allowed = allowed == null ? null : allowed.copy(); 1498 dst.reason = reason == null ? null : reason.copy(); 1499 } 1500 1501 @Override 1502 public boolean equalsDeep(Base other_) { 1503 if (!super.equalsDeep(other_)) 1504 return false; 1505 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1506 return false; 1507 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1508 return compareDeep(allowed, o.allowed, true) && compareDeep(reason, o.reason, true); 1509 } 1510 1511 @Override 1512 public boolean equalsShallow(Base other_) { 1513 if (!super.equalsShallow(other_)) 1514 return false; 1515 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1516 return false; 1517 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1518 return true; 1519 } 1520 1521 public boolean isEmpty() { 1522 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(allowed, reason); 1523 } 1524 1525 public String fhirType() { 1526 return "MedicationRequest.substitution"; 1527 1528 } 1529 1530 } 1531 1532 /** 1533 * Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server. 1534 */ 1535 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1536 @Description(shortDefinition="External ids for this request", formalDefinition="Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server." ) 1537 protected List<Identifier> identifier; 1538 1539 /** 1540 * A plan or request that is fulfilled in whole or in part by this medication request. 1541 */ 1542 @Child(name = "basedOn", type = {CarePlan.class, MedicationRequest.class, ServiceRequest.class, ImmunizationRecommendation.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1543 @Description(shortDefinition="A plan or request that is fulfilled in whole or in part by this medication request", formalDefinition="A plan or request that is fulfilled in whole or in part by this medication request." ) 1544 protected List<Reference> basedOn; 1545 1546 /** 1547 * Reference to an order/prescription that is being replaced by this MedicationRequest. 1548 */ 1549 @Child(name = "priorPrescription", type = {MedicationRequest.class}, order=2, min=0, max=1, modifier=false, summary=false) 1550 @Description(shortDefinition="Reference to an order/prescription that is being replaced by this MedicationRequest", formalDefinition="Reference to an order/prescription that is being replaced by this MedicationRequest." ) 1551 protected Reference priorPrescription; 1552 1553 /** 1554 * A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time. 1555 */ 1556 @Child(name = "groupIdentifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 1557 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time." ) 1558 protected Identifier groupIdentifier; 1559 1560 /** 1561 * A code specifying the current state of the order. Generally, this will be active or completed state. 1562 */ 1563 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 1564 @Description(shortDefinition="active | on-hold | ended | stopped | completed | cancelled | entered-in-error | draft | unknown", formalDefinition="A code specifying the current state of the order. Generally, this will be active or completed state." ) 1565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-status") 1566 protected Enumeration<MedicationrequestStatus> status; 1567 1568 /** 1569 * Captures the reason for the current state of the MedicationRequest. 1570 */ 1571 @Child(name = "statusReason", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1572 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current state of the MedicationRequest." ) 1573 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-status-reason") 1574 protected CodeableConcept statusReason; 1575 1576 /** 1577 * The date (and perhaps time) when the status was changed. 1578 */ 1579 @Child(name = "statusChanged", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1580 @Description(shortDefinition="When the status was changed", formalDefinition="The date (and perhaps time) when the status was changed." ) 1581 protected DateTimeType statusChanged; 1582 1583 /** 1584 * Whether the request is a proposal, plan, or an original order. 1585 */ 1586 @Child(name = "intent", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 1587 @Description(shortDefinition="proposal | plan | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Whether the request is a proposal, plan, or an original order." ) 1588 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-intent") 1589 protected Enumeration<MedicationRequestIntent> intent; 1590 1591 /** 1592 * An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication. 1593 */ 1594 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1595 @Description(shortDefinition="Grouping or category of medication request", formalDefinition="An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication." ) 1596 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-admin-location") 1597 protected List<CodeableConcept> category; 1598 1599 /** 1600 * Indicates how quickly the Medication Request should be addressed with respect to other requests. 1601 */ 1602 @Child(name = "priority", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1603 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the Medication Request should be addressed with respect to other requests." ) 1604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 1605 protected Enumeration<RequestPriority> priority; 1606 1607 /** 1608 * If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication. 1609 */ 1610 @Child(name = "doNotPerform", type = {BooleanType.class}, order=10, min=0, max=1, modifier=true, summary=true) 1611 @Description(shortDefinition="True if patient is to stop taking or not to start taking the medication", formalDefinition="If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication." ) 1612 protected BooleanType doNotPerform; 1613 1614 /** 1615 * Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications. 1616 */ 1617 @Child(name = "medication", type = {CodeableReference.class}, order=11, min=1, max=1, modifier=false, summary=true) 1618 @Description(shortDefinition="Medication to be taken", formalDefinition="Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications." ) 1619 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 1620 protected CodeableReference medication; 1621 1622 /** 1623 * The individual or group for whom the medication has been requested. 1624 */ 1625 @Child(name = "subject", type = {Patient.class, Group.class}, order=12, min=1, max=1, modifier=false, summary=true) 1626 @Description(shortDefinition="Individual or group for whom the medication has been requested", formalDefinition="The individual or group for whom the medication has been requested." ) 1627 protected Reference subject; 1628 1629 /** 1630 * The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person. 1631 */ 1632 @Child(name = "informationSource", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Organization.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1633 @Description(shortDefinition="The person or organization who provided the information about this request, if the source is someone other than the requestor", formalDefinition="The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person." ) 1634 protected List<Reference> informationSource; 1635 1636 /** 1637 * The Encounter during which this [x] was created or to which the creation of this record is tightly associated. 1638 */ 1639 @Child(name = "encounter", type = {Encounter.class}, order=14, min=0, max=1, modifier=false, summary=false) 1640 @Description(shortDefinition="Encounter created as part of encounter/admission/stay", formalDefinition="The Encounter during which this [x] was created or to which the creation of this record is tightly associated." ) 1641 protected Reference encounter; 1642 1643 /** 1644 * Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient). 1645 */ 1646 @Child(name = "supportingInformation", type = {Reference.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1647 @Description(shortDefinition="Information to support fulfilling of the medication", formalDefinition="Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient)." ) 1648 protected List<Reference> supportingInformation; 1649 1650 /** 1651 * The date (and perhaps time) when the prescription was initially written or authored on. 1652 */ 1653 @Child(name = "authoredOn", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=true) 1654 @Description(shortDefinition="When request was initially authored", formalDefinition="The date (and perhaps time) when the prescription was initially written or authored on." ) 1655 protected DateTimeType authoredOn; 1656 1657 /** 1658 * The individual, organization, or device that initiated the request and has responsibility for its activation. 1659 */ 1660 @Child(name = "requester", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=17, min=0, max=1, modifier=false, summary=true) 1661 @Description(shortDefinition="Who/What requested the Request", formalDefinition="The individual, organization, or device that initiated the request and has responsibility for its activation." ) 1662 protected Reference requester; 1663 1664 /** 1665 * Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report. 1666 */ 1667 @Child(name = "reported", type = {BooleanType.class}, order=18, min=0, max=1, modifier=false, summary=true) 1668 @Description(shortDefinition="Reported rather than primary record", formalDefinition="Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report." ) 1669 protected BooleanType reported; 1670 1671 /** 1672 * Indicates the type of performer of the administration of the medication. 1673 */ 1674 @Child(name = "performerType", type = {CodeableConcept.class}, order=19, min=0, max=1, modifier=false, summary=true) 1675 @Description(shortDefinition="Desired kind of performer of the medication administration", formalDefinition="Indicates the type of performer of the administration of the medication." ) 1676 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-intended-performer-role") 1677 protected CodeableConcept performerType; 1678 1679 /** 1680 * The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers. 1681 */ 1682 @Child(name = "performer", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, DeviceDefinition.class, RelatedPerson.class, CareTeam.class, HealthcareService.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1683 @Description(shortDefinition="Intended performer of administration", formalDefinition="The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers." ) 1684 protected List<Reference> performer; 1685 1686 /** 1687 * The intended type of device that is to be used for the administration of the medication (for example, PCA Pump). 1688 */ 1689 @Child(name = "device", type = {CodeableReference.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1690 @Description(shortDefinition="Intended type of device for the administration", formalDefinition="The intended type of device that is to be used for the administration of the medication (for example, PCA Pump)." ) 1691 protected List<CodeableReference> device; 1692 1693 /** 1694 * The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order. 1695 */ 1696 @Child(name = "recorder", type = {Practitioner.class, PractitionerRole.class}, order=22, min=0, max=1, modifier=false, summary=false) 1697 @Description(shortDefinition="Person who entered the request", formalDefinition="The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order." ) 1698 protected Reference recorder; 1699 1700 /** 1701 * The reason or the indication for ordering or not ordering the medication. 1702 */ 1703 @Child(name = "reason", type = {CodeableReference.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1704 @Description(shortDefinition="Reason or indication for ordering or not ordering the medication", formalDefinition="The reason or the indication for ordering or not ordering the medication." ) 1705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 1706 protected List<CodeableReference> reason; 1707 1708 /** 1709 * The description of the overall pattern of the administration of the medication to the patient. 1710 */ 1711 @Child(name = "courseOfTherapyType", type = {CodeableConcept.class}, order=24, min=0, max=1, modifier=false, summary=false) 1712 @Description(shortDefinition="Overall pattern of medication administration", formalDefinition="The description of the overall pattern of the administration of the medication to the patient." ) 1713 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-course-of-therapy") 1714 protected CodeableConcept courseOfTherapyType; 1715 1716 /** 1717 * Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service. 1718 */ 1719 @Child(name = "insurance", type = {Coverage.class, ClaimResponse.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1720 @Description(shortDefinition="Associated insurance coverage", formalDefinition="Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service." ) 1721 protected List<Reference> insurance; 1722 1723 /** 1724 * Extra information about the prescription that could not be conveyed by the other attributes. 1725 */ 1726 @Child(name = "note", type = {Annotation.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1727 @Description(shortDefinition="Information about the prescription", formalDefinition="Extra information about the prescription that could not be conveyed by the other attributes." ) 1728 protected List<Annotation> note; 1729 1730 /** 1731 * The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 1732 */ 1733 @Child(name = "renderedDosageInstruction", type = {MarkdownType.class}, order=27, min=0, max=1, modifier=false, summary=false) 1734 @Description(shortDefinition="Full representation of the dosage instructions", formalDefinition="The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses." ) 1735 protected MarkdownType renderedDosageInstruction; 1736 1737 /** 1738 * The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions. 1739 */ 1740 @Child(name = "effectiveDosePeriod", type = {Period.class}, order=28, min=0, max=1, modifier=false, summary=false) 1741 @Description(shortDefinition="Period over which the medication is to be taken", formalDefinition="The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions." ) 1742 protected Period effectiveDosePeriod; 1743 1744 /** 1745 * Specific instructions for how the medication is to be used by the patient. 1746 */ 1747 @Child(name = "dosageInstruction", type = {Dosage.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1748 @Description(shortDefinition="Specific instructions for how the medication should be taken", formalDefinition="Specific instructions for how the medication is to be used by the patient." ) 1749 protected List<Dosage> dosageInstruction; 1750 1751 /** 1752 * Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department. 1753 */ 1754 @Child(name = "dispenseRequest", type = {}, order=30, min=0, max=1, modifier=false, summary=false) 1755 @Description(shortDefinition="Medication supply authorization", formalDefinition="Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department." ) 1756 protected MedicationRequestDispenseRequestComponent dispenseRequest; 1757 1758 /** 1759 * Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done. 1760 */ 1761 @Child(name = "substitution", type = {}, order=31, min=0, max=1, modifier=false, summary=false) 1762 @Description(shortDefinition="Any restrictions on medication substitution", formalDefinition="Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done." ) 1763 protected MedicationRequestSubstitutionComponent substitution; 1764 1765 /** 1766 * Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource. 1767 */ 1768 @Child(name = "eventHistory", type = {Provenance.class}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1769 @Description(shortDefinition="A list of events of interest in the lifecycle", formalDefinition="Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource." ) 1770 protected List<Reference> eventHistory; 1771 1772 private static final long serialVersionUID = 1013423544L; 1773 1774 /** 1775 * Constructor 1776 */ 1777 public MedicationRequest() { 1778 super(); 1779 } 1780 1781 /** 1782 * Constructor 1783 */ 1784 public MedicationRequest(MedicationrequestStatus status, MedicationRequestIntent intent, CodeableReference medication, Reference subject) { 1785 super(); 1786 this.setStatus(status); 1787 this.setIntent(intent); 1788 this.setMedication(medication); 1789 this.setSubject(subject); 1790 } 1791 1792 /** 1793 * @return {@link #identifier} (Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.) 1794 */ 1795 public List<Identifier> getIdentifier() { 1796 if (this.identifier == null) 1797 this.identifier = new ArrayList<Identifier>(); 1798 return this.identifier; 1799 } 1800 1801 /** 1802 * @return Returns a reference to <code>this</code> for easy method chaining 1803 */ 1804 public MedicationRequest setIdentifier(List<Identifier> theIdentifier) { 1805 this.identifier = theIdentifier; 1806 return this; 1807 } 1808 1809 public boolean hasIdentifier() { 1810 if (this.identifier == null) 1811 return false; 1812 for (Identifier item : this.identifier) 1813 if (!item.isEmpty()) 1814 return true; 1815 return false; 1816 } 1817 1818 public Identifier addIdentifier() { //3 1819 Identifier t = new Identifier(); 1820 if (this.identifier == null) 1821 this.identifier = new ArrayList<Identifier>(); 1822 this.identifier.add(t); 1823 return t; 1824 } 1825 1826 public MedicationRequest addIdentifier(Identifier t) { //3 1827 if (t == null) 1828 return this; 1829 if (this.identifier == null) 1830 this.identifier = new ArrayList<Identifier>(); 1831 this.identifier.add(t); 1832 return this; 1833 } 1834 1835 /** 1836 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1837 */ 1838 public Identifier getIdentifierFirstRep() { 1839 if (getIdentifier().isEmpty()) { 1840 addIdentifier(); 1841 } 1842 return getIdentifier().get(0); 1843 } 1844 1845 /** 1846 * @return {@link #basedOn} (A plan or request that is fulfilled in whole or in part by this medication request.) 1847 */ 1848 public List<Reference> getBasedOn() { 1849 if (this.basedOn == null) 1850 this.basedOn = new ArrayList<Reference>(); 1851 return this.basedOn; 1852 } 1853 1854 /** 1855 * @return Returns a reference to <code>this</code> for easy method chaining 1856 */ 1857 public MedicationRequest setBasedOn(List<Reference> theBasedOn) { 1858 this.basedOn = theBasedOn; 1859 return this; 1860 } 1861 1862 public boolean hasBasedOn() { 1863 if (this.basedOn == null) 1864 return false; 1865 for (Reference item : this.basedOn) 1866 if (!item.isEmpty()) 1867 return true; 1868 return false; 1869 } 1870 1871 public Reference addBasedOn() { //3 1872 Reference t = new Reference(); 1873 if (this.basedOn == null) 1874 this.basedOn = new ArrayList<Reference>(); 1875 this.basedOn.add(t); 1876 return t; 1877 } 1878 1879 public MedicationRequest addBasedOn(Reference t) { //3 1880 if (t == null) 1881 return this; 1882 if (this.basedOn == null) 1883 this.basedOn = new ArrayList<Reference>(); 1884 this.basedOn.add(t); 1885 return this; 1886 } 1887 1888 /** 1889 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1890 */ 1891 public Reference getBasedOnFirstRep() { 1892 if (getBasedOn().isEmpty()) { 1893 addBasedOn(); 1894 } 1895 return getBasedOn().get(0); 1896 } 1897 1898 /** 1899 * @return {@link #priorPrescription} (Reference to an order/prescription that is being replaced by this MedicationRequest.) 1900 */ 1901 public Reference getPriorPrescription() { 1902 if (this.priorPrescription == null) 1903 if (Configuration.errorOnAutoCreate()) 1904 throw new Error("Attempt to auto-create MedicationRequest.priorPrescription"); 1905 else if (Configuration.doAutoCreate()) 1906 this.priorPrescription = new Reference(); // cc 1907 return this.priorPrescription; 1908 } 1909 1910 public boolean hasPriorPrescription() { 1911 return this.priorPrescription != null && !this.priorPrescription.isEmpty(); 1912 } 1913 1914 /** 1915 * @param value {@link #priorPrescription} (Reference to an order/prescription that is being replaced by this MedicationRequest.) 1916 */ 1917 public MedicationRequest setPriorPrescription(Reference value) { 1918 this.priorPrescription = value; 1919 return this; 1920 } 1921 1922 /** 1923 * @return {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 1924 */ 1925 public Identifier getGroupIdentifier() { 1926 if (this.groupIdentifier == null) 1927 if (Configuration.errorOnAutoCreate()) 1928 throw new Error("Attempt to auto-create MedicationRequest.groupIdentifier"); 1929 else if (Configuration.doAutoCreate()) 1930 this.groupIdentifier = new Identifier(); // cc 1931 return this.groupIdentifier; 1932 } 1933 1934 public boolean hasGroupIdentifier() { 1935 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1936 } 1937 1938 /** 1939 * @param value {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 1940 */ 1941 public MedicationRequest setGroupIdentifier(Identifier value) { 1942 this.groupIdentifier = value; 1943 return this; 1944 } 1945 1946 /** 1947 * @return {@link #status} (A code specifying the current state of the order. Generally, this will be active or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1948 */ 1949 public Enumeration<MedicationrequestStatus> getStatusElement() { 1950 if (this.status == null) 1951 if (Configuration.errorOnAutoCreate()) 1952 throw new Error("Attempt to auto-create MedicationRequest.status"); 1953 else if (Configuration.doAutoCreate()) 1954 this.status = new Enumeration<MedicationrequestStatus>(new MedicationrequestStatusEnumFactory()); // bb 1955 return this.status; 1956 } 1957 1958 public boolean hasStatusElement() { 1959 return this.status != null && !this.status.isEmpty(); 1960 } 1961 1962 public boolean hasStatus() { 1963 return this.status != null && !this.status.isEmpty(); 1964 } 1965 1966 /** 1967 * @param value {@link #status} (A code specifying the current state of the order. Generally, this will be active or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1968 */ 1969 public MedicationRequest setStatusElement(Enumeration<MedicationrequestStatus> value) { 1970 this.status = value; 1971 return this; 1972 } 1973 1974 /** 1975 * @return A code specifying the current state of the order. Generally, this will be active or completed state. 1976 */ 1977 public MedicationrequestStatus getStatus() { 1978 return this.status == null ? null : this.status.getValue(); 1979 } 1980 1981 /** 1982 * @param value A code specifying the current state of the order. Generally, this will be active or completed state. 1983 */ 1984 public MedicationRequest setStatus(MedicationrequestStatus value) { 1985 if (this.status == null) 1986 this.status = new Enumeration<MedicationrequestStatus>(new MedicationrequestStatusEnumFactory()); 1987 this.status.setValue(value); 1988 return this; 1989 } 1990 1991 /** 1992 * @return {@link #statusReason} (Captures the reason for the current state of the MedicationRequest.) 1993 */ 1994 public CodeableConcept getStatusReason() { 1995 if (this.statusReason == null) 1996 if (Configuration.errorOnAutoCreate()) 1997 throw new Error("Attempt to auto-create MedicationRequest.statusReason"); 1998 else if (Configuration.doAutoCreate()) 1999 this.statusReason = new CodeableConcept(); // cc 2000 return this.statusReason; 2001 } 2002 2003 public boolean hasStatusReason() { 2004 return this.statusReason != null && !this.statusReason.isEmpty(); 2005 } 2006 2007 /** 2008 * @param value {@link #statusReason} (Captures the reason for the current state of the MedicationRequest.) 2009 */ 2010 public MedicationRequest setStatusReason(CodeableConcept value) { 2011 this.statusReason = value; 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #statusChanged} (The date (and perhaps time) when the status was changed.). This is the underlying object with id, value and extensions. The accessor "getStatusChanged" gives direct access to the value 2017 */ 2018 public DateTimeType getStatusChangedElement() { 2019 if (this.statusChanged == null) 2020 if (Configuration.errorOnAutoCreate()) 2021 throw new Error("Attempt to auto-create MedicationRequest.statusChanged"); 2022 else if (Configuration.doAutoCreate()) 2023 this.statusChanged = new DateTimeType(); // bb 2024 return this.statusChanged; 2025 } 2026 2027 public boolean hasStatusChangedElement() { 2028 return this.statusChanged != null && !this.statusChanged.isEmpty(); 2029 } 2030 2031 public boolean hasStatusChanged() { 2032 return this.statusChanged != null && !this.statusChanged.isEmpty(); 2033 } 2034 2035 /** 2036 * @param value {@link #statusChanged} (The date (and perhaps time) when the status was changed.). This is the underlying object with id, value and extensions. The accessor "getStatusChanged" gives direct access to the value 2037 */ 2038 public MedicationRequest setStatusChangedElement(DateTimeType value) { 2039 this.statusChanged = value; 2040 return this; 2041 } 2042 2043 /** 2044 * @return The date (and perhaps time) when the status was changed. 2045 */ 2046 public Date getStatusChanged() { 2047 return this.statusChanged == null ? null : this.statusChanged.getValue(); 2048 } 2049 2050 /** 2051 * @param value The date (and perhaps time) when the status was changed. 2052 */ 2053 public MedicationRequest setStatusChanged(Date value) { 2054 if (value == null) 2055 this.statusChanged = null; 2056 else { 2057 if (this.statusChanged == null) 2058 this.statusChanged = new DateTimeType(); 2059 this.statusChanged.setValue(value); 2060 } 2061 return this; 2062 } 2063 2064 /** 2065 * @return {@link #intent} (Whether the request is a proposal, plan, or an original order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2066 */ 2067 public Enumeration<MedicationRequestIntent> getIntentElement() { 2068 if (this.intent == null) 2069 if (Configuration.errorOnAutoCreate()) 2070 throw new Error("Attempt to auto-create MedicationRequest.intent"); 2071 else if (Configuration.doAutoCreate()) 2072 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); // bb 2073 return this.intent; 2074 } 2075 2076 public boolean hasIntentElement() { 2077 return this.intent != null && !this.intent.isEmpty(); 2078 } 2079 2080 public boolean hasIntent() { 2081 return this.intent != null && !this.intent.isEmpty(); 2082 } 2083 2084 /** 2085 * @param value {@link #intent} (Whether the request is a proposal, plan, or an original order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2086 */ 2087 public MedicationRequest setIntentElement(Enumeration<MedicationRequestIntent> value) { 2088 this.intent = value; 2089 return this; 2090 } 2091 2092 /** 2093 * @return Whether the request is a proposal, plan, or an original order. 2094 */ 2095 public MedicationRequestIntent getIntent() { 2096 return this.intent == null ? null : this.intent.getValue(); 2097 } 2098 2099 /** 2100 * @param value Whether the request is a proposal, plan, or an original order. 2101 */ 2102 public MedicationRequest setIntent(MedicationRequestIntent value) { 2103 if (this.intent == null) 2104 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); 2105 this.intent.setValue(value); 2106 return this; 2107 } 2108 2109 /** 2110 * @return {@link #category} (An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication.) 2111 */ 2112 public List<CodeableConcept> getCategory() { 2113 if (this.category == null) 2114 this.category = new ArrayList<CodeableConcept>(); 2115 return this.category; 2116 } 2117 2118 /** 2119 * @return Returns a reference to <code>this</code> for easy method chaining 2120 */ 2121 public MedicationRequest setCategory(List<CodeableConcept> theCategory) { 2122 this.category = theCategory; 2123 return this; 2124 } 2125 2126 public boolean hasCategory() { 2127 if (this.category == null) 2128 return false; 2129 for (CodeableConcept item : this.category) 2130 if (!item.isEmpty()) 2131 return true; 2132 return false; 2133 } 2134 2135 public CodeableConcept addCategory() { //3 2136 CodeableConcept t = new CodeableConcept(); 2137 if (this.category == null) 2138 this.category = new ArrayList<CodeableConcept>(); 2139 this.category.add(t); 2140 return t; 2141 } 2142 2143 public MedicationRequest addCategory(CodeableConcept t) { //3 2144 if (t == null) 2145 return this; 2146 if (this.category == null) 2147 this.category = new ArrayList<CodeableConcept>(); 2148 this.category.add(t); 2149 return this; 2150 } 2151 2152 /** 2153 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2154 */ 2155 public CodeableConcept getCategoryFirstRep() { 2156 if (getCategory().isEmpty()) { 2157 addCategory(); 2158 } 2159 return getCategory().get(0); 2160 } 2161 2162 /** 2163 * @return {@link #priority} (Indicates how quickly the Medication Request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2164 */ 2165 public Enumeration<RequestPriority> getPriorityElement() { 2166 if (this.priority == null) 2167 if (Configuration.errorOnAutoCreate()) 2168 throw new Error("Attempt to auto-create MedicationRequest.priority"); 2169 else if (Configuration.doAutoCreate()) 2170 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 2171 return this.priority; 2172 } 2173 2174 public boolean hasPriorityElement() { 2175 return this.priority != null && !this.priority.isEmpty(); 2176 } 2177 2178 public boolean hasPriority() { 2179 return this.priority != null && !this.priority.isEmpty(); 2180 } 2181 2182 /** 2183 * @param value {@link #priority} (Indicates how quickly the Medication Request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2184 */ 2185 public MedicationRequest setPriorityElement(Enumeration<RequestPriority> value) { 2186 this.priority = value; 2187 return this; 2188 } 2189 2190 /** 2191 * @return Indicates how quickly the Medication Request should be addressed with respect to other requests. 2192 */ 2193 public RequestPriority getPriority() { 2194 return this.priority == null ? null : this.priority.getValue(); 2195 } 2196 2197 /** 2198 * @param value Indicates how quickly the Medication Request should be addressed with respect to other requests. 2199 */ 2200 public MedicationRequest setPriority(RequestPriority value) { 2201 if (value == null) 2202 this.priority = null; 2203 else { 2204 if (this.priority == null) 2205 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 2206 this.priority.setValue(value); 2207 } 2208 return this; 2209 } 2210 2211 /** 2212 * @return {@link #doNotPerform} (If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 2213 */ 2214 public BooleanType getDoNotPerformElement() { 2215 if (this.doNotPerform == null) 2216 if (Configuration.errorOnAutoCreate()) 2217 throw new Error("Attempt to auto-create MedicationRequest.doNotPerform"); 2218 else if (Configuration.doAutoCreate()) 2219 this.doNotPerform = new BooleanType(); // bb 2220 return this.doNotPerform; 2221 } 2222 2223 public boolean hasDoNotPerformElement() { 2224 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2225 } 2226 2227 public boolean hasDoNotPerform() { 2228 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2229 } 2230 2231 /** 2232 * @param value {@link #doNotPerform} (If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 2233 */ 2234 public MedicationRequest setDoNotPerformElement(BooleanType value) { 2235 this.doNotPerform = value; 2236 return this; 2237 } 2238 2239 /** 2240 * @return If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication. 2241 */ 2242 public boolean getDoNotPerform() { 2243 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 2244 } 2245 2246 /** 2247 * @param value If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication. 2248 */ 2249 public MedicationRequest setDoNotPerform(boolean value) { 2250 if (this.doNotPerform == null) 2251 this.doNotPerform = new BooleanType(); 2252 this.doNotPerform.setValue(value); 2253 return this; 2254 } 2255 2256 /** 2257 * @return {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 2258 */ 2259 public CodeableReference getMedication() { 2260 if (this.medication == null) 2261 if (Configuration.errorOnAutoCreate()) 2262 throw new Error("Attempt to auto-create MedicationRequest.medication"); 2263 else if (Configuration.doAutoCreate()) 2264 this.medication = new CodeableReference(); // cc 2265 return this.medication; 2266 } 2267 2268 public boolean hasMedication() { 2269 return this.medication != null && !this.medication.isEmpty(); 2270 } 2271 2272 /** 2273 * @param value {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 2274 */ 2275 public MedicationRequest setMedication(CodeableReference value) { 2276 this.medication = value; 2277 return this; 2278 } 2279 2280 /** 2281 * @return {@link #subject} (The individual or group for whom the medication has been requested.) 2282 */ 2283 public Reference getSubject() { 2284 if (this.subject == null) 2285 if (Configuration.errorOnAutoCreate()) 2286 throw new Error("Attempt to auto-create MedicationRequest.subject"); 2287 else if (Configuration.doAutoCreate()) 2288 this.subject = new Reference(); // cc 2289 return this.subject; 2290 } 2291 2292 public boolean hasSubject() { 2293 return this.subject != null && !this.subject.isEmpty(); 2294 } 2295 2296 /** 2297 * @param value {@link #subject} (The individual or group for whom the medication has been requested.) 2298 */ 2299 public MedicationRequest setSubject(Reference value) { 2300 this.subject = value; 2301 return this; 2302 } 2303 2304 /** 2305 * @return {@link #informationSource} (The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person.) 2306 */ 2307 public List<Reference> getInformationSource() { 2308 if (this.informationSource == null) 2309 this.informationSource = new ArrayList<Reference>(); 2310 return this.informationSource; 2311 } 2312 2313 /** 2314 * @return Returns a reference to <code>this</code> for easy method chaining 2315 */ 2316 public MedicationRequest setInformationSource(List<Reference> theInformationSource) { 2317 this.informationSource = theInformationSource; 2318 return this; 2319 } 2320 2321 public boolean hasInformationSource() { 2322 if (this.informationSource == null) 2323 return false; 2324 for (Reference item : this.informationSource) 2325 if (!item.isEmpty()) 2326 return true; 2327 return false; 2328 } 2329 2330 public Reference addInformationSource() { //3 2331 Reference t = new Reference(); 2332 if (this.informationSource == null) 2333 this.informationSource = new ArrayList<Reference>(); 2334 this.informationSource.add(t); 2335 return t; 2336 } 2337 2338 public MedicationRequest addInformationSource(Reference t) { //3 2339 if (t == null) 2340 return this; 2341 if (this.informationSource == null) 2342 this.informationSource = new ArrayList<Reference>(); 2343 this.informationSource.add(t); 2344 return this; 2345 } 2346 2347 /** 2348 * @return The first repetition of repeating field {@link #informationSource}, creating it if it does not already exist {3} 2349 */ 2350 public Reference getInformationSourceFirstRep() { 2351 if (getInformationSource().isEmpty()) { 2352 addInformationSource(); 2353 } 2354 return getInformationSource().get(0); 2355 } 2356 2357 /** 2358 * @return {@link #encounter} (The Encounter during which this [x] was created or to which the creation of this record is tightly associated.) 2359 */ 2360 public Reference getEncounter() { 2361 if (this.encounter == null) 2362 if (Configuration.errorOnAutoCreate()) 2363 throw new Error("Attempt to auto-create MedicationRequest.encounter"); 2364 else if (Configuration.doAutoCreate()) 2365 this.encounter = new Reference(); // cc 2366 return this.encounter; 2367 } 2368 2369 public boolean hasEncounter() { 2370 return this.encounter != null && !this.encounter.isEmpty(); 2371 } 2372 2373 /** 2374 * @param value {@link #encounter} (The Encounter during which this [x] was created or to which the creation of this record is tightly associated.) 2375 */ 2376 public MedicationRequest setEncounter(Reference value) { 2377 this.encounter = value; 2378 return this; 2379 } 2380 2381 /** 2382 * @return {@link #supportingInformation} (Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient).) 2383 */ 2384 public List<Reference> getSupportingInformation() { 2385 if (this.supportingInformation == null) 2386 this.supportingInformation = new ArrayList<Reference>(); 2387 return this.supportingInformation; 2388 } 2389 2390 /** 2391 * @return Returns a reference to <code>this</code> for easy method chaining 2392 */ 2393 public MedicationRequest setSupportingInformation(List<Reference> theSupportingInformation) { 2394 this.supportingInformation = theSupportingInformation; 2395 return this; 2396 } 2397 2398 public boolean hasSupportingInformation() { 2399 if (this.supportingInformation == null) 2400 return false; 2401 for (Reference item : this.supportingInformation) 2402 if (!item.isEmpty()) 2403 return true; 2404 return false; 2405 } 2406 2407 public Reference addSupportingInformation() { //3 2408 Reference t = new Reference(); 2409 if (this.supportingInformation == null) 2410 this.supportingInformation = new ArrayList<Reference>(); 2411 this.supportingInformation.add(t); 2412 return t; 2413 } 2414 2415 public MedicationRequest addSupportingInformation(Reference t) { //3 2416 if (t == null) 2417 return this; 2418 if (this.supportingInformation == null) 2419 this.supportingInformation = new ArrayList<Reference>(); 2420 this.supportingInformation.add(t); 2421 return this; 2422 } 2423 2424 /** 2425 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 2426 */ 2427 public Reference getSupportingInformationFirstRep() { 2428 if (getSupportingInformation().isEmpty()) { 2429 addSupportingInformation(); 2430 } 2431 return getSupportingInformation().get(0); 2432 } 2433 2434 /** 2435 * @return {@link #authoredOn} (The date (and perhaps time) when the prescription was initially written or authored on.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2436 */ 2437 public DateTimeType getAuthoredOnElement() { 2438 if (this.authoredOn == null) 2439 if (Configuration.errorOnAutoCreate()) 2440 throw new Error("Attempt to auto-create MedicationRequest.authoredOn"); 2441 else if (Configuration.doAutoCreate()) 2442 this.authoredOn = new DateTimeType(); // bb 2443 return this.authoredOn; 2444 } 2445 2446 public boolean hasAuthoredOnElement() { 2447 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2448 } 2449 2450 public boolean hasAuthoredOn() { 2451 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2452 } 2453 2454 /** 2455 * @param value {@link #authoredOn} (The date (and perhaps time) when the prescription was initially written or authored on.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2456 */ 2457 public MedicationRequest setAuthoredOnElement(DateTimeType value) { 2458 this.authoredOn = value; 2459 return this; 2460 } 2461 2462 /** 2463 * @return The date (and perhaps time) when the prescription was initially written or authored on. 2464 */ 2465 public Date getAuthoredOn() { 2466 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2467 } 2468 2469 /** 2470 * @param value The date (and perhaps time) when the prescription was initially written or authored on. 2471 */ 2472 public MedicationRequest setAuthoredOn(Date value) { 2473 if (value == null) 2474 this.authoredOn = null; 2475 else { 2476 if (this.authoredOn == null) 2477 this.authoredOn = new DateTimeType(); 2478 this.authoredOn.setValue(value); 2479 } 2480 return this; 2481 } 2482 2483 /** 2484 * @return {@link #requester} (The individual, organization, or device that initiated the request and has responsibility for its activation.) 2485 */ 2486 public Reference getRequester() { 2487 if (this.requester == null) 2488 if (Configuration.errorOnAutoCreate()) 2489 throw new Error("Attempt to auto-create MedicationRequest.requester"); 2490 else if (Configuration.doAutoCreate()) 2491 this.requester = new Reference(); // cc 2492 return this.requester; 2493 } 2494 2495 public boolean hasRequester() { 2496 return this.requester != null && !this.requester.isEmpty(); 2497 } 2498 2499 /** 2500 * @param value {@link #requester} (The individual, organization, or device that initiated the request and has responsibility for its activation.) 2501 */ 2502 public MedicationRequest setRequester(Reference value) { 2503 this.requester = value; 2504 return this; 2505 } 2506 2507 /** 2508 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 2509 */ 2510 public BooleanType getReportedElement() { 2511 if (this.reported == null) 2512 if (Configuration.errorOnAutoCreate()) 2513 throw new Error("Attempt to auto-create MedicationRequest.reported"); 2514 else if (Configuration.doAutoCreate()) 2515 this.reported = new BooleanType(); // bb 2516 return this.reported; 2517 } 2518 2519 public boolean hasReportedElement() { 2520 return this.reported != null && !this.reported.isEmpty(); 2521 } 2522 2523 public boolean hasReported() { 2524 return this.reported != null && !this.reported.isEmpty(); 2525 } 2526 2527 /** 2528 * @param value {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 2529 */ 2530 public MedicationRequest setReportedElement(BooleanType value) { 2531 this.reported = value; 2532 return this; 2533 } 2534 2535 /** 2536 * @return Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report. 2537 */ 2538 public boolean getReported() { 2539 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 2540 } 2541 2542 /** 2543 * @param value Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report. 2544 */ 2545 public MedicationRequest setReported(boolean value) { 2546 if (this.reported == null) 2547 this.reported = new BooleanType(); 2548 this.reported.setValue(value); 2549 return this; 2550 } 2551 2552 /** 2553 * @return {@link #performerType} (Indicates the type of performer of the administration of the medication.) 2554 */ 2555 public CodeableConcept getPerformerType() { 2556 if (this.performerType == null) 2557 if (Configuration.errorOnAutoCreate()) 2558 throw new Error("Attempt to auto-create MedicationRequest.performerType"); 2559 else if (Configuration.doAutoCreate()) 2560 this.performerType = new CodeableConcept(); // cc 2561 return this.performerType; 2562 } 2563 2564 public boolean hasPerformerType() { 2565 return this.performerType != null && !this.performerType.isEmpty(); 2566 } 2567 2568 /** 2569 * @param value {@link #performerType} (Indicates the type of performer of the administration of the medication.) 2570 */ 2571 public MedicationRequest setPerformerType(CodeableConcept value) { 2572 this.performerType = value; 2573 return this; 2574 } 2575 2576 /** 2577 * @return {@link #performer} (The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.) 2578 */ 2579 public List<Reference> getPerformer() { 2580 if (this.performer == null) 2581 this.performer = new ArrayList<Reference>(); 2582 return this.performer; 2583 } 2584 2585 /** 2586 * @return Returns a reference to <code>this</code> for easy method chaining 2587 */ 2588 public MedicationRequest setPerformer(List<Reference> thePerformer) { 2589 this.performer = thePerformer; 2590 return this; 2591 } 2592 2593 public boolean hasPerformer() { 2594 if (this.performer == null) 2595 return false; 2596 for (Reference item : this.performer) 2597 if (!item.isEmpty()) 2598 return true; 2599 return false; 2600 } 2601 2602 public Reference addPerformer() { //3 2603 Reference t = new Reference(); 2604 if (this.performer == null) 2605 this.performer = new ArrayList<Reference>(); 2606 this.performer.add(t); 2607 return t; 2608 } 2609 2610 public MedicationRequest addPerformer(Reference t) { //3 2611 if (t == null) 2612 return this; 2613 if (this.performer == null) 2614 this.performer = new ArrayList<Reference>(); 2615 this.performer.add(t); 2616 return this; 2617 } 2618 2619 /** 2620 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2621 */ 2622 public Reference getPerformerFirstRep() { 2623 if (getPerformer().isEmpty()) { 2624 addPerformer(); 2625 } 2626 return getPerformer().get(0); 2627 } 2628 2629 /** 2630 * @return {@link #device} (The intended type of device that is to be used for the administration of the medication (for example, PCA Pump).) 2631 */ 2632 public List<CodeableReference> getDevice() { 2633 if (this.device == null) 2634 this.device = new ArrayList<CodeableReference>(); 2635 return this.device; 2636 } 2637 2638 /** 2639 * @return Returns a reference to <code>this</code> for easy method chaining 2640 */ 2641 public MedicationRequest setDevice(List<CodeableReference> theDevice) { 2642 this.device = theDevice; 2643 return this; 2644 } 2645 2646 public boolean hasDevice() { 2647 if (this.device == null) 2648 return false; 2649 for (CodeableReference item : this.device) 2650 if (!item.isEmpty()) 2651 return true; 2652 return false; 2653 } 2654 2655 public CodeableReference addDevice() { //3 2656 CodeableReference t = new CodeableReference(); 2657 if (this.device == null) 2658 this.device = new ArrayList<CodeableReference>(); 2659 this.device.add(t); 2660 return t; 2661 } 2662 2663 public MedicationRequest addDevice(CodeableReference t) { //3 2664 if (t == null) 2665 return this; 2666 if (this.device == null) 2667 this.device = new ArrayList<CodeableReference>(); 2668 this.device.add(t); 2669 return this; 2670 } 2671 2672 /** 2673 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 2674 */ 2675 public CodeableReference getDeviceFirstRep() { 2676 if (getDevice().isEmpty()) { 2677 addDevice(); 2678 } 2679 return getDevice().get(0); 2680 } 2681 2682 /** 2683 * @return {@link #recorder} (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2684 */ 2685 public Reference getRecorder() { 2686 if (this.recorder == null) 2687 if (Configuration.errorOnAutoCreate()) 2688 throw new Error("Attempt to auto-create MedicationRequest.recorder"); 2689 else if (Configuration.doAutoCreate()) 2690 this.recorder = new Reference(); // cc 2691 return this.recorder; 2692 } 2693 2694 public boolean hasRecorder() { 2695 return this.recorder != null && !this.recorder.isEmpty(); 2696 } 2697 2698 /** 2699 * @param value {@link #recorder} (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2700 */ 2701 public MedicationRequest setRecorder(Reference value) { 2702 this.recorder = value; 2703 return this; 2704 } 2705 2706 /** 2707 * @return {@link #reason} (The reason or the indication for ordering or not ordering the medication.) 2708 */ 2709 public List<CodeableReference> getReason() { 2710 if (this.reason == null) 2711 this.reason = new ArrayList<CodeableReference>(); 2712 return this.reason; 2713 } 2714 2715 /** 2716 * @return Returns a reference to <code>this</code> for easy method chaining 2717 */ 2718 public MedicationRequest setReason(List<CodeableReference> theReason) { 2719 this.reason = theReason; 2720 return this; 2721 } 2722 2723 public boolean hasReason() { 2724 if (this.reason == null) 2725 return false; 2726 for (CodeableReference item : this.reason) 2727 if (!item.isEmpty()) 2728 return true; 2729 return false; 2730 } 2731 2732 public CodeableReference addReason() { //3 2733 CodeableReference t = new CodeableReference(); 2734 if (this.reason == null) 2735 this.reason = new ArrayList<CodeableReference>(); 2736 this.reason.add(t); 2737 return t; 2738 } 2739 2740 public MedicationRequest addReason(CodeableReference t) { //3 2741 if (t == null) 2742 return this; 2743 if (this.reason == null) 2744 this.reason = new ArrayList<CodeableReference>(); 2745 this.reason.add(t); 2746 return this; 2747 } 2748 2749 /** 2750 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2751 */ 2752 public CodeableReference getReasonFirstRep() { 2753 if (getReason().isEmpty()) { 2754 addReason(); 2755 } 2756 return getReason().get(0); 2757 } 2758 2759 /** 2760 * @return {@link #courseOfTherapyType} (The description of the overall pattern of the administration of the medication to the patient.) 2761 */ 2762 public CodeableConcept getCourseOfTherapyType() { 2763 if (this.courseOfTherapyType == null) 2764 if (Configuration.errorOnAutoCreate()) 2765 throw new Error("Attempt to auto-create MedicationRequest.courseOfTherapyType"); 2766 else if (Configuration.doAutoCreate()) 2767 this.courseOfTherapyType = new CodeableConcept(); // cc 2768 return this.courseOfTherapyType; 2769 } 2770 2771 public boolean hasCourseOfTherapyType() { 2772 return this.courseOfTherapyType != null && !this.courseOfTherapyType.isEmpty(); 2773 } 2774 2775 /** 2776 * @param value {@link #courseOfTherapyType} (The description of the overall pattern of the administration of the medication to the patient.) 2777 */ 2778 public MedicationRequest setCourseOfTherapyType(CodeableConcept value) { 2779 this.courseOfTherapyType = value; 2780 return this; 2781 } 2782 2783 /** 2784 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.) 2785 */ 2786 public List<Reference> getInsurance() { 2787 if (this.insurance == null) 2788 this.insurance = new ArrayList<Reference>(); 2789 return this.insurance; 2790 } 2791 2792 /** 2793 * @return Returns a reference to <code>this</code> for easy method chaining 2794 */ 2795 public MedicationRequest setInsurance(List<Reference> theInsurance) { 2796 this.insurance = theInsurance; 2797 return this; 2798 } 2799 2800 public boolean hasInsurance() { 2801 if (this.insurance == null) 2802 return false; 2803 for (Reference item : this.insurance) 2804 if (!item.isEmpty()) 2805 return true; 2806 return false; 2807 } 2808 2809 public Reference addInsurance() { //3 2810 Reference t = new Reference(); 2811 if (this.insurance == null) 2812 this.insurance = new ArrayList<Reference>(); 2813 this.insurance.add(t); 2814 return t; 2815 } 2816 2817 public MedicationRequest addInsurance(Reference t) { //3 2818 if (t == null) 2819 return this; 2820 if (this.insurance == null) 2821 this.insurance = new ArrayList<Reference>(); 2822 this.insurance.add(t); 2823 return this; 2824 } 2825 2826 /** 2827 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 2828 */ 2829 public Reference getInsuranceFirstRep() { 2830 if (getInsurance().isEmpty()) { 2831 addInsurance(); 2832 } 2833 return getInsurance().get(0); 2834 } 2835 2836 /** 2837 * @return {@link #note} (Extra information about the prescription that could not be conveyed by the other attributes.) 2838 */ 2839 public List<Annotation> getNote() { 2840 if (this.note == null) 2841 this.note = new ArrayList<Annotation>(); 2842 return this.note; 2843 } 2844 2845 /** 2846 * @return Returns a reference to <code>this</code> for easy method chaining 2847 */ 2848 public MedicationRequest setNote(List<Annotation> theNote) { 2849 this.note = theNote; 2850 return this; 2851 } 2852 2853 public boolean hasNote() { 2854 if (this.note == null) 2855 return false; 2856 for (Annotation item : this.note) 2857 if (!item.isEmpty()) 2858 return true; 2859 return false; 2860 } 2861 2862 public Annotation addNote() { //3 2863 Annotation t = new Annotation(); 2864 if (this.note == null) 2865 this.note = new ArrayList<Annotation>(); 2866 this.note.add(t); 2867 return t; 2868 } 2869 2870 public MedicationRequest addNote(Annotation t) { //3 2871 if (t == null) 2872 return this; 2873 if (this.note == null) 2874 this.note = new ArrayList<Annotation>(); 2875 this.note.add(t); 2876 return this; 2877 } 2878 2879 /** 2880 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2881 */ 2882 public Annotation getNoteFirstRep() { 2883 if (getNote().isEmpty()) { 2884 addNote(); 2885 } 2886 return getNote().get(0); 2887 } 2888 2889 /** 2890 * @return {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 2891 */ 2892 public MarkdownType getRenderedDosageInstructionElement() { 2893 if (this.renderedDosageInstruction == null) 2894 if (Configuration.errorOnAutoCreate()) 2895 throw new Error("Attempt to auto-create MedicationRequest.renderedDosageInstruction"); 2896 else if (Configuration.doAutoCreate()) 2897 this.renderedDosageInstruction = new MarkdownType(); // bb 2898 return this.renderedDosageInstruction; 2899 } 2900 2901 public boolean hasRenderedDosageInstructionElement() { 2902 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 2903 } 2904 2905 public boolean hasRenderedDosageInstruction() { 2906 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 2907 } 2908 2909 /** 2910 * @param value {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 2911 */ 2912 public MedicationRequest setRenderedDosageInstructionElement(MarkdownType value) { 2913 this.renderedDosageInstruction = value; 2914 return this; 2915 } 2916 2917 /** 2918 * @return The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 2919 */ 2920 public String getRenderedDosageInstruction() { 2921 return this.renderedDosageInstruction == null ? null : this.renderedDosageInstruction.getValue(); 2922 } 2923 2924 /** 2925 * @param value The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 2926 */ 2927 public MedicationRequest setRenderedDosageInstruction(String value) { 2928 if (Utilities.noString(value)) 2929 this.renderedDosageInstruction = null; 2930 else { 2931 if (this.renderedDosageInstruction == null) 2932 this.renderedDosageInstruction = new MarkdownType(); 2933 this.renderedDosageInstruction.setValue(value); 2934 } 2935 return this; 2936 } 2937 2938 /** 2939 * @return {@link #effectiveDosePeriod} (The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.) 2940 */ 2941 public Period getEffectiveDosePeriod() { 2942 if (this.effectiveDosePeriod == null) 2943 if (Configuration.errorOnAutoCreate()) 2944 throw new Error("Attempt to auto-create MedicationRequest.effectiveDosePeriod"); 2945 else if (Configuration.doAutoCreate()) 2946 this.effectiveDosePeriod = new Period(); // cc 2947 return this.effectiveDosePeriod; 2948 } 2949 2950 public boolean hasEffectiveDosePeriod() { 2951 return this.effectiveDosePeriod != null && !this.effectiveDosePeriod.isEmpty(); 2952 } 2953 2954 /** 2955 * @param value {@link #effectiveDosePeriod} (The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.) 2956 */ 2957 public MedicationRequest setEffectiveDosePeriod(Period value) { 2958 this.effectiveDosePeriod = value; 2959 return this; 2960 } 2961 2962 /** 2963 * @return {@link #dosageInstruction} (Specific instructions for how the medication is to be used by the patient.) 2964 */ 2965 public List<Dosage> getDosageInstruction() { 2966 if (this.dosageInstruction == null) 2967 this.dosageInstruction = new ArrayList<Dosage>(); 2968 return this.dosageInstruction; 2969 } 2970 2971 /** 2972 * @return Returns a reference to <code>this</code> for easy method chaining 2973 */ 2974 public MedicationRequest setDosageInstruction(List<Dosage> theDosageInstruction) { 2975 this.dosageInstruction = theDosageInstruction; 2976 return this; 2977 } 2978 2979 public boolean hasDosageInstruction() { 2980 if (this.dosageInstruction == null) 2981 return false; 2982 for (Dosage item : this.dosageInstruction) 2983 if (!item.isEmpty()) 2984 return true; 2985 return false; 2986 } 2987 2988 public Dosage addDosageInstruction() { //3 2989 Dosage t = new Dosage(); 2990 if (this.dosageInstruction == null) 2991 this.dosageInstruction = new ArrayList<Dosage>(); 2992 this.dosageInstruction.add(t); 2993 return t; 2994 } 2995 2996 public MedicationRequest addDosageInstruction(Dosage t) { //3 2997 if (t == null) 2998 return this; 2999 if (this.dosageInstruction == null) 3000 this.dosageInstruction = new ArrayList<Dosage>(); 3001 this.dosageInstruction.add(t); 3002 return this; 3003 } 3004 3005 /** 3006 * @return The first repetition of repeating field {@link #dosageInstruction}, creating it if it does not already exist {3} 3007 */ 3008 public Dosage getDosageInstructionFirstRep() { 3009 if (getDosageInstruction().isEmpty()) { 3010 addDosageInstruction(); 3011 } 3012 return getDosageInstruction().get(0); 3013 } 3014 3015 /** 3016 * @return {@link #dispenseRequest} (Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.) 3017 */ 3018 public MedicationRequestDispenseRequestComponent getDispenseRequest() { 3019 if (this.dispenseRequest == null) 3020 if (Configuration.errorOnAutoCreate()) 3021 throw new Error("Attempt to auto-create MedicationRequest.dispenseRequest"); 3022 else if (Configuration.doAutoCreate()) 3023 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); // cc 3024 return this.dispenseRequest; 3025 } 3026 3027 public boolean hasDispenseRequest() { 3028 return this.dispenseRequest != null && !this.dispenseRequest.isEmpty(); 3029 } 3030 3031 /** 3032 * @param value {@link #dispenseRequest} (Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.) 3033 */ 3034 public MedicationRequest setDispenseRequest(MedicationRequestDispenseRequestComponent value) { 3035 this.dispenseRequest = value; 3036 return this; 3037 } 3038 3039 /** 3040 * @return {@link #substitution} (Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.) 3041 */ 3042 public MedicationRequestSubstitutionComponent getSubstitution() { 3043 if (this.substitution == null) 3044 if (Configuration.errorOnAutoCreate()) 3045 throw new Error("Attempt to auto-create MedicationRequest.substitution"); 3046 else if (Configuration.doAutoCreate()) 3047 this.substitution = new MedicationRequestSubstitutionComponent(); // cc 3048 return this.substitution; 3049 } 3050 3051 public boolean hasSubstitution() { 3052 return this.substitution != null && !this.substitution.isEmpty(); 3053 } 3054 3055 /** 3056 * @param value {@link #substitution} (Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.) 3057 */ 3058 public MedicationRequest setSubstitution(MedicationRequestSubstitutionComponent value) { 3059 this.substitution = value; 3060 return this; 3061 } 3062 3063 /** 3064 * @return {@link #eventHistory} (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 3065 */ 3066 public List<Reference> getEventHistory() { 3067 if (this.eventHistory == null) 3068 this.eventHistory = new ArrayList<Reference>(); 3069 return this.eventHistory; 3070 } 3071 3072 /** 3073 * @return Returns a reference to <code>this</code> for easy method chaining 3074 */ 3075 public MedicationRequest setEventHistory(List<Reference> theEventHistory) { 3076 this.eventHistory = theEventHistory; 3077 return this; 3078 } 3079 3080 public boolean hasEventHistory() { 3081 if (this.eventHistory == null) 3082 return false; 3083 for (Reference item : this.eventHistory) 3084 if (!item.isEmpty()) 3085 return true; 3086 return false; 3087 } 3088 3089 public Reference addEventHistory() { //3 3090 Reference t = new Reference(); 3091 if (this.eventHistory == null) 3092 this.eventHistory = new ArrayList<Reference>(); 3093 this.eventHistory.add(t); 3094 return t; 3095 } 3096 3097 public MedicationRequest addEventHistory(Reference t) { //3 3098 if (t == null) 3099 return this; 3100 if (this.eventHistory == null) 3101 this.eventHistory = new ArrayList<Reference>(); 3102 this.eventHistory.add(t); 3103 return this; 3104 } 3105 3106 /** 3107 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist {3} 3108 */ 3109 public Reference getEventHistoryFirstRep() { 3110 if (getEventHistory().isEmpty()) { 3111 addEventHistory(); 3112 } 3113 return getEventHistory().get(0); 3114 } 3115 3116 protected void listChildren(List<Property> children) { 3117 super.listChildren(children); 3118 children.add(new Property("identifier", "Identifier", "Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3119 children.add(new Property("basedOn", "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", "A plan or request that is fulfilled in whole or in part by this medication request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3120 children.add(new Property("priorPrescription", "Reference(MedicationRequest)", "Reference to an order/prescription that is being replaced by this MedicationRequest.", 0, 1, priorPrescription)); 3121 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier)); 3122 children.add(new Property("status", "code", "A code specifying the current state of the order. Generally, this will be active or completed state.", 0, 1, status)); 3123 children.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the MedicationRequest.", 0, 1, statusReason)); 3124 children.add(new Property("statusChanged", "dateTime", "The date (and perhaps time) when the status was changed.", 0, 1, statusChanged)); 3125 children.add(new Property("intent", "code", "Whether the request is a proposal, plan, or an original order.", 0, 1, intent)); 3126 children.add(new Property("category", "CodeableConcept", "An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication.", 0, java.lang.Integer.MAX_VALUE, category)); 3127 children.add(new Property("priority", "code", "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, priority)); 3128 children.add(new Property("doNotPerform", "boolean", "If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.", 0, 1, doNotPerform)); 3129 children.add(new Property("medication", "CodeableReference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 3130 children.add(new Property("subject", "Reference(Patient|Group)", "The individual or group for whom the medication has been requested.", 0, 1, subject)); 3131 children.add(new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person.", 0, java.lang.Integer.MAX_VALUE, informationSource)); 3132 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this [x] was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 3133 children.add(new Property("supportingInformation", "Reference(Any)", "Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient).", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 3134 children.add(new Property("authoredOn", "dateTime", "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn)); 3135 children.add(new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The individual, organization, or device that initiated the request and has responsibility for its activation.", 0, 1, requester)); 3136 children.add(new Property("reported", "boolean", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported)); 3137 children.add(new Property("performerType", "CodeableConcept", "Indicates the type of performer of the administration of the medication.", 0, 1, performerType)); 3138 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|Patient|DeviceDefinition|RelatedPerson|CareTeam|HealthcareService)", "The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.", 0, java.lang.Integer.MAX_VALUE, performer)); 3139 children.add(new Property("device", "CodeableReference(DeviceDefinition)", "The intended type of device that is to be used for the administration of the medication (for example, PCA Pump).", 0, java.lang.Integer.MAX_VALUE, device)); 3140 children.add(new Property("recorder", "Reference(Practitioner|PractitionerRole)", "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 0, 1, recorder)); 3141 children.add(new Property("reason", "CodeableReference(Condition|Observation)", "The reason or the indication for ordering or not ordering the medication.", 0, java.lang.Integer.MAX_VALUE, reason)); 3142 children.add(new Property("courseOfTherapyType", "CodeableConcept", "The description of the overall pattern of the administration of the medication to the patient.", 0, 1, courseOfTherapyType)); 3143 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance)); 3144 children.add(new Property("note", "Annotation", "Extra information about the prescription that could not be conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 3145 children.add(new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction)); 3146 children.add(new Property("effectiveDosePeriod", "Period", "The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.", 0, 1, effectiveDosePeriod)); 3147 children.add(new Property("dosageInstruction", "Dosage", "Specific instructions for how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 3148 children.add(new Property("dispenseRequest", "", "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 0, 1, dispenseRequest)); 3149 children.add(new Property("substitution", "", "Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 0, 1, substitution)); 3150 children.add(new Property("eventHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 3151 } 3152 3153 @Override 3154 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3155 switch (_hash) { 3156 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 3157 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", "A plan or request that is fulfilled in whole or in part by this medication request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3158 case -486355964: /*priorPrescription*/ return new Property("priorPrescription", "Reference(MedicationRequest)", "Reference to an order/prescription that is being replaced by this MedicationRequest.", 0, 1, priorPrescription); 3159 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier); 3160 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the current state of the order. Generally, this will be active or completed state.", 0, 1, status); 3161 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the MedicationRequest.", 0, 1, statusReason); 3162 case -1174686110: /*statusChanged*/ return new Property("statusChanged", "dateTime", "The date (and perhaps time) when the status was changed.", 0, 1, statusChanged); 3163 case -1183762788: /*intent*/ return new Property("intent", "code", "Whether the request is a proposal, plan, or an original order.", 0, 1, intent); 3164 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication.", 0, java.lang.Integer.MAX_VALUE, category); 3165 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, priority); 3166 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.", 0, 1, doNotPerform); 3167 case 1998965455: /*medication*/ return new Property("medication", "CodeableReference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 3168 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The individual or group for whom the medication has been requested.", 0, 1, subject); 3169 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person.", 0, java.lang.Integer.MAX_VALUE, informationSource); 3170 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this [x] was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 3171 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient).", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 3172 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn); 3173 case 693933948: /*requester*/ return new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The individual, organization, or device that initiated the request and has responsibility for its activation.", 0, 1, requester); 3174 case -427039533: /*reported*/ return new Property("reported", "boolean", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported); 3175 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "Indicates the type of performer of the administration of the medication.", 0, 1, performerType); 3176 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|Patient|DeviceDefinition|RelatedPerson|CareTeam|HealthcareService)", "The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.", 0, java.lang.Integer.MAX_VALUE, performer); 3177 case -1335157162: /*device*/ return new Property("device", "CodeableReference(DeviceDefinition)", "The intended type of device that is to be used for the administration of the medication (for example, PCA Pump).", 0, java.lang.Integer.MAX_VALUE, device); 3178 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Practitioner|PractitionerRole)", "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 0, 1, recorder); 3179 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation)", "The reason or the indication for ordering or not ordering the medication.", 0, java.lang.Integer.MAX_VALUE, reason); 3180 case -447282031: /*courseOfTherapyType*/ return new Property("courseOfTherapyType", "CodeableConcept", "The description of the overall pattern of the administration of the medication to the patient.", 0, 1, courseOfTherapyType); 3181 case 73049818: /*insurance*/ return new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance); 3182 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the prescription that could not be conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 3183 case 1718902050: /*renderedDosageInstruction*/ return new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction); 3184 case 322608453: /*effectiveDosePeriod*/ return new Property("effectiveDosePeriod", "Period", "The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.", 0, 1, effectiveDosePeriod); 3185 case -1201373865: /*dosageInstruction*/ return new Property("dosageInstruction", "Dosage", "Specific instructions for how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction); 3186 case 824620658: /*dispenseRequest*/ return new Property("dispenseRequest", "", "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 0, 1, dispenseRequest); 3187 case 826147581: /*substitution*/ return new Property("substitution", "", "Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 0, 1, substitution); 3188 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 3189 default: return super.getNamedProperty(_hash, _name, _checkValid); 3190 } 3191 3192 } 3193 3194 @Override 3195 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3196 switch (hash) { 3197 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3198 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3199 case -486355964: /*priorPrescription*/ return this.priorPrescription == null ? new Base[0] : new Base[] {this.priorPrescription}; // Reference 3200 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 3201 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationrequestStatus> 3202 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 3203 case -1174686110: /*statusChanged*/ return this.statusChanged == null ? new Base[0] : new Base[] {this.statusChanged}; // DateTimeType 3204 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<MedicationRequestIntent> 3205 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3206 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 3207 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 3208 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // CodeableReference 3209 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3210 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : this.informationSource.toArray(new Base[this.informationSource.size()]); // Reference 3211 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3212 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 3213 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 3214 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 3215 case -427039533: /*reported*/ return this.reported == null ? new Base[0] : new Base[] {this.reported}; // BooleanType 3216 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 3217 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 3218 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // CodeableReference 3219 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 3220 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 3221 case -447282031: /*courseOfTherapyType*/ return this.courseOfTherapyType == null ? new Base[0] : new Base[] {this.courseOfTherapyType}; // CodeableConcept 3222 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 3223 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3224 case 1718902050: /*renderedDosageInstruction*/ return this.renderedDosageInstruction == null ? new Base[0] : new Base[] {this.renderedDosageInstruction}; // MarkdownType 3225 case 322608453: /*effectiveDosePeriod*/ return this.effectiveDosePeriod == null ? new Base[0] : new Base[] {this.effectiveDosePeriod}; // Period 3226 case -1201373865: /*dosageInstruction*/ return this.dosageInstruction == null ? new Base[0] : this.dosageInstruction.toArray(new Base[this.dosageInstruction.size()]); // Dosage 3227 case 824620658: /*dispenseRequest*/ return this.dispenseRequest == null ? new Base[0] : new Base[] {this.dispenseRequest}; // MedicationRequestDispenseRequestComponent 3228 case 826147581: /*substitution*/ return this.substitution == null ? new Base[0] : new Base[] {this.substitution}; // MedicationRequestSubstitutionComponent 3229 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 3230 default: return super.getProperty(hash, name, checkValid); 3231 } 3232 3233 } 3234 3235 @Override 3236 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3237 switch (hash) { 3238 case -1618432855: // identifier 3239 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3240 return value; 3241 case -332612366: // basedOn 3242 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 3243 return value; 3244 case -486355964: // priorPrescription 3245 this.priorPrescription = TypeConvertor.castToReference(value); // Reference 3246 return value; 3247 case -445338488: // groupIdentifier 3248 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 3249 return value; 3250 case -892481550: // status 3251 value = new MedicationrequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3252 this.status = (Enumeration) value; // Enumeration<MedicationrequestStatus> 3253 return value; 3254 case 2051346646: // statusReason 3255 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3256 return value; 3257 case -1174686110: // statusChanged 3258 this.statusChanged = TypeConvertor.castToDateTime(value); // DateTimeType 3259 return value; 3260 case -1183762788: // intent 3261 value = new MedicationRequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 3262 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 3263 return value; 3264 case 50511102: // category 3265 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3266 return value; 3267 case -1165461084: // priority 3268 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3269 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3270 return value; 3271 case -1788508167: // doNotPerform 3272 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 3273 return value; 3274 case 1998965455: // medication 3275 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 3276 return value; 3277 case -1867885268: // subject 3278 this.subject = TypeConvertor.castToReference(value); // Reference 3279 return value; 3280 case -2123220889: // informationSource 3281 this.getInformationSource().add(TypeConvertor.castToReference(value)); // Reference 3282 return value; 3283 case 1524132147: // encounter 3284 this.encounter = TypeConvertor.castToReference(value); // Reference 3285 return value; 3286 case -1248768647: // supportingInformation 3287 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 3288 return value; 3289 case -1500852503: // authoredOn 3290 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 3291 return value; 3292 case 693933948: // requester 3293 this.requester = TypeConvertor.castToReference(value); // Reference 3294 return value; 3295 case -427039533: // reported 3296 this.reported = TypeConvertor.castToBoolean(value); // BooleanType 3297 return value; 3298 case -901444568: // performerType 3299 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3300 return value; 3301 case 481140686: // performer 3302 this.getPerformer().add(TypeConvertor.castToReference(value)); // Reference 3303 return value; 3304 case -1335157162: // device 3305 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 3306 return value; 3307 case -799233858: // recorder 3308 this.recorder = TypeConvertor.castToReference(value); // Reference 3309 return value; 3310 case -934964668: // reason 3311 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 3312 return value; 3313 case -447282031: // courseOfTherapyType 3314 this.courseOfTherapyType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3315 return value; 3316 case 73049818: // insurance 3317 this.getInsurance().add(TypeConvertor.castToReference(value)); // Reference 3318 return value; 3319 case 3387378: // note 3320 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3321 return value; 3322 case 1718902050: // renderedDosageInstruction 3323 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 3324 return value; 3325 case 322608453: // effectiveDosePeriod 3326 this.effectiveDosePeriod = TypeConvertor.castToPeriod(value); // Period 3327 return value; 3328 case -1201373865: // dosageInstruction 3329 this.getDosageInstruction().add(TypeConvertor.castToDosage(value)); // Dosage 3330 return value; 3331 case 824620658: // dispenseRequest 3332 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 3333 return value; 3334 case 826147581: // substitution 3335 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 3336 return value; 3337 case 1835190426: // eventHistory 3338 this.getEventHistory().add(TypeConvertor.castToReference(value)); // Reference 3339 return value; 3340 default: return super.setProperty(hash, name, value); 3341 } 3342 3343 } 3344 3345 @Override 3346 public Base setProperty(String name, Base value) throws FHIRException { 3347 if (name.equals("identifier")) { 3348 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3349 } else if (name.equals("basedOn")) { 3350 this.getBasedOn().add(TypeConvertor.castToReference(value)); 3351 } else if (name.equals("priorPrescription")) { 3352 this.priorPrescription = TypeConvertor.castToReference(value); // Reference 3353 } else if (name.equals("groupIdentifier")) { 3354 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 3355 } else if (name.equals("status")) { 3356 value = new MedicationrequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3357 this.status = (Enumeration) value; // Enumeration<MedicationrequestStatus> 3358 } else if (name.equals("statusReason")) { 3359 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3360 } else if (name.equals("statusChanged")) { 3361 this.statusChanged = TypeConvertor.castToDateTime(value); // DateTimeType 3362 } else if (name.equals("intent")) { 3363 value = new MedicationRequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 3364 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 3365 } else if (name.equals("category")) { 3366 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3367 } else if (name.equals("priority")) { 3368 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3369 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3370 } else if (name.equals("doNotPerform")) { 3371 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 3372 } else if (name.equals("medication")) { 3373 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 3374 } else if (name.equals("subject")) { 3375 this.subject = TypeConvertor.castToReference(value); // Reference 3376 } else if (name.equals("informationSource")) { 3377 this.getInformationSource().add(TypeConvertor.castToReference(value)); 3378 } else if (name.equals("encounter")) { 3379 this.encounter = TypeConvertor.castToReference(value); // Reference 3380 } else if (name.equals("supportingInformation")) { 3381 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 3382 } else if (name.equals("authoredOn")) { 3383 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 3384 } else if (name.equals("requester")) { 3385 this.requester = TypeConvertor.castToReference(value); // Reference 3386 } else if (name.equals("reported")) { 3387 this.reported = TypeConvertor.castToBoolean(value); // BooleanType 3388 } else if (name.equals("performerType")) { 3389 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3390 } else if (name.equals("performer")) { 3391 this.getPerformer().add(TypeConvertor.castToReference(value)); 3392 } else if (name.equals("device")) { 3393 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); 3394 } else if (name.equals("recorder")) { 3395 this.recorder = TypeConvertor.castToReference(value); // Reference 3396 } else if (name.equals("reason")) { 3397 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 3398 } else if (name.equals("courseOfTherapyType")) { 3399 this.courseOfTherapyType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3400 } else if (name.equals("insurance")) { 3401 this.getInsurance().add(TypeConvertor.castToReference(value)); 3402 } else if (name.equals("note")) { 3403 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3404 } else if (name.equals("renderedDosageInstruction")) { 3405 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 3406 } else if (name.equals("effectiveDosePeriod")) { 3407 this.effectiveDosePeriod = TypeConvertor.castToPeriod(value); // Period 3408 } else if (name.equals("dosageInstruction")) { 3409 this.getDosageInstruction().add(TypeConvertor.castToDosage(value)); 3410 } else if (name.equals("dispenseRequest")) { 3411 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 3412 } else if (name.equals("substitution")) { 3413 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 3414 } else if (name.equals("eventHistory")) { 3415 this.getEventHistory().add(TypeConvertor.castToReference(value)); 3416 } else 3417 return super.setProperty(name, value); 3418 return value; 3419 } 3420 3421 @Override 3422 public void removeChild(String name, Base value) throws FHIRException { 3423 if (name.equals("identifier")) { 3424 this.getIdentifier().remove(value); 3425 } else if (name.equals("basedOn")) { 3426 this.getBasedOn().remove(value); 3427 } else if (name.equals("priorPrescription")) { 3428 this.priorPrescription = null; 3429 } else if (name.equals("groupIdentifier")) { 3430 this.groupIdentifier = null; 3431 } else if (name.equals("status")) { 3432 value = new MedicationrequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3433 this.status = (Enumeration) value; // Enumeration<MedicationrequestStatus> 3434 } else if (name.equals("statusReason")) { 3435 this.statusReason = null; 3436 } else if (name.equals("statusChanged")) { 3437 this.statusChanged = null; 3438 } else if (name.equals("intent")) { 3439 value = new MedicationRequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 3440 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 3441 } else if (name.equals("category")) { 3442 this.getCategory().remove(value); 3443 } else if (name.equals("priority")) { 3444 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3445 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3446 } else if (name.equals("doNotPerform")) { 3447 this.doNotPerform = null; 3448 } else if (name.equals("medication")) { 3449 this.medication = null; 3450 } else if (name.equals("subject")) { 3451 this.subject = null; 3452 } else if (name.equals("informationSource")) { 3453 this.getInformationSource().remove(value); 3454 } else if (name.equals("encounter")) { 3455 this.encounter = null; 3456 } else if (name.equals("supportingInformation")) { 3457 this.getSupportingInformation().remove(value); 3458 } else if (name.equals("authoredOn")) { 3459 this.authoredOn = null; 3460 } else if (name.equals("requester")) { 3461 this.requester = null; 3462 } else if (name.equals("reported")) { 3463 this.reported = null; 3464 } else if (name.equals("performerType")) { 3465 this.performerType = null; 3466 } else if (name.equals("performer")) { 3467 this.getPerformer().remove(value); 3468 } else if (name.equals("device")) { 3469 this.getDevice().remove(value); 3470 } else if (name.equals("recorder")) { 3471 this.recorder = null; 3472 } else if (name.equals("reason")) { 3473 this.getReason().remove(value); 3474 } else if (name.equals("courseOfTherapyType")) { 3475 this.courseOfTherapyType = null; 3476 } else if (name.equals("insurance")) { 3477 this.getInsurance().remove(value); 3478 } else if (name.equals("note")) { 3479 this.getNote().remove(value); 3480 } else if (name.equals("renderedDosageInstruction")) { 3481 this.renderedDosageInstruction = null; 3482 } else if (name.equals("effectiveDosePeriod")) { 3483 this.effectiveDosePeriod = null; 3484 } else if (name.equals("dosageInstruction")) { 3485 this.getDosageInstruction().remove(value); 3486 } else if (name.equals("dispenseRequest")) { 3487 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 3488 } else if (name.equals("substitution")) { 3489 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 3490 } else if (name.equals("eventHistory")) { 3491 this.getEventHistory().remove(value); 3492 } else 3493 super.removeChild(name, value); 3494 3495 } 3496 3497 @Override 3498 public Base makeProperty(int hash, String name) throws FHIRException { 3499 switch (hash) { 3500 case -1618432855: return addIdentifier(); 3501 case -332612366: return addBasedOn(); 3502 case -486355964: return getPriorPrescription(); 3503 case -445338488: return getGroupIdentifier(); 3504 case -892481550: return getStatusElement(); 3505 case 2051346646: return getStatusReason(); 3506 case -1174686110: return getStatusChangedElement(); 3507 case -1183762788: return getIntentElement(); 3508 case 50511102: return addCategory(); 3509 case -1165461084: return getPriorityElement(); 3510 case -1788508167: return getDoNotPerformElement(); 3511 case 1998965455: return getMedication(); 3512 case -1867885268: return getSubject(); 3513 case -2123220889: return addInformationSource(); 3514 case 1524132147: return getEncounter(); 3515 case -1248768647: return addSupportingInformation(); 3516 case -1500852503: return getAuthoredOnElement(); 3517 case 693933948: return getRequester(); 3518 case -427039533: return getReportedElement(); 3519 case -901444568: return getPerformerType(); 3520 case 481140686: return addPerformer(); 3521 case -1335157162: return addDevice(); 3522 case -799233858: return getRecorder(); 3523 case -934964668: return addReason(); 3524 case -447282031: return getCourseOfTherapyType(); 3525 case 73049818: return addInsurance(); 3526 case 3387378: return addNote(); 3527 case 1718902050: return getRenderedDosageInstructionElement(); 3528 case 322608453: return getEffectiveDosePeriod(); 3529 case -1201373865: return addDosageInstruction(); 3530 case 824620658: return getDispenseRequest(); 3531 case 826147581: return getSubstitution(); 3532 case 1835190426: return addEventHistory(); 3533 default: return super.makeProperty(hash, name); 3534 } 3535 3536 } 3537 3538 @Override 3539 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3540 switch (hash) { 3541 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3542 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3543 case -486355964: /*priorPrescription*/ return new String[] {"Reference"}; 3544 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 3545 case -892481550: /*status*/ return new String[] {"code"}; 3546 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 3547 case -1174686110: /*statusChanged*/ return new String[] {"dateTime"}; 3548 case -1183762788: /*intent*/ return new String[] {"code"}; 3549 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3550 case -1165461084: /*priority*/ return new String[] {"code"}; 3551 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 3552 case 1998965455: /*medication*/ return new String[] {"CodeableReference"}; 3553 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3554 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 3555 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3556 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 3557 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 3558 case 693933948: /*requester*/ return new String[] {"Reference"}; 3559 case -427039533: /*reported*/ return new String[] {"boolean"}; 3560 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 3561 case 481140686: /*performer*/ return new String[] {"Reference"}; 3562 case -1335157162: /*device*/ return new String[] {"CodeableReference"}; 3563 case -799233858: /*recorder*/ return new String[] {"Reference"}; 3564 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 3565 case -447282031: /*courseOfTherapyType*/ return new String[] {"CodeableConcept"}; 3566 case 73049818: /*insurance*/ return new String[] {"Reference"}; 3567 case 3387378: /*note*/ return new String[] {"Annotation"}; 3568 case 1718902050: /*renderedDosageInstruction*/ return new String[] {"markdown"}; 3569 case 322608453: /*effectiveDosePeriod*/ return new String[] {"Period"}; 3570 case -1201373865: /*dosageInstruction*/ return new String[] {"Dosage"}; 3571 case 824620658: /*dispenseRequest*/ return new String[] {}; 3572 case 826147581: /*substitution*/ return new String[] {}; 3573 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 3574 default: return super.getTypesForProperty(hash, name); 3575 } 3576 3577 } 3578 3579 @Override 3580 public Base addChild(String name) throws FHIRException { 3581 if (name.equals("identifier")) { 3582 return addIdentifier(); 3583 } 3584 else if (name.equals("basedOn")) { 3585 return addBasedOn(); 3586 } 3587 else if (name.equals("priorPrescription")) { 3588 this.priorPrescription = new Reference(); 3589 return this.priorPrescription; 3590 } 3591 else if (name.equals("groupIdentifier")) { 3592 this.groupIdentifier = new Identifier(); 3593 return this.groupIdentifier; 3594 } 3595 else if (name.equals("status")) { 3596 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.status"); 3597 } 3598 else if (name.equals("statusReason")) { 3599 this.statusReason = new CodeableConcept(); 3600 return this.statusReason; 3601 } 3602 else if (name.equals("statusChanged")) { 3603 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.statusChanged"); 3604 } 3605 else if (name.equals("intent")) { 3606 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.intent"); 3607 } 3608 else if (name.equals("category")) { 3609 return addCategory(); 3610 } 3611 else if (name.equals("priority")) { 3612 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.priority"); 3613 } 3614 else if (name.equals("doNotPerform")) { 3615 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.doNotPerform"); 3616 } 3617 else if (name.equals("medication")) { 3618 this.medication = new CodeableReference(); 3619 return this.medication; 3620 } 3621 else if (name.equals("subject")) { 3622 this.subject = new Reference(); 3623 return this.subject; 3624 } 3625 else if (name.equals("informationSource")) { 3626 return addInformationSource(); 3627 } 3628 else if (name.equals("encounter")) { 3629 this.encounter = new Reference(); 3630 return this.encounter; 3631 } 3632 else if (name.equals("supportingInformation")) { 3633 return addSupportingInformation(); 3634 } 3635 else if (name.equals("authoredOn")) { 3636 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.authoredOn"); 3637 } 3638 else if (name.equals("requester")) { 3639 this.requester = new Reference(); 3640 return this.requester; 3641 } 3642 else if (name.equals("reported")) { 3643 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.reported"); 3644 } 3645 else if (name.equals("performerType")) { 3646 this.performerType = new CodeableConcept(); 3647 return this.performerType; 3648 } 3649 else if (name.equals("performer")) { 3650 return addPerformer(); 3651 } 3652 else if (name.equals("device")) { 3653 return addDevice(); 3654 } 3655 else if (name.equals("recorder")) { 3656 this.recorder = new Reference(); 3657 return this.recorder; 3658 } 3659 else if (name.equals("reason")) { 3660 return addReason(); 3661 } 3662 else if (name.equals("courseOfTherapyType")) { 3663 this.courseOfTherapyType = new CodeableConcept(); 3664 return this.courseOfTherapyType; 3665 } 3666 else if (name.equals("insurance")) { 3667 return addInsurance(); 3668 } 3669 else if (name.equals("note")) { 3670 return addNote(); 3671 } 3672 else if (name.equals("renderedDosageInstruction")) { 3673 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.renderedDosageInstruction"); 3674 } 3675 else if (name.equals("effectiveDosePeriod")) { 3676 this.effectiveDosePeriod = new Period(); 3677 return this.effectiveDosePeriod; 3678 } 3679 else if (name.equals("dosageInstruction")) { 3680 return addDosageInstruction(); 3681 } 3682 else if (name.equals("dispenseRequest")) { 3683 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); 3684 return this.dispenseRequest; 3685 } 3686 else if (name.equals("substitution")) { 3687 this.substitution = new MedicationRequestSubstitutionComponent(); 3688 return this.substitution; 3689 } 3690 else if (name.equals("eventHistory")) { 3691 return addEventHistory(); 3692 } 3693 else 3694 return super.addChild(name); 3695 } 3696 3697 public String fhirType() { 3698 return "MedicationRequest"; 3699 3700 } 3701 3702 public MedicationRequest copy() { 3703 MedicationRequest dst = new MedicationRequest(); 3704 copyValues(dst); 3705 return dst; 3706 } 3707 3708 public void copyValues(MedicationRequest dst) { 3709 super.copyValues(dst); 3710 if (identifier != null) { 3711 dst.identifier = new ArrayList<Identifier>(); 3712 for (Identifier i : identifier) 3713 dst.identifier.add(i.copy()); 3714 }; 3715 if (basedOn != null) { 3716 dst.basedOn = new ArrayList<Reference>(); 3717 for (Reference i : basedOn) 3718 dst.basedOn.add(i.copy()); 3719 }; 3720 dst.priorPrescription = priorPrescription == null ? null : priorPrescription.copy(); 3721 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 3722 dst.status = status == null ? null : status.copy(); 3723 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3724 dst.statusChanged = statusChanged == null ? null : statusChanged.copy(); 3725 dst.intent = intent == null ? null : intent.copy(); 3726 if (category != null) { 3727 dst.category = new ArrayList<CodeableConcept>(); 3728 for (CodeableConcept i : category) 3729 dst.category.add(i.copy()); 3730 }; 3731 dst.priority = priority == null ? null : priority.copy(); 3732 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 3733 dst.medication = medication == null ? null : medication.copy(); 3734 dst.subject = subject == null ? null : subject.copy(); 3735 if (informationSource != null) { 3736 dst.informationSource = new ArrayList<Reference>(); 3737 for (Reference i : informationSource) 3738 dst.informationSource.add(i.copy()); 3739 }; 3740 dst.encounter = encounter == null ? null : encounter.copy(); 3741 if (supportingInformation != null) { 3742 dst.supportingInformation = new ArrayList<Reference>(); 3743 for (Reference i : supportingInformation) 3744 dst.supportingInformation.add(i.copy()); 3745 }; 3746 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 3747 dst.requester = requester == null ? null : requester.copy(); 3748 dst.reported = reported == null ? null : reported.copy(); 3749 dst.performerType = performerType == null ? null : performerType.copy(); 3750 if (performer != null) { 3751 dst.performer = new ArrayList<Reference>(); 3752 for (Reference i : performer) 3753 dst.performer.add(i.copy()); 3754 }; 3755 if (device != null) { 3756 dst.device = new ArrayList<CodeableReference>(); 3757 for (CodeableReference i : device) 3758 dst.device.add(i.copy()); 3759 }; 3760 dst.recorder = recorder == null ? null : recorder.copy(); 3761 if (reason != null) { 3762 dst.reason = new ArrayList<CodeableReference>(); 3763 for (CodeableReference i : reason) 3764 dst.reason.add(i.copy()); 3765 }; 3766 dst.courseOfTherapyType = courseOfTherapyType == null ? null : courseOfTherapyType.copy(); 3767 if (insurance != null) { 3768 dst.insurance = new ArrayList<Reference>(); 3769 for (Reference i : insurance) 3770 dst.insurance.add(i.copy()); 3771 }; 3772 if (note != null) { 3773 dst.note = new ArrayList<Annotation>(); 3774 for (Annotation i : note) 3775 dst.note.add(i.copy()); 3776 }; 3777 dst.renderedDosageInstruction = renderedDosageInstruction == null ? null : renderedDosageInstruction.copy(); 3778 dst.effectiveDosePeriod = effectiveDosePeriod == null ? null : effectiveDosePeriod.copy(); 3779 if (dosageInstruction != null) { 3780 dst.dosageInstruction = new ArrayList<Dosage>(); 3781 for (Dosage i : dosageInstruction) 3782 dst.dosageInstruction.add(i.copy()); 3783 }; 3784 dst.dispenseRequest = dispenseRequest == null ? null : dispenseRequest.copy(); 3785 dst.substitution = substitution == null ? null : substitution.copy(); 3786 if (eventHistory != null) { 3787 dst.eventHistory = new ArrayList<Reference>(); 3788 for (Reference i : eventHistory) 3789 dst.eventHistory.add(i.copy()); 3790 }; 3791 } 3792 3793 protected MedicationRequest typedCopy() { 3794 return copy(); 3795 } 3796 3797 @Override 3798 public boolean equalsDeep(Base other_) { 3799 if (!super.equalsDeep(other_)) 3800 return false; 3801 if (!(other_ instanceof MedicationRequest)) 3802 return false; 3803 MedicationRequest o = (MedicationRequest) other_; 3804 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(priorPrescription, o.priorPrescription, true) 3805 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(status, o.status, true) 3806 && compareDeep(statusReason, o.statusReason, true) && compareDeep(statusChanged, o.statusChanged, true) 3807 && compareDeep(intent, o.intent, true) && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 3808 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(medication, o.medication, true) 3809 && compareDeep(subject, o.subject, true) && compareDeep(informationSource, o.informationSource, true) 3810 && compareDeep(encounter, o.encounter, true) && compareDeep(supportingInformation, o.supportingInformation, true) 3811 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) && compareDeep(reported, o.reported, true) 3812 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 3813 && compareDeep(device, o.device, true) && compareDeep(recorder, o.recorder, true) && compareDeep(reason, o.reason, true) 3814 && compareDeep(courseOfTherapyType, o.courseOfTherapyType, true) && compareDeep(insurance, o.insurance, true) 3815 && compareDeep(note, o.note, true) && compareDeep(renderedDosageInstruction, o.renderedDosageInstruction, true) 3816 && compareDeep(effectiveDosePeriod, o.effectiveDosePeriod, true) && compareDeep(dosageInstruction, o.dosageInstruction, true) 3817 && compareDeep(dispenseRequest, o.dispenseRequest, true) && compareDeep(substitution, o.substitution, true) 3818 && compareDeep(eventHistory, o.eventHistory, true); 3819 } 3820 3821 @Override 3822 public boolean equalsShallow(Base other_) { 3823 if (!super.equalsShallow(other_)) 3824 return false; 3825 if (!(other_ instanceof MedicationRequest)) 3826 return false; 3827 MedicationRequest o = (MedicationRequest) other_; 3828 return compareValues(status, o.status, true) && compareValues(statusChanged, o.statusChanged, true) 3829 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) && compareValues(doNotPerform, o.doNotPerform, true) 3830 && compareValues(authoredOn, o.authoredOn, true) && compareValues(reported, o.reported, true) && compareValues(renderedDosageInstruction, o.renderedDosageInstruction, true) 3831 ; 3832 } 3833 3834 public boolean isEmpty() { 3835 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, priorPrescription 3836 , groupIdentifier, status, statusReason, statusChanged, intent, category, priority 3837 , doNotPerform, medication, subject, informationSource, encounter, supportingInformation 3838 , authoredOn, requester, reported, performerType, performer, device, recorder 3839 , reason, courseOfTherapyType, insurance, note, renderedDosageInstruction, effectiveDosePeriod 3840 , dosageInstruction, dispenseRequest, substitution, eventHistory); 3841 } 3842 3843 @Override 3844 public ResourceType getResourceType() { 3845 return ResourceType.MedicationRequest; 3846 } 3847 3848 /** 3849 * Search parameter: <b>authoredon</b> 3850 * <p> 3851 * Description: <b>Return prescriptions written on this date</b><br> 3852 * Type: <b>date</b><br> 3853 * Path: <b>MedicationRequest.authoredOn</b><br> 3854 * </p> 3855 */ 3856 @SearchParamDefinition(name="authoredon", path="MedicationRequest.authoredOn", description="Return prescriptions written on this date", type="date" ) 3857 public static final String SP_AUTHOREDON = "authoredon"; 3858 /** 3859 * <b>Fluent Client</b> search parameter constant for <b>authoredon</b> 3860 * <p> 3861 * Description: <b>Return prescriptions written on this date</b><br> 3862 * Type: <b>date</b><br> 3863 * Path: <b>MedicationRequest.authoredOn</b><br> 3864 * </p> 3865 */ 3866 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHOREDON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHOREDON); 3867 3868 /** 3869 * Search parameter: <b>category</b> 3870 * <p> 3871 * Description: <b>Returns prescriptions with different categories</b><br> 3872 * Type: <b>token</b><br> 3873 * Path: <b>MedicationRequest.category</b><br> 3874 * </p> 3875 */ 3876 @SearchParamDefinition(name="category", path="MedicationRequest.category", description="Returns prescriptions with different categories", type="token" ) 3877 public static final String SP_CATEGORY = "category"; 3878 /** 3879 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3880 * <p> 3881 * Description: <b>Returns prescriptions with different categories</b><br> 3882 * Type: <b>token</b><br> 3883 * Path: <b>MedicationRequest.category</b><br> 3884 * </p> 3885 */ 3886 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3887 3888 /** 3889 * Search parameter: <b>combo-date</b> 3890 * <p> 3891 * Description: <b>Returns medication request to be administered on a specific date or within a date range</b><br> 3892 * Type: <b>date</b><br> 3893 * Path: <b>MedicationRequest.dosageInstruction.timing.event | (MedicationRequest.dosageInstruction.timing.repeat.bounds.ofType(Period))</b><br> 3894 * </p> 3895 */ 3896 @SearchParamDefinition(name="combo-date", path="MedicationRequest.dosageInstruction.timing.event | (MedicationRequest.dosageInstruction.timing.repeat.bounds.ofType(Period))", description="Returns medication request to be administered on a specific date or within a date range", type="date" ) 3897 public static final String SP_COMBO_DATE = "combo-date"; 3898 /** 3899 * <b>Fluent Client</b> search parameter constant for <b>combo-date</b> 3900 * <p> 3901 * Description: <b>Returns medication request to be administered on a specific date or within a date range</b><br> 3902 * Type: <b>date</b><br> 3903 * Path: <b>MedicationRequest.dosageInstruction.timing.event | (MedicationRequest.dosageInstruction.timing.repeat.bounds.ofType(Period))</b><br> 3904 * </p> 3905 */ 3906 public static final ca.uhn.fhir.rest.gclient.DateClientParam COMBO_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_COMBO_DATE); 3907 3908 /** 3909 * Search parameter: <b>group-identifier</b> 3910 * <p> 3911 * Description: <b>Composite request this is part of</b><br> 3912 * Type: <b>token</b><br> 3913 * Path: <b>MedicationRequest.groupIdentifier</b><br> 3914 * </p> 3915 */ 3916 @SearchParamDefinition(name="group-identifier", path="MedicationRequest.groupIdentifier", description="Composite request this is part of", type="token" ) 3917 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 3918 /** 3919 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 3920 * <p> 3921 * Description: <b>Composite request this is part of</b><br> 3922 * Type: <b>token</b><br> 3923 * Path: <b>MedicationRequest.groupIdentifier</b><br> 3924 * </p> 3925 */ 3926 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 3927 3928 /** 3929 * Search parameter: <b>intended-dispenser</b> 3930 * <p> 3931 * Description: <b>Returns prescriptions intended to be dispensed by this Organization</b><br> 3932 * Type: <b>reference</b><br> 3933 * Path: <b>MedicationRequest.dispenseRequest.dispenser</b><br> 3934 * </p> 3935 */ 3936 @SearchParamDefinition(name="intended-dispenser", path="MedicationRequest.dispenseRequest.dispenser", description="Returns prescriptions intended to be dispensed by this Organization", type="reference", target={Organization.class } ) 3937 public static final String SP_INTENDED_DISPENSER = "intended-dispenser"; 3938 /** 3939 * <b>Fluent Client</b> search parameter constant for <b>intended-dispenser</b> 3940 * <p> 3941 * Description: <b>Returns prescriptions intended to be dispensed by this Organization</b><br> 3942 * Type: <b>reference</b><br> 3943 * Path: <b>MedicationRequest.dispenseRequest.dispenser</b><br> 3944 * </p> 3945 */ 3946 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTENDED_DISPENSER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INTENDED_DISPENSER); 3947 3948/** 3949 * Constant for fluent queries to be used to add include statements. Specifies 3950 * the path value of "<b>MedicationRequest:intended-dispenser</b>". 3951 */ 3952 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTENDED_DISPENSER = new ca.uhn.fhir.model.api.Include("MedicationRequest:intended-dispenser").toLocked(); 3953 3954 /** 3955 * Search parameter: <b>intended-performer</b> 3956 * <p> 3957 * Description: <b>Returns the intended performer of the administration of the medication request</b><br> 3958 * Type: <b>reference</b><br> 3959 * Path: <b>MedicationRequest.performer</b><br> 3960 * </p> 3961 */ 3962 @SearchParamDefinition(name="intended-performer", path="MedicationRequest.performer", description="Returns the intended performer of the administration of the medication request", type="reference", target={CareTeam.class, DeviceDefinition.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3963 public static final String SP_INTENDED_PERFORMER = "intended-performer"; 3964 /** 3965 * <b>Fluent Client</b> search parameter constant for <b>intended-performer</b> 3966 * <p> 3967 * Description: <b>Returns the intended performer of the administration of the medication request</b><br> 3968 * Type: <b>reference</b><br> 3969 * Path: <b>MedicationRequest.performer</b><br> 3970 * </p> 3971 */ 3972 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTENDED_PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INTENDED_PERFORMER); 3973 3974/** 3975 * Constant for fluent queries to be used to add include statements. Specifies 3976 * the path value of "<b>MedicationRequest:intended-performer</b>". 3977 */ 3978 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTENDED_PERFORMER = new ca.uhn.fhir.model.api.Include("MedicationRequest:intended-performer").toLocked(); 3979 3980 /** 3981 * Search parameter: <b>intended-performertype</b> 3982 * <p> 3983 * Description: <b>Returns requests for a specific type of performer</b><br> 3984 * Type: <b>token</b><br> 3985 * Path: <b>MedicationRequest.performerType</b><br> 3986 * </p> 3987 */ 3988 @SearchParamDefinition(name="intended-performertype", path="MedicationRequest.performerType", description="Returns requests for a specific type of performer", type="token" ) 3989 public static final String SP_INTENDED_PERFORMERTYPE = "intended-performertype"; 3990 /** 3991 * <b>Fluent Client</b> search parameter constant for <b>intended-performertype</b> 3992 * <p> 3993 * Description: <b>Returns requests for a specific type of performer</b><br> 3994 * Type: <b>token</b><br> 3995 * Path: <b>MedicationRequest.performerType</b><br> 3996 * </p> 3997 */ 3998 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENDED_PERFORMERTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENDED_PERFORMERTYPE); 3999 4000 /** 4001 * Search parameter: <b>intent</b> 4002 * <p> 4003 * Description: <b>Returns prescriptions with different intents</b><br> 4004 * Type: <b>token</b><br> 4005 * Path: <b>MedicationRequest.intent</b><br> 4006 * </p> 4007 */ 4008 @SearchParamDefinition(name="intent", path="MedicationRequest.intent", description="Returns prescriptions with different intents", type="token" ) 4009 public static final String SP_INTENT = "intent"; 4010 /** 4011 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 4012 * <p> 4013 * Description: <b>Returns prescriptions with different intents</b><br> 4014 * Type: <b>token</b><br> 4015 * Path: <b>MedicationRequest.intent</b><br> 4016 * </p> 4017 */ 4018 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 4019 4020 /** 4021 * Search parameter: <b>priority</b> 4022 * <p> 4023 * Description: <b>Returns prescriptions with different priorities</b><br> 4024 * Type: <b>token</b><br> 4025 * Path: <b>MedicationRequest.priority</b><br> 4026 * </p> 4027 */ 4028 @SearchParamDefinition(name="priority", path="MedicationRequest.priority", description="Returns prescriptions with different priorities", type="token" ) 4029 public static final String SP_PRIORITY = "priority"; 4030 /** 4031 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 4032 * <p> 4033 * Description: <b>Returns prescriptions with different priorities</b><br> 4034 * Type: <b>token</b><br> 4035 * Path: <b>MedicationRequest.priority</b><br> 4036 * </p> 4037 */ 4038 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 4039 4040 /** 4041 * Search parameter: <b>requester</b> 4042 * <p> 4043 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 4044 * Type: <b>reference</b><br> 4045 * Path: <b>MedicationRequest.requester</b><br> 4046 * </p> 4047 */ 4048 @SearchParamDefinition(name="requester", path="MedicationRequest.requester", description="Returns prescriptions prescribed by this prescriber", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 4049 public static final String SP_REQUESTER = "requester"; 4050 /** 4051 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 4052 * <p> 4053 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 4054 * Type: <b>reference</b><br> 4055 * Path: <b>MedicationRequest.requester</b><br> 4056 * </p> 4057 */ 4058 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 4059 4060/** 4061 * Constant for fluent queries to be used to add include statements. Specifies 4062 * the path value of "<b>MedicationRequest:requester</b>". 4063 */ 4064 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("MedicationRequest:requester").toLocked(); 4065 4066 /** 4067 * Search parameter: <b>subject</b> 4068 * <p> 4069 * Description: <b>The identity of a patient to list orders for</b><br> 4070 * Type: <b>reference</b><br> 4071 * Path: <b>MedicationRequest.subject</b><br> 4072 * </p> 4073 */ 4074 @SearchParamDefinition(name="subject", path="MedicationRequest.subject", description="The identity of a patient to list orders for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 4075 public static final String SP_SUBJECT = "subject"; 4076 /** 4077 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4078 * <p> 4079 * Description: <b>The identity of a patient to list orders for</b><br> 4080 * Type: <b>reference</b><br> 4081 * Path: <b>MedicationRequest.subject</b><br> 4082 * </p> 4083 */ 4084 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4085 4086/** 4087 * Constant for fluent queries to be used to add include statements. Specifies 4088 * the path value of "<b>MedicationRequest:subject</b>". 4089 */ 4090 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationRequest:subject").toLocked(); 4091 4092 /** 4093 * Search parameter: <b>code</b> 4094 * <p> 4095 * Description: <b>Multiple Resources: 4096 4097* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4098* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4099* [AuditEvent](auditevent.html): More specific code for the event 4100* [Basic](basic.html): Kind of Resource 4101* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4102* [Condition](condition.html): Code for the condition 4103* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4104* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4105* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4106* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4107* [ImagingSelection](imagingselection.html): The imaging selection status 4108* [List](list.html): What the purpose of this list is 4109* [Medication](medication.html): Returns medications for a specific code 4110* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4111* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4112* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4113* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4114* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4115* [Observation](observation.html): The code of the observation type 4116* [Procedure](procedure.html): A code to identify a procedure 4117* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4118* [Task](task.html): Search by task code 4119</b><br> 4120 * Type: <b>token</b><br> 4121 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4122 * </p> 4123 */ 4124 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 4125 public static final String SP_CODE = "code"; 4126 /** 4127 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4128 * <p> 4129 * Description: <b>Multiple Resources: 4130 4131* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4132* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4133* [AuditEvent](auditevent.html): More specific code for the event 4134* [Basic](basic.html): Kind of Resource 4135* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4136* [Condition](condition.html): Code for the condition 4137* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4138* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4139* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4140* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4141* [ImagingSelection](imagingselection.html): The imaging selection status 4142* [List](list.html): What the purpose of this list is 4143* [Medication](medication.html): Returns medications for a specific code 4144* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4145* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4146* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4147* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4148* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4149* [Observation](observation.html): The code of the observation type 4150* [Procedure](procedure.html): A code to identify a procedure 4151* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4152* [Task](task.html): Search by task code 4153</b><br> 4154 * Type: <b>token</b><br> 4155 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4156 * </p> 4157 */ 4158 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4159 4160 /** 4161 * Search parameter: <b>identifier</b> 4162 * <p> 4163 * Description: <b>Multiple Resources: 4164 4165* [Account](account.html): Account number 4166* [AdverseEvent](adverseevent.html): Business identifier for the event 4167* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4168* [Appointment](appointment.html): An Identifier of the Appointment 4169* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4170* [Basic](basic.html): Business identifier 4171* [BodyStructure](bodystructure.html): Bodystructure identifier 4172* [CarePlan](careplan.html): External Ids for this plan 4173* [CareTeam](careteam.html): External Ids for this team 4174* [ChargeItem](chargeitem.html): Business Identifier for item 4175* [Claim](claim.html): The primary identifier of the financial resource 4176* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4177* [ClinicalImpression](clinicalimpression.html): Business identifier 4178* [Communication](communication.html): Unique identifier 4179* [CommunicationRequest](communicationrequest.html): Unique identifier 4180* [Composition](composition.html): Version-independent identifier for the Composition 4181* [Condition](condition.html): A unique identifier of the condition record 4182* [Consent](consent.html): Identifier for this record (external references) 4183* [Contract](contract.html): The identity of the contract 4184* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4185* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4186* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4187* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4188* [DeviceRequest](devicerequest.html): Business identifier for request/order 4189* [DeviceUsage](deviceusage.html): Search by identifier 4190* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4191* [DocumentReference](documentreference.html): Identifier of the attachment binary 4192* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4193* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4194* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4195* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4196* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4197* [Flag](flag.html): Business identifier 4198* [Goal](goal.html): External Ids for this goal 4199* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4200* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4201* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4202* [Immunization](immunization.html): Business identifier 4203* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4204* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4205* [Invoice](invoice.html): Business Identifier for item 4206* [List](list.html): Business identifier 4207* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4208* [Medication](medication.html): Returns medications with this external identifier 4209* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4210* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4211* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4212* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4213* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4214* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4215* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4216* [Observation](observation.html): The unique id for a particular observation 4217* [Person](person.html): A person Identifier 4218* [Procedure](procedure.html): A unique identifier for a procedure 4219* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4220* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4221* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4222* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4223* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4224* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4225* [Specimen](specimen.html): The unique identifier associated with the specimen 4226* [SupplyDelivery](supplydelivery.html): External identifier 4227* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4228* [Task](task.html): Search for a task instance by its business identifier 4229* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4230</b><br> 4231 * Type: <b>token</b><br> 4232 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4233 * </p> 4234 */ 4235 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4236 public static final String SP_IDENTIFIER = "identifier"; 4237 /** 4238 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4239 * <p> 4240 * Description: <b>Multiple Resources: 4241 4242* [Account](account.html): Account number 4243* [AdverseEvent](adverseevent.html): Business identifier for the event 4244* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4245* [Appointment](appointment.html): An Identifier of the Appointment 4246* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4247* [Basic](basic.html): Business identifier 4248* [BodyStructure](bodystructure.html): Bodystructure identifier 4249* [CarePlan](careplan.html): External Ids for this plan 4250* [CareTeam](careteam.html): External Ids for this team 4251* [ChargeItem](chargeitem.html): Business Identifier for item 4252* [Claim](claim.html): The primary identifier of the financial resource 4253* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4254* [ClinicalImpression](clinicalimpression.html): Business identifier 4255* [Communication](communication.html): Unique identifier 4256* [CommunicationRequest](communicationrequest.html): Unique identifier 4257* [Composition](composition.html): Version-independent identifier for the Composition 4258* [Condition](condition.html): A unique identifier of the condition record 4259* [Consent](consent.html): Identifier for this record (external references) 4260* [Contract](contract.html): The identity of the contract 4261* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4262* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4263* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4264* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4265* [DeviceRequest](devicerequest.html): Business identifier for request/order 4266* [DeviceUsage](deviceusage.html): Search by identifier 4267* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4268* [DocumentReference](documentreference.html): Identifier of the attachment binary 4269* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4270* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4271* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4272* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4273* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4274* [Flag](flag.html): Business identifier 4275* [Goal](goal.html): External Ids for this goal 4276* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4277* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4278* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4279* [Immunization](immunization.html): Business identifier 4280* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4281* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4282* [Invoice](invoice.html): Business Identifier for item 4283* [List](list.html): Business identifier 4284* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4285* [Medication](medication.html): Returns medications with this external identifier 4286* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4287* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4288* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4289* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4290* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4291* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4292* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4293* [Observation](observation.html): The unique id for a particular observation 4294* [Person](person.html): A person Identifier 4295* [Procedure](procedure.html): A unique identifier for a procedure 4296* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4297* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4298* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4299* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4300* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4301* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4302* [Specimen](specimen.html): The unique identifier associated with the specimen 4303* [SupplyDelivery](supplydelivery.html): External identifier 4304* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4305* [Task](task.html): Search for a task instance by its business identifier 4306* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4307</b><br> 4308 * Type: <b>token</b><br> 4309 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4310 * </p> 4311 */ 4312 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4313 4314 /** 4315 * Search parameter: <b>patient</b> 4316 * <p> 4317 * Description: <b>Multiple Resources: 4318 4319* [Account](account.html): The entity that caused the expenses 4320* [AdverseEvent](adverseevent.html): Subject impacted by event 4321* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4322* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4323* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4324* [AuditEvent](auditevent.html): Where the activity involved patient data 4325* [Basic](basic.html): Identifies the focus of this resource 4326* [BodyStructure](bodystructure.html): Who this is about 4327* [CarePlan](careplan.html): Who the care plan is for 4328* [CareTeam](careteam.html): Who care team is for 4329* [ChargeItem](chargeitem.html): Individual service was done for/to 4330* [Claim](claim.html): Patient receiving the products or services 4331* [ClaimResponse](claimresponse.html): The subject of care 4332* [ClinicalImpression](clinicalimpression.html): Patient assessed 4333* [Communication](communication.html): Focus of message 4334* [CommunicationRequest](communicationrequest.html): Focus of message 4335* [Composition](composition.html): Who and/or what the composition is about 4336* [Condition](condition.html): Who has the condition? 4337* [Consent](consent.html): Who the consent applies to 4338* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4339* [Coverage](coverage.html): Retrieve coverages for a patient 4340* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4341* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4342* [DetectedIssue](detectedissue.html): Associated patient 4343* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4344* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4345* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4346* [DocumentReference](documentreference.html): Who/what is the subject of the document 4347* [Encounter](encounter.html): The patient present at the encounter 4348* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4349* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4350* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4351* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4352* [Flag](flag.html): The identity of a subject to list flags for 4353* [Goal](goal.html): Who this goal is intended for 4354* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4355* [ImagingSelection](imagingselection.html): Who the study is about 4356* [ImagingStudy](imagingstudy.html): Who the study is about 4357* [Immunization](immunization.html): The patient for the vaccination record 4358* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4359* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4360* [Invoice](invoice.html): Recipient(s) of goods and services 4361* [List](list.html): If all resources have the same subject 4362* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4363* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4364* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4365* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4366* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4367* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4368* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4369* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4370* [Observation](observation.html): The subject that the observation is about (if patient) 4371* [Person](person.html): The Person links to this Patient 4372* [Procedure](procedure.html): Search by subject - a patient 4373* [Provenance](provenance.html): Where the activity involved patient data 4374* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4375* [RelatedPerson](relatedperson.html): The patient this related person is related to 4376* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4377* [ResearchSubject](researchsubject.html): Who or what is part of study 4378* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4379* [ServiceRequest](servicerequest.html): Search by subject - a patient 4380* [Specimen](specimen.html): The patient the specimen comes from 4381* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4382* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4383* [Task](task.html): Search by patient 4384* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4385</b><br> 4386 * Type: <b>reference</b><br> 4387 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4388 * </p> 4389 */ 4390 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4391 public static final String SP_PATIENT = "patient"; 4392 /** 4393 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4394 * <p> 4395 * Description: <b>Multiple Resources: 4396 4397* [Account](account.html): The entity that caused the expenses 4398* [AdverseEvent](adverseevent.html): Subject impacted by event 4399* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4400* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4401* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4402* [AuditEvent](auditevent.html): Where the activity involved patient data 4403* [Basic](basic.html): Identifies the focus of this resource 4404* [BodyStructure](bodystructure.html): Who this is about 4405* [CarePlan](careplan.html): Who the care plan is for 4406* [CareTeam](careteam.html): Who care team is for 4407* [ChargeItem](chargeitem.html): Individual service was done for/to 4408* [Claim](claim.html): Patient receiving the products or services 4409* [ClaimResponse](claimresponse.html): The subject of care 4410* [ClinicalImpression](clinicalimpression.html): Patient assessed 4411* [Communication](communication.html): Focus of message 4412* [CommunicationRequest](communicationrequest.html): Focus of message 4413* [Composition](composition.html): Who and/or what the composition is about 4414* [Condition](condition.html): Who has the condition? 4415* [Consent](consent.html): Who the consent applies to 4416* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4417* [Coverage](coverage.html): Retrieve coverages for a patient 4418* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4419* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4420* [DetectedIssue](detectedissue.html): Associated patient 4421* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4422* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4423* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4424* [DocumentReference](documentreference.html): Who/what is the subject of the document 4425* [Encounter](encounter.html): The patient present at the encounter 4426* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4427* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4428* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4429* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4430* [Flag](flag.html): The identity of a subject to list flags for 4431* [Goal](goal.html): Who this goal is intended for 4432* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4433* [ImagingSelection](imagingselection.html): Who the study is about 4434* [ImagingStudy](imagingstudy.html): Who the study is about 4435* [Immunization](immunization.html): The patient for the vaccination record 4436* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4437* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4438* [Invoice](invoice.html): Recipient(s) of goods and services 4439* [List](list.html): If all resources have the same subject 4440* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4441* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4442* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4443* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4444* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4445* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4446* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4447* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4448* [Observation](observation.html): The subject that the observation is about (if patient) 4449* [Person](person.html): The Person links to this Patient 4450* [Procedure](procedure.html): Search by subject - a patient 4451* [Provenance](provenance.html): Where the activity involved patient data 4452* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4453* [RelatedPerson](relatedperson.html): The patient this related person is related to 4454* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4455* [ResearchSubject](researchsubject.html): Who or what is part of study 4456* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4457* [ServiceRequest](servicerequest.html): Search by subject - a patient 4458* [Specimen](specimen.html): The patient the specimen comes from 4459* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4460* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4461* [Task](task.html): Search by patient 4462* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4463</b><br> 4464 * Type: <b>reference</b><br> 4465 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4466 * </p> 4467 */ 4468 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4469 4470/** 4471 * Constant for fluent queries to be used to add include statements. Specifies 4472 * the path value of "<b>MedicationRequest:patient</b>". 4473 */ 4474 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationRequest:patient").toLocked(); 4475 4476 /** 4477 * Search parameter: <b>encounter</b> 4478 * <p> 4479 * Description: <b>Multiple Resources: 4480 4481* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter 4482* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier 4483</b><br> 4484 * Type: <b>reference</b><br> 4485 * Path: <b>MedicationAdministration.encounter | MedicationRequest.encounter</b><br> 4486 * </p> 4487 */ 4488 @SearchParamDefinition(name="encounter", path="MedicationAdministration.encounter | MedicationRequest.encounter", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 4489 public static final String SP_ENCOUNTER = "encounter"; 4490 /** 4491 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4492 * <p> 4493 * Description: <b>Multiple Resources: 4494 4495* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter 4496* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier 4497</b><br> 4498 * Type: <b>reference</b><br> 4499 * Path: <b>MedicationAdministration.encounter | MedicationRequest.encounter</b><br> 4500 * </p> 4501 */ 4502 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4503 4504/** 4505 * Constant for fluent queries to be used to add include statements. Specifies 4506 * the path value of "<b>MedicationRequest:encounter</b>". 4507 */ 4508 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("MedicationRequest:encounter").toLocked(); 4509 4510 /** 4511 * Search parameter: <b>medication</b> 4512 * <p> 4513 * Description: <b>Multiple Resources: 4514 4515* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 4516* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 4517* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 4518* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 4519</b><br> 4520 * Type: <b>reference</b><br> 4521 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 4522 * </p> 4523 */ 4524 @SearchParamDefinition(name="medication", path="MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication reference\r\n", type="reference", target={Medication.class } ) 4525 public static final String SP_MEDICATION = "medication"; 4526 /** 4527 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 4528 * <p> 4529 * Description: <b>Multiple Resources: 4530 4531* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 4532* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 4533* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 4534* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 4535</b><br> 4536 * Type: <b>reference</b><br> 4537 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 4538 * </p> 4539 */ 4540 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 4541 4542/** 4543 * Constant for fluent queries to be used to add include statements. Specifies 4544 * the path value of "<b>MedicationRequest:medication</b>". 4545 */ 4546 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationRequest:medication").toLocked(); 4547 4548 /** 4549 * Search parameter: <b>status</b> 4550 * <p> 4551 * Description: <b>Multiple Resources: 4552 4553* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 4554* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 4555* [MedicationRequest](medicationrequest.html): Status of the prescription 4556* [MedicationStatement](medicationstatement.html): Return statements that match the given status 4557</b><br> 4558 * Type: <b>token</b><br> 4559 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 4560 * </p> 4561 */ 4562 @SearchParamDefinition(name="status", path="MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified)\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status\r\n* [MedicationRequest](medicationrequest.html): Status of the prescription\r\n* [MedicationStatement](medicationstatement.html): Return statements that match the given status\r\n", type="token" ) 4563 public static final String SP_STATUS = "status"; 4564 /** 4565 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4566 * <p> 4567 * Description: <b>Multiple Resources: 4568 4569* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 4570* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 4571* [MedicationRequest](medicationrequest.html): Status of the prescription 4572* [MedicationStatement](medicationstatement.html): Return statements that match the given status 4573</b><br> 4574 * Type: <b>token</b><br> 4575 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 4576 * </p> 4577 */ 4578 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4579 4580 4581} 4582