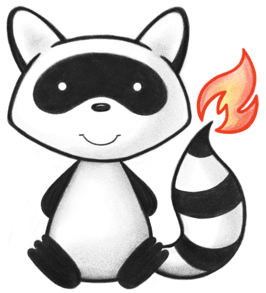
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 052 */ 053@ResourceDef(name="MessageDefinition", profile="http://hl7.org/fhir/StructureDefinition/MessageDefinition") 054public class MessageDefinition extends CanonicalResource { 055 056 public enum MessageSignificanceCategory { 057 /** 058 * The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment. 059 */ 060 CONSEQUENCE, 061 /** 062 * The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful. 063 */ 064 CURRENCY, 065 /** 066 * The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications. 067 */ 068 NOTIFICATION, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("consequence".equals(codeString)) 077 return CONSEQUENCE; 078 if ("currency".equals(codeString)) 079 return CURRENCY; 080 if ("notification".equals(codeString)) 081 return NOTIFICATION; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case CONSEQUENCE: return "consequence"; 090 case CURRENCY: return "currency"; 091 case NOTIFICATION: return "notification"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case CONSEQUENCE: return "http://hl7.org/fhir/message-significance-category"; 099 case CURRENCY: return "http://hl7.org/fhir/message-significance-category"; 100 case NOTIFICATION: return "http://hl7.org/fhir/message-significance-category"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case CONSEQUENCE: return "The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment."; 108 case CURRENCY: return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 109 case NOTIFICATION: return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case CONSEQUENCE: return "Consequence"; 117 case CURRENCY: return "Currency"; 118 case NOTIFICATION: return "Notification"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 126 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("consequence".equals(codeString)) 131 return MessageSignificanceCategory.CONSEQUENCE; 132 if ("currency".equals(codeString)) 133 return MessageSignificanceCategory.CURRENCY; 134 if ("notification".equals(codeString)) 135 return MessageSignificanceCategory.NOTIFICATION; 136 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 137 } 138 public Enumeration<MessageSignificanceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 146 if ("consequence".equals(codeString)) 147 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE, code); 148 if ("currency".equals(codeString)) 149 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY, code); 150 if ("notification".equals(codeString)) 151 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION, code); 152 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 153 } 154 public String toCode(MessageSignificanceCategory code) { 155 if (code == MessageSignificanceCategory.NULL) 156 return null; 157 if (code == MessageSignificanceCategory.CONSEQUENCE) 158 return "consequence"; 159 if (code == MessageSignificanceCategory.CURRENCY) 160 return "currency"; 161 if (code == MessageSignificanceCategory.NOTIFICATION) 162 return "notification"; 163 return "?"; 164 } 165 public String toSystem(MessageSignificanceCategory code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum MessageheaderResponseRequest { 171 /** 172 * initiator expects a response for this message. 173 */ 174 ALWAYS, 175 /** 176 * initiator expects a response only if in error. 177 */ 178 ONERROR, 179 /** 180 * initiator does not expect a response. 181 */ 182 NEVER, 183 /** 184 * initiator expects a response only if successful. 185 */ 186 ONSUCCESS, 187 /** 188 * added to help the parsers with the generic types 189 */ 190 NULL; 191 public static MessageheaderResponseRequest fromCode(String codeString) throws FHIRException { 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("always".equals(codeString)) 195 return ALWAYS; 196 if ("on-error".equals(codeString)) 197 return ONERROR; 198 if ("never".equals(codeString)) 199 return NEVER; 200 if ("on-success".equals(codeString)) 201 return ONSUCCESS; 202 if (Configuration.isAcceptInvalidEnums()) 203 return null; 204 else 205 throw new FHIRException("Unknown MessageheaderResponseRequest code '"+codeString+"'"); 206 } 207 public String toCode() { 208 switch (this) { 209 case ALWAYS: return "always"; 210 case ONERROR: return "on-error"; 211 case NEVER: return "never"; 212 case ONSUCCESS: return "on-success"; 213 case NULL: return null; 214 default: return "?"; 215 } 216 } 217 public String getSystem() { 218 switch (this) { 219 case ALWAYS: return "http://hl7.org/fhir/messageheader-response-request"; 220 case ONERROR: return "http://hl7.org/fhir/messageheader-response-request"; 221 case NEVER: return "http://hl7.org/fhir/messageheader-response-request"; 222 case ONSUCCESS: return "http://hl7.org/fhir/messageheader-response-request"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 public String getDefinition() { 228 switch (this) { 229 case ALWAYS: return "initiator expects a response for this message."; 230 case ONERROR: return "initiator expects a response only if in error."; 231 case NEVER: return "initiator does not expect a response."; 232 case ONSUCCESS: return "initiator expects a response only if successful."; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 public String getDisplay() { 238 switch (this) { 239 case ALWAYS: return "Always"; 240 case ONERROR: return "Error/reject conditions only"; 241 case NEVER: return "Never"; 242 case ONSUCCESS: return "Successful completion only"; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 } 248 249 public static class MessageheaderResponseRequestEnumFactory implements EnumFactory<MessageheaderResponseRequest> { 250 public MessageheaderResponseRequest fromCode(String codeString) throws IllegalArgumentException { 251 if (codeString == null || "".equals(codeString)) 252 if (codeString == null || "".equals(codeString)) 253 return null; 254 if ("always".equals(codeString)) 255 return MessageheaderResponseRequest.ALWAYS; 256 if ("on-error".equals(codeString)) 257 return MessageheaderResponseRequest.ONERROR; 258 if ("never".equals(codeString)) 259 return MessageheaderResponseRequest.NEVER; 260 if ("on-success".equals(codeString)) 261 return MessageheaderResponseRequest.ONSUCCESS; 262 throw new IllegalArgumentException("Unknown MessageheaderResponseRequest code '"+codeString+"'"); 263 } 264 public Enumeration<MessageheaderResponseRequest> fromType(PrimitiveType<?> code) throws FHIRException { 265 if (code == null) 266 return null; 267 if (code.isEmpty()) 268 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 269 String codeString = ((PrimitiveType) code).asStringValue(); 270 if (codeString == null || "".equals(codeString)) 271 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 272 if ("always".equals(codeString)) 273 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ALWAYS, code); 274 if ("on-error".equals(codeString)) 275 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONERROR, code); 276 if ("never".equals(codeString)) 277 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NEVER, code); 278 if ("on-success".equals(codeString)) 279 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONSUCCESS, code); 280 throw new FHIRException("Unknown MessageheaderResponseRequest code '"+codeString+"'"); 281 } 282 public String toCode(MessageheaderResponseRequest code) { 283 if (code == MessageheaderResponseRequest.NULL) 284 return null; 285 if (code == MessageheaderResponseRequest.ALWAYS) 286 return "always"; 287 if (code == MessageheaderResponseRequest.ONERROR) 288 return "on-error"; 289 if (code == MessageheaderResponseRequest.NEVER) 290 return "never"; 291 if (code == MessageheaderResponseRequest.ONSUCCESS) 292 return "on-success"; 293 return "?"; 294 } 295 public String toSystem(MessageheaderResponseRequest code) { 296 return code.getSystem(); 297 } 298 } 299 300 @Block() 301 public static class MessageDefinitionFocusComponent extends BackboneElement implements IBaseBackboneElement { 302 /** 303 * The kind of resource that must be the focus for this message. 304 */ 305 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 306 @Description(shortDefinition="Type of resource", formalDefinition="The kind of resource that must be the focus for this message." ) 307 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 308 protected CodeType code; 309 310 /** 311 * A profile that reflects constraints for the focal resource (and potentially for related resources). 312 */ 313 @Child(name = "profile", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=false) 314 @Description(shortDefinition="Profile that must be adhered to by focus", formalDefinition="A profile that reflects constraints for the focal resource (and potentially for related resources)." ) 315 protected CanonicalType profile; 316 317 /** 318 * Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 319 */ 320 @Child(name = "min", type = {UnsignedIntType.class}, order=3, min=1, max=1, modifier=false, summary=true) 321 @Description(shortDefinition="Minimum number of focuses of this type", formalDefinition="Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition." ) 322 protected UnsignedIntType min; 323 324 /** 325 * Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 326 */ 327 @Child(name = "max", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 328 @Description(shortDefinition="Maximum number of focuses of this type", formalDefinition="Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition." ) 329 protected StringType max; 330 331 private static final long serialVersionUID = -68504836L; 332 333 /** 334 * Constructor 335 */ 336 public MessageDefinitionFocusComponent() { 337 super(); 338 } 339 340 /** 341 * Constructor 342 */ 343 public MessageDefinitionFocusComponent(String code, int min) { 344 super(); 345 this.setCode(code); 346 this.setMin(min); 347 } 348 349 /** 350 * @return {@link #code} (The kind of resource that must be the focus for this message.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 351 */ 352 public CodeType getCodeElement() { 353 if (this.code == null) 354 if (Configuration.errorOnAutoCreate()) 355 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.code"); 356 else if (Configuration.doAutoCreate()) 357 this.code = new CodeType(); // bb 358 return this.code; 359 } 360 361 public boolean hasCodeElement() { 362 return this.code != null && !this.code.isEmpty(); 363 } 364 365 public boolean hasCode() { 366 return this.code != null && !this.code.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #code} (The kind of resource that must be the focus for this message.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 371 */ 372 public MessageDefinitionFocusComponent setCodeElement(CodeType value) { 373 this.code = value; 374 return this; 375 } 376 377 /** 378 * @return The kind of resource that must be the focus for this message. 379 */ 380 public String getCode() { 381 return this.code == null ? null : this.code.getValue(); 382 } 383 384 /** 385 * @param value The kind of resource that must be the focus for this message. 386 */ 387 public MessageDefinitionFocusComponent setCode(String value) { 388 if (this.code == null) 389 this.code = new CodeType(); 390 this.code.setValue(value); 391 return this; 392 } 393 394 /** 395 * @return {@link #profile} (A profile that reflects constraints for the focal resource (and potentially for related resources).). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 396 */ 397 public CanonicalType getProfileElement() { 398 if (this.profile == null) 399 if (Configuration.errorOnAutoCreate()) 400 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.profile"); 401 else if (Configuration.doAutoCreate()) 402 this.profile = new CanonicalType(); // bb 403 return this.profile; 404 } 405 406 public boolean hasProfileElement() { 407 return this.profile != null && !this.profile.isEmpty(); 408 } 409 410 public boolean hasProfile() { 411 return this.profile != null && !this.profile.isEmpty(); 412 } 413 414 /** 415 * @param value {@link #profile} (A profile that reflects constraints for the focal resource (and potentially for related resources).). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 416 */ 417 public MessageDefinitionFocusComponent setProfileElement(CanonicalType value) { 418 this.profile = value; 419 return this; 420 } 421 422 /** 423 * @return A profile that reflects constraints for the focal resource (and potentially for related resources). 424 */ 425 public String getProfile() { 426 return this.profile == null ? null : this.profile.getValue(); 427 } 428 429 /** 430 * @param value A profile that reflects constraints for the focal resource (and potentially for related resources). 431 */ 432 public MessageDefinitionFocusComponent setProfile(String value) { 433 if (Utilities.noString(value)) 434 this.profile = null; 435 else { 436 if (this.profile == null) 437 this.profile = new CanonicalType(); 438 this.profile.setValue(value); 439 } 440 return this; 441 } 442 443 /** 444 * @return {@link #min} (Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 445 */ 446 public UnsignedIntType getMinElement() { 447 if (this.min == null) 448 if (Configuration.errorOnAutoCreate()) 449 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.min"); 450 else if (Configuration.doAutoCreate()) 451 this.min = new UnsignedIntType(); // bb 452 return this.min; 453 } 454 455 public boolean hasMinElement() { 456 return this.min != null && !this.min.isEmpty(); 457 } 458 459 public boolean hasMin() { 460 return this.min != null && !this.min.isEmpty(); 461 } 462 463 /** 464 * @param value {@link #min} (Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 465 */ 466 public MessageDefinitionFocusComponent setMinElement(UnsignedIntType value) { 467 this.min = value; 468 return this; 469 } 470 471 /** 472 * @return Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 473 */ 474 public int getMin() { 475 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 476 } 477 478 /** 479 * @param value Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 480 */ 481 public MessageDefinitionFocusComponent setMin(int value) { 482 if (this.min == null) 483 this.min = new UnsignedIntType(); 484 this.min.setValue(value); 485 return this; 486 } 487 488 /** 489 * @return {@link #max} (Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 490 */ 491 public StringType getMaxElement() { 492 if (this.max == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.max"); 495 else if (Configuration.doAutoCreate()) 496 this.max = new StringType(); // bb 497 return this.max; 498 } 499 500 public boolean hasMaxElement() { 501 return this.max != null && !this.max.isEmpty(); 502 } 503 504 public boolean hasMax() { 505 return this.max != null && !this.max.isEmpty(); 506 } 507 508 /** 509 * @param value {@link #max} (Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 510 */ 511 public MessageDefinitionFocusComponent setMaxElement(StringType value) { 512 this.max = value; 513 return this; 514 } 515 516 /** 517 * @return Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 518 */ 519 public String getMax() { 520 return this.max == null ? null : this.max.getValue(); 521 } 522 523 /** 524 * @param value Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 525 */ 526 public MessageDefinitionFocusComponent setMax(String value) { 527 if (Utilities.noString(value)) 528 this.max = null; 529 else { 530 if (this.max == null) 531 this.max = new StringType(); 532 this.max.setValue(value); 533 } 534 return this; 535 } 536 537 protected void listChildren(List<Property> children) { 538 super.listChildren(children); 539 children.add(new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code)); 540 children.add(new Property("profile", "canonical(StructureDefinition)", "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, profile)); 541 children.add(new Property("min", "unsignedInt", "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, min)); 542 children.add(new Property("max", "string", "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, max)); 543 } 544 545 @Override 546 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 547 switch (_hash) { 548 case 3059181: /*code*/ return new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code); 549 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, profile); 550 case 108114: /*min*/ return new Property("min", "unsignedInt", "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, min); 551 case 107876: /*max*/ return new Property("max", "string", "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, max); 552 default: return super.getNamedProperty(_hash, _name, _checkValid); 553 } 554 555 } 556 557 @Override 558 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 559 switch (hash) { 560 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 561 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 562 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 563 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 564 default: return super.getProperty(hash, name, checkValid); 565 } 566 567 } 568 569 @Override 570 public Base setProperty(int hash, String name, Base value) throws FHIRException { 571 switch (hash) { 572 case 3059181: // code 573 this.code = TypeConvertor.castToCode(value); // CodeType 574 return value; 575 case -309425751: // profile 576 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 577 return value; 578 case 108114: // min 579 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 580 return value; 581 case 107876: // max 582 this.max = TypeConvertor.castToString(value); // StringType 583 return value; 584 default: return super.setProperty(hash, name, value); 585 } 586 587 } 588 589 @Override 590 public Base setProperty(String name, Base value) throws FHIRException { 591 if (name.equals("code")) { 592 this.code = TypeConvertor.castToCode(value); // CodeType 593 } else if (name.equals("profile")) { 594 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 595 } else if (name.equals("min")) { 596 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 597 } else if (name.equals("max")) { 598 this.max = TypeConvertor.castToString(value); // StringType 599 } else 600 return super.setProperty(name, value); 601 return value; 602 } 603 604 @Override 605 public void removeChild(String name, Base value) throws FHIRException { 606 if (name.equals("code")) { 607 this.code = null; 608 } else if (name.equals("profile")) { 609 this.profile = null; 610 } else if (name.equals("min")) { 611 this.min = null; 612 } else if (name.equals("max")) { 613 this.max = null; 614 } else 615 super.removeChild(name, value); 616 617 } 618 619 @Override 620 public Base makeProperty(int hash, String name) throws FHIRException { 621 switch (hash) { 622 case 3059181: return getCodeElement(); 623 case -309425751: return getProfileElement(); 624 case 108114: return getMinElement(); 625 case 107876: return getMaxElement(); 626 default: return super.makeProperty(hash, name); 627 } 628 629 } 630 631 @Override 632 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 633 switch (hash) { 634 case 3059181: /*code*/ return new String[] {"code"}; 635 case -309425751: /*profile*/ return new String[] {"canonical"}; 636 case 108114: /*min*/ return new String[] {"unsignedInt"}; 637 case 107876: /*max*/ return new String[] {"string"}; 638 default: return super.getTypesForProperty(hash, name); 639 } 640 641 } 642 643 @Override 644 public Base addChild(String name) throws FHIRException { 645 if (name.equals("code")) { 646 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.code"); 647 } 648 else if (name.equals("profile")) { 649 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.profile"); 650 } 651 else if (name.equals("min")) { 652 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.min"); 653 } 654 else if (name.equals("max")) { 655 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.max"); 656 } 657 else 658 return super.addChild(name); 659 } 660 661 public MessageDefinitionFocusComponent copy() { 662 MessageDefinitionFocusComponent dst = new MessageDefinitionFocusComponent(); 663 copyValues(dst); 664 return dst; 665 } 666 667 public void copyValues(MessageDefinitionFocusComponent dst) { 668 super.copyValues(dst); 669 dst.code = code == null ? null : code.copy(); 670 dst.profile = profile == null ? null : profile.copy(); 671 dst.min = min == null ? null : min.copy(); 672 dst.max = max == null ? null : max.copy(); 673 } 674 675 @Override 676 public boolean equalsDeep(Base other_) { 677 if (!super.equalsDeep(other_)) 678 return false; 679 if (!(other_ instanceof MessageDefinitionFocusComponent)) 680 return false; 681 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 682 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(min, o.min, true) 683 && compareDeep(max, o.max, true); 684 } 685 686 @Override 687 public boolean equalsShallow(Base other_) { 688 if (!super.equalsShallow(other_)) 689 return false; 690 if (!(other_ instanceof MessageDefinitionFocusComponent)) 691 return false; 692 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 693 return compareValues(code, o.code, true) && compareValues(profile, o.profile, true) && compareValues(min, o.min, true) 694 && compareValues(max, o.max, true); 695 } 696 697 public boolean isEmpty() { 698 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, min, max 699 ); 700 } 701 702 public String fhirType() { 703 return "MessageDefinition.focus"; 704 705 } 706 707 } 708 709 @Block() 710 public static class MessageDefinitionAllowedResponseComponent extends BackboneElement implements IBaseBackboneElement { 711 /** 712 * A reference to the message definition that must be adhered to by this supported response. 713 */ 714 @Child(name = "message", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 715 @Description(shortDefinition="Reference to allowed message definition response", formalDefinition="A reference to the message definition that must be adhered to by this supported response." ) 716 protected CanonicalType message; 717 718 /** 719 * Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 720 */ 721 @Child(name = "situation", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 722 @Description(shortDefinition="When should this response be used", formalDefinition="Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses)." ) 723 protected MarkdownType situation; 724 725 private static final long serialVersionUID = -1943810550L; 726 727 /** 728 * Constructor 729 */ 730 public MessageDefinitionAllowedResponseComponent() { 731 super(); 732 } 733 734 /** 735 * Constructor 736 */ 737 public MessageDefinitionAllowedResponseComponent(String message) { 738 super(); 739 this.setMessage(message); 740 } 741 742 /** 743 * @return {@link #message} (A reference to the message definition that must be adhered to by this supported response.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 744 */ 745 public CanonicalType getMessageElement() { 746 if (this.message == null) 747 if (Configuration.errorOnAutoCreate()) 748 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.message"); 749 else if (Configuration.doAutoCreate()) 750 this.message = new CanonicalType(); // bb 751 return this.message; 752 } 753 754 public boolean hasMessageElement() { 755 return this.message != null && !this.message.isEmpty(); 756 } 757 758 public boolean hasMessage() { 759 return this.message != null && !this.message.isEmpty(); 760 } 761 762 /** 763 * @param value {@link #message} (A reference to the message definition that must be adhered to by this supported response.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 764 */ 765 public MessageDefinitionAllowedResponseComponent setMessageElement(CanonicalType value) { 766 this.message = value; 767 return this; 768 } 769 770 /** 771 * @return A reference to the message definition that must be adhered to by this supported response. 772 */ 773 public String getMessage() { 774 return this.message == null ? null : this.message.getValue(); 775 } 776 777 /** 778 * @param value A reference to the message definition that must be adhered to by this supported response. 779 */ 780 public MessageDefinitionAllowedResponseComponent setMessage(String value) { 781 if (this.message == null) 782 this.message = new CanonicalType(); 783 this.message.setValue(value); 784 return this; 785 } 786 787 /** 788 * @return {@link #situation} (Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).). This is the underlying object with id, value and extensions. The accessor "getSituation" gives direct access to the value 789 */ 790 public MarkdownType getSituationElement() { 791 if (this.situation == null) 792 if (Configuration.errorOnAutoCreate()) 793 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.situation"); 794 else if (Configuration.doAutoCreate()) 795 this.situation = new MarkdownType(); // bb 796 return this.situation; 797 } 798 799 public boolean hasSituationElement() { 800 return this.situation != null && !this.situation.isEmpty(); 801 } 802 803 public boolean hasSituation() { 804 return this.situation != null && !this.situation.isEmpty(); 805 } 806 807 /** 808 * @param value {@link #situation} (Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).). This is the underlying object with id, value and extensions. The accessor "getSituation" gives direct access to the value 809 */ 810 public MessageDefinitionAllowedResponseComponent setSituationElement(MarkdownType value) { 811 this.situation = value; 812 return this; 813 } 814 815 /** 816 * @return Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 817 */ 818 public String getSituation() { 819 return this.situation == null ? null : this.situation.getValue(); 820 } 821 822 /** 823 * @param value Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 824 */ 825 public MessageDefinitionAllowedResponseComponent setSituation(String value) { 826 if (Utilities.noString(value)) 827 this.situation = null; 828 else { 829 if (this.situation == null) 830 this.situation = new MarkdownType(); 831 this.situation.setValue(value); 832 } 833 return this; 834 } 835 836 protected void listChildren(List<Property> children) { 837 super.listChildren(children); 838 children.add(new Property("message", "canonical(MessageDefinition)", "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message)); 839 children.add(new Property("situation", "markdown", "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 0, 1, situation)); 840 } 841 842 @Override 843 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 844 switch (_hash) { 845 case 954925063: /*message*/ return new Property("message", "canonical(MessageDefinition)", "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message); 846 case -73377282: /*situation*/ return new Property("situation", "markdown", "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 0, 1, situation); 847 default: return super.getNamedProperty(_hash, _name, _checkValid); 848 } 849 850 } 851 852 @Override 853 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 854 switch (hash) { 855 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // CanonicalType 856 case -73377282: /*situation*/ return this.situation == null ? new Base[0] : new Base[] {this.situation}; // MarkdownType 857 default: return super.getProperty(hash, name, checkValid); 858 } 859 860 } 861 862 @Override 863 public Base setProperty(int hash, String name, Base value) throws FHIRException { 864 switch (hash) { 865 case 954925063: // message 866 this.message = TypeConvertor.castToCanonical(value); // CanonicalType 867 return value; 868 case -73377282: // situation 869 this.situation = TypeConvertor.castToMarkdown(value); // MarkdownType 870 return value; 871 default: return super.setProperty(hash, name, value); 872 } 873 874 } 875 876 @Override 877 public Base setProperty(String name, Base value) throws FHIRException { 878 if (name.equals("message")) { 879 this.message = TypeConvertor.castToCanonical(value); // CanonicalType 880 } else if (name.equals("situation")) { 881 this.situation = TypeConvertor.castToMarkdown(value); // MarkdownType 882 } else 883 return super.setProperty(name, value); 884 return value; 885 } 886 887 @Override 888 public void removeChild(String name, Base value) throws FHIRException { 889 if (name.equals("message")) { 890 this.message = null; 891 } else if (name.equals("situation")) { 892 this.situation = null; 893 } else 894 super.removeChild(name, value); 895 896 } 897 898 @Override 899 public Base makeProperty(int hash, String name) throws FHIRException { 900 switch (hash) { 901 case 954925063: return getMessageElement(); 902 case -73377282: return getSituationElement(); 903 default: return super.makeProperty(hash, name); 904 } 905 906 } 907 908 @Override 909 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 910 switch (hash) { 911 case 954925063: /*message*/ return new String[] {"canonical"}; 912 case -73377282: /*situation*/ return new String[] {"markdown"}; 913 default: return super.getTypesForProperty(hash, name); 914 } 915 916 } 917 918 @Override 919 public Base addChild(String name) throws FHIRException { 920 if (name.equals("message")) { 921 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.allowedResponse.message"); 922 } 923 else if (name.equals("situation")) { 924 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.allowedResponse.situation"); 925 } 926 else 927 return super.addChild(name); 928 } 929 930 public MessageDefinitionAllowedResponseComponent copy() { 931 MessageDefinitionAllowedResponseComponent dst = new MessageDefinitionAllowedResponseComponent(); 932 copyValues(dst); 933 return dst; 934 } 935 936 public void copyValues(MessageDefinitionAllowedResponseComponent dst) { 937 super.copyValues(dst); 938 dst.message = message == null ? null : message.copy(); 939 dst.situation = situation == null ? null : situation.copy(); 940 } 941 942 @Override 943 public boolean equalsDeep(Base other_) { 944 if (!super.equalsDeep(other_)) 945 return false; 946 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 947 return false; 948 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 949 return compareDeep(message, o.message, true) && compareDeep(situation, o.situation, true); 950 } 951 952 @Override 953 public boolean equalsShallow(Base other_) { 954 if (!super.equalsShallow(other_)) 955 return false; 956 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 957 return false; 958 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 959 return compareValues(message, o.message, true) && compareValues(situation, o.situation, true); 960 } 961 962 public boolean isEmpty() { 963 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(message, situation); 964 } 965 966 public String fhirType() { 967 return "MessageDefinition.allowedResponse"; 968 969 } 970 971 } 972 973 /** 974 * The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server. 975 */ 976 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 977 @Description(shortDefinition="The cannonical URL for a given MessageDefinition", formalDefinition="The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server." ) 978 protected UriType url; 979 980 /** 981 * A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 982 */ 983 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 984 @Description(shortDefinition="Business Identifier for a given MessageDefinition", formalDefinition="A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 985 protected List<Identifier> identifier; 986 987 /** 988 * The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 989 */ 990 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 991 @Description(shortDefinition="Business version of the message definition", formalDefinition="The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 992 protected StringType version; 993 994 /** 995 * Indicates the mechanism used to compare versions to determine which is more current. 996 */ 997 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 998 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 999 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1000 protected DataType versionAlgorithm; 1001 1002 /** 1003 * A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1004 */ 1005 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1006 @Description(shortDefinition="Name for this message definition (computer friendly)", formalDefinition="A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1007 protected StringType name; 1008 1009 /** 1010 * A short, descriptive, user-friendly title for the message definition. 1011 */ 1012 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1013 @Description(shortDefinition="Name for this message definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the message definition." ) 1014 protected StringType title; 1015 1016 /** 1017 * A MessageDefinition that is superseded by this definition. 1018 */ 1019 @Child(name = "replaces", type = {CanonicalType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1020 @Description(shortDefinition="Takes the place of", formalDefinition="A MessageDefinition that is superseded by this definition." ) 1021 protected List<CanonicalType> replaces; 1022 1023 /** 1024 * The status of this message definition. Enables tracking the life-cycle of the content. 1025 */ 1026 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 1027 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this message definition. Enables tracking the life-cycle of the content." ) 1028 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1029 protected Enumeration<PublicationStatus> status; 1030 1031 /** 1032 * A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1033 */ 1034 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1035 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1036 protected BooleanType experimental; 1037 1038 /** 1039 * The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1040 */ 1041 @Child(name = "date", type = {DateTimeType.class}, order=9, min=1, max=1, modifier=false, summary=true) 1042 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes." ) 1043 protected DateTimeType date; 1044 1045 /** 1046 * The name of the organization or individual responsible for the release and ongoing maintenance of the message definition. 1047 */ 1048 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1049 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the message definition." ) 1050 protected StringType publisher; 1051 1052 /** 1053 * Contact details to assist a user in finding and communicating with the publisher. 1054 */ 1055 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1056 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1057 protected List<ContactDetail> contact; 1058 1059 /** 1060 * A free text natural language description of the message definition from a consumer's perspective. 1061 */ 1062 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1063 @Description(shortDefinition="Natural language description of the message definition", formalDefinition="A free text natural language description of the message definition from a consumer's perspective." ) 1064 protected MarkdownType description; 1065 1066 /** 1067 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances. 1068 */ 1069 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1070 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances." ) 1071 protected List<UsageContext> useContext; 1072 1073 /** 1074 * A legal or geographic region in which the message definition is intended to be used. 1075 */ 1076 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1077 @Description(shortDefinition="Intended jurisdiction for message definition (if applicable)", formalDefinition="A legal or geographic region in which the message definition is intended to be used." ) 1078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1079 protected List<CodeableConcept> jurisdiction; 1080 1081 /** 1082 * Explanation of why this message definition is needed and why it has been designed as it has. 1083 */ 1084 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=true) 1085 @Description(shortDefinition="Why this message definition is defined", formalDefinition="Explanation of why this message definition is needed and why it has been designed as it has." ) 1086 protected MarkdownType purpose; 1087 1088 /** 1089 * A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 1090 */ 1091 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1092 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition." ) 1093 protected MarkdownType copyright; 1094 1095 /** 1096 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1097 */ 1098 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1099 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1100 protected StringType copyrightLabel; 1101 1102 /** 1103 * The MessageDefinition that is the basis for the contents of this resource. 1104 */ 1105 @Child(name = "base", type = {CanonicalType.class}, order=18, min=0, max=1, modifier=false, summary=true) 1106 @Description(shortDefinition="Definition this one is based on", formalDefinition="The MessageDefinition that is the basis for the contents of this resource." ) 1107 protected CanonicalType base; 1108 1109 /** 1110 * Identifies a protocol or workflow that this MessageDefinition represents a step in. 1111 */ 1112 @Child(name = "parent", type = {CanonicalType.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1113 @Description(shortDefinition="Protocol/workflow this is part of", formalDefinition="Identifies a protocol or workflow that this MessageDefinition represents a step in." ) 1114 protected List<CanonicalType> parent; 1115 1116 /** 1117 * Event code or link to the EventDefinition. 1118 */ 1119 @Child(name = "event", type = {Coding.class, UriType.class}, order=20, min=1, max=1, modifier=false, summary=true) 1120 @Description(shortDefinition="Event code or link to the EventDefinition", formalDefinition="Event code or link to the EventDefinition." ) 1121 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-events") 1122 protected DataType event; 1123 1124 /** 1125 * The impact of the content of the message. 1126 */ 1127 @Child(name = "category", type = {CodeType.class}, order=21, min=0, max=1, modifier=false, summary=true) 1128 @Description(shortDefinition="consequence | currency | notification", formalDefinition="The impact of the content of the message." ) 1129 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-significance-category") 1130 protected Enumeration<MessageSignificanceCategory> category; 1131 1132 /** 1133 * Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge. 1134 */ 1135 @Child(name = "focus", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1136 @Description(shortDefinition="Resource(s) that are the subject of the event", formalDefinition="Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge." ) 1137 protected List<MessageDefinitionFocusComponent> focus; 1138 1139 /** 1140 * Declare at a message definition level whether a response is required or only upon error or success, or never. 1141 */ 1142 @Child(name = "responseRequired", type = {CodeType.class}, order=23, min=0, max=1, modifier=false, summary=false) 1143 @Description(shortDefinition="always | on-error | never | on-success", formalDefinition="Declare at a message definition level whether a response is required or only upon error or success, or never." ) 1144 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/messageheader-response-request") 1145 protected Enumeration<MessageheaderResponseRequest> responseRequired; 1146 1147 /** 1148 * Indicates what types of messages may be sent as an application-level response to this message. 1149 */ 1150 @Child(name = "allowedResponse", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1151 @Description(shortDefinition="Responses to this message", formalDefinition="Indicates what types of messages may be sent as an application-level response to this message." ) 1152 protected List<MessageDefinitionAllowedResponseComponent> allowedResponse; 1153 1154 /** 1155 * Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources. 1156 */ 1157 @Child(name = "graph", type = {CanonicalType.class}, order=25, min=0, max=1, modifier=false, summary=false) 1158 @Description(shortDefinition="Canonical reference to a GraphDefinition", formalDefinition="Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources." ) 1159 protected CanonicalType graph; 1160 1161 private static final long serialVersionUID = 1378164694L; 1162 1163 /** 1164 * Constructor 1165 */ 1166 public MessageDefinition() { 1167 super(); 1168 } 1169 1170 /** 1171 * Constructor 1172 */ 1173 public MessageDefinition(PublicationStatus status, Date date, DataType event) { 1174 super(); 1175 this.setStatus(status); 1176 this.setDate(date); 1177 this.setEvent(event); 1178 } 1179 1180 /** 1181 * @return {@link #url} (The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1182 */ 1183 public UriType getUrlElement() { 1184 if (this.url == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create MessageDefinition.url"); 1187 else if (Configuration.doAutoCreate()) 1188 this.url = new UriType(); // bb 1189 return this.url; 1190 } 1191 1192 public boolean hasUrlElement() { 1193 return this.url != null && !this.url.isEmpty(); 1194 } 1195 1196 public boolean hasUrl() { 1197 return this.url != null && !this.url.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #url} (The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1202 */ 1203 public MessageDefinition setUrlElement(UriType value) { 1204 this.url = value; 1205 return this; 1206 } 1207 1208 /** 1209 * @return The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server. 1210 */ 1211 public String getUrl() { 1212 return this.url == null ? null : this.url.getValue(); 1213 } 1214 1215 /** 1216 * @param value The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server. 1217 */ 1218 public MessageDefinition setUrl(String value) { 1219 if (Utilities.noString(value)) 1220 this.url = null; 1221 else { 1222 if (this.url == null) 1223 this.url = new UriType(); 1224 this.url.setValue(value); 1225 } 1226 return this; 1227 } 1228 1229 /** 1230 * @return {@link #identifier} (A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1231 */ 1232 public List<Identifier> getIdentifier() { 1233 if (this.identifier == null) 1234 this.identifier = new ArrayList<Identifier>(); 1235 return this.identifier; 1236 } 1237 1238 /** 1239 * @return Returns a reference to <code>this</code> for easy method chaining 1240 */ 1241 public MessageDefinition setIdentifier(List<Identifier> theIdentifier) { 1242 this.identifier = theIdentifier; 1243 return this; 1244 } 1245 1246 public boolean hasIdentifier() { 1247 if (this.identifier == null) 1248 return false; 1249 for (Identifier item : this.identifier) 1250 if (!item.isEmpty()) 1251 return true; 1252 return false; 1253 } 1254 1255 public Identifier addIdentifier() { //3 1256 Identifier t = new Identifier(); 1257 if (this.identifier == null) 1258 this.identifier = new ArrayList<Identifier>(); 1259 this.identifier.add(t); 1260 return t; 1261 } 1262 1263 public MessageDefinition addIdentifier(Identifier t) { //3 1264 if (t == null) 1265 return this; 1266 if (this.identifier == null) 1267 this.identifier = new ArrayList<Identifier>(); 1268 this.identifier.add(t); 1269 return this; 1270 } 1271 1272 /** 1273 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1274 */ 1275 public Identifier getIdentifierFirstRep() { 1276 if (getIdentifier().isEmpty()) { 1277 addIdentifier(); 1278 } 1279 return getIdentifier().get(0); 1280 } 1281 1282 /** 1283 * @return {@link #version} (The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1284 */ 1285 public StringType getVersionElement() { 1286 if (this.version == null) 1287 if (Configuration.errorOnAutoCreate()) 1288 throw new Error("Attempt to auto-create MessageDefinition.version"); 1289 else if (Configuration.doAutoCreate()) 1290 this.version = new StringType(); // bb 1291 return this.version; 1292 } 1293 1294 public boolean hasVersionElement() { 1295 return this.version != null && !this.version.isEmpty(); 1296 } 1297 1298 public boolean hasVersion() { 1299 return this.version != null && !this.version.isEmpty(); 1300 } 1301 1302 /** 1303 * @param value {@link #version} (The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1304 */ 1305 public MessageDefinition setVersionElement(StringType value) { 1306 this.version = value; 1307 return this; 1308 } 1309 1310 /** 1311 * @return The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1312 */ 1313 public String getVersion() { 1314 return this.version == null ? null : this.version.getValue(); 1315 } 1316 1317 /** 1318 * @param value The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1319 */ 1320 public MessageDefinition setVersion(String value) { 1321 if (Utilities.noString(value)) 1322 this.version = null; 1323 else { 1324 if (this.version == null) 1325 this.version = new StringType(); 1326 this.version.setValue(value); 1327 } 1328 return this; 1329 } 1330 1331 /** 1332 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1333 */ 1334 public DataType getVersionAlgorithm() { 1335 return this.versionAlgorithm; 1336 } 1337 1338 /** 1339 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1340 */ 1341 public StringType getVersionAlgorithmStringType() throws FHIRException { 1342 if (this.versionAlgorithm == null) 1343 this.versionAlgorithm = new StringType(); 1344 if (!(this.versionAlgorithm instanceof StringType)) 1345 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1346 return (StringType) this.versionAlgorithm; 1347 } 1348 1349 public boolean hasVersionAlgorithmStringType() { 1350 return this.versionAlgorithm instanceof StringType; 1351 } 1352 1353 /** 1354 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1355 */ 1356 public Coding getVersionAlgorithmCoding() throws FHIRException { 1357 if (this.versionAlgorithm == null) 1358 this.versionAlgorithm = new Coding(); 1359 if (!(this.versionAlgorithm instanceof Coding)) 1360 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1361 return (Coding) this.versionAlgorithm; 1362 } 1363 1364 public boolean hasVersionAlgorithmCoding() { 1365 return this.versionAlgorithm instanceof Coding; 1366 } 1367 1368 public boolean hasVersionAlgorithm() { 1369 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1374 */ 1375 public MessageDefinition setVersionAlgorithm(DataType value) { 1376 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1377 throw new FHIRException("Not the right type for MessageDefinition.versionAlgorithm[x]: "+value.fhirType()); 1378 this.versionAlgorithm = value; 1379 return this; 1380 } 1381 1382 /** 1383 * @return {@link #name} (A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1384 */ 1385 public StringType getNameElement() { 1386 if (this.name == null) 1387 if (Configuration.errorOnAutoCreate()) 1388 throw new Error("Attempt to auto-create MessageDefinition.name"); 1389 else if (Configuration.doAutoCreate()) 1390 this.name = new StringType(); // bb 1391 return this.name; 1392 } 1393 1394 public boolean hasNameElement() { 1395 return this.name != null && !this.name.isEmpty(); 1396 } 1397 1398 public boolean hasName() { 1399 return this.name != null && !this.name.isEmpty(); 1400 } 1401 1402 /** 1403 * @param value {@link #name} (A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1404 */ 1405 public MessageDefinition setNameElement(StringType value) { 1406 this.name = value; 1407 return this; 1408 } 1409 1410 /** 1411 * @return A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1412 */ 1413 public String getName() { 1414 return this.name == null ? null : this.name.getValue(); 1415 } 1416 1417 /** 1418 * @param value A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1419 */ 1420 public MessageDefinition setName(String value) { 1421 if (Utilities.noString(value)) 1422 this.name = null; 1423 else { 1424 if (this.name == null) 1425 this.name = new StringType(); 1426 this.name.setValue(value); 1427 } 1428 return this; 1429 } 1430 1431 /** 1432 * @return {@link #title} (A short, descriptive, user-friendly title for the message definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1433 */ 1434 public StringType getTitleElement() { 1435 if (this.title == null) 1436 if (Configuration.errorOnAutoCreate()) 1437 throw new Error("Attempt to auto-create MessageDefinition.title"); 1438 else if (Configuration.doAutoCreate()) 1439 this.title = new StringType(); // bb 1440 return this.title; 1441 } 1442 1443 public boolean hasTitleElement() { 1444 return this.title != null && !this.title.isEmpty(); 1445 } 1446 1447 public boolean hasTitle() { 1448 return this.title != null && !this.title.isEmpty(); 1449 } 1450 1451 /** 1452 * @param value {@link #title} (A short, descriptive, user-friendly title for the message definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1453 */ 1454 public MessageDefinition setTitleElement(StringType value) { 1455 this.title = value; 1456 return this; 1457 } 1458 1459 /** 1460 * @return A short, descriptive, user-friendly title for the message definition. 1461 */ 1462 public String getTitle() { 1463 return this.title == null ? null : this.title.getValue(); 1464 } 1465 1466 /** 1467 * @param value A short, descriptive, user-friendly title for the message definition. 1468 */ 1469 public MessageDefinition setTitle(String value) { 1470 if (Utilities.noString(value)) 1471 this.title = null; 1472 else { 1473 if (this.title == null) 1474 this.title = new StringType(); 1475 this.title.setValue(value); 1476 } 1477 return this; 1478 } 1479 1480 /** 1481 * @return {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1482 */ 1483 public List<CanonicalType> getReplaces() { 1484 if (this.replaces == null) 1485 this.replaces = new ArrayList<CanonicalType>(); 1486 return this.replaces; 1487 } 1488 1489 /** 1490 * @return Returns a reference to <code>this</code> for easy method chaining 1491 */ 1492 public MessageDefinition setReplaces(List<CanonicalType> theReplaces) { 1493 this.replaces = theReplaces; 1494 return this; 1495 } 1496 1497 public boolean hasReplaces() { 1498 if (this.replaces == null) 1499 return false; 1500 for (CanonicalType item : this.replaces) 1501 if (!item.isEmpty()) 1502 return true; 1503 return false; 1504 } 1505 1506 /** 1507 * @return {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1508 */ 1509 public CanonicalType addReplacesElement() {//2 1510 CanonicalType t = new CanonicalType(); 1511 if (this.replaces == null) 1512 this.replaces = new ArrayList<CanonicalType>(); 1513 this.replaces.add(t); 1514 return t; 1515 } 1516 1517 /** 1518 * @param value {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1519 */ 1520 public MessageDefinition addReplaces(String value) { //1 1521 CanonicalType t = new CanonicalType(); 1522 t.setValue(value); 1523 if (this.replaces == null) 1524 this.replaces = new ArrayList<CanonicalType>(); 1525 this.replaces.add(t); 1526 return this; 1527 } 1528 1529 /** 1530 * @param value {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1531 */ 1532 public boolean hasReplaces(String value) { 1533 if (this.replaces == null) 1534 return false; 1535 for (CanonicalType v : this.replaces) 1536 if (v.getValue().equals(value)) // canonical 1537 return true; 1538 return false; 1539 } 1540 1541 /** 1542 * @return {@link #status} (The status of this message definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1543 */ 1544 public Enumeration<PublicationStatus> getStatusElement() { 1545 if (this.status == null) 1546 if (Configuration.errorOnAutoCreate()) 1547 throw new Error("Attempt to auto-create MessageDefinition.status"); 1548 else if (Configuration.doAutoCreate()) 1549 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1550 return this.status; 1551 } 1552 1553 public boolean hasStatusElement() { 1554 return this.status != null && !this.status.isEmpty(); 1555 } 1556 1557 public boolean hasStatus() { 1558 return this.status != null && !this.status.isEmpty(); 1559 } 1560 1561 /** 1562 * @param value {@link #status} (The status of this message definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1563 */ 1564 public MessageDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1565 this.status = value; 1566 return this; 1567 } 1568 1569 /** 1570 * @return The status of this message definition. Enables tracking the life-cycle of the content. 1571 */ 1572 public PublicationStatus getStatus() { 1573 return this.status == null ? null : this.status.getValue(); 1574 } 1575 1576 /** 1577 * @param value The status of this message definition. Enables tracking the life-cycle of the content. 1578 */ 1579 public MessageDefinition setStatus(PublicationStatus value) { 1580 if (this.status == null) 1581 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1582 this.status.setValue(value); 1583 return this; 1584 } 1585 1586 /** 1587 * @return {@link #experimental} (A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1588 */ 1589 public BooleanType getExperimentalElement() { 1590 if (this.experimental == null) 1591 if (Configuration.errorOnAutoCreate()) 1592 throw new Error("Attempt to auto-create MessageDefinition.experimental"); 1593 else if (Configuration.doAutoCreate()) 1594 this.experimental = new BooleanType(); // bb 1595 return this.experimental; 1596 } 1597 1598 public boolean hasExperimentalElement() { 1599 return this.experimental != null && !this.experimental.isEmpty(); 1600 } 1601 1602 public boolean hasExperimental() { 1603 return this.experimental != null && !this.experimental.isEmpty(); 1604 } 1605 1606 /** 1607 * @param value {@link #experimental} (A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1608 */ 1609 public MessageDefinition setExperimentalElement(BooleanType value) { 1610 this.experimental = value; 1611 return this; 1612 } 1613 1614 /** 1615 * @return A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1616 */ 1617 public boolean getExperimental() { 1618 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1619 } 1620 1621 /** 1622 * @param value A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1623 */ 1624 public MessageDefinition setExperimental(boolean value) { 1625 if (this.experimental == null) 1626 this.experimental = new BooleanType(); 1627 this.experimental.setValue(value); 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #date} (The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1633 */ 1634 public DateTimeType getDateElement() { 1635 if (this.date == null) 1636 if (Configuration.errorOnAutoCreate()) 1637 throw new Error("Attempt to auto-create MessageDefinition.date"); 1638 else if (Configuration.doAutoCreate()) 1639 this.date = new DateTimeType(); // bb 1640 return this.date; 1641 } 1642 1643 public boolean hasDateElement() { 1644 return this.date != null && !this.date.isEmpty(); 1645 } 1646 1647 public boolean hasDate() { 1648 return this.date != null && !this.date.isEmpty(); 1649 } 1650 1651 /** 1652 * @param value {@link #date} (The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1653 */ 1654 public MessageDefinition setDateElement(DateTimeType value) { 1655 this.date = value; 1656 return this; 1657 } 1658 1659 /** 1660 * @return The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1661 */ 1662 public Date getDate() { 1663 return this.date == null ? null : this.date.getValue(); 1664 } 1665 1666 /** 1667 * @param value The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1668 */ 1669 public MessageDefinition setDate(Date value) { 1670 if (this.date == null) 1671 this.date = new DateTimeType(); 1672 this.date.setValue(value); 1673 return this; 1674 } 1675 1676 /** 1677 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1678 */ 1679 public StringType getPublisherElement() { 1680 if (this.publisher == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create MessageDefinition.publisher"); 1683 else if (Configuration.doAutoCreate()) 1684 this.publisher = new StringType(); // bb 1685 return this.publisher; 1686 } 1687 1688 public boolean hasPublisherElement() { 1689 return this.publisher != null && !this.publisher.isEmpty(); 1690 } 1691 1692 public boolean hasPublisher() { 1693 return this.publisher != null && !this.publisher.isEmpty(); 1694 } 1695 1696 /** 1697 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1698 */ 1699 public MessageDefinition setPublisherElement(StringType value) { 1700 this.publisher = value; 1701 return this; 1702 } 1703 1704 /** 1705 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the message definition. 1706 */ 1707 public String getPublisher() { 1708 return this.publisher == null ? null : this.publisher.getValue(); 1709 } 1710 1711 /** 1712 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the message definition. 1713 */ 1714 public MessageDefinition setPublisher(String value) { 1715 if (Utilities.noString(value)) 1716 this.publisher = null; 1717 else { 1718 if (this.publisher == null) 1719 this.publisher = new StringType(); 1720 this.publisher.setValue(value); 1721 } 1722 return this; 1723 } 1724 1725 /** 1726 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1727 */ 1728 public List<ContactDetail> getContact() { 1729 if (this.contact == null) 1730 this.contact = new ArrayList<ContactDetail>(); 1731 return this.contact; 1732 } 1733 1734 /** 1735 * @return Returns a reference to <code>this</code> for easy method chaining 1736 */ 1737 public MessageDefinition setContact(List<ContactDetail> theContact) { 1738 this.contact = theContact; 1739 return this; 1740 } 1741 1742 public boolean hasContact() { 1743 if (this.contact == null) 1744 return false; 1745 for (ContactDetail item : this.contact) 1746 if (!item.isEmpty()) 1747 return true; 1748 return false; 1749 } 1750 1751 public ContactDetail addContact() { //3 1752 ContactDetail t = new ContactDetail(); 1753 if (this.contact == null) 1754 this.contact = new ArrayList<ContactDetail>(); 1755 this.contact.add(t); 1756 return t; 1757 } 1758 1759 public MessageDefinition addContact(ContactDetail t) { //3 1760 if (t == null) 1761 return this; 1762 if (this.contact == null) 1763 this.contact = new ArrayList<ContactDetail>(); 1764 this.contact.add(t); 1765 return this; 1766 } 1767 1768 /** 1769 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1770 */ 1771 public ContactDetail getContactFirstRep() { 1772 if (getContact().isEmpty()) { 1773 addContact(); 1774 } 1775 return getContact().get(0); 1776 } 1777 1778 /** 1779 * @return {@link #description} (A free text natural language description of the message definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1780 */ 1781 public MarkdownType getDescriptionElement() { 1782 if (this.description == null) 1783 if (Configuration.errorOnAutoCreate()) 1784 throw new Error("Attempt to auto-create MessageDefinition.description"); 1785 else if (Configuration.doAutoCreate()) 1786 this.description = new MarkdownType(); // bb 1787 return this.description; 1788 } 1789 1790 public boolean hasDescriptionElement() { 1791 return this.description != null && !this.description.isEmpty(); 1792 } 1793 1794 public boolean hasDescription() { 1795 return this.description != null && !this.description.isEmpty(); 1796 } 1797 1798 /** 1799 * @param value {@link #description} (A free text natural language description of the message definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1800 */ 1801 public MessageDefinition setDescriptionElement(MarkdownType value) { 1802 this.description = value; 1803 return this; 1804 } 1805 1806 /** 1807 * @return A free text natural language description of the message definition from a consumer's perspective. 1808 */ 1809 public String getDescription() { 1810 return this.description == null ? null : this.description.getValue(); 1811 } 1812 1813 /** 1814 * @param value A free text natural language description of the message definition from a consumer's perspective. 1815 */ 1816 public MessageDefinition setDescription(String value) { 1817 if (Utilities.noString(value)) 1818 this.description = null; 1819 else { 1820 if (this.description == null) 1821 this.description = new MarkdownType(); 1822 this.description.setValue(value); 1823 } 1824 return this; 1825 } 1826 1827 /** 1828 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.) 1829 */ 1830 public List<UsageContext> getUseContext() { 1831 if (this.useContext == null) 1832 this.useContext = new ArrayList<UsageContext>(); 1833 return this.useContext; 1834 } 1835 1836 /** 1837 * @return Returns a reference to <code>this</code> for easy method chaining 1838 */ 1839 public MessageDefinition setUseContext(List<UsageContext> theUseContext) { 1840 this.useContext = theUseContext; 1841 return this; 1842 } 1843 1844 public boolean hasUseContext() { 1845 if (this.useContext == null) 1846 return false; 1847 for (UsageContext item : this.useContext) 1848 if (!item.isEmpty()) 1849 return true; 1850 return false; 1851 } 1852 1853 public UsageContext addUseContext() { //3 1854 UsageContext t = new UsageContext(); 1855 if (this.useContext == null) 1856 this.useContext = new ArrayList<UsageContext>(); 1857 this.useContext.add(t); 1858 return t; 1859 } 1860 1861 public MessageDefinition addUseContext(UsageContext t) { //3 1862 if (t == null) 1863 return this; 1864 if (this.useContext == null) 1865 this.useContext = new ArrayList<UsageContext>(); 1866 this.useContext.add(t); 1867 return this; 1868 } 1869 1870 /** 1871 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1872 */ 1873 public UsageContext getUseContextFirstRep() { 1874 if (getUseContext().isEmpty()) { 1875 addUseContext(); 1876 } 1877 return getUseContext().get(0); 1878 } 1879 1880 /** 1881 * @return {@link #jurisdiction} (A legal or geographic region in which the message definition is intended to be used.) 1882 */ 1883 public List<CodeableConcept> getJurisdiction() { 1884 if (this.jurisdiction == null) 1885 this.jurisdiction = new ArrayList<CodeableConcept>(); 1886 return this.jurisdiction; 1887 } 1888 1889 /** 1890 * @return Returns a reference to <code>this</code> for easy method chaining 1891 */ 1892 public MessageDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1893 this.jurisdiction = theJurisdiction; 1894 return this; 1895 } 1896 1897 public boolean hasJurisdiction() { 1898 if (this.jurisdiction == null) 1899 return false; 1900 for (CodeableConcept item : this.jurisdiction) 1901 if (!item.isEmpty()) 1902 return true; 1903 return false; 1904 } 1905 1906 public CodeableConcept addJurisdiction() { //3 1907 CodeableConcept t = new CodeableConcept(); 1908 if (this.jurisdiction == null) 1909 this.jurisdiction = new ArrayList<CodeableConcept>(); 1910 this.jurisdiction.add(t); 1911 return t; 1912 } 1913 1914 public MessageDefinition addJurisdiction(CodeableConcept t) { //3 1915 if (t == null) 1916 return this; 1917 if (this.jurisdiction == null) 1918 this.jurisdiction = new ArrayList<CodeableConcept>(); 1919 this.jurisdiction.add(t); 1920 return this; 1921 } 1922 1923 /** 1924 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1925 */ 1926 public CodeableConcept getJurisdictionFirstRep() { 1927 if (getJurisdiction().isEmpty()) { 1928 addJurisdiction(); 1929 } 1930 return getJurisdiction().get(0); 1931 } 1932 1933 /** 1934 * @return {@link #purpose} (Explanation of why this message definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1935 */ 1936 public MarkdownType getPurposeElement() { 1937 if (this.purpose == null) 1938 if (Configuration.errorOnAutoCreate()) 1939 throw new Error("Attempt to auto-create MessageDefinition.purpose"); 1940 else if (Configuration.doAutoCreate()) 1941 this.purpose = new MarkdownType(); // bb 1942 return this.purpose; 1943 } 1944 1945 public boolean hasPurposeElement() { 1946 return this.purpose != null && !this.purpose.isEmpty(); 1947 } 1948 1949 public boolean hasPurpose() { 1950 return this.purpose != null && !this.purpose.isEmpty(); 1951 } 1952 1953 /** 1954 * @param value {@link #purpose} (Explanation of why this message definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1955 */ 1956 public MessageDefinition setPurposeElement(MarkdownType value) { 1957 this.purpose = value; 1958 return this; 1959 } 1960 1961 /** 1962 * @return Explanation of why this message definition is needed and why it has been designed as it has. 1963 */ 1964 public String getPurpose() { 1965 return this.purpose == null ? null : this.purpose.getValue(); 1966 } 1967 1968 /** 1969 * @param value Explanation of why this message definition is needed and why it has been designed as it has. 1970 */ 1971 public MessageDefinition setPurpose(String value) { 1972 if (Utilities.noString(value)) 1973 this.purpose = null; 1974 else { 1975 if (this.purpose == null) 1976 this.purpose = new MarkdownType(); 1977 this.purpose.setValue(value); 1978 } 1979 return this; 1980 } 1981 1982 /** 1983 * @return {@link #copyright} (A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1984 */ 1985 public MarkdownType getCopyrightElement() { 1986 if (this.copyright == null) 1987 if (Configuration.errorOnAutoCreate()) 1988 throw new Error("Attempt to auto-create MessageDefinition.copyright"); 1989 else if (Configuration.doAutoCreate()) 1990 this.copyright = new MarkdownType(); // bb 1991 return this.copyright; 1992 } 1993 1994 public boolean hasCopyrightElement() { 1995 return this.copyright != null && !this.copyright.isEmpty(); 1996 } 1997 1998 public boolean hasCopyright() { 1999 return this.copyright != null && !this.copyright.isEmpty(); 2000 } 2001 2002 /** 2003 * @param value {@link #copyright} (A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2004 */ 2005 public MessageDefinition setCopyrightElement(MarkdownType value) { 2006 this.copyright = value; 2007 return this; 2008 } 2009 2010 /** 2011 * @return A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 2012 */ 2013 public String getCopyright() { 2014 return this.copyright == null ? null : this.copyright.getValue(); 2015 } 2016 2017 /** 2018 * @param value A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 2019 */ 2020 public MessageDefinition setCopyright(String value) { 2021 if (Utilities.noString(value)) 2022 this.copyright = null; 2023 else { 2024 if (this.copyright == null) 2025 this.copyright = new MarkdownType(); 2026 this.copyright.setValue(value); 2027 } 2028 return this; 2029 } 2030 2031 /** 2032 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2033 */ 2034 public StringType getCopyrightLabelElement() { 2035 if (this.copyrightLabel == null) 2036 if (Configuration.errorOnAutoCreate()) 2037 throw new Error("Attempt to auto-create MessageDefinition.copyrightLabel"); 2038 else if (Configuration.doAutoCreate()) 2039 this.copyrightLabel = new StringType(); // bb 2040 return this.copyrightLabel; 2041 } 2042 2043 public boolean hasCopyrightLabelElement() { 2044 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2045 } 2046 2047 public boolean hasCopyrightLabel() { 2048 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2049 } 2050 2051 /** 2052 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2053 */ 2054 public MessageDefinition setCopyrightLabelElement(StringType value) { 2055 this.copyrightLabel = value; 2056 return this; 2057 } 2058 2059 /** 2060 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2061 */ 2062 public String getCopyrightLabel() { 2063 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2064 } 2065 2066 /** 2067 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2068 */ 2069 public MessageDefinition setCopyrightLabel(String value) { 2070 if (Utilities.noString(value)) 2071 this.copyrightLabel = null; 2072 else { 2073 if (this.copyrightLabel == null) 2074 this.copyrightLabel = new StringType(); 2075 this.copyrightLabel.setValue(value); 2076 } 2077 return this; 2078 } 2079 2080 /** 2081 * @return {@link #base} (The MessageDefinition that is the basis for the contents of this resource.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 2082 */ 2083 public CanonicalType getBaseElement() { 2084 if (this.base == null) 2085 if (Configuration.errorOnAutoCreate()) 2086 throw new Error("Attempt to auto-create MessageDefinition.base"); 2087 else if (Configuration.doAutoCreate()) 2088 this.base = new CanonicalType(); // bb 2089 return this.base; 2090 } 2091 2092 public boolean hasBaseElement() { 2093 return this.base != null && !this.base.isEmpty(); 2094 } 2095 2096 public boolean hasBase() { 2097 return this.base != null && !this.base.isEmpty(); 2098 } 2099 2100 /** 2101 * @param value {@link #base} (The MessageDefinition that is the basis for the contents of this resource.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 2102 */ 2103 public MessageDefinition setBaseElement(CanonicalType value) { 2104 this.base = value; 2105 return this; 2106 } 2107 2108 /** 2109 * @return The MessageDefinition that is the basis for the contents of this resource. 2110 */ 2111 public String getBase() { 2112 return this.base == null ? null : this.base.getValue(); 2113 } 2114 2115 /** 2116 * @param value The MessageDefinition that is the basis for the contents of this resource. 2117 */ 2118 public MessageDefinition setBase(String value) { 2119 if (Utilities.noString(value)) 2120 this.base = null; 2121 else { 2122 if (this.base == null) 2123 this.base = new CanonicalType(); 2124 this.base.setValue(value); 2125 } 2126 return this; 2127 } 2128 2129 /** 2130 * @return {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2131 */ 2132 public List<CanonicalType> getParent() { 2133 if (this.parent == null) 2134 this.parent = new ArrayList<CanonicalType>(); 2135 return this.parent; 2136 } 2137 2138 /** 2139 * @return Returns a reference to <code>this</code> for easy method chaining 2140 */ 2141 public MessageDefinition setParent(List<CanonicalType> theParent) { 2142 this.parent = theParent; 2143 return this; 2144 } 2145 2146 public boolean hasParent() { 2147 if (this.parent == null) 2148 return false; 2149 for (CanonicalType item : this.parent) 2150 if (!item.isEmpty()) 2151 return true; 2152 return false; 2153 } 2154 2155 /** 2156 * @return {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2157 */ 2158 public CanonicalType addParentElement() {//2 2159 CanonicalType t = new CanonicalType(); 2160 if (this.parent == null) 2161 this.parent = new ArrayList<CanonicalType>(); 2162 this.parent.add(t); 2163 return t; 2164 } 2165 2166 /** 2167 * @param value {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2168 */ 2169 public MessageDefinition addParent(String value) { //1 2170 CanonicalType t = new CanonicalType(); 2171 t.setValue(value); 2172 if (this.parent == null) 2173 this.parent = new ArrayList<CanonicalType>(); 2174 this.parent.add(t); 2175 return this; 2176 } 2177 2178 /** 2179 * @param value {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2180 */ 2181 public boolean hasParent(String value) { 2182 if (this.parent == null) 2183 return false; 2184 for (CanonicalType v : this.parent) 2185 if (v.getValue().equals(value)) // canonical 2186 return true; 2187 return false; 2188 } 2189 2190 /** 2191 * @return {@link #event} (Event code or link to the EventDefinition.) 2192 */ 2193 public DataType getEvent() { 2194 return this.event; 2195 } 2196 2197 /** 2198 * @return {@link #event} (Event code or link to the EventDefinition.) 2199 */ 2200 public Coding getEventCoding() throws FHIRException { 2201 if (this.event == null) 2202 this.event = new Coding(); 2203 if (!(this.event instanceof Coding)) 2204 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.event.getClass().getName()+" was encountered"); 2205 return (Coding) this.event; 2206 } 2207 2208 public boolean hasEventCoding() { 2209 return this.event instanceof Coding; 2210 } 2211 2212 /** 2213 * @return {@link #event} (Event code or link to the EventDefinition.) 2214 */ 2215 public UriType getEventUriType() throws FHIRException { 2216 if (this.event == null) 2217 this.event = new UriType(); 2218 if (!(this.event instanceof UriType)) 2219 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.event.getClass().getName()+" was encountered"); 2220 return (UriType) this.event; 2221 } 2222 2223 public boolean hasEventUriType() { 2224 return this.event instanceof UriType; 2225 } 2226 2227 public boolean hasEvent() { 2228 return this.event != null && !this.event.isEmpty(); 2229 } 2230 2231 /** 2232 * @param value {@link #event} (Event code or link to the EventDefinition.) 2233 */ 2234 public MessageDefinition setEvent(DataType value) { 2235 if (value != null && !(value instanceof Coding || value instanceof UriType)) 2236 throw new FHIRException("Not the right type for MessageDefinition.event[x]: "+value.fhirType()); 2237 this.event = value; 2238 return this; 2239 } 2240 2241 /** 2242 * @return {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 2243 */ 2244 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 2245 if (this.category == null) 2246 if (Configuration.errorOnAutoCreate()) 2247 throw new Error("Attempt to auto-create MessageDefinition.category"); 2248 else if (Configuration.doAutoCreate()) 2249 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 2250 return this.category; 2251 } 2252 2253 public boolean hasCategoryElement() { 2254 return this.category != null && !this.category.isEmpty(); 2255 } 2256 2257 public boolean hasCategory() { 2258 return this.category != null && !this.category.isEmpty(); 2259 } 2260 2261 /** 2262 * @param value {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 2263 */ 2264 public MessageDefinition setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 2265 this.category = value; 2266 return this; 2267 } 2268 2269 /** 2270 * @return The impact of the content of the message. 2271 */ 2272 public MessageSignificanceCategory getCategory() { 2273 return this.category == null ? null : this.category.getValue(); 2274 } 2275 2276 /** 2277 * @param value The impact of the content of the message. 2278 */ 2279 public MessageDefinition setCategory(MessageSignificanceCategory value) { 2280 if (value == null) 2281 this.category = null; 2282 else { 2283 if (this.category == null) 2284 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 2285 this.category.setValue(value); 2286 } 2287 return this; 2288 } 2289 2290 /** 2291 * @return {@link #focus} (Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.) 2292 */ 2293 public List<MessageDefinitionFocusComponent> getFocus() { 2294 if (this.focus == null) 2295 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2296 return this.focus; 2297 } 2298 2299 /** 2300 * @return Returns a reference to <code>this</code> for easy method chaining 2301 */ 2302 public MessageDefinition setFocus(List<MessageDefinitionFocusComponent> theFocus) { 2303 this.focus = theFocus; 2304 return this; 2305 } 2306 2307 public boolean hasFocus() { 2308 if (this.focus == null) 2309 return false; 2310 for (MessageDefinitionFocusComponent item : this.focus) 2311 if (!item.isEmpty()) 2312 return true; 2313 return false; 2314 } 2315 2316 public MessageDefinitionFocusComponent addFocus() { //3 2317 MessageDefinitionFocusComponent t = new MessageDefinitionFocusComponent(); 2318 if (this.focus == null) 2319 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2320 this.focus.add(t); 2321 return t; 2322 } 2323 2324 public MessageDefinition addFocus(MessageDefinitionFocusComponent t) { //3 2325 if (t == null) 2326 return this; 2327 if (this.focus == null) 2328 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2329 this.focus.add(t); 2330 return this; 2331 } 2332 2333 /** 2334 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 2335 */ 2336 public MessageDefinitionFocusComponent getFocusFirstRep() { 2337 if (getFocus().isEmpty()) { 2338 addFocus(); 2339 } 2340 return getFocus().get(0); 2341 } 2342 2343 /** 2344 * @return {@link #responseRequired} (Declare at a message definition level whether a response is required or only upon error or success, or never.). This is the underlying object with id, value and extensions. The accessor "getResponseRequired" gives direct access to the value 2345 */ 2346 public Enumeration<MessageheaderResponseRequest> getResponseRequiredElement() { 2347 if (this.responseRequired == null) 2348 if (Configuration.errorOnAutoCreate()) 2349 throw new Error("Attempt to auto-create MessageDefinition.responseRequired"); 2350 else if (Configuration.doAutoCreate()) 2351 this.responseRequired = new Enumeration<MessageheaderResponseRequest>(new MessageheaderResponseRequestEnumFactory()); // bb 2352 return this.responseRequired; 2353 } 2354 2355 public boolean hasResponseRequiredElement() { 2356 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2357 } 2358 2359 public boolean hasResponseRequired() { 2360 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2361 } 2362 2363 /** 2364 * @param value {@link #responseRequired} (Declare at a message definition level whether a response is required or only upon error or success, or never.). This is the underlying object with id, value and extensions. The accessor "getResponseRequired" gives direct access to the value 2365 */ 2366 public MessageDefinition setResponseRequiredElement(Enumeration<MessageheaderResponseRequest> value) { 2367 this.responseRequired = value; 2368 return this; 2369 } 2370 2371 /** 2372 * @return Declare at a message definition level whether a response is required or only upon error or success, or never. 2373 */ 2374 public MessageheaderResponseRequest getResponseRequired() { 2375 return this.responseRequired == null ? null : this.responseRequired.getValue(); 2376 } 2377 2378 /** 2379 * @param value Declare at a message definition level whether a response is required or only upon error or success, or never. 2380 */ 2381 public MessageDefinition setResponseRequired(MessageheaderResponseRequest value) { 2382 if (value == null) 2383 this.responseRequired = null; 2384 else { 2385 if (this.responseRequired == null) 2386 this.responseRequired = new Enumeration<MessageheaderResponseRequest>(new MessageheaderResponseRequestEnumFactory()); 2387 this.responseRequired.setValue(value); 2388 } 2389 return this; 2390 } 2391 2392 /** 2393 * @return {@link #allowedResponse} (Indicates what types of messages may be sent as an application-level response to this message.) 2394 */ 2395 public List<MessageDefinitionAllowedResponseComponent> getAllowedResponse() { 2396 if (this.allowedResponse == null) 2397 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2398 return this.allowedResponse; 2399 } 2400 2401 /** 2402 * @return Returns a reference to <code>this</code> for easy method chaining 2403 */ 2404 public MessageDefinition setAllowedResponse(List<MessageDefinitionAllowedResponseComponent> theAllowedResponse) { 2405 this.allowedResponse = theAllowedResponse; 2406 return this; 2407 } 2408 2409 public boolean hasAllowedResponse() { 2410 if (this.allowedResponse == null) 2411 return false; 2412 for (MessageDefinitionAllowedResponseComponent item : this.allowedResponse) 2413 if (!item.isEmpty()) 2414 return true; 2415 return false; 2416 } 2417 2418 public MessageDefinitionAllowedResponseComponent addAllowedResponse() { //3 2419 MessageDefinitionAllowedResponseComponent t = new MessageDefinitionAllowedResponseComponent(); 2420 if (this.allowedResponse == null) 2421 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2422 this.allowedResponse.add(t); 2423 return t; 2424 } 2425 2426 public MessageDefinition addAllowedResponse(MessageDefinitionAllowedResponseComponent t) { //3 2427 if (t == null) 2428 return this; 2429 if (this.allowedResponse == null) 2430 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2431 this.allowedResponse.add(t); 2432 return this; 2433 } 2434 2435 /** 2436 * @return The first repetition of repeating field {@link #allowedResponse}, creating it if it does not already exist {3} 2437 */ 2438 public MessageDefinitionAllowedResponseComponent getAllowedResponseFirstRep() { 2439 if (getAllowedResponse().isEmpty()) { 2440 addAllowedResponse(); 2441 } 2442 return getAllowedResponse().get(0); 2443 } 2444 2445 /** 2446 * @return {@link #graph} (Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.). This is the underlying object with id, value and extensions. The accessor "getGraph" gives direct access to the value 2447 */ 2448 public CanonicalType getGraphElement() { 2449 if (this.graph == null) 2450 if (Configuration.errorOnAutoCreate()) 2451 throw new Error("Attempt to auto-create MessageDefinition.graph"); 2452 else if (Configuration.doAutoCreate()) 2453 this.graph = new CanonicalType(); // bb 2454 return this.graph; 2455 } 2456 2457 public boolean hasGraphElement() { 2458 return this.graph != null && !this.graph.isEmpty(); 2459 } 2460 2461 public boolean hasGraph() { 2462 return this.graph != null && !this.graph.isEmpty(); 2463 } 2464 2465 /** 2466 * @param value {@link #graph} (Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.). This is the underlying object with id, value and extensions. The accessor "getGraph" gives direct access to the value 2467 */ 2468 public MessageDefinition setGraphElement(CanonicalType value) { 2469 this.graph = value; 2470 return this; 2471 } 2472 2473 /** 2474 * @return Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources. 2475 */ 2476 public String getGraph() { 2477 return this.graph == null ? null : this.graph.getValue(); 2478 } 2479 2480 /** 2481 * @param value Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources. 2482 */ 2483 public MessageDefinition setGraph(String value) { 2484 if (Utilities.noString(value)) 2485 this.graph = null; 2486 else { 2487 if (this.graph == null) 2488 this.graph = new CanonicalType(); 2489 this.graph.setValue(value); 2490 } 2491 return this; 2492 } 2493 2494 protected void listChildren(List<Property> children) { 2495 super.listChildren(children); 2496 children.add(new Property("url", "uri", "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 0, 1, url)); 2497 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2498 children.add(new Property("version", "string", "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2499 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2500 children.add(new Property("name", "string", "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2501 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the message definition.", 0, 1, title)); 2502 children.add(new Property("replaces", "canonical(MessageDefinition)", "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2503 children.add(new Property("status", "code", "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2504 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2505 children.add(new Property("date", "dateTime", "The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 0, 1, date)); 2506 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.", 0, 1, publisher)); 2507 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2508 children.add(new Property("description", "markdown", "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, description)); 2509 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2510 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the message definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2511 children.add(new Property("purpose", "markdown", "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2512 children.add(new Property("copyright", "markdown", "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 0, 1, copyright)); 2513 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2514 children.add(new Property("base", "canonical(MessageDefinition)", "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base)); 2515 children.add(new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, java.lang.Integer.MAX_VALUE, parent)); 2516 children.add(new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event)); 2517 children.add(new Property("category", "code", "The impact of the content of the message.", 0, 1, category)); 2518 children.add(new Property("focus", "", "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 0, java.lang.Integer.MAX_VALUE, focus)); 2519 children.add(new Property("responseRequired", "code", "Declare at a message definition level whether a response is required or only upon error or success, or never.", 0, 1, responseRequired)); 2520 children.add(new Property("allowedResponse", "", "Indicates what types of messages may be sent as an application-level response to this message.", 0, java.lang.Integer.MAX_VALUE, allowedResponse)); 2521 children.add(new Property("graph", "canonical(GraphDefinition)", "Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.", 0, 1, graph)); 2522 } 2523 2524 @Override 2525 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2526 switch (_hash) { 2527 case 116079: /*url*/ return new Property("url", "uri", "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 0, 1, url); 2528 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2529 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2530 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2531 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2532 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2533 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2534 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2535 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the message definition.", 0, 1, title); 2536 case -430332865: /*replaces*/ return new Property("replaces", "canonical(MessageDefinition)", "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces); 2537 case -892481550: /*status*/ return new Property("status", "code", "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2538 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2539 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 0, 1, date); 2540 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.", 0, 1, publisher); 2541 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2542 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, description); 2543 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2544 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the message definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2545 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose); 2546 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 0, 1, copyright); 2547 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2548 case 3016401: /*base*/ return new Property("base", "canonical(MessageDefinition)", "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base); 2549 case -995424086: /*parent*/ return new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, java.lang.Integer.MAX_VALUE, parent); 2550 case 278115238: /*event[x]*/ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event); 2551 case 96891546: /*event*/ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event); 2552 case -355957084: /*eventCoding*/ return new Property("event[x]", "Coding", "Event code or link to the EventDefinition.", 0, 1, event); 2553 case 278109298: /*eventUri*/ return new Property("event[x]", "uri", "Event code or link to the EventDefinition.", 0, 1, event); 2554 case 50511102: /*category*/ return new Property("category", "code", "The impact of the content of the message.", 0, 1, category); 2555 case 97604824: /*focus*/ return new Property("focus", "", "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 0, java.lang.Integer.MAX_VALUE, focus); 2556 case 791597824: /*responseRequired*/ return new Property("responseRequired", "code", "Declare at a message definition level whether a response is required or only upon error or success, or never.", 0, 1, responseRequired); 2557 case -1130933751: /*allowedResponse*/ return new Property("allowedResponse", "", "Indicates what types of messages may be sent as an application-level response to this message.", 0, java.lang.Integer.MAX_VALUE, allowedResponse); 2558 case 98615630: /*graph*/ return new Property("graph", "canonical(GraphDefinition)", "Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.", 0, 1, graph); 2559 default: return super.getNamedProperty(_hash, _name, _checkValid); 2560 } 2561 2562 } 2563 2564 @Override 2565 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2566 switch (hash) { 2567 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2568 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2569 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2570 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2571 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2572 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2573 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 2574 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2575 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2576 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2577 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2578 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2579 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2580 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2581 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2582 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2583 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2584 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2585 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // CanonicalType 2586 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // CanonicalType 2587 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // DataType 2588 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<MessageSignificanceCategory> 2589 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // MessageDefinitionFocusComponent 2590 case 791597824: /*responseRequired*/ return this.responseRequired == null ? new Base[0] : new Base[] {this.responseRequired}; // Enumeration<MessageheaderResponseRequest> 2591 case -1130933751: /*allowedResponse*/ return this.allowedResponse == null ? new Base[0] : this.allowedResponse.toArray(new Base[this.allowedResponse.size()]); // MessageDefinitionAllowedResponseComponent 2592 case 98615630: /*graph*/ return this.graph == null ? new Base[0] : new Base[] {this.graph}; // CanonicalType 2593 default: return super.getProperty(hash, name, checkValid); 2594 } 2595 2596 } 2597 2598 @Override 2599 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2600 switch (hash) { 2601 case 116079: // url 2602 this.url = TypeConvertor.castToUri(value); // UriType 2603 return value; 2604 case -1618432855: // identifier 2605 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2606 return value; 2607 case 351608024: // version 2608 this.version = TypeConvertor.castToString(value); // StringType 2609 return value; 2610 case 1508158071: // versionAlgorithm 2611 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2612 return value; 2613 case 3373707: // name 2614 this.name = TypeConvertor.castToString(value); // StringType 2615 return value; 2616 case 110371416: // title 2617 this.title = TypeConvertor.castToString(value); // StringType 2618 return value; 2619 case -430332865: // replaces 2620 this.getReplaces().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2621 return value; 2622 case -892481550: // status 2623 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2624 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2625 return value; 2626 case -404562712: // experimental 2627 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2628 return value; 2629 case 3076014: // date 2630 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2631 return value; 2632 case 1447404028: // publisher 2633 this.publisher = TypeConvertor.castToString(value); // StringType 2634 return value; 2635 case 951526432: // contact 2636 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2637 return value; 2638 case -1724546052: // description 2639 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2640 return value; 2641 case -669707736: // useContext 2642 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2643 return value; 2644 case -507075711: // jurisdiction 2645 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2646 return value; 2647 case -220463842: // purpose 2648 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2649 return value; 2650 case 1522889671: // copyright 2651 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2652 return value; 2653 case 765157229: // copyrightLabel 2654 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2655 return value; 2656 case 3016401: // base 2657 this.base = TypeConvertor.castToCanonical(value); // CanonicalType 2658 return value; 2659 case -995424086: // parent 2660 this.getParent().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2661 return value; 2662 case 96891546: // event 2663 this.event = TypeConvertor.castToType(value); // DataType 2664 return value; 2665 case 50511102: // category 2666 value = new MessageSignificanceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2667 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2668 return value; 2669 case 97604824: // focus 2670 this.getFocus().add((MessageDefinitionFocusComponent) value); // MessageDefinitionFocusComponent 2671 return value; 2672 case 791597824: // responseRequired 2673 value = new MessageheaderResponseRequestEnumFactory().fromType(TypeConvertor.castToCode(value)); 2674 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 2675 return value; 2676 case -1130933751: // allowedResponse 2677 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); // MessageDefinitionAllowedResponseComponent 2678 return value; 2679 case 98615630: // graph 2680 this.graph = TypeConvertor.castToCanonical(value); // CanonicalType 2681 return value; 2682 default: return super.setProperty(hash, name, value); 2683 } 2684 2685 } 2686 2687 @Override 2688 public Base setProperty(String name, Base value) throws FHIRException { 2689 if (name.equals("url")) { 2690 this.url = TypeConvertor.castToUri(value); // UriType 2691 } else if (name.equals("identifier")) { 2692 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2693 } else if (name.equals("version")) { 2694 this.version = TypeConvertor.castToString(value); // StringType 2695 } else if (name.equals("versionAlgorithm[x]")) { 2696 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2697 } else if (name.equals("name")) { 2698 this.name = TypeConvertor.castToString(value); // StringType 2699 } else if (name.equals("title")) { 2700 this.title = TypeConvertor.castToString(value); // StringType 2701 } else if (name.equals("replaces")) { 2702 this.getReplaces().add(TypeConvertor.castToCanonical(value)); 2703 } else if (name.equals("status")) { 2704 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2705 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2706 } else if (name.equals("experimental")) { 2707 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2708 } else if (name.equals("date")) { 2709 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2710 } else if (name.equals("publisher")) { 2711 this.publisher = TypeConvertor.castToString(value); // StringType 2712 } else if (name.equals("contact")) { 2713 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2714 } else if (name.equals("description")) { 2715 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2716 } else if (name.equals("useContext")) { 2717 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2718 } else if (name.equals("jurisdiction")) { 2719 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2720 } else if (name.equals("purpose")) { 2721 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2722 } else if (name.equals("copyright")) { 2723 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2724 } else if (name.equals("copyrightLabel")) { 2725 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2726 } else if (name.equals("base")) { 2727 this.base = TypeConvertor.castToCanonical(value); // CanonicalType 2728 } else if (name.equals("parent")) { 2729 this.getParent().add(TypeConvertor.castToCanonical(value)); 2730 } else if (name.equals("event[x]")) { 2731 this.event = TypeConvertor.castToType(value); // DataType 2732 } else if (name.equals("category")) { 2733 value = new MessageSignificanceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2734 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2735 } else if (name.equals("focus")) { 2736 this.getFocus().add((MessageDefinitionFocusComponent) value); 2737 } else if (name.equals("responseRequired")) { 2738 value = new MessageheaderResponseRequestEnumFactory().fromType(TypeConvertor.castToCode(value)); 2739 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 2740 } else if (name.equals("allowedResponse")) { 2741 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); 2742 } else if (name.equals("graph")) { 2743 this.graph = TypeConvertor.castToCanonical(value); // CanonicalType 2744 } else 2745 return super.setProperty(name, value); 2746 return value; 2747 } 2748 2749 @Override 2750 public void removeChild(String name, Base value) throws FHIRException { 2751 if (name.equals("url")) { 2752 this.url = null; 2753 } else if (name.equals("identifier")) { 2754 this.getIdentifier().remove(value); 2755 } else if (name.equals("version")) { 2756 this.version = null; 2757 } else if (name.equals("versionAlgorithm[x]")) { 2758 this.versionAlgorithm = null; 2759 } else if (name.equals("name")) { 2760 this.name = null; 2761 } else if (name.equals("title")) { 2762 this.title = null; 2763 } else if (name.equals("replaces")) { 2764 this.getReplaces().remove(value); 2765 } else if (name.equals("status")) { 2766 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2767 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2768 } else if (name.equals("experimental")) { 2769 this.experimental = null; 2770 } else if (name.equals("date")) { 2771 this.date = null; 2772 } else if (name.equals("publisher")) { 2773 this.publisher = null; 2774 } else if (name.equals("contact")) { 2775 this.getContact().remove(value); 2776 } else if (name.equals("description")) { 2777 this.description = null; 2778 } else if (name.equals("useContext")) { 2779 this.getUseContext().remove(value); 2780 } else if (name.equals("jurisdiction")) { 2781 this.getJurisdiction().remove(value); 2782 } else if (name.equals("purpose")) { 2783 this.purpose = null; 2784 } else if (name.equals("copyright")) { 2785 this.copyright = null; 2786 } else if (name.equals("copyrightLabel")) { 2787 this.copyrightLabel = null; 2788 } else if (name.equals("base")) { 2789 this.base = null; 2790 } else if (name.equals("parent")) { 2791 this.getParent().remove(value); 2792 } else if (name.equals("event[x]")) { 2793 this.event = null; 2794 } else if (name.equals("category")) { 2795 value = new MessageSignificanceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2796 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2797 } else if (name.equals("focus")) { 2798 this.getFocus().remove((MessageDefinitionFocusComponent) value); 2799 } else if (name.equals("responseRequired")) { 2800 value = new MessageheaderResponseRequestEnumFactory().fromType(TypeConvertor.castToCode(value)); 2801 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 2802 } else if (name.equals("allowedResponse")) { 2803 this.getAllowedResponse().remove((MessageDefinitionAllowedResponseComponent) value); 2804 } else if (name.equals("graph")) { 2805 this.graph = null; 2806 } else 2807 super.removeChild(name, value); 2808 2809 } 2810 2811 @Override 2812 public Base makeProperty(int hash, String name) throws FHIRException { 2813 switch (hash) { 2814 case 116079: return getUrlElement(); 2815 case -1618432855: return addIdentifier(); 2816 case 351608024: return getVersionElement(); 2817 case -115699031: return getVersionAlgorithm(); 2818 case 1508158071: return getVersionAlgorithm(); 2819 case 3373707: return getNameElement(); 2820 case 110371416: return getTitleElement(); 2821 case -430332865: return addReplacesElement(); 2822 case -892481550: return getStatusElement(); 2823 case -404562712: return getExperimentalElement(); 2824 case 3076014: return getDateElement(); 2825 case 1447404028: return getPublisherElement(); 2826 case 951526432: return addContact(); 2827 case -1724546052: return getDescriptionElement(); 2828 case -669707736: return addUseContext(); 2829 case -507075711: return addJurisdiction(); 2830 case -220463842: return getPurposeElement(); 2831 case 1522889671: return getCopyrightElement(); 2832 case 765157229: return getCopyrightLabelElement(); 2833 case 3016401: return getBaseElement(); 2834 case -995424086: return addParentElement(); 2835 case 278115238: return getEvent(); 2836 case 96891546: return getEvent(); 2837 case 50511102: return getCategoryElement(); 2838 case 97604824: return addFocus(); 2839 case 791597824: return getResponseRequiredElement(); 2840 case -1130933751: return addAllowedResponse(); 2841 case 98615630: return getGraphElement(); 2842 default: return super.makeProperty(hash, name); 2843 } 2844 2845 } 2846 2847 @Override 2848 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2849 switch (hash) { 2850 case 116079: /*url*/ return new String[] {"uri"}; 2851 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2852 case 351608024: /*version*/ return new String[] {"string"}; 2853 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2854 case 3373707: /*name*/ return new String[] {"string"}; 2855 case 110371416: /*title*/ return new String[] {"string"}; 2856 case -430332865: /*replaces*/ return new String[] {"canonical"}; 2857 case -892481550: /*status*/ return new String[] {"code"}; 2858 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2859 case 3076014: /*date*/ return new String[] {"dateTime"}; 2860 case 1447404028: /*publisher*/ return new String[] {"string"}; 2861 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2862 case -1724546052: /*description*/ return new String[] {"markdown"}; 2863 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2864 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2865 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2866 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2867 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2868 case 3016401: /*base*/ return new String[] {"canonical"}; 2869 case -995424086: /*parent*/ return new String[] {"canonical"}; 2870 case 96891546: /*event*/ return new String[] {"Coding", "uri"}; 2871 case 50511102: /*category*/ return new String[] {"code"}; 2872 case 97604824: /*focus*/ return new String[] {}; 2873 case 791597824: /*responseRequired*/ return new String[] {"code"}; 2874 case -1130933751: /*allowedResponse*/ return new String[] {}; 2875 case 98615630: /*graph*/ return new String[] {"canonical"}; 2876 default: return super.getTypesForProperty(hash, name); 2877 } 2878 2879 } 2880 2881 @Override 2882 public Base addChild(String name) throws FHIRException { 2883 if (name.equals("url")) { 2884 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.url"); 2885 } 2886 else if (name.equals("identifier")) { 2887 return addIdentifier(); 2888 } 2889 else if (name.equals("version")) { 2890 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.version"); 2891 } 2892 else if (name.equals("versionAlgorithmString")) { 2893 this.versionAlgorithm = new StringType(); 2894 return this.versionAlgorithm; 2895 } 2896 else if (name.equals("versionAlgorithmCoding")) { 2897 this.versionAlgorithm = new Coding(); 2898 return this.versionAlgorithm; 2899 } 2900 else if (name.equals("name")) { 2901 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.name"); 2902 } 2903 else if (name.equals("title")) { 2904 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.title"); 2905 } 2906 else if (name.equals("replaces")) { 2907 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.replaces"); 2908 } 2909 else if (name.equals("status")) { 2910 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.status"); 2911 } 2912 else if (name.equals("experimental")) { 2913 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.experimental"); 2914 } 2915 else if (name.equals("date")) { 2916 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.date"); 2917 } 2918 else if (name.equals("publisher")) { 2919 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.publisher"); 2920 } 2921 else if (name.equals("contact")) { 2922 return addContact(); 2923 } 2924 else if (name.equals("description")) { 2925 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.description"); 2926 } 2927 else if (name.equals("useContext")) { 2928 return addUseContext(); 2929 } 2930 else if (name.equals("jurisdiction")) { 2931 return addJurisdiction(); 2932 } 2933 else if (name.equals("purpose")) { 2934 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.purpose"); 2935 } 2936 else if (name.equals("copyright")) { 2937 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.copyright"); 2938 } 2939 else if (name.equals("copyrightLabel")) { 2940 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.copyrightLabel"); 2941 } 2942 else if (name.equals("base")) { 2943 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.base"); 2944 } 2945 else if (name.equals("parent")) { 2946 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.parent"); 2947 } 2948 else if (name.equals("eventCoding")) { 2949 this.event = new Coding(); 2950 return this.event; 2951 } 2952 else if (name.equals("eventUri")) { 2953 this.event = new UriType(); 2954 return this.event; 2955 } 2956 else if (name.equals("category")) { 2957 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.category"); 2958 } 2959 else if (name.equals("focus")) { 2960 return addFocus(); 2961 } 2962 else if (name.equals("responseRequired")) { 2963 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.responseRequired"); 2964 } 2965 else if (name.equals("allowedResponse")) { 2966 return addAllowedResponse(); 2967 } 2968 else if (name.equals("graph")) { 2969 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.graph"); 2970 } 2971 else 2972 return super.addChild(name); 2973 } 2974 2975 public String fhirType() { 2976 return "MessageDefinition"; 2977 2978 } 2979 2980 public MessageDefinition copy() { 2981 MessageDefinition dst = new MessageDefinition(); 2982 copyValues(dst); 2983 return dst; 2984 } 2985 2986 public void copyValues(MessageDefinition dst) { 2987 super.copyValues(dst); 2988 dst.url = url == null ? null : url.copy(); 2989 if (identifier != null) { 2990 dst.identifier = new ArrayList<Identifier>(); 2991 for (Identifier i : identifier) 2992 dst.identifier.add(i.copy()); 2993 }; 2994 dst.version = version == null ? null : version.copy(); 2995 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2996 dst.name = name == null ? null : name.copy(); 2997 dst.title = title == null ? null : title.copy(); 2998 if (replaces != null) { 2999 dst.replaces = new ArrayList<CanonicalType>(); 3000 for (CanonicalType i : replaces) 3001 dst.replaces.add(i.copy()); 3002 }; 3003 dst.status = status == null ? null : status.copy(); 3004 dst.experimental = experimental == null ? null : experimental.copy(); 3005 dst.date = date == null ? null : date.copy(); 3006 dst.publisher = publisher == null ? null : publisher.copy(); 3007 if (contact != null) { 3008 dst.contact = new ArrayList<ContactDetail>(); 3009 for (ContactDetail i : contact) 3010 dst.contact.add(i.copy()); 3011 }; 3012 dst.description = description == null ? null : description.copy(); 3013 if (useContext != null) { 3014 dst.useContext = new ArrayList<UsageContext>(); 3015 for (UsageContext i : useContext) 3016 dst.useContext.add(i.copy()); 3017 }; 3018 if (jurisdiction != null) { 3019 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3020 for (CodeableConcept i : jurisdiction) 3021 dst.jurisdiction.add(i.copy()); 3022 }; 3023 dst.purpose = purpose == null ? null : purpose.copy(); 3024 dst.copyright = copyright == null ? null : copyright.copy(); 3025 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 3026 dst.base = base == null ? null : base.copy(); 3027 if (parent != null) { 3028 dst.parent = new ArrayList<CanonicalType>(); 3029 for (CanonicalType i : parent) 3030 dst.parent.add(i.copy()); 3031 }; 3032 dst.event = event == null ? null : event.copy(); 3033 dst.category = category == null ? null : category.copy(); 3034 if (focus != null) { 3035 dst.focus = new ArrayList<MessageDefinitionFocusComponent>(); 3036 for (MessageDefinitionFocusComponent i : focus) 3037 dst.focus.add(i.copy()); 3038 }; 3039 dst.responseRequired = responseRequired == null ? null : responseRequired.copy(); 3040 if (allowedResponse != null) { 3041 dst.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 3042 for (MessageDefinitionAllowedResponseComponent i : allowedResponse) 3043 dst.allowedResponse.add(i.copy()); 3044 }; 3045 dst.graph = graph == null ? null : graph.copy(); 3046 } 3047 3048 protected MessageDefinition typedCopy() { 3049 return copy(); 3050 } 3051 3052 @Override 3053 public boolean equalsDeep(Base other_) { 3054 if (!super.equalsDeep(other_)) 3055 return false; 3056 if (!(other_ instanceof MessageDefinition)) 3057 return false; 3058 MessageDefinition o = (MessageDefinition) other_; 3059 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 3060 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 3061 && compareDeep(replaces, o.replaces, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 3062 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 3063 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 3064 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 3065 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(base, o.base, true) && compareDeep(parent, o.parent, true) 3066 && compareDeep(event, o.event, true) && compareDeep(category, o.category, true) && compareDeep(focus, o.focus, true) 3067 && compareDeep(responseRequired, o.responseRequired, true) && compareDeep(allowedResponse, o.allowedResponse, true) 3068 && compareDeep(graph, o.graph, true); 3069 } 3070 3071 @Override 3072 public boolean equalsShallow(Base other_) { 3073 if (!super.equalsShallow(other_)) 3074 return false; 3075 if (!(other_ instanceof MessageDefinition)) 3076 return false; 3077 MessageDefinition o = (MessageDefinition) other_; 3078 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 3079 && compareValues(title, o.title, true) && compareValues(replaces, o.replaces, true) && compareValues(status, o.status, true) 3080 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 3081 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 3082 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(base, o.base, true) && compareValues(parent, o.parent, true) 3083 && compareValues(category, o.category, true) && compareValues(responseRequired, o.responseRequired, true) 3084 && compareValues(graph, o.graph, true); 3085 } 3086 3087 public boolean isEmpty() { 3088 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 3089 , versionAlgorithm, name, title, replaces, status, experimental, date, publisher 3090 , contact, description, useContext, jurisdiction, purpose, copyright, copyrightLabel 3091 , base, parent, event, category, focus, responseRequired, allowedResponse, graph 3092 ); 3093 } 3094 3095 @Override 3096 public ResourceType getResourceType() { 3097 return ResourceType.MessageDefinition; 3098 } 3099 3100 /** 3101 * Search parameter: <b>context-quantity</b> 3102 * <p> 3103 * Description: <b>Multiple Resources: 3104 3105* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3106* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3107* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3108* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3109* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3110* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3111* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3112* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3113* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3114* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3115* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3116* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3117* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3118* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3119* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3120* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3121* [Library](library.html): A quantity- or range-valued use context assigned to the library 3122* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3123* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3124* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3125* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3126* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3127* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3128* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3129* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3130* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3131* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3132* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3133* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3134* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3135</b><br> 3136 * Type: <b>quantity</b><br> 3137 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3138 * </p> 3139 */ 3140 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 3141 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3142 /** 3143 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3144 * <p> 3145 * Description: <b>Multiple Resources: 3146 3147* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3148* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3149* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3150* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3151* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3152* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3153* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3154* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3155* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3156* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3157* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3158* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3159* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3160* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3161* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3162* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3163* [Library](library.html): A quantity- or range-valued use context assigned to the library 3164* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3165* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3166* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3167* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3168* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3169* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3170* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3171* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3172* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3173* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3174* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3175* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3176* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3177</b><br> 3178 * Type: <b>quantity</b><br> 3179 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3180 * </p> 3181 */ 3182 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3183 3184 /** 3185 * Search parameter: <b>context-type-quantity</b> 3186 * <p> 3187 * Description: <b>Multiple Resources: 3188 3189* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3190* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3191* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3192* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3193* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3194* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3195* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3196* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3197* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3198* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3199* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3200* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3201* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3202* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3203* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3204* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3205* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3206* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3207* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3208* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3209* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3210* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3211* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3212* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3213* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3214* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3215* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3216* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3217* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3218* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3219</b><br> 3220 * Type: <b>composite</b><br> 3221 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3222 * </p> 3223 */ 3224 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3225 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3226 /** 3227 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3228 * <p> 3229 * Description: <b>Multiple Resources: 3230 3231* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3232* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3233* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3234* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3235* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3236* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3237* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3238* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3239* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3240* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3241* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3242* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3243* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3244* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3245* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3246* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3247* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3248* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3249* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3250* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3251* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3252* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3253* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3254* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3255* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3256* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3257* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3258* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3259* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3260* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3261</b><br> 3262 * Type: <b>composite</b><br> 3263 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3264 * </p> 3265 */ 3266 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3267 3268 /** 3269 * Search parameter: <b>context-type-value</b> 3270 * <p> 3271 * Description: <b>Multiple Resources: 3272 3273* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3274* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3275* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3276* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3277* [Citation](citation.html): A use context type and value assigned to the citation 3278* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3279* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3280* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3281* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3282* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3283* [Evidence](evidence.html): A use context type and value assigned to the evidence 3284* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3285* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3286* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3287* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3288* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3289* [Library](library.html): A use context type and value assigned to the library 3290* [Measure](measure.html): A use context type and value assigned to the measure 3291* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3292* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3293* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3294* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3295* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3296* [Requirements](requirements.html): A use context type and value assigned to the requirements 3297* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3298* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3299* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3300* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3301* [TestScript](testscript.html): A use context type and value assigned to the test script 3302* [ValueSet](valueset.html): A use context type and value assigned to the value set 3303</b><br> 3304 * Type: <b>composite</b><br> 3305 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3306 * </p> 3307 */ 3308 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3309 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3310 /** 3311 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3312 * <p> 3313 * Description: <b>Multiple Resources: 3314 3315* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3316* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3317* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3318* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3319* [Citation](citation.html): A use context type and value assigned to the citation 3320* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3321* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3322* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3323* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3324* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3325* [Evidence](evidence.html): A use context type and value assigned to the evidence 3326* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3327* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3328* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3329* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3330* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3331* [Library](library.html): A use context type and value assigned to the library 3332* [Measure](measure.html): A use context type and value assigned to the measure 3333* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3334* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3335* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3336* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3337* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3338* [Requirements](requirements.html): A use context type and value assigned to the requirements 3339* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3340* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3341* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3342* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3343* [TestScript](testscript.html): A use context type and value assigned to the test script 3344* [ValueSet](valueset.html): A use context type and value assigned to the value set 3345</b><br> 3346 * Type: <b>composite</b><br> 3347 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3348 * </p> 3349 */ 3350 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3351 3352 /** 3353 * Search parameter: <b>context-type</b> 3354 * <p> 3355 * Description: <b>Multiple Resources: 3356 3357* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3358* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3359* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3360* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3361* [Citation](citation.html): A type of use context assigned to the citation 3362* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3363* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3364* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3365* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3366* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3367* [Evidence](evidence.html): A type of use context assigned to the evidence 3368* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3369* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3370* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3371* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3372* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3373* [Library](library.html): A type of use context assigned to the library 3374* [Measure](measure.html): A type of use context assigned to the measure 3375* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3376* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3377* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3378* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3379* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3380* [Requirements](requirements.html): A type of use context assigned to the requirements 3381* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3382* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3383* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3384* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3385* [TestScript](testscript.html): A type of use context assigned to the test script 3386* [ValueSet](valueset.html): A type of use context assigned to the value set 3387</b><br> 3388 * Type: <b>token</b><br> 3389 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3390 * </p> 3391 */ 3392 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3393 public static final String SP_CONTEXT_TYPE = "context-type"; 3394 /** 3395 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3396 * <p> 3397 * Description: <b>Multiple Resources: 3398 3399* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3400* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3401* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3402* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3403* [Citation](citation.html): A type of use context assigned to the citation 3404* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3405* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3406* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3407* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3408* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3409* [Evidence](evidence.html): A type of use context assigned to the evidence 3410* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3411* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3412* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3413* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3414* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3415* [Library](library.html): A type of use context assigned to the library 3416* [Measure](measure.html): A type of use context assigned to the measure 3417* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3418* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3419* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3420* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3421* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3422* [Requirements](requirements.html): A type of use context assigned to the requirements 3423* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3424* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3425* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3426* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3427* [TestScript](testscript.html): A type of use context assigned to the test script 3428* [ValueSet](valueset.html): A type of use context assigned to the value set 3429</b><br> 3430 * Type: <b>token</b><br> 3431 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3432 * </p> 3433 */ 3434 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3435 3436 /** 3437 * Search parameter: <b>context</b> 3438 * <p> 3439 * Description: <b>Multiple Resources: 3440 3441* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3442* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3443* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3444* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3445* [Citation](citation.html): A use context assigned to the citation 3446* [CodeSystem](codesystem.html): A use context assigned to the code system 3447* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3448* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3449* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3450* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3451* [Evidence](evidence.html): A use context assigned to the evidence 3452* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3453* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3454* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3455* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3456* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3457* [Library](library.html): A use context assigned to the library 3458* [Measure](measure.html): A use context assigned to the measure 3459* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3460* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3461* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3462* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3463* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3464* [Requirements](requirements.html): A use context assigned to the requirements 3465* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3466* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3467* [StructureMap](structuremap.html): A use context assigned to the structure map 3468* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3469* [TestScript](testscript.html): A use context assigned to the test script 3470* [ValueSet](valueset.html): A use context assigned to the value set 3471</b><br> 3472 * Type: <b>token</b><br> 3473 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3474 * </p> 3475 */ 3476 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3477 public static final String SP_CONTEXT = "context"; 3478 /** 3479 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3480 * <p> 3481 * Description: <b>Multiple Resources: 3482 3483* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3484* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3485* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3486* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3487* [Citation](citation.html): A use context assigned to the citation 3488* [CodeSystem](codesystem.html): A use context assigned to the code system 3489* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3490* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3491* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3492* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3493* [Evidence](evidence.html): A use context assigned to the evidence 3494* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3495* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3496* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3497* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3498* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3499* [Library](library.html): A use context assigned to the library 3500* [Measure](measure.html): A use context assigned to the measure 3501* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3502* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3503* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3504* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3505* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3506* [Requirements](requirements.html): A use context assigned to the requirements 3507* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3508* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3509* [StructureMap](structuremap.html): A use context assigned to the structure map 3510* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3511* [TestScript](testscript.html): A use context assigned to the test script 3512* [ValueSet](valueset.html): A use context assigned to the value set 3513</b><br> 3514 * Type: <b>token</b><br> 3515 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3516 * </p> 3517 */ 3518 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3519 3520 /** 3521 * Search parameter: <b>date</b> 3522 * <p> 3523 * Description: <b>Multiple Resources: 3524 3525* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3526* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3527* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3528* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3529* [Citation](citation.html): The citation publication date 3530* [CodeSystem](codesystem.html): The code system publication date 3531* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3532* [ConceptMap](conceptmap.html): The concept map publication date 3533* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3534* [EventDefinition](eventdefinition.html): The event definition publication date 3535* [Evidence](evidence.html): The evidence publication date 3536* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3537* [ExampleScenario](examplescenario.html): The example scenario publication date 3538* [GraphDefinition](graphdefinition.html): The graph definition publication date 3539* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3540* [Library](library.html): The library publication date 3541* [Measure](measure.html): The measure publication date 3542* [MessageDefinition](messagedefinition.html): The message definition publication date 3543* [NamingSystem](namingsystem.html): The naming system publication date 3544* [OperationDefinition](operationdefinition.html): The operation definition publication date 3545* [PlanDefinition](plandefinition.html): The plan definition publication date 3546* [Questionnaire](questionnaire.html): The questionnaire publication date 3547* [Requirements](requirements.html): The requirements publication date 3548* [SearchParameter](searchparameter.html): The search parameter publication date 3549* [StructureDefinition](structuredefinition.html): The structure definition publication date 3550* [StructureMap](structuremap.html): The structure map publication date 3551* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3552* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3553* [TestScript](testscript.html): The test script publication date 3554* [ValueSet](valueset.html): The value set publication date 3555</b><br> 3556 * Type: <b>date</b><br> 3557 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3558 * </p> 3559 */ 3560 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3561 public static final String SP_DATE = "date"; 3562 /** 3563 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3564 * <p> 3565 * Description: <b>Multiple Resources: 3566 3567* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3568* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3569* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3570* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3571* [Citation](citation.html): The citation publication date 3572* [CodeSystem](codesystem.html): The code system publication date 3573* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3574* [ConceptMap](conceptmap.html): The concept map publication date 3575* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3576* [EventDefinition](eventdefinition.html): The event definition publication date 3577* [Evidence](evidence.html): The evidence publication date 3578* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3579* [ExampleScenario](examplescenario.html): The example scenario publication date 3580* [GraphDefinition](graphdefinition.html): The graph definition publication date 3581* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3582* [Library](library.html): The library publication date 3583* [Measure](measure.html): The measure publication date 3584* [MessageDefinition](messagedefinition.html): The message definition publication date 3585* [NamingSystem](namingsystem.html): The naming system publication date 3586* [OperationDefinition](operationdefinition.html): The operation definition publication date 3587* [PlanDefinition](plandefinition.html): The plan definition publication date 3588* [Questionnaire](questionnaire.html): The questionnaire publication date 3589* [Requirements](requirements.html): The requirements publication date 3590* [SearchParameter](searchparameter.html): The search parameter publication date 3591* [StructureDefinition](structuredefinition.html): The structure definition publication date 3592* [StructureMap](structuremap.html): The structure map publication date 3593* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3594* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3595* [TestScript](testscript.html): The test script publication date 3596* [ValueSet](valueset.html): The value set publication date 3597</b><br> 3598 * Type: <b>date</b><br> 3599 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3600 * </p> 3601 */ 3602 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3603 3604 /** 3605 * Search parameter: <b>description</b> 3606 * <p> 3607 * Description: <b>Multiple Resources: 3608 3609* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3610* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3611* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3612* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3613* [Citation](citation.html): The description of the citation 3614* [CodeSystem](codesystem.html): The description of the code system 3615* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3616* [ConceptMap](conceptmap.html): The description of the concept map 3617* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3618* [EventDefinition](eventdefinition.html): The description of the event definition 3619* [Evidence](evidence.html): The description of the evidence 3620* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3621* [GraphDefinition](graphdefinition.html): The description of the graph definition 3622* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3623* [Library](library.html): The description of the library 3624* [Measure](measure.html): The description of the measure 3625* [MessageDefinition](messagedefinition.html): The description of the message definition 3626* [NamingSystem](namingsystem.html): The description of the naming system 3627* [OperationDefinition](operationdefinition.html): The description of the operation definition 3628* [PlanDefinition](plandefinition.html): The description of the plan definition 3629* [Questionnaire](questionnaire.html): The description of the questionnaire 3630* [Requirements](requirements.html): The description of the requirements 3631* [SearchParameter](searchparameter.html): The description of the search parameter 3632* [StructureDefinition](structuredefinition.html): The description of the structure definition 3633* [StructureMap](structuremap.html): The description of the structure map 3634* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3635* [TestScript](testscript.html): The description of the test script 3636* [ValueSet](valueset.html): The description of the value set 3637</b><br> 3638 * Type: <b>string</b><br> 3639 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3640 * </p> 3641 */ 3642 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3643 public static final String SP_DESCRIPTION = "description"; 3644 /** 3645 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3646 * <p> 3647 * Description: <b>Multiple Resources: 3648 3649* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3650* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3651* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3652* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3653* [Citation](citation.html): The description of the citation 3654* [CodeSystem](codesystem.html): The description of the code system 3655* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3656* [ConceptMap](conceptmap.html): The description of the concept map 3657* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3658* [EventDefinition](eventdefinition.html): The description of the event definition 3659* [Evidence](evidence.html): The description of the evidence 3660* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3661* [GraphDefinition](graphdefinition.html): The description of the graph definition 3662* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3663* [Library](library.html): The description of the library 3664* [Measure](measure.html): The description of the measure 3665* [MessageDefinition](messagedefinition.html): The description of the message definition 3666* [NamingSystem](namingsystem.html): The description of the naming system 3667* [OperationDefinition](operationdefinition.html): The description of the operation definition 3668* [PlanDefinition](plandefinition.html): The description of the plan definition 3669* [Questionnaire](questionnaire.html): The description of the questionnaire 3670* [Requirements](requirements.html): The description of the requirements 3671* [SearchParameter](searchparameter.html): The description of the search parameter 3672* [StructureDefinition](structuredefinition.html): The description of the structure definition 3673* [StructureMap](structuremap.html): The description of the structure map 3674* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3675* [TestScript](testscript.html): The description of the test script 3676* [ValueSet](valueset.html): The description of the value set 3677</b><br> 3678 * Type: <b>string</b><br> 3679 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3680 * </p> 3681 */ 3682 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3683 3684 /** 3685 * Search parameter: <b>identifier</b> 3686 * <p> 3687 * Description: <b>Multiple Resources: 3688 3689* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3690* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3691* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3692* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3693* [Citation](citation.html): External identifier for the citation 3694* [CodeSystem](codesystem.html): External identifier for the code system 3695* [ConceptMap](conceptmap.html): External identifier for the concept map 3696* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3697* [EventDefinition](eventdefinition.html): External identifier for the event definition 3698* [Evidence](evidence.html): External identifier for the evidence 3699* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3700* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3701* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3702* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3703* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3704* [Library](library.html): External identifier for the library 3705* [Measure](measure.html): External identifier for the measure 3706* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3707* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3708* [NamingSystem](namingsystem.html): External identifier for the naming system 3709* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3710* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3711* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3712* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3713* [Requirements](requirements.html): External identifier for the requirements 3714* [SearchParameter](searchparameter.html): External identifier for the search parameter 3715* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3716* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3717* [StructureMap](structuremap.html): External identifier for the structure map 3718* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3719* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3720* [TestPlan](testplan.html): An identifier for the test plan 3721* [TestScript](testscript.html): External identifier for the test script 3722* [ValueSet](valueset.html): External identifier for the value set 3723</b><br> 3724 * Type: <b>token</b><br> 3725 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3726 * </p> 3727 */ 3728 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3729 public static final String SP_IDENTIFIER = "identifier"; 3730 /** 3731 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3732 * <p> 3733 * Description: <b>Multiple Resources: 3734 3735* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3736* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3737* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3738* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3739* [Citation](citation.html): External identifier for the citation 3740* [CodeSystem](codesystem.html): External identifier for the code system 3741* [ConceptMap](conceptmap.html): External identifier for the concept map 3742* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3743* [EventDefinition](eventdefinition.html): External identifier for the event definition 3744* [Evidence](evidence.html): External identifier for the evidence 3745* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3746* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3747* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3748* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3749* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3750* [Library](library.html): External identifier for the library 3751* [Measure](measure.html): External identifier for the measure 3752* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3753* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3754* [NamingSystem](namingsystem.html): External identifier for the naming system 3755* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3756* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3757* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3758* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3759* [Requirements](requirements.html): External identifier for the requirements 3760* [SearchParameter](searchparameter.html): External identifier for the search parameter 3761* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3762* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3763* [StructureMap](structuremap.html): External identifier for the structure map 3764* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3765* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3766* [TestPlan](testplan.html): An identifier for the test plan 3767* [TestScript](testscript.html): External identifier for the test script 3768* [ValueSet](valueset.html): External identifier for the value set 3769</b><br> 3770 * Type: <b>token</b><br> 3771 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3772 * </p> 3773 */ 3774 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3775 3776 /** 3777 * Search parameter: <b>jurisdiction</b> 3778 * <p> 3779 * Description: <b>Multiple Resources: 3780 3781* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3782* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3783* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3784* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3785* [Citation](citation.html): Intended jurisdiction for the citation 3786* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3787* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3788* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3789* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3790* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3791* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3792* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3793* [Library](library.html): Intended jurisdiction for the library 3794* [Measure](measure.html): Intended jurisdiction for the measure 3795* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3796* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3797* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3798* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3799* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3800* [Requirements](requirements.html): Intended jurisdiction for the requirements 3801* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3802* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3803* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3804* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3805* [TestScript](testscript.html): Intended jurisdiction for the test script 3806* [ValueSet](valueset.html): Intended jurisdiction for the value set 3807</b><br> 3808 * Type: <b>token</b><br> 3809 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3810 * </p> 3811 */ 3812 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3813 public static final String SP_JURISDICTION = "jurisdiction"; 3814 /** 3815 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3816 * <p> 3817 * Description: <b>Multiple Resources: 3818 3819* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3820* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3821* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3822* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3823* [Citation](citation.html): Intended jurisdiction for the citation 3824* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3825* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3826* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3827* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3828* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3829* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3830* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3831* [Library](library.html): Intended jurisdiction for the library 3832* [Measure](measure.html): Intended jurisdiction for the measure 3833* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3834* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3835* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3836* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3837* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3838* [Requirements](requirements.html): Intended jurisdiction for the requirements 3839* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3840* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3841* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3842* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3843* [TestScript](testscript.html): Intended jurisdiction for the test script 3844* [ValueSet](valueset.html): Intended jurisdiction for the value set 3845</b><br> 3846 * Type: <b>token</b><br> 3847 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3848 * </p> 3849 */ 3850 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3851 3852 /** 3853 * Search parameter: <b>name</b> 3854 * <p> 3855 * Description: <b>Multiple Resources: 3856 3857* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3858* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3859* [Citation](citation.html): Computationally friendly name of the citation 3860* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3861* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3862* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3863* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3864* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3865* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3866* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3867* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3868* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3869* [Library](library.html): Computationally friendly name of the library 3870* [Measure](measure.html): Computationally friendly name of the measure 3871* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3872* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3873* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3874* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3875* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3876* [Requirements](requirements.html): Computationally friendly name of the requirements 3877* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3878* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3879* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3880* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3881* [TestScript](testscript.html): Computationally friendly name of the test script 3882* [ValueSet](valueset.html): Computationally friendly name of the value set 3883</b><br> 3884 * Type: <b>string</b><br> 3885 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3886 * </p> 3887 */ 3888 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3889 public static final String SP_NAME = "name"; 3890 /** 3891 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3892 * <p> 3893 * Description: <b>Multiple Resources: 3894 3895* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3896* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3897* [Citation](citation.html): Computationally friendly name of the citation 3898* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3899* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3900* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3901* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3902* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3903* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3904* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3905* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3906* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3907* [Library](library.html): Computationally friendly name of the library 3908* [Measure](measure.html): Computationally friendly name of the measure 3909* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3910* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3911* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3912* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3913* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3914* [Requirements](requirements.html): Computationally friendly name of the requirements 3915* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3916* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3917* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3918* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3919* [TestScript](testscript.html): Computationally friendly name of the test script 3920* [ValueSet](valueset.html): Computationally friendly name of the value set 3921</b><br> 3922 * Type: <b>string</b><br> 3923 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3924 * </p> 3925 */ 3926 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3927 3928 /** 3929 * Search parameter: <b>publisher</b> 3930 * <p> 3931 * Description: <b>Multiple Resources: 3932 3933* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3934* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3935* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3936* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3937* [Citation](citation.html): Name of the publisher of the citation 3938* [CodeSystem](codesystem.html): Name of the publisher of the code system 3939* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3940* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3941* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3942* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3943* [Evidence](evidence.html): Name of the publisher of the evidence 3944* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3945* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3946* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3947* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3948* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3949* [Library](library.html): Name of the publisher of the library 3950* [Measure](measure.html): Name of the publisher of the measure 3951* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3952* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3953* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3954* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3955* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3956* [Requirements](requirements.html): Name of the publisher of the requirements 3957* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3958* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3959* [StructureMap](structuremap.html): Name of the publisher of the structure map 3960* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3961* [TestScript](testscript.html): Name of the publisher of the test script 3962* [ValueSet](valueset.html): Name of the publisher of the value set 3963</b><br> 3964 * Type: <b>string</b><br> 3965 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3966 * </p> 3967 */ 3968 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3969 public static final String SP_PUBLISHER = "publisher"; 3970 /** 3971 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3972 * <p> 3973 * Description: <b>Multiple Resources: 3974 3975* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3976* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3977* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3978* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3979* [Citation](citation.html): Name of the publisher of the citation 3980* [CodeSystem](codesystem.html): Name of the publisher of the code system 3981* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3982* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3983* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3984* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3985* [Evidence](evidence.html): Name of the publisher of the evidence 3986* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3987* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3988* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3989* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3990* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3991* [Library](library.html): Name of the publisher of the library 3992* [Measure](measure.html): Name of the publisher of the measure 3993* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3994* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3995* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3996* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3997* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3998* [Requirements](requirements.html): Name of the publisher of the requirements 3999* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4000* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4001* [StructureMap](structuremap.html): Name of the publisher of the structure map 4002* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4003* [TestScript](testscript.html): Name of the publisher of the test script 4004* [ValueSet](valueset.html): Name of the publisher of the value set 4005</b><br> 4006 * Type: <b>string</b><br> 4007 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4008 * </p> 4009 */ 4010 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4011 4012 /** 4013 * Search parameter: <b>status</b> 4014 * <p> 4015 * Description: <b>Multiple Resources: 4016 4017* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4018* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4019* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4020* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4021* [Citation](citation.html): The current status of the citation 4022* [CodeSystem](codesystem.html): The current status of the code system 4023* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4024* [ConceptMap](conceptmap.html): The current status of the concept map 4025* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4026* [EventDefinition](eventdefinition.html): The current status of the event definition 4027* [Evidence](evidence.html): The current status of the evidence 4028* [EvidenceReport](evidencereport.html): The current status of the evidence report 4029* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4030* [ExampleScenario](examplescenario.html): The current status of the example scenario 4031* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4032* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4033* [Library](library.html): The current status of the library 4034* [Measure](measure.html): The current status of the measure 4035* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4036* [MessageDefinition](messagedefinition.html): The current status of the message definition 4037* [NamingSystem](namingsystem.html): The current status of the naming system 4038* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4039* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4040* [PlanDefinition](plandefinition.html): The current status of the plan definition 4041* [Questionnaire](questionnaire.html): The current status of the questionnaire 4042* [Requirements](requirements.html): The current status of the requirements 4043* [SearchParameter](searchparameter.html): The current status of the search parameter 4044* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4045* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4046* [StructureMap](structuremap.html): The current status of the structure map 4047* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4048* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4049* [TestPlan](testplan.html): The current status of the test plan 4050* [TestScript](testscript.html): The current status of the test script 4051* [ValueSet](valueset.html): The current status of the value set 4052</b><br> 4053 * Type: <b>token</b><br> 4054 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4055 * </p> 4056 */ 4057 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4058 public static final String SP_STATUS = "status"; 4059 /** 4060 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4061 * <p> 4062 * Description: <b>Multiple Resources: 4063 4064* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4065* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4066* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4067* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4068* [Citation](citation.html): The current status of the citation 4069* [CodeSystem](codesystem.html): The current status of the code system 4070* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4071* [ConceptMap](conceptmap.html): The current status of the concept map 4072* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4073* [EventDefinition](eventdefinition.html): The current status of the event definition 4074* [Evidence](evidence.html): The current status of the evidence 4075* [EvidenceReport](evidencereport.html): The current status of the evidence report 4076* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4077* [ExampleScenario](examplescenario.html): The current status of the example scenario 4078* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4079* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4080* [Library](library.html): The current status of the library 4081* [Measure](measure.html): The current status of the measure 4082* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4083* [MessageDefinition](messagedefinition.html): The current status of the message definition 4084* [NamingSystem](namingsystem.html): The current status of the naming system 4085* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4086* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4087* [PlanDefinition](plandefinition.html): The current status of the plan definition 4088* [Questionnaire](questionnaire.html): The current status of the questionnaire 4089* [Requirements](requirements.html): The current status of the requirements 4090* [SearchParameter](searchparameter.html): The current status of the search parameter 4091* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4092* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4093* [StructureMap](structuremap.html): The current status of the structure map 4094* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4095* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4096* [TestPlan](testplan.html): The current status of the test plan 4097* [TestScript](testscript.html): The current status of the test script 4098* [ValueSet](valueset.html): The current status of the value set 4099</b><br> 4100 * Type: <b>token</b><br> 4101 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4102 * </p> 4103 */ 4104 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4105 4106 /** 4107 * Search parameter: <b>title</b> 4108 * <p> 4109 * Description: <b>Multiple Resources: 4110 4111* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4112* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4113* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4114* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4115* [Citation](citation.html): The human-friendly name of the citation 4116* [CodeSystem](codesystem.html): The human-friendly name of the code system 4117* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4118* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4119* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4120* [Evidence](evidence.html): The human-friendly name of the evidence 4121* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4122* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4123* [Library](library.html): The human-friendly name of the library 4124* [Measure](measure.html): The human-friendly name of the measure 4125* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4126* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4127* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4128* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4129* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4130* [Requirements](requirements.html): The human-friendly name of the requirements 4131* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4132* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4133* [StructureMap](structuremap.html): The human-friendly name of the structure map 4134* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4135* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4136* [TestScript](testscript.html): The human-friendly name of the test script 4137* [ValueSet](valueset.html): The human-friendly name of the value set 4138</b><br> 4139 * Type: <b>string</b><br> 4140 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4141 * </p> 4142 */ 4143 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4144 public static final String SP_TITLE = "title"; 4145 /** 4146 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4147 * <p> 4148 * Description: <b>Multiple Resources: 4149 4150* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4151* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4152* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4153* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4154* [Citation](citation.html): The human-friendly name of the citation 4155* [CodeSystem](codesystem.html): The human-friendly name of the code system 4156* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4157* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4158* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4159* [Evidence](evidence.html): The human-friendly name of the evidence 4160* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4161* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4162* [Library](library.html): The human-friendly name of the library 4163* [Measure](measure.html): The human-friendly name of the measure 4164* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4165* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4166* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4167* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4168* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4169* [Requirements](requirements.html): The human-friendly name of the requirements 4170* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4171* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4172* [StructureMap](structuremap.html): The human-friendly name of the structure map 4173* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4174* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4175* [TestScript](testscript.html): The human-friendly name of the test script 4176* [ValueSet](valueset.html): The human-friendly name of the value set 4177</b><br> 4178 * Type: <b>string</b><br> 4179 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4180 * </p> 4181 */ 4182 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4183 4184 /** 4185 * Search parameter: <b>url</b> 4186 * <p> 4187 * Description: <b>Multiple Resources: 4188 4189* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4190* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4191* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4192* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4193* [Citation](citation.html): The uri that identifies the citation 4194* [CodeSystem](codesystem.html): The uri that identifies the code system 4195* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4196* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4197* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4198* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4199* [Evidence](evidence.html): The uri that identifies the evidence 4200* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4201* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4202* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4203* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4204* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4205* [Library](library.html): The uri that identifies the library 4206* [Measure](measure.html): The uri that identifies the measure 4207* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4208* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4209* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4210* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4211* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4212* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4213* [Requirements](requirements.html): The uri that identifies the requirements 4214* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4215* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4216* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4217* [StructureMap](structuremap.html): The uri that identifies the structure map 4218* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4219* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4220* [TestPlan](testplan.html): The uri that identifies the test plan 4221* [TestScript](testscript.html): The uri that identifies the test script 4222* [ValueSet](valueset.html): The uri that identifies the value set 4223</b><br> 4224 * Type: <b>uri</b><br> 4225 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4226 * </p> 4227 */ 4228 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4229 public static final String SP_URL = "url"; 4230 /** 4231 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4232 * <p> 4233 * Description: <b>Multiple Resources: 4234 4235* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4236* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4237* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4238* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4239* [Citation](citation.html): The uri that identifies the citation 4240* [CodeSystem](codesystem.html): The uri that identifies the code system 4241* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4242* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4243* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4244* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4245* [Evidence](evidence.html): The uri that identifies the evidence 4246* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4247* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4248* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4249* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4250* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4251* [Library](library.html): The uri that identifies the library 4252* [Measure](measure.html): The uri that identifies the measure 4253* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4254* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4255* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4256* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4257* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4258* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4259* [Requirements](requirements.html): The uri that identifies the requirements 4260* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4261* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4262* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4263* [StructureMap](structuremap.html): The uri that identifies the structure map 4264* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4265* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4266* [TestPlan](testplan.html): The uri that identifies the test plan 4267* [TestScript](testscript.html): The uri that identifies the test script 4268* [ValueSet](valueset.html): The uri that identifies the value set 4269</b><br> 4270 * Type: <b>uri</b><br> 4271 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4272 * </p> 4273 */ 4274 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4275 4276 /** 4277 * Search parameter: <b>version</b> 4278 * <p> 4279 * Description: <b>Multiple Resources: 4280 4281* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4282* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4283* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4284* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4285* [Citation](citation.html): The business version of the citation 4286* [CodeSystem](codesystem.html): The business version of the code system 4287* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4288* [ConceptMap](conceptmap.html): The business version of the concept map 4289* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4290* [EventDefinition](eventdefinition.html): The business version of the event definition 4291* [Evidence](evidence.html): The business version of the evidence 4292* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4293* [ExampleScenario](examplescenario.html): The business version of the example scenario 4294* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4295* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4296* [Library](library.html): The business version of the library 4297* [Measure](measure.html): The business version of the measure 4298* [MessageDefinition](messagedefinition.html): The business version of the message definition 4299* [NamingSystem](namingsystem.html): The business version of the naming system 4300* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4301* [PlanDefinition](plandefinition.html): The business version of the plan definition 4302* [Questionnaire](questionnaire.html): The business version of the questionnaire 4303* [Requirements](requirements.html): The business version of the requirements 4304* [SearchParameter](searchparameter.html): The business version of the search parameter 4305* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4306* [StructureMap](structuremap.html): The business version of the structure map 4307* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4308* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4309* [TestScript](testscript.html): The business version of the test script 4310* [ValueSet](valueset.html): The business version of the value set 4311</b><br> 4312 * Type: <b>token</b><br> 4313 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4314 * </p> 4315 */ 4316 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4317 public static final String SP_VERSION = "version"; 4318 /** 4319 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4320 * <p> 4321 * Description: <b>Multiple Resources: 4322 4323* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4324* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4325* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4326* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4327* [Citation](citation.html): The business version of the citation 4328* [CodeSystem](codesystem.html): The business version of the code system 4329* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4330* [ConceptMap](conceptmap.html): The business version of the concept map 4331* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4332* [EventDefinition](eventdefinition.html): The business version of the event definition 4333* [Evidence](evidence.html): The business version of the evidence 4334* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4335* [ExampleScenario](examplescenario.html): The business version of the example scenario 4336* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4337* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4338* [Library](library.html): The business version of the library 4339* [Measure](measure.html): The business version of the measure 4340* [MessageDefinition](messagedefinition.html): The business version of the message definition 4341* [NamingSystem](namingsystem.html): The business version of the naming system 4342* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4343* [PlanDefinition](plandefinition.html): The business version of the plan definition 4344* [Questionnaire](questionnaire.html): The business version of the questionnaire 4345* [Requirements](requirements.html): The business version of the requirements 4346* [SearchParameter](searchparameter.html): The business version of the search parameter 4347* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4348* [StructureMap](structuremap.html): The business version of the structure map 4349* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4350* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4351* [TestScript](testscript.html): The business version of the test script 4352* [ValueSet](valueset.html): The business version of the value set 4353</b><br> 4354 * Type: <b>token</b><br> 4355 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4356 * </p> 4357 */ 4358 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4359 4360 /** 4361 * Search parameter: <b>category</b> 4362 * <p> 4363 * Description: <b>The behavior associated with the message</b><br> 4364 * Type: <b>token</b><br> 4365 * Path: <b>MessageDefinition.category</b><br> 4366 * </p> 4367 */ 4368 @SearchParamDefinition(name="category", path="MessageDefinition.category", description="The behavior associated with the message", type="token" ) 4369 public static final String SP_CATEGORY = "category"; 4370 /** 4371 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4372 * <p> 4373 * Description: <b>The behavior associated with the message</b><br> 4374 * Type: <b>token</b><br> 4375 * Path: <b>MessageDefinition.category</b><br> 4376 * </p> 4377 */ 4378 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4379 4380 /** 4381 * Search parameter: <b>event</b> 4382 * <p> 4383 * Description: <b>The event that triggers the message or link to the event definition.</b><br> 4384 * Type: <b>token</b><br> 4385 * Path: <b>MessageDefinition.event.ofType(Coding)</b><br> 4386 * </p> 4387 */ 4388 @SearchParamDefinition(name="event", path="MessageDefinition.event.ofType(Coding)", description="The event that triggers the message or link to the event definition.", type="token" ) 4389 public static final String SP_EVENT = "event"; 4390 /** 4391 * <b>Fluent Client</b> search parameter constant for <b>event</b> 4392 * <p> 4393 * Description: <b>The event that triggers the message or link to the event definition.</b><br> 4394 * Type: <b>token</b><br> 4395 * Path: <b>MessageDefinition.event.ofType(Coding)</b><br> 4396 * </p> 4397 */ 4398 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 4399 4400 /** 4401 * Search parameter: <b>focus</b> 4402 * <p> 4403 * Description: <b>A resource that is a permitted focus of the message</b><br> 4404 * Type: <b>token</b><br> 4405 * Path: <b>MessageDefinition.focus.code</b><br> 4406 * </p> 4407 */ 4408 @SearchParamDefinition(name="focus", path="MessageDefinition.focus.code", description="A resource that is a permitted focus of the message", type="token" ) 4409 public static final String SP_FOCUS = "focus"; 4410 /** 4411 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 4412 * <p> 4413 * Description: <b>A resource that is a permitted focus of the message</b><br> 4414 * Type: <b>token</b><br> 4415 * Path: <b>MessageDefinition.focus.code</b><br> 4416 * </p> 4417 */ 4418 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FOCUS); 4419 4420 /** 4421 * Search parameter: <b>parent</b> 4422 * <p> 4423 * Description: <b>A resource that is the parent of the definition</b><br> 4424 * Type: <b>reference</b><br> 4425 * Path: <b>MessageDefinition.parent</b><br> 4426 * </p> 4427 */ 4428 @SearchParamDefinition(name="parent", path="MessageDefinition.parent", description="A resource that is the parent of the definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 4429 public static final String SP_PARENT = "parent"; 4430 /** 4431 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 4432 * <p> 4433 * Description: <b>A resource that is the parent of the definition</b><br> 4434 * Type: <b>reference</b><br> 4435 * Path: <b>MessageDefinition.parent</b><br> 4436 * </p> 4437 */ 4438 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 4439 4440/** 4441 * Constant for fluent queries to be used to add include statements. Specifies 4442 * the path value of "<b>MessageDefinition:parent</b>". 4443 */ 4444 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("MessageDefinition:parent").toLocked(); 4445 4446 4447} 4448