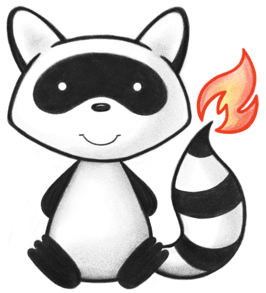
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use, drug catalogs, to support prescribing, adverse events management etc.). 052 */ 053@ResourceDef(name="MedicinalProductDefinition", profile="http://hl7.org/fhir/StructureDefinition/MedicinalProductDefinition") 054public class MedicinalProductDefinition extends DomainResource { 055 056 @Block() 057 public static class MedicinalProductDefinitionContactComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Allows the contact to be classified, for example QPPV, Pharmacovigilance Enquiry Information. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Allows the contact to be classified, for example QPPV, Pharmacovigilance Enquiry Information", formalDefinition="Allows the contact to be classified, for example QPPV, Pharmacovigilance Enquiry Information." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-contact-type") 064 protected CodeableConcept type; 065 066 /** 067 * A product specific contact, person (in a role), or an organization. 068 */ 069 @Child(name = "contact", type = {Organization.class, PractitionerRole.class}, order=2, min=1, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="A product specific contact, person (in a role), or an organization", formalDefinition="A product specific contact, person (in a role), or an organization." ) 071 protected Reference contact; 072 073 private static final long serialVersionUID = -587616244L; 074 075 /** 076 * Constructor 077 */ 078 public MedicinalProductDefinitionContactComponent() { 079 super(); 080 } 081 082 /** 083 * Constructor 084 */ 085 public MedicinalProductDefinitionContactComponent(Reference contact) { 086 super(); 087 this.setContact(contact); 088 } 089 090 /** 091 * @return {@link #type} (Allows the contact to be classified, for example QPPV, Pharmacovigilance Enquiry Information.) 092 */ 093 public CodeableConcept getType() { 094 if (this.type == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create MedicinalProductDefinitionContactComponent.type"); 097 else if (Configuration.doAutoCreate()) 098 this.type = new CodeableConcept(); // cc 099 return this.type; 100 } 101 102 public boolean hasType() { 103 return this.type != null && !this.type.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #type} (Allows the contact to be classified, for example QPPV, Pharmacovigilance Enquiry Information.) 108 */ 109 public MedicinalProductDefinitionContactComponent setType(CodeableConcept value) { 110 this.type = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #contact} (A product specific contact, person (in a role), or an organization.) 116 */ 117 public Reference getContact() { 118 if (this.contact == null) 119 if (Configuration.errorOnAutoCreate()) 120 throw new Error("Attempt to auto-create MedicinalProductDefinitionContactComponent.contact"); 121 else if (Configuration.doAutoCreate()) 122 this.contact = new Reference(); // cc 123 return this.contact; 124 } 125 126 public boolean hasContact() { 127 return this.contact != null && !this.contact.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #contact} (A product specific contact, person (in a role), or an organization.) 132 */ 133 public MedicinalProductDefinitionContactComponent setContact(Reference value) { 134 this.contact = value; 135 return this; 136 } 137 138 protected void listChildren(List<Property> children) { 139 super.listChildren(children); 140 children.add(new Property("type", "CodeableConcept", "Allows the contact to be classified, for example QPPV, Pharmacovigilance Enquiry Information.", 0, 1, type)); 141 children.add(new Property("contact", "Reference(Organization|PractitionerRole)", "A product specific contact, person (in a role), or an organization.", 0, 1, contact)); 142 } 143 144 @Override 145 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 146 switch (_hash) { 147 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Allows the contact to be classified, for example QPPV, Pharmacovigilance Enquiry Information.", 0, 1, type); 148 case 951526432: /*contact*/ return new Property("contact", "Reference(Organization|PractitionerRole)", "A product specific contact, person (in a role), or an organization.", 0, 1, contact); 149 default: return super.getNamedProperty(_hash, _name, _checkValid); 150 } 151 152 } 153 154 @Override 155 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 156 switch (hash) { 157 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 158 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : new Base[] {this.contact}; // Reference 159 default: return super.getProperty(hash, name, checkValid); 160 } 161 162 } 163 164 @Override 165 public Base setProperty(int hash, String name, Base value) throws FHIRException { 166 switch (hash) { 167 case 3575610: // type 168 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 169 return value; 170 case 951526432: // contact 171 this.contact = TypeConvertor.castToReference(value); // Reference 172 return value; 173 default: return super.setProperty(hash, name, value); 174 } 175 176 } 177 178 @Override 179 public Base setProperty(String name, Base value) throws FHIRException { 180 if (name.equals("type")) { 181 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 182 } else if (name.equals("contact")) { 183 this.contact = TypeConvertor.castToReference(value); // Reference 184 } else 185 return super.setProperty(name, value); 186 return value; 187 } 188 189 @Override 190 public void removeChild(String name, Base value) throws FHIRException { 191 if (name.equals("type")) { 192 this.type = null; 193 } else if (name.equals("contact")) { 194 this.contact = null; 195 } else 196 super.removeChild(name, value); 197 198 } 199 200 @Override 201 public Base makeProperty(int hash, String name) throws FHIRException { 202 switch (hash) { 203 case 3575610: return getType(); 204 case 951526432: return getContact(); 205 default: return super.makeProperty(hash, name); 206 } 207 208 } 209 210 @Override 211 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 212 switch (hash) { 213 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 214 case 951526432: /*contact*/ return new String[] {"Reference"}; 215 default: return super.getTypesForProperty(hash, name); 216 } 217 218 } 219 220 @Override 221 public Base addChild(String name) throws FHIRException { 222 if (name.equals("type")) { 223 this.type = new CodeableConcept(); 224 return this.type; 225 } 226 else if (name.equals("contact")) { 227 this.contact = new Reference(); 228 return this.contact; 229 } 230 else 231 return super.addChild(name); 232 } 233 234 public MedicinalProductDefinitionContactComponent copy() { 235 MedicinalProductDefinitionContactComponent dst = new MedicinalProductDefinitionContactComponent(); 236 copyValues(dst); 237 return dst; 238 } 239 240 public void copyValues(MedicinalProductDefinitionContactComponent dst) { 241 super.copyValues(dst); 242 dst.type = type == null ? null : type.copy(); 243 dst.contact = contact == null ? null : contact.copy(); 244 } 245 246 @Override 247 public boolean equalsDeep(Base other_) { 248 if (!super.equalsDeep(other_)) 249 return false; 250 if (!(other_ instanceof MedicinalProductDefinitionContactComponent)) 251 return false; 252 MedicinalProductDefinitionContactComponent o = (MedicinalProductDefinitionContactComponent) other_; 253 return compareDeep(type, o.type, true) && compareDeep(contact, o.contact, true); 254 } 255 256 @Override 257 public boolean equalsShallow(Base other_) { 258 if (!super.equalsShallow(other_)) 259 return false; 260 if (!(other_ instanceof MedicinalProductDefinitionContactComponent)) 261 return false; 262 MedicinalProductDefinitionContactComponent o = (MedicinalProductDefinitionContactComponent) other_; 263 return true; 264 } 265 266 public boolean isEmpty() { 267 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, contact); 268 } 269 270 public String fhirType() { 271 return "MedicinalProductDefinition.contact"; 272 273 } 274 275 } 276 277 @Block() 278 public static class MedicinalProductDefinitionNameComponent extends BackboneElement implements IBaseBackboneElement { 279 /** 280 * The full product name. 281 */ 282 @Child(name = "productName", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 283 @Description(shortDefinition="The full product name", formalDefinition="The full product name." ) 284 protected StringType productName; 285 286 /** 287 * Type of product name, such as rINN, BAN, Proprietary, Non-Proprietary. 288 */ 289 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 290 @Description(shortDefinition="Type of product name, such as rINN, BAN, Proprietary, Non-Proprietary", formalDefinition="Type of product name, such as rINN, BAN, Proprietary, Non-Proprietary." ) 291 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-name-type") 292 protected CodeableConcept type; 293 294 /** 295 * Coding words or phrases of the name. 296 */ 297 @Child(name = "part", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 298 @Description(shortDefinition="Coding words or phrases of the name", formalDefinition="Coding words or phrases of the name." ) 299 protected List<MedicinalProductDefinitionNamePartComponent> part; 300 301 /** 302 * Country and jurisdiction where the name applies, and associated language. 303 */ 304 @Child(name = "usage", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 305 @Description(shortDefinition="Country and jurisdiction where the name applies", formalDefinition="Country and jurisdiction where the name applies, and associated language." ) 306 protected List<MedicinalProductDefinitionNameUsageComponent> usage; 307 308 private static final long serialVersionUID = 890277480L; 309 310 /** 311 * Constructor 312 */ 313 public MedicinalProductDefinitionNameComponent() { 314 super(); 315 } 316 317 /** 318 * Constructor 319 */ 320 public MedicinalProductDefinitionNameComponent(String productName) { 321 super(); 322 this.setProductName(productName); 323 } 324 325 /** 326 * @return {@link #productName} (The full product name.). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 327 */ 328 public StringType getProductNameElement() { 329 if (this.productName == null) 330 if (Configuration.errorOnAutoCreate()) 331 throw new Error("Attempt to auto-create MedicinalProductDefinitionNameComponent.productName"); 332 else if (Configuration.doAutoCreate()) 333 this.productName = new StringType(); // bb 334 return this.productName; 335 } 336 337 public boolean hasProductNameElement() { 338 return this.productName != null && !this.productName.isEmpty(); 339 } 340 341 public boolean hasProductName() { 342 return this.productName != null && !this.productName.isEmpty(); 343 } 344 345 /** 346 * @param value {@link #productName} (The full product name.). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 347 */ 348 public MedicinalProductDefinitionNameComponent setProductNameElement(StringType value) { 349 this.productName = value; 350 return this; 351 } 352 353 /** 354 * @return The full product name. 355 */ 356 public String getProductName() { 357 return this.productName == null ? null : this.productName.getValue(); 358 } 359 360 /** 361 * @param value The full product name. 362 */ 363 public MedicinalProductDefinitionNameComponent setProductName(String value) { 364 if (this.productName == null) 365 this.productName = new StringType(); 366 this.productName.setValue(value); 367 return this; 368 } 369 370 /** 371 * @return {@link #type} (Type of product name, such as rINN, BAN, Proprietary, Non-Proprietary.) 372 */ 373 public CodeableConcept getType() { 374 if (this.type == null) 375 if (Configuration.errorOnAutoCreate()) 376 throw new Error("Attempt to auto-create MedicinalProductDefinitionNameComponent.type"); 377 else if (Configuration.doAutoCreate()) 378 this.type = new CodeableConcept(); // cc 379 return this.type; 380 } 381 382 public boolean hasType() { 383 return this.type != null && !this.type.isEmpty(); 384 } 385 386 /** 387 * @param value {@link #type} (Type of product name, such as rINN, BAN, Proprietary, Non-Proprietary.) 388 */ 389 public MedicinalProductDefinitionNameComponent setType(CodeableConcept value) { 390 this.type = value; 391 return this; 392 } 393 394 /** 395 * @return {@link #part} (Coding words or phrases of the name.) 396 */ 397 public List<MedicinalProductDefinitionNamePartComponent> getPart() { 398 if (this.part == null) 399 this.part = new ArrayList<MedicinalProductDefinitionNamePartComponent>(); 400 return this.part; 401 } 402 403 /** 404 * @return Returns a reference to <code>this</code> for easy method chaining 405 */ 406 public MedicinalProductDefinitionNameComponent setPart(List<MedicinalProductDefinitionNamePartComponent> thePart) { 407 this.part = thePart; 408 return this; 409 } 410 411 public boolean hasPart() { 412 if (this.part == null) 413 return false; 414 for (MedicinalProductDefinitionNamePartComponent item : this.part) 415 if (!item.isEmpty()) 416 return true; 417 return false; 418 } 419 420 public MedicinalProductDefinitionNamePartComponent addPart() { //3 421 MedicinalProductDefinitionNamePartComponent t = new MedicinalProductDefinitionNamePartComponent(); 422 if (this.part == null) 423 this.part = new ArrayList<MedicinalProductDefinitionNamePartComponent>(); 424 this.part.add(t); 425 return t; 426 } 427 428 public MedicinalProductDefinitionNameComponent addPart(MedicinalProductDefinitionNamePartComponent t) { //3 429 if (t == null) 430 return this; 431 if (this.part == null) 432 this.part = new ArrayList<MedicinalProductDefinitionNamePartComponent>(); 433 this.part.add(t); 434 return this; 435 } 436 437 /** 438 * @return The first repetition of repeating field {@link #part}, creating it if it does not already exist {3} 439 */ 440 public MedicinalProductDefinitionNamePartComponent getPartFirstRep() { 441 if (getPart().isEmpty()) { 442 addPart(); 443 } 444 return getPart().get(0); 445 } 446 447 /** 448 * @return {@link #usage} (Country and jurisdiction where the name applies, and associated language.) 449 */ 450 public List<MedicinalProductDefinitionNameUsageComponent> getUsage() { 451 if (this.usage == null) 452 this.usage = new ArrayList<MedicinalProductDefinitionNameUsageComponent>(); 453 return this.usage; 454 } 455 456 /** 457 * @return Returns a reference to <code>this</code> for easy method chaining 458 */ 459 public MedicinalProductDefinitionNameComponent setUsage(List<MedicinalProductDefinitionNameUsageComponent> theUsage) { 460 this.usage = theUsage; 461 return this; 462 } 463 464 public boolean hasUsage() { 465 if (this.usage == null) 466 return false; 467 for (MedicinalProductDefinitionNameUsageComponent item : this.usage) 468 if (!item.isEmpty()) 469 return true; 470 return false; 471 } 472 473 public MedicinalProductDefinitionNameUsageComponent addUsage() { //3 474 MedicinalProductDefinitionNameUsageComponent t = new MedicinalProductDefinitionNameUsageComponent(); 475 if (this.usage == null) 476 this.usage = new ArrayList<MedicinalProductDefinitionNameUsageComponent>(); 477 this.usage.add(t); 478 return t; 479 } 480 481 public MedicinalProductDefinitionNameComponent addUsage(MedicinalProductDefinitionNameUsageComponent t) { //3 482 if (t == null) 483 return this; 484 if (this.usage == null) 485 this.usage = new ArrayList<MedicinalProductDefinitionNameUsageComponent>(); 486 this.usage.add(t); 487 return this; 488 } 489 490 /** 491 * @return The first repetition of repeating field {@link #usage}, creating it if it does not already exist {3} 492 */ 493 public MedicinalProductDefinitionNameUsageComponent getUsageFirstRep() { 494 if (getUsage().isEmpty()) { 495 addUsage(); 496 } 497 return getUsage().get(0); 498 } 499 500 protected void listChildren(List<Property> children) { 501 super.listChildren(children); 502 children.add(new Property("productName", "string", "The full product name.", 0, 1, productName)); 503 children.add(new Property("type", "CodeableConcept", "Type of product name, such as rINN, BAN, Proprietary, Non-Proprietary.", 0, 1, type)); 504 children.add(new Property("part", "", "Coding words or phrases of the name.", 0, java.lang.Integer.MAX_VALUE, part)); 505 children.add(new Property("usage", "", "Country and jurisdiction where the name applies, and associated language.", 0, java.lang.Integer.MAX_VALUE, usage)); 506 } 507 508 @Override 509 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 510 switch (_hash) { 511 case -1491817446: /*productName*/ return new Property("productName", "string", "The full product name.", 0, 1, productName); 512 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of product name, such as rINN, BAN, Proprietary, Non-Proprietary.", 0, 1, type); 513 case 3433459: /*part*/ return new Property("part", "", "Coding words or phrases of the name.", 0, java.lang.Integer.MAX_VALUE, part); 514 case 111574433: /*usage*/ return new Property("usage", "", "Country and jurisdiction where the name applies, and associated language.", 0, java.lang.Integer.MAX_VALUE, usage); 515 default: return super.getNamedProperty(_hash, _name, _checkValid); 516 } 517 518 } 519 520 @Override 521 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 522 switch (hash) { 523 case -1491817446: /*productName*/ return this.productName == null ? new Base[0] : new Base[] {this.productName}; // StringType 524 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 525 case 3433459: /*part*/ return this.part == null ? new Base[0] : this.part.toArray(new Base[this.part.size()]); // MedicinalProductDefinitionNamePartComponent 526 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : this.usage.toArray(new Base[this.usage.size()]); // MedicinalProductDefinitionNameUsageComponent 527 default: return super.getProperty(hash, name, checkValid); 528 } 529 530 } 531 532 @Override 533 public Base setProperty(int hash, String name, Base value) throws FHIRException { 534 switch (hash) { 535 case -1491817446: // productName 536 this.productName = TypeConvertor.castToString(value); // StringType 537 return value; 538 case 3575610: // type 539 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 540 return value; 541 case 3433459: // part 542 this.getPart().add((MedicinalProductDefinitionNamePartComponent) value); // MedicinalProductDefinitionNamePartComponent 543 return value; 544 case 111574433: // usage 545 this.getUsage().add((MedicinalProductDefinitionNameUsageComponent) value); // MedicinalProductDefinitionNameUsageComponent 546 return value; 547 default: return super.setProperty(hash, name, value); 548 } 549 550 } 551 552 @Override 553 public Base setProperty(String name, Base value) throws FHIRException { 554 if (name.equals("productName")) { 555 this.productName = TypeConvertor.castToString(value); // StringType 556 } else if (name.equals("type")) { 557 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 558 } else if (name.equals("part")) { 559 this.getPart().add((MedicinalProductDefinitionNamePartComponent) value); 560 } else if (name.equals("usage")) { 561 this.getUsage().add((MedicinalProductDefinitionNameUsageComponent) value); 562 } else 563 return super.setProperty(name, value); 564 return value; 565 } 566 567 @Override 568 public void removeChild(String name, Base value) throws FHIRException { 569 if (name.equals("productName")) { 570 this.productName = null; 571 } else if (name.equals("type")) { 572 this.type = null; 573 } else if (name.equals("part")) { 574 this.getPart().remove((MedicinalProductDefinitionNamePartComponent) value); 575 } else if (name.equals("usage")) { 576 this.getUsage().remove((MedicinalProductDefinitionNameUsageComponent) value); 577 } else 578 super.removeChild(name, value); 579 580 } 581 582 @Override 583 public Base makeProperty(int hash, String name) throws FHIRException { 584 switch (hash) { 585 case -1491817446: return getProductNameElement(); 586 case 3575610: return getType(); 587 case 3433459: return addPart(); 588 case 111574433: return addUsage(); 589 default: return super.makeProperty(hash, name); 590 } 591 592 } 593 594 @Override 595 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 596 switch (hash) { 597 case -1491817446: /*productName*/ return new String[] {"string"}; 598 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 599 case 3433459: /*part*/ return new String[] {}; 600 case 111574433: /*usage*/ return new String[] {}; 601 default: return super.getTypesForProperty(hash, name); 602 } 603 604 } 605 606 @Override 607 public Base addChild(String name) throws FHIRException { 608 if (name.equals("productName")) { 609 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductDefinition.name.productName"); 610 } 611 else if (name.equals("type")) { 612 this.type = new CodeableConcept(); 613 return this.type; 614 } 615 else if (name.equals("part")) { 616 return addPart(); 617 } 618 else if (name.equals("usage")) { 619 return addUsage(); 620 } 621 else 622 return super.addChild(name); 623 } 624 625 public MedicinalProductDefinitionNameComponent copy() { 626 MedicinalProductDefinitionNameComponent dst = new MedicinalProductDefinitionNameComponent(); 627 copyValues(dst); 628 return dst; 629 } 630 631 public void copyValues(MedicinalProductDefinitionNameComponent dst) { 632 super.copyValues(dst); 633 dst.productName = productName == null ? null : productName.copy(); 634 dst.type = type == null ? null : type.copy(); 635 if (part != null) { 636 dst.part = new ArrayList<MedicinalProductDefinitionNamePartComponent>(); 637 for (MedicinalProductDefinitionNamePartComponent i : part) 638 dst.part.add(i.copy()); 639 }; 640 if (usage != null) { 641 dst.usage = new ArrayList<MedicinalProductDefinitionNameUsageComponent>(); 642 for (MedicinalProductDefinitionNameUsageComponent i : usage) 643 dst.usage.add(i.copy()); 644 }; 645 } 646 647 @Override 648 public boolean equalsDeep(Base other_) { 649 if (!super.equalsDeep(other_)) 650 return false; 651 if (!(other_ instanceof MedicinalProductDefinitionNameComponent)) 652 return false; 653 MedicinalProductDefinitionNameComponent o = (MedicinalProductDefinitionNameComponent) other_; 654 return compareDeep(productName, o.productName, true) && compareDeep(type, o.type, true) && compareDeep(part, o.part, true) 655 && compareDeep(usage, o.usage, true); 656 } 657 658 @Override 659 public boolean equalsShallow(Base other_) { 660 if (!super.equalsShallow(other_)) 661 return false; 662 if (!(other_ instanceof MedicinalProductDefinitionNameComponent)) 663 return false; 664 MedicinalProductDefinitionNameComponent o = (MedicinalProductDefinitionNameComponent) other_; 665 return compareValues(productName, o.productName, true); 666 } 667 668 public boolean isEmpty() { 669 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productName, type, part 670 , usage); 671 } 672 673 public String fhirType() { 674 return "MedicinalProductDefinition.name"; 675 676 } 677 678 } 679 680 @Block() 681 public static class MedicinalProductDefinitionNamePartComponent extends BackboneElement implements IBaseBackboneElement { 682 /** 683 * A fragment of a product name. 684 */ 685 @Child(name = "part", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 686 @Description(shortDefinition="A fragment of a product name", formalDefinition="A fragment of a product name." ) 687 protected StringType part; 688 689 /** 690 * Identifying type for this part of the name (e.g. strength part). 691 */ 692 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 693 @Description(shortDefinition="Identifying type for this part of the name (e.g. strength part)", formalDefinition="Identifying type for this part of the name (e.g. strength part)." ) 694 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-name-part-type") 695 protected CodeableConcept type; 696 697 private static final long serialVersionUID = -1359126549L; 698 699 /** 700 * Constructor 701 */ 702 public MedicinalProductDefinitionNamePartComponent() { 703 super(); 704 } 705 706 /** 707 * Constructor 708 */ 709 public MedicinalProductDefinitionNamePartComponent(String part, CodeableConcept type) { 710 super(); 711 this.setPart(part); 712 this.setType(type); 713 } 714 715 /** 716 * @return {@link #part} (A fragment of a product name.). This is the underlying object with id, value and extensions. The accessor "getPart" gives direct access to the value 717 */ 718 public StringType getPartElement() { 719 if (this.part == null) 720 if (Configuration.errorOnAutoCreate()) 721 throw new Error("Attempt to auto-create MedicinalProductDefinitionNamePartComponent.part"); 722 else if (Configuration.doAutoCreate()) 723 this.part = new StringType(); // bb 724 return this.part; 725 } 726 727 public boolean hasPartElement() { 728 return this.part != null && !this.part.isEmpty(); 729 } 730 731 public boolean hasPart() { 732 return this.part != null && !this.part.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #part} (A fragment of a product name.). This is the underlying object with id, value and extensions. The accessor "getPart" gives direct access to the value 737 */ 738 public MedicinalProductDefinitionNamePartComponent setPartElement(StringType value) { 739 this.part = value; 740 return this; 741 } 742 743 /** 744 * @return A fragment of a product name. 745 */ 746 public String getPart() { 747 return this.part == null ? null : this.part.getValue(); 748 } 749 750 /** 751 * @param value A fragment of a product name. 752 */ 753 public MedicinalProductDefinitionNamePartComponent setPart(String value) { 754 if (this.part == null) 755 this.part = new StringType(); 756 this.part.setValue(value); 757 return this; 758 } 759 760 /** 761 * @return {@link #type} (Identifying type for this part of the name (e.g. strength part).) 762 */ 763 public CodeableConcept getType() { 764 if (this.type == null) 765 if (Configuration.errorOnAutoCreate()) 766 throw new Error("Attempt to auto-create MedicinalProductDefinitionNamePartComponent.type"); 767 else if (Configuration.doAutoCreate()) 768 this.type = new CodeableConcept(); // cc 769 return this.type; 770 } 771 772 public boolean hasType() { 773 return this.type != null && !this.type.isEmpty(); 774 } 775 776 /** 777 * @param value {@link #type} (Identifying type for this part of the name (e.g. strength part).) 778 */ 779 public MedicinalProductDefinitionNamePartComponent setType(CodeableConcept value) { 780 this.type = value; 781 return this; 782 } 783 784 protected void listChildren(List<Property> children) { 785 super.listChildren(children); 786 children.add(new Property("part", "string", "A fragment of a product name.", 0, 1, part)); 787 children.add(new Property("type", "CodeableConcept", "Identifying type for this part of the name (e.g. strength part).", 0, 1, type)); 788 } 789 790 @Override 791 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 792 switch (_hash) { 793 case 3433459: /*part*/ return new Property("part", "string", "A fragment of a product name.", 0, 1, part); 794 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Identifying type for this part of the name (e.g. strength part).", 0, 1, type); 795 default: return super.getNamedProperty(_hash, _name, _checkValid); 796 } 797 798 } 799 800 @Override 801 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 802 switch (hash) { 803 case 3433459: /*part*/ return this.part == null ? new Base[0] : new Base[] {this.part}; // StringType 804 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 805 default: return super.getProperty(hash, name, checkValid); 806 } 807 808 } 809 810 @Override 811 public Base setProperty(int hash, String name, Base value) throws FHIRException { 812 switch (hash) { 813 case 3433459: // part 814 this.part = TypeConvertor.castToString(value); // StringType 815 return value; 816 case 3575610: // type 817 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 818 return value; 819 default: return super.setProperty(hash, name, value); 820 } 821 822 } 823 824 @Override 825 public Base setProperty(String name, Base value) throws FHIRException { 826 if (name.equals("part")) { 827 this.part = TypeConvertor.castToString(value); // StringType 828 } else if (name.equals("type")) { 829 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 830 } else 831 return super.setProperty(name, value); 832 return value; 833 } 834 835 @Override 836 public void removeChild(String name, Base value) throws FHIRException { 837 if (name.equals("part")) { 838 this.part = null; 839 } else if (name.equals("type")) { 840 this.type = null; 841 } else 842 super.removeChild(name, value); 843 844 } 845 846 @Override 847 public Base makeProperty(int hash, String name) throws FHIRException { 848 switch (hash) { 849 case 3433459: return getPartElement(); 850 case 3575610: return getType(); 851 default: return super.makeProperty(hash, name); 852 } 853 854 } 855 856 @Override 857 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 858 switch (hash) { 859 case 3433459: /*part*/ return new String[] {"string"}; 860 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 861 default: return super.getTypesForProperty(hash, name); 862 } 863 864 } 865 866 @Override 867 public Base addChild(String name) throws FHIRException { 868 if (name.equals("part")) { 869 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductDefinition.name.part.part"); 870 } 871 else if (name.equals("type")) { 872 this.type = new CodeableConcept(); 873 return this.type; 874 } 875 else 876 return super.addChild(name); 877 } 878 879 public MedicinalProductDefinitionNamePartComponent copy() { 880 MedicinalProductDefinitionNamePartComponent dst = new MedicinalProductDefinitionNamePartComponent(); 881 copyValues(dst); 882 return dst; 883 } 884 885 public void copyValues(MedicinalProductDefinitionNamePartComponent dst) { 886 super.copyValues(dst); 887 dst.part = part == null ? null : part.copy(); 888 dst.type = type == null ? null : type.copy(); 889 } 890 891 @Override 892 public boolean equalsDeep(Base other_) { 893 if (!super.equalsDeep(other_)) 894 return false; 895 if (!(other_ instanceof MedicinalProductDefinitionNamePartComponent)) 896 return false; 897 MedicinalProductDefinitionNamePartComponent o = (MedicinalProductDefinitionNamePartComponent) other_; 898 return compareDeep(part, o.part, true) && compareDeep(type, o.type, true); 899 } 900 901 @Override 902 public boolean equalsShallow(Base other_) { 903 if (!super.equalsShallow(other_)) 904 return false; 905 if (!(other_ instanceof MedicinalProductDefinitionNamePartComponent)) 906 return false; 907 MedicinalProductDefinitionNamePartComponent o = (MedicinalProductDefinitionNamePartComponent) other_; 908 return compareValues(part, o.part, true); 909 } 910 911 public boolean isEmpty() { 912 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(part, type); 913 } 914 915 public String fhirType() { 916 return "MedicinalProductDefinition.name.part"; 917 918 } 919 920 } 921 922 @Block() 923 public static class MedicinalProductDefinitionNameUsageComponent extends BackboneElement implements IBaseBackboneElement { 924 /** 925 * Country code for where this name applies. 926 */ 927 @Child(name = "country", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 928 @Description(shortDefinition="Country code for where this name applies", formalDefinition="Country code for where this name applies." ) 929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/country") 930 protected CodeableConcept country; 931 932 /** 933 * Jurisdiction code for where this name applies. A jurisdiction may be a sub- or supra-national entity (e.g. a state or a geographic region). 934 */ 935 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 936 @Description(shortDefinition="Jurisdiction code for where this name applies", formalDefinition="Jurisdiction code for where this name applies. A jurisdiction may be a sub- or supra-national entity (e.g. a state or a geographic region)." ) 937 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 938 protected CodeableConcept jurisdiction; 939 940 /** 941 * Language code for this name. 942 */ 943 @Child(name = "language", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 944 @Description(shortDefinition="Language code for this name", formalDefinition="Language code for this name." ) 945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 946 protected CodeableConcept language; 947 948 private static final long serialVersionUID = 1627157564L; 949 950 /** 951 * Constructor 952 */ 953 public MedicinalProductDefinitionNameUsageComponent() { 954 super(); 955 } 956 957 /** 958 * Constructor 959 */ 960 public MedicinalProductDefinitionNameUsageComponent(CodeableConcept country, CodeableConcept language) { 961 super(); 962 this.setCountry(country); 963 this.setLanguage(language); 964 } 965 966 /** 967 * @return {@link #country} (Country code for where this name applies.) 968 */ 969 public CodeableConcept getCountry() { 970 if (this.country == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create MedicinalProductDefinitionNameUsageComponent.country"); 973 else if (Configuration.doAutoCreate()) 974 this.country = new CodeableConcept(); // cc 975 return this.country; 976 } 977 978 public boolean hasCountry() { 979 return this.country != null && !this.country.isEmpty(); 980 } 981 982 /** 983 * @param value {@link #country} (Country code for where this name applies.) 984 */ 985 public MedicinalProductDefinitionNameUsageComponent setCountry(CodeableConcept value) { 986 this.country = value; 987 return this; 988 } 989 990 /** 991 * @return {@link #jurisdiction} (Jurisdiction code for where this name applies. A jurisdiction may be a sub- or supra-national entity (e.g. a state or a geographic region).) 992 */ 993 public CodeableConcept getJurisdiction() { 994 if (this.jurisdiction == null) 995 if (Configuration.errorOnAutoCreate()) 996 throw new Error("Attempt to auto-create MedicinalProductDefinitionNameUsageComponent.jurisdiction"); 997 else if (Configuration.doAutoCreate()) 998 this.jurisdiction = new CodeableConcept(); // cc 999 return this.jurisdiction; 1000 } 1001 1002 public boolean hasJurisdiction() { 1003 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 1004 } 1005 1006 /** 1007 * @param value {@link #jurisdiction} (Jurisdiction code for where this name applies. A jurisdiction may be a sub- or supra-national entity (e.g. a state or a geographic region).) 1008 */ 1009 public MedicinalProductDefinitionNameUsageComponent setJurisdiction(CodeableConcept value) { 1010 this.jurisdiction = value; 1011 return this; 1012 } 1013 1014 /** 1015 * @return {@link #language} (Language code for this name.) 1016 */ 1017 public CodeableConcept getLanguage() { 1018 if (this.language == null) 1019 if (Configuration.errorOnAutoCreate()) 1020 throw new Error("Attempt to auto-create MedicinalProductDefinitionNameUsageComponent.language"); 1021 else if (Configuration.doAutoCreate()) 1022 this.language = new CodeableConcept(); // cc 1023 return this.language; 1024 } 1025 1026 public boolean hasLanguage() { 1027 return this.language != null && !this.language.isEmpty(); 1028 } 1029 1030 /** 1031 * @param value {@link #language} (Language code for this name.) 1032 */ 1033 public MedicinalProductDefinitionNameUsageComponent setLanguage(CodeableConcept value) { 1034 this.language = value; 1035 return this; 1036 } 1037 1038 protected void listChildren(List<Property> children) { 1039 super.listChildren(children); 1040 children.add(new Property("country", "CodeableConcept", "Country code for where this name applies.", 0, 1, country)); 1041 children.add(new Property("jurisdiction", "CodeableConcept", "Jurisdiction code for where this name applies. A jurisdiction may be a sub- or supra-national entity (e.g. a state or a geographic region).", 0, 1, jurisdiction)); 1042 children.add(new Property("language", "CodeableConcept", "Language code for this name.", 0, 1, language)); 1043 } 1044 1045 @Override 1046 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1047 switch (_hash) { 1048 case 957831062: /*country*/ return new Property("country", "CodeableConcept", "Country code for where this name applies.", 0, 1, country); 1049 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "Jurisdiction code for where this name applies. A jurisdiction may be a sub- or supra-national entity (e.g. a state or a geographic region).", 0, 1, jurisdiction); 1050 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "Language code for this name.", 0, 1, language); 1051 default: return super.getNamedProperty(_hash, _name, _checkValid); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1058 switch (hash) { 1059 case 957831062: /*country*/ return this.country == null ? new Base[0] : new Base[] {this.country}; // CodeableConcept 1060 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // CodeableConcept 1061 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 1062 default: return super.getProperty(hash, name, checkValid); 1063 } 1064 1065 } 1066 1067 @Override 1068 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1069 switch (hash) { 1070 case 957831062: // country 1071 this.country = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1072 return value; 1073 case -507075711: // jurisdiction 1074 this.jurisdiction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1075 return value; 1076 case -1613589672: // language 1077 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1078 return value; 1079 default: return super.setProperty(hash, name, value); 1080 } 1081 1082 } 1083 1084 @Override 1085 public Base setProperty(String name, Base value) throws FHIRException { 1086 if (name.equals("country")) { 1087 this.country = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1088 } else if (name.equals("jurisdiction")) { 1089 this.jurisdiction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1090 } else if (name.equals("language")) { 1091 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1092 } else 1093 return super.setProperty(name, value); 1094 return value; 1095 } 1096 1097 @Override 1098 public void removeChild(String name, Base value) throws FHIRException { 1099 if (name.equals("country")) { 1100 this.country = null; 1101 } else if (name.equals("jurisdiction")) { 1102 this.jurisdiction = null; 1103 } else if (name.equals("language")) { 1104 this.language = null; 1105 } else 1106 super.removeChild(name, value); 1107 1108 } 1109 1110 @Override 1111 public Base makeProperty(int hash, String name) throws FHIRException { 1112 switch (hash) { 1113 case 957831062: return getCountry(); 1114 case -507075711: return getJurisdiction(); 1115 case -1613589672: return getLanguage(); 1116 default: return super.makeProperty(hash, name); 1117 } 1118 1119 } 1120 1121 @Override 1122 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1123 switch (hash) { 1124 case 957831062: /*country*/ return new String[] {"CodeableConcept"}; 1125 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1126 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 1127 default: return super.getTypesForProperty(hash, name); 1128 } 1129 1130 } 1131 1132 @Override 1133 public Base addChild(String name) throws FHIRException { 1134 if (name.equals("country")) { 1135 this.country = new CodeableConcept(); 1136 return this.country; 1137 } 1138 else if (name.equals("jurisdiction")) { 1139 this.jurisdiction = new CodeableConcept(); 1140 return this.jurisdiction; 1141 } 1142 else if (name.equals("language")) { 1143 this.language = new CodeableConcept(); 1144 return this.language; 1145 } 1146 else 1147 return super.addChild(name); 1148 } 1149 1150 public MedicinalProductDefinitionNameUsageComponent copy() { 1151 MedicinalProductDefinitionNameUsageComponent dst = new MedicinalProductDefinitionNameUsageComponent(); 1152 copyValues(dst); 1153 return dst; 1154 } 1155 1156 public void copyValues(MedicinalProductDefinitionNameUsageComponent dst) { 1157 super.copyValues(dst); 1158 dst.country = country == null ? null : country.copy(); 1159 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 1160 dst.language = language == null ? null : language.copy(); 1161 } 1162 1163 @Override 1164 public boolean equalsDeep(Base other_) { 1165 if (!super.equalsDeep(other_)) 1166 return false; 1167 if (!(other_ instanceof MedicinalProductDefinitionNameUsageComponent)) 1168 return false; 1169 MedicinalProductDefinitionNameUsageComponent o = (MedicinalProductDefinitionNameUsageComponent) other_; 1170 return compareDeep(country, o.country, true) && compareDeep(jurisdiction, o.jurisdiction, true) 1171 && compareDeep(language, o.language, true); 1172 } 1173 1174 @Override 1175 public boolean equalsShallow(Base other_) { 1176 if (!super.equalsShallow(other_)) 1177 return false; 1178 if (!(other_ instanceof MedicinalProductDefinitionNameUsageComponent)) 1179 return false; 1180 MedicinalProductDefinitionNameUsageComponent o = (MedicinalProductDefinitionNameUsageComponent) other_; 1181 return true; 1182 } 1183 1184 public boolean isEmpty() { 1185 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(country, jurisdiction, language 1186 ); 1187 } 1188 1189 public String fhirType() { 1190 return "MedicinalProductDefinition.name.usage"; 1191 1192 } 1193 1194 } 1195 1196 @Block() 1197 public static class MedicinalProductDefinitionCrossReferenceComponent extends BackboneElement implements IBaseBackboneElement { 1198 /** 1199 * Reference to another product, e.g. for linking authorised to investigational product. 1200 */ 1201 @Child(name = "product", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=true) 1202 @Description(shortDefinition="Reference to another product, e.g. for linking authorised to investigational product", formalDefinition="Reference to another product, e.g. for linking authorised to investigational product." ) 1203 protected CodeableReference product; 1204 1205 /** 1206 * The type of relationship, for instance branded to generic, virtual to actual product, product to development product (investigational), parallel import version. 1207 */ 1208 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1209 @Description(shortDefinition="The type of relationship, for instance branded to generic or virtual to actual product", formalDefinition="The type of relationship, for instance branded to generic, virtual to actual product, product to development product (investigational), parallel import version." ) 1210 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-cross-reference-type") 1211 protected CodeableConcept type; 1212 1213 private static final long serialVersionUID = -1746125578L; 1214 1215 /** 1216 * Constructor 1217 */ 1218 public MedicinalProductDefinitionCrossReferenceComponent() { 1219 super(); 1220 } 1221 1222 /** 1223 * Constructor 1224 */ 1225 public MedicinalProductDefinitionCrossReferenceComponent(CodeableReference product) { 1226 super(); 1227 this.setProduct(product); 1228 } 1229 1230 /** 1231 * @return {@link #product} (Reference to another product, e.g. for linking authorised to investigational product.) 1232 */ 1233 public CodeableReference getProduct() { 1234 if (this.product == null) 1235 if (Configuration.errorOnAutoCreate()) 1236 throw new Error("Attempt to auto-create MedicinalProductDefinitionCrossReferenceComponent.product"); 1237 else if (Configuration.doAutoCreate()) 1238 this.product = new CodeableReference(); // cc 1239 return this.product; 1240 } 1241 1242 public boolean hasProduct() { 1243 return this.product != null && !this.product.isEmpty(); 1244 } 1245 1246 /** 1247 * @param value {@link #product} (Reference to another product, e.g. for linking authorised to investigational product.) 1248 */ 1249 public MedicinalProductDefinitionCrossReferenceComponent setProduct(CodeableReference value) { 1250 this.product = value; 1251 return this; 1252 } 1253 1254 /** 1255 * @return {@link #type} (The type of relationship, for instance branded to generic, virtual to actual product, product to development product (investigational), parallel import version.) 1256 */ 1257 public CodeableConcept getType() { 1258 if (this.type == null) 1259 if (Configuration.errorOnAutoCreate()) 1260 throw new Error("Attempt to auto-create MedicinalProductDefinitionCrossReferenceComponent.type"); 1261 else if (Configuration.doAutoCreate()) 1262 this.type = new CodeableConcept(); // cc 1263 return this.type; 1264 } 1265 1266 public boolean hasType() { 1267 return this.type != null && !this.type.isEmpty(); 1268 } 1269 1270 /** 1271 * @param value {@link #type} (The type of relationship, for instance branded to generic, virtual to actual product, product to development product (investigational), parallel import version.) 1272 */ 1273 public MedicinalProductDefinitionCrossReferenceComponent setType(CodeableConcept value) { 1274 this.type = value; 1275 return this; 1276 } 1277 1278 protected void listChildren(List<Property> children) { 1279 super.listChildren(children); 1280 children.add(new Property("product", "CodeableReference(MedicinalProductDefinition)", "Reference to another product, e.g. for linking authorised to investigational product.", 0, 1, product)); 1281 children.add(new Property("type", "CodeableConcept", "The type of relationship, for instance branded to generic, virtual to actual product, product to development product (investigational), parallel import version.", 0, 1, type)); 1282 } 1283 1284 @Override 1285 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1286 switch (_hash) { 1287 case -309474065: /*product*/ return new Property("product", "CodeableReference(MedicinalProductDefinition)", "Reference to another product, e.g. for linking authorised to investigational product.", 0, 1, product); 1288 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of relationship, for instance branded to generic, virtual to actual product, product to development product (investigational), parallel import version.", 0, 1, type); 1289 default: return super.getNamedProperty(_hash, _name, _checkValid); 1290 } 1291 1292 } 1293 1294 @Override 1295 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1296 switch (hash) { 1297 case -309474065: /*product*/ return this.product == null ? new Base[0] : new Base[] {this.product}; // CodeableReference 1298 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1299 default: return super.getProperty(hash, name, checkValid); 1300 } 1301 1302 } 1303 1304 @Override 1305 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1306 switch (hash) { 1307 case -309474065: // product 1308 this.product = TypeConvertor.castToCodeableReference(value); // CodeableReference 1309 return value; 1310 case 3575610: // type 1311 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1312 return value; 1313 default: return super.setProperty(hash, name, value); 1314 } 1315 1316 } 1317 1318 @Override 1319 public Base setProperty(String name, Base value) throws FHIRException { 1320 if (name.equals("product")) { 1321 this.product = TypeConvertor.castToCodeableReference(value); // CodeableReference 1322 } else if (name.equals("type")) { 1323 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1324 } else 1325 return super.setProperty(name, value); 1326 return value; 1327 } 1328 1329 @Override 1330 public void removeChild(String name, Base value) throws FHIRException { 1331 if (name.equals("product")) { 1332 this.product = null; 1333 } else if (name.equals("type")) { 1334 this.type = null; 1335 } else 1336 super.removeChild(name, value); 1337 1338 } 1339 1340 @Override 1341 public Base makeProperty(int hash, String name) throws FHIRException { 1342 switch (hash) { 1343 case -309474065: return getProduct(); 1344 case 3575610: return getType(); 1345 default: return super.makeProperty(hash, name); 1346 } 1347 1348 } 1349 1350 @Override 1351 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1352 switch (hash) { 1353 case -309474065: /*product*/ return new String[] {"CodeableReference"}; 1354 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1355 default: return super.getTypesForProperty(hash, name); 1356 } 1357 1358 } 1359 1360 @Override 1361 public Base addChild(String name) throws FHIRException { 1362 if (name.equals("product")) { 1363 this.product = new CodeableReference(); 1364 return this.product; 1365 } 1366 else if (name.equals("type")) { 1367 this.type = new CodeableConcept(); 1368 return this.type; 1369 } 1370 else 1371 return super.addChild(name); 1372 } 1373 1374 public MedicinalProductDefinitionCrossReferenceComponent copy() { 1375 MedicinalProductDefinitionCrossReferenceComponent dst = new MedicinalProductDefinitionCrossReferenceComponent(); 1376 copyValues(dst); 1377 return dst; 1378 } 1379 1380 public void copyValues(MedicinalProductDefinitionCrossReferenceComponent dst) { 1381 super.copyValues(dst); 1382 dst.product = product == null ? null : product.copy(); 1383 dst.type = type == null ? null : type.copy(); 1384 } 1385 1386 @Override 1387 public boolean equalsDeep(Base other_) { 1388 if (!super.equalsDeep(other_)) 1389 return false; 1390 if (!(other_ instanceof MedicinalProductDefinitionCrossReferenceComponent)) 1391 return false; 1392 MedicinalProductDefinitionCrossReferenceComponent o = (MedicinalProductDefinitionCrossReferenceComponent) other_; 1393 return compareDeep(product, o.product, true) && compareDeep(type, o.type, true); 1394 } 1395 1396 @Override 1397 public boolean equalsShallow(Base other_) { 1398 if (!super.equalsShallow(other_)) 1399 return false; 1400 if (!(other_ instanceof MedicinalProductDefinitionCrossReferenceComponent)) 1401 return false; 1402 MedicinalProductDefinitionCrossReferenceComponent o = (MedicinalProductDefinitionCrossReferenceComponent) other_; 1403 return true; 1404 } 1405 1406 public boolean isEmpty() { 1407 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(product, type); 1408 } 1409 1410 public String fhirType() { 1411 return "MedicinalProductDefinition.crossReference"; 1412 1413 } 1414 1415 } 1416 1417 @Block() 1418 public static class MedicinalProductDefinitionOperationComponent extends BackboneElement implements IBaseBackboneElement { 1419 /** 1420 * The type of manufacturing operation e.g. manufacturing itself, re-packaging. For the authorization of this, a RegulatedAuthorization would point to the same plan or activity referenced here. 1421 */ 1422 @Child(name = "type", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 1423 @Description(shortDefinition="The type of manufacturing operation e.g. manufacturing itself, re-packaging", formalDefinition="The type of manufacturing operation e.g. manufacturing itself, re-packaging. For the authorization of this, a RegulatedAuthorization would point to the same plan or activity referenced here." ) 1424 protected CodeableReference type; 1425 1426 /** 1427 * Date range of applicability. 1428 */ 1429 @Child(name = "effectiveDate", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 1430 @Description(shortDefinition="Date range of applicability", formalDefinition="Date range of applicability." ) 1431 protected Period effectiveDate; 1432 1433 /** 1434 * The organization or establishment responsible for (or associated with) the particular process or step, examples include the manufacturer, importer, agent. 1435 */ 1436 @Child(name = "organization", type = {Organization.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1437 @Description(shortDefinition="The organization responsible for the particular process, e.g. the manufacturer or importer", formalDefinition="The organization or establishment responsible for (or associated with) the particular process or step, examples include the manufacturer, importer, agent." ) 1438 protected List<Reference> organization; 1439 1440 /** 1441 * Specifies whether this particular business or manufacturing process is considered proprietary or confidential. 1442 */ 1443 @Child(name = "confidentialityIndicator", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1444 @Description(shortDefinition="Specifies whether this process is considered proprietary or confidential", formalDefinition="Specifies whether this particular business or manufacturing process is considered proprietary or confidential." ) 1445 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-confidentiality") 1446 protected CodeableConcept confidentialityIndicator; 1447 1448 private static final long serialVersionUID = 1036054906L; 1449 1450 /** 1451 * Constructor 1452 */ 1453 public MedicinalProductDefinitionOperationComponent() { 1454 super(); 1455 } 1456 1457 /** 1458 * @return {@link #type} (The type of manufacturing operation e.g. manufacturing itself, re-packaging. For the authorization of this, a RegulatedAuthorization would point to the same plan or activity referenced here.) 1459 */ 1460 public CodeableReference getType() { 1461 if (this.type == null) 1462 if (Configuration.errorOnAutoCreate()) 1463 throw new Error("Attempt to auto-create MedicinalProductDefinitionOperationComponent.type"); 1464 else if (Configuration.doAutoCreate()) 1465 this.type = new CodeableReference(); // cc 1466 return this.type; 1467 } 1468 1469 public boolean hasType() { 1470 return this.type != null && !this.type.isEmpty(); 1471 } 1472 1473 /** 1474 * @param value {@link #type} (The type of manufacturing operation e.g. manufacturing itself, re-packaging. For the authorization of this, a RegulatedAuthorization would point to the same plan or activity referenced here.) 1475 */ 1476 public MedicinalProductDefinitionOperationComponent setType(CodeableReference value) { 1477 this.type = value; 1478 return this; 1479 } 1480 1481 /** 1482 * @return {@link #effectiveDate} (Date range of applicability.) 1483 */ 1484 public Period getEffectiveDate() { 1485 if (this.effectiveDate == null) 1486 if (Configuration.errorOnAutoCreate()) 1487 throw new Error("Attempt to auto-create MedicinalProductDefinitionOperationComponent.effectiveDate"); 1488 else if (Configuration.doAutoCreate()) 1489 this.effectiveDate = new Period(); // cc 1490 return this.effectiveDate; 1491 } 1492 1493 public boolean hasEffectiveDate() { 1494 return this.effectiveDate != null && !this.effectiveDate.isEmpty(); 1495 } 1496 1497 /** 1498 * @param value {@link #effectiveDate} (Date range of applicability.) 1499 */ 1500 public MedicinalProductDefinitionOperationComponent setEffectiveDate(Period value) { 1501 this.effectiveDate = value; 1502 return this; 1503 } 1504 1505 /** 1506 * @return {@link #organization} (The organization or establishment responsible for (or associated with) the particular process or step, examples include the manufacturer, importer, agent.) 1507 */ 1508 public List<Reference> getOrganization() { 1509 if (this.organization == null) 1510 this.organization = new ArrayList<Reference>(); 1511 return this.organization; 1512 } 1513 1514 /** 1515 * @return Returns a reference to <code>this</code> for easy method chaining 1516 */ 1517 public MedicinalProductDefinitionOperationComponent setOrganization(List<Reference> theOrganization) { 1518 this.organization = theOrganization; 1519 return this; 1520 } 1521 1522 public boolean hasOrganization() { 1523 if (this.organization == null) 1524 return false; 1525 for (Reference item : this.organization) 1526 if (!item.isEmpty()) 1527 return true; 1528 return false; 1529 } 1530 1531 public Reference addOrganization() { //3 1532 Reference t = new Reference(); 1533 if (this.organization == null) 1534 this.organization = new ArrayList<Reference>(); 1535 this.organization.add(t); 1536 return t; 1537 } 1538 1539 public MedicinalProductDefinitionOperationComponent addOrganization(Reference t) { //3 1540 if (t == null) 1541 return this; 1542 if (this.organization == null) 1543 this.organization = new ArrayList<Reference>(); 1544 this.organization.add(t); 1545 return this; 1546 } 1547 1548 /** 1549 * @return The first repetition of repeating field {@link #organization}, creating it if it does not already exist {3} 1550 */ 1551 public Reference getOrganizationFirstRep() { 1552 if (getOrganization().isEmpty()) { 1553 addOrganization(); 1554 } 1555 return getOrganization().get(0); 1556 } 1557 1558 /** 1559 * @return {@link #confidentialityIndicator} (Specifies whether this particular business or manufacturing process is considered proprietary or confidential.) 1560 */ 1561 public CodeableConcept getConfidentialityIndicator() { 1562 if (this.confidentialityIndicator == null) 1563 if (Configuration.errorOnAutoCreate()) 1564 throw new Error("Attempt to auto-create MedicinalProductDefinitionOperationComponent.confidentialityIndicator"); 1565 else if (Configuration.doAutoCreate()) 1566 this.confidentialityIndicator = new CodeableConcept(); // cc 1567 return this.confidentialityIndicator; 1568 } 1569 1570 public boolean hasConfidentialityIndicator() { 1571 return this.confidentialityIndicator != null && !this.confidentialityIndicator.isEmpty(); 1572 } 1573 1574 /** 1575 * @param value {@link #confidentialityIndicator} (Specifies whether this particular business or manufacturing process is considered proprietary or confidential.) 1576 */ 1577 public MedicinalProductDefinitionOperationComponent setConfidentialityIndicator(CodeableConcept value) { 1578 this.confidentialityIndicator = value; 1579 return this; 1580 } 1581 1582 protected void listChildren(List<Property> children) { 1583 super.listChildren(children); 1584 children.add(new Property("type", "CodeableReference(ActivityDefinition|PlanDefinition)", "The type of manufacturing operation e.g. manufacturing itself, re-packaging. For the authorization of this, a RegulatedAuthorization would point to the same plan or activity referenced here.", 0, 1, type)); 1585 children.add(new Property("effectiveDate", "Period", "Date range of applicability.", 0, 1, effectiveDate)); 1586 children.add(new Property("organization", "Reference(Organization)", "The organization or establishment responsible for (or associated with) the particular process or step, examples include the manufacturer, importer, agent.", 0, java.lang.Integer.MAX_VALUE, organization)); 1587 children.add(new Property("confidentialityIndicator", "CodeableConcept", "Specifies whether this particular business or manufacturing process is considered proprietary or confidential.", 0, 1, confidentialityIndicator)); 1588 } 1589 1590 @Override 1591 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1592 switch (_hash) { 1593 case 3575610: /*type*/ return new Property("type", "CodeableReference(ActivityDefinition|PlanDefinition)", "The type of manufacturing operation e.g. manufacturing itself, re-packaging. For the authorization of this, a RegulatedAuthorization would point to the same plan or activity referenced here.", 0, 1, type); 1594 case -930389515: /*effectiveDate*/ return new Property("effectiveDate", "Period", "Date range of applicability.", 0, 1, effectiveDate); 1595 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization or establishment responsible for (or associated with) the particular process or step, examples include the manufacturer, importer, agent.", 0, java.lang.Integer.MAX_VALUE, organization); 1596 case -1449404791: /*confidentialityIndicator*/ return new Property("confidentialityIndicator", "CodeableConcept", "Specifies whether this particular business or manufacturing process is considered proprietary or confidential.", 0, 1, confidentialityIndicator); 1597 default: return super.getNamedProperty(_hash, _name, _checkValid); 1598 } 1599 1600 } 1601 1602 @Override 1603 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1604 switch (hash) { 1605 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableReference 1606 case -930389515: /*effectiveDate*/ return this.effectiveDate == null ? new Base[0] : new Base[] {this.effectiveDate}; // Period 1607 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : this.organization.toArray(new Base[this.organization.size()]); // Reference 1608 case -1449404791: /*confidentialityIndicator*/ return this.confidentialityIndicator == null ? new Base[0] : new Base[] {this.confidentialityIndicator}; // CodeableConcept 1609 default: return super.getProperty(hash, name, checkValid); 1610 } 1611 1612 } 1613 1614 @Override 1615 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1616 switch (hash) { 1617 case 3575610: // type 1618 this.type = TypeConvertor.castToCodeableReference(value); // CodeableReference 1619 return value; 1620 case -930389515: // effectiveDate 1621 this.effectiveDate = TypeConvertor.castToPeriod(value); // Period 1622 return value; 1623 case 1178922291: // organization 1624 this.getOrganization().add(TypeConvertor.castToReference(value)); // Reference 1625 return value; 1626 case -1449404791: // confidentialityIndicator 1627 this.confidentialityIndicator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1628 return value; 1629 default: return super.setProperty(hash, name, value); 1630 } 1631 1632 } 1633 1634 @Override 1635 public Base setProperty(String name, Base value) throws FHIRException { 1636 if (name.equals("type")) { 1637 this.type = TypeConvertor.castToCodeableReference(value); // CodeableReference 1638 } else if (name.equals("effectiveDate")) { 1639 this.effectiveDate = TypeConvertor.castToPeriod(value); // Period 1640 } else if (name.equals("organization")) { 1641 this.getOrganization().add(TypeConvertor.castToReference(value)); 1642 } else if (name.equals("confidentialityIndicator")) { 1643 this.confidentialityIndicator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1644 } else 1645 return super.setProperty(name, value); 1646 return value; 1647 } 1648 1649 @Override 1650 public void removeChild(String name, Base value) throws FHIRException { 1651 if (name.equals("type")) { 1652 this.type = null; 1653 } else if (name.equals("effectiveDate")) { 1654 this.effectiveDate = null; 1655 } else if (name.equals("organization")) { 1656 this.getOrganization().remove(value); 1657 } else if (name.equals("confidentialityIndicator")) { 1658 this.confidentialityIndicator = null; 1659 } else 1660 super.removeChild(name, value); 1661 1662 } 1663 1664 @Override 1665 public Base makeProperty(int hash, String name) throws FHIRException { 1666 switch (hash) { 1667 case 3575610: return getType(); 1668 case -930389515: return getEffectiveDate(); 1669 case 1178922291: return addOrganization(); 1670 case -1449404791: return getConfidentialityIndicator(); 1671 default: return super.makeProperty(hash, name); 1672 } 1673 1674 } 1675 1676 @Override 1677 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1678 switch (hash) { 1679 case 3575610: /*type*/ return new String[] {"CodeableReference"}; 1680 case -930389515: /*effectiveDate*/ return new String[] {"Period"}; 1681 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1682 case -1449404791: /*confidentialityIndicator*/ return new String[] {"CodeableConcept"}; 1683 default: return super.getTypesForProperty(hash, name); 1684 } 1685 1686 } 1687 1688 @Override 1689 public Base addChild(String name) throws FHIRException { 1690 if (name.equals("type")) { 1691 this.type = new CodeableReference(); 1692 return this.type; 1693 } 1694 else if (name.equals("effectiveDate")) { 1695 this.effectiveDate = new Period(); 1696 return this.effectiveDate; 1697 } 1698 else if (name.equals("organization")) { 1699 return addOrganization(); 1700 } 1701 else if (name.equals("confidentialityIndicator")) { 1702 this.confidentialityIndicator = new CodeableConcept(); 1703 return this.confidentialityIndicator; 1704 } 1705 else 1706 return super.addChild(name); 1707 } 1708 1709 public MedicinalProductDefinitionOperationComponent copy() { 1710 MedicinalProductDefinitionOperationComponent dst = new MedicinalProductDefinitionOperationComponent(); 1711 copyValues(dst); 1712 return dst; 1713 } 1714 1715 public void copyValues(MedicinalProductDefinitionOperationComponent dst) { 1716 super.copyValues(dst); 1717 dst.type = type == null ? null : type.copy(); 1718 dst.effectiveDate = effectiveDate == null ? null : effectiveDate.copy(); 1719 if (organization != null) { 1720 dst.organization = new ArrayList<Reference>(); 1721 for (Reference i : organization) 1722 dst.organization.add(i.copy()); 1723 }; 1724 dst.confidentialityIndicator = confidentialityIndicator == null ? null : confidentialityIndicator.copy(); 1725 } 1726 1727 @Override 1728 public boolean equalsDeep(Base other_) { 1729 if (!super.equalsDeep(other_)) 1730 return false; 1731 if (!(other_ instanceof MedicinalProductDefinitionOperationComponent)) 1732 return false; 1733 MedicinalProductDefinitionOperationComponent o = (MedicinalProductDefinitionOperationComponent) other_; 1734 return compareDeep(type, o.type, true) && compareDeep(effectiveDate, o.effectiveDate, true) && compareDeep(organization, o.organization, true) 1735 && compareDeep(confidentialityIndicator, o.confidentialityIndicator, true); 1736 } 1737 1738 @Override 1739 public boolean equalsShallow(Base other_) { 1740 if (!super.equalsShallow(other_)) 1741 return false; 1742 if (!(other_ instanceof MedicinalProductDefinitionOperationComponent)) 1743 return false; 1744 MedicinalProductDefinitionOperationComponent o = (MedicinalProductDefinitionOperationComponent) other_; 1745 return true; 1746 } 1747 1748 public boolean isEmpty() { 1749 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, effectiveDate, organization 1750 , confidentialityIndicator); 1751 } 1752 1753 public String fhirType() { 1754 return "MedicinalProductDefinition.operation"; 1755 1756 } 1757 1758 } 1759 1760 @Block() 1761 public static class MedicinalProductDefinitionCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 1762 /** 1763 * A code expressing the type of characteristic. 1764 */ 1765 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1766 @Description(shortDefinition="A code expressing the type of characteristic", formalDefinition="A code expressing the type of characteristic." ) 1767 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-characteristic-codes") 1768 protected CodeableConcept type; 1769 1770 /** 1771 * A value for the characteristic.text. 1772 */ 1773 @Child(name = "value", type = {CodeableConcept.class, MarkdownType.class, Quantity.class, IntegerType.class, DateType.class, BooleanType.class, Attachment.class}, order=2, min=0, max=1, modifier=false, summary=true) 1774 @Description(shortDefinition="A value for the characteristic", formalDefinition="A value for the characteristic.text." ) 1775 protected DataType value; 1776 1777 private static final long serialVersionUID = -1659186716L; 1778 1779 /** 1780 * Constructor 1781 */ 1782 public MedicinalProductDefinitionCharacteristicComponent() { 1783 super(); 1784 } 1785 1786 /** 1787 * Constructor 1788 */ 1789 public MedicinalProductDefinitionCharacteristicComponent(CodeableConcept type) { 1790 super(); 1791 this.setType(type); 1792 } 1793 1794 /** 1795 * @return {@link #type} (A code expressing the type of characteristic.) 1796 */ 1797 public CodeableConcept getType() { 1798 if (this.type == null) 1799 if (Configuration.errorOnAutoCreate()) 1800 throw new Error("Attempt to auto-create MedicinalProductDefinitionCharacteristicComponent.type"); 1801 else if (Configuration.doAutoCreate()) 1802 this.type = new CodeableConcept(); // cc 1803 return this.type; 1804 } 1805 1806 public boolean hasType() { 1807 return this.type != null && !this.type.isEmpty(); 1808 } 1809 1810 /** 1811 * @param value {@link #type} (A code expressing the type of characteristic.) 1812 */ 1813 public MedicinalProductDefinitionCharacteristicComponent setType(CodeableConcept value) { 1814 this.type = value; 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #value} (A value for the characteristic.text.) 1820 */ 1821 public DataType getValue() { 1822 return this.value; 1823 } 1824 1825 /** 1826 * @return {@link #value} (A value for the characteristic.text.) 1827 */ 1828 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1829 if (this.value == null) 1830 this.value = new CodeableConcept(); 1831 if (!(this.value instanceof CodeableConcept)) 1832 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1833 return (CodeableConcept) this.value; 1834 } 1835 1836 public boolean hasValueCodeableConcept() { 1837 return this != null && this.value instanceof CodeableConcept; 1838 } 1839 1840 /** 1841 * @return {@link #value} (A value for the characteristic.text.) 1842 */ 1843 public MarkdownType getValueMarkdownType() throws FHIRException { 1844 if (this.value == null) 1845 this.value = new MarkdownType(); 1846 if (!(this.value instanceof MarkdownType)) 1847 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 1848 return (MarkdownType) this.value; 1849 } 1850 1851 public boolean hasValueMarkdownType() { 1852 return this != null && this.value instanceof MarkdownType; 1853 } 1854 1855 /** 1856 * @return {@link #value} (A value for the characteristic.text.) 1857 */ 1858 public Quantity getValueQuantity() throws FHIRException { 1859 if (this.value == null) 1860 this.value = new Quantity(); 1861 if (!(this.value instanceof Quantity)) 1862 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1863 return (Quantity) this.value; 1864 } 1865 1866 public boolean hasValueQuantity() { 1867 return this != null && this.value instanceof Quantity; 1868 } 1869 1870 /** 1871 * @return {@link #value} (A value for the characteristic.text.) 1872 */ 1873 public IntegerType getValueIntegerType() throws FHIRException { 1874 if (this.value == null) 1875 this.value = new IntegerType(); 1876 if (!(this.value instanceof IntegerType)) 1877 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 1878 return (IntegerType) this.value; 1879 } 1880 1881 public boolean hasValueIntegerType() { 1882 return this != null && this.value instanceof IntegerType; 1883 } 1884 1885 /** 1886 * @return {@link #value} (A value for the characteristic.text.) 1887 */ 1888 public DateType getValueDateType() throws FHIRException { 1889 if (this.value == null) 1890 this.value = new DateType(); 1891 if (!(this.value instanceof DateType)) 1892 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 1893 return (DateType) this.value; 1894 } 1895 1896 public boolean hasValueDateType() { 1897 return this != null && this.value instanceof DateType; 1898 } 1899 1900 /** 1901 * @return {@link #value} (A value for the characteristic.text.) 1902 */ 1903 public BooleanType getValueBooleanType() throws FHIRException { 1904 if (this.value == null) 1905 this.value = new BooleanType(); 1906 if (!(this.value instanceof BooleanType)) 1907 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1908 return (BooleanType) this.value; 1909 } 1910 1911 public boolean hasValueBooleanType() { 1912 return this != null && this.value instanceof BooleanType; 1913 } 1914 1915 /** 1916 * @return {@link #value} (A value for the characteristic.text.) 1917 */ 1918 public Attachment getValueAttachment() throws FHIRException { 1919 if (this.value == null) 1920 this.value = new Attachment(); 1921 if (!(this.value instanceof Attachment)) 1922 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1923 return (Attachment) this.value; 1924 } 1925 1926 public boolean hasValueAttachment() { 1927 return this != null && this.value instanceof Attachment; 1928 } 1929 1930 public boolean hasValue() { 1931 return this.value != null && !this.value.isEmpty(); 1932 } 1933 1934 /** 1935 * @param value {@link #value} (A value for the characteristic.text.) 1936 */ 1937 public MedicinalProductDefinitionCharacteristicComponent setValue(DataType value) { 1938 if (value != null && !(value instanceof CodeableConcept || value instanceof MarkdownType || value instanceof Quantity || value instanceof IntegerType || value instanceof DateType || value instanceof BooleanType || value instanceof Attachment)) 1939 throw new FHIRException("Not the right type for MedicinalProductDefinition.characteristic.value[x]: "+value.fhirType()); 1940 this.value = value; 1941 return this; 1942 } 1943 1944 protected void listChildren(List<Property> children) { 1945 super.listChildren(children); 1946 children.add(new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type)); 1947 children.add(new Property("value[x]", "CodeableConcept|markdown|Quantity|integer|date|boolean|Attachment", "A value for the characteristic.text.", 0, 1, value)); 1948 } 1949 1950 @Override 1951 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1952 switch (_hash) { 1953 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type); 1954 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|markdown|Quantity|integer|date|boolean|Attachment", "A value for the characteristic.text.", 0, 1, value); 1955 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|markdown|Quantity|integer|date|boolean|Attachment", "A value for the characteristic.text.", 0, 1, value); 1956 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "A value for the characteristic.text.", 0, 1, value); 1957 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "A value for the characteristic.text.", 0, 1, value); 1958 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "A value for the characteristic.text.", 0, 1, value); 1959 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "A value for the characteristic.text.", 0, 1, value); 1960 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "A value for the characteristic.text.", 0, 1, value); 1961 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "A value for the characteristic.text.", 0, 1, value); 1962 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "A value for the characteristic.text.", 0, 1, value); 1963 default: return super.getNamedProperty(_hash, _name, _checkValid); 1964 } 1965 1966 } 1967 1968 @Override 1969 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1970 switch (hash) { 1971 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1972 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1973 default: return super.getProperty(hash, name, checkValid); 1974 } 1975 1976 } 1977 1978 @Override 1979 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1980 switch (hash) { 1981 case 3575610: // type 1982 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1983 return value; 1984 case 111972721: // value 1985 this.value = TypeConvertor.castToType(value); // DataType 1986 return value; 1987 default: return super.setProperty(hash, name, value); 1988 } 1989 1990 } 1991 1992 @Override 1993 public Base setProperty(String name, Base value) throws FHIRException { 1994 if (name.equals("type")) { 1995 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1996 } else if (name.equals("value[x]")) { 1997 this.value = TypeConvertor.castToType(value); // DataType 1998 } else 1999 return super.setProperty(name, value); 2000 return value; 2001 } 2002 2003 @Override 2004 public void removeChild(String name, Base value) throws FHIRException { 2005 if (name.equals("type")) { 2006 this.type = null; 2007 } else if (name.equals("value[x]")) { 2008 this.value = null; 2009 } else 2010 super.removeChild(name, value); 2011 2012 } 2013 2014 @Override 2015 public Base makeProperty(int hash, String name) throws FHIRException { 2016 switch (hash) { 2017 case 3575610: return getType(); 2018 case -1410166417: return getValue(); 2019 case 111972721: return getValue(); 2020 default: return super.makeProperty(hash, name); 2021 } 2022 2023 } 2024 2025 @Override 2026 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2027 switch (hash) { 2028 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2029 case 111972721: /*value*/ return new String[] {"CodeableConcept", "markdown", "Quantity", "integer", "date", "boolean", "Attachment"}; 2030 default: return super.getTypesForProperty(hash, name); 2031 } 2032 2033 } 2034 2035 @Override 2036 public Base addChild(String name) throws FHIRException { 2037 if (name.equals("type")) { 2038 this.type = new CodeableConcept(); 2039 return this.type; 2040 } 2041 else if (name.equals("valueCodeableConcept")) { 2042 this.value = new CodeableConcept(); 2043 return this.value; 2044 } 2045 else if (name.equals("valueMarkdown")) { 2046 this.value = new MarkdownType(); 2047 return this.value; 2048 } 2049 else if (name.equals("valueQuantity")) { 2050 this.value = new Quantity(); 2051 return this.value; 2052 } 2053 else if (name.equals("valueInteger")) { 2054 this.value = new IntegerType(); 2055 return this.value; 2056 } 2057 else if (name.equals("valueDate")) { 2058 this.value = new DateType(); 2059 return this.value; 2060 } 2061 else if (name.equals("valueBoolean")) { 2062 this.value = new BooleanType(); 2063 return this.value; 2064 } 2065 else if (name.equals("valueAttachment")) { 2066 this.value = new Attachment(); 2067 return this.value; 2068 } 2069 else 2070 return super.addChild(name); 2071 } 2072 2073 public MedicinalProductDefinitionCharacteristicComponent copy() { 2074 MedicinalProductDefinitionCharacteristicComponent dst = new MedicinalProductDefinitionCharacteristicComponent(); 2075 copyValues(dst); 2076 return dst; 2077 } 2078 2079 public void copyValues(MedicinalProductDefinitionCharacteristicComponent dst) { 2080 super.copyValues(dst); 2081 dst.type = type == null ? null : type.copy(); 2082 dst.value = value == null ? null : value.copy(); 2083 } 2084 2085 @Override 2086 public boolean equalsDeep(Base other_) { 2087 if (!super.equalsDeep(other_)) 2088 return false; 2089 if (!(other_ instanceof MedicinalProductDefinitionCharacteristicComponent)) 2090 return false; 2091 MedicinalProductDefinitionCharacteristicComponent o = (MedicinalProductDefinitionCharacteristicComponent) other_; 2092 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2093 } 2094 2095 @Override 2096 public boolean equalsShallow(Base other_) { 2097 if (!super.equalsShallow(other_)) 2098 return false; 2099 if (!(other_ instanceof MedicinalProductDefinitionCharacteristicComponent)) 2100 return false; 2101 MedicinalProductDefinitionCharacteristicComponent o = (MedicinalProductDefinitionCharacteristicComponent) other_; 2102 return true; 2103 } 2104 2105 public boolean isEmpty() { 2106 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2107 } 2108 2109 public String fhirType() { 2110 return "MedicinalProductDefinition.characteristic"; 2111 2112 } 2113 2114 } 2115 2116 /** 2117 * Business identifier for this product. Could be an MPID. When in development or being regulated, products are typically referenced by official identifiers, assigned by a manufacturer or regulator, and unique to a product (which, when compared to a product instance being prescribed, is actually a product type). See also MedicinalProductDefinition.code. 2118 */ 2119 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2120 @Description(shortDefinition="Business identifier for this product. Could be an MPID", formalDefinition="Business identifier for this product. Could be an MPID. When in development or being regulated, products are typically referenced by official identifiers, assigned by a manufacturer or regulator, and unique to a product (which, when compared to a product instance being prescribed, is actually a product type). See also MedicinalProductDefinition.code." ) 2121 protected List<Identifier> identifier; 2122 2123 /** 2124 * Regulatory type, e.g. Investigational or Authorized. 2125 */ 2126 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 2127 @Description(shortDefinition="Regulatory type, e.g. Investigational or Authorized", formalDefinition="Regulatory type, e.g. Investigational or Authorized." ) 2128 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-type") 2129 protected CodeableConcept type; 2130 2131 /** 2132 * If this medicine applies to human or veterinary uses. 2133 */ 2134 @Child(name = "domain", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2135 @Description(shortDefinition="If this medicine applies to human or veterinary uses", formalDefinition="If this medicine applies to human or veterinary uses." ) 2136 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-domain") 2137 protected CodeableConcept domain; 2138 2139 /** 2140 * A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product. 2141 */ 2142 @Child(name = "version", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2143 @Description(shortDefinition="A business identifier relating to a specific version of the product", formalDefinition="A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product." ) 2144 protected StringType version; 2145 2146 /** 2147 * The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status. 2148 */ 2149 @Child(name = "status", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=true, summary=true) 2150 @Description(shortDefinition="The status within the lifecycle of this product record", formalDefinition="The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status." ) 2151 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2152 protected CodeableConcept status; 2153 2154 /** 2155 * The date at which the given status became applicable. 2156 */ 2157 @Child(name = "statusDate", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2158 @Description(shortDefinition="The date at which the given status became applicable", formalDefinition="The date at which the given status became applicable." ) 2159 protected DateTimeType statusDate; 2160 2161 /** 2162 * General description of this product. 2163 */ 2164 @Child(name = "description", type = {MarkdownType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2165 @Description(shortDefinition="General description of this product", formalDefinition="General description of this product." ) 2166 protected MarkdownType description; 2167 2168 /** 2169 * The dose form for a single part product, or combined form of a multiple part product. This is one concept that describes all the components. It does not represent the form with components physically mixed, if that might be necessary, for which see (AdministrableProductDefinition.administrableDoseForm). 2170 */ 2171 @Child(name = "combinedPharmaceuticalDoseForm", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 2172 @Description(shortDefinition="The dose form for a single part product, or combined form of a multiple part product", formalDefinition="The dose form for a single part product, or combined form of a multiple part product. This is one concept that describes all the components. It does not represent the form with components physically mixed, if that might be necessary, for which see (AdministrableProductDefinition.administrableDoseForm)." ) 2173 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/combined-dose-form") 2174 protected CodeableConcept combinedPharmaceuticalDoseForm; 2175 2176 /** 2177 * The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. See also AdministrableProductDefinition resource. MedicinalProductDefinition.route is the same concept as AdministrableProductDefinition.routeOfAdministration.code, and they cannot be used together. 2178 */ 2179 @Child(name = "route", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2180 @Description(shortDefinition="The path by which the product is taken into or makes contact with the body", formalDefinition="The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. See also AdministrableProductDefinition resource. MedicinalProductDefinition.route is the same concept as AdministrableProductDefinition.routeOfAdministration.code, and they cannot be used together." ) 2181 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 2182 protected List<CodeableConcept> route; 2183 2184 /** 2185 * Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate. 2186 */ 2187 @Child(name = "indication", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2188 @Description(shortDefinition="Description of indication(s) for this product, used when structured indications are not required", formalDefinition="Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate." ) 2189 protected MarkdownType indication; 2190 2191 /** 2192 * The legal status of supply of the medicinal product as classified by the regulator. 2193 */ 2194 @Child(name = "legalStatusOfSupply", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 2195 @Description(shortDefinition="The legal status of supply of the medicinal product as classified by the regulator", formalDefinition="The legal status of supply of the medicinal product as classified by the regulator." ) 2196 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/legal-status-of-supply") 2197 protected CodeableConcept legalStatusOfSupply; 2198 2199 /** 2200 * Whether the Medicinal Product is subject to additional monitoring for regulatory reasons, such as heightened reporting requirements. 2201 */ 2202 @Child(name = "additionalMonitoringIndicator", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=true) 2203 @Description(shortDefinition="Whether the Medicinal Product is subject to additional monitoring for regulatory reasons", formalDefinition="Whether the Medicinal Product is subject to additional monitoring for regulatory reasons, such as heightened reporting requirements." ) 2204 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-additional-monitoring") 2205 protected CodeableConcept additionalMonitoringIndicator; 2206 2207 /** 2208 * Whether the Medicinal Product is subject to special measures for regulatory reasons, such as a requirement to conduct post-authorization studies. 2209 */ 2210 @Child(name = "specialMeasures", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2211 @Description(shortDefinition="Whether the Medicinal Product is subject to special measures for regulatory reasons", formalDefinition="Whether the Medicinal Product is subject to special measures for regulatory reasons, such as a requirement to conduct post-authorization studies." ) 2212 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-special-measures") 2213 protected List<CodeableConcept> specialMeasures; 2214 2215 /** 2216 * If authorised for use in children, or infants, neonates etc. 2217 */ 2218 @Child(name = "pediatricUseIndicator", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=true) 2219 @Description(shortDefinition="If authorised for use in children", formalDefinition="If authorised for use in children, or infants, neonates etc." ) 2220 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-pediatric-use") 2221 protected CodeableConcept pediatricUseIndicator; 2222 2223 /** 2224 * Allows the product to be classified by various systems, commonly WHO ATC. 2225 */ 2226 @Child(name = "classification", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2227 @Description(shortDefinition="Allows the product to be classified by various systems", formalDefinition="Allows the product to be classified by various systems, commonly WHO ATC." ) 2228 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-classification") 2229 protected List<CodeableConcept> classification; 2230 2231 /** 2232 * Marketing status of the medicinal product, in contrast to marketing authorization. This refers to the product being actually 'on the market' as opposed to being allowed to be on the market (which is an authorization). 2233 */ 2234 @Child(name = "marketingStatus", type = {MarketingStatus.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2235 @Description(shortDefinition="Marketing status of the medicinal product, in contrast to marketing authorization", formalDefinition="Marketing status of the medicinal product, in contrast to marketing authorization. This refers to the product being actually 'on the market' as opposed to being allowed to be on the market (which is an authorization)." ) 2236 protected List<MarketingStatus> marketingStatus; 2237 2238 /** 2239 * Package type for the product. See also the PackagedProductDefinition resource. 2240 */ 2241 @Child(name = "packagedMedicinalProduct", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2242 @Description(shortDefinition="Package type for the product", formalDefinition="Package type for the product. See also the PackagedProductDefinition resource." ) 2243 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-package-type") 2244 protected List<CodeableConcept> packagedMedicinalProduct; 2245 2246 /** 2247 * Types of medicinal manufactured items and/or devices that this product consists of, such as tablets, capsule, or syringes. Used as a direct link when the item's packaging is not being recorded (see also PackagedProductDefinition.package.containedItem.item). 2248 */ 2249 @Child(name = "comprisedOf", type = {ManufacturedItemDefinition.class, DeviceDefinition.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2250 @Description(shortDefinition="Types of medicinal manufactured items and/or devices that this product consists of, such as tablets, capsule, or syringes", formalDefinition="Types of medicinal manufactured items and/or devices that this product consists of, such as tablets, capsule, or syringes. Used as a direct link when the item's packaging is not being recorded (see also PackagedProductDefinition.package.containedItem.item)." ) 2251 protected List<Reference> comprisedOf; 2252 2253 /** 2254 * The ingredients of this medicinal product - when not detailed in other resources. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource, or indirectly via incoming AdministrableProductDefinition, PackagedProductDefinition or ManufacturedItemDefinition references. In cases where those levels of detail are not used, the ingredients may be specified directly here as codes. 2255 */ 2256 @Child(name = "ingredient", type = {CodeableConcept.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2257 @Description(shortDefinition="The ingredients of this medicinal product - when not detailed in other resources", formalDefinition="The ingredients of this medicinal product - when not detailed in other resources. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource, or indirectly via incoming AdministrableProductDefinition, PackagedProductDefinition or ManufacturedItemDefinition references. In cases where those levels of detail are not used, the ingredients may be specified directly here as codes." ) 2258 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-codes") 2259 protected List<CodeableConcept> ingredient; 2260 2261 /** 2262 * Any component of the drug product which is not the chemical entity defined as the drug substance, or an excipient in the drug product. This includes process-related impurities and contaminants, product-related impurities including degradation products. 2263 */ 2264 @Child(name = "impurity", type = {CodeableReference.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2265 @Description(shortDefinition="Any component of the drug product which is not the chemical entity defined as the drug substance, or an excipient in the drug product", formalDefinition="Any component of the drug product which is not the chemical entity defined as the drug substance, or an excipient in the drug product. This includes process-related impurities and contaminants, product-related impurities including degradation products." ) 2266 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-codes") 2267 protected List<CodeableReference> impurity; 2268 2269 /** 2270 * Additional information or supporting documentation about the medicinal product. 2271 */ 2272 @Child(name = "attachedDocument", type = {DocumentReference.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2273 @Description(shortDefinition="Additional documentation about the medicinal product", formalDefinition="Additional information or supporting documentation about the medicinal product." ) 2274 protected List<Reference> attachedDocument; 2275 2276 /** 2277 * A master file for the medicinal product (e.g. Pharmacovigilance System Master File). Drug master files (DMFs) are documents submitted to regulatory agencies to provide confidential detailed information about facilities, processes or articles used in the manufacturing, processing, packaging and storing of drug products. 2278 */ 2279 @Child(name = "masterFile", type = {DocumentReference.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2280 @Description(shortDefinition="A master file for the medicinal product (e.g. Pharmacovigilance System Master File)", formalDefinition="A master file for the medicinal product (e.g. Pharmacovigilance System Master File). Drug master files (DMFs) are documents submitted to regulatory agencies to provide confidential detailed information about facilities, processes or articles used in the manufacturing, processing, packaging and storing of drug products." ) 2281 protected List<Reference> masterFile; 2282 2283 /** 2284 * A product specific contact, person (in a role), or an organization. 2285 */ 2286 @Child(name = "contact", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2287 @Description(shortDefinition="A product specific contact, person (in a role), or an organization", formalDefinition="A product specific contact, person (in a role), or an organization." ) 2288 protected List<MedicinalProductDefinitionContactComponent> contact; 2289 2290 /** 2291 * Clinical trials or studies that this product is involved in. 2292 */ 2293 @Child(name = "clinicalTrial", type = {ResearchStudy.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2294 @Description(shortDefinition="Clinical trials or studies that this product is involved in", formalDefinition="Clinical trials or studies that this product is involved in." ) 2295 protected List<Reference> clinicalTrial; 2296 2297 /** 2298 * A code that this product is known by, usually within some formal terminology, perhaps assigned by a third party (i.e. not the manufacturer or regulator). Products (types of medications) tend to be known by identifiers during development and within regulatory process. However when they are prescribed they tend to be identified by codes. The same product may be have multiple codes, applied to it by multiple organizations. 2299 */ 2300 @Child(name = "code", type = {Coding.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2301 @Description(shortDefinition="A code that this product is known by, within some formal terminology", formalDefinition="A code that this product is known by, usually within some formal terminology, perhaps assigned by a third party (i.e. not the manufacturer or regulator). Products (types of medications) tend to be known by identifiers during development and within regulatory process. However when they are prescribed they tend to be identified by codes. The same product may be have multiple codes, applied to it by multiple organizations." ) 2302 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 2303 protected List<Coding> code; 2304 2305 /** 2306 * The product's name, including full name and possibly coded parts. 2307 */ 2308 @Child(name = "name", type = {}, order=25, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2309 @Description(shortDefinition="The product's name, including full name and possibly coded parts", formalDefinition="The product's name, including full name and possibly coded parts." ) 2310 protected List<MedicinalProductDefinitionNameComponent> name; 2311 2312 /** 2313 * Reference to another product, e.g. for linking authorised to investigational product, or a virtual product. 2314 */ 2315 @Child(name = "crossReference", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2316 @Description(shortDefinition="Reference to another product, e.g. for linking authorised to investigational product", formalDefinition="Reference to another product, e.g. for linking authorised to investigational product, or a virtual product." ) 2317 protected List<MedicinalProductDefinitionCrossReferenceComponent> crossReference; 2318 2319 /** 2320 * A manufacturing or administrative process or step associated with (or performed on) the medicinal product. 2321 */ 2322 @Child(name = "operation", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2323 @Description(shortDefinition="A manufacturing or administrative process for the medicinal product", formalDefinition="A manufacturing or administrative process or step associated with (or performed on) the medicinal product." ) 2324 protected List<MedicinalProductDefinitionOperationComponent> operation; 2325 2326 /** 2327 * Allows the key product features to be recorded, such as "sugar free", "modified release", "parallel import". 2328 */ 2329 @Child(name = "characteristic", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2330 @Description(shortDefinition="Key product features such as \"sugar free\", \"modified release\"", formalDefinition="Allows the key product features to be recorded, such as \"sugar free\", \"modified release\", \"parallel import\"." ) 2331 protected List<MedicinalProductDefinitionCharacteristicComponent> characteristic; 2332 2333 private static final long serialVersionUID = 1105265034L; 2334 2335 /** 2336 * Constructor 2337 */ 2338 public MedicinalProductDefinition() { 2339 super(); 2340 } 2341 2342 /** 2343 * Constructor 2344 */ 2345 public MedicinalProductDefinition(MedicinalProductDefinitionNameComponent name) { 2346 super(); 2347 this.addName(name); 2348 } 2349 2350 /** 2351 * @return {@link #identifier} (Business identifier for this product. Could be an MPID. When in development or being regulated, products are typically referenced by official identifiers, assigned by a manufacturer or regulator, and unique to a product (which, when compared to a product instance being prescribed, is actually a product type). See also MedicinalProductDefinition.code.) 2352 */ 2353 public List<Identifier> getIdentifier() { 2354 if (this.identifier == null) 2355 this.identifier = new ArrayList<Identifier>(); 2356 return this.identifier; 2357 } 2358 2359 /** 2360 * @return Returns a reference to <code>this</code> for easy method chaining 2361 */ 2362 public MedicinalProductDefinition setIdentifier(List<Identifier> theIdentifier) { 2363 this.identifier = theIdentifier; 2364 return this; 2365 } 2366 2367 public boolean hasIdentifier() { 2368 if (this.identifier == null) 2369 return false; 2370 for (Identifier item : this.identifier) 2371 if (!item.isEmpty()) 2372 return true; 2373 return false; 2374 } 2375 2376 public Identifier addIdentifier() { //3 2377 Identifier t = new Identifier(); 2378 if (this.identifier == null) 2379 this.identifier = new ArrayList<Identifier>(); 2380 this.identifier.add(t); 2381 return t; 2382 } 2383 2384 public MedicinalProductDefinition addIdentifier(Identifier t) { //3 2385 if (t == null) 2386 return this; 2387 if (this.identifier == null) 2388 this.identifier = new ArrayList<Identifier>(); 2389 this.identifier.add(t); 2390 return this; 2391 } 2392 2393 /** 2394 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2395 */ 2396 public Identifier getIdentifierFirstRep() { 2397 if (getIdentifier().isEmpty()) { 2398 addIdentifier(); 2399 } 2400 return getIdentifier().get(0); 2401 } 2402 2403 /** 2404 * @return {@link #type} (Regulatory type, e.g. Investigational or Authorized.) 2405 */ 2406 public CodeableConcept getType() { 2407 if (this.type == null) 2408 if (Configuration.errorOnAutoCreate()) 2409 throw new Error("Attempt to auto-create MedicinalProductDefinition.type"); 2410 else if (Configuration.doAutoCreate()) 2411 this.type = new CodeableConcept(); // cc 2412 return this.type; 2413 } 2414 2415 public boolean hasType() { 2416 return this.type != null && !this.type.isEmpty(); 2417 } 2418 2419 /** 2420 * @param value {@link #type} (Regulatory type, e.g. Investigational or Authorized.) 2421 */ 2422 public MedicinalProductDefinition setType(CodeableConcept value) { 2423 this.type = value; 2424 return this; 2425 } 2426 2427 /** 2428 * @return {@link #domain} (If this medicine applies to human or veterinary uses.) 2429 */ 2430 public CodeableConcept getDomain() { 2431 if (this.domain == null) 2432 if (Configuration.errorOnAutoCreate()) 2433 throw new Error("Attempt to auto-create MedicinalProductDefinition.domain"); 2434 else if (Configuration.doAutoCreate()) 2435 this.domain = new CodeableConcept(); // cc 2436 return this.domain; 2437 } 2438 2439 public boolean hasDomain() { 2440 return this.domain != null && !this.domain.isEmpty(); 2441 } 2442 2443 /** 2444 * @param value {@link #domain} (If this medicine applies to human or veterinary uses.) 2445 */ 2446 public MedicinalProductDefinition setDomain(CodeableConcept value) { 2447 this.domain = value; 2448 return this; 2449 } 2450 2451 /** 2452 * @return {@link #version} (A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2453 */ 2454 public StringType getVersionElement() { 2455 if (this.version == null) 2456 if (Configuration.errorOnAutoCreate()) 2457 throw new Error("Attempt to auto-create MedicinalProductDefinition.version"); 2458 else if (Configuration.doAutoCreate()) 2459 this.version = new StringType(); // bb 2460 return this.version; 2461 } 2462 2463 public boolean hasVersionElement() { 2464 return this.version != null && !this.version.isEmpty(); 2465 } 2466 2467 public boolean hasVersion() { 2468 return this.version != null && !this.version.isEmpty(); 2469 } 2470 2471 /** 2472 * @param value {@link #version} (A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2473 */ 2474 public MedicinalProductDefinition setVersionElement(StringType value) { 2475 this.version = value; 2476 return this; 2477 } 2478 2479 /** 2480 * @return A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product. 2481 */ 2482 public String getVersion() { 2483 return this.version == null ? null : this.version.getValue(); 2484 } 2485 2486 /** 2487 * @param value A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product. 2488 */ 2489 public MedicinalProductDefinition setVersion(String value) { 2490 if (Utilities.noString(value)) 2491 this.version = null; 2492 else { 2493 if (this.version == null) 2494 this.version = new StringType(); 2495 this.version.setValue(value); 2496 } 2497 return this; 2498 } 2499 2500 /** 2501 * @return {@link #status} (The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status.) 2502 */ 2503 public CodeableConcept getStatus() { 2504 if (this.status == null) 2505 if (Configuration.errorOnAutoCreate()) 2506 throw new Error("Attempt to auto-create MedicinalProductDefinition.status"); 2507 else if (Configuration.doAutoCreate()) 2508 this.status = new CodeableConcept(); // cc 2509 return this.status; 2510 } 2511 2512 public boolean hasStatus() { 2513 return this.status != null && !this.status.isEmpty(); 2514 } 2515 2516 /** 2517 * @param value {@link #status} (The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status.) 2518 */ 2519 public MedicinalProductDefinition setStatus(CodeableConcept value) { 2520 this.status = value; 2521 return this; 2522 } 2523 2524 /** 2525 * @return {@link #statusDate} (The date at which the given status became applicable.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 2526 */ 2527 public DateTimeType getStatusDateElement() { 2528 if (this.statusDate == null) 2529 if (Configuration.errorOnAutoCreate()) 2530 throw new Error("Attempt to auto-create MedicinalProductDefinition.statusDate"); 2531 else if (Configuration.doAutoCreate()) 2532 this.statusDate = new DateTimeType(); // bb 2533 return this.statusDate; 2534 } 2535 2536 public boolean hasStatusDateElement() { 2537 return this.statusDate != null && !this.statusDate.isEmpty(); 2538 } 2539 2540 public boolean hasStatusDate() { 2541 return this.statusDate != null && !this.statusDate.isEmpty(); 2542 } 2543 2544 /** 2545 * @param value {@link #statusDate} (The date at which the given status became applicable.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 2546 */ 2547 public MedicinalProductDefinition setStatusDateElement(DateTimeType value) { 2548 this.statusDate = value; 2549 return this; 2550 } 2551 2552 /** 2553 * @return The date at which the given status became applicable. 2554 */ 2555 public Date getStatusDate() { 2556 return this.statusDate == null ? null : this.statusDate.getValue(); 2557 } 2558 2559 /** 2560 * @param value The date at which the given status became applicable. 2561 */ 2562 public MedicinalProductDefinition setStatusDate(Date value) { 2563 if (value == null) 2564 this.statusDate = null; 2565 else { 2566 if (this.statusDate == null) 2567 this.statusDate = new DateTimeType(); 2568 this.statusDate.setValue(value); 2569 } 2570 return this; 2571 } 2572 2573 /** 2574 * @return {@link #description} (General description of this product.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2575 */ 2576 public MarkdownType getDescriptionElement() { 2577 if (this.description == null) 2578 if (Configuration.errorOnAutoCreate()) 2579 throw new Error("Attempt to auto-create MedicinalProductDefinition.description"); 2580 else if (Configuration.doAutoCreate()) 2581 this.description = new MarkdownType(); // bb 2582 return this.description; 2583 } 2584 2585 public boolean hasDescriptionElement() { 2586 return this.description != null && !this.description.isEmpty(); 2587 } 2588 2589 public boolean hasDescription() { 2590 return this.description != null && !this.description.isEmpty(); 2591 } 2592 2593 /** 2594 * @param value {@link #description} (General description of this product.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2595 */ 2596 public MedicinalProductDefinition setDescriptionElement(MarkdownType value) { 2597 this.description = value; 2598 return this; 2599 } 2600 2601 /** 2602 * @return General description of this product. 2603 */ 2604 public String getDescription() { 2605 return this.description == null ? null : this.description.getValue(); 2606 } 2607 2608 /** 2609 * @param value General description of this product. 2610 */ 2611 public MedicinalProductDefinition setDescription(String value) { 2612 if (Utilities.noString(value)) 2613 this.description = null; 2614 else { 2615 if (this.description == null) 2616 this.description = new MarkdownType(); 2617 this.description.setValue(value); 2618 } 2619 return this; 2620 } 2621 2622 /** 2623 * @return {@link #combinedPharmaceuticalDoseForm} (The dose form for a single part product, or combined form of a multiple part product. This is one concept that describes all the components. It does not represent the form with components physically mixed, if that might be necessary, for which see (AdministrableProductDefinition.administrableDoseForm).) 2624 */ 2625 public CodeableConcept getCombinedPharmaceuticalDoseForm() { 2626 if (this.combinedPharmaceuticalDoseForm == null) 2627 if (Configuration.errorOnAutoCreate()) 2628 throw new Error("Attempt to auto-create MedicinalProductDefinition.combinedPharmaceuticalDoseForm"); 2629 else if (Configuration.doAutoCreate()) 2630 this.combinedPharmaceuticalDoseForm = new CodeableConcept(); // cc 2631 return this.combinedPharmaceuticalDoseForm; 2632 } 2633 2634 public boolean hasCombinedPharmaceuticalDoseForm() { 2635 return this.combinedPharmaceuticalDoseForm != null && !this.combinedPharmaceuticalDoseForm.isEmpty(); 2636 } 2637 2638 /** 2639 * @param value {@link #combinedPharmaceuticalDoseForm} (The dose form for a single part product, or combined form of a multiple part product. This is one concept that describes all the components. It does not represent the form with components physically mixed, if that might be necessary, for which see (AdministrableProductDefinition.administrableDoseForm).) 2640 */ 2641 public MedicinalProductDefinition setCombinedPharmaceuticalDoseForm(CodeableConcept value) { 2642 this.combinedPharmaceuticalDoseForm = value; 2643 return this; 2644 } 2645 2646 /** 2647 * @return {@link #route} (The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. See also AdministrableProductDefinition resource. MedicinalProductDefinition.route is the same concept as AdministrableProductDefinition.routeOfAdministration.code, and they cannot be used together.) 2648 */ 2649 public List<CodeableConcept> getRoute() { 2650 if (this.route == null) 2651 this.route = new ArrayList<CodeableConcept>(); 2652 return this.route; 2653 } 2654 2655 /** 2656 * @return Returns a reference to <code>this</code> for easy method chaining 2657 */ 2658 public MedicinalProductDefinition setRoute(List<CodeableConcept> theRoute) { 2659 this.route = theRoute; 2660 return this; 2661 } 2662 2663 public boolean hasRoute() { 2664 if (this.route == null) 2665 return false; 2666 for (CodeableConcept item : this.route) 2667 if (!item.isEmpty()) 2668 return true; 2669 return false; 2670 } 2671 2672 public CodeableConcept addRoute() { //3 2673 CodeableConcept t = new CodeableConcept(); 2674 if (this.route == null) 2675 this.route = new ArrayList<CodeableConcept>(); 2676 this.route.add(t); 2677 return t; 2678 } 2679 2680 public MedicinalProductDefinition addRoute(CodeableConcept t) { //3 2681 if (t == null) 2682 return this; 2683 if (this.route == null) 2684 this.route = new ArrayList<CodeableConcept>(); 2685 this.route.add(t); 2686 return this; 2687 } 2688 2689 /** 2690 * @return The first repetition of repeating field {@link #route}, creating it if it does not already exist {3} 2691 */ 2692 public CodeableConcept getRouteFirstRep() { 2693 if (getRoute().isEmpty()) { 2694 addRoute(); 2695 } 2696 return getRoute().get(0); 2697 } 2698 2699 /** 2700 * @return {@link #indication} (Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate.). This is the underlying object with id, value and extensions. The accessor "getIndication" gives direct access to the value 2701 */ 2702 public MarkdownType getIndicationElement() { 2703 if (this.indication == null) 2704 if (Configuration.errorOnAutoCreate()) 2705 throw new Error("Attempt to auto-create MedicinalProductDefinition.indication"); 2706 else if (Configuration.doAutoCreate()) 2707 this.indication = new MarkdownType(); // bb 2708 return this.indication; 2709 } 2710 2711 public boolean hasIndicationElement() { 2712 return this.indication != null && !this.indication.isEmpty(); 2713 } 2714 2715 public boolean hasIndication() { 2716 return this.indication != null && !this.indication.isEmpty(); 2717 } 2718 2719 /** 2720 * @param value {@link #indication} (Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate.). This is the underlying object with id, value and extensions. The accessor "getIndication" gives direct access to the value 2721 */ 2722 public MedicinalProductDefinition setIndicationElement(MarkdownType value) { 2723 this.indication = value; 2724 return this; 2725 } 2726 2727 /** 2728 * @return Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate. 2729 */ 2730 public String getIndication() { 2731 return this.indication == null ? null : this.indication.getValue(); 2732 } 2733 2734 /** 2735 * @param value Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate. 2736 */ 2737 public MedicinalProductDefinition setIndication(String value) { 2738 if (Utilities.noString(value)) 2739 this.indication = null; 2740 else { 2741 if (this.indication == null) 2742 this.indication = new MarkdownType(); 2743 this.indication.setValue(value); 2744 } 2745 return this; 2746 } 2747 2748 /** 2749 * @return {@link #legalStatusOfSupply} (The legal status of supply of the medicinal product as classified by the regulator.) 2750 */ 2751 public CodeableConcept getLegalStatusOfSupply() { 2752 if (this.legalStatusOfSupply == null) 2753 if (Configuration.errorOnAutoCreate()) 2754 throw new Error("Attempt to auto-create MedicinalProductDefinition.legalStatusOfSupply"); 2755 else if (Configuration.doAutoCreate()) 2756 this.legalStatusOfSupply = new CodeableConcept(); // cc 2757 return this.legalStatusOfSupply; 2758 } 2759 2760 public boolean hasLegalStatusOfSupply() { 2761 return this.legalStatusOfSupply != null && !this.legalStatusOfSupply.isEmpty(); 2762 } 2763 2764 /** 2765 * @param value {@link #legalStatusOfSupply} (The legal status of supply of the medicinal product as classified by the regulator.) 2766 */ 2767 public MedicinalProductDefinition setLegalStatusOfSupply(CodeableConcept value) { 2768 this.legalStatusOfSupply = value; 2769 return this; 2770 } 2771 2772 /** 2773 * @return {@link #additionalMonitoringIndicator} (Whether the Medicinal Product is subject to additional monitoring for regulatory reasons, such as heightened reporting requirements.) 2774 */ 2775 public CodeableConcept getAdditionalMonitoringIndicator() { 2776 if (this.additionalMonitoringIndicator == null) 2777 if (Configuration.errorOnAutoCreate()) 2778 throw new Error("Attempt to auto-create MedicinalProductDefinition.additionalMonitoringIndicator"); 2779 else if (Configuration.doAutoCreate()) 2780 this.additionalMonitoringIndicator = new CodeableConcept(); // cc 2781 return this.additionalMonitoringIndicator; 2782 } 2783 2784 public boolean hasAdditionalMonitoringIndicator() { 2785 return this.additionalMonitoringIndicator != null && !this.additionalMonitoringIndicator.isEmpty(); 2786 } 2787 2788 /** 2789 * @param value {@link #additionalMonitoringIndicator} (Whether the Medicinal Product is subject to additional monitoring for regulatory reasons, such as heightened reporting requirements.) 2790 */ 2791 public MedicinalProductDefinition setAdditionalMonitoringIndicator(CodeableConcept value) { 2792 this.additionalMonitoringIndicator = value; 2793 return this; 2794 } 2795 2796 /** 2797 * @return {@link #specialMeasures} (Whether the Medicinal Product is subject to special measures for regulatory reasons, such as a requirement to conduct post-authorization studies.) 2798 */ 2799 public List<CodeableConcept> getSpecialMeasures() { 2800 if (this.specialMeasures == null) 2801 this.specialMeasures = new ArrayList<CodeableConcept>(); 2802 return this.specialMeasures; 2803 } 2804 2805 /** 2806 * @return Returns a reference to <code>this</code> for easy method chaining 2807 */ 2808 public MedicinalProductDefinition setSpecialMeasures(List<CodeableConcept> theSpecialMeasures) { 2809 this.specialMeasures = theSpecialMeasures; 2810 return this; 2811 } 2812 2813 public boolean hasSpecialMeasures() { 2814 if (this.specialMeasures == null) 2815 return false; 2816 for (CodeableConcept item : this.specialMeasures) 2817 if (!item.isEmpty()) 2818 return true; 2819 return false; 2820 } 2821 2822 public CodeableConcept addSpecialMeasures() { //3 2823 CodeableConcept t = new CodeableConcept(); 2824 if (this.specialMeasures == null) 2825 this.specialMeasures = new ArrayList<CodeableConcept>(); 2826 this.specialMeasures.add(t); 2827 return t; 2828 } 2829 2830 public MedicinalProductDefinition addSpecialMeasures(CodeableConcept t) { //3 2831 if (t == null) 2832 return this; 2833 if (this.specialMeasures == null) 2834 this.specialMeasures = new ArrayList<CodeableConcept>(); 2835 this.specialMeasures.add(t); 2836 return this; 2837 } 2838 2839 /** 2840 * @return The first repetition of repeating field {@link #specialMeasures}, creating it if it does not already exist {3} 2841 */ 2842 public CodeableConcept getSpecialMeasuresFirstRep() { 2843 if (getSpecialMeasures().isEmpty()) { 2844 addSpecialMeasures(); 2845 } 2846 return getSpecialMeasures().get(0); 2847 } 2848 2849 /** 2850 * @return {@link #pediatricUseIndicator} (If authorised for use in children, or infants, neonates etc.) 2851 */ 2852 public CodeableConcept getPediatricUseIndicator() { 2853 if (this.pediatricUseIndicator == null) 2854 if (Configuration.errorOnAutoCreate()) 2855 throw new Error("Attempt to auto-create MedicinalProductDefinition.pediatricUseIndicator"); 2856 else if (Configuration.doAutoCreate()) 2857 this.pediatricUseIndicator = new CodeableConcept(); // cc 2858 return this.pediatricUseIndicator; 2859 } 2860 2861 public boolean hasPediatricUseIndicator() { 2862 return this.pediatricUseIndicator != null && !this.pediatricUseIndicator.isEmpty(); 2863 } 2864 2865 /** 2866 * @param value {@link #pediatricUseIndicator} (If authorised for use in children, or infants, neonates etc.) 2867 */ 2868 public MedicinalProductDefinition setPediatricUseIndicator(CodeableConcept value) { 2869 this.pediatricUseIndicator = value; 2870 return this; 2871 } 2872 2873 /** 2874 * @return {@link #classification} (Allows the product to be classified by various systems, commonly WHO ATC.) 2875 */ 2876 public List<CodeableConcept> getClassification() { 2877 if (this.classification == null) 2878 this.classification = new ArrayList<CodeableConcept>(); 2879 return this.classification; 2880 } 2881 2882 /** 2883 * @return Returns a reference to <code>this</code> for easy method chaining 2884 */ 2885 public MedicinalProductDefinition setClassification(List<CodeableConcept> theClassification) { 2886 this.classification = theClassification; 2887 return this; 2888 } 2889 2890 public boolean hasClassification() { 2891 if (this.classification == null) 2892 return false; 2893 for (CodeableConcept item : this.classification) 2894 if (!item.isEmpty()) 2895 return true; 2896 return false; 2897 } 2898 2899 public CodeableConcept addClassification() { //3 2900 CodeableConcept t = new CodeableConcept(); 2901 if (this.classification == null) 2902 this.classification = new ArrayList<CodeableConcept>(); 2903 this.classification.add(t); 2904 return t; 2905 } 2906 2907 public MedicinalProductDefinition addClassification(CodeableConcept t) { //3 2908 if (t == null) 2909 return this; 2910 if (this.classification == null) 2911 this.classification = new ArrayList<CodeableConcept>(); 2912 this.classification.add(t); 2913 return this; 2914 } 2915 2916 /** 2917 * @return The first repetition of repeating field {@link #classification}, creating it if it does not already exist {3} 2918 */ 2919 public CodeableConcept getClassificationFirstRep() { 2920 if (getClassification().isEmpty()) { 2921 addClassification(); 2922 } 2923 return getClassification().get(0); 2924 } 2925 2926 /** 2927 * @return {@link #marketingStatus} (Marketing status of the medicinal product, in contrast to marketing authorization. This refers to the product being actually 'on the market' as opposed to being allowed to be on the market (which is an authorization).) 2928 */ 2929 public List<MarketingStatus> getMarketingStatus() { 2930 if (this.marketingStatus == null) 2931 this.marketingStatus = new ArrayList<MarketingStatus>(); 2932 return this.marketingStatus; 2933 } 2934 2935 /** 2936 * @return Returns a reference to <code>this</code> for easy method chaining 2937 */ 2938 public MedicinalProductDefinition setMarketingStatus(List<MarketingStatus> theMarketingStatus) { 2939 this.marketingStatus = theMarketingStatus; 2940 return this; 2941 } 2942 2943 public boolean hasMarketingStatus() { 2944 if (this.marketingStatus == null) 2945 return false; 2946 for (MarketingStatus item : this.marketingStatus) 2947 if (!item.isEmpty()) 2948 return true; 2949 return false; 2950 } 2951 2952 public MarketingStatus addMarketingStatus() { //3 2953 MarketingStatus t = new MarketingStatus(); 2954 if (this.marketingStatus == null) 2955 this.marketingStatus = new ArrayList<MarketingStatus>(); 2956 this.marketingStatus.add(t); 2957 return t; 2958 } 2959 2960 public MedicinalProductDefinition addMarketingStatus(MarketingStatus t) { //3 2961 if (t == null) 2962 return this; 2963 if (this.marketingStatus == null) 2964 this.marketingStatus = new ArrayList<MarketingStatus>(); 2965 this.marketingStatus.add(t); 2966 return this; 2967 } 2968 2969 /** 2970 * @return The first repetition of repeating field {@link #marketingStatus}, creating it if it does not already exist {3} 2971 */ 2972 public MarketingStatus getMarketingStatusFirstRep() { 2973 if (getMarketingStatus().isEmpty()) { 2974 addMarketingStatus(); 2975 } 2976 return getMarketingStatus().get(0); 2977 } 2978 2979 /** 2980 * @return {@link #packagedMedicinalProduct} (Package type for the product. See also the PackagedProductDefinition resource.) 2981 */ 2982 public List<CodeableConcept> getPackagedMedicinalProduct() { 2983 if (this.packagedMedicinalProduct == null) 2984 this.packagedMedicinalProduct = new ArrayList<CodeableConcept>(); 2985 return this.packagedMedicinalProduct; 2986 } 2987 2988 /** 2989 * @return Returns a reference to <code>this</code> for easy method chaining 2990 */ 2991 public MedicinalProductDefinition setPackagedMedicinalProduct(List<CodeableConcept> thePackagedMedicinalProduct) { 2992 this.packagedMedicinalProduct = thePackagedMedicinalProduct; 2993 return this; 2994 } 2995 2996 public boolean hasPackagedMedicinalProduct() { 2997 if (this.packagedMedicinalProduct == null) 2998 return false; 2999 for (CodeableConcept item : this.packagedMedicinalProduct) 3000 if (!item.isEmpty()) 3001 return true; 3002 return false; 3003 } 3004 3005 public CodeableConcept addPackagedMedicinalProduct() { //3 3006 CodeableConcept t = new CodeableConcept(); 3007 if (this.packagedMedicinalProduct == null) 3008 this.packagedMedicinalProduct = new ArrayList<CodeableConcept>(); 3009 this.packagedMedicinalProduct.add(t); 3010 return t; 3011 } 3012 3013 public MedicinalProductDefinition addPackagedMedicinalProduct(CodeableConcept t) { //3 3014 if (t == null) 3015 return this; 3016 if (this.packagedMedicinalProduct == null) 3017 this.packagedMedicinalProduct = new ArrayList<CodeableConcept>(); 3018 this.packagedMedicinalProduct.add(t); 3019 return this; 3020 } 3021 3022 /** 3023 * @return The first repetition of repeating field {@link #packagedMedicinalProduct}, creating it if it does not already exist {3} 3024 */ 3025 public CodeableConcept getPackagedMedicinalProductFirstRep() { 3026 if (getPackagedMedicinalProduct().isEmpty()) { 3027 addPackagedMedicinalProduct(); 3028 } 3029 return getPackagedMedicinalProduct().get(0); 3030 } 3031 3032 /** 3033 * @return {@link #comprisedOf} (Types of medicinal manufactured items and/or devices that this product consists of, such as tablets, capsule, or syringes. Used as a direct link when the item's packaging is not being recorded (see also PackagedProductDefinition.package.containedItem.item).) 3034 */ 3035 public List<Reference> getComprisedOf() { 3036 if (this.comprisedOf == null) 3037 this.comprisedOf = new ArrayList<Reference>(); 3038 return this.comprisedOf; 3039 } 3040 3041 /** 3042 * @return Returns a reference to <code>this</code> for easy method chaining 3043 */ 3044 public MedicinalProductDefinition setComprisedOf(List<Reference> theComprisedOf) { 3045 this.comprisedOf = theComprisedOf; 3046 return this; 3047 } 3048 3049 public boolean hasComprisedOf() { 3050 if (this.comprisedOf == null) 3051 return false; 3052 for (Reference item : this.comprisedOf) 3053 if (!item.isEmpty()) 3054 return true; 3055 return false; 3056 } 3057 3058 public Reference addComprisedOf() { //3 3059 Reference t = new Reference(); 3060 if (this.comprisedOf == null) 3061 this.comprisedOf = new ArrayList<Reference>(); 3062 this.comprisedOf.add(t); 3063 return t; 3064 } 3065 3066 public MedicinalProductDefinition addComprisedOf(Reference t) { //3 3067 if (t == null) 3068 return this; 3069 if (this.comprisedOf == null) 3070 this.comprisedOf = new ArrayList<Reference>(); 3071 this.comprisedOf.add(t); 3072 return this; 3073 } 3074 3075 /** 3076 * @return The first repetition of repeating field {@link #comprisedOf}, creating it if it does not already exist {3} 3077 */ 3078 public Reference getComprisedOfFirstRep() { 3079 if (getComprisedOf().isEmpty()) { 3080 addComprisedOf(); 3081 } 3082 return getComprisedOf().get(0); 3083 } 3084 3085 /** 3086 * @return {@link #ingredient} (The ingredients of this medicinal product - when not detailed in other resources. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource, or indirectly via incoming AdministrableProductDefinition, PackagedProductDefinition or ManufacturedItemDefinition references. In cases where those levels of detail are not used, the ingredients may be specified directly here as codes.) 3087 */ 3088 public List<CodeableConcept> getIngredient() { 3089 if (this.ingredient == null) 3090 this.ingredient = new ArrayList<CodeableConcept>(); 3091 return this.ingredient; 3092 } 3093 3094 /** 3095 * @return Returns a reference to <code>this</code> for easy method chaining 3096 */ 3097 public MedicinalProductDefinition setIngredient(List<CodeableConcept> theIngredient) { 3098 this.ingredient = theIngredient; 3099 return this; 3100 } 3101 3102 public boolean hasIngredient() { 3103 if (this.ingredient == null) 3104 return false; 3105 for (CodeableConcept item : this.ingredient) 3106 if (!item.isEmpty()) 3107 return true; 3108 return false; 3109 } 3110 3111 public CodeableConcept addIngredient() { //3 3112 CodeableConcept t = new CodeableConcept(); 3113 if (this.ingredient == null) 3114 this.ingredient = new ArrayList<CodeableConcept>(); 3115 this.ingredient.add(t); 3116 return t; 3117 } 3118 3119 public MedicinalProductDefinition addIngredient(CodeableConcept t) { //3 3120 if (t == null) 3121 return this; 3122 if (this.ingredient == null) 3123 this.ingredient = new ArrayList<CodeableConcept>(); 3124 this.ingredient.add(t); 3125 return this; 3126 } 3127 3128 /** 3129 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 3130 */ 3131 public CodeableConcept getIngredientFirstRep() { 3132 if (getIngredient().isEmpty()) { 3133 addIngredient(); 3134 } 3135 return getIngredient().get(0); 3136 } 3137 3138 /** 3139 * @return {@link #impurity} (Any component of the drug product which is not the chemical entity defined as the drug substance, or an excipient in the drug product. This includes process-related impurities and contaminants, product-related impurities including degradation products.) 3140 */ 3141 public List<CodeableReference> getImpurity() { 3142 if (this.impurity == null) 3143 this.impurity = new ArrayList<CodeableReference>(); 3144 return this.impurity; 3145 } 3146 3147 /** 3148 * @return Returns a reference to <code>this</code> for easy method chaining 3149 */ 3150 public MedicinalProductDefinition setImpurity(List<CodeableReference> theImpurity) { 3151 this.impurity = theImpurity; 3152 return this; 3153 } 3154 3155 public boolean hasImpurity() { 3156 if (this.impurity == null) 3157 return false; 3158 for (CodeableReference item : this.impurity) 3159 if (!item.isEmpty()) 3160 return true; 3161 return false; 3162 } 3163 3164 public CodeableReference addImpurity() { //3 3165 CodeableReference t = new CodeableReference(); 3166 if (this.impurity == null) 3167 this.impurity = new ArrayList<CodeableReference>(); 3168 this.impurity.add(t); 3169 return t; 3170 } 3171 3172 public MedicinalProductDefinition addImpurity(CodeableReference t) { //3 3173 if (t == null) 3174 return this; 3175 if (this.impurity == null) 3176 this.impurity = new ArrayList<CodeableReference>(); 3177 this.impurity.add(t); 3178 return this; 3179 } 3180 3181 /** 3182 * @return The first repetition of repeating field {@link #impurity}, creating it if it does not already exist {3} 3183 */ 3184 public CodeableReference getImpurityFirstRep() { 3185 if (getImpurity().isEmpty()) { 3186 addImpurity(); 3187 } 3188 return getImpurity().get(0); 3189 } 3190 3191 /** 3192 * @return {@link #attachedDocument} (Additional information or supporting documentation about the medicinal product.) 3193 */ 3194 public List<Reference> getAttachedDocument() { 3195 if (this.attachedDocument == null) 3196 this.attachedDocument = new ArrayList<Reference>(); 3197 return this.attachedDocument; 3198 } 3199 3200 /** 3201 * @return Returns a reference to <code>this</code> for easy method chaining 3202 */ 3203 public MedicinalProductDefinition setAttachedDocument(List<Reference> theAttachedDocument) { 3204 this.attachedDocument = theAttachedDocument; 3205 return this; 3206 } 3207 3208 public boolean hasAttachedDocument() { 3209 if (this.attachedDocument == null) 3210 return false; 3211 for (Reference item : this.attachedDocument) 3212 if (!item.isEmpty()) 3213 return true; 3214 return false; 3215 } 3216 3217 public Reference addAttachedDocument() { //3 3218 Reference t = new Reference(); 3219 if (this.attachedDocument == null) 3220 this.attachedDocument = new ArrayList<Reference>(); 3221 this.attachedDocument.add(t); 3222 return t; 3223 } 3224 3225 public MedicinalProductDefinition addAttachedDocument(Reference t) { //3 3226 if (t == null) 3227 return this; 3228 if (this.attachedDocument == null) 3229 this.attachedDocument = new ArrayList<Reference>(); 3230 this.attachedDocument.add(t); 3231 return this; 3232 } 3233 3234 /** 3235 * @return The first repetition of repeating field {@link #attachedDocument}, creating it if it does not already exist {3} 3236 */ 3237 public Reference getAttachedDocumentFirstRep() { 3238 if (getAttachedDocument().isEmpty()) { 3239 addAttachedDocument(); 3240 } 3241 return getAttachedDocument().get(0); 3242 } 3243 3244 /** 3245 * @return {@link #masterFile} (A master file for the medicinal product (e.g. Pharmacovigilance System Master File). Drug master files (DMFs) are documents submitted to regulatory agencies to provide confidential detailed information about facilities, processes or articles used in the manufacturing, processing, packaging and storing of drug products.) 3246 */ 3247 public List<Reference> getMasterFile() { 3248 if (this.masterFile == null) 3249 this.masterFile = new ArrayList<Reference>(); 3250 return this.masterFile; 3251 } 3252 3253 /** 3254 * @return Returns a reference to <code>this</code> for easy method chaining 3255 */ 3256 public MedicinalProductDefinition setMasterFile(List<Reference> theMasterFile) { 3257 this.masterFile = theMasterFile; 3258 return this; 3259 } 3260 3261 public boolean hasMasterFile() { 3262 if (this.masterFile == null) 3263 return false; 3264 for (Reference item : this.masterFile) 3265 if (!item.isEmpty()) 3266 return true; 3267 return false; 3268 } 3269 3270 public Reference addMasterFile() { //3 3271 Reference t = new Reference(); 3272 if (this.masterFile == null) 3273 this.masterFile = new ArrayList<Reference>(); 3274 this.masterFile.add(t); 3275 return t; 3276 } 3277 3278 public MedicinalProductDefinition addMasterFile(Reference t) { //3 3279 if (t == null) 3280 return this; 3281 if (this.masterFile == null) 3282 this.masterFile = new ArrayList<Reference>(); 3283 this.masterFile.add(t); 3284 return this; 3285 } 3286 3287 /** 3288 * @return The first repetition of repeating field {@link #masterFile}, creating it if it does not already exist {3} 3289 */ 3290 public Reference getMasterFileFirstRep() { 3291 if (getMasterFile().isEmpty()) { 3292 addMasterFile(); 3293 } 3294 return getMasterFile().get(0); 3295 } 3296 3297 /** 3298 * @return {@link #contact} (A product specific contact, person (in a role), or an organization.) 3299 */ 3300 public List<MedicinalProductDefinitionContactComponent> getContact() { 3301 if (this.contact == null) 3302 this.contact = new ArrayList<MedicinalProductDefinitionContactComponent>(); 3303 return this.contact; 3304 } 3305 3306 /** 3307 * @return Returns a reference to <code>this</code> for easy method chaining 3308 */ 3309 public MedicinalProductDefinition setContact(List<MedicinalProductDefinitionContactComponent> theContact) { 3310 this.contact = theContact; 3311 return this; 3312 } 3313 3314 public boolean hasContact() { 3315 if (this.contact == null) 3316 return false; 3317 for (MedicinalProductDefinitionContactComponent item : this.contact) 3318 if (!item.isEmpty()) 3319 return true; 3320 return false; 3321 } 3322 3323 public MedicinalProductDefinitionContactComponent addContact() { //3 3324 MedicinalProductDefinitionContactComponent t = new MedicinalProductDefinitionContactComponent(); 3325 if (this.contact == null) 3326 this.contact = new ArrayList<MedicinalProductDefinitionContactComponent>(); 3327 this.contact.add(t); 3328 return t; 3329 } 3330 3331 public MedicinalProductDefinition addContact(MedicinalProductDefinitionContactComponent t) { //3 3332 if (t == null) 3333 return this; 3334 if (this.contact == null) 3335 this.contact = new ArrayList<MedicinalProductDefinitionContactComponent>(); 3336 this.contact.add(t); 3337 return this; 3338 } 3339 3340 /** 3341 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3342 */ 3343 public MedicinalProductDefinitionContactComponent getContactFirstRep() { 3344 if (getContact().isEmpty()) { 3345 addContact(); 3346 } 3347 return getContact().get(0); 3348 } 3349 3350 /** 3351 * @return {@link #clinicalTrial} (Clinical trials or studies that this product is involved in.) 3352 */ 3353 public List<Reference> getClinicalTrial() { 3354 if (this.clinicalTrial == null) 3355 this.clinicalTrial = new ArrayList<Reference>(); 3356 return this.clinicalTrial; 3357 } 3358 3359 /** 3360 * @return Returns a reference to <code>this</code> for easy method chaining 3361 */ 3362 public MedicinalProductDefinition setClinicalTrial(List<Reference> theClinicalTrial) { 3363 this.clinicalTrial = theClinicalTrial; 3364 return this; 3365 } 3366 3367 public boolean hasClinicalTrial() { 3368 if (this.clinicalTrial == null) 3369 return false; 3370 for (Reference item : this.clinicalTrial) 3371 if (!item.isEmpty()) 3372 return true; 3373 return false; 3374 } 3375 3376 public Reference addClinicalTrial() { //3 3377 Reference t = new Reference(); 3378 if (this.clinicalTrial == null) 3379 this.clinicalTrial = new ArrayList<Reference>(); 3380 this.clinicalTrial.add(t); 3381 return t; 3382 } 3383 3384 public MedicinalProductDefinition addClinicalTrial(Reference t) { //3 3385 if (t == null) 3386 return this; 3387 if (this.clinicalTrial == null) 3388 this.clinicalTrial = new ArrayList<Reference>(); 3389 this.clinicalTrial.add(t); 3390 return this; 3391 } 3392 3393 /** 3394 * @return The first repetition of repeating field {@link #clinicalTrial}, creating it if it does not already exist {3} 3395 */ 3396 public Reference getClinicalTrialFirstRep() { 3397 if (getClinicalTrial().isEmpty()) { 3398 addClinicalTrial(); 3399 } 3400 return getClinicalTrial().get(0); 3401 } 3402 3403 /** 3404 * @return {@link #code} (A code that this product is known by, usually within some formal terminology, perhaps assigned by a third party (i.e. not the manufacturer or regulator). Products (types of medications) tend to be known by identifiers during development and within regulatory process. However when they are prescribed they tend to be identified by codes. The same product may be have multiple codes, applied to it by multiple organizations.) 3405 */ 3406 public List<Coding> getCode() { 3407 if (this.code == null) 3408 this.code = new ArrayList<Coding>(); 3409 return this.code; 3410 } 3411 3412 /** 3413 * @return Returns a reference to <code>this</code> for easy method chaining 3414 */ 3415 public MedicinalProductDefinition setCode(List<Coding> theCode) { 3416 this.code = theCode; 3417 return this; 3418 } 3419 3420 public boolean hasCode() { 3421 if (this.code == null) 3422 return false; 3423 for (Coding item : this.code) 3424 if (!item.isEmpty()) 3425 return true; 3426 return false; 3427 } 3428 3429 public Coding addCode() { //3 3430 Coding t = new Coding(); 3431 if (this.code == null) 3432 this.code = new ArrayList<Coding>(); 3433 this.code.add(t); 3434 return t; 3435 } 3436 3437 public MedicinalProductDefinition addCode(Coding t) { //3 3438 if (t == null) 3439 return this; 3440 if (this.code == null) 3441 this.code = new ArrayList<Coding>(); 3442 this.code.add(t); 3443 return this; 3444 } 3445 3446 /** 3447 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 3448 */ 3449 public Coding getCodeFirstRep() { 3450 if (getCode().isEmpty()) { 3451 addCode(); 3452 } 3453 return getCode().get(0); 3454 } 3455 3456 /** 3457 * @return {@link #name} (The product's name, including full name and possibly coded parts.) 3458 */ 3459 public List<MedicinalProductDefinitionNameComponent> getName() { 3460 if (this.name == null) 3461 this.name = new ArrayList<MedicinalProductDefinitionNameComponent>(); 3462 return this.name; 3463 } 3464 3465 /** 3466 * @return Returns a reference to <code>this</code> for easy method chaining 3467 */ 3468 public MedicinalProductDefinition setName(List<MedicinalProductDefinitionNameComponent> theName) { 3469 this.name = theName; 3470 return this; 3471 } 3472 3473 public boolean hasName() { 3474 if (this.name == null) 3475 return false; 3476 for (MedicinalProductDefinitionNameComponent item : this.name) 3477 if (!item.isEmpty()) 3478 return true; 3479 return false; 3480 } 3481 3482 public MedicinalProductDefinitionNameComponent addName() { //3 3483 MedicinalProductDefinitionNameComponent t = new MedicinalProductDefinitionNameComponent(); 3484 if (this.name == null) 3485 this.name = new ArrayList<MedicinalProductDefinitionNameComponent>(); 3486 this.name.add(t); 3487 return t; 3488 } 3489 3490 public MedicinalProductDefinition addName(MedicinalProductDefinitionNameComponent t) { //3 3491 if (t == null) 3492 return this; 3493 if (this.name == null) 3494 this.name = new ArrayList<MedicinalProductDefinitionNameComponent>(); 3495 this.name.add(t); 3496 return this; 3497 } 3498 3499 /** 3500 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 3501 */ 3502 public MedicinalProductDefinitionNameComponent getNameFirstRep() { 3503 if (getName().isEmpty()) { 3504 addName(); 3505 } 3506 return getName().get(0); 3507 } 3508 3509 /** 3510 * @return {@link #crossReference} (Reference to another product, e.g. for linking authorised to investigational product, or a virtual product.) 3511 */ 3512 public List<MedicinalProductDefinitionCrossReferenceComponent> getCrossReference() { 3513 if (this.crossReference == null) 3514 this.crossReference = new ArrayList<MedicinalProductDefinitionCrossReferenceComponent>(); 3515 return this.crossReference; 3516 } 3517 3518 /** 3519 * @return Returns a reference to <code>this</code> for easy method chaining 3520 */ 3521 public MedicinalProductDefinition setCrossReference(List<MedicinalProductDefinitionCrossReferenceComponent> theCrossReference) { 3522 this.crossReference = theCrossReference; 3523 return this; 3524 } 3525 3526 public boolean hasCrossReference() { 3527 if (this.crossReference == null) 3528 return false; 3529 for (MedicinalProductDefinitionCrossReferenceComponent item : this.crossReference) 3530 if (!item.isEmpty()) 3531 return true; 3532 return false; 3533 } 3534 3535 public MedicinalProductDefinitionCrossReferenceComponent addCrossReference() { //3 3536 MedicinalProductDefinitionCrossReferenceComponent t = new MedicinalProductDefinitionCrossReferenceComponent(); 3537 if (this.crossReference == null) 3538 this.crossReference = new ArrayList<MedicinalProductDefinitionCrossReferenceComponent>(); 3539 this.crossReference.add(t); 3540 return t; 3541 } 3542 3543 public MedicinalProductDefinition addCrossReference(MedicinalProductDefinitionCrossReferenceComponent t) { //3 3544 if (t == null) 3545 return this; 3546 if (this.crossReference == null) 3547 this.crossReference = new ArrayList<MedicinalProductDefinitionCrossReferenceComponent>(); 3548 this.crossReference.add(t); 3549 return this; 3550 } 3551 3552 /** 3553 * @return The first repetition of repeating field {@link #crossReference}, creating it if it does not already exist {3} 3554 */ 3555 public MedicinalProductDefinitionCrossReferenceComponent getCrossReferenceFirstRep() { 3556 if (getCrossReference().isEmpty()) { 3557 addCrossReference(); 3558 } 3559 return getCrossReference().get(0); 3560 } 3561 3562 /** 3563 * @return {@link #operation} (A manufacturing or administrative process or step associated with (or performed on) the medicinal product.) 3564 */ 3565 public List<MedicinalProductDefinitionOperationComponent> getOperation() { 3566 if (this.operation == null) 3567 this.operation = new ArrayList<MedicinalProductDefinitionOperationComponent>(); 3568 return this.operation; 3569 } 3570 3571 /** 3572 * @return Returns a reference to <code>this</code> for easy method chaining 3573 */ 3574 public MedicinalProductDefinition setOperation(List<MedicinalProductDefinitionOperationComponent> theOperation) { 3575 this.operation = theOperation; 3576 return this; 3577 } 3578 3579 public boolean hasOperation() { 3580 if (this.operation == null) 3581 return false; 3582 for (MedicinalProductDefinitionOperationComponent item : this.operation) 3583 if (!item.isEmpty()) 3584 return true; 3585 return false; 3586 } 3587 3588 public MedicinalProductDefinitionOperationComponent addOperation() { //3 3589 MedicinalProductDefinitionOperationComponent t = new MedicinalProductDefinitionOperationComponent(); 3590 if (this.operation == null) 3591 this.operation = new ArrayList<MedicinalProductDefinitionOperationComponent>(); 3592 this.operation.add(t); 3593 return t; 3594 } 3595 3596 public MedicinalProductDefinition addOperation(MedicinalProductDefinitionOperationComponent t) { //3 3597 if (t == null) 3598 return this; 3599 if (this.operation == null) 3600 this.operation = new ArrayList<MedicinalProductDefinitionOperationComponent>(); 3601 this.operation.add(t); 3602 return this; 3603 } 3604 3605 /** 3606 * @return The first repetition of repeating field {@link #operation}, creating it if it does not already exist {3} 3607 */ 3608 public MedicinalProductDefinitionOperationComponent getOperationFirstRep() { 3609 if (getOperation().isEmpty()) { 3610 addOperation(); 3611 } 3612 return getOperation().get(0); 3613 } 3614 3615 /** 3616 * @return {@link #characteristic} (Allows the key product features to be recorded, such as "sugar free", "modified release", "parallel import".) 3617 */ 3618 public List<MedicinalProductDefinitionCharacteristicComponent> getCharacteristic() { 3619 if (this.characteristic == null) 3620 this.characteristic = new ArrayList<MedicinalProductDefinitionCharacteristicComponent>(); 3621 return this.characteristic; 3622 } 3623 3624 /** 3625 * @return Returns a reference to <code>this</code> for easy method chaining 3626 */ 3627 public MedicinalProductDefinition setCharacteristic(List<MedicinalProductDefinitionCharacteristicComponent> theCharacteristic) { 3628 this.characteristic = theCharacteristic; 3629 return this; 3630 } 3631 3632 public boolean hasCharacteristic() { 3633 if (this.characteristic == null) 3634 return false; 3635 for (MedicinalProductDefinitionCharacteristicComponent item : this.characteristic) 3636 if (!item.isEmpty()) 3637 return true; 3638 return false; 3639 } 3640 3641 public MedicinalProductDefinitionCharacteristicComponent addCharacteristic() { //3 3642 MedicinalProductDefinitionCharacteristicComponent t = new MedicinalProductDefinitionCharacteristicComponent(); 3643 if (this.characteristic == null) 3644 this.characteristic = new ArrayList<MedicinalProductDefinitionCharacteristicComponent>(); 3645 this.characteristic.add(t); 3646 return t; 3647 } 3648 3649 public MedicinalProductDefinition addCharacteristic(MedicinalProductDefinitionCharacteristicComponent t) { //3 3650 if (t == null) 3651 return this; 3652 if (this.characteristic == null) 3653 this.characteristic = new ArrayList<MedicinalProductDefinitionCharacteristicComponent>(); 3654 this.characteristic.add(t); 3655 return this; 3656 } 3657 3658 /** 3659 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 3660 */ 3661 public MedicinalProductDefinitionCharacteristicComponent getCharacteristicFirstRep() { 3662 if (getCharacteristic().isEmpty()) { 3663 addCharacteristic(); 3664 } 3665 return getCharacteristic().get(0); 3666 } 3667 3668 protected void listChildren(List<Property> children) { 3669 super.listChildren(children); 3670 children.add(new Property("identifier", "Identifier", "Business identifier for this product. Could be an MPID. When in development or being regulated, products are typically referenced by official identifiers, assigned by a manufacturer or regulator, and unique to a product (which, when compared to a product instance being prescribed, is actually a product type). See also MedicinalProductDefinition.code.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3671 children.add(new Property("type", "CodeableConcept", "Regulatory type, e.g. Investigational or Authorized.", 0, 1, type)); 3672 children.add(new Property("domain", "CodeableConcept", "If this medicine applies to human or veterinary uses.", 0, 1, domain)); 3673 children.add(new Property("version", "string", "A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product.", 0, 1, version)); 3674 children.add(new Property("status", "CodeableConcept", "The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status.", 0, 1, status)); 3675 children.add(new Property("statusDate", "dateTime", "The date at which the given status became applicable.", 0, 1, statusDate)); 3676 children.add(new Property("description", "markdown", "General description of this product.", 0, 1, description)); 3677 children.add(new Property("combinedPharmaceuticalDoseForm", "CodeableConcept", "The dose form for a single part product, or combined form of a multiple part product. This is one concept that describes all the components. It does not represent the form with components physically mixed, if that might be necessary, for which see (AdministrableProductDefinition.administrableDoseForm).", 0, 1, combinedPharmaceuticalDoseForm)); 3678 children.add(new Property("route", "CodeableConcept", "The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. See also AdministrableProductDefinition resource. MedicinalProductDefinition.route is the same concept as AdministrableProductDefinition.routeOfAdministration.code, and they cannot be used together.", 0, java.lang.Integer.MAX_VALUE, route)); 3679 children.add(new Property("indication", "markdown", "Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate.", 0, 1, indication)); 3680 children.add(new Property("legalStatusOfSupply", "CodeableConcept", "The legal status of supply of the medicinal product as classified by the regulator.", 0, 1, legalStatusOfSupply)); 3681 children.add(new Property("additionalMonitoringIndicator", "CodeableConcept", "Whether the Medicinal Product is subject to additional monitoring for regulatory reasons, such as heightened reporting requirements.", 0, 1, additionalMonitoringIndicator)); 3682 children.add(new Property("specialMeasures", "CodeableConcept", "Whether the Medicinal Product is subject to special measures for regulatory reasons, such as a requirement to conduct post-authorization studies.", 0, java.lang.Integer.MAX_VALUE, specialMeasures)); 3683 children.add(new Property("pediatricUseIndicator", "CodeableConcept", "If authorised for use in children, or infants, neonates etc.", 0, 1, pediatricUseIndicator)); 3684 children.add(new Property("classification", "CodeableConcept", "Allows the product to be classified by various systems, commonly WHO ATC.", 0, java.lang.Integer.MAX_VALUE, classification)); 3685 children.add(new Property("marketingStatus", "MarketingStatus", "Marketing status of the medicinal product, in contrast to marketing authorization. This refers to the product being actually 'on the market' as opposed to being allowed to be on the market (which is an authorization).", 0, java.lang.Integer.MAX_VALUE, marketingStatus)); 3686 children.add(new Property("packagedMedicinalProduct", "CodeableConcept", "Package type for the product. See also the PackagedProductDefinition resource.", 0, java.lang.Integer.MAX_VALUE, packagedMedicinalProduct)); 3687 children.add(new Property("comprisedOf", "Reference(ManufacturedItemDefinition|DeviceDefinition)", "Types of medicinal manufactured items and/or devices that this product consists of, such as tablets, capsule, or syringes. Used as a direct link when the item's packaging is not being recorded (see also PackagedProductDefinition.package.containedItem.item).", 0, java.lang.Integer.MAX_VALUE, comprisedOf)); 3688 children.add(new Property("ingredient", "CodeableConcept", "The ingredients of this medicinal product - when not detailed in other resources. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource, or indirectly via incoming AdministrableProductDefinition, PackagedProductDefinition or ManufacturedItemDefinition references. In cases where those levels of detail are not used, the ingredients may be specified directly here as codes.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 3689 children.add(new Property("impurity", "CodeableReference(SubstanceDefinition)", "Any component of the drug product which is not the chemical entity defined as the drug substance, or an excipient in the drug product. This includes process-related impurities and contaminants, product-related impurities including degradation products.", 0, java.lang.Integer.MAX_VALUE, impurity)); 3690 children.add(new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the medicinal product.", 0, java.lang.Integer.MAX_VALUE, attachedDocument)); 3691 children.add(new Property("masterFile", "Reference(DocumentReference)", "A master file for the medicinal product (e.g. Pharmacovigilance System Master File). Drug master files (DMFs) are documents submitted to regulatory agencies to provide confidential detailed information about facilities, processes or articles used in the manufacturing, processing, packaging and storing of drug products.", 0, java.lang.Integer.MAX_VALUE, masterFile)); 3692 children.add(new Property("contact", "", "A product specific contact, person (in a role), or an organization.", 0, java.lang.Integer.MAX_VALUE, contact)); 3693 children.add(new Property("clinicalTrial", "Reference(ResearchStudy)", "Clinical trials or studies that this product is involved in.", 0, java.lang.Integer.MAX_VALUE, clinicalTrial)); 3694 children.add(new Property("code", "Coding", "A code that this product is known by, usually within some formal terminology, perhaps assigned by a third party (i.e. not the manufacturer or regulator). Products (types of medications) tend to be known by identifiers during development and within regulatory process. However when they are prescribed they tend to be identified by codes. The same product may be have multiple codes, applied to it by multiple organizations.", 0, java.lang.Integer.MAX_VALUE, code)); 3695 children.add(new Property("name", "", "The product's name, including full name and possibly coded parts.", 0, java.lang.Integer.MAX_VALUE, name)); 3696 children.add(new Property("crossReference", "", "Reference to another product, e.g. for linking authorised to investigational product, or a virtual product.", 0, java.lang.Integer.MAX_VALUE, crossReference)); 3697 children.add(new Property("operation", "", "A manufacturing or administrative process or step associated with (or performed on) the medicinal product.", 0, java.lang.Integer.MAX_VALUE, operation)); 3698 children.add(new Property("characteristic", "", "Allows the key product features to be recorded, such as \"sugar free\", \"modified release\", \"parallel import\".", 0, java.lang.Integer.MAX_VALUE, characteristic)); 3699 } 3700 3701 @Override 3702 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3703 switch (_hash) { 3704 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for this product. Could be an MPID. When in development or being regulated, products are typically referenced by official identifiers, assigned by a manufacturer or regulator, and unique to a product (which, when compared to a product instance being prescribed, is actually a product type). See also MedicinalProductDefinition.code.", 0, java.lang.Integer.MAX_VALUE, identifier); 3705 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Regulatory type, e.g. Investigational or Authorized.", 0, 1, type); 3706 case -1326197564: /*domain*/ return new Property("domain", "CodeableConcept", "If this medicine applies to human or veterinary uses.", 0, 1, domain); 3707 case 351608024: /*version*/ return new Property("version", "string", "A business identifier relating to a specific version of the product, this is commonly used to support revisions to an existing product.", 0, 1, version); 3708 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status.", 0, 1, status); 3709 case 247524032: /*statusDate*/ return new Property("statusDate", "dateTime", "The date at which the given status became applicable.", 0, 1, statusDate); 3710 case -1724546052: /*description*/ return new Property("description", "markdown", "General description of this product.", 0, 1, description); 3711 case -1992898487: /*combinedPharmaceuticalDoseForm*/ return new Property("combinedPharmaceuticalDoseForm", "CodeableConcept", "The dose form for a single part product, or combined form of a multiple part product. This is one concept that describes all the components. It does not represent the form with components physically mixed, if that might be necessary, for which see (AdministrableProductDefinition.administrableDoseForm).", 0, 1, combinedPharmaceuticalDoseForm); 3712 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. See also AdministrableProductDefinition resource. MedicinalProductDefinition.route is the same concept as AdministrableProductDefinition.routeOfAdministration.code, and they cannot be used together.", 0, java.lang.Integer.MAX_VALUE, route); 3713 case -597168804: /*indication*/ return new Property("indication", "markdown", "Description of indication(s) for this product, used when structured indications are not required. In cases where structured indications are required, they are captured using the ClinicalUseDefinition resource. An indication is a medical situation for which using the product is appropriate.", 0, 1, indication); 3714 case -844874031: /*legalStatusOfSupply*/ return new Property("legalStatusOfSupply", "CodeableConcept", "The legal status of supply of the medicinal product as classified by the regulator.", 0, 1, legalStatusOfSupply); 3715 case 1935999744: /*additionalMonitoringIndicator*/ return new Property("additionalMonitoringIndicator", "CodeableConcept", "Whether the Medicinal Product is subject to additional monitoring for regulatory reasons, such as heightened reporting requirements.", 0, 1, additionalMonitoringIndicator); 3716 case 975102638: /*specialMeasures*/ return new Property("specialMeasures", "CodeableConcept", "Whether the Medicinal Product is subject to special measures for regulatory reasons, such as a requirement to conduct post-authorization studies.", 0, java.lang.Integer.MAX_VALUE, specialMeasures); 3717 case -1515533081: /*pediatricUseIndicator*/ return new Property("pediatricUseIndicator", "CodeableConcept", "If authorised for use in children, or infants, neonates etc.", 0, 1, pediatricUseIndicator); 3718 case 382350310: /*classification*/ return new Property("classification", "CodeableConcept", "Allows the product to be classified by various systems, commonly WHO ATC.", 0, java.lang.Integer.MAX_VALUE, classification); 3719 case 70767032: /*marketingStatus*/ return new Property("marketingStatus", "MarketingStatus", "Marketing status of the medicinal product, in contrast to marketing authorization. This refers to the product being actually 'on the market' as opposed to being allowed to be on the market (which is an authorization).", 0, java.lang.Integer.MAX_VALUE, marketingStatus); 3720 case -361025513: /*packagedMedicinalProduct*/ return new Property("packagedMedicinalProduct", "CodeableConcept", "Package type for the product. See also the PackagedProductDefinition resource.", 0, java.lang.Integer.MAX_VALUE, packagedMedicinalProduct); 3721 case 1546078211: /*comprisedOf*/ return new Property("comprisedOf", "Reference(ManufacturedItemDefinition|DeviceDefinition)", "Types of medicinal manufactured items and/or devices that this product consists of, such as tablets, capsule, or syringes. Used as a direct link when the item's packaging is not being recorded (see also PackagedProductDefinition.package.containedItem.item).", 0, java.lang.Integer.MAX_VALUE, comprisedOf); 3722 case -206409263: /*ingredient*/ return new Property("ingredient", "CodeableConcept", "The ingredients of this medicinal product - when not detailed in other resources. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource, or indirectly via incoming AdministrableProductDefinition, PackagedProductDefinition or ManufacturedItemDefinition references. In cases where those levels of detail are not used, the ingredients may be specified directly here as codes.", 0, java.lang.Integer.MAX_VALUE, ingredient); 3723 case -416837467: /*impurity*/ return new Property("impurity", "CodeableReference(SubstanceDefinition)", "Any component of the drug product which is not the chemical entity defined as the drug substance, or an excipient in the drug product. This includes process-related impurities and contaminants, product-related impurities including degradation products.", 0, java.lang.Integer.MAX_VALUE, impurity); 3724 case -513945889: /*attachedDocument*/ return new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the medicinal product.", 0, java.lang.Integer.MAX_VALUE, attachedDocument); 3725 case -2039573762: /*masterFile*/ return new Property("masterFile", "Reference(DocumentReference)", "A master file for the medicinal product (e.g. Pharmacovigilance System Master File). Drug master files (DMFs) are documents submitted to regulatory agencies to provide confidential detailed information about facilities, processes or articles used in the manufacturing, processing, packaging and storing of drug products.", 0, java.lang.Integer.MAX_VALUE, masterFile); 3726 case 951526432: /*contact*/ return new Property("contact", "", "A product specific contact, person (in a role), or an organization.", 0, java.lang.Integer.MAX_VALUE, contact); 3727 case 1232866243: /*clinicalTrial*/ return new Property("clinicalTrial", "Reference(ResearchStudy)", "Clinical trials or studies that this product is involved in.", 0, java.lang.Integer.MAX_VALUE, clinicalTrial); 3728 case 3059181: /*code*/ return new Property("code", "Coding", "A code that this product is known by, usually within some formal terminology, perhaps assigned by a third party (i.e. not the manufacturer or regulator). Products (types of medications) tend to be known by identifiers during development and within regulatory process. However when they are prescribed they tend to be identified by codes. The same product may be have multiple codes, applied to it by multiple organizations.", 0, java.lang.Integer.MAX_VALUE, code); 3729 case 3373707: /*name*/ return new Property("name", "", "The product's name, including full name and possibly coded parts.", 0, java.lang.Integer.MAX_VALUE, name); 3730 case -986968341: /*crossReference*/ return new Property("crossReference", "", "Reference to another product, e.g. for linking authorised to investigational product, or a virtual product.", 0, java.lang.Integer.MAX_VALUE, crossReference); 3731 case 1662702951: /*operation*/ return new Property("operation", "", "A manufacturing or administrative process or step associated with (or performed on) the medicinal product.", 0, java.lang.Integer.MAX_VALUE, operation); 3732 case 366313883: /*characteristic*/ return new Property("characteristic", "", "Allows the key product features to be recorded, such as \"sugar free\", \"modified release\", \"parallel import\".", 0, java.lang.Integer.MAX_VALUE, characteristic); 3733 default: return super.getNamedProperty(_hash, _name, _checkValid); 3734 } 3735 3736 } 3737 3738 @Override 3739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3740 switch (hash) { 3741 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3742 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3743 case -1326197564: /*domain*/ return this.domain == null ? new Base[0] : new Base[] {this.domain}; // CodeableConcept 3744 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3745 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 3746 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateTimeType 3747 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3748 case -1992898487: /*combinedPharmaceuticalDoseForm*/ return this.combinedPharmaceuticalDoseForm == null ? new Base[0] : new Base[] {this.combinedPharmaceuticalDoseForm}; // CodeableConcept 3749 case 108704329: /*route*/ return this.route == null ? new Base[0] : this.route.toArray(new Base[this.route.size()]); // CodeableConcept 3750 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : new Base[] {this.indication}; // MarkdownType 3751 case -844874031: /*legalStatusOfSupply*/ return this.legalStatusOfSupply == null ? new Base[0] : new Base[] {this.legalStatusOfSupply}; // CodeableConcept 3752 case 1935999744: /*additionalMonitoringIndicator*/ return this.additionalMonitoringIndicator == null ? new Base[0] : new Base[] {this.additionalMonitoringIndicator}; // CodeableConcept 3753 case 975102638: /*specialMeasures*/ return this.specialMeasures == null ? new Base[0] : this.specialMeasures.toArray(new Base[this.specialMeasures.size()]); // CodeableConcept 3754 case -1515533081: /*pediatricUseIndicator*/ return this.pediatricUseIndicator == null ? new Base[0] : new Base[] {this.pediatricUseIndicator}; // CodeableConcept 3755 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : this.classification.toArray(new Base[this.classification.size()]); // CodeableConcept 3756 case 70767032: /*marketingStatus*/ return this.marketingStatus == null ? new Base[0] : this.marketingStatus.toArray(new Base[this.marketingStatus.size()]); // MarketingStatus 3757 case -361025513: /*packagedMedicinalProduct*/ return this.packagedMedicinalProduct == null ? new Base[0] : this.packagedMedicinalProduct.toArray(new Base[this.packagedMedicinalProduct.size()]); // CodeableConcept 3758 case 1546078211: /*comprisedOf*/ return this.comprisedOf == null ? new Base[0] : this.comprisedOf.toArray(new Base[this.comprisedOf.size()]); // Reference 3759 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // CodeableConcept 3760 case -416837467: /*impurity*/ return this.impurity == null ? new Base[0] : this.impurity.toArray(new Base[this.impurity.size()]); // CodeableReference 3761 case -513945889: /*attachedDocument*/ return this.attachedDocument == null ? new Base[0] : this.attachedDocument.toArray(new Base[this.attachedDocument.size()]); // Reference 3762 case -2039573762: /*masterFile*/ return this.masterFile == null ? new Base[0] : this.masterFile.toArray(new Base[this.masterFile.size()]); // Reference 3763 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // MedicinalProductDefinitionContactComponent 3764 case 1232866243: /*clinicalTrial*/ return this.clinicalTrial == null ? new Base[0] : this.clinicalTrial.toArray(new Base[this.clinicalTrial.size()]); // Reference 3765 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 3766 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // MedicinalProductDefinitionNameComponent 3767 case -986968341: /*crossReference*/ return this.crossReference == null ? new Base[0] : this.crossReference.toArray(new Base[this.crossReference.size()]); // MedicinalProductDefinitionCrossReferenceComponent 3768 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : this.operation.toArray(new Base[this.operation.size()]); // MedicinalProductDefinitionOperationComponent 3769 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // MedicinalProductDefinitionCharacteristicComponent 3770 default: return super.getProperty(hash, name, checkValid); 3771 } 3772 3773 } 3774 3775 @Override 3776 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3777 switch (hash) { 3778 case -1618432855: // identifier 3779 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3780 return value; 3781 case 3575610: // type 3782 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3783 return value; 3784 case -1326197564: // domain 3785 this.domain = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3786 return value; 3787 case 351608024: // version 3788 this.version = TypeConvertor.castToString(value); // StringType 3789 return value; 3790 case -892481550: // status 3791 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3792 return value; 3793 case 247524032: // statusDate 3794 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 3795 return value; 3796 case -1724546052: // description 3797 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3798 return value; 3799 case -1992898487: // combinedPharmaceuticalDoseForm 3800 this.combinedPharmaceuticalDoseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3801 return value; 3802 case 108704329: // route 3803 this.getRoute().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3804 return value; 3805 case -597168804: // indication 3806 this.indication = TypeConvertor.castToMarkdown(value); // MarkdownType 3807 return value; 3808 case -844874031: // legalStatusOfSupply 3809 this.legalStatusOfSupply = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3810 return value; 3811 case 1935999744: // additionalMonitoringIndicator 3812 this.additionalMonitoringIndicator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3813 return value; 3814 case 975102638: // specialMeasures 3815 this.getSpecialMeasures().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3816 return value; 3817 case -1515533081: // pediatricUseIndicator 3818 this.pediatricUseIndicator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3819 return value; 3820 case 382350310: // classification 3821 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3822 return value; 3823 case 70767032: // marketingStatus 3824 this.getMarketingStatus().add(TypeConvertor.castToMarketingStatus(value)); // MarketingStatus 3825 return value; 3826 case -361025513: // packagedMedicinalProduct 3827 this.getPackagedMedicinalProduct().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3828 return value; 3829 case 1546078211: // comprisedOf 3830 this.getComprisedOf().add(TypeConvertor.castToReference(value)); // Reference 3831 return value; 3832 case -206409263: // ingredient 3833 this.getIngredient().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3834 return value; 3835 case -416837467: // impurity 3836 this.getImpurity().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 3837 return value; 3838 case -513945889: // attachedDocument 3839 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); // Reference 3840 return value; 3841 case -2039573762: // masterFile 3842 this.getMasterFile().add(TypeConvertor.castToReference(value)); // Reference 3843 return value; 3844 case 951526432: // contact 3845 this.getContact().add((MedicinalProductDefinitionContactComponent) value); // MedicinalProductDefinitionContactComponent 3846 return value; 3847 case 1232866243: // clinicalTrial 3848 this.getClinicalTrial().add(TypeConvertor.castToReference(value)); // Reference 3849 return value; 3850 case 3059181: // code 3851 this.getCode().add(TypeConvertor.castToCoding(value)); // Coding 3852 return value; 3853 case 3373707: // name 3854 this.getName().add((MedicinalProductDefinitionNameComponent) value); // MedicinalProductDefinitionNameComponent 3855 return value; 3856 case -986968341: // crossReference 3857 this.getCrossReference().add((MedicinalProductDefinitionCrossReferenceComponent) value); // MedicinalProductDefinitionCrossReferenceComponent 3858 return value; 3859 case 1662702951: // operation 3860 this.getOperation().add((MedicinalProductDefinitionOperationComponent) value); // MedicinalProductDefinitionOperationComponent 3861 return value; 3862 case 366313883: // characteristic 3863 this.getCharacteristic().add((MedicinalProductDefinitionCharacteristicComponent) value); // MedicinalProductDefinitionCharacteristicComponent 3864 return value; 3865 default: return super.setProperty(hash, name, value); 3866 } 3867 3868 } 3869 3870 @Override 3871 public Base setProperty(String name, Base value) throws FHIRException { 3872 if (name.equals("identifier")) { 3873 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3874 } else if (name.equals("type")) { 3875 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3876 } else if (name.equals("domain")) { 3877 this.domain = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3878 } else if (name.equals("version")) { 3879 this.version = TypeConvertor.castToString(value); // StringType 3880 } else if (name.equals("status")) { 3881 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3882 } else if (name.equals("statusDate")) { 3883 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 3884 } else if (name.equals("description")) { 3885 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3886 } else if (name.equals("combinedPharmaceuticalDoseForm")) { 3887 this.combinedPharmaceuticalDoseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3888 } else if (name.equals("route")) { 3889 this.getRoute().add(TypeConvertor.castToCodeableConcept(value)); 3890 } else if (name.equals("indication")) { 3891 this.indication = TypeConvertor.castToMarkdown(value); // MarkdownType 3892 } else if (name.equals("legalStatusOfSupply")) { 3893 this.legalStatusOfSupply = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3894 } else if (name.equals("additionalMonitoringIndicator")) { 3895 this.additionalMonitoringIndicator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3896 } else if (name.equals("specialMeasures")) { 3897 this.getSpecialMeasures().add(TypeConvertor.castToCodeableConcept(value)); 3898 } else if (name.equals("pediatricUseIndicator")) { 3899 this.pediatricUseIndicator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3900 } else if (name.equals("classification")) { 3901 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); 3902 } else if (name.equals("marketingStatus")) { 3903 this.getMarketingStatus().add(TypeConvertor.castToMarketingStatus(value)); 3904 } else if (name.equals("packagedMedicinalProduct")) { 3905 this.getPackagedMedicinalProduct().add(TypeConvertor.castToCodeableConcept(value)); 3906 } else if (name.equals("comprisedOf")) { 3907 this.getComprisedOf().add(TypeConvertor.castToReference(value)); 3908 } else if (name.equals("ingredient")) { 3909 this.getIngredient().add(TypeConvertor.castToCodeableConcept(value)); 3910 } else if (name.equals("impurity")) { 3911 this.getImpurity().add(TypeConvertor.castToCodeableReference(value)); 3912 } else if (name.equals("attachedDocument")) { 3913 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); 3914 } else if (name.equals("masterFile")) { 3915 this.getMasterFile().add(TypeConvertor.castToReference(value)); 3916 } else if (name.equals("contact")) { 3917 this.getContact().add((MedicinalProductDefinitionContactComponent) value); 3918 } else if (name.equals("clinicalTrial")) { 3919 this.getClinicalTrial().add(TypeConvertor.castToReference(value)); 3920 } else if (name.equals("code")) { 3921 this.getCode().add(TypeConvertor.castToCoding(value)); 3922 } else if (name.equals("name")) { 3923 this.getName().add((MedicinalProductDefinitionNameComponent) value); 3924 } else if (name.equals("crossReference")) { 3925 this.getCrossReference().add((MedicinalProductDefinitionCrossReferenceComponent) value); 3926 } else if (name.equals("operation")) { 3927 this.getOperation().add((MedicinalProductDefinitionOperationComponent) value); 3928 } else if (name.equals("characteristic")) { 3929 this.getCharacteristic().add((MedicinalProductDefinitionCharacteristicComponent) value); 3930 } else 3931 return super.setProperty(name, value); 3932 return value; 3933 } 3934 3935 @Override 3936 public void removeChild(String name, Base value) throws FHIRException { 3937 if (name.equals("identifier")) { 3938 this.getIdentifier().remove(value); 3939 } else if (name.equals("type")) { 3940 this.type = null; 3941 } else if (name.equals("domain")) { 3942 this.domain = null; 3943 } else if (name.equals("version")) { 3944 this.version = null; 3945 } else if (name.equals("status")) { 3946 this.status = null; 3947 } else if (name.equals("statusDate")) { 3948 this.statusDate = null; 3949 } else if (name.equals("description")) { 3950 this.description = null; 3951 } else if (name.equals("combinedPharmaceuticalDoseForm")) { 3952 this.combinedPharmaceuticalDoseForm = null; 3953 } else if (name.equals("route")) { 3954 this.getRoute().remove(value); 3955 } else if (name.equals("indication")) { 3956 this.indication = null; 3957 } else if (name.equals("legalStatusOfSupply")) { 3958 this.legalStatusOfSupply = null; 3959 } else if (name.equals("additionalMonitoringIndicator")) { 3960 this.additionalMonitoringIndicator = null; 3961 } else if (name.equals("specialMeasures")) { 3962 this.getSpecialMeasures().remove(value); 3963 } else if (name.equals("pediatricUseIndicator")) { 3964 this.pediatricUseIndicator = null; 3965 } else if (name.equals("classification")) { 3966 this.getClassification().remove(value); 3967 } else if (name.equals("marketingStatus")) { 3968 this.getMarketingStatus().remove(value); 3969 } else if (name.equals("packagedMedicinalProduct")) { 3970 this.getPackagedMedicinalProduct().remove(value); 3971 } else if (name.equals("comprisedOf")) { 3972 this.getComprisedOf().remove(value); 3973 } else if (name.equals("ingredient")) { 3974 this.getIngredient().remove(value); 3975 } else if (name.equals("impurity")) { 3976 this.getImpurity().remove(value); 3977 } else if (name.equals("attachedDocument")) { 3978 this.getAttachedDocument().remove(value); 3979 } else if (name.equals("masterFile")) { 3980 this.getMasterFile().remove(value); 3981 } else if (name.equals("contact")) { 3982 this.getContact().remove((MedicinalProductDefinitionContactComponent) value); 3983 } else if (name.equals("clinicalTrial")) { 3984 this.getClinicalTrial().remove(value); 3985 } else if (name.equals("code")) { 3986 this.getCode().remove(value); 3987 } else if (name.equals("name")) { 3988 this.getName().remove((MedicinalProductDefinitionNameComponent) value); 3989 } else if (name.equals("crossReference")) { 3990 this.getCrossReference().remove((MedicinalProductDefinitionCrossReferenceComponent) value); 3991 } else if (name.equals("operation")) { 3992 this.getOperation().remove((MedicinalProductDefinitionOperationComponent) value); 3993 } else if (name.equals("characteristic")) { 3994 this.getCharacteristic().remove((MedicinalProductDefinitionCharacteristicComponent) value); 3995 } else 3996 super.removeChild(name, value); 3997 3998 } 3999 4000 @Override 4001 public Base makeProperty(int hash, String name) throws FHIRException { 4002 switch (hash) { 4003 case -1618432855: return addIdentifier(); 4004 case 3575610: return getType(); 4005 case -1326197564: return getDomain(); 4006 case 351608024: return getVersionElement(); 4007 case -892481550: return getStatus(); 4008 case 247524032: return getStatusDateElement(); 4009 case -1724546052: return getDescriptionElement(); 4010 case -1992898487: return getCombinedPharmaceuticalDoseForm(); 4011 case 108704329: return addRoute(); 4012 case -597168804: return getIndicationElement(); 4013 case -844874031: return getLegalStatusOfSupply(); 4014 case 1935999744: return getAdditionalMonitoringIndicator(); 4015 case 975102638: return addSpecialMeasures(); 4016 case -1515533081: return getPediatricUseIndicator(); 4017 case 382350310: return addClassification(); 4018 case 70767032: return addMarketingStatus(); 4019 case -361025513: return addPackagedMedicinalProduct(); 4020 case 1546078211: return addComprisedOf(); 4021 case -206409263: return addIngredient(); 4022 case -416837467: return addImpurity(); 4023 case -513945889: return addAttachedDocument(); 4024 case -2039573762: return addMasterFile(); 4025 case 951526432: return addContact(); 4026 case 1232866243: return addClinicalTrial(); 4027 case 3059181: return addCode(); 4028 case 3373707: return addName(); 4029 case -986968341: return addCrossReference(); 4030 case 1662702951: return addOperation(); 4031 case 366313883: return addCharacteristic(); 4032 default: return super.makeProperty(hash, name); 4033 } 4034 4035 } 4036 4037 @Override 4038 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4039 switch (hash) { 4040 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4041 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4042 case -1326197564: /*domain*/ return new String[] {"CodeableConcept"}; 4043 case 351608024: /*version*/ return new String[] {"string"}; 4044 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 4045 case 247524032: /*statusDate*/ return new String[] {"dateTime"}; 4046 case -1724546052: /*description*/ return new String[] {"markdown"}; 4047 case -1992898487: /*combinedPharmaceuticalDoseForm*/ return new String[] {"CodeableConcept"}; 4048 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 4049 case -597168804: /*indication*/ return new String[] {"markdown"}; 4050 case -844874031: /*legalStatusOfSupply*/ return new String[] {"CodeableConcept"}; 4051 case 1935999744: /*additionalMonitoringIndicator*/ return new String[] {"CodeableConcept"}; 4052 case 975102638: /*specialMeasures*/ return new String[] {"CodeableConcept"}; 4053 case -1515533081: /*pediatricUseIndicator*/ return new String[] {"CodeableConcept"}; 4054 case 382350310: /*classification*/ return new String[] {"CodeableConcept"}; 4055 case 70767032: /*marketingStatus*/ return new String[] {"MarketingStatus"}; 4056 case -361025513: /*packagedMedicinalProduct*/ return new String[] {"CodeableConcept"}; 4057 case 1546078211: /*comprisedOf*/ return new String[] {"Reference"}; 4058 case -206409263: /*ingredient*/ return new String[] {"CodeableConcept"}; 4059 case -416837467: /*impurity*/ return new String[] {"CodeableReference"}; 4060 case -513945889: /*attachedDocument*/ return new String[] {"Reference"}; 4061 case -2039573762: /*masterFile*/ return new String[] {"Reference"}; 4062 case 951526432: /*contact*/ return new String[] {}; 4063 case 1232866243: /*clinicalTrial*/ return new String[] {"Reference"}; 4064 case 3059181: /*code*/ return new String[] {"Coding"}; 4065 case 3373707: /*name*/ return new String[] {}; 4066 case -986968341: /*crossReference*/ return new String[] {}; 4067 case 1662702951: /*operation*/ return new String[] {}; 4068 case 366313883: /*characteristic*/ return new String[] {}; 4069 default: return super.getTypesForProperty(hash, name); 4070 } 4071 4072 } 4073 4074 @Override 4075 public Base addChild(String name) throws FHIRException { 4076 if (name.equals("identifier")) { 4077 return addIdentifier(); 4078 } 4079 else if (name.equals("type")) { 4080 this.type = new CodeableConcept(); 4081 return this.type; 4082 } 4083 else if (name.equals("domain")) { 4084 this.domain = new CodeableConcept(); 4085 return this.domain; 4086 } 4087 else if (name.equals("version")) { 4088 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductDefinition.version"); 4089 } 4090 else if (name.equals("status")) { 4091 this.status = new CodeableConcept(); 4092 return this.status; 4093 } 4094 else if (name.equals("statusDate")) { 4095 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductDefinition.statusDate"); 4096 } 4097 else if (name.equals("description")) { 4098 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductDefinition.description"); 4099 } 4100 else if (name.equals("combinedPharmaceuticalDoseForm")) { 4101 this.combinedPharmaceuticalDoseForm = new CodeableConcept(); 4102 return this.combinedPharmaceuticalDoseForm; 4103 } 4104 else if (name.equals("route")) { 4105 return addRoute(); 4106 } 4107 else if (name.equals("indication")) { 4108 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductDefinition.indication"); 4109 } 4110 else if (name.equals("legalStatusOfSupply")) { 4111 this.legalStatusOfSupply = new CodeableConcept(); 4112 return this.legalStatusOfSupply; 4113 } 4114 else if (name.equals("additionalMonitoringIndicator")) { 4115 this.additionalMonitoringIndicator = new CodeableConcept(); 4116 return this.additionalMonitoringIndicator; 4117 } 4118 else if (name.equals("specialMeasures")) { 4119 return addSpecialMeasures(); 4120 } 4121 else if (name.equals("pediatricUseIndicator")) { 4122 this.pediatricUseIndicator = new CodeableConcept(); 4123 return this.pediatricUseIndicator; 4124 } 4125 else if (name.equals("classification")) { 4126 return addClassification(); 4127 } 4128 else if (name.equals("marketingStatus")) { 4129 return addMarketingStatus(); 4130 } 4131 else if (name.equals("packagedMedicinalProduct")) { 4132 return addPackagedMedicinalProduct(); 4133 } 4134 else if (name.equals("comprisedOf")) { 4135 return addComprisedOf(); 4136 } 4137 else if (name.equals("ingredient")) { 4138 return addIngredient(); 4139 } 4140 else if (name.equals("impurity")) { 4141 return addImpurity(); 4142 } 4143 else if (name.equals("attachedDocument")) { 4144 return addAttachedDocument(); 4145 } 4146 else if (name.equals("masterFile")) { 4147 return addMasterFile(); 4148 } 4149 else if (name.equals("contact")) { 4150 return addContact(); 4151 } 4152 else if (name.equals("clinicalTrial")) { 4153 return addClinicalTrial(); 4154 } 4155 else if (name.equals("code")) { 4156 return addCode(); 4157 } 4158 else if (name.equals("name")) { 4159 return addName(); 4160 } 4161 else if (name.equals("crossReference")) { 4162 return addCrossReference(); 4163 } 4164 else if (name.equals("operation")) { 4165 return addOperation(); 4166 } 4167 else if (name.equals("characteristic")) { 4168 return addCharacteristic(); 4169 } 4170 else 4171 return super.addChild(name); 4172 } 4173 4174 public String fhirType() { 4175 return "MedicinalProductDefinition"; 4176 4177 } 4178 4179 public MedicinalProductDefinition copy() { 4180 MedicinalProductDefinition dst = new MedicinalProductDefinition(); 4181 copyValues(dst); 4182 return dst; 4183 } 4184 4185 public void copyValues(MedicinalProductDefinition dst) { 4186 super.copyValues(dst); 4187 if (identifier != null) { 4188 dst.identifier = new ArrayList<Identifier>(); 4189 for (Identifier i : identifier) 4190 dst.identifier.add(i.copy()); 4191 }; 4192 dst.type = type == null ? null : type.copy(); 4193 dst.domain = domain == null ? null : domain.copy(); 4194 dst.version = version == null ? null : version.copy(); 4195 dst.status = status == null ? null : status.copy(); 4196 dst.statusDate = statusDate == null ? null : statusDate.copy(); 4197 dst.description = description == null ? null : description.copy(); 4198 dst.combinedPharmaceuticalDoseForm = combinedPharmaceuticalDoseForm == null ? null : combinedPharmaceuticalDoseForm.copy(); 4199 if (route != null) { 4200 dst.route = new ArrayList<CodeableConcept>(); 4201 for (CodeableConcept i : route) 4202 dst.route.add(i.copy()); 4203 }; 4204 dst.indication = indication == null ? null : indication.copy(); 4205 dst.legalStatusOfSupply = legalStatusOfSupply == null ? null : legalStatusOfSupply.copy(); 4206 dst.additionalMonitoringIndicator = additionalMonitoringIndicator == null ? null : additionalMonitoringIndicator.copy(); 4207 if (specialMeasures != null) { 4208 dst.specialMeasures = new ArrayList<CodeableConcept>(); 4209 for (CodeableConcept i : specialMeasures) 4210 dst.specialMeasures.add(i.copy()); 4211 }; 4212 dst.pediatricUseIndicator = pediatricUseIndicator == null ? null : pediatricUseIndicator.copy(); 4213 if (classification != null) { 4214 dst.classification = new ArrayList<CodeableConcept>(); 4215 for (CodeableConcept i : classification) 4216 dst.classification.add(i.copy()); 4217 }; 4218 if (marketingStatus != null) { 4219 dst.marketingStatus = new ArrayList<MarketingStatus>(); 4220 for (MarketingStatus i : marketingStatus) 4221 dst.marketingStatus.add(i.copy()); 4222 }; 4223 if (packagedMedicinalProduct != null) { 4224 dst.packagedMedicinalProduct = new ArrayList<CodeableConcept>(); 4225 for (CodeableConcept i : packagedMedicinalProduct) 4226 dst.packagedMedicinalProduct.add(i.copy()); 4227 }; 4228 if (comprisedOf != null) { 4229 dst.comprisedOf = new ArrayList<Reference>(); 4230 for (Reference i : comprisedOf) 4231 dst.comprisedOf.add(i.copy()); 4232 }; 4233 if (ingredient != null) { 4234 dst.ingredient = new ArrayList<CodeableConcept>(); 4235 for (CodeableConcept i : ingredient) 4236 dst.ingredient.add(i.copy()); 4237 }; 4238 if (impurity != null) { 4239 dst.impurity = new ArrayList<CodeableReference>(); 4240 for (CodeableReference i : impurity) 4241 dst.impurity.add(i.copy()); 4242 }; 4243 if (attachedDocument != null) { 4244 dst.attachedDocument = new ArrayList<Reference>(); 4245 for (Reference i : attachedDocument) 4246 dst.attachedDocument.add(i.copy()); 4247 }; 4248 if (masterFile != null) { 4249 dst.masterFile = new ArrayList<Reference>(); 4250 for (Reference i : masterFile) 4251 dst.masterFile.add(i.copy()); 4252 }; 4253 if (contact != null) { 4254 dst.contact = new ArrayList<MedicinalProductDefinitionContactComponent>(); 4255 for (MedicinalProductDefinitionContactComponent i : contact) 4256 dst.contact.add(i.copy()); 4257 }; 4258 if (clinicalTrial != null) { 4259 dst.clinicalTrial = new ArrayList<Reference>(); 4260 for (Reference i : clinicalTrial) 4261 dst.clinicalTrial.add(i.copy()); 4262 }; 4263 if (code != null) { 4264 dst.code = new ArrayList<Coding>(); 4265 for (Coding i : code) 4266 dst.code.add(i.copy()); 4267 }; 4268 if (name != null) { 4269 dst.name = new ArrayList<MedicinalProductDefinitionNameComponent>(); 4270 for (MedicinalProductDefinitionNameComponent i : name) 4271 dst.name.add(i.copy()); 4272 }; 4273 if (crossReference != null) { 4274 dst.crossReference = new ArrayList<MedicinalProductDefinitionCrossReferenceComponent>(); 4275 for (MedicinalProductDefinitionCrossReferenceComponent i : crossReference) 4276 dst.crossReference.add(i.copy()); 4277 }; 4278 if (operation != null) { 4279 dst.operation = new ArrayList<MedicinalProductDefinitionOperationComponent>(); 4280 for (MedicinalProductDefinitionOperationComponent i : operation) 4281 dst.operation.add(i.copy()); 4282 }; 4283 if (characteristic != null) { 4284 dst.characteristic = new ArrayList<MedicinalProductDefinitionCharacteristicComponent>(); 4285 for (MedicinalProductDefinitionCharacteristicComponent i : characteristic) 4286 dst.characteristic.add(i.copy()); 4287 }; 4288 } 4289 4290 protected MedicinalProductDefinition typedCopy() { 4291 return copy(); 4292 } 4293 4294 @Override 4295 public boolean equalsDeep(Base other_) { 4296 if (!super.equalsDeep(other_)) 4297 return false; 4298 if (!(other_ instanceof MedicinalProductDefinition)) 4299 return false; 4300 MedicinalProductDefinition o = (MedicinalProductDefinition) other_; 4301 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(domain, o.domain, true) 4302 && compareDeep(version, o.version, true) && compareDeep(status, o.status, true) && compareDeep(statusDate, o.statusDate, true) 4303 && compareDeep(description, o.description, true) && compareDeep(combinedPharmaceuticalDoseForm, o.combinedPharmaceuticalDoseForm, true) 4304 && compareDeep(route, o.route, true) && compareDeep(indication, o.indication, true) && compareDeep(legalStatusOfSupply, o.legalStatusOfSupply, true) 4305 && compareDeep(additionalMonitoringIndicator, o.additionalMonitoringIndicator, true) && compareDeep(specialMeasures, o.specialMeasures, true) 4306 && compareDeep(pediatricUseIndicator, o.pediatricUseIndicator, true) && compareDeep(classification, o.classification, true) 4307 && compareDeep(marketingStatus, o.marketingStatus, true) && compareDeep(packagedMedicinalProduct, o.packagedMedicinalProduct, true) 4308 && compareDeep(comprisedOf, o.comprisedOf, true) && compareDeep(ingredient, o.ingredient, true) 4309 && compareDeep(impurity, o.impurity, true) && compareDeep(attachedDocument, o.attachedDocument, true) 4310 && compareDeep(masterFile, o.masterFile, true) && compareDeep(contact, o.contact, true) && compareDeep(clinicalTrial, o.clinicalTrial, true) 4311 && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) && compareDeep(crossReference, o.crossReference, true) 4312 && compareDeep(operation, o.operation, true) && compareDeep(characteristic, o.characteristic, true) 4313 ; 4314 } 4315 4316 @Override 4317 public boolean equalsShallow(Base other_) { 4318 if (!super.equalsShallow(other_)) 4319 return false; 4320 if (!(other_ instanceof MedicinalProductDefinition)) 4321 return false; 4322 MedicinalProductDefinition o = (MedicinalProductDefinition) other_; 4323 return compareValues(version, o.version, true) && compareValues(statusDate, o.statusDate, true) && compareValues(description, o.description, true) 4324 && compareValues(indication, o.indication, true); 4325 } 4326 4327 public boolean isEmpty() { 4328 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, domain 4329 , version, status, statusDate, description, combinedPharmaceuticalDoseForm, route 4330 , indication, legalStatusOfSupply, additionalMonitoringIndicator, specialMeasures, pediatricUseIndicator 4331 , classification, marketingStatus, packagedMedicinalProduct, comprisedOf, ingredient 4332 , impurity, attachedDocument, masterFile, contact, clinicalTrial, code, name, crossReference 4333 , operation, characteristic); 4334 } 4335 4336 @Override 4337 public ResourceType getResourceType() { 4338 return ResourceType.MedicinalProductDefinition; 4339 } 4340 4341 /** 4342 * Search parameter: <b>characteristic-type</b> 4343 * <p> 4344 * Description: <b>A category for the characteristic</b><br> 4345 * Type: <b>token</b><br> 4346 * Path: <b>MedicinalProductDefinition.characteristic.type</b><br> 4347 * </p> 4348 */ 4349 @SearchParamDefinition(name="characteristic-type", path="MedicinalProductDefinition.characteristic.type", description="A category for the characteristic", type="token" ) 4350 public static final String SP_CHARACTERISTIC_TYPE = "characteristic-type"; 4351 /** 4352 * <b>Fluent Client</b> search parameter constant for <b>characteristic-type</b> 4353 * <p> 4354 * Description: <b>A category for the characteristic</b><br> 4355 * Type: <b>token</b><br> 4356 * Path: <b>MedicinalProductDefinition.characteristic.type</b><br> 4357 * </p> 4358 */ 4359 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC_TYPE); 4360 4361 /** 4362 * Search parameter: <b>characteristic</b> 4363 * <p> 4364 * Description: <b>Allows the key product features to be recorded, such as "sugar free", "modified release", "parallel import"</b><br> 4365 * Type: <b>token</b><br> 4366 * Path: <b>MedicinalProductDefinition.characteristic.value.ofType(Quantity) | MedicinalProductDefinition.characteristic.value.ofType(CodeableConcept)</b><br> 4367 * </p> 4368 */ 4369 @SearchParamDefinition(name="characteristic", path="MedicinalProductDefinition.characteristic.value.ofType(Quantity) | MedicinalProductDefinition.characteristic.value.ofType(CodeableConcept)", description="Allows the key product features to be recorded, such as \"sugar free\", \"modified release\", \"parallel import\"", type="token" ) 4370 public static final String SP_CHARACTERISTIC = "characteristic"; 4371 /** 4372 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 4373 * <p> 4374 * Description: <b>Allows the key product features to be recorded, such as "sugar free", "modified release", "parallel import"</b><br> 4375 * Type: <b>token</b><br> 4376 * Path: <b>MedicinalProductDefinition.characteristic.value.ofType(Quantity) | MedicinalProductDefinition.characteristic.value.ofType(CodeableConcept)</b><br> 4377 * </p> 4378 */ 4379 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC); 4380 4381 /** 4382 * Search parameter: <b>contact</b> 4383 * <p> 4384 * Description: <b>A product specific contact, person (in a role), or an organization</b><br> 4385 * Type: <b>reference</b><br> 4386 * Path: <b>MedicinalProductDefinition.contact.contact</b><br> 4387 * </p> 4388 */ 4389 @SearchParamDefinition(name="contact", path="MedicinalProductDefinition.contact.contact", description="A product specific contact, person (in a role), or an organization", type="reference", target={Organization.class, PractitionerRole.class } ) 4390 public static final String SP_CONTACT = "contact"; 4391 /** 4392 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 4393 * <p> 4394 * Description: <b>A product specific contact, person (in a role), or an organization</b><br> 4395 * Type: <b>reference</b><br> 4396 * Path: <b>MedicinalProductDefinition.contact.contact</b><br> 4397 * </p> 4398 */ 4399 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTACT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTACT); 4400 4401/** 4402 * Constant for fluent queries to be used to add include statements. Specifies 4403 * the path value of "<b>MedicinalProductDefinition:contact</b>". 4404 */ 4405 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTACT = new ca.uhn.fhir.model.api.Include("MedicinalProductDefinition:contact").toLocked(); 4406 4407 /** 4408 * Search parameter: <b>domain</b> 4409 * <p> 4410 * Description: <b>If this medicine applies to human or veterinary uses</b><br> 4411 * Type: <b>token</b><br> 4412 * Path: <b>MedicinalProductDefinition.domain</b><br> 4413 * </p> 4414 */ 4415 @SearchParamDefinition(name="domain", path="MedicinalProductDefinition.domain", description="If this medicine applies to human or veterinary uses", type="token" ) 4416 public static final String SP_DOMAIN = "domain"; 4417 /** 4418 * <b>Fluent Client</b> search parameter constant for <b>domain</b> 4419 * <p> 4420 * Description: <b>If this medicine applies to human or veterinary uses</b><br> 4421 * Type: <b>token</b><br> 4422 * Path: <b>MedicinalProductDefinition.domain</b><br> 4423 * </p> 4424 */ 4425 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOMAIN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOMAIN); 4426 4427 /** 4428 * Search parameter: <b>identifier</b> 4429 * <p> 4430 * Description: <b>Business identifier for this product. Could be an MPID</b><br> 4431 * Type: <b>token</b><br> 4432 * Path: <b>MedicinalProductDefinition.identifier</b><br> 4433 * </p> 4434 */ 4435 @SearchParamDefinition(name="identifier", path="MedicinalProductDefinition.identifier", description="Business identifier for this product. Could be an MPID", type="token" ) 4436 public static final String SP_IDENTIFIER = "identifier"; 4437 /** 4438 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4439 * <p> 4440 * Description: <b>Business identifier for this product. Could be an MPID</b><br> 4441 * Type: <b>token</b><br> 4442 * Path: <b>MedicinalProductDefinition.identifier</b><br> 4443 * </p> 4444 */ 4445 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4446 4447 /** 4448 * Search parameter: <b>ingredient</b> 4449 * <p> 4450 * Description: <b>An ingredient of this product</b><br> 4451 * Type: <b>token</b><br> 4452 * Path: <b>MedicinalProductDefinition.ingredient</b><br> 4453 * </p> 4454 */ 4455 @SearchParamDefinition(name="ingredient", path="MedicinalProductDefinition.ingredient", description="An ingredient of this product", type="token" ) 4456 public static final String SP_INGREDIENT = "ingredient"; 4457 /** 4458 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 4459 * <p> 4460 * Description: <b>An ingredient of this product</b><br> 4461 * Type: <b>token</b><br> 4462 * Path: <b>MedicinalProductDefinition.ingredient</b><br> 4463 * </p> 4464 */ 4465 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INGREDIENT); 4466 4467 /** 4468 * Search parameter: <b>master-file</b> 4469 * <p> 4470 * Description: <b>A master file for to the medicinal product (e.g. Pharmacovigilance System Master File)</b><br> 4471 * Type: <b>reference</b><br> 4472 * Path: <b>MedicinalProductDefinition.masterFile</b><br> 4473 * </p> 4474 */ 4475 @SearchParamDefinition(name="master-file", path="MedicinalProductDefinition.masterFile", description="A master file for to the medicinal product (e.g. Pharmacovigilance System Master File)", type="reference", target={DocumentReference.class } ) 4476 public static final String SP_MASTER_FILE = "master-file"; 4477 /** 4478 * <b>Fluent Client</b> search parameter constant for <b>master-file</b> 4479 * <p> 4480 * Description: <b>A master file for to the medicinal product (e.g. Pharmacovigilance System Master File)</b><br> 4481 * Type: <b>reference</b><br> 4482 * Path: <b>MedicinalProductDefinition.masterFile</b><br> 4483 * </p> 4484 */ 4485 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MASTER_FILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MASTER_FILE); 4486 4487/** 4488 * Constant for fluent queries to be used to add include statements. Specifies 4489 * the path value of "<b>MedicinalProductDefinition:master-file</b>". 4490 */ 4491 public static final ca.uhn.fhir.model.api.Include INCLUDE_MASTER_FILE = new ca.uhn.fhir.model.api.Include("MedicinalProductDefinition:master-file").toLocked(); 4492 4493 /** 4494 * Search parameter: <b>name-language</b> 4495 * <p> 4496 * Description: <b>Language code for this name</b><br> 4497 * Type: <b>token</b><br> 4498 * Path: <b>MedicinalProductDefinition.name.usage.language</b><br> 4499 * </p> 4500 */ 4501 @SearchParamDefinition(name="name-language", path="MedicinalProductDefinition.name.usage.language", description="Language code for this name", type="token" ) 4502 public static final String SP_NAME_LANGUAGE = "name-language"; 4503 /** 4504 * <b>Fluent Client</b> search parameter constant for <b>name-language</b> 4505 * <p> 4506 * Description: <b>Language code for this name</b><br> 4507 * Type: <b>token</b><br> 4508 * Path: <b>MedicinalProductDefinition.name.usage.language</b><br> 4509 * </p> 4510 */ 4511 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NAME_LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NAME_LANGUAGE); 4512 4513 /** 4514 * Search parameter: <b>name</b> 4515 * <p> 4516 * Description: <b>The full product name</b><br> 4517 * Type: <b>string</b><br> 4518 * Path: <b>MedicinalProductDefinition.name.productName</b><br> 4519 * </p> 4520 */ 4521 @SearchParamDefinition(name="name", path="MedicinalProductDefinition.name.productName", description="The full product name", type="string" ) 4522 public static final String SP_NAME = "name"; 4523 /** 4524 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4525 * <p> 4526 * Description: <b>The full product name</b><br> 4527 * Type: <b>string</b><br> 4528 * Path: <b>MedicinalProductDefinition.name.productName</b><br> 4529 * </p> 4530 */ 4531 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4532 4533 /** 4534 * Search parameter: <b>product-classification</b> 4535 * <p> 4536 * Description: <b>Allows the product to be classified by various systems</b><br> 4537 * Type: <b>token</b><br> 4538 * Path: <b>MedicinalProductDefinition.classification</b><br> 4539 * </p> 4540 */ 4541 @SearchParamDefinition(name="product-classification", path="MedicinalProductDefinition.classification", description="Allows the product to be classified by various systems", type="token" ) 4542 public static final String SP_PRODUCT_CLASSIFICATION = "product-classification"; 4543 /** 4544 * <b>Fluent Client</b> search parameter constant for <b>product-classification</b> 4545 * <p> 4546 * Description: <b>Allows the product to be classified by various systems</b><br> 4547 * Type: <b>token</b><br> 4548 * Path: <b>MedicinalProductDefinition.classification</b><br> 4549 * </p> 4550 */ 4551 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRODUCT_CLASSIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRODUCT_CLASSIFICATION); 4552 4553 /** 4554 * Search parameter: <b>status</b> 4555 * <p> 4556 * Description: <b>The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status</b><br> 4557 * Type: <b>token</b><br> 4558 * Path: <b>MedicinalProductDefinition.status</b><br> 4559 * </p> 4560 */ 4561 @SearchParamDefinition(name="status", path="MedicinalProductDefinition.status", description="The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status", type="token" ) 4562 public static final String SP_STATUS = "status"; 4563 /** 4564 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4565 * <p> 4566 * Description: <b>The status within the lifecycle of this product record. A high-level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization status</b><br> 4567 * Type: <b>token</b><br> 4568 * Path: <b>MedicinalProductDefinition.status</b><br> 4569 * </p> 4570 */ 4571 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4572 4573 /** 4574 * Search parameter: <b>type</b> 4575 * <p> 4576 * Description: <b>Regulatory type, e.g. Investigational or Authorized</b><br> 4577 * Type: <b>token</b><br> 4578 * Path: <b>MedicinalProductDefinition.type</b><br> 4579 * </p> 4580 */ 4581 @SearchParamDefinition(name="type", path="MedicinalProductDefinition.type", description="Regulatory type, e.g. Investigational or Authorized", type="token" ) 4582 public static final String SP_TYPE = "type"; 4583 /** 4584 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4585 * <p> 4586 * Description: <b>Regulatory type, e.g. Investigational or Authorized</b><br> 4587 * Type: <b>token</b><br> 4588 * Path: <b>MedicinalProductDefinition.type</b><br> 4589 * </p> 4590 */ 4591 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4592 4593 4594} 4595