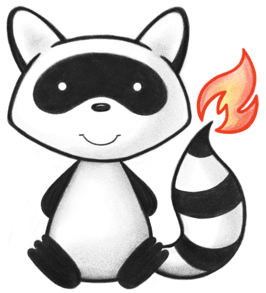
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 052 */ 053@ResourceDef(name="MessageDefinition", profile="http://hl7.org/fhir/StructureDefinition/MessageDefinition") 054public class MessageDefinition extends CanonicalResource { 055 056 public enum MessageSignificanceCategory { 057 /** 058 * The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment. 059 */ 060 CONSEQUENCE, 061 /** 062 * The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful. 063 */ 064 CURRENCY, 065 /** 066 * The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications. 067 */ 068 NOTIFICATION, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("consequence".equals(codeString)) 077 return CONSEQUENCE; 078 if ("currency".equals(codeString)) 079 return CURRENCY; 080 if ("notification".equals(codeString)) 081 return NOTIFICATION; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case CONSEQUENCE: return "consequence"; 090 case CURRENCY: return "currency"; 091 case NOTIFICATION: return "notification"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case CONSEQUENCE: return "http://hl7.org/fhir/message-significance-category"; 099 case CURRENCY: return "http://hl7.org/fhir/message-significance-category"; 100 case NOTIFICATION: return "http://hl7.org/fhir/message-significance-category"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case CONSEQUENCE: return "The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment."; 108 case CURRENCY: return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 109 case NOTIFICATION: return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case CONSEQUENCE: return "Consequence"; 117 case CURRENCY: return "Currency"; 118 case NOTIFICATION: return "Notification"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 126 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("consequence".equals(codeString)) 131 return MessageSignificanceCategory.CONSEQUENCE; 132 if ("currency".equals(codeString)) 133 return MessageSignificanceCategory.CURRENCY; 134 if ("notification".equals(codeString)) 135 return MessageSignificanceCategory.NOTIFICATION; 136 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 137 } 138 public Enumeration<MessageSignificanceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 146 if ("consequence".equals(codeString)) 147 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE, code); 148 if ("currency".equals(codeString)) 149 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY, code); 150 if ("notification".equals(codeString)) 151 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION, code); 152 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 153 } 154 public String toCode(MessageSignificanceCategory code) { 155 if (code == MessageSignificanceCategory.CONSEQUENCE) 156 return "consequence"; 157 if (code == MessageSignificanceCategory.CURRENCY) 158 return "currency"; 159 if (code == MessageSignificanceCategory.NOTIFICATION) 160 return "notification"; 161 return "?"; 162 } 163 public String toSystem(MessageSignificanceCategory code) { 164 return code.getSystem(); 165 } 166 } 167 168 public enum MessageheaderResponseRequest { 169 /** 170 * initiator expects a response for this message. 171 */ 172 ALWAYS, 173 /** 174 * initiator expects a response only if in error. 175 */ 176 ONERROR, 177 /** 178 * initiator does not expect a response. 179 */ 180 NEVER, 181 /** 182 * initiator expects a response only if successful. 183 */ 184 ONSUCCESS, 185 /** 186 * added to help the parsers with the generic types 187 */ 188 NULL; 189 public static MessageheaderResponseRequest fromCode(String codeString) throws FHIRException { 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("always".equals(codeString)) 193 return ALWAYS; 194 if ("on-error".equals(codeString)) 195 return ONERROR; 196 if ("never".equals(codeString)) 197 return NEVER; 198 if ("on-success".equals(codeString)) 199 return ONSUCCESS; 200 if (Configuration.isAcceptInvalidEnums()) 201 return null; 202 else 203 throw new FHIRException("Unknown MessageheaderResponseRequest code '"+codeString+"'"); 204 } 205 public String toCode() { 206 switch (this) { 207 case ALWAYS: return "always"; 208 case ONERROR: return "on-error"; 209 case NEVER: return "never"; 210 case ONSUCCESS: return "on-success"; 211 case NULL: return null; 212 default: return "?"; 213 } 214 } 215 public String getSystem() { 216 switch (this) { 217 case ALWAYS: return "http://hl7.org/fhir/messageheader-response-request"; 218 case ONERROR: return "http://hl7.org/fhir/messageheader-response-request"; 219 case NEVER: return "http://hl7.org/fhir/messageheader-response-request"; 220 case ONSUCCESS: return "http://hl7.org/fhir/messageheader-response-request"; 221 case NULL: return null; 222 default: return "?"; 223 } 224 } 225 public String getDefinition() { 226 switch (this) { 227 case ALWAYS: return "initiator expects a response for this message."; 228 case ONERROR: return "initiator expects a response only if in error."; 229 case NEVER: return "initiator does not expect a response."; 230 case ONSUCCESS: return "initiator expects a response only if successful."; 231 case NULL: return null; 232 default: return "?"; 233 } 234 } 235 public String getDisplay() { 236 switch (this) { 237 case ALWAYS: return "Always"; 238 case ONERROR: return "Error/reject conditions only"; 239 case NEVER: return "Never"; 240 case ONSUCCESS: return "Successful completion only"; 241 case NULL: return null; 242 default: return "?"; 243 } 244 } 245 } 246 247 public static class MessageheaderResponseRequestEnumFactory implements EnumFactory<MessageheaderResponseRequest> { 248 public MessageheaderResponseRequest fromCode(String codeString) throws IllegalArgumentException { 249 if (codeString == null || "".equals(codeString)) 250 if (codeString == null || "".equals(codeString)) 251 return null; 252 if ("always".equals(codeString)) 253 return MessageheaderResponseRequest.ALWAYS; 254 if ("on-error".equals(codeString)) 255 return MessageheaderResponseRequest.ONERROR; 256 if ("never".equals(codeString)) 257 return MessageheaderResponseRequest.NEVER; 258 if ("on-success".equals(codeString)) 259 return MessageheaderResponseRequest.ONSUCCESS; 260 throw new IllegalArgumentException("Unknown MessageheaderResponseRequest code '"+codeString+"'"); 261 } 262 public Enumeration<MessageheaderResponseRequest> fromType(PrimitiveType<?> code) throws FHIRException { 263 if (code == null) 264 return null; 265 if (code.isEmpty()) 266 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 267 String codeString = ((PrimitiveType) code).asStringValue(); 268 if (codeString == null || "".equals(codeString)) 269 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 270 if ("always".equals(codeString)) 271 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ALWAYS, code); 272 if ("on-error".equals(codeString)) 273 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONERROR, code); 274 if ("never".equals(codeString)) 275 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NEVER, code); 276 if ("on-success".equals(codeString)) 277 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONSUCCESS, code); 278 throw new FHIRException("Unknown MessageheaderResponseRequest code '"+codeString+"'"); 279 } 280 public String toCode(MessageheaderResponseRequest code) { 281 if (code == MessageheaderResponseRequest.ALWAYS) 282 return "always"; 283 if (code == MessageheaderResponseRequest.ONERROR) 284 return "on-error"; 285 if (code == MessageheaderResponseRequest.NEVER) 286 return "never"; 287 if (code == MessageheaderResponseRequest.ONSUCCESS) 288 return "on-success"; 289 return "?"; 290 } 291 public String toSystem(MessageheaderResponseRequest code) { 292 return code.getSystem(); 293 } 294 } 295 296 @Block() 297 public static class MessageDefinitionFocusComponent extends BackboneElement implements IBaseBackboneElement { 298 /** 299 * The kind of resource that must be the focus for this message. 300 */ 301 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 302 @Description(shortDefinition="Type of resource", formalDefinition="The kind of resource that must be the focus for this message." ) 303 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 304 protected CodeType code; 305 306 /** 307 * A profile that reflects constraints for the focal resource (and potentially for related resources). 308 */ 309 @Child(name = "profile", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=false) 310 @Description(shortDefinition="Profile that must be adhered to by focus", formalDefinition="A profile that reflects constraints for the focal resource (and potentially for related resources)." ) 311 protected CanonicalType profile; 312 313 /** 314 * Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 315 */ 316 @Child(name = "min", type = {UnsignedIntType.class}, order=3, min=1, max=1, modifier=false, summary=true) 317 @Description(shortDefinition="Minimum number of focuses of this type", formalDefinition="Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition." ) 318 protected UnsignedIntType min; 319 320 /** 321 * Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 322 */ 323 @Child(name = "max", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 324 @Description(shortDefinition="Maximum number of focuses of this type", formalDefinition="Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition." ) 325 protected StringType max; 326 327 private static final long serialVersionUID = -68504836L; 328 329 /** 330 * Constructor 331 */ 332 public MessageDefinitionFocusComponent() { 333 super(); 334 } 335 336 /** 337 * Constructor 338 */ 339 public MessageDefinitionFocusComponent(String code, int min) { 340 super(); 341 this.setCode(code); 342 this.setMin(min); 343 } 344 345 /** 346 * @return {@link #code} (The kind of resource that must be the focus for this message.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 347 */ 348 public CodeType getCodeElement() { 349 if (this.code == null) 350 if (Configuration.errorOnAutoCreate()) 351 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.code"); 352 else if (Configuration.doAutoCreate()) 353 this.code = new CodeType(); // bb 354 return this.code; 355 } 356 357 public boolean hasCodeElement() { 358 return this.code != null && !this.code.isEmpty(); 359 } 360 361 public boolean hasCode() { 362 return this.code != null && !this.code.isEmpty(); 363 } 364 365 /** 366 * @param value {@link #code} (The kind of resource that must be the focus for this message.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 367 */ 368 public MessageDefinitionFocusComponent setCodeElement(CodeType value) { 369 this.code = value; 370 return this; 371 } 372 373 /** 374 * @return The kind of resource that must be the focus for this message. 375 */ 376 public String getCode() { 377 return this.code == null ? null : this.code.getValue(); 378 } 379 380 /** 381 * @param value The kind of resource that must be the focus for this message. 382 */ 383 public MessageDefinitionFocusComponent setCode(String value) { 384 if (this.code == null) 385 this.code = new CodeType(); 386 this.code.setValue(value); 387 return this; 388 } 389 390 /** 391 * @return {@link #profile} (A profile that reflects constraints for the focal resource (and potentially for related resources).). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 392 */ 393 public CanonicalType getProfileElement() { 394 if (this.profile == null) 395 if (Configuration.errorOnAutoCreate()) 396 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.profile"); 397 else if (Configuration.doAutoCreate()) 398 this.profile = new CanonicalType(); // bb 399 return this.profile; 400 } 401 402 public boolean hasProfileElement() { 403 return this.profile != null && !this.profile.isEmpty(); 404 } 405 406 public boolean hasProfile() { 407 return this.profile != null && !this.profile.isEmpty(); 408 } 409 410 /** 411 * @param value {@link #profile} (A profile that reflects constraints for the focal resource (and potentially for related resources).). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 412 */ 413 public MessageDefinitionFocusComponent setProfileElement(CanonicalType value) { 414 this.profile = value; 415 return this; 416 } 417 418 /** 419 * @return A profile that reflects constraints for the focal resource (and potentially for related resources). 420 */ 421 public String getProfile() { 422 return this.profile == null ? null : this.profile.getValue(); 423 } 424 425 /** 426 * @param value A profile that reflects constraints for the focal resource (and potentially for related resources). 427 */ 428 public MessageDefinitionFocusComponent setProfile(String value) { 429 if (Utilities.noString(value)) 430 this.profile = null; 431 else { 432 if (this.profile == null) 433 this.profile = new CanonicalType(); 434 this.profile.setValue(value); 435 } 436 return this; 437 } 438 439 /** 440 * @return {@link #min} (Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 441 */ 442 public UnsignedIntType getMinElement() { 443 if (this.min == null) 444 if (Configuration.errorOnAutoCreate()) 445 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.min"); 446 else if (Configuration.doAutoCreate()) 447 this.min = new UnsignedIntType(); // bb 448 return this.min; 449 } 450 451 public boolean hasMinElement() { 452 return this.min != null && !this.min.isEmpty(); 453 } 454 455 public boolean hasMin() { 456 return this.min != null && !this.min.isEmpty(); 457 } 458 459 /** 460 * @param value {@link #min} (Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 461 */ 462 public MessageDefinitionFocusComponent setMinElement(UnsignedIntType value) { 463 this.min = value; 464 return this; 465 } 466 467 /** 468 * @return Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 469 */ 470 public int getMin() { 471 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 472 } 473 474 /** 475 * @param value Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 476 */ 477 public MessageDefinitionFocusComponent setMin(int value) { 478 if (this.min == null) 479 this.min = new UnsignedIntType(); 480 this.min.setValue(value); 481 return this; 482 } 483 484 /** 485 * @return {@link #max} (Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 486 */ 487 public StringType getMaxElement() { 488 if (this.max == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.max"); 491 else if (Configuration.doAutoCreate()) 492 this.max = new StringType(); // bb 493 return this.max; 494 } 495 496 public boolean hasMaxElement() { 497 return this.max != null && !this.max.isEmpty(); 498 } 499 500 public boolean hasMax() { 501 return this.max != null && !this.max.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #max} (Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 506 */ 507 public MessageDefinitionFocusComponent setMaxElement(StringType value) { 508 this.max = value; 509 return this; 510 } 511 512 /** 513 * @return Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 514 */ 515 public String getMax() { 516 return this.max == null ? null : this.max.getValue(); 517 } 518 519 /** 520 * @param value Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 521 */ 522 public MessageDefinitionFocusComponent setMax(String value) { 523 if (Utilities.noString(value)) 524 this.max = null; 525 else { 526 if (this.max == null) 527 this.max = new StringType(); 528 this.max.setValue(value); 529 } 530 return this; 531 } 532 533 protected void listChildren(List<Property> children) { 534 super.listChildren(children); 535 children.add(new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code)); 536 children.add(new Property("profile", "canonical(StructureDefinition)", "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, profile)); 537 children.add(new Property("min", "unsignedInt", "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, min)); 538 children.add(new Property("max", "string", "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, max)); 539 } 540 541 @Override 542 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 543 switch (_hash) { 544 case 3059181: /*code*/ return new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code); 545 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, profile); 546 case 108114: /*min*/ return new Property("min", "unsignedInt", "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, min); 547 case 107876: /*max*/ return new Property("max", "string", "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, max); 548 default: return super.getNamedProperty(_hash, _name, _checkValid); 549 } 550 551 } 552 553 @Override 554 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 555 switch (hash) { 556 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 557 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 558 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 559 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 560 default: return super.getProperty(hash, name, checkValid); 561 } 562 563 } 564 565 @Override 566 public Base setProperty(int hash, String name, Base value) throws FHIRException { 567 switch (hash) { 568 case 3059181: // code 569 this.code = TypeConvertor.castToCode(value); // CodeType 570 return value; 571 case -309425751: // profile 572 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 573 return value; 574 case 108114: // min 575 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 576 return value; 577 case 107876: // max 578 this.max = TypeConvertor.castToString(value); // StringType 579 return value; 580 default: return super.setProperty(hash, name, value); 581 } 582 583 } 584 585 @Override 586 public Base setProperty(String name, Base value) throws FHIRException { 587 if (name.equals("code")) { 588 this.code = TypeConvertor.castToCode(value); // CodeType 589 } else if (name.equals("profile")) { 590 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 591 } else if (name.equals("min")) { 592 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 593 } else if (name.equals("max")) { 594 this.max = TypeConvertor.castToString(value); // StringType 595 } else 596 return super.setProperty(name, value); 597 return value; 598 } 599 600 @Override 601 public Base makeProperty(int hash, String name) throws FHIRException { 602 switch (hash) { 603 case 3059181: return getCodeElement(); 604 case -309425751: return getProfileElement(); 605 case 108114: return getMinElement(); 606 case 107876: return getMaxElement(); 607 default: return super.makeProperty(hash, name); 608 } 609 610 } 611 612 @Override 613 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 614 switch (hash) { 615 case 3059181: /*code*/ return new String[] {"code"}; 616 case -309425751: /*profile*/ return new String[] {"canonical"}; 617 case 108114: /*min*/ return new String[] {"unsignedInt"}; 618 case 107876: /*max*/ return new String[] {"string"}; 619 default: return super.getTypesForProperty(hash, name); 620 } 621 622 } 623 624 @Override 625 public Base addChild(String name) throws FHIRException { 626 if (name.equals("code")) { 627 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.code"); 628 } 629 else if (name.equals("profile")) { 630 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.profile"); 631 } 632 else if (name.equals("min")) { 633 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.min"); 634 } 635 else if (name.equals("max")) { 636 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.focus.max"); 637 } 638 else 639 return super.addChild(name); 640 } 641 642 public MessageDefinitionFocusComponent copy() { 643 MessageDefinitionFocusComponent dst = new MessageDefinitionFocusComponent(); 644 copyValues(dst); 645 return dst; 646 } 647 648 public void copyValues(MessageDefinitionFocusComponent dst) { 649 super.copyValues(dst); 650 dst.code = code == null ? null : code.copy(); 651 dst.profile = profile == null ? null : profile.copy(); 652 dst.min = min == null ? null : min.copy(); 653 dst.max = max == null ? null : max.copy(); 654 } 655 656 @Override 657 public boolean equalsDeep(Base other_) { 658 if (!super.equalsDeep(other_)) 659 return false; 660 if (!(other_ instanceof MessageDefinitionFocusComponent)) 661 return false; 662 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 663 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(min, o.min, true) 664 && compareDeep(max, o.max, true); 665 } 666 667 @Override 668 public boolean equalsShallow(Base other_) { 669 if (!super.equalsShallow(other_)) 670 return false; 671 if (!(other_ instanceof MessageDefinitionFocusComponent)) 672 return false; 673 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 674 return compareValues(code, o.code, true) && compareValues(profile, o.profile, true) && compareValues(min, o.min, true) 675 && compareValues(max, o.max, true); 676 } 677 678 public boolean isEmpty() { 679 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, min, max 680 ); 681 } 682 683 public String fhirType() { 684 return "MessageDefinition.focus"; 685 686 } 687 688 } 689 690 @Block() 691 public static class MessageDefinitionAllowedResponseComponent extends BackboneElement implements IBaseBackboneElement { 692 /** 693 * A reference to the message definition that must be adhered to by this supported response. 694 */ 695 @Child(name = "message", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 696 @Description(shortDefinition="Reference to allowed message definition response", formalDefinition="A reference to the message definition that must be adhered to by this supported response." ) 697 protected CanonicalType message; 698 699 /** 700 * Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 701 */ 702 @Child(name = "situation", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 703 @Description(shortDefinition="When should this response be used", formalDefinition="Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses)." ) 704 protected MarkdownType situation; 705 706 private static final long serialVersionUID = -1943810550L; 707 708 /** 709 * Constructor 710 */ 711 public MessageDefinitionAllowedResponseComponent() { 712 super(); 713 } 714 715 /** 716 * Constructor 717 */ 718 public MessageDefinitionAllowedResponseComponent(String message) { 719 super(); 720 this.setMessage(message); 721 } 722 723 /** 724 * @return {@link #message} (A reference to the message definition that must be adhered to by this supported response.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 725 */ 726 public CanonicalType getMessageElement() { 727 if (this.message == null) 728 if (Configuration.errorOnAutoCreate()) 729 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.message"); 730 else if (Configuration.doAutoCreate()) 731 this.message = new CanonicalType(); // bb 732 return this.message; 733 } 734 735 public boolean hasMessageElement() { 736 return this.message != null && !this.message.isEmpty(); 737 } 738 739 public boolean hasMessage() { 740 return this.message != null && !this.message.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #message} (A reference to the message definition that must be adhered to by this supported response.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 745 */ 746 public MessageDefinitionAllowedResponseComponent setMessageElement(CanonicalType value) { 747 this.message = value; 748 return this; 749 } 750 751 /** 752 * @return A reference to the message definition that must be adhered to by this supported response. 753 */ 754 public String getMessage() { 755 return this.message == null ? null : this.message.getValue(); 756 } 757 758 /** 759 * @param value A reference to the message definition that must be adhered to by this supported response. 760 */ 761 public MessageDefinitionAllowedResponseComponent setMessage(String value) { 762 if (this.message == null) 763 this.message = new CanonicalType(); 764 this.message.setValue(value); 765 return this; 766 } 767 768 /** 769 * @return {@link #situation} (Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).). This is the underlying object with id, value and extensions. The accessor "getSituation" gives direct access to the value 770 */ 771 public MarkdownType getSituationElement() { 772 if (this.situation == null) 773 if (Configuration.errorOnAutoCreate()) 774 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.situation"); 775 else if (Configuration.doAutoCreate()) 776 this.situation = new MarkdownType(); // bb 777 return this.situation; 778 } 779 780 public boolean hasSituationElement() { 781 return this.situation != null && !this.situation.isEmpty(); 782 } 783 784 public boolean hasSituation() { 785 return this.situation != null && !this.situation.isEmpty(); 786 } 787 788 /** 789 * @param value {@link #situation} (Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).). This is the underlying object with id, value and extensions. The accessor "getSituation" gives direct access to the value 790 */ 791 public MessageDefinitionAllowedResponseComponent setSituationElement(MarkdownType value) { 792 this.situation = value; 793 return this; 794 } 795 796 /** 797 * @return Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 798 */ 799 public String getSituation() { 800 return this.situation == null ? null : this.situation.getValue(); 801 } 802 803 /** 804 * @param value Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 805 */ 806 public MessageDefinitionAllowedResponseComponent setSituation(String value) { 807 if (Utilities.noString(value)) 808 this.situation = null; 809 else { 810 if (this.situation == null) 811 this.situation = new MarkdownType(); 812 this.situation.setValue(value); 813 } 814 return this; 815 } 816 817 protected void listChildren(List<Property> children) { 818 super.listChildren(children); 819 children.add(new Property("message", "canonical(MessageDefinition)", "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message)); 820 children.add(new Property("situation", "markdown", "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 0, 1, situation)); 821 } 822 823 @Override 824 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 825 switch (_hash) { 826 case 954925063: /*message*/ return new Property("message", "canonical(MessageDefinition)", "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message); 827 case -73377282: /*situation*/ return new Property("situation", "markdown", "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 0, 1, situation); 828 default: return super.getNamedProperty(_hash, _name, _checkValid); 829 } 830 831 } 832 833 @Override 834 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 835 switch (hash) { 836 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // CanonicalType 837 case -73377282: /*situation*/ return this.situation == null ? new Base[0] : new Base[] {this.situation}; // MarkdownType 838 default: return super.getProperty(hash, name, checkValid); 839 } 840 841 } 842 843 @Override 844 public Base setProperty(int hash, String name, Base value) throws FHIRException { 845 switch (hash) { 846 case 954925063: // message 847 this.message = TypeConvertor.castToCanonical(value); // CanonicalType 848 return value; 849 case -73377282: // situation 850 this.situation = TypeConvertor.castToMarkdown(value); // MarkdownType 851 return value; 852 default: return super.setProperty(hash, name, value); 853 } 854 855 } 856 857 @Override 858 public Base setProperty(String name, Base value) throws FHIRException { 859 if (name.equals("message")) { 860 this.message = TypeConvertor.castToCanonical(value); // CanonicalType 861 } else if (name.equals("situation")) { 862 this.situation = TypeConvertor.castToMarkdown(value); // MarkdownType 863 } else 864 return super.setProperty(name, value); 865 return value; 866 } 867 868 @Override 869 public Base makeProperty(int hash, String name) throws FHIRException { 870 switch (hash) { 871 case 954925063: return getMessageElement(); 872 case -73377282: return getSituationElement(); 873 default: return super.makeProperty(hash, name); 874 } 875 876 } 877 878 @Override 879 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 880 switch (hash) { 881 case 954925063: /*message*/ return new String[] {"canonical"}; 882 case -73377282: /*situation*/ return new String[] {"markdown"}; 883 default: return super.getTypesForProperty(hash, name); 884 } 885 886 } 887 888 @Override 889 public Base addChild(String name) throws FHIRException { 890 if (name.equals("message")) { 891 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.allowedResponse.message"); 892 } 893 else if (name.equals("situation")) { 894 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.allowedResponse.situation"); 895 } 896 else 897 return super.addChild(name); 898 } 899 900 public MessageDefinitionAllowedResponseComponent copy() { 901 MessageDefinitionAllowedResponseComponent dst = new MessageDefinitionAllowedResponseComponent(); 902 copyValues(dst); 903 return dst; 904 } 905 906 public void copyValues(MessageDefinitionAllowedResponseComponent dst) { 907 super.copyValues(dst); 908 dst.message = message == null ? null : message.copy(); 909 dst.situation = situation == null ? null : situation.copy(); 910 } 911 912 @Override 913 public boolean equalsDeep(Base other_) { 914 if (!super.equalsDeep(other_)) 915 return false; 916 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 917 return false; 918 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 919 return compareDeep(message, o.message, true) && compareDeep(situation, o.situation, true); 920 } 921 922 @Override 923 public boolean equalsShallow(Base other_) { 924 if (!super.equalsShallow(other_)) 925 return false; 926 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 927 return false; 928 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 929 return compareValues(message, o.message, true) && compareValues(situation, o.situation, true); 930 } 931 932 public boolean isEmpty() { 933 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(message, situation); 934 } 935 936 public String fhirType() { 937 return "MessageDefinition.allowedResponse"; 938 939 } 940 941 } 942 943 /** 944 * The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server. 945 */ 946 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 947 @Description(shortDefinition="The cannonical URL for a given MessageDefinition", formalDefinition="The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server." ) 948 protected UriType url; 949 950 /** 951 * A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 952 */ 953 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 954 @Description(shortDefinition="Business Identifier for a given MessageDefinition", formalDefinition="A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 955 protected List<Identifier> identifier; 956 957 /** 958 * The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 959 */ 960 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 961 @Description(shortDefinition="Business version of the message definition", formalDefinition="The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 962 protected StringType version; 963 964 /** 965 * Indicates the mechanism used to compare versions to determine which is more current. 966 */ 967 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 968 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 969 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 970 protected DataType versionAlgorithm; 971 972 /** 973 * A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 974 */ 975 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 976 @Description(shortDefinition="Name for this message definition (computer friendly)", formalDefinition="A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 977 protected StringType name; 978 979 /** 980 * A short, descriptive, user-friendly title for the message definition. 981 */ 982 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 983 @Description(shortDefinition="Name for this message definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the message definition." ) 984 protected StringType title; 985 986 /** 987 * A MessageDefinition that is superseded by this definition. 988 */ 989 @Child(name = "replaces", type = {CanonicalType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 990 @Description(shortDefinition="Takes the place of", formalDefinition="A MessageDefinition that is superseded by this definition." ) 991 protected List<CanonicalType> replaces; 992 993 /** 994 * The status of this message definition. Enables tracking the life-cycle of the content. 995 */ 996 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 997 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this message definition. Enables tracking the life-cycle of the content." ) 998 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 999 protected Enumeration<PublicationStatus> status; 1000 1001 /** 1002 * A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1003 */ 1004 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1005 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1006 protected BooleanType experimental; 1007 1008 /** 1009 * The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1010 */ 1011 @Child(name = "date", type = {DateTimeType.class}, order=9, min=1, max=1, modifier=false, summary=true) 1012 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes." ) 1013 protected DateTimeType date; 1014 1015 /** 1016 * The name of the organization or individual responsible for the release and ongoing maintenance of the message definition. 1017 */ 1018 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1019 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the message definition." ) 1020 protected StringType publisher; 1021 1022 /** 1023 * Contact details to assist a user in finding and communicating with the publisher. 1024 */ 1025 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1026 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1027 protected List<ContactDetail> contact; 1028 1029 /** 1030 * A free text natural language description of the message definition from a consumer's perspective. 1031 */ 1032 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1033 @Description(shortDefinition="Natural language description of the message definition", formalDefinition="A free text natural language description of the message definition from a consumer's perspective." ) 1034 protected MarkdownType description; 1035 1036 /** 1037 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances. 1038 */ 1039 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1040 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances." ) 1041 protected List<UsageContext> useContext; 1042 1043 /** 1044 * A legal or geographic region in which the message definition is intended to be used. 1045 */ 1046 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1047 @Description(shortDefinition="Intended jurisdiction for message definition (if applicable)", formalDefinition="A legal or geographic region in which the message definition is intended to be used." ) 1048 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1049 protected List<CodeableConcept> jurisdiction; 1050 1051 /** 1052 * Explanation of why this message definition is needed and why it has been designed as it has. 1053 */ 1054 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=true) 1055 @Description(shortDefinition="Why this message definition is defined", formalDefinition="Explanation of why this message definition is needed and why it has been designed as it has." ) 1056 protected MarkdownType purpose; 1057 1058 /** 1059 * A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 1060 */ 1061 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1062 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition." ) 1063 protected MarkdownType copyright; 1064 1065 /** 1066 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1067 */ 1068 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1069 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1070 protected StringType copyrightLabel; 1071 1072 /** 1073 * The MessageDefinition that is the basis for the contents of this resource. 1074 */ 1075 @Child(name = "base", type = {CanonicalType.class}, order=18, min=0, max=1, modifier=false, summary=true) 1076 @Description(shortDefinition="Definition this one is based on", formalDefinition="The MessageDefinition that is the basis for the contents of this resource." ) 1077 protected CanonicalType base; 1078 1079 /** 1080 * Identifies a protocol or workflow that this MessageDefinition represents a step in. 1081 */ 1082 @Child(name = "parent", type = {CanonicalType.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1083 @Description(shortDefinition="Protocol/workflow this is part of", formalDefinition="Identifies a protocol or workflow that this MessageDefinition represents a step in." ) 1084 protected List<CanonicalType> parent; 1085 1086 /** 1087 * Event code or link to the EventDefinition. 1088 */ 1089 @Child(name = "event", type = {Coding.class, UriType.class}, order=20, min=1, max=1, modifier=false, summary=true) 1090 @Description(shortDefinition="Event code or link to the EventDefinition", formalDefinition="Event code or link to the EventDefinition." ) 1091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-events") 1092 protected DataType event; 1093 1094 /** 1095 * The impact of the content of the message. 1096 */ 1097 @Child(name = "category", type = {CodeType.class}, order=21, min=0, max=1, modifier=false, summary=true) 1098 @Description(shortDefinition="consequence | currency | notification", formalDefinition="The impact of the content of the message." ) 1099 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-significance-category") 1100 protected Enumeration<MessageSignificanceCategory> category; 1101 1102 /** 1103 * Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge. 1104 */ 1105 @Child(name = "focus", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1106 @Description(shortDefinition="Resource(s) that are the subject of the event", formalDefinition="Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge." ) 1107 protected List<MessageDefinitionFocusComponent> focus; 1108 1109 /** 1110 * Declare at a message definition level whether a response is required or only upon error or success, or never. 1111 */ 1112 @Child(name = "responseRequired", type = {CodeType.class}, order=23, min=0, max=1, modifier=false, summary=false) 1113 @Description(shortDefinition="always | on-error | never | on-success", formalDefinition="Declare at a message definition level whether a response is required or only upon error or success, or never." ) 1114 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/messageheader-response-request") 1115 protected Enumeration<MessageheaderResponseRequest> responseRequired; 1116 1117 /** 1118 * Indicates what types of messages may be sent as an application-level response to this message. 1119 */ 1120 @Child(name = "allowedResponse", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1121 @Description(shortDefinition="Responses to this message", formalDefinition="Indicates what types of messages may be sent as an application-level response to this message." ) 1122 protected List<MessageDefinitionAllowedResponseComponent> allowedResponse; 1123 1124 /** 1125 * Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources. 1126 */ 1127 @Child(name = "graph", type = {CanonicalType.class}, order=25, min=0, max=1, modifier=false, summary=false) 1128 @Description(shortDefinition="Canonical reference to a GraphDefinition", formalDefinition="Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources." ) 1129 protected CanonicalType graph; 1130 1131 private static final long serialVersionUID = 1378164694L; 1132 1133 /** 1134 * Constructor 1135 */ 1136 public MessageDefinition() { 1137 super(); 1138 } 1139 1140 /** 1141 * Constructor 1142 */ 1143 public MessageDefinition(PublicationStatus status, Date date, DataType event) { 1144 super(); 1145 this.setStatus(status); 1146 this.setDate(date); 1147 this.setEvent(event); 1148 } 1149 1150 /** 1151 * @return {@link #url} (The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1152 */ 1153 public UriType getUrlElement() { 1154 if (this.url == null) 1155 if (Configuration.errorOnAutoCreate()) 1156 throw new Error("Attempt to auto-create MessageDefinition.url"); 1157 else if (Configuration.doAutoCreate()) 1158 this.url = new UriType(); // bb 1159 return this.url; 1160 } 1161 1162 public boolean hasUrlElement() { 1163 return this.url != null && !this.url.isEmpty(); 1164 } 1165 1166 public boolean hasUrl() { 1167 return this.url != null && !this.url.isEmpty(); 1168 } 1169 1170 /** 1171 * @param value {@link #url} (The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1172 */ 1173 public MessageDefinition setUrlElement(UriType value) { 1174 this.url = value; 1175 return this; 1176 } 1177 1178 /** 1179 * @return The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server. 1180 */ 1181 public String getUrl() { 1182 return this.url == null ? null : this.url.getValue(); 1183 } 1184 1185 /** 1186 * @param value The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server. 1187 */ 1188 public MessageDefinition setUrl(String value) { 1189 if (Utilities.noString(value)) 1190 this.url = null; 1191 else { 1192 if (this.url == null) 1193 this.url = new UriType(); 1194 this.url.setValue(value); 1195 } 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #identifier} (A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1201 */ 1202 public List<Identifier> getIdentifier() { 1203 if (this.identifier == null) 1204 this.identifier = new ArrayList<Identifier>(); 1205 return this.identifier; 1206 } 1207 1208 /** 1209 * @return Returns a reference to <code>this</code> for easy method chaining 1210 */ 1211 public MessageDefinition setIdentifier(List<Identifier> theIdentifier) { 1212 this.identifier = theIdentifier; 1213 return this; 1214 } 1215 1216 public boolean hasIdentifier() { 1217 if (this.identifier == null) 1218 return false; 1219 for (Identifier item : this.identifier) 1220 if (!item.isEmpty()) 1221 return true; 1222 return false; 1223 } 1224 1225 public Identifier addIdentifier() { //3 1226 Identifier t = new Identifier(); 1227 if (this.identifier == null) 1228 this.identifier = new ArrayList<Identifier>(); 1229 this.identifier.add(t); 1230 return t; 1231 } 1232 1233 public MessageDefinition addIdentifier(Identifier t) { //3 1234 if (t == null) 1235 return this; 1236 if (this.identifier == null) 1237 this.identifier = new ArrayList<Identifier>(); 1238 this.identifier.add(t); 1239 return this; 1240 } 1241 1242 /** 1243 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1244 */ 1245 public Identifier getIdentifierFirstRep() { 1246 if (getIdentifier().isEmpty()) { 1247 addIdentifier(); 1248 } 1249 return getIdentifier().get(0); 1250 } 1251 1252 /** 1253 * @return {@link #version} (The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1254 */ 1255 public StringType getVersionElement() { 1256 if (this.version == null) 1257 if (Configuration.errorOnAutoCreate()) 1258 throw new Error("Attempt to auto-create MessageDefinition.version"); 1259 else if (Configuration.doAutoCreate()) 1260 this.version = new StringType(); // bb 1261 return this.version; 1262 } 1263 1264 public boolean hasVersionElement() { 1265 return this.version != null && !this.version.isEmpty(); 1266 } 1267 1268 public boolean hasVersion() { 1269 return this.version != null && !this.version.isEmpty(); 1270 } 1271 1272 /** 1273 * @param value {@link #version} (The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1274 */ 1275 public MessageDefinition setVersionElement(StringType value) { 1276 this.version = value; 1277 return this; 1278 } 1279 1280 /** 1281 * @return The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1282 */ 1283 public String getVersion() { 1284 return this.version == null ? null : this.version.getValue(); 1285 } 1286 1287 /** 1288 * @param value The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1289 */ 1290 public MessageDefinition setVersion(String value) { 1291 if (Utilities.noString(value)) 1292 this.version = null; 1293 else { 1294 if (this.version == null) 1295 this.version = new StringType(); 1296 this.version.setValue(value); 1297 } 1298 return this; 1299 } 1300 1301 /** 1302 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1303 */ 1304 public DataType getVersionAlgorithm() { 1305 return this.versionAlgorithm; 1306 } 1307 1308 /** 1309 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1310 */ 1311 public StringType getVersionAlgorithmStringType() throws FHIRException { 1312 if (this.versionAlgorithm == null) 1313 this.versionAlgorithm = new StringType(); 1314 if (!(this.versionAlgorithm instanceof StringType)) 1315 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1316 return (StringType) this.versionAlgorithm; 1317 } 1318 1319 public boolean hasVersionAlgorithmStringType() { 1320 return this != null && this.versionAlgorithm instanceof StringType; 1321 } 1322 1323 /** 1324 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1325 */ 1326 public Coding getVersionAlgorithmCoding() throws FHIRException { 1327 if (this.versionAlgorithm == null) 1328 this.versionAlgorithm = new Coding(); 1329 if (!(this.versionAlgorithm instanceof Coding)) 1330 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1331 return (Coding) this.versionAlgorithm; 1332 } 1333 1334 public boolean hasVersionAlgorithmCoding() { 1335 return this != null && this.versionAlgorithm instanceof Coding; 1336 } 1337 1338 public boolean hasVersionAlgorithm() { 1339 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1340 } 1341 1342 /** 1343 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1344 */ 1345 public MessageDefinition setVersionAlgorithm(DataType value) { 1346 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1347 throw new FHIRException("Not the right type for MessageDefinition.versionAlgorithm[x]: "+value.fhirType()); 1348 this.versionAlgorithm = value; 1349 return this; 1350 } 1351 1352 /** 1353 * @return {@link #name} (A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1354 */ 1355 public StringType getNameElement() { 1356 if (this.name == null) 1357 if (Configuration.errorOnAutoCreate()) 1358 throw new Error("Attempt to auto-create MessageDefinition.name"); 1359 else if (Configuration.doAutoCreate()) 1360 this.name = new StringType(); // bb 1361 return this.name; 1362 } 1363 1364 public boolean hasNameElement() { 1365 return this.name != null && !this.name.isEmpty(); 1366 } 1367 1368 public boolean hasName() { 1369 return this.name != null && !this.name.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #name} (A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1374 */ 1375 public MessageDefinition setNameElement(StringType value) { 1376 this.name = value; 1377 return this; 1378 } 1379 1380 /** 1381 * @return A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1382 */ 1383 public String getName() { 1384 return this.name == null ? null : this.name.getValue(); 1385 } 1386 1387 /** 1388 * @param value A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1389 */ 1390 public MessageDefinition setName(String value) { 1391 if (Utilities.noString(value)) 1392 this.name = null; 1393 else { 1394 if (this.name == null) 1395 this.name = new StringType(); 1396 this.name.setValue(value); 1397 } 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #title} (A short, descriptive, user-friendly title for the message definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1403 */ 1404 public StringType getTitleElement() { 1405 if (this.title == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create MessageDefinition.title"); 1408 else if (Configuration.doAutoCreate()) 1409 this.title = new StringType(); // bb 1410 return this.title; 1411 } 1412 1413 public boolean hasTitleElement() { 1414 return this.title != null && !this.title.isEmpty(); 1415 } 1416 1417 public boolean hasTitle() { 1418 return this.title != null && !this.title.isEmpty(); 1419 } 1420 1421 /** 1422 * @param value {@link #title} (A short, descriptive, user-friendly title for the message definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1423 */ 1424 public MessageDefinition setTitleElement(StringType value) { 1425 this.title = value; 1426 return this; 1427 } 1428 1429 /** 1430 * @return A short, descriptive, user-friendly title for the message definition. 1431 */ 1432 public String getTitle() { 1433 return this.title == null ? null : this.title.getValue(); 1434 } 1435 1436 /** 1437 * @param value A short, descriptive, user-friendly title for the message definition. 1438 */ 1439 public MessageDefinition setTitle(String value) { 1440 if (Utilities.noString(value)) 1441 this.title = null; 1442 else { 1443 if (this.title == null) 1444 this.title = new StringType(); 1445 this.title.setValue(value); 1446 } 1447 return this; 1448 } 1449 1450 /** 1451 * @return {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1452 */ 1453 public List<CanonicalType> getReplaces() { 1454 if (this.replaces == null) 1455 this.replaces = new ArrayList<CanonicalType>(); 1456 return this.replaces; 1457 } 1458 1459 /** 1460 * @return Returns a reference to <code>this</code> for easy method chaining 1461 */ 1462 public MessageDefinition setReplaces(List<CanonicalType> theReplaces) { 1463 this.replaces = theReplaces; 1464 return this; 1465 } 1466 1467 public boolean hasReplaces() { 1468 if (this.replaces == null) 1469 return false; 1470 for (CanonicalType item : this.replaces) 1471 if (!item.isEmpty()) 1472 return true; 1473 return false; 1474 } 1475 1476 /** 1477 * @return {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1478 */ 1479 public CanonicalType addReplacesElement() {//2 1480 CanonicalType t = new CanonicalType(); 1481 if (this.replaces == null) 1482 this.replaces = new ArrayList<CanonicalType>(); 1483 this.replaces.add(t); 1484 return t; 1485 } 1486 1487 /** 1488 * @param value {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1489 */ 1490 public MessageDefinition addReplaces(String value) { //1 1491 CanonicalType t = new CanonicalType(); 1492 t.setValue(value); 1493 if (this.replaces == null) 1494 this.replaces = new ArrayList<CanonicalType>(); 1495 this.replaces.add(t); 1496 return this; 1497 } 1498 1499 /** 1500 * @param value {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1501 */ 1502 public boolean hasReplaces(String value) { 1503 if (this.replaces == null) 1504 return false; 1505 for (CanonicalType v : this.replaces) 1506 if (v.getValue().equals(value)) // canonical 1507 return true; 1508 return false; 1509 } 1510 1511 /** 1512 * @return {@link #status} (The status of this message definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1513 */ 1514 public Enumeration<PublicationStatus> getStatusElement() { 1515 if (this.status == null) 1516 if (Configuration.errorOnAutoCreate()) 1517 throw new Error("Attempt to auto-create MessageDefinition.status"); 1518 else if (Configuration.doAutoCreate()) 1519 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1520 return this.status; 1521 } 1522 1523 public boolean hasStatusElement() { 1524 return this.status != null && !this.status.isEmpty(); 1525 } 1526 1527 public boolean hasStatus() { 1528 return this.status != null && !this.status.isEmpty(); 1529 } 1530 1531 /** 1532 * @param value {@link #status} (The status of this message definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1533 */ 1534 public MessageDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1535 this.status = value; 1536 return this; 1537 } 1538 1539 /** 1540 * @return The status of this message definition. Enables tracking the life-cycle of the content. 1541 */ 1542 public PublicationStatus getStatus() { 1543 return this.status == null ? null : this.status.getValue(); 1544 } 1545 1546 /** 1547 * @param value The status of this message definition. Enables tracking the life-cycle of the content. 1548 */ 1549 public MessageDefinition setStatus(PublicationStatus value) { 1550 if (this.status == null) 1551 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1552 this.status.setValue(value); 1553 return this; 1554 } 1555 1556 /** 1557 * @return {@link #experimental} (A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1558 */ 1559 public BooleanType getExperimentalElement() { 1560 if (this.experimental == null) 1561 if (Configuration.errorOnAutoCreate()) 1562 throw new Error("Attempt to auto-create MessageDefinition.experimental"); 1563 else if (Configuration.doAutoCreate()) 1564 this.experimental = new BooleanType(); // bb 1565 return this.experimental; 1566 } 1567 1568 public boolean hasExperimentalElement() { 1569 return this.experimental != null && !this.experimental.isEmpty(); 1570 } 1571 1572 public boolean hasExperimental() { 1573 return this.experimental != null && !this.experimental.isEmpty(); 1574 } 1575 1576 /** 1577 * @param value {@link #experimental} (A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1578 */ 1579 public MessageDefinition setExperimentalElement(BooleanType value) { 1580 this.experimental = value; 1581 return this; 1582 } 1583 1584 /** 1585 * @return A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1586 */ 1587 public boolean getExperimental() { 1588 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1589 } 1590 1591 /** 1592 * @param value A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1593 */ 1594 public MessageDefinition setExperimental(boolean value) { 1595 if (this.experimental == null) 1596 this.experimental = new BooleanType(); 1597 this.experimental.setValue(value); 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #date} (The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1603 */ 1604 public DateTimeType getDateElement() { 1605 if (this.date == null) 1606 if (Configuration.errorOnAutoCreate()) 1607 throw new Error("Attempt to auto-create MessageDefinition.date"); 1608 else if (Configuration.doAutoCreate()) 1609 this.date = new DateTimeType(); // bb 1610 return this.date; 1611 } 1612 1613 public boolean hasDateElement() { 1614 return this.date != null && !this.date.isEmpty(); 1615 } 1616 1617 public boolean hasDate() { 1618 return this.date != null && !this.date.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #date} (The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1623 */ 1624 public MessageDefinition setDateElement(DateTimeType value) { 1625 this.date = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1631 */ 1632 public Date getDate() { 1633 return this.date == null ? null : this.date.getValue(); 1634 } 1635 1636 /** 1637 * @param value The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1638 */ 1639 public MessageDefinition setDate(Date value) { 1640 if (this.date == null) 1641 this.date = new DateTimeType(); 1642 this.date.setValue(value); 1643 return this; 1644 } 1645 1646 /** 1647 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1648 */ 1649 public StringType getPublisherElement() { 1650 if (this.publisher == null) 1651 if (Configuration.errorOnAutoCreate()) 1652 throw new Error("Attempt to auto-create MessageDefinition.publisher"); 1653 else if (Configuration.doAutoCreate()) 1654 this.publisher = new StringType(); // bb 1655 return this.publisher; 1656 } 1657 1658 public boolean hasPublisherElement() { 1659 return this.publisher != null && !this.publisher.isEmpty(); 1660 } 1661 1662 public boolean hasPublisher() { 1663 return this.publisher != null && !this.publisher.isEmpty(); 1664 } 1665 1666 /** 1667 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1668 */ 1669 public MessageDefinition setPublisherElement(StringType value) { 1670 this.publisher = value; 1671 return this; 1672 } 1673 1674 /** 1675 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the message definition. 1676 */ 1677 public String getPublisher() { 1678 return this.publisher == null ? null : this.publisher.getValue(); 1679 } 1680 1681 /** 1682 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the message definition. 1683 */ 1684 public MessageDefinition setPublisher(String value) { 1685 if (Utilities.noString(value)) 1686 this.publisher = null; 1687 else { 1688 if (this.publisher == null) 1689 this.publisher = new StringType(); 1690 this.publisher.setValue(value); 1691 } 1692 return this; 1693 } 1694 1695 /** 1696 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1697 */ 1698 public List<ContactDetail> getContact() { 1699 if (this.contact == null) 1700 this.contact = new ArrayList<ContactDetail>(); 1701 return this.contact; 1702 } 1703 1704 /** 1705 * @return Returns a reference to <code>this</code> for easy method chaining 1706 */ 1707 public MessageDefinition setContact(List<ContactDetail> theContact) { 1708 this.contact = theContact; 1709 return this; 1710 } 1711 1712 public boolean hasContact() { 1713 if (this.contact == null) 1714 return false; 1715 for (ContactDetail item : this.contact) 1716 if (!item.isEmpty()) 1717 return true; 1718 return false; 1719 } 1720 1721 public ContactDetail addContact() { //3 1722 ContactDetail t = new ContactDetail(); 1723 if (this.contact == null) 1724 this.contact = new ArrayList<ContactDetail>(); 1725 this.contact.add(t); 1726 return t; 1727 } 1728 1729 public MessageDefinition addContact(ContactDetail t) { //3 1730 if (t == null) 1731 return this; 1732 if (this.contact == null) 1733 this.contact = new ArrayList<ContactDetail>(); 1734 this.contact.add(t); 1735 return this; 1736 } 1737 1738 /** 1739 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1740 */ 1741 public ContactDetail getContactFirstRep() { 1742 if (getContact().isEmpty()) { 1743 addContact(); 1744 } 1745 return getContact().get(0); 1746 } 1747 1748 /** 1749 * @return {@link #description} (A free text natural language description of the message definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1750 */ 1751 public MarkdownType getDescriptionElement() { 1752 if (this.description == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create MessageDefinition.description"); 1755 else if (Configuration.doAutoCreate()) 1756 this.description = new MarkdownType(); // bb 1757 return this.description; 1758 } 1759 1760 public boolean hasDescriptionElement() { 1761 return this.description != null && !this.description.isEmpty(); 1762 } 1763 1764 public boolean hasDescription() { 1765 return this.description != null && !this.description.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #description} (A free text natural language description of the message definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1770 */ 1771 public MessageDefinition setDescriptionElement(MarkdownType value) { 1772 this.description = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return A free text natural language description of the message definition from a consumer's perspective. 1778 */ 1779 public String getDescription() { 1780 return this.description == null ? null : this.description.getValue(); 1781 } 1782 1783 /** 1784 * @param value A free text natural language description of the message definition from a consumer's perspective. 1785 */ 1786 public MessageDefinition setDescription(String value) { 1787 if (Utilities.noString(value)) 1788 this.description = null; 1789 else { 1790 if (this.description == null) 1791 this.description = new MarkdownType(); 1792 this.description.setValue(value); 1793 } 1794 return this; 1795 } 1796 1797 /** 1798 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.) 1799 */ 1800 public List<UsageContext> getUseContext() { 1801 if (this.useContext == null) 1802 this.useContext = new ArrayList<UsageContext>(); 1803 return this.useContext; 1804 } 1805 1806 /** 1807 * @return Returns a reference to <code>this</code> for easy method chaining 1808 */ 1809 public MessageDefinition setUseContext(List<UsageContext> theUseContext) { 1810 this.useContext = theUseContext; 1811 return this; 1812 } 1813 1814 public boolean hasUseContext() { 1815 if (this.useContext == null) 1816 return false; 1817 for (UsageContext item : this.useContext) 1818 if (!item.isEmpty()) 1819 return true; 1820 return false; 1821 } 1822 1823 public UsageContext addUseContext() { //3 1824 UsageContext t = new UsageContext(); 1825 if (this.useContext == null) 1826 this.useContext = new ArrayList<UsageContext>(); 1827 this.useContext.add(t); 1828 return t; 1829 } 1830 1831 public MessageDefinition addUseContext(UsageContext t) { //3 1832 if (t == null) 1833 return this; 1834 if (this.useContext == null) 1835 this.useContext = new ArrayList<UsageContext>(); 1836 this.useContext.add(t); 1837 return this; 1838 } 1839 1840 /** 1841 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1842 */ 1843 public UsageContext getUseContextFirstRep() { 1844 if (getUseContext().isEmpty()) { 1845 addUseContext(); 1846 } 1847 return getUseContext().get(0); 1848 } 1849 1850 /** 1851 * @return {@link #jurisdiction} (A legal or geographic region in which the message definition is intended to be used.) 1852 */ 1853 public List<CodeableConcept> getJurisdiction() { 1854 if (this.jurisdiction == null) 1855 this.jurisdiction = new ArrayList<CodeableConcept>(); 1856 return this.jurisdiction; 1857 } 1858 1859 /** 1860 * @return Returns a reference to <code>this</code> for easy method chaining 1861 */ 1862 public MessageDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1863 this.jurisdiction = theJurisdiction; 1864 return this; 1865 } 1866 1867 public boolean hasJurisdiction() { 1868 if (this.jurisdiction == null) 1869 return false; 1870 for (CodeableConcept item : this.jurisdiction) 1871 if (!item.isEmpty()) 1872 return true; 1873 return false; 1874 } 1875 1876 public CodeableConcept addJurisdiction() { //3 1877 CodeableConcept t = new CodeableConcept(); 1878 if (this.jurisdiction == null) 1879 this.jurisdiction = new ArrayList<CodeableConcept>(); 1880 this.jurisdiction.add(t); 1881 return t; 1882 } 1883 1884 public MessageDefinition addJurisdiction(CodeableConcept t) { //3 1885 if (t == null) 1886 return this; 1887 if (this.jurisdiction == null) 1888 this.jurisdiction = new ArrayList<CodeableConcept>(); 1889 this.jurisdiction.add(t); 1890 return this; 1891 } 1892 1893 /** 1894 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1895 */ 1896 public CodeableConcept getJurisdictionFirstRep() { 1897 if (getJurisdiction().isEmpty()) { 1898 addJurisdiction(); 1899 } 1900 return getJurisdiction().get(0); 1901 } 1902 1903 /** 1904 * @return {@link #purpose} (Explanation of why this message definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1905 */ 1906 public MarkdownType getPurposeElement() { 1907 if (this.purpose == null) 1908 if (Configuration.errorOnAutoCreate()) 1909 throw new Error("Attempt to auto-create MessageDefinition.purpose"); 1910 else if (Configuration.doAutoCreate()) 1911 this.purpose = new MarkdownType(); // bb 1912 return this.purpose; 1913 } 1914 1915 public boolean hasPurposeElement() { 1916 return this.purpose != null && !this.purpose.isEmpty(); 1917 } 1918 1919 public boolean hasPurpose() { 1920 return this.purpose != null && !this.purpose.isEmpty(); 1921 } 1922 1923 /** 1924 * @param value {@link #purpose} (Explanation of why this message definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1925 */ 1926 public MessageDefinition setPurposeElement(MarkdownType value) { 1927 this.purpose = value; 1928 return this; 1929 } 1930 1931 /** 1932 * @return Explanation of why this message definition is needed and why it has been designed as it has. 1933 */ 1934 public String getPurpose() { 1935 return this.purpose == null ? null : this.purpose.getValue(); 1936 } 1937 1938 /** 1939 * @param value Explanation of why this message definition is needed and why it has been designed as it has. 1940 */ 1941 public MessageDefinition setPurpose(String value) { 1942 if (Utilities.noString(value)) 1943 this.purpose = null; 1944 else { 1945 if (this.purpose == null) 1946 this.purpose = new MarkdownType(); 1947 this.purpose.setValue(value); 1948 } 1949 return this; 1950 } 1951 1952 /** 1953 * @return {@link #copyright} (A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1954 */ 1955 public MarkdownType getCopyrightElement() { 1956 if (this.copyright == null) 1957 if (Configuration.errorOnAutoCreate()) 1958 throw new Error("Attempt to auto-create MessageDefinition.copyright"); 1959 else if (Configuration.doAutoCreate()) 1960 this.copyright = new MarkdownType(); // bb 1961 return this.copyright; 1962 } 1963 1964 public boolean hasCopyrightElement() { 1965 return this.copyright != null && !this.copyright.isEmpty(); 1966 } 1967 1968 public boolean hasCopyright() { 1969 return this.copyright != null && !this.copyright.isEmpty(); 1970 } 1971 1972 /** 1973 * @param value {@link #copyright} (A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1974 */ 1975 public MessageDefinition setCopyrightElement(MarkdownType value) { 1976 this.copyright = value; 1977 return this; 1978 } 1979 1980 /** 1981 * @return A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 1982 */ 1983 public String getCopyright() { 1984 return this.copyright == null ? null : this.copyright.getValue(); 1985 } 1986 1987 /** 1988 * @param value A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 1989 */ 1990 public MessageDefinition setCopyright(String value) { 1991 if (Utilities.noString(value)) 1992 this.copyright = null; 1993 else { 1994 if (this.copyright == null) 1995 this.copyright = new MarkdownType(); 1996 this.copyright.setValue(value); 1997 } 1998 return this; 1999 } 2000 2001 /** 2002 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2003 */ 2004 public StringType getCopyrightLabelElement() { 2005 if (this.copyrightLabel == null) 2006 if (Configuration.errorOnAutoCreate()) 2007 throw new Error("Attempt to auto-create MessageDefinition.copyrightLabel"); 2008 else if (Configuration.doAutoCreate()) 2009 this.copyrightLabel = new StringType(); // bb 2010 return this.copyrightLabel; 2011 } 2012 2013 public boolean hasCopyrightLabelElement() { 2014 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2015 } 2016 2017 public boolean hasCopyrightLabel() { 2018 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2019 } 2020 2021 /** 2022 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2023 */ 2024 public MessageDefinition setCopyrightLabelElement(StringType value) { 2025 this.copyrightLabel = value; 2026 return this; 2027 } 2028 2029 /** 2030 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2031 */ 2032 public String getCopyrightLabel() { 2033 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2034 } 2035 2036 /** 2037 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2038 */ 2039 public MessageDefinition setCopyrightLabel(String value) { 2040 if (Utilities.noString(value)) 2041 this.copyrightLabel = null; 2042 else { 2043 if (this.copyrightLabel == null) 2044 this.copyrightLabel = new StringType(); 2045 this.copyrightLabel.setValue(value); 2046 } 2047 return this; 2048 } 2049 2050 /** 2051 * @return {@link #base} (The MessageDefinition that is the basis for the contents of this resource.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 2052 */ 2053 public CanonicalType getBaseElement() { 2054 if (this.base == null) 2055 if (Configuration.errorOnAutoCreate()) 2056 throw new Error("Attempt to auto-create MessageDefinition.base"); 2057 else if (Configuration.doAutoCreate()) 2058 this.base = new CanonicalType(); // bb 2059 return this.base; 2060 } 2061 2062 public boolean hasBaseElement() { 2063 return this.base != null && !this.base.isEmpty(); 2064 } 2065 2066 public boolean hasBase() { 2067 return this.base != null && !this.base.isEmpty(); 2068 } 2069 2070 /** 2071 * @param value {@link #base} (The MessageDefinition that is the basis for the contents of this resource.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 2072 */ 2073 public MessageDefinition setBaseElement(CanonicalType value) { 2074 this.base = value; 2075 return this; 2076 } 2077 2078 /** 2079 * @return The MessageDefinition that is the basis for the contents of this resource. 2080 */ 2081 public String getBase() { 2082 return this.base == null ? null : this.base.getValue(); 2083 } 2084 2085 /** 2086 * @param value The MessageDefinition that is the basis for the contents of this resource. 2087 */ 2088 public MessageDefinition setBase(String value) { 2089 if (Utilities.noString(value)) 2090 this.base = null; 2091 else { 2092 if (this.base == null) 2093 this.base = new CanonicalType(); 2094 this.base.setValue(value); 2095 } 2096 return this; 2097 } 2098 2099 /** 2100 * @return {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2101 */ 2102 public List<CanonicalType> getParent() { 2103 if (this.parent == null) 2104 this.parent = new ArrayList<CanonicalType>(); 2105 return this.parent; 2106 } 2107 2108 /** 2109 * @return Returns a reference to <code>this</code> for easy method chaining 2110 */ 2111 public MessageDefinition setParent(List<CanonicalType> theParent) { 2112 this.parent = theParent; 2113 return this; 2114 } 2115 2116 public boolean hasParent() { 2117 if (this.parent == null) 2118 return false; 2119 for (CanonicalType item : this.parent) 2120 if (!item.isEmpty()) 2121 return true; 2122 return false; 2123 } 2124 2125 /** 2126 * @return {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2127 */ 2128 public CanonicalType addParentElement() {//2 2129 CanonicalType t = new CanonicalType(); 2130 if (this.parent == null) 2131 this.parent = new ArrayList<CanonicalType>(); 2132 this.parent.add(t); 2133 return t; 2134 } 2135 2136 /** 2137 * @param value {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2138 */ 2139 public MessageDefinition addParent(String value) { //1 2140 CanonicalType t = new CanonicalType(); 2141 t.setValue(value); 2142 if (this.parent == null) 2143 this.parent = new ArrayList<CanonicalType>(); 2144 this.parent.add(t); 2145 return this; 2146 } 2147 2148 /** 2149 * @param value {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 2150 */ 2151 public boolean hasParent(String value) { 2152 if (this.parent == null) 2153 return false; 2154 for (CanonicalType v : this.parent) 2155 if (v.getValue().equals(value)) // canonical 2156 return true; 2157 return false; 2158 } 2159 2160 /** 2161 * @return {@link #event} (Event code or link to the EventDefinition.) 2162 */ 2163 public DataType getEvent() { 2164 return this.event; 2165 } 2166 2167 /** 2168 * @return {@link #event} (Event code or link to the EventDefinition.) 2169 */ 2170 public Coding getEventCoding() throws FHIRException { 2171 if (this.event == null) 2172 this.event = new Coding(); 2173 if (!(this.event instanceof Coding)) 2174 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.event.getClass().getName()+" was encountered"); 2175 return (Coding) this.event; 2176 } 2177 2178 public boolean hasEventCoding() { 2179 return this != null && this.event instanceof Coding; 2180 } 2181 2182 /** 2183 * @return {@link #event} (Event code or link to the EventDefinition.) 2184 */ 2185 public UriType getEventUriType() throws FHIRException { 2186 if (this.event == null) 2187 this.event = new UriType(); 2188 if (!(this.event instanceof UriType)) 2189 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.event.getClass().getName()+" was encountered"); 2190 return (UriType) this.event; 2191 } 2192 2193 public boolean hasEventUriType() { 2194 return this != null && this.event instanceof UriType; 2195 } 2196 2197 public boolean hasEvent() { 2198 return this.event != null && !this.event.isEmpty(); 2199 } 2200 2201 /** 2202 * @param value {@link #event} (Event code or link to the EventDefinition.) 2203 */ 2204 public MessageDefinition setEvent(DataType value) { 2205 if (value != null && !(value instanceof Coding || value instanceof UriType)) 2206 throw new FHIRException("Not the right type for MessageDefinition.event[x]: "+value.fhirType()); 2207 this.event = value; 2208 return this; 2209 } 2210 2211 /** 2212 * @return {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 2213 */ 2214 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 2215 if (this.category == null) 2216 if (Configuration.errorOnAutoCreate()) 2217 throw new Error("Attempt to auto-create MessageDefinition.category"); 2218 else if (Configuration.doAutoCreate()) 2219 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 2220 return this.category; 2221 } 2222 2223 public boolean hasCategoryElement() { 2224 return this.category != null && !this.category.isEmpty(); 2225 } 2226 2227 public boolean hasCategory() { 2228 return this.category != null && !this.category.isEmpty(); 2229 } 2230 2231 /** 2232 * @param value {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 2233 */ 2234 public MessageDefinition setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 2235 this.category = value; 2236 return this; 2237 } 2238 2239 /** 2240 * @return The impact of the content of the message. 2241 */ 2242 public MessageSignificanceCategory getCategory() { 2243 return this.category == null ? null : this.category.getValue(); 2244 } 2245 2246 /** 2247 * @param value The impact of the content of the message. 2248 */ 2249 public MessageDefinition setCategory(MessageSignificanceCategory value) { 2250 if (value == null) 2251 this.category = null; 2252 else { 2253 if (this.category == null) 2254 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 2255 this.category.setValue(value); 2256 } 2257 return this; 2258 } 2259 2260 /** 2261 * @return {@link #focus} (Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.) 2262 */ 2263 public List<MessageDefinitionFocusComponent> getFocus() { 2264 if (this.focus == null) 2265 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2266 return this.focus; 2267 } 2268 2269 /** 2270 * @return Returns a reference to <code>this</code> for easy method chaining 2271 */ 2272 public MessageDefinition setFocus(List<MessageDefinitionFocusComponent> theFocus) { 2273 this.focus = theFocus; 2274 return this; 2275 } 2276 2277 public boolean hasFocus() { 2278 if (this.focus == null) 2279 return false; 2280 for (MessageDefinitionFocusComponent item : this.focus) 2281 if (!item.isEmpty()) 2282 return true; 2283 return false; 2284 } 2285 2286 public MessageDefinitionFocusComponent addFocus() { //3 2287 MessageDefinitionFocusComponent t = new MessageDefinitionFocusComponent(); 2288 if (this.focus == null) 2289 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2290 this.focus.add(t); 2291 return t; 2292 } 2293 2294 public MessageDefinition addFocus(MessageDefinitionFocusComponent t) { //3 2295 if (t == null) 2296 return this; 2297 if (this.focus == null) 2298 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2299 this.focus.add(t); 2300 return this; 2301 } 2302 2303 /** 2304 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 2305 */ 2306 public MessageDefinitionFocusComponent getFocusFirstRep() { 2307 if (getFocus().isEmpty()) { 2308 addFocus(); 2309 } 2310 return getFocus().get(0); 2311 } 2312 2313 /** 2314 * @return {@link #responseRequired} (Declare at a message definition level whether a response is required or only upon error or success, or never.). This is the underlying object with id, value and extensions. The accessor "getResponseRequired" gives direct access to the value 2315 */ 2316 public Enumeration<MessageheaderResponseRequest> getResponseRequiredElement() { 2317 if (this.responseRequired == null) 2318 if (Configuration.errorOnAutoCreate()) 2319 throw new Error("Attempt to auto-create MessageDefinition.responseRequired"); 2320 else if (Configuration.doAutoCreate()) 2321 this.responseRequired = new Enumeration<MessageheaderResponseRequest>(new MessageheaderResponseRequestEnumFactory()); // bb 2322 return this.responseRequired; 2323 } 2324 2325 public boolean hasResponseRequiredElement() { 2326 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2327 } 2328 2329 public boolean hasResponseRequired() { 2330 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2331 } 2332 2333 /** 2334 * @param value {@link #responseRequired} (Declare at a message definition level whether a response is required or only upon error or success, or never.). This is the underlying object with id, value and extensions. The accessor "getResponseRequired" gives direct access to the value 2335 */ 2336 public MessageDefinition setResponseRequiredElement(Enumeration<MessageheaderResponseRequest> value) { 2337 this.responseRequired = value; 2338 return this; 2339 } 2340 2341 /** 2342 * @return Declare at a message definition level whether a response is required or only upon error or success, or never. 2343 */ 2344 public MessageheaderResponseRequest getResponseRequired() { 2345 return this.responseRequired == null ? null : this.responseRequired.getValue(); 2346 } 2347 2348 /** 2349 * @param value Declare at a message definition level whether a response is required or only upon error or success, or never. 2350 */ 2351 public MessageDefinition setResponseRequired(MessageheaderResponseRequest value) { 2352 if (value == null) 2353 this.responseRequired = null; 2354 else { 2355 if (this.responseRequired == null) 2356 this.responseRequired = new Enumeration<MessageheaderResponseRequest>(new MessageheaderResponseRequestEnumFactory()); 2357 this.responseRequired.setValue(value); 2358 } 2359 return this; 2360 } 2361 2362 /** 2363 * @return {@link #allowedResponse} (Indicates what types of messages may be sent as an application-level response to this message.) 2364 */ 2365 public List<MessageDefinitionAllowedResponseComponent> getAllowedResponse() { 2366 if (this.allowedResponse == null) 2367 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2368 return this.allowedResponse; 2369 } 2370 2371 /** 2372 * @return Returns a reference to <code>this</code> for easy method chaining 2373 */ 2374 public MessageDefinition setAllowedResponse(List<MessageDefinitionAllowedResponseComponent> theAllowedResponse) { 2375 this.allowedResponse = theAllowedResponse; 2376 return this; 2377 } 2378 2379 public boolean hasAllowedResponse() { 2380 if (this.allowedResponse == null) 2381 return false; 2382 for (MessageDefinitionAllowedResponseComponent item : this.allowedResponse) 2383 if (!item.isEmpty()) 2384 return true; 2385 return false; 2386 } 2387 2388 public MessageDefinitionAllowedResponseComponent addAllowedResponse() { //3 2389 MessageDefinitionAllowedResponseComponent t = new MessageDefinitionAllowedResponseComponent(); 2390 if (this.allowedResponse == null) 2391 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2392 this.allowedResponse.add(t); 2393 return t; 2394 } 2395 2396 public MessageDefinition addAllowedResponse(MessageDefinitionAllowedResponseComponent t) { //3 2397 if (t == null) 2398 return this; 2399 if (this.allowedResponse == null) 2400 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2401 this.allowedResponse.add(t); 2402 return this; 2403 } 2404 2405 /** 2406 * @return The first repetition of repeating field {@link #allowedResponse}, creating it if it does not already exist {3} 2407 */ 2408 public MessageDefinitionAllowedResponseComponent getAllowedResponseFirstRep() { 2409 if (getAllowedResponse().isEmpty()) { 2410 addAllowedResponse(); 2411 } 2412 return getAllowedResponse().get(0); 2413 } 2414 2415 /** 2416 * @return {@link #graph} (Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.). This is the underlying object with id, value and extensions. The accessor "getGraph" gives direct access to the value 2417 */ 2418 public CanonicalType getGraphElement() { 2419 if (this.graph == null) 2420 if (Configuration.errorOnAutoCreate()) 2421 throw new Error("Attempt to auto-create MessageDefinition.graph"); 2422 else if (Configuration.doAutoCreate()) 2423 this.graph = new CanonicalType(); // bb 2424 return this.graph; 2425 } 2426 2427 public boolean hasGraphElement() { 2428 return this.graph != null && !this.graph.isEmpty(); 2429 } 2430 2431 public boolean hasGraph() { 2432 return this.graph != null && !this.graph.isEmpty(); 2433 } 2434 2435 /** 2436 * @param value {@link #graph} (Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.). This is the underlying object with id, value and extensions. The accessor "getGraph" gives direct access to the value 2437 */ 2438 public MessageDefinition setGraphElement(CanonicalType value) { 2439 this.graph = value; 2440 return this; 2441 } 2442 2443 /** 2444 * @return Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources. 2445 */ 2446 public String getGraph() { 2447 return this.graph == null ? null : this.graph.getValue(); 2448 } 2449 2450 /** 2451 * @param value Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources. 2452 */ 2453 public MessageDefinition setGraph(String value) { 2454 if (Utilities.noString(value)) 2455 this.graph = null; 2456 else { 2457 if (this.graph == null) 2458 this.graph = new CanonicalType(); 2459 this.graph.setValue(value); 2460 } 2461 return this; 2462 } 2463 2464 protected void listChildren(List<Property> children) { 2465 super.listChildren(children); 2466 children.add(new Property("url", "uri", "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 0, 1, url)); 2467 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2468 children.add(new Property("version", "string", "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2469 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2470 children.add(new Property("name", "string", "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2471 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the message definition.", 0, 1, title)); 2472 children.add(new Property("replaces", "canonical(MessageDefinition)", "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2473 children.add(new Property("status", "code", "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2474 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2475 children.add(new Property("date", "dateTime", "The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 0, 1, date)); 2476 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.", 0, 1, publisher)); 2477 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2478 children.add(new Property("description", "markdown", "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, description)); 2479 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2480 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the message definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2481 children.add(new Property("purpose", "markdown", "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2482 children.add(new Property("copyright", "markdown", "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 0, 1, copyright)); 2483 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2484 children.add(new Property("base", "canonical(MessageDefinition)", "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base)); 2485 children.add(new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, java.lang.Integer.MAX_VALUE, parent)); 2486 children.add(new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event)); 2487 children.add(new Property("category", "code", "The impact of the content of the message.", 0, 1, category)); 2488 children.add(new Property("focus", "", "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 0, java.lang.Integer.MAX_VALUE, focus)); 2489 children.add(new Property("responseRequired", "code", "Declare at a message definition level whether a response is required or only upon error or success, or never.", 0, 1, responseRequired)); 2490 children.add(new Property("allowedResponse", "", "Indicates what types of messages may be sent as an application-level response to this message.", 0, java.lang.Integer.MAX_VALUE, allowedResponse)); 2491 children.add(new Property("graph", "canonical(GraphDefinition)", "Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.", 0, 1, graph)); 2492 } 2493 2494 @Override 2495 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2496 switch (_hash) { 2497 case 116079: /*url*/ return new Property("url", "uri", "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 0, 1, url); 2498 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2499 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2500 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2501 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2502 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2503 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2504 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2505 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the message definition.", 0, 1, title); 2506 case -430332865: /*replaces*/ return new Property("replaces", "canonical(MessageDefinition)", "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces); 2507 case -892481550: /*status*/ return new Property("status", "code", "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2508 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2509 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the message definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 0, 1, date); 2510 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the message definition.", 0, 1, publisher); 2511 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2512 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, description); 2513 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2514 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the message definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2515 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose); 2516 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 0, 1, copyright); 2517 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2518 case 3016401: /*base*/ return new Property("base", "canonical(MessageDefinition)", "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base); 2519 case -995424086: /*parent*/ return new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, java.lang.Integer.MAX_VALUE, parent); 2520 case 278115238: /*event[x]*/ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event); 2521 case 96891546: /*event*/ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event); 2522 case -355957084: /*eventCoding*/ return new Property("event[x]", "Coding", "Event code or link to the EventDefinition.", 0, 1, event); 2523 case 278109298: /*eventUri*/ return new Property("event[x]", "uri", "Event code or link to the EventDefinition.", 0, 1, event); 2524 case 50511102: /*category*/ return new Property("category", "code", "The impact of the content of the message.", 0, 1, category); 2525 case 97604824: /*focus*/ return new Property("focus", "", "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 0, java.lang.Integer.MAX_VALUE, focus); 2526 case 791597824: /*responseRequired*/ return new Property("responseRequired", "code", "Declare at a message definition level whether a response is required or only upon error or success, or never.", 0, 1, responseRequired); 2527 case -1130933751: /*allowedResponse*/ return new Property("allowedResponse", "", "Indicates what types of messages may be sent as an application-level response to this message.", 0, java.lang.Integer.MAX_VALUE, allowedResponse); 2528 case 98615630: /*graph*/ return new Property("graph", "canonical(GraphDefinition)", "Graph is Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a GraphDefinition that it controls what additional resources are to be added to the Bundle when building the message. The GraphDefinition can also specify profiles that apply to the various resources.", 0, 1, graph); 2529 default: return super.getNamedProperty(_hash, _name, _checkValid); 2530 } 2531 2532 } 2533 2534 @Override 2535 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2536 switch (hash) { 2537 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2538 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2539 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2540 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2541 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2542 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2543 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 2544 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2545 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2546 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2547 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2548 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2549 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2550 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2551 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2552 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2553 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2554 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2555 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // CanonicalType 2556 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // CanonicalType 2557 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // DataType 2558 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<MessageSignificanceCategory> 2559 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // MessageDefinitionFocusComponent 2560 case 791597824: /*responseRequired*/ return this.responseRequired == null ? new Base[0] : new Base[] {this.responseRequired}; // Enumeration<MessageheaderResponseRequest> 2561 case -1130933751: /*allowedResponse*/ return this.allowedResponse == null ? new Base[0] : this.allowedResponse.toArray(new Base[this.allowedResponse.size()]); // MessageDefinitionAllowedResponseComponent 2562 case 98615630: /*graph*/ return this.graph == null ? new Base[0] : new Base[] {this.graph}; // CanonicalType 2563 default: return super.getProperty(hash, name, checkValid); 2564 } 2565 2566 } 2567 2568 @Override 2569 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2570 switch (hash) { 2571 case 116079: // url 2572 this.url = TypeConvertor.castToUri(value); // UriType 2573 return value; 2574 case -1618432855: // identifier 2575 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2576 return value; 2577 case 351608024: // version 2578 this.version = TypeConvertor.castToString(value); // StringType 2579 return value; 2580 case 1508158071: // versionAlgorithm 2581 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2582 return value; 2583 case 3373707: // name 2584 this.name = TypeConvertor.castToString(value); // StringType 2585 return value; 2586 case 110371416: // title 2587 this.title = TypeConvertor.castToString(value); // StringType 2588 return value; 2589 case -430332865: // replaces 2590 this.getReplaces().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2591 return value; 2592 case -892481550: // status 2593 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2594 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2595 return value; 2596 case -404562712: // experimental 2597 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2598 return value; 2599 case 3076014: // date 2600 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2601 return value; 2602 case 1447404028: // publisher 2603 this.publisher = TypeConvertor.castToString(value); // StringType 2604 return value; 2605 case 951526432: // contact 2606 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2607 return value; 2608 case -1724546052: // description 2609 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2610 return value; 2611 case -669707736: // useContext 2612 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2613 return value; 2614 case -507075711: // jurisdiction 2615 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2616 return value; 2617 case -220463842: // purpose 2618 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2619 return value; 2620 case 1522889671: // copyright 2621 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2622 return value; 2623 case 765157229: // copyrightLabel 2624 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2625 return value; 2626 case 3016401: // base 2627 this.base = TypeConvertor.castToCanonical(value); // CanonicalType 2628 return value; 2629 case -995424086: // parent 2630 this.getParent().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2631 return value; 2632 case 96891546: // event 2633 this.event = TypeConvertor.castToType(value); // DataType 2634 return value; 2635 case 50511102: // category 2636 value = new MessageSignificanceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2637 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2638 return value; 2639 case 97604824: // focus 2640 this.getFocus().add((MessageDefinitionFocusComponent) value); // MessageDefinitionFocusComponent 2641 return value; 2642 case 791597824: // responseRequired 2643 value = new MessageheaderResponseRequestEnumFactory().fromType(TypeConvertor.castToCode(value)); 2644 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 2645 return value; 2646 case -1130933751: // allowedResponse 2647 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); // MessageDefinitionAllowedResponseComponent 2648 return value; 2649 case 98615630: // graph 2650 this.graph = TypeConvertor.castToCanonical(value); // CanonicalType 2651 return value; 2652 default: return super.setProperty(hash, name, value); 2653 } 2654 2655 } 2656 2657 @Override 2658 public Base setProperty(String name, Base value) throws FHIRException { 2659 if (name.equals("url")) { 2660 this.url = TypeConvertor.castToUri(value); // UriType 2661 } else if (name.equals("identifier")) { 2662 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2663 } else if (name.equals("version")) { 2664 this.version = TypeConvertor.castToString(value); // StringType 2665 } else if (name.equals("versionAlgorithm[x]")) { 2666 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2667 } else if (name.equals("name")) { 2668 this.name = TypeConvertor.castToString(value); // StringType 2669 } else if (name.equals("title")) { 2670 this.title = TypeConvertor.castToString(value); // StringType 2671 } else if (name.equals("replaces")) { 2672 this.getReplaces().add(TypeConvertor.castToCanonical(value)); 2673 } else if (name.equals("status")) { 2674 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2675 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2676 } else if (name.equals("experimental")) { 2677 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2678 } else if (name.equals("date")) { 2679 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2680 } else if (name.equals("publisher")) { 2681 this.publisher = TypeConvertor.castToString(value); // StringType 2682 } else if (name.equals("contact")) { 2683 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2684 } else if (name.equals("description")) { 2685 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2686 } else if (name.equals("useContext")) { 2687 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2688 } else if (name.equals("jurisdiction")) { 2689 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2690 } else if (name.equals("purpose")) { 2691 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2692 } else if (name.equals("copyright")) { 2693 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2694 } else if (name.equals("copyrightLabel")) { 2695 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2696 } else if (name.equals("base")) { 2697 this.base = TypeConvertor.castToCanonical(value); // CanonicalType 2698 } else if (name.equals("parent")) { 2699 this.getParent().add(TypeConvertor.castToCanonical(value)); 2700 } else if (name.equals("event[x]")) { 2701 this.event = TypeConvertor.castToType(value); // DataType 2702 } else if (name.equals("category")) { 2703 value = new MessageSignificanceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2704 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2705 } else if (name.equals("focus")) { 2706 this.getFocus().add((MessageDefinitionFocusComponent) value); 2707 } else if (name.equals("responseRequired")) { 2708 value = new MessageheaderResponseRequestEnumFactory().fromType(TypeConvertor.castToCode(value)); 2709 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 2710 } else if (name.equals("allowedResponse")) { 2711 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); 2712 } else if (name.equals("graph")) { 2713 this.graph = TypeConvertor.castToCanonical(value); // CanonicalType 2714 } else 2715 return super.setProperty(name, value); 2716 return value; 2717 } 2718 2719 @Override 2720 public Base makeProperty(int hash, String name) throws FHIRException { 2721 switch (hash) { 2722 case 116079: return getUrlElement(); 2723 case -1618432855: return addIdentifier(); 2724 case 351608024: return getVersionElement(); 2725 case -115699031: return getVersionAlgorithm(); 2726 case 1508158071: return getVersionAlgorithm(); 2727 case 3373707: return getNameElement(); 2728 case 110371416: return getTitleElement(); 2729 case -430332865: return addReplacesElement(); 2730 case -892481550: return getStatusElement(); 2731 case -404562712: return getExperimentalElement(); 2732 case 3076014: return getDateElement(); 2733 case 1447404028: return getPublisherElement(); 2734 case 951526432: return addContact(); 2735 case -1724546052: return getDescriptionElement(); 2736 case -669707736: return addUseContext(); 2737 case -507075711: return addJurisdiction(); 2738 case -220463842: return getPurposeElement(); 2739 case 1522889671: return getCopyrightElement(); 2740 case 765157229: return getCopyrightLabelElement(); 2741 case 3016401: return getBaseElement(); 2742 case -995424086: return addParentElement(); 2743 case 278115238: return getEvent(); 2744 case 96891546: return getEvent(); 2745 case 50511102: return getCategoryElement(); 2746 case 97604824: return addFocus(); 2747 case 791597824: return getResponseRequiredElement(); 2748 case -1130933751: return addAllowedResponse(); 2749 case 98615630: return getGraphElement(); 2750 default: return super.makeProperty(hash, name); 2751 } 2752 2753 } 2754 2755 @Override 2756 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2757 switch (hash) { 2758 case 116079: /*url*/ return new String[] {"uri"}; 2759 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2760 case 351608024: /*version*/ return new String[] {"string"}; 2761 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2762 case 3373707: /*name*/ return new String[] {"string"}; 2763 case 110371416: /*title*/ return new String[] {"string"}; 2764 case -430332865: /*replaces*/ return new String[] {"canonical"}; 2765 case -892481550: /*status*/ return new String[] {"code"}; 2766 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2767 case 3076014: /*date*/ return new String[] {"dateTime"}; 2768 case 1447404028: /*publisher*/ return new String[] {"string"}; 2769 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2770 case -1724546052: /*description*/ return new String[] {"markdown"}; 2771 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2772 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2773 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2774 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2775 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2776 case 3016401: /*base*/ return new String[] {"canonical"}; 2777 case -995424086: /*parent*/ return new String[] {"canonical"}; 2778 case 96891546: /*event*/ return new String[] {"Coding", "uri"}; 2779 case 50511102: /*category*/ return new String[] {"code"}; 2780 case 97604824: /*focus*/ return new String[] {}; 2781 case 791597824: /*responseRequired*/ return new String[] {"code"}; 2782 case -1130933751: /*allowedResponse*/ return new String[] {}; 2783 case 98615630: /*graph*/ return new String[] {"canonical"}; 2784 default: return super.getTypesForProperty(hash, name); 2785 } 2786 2787 } 2788 2789 @Override 2790 public Base addChild(String name) throws FHIRException { 2791 if (name.equals("url")) { 2792 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.url"); 2793 } 2794 else if (name.equals("identifier")) { 2795 return addIdentifier(); 2796 } 2797 else if (name.equals("version")) { 2798 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.version"); 2799 } 2800 else if (name.equals("versionAlgorithmString")) { 2801 this.versionAlgorithm = new StringType(); 2802 return this.versionAlgorithm; 2803 } 2804 else if (name.equals("versionAlgorithmCoding")) { 2805 this.versionAlgorithm = new Coding(); 2806 return this.versionAlgorithm; 2807 } 2808 else if (name.equals("name")) { 2809 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.name"); 2810 } 2811 else if (name.equals("title")) { 2812 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.title"); 2813 } 2814 else if (name.equals("replaces")) { 2815 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.replaces"); 2816 } 2817 else if (name.equals("status")) { 2818 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.status"); 2819 } 2820 else if (name.equals("experimental")) { 2821 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.experimental"); 2822 } 2823 else if (name.equals("date")) { 2824 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.date"); 2825 } 2826 else if (name.equals("publisher")) { 2827 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.publisher"); 2828 } 2829 else if (name.equals("contact")) { 2830 return addContact(); 2831 } 2832 else if (name.equals("description")) { 2833 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.description"); 2834 } 2835 else if (name.equals("useContext")) { 2836 return addUseContext(); 2837 } 2838 else if (name.equals("jurisdiction")) { 2839 return addJurisdiction(); 2840 } 2841 else if (name.equals("purpose")) { 2842 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.purpose"); 2843 } 2844 else if (name.equals("copyright")) { 2845 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.copyright"); 2846 } 2847 else if (name.equals("copyrightLabel")) { 2848 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.copyrightLabel"); 2849 } 2850 else if (name.equals("base")) { 2851 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.base"); 2852 } 2853 else if (name.equals("parent")) { 2854 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.parent"); 2855 } 2856 else if (name.equals("eventCoding")) { 2857 this.event = new Coding(); 2858 return this.event; 2859 } 2860 else if (name.equals("eventUri")) { 2861 this.event = new UriType(); 2862 return this.event; 2863 } 2864 else if (name.equals("category")) { 2865 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.category"); 2866 } 2867 else if (name.equals("focus")) { 2868 return addFocus(); 2869 } 2870 else if (name.equals("responseRequired")) { 2871 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.responseRequired"); 2872 } 2873 else if (name.equals("allowedResponse")) { 2874 return addAllowedResponse(); 2875 } 2876 else if (name.equals("graph")) { 2877 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.graph"); 2878 } 2879 else 2880 return super.addChild(name); 2881 } 2882 2883 public String fhirType() { 2884 return "MessageDefinition"; 2885 2886 } 2887 2888 public MessageDefinition copy() { 2889 MessageDefinition dst = new MessageDefinition(); 2890 copyValues(dst); 2891 return dst; 2892 } 2893 2894 public void copyValues(MessageDefinition dst) { 2895 super.copyValues(dst); 2896 dst.url = url == null ? null : url.copy(); 2897 if (identifier != null) { 2898 dst.identifier = new ArrayList<Identifier>(); 2899 for (Identifier i : identifier) 2900 dst.identifier.add(i.copy()); 2901 }; 2902 dst.version = version == null ? null : version.copy(); 2903 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2904 dst.name = name == null ? null : name.copy(); 2905 dst.title = title == null ? null : title.copy(); 2906 if (replaces != null) { 2907 dst.replaces = new ArrayList<CanonicalType>(); 2908 for (CanonicalType i : replaces) 2909 dst.replaces.add(i.copy()); 2910 }; 2911 dst.status = status == null ? null : status.copy(); 2912 dst.experimental = experimental == null ? null : experimental.copy(); 2913 dst.date = date == null ? null : date.copy(); 2914 dst.publisher = publisher == null ? null : publisher.copy(); 2915 if (contact != null) { 2916 dst.contact = new ArrayList<ContactDetail>(); 2917 for (ContactDetail i : contact) 2918 dst.contact.add(i.copy()); 2919 }; 2920 dst.description = description == null ? null : description.copy(); 2921 if (useContext != null) { 2922 dst.useContext = new ArrayList<UsageContext>(); 2923 for (UsageContext i : useContext) 2924 dst.useContext.add(i.copy()); 2925 }; 2926 if (jurisdiction != null) { 2927 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2928 for (CodeableConcept i : jurisdiction) 2929 dst.jurisdiction.add(i.copy()); 2930 }; 2931 dst.purpose = purpose == null ? null : purpose.copy(); 2932 dst.copyright = copyright == null ? null : copyright.copy(); 2933 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2934 dst.base = base == null ? null : base.copy(); 2935 if (parent != null) { 2936 dst.parent = new ArrayList<CanonicalType>(); 2937 for (CanonicalType i : parent) 2938 dst.parent.add(i.copy()); 2939 }; 2940 dst.event = event == null ? null : event.copy(); 2941 dst.category = category == null ? null : category.copy(); 2942 if (focus != null) { 2943 dst.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2944 for (MessageDefinitionFocusComponent i : focus) 2945 dst.focus.add(i.copy()); 2946 }; 2947 dst.responseRequired = responseRequired == null ? null : responseRequired.copy(); 2948 if (allowedResponse != null) { 2949 dst.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2950 for (MessageDefinitionAllowedResponseComponent i : allowedResponse) 2951 dst.allowedResponse.add(i.copy()); 2952 }; 2953 dst.graph = graph == null ? null : graph.copy(); 2954 } 2955 2956 protected MessageDefinition typedCopy() { 2957 return copy(); 2958 } 2959 2960 @Override 2961 public boolean equalsDeep(Base other_) { 2962 if (!super.equalsDeep(other_)) 2963 return false; 2964 if (!(other_ instanceof MessageDefinition)) 2965 return false; 2966 MessageDefinition o = (MessageDefinition) other_; 2967 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2968 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2969 && compareDeep(replaces, o.replaces, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 2970 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 2971 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 2972 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 2973 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(base, o.base, true) && compareDeep(parent, o.parent, true) 2974 && compareDeep(event, o.event, true) && compareDeep(category, o.category, true) && compareDeep(focus, o.focus, true) 2975 && compareDeep(responseRequired, o.responseRequired, true) && compareDeep(allowedResponse, o.allowedResponse, true) 2976 && compareDeep(graph, o.graph, true); 2977 } 2978 2979 @Override 2980 public boolean equalsShallow(Base other_) { 2981 if (!super.equalsShallow(other_)) 2982 return false; 2983 if (!(other_ instanceof MessageDefinition)) 2984 return false; 2985 MessageDefinition o = (MessageDefinition) other_; 2986 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2987 && compareValues(title, o.title, true) && compareValues(replaces, o.replaces, true) && compareValues(status, o.status, true) 2988 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 2989 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 2990 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(base, o.base, true) && compareValues(parent, o.parent, true) 2991 && compareValues(category, o.category, true) && compareValues(responseRequired, o.responseRequired, true) 2992 && compareValues(graph, o.graph, true); 2993 } 2994 2995 public boolean isEmpty() { 2996 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2997 , versionAlgorithm, name, title, replaces, status, experimental, date, publisher 2998 , contact, description, useContext, jurisdiction, purpose, copyright, copyrightLabel 2999 , base, parent, event, category, focus, responseRequired, allowedResponse, graph 3000 ); 3001 } 3002 3003 @Override 3004 public ResourceType getResourceType() { 3005 return ResourceType.MessageDefinition; 3006 } 3007 3008 /** 3009 * Search parameter: <b>context-quantity</b> 3010 * <p> 3011 * Description: <b>Multiple Resources: 3012 3013* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3014* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3015* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3016* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3017* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3018* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3019* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3020* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3021* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3022* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3023* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3024* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3025* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3026* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3027* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3028* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3029* [Library](library.html): A quantity- or range-valued use context assigned to the library 3030* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3031* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3032* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3033* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3034* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3035* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3036* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3037* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3038* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3039* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3040* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3041* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3042* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3043</b><br> 3044 * Type: <b>quantity</b><br> 3045 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3046 * </p> 3047 */ 3048 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 3049 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3050 /** 3051 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3052 * <p> 3053 * Description: <b>Multiple Resources: 3054 3055* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3056* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3057* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3058* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3059* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3060* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3061* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3062* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3063* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3064* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3065* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3066* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3067* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3068* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3069* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3070* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3071* [Library](library.html): A quantity- or range-valued use context assigned to the library 3072* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3073* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3074* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3075* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3076* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3077* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3078* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3079* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3080* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3081* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3082* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3083* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3084* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3085</b><br> 3086 * Type: <b>quantity</b><br> 3087 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3088 * </p> 3089 */ 3090 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3091 3092 /** 3093 * Search parameter: <b>context-type-quantity</b> 3094 * <p> 3095 * Description: <b>Multiple Resources: 3096 3097* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3098* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3099* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3100* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3101* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3102* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3103* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3104* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3105* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3106* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3107* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3108* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3109* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3110* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3111* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3112* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3113* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3114* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3115* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3116* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3117* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3118* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3119* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3120* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3121* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3122* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3123* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3124* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3125* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3126* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3127</b><br> 3128 * Type: <b>composite</b><br> 3129 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3130 * </p> 3131 */ 3132 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3133 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3134 /** 3135 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3136 * <p> 3137 * Description: <b>Multiple Resources: 3138 3139* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3140* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3141* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3142* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3143* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3144* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3145* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3146* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3147* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3148* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3149* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3150* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3151* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3152* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3153* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3154* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3155* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3156* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3157* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3158* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3159* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3160* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3161* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3162* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3163* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3164* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3165* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3166* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3167* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3168* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3169</b><br> 3170 * Type: <b>composite</b><br> 3171 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3172 * </p> 3173 */ 3174 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3175 3176 /** 3177 * Search parameter: <b>context-type-value</b> 3178 * <p> 3179 * Description: <b>Multiple Resources: 3180 3181* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3182* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3183* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3184* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3185* [Citation](citation.html): A use context type and value assigned to the citation 3186* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3187* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3188* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3189* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3190* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3191* [Evidence](evidence.html): A use context type and value assigned to the evidence 3192* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3193* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3194* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3195* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3196* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3197* [Library](library.html): A use context type and value assigned to the library 3198* [Measure](measure.html): A use context type and value assigned to the measure 3199* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3200* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3201* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3202* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3203* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3204* [Requirements](requirements.html): A use context type and value assigned to the requirements 3205* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3206* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3207* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3208* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3209* [TestScript](testscript.html): A use context type and value assigned to the test script 3210* [ValueSet](valueset.html): A use context type and value assigned to the value set 3211</b><br> 3212 * Type: <b>composite</b><br> 3213 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3214 * </p> 3215 */ 3216 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3217 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3218 /** 3219 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3220 * <p> 3221 * Description: <b>Multiple Resources: 3222 3223* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3224* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3225* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3226* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3227* [Citation](citation.html): A use context type and value assigned to the citation 3228* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3229* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3230* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3231* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3232* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3233* [Evidence](evidence.html): A use context type and value assigned to the evidence 3234* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3235* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3236* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3237* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3238* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3239* [Library](library.html): A use context type and value assigned to the library 3240* [Measure](measure.html): A use context type and value assigned to the measure 3241* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3242* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3243* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3244* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3245* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3246* [Requirements](requirements.html): A use context type and value assigned to the requirements 3247* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3248* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3249* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3250* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3251* [TestScript](testscript.html): A use context type and value assigned to the test script 3252* [ValueSet](valueset.html): A use context type and value assigned to the value set 3253</b><br> 3254 * Type: <b>composite</b><br> 3255 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3256 * </p> 3257 */ 3258 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3259 3260 /** 3261 * Search parameter: <b>context-type</b> 3262 * <p> 3263 * Description: <b>Multiple Resources: 3264 3265* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3266* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3267* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3268* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3269* [Citation](citation.html): A type of use context assigned to the citation 3270* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3271* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3272* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3273* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3274* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3275* [Evidence](evidence.html): A type of use context assigned to the evidence 3276* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3277* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3278* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3279* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3280* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3281* [Library](library.html): A type of use context assigned to the library 3282* [Measure](measure.html): A type of use context assigned to the measure 3283* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3284* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3285* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3286* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3287* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3288* [Requirements](requirements.html): A type of use context assigned to the requirements 3289* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3290* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3291* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3292* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3293* [TestScript](testscript.html): A type of use context assigned to the test script 3294* [ValueSet](valueset.html): A type of use context assigned to the value set 3295</b><br> 3296 * Type: <b>token</b><br> 3297 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3298 * </p> 3299 */ 3300 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3301 public static final String SP_CONTEXT_TYPE = "context-type"; 3302 /** 3303 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3304 * <p> 3305 * Description: <b>Multiple Resources: 3306 3307* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3308* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3309* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3310* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3311* [Citation](citation.html): A type of use context assigned to the citation 3312* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3313* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3314* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3315* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3316* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3317* [Evidence](evidence.html): A type of use context assigned to the evidence 3318* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3319* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3320* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3321* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3322* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3323* [Library](library.html): A type of use context assigned to the library 3324* [Measure](measure.html): A type of use context assigned to the measure 3325* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3326* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3327* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3328* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3329* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3330* [Requirements](requirements.html): A type of use context assigned to the requirements 3331* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3332* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3333* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3334* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3335* [TestScript](testscript.html): A type of use context assigned to the test script 3336* [ValueSet](valueset.html): A type of use context assigned to the value set 3337</b><br> 3338 * Type: <b>token</b><br> 3339 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3340 * </p> 3341 */ 3342 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3343 3344 /** 3345 * Search parameter: <b>context</b> 3346 * <p> 3347 * Description: <b>Multiple Resources: 3348 3349* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3350* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3351* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3352* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3353* [Citation](citation.html): A use context assigned to the citation 3354* [CodeSystem](codesystem.html): A use context assigned to the code system 3355* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3356* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3357* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3358* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3359* [Evidence](evidence.html): A use context assigned to the evidence 3360* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3361* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3362* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3363* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3364* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3365* [Library](library.html): A use context assigned to the library 3366* [Measure](measure.html): A use context assigned to the measure 3367* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3368* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3369* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3370* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3371* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3372* [Requirements](requirements.html): A use context assigned to the requirements 3373* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3374* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3375* [StructureMap](structuremap.html): A use context assigned to the structure map 3376* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3377* [TestScript](testscript.html): A use context assigned to the test script 3378* [ValueSet](valueset.html): A use context assigned to the value set 3379</b><br> 3380 * Type: <b>token</b><br> 3381 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3382 * </p> 3383 */ 3384 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3385 public static final String SP_CONTEXT = "context"; 3386 /** 3387 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3388 * <p> 3389 * Description: <b>Multiple Resources: 3390 3391* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3392* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3393* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3394* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3395* [Citation](citation.html): A use context assigned to the citation 3396* [CodeSystem](codesystem.html): A use context assigned to the code system 3397* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3398* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3399* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3400* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3401* [Evidence](evidence.html): A use context assigned to the evidence 3402* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3403* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3404* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3405* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3406* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3407* [Library](library.html): A use context assigned to the library 3408* [Measure](measure.html): A use context assigned to the measure 3409* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3410* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3411* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3412* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3413* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3414* [Requirements](requirements.html): A use context assigned to the requirements 3415* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3416* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3417* [StructureMap](structuremap.html): A use context assigned to the structure map 3418* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3419* [TestScript](testscript.html): A use context assigned to the test script 3420* [ValueSet](valueset.html): A use context assigned to the value set 3421</b><br> 3422 * Type: <b>token</b><br> 3423 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3424 * </p> 3425 */ 3426 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3427 3428 /** 3429 * Search parameter: <b>date</b> 3430 * <p> 3431 * Description: <b>Multiple Resources: 3432 3433* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3434* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3435* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3436* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3437* [Citation](citation.html): The citation publication date 3438* [CodeSystem](codesystem.html): The code system publication date 3439* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3440* [ConceptMap](conceptmap.html): The concept map publication date 3441* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3442* [EventDefinition](eventdefinition.html): The event definition publication date 3443* [Evidence](evidence.html): The evidence publication date 3444* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3445* [ExampleScenario](examplescenario.html): The example scenario publication date 3446* [GraphDefinition](graphdefinition.html): The graph definition publication date 3447* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3448* [Library](library.html): The library publication date 3449* [Measure](measure.html): The measure publication date 3450* [MessageDefinition](messagedefinition.html): The message definition publication date 3451* [NamingSystem](namingsystem.html): The naming system publication date 3452* [OperationDefinition](operationdefinition.html): The operation definition publication date 3453* [PlanDefinition](plandefinition.html): The plan definition publication date 3454* [Questionnaire](questionnaire.html): The questionnaire publication date 3455* [Requirements](requirements.html): The requirements publication date 3456* [SearchParameter](searchparameter.html): The search parameter publication date 3457* [StructureDefinition](structuredefinition.html): The structure definition publication date 3458* [StructureMap](structuremap.html): The structure map publication date 3459* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3460* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3461* [TestScript](testscript.html): The test script publication date 3462* [ValueSet](valueset.html): The value set publication date 3463</b><br> 3464 * Type: <b>date</b><br> 3465 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3466 * </p> 3467 */ 3468 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3469 public static final String SP_DATE = "date"; 3470 /** 3471 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3472 * <p> 3473 * Description: <b>Multiple Resources: 3474 3475* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3476* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3477* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3478* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3479* [Citation](citation.html): The citation publication date 3480* [CodeSystem](codesystem.html): The code system publication date 3481* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3482* [ConceptMap](conceptmap.html): The concept map publication date 3483* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3484* [EventDefinition](eventdefinition.html): The event definition publication date 3485* [Evidence](evidence.html): The evidence publication date 3486* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3487* [ExampleScenario](examplescenario.html): The example scenario publication date 3488* [GraphDefinition](graphdefinition.html): The graph definition publication date 3489* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3490* [Library](library.html): The library publication date 3491* [Measure](measure.html): The measure publication date 3492* [MessageDefinition](messagedefinition.html): The message definition publication date 3493* [NamingSystem](namingsystem.html): The naming system publication date 3494* [OperationDefinition](operationdefinition.html): The operation definition publication date 3495* [PlanDefinition](plandefinition.html): The plan definition publication date 3496* [Questionnaire](questionnaire.html): The questionnaire publication date 3497* [Requirements](requirements.html): The requirements publication date 3498* [SearchParameter](searchparameter.html): The search parameter publication date 3499* [StructureDefinition](structuredefinition.html): The structure definition publication date 3500* [StructureMap](structuremap.html): The structure map publication date 3501* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3502* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3503* [TestScript](testscript.html): The test script publication date 3504* [ValueSet](valueset.html): The value set publication date 3505</b><br> 3506 * Type: <b>date</b><br> 3507 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3508 * </p> 3509 */ 3510 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3511 3512 /** 3513 * Search parameter: <b>description</b> 3514 * <p> 3515 * Description: <b>Multiple Resources: 3516 3517* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3518* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3519* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3520* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3521* [Citation](citation.html): The description of the citation 3522* [CodeSystem](codesystem.html): The description of the code system 3523* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3524* [ConceptMap](conceptmap.html): The description of the concept map 3525* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3526* [EventDefinition](eventdefinition.html): The description of the event definition 3527* [Evidence](evidence.html): The description of the evidence 3528* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3529* [GraphDefinition](graphdefinition.html): The description of the graph definition 3530* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3531* [Library](library.html): The description of the library 3532* [Measure](measure.html): The description of the measure 3533* [MessageDefinition](messagedefinition.html): The description of the message definition 3534* [NamingSystem](namingsystem.html): The description of the naming system 3535* [OperationDefinition](operationdefinition.html): The description of the operation definition 3536* [PlanDefinition](plandefinition.html): The description of the plan definition 3537* [Questionnaire](questionnaire.html): The description of the questionnaire 3538* [Requirements](requirements.html): The description of the requirements 3539* [SearchParameter](searchparameter.html): The description of the search parameter 3540* [StructureDefinition](structuredefinition.html): The description of the structure definition 3541* [StructureMap](structuremap.html): The description of the structure map 3542* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3543* [TestScript](testscript.html): The description of the test script 3544* [ValueSet](valueset.html): The description of the value set 3545</b><br> 3546 * Type: <b>string</b><br> 3547 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3548 * </p> 3549 */ 3550 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3551 public static final String SP_DESCRIPTION = "description"; 3552 /** 3553 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3554 * <p> 3555 * Description: <b>Multiple Resources: 3556 3557* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3558* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3559* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3560* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3561* [Citation](citation.html): The description of the citation 3562* [CodeSystem](codesystem.html): The description of the code system 3563* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3564* [ConceptMap](conceptmap.html): The description of the concept map 3565* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3566* [EventDefinition](eventdefinition.html): The description of the event definition 3567* [Evidence](evidence.html): The description of the evidence 3568* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3569* [GraphDefinition](graphdefinition.html): The description of the graph definition 3570* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3571* [Library](library.html): The description of the library 3572* [Measure](measure.html): The description of the measure 3573* [MessageDefinition](messagedefinition.html): The description of the message definition 3574* [NamingSystem](namingsystem.html): The description of the naming system 3575* [OperationDefinition](operationdefinition.html): The description of the operation definition 3576* [PlanDefinition](plandefinition.html): The description of the plan definition 3577* [Questionnaire](questionnaire.html): The description of the questionnaire 3578* [Requirements](requirements.html): The description of the requirements 3579* [SearchParameter](searchparameter.html): The description of the search parameter 3580* [StructureDefinition](structuredefinition.html): The description of the structure definition 3581* [StructureMap](structuremap.html): The description of the structure map 3582* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3583* [TestScript](testscript.html): The description of the test script 3584* [ValueSet](valueset.html): The description of the value set 3585</b><br> 3586 * Type: <b>string</b><br> 3587 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3588 * </p> 3589 */ 3590 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3591 3592 /** 3593 * Search parameter: <b>identifier</b> 3594 * <p> 3595 * Description: <b>Multiple Resources: 3596 3597* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3598* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3599* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3600* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3601* [Citation](citation.html): External identifier for the citation 3602* [CodeSystem](codesystem.html): External identifier for the code system 3603* [ConceptMap](conceptmap.html): External identifier for the concept map 3604* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3605* [EventDefinition](eventdefinition.html): External identifier for the event definition 3606* [Evidence](evidence.html): External identifier for the evidence 3607* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3608* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3609* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3610* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3611* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3612* [Library](library.html): External identifier for the library 3613* [Measure](measure.html): External identifier for the measure 3614* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3615* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3616* [NamingSystem](namingsystem.html): External identifier for the naming system 3617* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3618* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3619* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3620* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3621* [Requirements](requirements.html): External identifier for the requirements 3622* [SearchParameter](searchparameter.html): External identifier for the search parameter 3623* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3624* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3625* [StructureMap](structuremap.html): External identifier for the structure map 3626* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3627* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3628* [TestPlan](testplan.html): An identifier for the test plan 3629* [TestScript](testscript.html): External identifier for the test script 3630* [ValueSet](valueset.html): External identifier for the value set 3631</b><br> 3632 * Type: <b>token</b><br> 3633 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3634 * </p> 3635 */ 3636 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3637 public static final String SP_IDENTIFIER = "identifier"; 3638 /** 3639 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3640 * <p> 3641 * Description: <b>Multiple Resources: 3642 3643* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3644* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3645* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3646* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3647* [Citation](citation.html): External identifier for the citation 3648* [CodeSystem](codesystem.html): External identifier for the code system 3649* [ConceptMap](conceptmap.html): External identifier for the concept map 3650* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3651* [EventDefinition](eventdefinition.html): External identifier for the event definition 3652* [Evidence](evidence.html): External identifier for the evidence 3653* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3654* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3655* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3656* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3657* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3658* [Library](library.html): External identifier for the library 3659* [Measure](measure.html): External identifier for the measure 3660* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3661* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3662* [NamingSystem](namingsystem.html): External identifier for the naming system 3663* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3664* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3665* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3666* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3667* [Requirements](requirements.html): External identifier for the requirements 3668* [SearchParameter](searchparameter.html): External identifier for the search parameter 3669* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3670* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3671* [StructureMap](structuremap.html): External identifier for the structure map 3672* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3673* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3674* [TestPlan](testplan.html): An identifier for the test plan 3675* [TestScript](testscript.html): External identifier for the test script 3676* [ValueSet](valueset.html): External identifier for the value set 3677</b><br> 3678 * Type: <b>token</b><br> 3679 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3680 * </p> 3681 */ 3682 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3683 3684 /** 3685 * Search parameter: <b>jurisdiction</b> 3686 * <p> 3687 * Description: <b>Multiple Resources: 3688 3689* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3690* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3691* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3692* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3693* [Citation](citation.html): Intended jurisdiction for the citation 3694* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3695* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3696* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3697* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3698* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3699* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3700* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3701* [Library](library.html): Intended jurisdiction for the library 3702* [Measure](measure.html): Intended jurisdiction for the measure 3703* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3704* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3705* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3706* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3707* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3708* [Requirements](requirements.html): Intended jurisdiction for the requirements 3709* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3710* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3711* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3712* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3713* [TestScript](testscript.html): Intended jurisdiction for the test script 3714* [ValueSet](valueset.html): Intended jurisdiction for the value set 3715</b><br> 3716 * Type: <b>token</b><br> 3717 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3718 * </p> 3719 */ 3720 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3721 public static final String SP_JURISDICTION = "jurisdiction"; 3722 /** 3723 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3724 * <p> 3725 * Description: <b>Multiple Resources: 3726 3727* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3728* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3729* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3730* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3731* [Citation](citation.html): Intended jurisdiction for the citation 3732* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3733* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3734* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3735* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3736* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3737* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3738* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3739* [Library](library.html): Intended jurisdiction for the library 3740* [Measure](measure.html): Intended jurisdiction for the measure 3741* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3742* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3743* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3744* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3745* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3746* [Requirements](requirements.html): Intended jurisdiction for the requirements 3747* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3748* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3749* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3750* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3751* [TestScript](testscript.html): Intended jurisdiction for the test script 3752* [ValueSet](valueset.html): Intended jurisdiction for the value set 3753</b><br> 3754 * Type: <b>token</b><br> 3755 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3756 * </p> 3757 */ 3758 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3759 3760 /** 3761 * Search parameter: <b>name</b> 3762 * <p> 3763 * Description: <b>Multiple Resources: 3764 3765* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3766* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3767* [Citation](citation.html): Computationally friendly name of the citation 3768* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3769* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3770* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3771* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3772* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3773* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3774* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3775* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3776* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3777* [Library](library.html): Computationally friendly name of the library 3778* [Measure](measure.html): Computationally friendly name of the measure 3779* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3780* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3781* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3782* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3783* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3784* [Requirements](requirements.html): Computationally friendly name of the requirements 3785* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3786* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3787* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3788* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3789* [TestScript](testscript.html): Computationally friendly name of the test script 3790* [ValueSet](valueset.html): Computationally friendly name of the value set 3791</b><br> 3792 * Type: <b>string</b><br> 3793 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3794 * </p> 3795 */ 3796 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3797 public static final String SP_NAME = "name"; 3798 /** 3799 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3800 * <p> 3801 * Description: <b>Multiple Resources: 3802 3803* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3804* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3805* [Citation](citation.html): Computationally friendly name of the citation 3806* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3807* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3808* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3809* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3810* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3811* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3812* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3813* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3814* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3815* [Library](library.html): Computationally friendly name of the library 3816* [Measure](measure.html): Computationally friendly name of the measure 3817* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3818* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3819* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3820* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3821* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3822* [Requirements](requirements.html): Computationally friendly name of the requirements 3823* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3824* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3825* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3826* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3827* [TestScript](testscript.html): Computationally friendly name of the test script 3828* [ValueSet](valueset.html): Computationally friendly name of the value set 3829</b><br> 3830 * Type: <b>string</b><br> 3831 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3832 * </p> 3833 */ 3834 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3835 3836 /** 3837 * Search parameter: <b>publisher</b> 3838 * <p> 3839 * Description: <b>Multiple Resources: 3840 3841* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3842* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3843* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3844* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3845* [Citation](citation.html): Name of the publisher of the citation 3846* [CodeSystem](codesystem.html): Name of the publisher of the code system 3847* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3848* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3849* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3850* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3851* [Evidence](evidence.html): Name of the publisher of the evidence 3852* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3853* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3854* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3855* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3856* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3857* [Library](library.html): Name of the publisher of the library 3858* [Measure](measure.html): Name of the publisher of the measure 3859* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3860* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3861* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3862* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3863* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3864* [Requirements](requirements.html): Name of the publisher of the requirements 3865* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3866* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3867* [StructureMap](structuremap.html): Name of the publisher of the structure map 3868* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3869* [TestScript](testscript.html): Name of the publisher of the test script 3870* [ValueSet](valueset.html): Name of the publisher of the value set 3871</b><br> 3872 * Type: <b>string</b><br> 3873 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3874 * </p> 3875 */ 3876 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3877 public static final String SP_PUBLISHER = "publisher"; 3878 /** 3879 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3880 * <p> 3881 * Description: <b>Multiple Resources: 3882 3883* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3884* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3885* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3886* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3887* [Citation](citation.html): Name of the publisher of the citation 3888* [CodeSystem](codesystem.html): Name of the publisher of the code system 3889* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3890* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3891* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3892* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3893* [Evidence](evidence.html): Name of the publisher of the evidence 3894* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3895* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3896* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3897* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3898* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3899* [Library](library.html): Name of the publisher of the library 3900* [Measure](measure.html): Name of the publisher of the measure 3901* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3902* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3903* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3904* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3905* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3906* [Requirements](requirements.html): Name of the publisher of the requirements 3907* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3908* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3909* [StructureMap](structuremap.html): Name of the publisher of the structure map 3910* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3911* [TestScript](testscript.html): Name of the publisher of the test script 3912* [ValueSet](valueset.html): Name of the publisher of the value set 3913</b><br> 3914 * Type: <b>string</b><br> 3915 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3916 * </p> 3917 */ 3918 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3919 3920 /** 3921 * Search parameter: <b>status</b> 3922 * <p> 3923 * Description: <b>Multiple Resources: 3924 3925* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3926* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3927* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3928* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3929* [Citation](citation.html): The current status of the citation 3930* [CodeSystem](codesystem.html): The current status of the code system 3931* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3932* [ConceptMap](conceptmap.html): The current status of the concept map 3933* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3934* [EventDefinition](eventdefinition.html): The current status of the event definition 3935* [Evidence](evidence.html): The current status of the evidence 3936* [EvidenceReport](evidencereport.html): The current status of the evidence report 3937* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3938* [ExampleScenario](examplescenario.html): The current status of the example scenario 3939* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3940* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3941* [Library](library.html): The current status of the library 3942* [Measure](measure.html): The current status of the measure 3943* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3944* [MessageDefinition](messagedefinition.html): The current status of the message definition 3945* [NamingSystem](namingsystem.html): The current status of the naming system 3946* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3947* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3948* [PlanDefinition](plandefinition.html): The current status of the plan definition 3949* [Questionnaire](questionnaire.html): The current status of the questionnaire 3950* [Requirements](requirements.html): The current status of the requirements 3951* [SearchParameter](searchparameter.html): The current status of the search parameter 3952* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3953* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3954* [StructureMap](structuremap.html): The current status of the structure map 3955* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3956* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3957* [TestPlan](testplan.html): The current status of the test plan 3958* [TestScript](testscript.html): The current status of the test script 3959* [ValueSet](valueset.html): The current status of the value set 3960</b><br> 3961 * Type: <b>token</b><br> 3962 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3963 * </p> 3964 */ 3965 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3966 public static final String SP_STATUS = "status"; 3967 /** 3968 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3969 * <p> 3970 * Description: <b>Multiple Resources: 3971 3972* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3973* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3974* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3975* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3976* [Citation](citation.html): The current status of the citation 3977* [CodeSystem](codesystem.html): The current status of the code system 3978* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3979* [ConceptMap](conceptmap.html): The current status of the concept map 3980* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3981* [EventDefinition](eventdefinition.html): The current status of the event definition 3982* [Evidence](evidence.html): The current status of the evidence 3983* [EvidenceReport](evidencereport.html): The current status of the evidence report 3984* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3985* [ExampleScenario](examplescenario.html): The current status of the example scenario 3986* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3987* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3988* [Library](library.html): The current status of the library 3989* [Measure](measure.html): The current status of the measure 3990* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3991* [MessageDefinition](messagedefinition.html): The current status of the message definition 3992* [NamingSystem](namingsystem.html): The current status of the naming system 3993* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3994* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3995* [PlanDefinition](plandefinition.html): The current status of the plan definition 3996* [Questionnaire](questionnaire.html): The current status of the questionnaire 3997* [Requirements](requirements.html): The current status of the requirements 3998* [SearchParameter](searchparameter.html): The current status of the search parameter 3999* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4000* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4001* [StructureMap](structuremap.html): The current status of the structure map 4002* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4003* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4004* [TestPlan](testplan.html): The current status of the test plan 4005* [TestScript](testscript.html): The current status of the test script 4006* [ValueSet](valueset.html): The current status of the value set 4007</b><br> 4008 * Type: <b>token</b><br> 4009 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4010 * </p> 4011 */ 4012 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4013 4014 /** 4015 * Search parameter: <b>title</b> 4016 * <p> 4017 * Description: <b>Multiple Resources: 4018 4019* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4020* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4021* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4022* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4023* [Citation](citation.html): The human-friendly name of the citation 4024* [CodeSystem](codesystem.html): The human-friendly name of the code system 4025* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4026* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4027* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4028* [Evidence](evidence.html): The human-friendly name of the evidence 4029* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4030* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4031* [Library](library.html): The human-friendly name of the library 4032* [Measure](measure.html): The human-friendly name of the measure 4033* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4034* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4035* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4036* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4037* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4038* [Requirements](requirements.html): The human-friendly name of the requirements 4039* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4040* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4041* [StructureMap](structuremap.html): The human-friendly name of the structure map 4042* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4043* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4044* [TestScript](testscript.html): The human-friendly name of the test script 4045* [ValueSet](valueset.html): The human-friendly name of the value set 4046</b><br> 4047 * Type: <b>string</b><br> 4048 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4049 * </p> 4050 */ 4051 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4052 public static final String SP_TITLE = "title"; 4053 /** 4054 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4055 * <p> 4056 * Description: <b>Multiple Resources: 4057 4058* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4059* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4060* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4061* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4062* [Citation](citation.html): The human-friendly name of the citation 4063* [CodeSystem](codesystem.html): The human-friendly name of the code system 4064* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4065* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4066* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4067* [Evidence](evidence.html): The human-friendly name of the evidence 4068* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4069* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4070* [Library](library.html): The human-friendly name of the library 4071* [Measure](measure.html): The human-friendly name of the measure 4072* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4073* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4074* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4075* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4076* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4077* [Requirements](requirements.html): The human-friendly name of the requirements 4078* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4079* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4080* [StructureMap](structuremap.html): The human-friendly name of the structure map 4081* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4082* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4083* [TestScript](testscript.html): The human-friendly name of the test script 4084* [ValueSet](valueset.html): The human-friendly name of the value set 4085</b><br> 4086 * Type: <b>string</b><br> 4087 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4088 * </p> 4089 */ 4090 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4091 4092 /** 4093 * Search parameter: <b>url</b> 4094 * <p> 4095 * Description: <b>Multiple Resources: 4096 4097* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4098* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4099* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4100* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4101* [Citation](citation.html): The uri that identifies the citation 4102* [CodeSystem](codesystem.html): The uri that identifies the code system 4103* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4104* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4105* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4106* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4107* [Evidence](evidence.html): The uri that identifies the evidence 4108* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4109* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4110* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4111* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4112* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4113* [Library](library.html): The uri that identifies the library 4114* [Measure](measure.html): The uri that identifies the measure 4115* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4116* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4117* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4118* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4119* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4120* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4121* [Requirements](requirements.html): The uri that identifies the requirements 4122* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4123* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4124* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4125* [StructureMap](structuremap.html): The uri that identifies the structure map 4126* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4127* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4128* [TestPlan](testplan.html): The uri that identifies the test plan 4129* [TestScript](testscript.html): The uri that identifies the test script 4130* [ValueSet](valueset.html): The uri that identifies the value set 4131</b><br> 4132 * Type: <b>uri</b><br> 4133 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4134 * </p> 4135 */ 4136 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4137 public static final String SP_URL = "url"; 4138 /** 4139 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4140 * <p> 4141 * Description: <b>Multiple Resources: 4142 4143* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4144* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4145* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4146* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4147* [Citation](citation.html): The uri that identifies the citation 4148* [CodeSystem](codesystem.html): The uri that identifies the code system 4149* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4150* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4151* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4152* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4153* [Evidence](evidence.html): The uri that identifies the evidence 4154* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4155* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4156* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4157* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4158* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4159* [Library](library.html): The uri that identifies the library 4160* [Measure](measure.html): The uri that identifies the measure 4161* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4162* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4163* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4164* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4165* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4166* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4167* [Requirements](requirements.html): The uri that identifies the requirements 4168* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4169* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4170* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4171* [StructureMap](structuremap.html): The uri that identifies the structure map 4172* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4173* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4174* [TestPlan](testplan.html): The uri that identifies the test plan 4175* [TestScript](testscript.html): The uri that identifies the test script 4176* [ValueSet](valueset.html): The uri that identifies the value set 4177</b><br> 4178 * Type: <b>uri</b><br> 4179 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4180 * </p> 4181 */ 4182 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4183 4184 /** 4185 * Search parameter: <b>version</b> 4186 * <p> 4187 * Description: <b>Multiple Resources: 4188 4189* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4190* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4191* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4192* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4193* [Citation](citation.html): The business version of the citation 4194* [CodeSystem](codesystem.html): The business version of the code system 4195* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4196* [ConceptMap](conceptmap.html): The business version of the concept map 4197* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4198* [EventDefinition](eventdefinition.html): The business version of the event definition 4199* [Evidence](evidence.html): The business version of the evidence 4200* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4201* [ExampleScenario](examplescenario.html): The business version of the example scenario 4202* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4203* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4204* [Library](library.html): The business version of the library 4205* [Measure](measure.html): The business version of the measure 4206* [MessageDefinition](messagedefinition.html): The business version of the message definition 4207* [NamingSystem](namingsystem.html): The business version of the naming system 4208* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4209* [PlanDefinition](plandefinition.html): The business version of the plan definition 4210* [Questionnaire](questionnaire.html): The business version of the questionnaire 4211* [Requirements](requirements.html): The business version of the requirements 4212* [SearchParameter](searchparameter.html): The business version of the search parameter 4213* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4214* [StructureMap](structuremap.html): The business version of the structure map 4215* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4216* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4217* [TestScript](testscript.html): The business version of the test script 4218* [ValueSet](valueset.html): The business version of the value set 4219</b><br> 4220 * Type: <b>token</b><br> 4221 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4222 * </p> 4223 */ 4224 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4225 public static final String SP_VERSION = "version"; 4226 /** 4227 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4228 * <p> 4229 * Description: <b>Multiple Resources: 4230 4231* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4232* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4233* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4234* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4235* [Citation](citation.html): The business version of the citation 4236* [CodeSystem](codesystem.html): The business version of the code system 4237* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4238* [ConceptMap](conceptmap.html): The business version of the concept map 4239* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4240* [EventDefinition](eventdefinition.html): The business version of the event definition 4241* [Evidence](evidence.html): The business version of the evidence 4242* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4243* [ExampleScenario](examplescenario.html): The business version of the example scenario 4244* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4245* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4246* [Library](library.html): The business version of the library 4247* [Measure](measure.html): The business version of the measure 4248* [MessageDefinition](messagedefinition.html): The business version of the message definition 4249* [NamingSystem](namingsystem.html): The business version of the naming system 4250* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4251* [PlanDefinition](plandefinition.html): The business version of the plan definition 4252* [Questionnaire](questionnaire.html): The business version of the questionnaire 4253* [Requirements](requirements.html): The business version of the requirements 4254* [SearchParameter](searchparameter.html): The business version of the search parameter 4255* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4256* [StructureMap](structuremap.html): The business version of the structure map 4257* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4258* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4259* [TestScript](testscript.html): The business version of the test script 4260* [ValueSet](valueset.html): The business version of the value set 4261</b><br> 4262 * Type: <b>token</b><br> 4263 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4264 * </p> 4265 */ 4266 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4267 4268 /** 4269 * Search parameter: <b>category</b> 4270 * <p> 4271 * Description: <b>The behavior associated with the message</b><br> 4272 * Type: <b>token</b><br> 4273 * Path: <b>MessageDefinition.category</b><br> 4274 * </p> 4275 */ 4276 @SearchParamDefinition(name="category", path="MessageDefinition.category", description="The behavior associated with the message", type="token" ) 4277 public static final String SP_CATEGORY = "category"; 4278 /** 4279 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4280 * <p> 4281 * Description: <b>The behavior associated with the message</b><br> 4282 * Type: <b>token</b><br> 4283 * Path: <b>MessageDefinition.category</b><br> 4284 * </p> 4285 */ 4286 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4287 4288 /** 4289 * Search parameter: <b>event</b> 4290 * <p> 4291 * Description: <b>The event that triggers the message or link to the event definition.</b><br> 4292 * Type: <b>token</b><br> 4293 * Path: <b>MessageDefinition.event.ofType(Coding)</b><br> 4294 * </p> 4295 */ 4296 @SearchParamDefinition(name="event", path="MessageDefinition.event.ofType(Coding)", description="The event that triggers the message or link to the event definition.", type="token" ) 4297 public static final String SP_EVENT = "event"; 4298 /** 4299 * <b>Fluent Client</b> search parameter constant for <b>event</b> 4300 * <p> 4301 * Description: <b>The event that triggers the message or link to the event definition.</b><br> 4302 * Type: <b>token</b><br> 4303 * Path: <b>MessageDefinition.event.ofType(Coding)</b><br> 4304 * </p> 4305 */ 4306 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 4307 4308 /** 4309 * Search parameter: <b>focus</b> 4310 * <p> 4311 * Description: <b>A resource that is a permitted focus of the message</b><br> 4312 * Type: <b>token</b><br> 4313 * Path: <b>MessageDefinition.focus.code</b><br> 4314 * </p> 4315 */ 4316 @SearchParamDefinition(name="focus", path="MessageDefinition.focus.code", description="A resource that is a permitted focus of the message", type="token" ) 4317 public static final String SP_FOCUS = "focus"; 4318 /** 4319 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 4320 * <p> 4321 * Description: <b>A resource that is a permitted focus of the message</b><br> 4322 * Type: <b>token</b><br> 4323 * Path: <b>MessageDefinition.focus.code</b><br> 4324 * </p> 4325 */ 4326 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FOCUS); 4327 4328 /** 4329 * Search parameter: <b>parent</b> 4330 * <p> 4331 * Description: <b>A resource that is the parent of the definition</b><br> 4332 * Type: <b>reference</b><br> 4333 * Path: <b>MessageDefinition.parent</b><br> 4334 * </p> 4335 */ 4336 @SearchParamDefinition(name="parent", path="MessageDefinition.parent", description="A resource that is the parent of the definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 4337 public static final String SP_PARENT = "parent"; 4338 /** 4339 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 4340 * <p> 4341 * Description: <b>A resource that is the parent of the definition</b><br> 4342 * Type: <b>reference</b><br> 4343 * Path: <b>MessageDefinition.parent</b><br> 4344 * </p> 4345 */ 4346 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 4347 4348/** 4349 * Constant for fluent queries to be used to add include statements. Specifies 4350 * the path value of "<b>MessageDefinition:parent</b>". 4351 */ 4352 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("MessageDefinition:parent").toLocked(); 4353 4354 4355} 4356