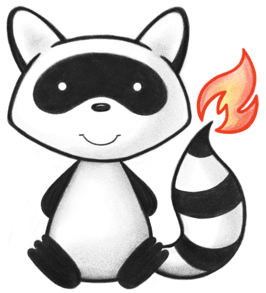
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 052 */ 053@ResourceDef(name="MedicationRequest", profile="http://hl7.org/fhir/StructureDefinition/MedicationRequest") 054public class MedicationRequest extends DomainResource { 055 056 public enum MedicationRequestIntent { 057 /** 058 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 059 */ 060 PROPOSAL, 061 /** 062 * The request represents an intention to ensure something occurs without providing an authorization for others to act. 063 */ 064 PLAN, 065 /** 066 * The request represents a request/demand and authorization for action 067 */ 068 ORDER, 069 /** 070 * The request represents the original authorization for the medication request. 071 */ 072 ORIGINALORDER, 073 /** 074 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization.. 075 */ 076 REFLEXORDER, 077 /** 078 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order. 079 */ 080 FILLERORDER, 081 /** 082 * The request represents an instance for the particular order and is used to generate a schedule of requests on a medication administration record (MAR). 083 */ 084 INSTANCEORDER, 085 /** 086 * The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. 087 */ 088 OPTION, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 public static MedicationRequestIntent fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("proposal".equals(codeString)) 097 return PROPOSAL; 098 if ("plan".equals(codeString)) 099 return PLAN; 100 if ("order".equals(codeString)) 101 return ORDER; 102 if ("original-order".equals(codeString)) 103 return ORIGINALORDER; 104 if ("reflex-order".equals(codeString)) 105 return REFLEXORDER; 106 if ("filler-order".equals(codeString)) 107 return FILLERORDER; 108 if ("instance-order".equals(codeString)) 109 return INSTANCEORDER; 110 if ("option".equals(codeString)) 111 return OPTION; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown MedicationRequestIntent code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case PROPOSAL: return "proposal"; 120 case PLAN: return "plan"; 121 case ORDER: return "order"; 122 case ORIGINALORDER: return "original-order"; 123 case REFLEXORDER: return "reflex-order"; 124 case FILLERORDER: return "filler-order"; 125 case INSTANCEORDER: return "instance-order"; 126 case OPTION: return "option"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getSystem() { 132 switch (this) { 133 case PROPOSAL: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 134 case PLAN: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 135 case ORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 136 case ORIGINALORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 137 case REFLEXORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 138 case FILLERORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 139 case INSTANCEORDER: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 140 case OPTION: return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getDefinition() { 146 switch (this) { 147 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 148 case PLAN: return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 149 case ORDER: return "The request represents a request/demand and authorization for action"; 150 case ORIGINALORDER: return "The request represents the original authorization for the medication request."; 151 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization.."; 152 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 153 case INSTANCEORDER: return "The request represents an instance for the particular order and is used to generate a schedule of requests on a medication administration record (MAR)."; 154 case OPTION: return "The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests."; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 public String getDisplay() { 160 switch (this) { 161 case PROPOSAL: return "Proposal"; 162 case PLAN: return "Plan"; 163 case ORDER: return "Order"; 164 case ORIGINALORDER: return "Original Order"; 165 case REFLEXORDER: return "Reflex Order"; 166 case FILLERORDER: return "Filler Order"; 167 case INSTANCEORDER: return "Instance Order"; 168 case OPTION: return "Option"; 169 case NULL: return null; 170 default: return "?"; 171 } 172 } 173 } 174 175 public static class MedicationRequestIntentEnumFactory implements EnumFactory<MedicationRequestIntent> { 176 public MedicationRequestIntent fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("proposal".equals(codeString)) 181 return MedicationRequestIntent.PROPOSAL; 182 if ("plan".equals(codeString)) 183 return MedicationRequestIntent.PLAN; 184 if ("order".equals(codeString)) 185 return MedicationRequestIntent.ORDER; 186 if ("original-order".equals(codeString)) 187 return MedicationRequestIntent.ORIGINALORDER; 188 if ("reflex-order".equals(codeString)) 189 return MedicationRequestIntent.REFLEXORDER; 190 if ("filler-order".equals(codeString)) 191 return MedicationRequestIntent.FILLERORDER; 192 if ("instance-order".equals(codeString)) 193 return MedicationRequestIntent.INSTANCEORDER; 194 if ("option".equals(codeString)) 195 return MedicationRequestIntent.OPTION; 196 throw new IllegalArgumentException("Unknown MedicationRequestIntent code '"+codeString+"'"); 197 } 198 public Enumeration<MedicationRequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.NULL, code); 203 String codeString = ((PrimitiveType) code).asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.NULL, code); 206 if ("proposal".equals(codeString)) 207 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PROPOSAL, code); 208 if ("plan".equals(codeString)) 209 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PLAN, code); 210 if ("order".equals(codeString)) 211 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.ORDER, code); 212 if ("original-order".equals(codeString)) 213 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.ORIGINALORDER, code); 214 if ("reflex-order".equals(codeString)) 215 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.REFLEXORDER, code); 216 if ("filler-order".equals(codeString)) 217 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.FILLERORDER, code); 218 if ("instance-order".equals(codeString)) 219 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.INSTANCEORDER, code); 220 if ("option".equals(codeString)) 221 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.OPTION, code); 222 throw new FHIRException("Unknown MedicationRequestIntent code '"+codeString+"'"); 223 } 224 public String toCode(MedicationRequestIntent code) { 225 if (code == MedicationRequestIntent.NULL) 226 return null; 227 if (code == MedicationRequestIntent.PROPOSAL) 228 return "proposal"; 229 if (code == MedicationRequestIntent.PLAN) 230 return "plan"; 231 if (code == MedicationRequestIntent.ORDER) 232 return "order"; 233 if (code == MedicationRequestIntent.ORIGINALORDER) 234 return "original-order"; 235 if (code == MedicationRequestIntent.REFLEXORDER) 236 return "reflex-order"; 237 if (code == MedicationRequestIntent.FILLERORDER) 238 return "filler-order"; 239 if (code == MedicationRequestIntent.INSTANCEORDER) 240 return "instance-order"; 241 if (code == MedicationRequestIntent.OPTION) 242 return "option"; 243 return "?"; 244 } 245 public String toSystem(MedicationRequestIntent code) { 246 return code.getSystem(); 247 } 248 } 249 250 public enum MedicationrequestStatus { 251 /** 252 * The request is 'actionable', but not all actions that are implied by it have occurred yet. 253 */ 254 ACTIVE, 255 /** 256 * Actions implied by the request are to be temporarily halted. The request might or might not be resumed. May also be called 'suspended'. 257 */ 258 ONHOLD, 259 /** 260 * The request is no longer active and the subject should no longer be taking the medication. 261 */ 262 ENDED, 263 /** 264 * Actions implied by the request are to be permanently halted, before all of the administrations occurred. This should not be used if the original order was entered in error 265 */ 266 STOPPED, 267 /** 268 * All actions that are implied by the request have occurred. 269 */ 270 COMPLETED, 271 /** 272 * The request has been withdrawn before any administrations have occurred 273 */ 274 CANCELLED, 275 /** 276 * The request was recorded against the wrong patient or for some reason should not have been recorded (e.g. wrong medication, wrong dose, etc.). Some of the actions that are implied by the medication request may have occurred. For example, the medication may have been dispensed and the patient may have taken some of the medication. 277 */ 278 ENTEREDINERROR, 279 /** 280 * The request is not yet 'actionable', e.g. it is a work in progress, requires sign-off, verification or needs to be run through decision support process. 281 */ 282 DRAFT, 283 /** 284 * The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 285 */ 286 UNKNOWN, 287 /** 288 * added to help the parsers with the generic types 289 */ 290 NULL; 291 public static MedicationrequestStatus fromCode(String codeString) throws FHIRException { 292 if (codeString == null || "".equals(codeString)) 293 return null; 294 if ("active".equals(codeString)) 295 return ACTIVE; 296 if ("on-hold".equals(codeString)) 297 return ONHOLD; 298 if ("ended".equals(codeString)) 299 return ENDED; 300 if ("stopped".equals(codeString)) 301 return STOPPED; 302 if ("completed".equals(codeString)) 303 return COMPLETED; 304 if ("cancelled".equals(codeString)) 305 return CANCELLED; 306 if ("entered-in-error".equals(codeString)) 307 return ENTEREDINERROR; 308 if ("draft".equals(codeString)) 309 return DRAFT; 310 if ("unknown".equals(codeString)) 311 return UNKNOWN; 312 if (Configuration.isAcceptInvalidEnums()) 313 return null; 314 else 315 throw new FHIRException("Unknown MedicationrequestStatus code '"+codeString+"'"); 316 } 317 public String toCode() { 318 switch (this) { 319 case ACTIVE: return "active"; 320 case ONHOLD: return "on-hold"; 321 case ENDED: return "ended"; 322 case STOPPED: return "stopped"; 323 case COMPLETED: return "completed"; 324 case CANCELLED: return "cancelled"; 325 case ENTEREDINERROR: return "entered-in-error"; 326 case DRAFT: return "draft"; 327 case UNKNOWN: return "unknown"; 328 case NULL: return null; 329 default: return "?"; 330 } 331 } 332 public String getSystem() { 333 switch (this) { 334 case ACTIVE: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 335 case ONHOLD: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 336 case ENDED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 337 case STOPPED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 338 case COMPLETED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 339 case CANCELLED: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 340 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 341 case DRAFT: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 342 case UNKNOWN: return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 343 case NULL: return null; 344 default: return "?"; 345 } 346 } 347 public String getDefinition() { 348 switch (this) { 349 case ACTIVE: return "The request is 'actionable', but not all actions that are implied by it have occurred yet."; 350 case ONHOLD: return "Actions implied by the request are to be temporarily halted. The request might or might not be resumed. May also be called 'suspended'."; 351 case ENDED: return "The request is no longer active and the subject should no longer be taking the medication."; 352 case STOPPED: return "Actions implied by the request are to be permanently halted, before all of the administrations occurred. This should not be used if the original order was entered in error"; 353 case COMPLETED: return "All actions that are implied by the request have occurred."; 354 case CANCELLED: return "The request has been withdrawn before any administrations have occurred"; 355 case ENTEREDINERROR: return "The request was recorded against the wrong patient or for some reason should not have been recorded (e.g. wrong medication, wrong dose, etc.). Some of the actions that are implied by the medication request may have occurred. For example, the medication may have been dispensed and the patient may have taken some of the medication."; 356 case DRAFT: return "The request is not yet 'actionable', e.g. it is a work in progress, requires sign-off, verification or needs to be run through decision support process."; 357 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 358 case NULL: return null; 359 default: return "?"; 360 } 361 } 362 public String getDisplay() { 363 switch (this) { 364 case ACTIVE: return "Active"; 365 case ONHOLD: return "On Hold"; 366 case ENDED: return "Ended"; 367 case STOPPED: return "Stopped"; 368 case COMPLETED: return "Completed"; 369 case CANCELLED: return "Cancelled"; 370 case ENTEREDINERROR: return "Entered in Error"; 371 case DRAFT: return "Draft"; 372 case UNKNOWN: return "Unknown"; 373 case NULL: return null; 374 default: return "?"; 375 } 376 } 377 } 378 379 public static class MedicationrequestStatusEnumFactory implements EnumFactory<MedicationrequestStatus> { 380 public MedicationrequestStatus fromCode(String codeString) throws IllegalArgumentException { 381 if (codeString == null || "".equals(codeString)) 382 if (codeString == null || "".equals(codeString)) 383 return null; 384 if ("active".equals(codeString)) 385 return MedicationrequestStatus.ACTIVE; 386 if ("on-hold".equals(codeString)) 387 return MedicationrequestStatus.ONHOLD; 388 if ("ended".equals(codeString)) 389 return MedicationrequestStatus.ENDED; 390 if ("stopped".equals(codeString)) 391 return MedicationrequestStatus.STOPPED; 392 if ("completed".equals(codeString)) 393 return MedicationrequestStatus.COMPLETED; 394 if ("cancelled".equals(codeString)) 395 return MedicationrequestStatus.CANCELLED; 396 if ("entered-in-error".equals(codeString)) 397 return MedicationrequestStatus.ENTEREDINERROR; 398 if ("draft".equals(codeString)) 399 return MedicationrequestStatus.DRAFT; 400 if ("unknown".equals(codeString)) 401 return MedicationrequestStatus.UNKNOWN; 402 throw new IllegalArgumentException("Unknown MedicationrequestStatus code '"+codeString+"'"); 403 } 404 public Enumeration<MedicationrequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 405 if (code == null) 406 return null; 407 if (code.isEmpty()) 408 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.NULL, code); 409 String codeString = ((PrimitiveType) code).asStringValue(); 410 if (codeString == null || "".equals(codeString)) 411 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.NULL, code); 412 if ("active".equals(codeString)) 413 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ACTIVE, code); 414 if ("on-hold".equals(codeString)) 415 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ONHOLD, code); 416 if ("ended".equals(codeString)) 417 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ENDED, code); 418 if ("stopped".equals(codeString)) 419 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.STOPPED, code); 420 if ("completed".equals(codeString)) 421 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.COMPLETED, code); 422 if ("cancelled".equals(codeString)) 423 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.CANCELLED, code); 424 if ("entered-in-error".equals(codeString)) 425 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.ENTEREDINERROR, code); 426 if ("draft".equals(codeString)) 427 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.DRAFT, code); 428 if ("unknown".equals(codeString)) 429 return new Enumeration<MedicationrequestStatus>(this, MedicationrequestStatus.UNKNOWN, code); 430 throw new FHIRException("Unknown MedicationrequestStatus code '"+codeString+"'"); 431 } 432 public String toCode(MedicationrequestStatus code) { 433 if (code == MedicationrequestStatus.NULL) 434 return null; 435 if (code == MedicationrequestStatus.ACTIVE) 436 return "active"; 437 if (code == MedicationrequestStatus.ONHOLD) 438 return "on-hold"; 439 if (code == MedicationrequestStatus.ENDED) 440 return "ended"; 441 if (code == MedicationrequestStatus.STOPPED) 442 return "stopped"; 443 if (code == MedicationrequestStatus.COMPLETED) 444 return "completed"; 445 if (code == MedicationrequestStatus.CANCELLED) 446 return "cancelled"; 447 if (code == MedicationrequestStatus.ENTEREDINERROR) 448 return "entered-in-error"; 449 if (code == MedicationrequestStatus.DRAFT) 450 return "draft"; 451 if (code == MedicationrequestStatus.UNKNOWN) 452 return "unknown"; 453 return "?"; 454 } 455 public String toSystem(MedicationrequestStatus code) { 456 return code.getSystem(); 457 } 458 } 459 460 @Block() 461 public static class MedicationRequestDispenseRequestComponent extends BackboneElement implements IBaseBackboneElement { 462 /** 463 * Indicates the quantity or duration for the first dispense of the medication. 464 */ 465 @Child(name = "initialFill", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 466 @Description(shortDefinition="First fill details", formalDefinition="Indicates the quantity or duration for the first dispense of the medication." ) 467 protected MedicationRequestDispenseRequestInitialFillComponent initialFill; 468 469 /** 470 * The minimum period of time that must occur between dispenses of the medication. 471 */ 472 @Child(name = "dispenseInterval", type = {Duration.class}, order=2, min=0, max=1, modifier=false, summary=false) 473 @Description(shortDefinition="Minimum period of time between dispenses", formalDefinition="The minimum period of time that must occur between dispenses of the medication." ) 474 protected Duration dispenseInterval; 475 476 /** 477 * This indicates the validity period of a prescription (stale dating the Prescription). 478 */ 479 @Child(name = "validityPeriod", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 480 @Description(shortDefinition="Time period supply is authorized for", formalDefinition="This indicates the validity period of a prescription (stale dating the Prescription)." ) 481 protected Period validityPeriod; 482 483 /** 484 * An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense. 485 */ 486 @Child(name = "numberOfRepeatsAllowed", type = {UnsignedIntType.class}, order=4, min=0, max=1, modifier=false, summary=false) 487 @Description(shortDefinition="Number of refills authorized", formalDefinition="An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense." ) 488 protected UnsignedIntType numberOfRepeatsAllowed; 489 490 /** 491 * The amount that is to be dispensed for one fill. 492 */ 493 @Child(name = "quantity", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 494 @Description(shortDefinition="Amount of medication to supply per dispense", formalDefinition="The amount that is to be dispensed for one fill." ) 495 protected Quantity quantity; 496 497 /** 498 * Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last. 499 */ 500 @Child(name = "expectedSupplyDuration", type = {Duration.class}, order=6, min=0, max=1, modifier=false, summary=false) 501 @Description(shortDefinition="Number of days supply per dispense", formalDefinition="Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last." ) 502 protected Duration expectedSupplyDuration; 503 504 /** 505 * Indicates the intended performing Organization that will dispense the medication as specified by the prescriber. 506 */ 507 @Child(name = "dispenser", type = {Organization.class}, order=7, min=0, max=1, modifier=false, summary=false) 508 @Description(shortDefinition="Intended performer of dispense", formalDefinition="Indicates the intended performing Organization that will dispense the medication as specified by the prescriber." ) 509 protected Reference dispenser; 510 511 /** 512 * Provides additional information to the dispenser, for example, counselling to be provided to the patient. 513 */ 514 @Child(name = "dispenserInstruction", type = {Annotation.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 515 @Description(shortDefinition="Additional information for the dispenser", formalDefinition="Provides additional information to the dispenser, for example, counselling to be provided to the patient." ) 516 protected List<Annotation> dispenserInstruction; 517 518 /** 519 * Provides information about the type of adherence packaging to be supplied for the medication dispense. 520 */ 521 @Child(name = "doseAdministrationAid", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 522 @Description(shortDefinition="Type of adherence packaging to use for the dispense", formalDefinition="Provides information about the type of adherence packaging to be supplied for the medication dispense." ) 523 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-dose-aid") 524 protected CodeableConcept doseAdministrationAid; 525 526 private static final long serialVersionUID = -916083616L; 527 528 /** 529 * Constructor 530 */ 531 public MedicationRequestDispenseRequestComponent() { 532 super(); 533 } 534 535 /** 536 * @return {@link #initialFill} (Indicates the quantity or duration for the first dispense of the medication.) 537 */ 538 public MedicationRequestDispenseRequestInitialFillComponent getInitialFill() { 539 if (this.initialFill == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.initialFill"); 542 else if (Configuration.doAutoCreate()) 543 this.initialFill = new MedicationRequestDispenseRequestInitialFillComponent(); // cc 544 return this.initialFill; 545 } 546 547 public boolean hasInitialFill() { 548 return this.initialFill != null && !this.initialFill.isEmpty(); 549 } 550 551 /** 552 * @param value {@link #initialFill} (Indicates the quantity or duration for the first dispense of the medication.) 553 */ 554 public MedicationRequestDispenseRequestComponent setInitialFill(MedicationRequestDispenseRequestInitialFillComponent value) { 555 this.initialFill = value; 556 return this; 557 } 558 559 /** 560 * @return {@link #dispenseInterval} (The minimum period of time that must occur between dispenses of the medication.) 561 */ 562 public Duration getDispenseInterval() { 563 if (this.dispenseInterval == null) 564 if (Configuration.errorOnAutoCreate()) 565 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.dispenseInterval"); 566 else if (Configuration.doAutoCreate()) 567 this.dispenseInterval = new Duration(); // cc 568 return this.dispenseInterval; 569 } 570 571 public boolean hasDispenseInterval() { 572 return this.dispenseInterval != null && !this.dispenseInterval.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #dispenseInterval} (The minimum period of time that must occur between dispenses of the medication.) 577 */ 578 public MedicationRequestDispenseRequestComponent setDispenseInterval(Duration value) { 579 this.dispenseInterval = value; 580 return this; 581 } 582 583 /** 584 * @return {@link #validityPeriod} (This indicates the validity period of a prescription (stale dating the Prescription).) 585 */ 586 public Period getValidityPeriod() { 587 if (this.validityPeriod == null) 588 if (Configuration.errorOnAutoCreate()) 589 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.validityPeriod"); 590 else if (Configuration.doAutoCreate()) 591 this.validityPeriod = new Period(); // cc 592 return this.validityPeriod; 593 } 594 595 public boolean hasValidityPeriod() { 596 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 597 } 598 599 /** 600 * @param value {@link #validityPeriod} (This indicates the validity period of a prescription (stale dating the Prescription).) 601 */ 602 public MedicationRequestDispenseRequestComponent setValidityPeriod(Period value) { 603 this.validityPeriod = value; 604 return this; 605 } 606 607 /** 608 * @return {@link #numberOfRepeatsAllowed} (An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.). This is the underlying object with id, value and extensions. The accessor "getNumberOfRepeatsAllowed" gives direct access to the value 609 */ 610 public UnsignedIntType getNumberOfRepeatsAllowedElement() { 611 if (this.numberOfRepeatsAllowed == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.numberOfRepeatsAllowed"); 614 else if (Configuration.doAutoCreate()) 615 this.numberOfRepeatsAllowed = new UnsignedIntType(); // bb 616 return this.numberOfRepeatsAllowed; 617 } 618 619 public boolean hasNumberOfRepeatsAllowedElement() { 620 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 621 } 622 623 public boolean hasNumberOfRepeatsAllowed() { 624 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 625 } 626 627 /** 628 * @param value {@link #numberOfRepeatsAllowed} (An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.). This is the underlying object with id, value and extensions. The accessor "getNumberOfRepeatsAllowed" gives direct access to the value 629 */ 630 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowedElement(UnsignedIntType value) { 631 this.numberOfRepeatsAllowed = value; 632 return this; 633 } 634 635 /** 636 * @return An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense. 637 */ 638 public int getNumberOfRepeatsAllowed() { 639 return this.numberOfRepeatsAllowed == null || this.numberOfRepeatsAllowed.isEmpty() ? 0 : this.numberOfRepeatsAllowed.getValue(); 640 } 641 642 /** 643 * @param value An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense. 644 */ 645 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowed(int value) { 646 if (this.numberOfRepeatsAllowed == null) 647 this.numberOfRepeatsAllowed = new UnsignedIntType(); 648 this.numberOfRepeatsAllowed.setValue(value); 649 return this; 650 } 651 652 /** 653 * @return {@link #quantity} (The amount that is to be dispensed for one fill.) 654 */ 655 public Quantity getQuantity() { 656 if (this.quantity == null) 657 if (Configuration.errorOnAutoCreate()) 658 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.quantity"); 659 else if (Configuration.doAutoCreate()) 660 this.quantity = new Quantity(); // cc 661 return this.quantity; 662 } 663 664 public boolean hasQuantity() { 665 return this.quantity != null && !this.quantity.isEmpty(); 666 } 667 668 /** 669 * @param value {@link #quantity} (The amount that is to be dispensed for one fill.) 670 */ 671 public MedicationRequestDispenseRequestComponent setQuantity(Quantity value) { 672 this.quantity = value; 673 return this; 674 } 675 676 /** 677 * @return {@link #expectedSupplyDuration} (Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.) 678 */ 679 public Duration getExpectedSupplyDuration() { 680 if (this.expectedSupplyDuration == null) 681 if (Configuration.errorOnAutoCreate()) 682 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.expectedSupplyDuration"); 683 else if (Configuration.doAutoCreate()) 684 this.expectedSupplyDuration = new Duration(); // cc 685 return this.expectedSupplyDuration; 686 } 687 688 public boolean hasExpectedSupplyDuration() { 689 return this.expectedSupplyDuration != null && !this.expectedSupplyDuration.isEmpty(); 690 } 691 692 /** 693 * @param value {@link #expectedSupplyDuration} (Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.) 694 */ 695 public MedicationRequestDispenseRequestComponent setExpectedSupplyDuration(Duration value) { 696 this.expectedSupplyDuration = value; 697 return this; 698 } 699 700 /** 701 * @return {@link #dispenser} (Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.) 702 */ 703 public Reference getDispenser() { 704 if (this.dispenser == null) 705 if (Configuration.errorOnAutoCreate()) 706 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.dispenser"); 707 else if (Configuration.doAutoCreate()) 708 this.dispenser = new Reference(); // cc 709 return this.dispenser; 710 } 711 712 public boolean hasDispenser() { 713 return this.dispenser != null && !this.dispenser.isEmpty(); 714 } 715 716 /** 717 * @param value {@link #dispenser} (Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.) 718 */ 719 public MedicationRequestDispenseRequestComponent setDispenser(Reference value) { 720 this.dispenser = value; 721 return this; 722 } 723 724 /** 725 * @return {@link #dispenserInstruction} (Provides additional information to the dispenser, for example, counselling to be provided to the patient.) 726 */ 727 public List<Annotation> getDispenserInstruction() { 728 if (this.dispenserInstruction == null) 729 this.dispenserInstruction = new ArrayList<Annotation>(); 730 return this.dispenserInstruction; 731 } 732 733 /** 734 * @return Returns a reference to <code>this</code> for easy method chaining 735 */ 736 public MedicationRequestDispenseRequestComponent setDispenserInstruction(List<Annotation> theDispenserInstruction) { 737 this.dispenserInstruction = theDispenserInstruction; 738 return this; 739 } 740 741 public boolean hasDispenserInstruction() { 742 if (this.dispenserInstruction == null) 743 return false; 744 for (Annotation item : this.dispenserInstruction) 745 if (!item.isEmpty()) 746 return true; 747 return false; 748 } 749 750 public Annotation addDispenserInstruction() { //3 751 Annotation t = new Annotation(); 752 if (this.dispenserInstruction == null) 753 this.dispenserInstruction = new ArrayList<Annotation>(); 754 this.dispenserInstruction.add(t); 755 return t; 756 } 757 758 public MedicationRequestDispenseRequestComponent addDispenserInstruction(Annotation t) { //3 759 if (t == null) 760 return this; 761 if (this.dispenserInstruction == null) 762 this.dispenserInstruction = new ArrayList<Annotation>(); 763 this.dispenserInstruction.add(t); 764 return this; 765 } 766 767 /** 768 * @return The first repetition of repeating field {@link #dispenserInstruction}, creating it if it does not already exist {3} 769 */ 770 public Annotation getDispenserInstructionFirstRep() { 771 if (getDispenserInstruction().isEmpty()) { 772 addDispenserInstruction(); 773 } 774 return getDispenserInstruction().get(0); 775 } 776 777 /** 778 * @return {@link #doseAdministrationAid} (Provides information about the type of adherence packaging to be supplied for the medication dispense.) 779 */ 780 public CodeableConcept getDoseAdministrationAid() { 781 if (this.doseAdministrationAid == null) 782 if (Configuration.errorOnAutoCreate()) 783 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.doseAdministrationAid"); 784 else if (Configuration.doAutoCreate()) 785 this.doseAdministrationAid = new CodeableConcept(); // cc 786 return this.doseAdministrationAid; 787 } 788 789 public boolean hasDoseAdministrationAid() { 790 return this.doseAdministrationAid != null && !this.doseAdministrationAid.isEmpty(); 791 } 792 793 /** 794 * @param value {@link #doseAdministrationAid} (Provides information about the type of adherence packaging to be supplied for the medication dispense.) 795 */ 796 public MedicationRequestDispenseRequestComponent setDoseAdministrationAid(CodeableConcept value) { 797 this.doseAdministrationAid = value; 798 return this; 799 } 800 801 protected void listChildren(List<Property> children) { 802 super.listChildren(children); 803 children.add(new Property("initialFill", "", "Indicates the quantity or duration for the first dispense of the medication.", 0, 1, initialFill)); 804 children.add(new Property("dispenseInterval", "Duration", "The minimum period of time that must occur between dispenses of the medication.", 0, 1, dispenseInterval)); 805 children.add(new Property("validityPeriod", "Period", "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, validityPeriod)); 806 children.add(new Property("numberOfRepeatsAllowed", "unsignedInt", "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.", 0, 1, numberOfRepeatsAllowed)); 807 children.add(new Property("quantity", "Quantity", "The amount that is to be dispensed for one fill.", 0, 1, quantity)); 808 children.add(new Property("expectedSupplyDuration", "Duration", "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 0, 1, expectedSupplyDuration)); 809 children.add(new Property("dispenser", "Reference(Organization)", "Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.", 0, 1, dispenser)); 810 children.add(new Property("dispenserInstruction", "Annotation", "Provides additional information to the dispenser, for example, counselling to be provided to the patient.", 0, java.lang.Integer.MAX_VALUE, dispenserInstruction)); 811 children.add(new Property("doseAdministrationAid", "CodeableConcept", "Provides information about the type of adherence packaging to be supplied for the medication dispense.", 0, 1, doseAdministrationAid)); 812 } 813 814 @Override 815 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 816 switch (_hash) { 817 case 1232961255: /*initialFill*/ return new Property("initialFill", "", "Indicates the quantity or duration for the first dispense of the medication.", 0, 1, initialFill); 818 case 757112130: /*dispenseInterval*/ return new Property("dispenseInterval", "Duration", "The minimum period of time that must occur between dispenses of the medication.", 0, 1, dispenseInterval); 819 case -1434195053: /*validityPeriod*/ return new Property("validityPeriod", "Period", "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, validityPeriod); 820 case -239736976: /*numberOfRepeatsAllowed*/ return new Property("numberOfRepeatsAllowed", "unsignedInt", "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.", 0, 1, numberOfRepeatsAllowed); 821 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount that is to be dispensed for one fill.", 0, 1, quantity); 822 case -1910182789: /*expectedSupplyDuration*/ return new Property("expectedSupplyDuration", "Duration", "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 0, 1, expectedSupplyDuration); 823 case 241511093: /*dispenser*/ return new Property("dispenser", "Reference(Organization)", "Indicates the intended performing Organization that will dispense the medication as specified by the prescriber.", 0, 1, dispenser); 824 case 2073630361: /*dispenserInstruction*/ return new Property("dispenserInstruction", "Annotation", "Provides additional information to the dispenser, for example, counselling to be provided to the patient.", 0, java.lang.Integer.MAX_VALUE, dispenserInstruction); 825 case 390821217: /*doseAdministrationAid*/ return new Property("doseAdministrationAid", "CodeableConcept", "Provides information about the type of adherence packaging to be supplied for the medication dispense.", 0, 1, doseAdministrationAid); 826 default: return super.getNamedProperty(_hash, _name, _checkValid); 827 } 828 829 } 830 831 @Override 832 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 833 switch (hash) { 834 case 1232961255: /*initialFill*/ return this.initialFill == null ? new Base[0] : new Base[] {this.initialFill}; // MedicationRequestDispenseRequestInitialFillComponent 835 case 757112130: /*dispenseInterval*/ return this.dispenseInterval == null ? new Base[0] : new Base[] {this.dispenseInterval}; // Duration 836 case -1434195053: /*validityPeriod*/ return this.validityPeriod == null ? new Base[0] : new Base[] {this.validityPeriod}; // Period 837 case -239736976: /*numberOfRepeatsAllowed*/ return this.numberOfRepeatsAllowed == null ? new Base[0] : new Base[] {this.numberOfRepeatsAllowed}; // UnsignedIntType 838 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 839 case -1910182789: /*expectedSupplyDuration*/ return this.expectedSupplyDuration == null ? new Base[0] : new Base[] {this.expectedSupplyDuration}; // Duration 840 case 241511093: /*dispenser*/ return this.dispenser == null ? new Base[0] : new Base[] {this.dispenser}; // Reference 841 case 2073630361: /*dispenserInstruction*/ return this.dispenserInstruction == null ? new Base[0] : this.dispenserInstruction.toArray(new Base[this.dispenserInstruction.size()]); // Annotation 842 case 390821217: /*doseAdministrationAid*/ return this.doseAdministrationAid == null ? new Base[0] : new Base[] {this.doseAdministrationAid}; // CodeableConcept 843 default: return super.getProperty(hash, name, checkValid); 844 } 845 846 } 847 848 @Override 849 public Base setProperty(int hash, String name, Base value) throws FHIRException { 850 switch (hash) { 851 case 1232961255: // initialFill 852 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 853 return value; 854 case 757112130: // dispenseInterval 855 this.dispenseInterval = TypeConvertor.castToDuration(value); // Duration 856 return value; 857 case -1434195053: // validityPeriod 858 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 859 return value; 860 case -239736976: // numberOfRepeatsAllowed 861 this.numberOfRepeatsAllowed = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 862 return value; 863 case -1285004149: // quantity 864 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 865 return value; 866 case -1910182789: // expectedSupplyDuration 867 this.expectedSupplyDuration = TypeConvertor.castToDuration(value); // Duration 868 return value; 869 case 241511093: // dispenser 870 this.dispenser = TypeConvertor.castToReference(value); // Reference 871 return value; 872 case 2073630361: // dispenserInstruction 873 this.getDispenserInstruction().add(TypeConvertor.castToAnnotation(value)); // Annotation 874 return value; 875 case 390821217: // doseAdministrationAid 876 this.doseAdministrationAid = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 877 return value; 878 default: return super.setProperty(hash, name, value); 879 } 880 881 } 882 883 @Override 884 public Base setProperty(String name, Base value) throws FHIRException { 885 if (name.equals("initialFill")) { 886 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 887 } else if (name.equals("dispenseInterval")) { 888 this.dispenseInterval = TypeConvertor.castToDuration(value); // Duration 889 } else if (name.equals("validityPeriod")) { 890 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 891 } else if (name.equals("numberOfRepeatsAllowed")) { 892 this.numberOfRepeatsAllowed = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 893 } else if (name.equals("quantity")) { 894 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 895 } else if (name.equals("expectedSupplyDuration")) { 896 this.expectedSupplyDuration = TypeConvertor.castToDuration(value); // Duration 897 } else if (name.equals("dispenser")) { 898 this.dispenser = TypeConvertor.castToReference(value); // Reference 899 } else if (name.equals("dispenserInstruction")) { 900 this.getDispenserInstruction().add(TypeConvertor.castToAnnotation(value)); 901 } else if (name.equals("doseAdministrationAid")) { 902 this.doseAdministrationAid = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 903 } else 904 return super.setProperty(name, value); 905 return value; 906 } 907 908 @Override 909 public void removeChild(String name, Base value) throws FHIRException { 910 if (name.equals("initialFill")) { 911 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 912 } else if (name.equals("dispenseInterval")) { 913 this.dispenseInterval = null; 914 } else if (name.equals("validityPeriod")) { 915 this.validityPeriod = null; 916 } else if (name.equals("numberOfRepeatsAllowed")) { 917 this.numberOfRepeatsAllowed = null; 918 } else if (name.equals("quantity")) { 919 this.quantity = null; 920 } else if (name.equals("expectedSupplyDuration")) { 921 this.expectedSupplyDuration = null; 922 } else if (name.equals("dispenser")) { 923 this.dispenser = null; 924 } else if (name.equals("dispenserInstruction")) { 925 this.getDispenserInstruction().remove(value); 926 } else if (name.equals("doseAdministrationAid")) { 927 this.doseAdministrationAid = null; 928 } else 929 super.removeChild(name, value); 930 931 } 932 933 @Override 934 public Base makeProperty(int hash, String name) throws FHIRException { 935 switch (hash) { 936 case 1232961255: return getInitialFill(); 937 case 757112130: return getDispenseInterval(); 938 case -1434195053: return getValidityPeriod(); 939 case -239736976: return getNumberOfRepeatsAllowedElement(); 940 case -1285004149: return getQuantity(); 941 case -1910182789: return getExpectedSupplyDuration(); 942 case 241511093: return getDispenser(); 943 case 2073630361: return addDispenserInstruction(); 944 case 390821217: return getDoseAdministrationAid(); 945 default: return super.makeProperty(hash, name); 946 } 947 948 } 949 950 @Override 951 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 952 switch (hash) { 953 case 1232961255: /*initialFill*/ return new String[] {}; 954 case 757112130: /*dispenseInterval*/ return new String[] {"Duration"}; 955 case -1434195053: /*validityPeriod*/ return new String[] {"Period"}; 956 case -239736976: /*numberOfRepeatsAllowed*/ return new String[] {"unsignedInt"}; 957 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 958 case -1910182789: /*expectedSupplyDuration*/ return new String[] {"Duration"}; 959 case 241511093: /*dispenser*/ return new String[] {"Reference"}; 960 case 2073630361: /*dispenserInstruction*/ return new String[] {"Annotation"}; 961 case 390821217: /*doseAdministrationAid*/ return new String[] {"CodeableConcept"}; 962 default: return super.getTypesForProperty(hash, name); 963 } 964 965 } 966 967 @Override 968 public Base addChild(String name) throws FHIRException { 969 if (name.equals("initialFill")) { 970 this.initialFill = new MedicationRequestDispenseRequestInitialFillComponent(); 971 return this.initialFill; 972 } 973 else if (name.equals("dispenseInterval")) { 974 this.dispenseInterval = new Duration(); 975 return this.dispenseInterval; 976 } 977 else if (name.equals("validityPeriod")) { 978 this.validityPeriod = new Period(); 979 return this.validityPeriod; 980 } 981 else if (name.equals("numberOfRepeatsAllowed")) { 982 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.dispenseRequest.numberOfRepeatsAllowed"); 983 } 984 else if (name.equals("quantity")) { 985 this.quantity = new Quantity(); 986 return this.quantity; 987 } 988 else if (name.equals("expectedSupplyDuration")) { 989 this.expectedSupplyDuration = new Duration(); 990 return this.expectedSupplyDuration; 991 } 992 else if (name.equals("dispenser")) { 993 this.dispenser = new Reference(); 994 return this.dispenser; 995 } 996 else if (name.equals("dispenserInstruction")) { 997 return addDispenserInstruction(); 998 } 999 else if (name.equals("doseAdministrationAid")) { 1000 this.doseAdministrationAid = new CodeableConcept(); 1001 return this.doseAdministrationAid; 1002 } 1003 else 1004 return super.addChild(name); 1005 } 1006 1007 public MedicationRequestDispenseRequestComponent copy() { 1008 MedicationRequestDispenseRequestComponent dst = new MedicationRequestDispenseRequestComponent(); 1009 copyValues(dst); 1010 return dst; 1011 } 1012 1013 public void copyValues(MedicationRequestDispenseRequestComponent dst) { 1014 super.copyValues(dst); 1015 dst.initialFill = initialFill == null ? null : initialFill.copy(); 1016 dst.dispenseInterval = dispenseInterval == null ? null : dispenseInterval.copy(); 1017 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1018 dst.numberOfRepeatsAllowed = numberOfRepeatsAllowed == null ? null : numberOfRepeatsAllowed.copy(); 1019 dst.quantity = quantity == null ? null : quantity.copy(); 1020 dst.expectedSupplyDuration = expectedSupplyDuration == null ? null : expectedSupplyDuration.copy(); 1021 dst.dispenser = dispenser == null ? null : dispenser.copy(); 1022 if (dispenserInstruction != null) { 1023 dst.dispenserInstruction = new ArrayList<Annotation>(); 1024 for (Annotation i : dispenserInstruction) 1025 dst.dispenserInstruction.add(i.copy()); 1026 }; 1027 dst.doseAdministrationAid = doseAdministrationAid == null ? null : doseAdministrationAid.copy(); 1028 } 1029 1030 @Override 1031 public boolean equalsDeep(Base other_) { 1032 if (!super.equalsDeep(other_)) 1033 return false; 1034 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1035 return false; 1036 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1037 return compareDeep(initialFill, o.initialFill, true) && compareDeep(dispenseInterval, o.dispenseInterval, true) 1038 && compareDeep(validityPeriod, o.validityPeriod, true) && compareDeep(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true) 1039 && compareDeep(quantity, o.quantity, true) && compareDeep(expectedSupplyDuration, o.expectedSupplyDuration, true) 1040 && compareDeep(dispenser, o.dispenser, true) && compareDeep(dispenserInstruction, o.dispenserInstruction, true) 1041 && compareDeep(doseAdministrationAid, o.doseAdministrationAid, true); 1042 } 1043 1044 @Override 1045 public boolean equalsShallow(Base other_) { 1046 if (!super.equalsShallow(other_)) 1047 return false; 1048 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1049 return false; 1050 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1051 return compareValues(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true); 1052 } 1053 1054 public boolean isEmpty() { 1055 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(initialFill, dispenseInterval 1056 , validityPeriod, numberOfRepeatsAllowed, quantity, expectedSupplyDuration, dispenser 1057 , dispenserInstruction, doseAdministrationAid); 1058 } 1059 1060 public String fhirType() { 1061 return "MedicationRequest.dispenseRequest"; 1062 1063 } 1064 1065 } 1066 1067 @Block() 1068 public static class MedicationRequestDispenseRequestInitialFillComponent extends BackboneElement implements IBaseBackboneElement { 1069 /** 1070 * The amount or quantity to provide as part of the first dispense. 1071 */ 1072 @Child(name = "quantity", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 1073 @Description(shortDefinition="First fill quantity", formalDefinition="The amount or quantity to provide as part of the first dispense." ) 1074 protected Quantity quantity; 1075 1076 /** 1077 * The length of time that the first dispense is expected to last. 1078 */ 1079 @Child(name = "duration", type = {Duration.class}, order=2, min=0, max=1, modifier=false, summary=false) 1080 @Description(shortDefinition="First fill duration", formalDefinition="The length of time that the first dispense is expected to last." ) 1081 protected Duration duration; 1082 1083 private static final long serialVersionUID = 1223227956L; 1084 1085 /** 1086 * Constructor 1087 */ 1088 public MedicationRequestDispenseRequestInitialFillComponent() { 1089 super(); 1090 } 1091 1092 /** 1093 * @return {@link #quantity} (The amount or quantity to provide as part of the first dispense.) 1094 */ 1095 public Quantity getQuantity() { 1096 if (this.quantity == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestInitialFillComponent.quantity"); 1099 else if (Configuration.doAutoCreate()) 1100 this.quantity = new Quantity(); // cc 1101 return this.quantity; 1102 } 1103 1104 public boolean hasQuantity() { 1105 return this.quantity != null && !this.quantity.isEmpty(); 1106 } 1107 1108 /** 1109 * @param value {@link #quantity} (The amount or quantity to provide as part of the first dispense.) 1110 */ 1111 public MedicationRequestDispenseRequestInitialFillComponent setQuantity(Quantity value) { 1112 this.quantity = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #duration} (The length of time that the first dispense is expected to last.) 1118 */ 1119 public Duration getDuration() { 1120 if (this.duration == null) 1121 if (Configuration.errorOnAutoCreate()) 1122 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestInitialFillComponent.duration"); 1123 else if (Configuration.doAutoCreate()) 1124 this.duration = new Duration(); // cc 1125 return this.duration; 1126 } 1127 1128 public boolean hasDuration() { 1129 return this.duration != null && !this.duration.isEmpty(); 1130 } 1131 1132 /** 1133 * @param value {@link #duration} (The length of time that the first dispense is expected to last.) 1134 */ 1135 public MedicationRequestDispenseRequestInitialFillComponent setDuration(Duration value) { 1136 this.duration = value; 1137 return this; 1138 } 1139 1140 protected void listChildren(List<Property> children) { 1141 super.listChildren(children); 1142 children.add(new Property("quantity", "Quantity", "The amount or quantity to provide as part of the first dispense.", 0, 1, quantity)); 1143 children.add(new Property("duration", "Duration", "The length of time that the first dispense is expected to last.", 0, 1, duration)); 1144 } 1145 1146 @Override 1147 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1148 switch (_hash) { 1149 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount or quantity to provide as part of the first dispense.", 0, 1, quantity); 1150 case -1992012396: /*duration*/ return new Property("duration", "Duration", "The length of time that the first dispense is expected to last.", 0, 1, duration); 1151 default: return super.getNamedProperty(_hash, _name, _checkValid); 1152 } 1153 1154 } 1155 1156 @Override 1157 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1158 switch (hash) { 1159 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1160 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // Duration 1161 default: return super.getProperty(hash, name, checkValid); 1162 } 1163 1164 } 1165 1166 @Override 1167 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1168 switch (hash) { 1169 case -1285004149: // quantity 1170 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1171 return value; 1172 case -1992012396: // duration 1173 this.duration = TypeConvertor.castToDuration(value); // Duration 1174 return value; 1175 default: return super.setProperty(hash, name, value); 1176 } 1177 1178 } 1179 1180 @Override 1181 public Base setProperty(String name, Base value) throws FHIRException { 1182 if (name.equals("quantity")) { 1183 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1184 } else if (name.equals("duration")) { 1185 this.duration = TypeConvertor.castToDuration(value); // Duration 1186 } else 1187 return super.setProperty(name, value); 1188 return value; 1189 } 1190 1191 @Override 1192 public void removeChild(String name, Base value) throws FHIRException { 1193 if (name.equals("quantity")) { 1194 this.quantity = null; 1195 } else if (name.equals("duration")) { 1196 this.duration = null; 1197 } else 1198 super.removeChild(name, value); 1199 1200 } 1201 1202 @Override 1203 public Base makeProperty(int hash, String name) throws FHIRException { 1204 switch (hash) { 1205 case -1285004149: return getQuantity(); 1206 case -1992012396: return getDuration(); 1207 default: return super.makeProperty(hash, name); 1208 } 1209 1210 } 1211 1212 @Override 1213 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1214 switch (hash) { 1215 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1216 case -1992012396: /*duration*/ return new String[] {"Duration"}; 1217 default: return super.getTypesForProperty(hash, name); 1218 } 1219 1220 } 1221 1222 @Override 1223 public Base addChild(String name) throws FHIRException { 1224 if (name.equals("quantity")) { 1225 this.quantity = new Quantity(); 1226 return this.quantity; 1227 } 1228 else if (name.equals("duration")) { 1229 this.duration = new Duration(); 1230 return this.duration; 1231 } 1232 else 1233 return super.addChild(name); 1234 } 1235 1236 public MedicationRequestDispenseRequestInitialFillComponent copy() { 1237 MedicationRequestDispenseRequestInitialFillComponent dst = new MedicationRequestDispenseRequestInitialFillComponent(); 1238 copyValues(dst); 1239 return dst; 1240 } 1241 1242 public void copyValues(MedicationRequestDispenseRequestInitialFillComponent dst) { 1243 super.copyValues(dst); 1244 dst.quantity = quantity == null ? null : quantity.copy(); 1245 dst.duration = duration == null ? null : duration.copy(); 1246 } 1247 1248 @Override 1249 public boolean equalsDeep(Base other_) { 1250 if (!super.equalsDeep(other_)) 1251 return false; 1252 if (!(other_ instanceof MedicationRequestDispenseRequestInitialFillComponent)) 1253 return false; 1254 MedicationRequestDispenseRequestInitialFillComponent o = (MedicationRequestDispenseRequestInitialFillComponent) other_; 1255 return compareDeep(quantity, o.quantity, true) && compareDeep(duration, o.duration, true); 1256 } 1257 1258 @Override 1259 public boolean equalsShallow(Base other_) { 1260 if (!super.equalsShallow(other_)) 1261 return false; 1262 if (!(other_ instanceof MedicationRequestDispenseRequestInitialFillComponent)) 1263 return false; 1264 MedicationRequestDispenseRequestInitialFillComponent o = (MedicationRequestDispenseRequestInitialFillComponent) other_; 1265 return true; 1266 } 1267 1268 public boolean isEmpty() { 1269 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, duration); 1270 } 1271 1272 public String fhirType() { 1273 return "MedicationRequest.dispenseRequest.initialFill"; 1274 1275 } 1276 1277 } 1278 1279 @Block() 1280 public static class MedicationRequestSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 1281 /** 1282 * True if the prescriber allows a different drug to be dispensed from what was prescribed. 1283 */ 1284 @Child(name = "allowed", type = {BooleanType.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1285 @Description(shortDefinition="Whether substitution is allowed or not", formalDefinition="True if the prescriber allows a different drug to be dispensed from what was prescribed." ) 1286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActSubstanceAdminSubstitutionCode") 1287 protected DataType allowed; 1288 1289 /** 1290 * Indicates the reason for the substitution, or why substitution must or must not be performed. 1291 */ 1292 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1293 @Description(shortDefinition="Why should (not) substitution be made", formalDefinition="Indicates the reason for the substitution, or why substitution must or must not be performed." ) 1294 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-SubstanceAdminSubstitutionReason") 1295 protected CodeableConcept reason; 1296 1297 private static final long serialVersionUID = -855005751L; 1298 1299 /** 1300 * Constructor 1301 */ 1302 public MedicationRequestSubstitutionComponent() { 1303 super(); 1304 } 1305 1306 /** 1307 * Constructor 1308 */ 1309 public MedicationRequestSubstitutionComponent(DataType allowed) { 1310 super(); 1311 this.setAllowed(allowed); 1312 } 1313 1314 /** 1315 * @return {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1316 */ 1317 public DataType getAllowed() { 1318 return this.allowed; 1319 } 1320 1321 /** 1322 * @return {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1323 */ 1324 public BooleanType getAllowedBooleanType() throws FHIRException { 1325 if (this.allowed == null) 1326 this.allowed = new BooleanType(); 1327 if (!(this.allowed instanceof BooleanType)) 1328 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 1329 return (BooleanType) this.allowed; 1330 } 1331 1332 public boolean hasAllowedBooleanType() { 1333 return this.allowed instanceof BooleanType; 1334 } 1335 1336 /** 1337 * @return {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1338 */ 1339 public CodeableConcept getAllowedCodeableConcept() throws FHIRException { 1340 if (this.allowed == null) 1341 this.allowed = new CodeableConcept(); 1342 if (!(this.allowed instanceof CodeableConcept)) 1343 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.allowed.getClass().getName()+" was encountered"); 1344 return (CodeableConcept) this.allowed; 1345 } 1346 1347 public boolean hasAllowedCodeableConcept() { 1348 return this.allowed instanceof CodeableConcept; 1349 } 1350 1351 public boolean hasAllowed() { 1352 return this.allowed != null && !this.allowed.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.) 1357 */ 1358 public MedicationRequestSubstitutionComponent setAllowed(DataType value) { 1359 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 1360 throw new FHIRException("Not the right type for MedicationRequest.substitution.allowed[x]: "+value.fhirType()); 1361 this.allowed = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #reason} (Indicates the reason for the substitution, or why substitution must or must not be performed.) 1367 */ 1368 public CodeableConcept getReason() { 1369 if (this.reason == null) 1370 if (Configuration.errorOnAutoCreate()) 1371 throw new Error("Attempt to auto-create MedicationRequestSubstitutionComponent.reason"); 1372 else if (Configuration.doAutoCreate()) 1373 this.reason = new CodeableConcept(); // cc 1374 return this.reason; 1375 } 1376 1377 public boolean hasReason() { 1378 return this.reason != null && !this.reason.isEmpty(); 1379 } 1380 1381 /** 1382 * @param value {@link #reason} (Indicates the reason for the substitution, or why substitution must or must not be performed.) 1383 */ 1384 public MedicationRequestSubstitutionComponent setReason(CodeableConcept value) { 1385 this.reason = value; 1386 return this; 1387 } 1388 1389 protected void listChildren(List<Property> children) { 1390 super.listChildren(children); 1391 children.add(new Property("allowed[x]", "boolean|CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed)); 1392 children.add(new Property("reason", "CodeableConcept", "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, reason)); 1393 } 1394 1395 @Override 1396 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1397 switch (_hash) { 1398 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "boolean|CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1399 case -911343192: /*allowed*/ return new Property("allowed[x]", "boolean|CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1400 case 177755488: /*allowedBoolean*/ return new Property("allowed[x]", "boolean", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1401 case 963125465: /*allowedCodeableConcept*/ return new Property("allowed[x]", "CodeableConcept", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1402 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, reason); 1403 default: return super.getNamedProperty(_hash, _name, _checkValid); 1404 } 1405 1406 } 1407 1408 @Override 1409 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1410 switch (hash) { 1411 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // DataType 1412 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1413 default: return super.getProperty(hash, name, checkValid); 1414 } 1415 1416 } 1417 1418 @Override 1419 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1420 switch (hash) { 1421 case -911343192: // allowed 1422 this.allowed = TypeConvertor.castToType(value); // DataType 1423 return value; 1424 case -934964668: // reason 1425 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1426 return value; 1427 default: return super.setProperty(hash, name, value); 1428 } 1429 1430 } 1431 1432 @Override 1433 public Base setProperty(String name, Base value) throws FHIRException { 1434 if (name.equals("allowed[x]")) { 1435 this.allowed = TypeConvertor.castToType(value); // DataType 1436 } else if (name.equals("reason")) { 1437 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1438 } else 1439 return super.setProperty(name, value); 1440 return value; 1441 } 1442 1443 @Override 1444 public void removeChild(String name, Base value) throws FHIRException { 1445 if (name.equals("allowed[x]")) { 1446 this.allowed = null; 1447 } else if (name.equals("reason")) { 1448 this.reason = null; 1449 } else 1450 super.removeChild(name, value); 1451 1452 } 1453 1454 @Override 1455 public Base makeProperty(int hash, String name) throws FHIRException { 1456 switch (hash) { 1457 case -1336663592: return getAllowed(); 1458 case -911343192: return getAllowed(); 1459 case -934964668: return getReason(); 1460 default: return super.makeProperty(hash, name); 1461 } 1462 1463 } 1464 1465 @Override 1466 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1467 switch (hash) { 1468 case -911343192: /*allowed*/ return new String[] {"boolean", "CodeableConcept"}; 1469 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1470 default: return super.getTypesForProperty(hash, name); 1471 } 1472 1473 } 1474 1475 @Override 1476 public Base addChild(String name) throws FHIRException { 1477 if (name.equals("allowedBoolean")) { 1478 this.allowed = new BooleanType(); 1479 return this.allowed; 1480 } 1481 else if (name.equals("allowedCodeableConcept")) { 1482 this.allowed = new CodeableConcept(); 1483 return this.allowed; 1484 } 1485 else if (name.equals("reason")) { 1486 this.reason = new CodeableConcept(); 1487 return this.reason; 1488 } 1489 else 1490 return super.addChild(name); 1491 } 1492 1493 public MedicationRequestSubstitutionComponent copy() { 1494 MedicationRequestSubstitutionComponent dst = new MedicationRequestSubstitutionComponent(); 1495 copyValues(dst); 1496 return dst; 1497 } 1498 1499 public void copyValues(MedicationRequestSubstitutionComponent dst) { 1500 super.copyValues(dst); 1501 dst.allowed = allowed == null ? null : allowed.copy(); 1502 dst.reason = reason == null ? null : reason.copy(); 1503 } 1504 1505 @Override 1506 public boolean equalsDeep(Base other_) { 1507 if (!super.equalsDeep(other_)) 1508 return false; 1509 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1510 return false; 1511 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1512 return compareDeep(allowed, o.allowed, true) && compareDeep(reason, o.reason, true); 1513 } 1514 1515 @Override 1516 public boolean equalsShallow(Base other_) { 1517 if (!super.equalsShallow(other_)) 1518 return false; 1519 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1520 return false; 1521 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1522 return true; 1523 } 1524 1525 public boolean isEmpty() { 1526 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(allowed, reason); 1527 } 1528 1529 public String fhirType() { 1530 return "MedicationRequest.substitution"; 1531 1532 } 1533 1534 } 1535 1536 /** 1537 * Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server. 1538 */ 1539 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1540 @Description(shortDefinition="External ids for this request", formalDefinition="Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server." ) 1541 protected List<Identifier> identifier; 1542 1543 /** 1544 * A plan or request that is fulfilled in whole or in part by this medication request. 1545 */ 1546 @Child(name = "basedOn", type = {CarePlan.class, MedicationRequest.class, ServiceRequest.class, ImmunizationRecommendation.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1547 @Description(shortDefinition="A plan or request that is fulfilled in whole or in part by this medication request", formalDefinition="A plan or request that is fulfilled in whole or in part by this medication request." ) 1548 protected List<Reference> basedOn; 1549 1550 /** 1551 * Reference to an order/prescription that is being replaced by this MedicationRequest. 1552 */ 1553 @Child(name = "priorPrescription", type = {MedicationRequest.class}, order=2, min=0, max=1, modifier=false, summary=false) 1554 @Description(shortDefinition="Reference to an order/prescription that is being replaced by this MedicationRequest", formalDefinition="Reference to an order/prescription that is being replaced by this MedicationRequest." ) 1555 protected Reference priorPrescription; 1556 1557 /** 1558 * A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time. 1559 */ 1560 @Child(name = "groupIdentifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 1561 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time." ) 1562 protected Identifier groupIdentifier; 1563 1564 /** 1565 * A code specifying the current state of the order. Generally, this will be active or completed state. 1566 */ 1567 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 1568 @Description(shortDefinition="active | on-hold | ended | stopped | completed | cancelled | entered-in-error | draft | unknown", formalDefinition="A code specifying the current state of the order. Generally, this will be active or completed state." ) 1569 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-status") 1570 protected Enumeration<MedicationrequestStatus> status; 1571 1572 /** 1573 * Captures the reason for the current state of the MedicationRequest. 1574 */ 1575 @Child(name = "statusReason", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1576 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current state of the MedicationRequest." ) 1577 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-status-reason") 1578 protected CodeableConcept statusReason; 1579 1580 /** 1581 * The date (and perhaps time) when the status was changed. 1582 */ 1583 @Child(name = "statusChanged", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1584 @Description(shortDefinition="When the status was changed", formalDefinition="The date (and perhaps time) when the status was changed." ) 1585 protected DateTimeType statusChanged; 1586 1587 /** 1588 * Whether the request is a proposal, plan, or an original order. 1589 */ 1590 @Child(name = "intent", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 1591 @Description(shortDefinition="proposal | plan | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Whether the request is a proposal, plan, or an original order." ) 1592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-intent") 1593 protected Enumeration<MedicationRequestIntent> intent; 1594 1595 /** 1596 * An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication. 1597 */ 1598 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1599 @Description(shortDefinition="Grouping or category of medication request", formalDefinition="An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication." ) 1600 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-admin-location") 1601 protected List<CodeableConcept> category; 1602 1603 /** 1604 * Indicates how quickly the Medication Request should be addressed with respect to other requests. 1605 */ 1606 @Child(name = "priority", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1607 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the Medication Request should be addressed with respect to other requests." ) 1608 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 1609 protected Enumeration<RequestPriority> priority; 1610 1611 /** 1612 * If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication. 1613 */ 1614 @Child(name = "doNotPerform", type = {BooleanType.class}, order=10, min=0, max=1, modifier=true, summary=true) 1615 @Description(shortDefinition="True if patient is to stop taking or not to start taking the medication", formalDefinition="If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication." ) 1616 protected BooleanType doNotPerform; 1617 1618 /** 1619 * Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications. 1620 */ 1621 @Child(name = "medication", type = {CodeableReference.class}, order=11, min=1, max=1, modifier=false, summary=true) 1622 @Description(shortDefinition="Medication to be taken", formalDefinition="Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications." ) 1623 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 1624 protected CodeableReference medication; 1625 1626 /** 1627 * The individual or group for whom the medication has been requested. 1628 */ 1629 @Child(name = "subject", type = {Patient.class, Group.class}, order=12, min=1, max=1, modifier=false, summary=true) 1630 @Description(shortDefinition="Individual or group for whom the medication has been requested", formalDefinition="The individual or group for whom the medication has been requested." ) 1631 protected Reference subject; 1632 1633 /** 1634 * The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person. 1635 */ 1636 @Child(name = "informationSource", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Organization.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1637 @Description(shortDefinition="The person or organization who provided the information about this request, if the source is someone other than the requestor", formalDefinition="The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person." ) 1638 protected List<Reference> informationSource; 1639 1640 /** 1641 * The Encounter during which this [x] was created or to which the creation of this record is tightly associated. 1642 */ 1643 @Child(name = "encounter", type = {Encounter.class}, order=14, min=0, max=1, modifier=false, summary=false) 1644 @Description(shortDefinition="Encounter created as part of encounter/admission/stay", formalDefinition="The Encounter during which this [x] was created or to which the creation of this record is tightly associated." ) 1645 protected Reference encounter; 1646 1647 /** 1648 * Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient). 1649 */ 1650 @Child(name = "supportingInformation", type = {Reference.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1651 @Description(shortDefinition="Information to support fulfilling of the medication", formalDefinition="Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient)." ) 1652 protected List<Reference> supportingInformation; 1653 1654 /** 1655 * The date (and perhaps time) when the prescription was initially written or authored on. 1656 */ 1657 @Child(name = "authoredOn", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=true) 1658 @Description(shortDefinition="When request was initially authored", formalDefinition="The date (and perhaps time) when the prescription was initially written or authored on." ) 1659 protected DateTimeType authoredOn; 1660 1661 /** 1662 * The individual, organization, or device that initiated the request and has responsibility for its activation. 1663 */ 1664 @Child(name = "requester", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=17, min=0, max=1, modifier=false, summary=true) 1665 @Description(shortDefinition="Who/What requested the Request", formalDefinition="The individual, organization, or device that initiated the request and has responsibility for its activation." ) 1666 protected Reference requester; 1667 1668 /** 1669 * Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report. 1670 */ 1671 @Child(name = "reported", type = {BooleanType.class}, order=18, min=0, max=1, modifier=false, summary=true) 1672 @Description(shortDefinition="Reported rather than primary record", formalDefinition="Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report." ) 1673 protected BooleanType reported; 1674 1675 /** 1676 * Indicates the type of performer of the administration of the medication. 1677 */ 1678 @Child(name = "performerType", type = {CodeableConcept.class}, order=19, min=0, max=1, modifier=false, summary=true) 1679 @Description(shortDefinition="Desired kind of performer of the medication administration", formalDefinition="Indicates the type of performer of the administration of the medication." ) 1680 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-intended-performer-role") 1681 protected CodeableConcept performerType; 1682 1683 /** 1684 * The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers. 1685 */ 1686 @Child(name = "performer", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, DeviceDefinition.class, RelatedPerson.class, CareTeam.class, HealthcareService.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1687 @Description(shortDefinition="Intended performer of administration", formalDefinition="The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers." ) 1688 protected List<Reference> performer; 1689 1690 /** 1691 * The intended type of device that is to be used for the administration of the medication (for example, PCA Pump). 1692 */ 1693 @Child(name = "device", type = {CodeableReference.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1694 @Description(shortDefinition="Intended type of device for the administration", formalDefinition="The intended type of device that is to be used for the administration of the medication (for example, PCA Pump)." ) 1695 protected List<CodeableReference> device; 1696 1697 /** 1698 * The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order. 1699 */ 1700 @Child(name = "recorder", type = {Practitioner.class, PractitionerRole.class}, order=22, min=0, max=1, modifier=false, summary=false) 1701 @Description(shortDefinition="Person who entered the request", formalDefinition="The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order." ) 1702 protected Reference recorder; 1703 1704 /** 1705 * The reason or the indication for ordering or not ordering the medication. 1706 */ 1707 @Child(name = "reason", type = {CodeableReference.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1708 @Description(shortDefinition="Reason or indication for ordering or not ordering the medication", formalDefinition="The reason or the indication for ordering or not ordering the medication." ) 1709 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 1710 protected List<CodeableReference> reason; 1711 1712 /** 1713 * The description of the overall pattern of the administration of the medication to the patient. 1714 */ 1715 @Child(name = "courseOfTherapyType", type = {CodeableConcept.class}, order=24, min=0, max=1, modifier=false, summary=false) 1716 @Description(shortDefinition="Overall pattern of medication administration", formalDefinition="The description of the overall pattern of the administration of the medication to the patient." ) 1717 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-course-of-therapy") 1718 protected CodeableConcept courseOfTherapyType; 1719 1720 /** 1721 * Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service. 1722 */ 1723 @Child(name = "insurance", type = {Coverage.class, ClaimResponse.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1724 @Description(shortDefinition="Associated insurance coverage", formalDefinition="Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service." ) 1725 protected List<Reference> insurance; 1726 1727 /** 1728 * Extra information about the prescription that could not be conveyed by the other attributes. 1729 */ 1730 @Child(name = "note", type = {Annotation.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1731 @Description(shortDefinition="Information about the prescription", formalDefinition="Extra information about the prescription that could not be conveyed by the other attributes." ) 1732 protected List<Annotation> note; 1733 1734 /** 1735 * The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 1736 */ 1737 @Child(name = "renderedDosageInstruction", type = {MarkdownType.class}, order=27, min=0, max=1, modifier=false, summary=false) 1738 @Description(shortDefinition="Full representation of the dosage instructions", formalDefinition="The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses." ) 1739 protected MarkdownType renderedDosageInstruction; 1740 1741 /** 1742 * The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions. 1743 */ 1744 @Child(name = "effectiveDosePeriod", type = {Period.class}, order=28, min=0, max=1, modifier=false, summary=false) 1745 @Description(shortDefinition="Period over which the medication is to be taken", formalDefinition="The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions." ) 1746 protected Period effectiveDosePeriod; 1747 1748 /** 1749 * Specific instructions for how the medication is to be used by the patient. 1750 */ 1751 @Child(name = "dosageInstruction", type = {Dosage.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1752 @Description(shortDefinition="Specific instructions for how the medication should be taken", formalDefinition="Specific instructions for how the medication is to be used by the patient." ) 1753 protected List<Dosage> dosageInstruction; 1754 1755 /** 1756 * Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department. 1757 */ 1758 @Child(name = "dispenseRequest", type = {}, order=30, min=0, max=1, modifier=false, summary=false) 1759 @Description(shortDefinition="Medication supply authorization", formalDefinition="Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department." ) 1760 protected MedicationRequestDispenseRequestComponent dispenseRequest; 1761 1762 /** 1763 * Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done. 1764 */ 1765 @Child(name = "substitution", type = {}, order=31, min=0, max=1, modifier=false, summary=false) 1766 @Description(shortDefinition="Any restrictions on medication substitution", formalDefinition="Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done." ) 1767 protected MedicationRequestSubstitutionComponent substitution; 1768 1769 /** 1770 * Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource. 1771 */ 1772 @Child(name = "eventHistory", type = {Provenance.class}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1773 @Description(shortDefinition="A list of events of interest in the lifecycle", formalDefinition="Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource." ) 1774 protected List<Reference> eventHistory; 1775 1776 private static final long serialVersionUID = 1013423544L; 1777 1778 /** 1779 * Constructor 1780 */ 1781 public MedicationRequest() { 1782 super(); 1783 } 1784 1785 /** 1786 * Constructor 1787 */ 1788 public MedicationRequest(MedicationrequestStatus status, MedicationRequestIntent intent, CodeableReference medication, Reference subject) { 1789 super(); 1790 this.setStatus(status); 1791 this.setIntent(intent); 1792 this.setMedication(medication); 1793 this.setSubject(subject); 1794 } 1795 1796 /** 1797 * @return {@link #identifier} (Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.) 1798 */ 1799 public List<Identifier> getIdentifier() { 1800 if (this.identifier == null) 1801 this.identifier = new ArrayList<Identifier>(); 1802 return this.identifier; 1803 } 1804 1805 /** 1806 * @return Returns a reference to <code>this</code> for easy method chaining 1807 */ 1808 public MedicationRequest setIdentifier(List<Identifier> theIdentifier) { 1809 this.identifier = theIdentifier; 1810 return this; 1811 } 1812 1813 public boolean hasIdentifier() { 1814 if (this.identifier == null) 1815 return false; 1816 for (Identifier item : this.identifier) 1817 if (!item.isEmpty()) 1818 return true; 1819 return false; 1820 } 1821 1822 public Identifier addIdentifier() { //3 1823 Identifier t = new Identifier(); 1824 if (this.identifier == null) 1825 this.identifier = new ArrayList<Identifier>(); 1826 this.identifier.add(t); 1827 return t; 1828 } 1829 1830 public MedicationRequest addIdentifier(Identifier t) { //3 1831 if (t == null) 1832 return this; 1833 if (this.identifier == null) 1834 this.identifier = new ArrayList<Identifier>(); 1835 this.identifier.add(t); 1836 return this; 1837 } 1838 1839 /** 1840 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1841 */ 1842 public Identifier getIdentifierFirstRep() { 1843 if (getIdentifier().isEmpty()) { 1844 addIdentifier(); 1845 } 1846 return getIdentifier().get(0); 1847 } 1848 1849 /** 1850 * @return {@link #basedOn} (A plan or request that is fulfilled in whole or in part by this medication request.) 1851 */ 1852 public List<Reference> getBasedOn() { 1853 if (this.basedOn == null) 1854 this.basedOn = new ArrayList<Reference>(); 1855 return this.basedOn; 1856 } 1857 1858 /** 1859 * @return Returns a reference to <code>this</code> for easy method chaining 1860 */ 1861 public MedicationRequest setBasedOn(List<Reference> theBasedOn) { 1862 this.basedOn = theBasedOn; 1863 return this; 1864 } 1865 1866 public boolean hasBasedOn() { 1867 if (this.basedOn == null) 1868 return false; 1869 for (Reference item : this.basedOn) 1870 if (!item.isEmpty()) 1871 return true; 1872 return false; 1873 } 1874 1875 public Reference addBasedOn() { //3 1876 Reference t = new Reference(); 1877 if (this.basedOn == null) 1878 this.basedOn = new ArrayList<Reference>(); 1879 this.basedOn.add(t); 1880 return t; 1881 } 1882 1883 public MedicationRequest addBasedOn(Reference t) { //3 1884 if (t == null) 1885 return this; 1886 if (this.basedOn == null) 1887 this.basedOn = new ArrayList<Reference>(); 1888 this.basedOn.add(t); 1889 return this; 1890 } 1891 1892 /** 1893 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1894 */ 1895 public Reference getBasedOnFirstRep() { 1896 if (getBasedOn().isEmpty()) { 1897 addBasedOn(); 1898 } 1899 return getBasedOn().get(0); 1900 } 1901 1902 /** 1903 * @return {@link #priorPrescription} (Reference to an order/prescription that is being replaced by this MedicationRequest.) 1904 */ 1905 public Reference getPriorPrescription() { 1906 if (this.priorPrescription == null) 1907 if (Configuration.errorOnAutoCreate()) 1908 throw new Error("Attempt to auto-create MedicationRequest.priorPrescription"); 1909 else if (Configuration.doAutoCreate()) 1910 this.priorPrescription = new Reference(); // cc 1911 return this.priorPrescription; 1912 } 1913 1914 public boolean hasPriorPrescription() { 1915 return this.priorPrescription != null && !this.priorPrescription.isEmpty(); 1916 } 1917 1918 /** 1919 * @param value {@link #priorPrescription} (Reference to an order/prescription that is being replaced by this MedicationRequest.) 1920 */ 1921 public MedicationRequest setPriorPrescription(Reference value) { 1922 this.priorPrescription = value; 1923 return this; 1924 } 1925 1926 /** 1927 * @return {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 1928 */ 1929 public Identifier getGroupIdentifier() { 1930 if (this.groupIdentifier == null) 1931 if (Configuration.errorOnAutoCreate()) 1932 throw new Error("Attempt to auto-create MedicationRequest.groupIdentifier"); 1933 else if (Configuration.doAutoCreate()) 1934 this.groupIdentifier = new Identifier(); // cc 1935 return this.groupIdentifier; 1936 } 1937 1938 public boolean hasGroupIdentifier() { 1939 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 1944 */ 1945 public MedicationRequest setGroupIdentifier(Identifier value) { 1946 this.groupIdentifier = value; 1947 return this; 1948 } 1949 1950 /** 1951 * @return {@link #status} (A code specifying the current state of the order. Generally, this will be active or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1952 */ 1953 public Enumeration<MedicationrequestStatus> getStatusElement() { 1954 if (this.status == null) 1955 if (Configuration.errorOnAutoCreate()) 1956 throw new Error("Attempt to auto-create MedicationRequest.status"); 1957 else if (Configuration.doAutoCreate()) 1958 this.status = new Enumeration<MedicationrequestStatus>(new MedicationrequestStatusEnumFactory()); // bb 1959 return this.status; 1960 } 1961 1962 public boolean hasStatusElement() { 1963 return this.status != null && !this.status.isEmpty(); 1964 } 1965 1966 public boolean hasStatus() { 1967 return this.status != null && !this.status.isEmpty(); 1968 } 1969 1970 /** 1971 * @param value {@link #status} (A code specifying the current state of the order. Generally, this will be active or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1972 */ 1973 public MedicationRequest setStatusElement(Enumeration<MedicationrequestStatus> value) { 1974 this.status = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return A code specifying the current state of the order. Generally, this will be active or completed state. 1980 */ 1981 public MedicationrequestStatus getStatus() { 1982 return this.status == null ? null : this.status.getValue(); 1983 } 1984 1985 /** 1986 * @param value A code specifying the current state of the order. Generally, this will be active or completed state. 1987 */ 1988 public MedicationRequest setStatus(MedicationrequestStatus value) { 1989 if (this.status == null) 1990 this.status = new Enumeration<MedicationrequestStatus>(new MedicationrequestStatusEnumFactory()); 1991 this.status.setValue(value); 1992 return this; 1993 } 1994 1995 /** 1996 * @return {@link #statusReason} (Captures the reason for the current state of the MedicationRequest.) 1997 */ 1998 public CodeableConcept getStatusReason() { 1999 if (this.statusReason == null) 2000 if (Configuration.errorOnAutoCreate()) 2001 throw new Error("Attempt to auto-create MedicationRequest.statusReason"); 2002 else if (Configuration.doAutoCreate()) 2003 this.statusReason = new CodeableConcept(); // cc 2004 return this.statusReason; 2005 } 2006 2007 public boolean hasStatusReason() { 2008 return this.statusReason != null && !this.statusReason.isEmpty(); 2009 } 2010 2011 /** 2012 * @param value {@link #statusReason} (Captures the reason for the current state of the MedicationRequest.) 2013 */ 2014 public MedicationRequest setStatusReason(CodeableConcept value) { 2015 this.statusReason = value; 2016 return this; 2017 } 2018 2019 /** 2020 * @return {@link #statusChanged} (The date (and perhaps time) when the status was changed.). This is the underlying object with id, value and extensions. The accessor "getStatusChanged" gives direct access to the value 2021 */ 2022 public DateTimeType getStatusChangedElement() { 2023 if (this.statusChanged == null) 2024 if (Configuration.errorOnAutoCreate()) 2025 throw new Error("Attempt to auto-create MedicationRequest.statusChanged"); 2026 else if (Configuration.doAutoCreate()) 2027 this.statusChanged = new DateTimeType(); // bb 2028 return this.statusChanged; 2029 } 2030 2031 public boolean hasStatusChangedElement() { 2032 return this.statusChanged != null && !this.statusChanged.isEmpty(); 2033 } 2034 2035 public boolean hasStatusChanged() { 2036 return this.statusChanged != null && !this.statusChanged.isEmpty(); 2037 } 2038 2039 /** 2040 * @param value {@link #statusChanged} (The date (and perhaps time) when the status was changed.). This is the underlying object with id, value and extensions. The accessor "getStatusChanged" gives direct access to the value 2041 */ 2042 public MedicationRequest setStatusChangedElement(DateTimeType value) { 2043 this.statusChanged = value; 2044 return this; 2045 } 2046 2047 /** 2048 * @return The date (and perhaps time) when the status was changed. 2049 */ 2050 public Date getStatusChanged() { 2051 return this.statusChanged == null ? null : this.statusChanged.getValue(); 2052 } 2053 2054 /** 2055 * @param value The date (and perhaps time) when the status was changed. 2056 */ 2057 public MedicationRequest setStatusChanged(Date value) { 2058 if (value == null) 2059 this.statusChanged = null; 2060 else { 2061 if (this.statusChanged == null) 2062 this.statusChanged = new DateTimeType(); 2063 this.statusChanged.setValue(value); 2064 } 2065 return this; 2066 } 2067 2068 /** 2069 * @return {@link #intent} (Whether the request is a proposal, plan, or an original order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2070 */ 2071 public Enumeration<MedicationRequestIntent> getIntentElement() { 2072 if (this.intent == null) 2073 if (Configuration.errorOnAutoCreate()) 2074 throw new Error("Attempt to auto-create MedicationRequest.intent"); 2075 else if (Configuration.doAutoCreate()) 2076 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); // bb 2077 return this.intent; 2078 } 2079 2080 public boolean hasIntentElement() { 2081 return this.intent != null && !this.intent.isEmpty(); 2082 } 2083 2084 public boolean hasIntent() { 2085 return this.intent != null && !this.intent.isEmpty(); 2086 } 2087 2088 /** 2089 * @param value {@link #intent} (Whether the request is a proposal, plan, or an original order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2090 */ 2091 public MedicationRequest setIntentElement(Enumeration<MedicationRequestIntent> value) { 2092 this.intent = value; 2093 return this; 2094 } 2095 2096 /** 2097 * @return Whether the request is a proposal, plan, or an original order. 2098 */ 2099 public MedicationRequestIntent getIntent() { 2100 return this.intent == null ? null : this.intent.getValue(); 2101 } 2102 2103 /** 2104 * @param value Whether the request is a proposal, plan, or an original order. 2105 */ 2106 public MedicationRequest setIntent(MedicationRequestIntent value) { 2107 if (this.intent == null) 2108 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); 2109 this.intent.setValue(value); 2110 return this; 2111 } 2112 2113 /** 2114 * @return {@link #category} (An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication.) 2115 */ 2116 public List<CodeableConcept> getCategory() { 2117 if (this.category == null) 2118 this.category = new ArrayList<CodeableConcept>(); 2119 return this.category; 2120 } 2121 2122 /** 2123 * @return Returns a reference to <code>this</code> for easy method chaining 2124 */ 2125 public MedicationRequest setCategory(List<CodeableConcept> theCategory) { 2126 this.category = theCategory; 2127 return this; 2128 } 2129 2130 public boolean hasCategory() { 2131 if (this.category == null) 2132 return false; 2133 for (CodeableConcept item : this.category) 2134 if (!item.isEmpty()) 2135 return true; 2136 return false; 2137 } 2138 2139 public CodeableConcept addCategory() { //3 2140 CodeableConcept t = new CodeableConcept(); 2141 if (this.category == null) 2142 this.category = new ArrayList<CodeableConcept>(); 2143 this.category.add(t); 2144 return t; 2145 } 2146 2147 public MedicationRequest addCategory(CodeableConcept t) { //3 2148 if (t == null) 2149 return this; 2150 if (this.category == null) 2151 this.category = new ArrayList<CodeableConcept>(); 2152 this.category.add(t); 2153 return this; 2154 } 2155 2156 /** 2157 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2158 */ 2159 public CodeableConcept getCategoryFirstRep() { 2160 if (getCategory().isEmpty()) { 2161 addCategory(); 2162 } 2163 return getCategory().get(0); 2164 } 2165 2166 /** 2167 * @return {@link #priority} (Indicates how quickly the Medication Request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2168 */ 2169 public Enumeration<RequestPriority> getPriorityElement() { 2170 if (this.priority == null) 2171 if (Configuration.errorOnAutoCreate()) 2172 throw new Error("Attempt to auto-create MedicationRequest.priority"); 2173 else if (Configuration.doAutoCreate()) 2174 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 2175 return this.priority; 2176 } 2177 2178 public boolean hasPriorityElement() { 2179 return this.priority != null && !this.priority.isEmpty(); 2180 } 2181 2182 public boolean hasPriority() { 2183 return this.priority != null && !this.priority.isEmpty(); 2184 } 2185 2186 /** 2187 * @param value {@link #priority} (Indicates how quickly the Medication Request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2188 */ 2189 public MedicationRequest setPriorityElement(Enumeration<RequestPriority> value) { 2190 this.priority = value; 2191 return this; 2192 } 2193 2194 /** 2195 * @return Indicates how quickly the Medication Request should be addressed with respect to other requests. 2196 */ 2197 public RequestPriority getPriority() { 2198 return this.priority == null ? null : this.priority.getValue(); 2199 } 2200 2201 /** 2202 * @param value Indicates how quickly the Medication Request should be addressed with respect to other requests. 2203 */ 2204 public MedicationRequest setPriority(RequestPriority value) { 2205 if (value == null) 2206 this.priority = null; 2207 else { 2208 if (this.priority == null) 2209 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 2210 this.priority.setValue(value); 2211 } 2212 return this; 2213 } 2214 2215 /** 2216 * @return {@link #doNotPerform} (If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 2217 */ 2218 public BooleanType getDoNotPerformElement() { 2219 if (this.doNotPerform == null) 2220 if (Configuration.errorOnAutoCreate()) 2221 throw new Error("Attempt to auto-create MedicationRequest.doNotPerform"); 2222 else if (Configuration.doAutoCreate()) 2223 this.doNotPerform = new BooleanType(); // bb 2224 return this.doNotPerform; 2225 } 2226 2227 public boolean hasDoNotPerformElement() { 2228 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2229 } 2230 2231 public boolean hasDoNotPerform() { 2232 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2233 } 2234 2235 /** 2236 * @param value {@link #doNotPerform} (If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 2237 */ 2238 public MedicationRequest setDoNotPerformElement(BooleanType value) { 2239 this.doNotPerform = value; 2240 return this; 2241 } 2242 2243 /** 2244 * @return If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication. 2245 */ 2246 public boolean getDoNotPerform() { 2247 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 2248 } 2249 2250 /** 2251 * @param value If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication. 2252 */ 2253 public MedicationRequest setDoNotPerform(boolean value) { 2254 if (this.doNotPerform == null) 2255 this.doNotPerform = new BooleanType(); 2256 this.doNotPerform.setValue(value); 2257 return this; 2258 } 2259 2260 /** 2261 * @return {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 2262 */ 2263 public CodeableReference getMedication() { 2264 if (this.medication == null) 2265 if (Configuration.errorOnAutoCreate()) 2266 throw new Error("Attempt to auto-create MedicationRequest.medication"); 2267 else if (Configuration.doAutoCreate()) 2268 this.medication = new CodeableReference(); // cc 2269 return this.medication; 2270 } 2271 2272 public boolean hasMedication() { 2273 return this.medication != null && !this.medication.isEmpty(); 2274 } 2275 2276 /** 2277 * @param value {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 2278 */ 2279 public MedicationRequest setMedication(CodeableReference value) { 2280 this.medication = value; 2281 return this; 2282 } 2283 2284 /** 2285 * @return {@link #subject} (The individual or group for whom the medication has been requested.) 2286 */ 2287 public Reference getSubject() { 2288 if (this.subject == null) 2289 if (Configuration.errorOnAutoCreate()) 2290 throw new Error("Attempt to auto-create MedicationRequest.subject"); 2291 else if (Configuration.doAutoCreate()) 2292 this.subject = new Reference(); // cc 2293 return this.subject; 2294 } 2295 2296 public boolean hasSubject() { 2297 return this.subject != null && !this.subject.isEmpty(); 2298 } 2299 2300 /** 2301 * @param value {@link #subject} (The individual or group for whom the medication has been requested.) 2302 */ 2303 public MedicationRequest setSubject(Reference value) { 2304 this.subject = value; 2305 return this; 2306 } 2307 2308 /** 2309 * @return {@link #informationSource} (The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person.) 2310 */ 2311 public List<Reference> getInformationSource() { 2312 if (this.informationSource == null) 2313 this.informationSource = new ArrayList<Reference>(); 2314 return this.informationSource; 2315 } 2316 2317 /** 2318 * @return Returns a reference to <code>this</code> for easy method chaining 2319 */ 2320 public MedicationRequest setInformationSource(List<Reference> theInformationSource) { 2321 this.informationSource = theInformationSource; 2322 return this; 2323 } 2324 2325 public boolean hasInformationSource() { 2326 if (this.informationSource == null) 2327 return false; 2328 for (Reference item : this.informationSource) 2329 if (!item.isEmpty()) 2330 return true; 2331 return false; 2332 } 2333 2334 public Reference addInformationSource() { //3 2335 Reference t = new Reference(); 2336 if (this.informationSource == null) 2337 this.informationSource = new ArrayList<Reference>(); 2338 this.informationSource.add(t); 2339 return t; 2340 } 2341 2342 public MedicationRequest addInformationSource(Reference t) { //3 2343 if (t == null) 2344 return this; 2345 if (this.informationSource == null) 2346 this.informationSource = new ArrayList<Reference>(); 2347 this.informationSource.add(t); 2348 return this; 2349 } 2350 2351 /** 2352 * @return The first repetition of repeating field {@link #informationSource}, creating it if it does not already exist {3} 2353 */ 2354 public Reference getInformationSourceFirstRep() { 2355 if (getInformationSource().isEmpty()) { 2356 addInformationSource(); 2357 } 2358 return getInformationSource().get(0); 2359 } 2360 2361 /** 2362 * @return {@link #encounter} (The Encounter during which this [x] was created or to which the creation of this record is tightly associated.) 2363 */ 2364 public Reference getEncounter() { 2365 if (this.encounter == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create MedicationRequest.encounter"); 2368 else if (Configuration.doAutoCreate()) 2369 this.encounter = new Reference(); // cc 2370 return this.encounter; 2371 } 2372 2373 public boolean hasEncounter() { 2374 return this.encounter != null && !this.encounter.isEmpty(); 2375 } 2376 2377 /** 2378 * @param value {@link #encounter} (The Encounter during which this [x] was created or to which the creation of this record is tightly associated.) 2379 */ 2380 public MedicationRequest setEncounter(Reference value) { 2381 this.encounter = value; 2382 return this; 2383 } 2384 2385 /** 2386 * @return {@link #supportingInformation} (Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient).) 2387 */ 2388 public List<Reference> getSupportingInformation() { 2389 if (this.supportingInformation == null) 2390 this.supportingInformation = new ArrayList<Reference>(); 2391 return this.supportingInformation; 2392 } 2393 2394 /** 2395 * @return Returns a reference to <code>this</code> for easy method chaining 2396 */ 2397 public MedicationRequest setSupportingInformation(List<Reference> theSupportingInformation) { 2398 this.supportingInformation = theSupportingInformation; 2399 return this; 2400 } 2401 2402 public boolean hasSupportingInformation() { 2403 if (this.supportingInformation == null) 2404 return false; 2405 for (Reference item : this.supportingInformation) 2406 if (!item.isEmpty()) 2407 return true; 2408 return false; 2409 } 2410 2411 public Reference addSupportingInformation() { //3 2412 Reference t = new Reference(); 2413 if (this.supportingInformation == null) 2414 this.supportingInformation = new ArrayList<Reference>(); 2415 this.supportingInformation.add(t); 2416 return t; 2417 } 2418 2419 public MedicationRequest addSupportingInformation(Reference t) { //3 2420 if (t == null) 2421 return this; 2422 if (this.supportingInformation == null) 2423 this.supportingInformation = new ArrayList<Reference>(); 2424 this.supportingInformation.add(t); 2425 return this; 2426 } 2427 2428 /** 2429 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 2430 */ 2431 public Reference getSupportingInformationFirstRep() { 2432 if (getSupportingInformation().isEmpty()) { 2433 addSupportingInformation(); 2434 } 2435 return getSupportingInformation().get(0); 2436 } 2437 2438 /** 2439 * @return {@link #authoredOn} (The date (and perhaps time) when the prescription was initially written or authored on.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2440 */ 2441 public DateTimeType getAuthoredOnElement() { 2442 if (this.authoredOn == null) 2443 if (Configuration.errorOnAutoCreate()) 2444 throw new Error("Attempt to auto-create MedicationRequest.authoredOn"); 2445 else if (Configuration.doAutoCreate()) 2446 this.authoredOn = new DateTimeType(); // bb 2447 return this.authoredOn; 2448 } 2449 2450 public boolean hasAuthoredOnElement() { 2451 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2452 } 2453 2454 public boolean hasAuthoredOn() { 2455 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2456 } 2457 2458 /** 2459 * @param value {@link #authoredOn} (The date (and perhaps time) when the prescription was initially written or authored on.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2460 */ 2461 public MedicationRequest setAuthoredOnElement(DateTimeType value) { 2462 this.authoredOn = value; 2463 return this; 2464 } 2465 2466 /** 2467 * @return The date (and perhaps time) when the prescription was initially written or authored on. 2468 */ 2469 public Date getAuthoredOn() { 2470 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2471 } 2472 2473 /** 2474 * @param value The date (and perhaps time) when the prescription was initially written or authored on. 2475 */ 2476 public MedicationRequest setAuthoredOn(Date value) { 2477 if (value == null) 2478 this.authoredOn = null; 2479 else { 2480 if (this.authoredOn == null) 2481 this.authoredOn = new DateTimeType(); 2482 this.authoredOn.setValue(value); 2483 } 2484 return this; 2485 } 2486 2487 /** 2488 * @return {@link #requester} (The individual, organization, or device that initiated the request and has responsibility for its activation.) 2489 */ 2490 public Reference getRequester() { 2491 if (this.requester == null) 2492 if (Configuration.errorOnAutoCreate()) 2493 throw new Error("Attempt to auto-create MedicationRequest.requester"); 2494 else if (Configuration.doAutoCreate()) 2495 this.requester = new Reference(); // cc 2496 return this.requester; 2497 } 2498 2499 public boolean hasRequester() { 2500 return this.requester != null && !this.requester.isEmpty(); 2501 } 2502 2503 /** 2504 * @param value {@link #requester} (The individual, organization, or device that initiated the request and has responsibility for its activation.) 2505 */ 2506 public MedicationRequest setRequester(Reference value) { 2507 this.requester = value; 2508 return this; 2509 } 2510 2511 /** 2512 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 2513 */ 2514 public BooleanType getReportedElement() { 2515 if (this.reported == null) 2516 if (Configuration.errorOnAutoCreate()) 2517 throw new Error("Attempt to auto-create MedicationRequest.reported"); 2518 else if (Configuration.doAutoCreate()) 2519 this.reported = new BooleanType(); // bb 2520 return this.reported; 2521 } 2522 2523 public boolean hasReportedElement() { 2524 return this.reported != null && !this.reported.isEmpty(); 2525 } 2526 2527 public boolean hasReported() { 2528 return this.reported != null && !this.reported.isEmpty(); 2529 } 2530 2531 /** 2532 * @param value {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 2533 */ 2534 public MedicationRequest setReportedElement(BooleanType value) { 2535 this.reported = value; 2536 return this; 2537 } 2538 2539 /** 2540 * @return Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report. 2541 */ 2542 public boolean getReported() { 2543 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 2544 } 2545 2546 /** 2547 * @param value Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report. 2548 */ 2549 public MedicationRequest setReported(boolean value) { 2550 if (this.reported == null) 2551 this.reported = new BooleanType(); 2552 this.reported.setValue(value); 2553 return this; 2554 } 2555 2556 /** 2557 * @return {@link #performerType} (Indicates the type of performer of the administration of the medication.) 2558 */ 2559 public CodeableConcept getPerformerType() { 2560 if (this.performerType == null) 2561 if (Configuration.errorOnAutoCreate()) 2562 throw new Error("Attempt to auto-create MedicationRequest.performerType"); 2563 else if (Configuration.doAutoCreate()) 2564 this.performerType = new CodeableConcept(); // cc 2565 return this.performerType; 2566 } 2567 2568 public boolean hasPerformerType() { 2569 return this.performerType != null && !this.performerType.isEmpty(); 2570 } 2571 2572 /** 2573 * @param value {@link #performerType} (Indicates the type of performer of the administration of the medication.) 2574 */ 2575 public MedicationRequest setPerformerType(CodeableConcept value) { 2576 this.performerType = value; 2577 return this; 2578 } 2579 2580 /** 2581 * @return {@link #performer} (The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.) 2582 */ 2583 public List<Reference> getPerformer() { 2584 if (this.performer == null) 2585 this.performer = new ArrayList<Reference>(); 2586 return this.performer; 2587 } 2588 2589 /** 2590 * @return Returns a reference to <code>this</code> for easy method chaining 2591 */ 2592 public MedicationRequest setPerformer(List<Reference> thePerformer) { 2593 this.performer = thePerformer; 2594 return this; 2595 } 2596 2597 public boolean hasPerformer() { 2598 if (this.performer == null) 2599 return false; 2600 for (Reference item : this.performer) 2601 if (!item.isEmpty()) 2602 return true; 2603 return false; 2604 } 2605 2606 public Reference addPerformer() { //3 2607 Reference t = new Reference(); 2608 if (this.performer == null) 2609 this.performer = new ArrayList<Reference>(); 2610 this.performer.add(t); 2611 return t; 2612 } 2613 2614 public MedicationRequest addPerformer(Reference t) { //3 2615 if (t == null) 2616 return this; 2617 if (this.performer == null) 2618 this.performer = new ArrayList<Reference>(); 2619 this.performer.add(t); 2620 return this; 2621 } 2622 2623 /** 2624 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2625 */ 2626 public Reference getPerformerFirstRep() { 2627 if (getPerformer().isEmpty()) { 2628 addPerformer(); 2629 } 2630 return getPerformer().get(0); 2631 } 2632 2633 /** 2634 * @return {@link #device} (The intended type of device that is to be used for the administration of the medication (for example, PCA Pump).) 2635 */ 2636 public List<CodeableReference> getDevice() { 2637 if (this.device == null) 2638 this.device = new ArrayList<CodeableReference>(); 2639 return this.device; 2640 } 2641 2642 /** 2643 * @return Returns a reference to <code>this</code> for easy method chaining 2644 */ 2645 public MedicationRequest setDevice(List<CodeableReference> theDevice) { 2646 this.device = theDevice; 2647 return this; 2648 } 2649 2650 public boolean hasDevice() { 2651 if (this.device == null) 2652 return false; 2653 for (CodeableReference item : this.device) 2654 if (!item.isEmpty()) 2655 return true; 2656 return false; 2657 } 2658 2659 public CodeableReference addDevice() { //3 2660 CodeableReference t = new CodeableReference(); 2661 if (this.device == null) 2662 this.device = new ArrayList<CodeableReference>(); 2663 this.device.add(t); 2664 return t; 2665 } 2666 2667 public MedicationRequest addDevice(CodeableReference t) { //3 2668 if (t == null) 2669 return this; 2670 if (this.device == null) 2671 this.device = new ArrayList<CodeableReference>(); 2672 this.device.add(t); 2673 return this; 2674 } 2675 2676 /** 2677 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 2678 */ 2679 public CodeableReference getDeviceFirstRep() { 2680 if (getDevice().isEmpty()) { 2681 addDevice(); 2682 } 2683 return getDevice().get(0); 2684 } 2685 2686 /** 2687 * @return {@link #recorder} (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2688 */ 2689 public Reference getRecorder() { 2690 if (this.recorder == null) 2691 if (Configuration.errorOnAutoCreate()) 2692 throw new Error("Attempt to auto-create MedicationRequest.recorder"); 2693 else if (Configuration.doAutoCreate()) 2694 this.recorder = new Reference(); // cc 2695 return this.recorder; 2696 } 2697 2698 public boolean hasRecorder() { 2699 return this.recorder != null && !this.recorder.isEmpty(); 2700 } 2701 2702 /** 2703 * @param value {@link #recorder} (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2704 */ 2705 public MedicationRequest setRecorder(Reference value) { 2706 this.recorder = value; 2707 return this; 2708 } 2709 2710 /** 2711 * @return {@link #reason} (The reason or the indication for ordering or not ordering the medication.) 2712 */ 2713 public List<CodeableReference> getReason() { 2714 if (this.reason == null) 2715 this.reason = new ArrayList<CodeableReference>(); 2716 return this.reason; 2717 } 2718 2719 /** 2720 * @return Returns a reference to <code>this</code> for easy method chaining 2721 */ 2722 public MedicationRequest setReason(List<CodeableReference> theReason) { 2723 this.reason = theReason; 2724 return this; 2725 } 2726 2727 public boolean hasReason() { 2728 if (this.reason == null) 2729 return false; 2730 for (CodeableReference item : this.reason) 2731 if (!item.isEmpty()) 2732 return true; 2733 return false; 2734 } 2735 2736 public CodeableReference addReason() { //3 2737 CodeableReference t = new CodeableReference(); 2738 if (this.reason == null) 2739 this.reason = new ArrayList<CodeableReference>(); 2740 this.reason.add(t); 2741 return t; 2742 } 2743 2744 public MedicationRequest addReason(CodeableReference t) { //3 2745 if (t == null) 2746 return this; 2747 if (this.reason == null) 2748 this.reason = new ArrayList<CodeableReference>(); 2749 this.reason.add(t); 2750 return this; 2751 } 2752 2753 /** 2754 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2755 */ 2756 public CodeableReference getReasonFirstRep() { 2757 if (getReason().isEmpty()) { 2758 addReason(); 2759 } 2760 return getReason().get(0); 2761 } 2762 2763 /** 2764 * @return {@link #courseOfTherapyType} (The description of the overall pattern of the administration of the medication to the patient.) 2765 */ 2766 public CodeableConcept getCourseOfTherapyType() { 2767 if (this.courseOfTherapyType == null) 2768 if (Configuration.errorOnAutoCreate()) 2769 throw new Error("Attempt to auto-create MedicationRequest.courseOfTherapyType"); 2770 else if (Configuration.doAutoCreate()) 2771 this.courseOfTherapyType = new CodeableConcept(); // cc 2772 return this.courseOfTherapyType; 2773 } 2774 2775 public boolean hasCourseOfTherapyType() { 2776 return this.courseOfTherapyType != null && !this.courseOfTherapyType.isEmpty(); 2777 } 2778 2779 /** 2780 * @param value {@link #courseOfTherapyType} (The description of the overall pattern of the administration of the medication to the patient.) 2781 */ 2782 public MedicationRequest setCourseOfTherapyType(CodeableConcept value) { 2783 this.courseOfTherapyType = value; 2784 return this; 2785 } 2786 2787 /** 2788 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.) 2789 */ 2790 public List<Reference> getInsurance() { 2791 if (this.insurance == null) 2792 this.insurance = new ArrayList<Reference>(); 2793 return this.insurance; 2794 } 2795 2796 /** 2797 * @return Returns a reference to <code>this</code> for easy method chaining 2798 */ 2799 public MedicationRequest setInsurance(List<Reference> theInsurance) { 2800 this.insurance = theInsurance; 2801 return this; 2802 } 2803 2804 public boolean hasInsurance() { 2805 if (this.insurance == null) 2806 return false; 2807 for (Reference item : this.insurance) 2808 if (!item.isEmpty()) 2809 return true; 2810 return false; 2811 } 2812 2813 public Reference addInsurance() { //3 2814 Reference t = new Reference(); 2815 if (this.insurance == null) 2816 this.insurance = new ArrayList<Reference>(); 2817 this.insurance.add(t); 2818 return t; 2819 } 2820 2821 public MedicationRequest addInsurance(Reference t) { //3 2822 if (t == null) 2823 return this; 2824 if (this.insurance == null) 2825 this.insurance = new ArrayList<Reference>(); 2826 this.insurance.add(t); 2827 return this; 2828 } 2829 2830 /** 2831 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 2832 */ 2833 public Reference getInsuranceFirstRep() { 2834 if (getInsurance().isEmpty()) { 2835 addInsurance(); 2836 } 2837 return getInsurance().get(0); 2838 } 2839 2840 /** 2841 * @return {@link #note} (Extra information about the prescription that could not be conveyed by the other attributes.) 2842 */ 2843 public List<Annotation> getNote() { 2844 if (this.note == null) 2845 this.note = new ArrayList<Annotation>(); 2846 return this.note; 2847 } 2848 2849 /** 2850 * @return Returns a reference to <code>this</code> for easy method chaining 2851 */ 2852 public MedicationRequest setNote(List<Annotation> theNote) { 2853 this.note = theNote; 2854 return this; 2855 } 2856 2857 public boolean hasNote() { 2858 if (this.note == null) 2859 return false; 2860 for (Annotation item : this.note) 2861 if (!item.isEmpty()) 2862 return true; 2863 return false; 2864 } 2865 2866 public Annotation addNote() { //3 2867 Annotation t = new Annotation(); 2868 if (this.note == null) 2869 this.note = new ArrayList<Annotation>(); 2870 this.note.add(t); 2871 return t; 2872 } 2873 2874 public MedicationRequest addNote(Annotation t) { //3 2875 if (t == null) 2876 return this; 2877 if (this.note == null) 2878 this.note = new ArrayList<Annotation>(); 2879 this.note.add(t); 2880 return this; 2881 } 2882 2883 /** 2884 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2885 */ 2886 public Annotation getNoteFirstRep() { 2887 if (getNote().isEmpty()) { 2888 addNote(); 2889 } 2890 return getNote().get(0); 2891 } 2892 2893 /** 2894 * @return {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 2895 */ 2896 public MarkdownType getRenderedDosageInstructionElement() { 2897 if (this.renderedDosageInstruction == null) 2898 if (Configuration.errorOnAutoCreate()) 2899 throw new Error("Attempt to auto-create MedicationRequest.renderedDosageInstruction"); 2900 else if (Configuration.doAutoCreate()) 2901 this.renderedDosageInstruction = new MarkdownType(); // bb 2902 return this.renderedDosageInstruction; 2903 } 2904 2905 public boolean hasRenderedDosageInstructionElement() { 2906 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 2907 } 2908 2909 public boolean hasRenderedDosageInstruction() { 2910 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 2911 } 2912 2913 /** 2914 * @param value {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 2915 */ 2916 public MedicationRequest setRenderedDosageInstructionElement(MarkdownType value) { 2917 this.renderedDosageInstruction = value; 2918 return this; 2919 } 2920 2921 /** 2922 * @return The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 2923 */ 2924 public String getRenderedDosageInstruction() { 2925 return this.renderedDosageInstruction == null ? null : this.renderedDosageInstruction.getValue(); 2926 } 2927 2928 /** 2929 * @param value The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 2930 */ 2931 public MedicationRequest setRenderedDosageInstruction(String value) { 2932 if (Utilities.noString(value)) 2933 this.renderedDosageInstruction = null; 2934 else { 2935 if (this.renderedDosageInstruction == null) 2936 this.renderedDosageInstruction = new MarkdownType(); 2937 this.renderedDosageInstruction.setValue(value); 2938 } 2939 return this; 2940 } 2941 2942 /** 2943 * @return {@link #effectiveDosePeriod} (The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.) 2944 */ 2945 public Period getEffectiveDosePeriod() { 2946 if (this.effectiveDosePeriod == null) 2947 if (Configuration.errorOnAutoCreate()) 2948 throw new Error("Attempt to auto-create MedicationRequest.effectiveDosePeriod"); 2949 else if (Configuration.doAutoCreate()) 2950 this.effectiveDosePeriod = new Period(); // cc 2951 return this.effectiveDosePeriod; 2952 } 2953 2954 public boolean hasEffectiveDosePeriod() { 2955 return this.effectiveDosePeriod != null && !this.effectiveDosePeriod.isEmpty(); 2956 } 2957 2958 /** 2959 * @param value {@link #effectiveDosePeriod} (The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.) 2960 */ 2961 public MedicationRequest setEffectiveDosePeriod(Period value) { 2962 this.effectiveDosePeriod = value; 2963 return this; 2964 } 2965 2966 /** 2967 * @return {@link #dosageInstruction} (Specific instructions for how the medication is to be used by the patient.) 2968 */ 2969 public List<Dosage> getDosageInstruction() { 2970 if (this.dosageInstruction == null) 2971 this.dosageInstruction = new ArrayList<Dosage>(); 2972 return this.dosageInstruction; 2973 } 2974 2975 /** 2976 * @return Returns a reference to <code>this</code> for easy method chaining 2977 */ 2978 public MedicationRequest setDosageInstruction(List<Dosage> theDosageInstruction) { 2979 this.dosageInstruction = theDosageInstruction; 2980 return this; 2981 } 2982 2983 public boolean hasDosageInstruction() { 2984 if (this.dosageInstruction == null) 2985 return false; 2986 for (Dosage item : this.dosageInstruction) 2987 if (!item.isEmpty()) 2988 return true; 2989 return false; 2990 } 2991 2992 public Dosage addDosageInstruction() { //3 2993 Dosage t = new Dosage(); 2994 if (this.dosageInstruction == null) 2995 this.dosageInstruction = new ArrayList<Dosage>(); 2996 this.dosageInstruction.add(t); 2997 return t; 2998 } 2999 3000 public MedicationRequest addDosageInstruction(Dosage t) { //3 3001 if (t == null) 3002 return this; 3003 if (this.dosageInstruction == null) 3004 this.dosageInstruction = new ArrayList<Dosage>(); 3005 this.dosageInstruction.add(t); 3006 return this; 3007 } 3008 3009 /** 3010 * @return The first repetition of repeating field {@link #dosageInstruction}, creating it if it does not already exist {3} 3011 */ 3012 public Dosage getDosageInstructionFirstRep() { 3013 if (getDosageInstruction().isEmpty()) { 3014 addDosageInstruction(); 3015 } 3016 return getDosageInstruction().get(0); 3017 } 3018 3019 /** 3020 * @return {@link #dispenseRequest} (Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.) 3021 */ 3022 public MedicationRequestDispenseRequestComponent getDispenseRequest() { 3023 if (this.dispenseRequest == null) 3024 if (Configuration.errorOnAutoCreate()) 3025 throw new Error("Attempt to auto-create MedicationRequest.dispenseRequest"); 3026 else if (Configuration.doAutoCreate()) 3027 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); // cc 3028 return this.dispenseRequest; 3029 } 3030 3031 public boolean hasDispenseRequest() { 3032 return this.dispenseRequest != null && !this.dispenseRequest.isEmpty(); 3033 } 3034 3035 /** 3036 * @param value {@link #dispenseRequest} (Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.) 3037 */ 3038 public MedicationRequest setDispenseRequest(MedicationRequestDispenseRequestComponent value) { 3039 this.dispenseRequest = value; 3040 return this; 3041 } 3042 3043 /** 3044 * @return {@link #substitution} (Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.) 3045 */ 3046 public MedicationRequestSubstitutionComponent getSubstitution() { 3047 if (this.substitution == null) 3048 if (Configuration.errorOnAutoCreate()) 3049 throw new Error("Attempt to auto-create MedicationRequest.substitution"); 3050 else if (Configuration.doAutoCreate()) 3051 this.substitution = new MedicationRequestSubstitutionComponent(); // cc 3052 return this.substitution; 3053 } 3054 3055 public boolean hasSubstitution() { 3056 return this.substitution != null && !this.substitution.isEmpty(); 3057 } 3058 3059 /** 3060 * @param value {@link #substitution} (Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.) 3061 */ 3062 public MedicationRequest setSubstitution(MedicationRequestSubstitutionComponent value) { 3063 this.substitution = value; 3064 return this; 3065 } 3066 3067 /** 3068 * @return {@link #eventHistory} (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 3069 */ 3070 public List<Reference> getEventHistory() { 3071 if (this.eventHistory == null) 3072 this.eventHistory = new ArrayList<Reference>(); 3073 return this.eventHistory; 3074 } 3075 3076 /** 3077 * @return Returns a reference to <code>this</code> for easy method chaining 3078 */ 3079 public MedicationRequest setEventHistory(List<Reference> theEventHistory) { 3080 this.eventHistory = theEventHistory; 3081 return this; 3082 } 3083 3084 public boolean hasEventHistory() { 3085 if (this.eventHistory == null) 3086 return false; 3087 for (Reference item : this.eventHistory) 3088 if (!item.isEmpty()) 3089 return true; 3090 return false; 3091 } 3092 3093 public Reference addEventHistory() { //3 3094 Reference t = new Reference(); 3095 if (this.eventHistory == null) 3096 this.eventHistory = new ArrayList<Reference>(); 3097 this.eventHistory.add(t); 3098 return t; 3099 } 3100 3101 public MedicationRequest addEventHistory(Reference t) { //3 3102 if (t == null) 3103 return this; 3104 if (this.eventHistory == null) 3105 this.eventHistory = new ArrayList<Reference>(); 3106 this.eventHistory.add(t); 3107 return this; 3108 } 3109 3110 /** 3111 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist {3} 3112 */ 3113 public Reference getEventHistoryFirstRep() { 3114 if (getEventHistory().isEmpty()) { 3115 addEventHistory(); 3116 } 3117 return getEventHistory().get(0); 3118 } 3119 3120 protected void listChildren(List<Property> children) { 3121 super.listChildren(children); 3122 children.add(new Property("identifier", "Identifier", "Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3123 children.add(new Property("basedOn", "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", "A plan or request that is fulfilled in whole or in part by this medication request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3124 children.add(new Property("priorPrescription", "Reference(MedicationRequest)", "Reference to an order/prescription that is being replaced by this MedicationRequest.", 0, 1, priorPrescription)); 3125 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier)); 3126 children.add(new Property("status", "code", "A code specifying the current state of the order. Generally, this will be active or completed state.", 0, 1, status)); 3127 children.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the MedicationRequest.", 0, 1, statusReason)); 3128 children.add(new Property("statusChanged", "dateTime", "The date (and perhaps time) when the status was changed.", 0, 1, statusChanged)); 3129 children.add(new Property("intent", "code", "Whether the request is a proposal, plan, or an original order.", 0, 1, intent)); 3130 children.add(new Property("category", "CodeableConcept", "An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication.", 0, java.lang.Integer.MAX_VALUE, category)); 3131 children.add(new Property("priority", "code", "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, priority)); 3132 children.add(new Property("doNotPerform", "boolean", "If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.", 0, 1, doNotPerform)); 3133 children.add(new Property("medication", "CodeableReference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 3134 children.add(new Property("subject", "Reference(Patient|Group)", "The individual or group for whom the medication has been requested.", 0, 1, subject)); 3135 children.add(new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person.", 0, java.lang.Integer.MAX_VALUE, informationSource)); 3136 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this [x] was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 3137 children.add(new Property("supportingInformation", "Reference(Any)", "Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient).", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 3138 children.add(new Property("authoredOn", "dateTime", "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn)); 3139 children.add(new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The individual, organization, or device that initiated the request and has responsibility for its activation.", 0, 1, requester)); 3140 children.add(new Property("reported", "boolean", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported)); 3141 children.add(new Property("performerType", "CodeableConcept", "Indicates the type of performer of the administration of the medication.", 0, 1, performerType)); 3142 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|Patient|DeviceDefinition|RelatedPerson|CareTeam|HealthcareService)", "The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.", 0, java.lang.Integer.MAX_VALUE, performer)); 3143 children.add(new Property("device", "CodeableReference(DeviceDefinition)", "The intended type of device that is to be used for the administration of the medication (for example, PCA Pump).", 0, java.lang.Integer.MAX_VALUE, device)); 3144 children.add(new Property("recorder", "Reference(Practitioner|PractitionerRole)", "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 0, 1, recorder)); 3145 children.add(new Property("reason", "CodeableReference(Condition|Observation)", "The reason or the indication for ordering or not ordering the medication.", 0, java.lang.Integer.MAX_VALUE, reason)); 3146 children.add(new Property("courseOfTherapyType", "CodeableConcept", "The description of the overall pattern of the administration of the medication to the patient.", 0, 1, courseOfTherapyType)); 3147 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance)); 3148 children.add(new Property("note", "Annotation", "Extra information about the prescription that could not be conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 3149 children.add(new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction)); 3150 children.add(new Property("effectiveDosePeriod", "Period", "The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.", 0, 1, effectiveDosePeriod)); 3151 children.add(new Property("dosageInstruction", "Dosage", "Specific instructions for how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 3152 children.add(new Property("dispenseRequest", "", "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 0, 1, dispenseRequest)); 3153 children.add(new Property("substitution", "", "Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 0, 1, substitution)); 3154 children.add(new Property("eventHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 3155 } 3156 3157 @Override 3158 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3159 switch (_hash) { 3160 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 3161 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", "A plan or request that is fulfilled in whole or in part by this medication request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3162 case -486355964: /*priorPrescription*/ return new Property("priorPrescription", "Reference(MedicationRequest)", "Reference to an order/prescription that is being replaced by this MedicationRequest.", 0, 1, priorPrescription); 3163 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier); 3164 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the current state of the order. Generally, this will be active or completed state.", 0, 1, status); 3165 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the MedicationRequest.", 0, 1, statusReason); 3166 case -1174686110: /*statusChanged*/ return new Property("statusChanged", "dateTime", "The date (and perhaps time) when the status was changed.", 0, 1, statusChanged); 3167 case -1183762788: /*intent*/ return new Property("intent", "code", "Whether the request is a proposal, plan, or an original order.", 0, 1, intent); 3168 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "An arbitrary categorization or grouping of the medication request. It could be used for indicating where meds are intended to be administered, eg. in an inpatient setting or in a patient's home, or a legal category of the medication.", 0, java.lang.Integer.MAX_VALUE, category); 3169 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, priority); 3170 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "If true, indicates that the provider is asking for the patient to either stop taking or to not start taking the specified medication. For example, the patient is taking an existing medication and the provider is changing their medication. They want to create two seperate requests: one to stop using the current medication and another to start the new medication.", 0, 1, doNotPerform); 3171 case 1998965455: /*medication*/ return new Property("medication", "CodeableReference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 3172 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The individual or group for whom the medication has been requested.", 0, 1, subject); 3173 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization who provided the information about this request, if the source is someone other than the requestor. This is often used when the MedicationRequest is reported by another person.", 0, java.lang.Integer.MAX_VALUE, informationSource); 3174 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this [x] was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 3175 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Information to support fulfilling (i.e. dispensing or administering) of the medication, for example, patient height and weight, a MedicationStatement for the patient).", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 3176 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn); 3177 case 693933948: /*requester*/ return new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The individual, organization, or device that initiated the request and has responsibility for its activation.", 0, 1, requester); 3178 case -427039533: /*reported*/ return new Property("reported", "boolean", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported); 3179 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "Indicates the type of performer of the administration of the medication.", 0, 1, performerType); 3180 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|Patient|DeviceDefinition|RelatedPerson|CareTeam|HealthcareService)", "The specified desired performer of the medication treatment (e.g. the performer of the medication administration). For devices, this is the device that is intended to perform the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.", 0, java.lang.Integer.MAX_VALUE, performer); 3181 case -1335157162: /*device*/ return new Property("device", "CodeableReference(DeviceDefinition)", "The intended type of device that is to be used for the administration of the medication (for example, PCA Pump).", 0, java.lang.Integer.MAX_VALUE, device); 3182 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Practitioner|PractitionerRole)", "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 0, 1, recorder); 3183 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation)", "The reason or the indication for ordering or not ordering the medication.", 0, java.lang.Integer.MAX_VALUE, reason); 3184 case -447282031: /*courseOfTherapyType*/ return new Property("courseOfTherapyType", "CodeableConcept", "The description of the overall pattern of the administration of the medication to the patient.", 0, 1, courseOfTherapyType); 3185 case 73049818: /*insurance*/ return new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance); 3186 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the prescription that could not be conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 3187 case 1718902050: /*renderedDosageInstruction*/ return new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction); 3188 case 322608453: /*effectiveDosePeriod*/ return new Property("effectiveDosePeriod", "Period", "The period over which the medication is to be taken. Where there are multiple dosageInstruction lines (for example, tapering doses), this is the earliest date and the latest end date of the dosageInstructions.", 0, 1, effectiveDosePeriod); 3189 case -1201373865: /*dosageInstruction*/ return new Property("dosageInstruction", "Dosage", "Specific instructions for how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction); 3190 case 824620658: /*dispenseRequest*/ return new Property("dispenseRequest", "", "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 0, 1, dispenseRequest); 3191 case 826147581: /*substitution*/ return new Property("substitution", "", "Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 0, 1, substitution); 3192 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 3193 default: return super.getNamedProperty(_hash, _name, _checkValid); 3194 } 3195 3196 } 3197 3198 @Override 3199 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3200 switch (hash) { 3201 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3202 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3203 case -486355964: /*priorPrescription*/ return this.priorPrescription == null ? new Base[0] : new Base[] {this.priorPrescription}; // Reference 3204 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 3205 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationrequestStatus> 3206 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 3207 case -1174686110: /*statusChanged*/ return this.statusChanged == null ? new Base[0] : new Base[] {this.statusChanged}; // DateTimeType 3208 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<MedicationRequestIntent> 3209 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3210 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 3211 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 3212 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // CodeableReference 3213 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3214 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : this.informationSource.toArray(new Base[this.informationSource.size()]); // Reference 3215 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3216 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 3217 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 3218 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 3219 case -427039533: /*reported*/ return this.reported == null ? new Base[0] : new Base[] {this.reported}; // BooleanType 3220 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 3221 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 3222 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // CodeableReference 3223 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 3224 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 3225 case -447282031: /*courseOfTherapyType*/ return this.courseOfTherapyType == null ? new Base[0] : new Base[] {this.courseOfTherapyType}; // CodeableConcept 3226 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 3227 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3228 case 1718902050: /*renderedDosageInstruction*/ return this.renderedDosageInstruction == null ? new Base[0] : new Base[] {this.renderedDosageInstruction}; // MarkdownType 3229 case 322608453: /*effectiveDosePeriod*/ return this.effectiveDosePeriod == null ? new Base[0] : new Base[] {this.effectiveDosePeriod}; // Period 3230 case -1201373865: /*dosageInstruction*/ return this.dosageInstruction == null ? new Base[0] : this.dosageInstruction.toArray(new Base[this.dosageInstruction.size()]); // Dosage 3231 case 824620658: /*dispenseRequest*/ return this.dispenseRequest == null ? new Base[0] : new Base[] {this.dispenseRequest}; // MedicationRequestDispenseRequestComponent 3232 case 826147581: /*substitution*/ return this.substitution == null ? new Base[0] : new Base[] {this.substitution}; // MedicationRequestSubstitutionComponent 3233 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 3234 default: return super.getProperty(hash, name, checkValid); 3235 } 3236 3237 } 3238 3239 @Override 3240 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3241 switch (hash) { 3242 case -1618432855: // identifier 3243 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3244 return value; 3245 case -332612366: // basedOn 3246 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 3247 return value; 3248 case -486355964: // priorPrescription 3249 this.priorPrescription = TypeConvertor.castToReference(value); // Reference 3250 return value; 3251 case -445338488: // groupIdentifier 3252 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 3253 return value; 3254 case -892481550: // status 3255 value = new MedicationrequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3256 this.status = (Enumeration) value; // Enumeration<MedicationrequestStatus> 3257 return value; 3258 case 2051346646: // statusReason 3259 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3260 return value; 3261 case -1174686110: // statusChanged 3262 this.statusChanged = TypeConvertor.castToDateTime(value); // DateTimeType 3263 return value; 3264 case -1183762788: // intent 3265 value = new MedicationRequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 3266 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 3267 return value; 3268 case 50511102: // category 3269 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3270 return value; 3271 case -1165461084: // priority 3272 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3273 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3274 return value; 3275 case -1788508167: // doNotPerform 3276 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 3277 return value; 3278 case 1998965455: // medication 3279 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 3280 return value; 3281 case -1867885268: // subject 3282 this.subject = TypeConvertor.castToReference(value); // Reference 3283 return value; 3284 case -2123220889: // informationSource 3285 this.getInformationSource().add(TypeConvertor.castToReference(value)); // Reference 3286 return value; 3287 case 1524132147: // encounter 3288 this.encounter = TypeConvertor.castToReference(value); // Reference 3289 return value; 3290 case -1248768647: // supportingInformation 3291 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 3292 return value; 3293 case -1500852503: // authoredOn 3294 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 3295 return value; 3296 case 693933948: // requester 3297 this.requester = TypeConvertor.castToReference(value); // Reference 3298 return value; 3299 case -427039533: // reported 3300 this.reported = TypeConvertor.castToBoolean(value); // BooleanType 3301 return value; 3302 case -901444568: // performerType 3303 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3304 return value; 3305 case 481140686: // performer 3306 this.getPerformer().add(TypeConvertor.castToReference(value)); // Reference 3307 return value; 3308 case -1335157162: // device 3309 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 3310 return value; 3311 case -799233858: // recorder 3312 this.recorder = TypeConvertor.castToReference(value); // Reference 3313 return value; 3314 case -934964668: // reason 3315 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 3316 return value; 3317 case -447282031: // courseOfTherapyType 3318 this.courseOfTherapyType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3319 return value; 3320 case 73049818: // insurance 3321 this.getInsurance().add(TypeConvertor.castToReference(value)); // Reference 3322 return value; 3323 case 3387378: // note 3324 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3325 return value; 3326 case 1718902050: // renderedDosageInstruction 3327 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 3328 return value; 3329 case 322608453: // effectiveDosePeriod 3330 this.effectiveDosePeriod = TypeConvertor.castToPeriod(value); // Period 3331 return value; 3332 case -1201373865: // dosageInstruction 3333 this.getDosageInstruction().add(TypeConvertor.castToDosage(value)); // Dosage 3334 return value; 3335 case 824620658: // dispenseRequest 3336 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 3337 return value; 3338 case 826147581: // substitution 3339 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 3340 return value; 3341 case 1835190426: // eventHistory 3342 this.getEventHistory().add(TypeConvertor.castToReference(value)); // Reference 3343 return value; 3344 default: return super.setProperty(hash, name, value); 3345 } 3346 3347 } 3348 3349 @Override 3350 public Base setProperty(String name, Base value) throws FHIRException { 3351 if (name.equals("identifier")) { 3352 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3353 } else if (name.equals("basedOn")) { 3354 this.getBasedOn().add(TypeConvertor.castToReference(value)); 3355 } else if (name.equals("priorPrescription")) { 3356 this.priorPrescription = TypeConvertor.castToReference(value); // Reference 3357 } else if (name.equals("groupIdentifier")) { 3358 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 3359 } else if (name.equals("status")) { 3360 value = new MedicationrequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3361 this.status = (Enumeration) value; // Enumeration<MedicationrequestStatus> 3362 } else if (name.equals("statusReason")) { 3363 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3364 } else if (name.equals("statusChanged")) { 3365 this.statusChanged = TypeConvertor.castToDateTime(value); // DateTimeType 3366 } else if (name.equals("intent")) { 3367 value = new MedicationRequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 3368 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 3369 } else if (name.equals("category")) { 3370 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3371 } else if (name.equals("priority")) { 3372 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3373 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3374 } else if (name.equals("doNotPerform")) { 3375 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 3376 } else if (name.equals("medication")) { 3377 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 3378 } else if (name.equals("subject")) { 3379 this.subject = TypeConvertor.castToReference(value); // Reference 3380 } else if (name.equals("informationSource")) { 3381 this.getInformationSource().add(TypeConvertor.castToReference(value)); 3382 } else if (name.equals("encounter")) { 3383 this.encounter = TypeConvertor.castToReference(value); // Reference 3384 } else if (name.equals("supportingInformation")) { 3385 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 3386 } else if (name.equals("authoredOn")) { 3387 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 3388 } else if (name.equals("requester")) { 3389 this.requester = TypeConvertor.castToReference(value); // Reference 3390 } else if (name.equals("reported")) { 3391 this.reported = TypeConvertor.castToBoolean(value); // BooleanType 3392 } else if (name.equals("performerType")) { 3393 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3394 } else if (name.equals("performer")) { 3395 this.getPerformer().add(TypeConvertor.castToReference(value)); 3396 } else if (name.equals("device")) { 3397 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); 3398 } else if (name.equals("recorder")) { 3399 this.recorder = TypeConvertor.castToReference(value); // Reference 3400 } else if (name.equals("reason")) { 3401 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 3402 } else if (name.equals("courseOfTherapyType")) { 3403 this.courseOfTherapyType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3404 } else if (name.equals("insurance")) { 3405 this.getInsurance().add(TypeConvertor.castToReference(value)); 3406 } else if (name.equals("note")) { 3407 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3408 } else if (name.equals("renderedDosageInstruction")) { 3409 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 3410 } else if (name.equals("effectiveDosePeriod")) { 3411 this.effectiveDosePeriod = TypeConvertor.castToPeriod(value); // Period 3412 } else if (name.equals("dosageInstruction")) { 3413 this.getDosageInstruction().add(TypeConvertor.castToDosage(value)); 3414 } else if (name.equals("dispenseRequest")) { 3415 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 3416 } else if (name.equals("substitution")) { 3417 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 3418 } else if (name.equals("eventHistory")) { 3419 this.getEventHistory().add(TypeConvertor.castToReference(value)); 3420 } else 3421 return super.setProperty(name, value); 3422 return value; 3423 } 3424 3425 @Override 3426 public void removeChild(String name, Base value) throws FHIRException { 3427 if (name.equals("identifier")) { 3428 this.getIdentifier().remove(value); 3429 } else if (name.equals("basedOn")) { 3430 this.getBasedOn().remove(value); 3431 } else if (name.equals("priorPrescription")) { 3432 this.priorPrescription = null; 3433 } else if (name.equals("groupIdentifier")) { 3434 this.groupIdentifier = null; 3435 } else if (name.equals("status")) { 3436 value = new MedicationrequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3437 this.status = (Enumeration) value; // Enumeration<MedicationrequestStatus> 3438 } else if (name.equals("statusReason")) { 3439 this.statusReason = null; 3440 } else if (name.equals("statusChanged")) { 3441 this.statusChanged = null; 3442 } else if (name.equals("intent")) { 3443 value = new MedicationRequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 3444 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 3445 } else if (name.equals("category")) { 3446 this.getCategory().remove(value); 3447 } else if (name.equals("priority")) { 3448 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3449 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3450 } else if (name.equals("doNotPerform")) { 3451 this.doNotPerform = null; 3452 } else if (name.equals("medication")) { 3453 this.medication = null; 3454 } else if (name.equals("subject")) { 3455 this.subject = null; 3456 } else if (name.equals("informationSource")) { 3457 this.getInformationSource().remove(value); 3458 } else if (name.equals("encounter")) { 3459 this.encounter = null; 3460 } else if (name.equals("supportingInformation")) { 3461 this.getSupportingInformation().remove(value); 3462 } else if (name.equals("authoredOn")) { 3463 this.authoredOn = null; 3464 } else if (name.equals("requester")) { 3465 this.requester = null; 3466 } else if (name.equals("reported")) { 3467 this.reported = null; 3468 } else if (name.equals("performerType")) { 3469 this.performerType = null; 3470 } else if (name.equals("performer")) { 3471 this.getPerformer().remove(value); 3472 } else if (name.equals("device")) { 3473 this.getDevice().remove(value); 3474 } else if (name.equals("recorder")) { 3475 this.recorder = null; 3476 } else if (name.equals("reason")) { 3477 this.getReason().remove(value); 3478 } else if (name.equals("courseOfTherapyType")) { 3479 this.courseOfTherapyType = null; 3480 } else if (name.equals("insurance")) { 3481 this.getInsurance().remove(value); 3482 } else if (name.equals("note")) { 3483 this.getNote().remove(value); 3484 } else if (name.equals("renderedDosageInstruction")) { 3485 this.renderedDosageInstruction = null; 3486 } else if (name.equals("effectiveDosePeriod")) { 3487 this.effectiveDosePeriod = null; 3488 } else if (name.equals("dosageInstruction")) { 3489 this.getDosageInstruction().remove(value); 3490 } else if (name.equals("dispenseRequest")) { 3491 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 3492 } else if (name.equals("substitution")) { 3493 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 3494 } else if (name.equals("eventHistory")) { 3495 this.getEventHistory().remove(value); 3496 } else 3497 super.removeChild(name, value); 3498 3499 } 3500 3501 @Override 3502 public Base makeProperty(int hash, String name) throws FHIRException { 3503 switch (hash) { 3504 case -1618432855: return addIdentifier(); 3505 case -332612366: return addBasedOn(); 3506 case -486355964: return getPriorPrescription(); 3507 case -445338488: return getGroupIdentifier(); 3508 case -892481550: return getStatusElement(); 3509 case 2051346646: return getStatusReason(); 3510 case -1174686110: return getStatusChangedElement(); 3511 case -1183762788: return getIntentElement(); 3512 case 50511102: return addCategory(); 3513 case -1165461084: return getPriorityElement(); 3514 case -1788508167: return getDoNotPerformElement(); 3515 case 1998965455: return getMedication(); 3516 case -1867885268: return getSubject(); 3517 case -2123220889: return addInformationSource(); 3518 case 1524132147: return getEncounter(); 3519 case -1248768647: return addSupportingInformation(); 3520 case -1500852503: return getAuthoredOnElement(); 3521 case 693933948: return getRequester(); 3522 case -427039533: return getReportedElement(); 3523 case -901444568: return getPerformerType(); 3524 case 481140686: return addPerformer(); 3525 case -1335157162: return addDevice(); 3526 case -799233858: return getRecorder(); 3527 case -934964668: return addReason(); 3528 case -447282031: return getCourseOfTherapyType(); 3529 case 73049818: return addInsurance(); 3530 case 3387378: return addNote(); 3531 case 1718902050: return getRenderedDosageInstructionElement(); 3532 case 322608453: return getEffectiveDosePeriod(); 3533 case -1201373865: return addDosageInstruction(); 3534 case 824620658: return getDispenseRequest(); 3535 case 826147581: return getSubstitution(); 3536 case 1835190426: return addEventHistory(); 3537 default: return super.makeProperty(hash, name); 3538 } 3539 3540 } 3541 3542 @Override 3543 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3544 switch (hash) { 3545 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3546 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3547 case -486355964: /*priorPrescription*/ return new String[] {"Reference"}; 3548 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 3549 case -892481550: /*status*/ return new String[] {"code"}; 3550 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 3551 case -1174686110: /*statusChanged*/ return new String[] {"dateTime"}; 3552 case -1183762788: /*intent*/ return new String[] {"code"}; 3553 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3554 case -1165461084: /*priority*/ return new String[] {"code"}; 3555 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 3556 case 1998965455: /*medication*/ return new String[] {"CodeableReference"}; 3557 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3558 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 3559 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3560 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 3561 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 3562 case 693933948: /*requester*/ return new String[] {"Reference"}; 3563 case -427039533: /*reported*/ return new String[] {"boolean"}; 3564 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 3565 case 481140686: /*performer*/ return new String[] {"Reference"}; 3566 case -1335157162: /*device*/ return new String[] {"CodeableReference"}; 3567 case -799233858: /*recorder*/ return new String[] {"Reference"}; 3568 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 3569 case -447282031: /*courseOfTherapyType*/ return new String[] {"CodeableConcept"}; 3570 case 73049818: /*insurance*/ return new String[] {"Reference"}; 3571 case 3387378: /*note*/ return new String[] {"Annotation"}; 3572 case 1718902050: /*renderedDosageInstruction*/ return new String[] {"markdown"}; 3573 case 322608453: /*effectiveDosePeriod*/ return new String[] {"Period"}; 3574 case -1201373865: /*dosageInstruction*/ return new String[] {"Dosage"}; 3575 case 824620658: /*dispenseRequest*/ return new String[] {}; 3576 case 826147581: /*substitution*/ return new String[] {}; 3577 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 3578 default: return super.getTypesForProperty(hash, name); 3579 } 3580 3581 } 3582 3583 @Override 3584 public Base addChild(String name) throws FHIRException { 3585 if (name.equals("identifier")) { 3586 return addIdentifier(); 3587 } 3588 else if (name.equals("basedOn")) { 3589 return addBasedOn(); 3590 } 3591 else if (name.equals("priorPrescription")) { 3592 this.priorPrescription = new Reference(); 3593 return this.priorPrescription; 3594 } 3595 else if (name.equals("groupIdentifier")) { 3596 this.groupIdentifier = new Identifier(); 3597 return this.groupIdentifier; 3598 } 3599 else if (name.equals("status")) { 3600 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.status"); 3601 } 3602 else if (name.equals("statusReason")) { 3603 this.statusReason = new CodeableConcept(); 3604 return this.statusReason; 3605 } 3606 else if (name.equals("statusChanged")) { 3607 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.statusChanged"); 3608 } 3609 else if (name.equals("intent")) { 3610 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.intent"); 3611 } 3612 else if (name.equals("category")) { 3613 return addCategory(); 3614 } 3615 else if (name.equals("priority")) { 3616 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.priority"); 3617 } 3618 else if (name.equals("doNotPerform")) { 3619 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.doNotPerform"); 3620 } 3621 else if (name.equals("medication")) { 3622 this.medication = new CodeableReference(); 3623 return this.medication; 3624 } 3625 else if (name.equals("subject")) { 3626 this.subject = new Reference(); 3627 return this.subject; 3628 } 3629 else if (name.equals("informationSource")) { 3630 return addInformationSource(); 3631 } 3632 else if (name.equals("encounter")) { 3633 this.encounter = new Reference(); 3634 return this.encounter; 3635 } 3636 else if (name.equals("supportingInformation")) { 3637 return addSupportingInformation(); 3638 } 3639 else if (name.equals("authoredOn")) { 3640 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.authoredOn"); 3641 } 3642 else if (name.equals("requester")) { 3643 this.requester = new Reference(); 3644 return this.requester; 3645 } 3646 else if (name.equals("reported")) { 3647 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.reported"); 3648 } 3649 else if (name.equals("performerType")) { 3650 this.performerType = new CodeableConcept(); 3651 return this.performerType; 3652 } 3653 else if (name.equals("performer")) { 3654 return addPerformer(); 3655 } 3656 else if (name.equals("device")) { 3657 return addDevice(); 3658 } 3659 else if (name.equals("recorder")) { 3660 this.recorder = new Reference(); 3661 return this.recorder; 3662 } 3663 else if (name.equals("reason")) { 3664 return addReason(); 3665 } 3666 else if (name.equals("courseOfTherapyType")) { 3667 this.courseOfTherapyType = new CodeableConcept(); 3668 return this.courseOfTherapyType; 3669 } 3670 else if (name.equals("insurance")) { 3671 return addInsurance(); 3672 } 3673 else if (name.equals("note")) { 3674 return addNote(); 3675 } 3676 else if (name.equals("renderedDosageInstruction")) { 3677 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.renderedDosageInstruction"); 3678 } 3679 else if (name.equals("effectiveDosePeriod")) { 3680 this.effectiveDosePeriod = new Period(); 3681 return this.effectiveDosePeriod; 3682 } 3683 else if (name.equals("dosageInstruction")) { 3684 return addDosageInstruction(); 3685 } 3686 else if (name.equals("dispenseRequest")) { 3687 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); 3688 return this.dispenseRequest; 3689 } 3690 else if (name.equals("substitution")) { 3691 this.substitution = new MedicationRequestSubstitutionComponent(); 3692 return this.substitution; 3693 } 3694 else if (name.equals("eventHistory")) { 3695 return addEventHistory(); 3696 } 3697 else 3698 return super.addChild(name); 3699 } 3700 3701 public String fhirType() { 3702 return "MedicationRequest"; 3703 3704 } 3705 3706 public MedicationRequest copy() { 3707 MedicationRequest dst = new MedicationRequest(); 3708 copyValues(dst); 3709 return dst; 3710 } 3711 3712 public void copyValues(MedicationRequest dst) { 3713 super.copyValues(dst); 3714 if (identifier != null) { 3715 dst.identifier = new ArrayList<Identifier>(); 3716 for (Identifier i : identifier) 3717 dst.identifier.add(i.copy()); 3718 }; 3719 if (basedOn != null) { 3720 dst.basedOn = new ArrayList<Reference>(); 3721 for (Reference i : basedOn) 3722 dst.basedOn.add(i.copy()); 3723 }; 3724 dst.priorPrescription = priorPrescription == null ? null : priorPrescription.copy(); 3725 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 3726 dst.status = status == null ? null : status.copy(); 3727 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3728 dst.statusChanged = statusChanged == null ? null : statusChanged.copy(); 3729 dst.intent = intent == null ? null : intent.copy(); 3730 if (category != null) { 3731 dst.category = new ArrayList<CodeableConcept>(); 3732 for (CodeableConcept i : category) 3733 dst.category.add(i.copy()); 3734 }; 3735 dst.priority = priority == null ? null : priority.copy(); 3736 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 3737 dst.medication = medication == null ? null : medication.copy(); 3738 dst.subject = subject == null ? null : subject.copy(); 3739 if (informationSource != null) { 3740 dst.informationSource = new ArrayList<Reference>(); 3741 for (Reference i : informationSource) 3742 dst.informationSource.add(i.copy()); 3743 }; 3744 dst.encounter = encounter == null ? null : encounter.copy(); 3745 if (supportingInformation != null) { 3746 dst.supportingInformation = new ArrayList<Reference>(); 3747 for (Reference i : supportingInformation) 3748 dst.supportingInformation.add(i.copy()); 3749 }; 3750 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 3751 dst.requester = requester == null ? null : requester.copy(); 3752 dst.reported = reported == null ? null : reported.copy(); 3753 dst.performerType = performerType == null ? null : performerType.copy(); 3754 if (performer != null) { 3755 dst.performer = new ArrayList<Reference>(); 3756 for (Reference i : performer) 3757 dst.performer.add(i.copy()); 3758 }; 3759 if (device != null) { 3760 dst.device = new ArrayList<CodeableReference>(); 3761 for (CodeableReference i : device) 3762 dst.device.add(i.copy()); 3763 }; 3764 dst.recorder = recorder == null ? null : recorder.copy(); 3765 if (reason != null) { 3766 dst.reason = new ArrayList<CodeableReference>(); 3767 for (CodeableReference i : reason) 3768 dst.reason.add(i.copy()); 3769 }; 3770 dst.courseOfTherapyType = courseOfTherapyType == null ? null : courseOfTherapyType.copy(); 3771 if (insurance != null) { 3772 dst.insurance = new ArrayList<Reference>(); 3773 for (Reference i : insurance) 3774 dst.insurance.add(i.copy()); 3775 }; 3776 if (note != null) { 3777 dst.note = new ArrayList<Annotation>(); 3778 for (Annotation i : note) 3779 dst.note.add(i.copy()); 3780 }; 3781 dst.renderedDosageInstruction = renderedDosageInstruction == null ? null : renderedDosageInstruction.copy(); 3782 dst.effectiveDosePeriod = effectiveDosePeriod == null ? null : effectiveDosePeriod.copy(); 3783 if (dosageInstruction != null) { 3784 dst.dosageInstruction = new ArrayList<Dosage>(); 3785 for (Dosage i : dosageInstruction) 3786 dst.dosageInstruction.add(i.copy()); 3787 }; 3788 dst.dispenseRequest = dispenseRequest == null ? null : dispenseRequest.copy(); 3789 dst.substitution = substitution == null ? null : substitution.copy(); 3790 if (eventHistory != null) { 3791 dst.eventHistory = new ArrayList<Reference>(); 3792 for (Reference i : eventHistory) 3793 dst.eventHistory.add(i.copy()); 3794 }; 3795 } 3796 3797 protected MedicationRequest typedCopy() { 3798 return copy(); 3799 } 3800 3801 @Override 3802 public boolean equalsDeep(Base other_) { 3803 if (!super.equalsDeep(other_)) 3804 return false; 3805 if (!(other_ instanceof MedicationRequest)) 3806 return false; 3807 MedicationRequest o = (MedicationRequest) other_; 3808 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(priorPrescription, o.priorPrescription, true) 3809 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(status, o.status, true) 3810 && compareDeep(statusReason, o.statusReason, true) && compareDeep(statusChanged, o.statusChanged, true) 3811 && compareDeep(intent, o.intent, true) && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 3812 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(medication, o.medication, true) 3813 && compareDeep(subject, o.subject, true) && compareDeep(informationSource, o.informationSource, true) 3814 && compareDeep(encounter, o.encounter, true) && compareDeep(supportingInformation, o.supportingInformation, true) 3815 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) && compareDeep(reported, o.reported, true) 3816 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 3817 && compareDeep(device, o.device, true) && compareDeep(recorder, o.recorder, true) && compareDeep(reason, o.reason, true) 3818 && compareDeep(courseOfTherapyType, o.courseOfTherapyType, true) && compareDeep(insurance, o.insurance, true) 3819 && compareDeep(note, o.note, true) && compareDeep(renderedDosageInstruction, o.renderedDosageInstruction, true) 3820 && compareDeep(effectiveDosePeriod, o.effectiveDosePeriod, true) && compareDeep(dosageInstruction, o.dosageInstruction, true) 3821 && compareDeep(dispenseRequest, o.dispenseRequest, true) && compareDeep(substitution, o.substitution, true) 3822 && compareDeep(eventHistory, o.eventHistory, true); 3823 } 3824 3825 @Override 3826 public boolean equalsShallow(Base other_) { 3827 if (!super.equalsShallow(other_)) 3828 return false; 3829 if (!(other_ instanceof MedicationRequest)) 3830 return false; 3831 MedicationRequest o = (MedicationRequest) other_; 3832 return compareValues(status, o.status, true) && compareValues(statusChanged, o.statusChanged, true) 3833 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) && compareValues(doNotPerform, o.doNotPerform, true) 3834 && compareValues(authoredOn, o.authoredOn, true) && compareValues(reported, o.reported, true) && compareValues(renderedDosageInstruction, o.renderedDosageInstruction, true) 3835 ; 3836 } 3837 3838 public boolean isEmpty() { 3839 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, priorPrescription 3840 , groupIdentifier, status, statusReason, statusChanged, intent, category, priority 3841 , doNotPerform, medication, subject, informationSource, encounter, supportingInformation 3842 , authoredOn, requester, reported, performerType, performer, device, recorder 3843 , reason, courseOfTherapyType, insurance, note, renderedDosageInstruction, effectiveDosePeriod 3844 , dosageInstruction, dispenseRequest, substitution, eventHistory); 3845 } 3846 3847 @Override 3848 public ResourceType getResourceType() { 3849 return ResourceType.MedicationRequest; 3850 } 3851 3852 /** 3853 * Search parameter: <b>authoredon</b> 3854 * <p> 3855 * Description: <b>Return prescriptions written on this date</b><br> 3856 * Type: <b>date</b><br> 3857 * Path: <b>MedicationRequest.authoredOn</b><br> 3858 * </p> 3859 */ 3860 @SearchParamDefinition(name="authoredon", path="MedicationRequest.authoredOn", description="Return prescriptions written on this date", type="date" ) 3861 public static final String SP_AUTHOREDON = "authoredon"; 3862 /** 3863 * <b>Fluent Client</b> search parameter constant for <b>authoredon</b> 3864 * <p> 3865 * Description: <b>Return prescriptions written on this date</b><br> 3866 * Type: <b>date</b><br> 3867 * Path: <b>MedicationRequest.authoredOn</b><br> 3868 * </p> 3869 */ 3870 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHOREDON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHOREDON); 3871 3872 /** 3873 * Search parameter: <b>category</b> 3874 * <p> 3875 * Description: <b>Returns prescriptions with different categories</b><br> 3876 * Type: <b>token</b><br> 3877 * Path: <b>MedicationRequest.category</b><br> 3878 * </p> 3879 */ 3880 @SearchParamDefinition(name="category", path="MedicationRequest.category", description="Returns prescriptions with different categories", type="token" ) 3881 public static final String SP_CATEGORY = "category"; 3882 /** 3883 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3884 * <p> 3885 * Description: <b>Returns prescriptions with different categories</b><br> 3886 * Type: <b>token</b><br> 3887 * Path: <b>MedicationRequest.category</b><br> 3888 * </p> 3889 */ 3890 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3891 3892 /** 3893 * Search parameter: <b>combo-date</b> 3894 * <p> 3895 * Description: <b>Returns medication request to be administered on a specific date or within a date range</b><br> 3896 * Type: <b>date</b><br> 3897 * Path: <b>MedicationRequest.dosageInstruction.timing.event | (MedicationRequest.dosageInstruction.timing.repeat.bounds.ofType(Period))</b><br> 3898 * </p> 3899 */ 3900 @SearchParamDefinition(name="combo-date", path="MedicationRequest.dosageInstruction.timing.event | (MedicationRequest.dosageInstruction.timing.repeat.bounds.ofType(Period))", description="Returns medication request to be administered on a specific date or within a date range", type="date" ) 3901 public static final String SP_COMBO_DATE = "combo-date"; 3902 /** 3903 * <b>Fluent Client</b> search parameter constant for <b>combo-date</b> 3904 * <p> 3905 * Description: <b>Returns medication request to be administered on a specific date or within a date range</b><br> 3906 * Type: <b>date</b><br> 3907 * Path: <b>MedicationRequest.dosageInstruction.timing.event | (MedicationRequest.dosageInstruction.timing.repeat.bounds.ofType(Period))</b><br> 3908 * </p> 3909 */ 3910 public static final ca.uhn.fhir.rest.gclient.DateClientParam COMBO_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_COMBO_DATE); 3911 3912 /** 3913 * Search parameter: <b>group-identifier</b> 3914 * <p> 3915 * Description: <b>Composite request this is part of</b><br> 3916 * Type: <b>token</b><br> 3917 * Path: <b>MedicationRequest.groupIdentifier</b><br> 3918 * </p> 3919 */ 3920 @SearchParamDefinition(name="group-identifier", path="MedicationRequest.groupIdentifier", description="Composite request this is part of", type="token" ) 3921 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 3922 /** 3923 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 3924 * <p> 3925 * Description: <b>Composite request this is part of</b><br> 3926 * Type: <b>token</b><br> 3927 * Path: <b>MedicationRequest.groupIdentifier</b><br> 3928 * </p> 3929 */ 3930 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 3931 3932 /** 3933 * Search parameter: <b>intended-dispenser</b> 3934 * <p> 3935 * Description: <b>Returns prescriptions intended to be dispensed by this Organization</b><br> 3936 * Type: <b>reference</b><br> 3937 * Path: <b>MedicationRequest.dispenseRequest.dispenser</b><br> 3938 * </p> 3939 */ 3940 @SearchParamDefinition(name="intended-dispenser", path="MedicationRequest.dispenseRequest.dispenser", description="Returns prescriptions intended to be dispensed by this Organization", type="reference", target={Organization.class } ) 3941 public static final String SP_INTENDED_DISPENSER = "intended-dispenser"; 3942 /** 3943 * <b>Fluent Client</b> search parameter constant for <b>intended-dispenser</b> 3944 * <p> 3945 * Description: <b>Returns prescriptions intended to be dispensed by this Organization</b><br> 3946 * Type: <b>reference</b><br> 3947 * Path: <b>MedicationRequest.dispenseRequest.dispenser</b><br> 3948 * </p> 3949 */ 3950 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTENDED_DISPENSER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INTENDED_DISPENSER); 3951 3952/** 3953 * Constant for fluent queries to be used to add include statements. Specifies 3954 * the path value of "<b>MedicationRequest:intended-dispenser</b>". 3955 */ 3956 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTENDED_DISPENSER = new ca.uhn.fhir.model.api.Include("MedicationRequest:intended-dispenser").toLocked(); 3957 3958 /** 3959 * Search parameter: <b>intended-performer</b> 3960 * <p> 3961 * Description: <b>Returns the intended performer of the administration of the medication request</b><br> 3962 * Type: <b>reference</b><br> 3963 * Path: <b>MedicationRequest.performer</b><br> 3964 * </p> 3965 */ 3966 @SearchParamDefinition(name="intended-performer", path="MedicationRequest.performer", description="Returns the intended performer of the administration of the medication request", type="reference", target={CareTeam.class, DeviceDefinition.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3967 public static final String SP_INTENDED_PERFORMER = "intended-performer"; 3968 /** 3969 * <b>Fluent Client</b> search parameter constant for <b>intended-performer</b> 3970 * <p> 3971 * Description: <b>Returns the intended performer of the administration of the medication request</b><br> 3972 * Type: <b>reference</b><br> 3973 * Path: <b>MedicationRequest.performer</b><br> 3974 * </p> 3975 */ 3976 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTENDED_PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INTENDED_PERFORMER); 3977 3978/** 3979 * Constant for fluent queries to be used to add include statements. Specifies 3980 * the path value of "<b>MedicationRequest:intended-performer</b>". 3981 */ 3982 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTENDED_PERFORMER = new ca.uhn.fhir.model.api.Include("MedicationRequest:intended-performer").toLocked(); 3983 3984 /** 3985 * Search parameter: <b>intended-performertype</b> 3986 * <p> 3987 * Description: <b>Returns requests for a specific type of performer</b><br> 3988 * Type: <b>token</b><br> 3989 * Path: <b>MedicationRequest.performerType</b><br> 3990 * </p> 3991 */ 3992 @SearchParamDefinition(name="intended-performertype", path="MedicationRequest.performerType", description="Returns requests for a specific type of performer", type="token" ) 3993 public static final String SP_INTENDED_PERFORMERTYPE = "intended-performertype"; 3994 /** 3995 * <b>Fluent Client</b> search parameter constant for <b>intended-performertype</b> 3996 * <p> 3997 * Description: <b>Returns requests for a specific type of performer</b><br> 3998 * Type: <b>token</b><br> 3999 * Path: <b>MedicationRequest.performerType</b><br> 4000 * </p> 4001 */ 4002 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENDED_PERFORMERTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENDED_PERFORMERTYPE); 4003 4004 /** 4005 * Search parameter: <b>intent</b> 4006 * <p> 4007 * Description: <b>Returns prescriptions with different intents</b><br> 4008 * Type: <b>token</b><br> 4009 * Path: <b>MedicationRequest.intent</b><br> 4010 * </p> 4011 */ 4012 @SearchParamDefinition(name="intent", path="MedicationRequest.intent", description="Returns prescriptions with different intents", type="token" ) 4013 public static final String SP_INTENT = "intent"; 4014 /** 4015 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 4016 * <p> 4017 * Description: <b>Returns prescriptions with different intents</b><br> 4018 * Type: <b>token</b><br> 4019 * Path: <b>MedicationRequest.intent</b><br> 4020 * </p> 4021 */ 4022 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 4023 4024 /** 4025 * Search parameter: <b>priority</b> 4026 * <p> 4027 * Description: <b>Returns prescriptions with different priorities</b><br> 4028 * Type: <b>token</b><br> 4029 * Path: <b>MedicationRequest.priority</b><br> 4030 * </p> 4031 */ 4032 @SearchParamDefinition(name="priority", path="MedicationRequest.priority", description="Returns prescriptions with different priorities", type="token" ) 4033 public static final String SP_PRIORITY = "priority"; 4034 /** 4035 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 4036 * <p> 4037 * Description: <b>Returns prescriptions with different priorities</b><br> 4038 * Type: <b>token</b><br> 4039 * Path: <b>MedicationRequest.priority</b><br> 4040 * </p> 4041 */ 4042 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 4043 4044 /** 4045 * Search parameter: <b>requester</b> 4046 * <p> 4047 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 4048 * Type: <b>reference</b><br> 4049 * Path: <b>MedicationRequest.requester</b><br> 4050 * </p> 4051 */ 4052 @SearchParamDefinition(name="requester", path="MedicationRequest.requester", description="Returns prescriptions prescribed by this prescriber", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 4053 public static final String SP_REQUESTER = "requester"; 4054 /** 4055 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 4056 * <p> 4057 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 4058 * Type: <b>reference</b><br> 4059 * Path: <b>MedicationRequest.requester</b><br> 4060 * </p> 4061 */ 4062 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 4063 4064/** 4065 * Constant for fluent queries to be used to add include statements. Specifies 4066 * the path value of "<b>MedicationRequest:requester</b>". 4067 */ 4068 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("MedicationRequest:requester").toLocked(); 4069 4070 /** 4071 * Search parameter: <b>subject</b> 4072 * <p> 4073 * Description: <b>The identity of a patient to list orders for</b><br> 4074 * Type: <b>reference</b><br> 4075 * Path: <b>MedicationRequest.subject</b><br> 4076 * </p> 4077 */ 4078 @SearchParamDefinition(name="subject", path="MedicationRequest.subject", description="The identity of a patient to list orders for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 4079 public static final String SP_SUBJECT = "subject"; 4080 /** 4081 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4082 * <p> 4083 * Description: <b>The identity of a patient to list orders for</b><br> 4084 * Type: <b>reference</b><br> 4085 * Path: <b>MedicationRequest.subject</b><br> 4086 * </p> 4087 */ 4088 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4089 4090/** 4091 * Constant for fluent queries to be used to add include statements. Specifies 4092 * the path value of "<b>MedicationRequest:subject</b>". 4093 */ 4094 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationRequest:subject").toLocked(); 4095 4096 /** 4097 * Search parameter: <b>code</b> 4098 * <p> 4099 * Description: <b>Multiple Resources: 4100 4101* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4102* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4103* [AuditEvent](auditevent.html): More specific code for the event 4104* [Basic](basic.html): Kind of Resource 4105* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4106* [Condition](condition.html): Code for the condition 4107* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4108* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4109* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4110* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4111* [ImagingSelection](imagingselection.html): The imaging selection status 4112* [List](list.html): What the purpose of this list is 4113* [Medication](medication.html): Returns medications for a specific code 4114* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4115* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4116* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4117* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4118* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4119* [Observation](observation.html): The code of the observation type 4120* [Procedure](procedure.html): A code to identify a procedure 4121* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4122* [Task](task.html): Search by task code 4123</b><br> 4124 * Type: <b>token</b><br> 4125 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4126 * </p> 4127 */ 4128 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 4129 public static final String SP_CODE = "code"; 4130 /** 4131 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4132 * <p> 4133 * Description: <b>Multiple Resources: 4134 4135* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4136* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4137* [AuditEvent](auditevent.html): More specific code for the event 4138* [Basic](basic.html): Kind of Resource 4139* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4140* [Condition](condition.html): Code for the condition 4141* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4142* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4143* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4144* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4145* [ImagingSelection](imagingselection.html): The imaging selection status 4146* [List](list.html): What the purpose of this list is 4147* [Medication](medication.html): Returns medications for a specific code 4148* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4149* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4150* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4151* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4152* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4153* [Observation](observation.html): The code of the observation type 4154* [Procedure](procedure.html): A code to identify a procedure 4155* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4156* [Task](task.html): Search by task code 4157</b><br> 4158 * Type: <b>token</b><br> 4159 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4160 * </p> 4161 */ 4162 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4163 4164 /** 4165 * Search parameter: <b>identifier</b> 4166 * <p> 4167 * Description: <b>Multiple Resources: 4168 4169* [Account](account.html): Account number 4170* [AdverseEvent](adverseevent.html): Business identifier for the event 4171* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4172* [Appointment](appointment.html): An Identifier of the Appointment 4173* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4174* [Basic](basic.html): Business identifier 4175* [BodyStructure](bodystructure.html): Bodystructure identifier 4176* [CarePlan](careplan.html): External Ids for this plan 4177* [CareTeam](careteam.html): External Ids for this team 4178* [ChargeItem](chargeitem.html): Business Identifier for item 4179* [Claim](claim.html): The primary identifier of the financial resource 4180* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4181* [ClinicalImpression](clinicalimpression.html): Business identifier 4182* [Communication](communication.html): Unique identifier 4183* [CommunicationRequest](communicationrequest.html): Unique identifier 4184* [Composition](composition.html): Version-independent identifier for the Composition 4185* [Condition](condition.html): A unique identifier of the condition record 4186* [Consent](consent.html): Identifier for this record (external references) 4187* [Contract](contract.html): The identity of the contract 4188* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4189* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4190* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4191* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4192* [DeviceRequest](devicerequest.html): Business identifier for request/order 4193* [DeviceUsage](deviceusage.html): Search by identifier 4194* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4195* [DocumentReference](documentreference.html): Identifier of the attachment binary 4196* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4197* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4198* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4199* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4200* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4201* [Flag](flag.html): Business identifier 4202* [Goal](goal.html): External Ids for this goal 4203* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4204* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4205* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4206* [Immunization](immunization.html): Business identifier 4207* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4208* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4209* [Invoice](invoice.html): Business Identifier for item 4210* [List](list.html): Business identifier 4211* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4212* [Medication](medication.html): Returns medications with this external identifier 4213* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4214* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4215* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4216* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4217* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4218* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4219* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4220* [Observation](observation.html): The unique id for a particular observation 4221* [Person](person.html): A person Identifier 4222* [Procedure](procedure.html): A unique identifier for a procedure 4223* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4224* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4225* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4226* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4227* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4228* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4229* [Specimen](specimen.html): The unique identifier associated with the specimen 4230* [SupplyDelivery](supplydelivery.html): External identifier 4231* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4232* [Task](task.html): Search for a task instance by its business identifier 4233* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4234</b><br> 4235 * Type: <b>token</b><br> 4236 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4237 * </p> 4238 */ 4239 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4240 public static final String SP_IDENTIFIER = "identifier"; 4241 /** 4242 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4243 * <p> 4244 * Description: <b>Multiple Resources: 4245 4246* [Account](account.html): Account number 4247* [AdverseEvent](adverseevent.html): Business identifier for the event 4248* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4249* [Appointment](appointment.html): An Identifier of the Appointment 4250* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4251* [Basic](basic.html): Business identifier 4252* [BodyStructure](bodystructure.html): Bodystructure identifier 4253* [CarePlan](careplan.html): External Ids for this plan 4254* [CareTeam](careteam.html): External Ids for this team 4255* [ChargeItem](chargeitem.html): Business Identifier for item 4256* [Claim](claim.html): The primary identifier of the financial resource 4257* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4258* [ClinicalImpression](clinicalimpression.html): Business identifier 4259* [Communication](communication.html): Unique identifier 4260* [CommunicationRequest](communicationrequest.html): Unique identifier 4261* [Composition](composition.html): Version-independent identifier for the Composition 4262* [Condition](condition.html): A unique identifier of the condition record 4263* [Consent](consent.html): Identifier for this record (external references) 4264* [Contract](contract.html): The identity of the contract 4265* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4266* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4267* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4268* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4269* [DeviceRequest](devicerequest.html): Business identifier for request/order 4270* [DeviceUsage](deviceusage.html): Search by identifier 4271* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4272* [DocumentReference](documentreference.html): Identifier of the attachment binary 4273* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4274* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4275* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4276* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4277* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4278* [Flag](flag.html): Business identifier 4279* [Goal](goal.html): External Ids for this goal 4280* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4281* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4282* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4283* [Immunization](immunization.html): Business identifier 4284* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4285* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4286* [Invoice](invoice.html): Business Identifier for item 4287* [List](list.html): Business identifier 4288* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4289* [Medication](medication.html): Returns medications with this external identifier 4290* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4291* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4292* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4293* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4294* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4295* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4296* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4297* [Observation](observation.html): The unique id for a particular observation 4298* [Person](person.html): A person Identifier 4299* [Procedure](procedure.html): A unique identifier for a procedure 4300* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4301* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4302* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4303* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4304* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4305* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4306* [Specimen](specimen.html): The unique identifier associated with the specimen 4307* [SupplyDelivery](supplydelivery.html): External identifier 4308* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4309* [Task](task.html): Search for a task instance by its business identifier 4310* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4311</b><br> 4312 * Type: <b>token</b><br> 4313 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4314 * </p> 4315 */ 4316 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4317 4318 /** 4319 * Search parameter: <b>patient</b> 4320 * <p> 4321 * Description: <b>Multiple Resources: 4322 4323* [Account](account.html): The entity that caused the expenses 4324* [AdverseEvent](adverseevent.html): Subject impacted by event 4325* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4326* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4327* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4328* [AuditEvent](auditevent.html): Where the activity involved patient data 4329* [Basic](basic.html): Identifies the focus of this resource 4330* [BodyStructure](bodystructure.html): Who this is about 4331* [CarePlan](careplan.html): Who the care plan is for 4332* [CareTeam](careteam.html): Who care team is for 4333* [ChargeItem](chargeitem.html): Individual service was done for/to 4334* [Claim](claim.html): Patient receiving the products or services 4335* [ClaimResponse](claimresponse.html): The subject of care 4336* [ClinicalImpression](clinicalimpression.html): Patient assessed 4337* [Communication](communication.html): Focus of message 4338* [CommunicationRequest](communicationrequest.html): Focus of message 4339* [Composition](composition.html): Who and/or what the composition is about 4340* [Condition](condition.html): Who has the condition? 4341* [Consent](consent.html): Who the consent applies to 4342* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4343* [Coverage](coverage.html): Retrieve coverages for a patient 4344* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4345* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4346* [DetectedIssue](detectedissue.html): Associated patient 4347* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4348* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4349* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4350* [DocumentReference](documentreference.html): Who/what is the subject of the document 4351* [Encounter](encounter.html): The patient present at the encounter 4352* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4353* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4354* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4355* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4356* [Flag](flag.html): The identity of a subject to list flags for 4357* [Goal](goal.html): Who this goal is intended for 4358* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4359* [ImagingSelection](imagingselection.html): Who the study is about 4360* [ImagingStudy](imagingstudy.html): Who the study is about 4361* [Immunization](immunization.html): The patient for the vaccination record 4362* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4363* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4364* [Invoice](invoice.html): Recipient(s) of goods and services 4365* [List](list.html): If all resources have the same subject 4366* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4367* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4368* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4369* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4370* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4371* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4372* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4373* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4374* [Observation](observation.html): The subject that the observation is about (if patient) 4375* [Person](person.html): The Person links to this Patient 4376* [Procedure](procedure.html): Search by subject - a patient 4377* [Provenance](provenance.html): Where the activity involved patient data 4378* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4379* [RelatedPerson](relatedperson.html): The patient this related person is related to 4380* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4381* [ResearchSubject](researchsubject.html): Who or what is part of study 4382* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4383* [ServiceRequest](servicerequest.html): Search by subject - a patient 4384* [Specimen](specimen.html): The patient the specimen comes from 4385* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4386* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4387* [Task](task.html): Search by patient 4388* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4389</b><br> 4390 * Type: <b>reference</b><br> 4391 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4392 * </p> 4393 */ 4394 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4395 public static final String SP_PATIENT = "patient"; 4396 /** 4397 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4398 * <p> 4399 * Description: <b>Multiple Resources: 4400 4401* [Account](account.html): The entity that caused the expenses 4402* [AdverseEvent](adverseevent.html): Subject impacted by event 4403* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4404* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4405* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4406* [AuditEvent](auditevent.html): Where the activity involved patient data 4407* [Basic](basic.html): Identifies the focus of this resource 4408* [BodyStructure](bodystructure.html): Who this is about 4409* [CarePlan](careplan.html): Who the care plan is for 4410* [CareTeam](careteam.html): Who care team is for 4411* [ChargeItem](chargeitem.html): Individual service was done for/to 4412* [Claim](claim.html): Patient receiving the products or services 4413* [ClaimResponse](claimresponse.html): The subject of care 4414* [ClinicalImpression](clinicalimpression.html): Patient assessed 4415* [Communication](communication.html): Focus of message 4416* [CommunicationRequest](communicationrequest.html): Focus of message 4417* [Composition](composition.html): Who and/or what the composition is about 4418* [Condition](condition.html): Who has the condition? 4419* [Consent](consent.html): Who the consent applies to 4420* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4421* [Coverage](coverage.html): Retrieve coverages for a patient 4422* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4423* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4424* [DetectedIssue](detectedissue.html): Associated patient 4425* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4426* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4427* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4428* [DocumentReference](documentreference.html): Who/what is the subject of the document 4429* [Encounter](encounter.html): The patient present at the encounter 4430* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4431* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4432* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4433* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4434* [Flag](flag.html): The identity of a subject to list flags for 4435* [Goal](goal.html): Who this goal is intended for 4436* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4437* [ImagingSelection](imagingselection.html): Who the study is about 4438* [ImagingStudy](imagingstudy.html): Who the study is about 4439* [Immunization](immunization.html): The patient for the vaccination record 4440* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4441* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4442* [Invoice](invoice.html): Recipient(s) of goods and services 4443* [List](list.html): If all resources have the same subject 4444* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4445* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4446* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4447* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4448* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4449* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4450* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4451* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4452* [Observation](observation.html): The subject that the observation is about (if patient) 4453* [Person](person.html): The Person links to this Patient 4454* [Procedure](procedure.html): Search by subject - a patient 4455* [Provenance](provenance.html): Where the activity involved patient data 4456* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4457* [RelatedPerson](relatedperson.html): The patient this related person is related to 4458* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4459* [ResearchSubject](researchsubject.html): Who or what is part of study 4460* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4461* [ServiceRequest](servicerequest.html): Search by subject - a patient 4462* [Specimen](specimen.html): The patient the specimen comes from 4463* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4464* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4465* [Task](task.html): Search by patient 4466* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4467</b><br> 4468 * Type: <b>reference</b><br> 4469 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4470 * </p> 4471 */ 4472 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4473 4474/** 4475 * Constant for fluent queries to be used to add include statements. Specifies 4476 * the path value of "<b>MedicationRequest:patient</b>". 4477 */ 4478 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationRequest:patient").toLocked(); 4479 4480 /** 4481 * Search parameter: <b>encounter</b> 4482 * <p> 4483 * Description: <b>Multiple Resources: 4484 4485* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter 4486* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier 4487</b><br> 4488 * Type: <b>reference</b><br> 4489 * Path: <b>MedicationAdministration.encounter | MedicationRequest.encounter</b><br> 4490 * </p> 4491 */ 4492 @SearchParamDefinition(name="encounter", path="MedicationAdministration.encounter | MedicationRequest.encounter", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 4493 public static final String SP_ENCOUNTER = "encounter"; 4494 /** 4495 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4496 * <p> 4497 * Description: <b>Multiple Resources: 4498 4499* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter 4500* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier 4501</b><br> 4502 * Type: <b>reference</b><br> 4503 * Path: <b>MedicationAdministration.encounter | MedicationRequest.encounter</b><br> 4504 * </p> 4505 */ 4506 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4507 4508/** 4509 * Constant for fluent queries to be used to add include statements. Specifies 4510 * the path value of "<b>MedicationRequest:encounter</b>". 4511 */ 4512 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("MedicationRequest:encounter").toLocked(); 4513 4514 /** 4515 * Search parameter: <b>medication</b> 4516 * <p> 4517 * Description: <b>Multiple Resources: 4518 4519* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 4520* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 4521* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 4522* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 4523</b><br> 4524 * Type: <b>reference</b><br> 4525 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 4526 * </p> 4527 */ 4528 @SearchParamDefinition(name="medication", path="MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication reference\r\n", type="reference", target={Medication.class } ) 4529 public static final String SP_MEDICATION = "medication"; 4530 /** 4531 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 4532 * <p> 4533 * Description: <b>Multiple Resources: 4534 4535* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 4536* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 4537* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 4538* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 4539</b><br> 4540 * Type: <b>reference</b><br> 4541 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 4542 * </p> 4543 */ 4544 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 4545 4546/** 4547 * Constant for fluent queries to be used to add include statements. Specifies 4548 * the path value of "<b>MedicationRequest:medication</b>". 4549 */ 4550 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationRequest:medication").toLocked(); 4551 4552 /** 4553 * Search parameter: <b>status</b> 4554 * <p> 4555 * Description: <b>Multiple Resources: 4556 4557* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 4558* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 4559* [MedicationRequest](medicationrequest.html): Status of the prescription 4560* [MedicationStatement](medicationstatement.html): Return statements that match the given status 4561</b><br> 4562 * Type: <b>token</b><br> 4563 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 4564 * </p> 4565 */ 4566 @SearchParamDefinition(name="status", path="MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified)\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status\r\n* [MedicationRequest](medicationrequest.html): Status of the prescription\r\n* [MedicationStatement](medicationstatement.html): Return statements that match the given status\r\n", type="token" ) 4567 public static final String SP_STATUS = "status"; 4568 /** 4569 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4570 * <p> 4571 * Description: <b>Multiple Resources: 4572 4573* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 4574* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 4575* [MedicationRequest](medicationrequest.html): Status of the prescription 4576* [MedicationStatement](medicationstatement.html): Return statements that match the given status 4577</b><br> 4578 * Type: <b>token</b><br> 4579 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 4580 * </p> 4581 */ 4582 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4583 4584 4585} 4586