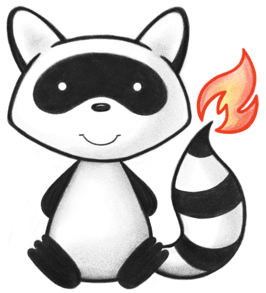
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. 052 053The primary difference between a medicationstatement and a medicationadministration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medicationstatement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the Medication Statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 054 */ 055@ResourceDef(name="MedicationStatement", profile="http://hl7.org/fhir/StructureDefinition/MedicationStatement") 056public class MedicationStatement extends DomainResource { 057 058 public enum MedicationStatementStatusCodes { 059 /** 060 * The action of recording the medication statement is finished. 061 */ 062 RECORDED, 063 /** 064 * Some of the actions that are implied by the medication usage may have occurred. For example, the patient may have taken some of the medication. Clinical decision support systems should take this status into account. 065 */ 066 ENTEREDINERROR, 067 /** 068 * The medication usage is draft or preliminary. 069 */ 070 DRAFT, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static MedicationStatementStatusCodes fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("recorded".equals(codeString)) 079 return RECORDED; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if ("draft".equals(codeString)) 083 return DRAFT; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown MedicationStatementStatusCodes code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case RECORDED: return "recorded"; 092 case ENTEREDINERROR: return "entered-in-error"; 093 case DRAFT: return "draft"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case RECORDED: return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 101 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 102 case DRAFT: return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case RECORDED: return "The action of recording the medication statement is finished."; 110 case ENTEREDINERROR: return "Some of the actions that are implied by the medication usage may have occurred. For example, the patient may have taken some of the medication. Clinical decision support systems should take this status into account."; 111 case DRAFT: return "The medication usage is draft or preliminary."; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDisplay() { 117 switch (this) { 118 case RECORDED: return "Recorded"; 119 case ENTEREDINERROR: return "Entered in Error"; 120 case DRAFT: return "Draft"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 } 126 127 public static class MedicationStatementStatusCodesEnumFactory implements EnumFactory<MedicationStatementStatusCodes> { 128 public MedicationStatementStatusCodes fromCode(String codeString) throws IllegalArgumentException { 129 if (codeString == null || "".equals(codeString)) 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("recorded".equals(codeString)) 133 return MedicationStatementStatusCodes.RECORDED; 134 if ("entered-in-error".equals(codeString)) 135 return MedicationStatementStatusCodes.ENTEREDINERROR; 136 if ("draft".equals(codeString)) 137 return MedicationStatementStatusCodes.DRAFT; 138 throw new IllegalArgumentException("Unknown MedicationStatementStatusCodes code '"+codeString+"'"); 139 } 140 public Enumeration<MedicationStatementStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 141 if (code == null) 142 return null; 143 if (code.isEmpty()) 144 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.NULL, code); 145 String codeString = ((PrimitiveType) code).asStringValue(); 146 if (codeString == null || "".equals(codeString)) 147 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.NULL, code); 148 if ("recorded".equals(codeString)) 149 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.RECORDED, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.ENTEREDINERROR, code); 152 if ("draft".equals(codeString)) 153 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.DRAFT, code); 154 throw new FHIRException("Unknown MedicationStatementStatusCodes code '"+codeString+"'"); 155 } 156 public String toCode(MedicationStatementStatusCodes code) { 157 if (code == MedicationStatementStatusCodes.RECORDED) 158 return "recorded"; 159 if (code == MedicationStatementStatusCodes.ENTEREDINERROR) 160 return "entered-in-error"; 161 if (code == MedicationStatementStatusCodes.DRAFT) 162 return "draft"; 163 return "?"; 164 } 165 public String toSystem(MedicationStatementStatusCodes code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class MedicationStatementAdherenceComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * Type of the adherence for the medication. 174 */ 175 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="Type of adherence", formalDefinition="Type of the adherence for the medication." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-adherence") 178 protected CodeableConcept code; 179 180 /** 181 * Captures the reason for the current use or adherence of a medication. 182 */ 183 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 184 @Description(shortDefinition="Details of the reason for the current use of the medication", formalDefinition="Captures the reason for the current use or adherence of a medication." ) 185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-status-codes") 186 protected CodeableConcept reason; 187 188 private static final long serialVersionUID = -1479626679L; 189 190 /** 191 * Constructor 192 */ 193 public MedicationStatementAdherenceComponent() { 194 super(); 195 } 196 197 /** 198 * Constructor 199 */ 200 public MedicationStatementAdherenceComponent(CodeableConcept code) { 201 super(); 202 this.setCode(code); 203 } 204 205 /** 206 * @return {@link #code} (Type of the adherence for the medication.) 207 */ 208 public CodeableConcept getCode() { 209 if (this.code == null) 210 if (Configuration.errorOnAutoCreate()) 211 throw new Error("Attempt to auto-create MedicationStatementAdherenceComponent.code"); 212 else if (Configuration.doAutoCreate()) 213 this.code = new CodeableConcept(); // cc 214 return this.code; 215 } 216 217 public boolean hasCode() { 218 return this.code != null && !this.code.isEmpty(); 219 } 220 221 /** 222 * @param value {@link #code} (Type of the adherence for the medication.) 223 */ 224 public MedicationStatementAdherenceComponent setCode(CodeableConcept value) { 225 this.code = value; 226 return this; 227 } 228 229 /** 230 * @return {@link #reason} (Captures the reason for the current use or adherence of a medication.) 231 */ 232 public CodeableConcept getReason() { 233 if (this.reason == null) 234 if (Configuration.errorOnAutoCreate()) 235 throw new Error("Attempt to auto-create MedicationStatementAdherenceComponent.reason"); 236 else if (Configuration.doAutoCreate()) 237 this.reason = new CodeableConcept(); // cc 238 return this.reason; 239 } 240 241 public boolean hasReason() { 242 return this.reason != null && !this.reason.isEmpty(); 243 } 244 245 /** 246 * @param value {@link #reason} (Captures the reason for the current use or adherence of a medication.) 247 */ 248 public MedicationStatementAdherenceComponent setReason(CodeableConcept value) { 249 this.reason = value; 250 return this; 251 } 252 253 protected void listChildren(List<Property> children) { 254 super.listChildren(children); 255 children.add(new Property("code", "CodeableConcept", "Type of the adherence for the medication.", 0, 1, code)); 256 children.add(new Property("reason", "CodeableConcept", "Captures the reason for the current use or adherence of a medication.", 0, 1, reason)); 257 } 258 259 @Override 260 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 261 switch (_hash) { 262 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Type of the adherence for the medication.", 0, 1, code); 263 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Captures the reason for the current use or adherence of a medication.", 0, 1, reason); 264 default: return super.getNamedProperty(_hash, _name, _checkValid); 265 } 266 267 } 268 269 @Override 270 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 271 switch (hash) { 272 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 273 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 274 default: return super.getProperty(hash, name, checkValid); 275 } 276 277 } 278 279 @Override 280 public Base setProperty(int hash, String name, Base value) throws FHIRException { 281 switch (hash) { 282 case 3059181: // code 283 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 284 return value; 285 case -934964668: // reason 286 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 287 return value; 288 default: return super.setProperty(hash, name, value); 289 } 290 291 } 292 293 @Override 294 public Base setProperty(String name, Base value) throws FHIRException { 295 if (name.equals("code")) { 296 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 297 } else if (name.equals("reason")) { 298 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 299 } else 300 return super.setProperty(name, value); 301 return value; 302 } 303 304 @Override 305 public Base makeProperty(int hash, String name) throws FHIRException { 306 switch (hash) { 307 case 3059181: return getCode(); 308 case -934964668: return getReason(); 309 default: return super.makeProperty(hash, name); 310 } 311 312 } 313 314 @Override 315 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 316 switch (hash) { 317 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 318 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 319 default: return super.getTypesForProperty(hash, name); 320 } 321 322 } 323 324 @Override 325 public Base addChild(String name) throws FHIRException { 326 if (name.equals("code")) { 327 this.code = new CodeableConcept(); 328 return this.code; 329 } 330 else if (name.equals("reason")) { 331 this.reason = new CodeableConcept(); 332 return this.reason; 333 } 334 else 335 return super.addChild(name); 336 } 337 338 public MedicationStatementAdherenceComponent copy() { 339 MedicationStatementAdherenceComponent dst = new MedicationStatementAdherenceComponent(); 340 copyValues(dst); 341 return dst; 342 } 343 344 public void copyValues(MedicationStatementAdherenceComponent dst) { 345 super.copyValues(dst); 346 dst.code = code == null ? null : code.copy(); 347 dst.reason = reason == null ? null : reason.copy(); 348 } 349 350 @Override 351 public boolean equalsDeep(Base other_) { 352 if (!super.equalsDeep(other_)) 353 return false; 354 if (!(other_ instanceof MedicationStatementAdherenceComponent)) 355 return false; 356 MedicationStatementAdherenceComponent o = (MedicationStatementAdherenceComponent) other_; 357 return compareDeep(code, o.code, true) && compareDeep(reason, o.reason, true); 358 } 359 360 @Override 361 public boolean equalsShallow(Base other_) { 362 if (!super.equalsShallow(other_)) 363 return false; 364 if (!(other_ instanceof MedicationStatementAdherenceComponent)) 365 return false; 366 MedicationStatementAdherenceComponent o = (MedicationStatementAdherenceComponent) other_; 367 return true; 368 } 369 370 public boolean isEmpty() { 371 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, reason); 372 } 373 374 public String fhirType() { 375 return "MedicationStatement.adherence"; 376 377 } 378 379 } 380 381 /** 382 * Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server. 383 */ 384 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 385 @Description(shortDefinition="External identifier", formalDefinition="Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server." ) 386 protected List<Identifier> identifier; 387 388 /** 389 * A larger event of which this particular MedicationStatement is a component or step. 390 */ 391 @Child(name = "partOf", type = {Procedure.class, MedicationStatement.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 392 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular MedicationStatement is a component or step." ) 393 protected List<Reference> partOf; 394 395 /** 396 * A code representing the status of recording the medication statement. 397 */ 398 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 399 @Description(shortDefinition="recorded | entered-in-error | draft", formalDefinition="A code representing the status of recording the medication statement." ) 400 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-status") 401 protected Enumeration<MedicationStatementStatusCodes> status; 402 403 /** 404 * Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.). 405 */ 406 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 407 @Description(shortDefinition="Type of medication statement", formalDefinition="Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.)." ) 408 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-admin-location") 409 protected List<CodeableConcept> category; 410 411 /** 412 * Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 413 */ 414 @Child(name = "medication", type = {CodeableReference.class}, order=4, min=1, max=1, modifier=false, summary=true) 415 @Description(shortDefinition="What medication was taken", formalDefinition="Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 416 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 417 protected CodeableReference medication; 418 419 /** 420 * The person, animal or group who is/was taking the medication. 421 */ 422 @Child(name = "subject", type = {Patient.class, Group.class}, order=5, min=1, max=1, modifier=false, summary=true) 423 @Description(shortDefinition="Who is/was taking the medication", formalDefinition="The person, animal or group who is/was taking the medication." ) 424 protected Reference subject; 425 426 /** 427 * The encounter that establishes the context for this MedicationStatement. 428 */ 429 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 430 @Description(shortDefinition="Encounter associated with MedicationStatement", formalDefinition="The encounter that establishes the context for this MedicationStatement." ) 431 protected Reference encounter; 432 433 /** 434 * The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking). 435 */ 436 @Child(name = "effective", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 437 @Description(shortDefinition="The date/time or interval when the medication is/was/will be taken", formalDefinition="The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking)." ) 438 protected DataType effective; 439 440 /** 441 * The date when the Medication Statement was asserted by the information source. 442 */ 443 @Child(name = "dateAsserted", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 444 @Description(shortDefinition="When the usage was asserted?", formalDefinition="The date when the Medication Statement was asserted by the information source." ) 445 protected DateTimeType dateAsserted; 446 447 /** 448 * The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest. 449 */ 450 @Child(name = "informationSource", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Organization.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 451 @Description(shortDefinition="Person or organization that provided the information about the taking of this medication", formalDefinition="The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest." ) 452 protected List<Reference> informationSource; 453 454 /** 455 * Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement. 456 */ 457 @Child(name = "derivedFrom", type = {Reference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 458 @Description(shortDefinition="Link to information used to derive the MedicationStatement", formalDefinition="Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement." ) 459 protected List<Reference> derivedFrom; 460 461 /** 462 * A concept, Condition or observation that supports why the medication is being/was taken. 463 */ 464 @Child(name = "reason", type = {CodeableReference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 465 @Description(shortDefinition="Reason for why the medication is being/was taken", formalDefinition="A concept, Condition or observation that supports why the medication is being/was taken." ) 466 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 467 protected List<CodeableReference> reason; 468 469 /** 470 * Provides extra information about the Medication Statement that is not conveyed by the other attributes. 471 */ 472 @Child(name = "note", type = {Annotation.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 473 @Description(shortDefinition="Further information about the usage", formalDefinition="Provides extra information about the Medication Statement that is not conveyed by the other attributes." ) 474 protected List<Annotation> note; 475 476 /** 477 * Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc. 478 */ 479 @Child(name = "relatedClinicalInformation", type = {Observation.class, Condition.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 480 @Description(shortDefinition="Link to information relevant to the usage of a medication", formalDefinition="Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc." ) 481 protected List<Reference> relatedClinicalInformation; 482 483 /** 484 * The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 485 */ 486 @Child(name = "renderedDosageInstruction", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 487 @Description(shortDefinition="Full representation of the dosage instructions", formalDefinition="The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses." ) 488 protected MarkdownType renderedDosageInstruction; 489 490 /** 491 * Indicates how the medication is/was or should be taken by the patient. 492 */ 493 @Child(name = "dosage", type = {Dosage.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 494 @Description(shortDefinition="Details of how medication is/was taken or should be taken", formalDefinition="Indicates how the medication is/was or should be taken by the patient." ) 495 protected List<Dosage> dosage; 496 497 /** 498 * Indicates whether the medication is or is not being consumed or administered. 499 */ 500 @Child(name = "adherence", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 501 @Description(shortDefinition="Indicates whether the medication is or is not being consumed or administered", formalDefinition="Indicates whether the medication is or is not being consumed or administered." ) 502 protected MedicationStatementAdherenceComponent adherence; 503 504 private static final long serialVersionUID = -1043616866L; 505 506 /** 507 * Constructor 508 */ 509 public MedicationStatement() { 510 super(); 511 } 512 513 /** 514 * Constructor 515 */ 516 public MedicationStatement(MedicationStatementStatusCodes status, CodeableReference medication, Reference subject) { 517 super(); 518 this.setStatus(status); 519 this.setMedication(medication); 520 this.setSubject(subject); 521 } 522 523 /** 524 * @return {@link #identifier} (Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.) 525 */ 526 public List<Identifier> getIdentifier() { 527 if (this.identifier == null) 528 this.identifier = new ArrayList<Identifier>(); 529 return this.identifier; 530 } 531 532 /** 533 * @return Returns a reference to <code>this</code> for easy method chaining 534 */ 535 public MedicationStatement setIdentifier(List<Identifier> theIdentifier) { 536 this.identifier = theIdentifier; 537 return this; 538 } 539 540 public boolean hasIdentifier() { 541 if (this.identifier == null) 542 return false; 543 for (Identifier item : this.identifier) 544 if (!item.isEmpty()) 545 return true; 546 return false; 547 } 548 549 public Identifier addIdentifier() { //3 550 Identifier t = new Identifier(); 551 if (this.identifier == null) 552 this.identifier = new ArrayList<Identifier>(); 553 this.identifier.add(t); 554 return t; 555 } 556 557 public MedicationStatement addIdentifier(Identifier t) { //3 558 if (t == null) 559 return this; 560 if (this.identifier == null) 561 this.identifier = new ArrayList<Identifier>(); 562 this.identifier.add(t); 563 return this; 564 } 565 566 /** 567 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 568 */ 569 public Identifier getIdentifierFirstRep() { 570 if (getIdentifier().isEmpty()) { 571 addIdentifier(); 572 } 573 return getIdentifier().get(0); 574 } 575 576 /** 577 * @return {@link #partOf} (A larger event of which this particular MedicationStatement is a component or step.) 578 */ 579 public List<Reference> getPartOf() { 580 if (this.partOf == null) 581 this.partOf = new ArrayList<Reference>(); 582 return this.partOf; 583 } 584 585 /** 586 * @return Returns a reference to <code>this</code> for easy method chaining 587 */ 588 public MedicationStatement setPartOf(List<Reference> thePartOf) { 589 this.partOf = thePartOf; 590 return this; 591 } 592 593 public boolean hasPartOf() { 594 if (this.partOf == null) 595 return false; 596 for (Reference item : this.partOf) 597 if (!item.isEmpty()) 598 return true; 599 return false; 600 } 601 602 public Reference addPartOf() { //3 603 Reference t = new Reference(); 604 if (this.partOf == null) 605 this.partOf = new ArrayList<Reference>(); 606 this.partOf.add(t); 607 return t; 608 } 609 610 public MedicationStatement addPartOf(Reference t) { //3 611 if (t == null) 612 return this; 613 if (this.partOf == null) 614 this.partOf = new ArrayList<Reference>(); 615 this.partOf.add(t); 616 return this; 617 } 618 619 /** 620 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 621 */ 622 public Reference getPartOfFirstRep() { 623 if (getPartOf().isEmpty()) { 624 addPartOf(); 625 } 626 return getPartOf().get(0); 627 } 628 629 /** 630 * @return {@link #status} (A code representing the status of recording the medication statement.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 631 */ 632 public Enumeration<MedicationStatementStatusCodes> getStatusElement() { 633 if (this.status == null) 634 if (Configuration.errorOnAutoCreate()) 635 throw new Error("Attempt to auto-create MedicationStatement.status"); 636 else if (Configuration.doAutoCreate()) 637 this.status = new Enumeration<MedicationStatementStatusCodes>(new MedicationStatementStatusCodesEnumFactory()); // bb 638 return this.status; 639 } 640 641 public boolean hasStatusElement() { 642 return this.status != null && !this.status.isEmpty(); 643 } 644 645 public boolean hasStatus() { 646 return this.status != null && !this.status.isEmpty(); 647 } 648 649 /** 650 * @param value {@link #status} (A code representing the status of recording the medication statement.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 651 */ 652 public MedicationStatement setStatusElement(Enumeration<MedicationStatementStatusCodes> value) { 653 this.status = value; 654 return this; 655 } 656 657 /** 658 * @return A code representing the status of recording the medication statement. 659 */ 660 public MedicationStatementStatusCodes getStatus() { 661 return this.status == null ? null : this.status.getValue(); 662 } 663 664 /** 665 * @param value A code representing the status of recording the medication statement. 666 */ 667 public MedicationStatement setStatus(MedicationStatementStatusCodes value) { 668 if (this.status == null) 669 this.status = new Enumeration<MedicationStatementStatusCodes>(new MedicationStatementStatusCodesEnumFactory()); 670 this.status.setValue(value); 671 return this; 672 } 673 674 /** 675 * @return {@link #category} (Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).) 676 */ 677 public List<CodeableConcept> getCategory() { 678 if (this.category == null) 679 this.category = new ArrayList<CodeableConcept>(); 680 return this.category; 681 } 682 683 /** 684 * @return Returns a reference to <code>this</code> for easy method chaining 685 */ 686 public MedicationStatement setCategory(List<CodeableConcept> theCategory) { 687 this.category = theCategory; 688 return this; 689 } 690 691 public boolean hasCategory() { 692 if (this.category == null) 693 return false; 694 for (CodeableConcept item : this.category) 695 if (!item.isEmpty()) 696 return true; 697 return false; 698 } 699 700 public CodeableConcept addCategory() { //3 701 CodeableConcept t = new CodeableConcept(); 702 if (this.category == null) 703 this.category = new ArrayList<CodeableConcept>(); 704 this.category.add(t); 705 return t; 706 } 707 708 public MedicationStatement addCategory(CodeableConcept t) { //3 709 if (t == null) 710 return this; 711 if (this.category == null) 712 this.category = new ArrayList<CodeableConcept>(); 713 this.category.add(t); 714 return this; 715 } 716 717 /** 718 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 719 */ 720 public CodeableConcept getCategoryFirstRep() { 721 if (getCategory().isEmpty()) { 722 addCategory(); 723 } 724 return getCategory().get(0); 725 } 726 727 /** 728 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 729 */ 730 public CodeableReference getMedication() { 731 if (this.medication == null) 732 if (Configuration.errorOnAutoCreate()) 733 throw new Error("Attempt to auto-create MedicationStatement.medication"); 734 else if (Configuration.doAutoCreate()) 735 this.medication = new CodeableReference(); // cc 736 return this.medication; 737 } 738 739 public boolean hasMedication() { 740 return this.medication != null && !this.medication.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 745 */ 746 public MedicationStatement setMedication(CodeableReference value) { 747 this.medication = value; 748 return this; 749 } 750 751 /** 752 * @return {@link #subject} (The person, animal or group who is/was taking the medication.) 753 */ 754 public Reference getSubject() { 755 if (this.subject == null) 756 if (Configuration.errorOnAutoCreate()) 757 throw new Error("Attempt to auto-create MedicationStatement.subject"); 758 else if (Configuration.doAutoCreate()) 759 this.subject = new Reference(); // cc 760 return this.subject; 761 } 762 763 public boolean hasSubject() { 764 return this.subject != null && !this.subject.isEmpty(); 765 } 766 767 /** 768 * @param value {@link #subject} (The person, animal or group who is/was taking the medication.) 769 */ 770 public MedicationStatement setSubject(Reference value) { 771 this.subject = value; 772 return this; 773 } 774 775 /** 776 * @return {@link #encounter} (The encounter that establishes the context for this MedicationStatement.) 777 */ 778 public Reference getEncounter() { 779 if (this.encounter == null) 780 if (Configuration.errorOnAutoCreate()) 781 throw new Error("Attempt to auto-create MedicationStatement.encounter"); 782 else if (Configuration.doAutoCreate()) 783 this.encounter = new Reference(); // cc 784 return this.encounter; 785 } 786 787 public boolean hasEncounter() { 788 return this.encounter != null && !this.encounter.isEmpty(); 789 } 790 791 /** 792 * @param value {@link #encounter} (The encounter that establishes the context for this MedicationStatement.) 793 */ 794 public MedicationStatement setEncounter(Reference value) { 795 this.encounter = value; 796 return this; 797 } 798 799 /** 800 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 801 */ 802 public DataType getEffective() { 803 return this.effective; 804 } 805 806 /** 807 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 808 */ 809 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 810 if (this.effective == null) 811 this.effective = new DateTimeType(); 812 if (!(this.effective instanceof DateTimeType)) 813 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 814 return (DateTimeType) this.effective; 815 } 816 817 public boolean hasEffectiveDateTimeType() { 818 return this != null && this.effective instanceof DateTimeType; 819 } 820 821 /** 822 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 823 */ 824 public Period getEffectivePeriod() throws FHIRException { 825 if (this.effective == null) 826 this.effective = new Period(); 827 if (!(this.effective instanceof Period)) 828 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 829 return (Period) this.effective; 830 } 831 832 public boolean hasEffectivePeriod() { 833 return this != null && this.effective instanceof Period; 834 } 835 836 /** 837 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 838 */ 839 public Timing getEffectiveTiming() throws FHIRException { 840 if (this.effective == null) 841 this.effective = new Timing(); 842 if (!(this.effective instanceof Timing)) 843 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.effective.getClass().getName()+" was encountered"); 844 return (Timing) this.effective; 845 } 846 847 public boolean hasEffectiveTiming() { 848 return this != null && this.effective instanceof Timing; 849 } 850 851 public boolean hasEffective() { 852 return this.effective != null && !this.effective.isEmpty(); 853 } 854 855 /** 856 * @param value {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 857 */ 858 public MedicationStatement setEffective(DataType value) { 859 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 860 throw new FHIRException("Not the right type for MedicationStatement.effective[x]: "+value.fhirType()); 861 this.effective = value; 862 return this; 863 } 864 865 /** 866 * @return {@link #dateAsserted} (The date when the Medication Statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 867 */ 868 public DateTimeType getDateAssertedElement() { 869 if (this.dateAsserted == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create MedicationStatement.dateAsserted"); 872 else if (Configuration.doAutoCreate()) 873 this.dateAsserted = new DateTimeType(); // bb 874 return this.dateAsserted; 875 } 876 877 public boolean hasDateAssertedElement() { 878 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 879 } 880 881 public boolean hasDateAsserted() { 882 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #dateAsserted} (The date when the Medication Statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 887 */ 888 public MedicationStatement setDateAssertedElement(DateTimeType value) { 889 this.dateAsserted = value; 890 return this; 891 } 892 893 /** 894 * @return The date when the Medication Statement was asserted by the information source. 895 */ 896 public Date getDateAsserted() { 897 return this.dateAsserted == null ? null : this.dateAsserted.getValue(); 898 } 899 900 /** 901 * @param value The date when the Medication Statement was asserted by the information source. 902 */ 903 public MedicationStatement setDateAsserted(Date value) { 904 if (value == null) 905 this.dateAsserted = null; 906 else { 907 if (this.dateAsserted == null) 908 this.dateAsserted = new DateTimeType(); 909 this.dateAsserted.setValue(value); 910 } 911 return this; 912 } 913 914 /** 915 * @return {@link #informationSource} (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.) 916 */ 917 public List<Reference> getInformationSource() { 918 if (this.informationSource == null) 919 this.informationSource = new ArrayList<Reference>(); 920 return this.informationSource; 921 } 922 923 /** 924 * @return Returns a reference to <code>this</code> for easy method chaining 925 */ 926 public MedicationStatement setInformationSource(List<Reference> theInformationSource) { 927 this.informationSource = theInformationSource; 928 return this; 929 } 930 931 public boolean hasInformationSource() { 932 if (this.informationSource == null) 933 return false; 934 for (Reference item : this.informationSource) 935 if (!item.isEmpty()) 936 return true; 937 return false; 938 } 939 940 public Reference addInformationSource() { //3 941 Reference t = new Reference(); 942 if (this.informationSource == null) 943 this.informationSource = new ArrayList<Reference>(); 944 this.informationSource.add(t); 945 return t; 946 } 947 948 public MedicationStatement addInformationSource(Reference t) { //3 949 if (t == null) 950 return this; 951 if (this.informationSource == null) 952 this.informationSource = new ArrayList<Reference>(); 953 this.informationSource.add(t); 954 return this; 955 } 956 957 /** 958 * @return The first repetition of repeating field {@link #informationSource}, creating it if it does not already exist {3} 959 */ 960 public Reference getInformationSourceFirstRep() { 961 if (getInformationSource().isEmpty()) { 962 addInformationSource(); 963 } 964 return getInformationSource().get(0); 965 } 966 967 /** 968 * @return {@link #derivedFrom} (Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.) 969 */ 970 public List<Reference> getDerivedFrom() { 971 if (this.derivedFrom == null) 972 this.derivedFrom = new ArrayList<Reference>(); 973 return this.derivedFrom; 974 } 975 976 /** 977 * @return Returns a reference to <code>this</code> for easy method chaining 978 */ 979 public MedicationStatement setDerivedFrom(List<Reference> theDerivedFrom) { 980 this.derivedFrom = theDerivedFrom; 981 return this; 982 } 983 984 public boolean hasDerivedFrom() { 985 if (this.derivedFrom == null) 986 return false; 987 for (Reference item : this.derivedFrom) 988 if (!item.isEmpty()) 989 return true; 990 return false; 991 } 992 993 public Reference addDerivedFrom() { //3 994 Reference t = new Reference(); 995 if (this.derivedFrom == null) 996 this.derivedFrom = new ArrayList<Reference>(); 997 this.derivedFrom.add(t); 998 return t; 999 } 1000 1001 public MedicationStatement addDerivedFrom(Reference t) { //3 1002 if (t == null) 1003 return this; 1004 if (this.derivedFrom == null) 1005 this.derivedFrom = new ArrayList<Reference>(); 1006 this.derivedFrom.add(t); 1007 return this; 1008 } 1009 1010 /** 1011 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 1012 */ 1013 public Reference getDerivedFromFirstRep() { 1014 if (getDerivedFrom().isEmpty()) { 1015 addDerivedFrom(); 1016 } 1017 return getDerivedFrom().get(0); 1018 } 1019 1020 /** 1021 * @return {@link #reason} (A concept, Condition or observation that supports why the medication is being/was taken.) 1022 */ 1023 public List<CodeableReference> getReason() { 1024 if (this.reason == null) 1025 this.reason = new ArrayList<CodeableReference>(); 1026 return this.reason; 1027 } 1028 1029 /** 1030 * @return Returns a reference to <code>this</code> for easy method chaining 1031 */ 1032 public MedicationStatement setReason(List<CodeableReference> theReason) { 1033 this.reason = theReason; 1034 return this; 1035 } 1036 1037 public boolean hasReason() { 1038 if (this.reason == null) 1039 return false; 1040 for (CodeableReference item : this.reason) 1041 if (!item.isEmpty()) 1042 return true; 1043 return false; 1044 } 1045 1046 public CodeableReference addReason() { //3 1047 CodeableReference t = new CodeableReference(); 1048 if (this.reason == null) 1049 this.reason = new ArrayList<CodeableReference>(); 1050 this.reason.add(t); 1051 return t; 1052 } 1053 1054 public MedicationStatement addReason(CodeableReference t) { //3 1055 if (t == null) 1056 return this; 1057 if (this.reason == null) 1058 this.reason = new ArrayList<CodeableReference>(); 1059 this.reason.add(t); 1060 return this; 1061 } 1062 1063 /** 1064 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1065 */ 1066 public CodeableReference getReasonFirstRep() { 1067 if (getReason().isEmpty()) { 1068 addReason(); 1069 } 1070 return getReason().get(0); 1071 } 1072 1073 /** 1074 * @return {@link #note} (Provides extra information about the Medication Statement that is not conveyed by the other attributes.) 1075 */ 1076 public List<Annotation> getNote() { 1077 if (this.note == null) 1078 this.note = new ArrayList<Annotation>(); 1079 return this.note; 1080 } 1081 1082 /** 1083 * @return Returns a reference to <code>this</code> for easy method chaining 1084 */ 1085 public MedicationStatement setNote(List<Annotation> theNote) { 1086 this.note = theNote; 1087 return this; 1088 } 1089 1090 public boolean hasNote() { 1091 if (this.note == null) 1092 return false; 1093 for (Annotation item : this.note) 1094 if (!item.isEmpty()) 1095 return true; 1096 return false; 1097 } 1098 1099 public Annotation addNote() { //3 1100 Annotation t = new Annotation(); 1101 if (this.note == null) 1102 this.note = new ArrayList<Annotation>(); 1103 this.note.add(t); 1104 return t; 1105 } 1106 1107 public MedicationStatement addNote(Annotation t) { //3 1108 if (t == null) 1109 return this; 1110 if (this.note == null) 1111 this.note = new ArrayList<Annotation>(); 1112 this.note.add(t); 1113 return this; 1114 } 1115 1116 /** 1117 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1118 */ 1119 public Annotation getNoteFirstRep() { 1120 if (getNote().isEmpty()) { 1121 addNote(); 1122 } 1123 return getNote().get(0); 1124 } 1125 1126 /** 1127 * @return {@link #relatedClinicalInformation} (Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc.) 1128 */ 1129 public List<Reference> getRelatedClinicalInformation() { 1130 if (this.relatedClinicalInformation == null) 1131 this.relatedClinicalInformation = new ArrayList<Reference>(); 1132 return this.relatedClinicalInformation; 1133 } 1134 1135 /** 1136 * @return Returns a reference to <code>this</code> for easy method chaining 1137 */ 1138 public MedicationStatement setRelatedClinicalInformation(List<Reference> theRelatedClinicalInformation) { 1139 this.relatedClinicalInformation = theRelatedClinicalInformation; 1140 return this; 1141 } 1142 1143 public boolean hasRelatedClinicalInformation() { 1144 if (this.relatedClinicalInformation == null) 1145 return false; 1146 for (Reference item : this.relatedClinicalInformation) 1147 if (!item.isEmpty()) 1148 return true; 1149 return false; 1150 } 1151 1152 public Reference addRelatedClinicalInformation() { //3 1153 Reference t = new Reference(); 1154 if (this.relatedClinicalInformation == null) 1155 this.relatedClinicalInformation = new ArrayList<Reference>(); 1156 this.relatedClinicalInformation.add(t); 1157 return t; 1158 } 1159 1160 public MedicationStatement addRelatedClinicalInformation(Reference t) { //3 1161 if (t == null) 1162 return this; 1163 if (this.relatedClinicalInformation == null) 1164 this.relatedClinicalInformation = new ArrayList<Reference>(); 1165 this.relatedClinicalInformation.add(t); 1166 return this; 1167 } 1168 1169 /** 1170 * @return The first repetition of repeating field {@link #relatedClinicalInformation}, creating it if it does not already exist {3} 1171 */ 1172 public Reference getRelatedClinicalInformationFirstRep() { 1173 if (getRelatedClinicalInformation().isEmpty()) { 1174 addRelatedClinicalInformation(); 1175 } 1176 return getRelatedClinicalInformation().get(0); 1177 } 1178 1179 /** 1180 * @return {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 1181 */ 1182 public MarkdownType getRenderedDosageInstructionElement() { 1183 if (this.renderedDosageInstruction == null) 1184 if (Configuration.errorOnAutoCreate()) 1185 throw new Error("Attempt to auto-create MedicationStatement.renderedDosageInstruction"); 1186 else if (Configuration.doAutoCreate()) 1187 this.renderedDosageInstruction = new MarkdownType(); // bb 1188 return this.renderedDosageInstruction; 1189 } 1190 1191 public boolean hasRenderedDosageInstructionElement() { 1192 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 1193 } 1194 1195 public boolean hasRenderedDosageInstruction() { 1196 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 1201 */ 1202 public MedicationStatement setRenderedDosageInstructionElement(MarkdownType value) { 1203 this.renderedDosageInstruction = value; 1204 return this; 1205 } 1206 1207 /** 1208 * @return The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 1209 */ 1210 public String getRenderedDosageInstruction() { 1211 return this.renderedDosageInstruction == null ? null : this.renderedDosageInstruction.getValue(); 1212 } 1213 1214 /** 1215 * @param value The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 1216 */ 1217 public MedicationStatement setRenderedDosageInstruction(String value) { 1218 if (Utilities.noString(value)) 1219 this.renderedDosageInstruction = null; 1220 else { 1221 if (this.renderedDosageInstruction == null) 1222 this.renderedDosageInstruction = new MarkdownType(); 1223 this.renderedDosageInstruction.setValue(value); 1224 } 1225 return this; 1226 } 1227 1228 /** 1229 * @return {@link #dosage} (Indicates how the medication is/was or should be taken by the patient.) 1230 */ 1231 public List<Dosage> getDosage() { 1232 if (this.dosage == null) 1233 this.dosage = new ArrayList<Dosage>(); 1234 return this.dosage; 1235 } 1236 1237 /** 1238 * @return Returns a reference to <code>this</code> for easy method chaining 1239 */ 1240 public MedicationStatement setDosage(List<Dosage> theDosage) { 1241 this.dosage = theDosage; 1242 return this; 1243 } 1244 1245 public boolean hasDosage() { 1246 if (this.dosage == null) 1247 return false; 1248 for (Dosage item : this.dosage) 1249 if (!item.isEmpty()) 1250 return true; 1251 return false; 1252 } 1253 1254 public Dosage addDosage() { //3 1255 Dosage t = new Dosage(); 1256 if (this.dosage == null) 1257 this.dosage = new ArrayList<Dosage>(); 1258 this.dosage.add(t); 1259 return t; 1260 } 1261 1262 public MedicationStatement addDosage(Dosage t) { //3 1263 if (t == null) 1264 return this; 1265 if (this.dosage == null) 1266 this.dosage = new ArrayList<Dosage>(); 1267 this.dosage.add(t); 1268 return this; 1269 } 1270 1271 /** 1272 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist {3} 1273 */ 1274 public Dosage getDosageFirstRep() { 1275 if (getDosage().isEmpty()) { 1276 addDosage(); 1277 } 1278 return getDosage().get(0); 1279 } 1280 1281 /** 1282 * @return {@link #adherence} (Indicates whether the medication is or is not being consumed or administered.) 1283 */ 1284 public MedicationStatementAdherenceComponent getAdherence() { 1285 if (this.adherence == null) 1286 if (Configuration.errorOnAutoCreate()) 1287 throw new Error("Attempt to auto-create MedicationStatement.adherence"); 1288 else if (Configuration.doAutoCreate()) 1289 this.adherence = new MedicationStatementAdherenceComponent(); // cc 1290 return this.adherence; 1291 } 1292 1293 public boolean hasAdherence() { 1294 return this.adherence != null && !this.adherence.isEmpty(); 1295 } 1296 1297 /** 1298 * @param value {@link #adherence} (Indicates whether the medication is or is not being consumed or administered.) 1299 */ 1300 public MedicationStatement setAdherence(MedicationStatementAdherenceComponent value) { 1301 this.adherence = value; 1302 return this; 1303 } 1304 1305 protected void listChildren(List<Property> children) { 1306 super.listChildren(children); 1307 children.add(new Property("identifier", "Identifier", "Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1308 children.add(new Property("partOf", "Reference(Procedure|MedicationStatement)", "A larger event of which this particular MedicationStatement is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1309 children.add(new Property("status", "code", "A code representing the status of recording the medication statement.", 0, 1, status)); 1310 children.add(new Property("category", "CodeableConcept", "Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).", 0, java.lang.Integer.MAX_VALUE, category)); 1311 children.add(new Property("medication", "CodeableReference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 1312 children.add(new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject)); 1313 children.add(new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this MedicationStatement.", 0, 1, encounter)); 1314 children.add(new Property("effective[x]", "dateTime|Period|Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective)); 1315 children.add(new Property("dateAsserted", "dateTime", "The date when the Medication Statement was asserted by the information source.", 0, 1, dateAsserted)); 1316 children.add(new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.", 0, java.lang.Integer.MAX_VALUE, informationSource)); 1317 children.add(new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1318 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "A concept, Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reason)); 1319 children.add(new Property("note", "Annotation", "Provides extra information about the Medication Statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 1320 children.add(new Property("relatedClinicalInformation", "Reference(Observation|Condition)", "Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc.", 0, java.lang.Integer.MAX_VALUE, relatedClinicalInformation)); 1321 children.add(new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction)); 1322 children.add(new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage)); 1323 children.add(new Property("adherence", "", "Indicates whether the medication is or is not being consumed or administered.", 0, 1, adherence)); 1324 } 1325 1326 @Override 1327 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1328 switch (_hash) { 1329 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1330 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure|MedicationStatement)", "A larger event of which this particular MedicationStatement is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1331 case -892481550: /*status*/ return new Property("status", "code", "A code representing the status of recording the medication statement.", 0, 1, status); 1332 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).", 0, java.lang.Integer.MAX_VALUE, category); 1333 case 1998965455: /*medication*/ return new Property("medication", "CodeableReference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1334 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject); 1335 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this MedicationStatement.", 0, 1, encounter); 1336 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period|Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1337 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period|Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1338 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1339 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "Period", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1340 case -285872943: /*effectiveTiming*/ return new Property("effective[x]", "Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1341 case -1980855245: /*dateAsserted*/ return new Property("dateAsserted", "dateTime", "The date when the Medication Statement was asserted by the information source.", 0, 1, dateAsserted); 1342 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.", 0, java.lang.Integer.MAX_VALUE, informationSource); 1343 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1344 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "A concept, Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reason); 1345 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides extra information about the Medication Statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 1346 case 1040787950: /*relatedClinicalInformation*/ return new Property("relatedClinicalInformation", "Reference(Observation|Condition)", "Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc.", 0, java.lang.Integer.MAX_VALUE, relatedClinicalInformation); 1347 case 1718902050: /*renderedDosageInstruction*/ return new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction); 1348 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage); 1349 case -231003683: /*adherence*/ return new Property("adherence", "", "Indicates whether the medication is or is not being consumed or administered.", 0, 1, adherence); 1350 default: return super.getNamedProperty(_hash, _name, _checkValid); 1351 } 1352 1353 } 1354 1355 @Override 1356 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1357 switch (hash) { 1358 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1359 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1360 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationStatementStatusCodes> 1361 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1362 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // CodeableReference 1363 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1364 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1365 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // DataType 1366 case -1980855245: /*dateAsserted*/ return this.dateAsserted == null ? new Base[0] : new Base[] {this.dateAsserted}; // DateTimeType 1367 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : this.informationSource.toArray(new Base[this.informationSource.size()]); // Reference 1368 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1369 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1370 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1371 case 1040787950: /*relatedClinicalInformation*/ return this.relatedClinicalInformation == null ? new Base[0] : this.relatedClinicalInformation.toArray(new Base[this.relatedClinicalInformation.size()]); // Reference 1372 case 1718902050: /*renderedDosageInstruction*/ return this.renderedDosageInstruction == null ? new Base[0] : new Base[] {this.renderedDosageInstruction}; // MarkdownType 1373 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 1374 case -231003683: /*adherence*/ return this.adherence == null ? new Base[0] : new Base[] {this.adherence}; // MedicationStatementAdherenceComponent 1375 default: return super.getProperty(hash, name, checkValid); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1382 switch (hash) { 1383 case -1618432855: // identifier 1384 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1385 return value; 1386 case -995410646: // partOf 1387 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1388 return value; 1389 case -892481550: // status 1390 value = new MedicationStatementStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1391 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatusCodes> 1392 return value; 1393 case 50511102: // category 1394 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1395 return value; 1396 case 1998965455: // medication 1397 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 1398 return value; 1399 case -1867885268: // subject 1400 this.subject = TypeConvertor.castToReference(value); // Reference 1401 return value; 1402 case 1524132147: // encounter 1403 this.encounter = TypeConvertor.castToReference(value); // Reference 1404 return value; 1405 case -1468651097: // effective 1406 this.effective = TypeConvertor.castToType(value); // DataType 1407 return value; 1408 case -1980855245: // dateAsserted 1409 this.dateAsserted = TypeConvertor.castToDateTime(value); // DateTimeType 1410 return value; 1411 case -2123220889: // informationSource 1412 this.getInformationSource().add(TypeConvertor.castToReference(value)); // Reference 1413 return value; 1414 case 1077922663: // derivedFrom 1415 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 1416 return value; 1417 case -934964668: // reason 1418 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1419 return value; 1420 case 3387378: // note 1421 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1422 return value; 1423 case 1040787950: // relatedClinicalInformation 1424 this.getRelatedClinicalInformation().add(TypeConvertor.castToReference(value)); // Reference 1425 return value; 1426 case 1718902050: // renderedDosageInstruction 1427 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 1428 return value; 1429 case -1326018889: // dosage 1430 this.getDosage().add(TypeConvertor.castToDosage(value)); // Dosage 1431 return value; 1432 case -231003683: // adherence 1433 this.adherence = (MedicationStatementAdherenceComponent) value; // MedicationStatementAdherenceComponent 1434 return value; 1435 default: return super.setProperty(hash, name, value); 1436 } 1437 1438 } 1439 1440 @Override 1441 public Base setProperty(String name, Base value) throws FHIRException { 1442 if (name.equals("identifier")) { 1443 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1444 } else if (name.equals("partOf")) { 1445 this.getPartOf().add(TypeConvertor.castToReference(value)); 1446 } else if (name.equals("status")) { 1447 value = new MedicationStatementStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1448 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatusCodes> 1449 } else if (name.equals("category")) { 1450 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1451 } else if (name.equals("medication")) { 1452 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 1453 } else if (name.equals("subject")) { 1454 this.subject = TypeConvertor.castToReference(value); // Reference 1455 } else if (name.equals("encounter")) { 1456 this.encounter = TypeConvertor.castToReference(value); // Reference 1457 } else if (name.equals("effective[x]")) { 1458 this.effective = TypeConvertor.castToType(value); // DataType 1459 } else if (name.equals("dateAsserted")) { 1460 this.dateAsserted = TypeConvertor.castToDateTime(value); // DateTimeType 1461 } else if (name.equals("informationSource")) { 1462 this.getInformationSource().add(TypeConvertor.castToReference(value)); 1463 } else if (name.equals("derivedFrom")) { 1464 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 1465 } else if (name.equals("reason")) { 1466 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1467 } else if (name.equals("note")) { 1468 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1469 } else if (name.equals("relatedClinicalInformation")) { 1470 this.getRelatedClinicalInformation().add(TypeConvertor.castToReference(value)); 1471 } else if (name.equals("renderedDosageInstruction")) { 1472 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 1473 } else if (name.equals("dosage")) { 1474 this.getDosage().add(TypeConvertor.castToDosage(value)); 1475 } else if (name.equals("adherence")) { 1476 this.adherence = (MedicationStatementAdherenceComponent) value; // MedicationStatementAdherenceComponent 1477 } else 1478 return super.setProperty(name, value); 1479 return value; 1480 } 1481 1482 @Override 1483 public Base makeProperty(int hash, String name) throws FHIRException { 1484 switch (hash) { 1485 case -1618432855: return addIdentifier(); 1486 case -995410646: return addPartOf(); 1487 case -892481550: return getStatusElement(); 1488 case 50511102: return addCategory(); 1489 case 1998965455: return getMedication(); 1490 case -1867885268: return getSubject(); 1491 case 1524132147: return getEncounter(); 1492 case 247104889: return getEffective(); 1493 case -1468651097: return getEffective(); 1494 case -1980855245: return getDateAssertedElement(); 1495 case -2123220889: return addInformationSource(); 1496 case 1077922663: return addDerivedFrom(); 1497 case -934964668: return addReason(); 1498 case 3387378: return addNote(); 1499 case 1040787950: return addRelatedClinicalInformation(); 1500 case 1718902050: return getRenderedDosageInstructionElement(); 1501 case -1326018889: return addDosage(); 1502 case -231003683: return getAdherence(); 1503 default: return super.makeProperty(hash, name); 1504 } 1505 1506 } 1507 1508 @Override 1509 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1510 switch (hash) { 1511 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1512 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1513 case -892481550: /*status*/ return new String[] {"code"}; 1514 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1515 case 1998965455: /*medication*/ return new String[] {"CodeableReference"}; 1516 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1517 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1518 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period", "Timing"}; 1519 case -1980855245: /*dateAsserted*/ return new String[] {"dateTime"}; 1520 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 1521 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 1522 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1523 case 3387378: /*note*/ return new String[] {"Annotation"}; 1524 case 1040787950: /*relatedClinicalInformation*/ return new String[] {"Reference"}; 1525 case 1718902050: /*renderedDosageInstruction*/ return new String[] {"markdown"}; 1526 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 1527 case -231003683: /*adherence*/ return new String[] {}; 1528 default: return super.getTypesForProperty(hash, name); 1529 } 1530 1531 } 1532 1533 @Override 1534 public Base addChild(String name) throws FHIRException { 1535 if (name.equals("identifier")) { 1536 return addIdentifier(); 1537 } 1538 else if (name.equals("partOf")) { 1539 return addPartOf(); 1540 } 1541 else if (name.equals("status")) { 1542 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.status"); 1543 } 1544 else if (name.equals("category")) { 1545 return addCategory(); 1546 } 1547 else if (name.equals("medication")) { 1548 this.medication = new CodeableReference(); 1549 return this.medication; 1550 } 1551 else if (name.equals("subject")) { 1552 this.subject = new Reference(); 1553 return this.subject; 1554 } 1555 else if (name.equals("encounter")) { 1556 this.encounter = new Reference(); 1557 return this.encounter; 1558 } 1559 else if (name.equals("effectiveDateTime")) { 1560 this.effective = new DateTimeType(); 1561 return this.effective; 1562 } 1563 else if (name.equals("effectivePeriod")) { 1564 this.effective = new Period(); 1565 return this.effective; 1566 } 1567 else if (name.equals("effectiveTiming")) { 1568 this.effective = new Timing(); 1569 return this.effective; 1570 } 1571 else if (name.equals("dateAsserted")) { 1572 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.dateAsserted"); 1573 } 1574 else if (name.equals("informationSource")) { 1575 return addInformationSource(); 1576 } 1577 else if (name.equals("derivedFrom")) { 1578 return addDerivedFrom(); 1579 } 1580 else if (name.equals("reason")) { 1581 return addReason(); 1582 } 1583 else if (name.equals("note")) { 1584 return addNote(); 1585 } 1586 else if (name.equals("relatedClinicalInformation")) { 1587 return addRelatedClinicalInformation(); 1588 } 1589 else if (name.equals("renderedDosageInstruction")) { 1590 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.renderedDosageInstruction"); 1591 } 1592 else if (name.equals("dosage")) { 1593 return addDosage(); 1594 } 1595 else if (name.equals("adherence")) { 1596 this.adherence = new MedicationStatementAdherenceComponent(); 1597 return this.adherence; 1598 } 1599 else 1600 return super.addChild(name); 1601 } 1602 1603 public String fhirType() { 1604 return "MedicationStatement"; 1605 1606 } 1607 1608 public MedicationStatement copy() { 1609 MedicationStatement dst = new MedicationStatement(); 1610 copyValues(dst); 1611 return dst; 1612 } 1613 1614 public void copyValues(MedicationStatement dst) { 1615 super.copyValues(dst); 1616 if (identifier != null) { 1617 dst.identifier = new ArrayList<Identifier>(); 1618 for (Identifier i : identifier) 1619 dst.identifier.add(i.copy()); 1620 }; 1621 if (partOf != null) { 1622 dst.partOf = new ArrayList<Reference>(); 1623 for (Reference i : partOf) 1624 dst.partOf.add(i.copy()); 1625 }; 1626 dst.status = status == null ? null : status.copy(); 1627 if (category != null) { 1628 dst.category = new ArrayList<CodeableConcept>(); 1629 for (CodeableConcept i : category) 1630 dst.category.add(i.copy()); 1631 }; 1632 dst.medication = medication == null ? null : medication.copy(); 1633 dst.subject = subject == null ? null : subject.copy(); 1634 dst.encounter = encounter == null ? null : encounter.copy(); 1635 dst.effective = effective == null ? null : effective.copy(); 1636 dst.dateAsserted = dateAsserted == null ? null : dateAsserted.copy(); 1637 if (informationSource != null) { 1638 dst.informationSource = new ArrayList<Reference>(); 1639 for (Reference i : informationSource) 1640 dst.informationSource.add(i.copy()); 1641 }; 1642 if (derivedFrom != null) { 1643 dst.derivedFrom = new ArrayList<Reference>(); 1644 for (Reference i : derivedFrom) 1645 dst.derivedFrom.add(i.copy()); 1646 }; 1647 if (reason != null) { 1648 dst.reason = new ArrayList<CodeableReference>(); 1649 for (CodeableReference i : reason) 1650 dst.reason.add(i.copy()); 1651 }; 1652 if (note != null) { 1653 dst.note = new ArrayList<Annotation>(); 1654 for (Annotation i : note) 1655 dst.note.add(i.copy()); 1656 }; 1657 if (relatedClinicalInformation != null) { 1658 dst.relatedClinicalInformation = new ArrayList<Reference>(); 1659 for (Reference i : relatedClinicalInformation) 1660 dst.relatedClinicalInformation.add(i.copy()); 1661 }; 1662 dst.renderedDosageInstruction = renderedDosageInstruction == null ? null : renderedDosageInstruction.copy(); 1663 if (dosage != null) { 1664 dst.dosage = new ArrayList<Dosage>(); 1665 for (Dosage i : dosage) 1666 dst.dosage.add(i.copy()); 1667 }; 1668 dst.adherence = adherence == null ? null : adherence.copy(); 1669 } 1670 1671 protected MedicationStatement typedCopy() { 1672 return copy(); 1673 } 1674 1675 @Override 1676 public boolean equalsDeep(Base other_) { 1677 if (!super.equalsDeep(other_)) 1678 return false; 1679 if (!(other_ instanceof MedicationStatement)) 1680 return false; 1681 MedicationStatement o = (MedicationStatement) other_; 1682 return compareDeep(identifier, o.identifier, true) && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 1683 && compareDeep(category, o.category, true) && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 1684 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) && compareDeep(dateAsserted, o.dateAsserted, true) 1685 && compareDeep(informationSource, o.informationSource, true) && compareDeep(derivedFrom, o.derivedFrom, true) 1686 && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) && compareDeep(relatedClinicalInformation, o.relatedClinicalInformation, true) 1687 && compareDeep(renderedDosageInstruction, o.renderedDosageInstruction, true) && compareDeep(dosage, o.dosage, true) 1688 && compareDeep(adherence, o.adherence, true); 1689 } 1690 1691 @Override 1692 public boolean equalsShallow(Base other_) { 1693 if (!super.equalsShallow(other_)) 1694 return false; 1695 if (!(other_ instanceof MedicationStatement)) 1696 return false; 1697 MedicationStatement o = (MedicationStatement) other_; 1698 return compareValues(status, o.status, true) && compareValues(dateAsserted, o.dateAsserted, true) && compareValues(renderedDosageInstruction, o.renderedDosageInstruction, true) 1699 ; 1700 } 1701 1702 public boolean isEmpty() { 1703 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, partOf, status 1704 , category, medication, subject, encounter, effective, dateAsserted, informationSource 1705 , derivedFrom, reason, note, relatedClinicalInformation, renderedDosageInstruction 1706 , dosage, adherence); 1707 } 1708 1709 @Override 1710 public ResourceType getResourceType() { 1711 return ResourceType.MedicationStatement; 1712 } 1713 1714 /** 1715 * Search parameter: <b>adherence</b> 1716 * <p> 1717 * Description: <b>Returns statements based on adherence or compliance</b><br> 1718 * Type: <b>token</b><br> 1719 * Path: <b>MedicationStatement.adherence.code</b><br> 1720 * </p> 1721 */ 1722 @SearchParamDefinition(name="adherence", path="MedicationStatement.adherence.code", description="Returns statements based on adherence or compliance", type="token" ) 1723 public static final String SP_ADHERENCE = "adherence"; 1724 /** 1725 * <b>Fluent Client</b> search parameter constant for <b>adherence</b> 1726 * <p> 1727 * Description: <b>Returns statements based on adherence or compliance</b><br> 1728 * Type: <b>token</b><br> 1729 * Path: <b>MedicationStatement.adherence.code</b><br> 1730 * </p> 1731 */ 1732 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADHERENCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADHERENCE); 1733 1734 /** 1735 * Search parameter: <b>category</b> 1736 * <p> 1737 * Description: <b>Returns statements of this category of MedicationStatement</b><br> 1738 * Type: <b>token</b><br> 1739 * Path: <b>MedicationStatement.category</b><br> 1740 * </p> 1741 */ 1742 @SearchParamDefinition(name="category", path="MedicationStatement.category", description="Returns statements of this category of MedicationStatement", type="token" ) 1743 public static final String SP_CATEGORY = "category"; 1744 /** 1745 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1746 * <p> 1747 * Description: <b>Returns statements of this category of MedicationStatement</b><br> 1748 * Type: <b>token</b><br> 1749 * Path: <b>MedicationStatement.category</b><br> 1750 * </p> 1751 */ 1752 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1753 1754 /** 1755 * Search parameter: <b>effective</b> 1756 * <p> 1757 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1758 * Type: <b>date</b><br> 1759 * Path: <b>MedicationStatement.effective.ofType(dateTime) | MedicationStatement.effective.ofType(Period)</b><br> 1760 * </p> 1761 */ 1762 @SearchParamDefinition(name="effective", path="MedicationStatement.effective.ofType(dateTime) | MedicationStatement.effective.ofType(Period)", description="Date when patient was taking (or not taking) the medication", type="date" ) 1763 public static final String SP_EFFECTIVE = "effective"; 1764 /** 1765 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 1766 * <p> 1767 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1768 * Type: <b>date</b><br> 1769 * Path: <b>MedicationStatement.effective.ofType(dateTime) | MedicationStatement.effective.ofType(Period)</b><br> 1770 * </p> 1771 */ 1772 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 1773 1774 /** 1775 * Search parameter: <b>source</b> 1776 * <p> 1777 * Description: <b>Who or where the information in the statement came from</b><br> 1778 * Type: <b>reference</b><br> 1779 * Path: <b>MedicationStatement.informationSource</b><br> 1780 * </p> 1781 */ 1782 @SearchParamDefinition(name="source", path="MedicationStatement.informationSource", description="Who or where the information in the statement came from", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1783 public static final String SP_SOURCE = "source"; 1784 /** 1785 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1786 * <p> 1787 * Description: <b>Who or where the information in the statement came from</b><br> 1788 * Type: <b>reference</b><br> 1789 * Path: <b>MedicationStatement.informationSource</b><br> 1790 * </p> 1791 */ 1792 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 1793 1794/** 1795 * Constant for fluent queries to be used to add include statements. Specifies 1796 * the path value of "<b>MedicationStatement:source</b>". 1797 */ 1798 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("MedicationStatement:source").toLocked(); 1799 1800 /** 1801 * Search parameter: <b>subject</b> 1802 * <p> 1803 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1804 * Type: <b>reference</b><br> 1805 * Path: <b>MedicationStatement.subject</b><br> 1806 * </p> 1807 */ 1808 @SearchParamDefinition(name="subject", path="MedicationStatement.subject", description="The identity of a patient, animal or group to list statements for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 1809 public static final String SP_SUBJECT = "subject"; 1810 /** 1811 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1812 * <p> 1813 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1814 * Type: <b>reference</b><br> 1815 * Path: <b>MedicationStatement.subject</b><br> 1816 * </p> 1817 */ 1818 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1819 1820/** 1821 * Constant for fluent queries to be used to add include statements. Specifies 1822 * the path value of "<b>MedicationStatement:subject</b>". 1823 */ 1824 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationStatement:subject").toLocked(); 1825 1826 /** 1827 * Search parameter: <b>code</b> 1828 * <p> 1829 * Description: <b>Multiple Resources: 1830 1831* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1832* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1833* [AuditEvent](auditevent.html): More specific code for the event 1834* [Basic](basic.html): Kind of Resource 1835* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1836* [Condition](condition.html): Code for the condition 1837* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1838* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1839* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1840* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1841* [ImagingSelection](imagingselection.html): The imaging selection status 1842* [List](list.html): What the purpose of this list is 1843* [Medication](medication.html): Returns medications for a specific code 1844* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1845* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1846* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1847* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1848* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1849* [Observation](observation.html): The code of the observation type 1850* [Procedure](procedure.html): A code to identify a procedure 1851* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1852* [Task](task.html): Search by task code 1853</b><br> 1854 * Type: <b>token</b><br> 1855 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1856 * </p> 1857 */ 1858 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 1859 public static final String SP_CODE = "code"; 1860 /** 1861 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1862 * <p> 1863 * Description: <b>Multiple Resources: 1864 1865* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1866* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1867* [AuditEvent](auditevent.html): More specific code for the event 1868* [Basic](basic.html): Kind of Resource 1869* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1870* [Condition](condition.html): Code for the condition 1871* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1872* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1873* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1874* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1875* [ImagingSelection](imagingselection.html): The imaging selection status 1876* [List](list.html): What the purpose of this list is 1877* [Medication](medication.html): Returns medications for a specific code 1878* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1879* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1880* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1881* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1882* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1883* [Observation](observation.html): The code of the observation type 1884* [Procedure](procedure.html): A code to identify a procedure 1885* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1886* [Task](task.html): Search by task code 1887</b><br> 1888 * Type: <b>token</b><br> 1889 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1890 * </p> 1891 */ 1892 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1893 1894 /** 1895 * Search parameter: <b>encounter</b> 1896 * <p> 1897 * Description: <b>Multiple Resources: 1898 1899* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1900* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1901* [ChargeItem](chargeitem.html): Encounter associated with event 1902* [Claim](claim.html): Encounters associated with a billed line item 1903* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1904* [Communication](communication.html): The Encounter during which this Communication was created 1905* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1906* [Composition](composition.html): Context of the Composition 1907* [Condition](condition.html): The Encounter during which this Condition was created 1908* [DeviceRequest](devicerequest.html): Encounter during which request was created 1909* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1910* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1911* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1912* [Flag](flag.html): Alert relevant during encounter 1913* [ImagingStudy](imagingstudy.html): The context of the study 1914* [List](list.html): Context in which list created 1915* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1916* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1917* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1918* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1919* [Observation](observation.html): Encounter related to the observation 1920* [Procedure](procedure.html): The Encounter during which this Procedure was created 1921* [Provenance](provenance.html): Encounter related to the Provenance 1922* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1923* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1924* [RiskAssessment](riskassessment.html): Where was assessment performed? 1925* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1926* [Task](task.html): Search by encounter 1927* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1928</b><br> 1929 * Type: <b>reference</b><br> 1930 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1931 * </p> 1932 */ 1933 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 1934 public static final String SP_ENCOUNTER = "encounter"; 1935 /** 1936 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1937 * <p> 1938 * Description: <b>Multiple Resources: 1939 1940* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1941* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1942* [ChargeItem](chargeitem.html): Encounter associated with event 1943* [Claim](claim.html): Encounters associated with a billed line item 1944* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1945* [Communication](communication.html): The Encounter during which this Communication was created 1946* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1947* [Composition](composition.html): Context of the Composition 1948* [Condition](condition.html): The Encounter during which this Condition was created 1949* [DeviceRequest](devicerequest.html): Encounter during which request was created 1950* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1951* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1952* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1953* [Flag](flag.html): Alert relevant during encounter 1954* [ImagingStudy](imagingstudy.html): The context of the study 1955* [List](list.html): Context in which list created 1956* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1957* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1958* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1959* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1960* [Observation](observation.html): Encounter related to the observation 1961* [Procedure](procedure.html): The Encounter during which this Procedure was created 1962* [Provenance](provenance.html): Encounter related to the Provenance 1963* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1964* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1965* [RiskAssessment](riskassessment.html): Where was assessment performed? 1966* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1967* [Task](task.html): Search by encounter 1968* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1969</b><br> 1970 * Type: <b>reference</b><br> 1971 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1972 * </p> 1973 */ 1974 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 1975 1976/** 1977 * Constant for fluent queries to be used to add include statements. Specifies 1978 * the path value of "<b>MedicationStatement:encounter</b>". 1979 */ 1980 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("MedicationStatement:encounter").toLocked(); 1981 1982 /** 1983 * Search parameter: <b>identifier</b> 1984 * <p> 1985 * Description: <b>Multiple Resources: 1986 1987* [Account](account.html): Account number 1988* [AdverseEvent](adverseevent.html): Business identifier for the event 1989* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1990* [Appointment](appointment.html): An Identifier of the Appointment 1991* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1992* [Basic](basic.html): Business identifier 1993* [BodyStructure](bodystructure.html): Bodystructure identifier 1994* [CarePlan](careplan.html): External Ids for this plan 1995* [CareTeam](careteam.html): External Ids for this team 1996* [ChargeItem](chargeitem.html): Business Identifier for item 1997* [Claim](claim.html): The primary identifier of the financial resource 1998* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1999* [ClinicalImpression](clinicalimpression.html): Business identifier 2000* [Communication](communication.html): Unique identifier 2001* [CommunicationRequest](communicationrequest.html): Unique identifier 2002* [Composition](composition.html): Version-independent identifier for the Composition 2003* [Condition](condition.html): A unique identifier of the condition record 2004* [Consent](consent.html): Identifier for this record (external references) 2005* [Contract](contract.html): The identity of the contract 2006* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2007* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2008* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2009* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2010* [DeviceRequest](devicerequest.html): Business identifier for request/order 2011* [DeviceUsage](deviceusage.html): Search by identifier 2012* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2013* [DocumentReference](documentreference.html): Identifier of the attachment binary 2014* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2015* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2016* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2017* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2018* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2019* [Flag](flag.html): Business identifier 2020* [Goal](goal.html): External Ids for this goal 2021* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2022* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2023* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2024* [Immunization](immunization.html): Business identifier 2025* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2026* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2027* [Invoice](invoice.html): Business Identifier for item 2028* [List](list.html): Business identifier 2029* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2030* [Medication](medication.html): Returns medications with this external identifier 2031* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2032* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2033* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2034* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2035* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2036* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2037* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2038* [Observation](observation.html): The unique id for a particular observation 2039* [Person](person.html): A person Identifier 2040* [Procedure](procedure.html): A unique identifier for a procedure 2041* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2042* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2043* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2044* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2045* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2046* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2047* [Specimen](specimen.html): The unique identifier associated with the specimen 2048* [SupplyDelivery](supplydelivery.html): External identifier 2049* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2050* [Task](task.html): Search for a task instance by its business identifier 2051* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2052</b><br> 2053 * Type: <b>token</b><br> 2054 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2055 * </p> 2056 */ 2057 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2058 public static final String SP_IDENTIFIER = "identifier"; 2059 /** 2060 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2061 * <p> 2062 * Description: <b>Multiple Resources: 2063 2064* [Account](account.html): Account number 2065* [AdverseEvent](adverseevent.html): Business identifier for the event 2066* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2067* [Appointment](appointment.html): An Identifier of the Appointment 2068* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2069* [Basic](basic.html): Business identifier 2070* [BodyStructure](bodystructure.html): Bodystructure identifier 2071* [CarePlan](careplan.html): External Ids for this plan 2072* [CareTeam](careteam.html): External Ids for this team 2073* [ChargeItem](chargeitem.html): Business Identifier for item 2074* [Claim](claim.html): The primary identifier of the financial resource 2075* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2076* [ClinicalImpression](clinicalimpression.html): Business identifier 2077* [Communication](communication.html): Unique identifier 2078* [CommunicationRequest](communicationrequest.html): Unique identifier 2079* [Composition](composition.html): Version-independent identifier for the Composition 2080* [Condition](condition.html): A unique identifier of the condition record 2081* [Consent](consent.html): Identifier for this record (external references) 2082* [Contract](contract.html): The identity of the contract 2083* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2084* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2085* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2086* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2087* [DeviceRequest](devicerequest.html): Business identifier for request/order 2088* [DeviceUsage](deviceusage.html): Search by identifier 2089* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2090* [DocumentReference](documentreference.html): Identifier of the attachment binary 2091* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2092* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2093* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2094* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2095* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2096* [Flag](flag.html): Business identifier 2097* [Goal](goal.html): External Ids for this goal 2098* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2099* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2100* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2101* [Immunization](immunization.html): Business identifier 2102* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2103* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2104* [Invoice](invoice.html): Business Identifier for item 2105* [List](list.html): Business identifier 2106* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2107* [Medication](medication.html): Returns medications with this external identifier 2108* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2109* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2110* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2111* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2112* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2113* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2114* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2115* [Observation](observation.html): The unique id for a particular observation 2116* [Person](person.html): A person Identifier 2117* [Procedure](procedure.html): A unique identifier for a procedure 2118* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2119* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2120* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2121* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2122* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2123* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2124* [Specimen](specimen.html): The unique identifier associated with the specimen 2125* [SupplyDelivery](supplydelivery.html): External identifier 2126* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2127* [Task](task.html): Search for a task instance by its business identifier 2128* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2129</b><br> 2130 * Type: <b>token</b><br> 2131 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2132 * </p> 2133 */ 2134 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2135 2136 /** 2137 * Search parameter: <b>patient</b> 2138 * <p> 2139 * Description: <b>Multiple Resources: 2140 2141* [Account](account.html): The entity that caused the expenses 2142* [AdverseEvent](adverseevent.html): Subject impacted by event 2143* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2144* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2145* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2146* [AuditEvent](auditevent.html): Where the activity involved patient data 2147* [Basic](basic.html): Identifies the focus of this resource 2148* [BodyStructure](bodystructure.html): Who this is about 2149* [CarePlan](careplan.html): Who the care plan is for 2150* [CareTeam](careteam.html): Who care team is for 2151* [ChargeItem](chargeitem.html): Individual service was done for/to 2152* [Claim](claim.html): Patient receiving the products or services 2153* [ClaimResponse](claimresponse.html): The subject of care 2154* [ClinicalImpression](clinicalimpression.html): Patient assessed 2155* [Communication](communication.html): Focus of message 2156* [CommunicationRequest](communicationrequest.html): Focus of message 2157* [Composition](composition.html): Who and/or what the composition is about 2158* [Condition](condition.html): Who has the condition? 2159* [Consent](consent.html): Who the consent applies to 2160* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2161* [Coverage](coverage.html): Retrieve coverages for a patient 2162* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2163* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2164* [DetectedIssue](detectedissue.html): Associated patient 2165* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2166* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2167* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2168* [DocumentReference](documentreference.html): Who/what is the subject of the document 2169* [Encounter](encounter.html): The patient present at the encounter 2170* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2171* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2172* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2173* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2174* [Flag](flag.html): The identity of a subject to list flags for 2175* [Goal](goal.html): Who this goal is intended for 2176* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2177* [ImagingSelection](imagingselection.html): Who the study is about 2178* [ImagingStudy](imagingstudy.html): Who the study is about 2179* [Immunization](immunization.html): The patient for the vaccination record 2180* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2181* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2182* [Invoice](invoice.html): Recipient(s) of goods and services 2183* [List](list.html): If all resources have the same subject 2184* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2185* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2186* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2187* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2188* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2189* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2190* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2191* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2192* [Observation](observation.html): The subject that the observation is about (if patient) 2193* [Person](person.html): The Person links to this Patient 2194* [Procedure](procedure.html): Search by subject - a patient 2195* [Provenance](provenance.html): Where the activity involved patient data 2196* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2197* [RelatedPerson](relatedperson.html): The patient this related person is related to 2198* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2199* [ResearchSubject](researchsubject.html): Who or what is part of study 2200* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2201* [ServiceRequest](servicerequest.html): Search by subject - a patient 2202* [Specimen](specimen.html): The patient the specimen comes from 2203* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2204* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2205* [Task](task.html): Search by patient 2206* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2207</b><br> 2208 * Type: <b>reference</b><br> 2209 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2210 * </p> 2211 */ 2212 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2213 public static final String SP_PATIENT = "patient"; 2214 /** 2215 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2216 * <p> 2217 * Description: <b>Multiple Resources: 2218 2219* [Account](account.html): The entity that caused the expenses 2220* [AdverseEvent](adverseevent.html): Subject impacted by event 2221* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2222* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2223* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2224* [AuditEvent](auditevent.html): Where the activity involved patient data 2225* [Basic](basic.html): Identifies the focus of this resource 2226* [BodyStructure](bodystructure.html): Who this is about 2227* [CarePlan](careplan.html): Who the care plan is for 2228* [CareTeam](careteam.html): Who care team is for 2229* [ChargeItem](chargeitem.html): Individual service was done for/to 2230* [Claim](claim.html): Patient receiving the products or services 2231* [ClaimResponse](claimresponse.html): The subject of care 2232* [ClinicalImpression](clinicalimpression.html): Patient assessed 2233* [Communication](communication.html): Focus of message 2234* [CommunicationRequest](communicationrequest.html): Focus of message 2235* [Composition](composition.html): Who and/or what the composition is about 2236* [Condition](condition.html): Who has the condition? 2237* [Consent](consent.html): Who the consent applies to 2238* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2239* [Coverage](coverage.html): Retrieve coverages for a patient 2240* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2241* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2242* [DetectedIssue](detectedissue.html): Associated patient 2243* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2244* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2245* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2246* [DocumentReference](documentreference.html): Who/what is the subject of the document 2247* [Encounter](encounter.html): The patient present at the encounter 2248* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2249* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2250* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2251* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2252* [Flag](flag.html): The identity of a subject to list flags for 2253* [Goal](goal.html): Who this goal is intended for 2254* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2255* [ImagingSelection](imagingselection.html): Who the study is about 2256* [ImagingStudy](imagingstudy.html): Who the study is about 2257* [Immunization](immunization.html): The patient for the vaccination record 2258* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2259* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2260* [Invoice](invoice.html): Recipient(s) of goods and services 2261* [List](list.html): If all resources have the same subject 2262* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2263* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2264* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2265* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2266* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2267* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2268* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2269* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2270* [Observation](observation.html): The subject that the observation is about (if patient) 2271* [Person](person.html): The Person links to this Patient 2272* [Procedure](procedure.html): Search by subject - a patient 2273* [Provenance](provenance.html): Where the activity involved patient data 2274* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2275* [RelatedPerson](relatedperson.html): The patient this related person is related to 2276* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2277* [ResearchSubject](researchsubject.html): Who or what is part of study 2278* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2279* [ServiceRequest](servicerequest.html): Search by subject - a patient 2280* [Specimen](specimen.html): The patient the specimen comes from 2281* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2282* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2283* [Task](task.html): Search by patient 2284* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2285</b><br> 2286 * Type: <b>reference</b><br> 2287 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2288 * </p> 2289 */ 2290 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2291 2292/** 2293 * Constant for fluent queries to be used to add include statements. Specifies 2294 * the path value of "<b>MedicationStatement:patient</b>". 2295 */ 2296 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationStatement:patient").toLocked(); 2297 2298 /** 2299 * Search parameter: <b>medication</b> 2300 * <p> 2301 * Description: <b>Multiple Resources: 2302 2303* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 2304* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 2305* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 2306* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 2307</b><br> 2308 * Type: <b>reference</b><br> 2309 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 2310 * </p> 2311 */ 2312 @SearchParamDefinition(name="medication", path="MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication reference\r\n", type="reference", target={Medication.class } ) 2313 public static final String SP_MEDICATION = "medication"; 2314 /** 2315 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 2316 * <p> 2317 * Description: <b>Multiple Resources: 2318 2319* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 2320* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 2321* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 2322* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 2323</b><br> 2324 * Type: <b>reference</b><br> 2325 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 2326 * </p> 2327 */ 2328 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 2329 2330/** 2331 * Constant for fluent queries to be used to add include statements. Specifies 2332 * the path value of "<b>MedicationStatement:medication</b>". 2333 */ 2334 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationStatement:medication").toLocked(); 2335 2336 /** 2337 * Search parameter: <b>status</b> 2338 * <p> 2339 * Description: <b>Multiple Resources: 2340 2341* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 2342* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 2343* [MedicationRequest](medicationrequest.html): Status of the prescription 2344* [MedicationStatement](medicationstatement.html): Return statements that match the given status 2345</b><br> 2346 * Type: <b>token</b><br> 2347 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 2348 * </p> 2349 */ 2350 @SearchParamDefinition(name="status", path="MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified)\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status\r\n* [MedicationRequest](medicationrequest.html): Status of the prescription\r\n* [MedicationStatement](medicationstatement.html): Return statements that match the given status\r\n", type="token" ) 2351 public static final String SP_STATUS = "status"; 2352 /** 2353 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2354 * <p> 2355 * Description: <b>Multiple Resources: 2356 2357* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 2358* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 2359* [MedicationRequest](medicationrequest.html): Status of the prescription 2360* [MedicationStatement](medicationstatement.html): Return statements that match the given status 2361</b><br> 2362 * Type: <b>token</b><br> 2363 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 2364 * </p> 2365 */ 2366 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2367 2368 2369} 2370