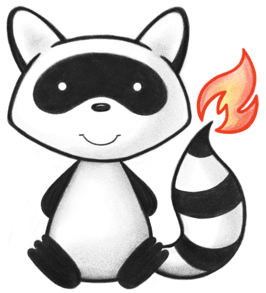
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. 052 053The primary difference between a medicationstatement and a medicationadministration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medicationstatement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the Medication Statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 054 */ 055@ResourceDef(name="MedicationStatement", profile="http://hl7.org/fhir/StructureDefinition/MedicationStatement") 056public class MedicationStatement extends DomainResource { 057 058 public enum MedicationStatementStatusCodes { 059 /** 060 * The action of recording the medication statement is finished. 061 */ 062 RECORDED, 063 /** 064 * Some of the actions that are implied by the medication usage may have occurred. For example, the patient may have taken some of the medication. Clinical decision support systems should take this status into account. 065 */ 066 ENTEREDINERROR, 067 /** 068 * The medication usage is draft or preliminary. 069 */ 070 DRAFT, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static MedicationStatementStatusCodes fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("recorded".equals(codeString)) 079 return RECORDED; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if ("draft".equals(codeString)) 083 return DRAFT; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown MedicationStatementStatusCodes code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case RECORDED: return "recorded"; 092 case ENTEREDINERROR: return "entered-in-error"; 093 case DRAFT: return "draft"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case RECORDED: return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 101 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 102 case DRAFT: return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case RECORDED: return "The action of recording the medication statement is finished."; 110 case ENTEREDINERROR: return "Some of the actions that are implied by the medication usage may have occurred. For example, the patient may have taken some of the medication. Clinical decision support systems should take this status into account."; 111 case DRAFT: return "The medication usage is draft or preliminary."; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDisplay() { 117 switch (this) { 118 case RECORDED: return "Recorded"; 119 case ENTEREDINERROR: return "Entered in Error"; 120 case DRAFT: return "Draft"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 } 126 127 public static class MedicationStatementStatusCodesEnumFactory implements EnumFactory<MedicationStatementStatusCodes> { 128 public MedicationStatementStatusCodes fromCode(String codeString) throws IllegalArgumentException { 129 if (codeString == null || "".equals(codeString)) 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("recorded".equals(codeString)) 133 return MedicationStatementStatusCodes.RECORDED; 134 if ("entered-in-error".equals(codeString)) 135 return MedicationStatementStatusCodes.ENTEREDINERROR; 136 if ("draft".equals(codeString)) 137 return MedicationStatementStatusCodes.DRAFT; 138 throw new IllegalArgumentException("Unknown MedicationStatementStatusCodes code '"+codeString+"'"); 139 } 140 public Enumeration<MedicationStatementStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 141 if (code == null) 142 return null; 143 if (code.isEmpty()) 144 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.NULL, code); 145 String codeString = ((PrimitiveType) code).asStringValue(); 146 if (codeString == null || "".equals(codeString)) 147 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.NULL, code); 148 if ("recorded".equals(codeString)) 149 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.RECORDED, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.ENTEREDINERROR, code); 152 if ("draft".equals(codeString)) 153 return new Enumeration<MedicationStatementStatusCodes>(this, MedicationStatementStatusCodes.DRAFT, code); 154 throw new FHIRException("Unknown MedicationStatementStatusCodes code '"+codeString+"'"); 155 } 156 public String toCode(MedicationStatementStatusCodes code) { 157 if (code == MedicationStatementStatusCodes.NULL) 158 return null; 159 if (code == MedicationStatementStatusCodes.RECORDED) 160 return "recorded"; 161 if (code == MedicationStatementStatusCodes.ENTEREDINERROR) 162 return "entered-in-error"; 163 if (code == MedicationStatementStatusCodes.DRAFT) 164 return "draft"; 165 return "?"; 166 } 167 public String toSystem(MedicationStatementStatusCodes code) { 168 return code.getSystem(); 169 } 170 } 171 172 @Block() 173 public static class MedicationStatementAdherenceComponent extends BackboneElement implements IBaseBackboneElement { 174 /** 175 * Type of the adherence for the medication. 176 */ 177 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 178 @Description(shortDefinition="Type of adherence", formalDefinition="Type of the adherence for the medication." ) 179 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-adherence") 180 protected CodeableConcept code; 181 182 /** 183 * Captures the reason for the current use or adherence of a medication. 184 */ 185 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 186 @Description(shortDefinition="Details of the reason for the current use of the medication", formalDefinition="Captures the reason for the current use or adherence of a medication." ) 187 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-status-codes") 188 protected CodeableConcept reason; 189 190 private static final long serialVersionUID = -1479626679L; 191 192 /** 193 * Constructor 194 */ 195 public MedicationStatementAdherenceComponent() { 196 super(); 197 } 198 199 /** 200 * Constructor 201 */ 202 public MedicationStatementAdherenceComponent(CodeableConcept code) { 203 super(); 204 this.setCode(code); 205 } 206 207 /** 208 * @return {@link #code} (Type of the adherence for the medication.) 209 */ 210 public CodeableConcept getCode() { 211 if (this.code == null) 212 if (Configuration.errorOnAutoCreate()) 213 throw new Error("Attempt to auto-create MedicationStatementAdherenceComponent.code"); 214 else if (Configuration.doAutoCreate()) 215 this.code = new CodeableConcept(); // cc 216 return this.code; 217 } 218 219 public boolean hasCode() { 220 return this.code != null && !this.code.isEmpty(); 221 } 222 223 /** 224 * @param value {@link #code} (Type of the adherence for the medication.) 225 */ 226 public MedicationStatementAdherenceComponent setCode(CodeableConcept value) { 227 this.code = value; 228 return this; 229 } 230 231 /** 232 * @return {@link #reason} (Captures the reason for the current use or adherence of a medication.) 233 */ 234 public CodeableConcept getReason() { 235 if (this.reason == null) 236 if (Configuration.errorOnAutoCreate()) 237 throw new Error("Attempt to auto-create MedicationStatementAdherenceComponent.reason"); 238 else if (Configuration.doAutoCreate()) 239 this.reason = new CodeableConcept(); // cc 240 return this.reason; 241 } 242 243 public boolean hasReason() { 244 return this.reason != null && !this.reason.isEmpty(); 245 } 246 247 /** 248 * @param value {@link #reason} (Captures the reason for the current use or adherence of a medication.) 249 */ 250 public MedicationStatementAdherenceComponent setReason(CodeableConcept value) { 251 this.reason = value; 252 return this; 253 } 254 255 protected void listChildren(List<Property> children) { 256 super.listChildren(children); 257 children.add(new Property("code", "CodeableConcept", "Type of the adherence for the medication.", 0, 1, code)); 258 children.add(new Property("reason", "CodeableConcept", "Captures the reason for the current use or adherence of a medication.", 0, 1, reason)); 259 } 260 261 @Override 262 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 263 switch (_hash) { 264 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Type of the adherence for the medication.", 0, 1, code); 265 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Captures the reason for the current use or adherence of a medication.", 0, 1, reason); 266 default: return super.getNamedProperty(_hash, _name, _checkValid); 267 } 268 269 } 270 271 @Override 272 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 273 switch (hash) { 274 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 275 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 276 default: return super.getProperty(hash, name, checkValid); 277 } 278 279 } 280 281 @Override 282 public Base setProperty(int hash, String name, Base value) throws FHIRException { 283 switch (hash) { 284 case 3059181: // code 285 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 286 return value; 287 case -934964668: // reason 288 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 289 return value; 290 default: return super.setProperty(hash, name, value); 291 } 292 293 } 294 295 @Override 296 public Base setProperty(String name, Base value) throws FHIRException { 297 if (name.equals("code")) { 298 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 299 } else if (name.equals("reason")) { 300 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 301 } else 302 return super.setProperty(name, value); 303 return value; 304 } 305 306 @Override 307 public void removeChild(String name, Base value) throws FHIRException { 308 if (name.equals("code")) { 309 this.code = null; 310 } else if (name.equals("reason")) { 311 this.reason = null; 312 } else 313 super.removeChild(name, value); 314 315 } 316 317 @Override 318 public Base makeProperty(int hash, String name) throws FHIRException { 319 switch (hash) { 320 case 3059181: return getCode(); 321 case -934964668: return getReason(); 322 default: return super.makeProperty(hash, name); 323 } 324 325 } 326 327 @Override 328 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 329 switch (hash) { 330 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 331 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 332 default: return super.getTypesForProperty(hash, name); 333 } 334 335 } 336 337 @Override 338 public Base addChild(String name) throws FHIRException { 339 if (name.equals("code")) { 340 this.code = new CodeableConcept(); 341 return this.code; 342 } 343 else if (name.equals("reason")) { 344 this.reason = new CodeableConcept(); 345 return this.reason; 346 } 347 else 348 return super.addChild(name); 349 } 350 351 public MedicationStatementAdherenceComponent copy() { 352 MedicationStatementAdherenceComponent dst = new MedicationStatementAdherenceComponent(); 353 copyValues(dst); 354 return dst; 355 } 356 357 public void copyValues(MedicationStatementAdherenceComponent dst) { 358 super.copyValues(dst); 359 dst.code = code == null ? null : code.copy(); 360 dst.reason = reason == null ? null : reason.copy(); 361 } 362 363 @Override 364 public boolean equalsDeep(Base other_) { 365 if (!super.equalsDeep(other_)) 366 return false; 367 if (!(other_ instanceof MedicationStatementAdherenceComponent)) 368 return false; 369 MedicationStatementAdherenceComponent o = (MedicationStatementAdherenceComponent) other_; 370 return compareDeep(code, o.code, true) && compareDeep(reason, o.reason, true); 371 } 372 373 @Override 374 public boolean equalsShallow(Base other_) { 375 if (!super.equalsShallow(other_)) 376 return false; 377 if (!(other_ instanceof MedicationStatementAdherenceComponent)) 378 return false; 379 MedicationStatementAdherenceComponent o = (MedicationStatementAdherenceComponent) other_; 380 return true; 381 } 382 383 public boolean isEmpty() { 384 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, reason); 385 } 386 387 public String fhirType() { 388 return "MedicationStatement.adherence"; 389 390 } 391 392 } 393 394 /** 395 * Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server. 396 */ 397 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 398 @Description(shortDefinition="External identifier", formalDefinition="Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server." ) 399 protected List<Identifier> identifier; 400 401 /** 402 * A larger event of which this particular MedicationStatement is a component or step. 403 */ 404 @Child(name = "partOf", type = {Procedure.class, MedicationStatement.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 405 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular MedicationStatement is a component or step." ) 406 protected List<Reference> partOf; 407 408 /** 409 * A code representing the status of recording the medication statement. 410 */ 411 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 412 @Description(shortDefinition="recorded | entered-in-error | draft", formalDefinition="A code representing the status of recording the medication statement." ) 413 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-status") 414 protected Enumeration<MedicationStatementStatusCodes> status; 415 416 /** 417 * Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.). 418 */ 419 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 420 @Description(shortDefinition="Type of medication statement", formalDefinition="Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.)." ) 421 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationrequest-admin-location") 422 protected List<CodeableConcept> category; 423 424 /** 425 * Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 426 */ 427 @Child(name = "medication", type = {CodeableReference.class}, order=4, min=1, max=1, modifier=false, summary=true) 428 @Description(shortDefinition="What medication was taken", formalDefinition="Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 429 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 430 protected CodeableReference medication; 431 432 /** 433 * The person, animal or group who is/was taking the medication. 434 */ 435 @Child(name = "subject", type = {Patient.class, Group.class}, order=5, min=1, max=1, modifier=false, summary=true) 436 @Description(shortDefinition="Who is/was taking the medication", formalDefinition="The person, animal or group who is/was taking the medication." ) 437 protected Reference subject; 438 439 /** 440 * The encounter that establishes the context for this MedicationStatement. 441 */ 442 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 443 @Description(shortDefinition="Encounter associated with MedicationStatement", formalDefinition="The encounter that establishes the context for this MedicationStatement." ) 444 protected Reference encounter; 445 446 /** 447 * The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking). 448 */ 449 @Child(name = "effective", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 450 @Description(shortDefinition="The date/time or interval when the medication is/was/will be taken", formalDefinition="The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking)." ) 451 protected DataType effective; 452 453 /** 454 * The date when the Medication Statement was asserted by the information source. 455 */ 456 @Child(name = "dateAsserted", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 457 @Description(shortDefinition="When the usage was asserted?", formalDefinition="The date when the Medication Statement was asserted by the information source." ) 458 protected DateTimeType dateAsserted; 459 460 /** 461 * The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest. 462 */ 463 @Child(name = "informationSource", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Organization.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 464 @Description(shortDefinition="Person or organization that provided the information about the taking of this medication", formalDefinition="The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest." ) 465 protected List<Reference> informationSource; 466 467 /** 468 * Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement. 469 */ 470 @Child(name = "derivedFrom", type = {Reference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 471 @Description(shortDefinition="Link to information used to derive the MedicationStatement", formalDefinition="Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement." ) 472 protected List<Reference> derivedFrom; 473 474 /** 475 * A concept, Condition or observation that supports why the medication is being/was taken. 476 */ 477 @Child(name = "reason", type = {CodeableReference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 478 @Description(shortDefinition="Reason for why the medication is being/was taken", formalDefinition="A concept, Condition or observation that supports why the medication is being/was taken." ) 479 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 480 protected List<CodeableReference> reason; 481 482 /** 483 * Provides extra information about the Medication Statement that is not conveyed by the other attributes. 484 */ 485 @Child(name = "note", type = {Annotation.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 486 @Description(shortDefinition="Further information about the usage", formalDefinition="Provides extra information about the Medication Statement that is not conveyed by the other attributes." ) 487 protected List<Annotation> note; 488 489 /** 490 * Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc. 491 */ 492 @Child(name = "relatedClinicalInformation", type = {Observation.class, Condition.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 493 @Description(shortDefinition="Link to information relevant to the usage of a medication", formalDefinition="Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc." ) 494 protected List<Reference> relatedClinicalInformation; 495 496 /** 497 * The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 498 */ 499 @Child(name = "renderedDosageInstruction", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 500 @Description(shortDefinition="Full representation of the dosage instructions", formalDefinition="The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses." ) 501 protected MarkdownType renderedDosageInstruction; 502 503 /** 504 * Indicates how the medication is/was or should be taken by the patient. 505 */ 506 @Child(name = "dosage", type = {Dosage.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 507 @Description(shortDefinition="Details of how medication is/was taken or should be taken", formalDefinition="Indicates how the medication is/was or should be taken by the patient." ) 508 protected List<Dosage> dosage; 509 510 /** 511 * Indicates whether the medication is or is not being consumed or administered. 512 */ 513 @Child(name = "adherence", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 514 @Description(shortDefinition="Indicates whether the medication is or is not being consumed or administered", formalDefinition="Indicates whether the medication is or is not being consumed or administered." ) 515 protected MedicationStatementAdherenceComponent adherence; 516 517 private static final long serialVersionUID = -1043616866L; 518 519 /** 520 * Constructor 521 */ 522 public MedicationStatement() { 523 super(); 524 } 525 526 /** 527 * Constructor 528 */ 529 public MedicationStatement(MedicationStatementStatusCodes status, CodeableReference medication, Reference subject) { 530 super(); 531 this.setStatus(status); 532 this.setMedication(medication); 533 this.setSubject(subject); 534 } 535 536 /** 537 * @return {@link #identifier} (Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.) 538 */ 539 public List<Identifier> getIdentifier() { 540 if (this.identifier == null) 541 this.identifier = new ArrayList<Identifier>(); 542 return this.identifier; 543 } 544 545 /** 546 * @return Returns a reference to <code>this</code> for easy method chaining 547 */ 548 public MedicationStatement setIdentifier(List<Identifier> theIdentifier) { 549 this.identifier = theIdentifier; 550 return this; 551 } 552 553 public boolean hasIdentifier() { 554 if (this.identifier == null) 555 return false; 556 for (Identifier item : this.identifier) 557 if (!item.isEmpty()) 558 return true; 559 return false; 560 } 561 562 public Identifier addIdentifier() { //3 563 Identifier t = new Identifier(); 564 if (this.identifier == null) 565 this.identifier = new ArrayList<Identifier>(); 566 this.identifier.add(t); 567 return t; 568 } 569 570 public MedicationStatement addIdentifier(Identifier t) { //3 571 if (t == null) 572 return this; 573 if (this.identifier == null) 574 this.identifier = new ArrayList<Identifier>(); 575 this.identifier.add(t); 576 return this; 577 } 578 579 /** 580 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 581 */ 582 public Identifier getIdentifierFirstRep() { 583 if (getIdentifier().isEmpty()) { 584 addIdentifier(); 585 } 586 return getIdentifier().get(0); 587 } 588 589 /** 590 * @return {@link #partOf} (A larger event of which this particular MedicationStatement is a component or step.) 591 */ 592 public List<Reference> getPartOf() { 593 if (this.partOf == null) 594 this.partOf = new ArrayList<Reference>(); 595 return this.partOf; 596 } 597 598 /** 599 * @return Returns a reference to <code>this</code> for easy method chaining 600 */ 601 public MedicationStatement setPartOf(List<Reference> thePartOf) { 602 this.partOf = thePartOf; 603 return this; 604 } 605 606 public boolean hasPartOf() { 607 if (this.partOf == null) 608 return false; 609 for (Reference item : this.partOf) 610 if (!item.isEmpty()) 611 return true; 612 return false; 613 } 614 615 public Reference addPartOf() { //3 616 Reference t = new Reference(); 617 if (this.partOf == null) 618 this.partOf = new ArrayList<Reference>(); 619 this.partOf.add(t); 620 return t; 621 } 622 623 public MedicationStatement addPartOf(Reference t) { //3 624 if (t == null) 625 return this; 626 if (this.partOf == null) 627 this.partOf = new ArrayList<Reference>(); 628 this.partOf.add(t); 629 return this; 630 } 631 632 /** 633 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 634 */ 635 public Reference getPartOfFirstRep() { 636 if (getPartOf().isEmpty()) { 637 addPartOf(); 638 } 639 return getPartOf().get(0); 640 } 641 642 /** 643 * @return {@link #status} (A code representing the status of recording the medication statement.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 644 */ 645 public Enumeration<MedicationStatementStatusCodes> getStatusElement() { 646 if (this.status == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create MedicationStatement.status"); 649 else if (Configuration.doAutoCreate()) 650 this.status = new Enumeration<MedicationStatementStatusCodes>(new MedicationStatementStatusCodesEnumFactory()); // bb 651 return this.status; 652 } 653 654 public boolean hasStatusElement() { 655 return this.status != null && !this.status.isEmpty(); 656 } 657 658 public boolean hasStatus() { 659 return this.status != null && !this.status.isEmpty(); 660 } 661 662 /** 663 * @param value {@link #status} (A code representing the status of recording the medication statement.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 664 */ 665 public MedicationStatement setStatusElement(Enumeration<MedicationStatementStatusCodes> value) { 666 this.status = value; 667 return this; 668 } 669 670 /** 671 * @return A code representing the status of recording the medication statement. 672 */ 673 public MedicationStatementStatusCodes getStatus() { 674 return this.status == null ? null : this.status.getValue(); 675 } 676 677 /** 678 * @param value A code representing the status of recording the medication statement. 679 */ 680 public MedicationStatement setStatus(MedicationStatementStatusCodes value) { 681 if (this.status == null) 682 this.status = new Enumeration<MedicationStatementStatusCodes>(new MedicationStatementStatusCodesEnumFactory()); 683 this.status.setValue(value); 684 return this; 685 } 686 687 /** 688 * @return {@link #category} (Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).) 689 */ 690 public List<CodeableConcept> getCategory() { 691 if (this.category == null) 692 this.category = new ArrayList<CodeableConcept>(); 693 return this.category; 694 } 695 696 /** 697 * @return Returns a reference to <code>this</code> for easy method chaining 698 */ 699 public MedicationStatement setCategory(List<CodeableConcept> theCategory) { 700 this.category = theCategory; 701 return this; 702 } 703 704 public boolean hasCategory() { 705 if (this.category == null) 706 return false; 707 for (CodeableConcept item : this.category) 708 if (!item.isEmpty()) 709 return true; 710 return false; 711 } 712 713 public CodeableConcept addCategory() { //3 714 CodeableConcept t = new CodeableConcept(); 715 if (this.category == null) 716 this.category = new ArrayList<CodeableConcept>(); 717 this.category.add(t); 718 return t; 719 } 720 721 public MedicationStatement addCategory(CodeableConcept t) { //3 722 if (t == null) 723 return this; 724 if (this.category == null) 725 this.category = new ArrayList<CodeableConcept>(); 726 this.category.add(t); 727 return this; 728 } 729 730 /** 731 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 732 */ 733 public CodeableConcept getCategoryFirstRep() { 734 if (getCategory().isEmpty()) { 735 addCategory(); 736 } 737 return getCategory().get(0); 738 } 739 740 /** 741 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 742 */ 743 public CodeableReference getMedication() { 744 if (this.medication == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create MedicationStatement.medication"); 747 else if (Configuration.doAutoCreate()) 748 this.medication = new CodeableReference(); // cc 749 return this.medication; 750 } 751 752 public boolean hasMedication() { 753 return this.medication != null && !this.medication.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 758 */ 759 public MedicationStatement setMedication(CodeableReference value) { 760 this.medication = value; 761 return this; 762 } 763 764 /** 765 * @return {@link #subject} (The person, animal or group who is/was taking the medication.) 766 */ 767 public Reference getSubject() { 768 if (this.subject == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create MedicationStatement.subject"); 771 else if (Configuration.doAutoCreate()) 772 this.subject = new Reference(); // cc 773 return this.subject; 774 } 775 776 public boolean hasSubject() { 777 return this.subject != null && !this.subject.isEmpty(); 778 } 779 780 /** 781 * @param value {@link #subject} (The person, animal or group who is/was taking the medication.) 782 */ 783 public MedicationStatement setSubject(Reference value) { 784 this.subject = value; 785 return this; 786 } 787 788 /** 789 * @return {@link #encounter} (The encounter that establishes the context for this MedicationStatement.) 790 */ 791 public Reference getEncounter() { 792 if (this.encounter == null) 793 if (Configuration.errorOnAutoCreate()) 794 throw new Error("Attempt to auto-create MedicationStatement.encounter"); 795 else if (Configuration.doAutoCreate()) 796 this.encounter = new Reference(); // cc 797 return this.encounter; 798 } 799 800 public boolean hasEncounter() { 801 return this.encounter != null && !this.encounter.isEmpty(); 802 } 803 804 /** 805 * @param value {@link #encounter} (The encounter that establishes the context for this MedicationStatement.) 806 */ 807 public MedicationStatement setEncounter(Reference value) { 808 this.encounter = value; 809 return this; 810 } 811 812 /** 813 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 814 */ 815 public DataType getEffective() { 816 return this.effective; 817 } 818 819 /** 820 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 821 */ 822 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 823 if (this.effective == null) 824 this.effective = new DateTimeType(); 825 if (!(this.effective instanceof DateTimeType)) 826 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 827 return (DateTimeType) this.effective; 828 } 829 830 public boolean hasEffectiveDateTimeType() { 831 return this != null && this.effective instanceof DateTimeType; 832 } 833 834 /** 835 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 836 */ 837 public Period getEffectivePeriod() throws FHIRException { 838 if (this.effective == null) 839 this.effective = new Period(); 840 if (!(this.effective instanceof Period)) 841 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 842 return (Period) this.effective; 843 } 844 845 public boolean hasEffectivePeriod() { 846 return this != null && this.effective instanceof Period; 847 } 848 849 /** 850 * @return {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 851 */ 852 public Timing getEffectiveTiming() throws FHIRException { 853 if (this.effective == null) 854 this.effective = new Timing(); 855 if (!(this.effective instanceof Timing)) 856 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.effective.getClass().getName()+" was encountered"); 857 return (Timing) this.effective; 858 } 859 860 public boolean hasEffectiveTiming() { 861 return this != null && this.effective instanceof Timing; 862 } 863 864 public boolean hasEffective() { 865 return this.effective != null && !this.effective.isEmpty(); 866 } 867 868 /** 869 * @param value {@link #effective} (The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).) 870 */ 871 public MedicationStatement setEffective(DataType value) { 872 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 873 throw new FHIRException("Not the right type for MedicationStatement.effective[x]: "+value.fhirType()); 874 this.effective = value; 875 return this; 876 } 877 878 /** 879 * @return {@link #dateAsserted} (The date when the Medication Statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 880 */ 881 public DateTimeType getDateAssertedElement() { 882 if (this.dateAsserted == null) 883 if (Configuration.errorOnAutoCreate()) 884 throw new Error("Attempt to auto-create MedicationStatement.dateAsserted"); 885 else if (Configuration.doAutoCreate()) 886 this.dateAsserted = new DateTimeType(); // bb 887 return this.dateAsserted; 888 } 889 890 public boolean hasDateAssertedElement() { 891 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 892 } 893 894 public boolean hasDateAsserted() { 895 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 896 } 897 898 /** 899 * @param value {@link #dateAsserted} (The date when the Medication Statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 900 */ 901 public MedicationStatement setDateAssertedElement(DateTimeType value) { 902 this.dateAsserted = value; 903 return this; 904 } 905 906 /** 907 * @return The date when the Medication Statement was asserted by the information source. 908 */ 909 public Date getDateAsserted() { 910 return this.dateAsserted == null ? null : this.dateAsserted.getValue(); 911 } 912 913 /** 914 * @param value The date when the Medication Statement was asserted by the information source. 915 */ 916 public MedicationStatement setDateAsserted(Date value) { 917 if (value == null) 918 this.dateAsserted = null; 919 else { 920 if (this.dateAsserted == null) 921 this.dateAsserted = new DateTimeType(); 922 this.dateAsserted.setValue(value); 923 } 924 return this; 925 } 926 927 /** 928 * @return {@link #informationSource} (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.) 929 */ 930 public List<Reference> getInformationSource() { 931 if (this.informationSource == null) 932 this.informationSource = new ArrayList<Reference>(); 933 return this.informationSource; 934 } 935 936 /** 937 * @return Returns a reference to <code>this</code> for easy method chaining 938 */ 939 public MedicationStatement setInformationSource(List<Reference> theInformationSource) { 940 this.informationSource = theInformationSource; 941 return this; 942 } 943 944 public boolean hasInformationSource() { 945 if (this.informationSource == null) 946 return false; 947 for (Reference item : this.informationSource) 948 if (!item.isEmpty()) 949 return true; 950 return false; 951 } 952 953 public Reference addInformationSource() { //3 954 Reference t = new Reference(); 955 if (this.informationSource == null) 956 this.informationSource = new ArrayList<Reference>(); 957 this.informationSource.add(t); 958 return t; 959 } 960 961 public MedicationStatement addInformationSource(Reference t) { //3 962 if (t == null) 963 return this; 964 if (this.informationSource == null) 965 this.informationSource = new ArrayList<Reference>(); 966 this.informationSource.add(t); 967 return this; 968 } 969 970 /** 971 * @return The first repetition of repeating field {@link #informationSource}, creating it if it does not already exist {3} 972 */ 973 public Reference getInformationSourceFirstRep() { 974 if (getInformationSource().isEmpty()) { 975 addInformationSource(); 976 } 977 return getInformationSource().get(0); 978 } 979 980 /** 981 * @return {@link #derivedFrom} (Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.) 982 */ 983 public List<Reference> getDerivedFrom() { 984 if (this.derivedFrom == null) 985 this.derivedFrom = new ArrayList<Reference>(); 986 return this.derivedFrom; 987 } 988 989 /** 990 * @return Returns a reference to <code>this</code> for easy method chaining 991 */ 992 public MedicationStatement setDerivedFrom(List<Reference> theDerivedFrom) { 993 this.derivedFrom = theDerivedFrom; 994 return this; 995 } 996 997 public boolean hasDerivedFrom() { 998 if (this.derivedFrom == null) 999 return false; 1000 for (Reference item : this.derivedFrom) 1001 if (!item.isEmpty()) 1002 return true; 1003 return false; 1004 } 1005 1006 public Reference addDerivedFrom() { //3 1007 Reference t = new Reference(); 1008 if (this.derivedFrom == null) 1009 this.derivedFrom = new ArrayList<Reference>(); 1010 this.derivedFrom.add(t); 1011 return t; 1012 } 1013 1014 public MedicationStatement addDerivedFrom(Reference t) { //3 1015 if (t == null) 1016 return this; 1017 if (this.derivedFrom == null) 1018 this.derivedFrom = new ArrayList<Reference>(); 1019 this.derivedFrom.add(t); 1020 return this; 1021 } 1022 1023 /** 1024 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 1025 */ 1026 public Reference getDerivedFromFirstRep() { 1027 if (getDerivedFrom().isEmpty()) { 1028 addDerivedFrom(); 1029 } 1030 return getDerivedFrom().get(0); 1031 } 1032 1033 /** 1034 * @return {@link #reason} (A concept, Condition or observation that supports why the medication is being/was taken.) 1035 */ 1036 public List<CodeableReference> getReason() { 1037 if (this.reason == null) 1038 this.reason = new ArrayList<CodeableReference>(); 1039 return this.reason; 1040 } 1041 1042 /** 1043 * @return Returns a reference to <code>this</code> for easy method chaining 1044 */ 1045 public MedicationStatement setReason(List<CodeableReference> theReason) { 1046 this.reason = theReason; 1047 return this; 1048 } 1049 1050 public boolean hasReason() { 1051 if (this.reason == null) 1052 return false; 1053 for (CodeableReference item : this.reason) 1054 if (!item.isEmpty()) 1055 return true; 1056 return false; 1057 } 1058 1059 public CodeableReference addReason() { //3 1060 CodeableReference t = new CodeableReference(); 1061 if (this.reason == null) 1062 this.reason = new ArrayList<CodeableReference>(); 1063 this.reason.add(t); 1064 return t; 1065 } 1066 1067 public MedicationStatement addReason(CodeableReference t) { //3 1068 if (t == null) 1069 return this; 1070 if (this.reason == null) 1071 this.reason = new ArrayList<CodeableReference>(); 1072 this.reason.add(t); 1073 return this; 1074 } 1075 1076 /** 1077 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1078 */ 1079 public CodeableReference getReasonFirstRep() { 1080 if (getReason().isEmpty()) { 1081 addReason(); 1082 } 1083 return getReason().get(0); 1084 } 1085 1086 /** 1087 * @return {@link #note} (Provides extra information about the Medication Statement that is not conveyed by the other attributes.) 1088 */ 1089 public List<Annotation> getNote() { 1090 if (this.note == null) 1091 this.note = new ArrayList<Annotation>(); 1092 return this.note; 1093 } 1094 1095 /** 1096 * @return Returns a reference to <code>this</code> for easy method chaining 1097 */ 1098 public MedicationStatement setNote(List<Annotation> theNote) { 1099 this.note = theNote; 1100 return this; 1101 } 1102 1103 public boolean hasNote() { 1104 if (this.note == null) 1105 return false; 1106 for (Annotation item : this.note) 1107 if (!item.isEmpty()) 1108 return true; 1109 return false; 1110 } 1111 1112 public Annotation addNote() { //3 1113 Annotation t = new Annotation(); 1114 if (this.note == null) 1115 this.note = new ArrayList<Annotation>(); 1116 this.note.add(t); 1117 return t; 1118 } 1119 1120 public MedicationStatement addNote(Annotation t) { //3 1121 if (t == null) 1122 return this; 1123 if (this.note == null) 1124 this.note = new ArrayList<Annotation>(); 1125 this.note.add(t); 1126 return this; 1127 } 1128 1129 /** 1130 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1131 */ 1132 public Annotation getNoteFirstRep() { 1133 if (getNote().isEmpty()) { 1134 addNote(); 1135 } 1136 return getNote().get(0); 1137 } 1138 1139 /** 1140 * @return {@link #relatedClinicalInformation} (Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc.) 1141 */ 1142 public List<Reference> getRelatedClinicalInformation() { 1143 if (this.relatedClinicalInformation == null) 1144 this.relatedClinicalInformation = new ArrayList<Reference>(); 1145 return this.relatedClinicalInformation; 1146 } 1147 1148 /** 1149 * @return Returns a reference to <code>this</code> for easy method chaining 1150 */ 1151 public MedicationStatement setRelatedClinicalInformation(List<Reference> theRelatedClinicalInformation) { 1152 this.relatedClinicalInformation = theRelatedClinicalInformation; 1153 return this; 1154 } 1155 1156 public boolean hasRelatedClinicalInformation() { 1157 if (this.relatedClinicalInformation == null) 1158 return false; 1159 for (Reference item : this.relatedClinicalInformation) 1160 if (!item.isEmpty()) 1161 return true; 1162 return false; 1163 } 1164 1165 public Reference addRelatedClinicalInformation() { //3 1166 Reference t = new Reference(); 1167 if (this.relatedClinicalInformation == null) 1168 this.relatedClinicalInformation = new ArrayList<Reference>(); 1169 this.relatedClinicalInformation.add(t); 1170 return t; 1171 } 1172 1173 public MedicationStatement addRelatedClinicalInformation(Reference t) { //3 1174 if (t == null) 1175 return this; 1176 if (this.relatedClinicalInformation == null) 1177 this.relatedClinicalInformation = new ArrayList<Reference>(); 1178 this.relatedClinicalInformation.add(t); 1179 return this; 1180 } 1181 1182 /** 1183 * @return The first repetition of repeating field {@link #relatedClinicalInformation}, creating it if it does not already exist {3} 1184 */ 1185 public Reference getRelatedClinicalInformationFirstRep() { 1186 if (getRelatedClinicalInformation().isEmpty()) { 1187 addRelatedClinicalInformation(); 1188 } 1189 return getRelatedClinicalInformation().get(0); 1190 } 1191 1192 /** 1193 * @return {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 1194 */ 1195 public MarkdownType getRenderedDosageInstructionElement() { 1196 if (this.renderedDosageInstruction == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create MedicationStatement.renderedDosageInstruction"); 1199 else if (Configuration.doAutoCreate()) 1200 this.renderedDosageInstruction = new MarkdownType(); // bb 1201 return this.renderedDosageInstruction; 1202 } 1203 1204 public boolean hasRenderedDosageInstructionElement() { 1205 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 1206 } 1207 1208 public boolean hasRenderedDosageInstruction() { 1209 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 1214 */ 1215 public MedicationStatement setRenderedDosageInstructionElement(MarkdownType value) { 1216 this.renderedDosageInstruction = value; 1217 return this; 1218 } 1219 1220 /** 1221 * @return The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 1222 */ 1223 public String getRenderedDosageInstruction() { 1224 return this.renderedDosageInstruction == null ? null : this.renderedDosageInstruction.getValue(); 1225 } 1226 1227 /** 1228 * @param value The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 1229 */ 1230 public MedicationStatement setRenderedDosageInstruction(String value) { 1231 if (Utilities.noString(value)) 1232 this.renderedDosageInstruction = null; 1233 else { 1234 if (this.renderedDosageInstruction == null) 1235 this.renderedDosageInstruction = new MarkdownType(); 1236 this.renderedDosageInstruction.setValue(value); 1237 } 1238 return this; 1239 } 1240 1241 /** 1242 * @return {@link #dosage} (Indicates how the medication is/was or should be taken by the patient.) 1243 */ 1244 public List<Dosage> getDosage() { 1245 if (this.dosage == null) 1246 this.dosage = new ArrayList<Dosage>(); 1247 return this.dosage; 1248 } 1249 1250 /** 1251 * @return Returns a reference to <code>this</code> for easy method chaining 1252 */ 1253 public MedicationStatement setDosage(List<Dosage> theDosage) { 1254 this.dosage = theDosage; 1255 return this; 1256 } 1257 1258 public boolean hasDosage() { 1259 if (this.dosage == null) 1260 return false; 1261 for (Dosage item : this.dosage) 1262 if (!item.isEmpty()) 1263 return true; 1264 return false; 1265 } 1266 1267 public Dosage addDosage() { //3 1268 Dosage t = new Dosage(); 1269 if (this.dosage == null) 1270 this.dosage = new ArrayList<Dosage>(); 1271 this.dosage.add(t); 1272 return t; 1273 } 1274 1275 public MedicationStatement addDosage(Dosage t) { //3 1276 if (t == null) 1277 return this; 1278 if (this.dosage == null) 1279 this.dosage = new ArrayList<Dosage>(); 1280 this.dosage.add(t); 1281 return this; 1282 } 1283 1284 /** 1285 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist {3} 1286 */ 1287 public Dosage getDosageFirstRep() { 1288 if (getDosage().isEmpty()) { 1289 addDosage(); 1290 } 1291 return getDosage().get(0); 1292 } 1293 1294 /** 1295 * @return {@link #adherence} (Indicates whether the medication is or is not being consumed or administered.) 1296 */ 1297 public MedicationStatementAdherenceComponent getAdherence() { 1298 if (this.adherence == null) 1299 if (Configuration.errorOnAutoCreate()) 1300 throw new Error("Attempt to auto-create MedicationStatement.adherence"); 1301 else if (Configuration.doAutoCreate()) 1302 this.adherence = new MedicationStatementAdherenceComponent(); // cc 1303 return this.adherence; 1304 } 1305 1306 public boolean hasAdherence() { 1307 return this.adherence != null && !this.adherence.isEmpty(); 1308 } 1309 1310 /** 1311 * @param value {@link #adherence} (Indicates whether the medication is or is not being consumed or administered.) 1312 */ 1313 public MedicationStatement setAdherence(MedicationStatementAdherenceComponent value) { 1314 this.adherence = value; 1315 return this; 1316 } 1317 1318 protected void listChildren(List<Property> children) { 1319 super.listChildren(children); 1320 children.add(new Property("identifier", "Identifier", "Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1321 children.add(new Property("partOf", "Reference(Procedure|MedicationStatement)", "A larger event of which this particular MedicationStatement is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1322 children.add(new Property("status", "code", "A code representing the status of recording the medication statement.", 0, 1, status)); 1323 children.add(new Property("category", "CodeableConcept", "Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).", 0, java.lang.Integer.MAX_VALUE, category)); 1324 children.add(new Property("medication", "CodeableReference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 1325 children.add(new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject)); 1326 children.add(new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this MedicationStatement.", 0, 1, encounter)); 1327 children.add(new Property("effective[x]", "dateTime|Period|Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective)); 1328 children.add(new Property("dateAsserted", "dateTime", "The date when the Medication Statement was asserted by the information source.", 0, 1, dateAsserted)); 1329 children.add(new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.", 0, java.lang.Integer.MAX_VALUE, informationSource)); 1330 children.add(new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1331 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "A concept, Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reason)); 1332 children.add(new Property("note", "Annotation", "Provides extra information about the Medication Statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 1333 children.add(new Property("relatedClinicalInformation", "Reference(Observation|Condition)", "Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc.", 0, java.lang.Integer.MAX_VALUE, relatedClinicalInformation)); 1334 children.add(new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction)); 1335 children.add(new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage)); 1336 children.add(new Property("adherence", "", "Indicates whether the medication is or is not being consumed or administered.", 0, 1, adherence)); 1337 } 1338 1339 @Override 1340 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1341 switch (_hash) { 1342 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1343 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure|MedicationStatement)", "A larger event of which this particular MedicationStatement is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1344 case -892481550: /*status*/ return new Property("status", "code", "A code representing the status of recording the medication statement.", 0, 1, status); 1345 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Type of medication statement (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).", 0, java.lang.Integer.MAX_VALUE, category); 1346 case 1998965455: /*medication*/ return new Property("medication", "CodeableReference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1347 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject); 1348 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this MedicationStatement.", 0, 1, encounter); 1349 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period|Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1350 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period|Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1351 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1352 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "Period", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1353 case -285872943: /*effectiveTiming*/ return new Property("effective[x]", "Timing", "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.adherence element is Not Taking).", 0, 1, effective); 1354 case -1980855245: /*dateAsserted*/ return new Property("dateAsserted", "dateTime", "The date when the Medication Statement was asserted by the information source.", 0, 1, dateAsserted); 1355 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.", 0, java.lang.Integer.MAX_VALUE, informationSource); 1356 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1357 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "A concept, Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reason); 1358 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides extra information about the Medication Statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 1359 case 1040787950: /*relatedClinicalInformation*/ return new Property("relatedClinicalInformation", "Reference(Observation|Condition)", "Link to information that is relevant to a medication statement, for example, illicit drug use, gestational age, etc.", 0, java.lang.Integer.MAX_VALUE, relatedClinicalInformation); 1360 case 1718902050: /*renderedDosageInstruction*/ return new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction); 1361 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage); 1362 case -231003683: /*adherence*/ return new Property("adherence", "", "Indicates whether the medication is or is not being consumed or administered.", 0, 1, adherence); 1363 default: return super.getNamedProperty(_hash, _name, _checkValid); 1364 } 1365 1366 } 1367 1368 @Override 1369 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1370 switch (hash) { 1371 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1372 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1373 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationStatementStatusCodes> 1374 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1375 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // CodeableReference 1376 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1377 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1378 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // DataType 1379 case -1980855245: /*dateAsserted*/ return this.dateAsserted == null ? new Base[0] : new Base[] {this.dateAsserted}; // DateTimeType 1380 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : this.informationSource.toArray(new Base[this.informationSource.size()]); // Reference 1381 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1382 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1383 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1384 case 1040787950: /*relatedClinicalInformation*/ return this.relatedClinicalInformation == null ? new Base[0] : this.relatedClinicalInformation.toArray(new Base[this.relatedClinicalInformation.size()]); // Reference 1385 case 1718902050: /*renderedDosageInstruction*/ return this.renderedDosageInstruction == null ? new Base[0] : new Base[] {this.renderedDosageInstruction}; // MarkdownType 1386 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 1387 case -231003683: /*adherence*/ return this.adherence == null ? new Base[0] : new Base[] {this.adherence}; // MedicationStatementAdherenceComponent 1388 default: return super.getProperty(hash, name, checkValid); 1389 } 1390 1391 } 1392 1393 @Override 1394 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1395 switch (hash) { 1396 case -1618432855: // identifier 1397 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1398 return value; 1399 case -995410646: // partOf 1400 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1401 return value; 1402 case -892481550: // status 1403 value = new MedicationStatementStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1404 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatusCodes> 1405 return value; 1406 case 50511102: // category 1407 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1408 return value; 1409 case 1998965455: // medication 1410 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 1411 return value; 1412 case -1867885268: // subject 1413 this.subject = TypeConvertor.castToReference(value); // Reference 1414 return value; 1415 case 1524132147: // encounter 1416 this.encounter = TypeConvertor.castToReference(value); // Reference 1417 return value; 1418 case -1468651097: // effective 1419 this.effective = TypeConvertor.castToType(value); // DataType 1420 return value; 1421 case -1980855245: // dateAsserted 1422 this.dateAsserted = TypeConvertor.castToDateTime(value); // DateTimeType 1423 return value; 1424 case -2123220889: // informationSource 1425 this.getInformationSource().add(TypeConvertor.castToReference(value)); // Reference 1426 return value; 1427 case 1077922663: // derivedFrom 1428 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 1429 return value; 1430 case -934964668: // reason 1431 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1432 return value; 1433 case 3387378: // note 1434 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1435 return value; 1436 case 1040787950: // relatedClinicalInformation 1437 this.getRelatedClinicalInformation().add(TypeConvertor.castToReference(value)); // Reference 1438 return value; 1439 case 1718902050: // renderedDosageInstruction 1440 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 1441 return value; 1442 case -1326018889: // dosage 1443 this.getDosage().add(TypeConvertor.castToDosage(value)); // Dosage 1444 return value; 1445 case -231003683: // adherence 1446 this.adherence = (MedicationStatementAdherenceComponent) value; // MedicationStatementAdherenceComponent 1447 return value; 1448 default: return super.setProperty(hash, name, value); 1449 } 1450 1451 } 1452 1453 @Override 1454 public Base setProperty(String name, Base value) throws FHIRException { 1455 if (name.equals("identifier")) { 1456 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1457 } else if (name.equals("partOf")) { 1458 this.getPartOf().add(TypeConvertor.castToReference(value)); 1459 } else if (name.equals("status")) { 1460 value = new MedicationStatementStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1461 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatusCodes> 1462 } else if (name.equals("category")) { 1463 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1464 } else if (name.equals("medication")) { 1465 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 1466 } else if (name.equals("subject")) { 1467 this.subject = TypeConvertor.castToReference(value); // Reference 1468 } else if (name.equals("encounter")) { 1469 this.encounter = TypeConvertor.castToReference(value); // Reference 1470 } else if (name.equals("effective[x]")) { 1471 this.effective = TypeConvertor.castToType(value); // DataType 1472 } else if (name.equals("dateAsserted")) { 1473 this.dateAsserted = TypeConvertor.castToDateTime(value); // DateTimeType 1474 } else if (name.equals("informationSource")) { 1475 this.getInformationSource().add(TypeConvertor.castToReference(value)); 1476 } else if (name.equals("derivedFrom")) { 1477 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 1478 } else if (name.equals("reason")) { 1479 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1480 } else if (name.equals("note")) { 1481 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1482 } else if (name.equals("relatedClinicalInformation")) { 1483 this.getRelatedClinicalInformation().add(TypeConvertor.castToReference(value)); 1484 } else if (name.equals("renderedDosageInstruction")) { 1485 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 1486 } else if (name.equals("dosage")) { 1487 this.getDosage().add(TypeConvertor.castToDosage(value)); 1488 } else if (name.equals("adherence")) { 1489 this.adherence = (MedicationStatementAdherenceComponent) value; // MedicationStatementAdherenceComponent 1490 } else 1491 return super.setProperty(name, value); 1492 return value; 1493 } 1494 1495 @Override 1496 public void removeChild(String name, Base value) throws FHIRException { 1497 if (name.equals("identifier")) { 1498 this.getIdentifier().remove(value); 1499 } else if (name.equals("partOf")) { 1500 this.getPartOf().remove(value); 1501 } else if (name.equals("status")) { 1502 value = new MedicationStatementStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1503 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatusCodes> 1504 } else if (name.equals("category")) { 1505 this.getCategory().remove(value); 1506 } else if (name.equals("medication")) { 1507 this.medication = null; 1508 } else if (name.equals("subject")) { 1509 this.subject = null; 1510 } else if (name.equals("encounter")) { 1511 this.encounter = null; 1512 } else if (name.equals("effective[x]")) { 1513 this.effective = null; 1514 } else if (name.equals("dateAsserted")) { 1515 this.dateAsserted = null; 1516 } else if (name.equals("informationSource")) { 1517 this.getInformationSource().remove(value); 1518 } else if (name.equals("derivedFrom")) { 1519 this.getDerivedFrom().remove(value); 1520 } else if (name.equals("reason")) { 1521 this.getReason().remove(value); 1522 } else if (name.equals("note")) { 1523 this.getNote().remove(value); 1524 } else if (name.equals("relatedClinicalInformation")) { 1525 this.getRelatedClinicalInformation().remove(value); 1526 } else if (name.equals("renderedDosageInstruction")) { 1527 this.renderedDosageInstruction = null; 1528 } else if (name.equals("dosage")) { 1529 this.getDosage().remove(value); 1530 } else if (name.equals("adherence")) { 1531 this.adherence = (MedicationStatementAdherenceComponent) value; // MedicationStatementAdherenceComponent 1532 } else 1533 super.removeChild(name, value); 1534 1535 } 1536 1537 @Override 1538 public Base makeProperty(int hash, String name) throws FHIRException { 1539 switch (hash) { 1540 case -1618432855: return addIdentifier(); 1541 case -995410646: return addPartOf(); 1542 case -892481550: return getStatusElement(); 1543 case 50511102: return addCategory(); 1544 case 1998965455: return getMedication(); 1545 case -1867885268: return getSubject(); 1546 case 1524132147: return getEncounter(); 1547 case 247104889: return getEffective(); 1548 case -1468651097: return getEffective(); 1549 case -1980855245: return getDateAssertedElement(); 1550 case -2123220889: return addInformationSource(); 1551 case 1077922663: return addDerivedFrom(); 1552 case -934964668: return addReason(); 1553 case 3387378: return addNote(); 1554 case 1040787950: return addRelatedClinicalInformation(); 1555 case 1718902050: return getRenderedDosageInstructionElement(); 1556 case -1326018889: return addDosage(); 1557 case -231003683: return getAdherence(); 1558 default: return super.makeProperty(hash, name); 1559 } 1560 1561 } 1562 1563 @Override 1564 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1565 switch (hash) { 1566 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1567 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1568 case -892481550: /*status*/ return new String[] {"code"}; 1569 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1570 case 1998965455: /*medication*/ return new String[] {"CodeableReference"}; 1571 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1572 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1573 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period", "Timing"}; 1574 case -1980855245: /*dateAsserted*/ return new String[] {"dateTime"}; 1575 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 1576 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 1577 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1578 case 3387378: /*note*/ return new String[] {"Annotation"}; 1579 case 1040787950: /*relatedClinicalInformation*/ return new String[] {"Reference"}; 1580 case 1718902050: /*renderedDosageInstruction*/ return new String[] {"markdown"}; 1581 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 1582 case -231003683: /*adherence*/ return new String[] {}; 1583 default: return super.getTypesForProperty(hash, name); 1584 } 1585 1586 } 1587 1588 @Override 1589 public Base addChild(String name) throws FHIRException { 1590 if (name.equals("identifier")) { 1591 return addIdentifier(); 1592 } 1593 else if (name.equals("partOf")) { 1594 return addPartOf(); 1595 } 1596 else if (name.equals("status")) { 1597 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.status"); 1598 } 1599 else if (name.equals("category")) { 1600 return addCategory(); 1601 } 1602 else if (name.equals("medication")) { 1603 this.medication = new CodeableReference(); 1604 return this.medication; 1605 } 1606 else if (name.equals("subject")) { 1607 this.subject = new Reference(); 1608 return this.subject; 1609 } 1610 else if (name.equals("encounter")) { 1611 this.encounter = new Reference(); 1612 return this.encounter; 1613 } 1614 else if (name.equals("effectiveDateTime")) { 1615 this.effective = new DateTimeType(); 1616 return this.effective; 1617 } 1618 else if (name.equals("effectivePeriod")) { 1619 this.effective = new Period(); 1620 return this.effective; 1621 } 1622 else if (name.equals("effectiveTiming")) { 1623 this.effective = new Timing(); 1624 return this.effective; 1625 } 1626 else if (name.equals("dateAsserted")) { 1627 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.dateAsserted"); 1628 } 1629 else if (name.equals("informationSource")) { 1630 return addInformationSource(); 1631 } 1632 else if (name.equals("derivedFrom")) { 1633 return addDerivedFrom(); 1634 } 1635 else if (name.equals("reason")) { 1636 return addReason(); 1637 } 1638 else if (name.equals("note")) { 1639 return addNote(); 1640 } 1641 else if (name.equals("relatedClinicalInformation")) { 1642 return addRelatedClinicalInformation(); 1643 } 1644 else if (name.equals("renderedDosageInstruction")) { 1645 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.renderedDosageInstruction"); 1646 } 1647 else if (name.equals("dosage")) { 1648 return addDosage(); 1649 } 1650 else if (name.equals("adherence")) { 1651 this.adherence = new MedicationStatementAdherenceComponent(); 1652 return this.adherence; 1653 } 1654 else 1655 return super.addChild(name); 1656 } 1657 1658 public String fhirType() { 1659 return "MedicationStatement"; 1660 1661 } 1662 1663 public MedicationStatement copy() { 1664 MedicationStatement dst = new MedicationStatement(); 1665 copyValues(dst); 1666 return dst; 1667 } 1668 1669 public void copyValues(MedicationStatement dst) { 1670 super.copyValues(dst); 1671 if (identifier != null) { 1672 dst.identifier = new ArrayList<Identifier>(); 1673 for (Identifier i : identifier) 1674 dst.identifier.add(i.copy()); 1675 }; 1676 if (partOf != null) { 1677 dst.partOf = new ArrayList<Reference>(); 1678 for (Reference i : partOf) 1679 dst.partOf.add(i.copy()); 1680 }; 1681 dst.status = status == null ? null : status.copy(); 1682 if (category != null) { 1683 dst.category = new ArrayList<CodeableConcept>(); 1684 for (CodeableConcept i : category) 1685 dst.category.add(i.copy()); 1686 }; 1687 dst.medication = medication == null ? null : medication.copy(); 1688 dst.subject = subject == null ? null : subject.copy(); 1689 dst.encounter = encounter == null ? null : encounter.copy(); 1690 dst.effective = effective == null ? null : effective.copy(); 1691 dst.dateAsserted = dateAsserted == null ? null : dateAsserted.copy(); 1692 if (informationSource != null) { 1693 dst.informationSource = new ArrayList<Reference>(); 1694 for (Reference i : informationSource) 1695 dst.informationSource.add(i.copy()); 1696 }; 1697 if (derivedFrom != null) { 1698 dst.derivedFrom = new ArrayList<Reference>(); 1699 for (Reference i : derivedFrom) 1700 dst.derivedFrom.add(i.copy()); 1701 }; 1702 if (reason != null) { 1703 dst.reason = new ArrayList<CodeableReference>(); 1704 for (CodeableReference i : reason) 1705 dst.reason.add(i.copy()); 1706 }; 1707 if (note != null) { 1708 dst.note = new ArrayList<Annotation>(); 1709 for (Annotation i : note) 1710 dst.note.add(i.copy()); 1711 }; 1712 if (relatedClinicalInformation != null) { 1713 dst.relatedClinicalInformation = new ArrayList<Reference>(); 1714 for (Reference i : relatedClinicalInformation) 1715 dst.relatedClinicalInformation.add(i.copy()); 1716 }; 1717 dst.renderedDosageInstruction = renderedDosageInstruction == null ? null : renderedDosageInstruction.copy(); 1718 if (dosage != null) { 1719 dst.dosage = new ArrayList<Dosage>(); 1720 for (Dosage i : dosage) 1721 dst.dosage.add(i.copy()); 1722 }; 1723 dst.adherence = adherence == null ? null : adherence.copy(); 1724 } 1725 1726 protected MedicationStatement typedCopy() { 1727 return copy(); 1728 } 1729 1730 @Override 1731 public boolean equalsDeep(Base other_) { 1732 if (!super.equalsDeep(other_)) 1733 return false; 1734 if (!(other_ instanceof MedicationStatement)) 1735 return false; 1736 MedicationStatement o = (MedicationStatement) other_; 1737 return compareDeep(identifier, o.identifier, true) && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 1738 && compareDeep(category, o.category, true) && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 1739 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) && compareDeep(dateAsserted, o.dateAsserted, true) 1740 && compareDeep(informationSource, o.informationSource, true) && compareDeep(derivedFrom, o.derivedFrom, true) 1741 && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) && compareDeep(relatedClinicalInformation, o.relatedClinicalInformation, true) 1742 && compareDeep(renderedDosageInstruction, o.renderedDosageInstruction, true) && compareDeep(dosage, o.dosage, true) 1743 && compareDeep(adherence, o.adherence, true); 1744 } 1745 1746 @Override 1747 public boolean equalsShallow(Base other_) { 1748 if (!super.equalsShallow(other_)) 1749 return false; 1750 if (!(other_ instanceof MedicationStatement)) 1751 return false; 1752 MedicationStatement o = (MedicationStatement) other_; 1753 return compareValues(status, o.status, true) && compareValues(dateAsserted, o.dateAsserted, true) && compareValues(renderedDosageInstruction, o.renderedDosageInstruction, true) 1754 ; 1755 } 1756 1757 public boolean isEmpty() { 1758 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, partOf, status 1759 , category, medication, subject, encounter, effective, dateAsserted, informationSource 1760 , derivedFrom, reason, note, relatedClinicalInformation, renderedDosageInstruction 1761 , dosage, adherence); 1762 } 1763 1764 @Override 1765 public ResourceType getResourceType() { 1766 return ResourceType.MedicationStatement; 1767 } 1768 1769 /** 1770 * Search parameter: <b>adherence</b> 1771 * <p> 1772 * Description: <b>Returns statements based on adherence or compliance</b><br> 1773 * Type: <b>token</b><br> 1774 * Path: <b>MedicationStatement.adherence.code</b><br> 1775 * </p> 1776 */ 1777 @SearchParamDefinition(name="adherence", path="MedicationStatement.adherence.code", description="Returns statements based on adherence or compliance", type="token" ) 1778 public static final String SP_ADHERENCE = "adherence"; 1779 /** 1780 * <b>Fluent Client</b> search parameter constant for <b>adherence</b> 1781 * <p> 1782 * Description: <b>Returns statements based on adherence or compliance</b><br> 1783 * Type: <b>token</b><br> 1784 * Path: <b>MedicationStatement.adherence.code</b><br> 1785 * </p> 1786 */ 1787 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADHERENCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADHERENCE); 1788 1789 /** 1790 * Search parameter: <b>category</b> 1791 * <p> 1792 * Description: <b>Returns statements of this category of MedicationStatement</b><br> 1793 * Type: <b>token</b><br> 1794 * Path: <b>MedicationStatement.category</b><br> 1795 * </p> 1796 */ 1797 @SearchParamDefinition(name="category", path="MedicationStatement.category", description="Returns statements of this category of MedicationStatement", type="token" ) 1798 public static final String SP_CATEGORY = "category"; 1799 /** 1800 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1801 * <p> 1802 * Description: <b>Returns statements of this category of MedicationStatement</b><br> 1803 * Type: <b>token</b><br> 1804 * Path: <b>MedicationStatement.category</b><br> 1805 * </p> 1806 */ 1807 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1808 1809 /** 1810 * Search parameter: <b>effective</b> 1811 * <p> 1812 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1813 * Type: <b>date</b><br> 1814 * Path: <b>MedicationStatement.effective.ofType(dateTime) | MedicationStatement.effective.ofType(Period)</b><br> 1815 * </p> 1816 */ 1817 @SearchParamDefinition(name="effective", path="MedicationStatement.effective.ofType(dateTime) | MedicationStatement.effective.ofType(Period)", description="Date when patient was taking (or not taking) the medication", type="date" ) 1818 public static final String SP_EFFECTIVE = "effective"; 1819 /** 1820 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 1821 * <p> 1822 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1823 * Type: <b>date</b><br> 1824 * Path: <b>MedicationStatement.effective.ofType(dateTime) | MedicationStatement.effective.ofType(Period)</b><br> 1825 * </p> 1826 */ 1827 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 1828 1829 /** 1830 * Search parameter: <b>source</b> 1831 * <p> 1832 * Description: <b>Who or where the information in the statement came from</b><br> 1833 * Type: <b>reference</b><br> 1834 * Path: <b>MedicationStatement.informationSource</b><br> 1835 * </p> 1836 */ 1837 @SearchParamDefinition(name="source", path="MedicationStatement.informationSource", description="Who or where the information in the statement came from", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1838 public static final String SP_SOURCE = "source"; 1839 /** 1840 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1841 * <p> 1842 * Description: <b>Who or where the information in the statement came from</b><br> 1843 * Type: <b>reference</b><br> 1844 * Path: <b>MedicationStatement.informationSource</b><br> 1845 * </p> 1846 */ 1847 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 1848 1849/** 1850 * Constant for fluent queries to be used to add include statements. Specifies 1851 * the path value of "<b>MedicationStatement:source</b>". 1852 */ 1853 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("MedicationStatement:source").toLocked(); 1854 1855 /** 1856 * Search parameter: <b>subject</b> 1857 * <p> 1858 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1859 * Type: <b>reference</b><br> 1860 * Path: <b>MedicationStatement.subject</b><br> 1861 * </p> 1862 */ 1863 @SearchParamDefinition(name="subject", path="MedicationStatement.subject", description="The identity of a patient, animal or group to list statements for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 1864 public static final String SP_SUBJECT = "subject"; 1865 /** 1866 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1867 * <p> 1868 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1869 * Type: <b>reference</b><br> 1870 * Path: <b>MedicationStatement.subject</b><br> 1871 * </p> 1872 */ 1873 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1874 1875/** 1876 * Constant for fluent queries to be used to add include statements. Specifies 1877 * the path value of "<b>MedicationStatement:subject</b>". 1878 */ 1879 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationStatement:subject").toLocked(); 1880 1881 /** 1882 * Search parameter: <b>code</b> 1883 * <p> 1884 * Description: <b>Multiple Resources: 1885 1886* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1887* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1888* [AuditEvent](auditevent.html): More specific code for the event 1889* [Basic](basic.html): Kind of Resource 1890* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1891* [Condition](condition.html): Code for the condition 1892* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1893* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1894* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1895* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1896* [ImagingSelection](imagingselection.html): The imaging selection status 1897* [List](list.html): What the purpose of this list is 1898* [Medication](medication.html): Returns medications for a specific code 1899* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1900* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1901* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1902* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1903* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1904* [Observation](observation.html): The code of the observation type 1905* [Procedure](procedure.html): A code to identify a procedure 1906* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1907* [Task](task.html): Search by task code 1908</b><br> 1909 * Type: <b>token</b><br> 1910 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1911 * </p> 1912 */ 1913 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 1914 public static final String SP_CODE = "code"; 1915 /** 1916 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1917 * <p> 1918 * Description: <b>Multiple Resources: 1919 1920* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1921* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1922* [AuditEvent](auditevent.html): More specific code for the event 1923* [Basic](basic.html): Kind of Resource 1924* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1925* [Condition](condition.html): Code for the condition 1926* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1927* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1928* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1929* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1930* [ImagingSelection](imagingselection.html): The imaging selection status 1931* [List](list.html): What the purpose of this list is 1932* [Medication](medication.html): Returns medications for a specific code 1933* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1934* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1935* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1936* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1937* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1938* [Observation](observation.html): The code of the observation type 1939* [Procedure](procedure.html): A code to identify a procedure 1940* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1941* [Task](task.html): Search by task code 1942</b><br> 1943 * Type: <b>token</b><br> 1944 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1945 * </p> 1946 */ 1947 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1948 1949 /** 1950 * Search parameter: <b>encounter</b> 1951 * <p> 1952 * Description: <b>Multiple Resources: 1953 1954* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1955* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1956* [ChargeItem](chargeitem.html): Encounter associated with event 1957* [Claim](claim.html): Encounters associated with a billed line item 1958* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1959* [Communication](communication.html): The Encounter during which this Communication was created 1960* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1961* [Composition](composition.html): Context of the Composition 1962* [Condition](condition.html): The Encounter during which this Condition was created 1963* [DeviceRequest](devicerequest.html): Encounter during which request was created 1964* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1965* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1966* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1967* [Flag](flag.html): Alert relevant during encounter 1968* [ImagingStudy](imagingstudy.html): The context of the study 1969* [List](list.html): Context in which list created 1970* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1971* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1972* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1973* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1974* [Observation](observation.html): Encounter related to the observation 1975* [Procedure](procedure.html): The Encounter during which this Procedure was created 1976* [Provenance](provenance.html): Encounter related to the Provenance 1977* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1978* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1979* [RiskAssessment](riskassessment.html): Where was assessment performed? 1980* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1981* [Task](task.html): Search by encounter 1982* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1983</b><br> 1984 * Type: <b>reference</b><br> 1985 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1986 * </p> 1987 */ 1988 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 1989 public static final String SP_ENCOUNTER = "encounter"; 1990 /** 1991 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1992 * <p> 1993 * Description: <b>Multiple Resources: 1994 1995* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1996* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1997* [ChargeItem](chargeitem.html): Encounter associated with event 1998* [Claim](claim.html): Encounters associated with a billed line item 1999* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2000* [Communication](communication.html): The Encounter during which this Communication was created 2001* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2002* [Composition](composition.html): Context of the Composition 2003* [Condition](condition.html): The Encounter during which this Condition was created 2004* [DeviceRequest](devicerequest.html): Encounter during which request was created 2005* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2006* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2007* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2008* [Flag](flag.html): Alert relevant during encounter 2009* [ImagingStudy](imagingstudy.html): The context of the study 2010* [List](list.html): Context in which list created 2011* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2012* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2013* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2014* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2015* [Observation](observation.html): Encounter related to the observation 2016* [Procedure](procedure.html): The Encounter during which this Procedure was created 2017* [Provenance](provenance.html): Encounter related to the Provenance 2018* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2019* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2020* [RiskAssessment](riskassessment.html): Where was assessment performed? 2021* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2022* [Task](task.html): Search by encounter 2023* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2024</b><br> 2025 * Type: <b>reference</b><br> 2026 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2027 * </p> 2028 */ 2029 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2030 2031/** 2032 * Constant for fluent queries to be used to add include statements. Specifies 2033 * the path value of "<b>MedicationStatement:encounter</b>". 2034 */ 2035 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("MedicationStatement:encounter").toLocked(); 2036 2037 /** 2038 * Search parameter: <b>identifier</b> 2039 * <p> 2040 * Description: <b>Multiple Resources: 2041 2042* [Account](account.html): Account number 2043* [AdverseEvent](adverseevent.html): Business identifier for the event 2044* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2045* [Appointment](appointment.html): An Identifier of the Appointment 2046* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2047* [Basic](basic.html): Business identifier 2048* [BodyStructure](bodystructure.html): Bodystructure identifier 2049* [CarePlan](careplan.html): External Ids for this plan 2050* [CareTeam](careteam.html): External Ids for this team 2051* [ChargeItem](chargeitem.html): Business Identifier for item 2052* [Claim](claim.html): The primary identifier of the financial resource 2053* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2054* [ClinicalImpression](clinicalimpression.html): Business identifier 2055* [Communication](communication.html): Unique identifier 2056* [CommunicationRequest](communicationrequest.html): Unique identifier 2057* [Composition](composition.html): Version-independent identifier for the Composition 2058* [Condition](condition.html): A unique identifier of the condition record 2059* [Consent](consent.html): Identifier for this record (external references) 2060* [Contract](contract.html): The identity of the contract 2061* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2062* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2063* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2064* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2065* [DeviceRequest](devicerequest.html): Business identifier for request/order 2066* [DeviceUsage](deviceusage.html): Search by identifier 2067* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2068* [DocumentReference](documentreference.html): Identifier of the attachment binary 2069* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2070* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2071* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2072* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2073* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2074* [Flag](flag.html): Business identifier 2075* [Goal](goal.html): External Ids for this goal 2076* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2077* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2078* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2079* [Immunization](immunization.html): Business identifier 2080* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2081* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2082* [Invoice](invoice.html): Business Identifier for item 2083* [List](list.html): Business identifier 2084* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2085* [Medication](medication.html): Returns medications with this external identifier 2086* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2087* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2088* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2089* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2090* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2091* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2092* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2093* [Observation](observation.html): The unique id for a particular observation 2094* [Person](person.html): A person Identifier 2095* [Procedure](procedure.html): A unique identifier for a procedure 2096* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2097* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2098* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2099* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2100* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2101* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2102* [Specimen](specimen.html): The unique identifier associated with the specimen 2103* [SupplyDelivery](supplydelivery.html): External identifier 2104* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2105* [Task](task.html): Search for a task instance by its business identifier 2106* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2107</b><br> 2108 * Type: <b>token</b><br> 2109 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2110 * </p> 2111 */ 2112 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2113 public static final String SP_IDENTIFIER = "identifier"; 2114 /** 2115 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2116 * <p> 2117 * Description: <b>Multiple Resources: 2118 2119* [Account](account.html): Account number 2120* [AdverseEvent](adverseevent.html): Business identifier for the event 2121* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2122* [Appointment](appointment.html): An Identifier of the Appointment 2123* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2124* [Basic](basic.html): Business identifier 2125* [BodyStructure](bodystructure.html): Bodystructure identifier 2126* [CarePlan](careplan.html): External Ids for this plan 2127* [CareTeam](careteam.html): External Ids for this team 2128* [ChargeItem](chargeitem.html): Business Identifier for item 2129* [Claim](claim.html): The primary identifier of the financial resource 2130* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2131* [ClinicalImpression](clinicalimpression.html): Business identifier 2132* [Communication](communication.html): Unique identifier 2133* [CommunicationRequest](communicationrequest.html): Unique identifier 2134* [Composition](composition.html): Version-independent identifier for the Composition 2135* [Condition](condition.html): A unique identifier of the condition record 2136* [Consent](consent.html): Identifier for this record (external references) 2137* [Contract](contract.html): The identity of the contract 2138* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2139* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2140* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2141* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2142* [DeviceRequest](devicerequest.html): Business identifier for request/order 2143* [DeviceUsage](deviceusage.html): Search by identifier 2144* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2145* [DocumentReference](documentreference.html): Identifier of the attachment binary 2146* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2147* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2148* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2149* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2150* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2151* [Flag](flag.html): Business identifier 2152* [Goal](goal.html): External Ids for this goal 2153* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2154* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2155* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2156* [Immunization](immunization.html): Business identifier 2157* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2158* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2159* [Invoice](invoice.html): Business Identifier for item 2160* [List](list.html): Business identifier 2161* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2162* [Medication](medication.html): Returns medications with this external identifier 2163* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2164* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2165* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2166* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2167* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2168* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2169* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2170* [Observation](observation.html): The unique id for a particular observation 2171* [Person](person.html): A person Identifier 2172* [Procedure](procedure.html): A unique identifier for a procedure 2173* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2174* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2175* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2176* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2177* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2178* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2179* [Specimen](specimen.html): The unique identifier associated with the specimen 2180* [SupplyDelivery](supplydelivery.html): External identifier 2181* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2182* [Task](task.html): Search for a task instance by its business identifier 2183* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2184</b><br> 2185 * Type: <b>token</b><br> 2186 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2187 * </p> 2188 */ 2189 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2190 2191 /** 2192 * Search parameter: <b>patient</b> 2193 * <p> 2194 * Description: <b>Multiple Resources: 2195 2196* [Account](account.html): The entity that caused the expenses 2197* [AdverseEvent](adverseevent.html): Subject impacted by event 2198* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2199* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2200* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2201* [AuditEvent](auditevent.html): Where the activity involved patient data 2202* [Basic](basic.html): Identifies the focus of this resource 2203* [BodyStructure](bodystructure.html): Who this is about 2204* [CarePlan](careplan.html): Who the care plan is for 2205* [CareTeam](careteam.html): Who care team is for 2206* [ChargeItem](chargeitem.html): Individual service was done for/to 2207* [Claim](claim.html): Patient receiving the products or services 2208* [ClaimResponse](claimresponse.html): The subject of care 2209* [ClinicalImpression](clinicalimpression.html): Patient assessed 2210* [Communication](communication.html): Focus of message 2211* [CommunicationRequest](communicationrequest.html): Focus of message 2212* [Composition](composition.html): Who and/or what the composition is about 2213* [Condition](condition.html): Who has the condition? 2214* [Consent](consent.html): Who the consent applies to 2215* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2216* [Coverage](coverage.html): Retrieve coverages for a patient 2217* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2218* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2219* [DetectedIssue](detectedissue.html): Associated patient 2220* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2221* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2222* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2223* [DocumentReference](documentreference.html): Who/what is the subject of the document 2224* [Encounter](encounter.html): The patient present at the encounter 2225* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2226* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2227* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2228* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2229* [Flag](flag.html): The identity of a subject to list flags for 2230* [Goal](goal.html): Who this goal is intended for 2231* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2232* [ImagingSelection](imagingselection.html): Who the study is about 2233* [ImagingStudy](imagingstudy.html): Who the study is about 2234* [Immunization](immunization.html): The patient for the vaccination record 2235* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2236* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2237* [Invoice](invoice.html): Recipient(s) of goods and services 2238* [List](list.html): If all resources have the same subject 2239* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2240* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2241* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2242* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2243* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2244* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2245* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2246* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2247* [Observation](observation.html): The subject that the observation is about (if patient) 2248* [Person](person.html): The Person links to this Patient 2249* [Procedure](procedure.html): Search by subject - a patient 2250* [Provenance](provenance.html): Where the activity involved patient data 2251* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2252* [RelatedPerson](relatedperson.html): The patient this related person is related to 2253* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2254* [ResearchSubject](researchsubject.html): Who or what is part of study 2255* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2256* [ServiceRequest](servicerequest.html): Search by subject - a patient 2257* [Specimen](specimen.html): The patient the specimen comes from 2258* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2259* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2260* [Task](task.html): Search by patient 2261* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2262</b><br> 2263 * Type: <b>reference</b><br> 2264 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2265 * </p> 2266 */ 2267 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2268 public static final String SP_PATIENT = "patient"; 2269 /** 2270 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2271 * <p> 2272 * Description: <b>Multiple Resources: 2273 2274* [Account](account.html): The entity that caused the expenses 2275* [AdverseEvent](adverseevent.html): Subject impacted by event 2276* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2277* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2278* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2279* [AuditEvent](auditevent.html): Where the activity involved patient data 2280* [Basic](basic.html): Identifies the focus of this resource 2281* [BodyStructure](bodystructure.html): Who this is about 2282* [CarePlan](careplan.html): Who the care plan is for 2283* [CareTeam](careteam.html): Who care team is for 2284* [ChargeItem](chargeitem.html): Individual service was done for/to 2285* [Claim](claim.html): Patient receiving the products or services 2286* [ClaimResponse](claimresponse.html): The subject of care 2287* [ClinicalImpression](clinicalimpression.html): Patient assessed 2288* [Communication](communication.html): Focus of message 2289* [CommunicationRequest](communicationrequest.html): Focus of message 2290* [Composition](composition.html): Who and/or what the composition is about 2291* [Condition](condition.html): Who has the condition? 2292* [Consent](consent.html): Who the consent applies to 2293* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2294* [Coverage](coverage.html): Retrieve coverages for a patient 2295* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2296* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2297* [DetectedIssue](detectedissue.html): Associated patient 2298* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2299* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2300* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2301* [DocumentReference](documentreference.html): Who/what is the subject of the document 2302* [Encounter](encounter.html): The patient present at the encounter 2303* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2304* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2305* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2306* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2307* [Flag](flag.html): The identity of a subject to list flags for 2308* [Goal](goal.html): Who this goal is intended for 2309* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2310* [ImagingSelection](imagingselection.html): Who the study is about 2311* [ImagingStudy](imagingstudy.html): Who the study is about 2312* [Immunization](immunization.html): The patient for the vaccination record 2313* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2314* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2315* [Invoice](invoice.html): Recipient(s) of goods and services 2316* [List](list.html): If all resources have the same subject 2317* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2318* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2319* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2320* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2321* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2322* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2323* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2324* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2325* [Observation](observation.html): The subject that the observation is about (if patient) 2326* [Person](person.html): The Person links to this Patient 2327* [Procedure](procedure.html): Search by subject - a patient 2328* [Provenance](provenance.html): Where the activity involved patient data 2329* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2330* [RelatedPerson](relatedperson.html): The patient this related person is related to 2331* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2332* [ResearchSubject](researchsubject.html): Who or what is part of study 2333* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2334* [ServiceRequest](servicerequest.html): Search by subject - a patient 2335* [Specimen](specimen.html): The patient the specimen comes from 2336* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2337* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2338* [Task](task.html): Search by patient 2339* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2340</b><br> 2341 * Type: <b>reference</b><br> 2342 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2343 * </p> 2344 */ 2345 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2346 2347/** 2348 * Constant for fluent queries to be used to add include statements. Specifies 2349 * the path value of "<b>MedicationStatement:patient</b>". 2350 */ 2351 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationStatement:patient").toLocked(); 2352 2353 /** 2354 * Search parameter: <b>medication</b> 2355 * <p> 2356 * Description: <b>Multiple Resources: 2357 2358* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 2359* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 2360* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 2361* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 2362</b><br> 2363 * Type: <b>reference</b><br> 2364 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 2365 * </p> 2366 */ 2367 @SearchParamDefinition(name="medication", path="MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication reference\r\n", type="reference", target={Medication.class } ) 2368 public static final String SP_MEDICATION = "medication"; 2369 /** 2370 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 2371 * <p> 2372 * Description: <b>Multiple Resources: 2373 2374* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 2375* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 2376* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 2377* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 2378</b><br> 2379 * Type: <b>reference</b><br> 2380 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 2381 * </p> 2382 */ 2383 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 2384 2385/** 2386 * Constant for fluent queries to be used to add include statements. Specifies 2387 * the path value of "<b>MedicationStatement:medication</b>". 2388 */ 2389 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationStatement:medication").toLocked(); 2390 2391 /** 2392 * Search parameter: <b>status</b> 2393 * <p> 2394 * Description: <b>Multiple Resources: 2395 2396* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 2397* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 2398* [MedicationRequest](medicationrequest.html): Status of the prescription 2399* [MedicationStatement](medicationstatement.html): Return statements that match the given status 2400</b><br> 2401 * Type: <b>token</b><br> 2402 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 2403 * </p> 2404 */ 2405 @SearchParamDefinition(name="status", path="MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified)\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status\r\n* [MedicationRequest](medicationrequest.html): Status of the prescription\r\n* [MedicationStatement](medicationstatement.html): Return statements that match the given status\r\n", type="token" ) 2406 public static final String SP_STATUS = "status"; 2407 /** 2408 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2409 * <p> 2410 * Description: <b>Multiple Resources: 2411 2412* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 2413* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 2414* [MedicationRequest](medicationrequest.html): Status of the prescription 2415* [MedicationStatement](medicationstatement.html): Return statements that match the given status 2416</b><br> 2417 * Type: <b>token</b><br> 2418 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 2419 * </p> 2420 */ 2421 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2422 2423 2424} 2425