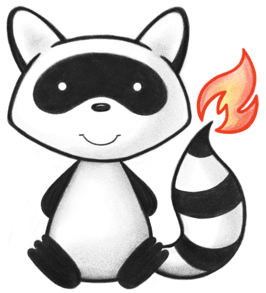
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 052 */ 053@ResourceDef(name="MessageHeader", profile="http://hl7.org/fhir/StructureDefinition/MessageHeader") 054public class MessageHeader extends DomainResource { 055 056 public enum ResponseType { 057 /** 058 * The message was accepted and processed without error. 059 */ 060 OK, 061 /** 062 * Some internal unexpected error occurred - wait and try again. Note - this is usually used for things like database unavailable, which may be expected to resolve, though human intervention may be required. 063 */ 064 TRANSIENTERROR, 065 /** 066 * The message was rejected because of a problem with the content. There is no point in re-sending without change. The response narrative SHALL describe the issue. 067 */ 068 FATALERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static ResponseType fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("ok".equals(codeString)) 077 return OK; 078 if ("transient-error".equals(codeString)) 079 return TRANSIENTERROR; 080 if ("fatal-error".equals(codeString)) 081 return FATALERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown ResponseType code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case OK: return "ok"; 090 case TRANSIENTERROR: return "transient-error"; 091 case FATALERROR: return "fatal-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case OK: return "http://hl7.org/fhir/response-code"; 099 case TRANSIENTERROR: return "http://hl7.org/fhir/response-code"; 100 case FATALERROR: return "http://hl7.org/fhir/response-code"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case OK: return "The message was accepted and processed without error."; 108 case TRANSIENTERROR: return "Some internal unexpected error occurred - wait and try again. Note - this is usually used for things like database unavailable, which may be expected to resolve, though human intervention may be required."; 109 case FATALERROR: return "The message was rejected because of a problem with the content. There is no point in re-sending without change. The response narrative SHALL describe the issue."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case OK: return "OK"; 117 case TRANSIENTERROR: return "Transient Error"; 118 case FATALERROR: return "Fatal Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class ResponseTypeEnumFactory implements EnumFactory<ResponseType> { 126 public ResponseType fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("ok".equals(codeString)) 131 return ResponseType.OK; 132 if ("transient-error".equals(codeString)) 133 return ResponseType.TRANSIENTERROR; 134 if ("fatal-error".equals(codeString)) 135 return ResponseType.FATALERROR; 136 throw new IllegalArgumentException("Unknown ResponseType code '"+codeString+"'"); 137 } 138 public Enumeration<ResponseType> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<ResponseType>(this, ResponseType.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<ResponseType>(this, ResponseType.NULL, code); 146 if ("ok".equals(codeString)) 147 return new Enumeration<ResponseType>(this, ResponseType.OK, code); 148 if ("transient-error".equals(codeString)) 149 return new Enumeration<ResponseType>(this, ResponseType.TRANSIENTERROR, code); 150 if ("fatal-error".equals(codeString)) 151 return new Enumeration<ResponseType>(this, ResponseType.FATALERROR, code); 152 throw new FHIRException("Unknown ResponseType code '"+codeString+"'"); 153 } 154 public String toCode(ResponseType code) { 155 if (code == ResponseType.NULL) 156 return null; 157 if (code == ResponseType.OK) 158 return "ok"; 159 if (code == ResponseType.TRANSIENTERROR) 160 return "transient-error"; 161 if (code == ResponseType.FATALERROR) 162 return "fatal-error"; 163 return "?"; 164 } 165 public String toSystem(ResponseType code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class MessageDestinationComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * Indicates where the message should be routed. 174 */ 175 @Child(name = "endpoint", type = {UrlType.class, Endpoint.class}, order=1, min=0, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="Actual destination address or Endpoint resource", formalDefinition="Indicates where the message should be routed." ) 177 protected DataType endpoint; 178 179 /** 180 * Human-readable name for the target system. 181 */ 182 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 183 @Description(shortDefinition="Name of system", formalDefinition="Human-readable name for the target system." ) 184 protected StringType name; 185 186 /** 187 * Identifies the target end system in situations where the initial message transmission is to an intermediary system. 188 */ 189 @Child(name = "target", type = {Device.class}, order=3, min=0, max=1, modifier=false, summary=true) 190 @Description(shortDefinition="Particular delivery destination within the destination", formalDefinition="Identifies the target end system in situations where the initial message transmission is to an intermediary system." ) 191 protected Reference target; 192 193 /** 194 * Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient. 195 */ 196 @Child(name = "receiver", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 197 @Description(shortDefinition="Intended \"real-world\" recipient for the data", formalDefinition="Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient." ) 198 protected Reference receiver; 199 200 private static final long serialVersionUID = -274385787L; 201 202 /** 203 * Constructor 204 */ 205 public MessageDestinationComponent() { 206 super(); 207 } 208 209 /** 210 * @return {@link #endpoint} (Indicates where the message should be routed.) 211 */ 212 public DataType getEndpoint() { 213 return this.endpoint; 214 } 215 216 /** 217 * @return {@link #endpoint} (Indicates where the message should be routed.) 218 */ 219 public UrlType getEndpointUrlType() throws FHIRException { 220 if (this.endpoint == null) 221 this.endpoint = new UrlType(); 222 if (!(this.endpoint instanceof UrlType)) 223 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.endpoint.getClass().getName()+" was encountered"); 224 return (UrlType) this.endpoint; 225 } 226 227 public boolean hasEndpointUrlType() { 228 return this != null && this.endpoint instanceof UrlType; 229 } 230 231 /** 232 * @return {@link #endpoint} (Indicates where the message should be routed.) 233 */ 234 public Reference getEndpointReference() throws FHIRException { 235 if (this.endpoint == null) 236 this.endpoint = new Reference(); 237 if (!(this.endpoint instanceof Reference)) 238 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.endpoint.getClass().getName()+" was encountered"); 239 return (Reference) this.endpoint; 240 } 241 242 public boolean hasEndpointReference() { 243 return this != null && this.endpoint instanceof Reference; 244 } 245 246 public boolean hasEndpoint() { 247 return this.endpoint != null && !this.endpoint.isEmpty(); 248 } 249 250 /** 251 * @param value {@link #endpoint} (Indicates where the message should be routed.) 252 */ 253 public MessageDestinationComponent setEndpoint(DataType value) { 254 if (value != null && !(value instanceof UrlType || value instanceof Reference)) 255 throw new FHIRException("Not the right type for MessageHeader.destination.endpoint[x]: "+value.fhirType()); 256 this.endpoint = value; 257 return this; 258 } 259 260 /** 261 * @return {@link #name} (Human-readable name for the target system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 262 */ 263 public StringType getNameElement() { 264 if (this.name == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create MessageDestinationComponent.name"); 267 else if (Configuration.doAutoCreate()) 268 this.name = new StringType(); // bb 269 return this.name; 270 } 271 272 public boolean hasNameElement() { 273 return this.name != null && !this.name.isEmpty(); 274 } 275 276 public boolean hasName() { 277 return this.name != null && !this.name.isEmpty(); 278 } 279 280 /** 281 * @param value {@link #name} (Human-readable name for the target system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 282 */ 283 public MessageDestinationComponent setNameElement(StringType value) { 284 this.name = value; 285 return this; 286 } 287 288 /** 289 * @return Human-readable name for the target system. 290 */ 291 public String getName() { 292 return this.name == null ? null : this.name.getValue(); 293 } 294 295 /** 296 * @param value Human-readable name for the target system. 297 */ 298 public MessageDestinationComponent setName(String value) { 299 if (Utilities.noString(value)) 300 this.name = null; 301 else { 302 if (this.name == null) 303 this.name = new StringType(); 304 this.name.setValue(value); 305 } 306 return this; 307 } 308 309 /** 310 * @return {@link #target} (Identifies the target end system in situations where the initial message transmission is to an intermediary system.) 311 */ 312 public Reference getTarget() { 313 if (this.target == null) 314 if (Configuration.errorOnAutoCreate()) 315 throw new Error("Attempt to auto-create MessageDestinationComponent.target"); 316 else if (Configuration.doAutoCreate()) 317 this.target = new Reference(); // cc 318 return this.target; 319 } 320 321 public boolean hasTarget() { 322 return this.target != null && !this.target.isEmpty(); 323 } 324 325 /** 326 * @param value {@link #target} (Identifies the target end system in situations where the initial message transmission is to an intermediary system.) 327 */ 328 public MessageDestinationComponent setTarget(Reference value) { 329 this.target = value; 330 return this; 331 } 332 333 /** 334 * @return {@link #receiver} (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) 335 */ 336 public Reference getReceiver() { 337 if (this.receiver == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create MessageDestinationComponent.receiver"); 340 else if (Configuration.doAutoCreate()) 341 this.receiver = new Reference(); // cc 342 return this.receiver; 343 } 344 345 public boolean hasReceiver() { 346 return this.receiver != null && !this.receiver.isEmpty(); 347 } 348 349 /** 350 * @param value {@link #receiver} (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) 351 */ 352 public MessageDestinationComponent setReceiver(Reference value) { 353 this.receiver = value; 354 return this; 355 } 356 357 protected void listChildren(List<Property> children) { 358 super.listChildren(children); 359 children.add(new Property("endpoint[x]", "url|Reference(Endpoint)", "Indicates where the message should be routed.", 0, 1, endpoint)); 360 children.add(new Property("name", "string", "Human-readable name for the target system.", 0, 1, name)); 361 children.add(new Property("target", "Reference(Device)", "Identifies the target end system in situations where the initial message transmission is to an intermediary system.", 0, 1, target)); 362 children.add(new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.", 0, 1, receiver)); 363 } 364 365 @Override 366 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 367 switch (_hash) { 368 case -1135811893: /*endpoint[x]*/ return new Property("endpoint[x]", "url|Reference(Endpoint)", "Indicates where the message should be routed.", 0, 1, endpoint); 369 case 1741102485: /*endpoint*/ return new Property("endpoint[x]", "url|Reference(Endpoint)", "Indicates where the message should be routed.", 0, 1, endpoint); 370 case -1135817830: /*endpointUrl*/ return new Property("endpoint[x]", "url", "Indicates where the message should be routed.", 0, 1, endpoint); 371 case 687192374: /*endpointReference*/ return new Property("endpoint[x]", "Reference(Endpoint)", "Indicates where the message should be routed.", 0, 1, endpoint); 372 case 3373707: /*name*/ return new Property("name", "string", "Human-readable name for the target system.", 0, 1, name); 373 case -880905839: /*target*/ return new Property("target", "Reference(Device)", "Identifies the target end system in situations where the initial message transmission is to an intermediary system.", 0, 1, target); 374 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.", 0, 1, receiver); 375 default: return super.getNamedProperty(_hash, _name, _checkValid); 376 } 377 378 } 379 380 @Override 381 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 382 switch (hash) { 383 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : new Base[] {this.endpoint}; // DataType 384 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 385 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 386 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : new Base[] {this.receiver}; // Reference 387 default: return super.getProperty(hash, name, checkValid); 388 } 389 390 } 391 392 @Override 393 public Base setProperty(int hash, String name, Base value) throws FHIRException { 394 switch (hash) { 395 case 1741102485: // endpoint 396 this.endpoint = TypeConvertor.castToType(value); // DataType 397 return value; 398 case 3373707: // name 399 this.name = TypeConvertor.castToString(value); // StringType 400 return value; 401 case -880905839: // target 402 this.target = TypeConvertor.castToReference(value); // Reference 403 return value; 404 case -808719889: // receiver 405 this.receiver = TypeConvertor.castToReference(value); // Reference 406 return value; 407 default: return super.setProperty(hash, name, value); 408 } 409 410 } 411 412 @Override 413 public Base setProperty(String name, Base value) throws FHIRException { 414 if (name.equals("endpoint[x]")) { 415 this.endpoint = TypeConvertor.castToType(value); // DataType 416 } else if (name.equals("name")) { 417 this.name = TypeConvertor.castToString(value); // StringType 418 } else if (name.equals("target")) { 419 this.target = TypeConvertor.castToReference(value); // Reference 420 } else if (name.equals("receiver")) { 421 this.receiver = TypeConvertor.castToReference(value); // Reference 422 } else 423 return super.setProperty(name, value); 424 return value; 425 } 426 427 @Override 428 public void removeChild(String name, Base value) throws FHIRException { 429 if (name.equals("endpoint[x]")) { 430 this.endpoint = null; 431 } else if (name.equals("name")) { 432 this.name = null; 433 } else if (name.equals("target")) { 434 this.target = null; 435 } else if (name.equals("receiver")) { 436 this.receiver = null; 437 } else 438 super.removeChild(name, value); 439 440 } 441 442 @Override 443 public Base makeProperty(int hash, String name) throws FHIRException { 444 switch (hash) { 445 case -1135811893: return getEndpoint(); 446 case 1741102485: return getEndpoint(); 447 case 3373707: return getNameElement(); 448 case -880905839: return getTarget(); 449 case -808719889: return getReceiver(); 450 default: return super.makeProperty(hash, name); 451 } 452 453 } 454 455 @Override 456 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 457 switch (hash) { 458 case 1741102485: /*endpoint*/ return new String[] {"url", "Reference"}; 459 case 3373707: /*name*/ return new String[] {"string"}; 460 case -880905839: /*target*/ return new String[] {"Reference"}; 461 case -808719889: /*receiver*/ return new String[] {"Reference"}; 462 default: return super.getTypesForProperty(hash, name); 463 } 464 465 } 466 467 @Override 468 public Base addChild(String name) throws FHIRException { 469 if (name.equals("endpointUrl")) { 470 this.endpoint = new UrlType(); 471 return this.endpoint; 472 } 473 else if (name.equals("endpointReference")) { 474 this.endpoint = new Reference(); 475 return this.endpoint; 476 } 477 else if (name.equals("name")) { 478 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.destination.name"); 479 } 480 else if (name.equals("target")) { 481 this.target = new Reference(); 482 return this.target; 483 } 484 else if (name.equals("receiver")) { 485 this.receiver = new Reference(); 486 return this.receiver; 487 } 488 else 489 return super.addChild(name); 490 } 491 492 public MessageDestinationComponent copy() { 493 MessageDestinationComponent dst = new MessageDestinationComponent(); 494 copyValues(dst); 495 return dst; 496 } 497 498 public void copyValues(MessageDestinationComponent dst) { 499 super.copyValues(dst); 500 dst.endpoint = endpoint == null ? null : endpoint.copy(); 501 dst.name = name == null ? null : name.copy(); 502 dst.target = target == null ? null : target.copy(); 503 dst.receiver = receiver == null ? null : receiver.copy(); 504 } 505 506 @Override 507 public boolean equalsDeep(Base other_) { 508 if (!super.equalsDeep(other_)) 509 return false; 510 if (!(other_ instanceof MessageDestinationComponent)) 511 return false; 512 MessageDestinationComponent o = (MessageDestinationComponent) other_; 513 return compareDeep(endpoint, o.endpoint, true) && compareDeep(name, o.name, true) && compareDeep(target, o.target, true) 514 && compareDeep(receiver, o.receiver, true); 515 } 516 517 @Override 518 public boolean equalsShallow(Base other_) { 519 if (!super.equalsShallow(other_)) 520 return false; 521 if (!(other_ instanceof MessageDestinationComponent)) 522 return false; 523 MessageDestinationComponent o = (MessageDestinationComponent) other_; 524 return compareValues(name, o.name, true); 525 } 526 527 public boolean isEmpty() { 528 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(endpoint, name, target, receiver 529 ); 530 } 531 532 public String fhirType() { 533 return "MessageHeader.destination"; 534 535 } 536 537 } 538 539 @Block() 540 public static class MessageSourceComponent extends BackboneElement implements IBaseBackboneElement { 541 /** 542 * Identifies the routing target to send acknowledgements to. 543 */ 544 @Child(name = "endpoint", type = {UrlType.class, Endpoint.class}, order=1, min=0, max=1, modifier=false, summary=true) 545 @Description(shortDefinition="Actual source address or Endpoint resource", formalDefinition="Identifies the routing target to send acknowledgements to." ) 546 protected DataType endpoint; 547 548 /** 549 * Human-readable name for the source system. 550 */ 551 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 552 @Description(shortDefinition="Name of system", formalDefinition="Human-readable name for the source system." ) 553 protected StringType name; 554 555 /** 556 * May include configuration or other information useful in debugging. 557 */ 558 @Child(name = "software", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 559 @Description(shortDefinition="Name of software running the system", formalDefinition="May include configuration or other information useful in debugging." ) 560 protected StringType software; 561 562 /** 563 * Can convey versions of multiple systems in situations where a message passes through multiple hands. 564 */ 565 @Child(name = "version", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 566 @Description(shortDefinition="Version of software running", formalDefinition="Can convey versions of multiple systems in situations where a message passes through multiple hands." ) 567 protected StringType version; 568 569 /** 570 * An e-mail, phone, website or other contact point to use to resolve issues with message communications. 571 */ 572 @Child(name = "contact", type = {ContactPoint.class}, order=5, min=0, max=1, modifier=false, summary=true) 573 @Description(shortDefinition="Human contact for problems", formalDefinition="An e-mail, phone, website or other contact point to use to resolve issues with message communications." ) 574 protected ContactPoint contact; 575 576 private static final long serialVersionUID = 62264996L; 577 578 /** 579 * Constructor 580 */ 581 public MessageSourceComponent() { 582 super(); 583 } 584 585 /** 586 * @return {@link #endpoint} (Identifies the routing target to send acknowledgements to.) 587 */ 588 public DataType getEndpoint() { 589 return this.endpoint; 590 } 591 592 /** 593 * @return {@link #endpoint} (Identifies the routing target to send acknowledgements to.) 594 */ 595 public UrlType getEndpointUrlType() throws FHIRException { 596 if (this.endpoint == null) 597 this.endpoint = new UrlType(); 598 if (!(this.endpoint instanceof UrlType)) 599 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.endpoint.getClass().getName()+" was encountered"); 600 return (UrlType) this.endpoint; 601 } 602 603 public boolean hasEndpointUrlType() { 604 return this != null && this.endpoint instanceof UrlType; 605 } 606 607 /** 608 * @return {@link #endpoint} (Identifies the routing target to send acknowledgements to.) 609 */ 610 public Reference getEndpointReference() throws FHIRException { 611 if (this.endpoint == null) 612 this.endpoint = new Reference(); 613 if (!(this.endpoint instanceof Reference)) 614 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.endpoint.getClass().getName()+" was encountered"); 615 return (Reference) this.endpoint; 616 } 617 618 public boolean hasEndpointReference() { 619 return this != null && this.endpoint instanceof Reference; 620 } 621 622 public boolean hasEndpoint() { 623 return this.endpoint != null && !this.endpoint.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #endpoint} (Identifies the routing target to send acknowledgements to.) 628 */ 629 public MessageSourceComponent setEndpoint(DataType value) { 630 if (value != null && !(value instanceof UrlType || value instanceof Reference)) 631 throw new FHIRException("Not the right type for MessageHeader.source.endpoint[x]: "+value.fhirType()); 632 this.endpoint = value; 633 return this; 634 } 635 636 /** 637 * @return {@link #name} (Human-readable name for the source system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 638 */ 639 public StringType getNameElement() { 640 if (this.name == null) 641 if (Configuration.errorOnAutoCreate()) 642 throw new Error("Attempt to auto-create MessageSourceComponent.name"); 643 else if (Configuration.doAutoCreate()) 644 this.name = new StringType(); // bb 645 return this.name; 646 } 647 648 public boolean hasNameElement() { 649 return this.name != null && !this.name.isEmpty(); 650 } 651 652 public boolean hasName() { 653 return this.name != null && !this.name.isEmpty(); 654 } 655 656 /** 657 * @param value {@link #name} (Human-readable name for the source system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 658 */ 659 public MessageSourceComponent setNameElement(StringType value) { 660 this.name = value; 661 return this; 662 } 663 664 /** 665 * @return Human-readable name for the source system. 666 */ 667 public String getName() { 668 return this.name == null ? null : this.name.getValue(); 669 } 670 671 /** 672 * @param value Human-readable name for the source system. 673 */ 674 public MessageSourceComponent setName(String value) { 675 if (Utilities.noString(value)) 676 this.name = null; 677 else { 678 if (this.name == null) 679 this.name = new StringType(); 680 this.name.setValue(value); 681 } 682 return this; 683 } 684 685 /** 686 * @return {@link #software} (May include configuration or other information useful in debugging.). This is the underlying object with id, value and extensions. The accessor "getSoftware" gives direct access to the value 687 */ 688 public StringType getSoftwareElement() { 689 if (this.software == null) 690 if (Configuration.errorOnAutoCreate()) 691 throw new Error("Attempt to auto-create MessageSourceComponent.software"); 692 else if (Configuration.doAutoCreate()) 693 this.software = new StringType(); // bb 694 return this.software; 695 } 696 697 public boolean hasSoftwareElement() { 698 return this.software != null && !this.software.isEmpty(); 699 } 700 701 public boolean hasSoftware() { 702 return this.software != null && !this.software.isEmpty(); 703 } 704 705 /** 706 * @param value {@link #software} (May include configuration or other information useful in debugging.). This is the underlying object with id, value and extensions. The accessor "getSoftware" gives direct access to the value 707 */ 708 public MessageSourceComponent setSoftwareElement(StringType value) { 709 this.software = value; 710 return this; 711 } 712 713 /** 714 * @return May include configuration or other information useful in debugging. 715 */ 716 public String getSoftware() { 717 return this.software == null ? null : this.software.getValue(); 718 } 719 720 /** 721 * @param value May include configuration or other information useful in debugging. 722 */ 723 public MessageSourceComponent setSoftware(String value) { 724 if (Utilities.noString(value)) 725 this.software = null; 726 else { 727 if (this.software == null) 728 this.software = new StringType(); 729 this.software.setValue(value); 730 } 731 return this; 732 } 733 734 /** 735 * @return {@link #version} (Can convey versions of multiple systems in situations where a message passes through multiple hands.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 736 */ 737 public StringType getVersionElement() { 738 if (this.version == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create MessageSourceComponent.version"); 741 else if (Configuration.doAutoCreate()) 742 this.version = new StringType(); // bb 743 return this.version; 744 } 745 746 public boolean hasVersionElement() { 747 return this.version != null && !this.version.isEmpty(); 748 } 749 750 public boolean hasVersion() { 751 return this.version != null && !this.version.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #version} (Can convey versions of multiple systems in situations where a message passes through multiple hands.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 756 */ 757 public MessageSourceComponent setVersionElement(StringType value) { 758 this.version = value; 759 return this; 760 } 761 762 /** 763 * @return Can convey versions of multiple systems in situations where a message passes through multiple hands. 764 */ 765 public String getVersion() { 766 return this.version == null ? null : this.version.getValue(); 767 } 768 769 /** 770 * @param value Can convey versions of multiple systems in situations where a message passes through multiple hands. 771 */ 772 public MessageSourceComponent setVersion(String value) { 773 if (Utilities.noString(value)) 774 this.version = null; 775 else { 776 if (this.version == null) 777 this.version = new StringType(); 778 this.version.setValue(value); 779 } 780 return this; 781 } 782 783 /** 784 * @return {@link #contact} (An e-mail, phone, website or other contact point to use to resolve issues with message communications.) 785 */ 786 public ContactPoint getContact() { 787 if (this.contact == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create MessageSourceComponent.contact"); 790 else if (Configuration.doAutoCreate()) 791 this.contact = new ContactPoint(); // cc 792 return this.contact; 793 } 794 795 public boolean hasContact() { 796 return this.contact != null && !this.contact.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #contact} (An e-mail, phone, website or other contact point to use to resolve issues with message communications.) 801 */ 802 public MessageSourceComponent setContact(ContactPoint value) { 803 this.contact = value; 804 return this; 805 } 806 807 protected void listChildren(List<Property> children) { 808 super.listChildren(children); 809 children.add(new Property("endpoint[x]", "url|Reference(Endpoint)", "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint)); 810 children.add(new Property("name", "string", "Human-readable name for the source system.", 0, 1, name)); 811 children.add(new Property("software", "string", "May include configuration or other information useful in debugging.", 0, 1, software)); 812 children.add(new Property("version", "string", "Can convey versions of multiple systems in situations where a message passes through multiple hands.", 0, 1, version)); 813 children.add(new Property("contact", "ContactPoint", "An e-mail, phone, website or other contact point to use to resolve issues with message communications.", 0, 1, contact)); 814 } 815 816 @Override 817 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 818 switch (_hash) { 819 case -1135811893: /*endpoint[x]*/ return new Property("endpoint[x]", "url|Reference(Endpoint)", "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint); 820 case 1741102485: /*endpoint*/ return new Property("endpoint[x]", "url|Reference(Endpoint)", "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint); 821 case -1135817830: /*endpointUrl*/ return new Property("endpoint[x]", "url", "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint); 822 case 687192374: /*endpointReference*/ return new Property("endpoint[x]", "Reference(Endpoint)", "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint); 823 case 3373707: /*name*/ return new Property("name", "string", "Human-readable name for the source system.", 0, 1, name); 824 case 1319330215: /*software*/ return new Property("software", "string", "May include configuration or other information useful in debugging.", 0, 1, software); 825 case 351608024: /*version*/ return new Property("version", "string", "Can convey versions of multiple systems in situations where a message passes through multiple hands.", 0, 1, version); 826 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "An e-mail, phone, website or other contact point to use to resolve issues with message communications.", 0, 1, contact); 827 default: return super.getNamedProperty(_hash, _name, _checkValid); 828 } 829 830 } 831 832 @Override 833 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 834 switch (hash) { 835 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : new Base[] {this.endpoint}; // DataType 836 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 837 case 1319330215: /*software*/ return this.software == null ? new Base[0] : new Base[] {this.software}; // StringType 838 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 839 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : new Base[] {this.contact}; // ContactPoint 840 default: return super.getProperty(hash, name, checkValid); 841 } 842 843 } 844 845 @Override 846 public Base setProperty(int hash, String name, Base value) throws FHIRException { 847 switch (hash) { 848 case 1741102485: // endpoint 849 this.endpoint = TypeConvertor.castToType(value); // DataType 850 return value; 851 case 3373707: // name 852 this.name = TypeConvertor.castToString(value); // StringType 853 return value; 854 case 1319330215: // software 855 this.software = TypeConvertor.castToString(value); // StringType 856 return value; 857 case 351608024: // version 858 this.version = TypeConvertor.castToString(value); // StringType 859 return value; 860 case 951526432: // contact 861 this.contact = TypeConvertor.castToContactPoint(value); // ContactPoint 862 return value; 863 default: return super.setProperty(hash, name, value); 864 } 865 866 } 867 868 @Override 869 public Base setProperty(String name, Base value) throws FHIRException { 870 if (name.equals("endpoint[x]")) { 871 this.endpoint = TypeConvertor.castToType(value); // DataType 872 } else if (name.equals("name")) { 873 this.name = TypeConvertor.castToString(value); // StringType 874 } else if (name.equals("software")) { 875 this.software = TypeConvertor.castToString(value); // StringType 876 } else if (name.equals("version")) { 877 this.version = TypeConvertor.castToString(value); // StringType 878 } else if (name.equals("contact")) { 879 this.contact = TypeConvertor.castToContactPoint(value); // ContactPoint 880 } else 881 return super.setProperty(name, value); 882 return value; 883 } 884 885 @Override 886 public void removeChild(String name, Base value) throws FHIRException { 887 if (name.equals("endpoint[x]")) { 888 this.endpoint = null; 889 } else if (name.equals("name")) { 890 this.name = null; 891 } else if (name.equals("software")) { 892 this.software = null; 893 } else if (name.equals("version")) { 894 this.version = null; 895 } else if (name.equals("contact")) { 896 this.contact = null; 897 } else 898 super.removeChild(name, value); 899 900 } 901 902 @Override 903 public Base makeProperty(int hash, String name) throws FHIRException { 904 switch (hash) { 905 case -1135811893: return getEndpoint(); 906 case 1741102485: return getEndpoint(); 907 case 3373707: return getNameElement(); 908 case 1319330215: return getSoftwareElement(); 909 case 351608024: return getVersionElement(); 910 case 951526432: return getContact(); 911 default: return super.makeProperty(hash, name); 912 } 913 914 } 915 916 @Override 917 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 918 switch (hash) { 919 case 1741102485: /*endpoint*/ return new String[] {"url", "Reference"}; 920 case 3373707: /*name*/ return new String[] {"string"}; 921 case 1319330215: /*software*/ return new String[] {"string"}; 922 case 351608024: /*version*/ return new String[] {"string"}; 923 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 924 default: return super.getTypesForProperty(hash, name); 925 } 926 927 } 928 929 @Override 930 public Base addChild(String name) throws FHIRException { 931 if (name.equals("endpointUrl")) { 932 this.endpoint = new UrlType(); 933 return this.endpoint; 934 } 935 else if (name.equals("endpointReference")) { 936 this.endpoint = new Reference(); 937 return this.endpoint; 938 } 939 else if (name.equals("name")) { 940 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.source.name"); 941 } 942 else if (name.equals("software")) { 943 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.source.software"); 944 } 945 else if (name.equals("version")) { 946 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.source.version"); 947 } 948 else if (name.equals("contact")) { 949 this.contact = new ContactPoint(); 950 return this.contact; 951 } 952 else 953 return super.addChild(name); 954 } 955 956 public MessageSourceComponent copy() { 957 MessageSourceComponent dst = new MessageSourceComponent(); 958 copyValues(dst); 959 return dst; 960 } 961 962 public void copyValues(MessageSourceComponent dst) { 963 super.copyValues(dst); 964 dst.endpoint = endpoint == null ? null : endpoint.copy(); 965 dst.name = name == null ? null : name.copy(); 966 dst.software = software == null ? null : software.copy(); 967 dst.version = version == null ? null : version.copy(); 968 dst.contact = contact == null ? null : contact.copy(); 969 } 970 971 @Override 972 public boolean equalsDeep(Base other_) { 973 if (!super.equalsDeep(other_)) 974 return false; 975 if (!(other_ instanceof MessageSourceComponent)) 976 return false; 977 MessageSourceComponent o = (MessageSourceComponent) other_; 978 return compareDeep(endpoint, o.endpoint, true) && compareDeep(name, o.name, true) && compareDeep(software, o.software, true) 979 && compareDeep(version, o.version, true) && compareDeep(contact, o.contact, true); 980 } 981 982 @Override 983 public boolean equalsShallow(Base other_) { 984 if (!super.equalsShallow(other_)) 985 return false; 986 if (!(other_ instanceof MessageSourceComponent)) 987 return false; 988 MessageSourceComponent o = (MessageSourceComponent) other_; 989 return compareValues(name, o.name, true) && compareValues(software, o.software, true) && compareValues(version, o.version, true) 990 ; 991 } 992 993 public boolean isEmpty() { 994 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(endpoint, name, software 995 , version, contact); 996 } 997 998 public String fhirType() { 999 return "MessageHeader.source"; 1000 1001 } 1002 1003 } 1004 1005 @Block() 1006 public static class MessageHeaderResponseComponent extends BackboneElement implements IBaseBackboneElement { 1007 /** 1008 * The Bundle.identifier of the message to which this message is a response. 1009 */ 1010 @Child(name = "identifier", type = {Identifier.class}, order=1, min=1, max=1, modifier=false, summary=true) 1011 @Description(shortDefinition="Bundle.identifier of original message", formalDefinition="The Bundle.identifier of the message to which this message is a response." ) 1012 protected Identifier identifier; 1013 1014 /** 1015 * Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not. 1016 */ 1017 @Child(name = "code", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1018 @Description(shortDefinition="ok | transient-error | fatal-error", formalDefinition="Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not." ) 1019 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/response-code") 1020 protected Enumeration<ResponseType> code; 1021 1022 /** 1023 * Full details of any issues found in the message. 1024 */ 1025 @Child(name = "details", type = {OperationOutcome.class}, order=3, min=0, max=1, modifier=false, summary=true) 1026 @Description(shortDefinition="Specific list of hints/warnings/errors", formalDefinition="Full details of any issues found in the message." ) 1027 protected Reference details; 1028 1029 private static final long serialVersionUID = 1091046778L; 1030 1031 /** 1032 * Constructor 1033 */ 1034 public MessageHeaderResponseComponent() { 1035 super(); 1036 } 1037 1038 /** 1039 * Constructor 1040 */ 1041 public MessageHeaderResponseComponent(Identifier identifier, ResponseType code) { 1042 super(); 1043 this.setIdentifier(identifier); 1044 this.setCode(code); 1045 } 1046 1047 /** 1048 * @return {@link #identifier} (The Bundle.identifier of the message to which this message is a response.) 1049 */ 1050 public Identifier getIdentifier() { 1051 if (this.identifier == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.identifier"); 1054 else if (Configuration.doAutoCreate()) 1055 this.identifier = new Identifier(); // cc 1056 return this.identifier; 1057 } 1058 1059 public boolean hasIdentifier() { 1060 return this.identifier != null && !this.identifier.isEmpty(); 1061 } 1062 1063 /** 1064 * @param value {@link #identifier} (The Bundle.identifier of the message to which this message is a response.) 1065 */ 1066 public MessageHeaderResponseComponent setIdentifier(Identifier value) { 1067 this.identifier = value; 1068 return this; 1069 } 1070 1071 /** 1072 * @return {@link #code} (Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1073 */ 1074 public Enumeration<ResponseType> getCodeElement() { 1075 if (this.code == null) 1076 if (Configuration.errorOnAutoCreate()) 1077 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.code"); 1078 else if (Configuration.doAutoCreate()) 1079 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); // bb 1080 return this.code; 1081 } 1082 1083 public boolean hasCodeElement() { 1084 return this.code != null && !this.code.isEmpty(); 1085 } 1086 1087 public boolean hasCode() { 1088 return this.code != null && !this.code.isEmpty(); 1089 } 1090 1091 /** 1092 * @param value {@link #code} (Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1093 */ 1094 public MessageHeaderResponseComponent setCodeElement(Enumeration<ResponseType> value) { 1095 this.code = value; 1096 return this; 1097 } 1098 1099 /** 1100 * @return Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not. 1101 */ 1102 public ResponseType getCode() { 1103 return this.code == null ? null : this.code.getValue(); 1104 } 1105 1106 /** 1107 * @param value Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not. 1108 */ 1109 public MessageHeaderResponseComponent setCode(ResponseType value) { 1110 if (this.code == null) 1111 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); 1112 this.code.setValue(value); 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #details} (Full details of any issues found in the message.) 1118 */ 1119 public Reference getDetails() { 1120 if (this.details == null) 1121 if (Configuration.errorOnAutoCreate()) 1122 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.details"); 1123 else if (Configuration.doAutoCreate()) 1124 this.details = new Reference(); // cc 1125 return this.details; 1126 } 1127 1128 public boolean hasDetails() { 1129 return this.details != null && !this.details.isEmpty(); 1130 } 1131 1132 /** 1133 * @param value {@link #details} (Full details of any issues found in the message.) 1134 */ 1135 public MessageHeaderResponseComponent setDetails(Reference value) { 1136 this.details = value; 1137 return this; 1138 } 1139 1140 protected void listChildren(List<Property> children) { 1141 super.listChildren(children); 1142 children.add(new Property("identifier", "Identifier", "The Bundle.identifier of the message to which this message is a response.", 0, 1, identifier)); 1143 children.add(new Property("code", "code", "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.", 0, 1, code)); 1144 children.add(new Property("details", "Reference(OperationOutcome)", "Full details of any issues found in the message.", 0, 1, details)); 1145 } 1146 1147 @Override 1148 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1149 switch (_hash) { 1150 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Bundle.identifier of the message to which this message is a response.", 0, 1, identifier); 1151 case 3059181: /*code*/ return new Property("code", "code", "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.", 0, 1, code); 1152 case 1557721666: /*details*/ return new Property("details", "Reference(OperationOutcome)", "Full details of any issues found in the message.", 0, 1, details); 1153 default: return super.getNamedProperty(_hash, _name, _checkValid); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1160 switch (hash) { 1161 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1162 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<ResponseType> 1163 case 1557721666: /*details*/ return this.details == null ? new Base[0] : new Base[] {this.details}; // Reference 1164 default: return super.getProperty(hash, name, checkValid); 1165 } 1166 1167 } 1168 1169 @Override 1170 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1171 switch (hash) { 1172 case -1618432855: // identifier 1173 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1174 return value; 1175 case 3059181: // code 1176 value = new ResponseTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1177 this.code = (Enumeration) value; // Enumeration<ResponseType> 1178 return value; 1179 case 1557721666: // details 1180 this.details = TypeConvertor.castToReference(value); // Reference 1181 return value; 1182 default: return super.setProperty(hash, name, value); 1183 } 1184 1185 } 1186 1187 @Override 1188 public Base setProperty(String name, Base value) throws FHIRException { 1189 if (name.equals("identifier")) { 1190 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1191 } else if (name.equals("code")) { 1192 value = new ResponseTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1193 this.code = (Enumeration) value; // Enumeration<ResponseType> 1194 } else if (name.equals("details")) { 1195 this.details = TypeConvertor.castToReference(value); // Reference 1196 } else 1197 return super.setProperty(name, value); 1198 return value; 1199 } 1200 1201 @Override 1202 public void removeChild(String name, Base value) throws FHIRException { 1203 if (name.equals("identifier")) { 1204 this.identifier = null; 1205 } else if (name.equals("code")) { 1206 value = new ResponseTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1207 this.code = (Enumeration) value; // Enumeration<ResponseType> 1208 } else if (name.equals("details")) { 1209 this.details = null; 1210 } else 1211 super.removeChild(name, value); 1212 1213 } 1214 1215 @Override 1216 public Base makeProperty(int hash, String name) throws FHIRException { 1217 switch (hash) { 1218 case -1618432855: return getIdentifier(); 1219 case 3059181: return getCodeElement(); 1220 case 1557721666: return getDetails(); 1221 default: return super.makeProperty(hash, name); 1222 } 1223 1224 } 1225 1226 @Override 1227 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1228 switch (hash) { 1229 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1230 case 3059181: /*code*/ return new String[] {"code"}; 1231 case 1557721666: /*details*/ return new String[] {"Reference"}; 1232 default: return super.getTypesForProperty(hash, name); 1233 } 1234 1235 } 1236 1237 @Override 1238 public Base addChild(String name) throws FHIRException { 1239 if (name.equals("identifier")) { 1240 this.identifier = new Identifier(); 1241 return this.identifier; 1242 } 1243 else if (name.equals("code")) { 1244 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.response.code"); 1245 } 1246 else if (name.equals("details")) { 1247 this.details = new Reference(); 1248 return this.details; 1249 } 1250 else 1251 return super.addChild(name); 1252 } 1253 1254 public MessageHeaderResponseComponent copy() { 1255 MessageHeaderResponseComponent dst = new MessageHeaderResponseComponent(); 1256 copyValues(dst); 1257 return dst; 1258 } 1259 1260 public void copyValues(MessageHeaderResponseComponent dst) { 1261 super.copyValues(dst); 1262 dst.identifier = identifier == null ? null : identifier.copy(); 1263 dst.code = code == null ? null : code.copy(); 1264 dst.details = details == null ? null : details.copy(); 1265 } 1266 1267 @Override 1268 public boolean equalsDeep(Base other_) { 1269 if (!super.equalsDeep(other_)) 1270 return false; 1271 if (!(other_ instanceof MessageHeaderResponseComponent)) 1272 return false; 1273 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other_; 1274 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(details, o.details, true) 1275 ; 1276 } 1277 1278 @Override 1279 public boolean equalsShallow(Base other_) { 1280 if (!super.equalsShallow(other_)) 1281 return false; 1282 if (!(other_ instanceof MessageHeaderResponseComponent)) 1283 return false; 1284 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other_; 1285 return compareValues(code, o.code, true); 1286 } 1287 1288 public boolean isEmpty() { 1289 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, details 1290 ); 1291 } 1292 1293 public String fhirType() { 1294 return "MessageHeader.response"; 1295 1296 } 1297 1298 } 1299 1300 /** 1301 * Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition. 1302 */ 1303 @Child(name = "event", type = {Coding.class, CanonicalType.class}, order=0, min=1, max=1, modifier=false, summary=true) 1304 @Description(shortDefinition="Event code or link to EventDefinition", formalDefinition="Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition." ) 1305 protected DataType event; 1306 1307 /** 1308 * The destination application which the message is intended for. 1309 */ 1310 @Child(name = "destination", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1311 @Description(shortDefinition="Message destination application(s)", formalDefinition="The destination application which the message is intended for." ) 1312 protected List<MessageDestinationComponent> destination; 1313 1314 /** 1315 * Identifies the sending system to allow the use of a trust relationship. 1316 */ 1317 @Child(name = "sender", type = {Practitioner.class, PractitionerRole.class, Device.class, Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 1318 @Description(shortDefinition="Real world sender of the message", formalDefinition="Identifies the sending system to allow the use of a trust relationship." ) 1319 protected Reference sender; 1320 1321 /** 1322 * The logical author of the message - the personor device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions. 1323 */ 1324 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Device.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 1325 @Description(shortDefinition="The source of the decision", formalDefinition="The logical author of the message - the personor device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions." ) 1326 protected Reference author; 1327 1328 /** 1329 * The source application from which this message originated. 1330 */ 1331 @Child(name = "source", type = {}, order=4, min=1, max=1, modifier=false, summary=true) 1332 @Description(shortDefinition="Message source application", formalDefinition="The source application from which this message originated." ) 1333 protected MessageSourceComponent source; 1334 1335 /** 1336 * The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party. 1337 */ 1338 @Child(name = "responsible", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=5, min=0, max=1, modifier=false, summary=true) 1339 @Description(shortDefinition="Final responsibility for event", formalDefinition="The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party." ) 1340 protected Reference responsible; 1341 1342 /** 1343 * Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message. 1344 */ 1345 @Child(name = "reason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 1346 @Description(shortDefinition="Cause of event", formalDefinition="Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message." ) 1347 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-reason-encounter") 1348 protected CodeableConcept reason; 1349 1350 /** 1351 * Information about the message that this message is a response to. Only present if this message is a response. 1352 */ 1353 @Child(name = "response", type = {}, order=7, min=0, max=1, modifier=false, summary=true) 1354 @Description(shortDefinition="If this is a reply to prior message", formalDefinition="Information about the message that this message is a response to. Only present if this message is a response." ) 1355 protected MessageHeaderResponseComponent response; 1356 1357 /** 1358 * The actual data of the message - a reference to the root/focus class of the event. This is allowed to be a Parameters resource. 1359 */ 1360 @Child(name = "focus", type = {Reference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1361 @Description(shortDefinition="The actual content of the message", formalDefinition="The actual data of the message - a reference to the root/focus class of the event. This is allowed to be a Parameters resource." ) 1362 protected List<Reference> focus; 1363 1364 /** 1365 * Permanent link to the MessageDefinition for this message. 1366 */ 1367 @Child(name = "definition", type = {CanonicalType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1368 @Description(shortDefinition="Link to the definition for this message", formalDefinition="Permanent link to the MessageDefinition for this message." ) 1369 protected CanonicalType definition; 1370 1371 private static final long serialVersionUID = 2129967751L; 1372 1373 /** 1374 * Constructor 1375 */ 1376 public MessageHeader() { 1377 super(); 1378 } 1379 1380 /** 1381 * Constructor 1382 */ 1383 public MessageHeader(DataType event, MessageSourceComponent source) { 1384 super(); 1385 this.setEvent(event); 1386 this.setSource(source); 1387 } 1388 1389 /** 1390 * @return {@link #event} (Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.) 1391 */ 1392 public DataType getEvent() { 1393 return this.event; 1394 } 1395 1396 /** 1397 * @return {@link #event} (Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.) 1398 */ 1399 public Coding getEventCoding() throws FHIRException { 1400 if (this.event == null) 1401 this.event = new Coding(); 1402 if (!(this.event instanceof Coding)) 1403 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.event.getClass().getName()+" was encountered"); 1404 return (Coding) this.event; 1405 } 1406 1407 public boolean hasEventCoding() { 1408 return this != null && this.event instanceof Coding; 1409 } 1410 1411 /** 1412 * @return {@link #event} (Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.) 1413 */ 1414 public CanonicalType getEventCanonicalType() throws FHIRException { 1415 if (this.event == null) 1416 this.event = new CanonicalType(); 1417 if (!(this.event instanceof CanonicalType)) 1418 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.event.getClass().getName()+" was encountered"); 1419 return (CanonicalType) this.event; 1420 } 1421 1422 public boolean hasEventCanonicalType() { 1423 return this != null && this.event instanceof CanonicalType; 1424 } 1425 1426 public boolean hasEvent() { 1427 return this.event != null && !this.event.isEmpty(); 1428 } 1429 1430 /** 1431 * @param value {@link #event} (Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.) 1432 */ 1433 public MessageHeader setEvent(DataType value) { 1434 if (value != null && !(value instanceof Coding || value instanceof CanonicalType)) 1435 throw new FHIRException("Not the right type for MessageHeader.event[x]: "+value.fhirType()); 1436 this.event = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #destination} (The destination application which the message is intended for.) 1442 */ 1443 public List<MessageDestinationComponent> getDestination() { 1444 if (this.destination == null) 1445 this.destination = new ArrayList<MessageDestinationComponent>(); 1446 return this.destination; 1447 } 1448 1449 /** 1450 * @return Returns a reference to <code>this</code> for easy method chaining 1451 */ 1452 public MessageHeader setDestination(List<MessageDestinationComponent> theDestination) { 1453 this.destination = theDestination; 1454 return this; 1455 } 1456 1457 public boolean hasDestination() { 1458 if (this.destination == null) 1459 return false; 1460 for (MessageDestinationComponent item : this.destination) 1461 if (!item.isEmpty()) 1462 return true; 1463 return false; 1464 } 1465 1466 public MessageDestinationComponent addDestination() { //3 1467 MessageDestinationComponent t = new MessageDestinationComponent(); 1468 if (this.destination == null) 1469 this.destination = new ArrayList<MessageDestinationComponent>(); 1470 this.destination.add(t); 1471 return t; 1472 } 1473 1474 public MessageHeader addDestination(MessageDestinationComponent t) { //3 1475 if (t == null) 1476 return this; 1477 if (this.destination == null) 1478 this.destination = new ArrayList<MessageDestinationComponent>(); 1479 this.destination.add(t); 1480 return this; 1481 } 1482 1483 /** 1484 * @return The first repetition of repeating field {@link #destination}, creating it if it does not already exist {3} 1485 */ 1486 public MessageDestinationComponent getDestinationFirstRep() { 1487 if (getDestination().isEmpty()) { 1488 addDestination(); 1489 } 1490 return getDestination().get(0); 1491 } 1492 1493 /** 1494 * @return {@link #sender} (Identifies the sending system to allow the use of a trust relationship.) 1495 */ 1496 public Reference getSender() { 1497 if (this.sender == null) 1498 if (Configuration.errorOnAutoCreate()) 1499 throw new Error("Attempt to auto-create MessageHeader.sender"); 1500 else if (Configuration.doAutoCreate()) 1501 this.sender = new Reference(); // cc 1502 return this.sender; 1503 } 1504 1505 public boolean hasSender() { 1506 return this.sender != null && !this.sender.isEmpty(); 1507 } 1508 1509 /** 1510 * @param value {@link #sender} (Identifies the sending system to allow the use of a trust relationship.) 1511 */ 1512 public MessageHeader setSender(Reference value) { 1513 this.sender = value; 1514 return this; 1515 } 1516 1517 /** 1518 * @return {@link #author} (The logical author of the message - the personor device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) 1519 */ 1520 public Reference getAuthor() { 1521 if (this.author == null) 1522 if (Configuration.errorOnAutoCreate()) 1523 throw new Error("Attempt to auto-create MessageHeader.author"); 1524 else if (Configuration.doAutoCreate()) 1525 this.author = new Reference(); // cc 1526 return this.author; 1527 } 1528 1529 public boolean hasAuthor() { 1530 return this.author != null && !this.author.isEmpty(); 1531 } 1532 1533 /** 1534 * @param value {@link #author} (The logical author of the message - the personor device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) 1535 */ 1536 public MessageHeader setAuthor(Reference value) { 1537 this.author = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return {@link #source} (The source application from which this message originated.) 1543 */ 1544 public MessageSourceComponent getSource() { 1545 if (this.source == null) 1546 if (Configuration.errorOnAutoCreate()) 1547 throw new Error("Attempt to auto-create MessageHeader.source"); 1548 else if (Configuration.doAutoCreate()) 1549 this.source = new MessageSourceComponent(); // cc 1550 return this.source; 1551 } 1552 1553 public boolean hasSource() { 1554 return this.source != null && !this.source.isEmpty(); 1555 } 1556 1557 /** 1558 * @param value {@link #source} (The source application from which this message originated.) 1559 */ 1560 public MessageHeader setSource(MessageSourceComponent value) { 1561 this.source = value; 1562 return this; 1563 } 1564 1565 /** 1566 * @return {@link #responsible} (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) 1567 */ 1568 public Reference getResponsible() { 1569 if (this.responsible == null) 1570 if (Configuration.errorOnAutoCreate()) 1571 throw new Error("Attempt to auto-create MessageHeader.responsible"); 1572 else if (Configuration.doAutoCreate()) 1573 this.responsible = new Reference(); // cc 1574 return this.responsible; 1575 } 1576 1577 public boolean hasResponsible() { 1578 return this.responsible != null && !this.responsible.isEmpty(); 1579 } 1580 1581 /** 1582 * @param value {@link #responsible} (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) 1583 */ 1584 public MessageHeader setResponsible(Reference value) { 1585 this.responsible = value; 1586 return this; 1587 } 1588 1589 /** 1590 * @return {@link #reason} (Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.) 1591 */ 1592 public CodeableConcept getReason() { 1593 if (this.reason == null) 1594 if (Configuration.errorOnAutoCreate()) 1595 throw new Error("Attempt to auto-create MessageHeader.reason"); 1596 else if (Configuration.doAutoCreate()) 1597 this.reason = new CodeableConcept(); // cc 1598 return this.reason; 1599 } 1600 1601 public boolean hasReason() { 1602 return this.reason != null && !this.reason.isEmpty(); 1603 } 1604 1605 /** 1606 * @param value {@link #reason} (Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.) 1607 */ 1608 public MessageHeader setReason(CodeableConcept value) { 1609 this.reason = value; 1610 return this; 1611 } 1612 1613 /** 1614 * @return {@link #response} (Information about the message that this message is a response to. Only present if this message is a response.) 1615 */ 1616 public MessageHeaderResponseComponent getResponse() { 1617 if (this.response == null) 1618 if (Configuration.errorOnAutoCreate()) 1619 throw new Error("Attempt to auto-create MessageHeader.response"); 1620 else if (Configuration.doAutoCreate()) 1621 this.response = new MessageHeaderResponseComponent(); // cc 1622 return this.response; 1623 } 1624 1625 public boolean hasResponse() { 1626 return this.response != null && !this.response.isEmpty(); 1627 } 1628 1629 /** 1630 * @param value {@link #response} (Information about the message that this message is a response to. Only present if this message is a response.) 1631 */ 1632 public MessageHeader setResponse(MessageHeaderResponseComponent value) { 1633 this.response = value; 1634 return this; 1635 } 1636 1637 /** 1638 * @return {@link #focus} (The actual data of the message - a reference to the root/focus class of the event. This is allowed to be a Parameters resource.) 1639 */ 1640 public List<Reference> getFocus() { 1641 if (this.focus == null) 1642 this.focus = new ArrayList<Reference>(); 1643 return this.focus; 1644 } 1645 1646 /** 1647 * @return Returns a reference to <code>this</code> for easy method chaining 1648 */ 1649 public MessageHeader setFocus(List<Reference> theFocus) { 1650 this.focus = theFocus; 1651 return this; 1652 } 1653 1654 public boolean hasFocus() { 1655 if (this.focus == null) 1656 return false; 1657 for (Reference item : this.focus) 1658 if (!item.isEmpty()) 1659 return true; 1660 return false; 1661 } 1662 1663 public Reference addFocus() { //3 1664 Reference t = new Reference(); 1665 if (this.focus == null) 1666 this.focus = new ArrayList<Reference>(); 1667 this.focus.add(t); 1668 return t; 1669 } 1670 1671 public MessageHeader addFocus(Reference t) { //3 1672 if (t == null) 1673 return this; 1674 if (this.focus == null) 1675 this.focus = new ArrayList<Reference>(); 1676 this.focus.add(t); 1677 return this; 1678 } 1679 1680 /** 1681 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 1682 */ 1683 public Reference getFocusFirstRep() { 1684 if (getFocus().isEmpty()) { 1685 addFocus(); 1686 } 1687 return getFocus().get(0); 1688 } 1689 1690 /** 1691 * @return {@link #definition} (Permanent link to the MessageDefinition for this message.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1692 */ 1693 public CanonicalType getDefinitionElement() { 1694 if (this.definition == null) 1695 if (Configuration.errorOnAutoCreate()) 1696 throw new Error("Attempt to auto-create MessageHeader.definition"); 1697 else if (Configuration.doAutoCreate()) 1698 this.definition = new CanonicalType(); // bb 1699 return this.definition; 1700 } 1701 1702 public boolean hasDefinitionElement() { 1703 return this.definition != null && !this.definition.isEmpty(); 1704 } 1705 1706 public boolean hasDefinition() { 1707 return this.definition != null && !this.definition.isEmpty(); 1708 } 1709 1710 /** 1711 * @param value {@link #definition} (Permanent link to the MessageDefinition for this message.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1712 */ 1713 public MessageHeader setDefinitionElement(CanonicalType value) { 1714 this.definition = value; 1715 return this; 1716 } 1717 1718 /** 1719 * @return Permanent link to the MessageDefinition for this message. 1720 */ 1721 public String getDefinition() { 1722 return this.definition == null ? null : this.definition.getValue(); 1723 } 1724 1725 /** 1726 * @param value Permanent link to the MessageDefinition for this message. 1727 */ 1728 public MessageHeader setDefinition(String value) { 1729 if (Utilities.noString(value)) 1730 this.definition = null; 1731 else { 1732 if (this.definition == null) 1733 this.definition = new CanonicalType(); 1734 this.definition.setValue(value); 1735 } 1736 return this; 1737 } 1738 1739 protected void listChildren(List<Property> children) { 1740 super.listChildren(children); 1741 children.add(new Property("event[x]", "Coding|canonical(EventDefinition)", "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.", 0, 1, event)); 1742 children.add(new Property("destination", "", "The destination application which the message is intended for.", 0, java.lang.Integer.MAX_VALUE, destination)); 1743 children.add(new Property("sender", "Reference(Practitioner|PractitionerRole|Device|Organization)", "Identifies the sending system to allow the use of a trust relationship.", 0, 1, sender)); 1744 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Device|Organization)", "The logical author of the message - the personor device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.", 0, 1, author)); 1745 children.add(new Property("source", "", "The source application from which this message originated.", 0, 1, source)); 1746 children.add(new Property("responsible", "Reference(Practitioner|PractitionerRole|Organization)", "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.", 0, 1, responsible)); 1747 children.add(new Property("reason", "CodeableConcept", "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.", 0, 1, reason)); 1748 children.add(new Property("response", "", "Information about the message that this message is a response to. Only present if this message is a response.", 0, 1, response)); 1749 children.add(new Property("focus", "Reference(Any)", "The actual data of the message - a reference to the root/focus class of the event. This is allowed to be a Parameters resource.", 0, java.lang.Integer.MAX_VALUE, focus)); 1750 children.add(new Property("definition", "canonical(MessageDefinition)", "Permanent link to the MessageDefinition for this message.", 0, 1, definition)); 1751 } 1752 1753 @Override 1754 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1755 switch (_hash) { 1756 case 278115238: /*event[x]*/ return new Property("event[x]", "Coding|canonical(EventDefinition)", "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.", 0, 1, event); 1757 case 96891546: /*event*/ return new Property("event[x]", "Coding|canonical(EventDefinition)", "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.", 0, 1, event); 1758 case -355957084: /*eventCoding*/ return new Property("event[x]", "Coding", "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.", 0, 1, event); 1759 case 1784258426: /*eventCanonical*/ return new Property("event[x]", "canonical(EventDefinition)", "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification are defined by the implementation. Alternatively a canonical uri to the EventDefinition.", 0, 1, event); 1760 case -1429847026: /*destination*/ return new Property("destination", "", "The destination application which the message is intended for.", 0, java.lang.Integer.MAX_VALUE, destination); 1761 case -905962955: /*sender*/ return new Property("sender", "Reference(Practitioner|PractitionerRole|Device|Organization)", "Identifies the sending system to allow the use of a trust relationship.", 0, 1, sender); 1762 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Device|Organization)", "The logical author of the message - the personor device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.", 0, 1, author); 1763 case -896505829: /*source*/ return new Property("source", "", "The source application from which this message originated.", 0, 1, source); 1764 case 1847674614: /*responsible*/ return new Property("responsible", "Reference(Practitioner|PractitionerRole|Organization)", "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.", 0, 1, responsible); 1765 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.", 0, 1, reason); 1766 case -340323263: /*response*/ return new Property("response", "", "Information about the message that this message is a response to. Only present if this message is a response.", 0, 1, response); 1767 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The actual data of the message - a reference to the root/focus class of the event. This is allowed to be a Parameters resource.", 0, java.lang.Integer.MAX_VALUE, focus); 1768 case -1014418093: /*definition*/ return new Property("definition", "canonical(MessageDefinition)", "Permanent link to the MessageDefinition for this message.", 0, 1, definition); 1769 default: return super.getNamedProperty(_hash, _name, _checkValid); 1770 } 1771 1772 } 1773 1774 @Override 1775 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1776 switch (hash) { 1777 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // DataType 1778 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : this.destination.toArray(new Base[this.destination.size()]); // MessageDestinationComponent 1779 case -905962955: /*sender*/ return this.sender == null ? new Base[0] : new Base[] {this.sender}; // Reference 1780 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1781 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // MessageSourceComponent 1782 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // Reference 1783 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1784 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // MessageHeaderResponseComponent 1785 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 1786 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CanonicalType 1787 default: return super.getProperty(hash, name, checkValid); 1788 } 1789 1790 } 1791 1792 @Override 1793 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1794 switch (hash) { 1795 case 96891546: // event 1796 this.event = TypeConvertor.castToType(value); // DataType 1797 return value; 1798 case -1429847026: // destination 1799 this.getDestination().add((MessageDestinationComponent) value); // MessageDestinationComponent 1800 return value; 1801 case -905962955: // sender 1802 this.sender = TypeConvertor.castToReference(value); // Reference 1803 return value; 1804 case -1406328437: // author 1805 this.author = TypeConvertor.castToReference(value); // Reference 1806 return value; 1807 case -896505829: // source 1808 this.source = (MessageSourceComponent) value; // MessageSourceComponent 1809 return value; 1810 case 1847674614: // responsible 1811 this.responsible = TypeConvertor.castToReference(value); // Reference 1812 return value; 1813 case -934964668: // reason 1814 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1815 return value; 1816 case -340323263: // response 1817 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 1818 return value; 1819 case 97604824: // focus 1820 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 1821 return value; 1822 case -1014418093: // definition 1823 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 1824 return value; 1825 default: return super.setProperty(hash, name, value); 1826 } 1827 1828 } 1829 1830 @Override 1831 public Base setProperty(String name, Base value) throws FHIRException { 1832 if (name.equals("event[x]")) { 1833 this.event = TypeConvertor.castToType(value); // DataType 1834 } else if (name.equals("destination")) { 1835 this.getDestination().add((MessageDestinationComponent) value); 1836 } else if (name.equals("sender")) { 1837 this.sender = TypeConvertor.castToReference(value); // Reference 1838 } else if (name.equals("author")) { 1839 this.author = TypeConvertor.castToReference(value); // Reference 1840 } else if (name.equals("source")) { 1841 this.source = (MessageSourceComponent) value; // MessageSourceComponent 1842 } else if (name.equals("responsible")) { 1843 this.responsible = TypeConvertor.castToReference(value); // Reference 1844 } else if (name.equals("reason")) { 1845 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1846 } else if (name.equals("response")) { 1847 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 1848 } else if (name.equals("focus")) { 1849 this.getFocus().add(TypeConvertor.castToReference(value)); 1850 } else if (name.equals("definition")) { 1851 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 1852 } else 1853 return super.setProperty(name, value); 1854 return value; 1855 } 1856 1857 @Override 1858 public void removeChild(String name, Base value) throws FHIRException { 1859 if (name.equals("event[x]")) { 1860 this.event = null; 1861 } else if (name.equals("destination")) { 1862 this.getDestination().remove((MessageDestinationComponent) value); 1863 } else if (name.equals("sender")) { 1864 this.sender = null; 1865 } else if (name.equals("author")) { 1866 this.author = null; 1867 } else if (name.equals("source")) { 1868 this.source = (MessageSourceComponent) value; // MessageSourceComponent 1869 } else if (name.equals("responsible")) { 1870 this.responsible = null; 1871 } else if (name.equals("reason")) { 1872 this.reason = null; 1873 } else if (name.equals("response")) { 1874 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 1875 } else if (name.equals("focus")) { 1876 this.getFocus().remove(value); 1877 } else if (name.equals("definition")) { 1878 this.definition = null; 1879 } else 1880 super.removeChild(name, value); 1881 1882 } 1883 1884 @Override 1885 public Base makeProperty(int hash, String name) throws FHIRException { 1886 switch (hash) { 1887 case 278115238: return getEvent(); 1888 case 96891546: return getEvent(); 1889 case -1429847026: return addDestination(); 1890 case -905962955: return getSender(); 1891 case -1406328437: return getAuthor(); 1892 case -896505829: return getSource(); 1893 case 1847674614: return getResponsible(); 1894 case -934964668: return getReason(); 1895 case -340323263: return getResponse(); 1896 case 97604824: return addFocus(); 1897 case -1014418093: return getDefinitionElement(); 1898 default: return super.makeProperty(hash, name); 1899 } 1900 1901 } 1902 1903 @Override 1904 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1905 switch (hash) { 1906 case 96891546: /*event*/ return new String[] {"Coding", "canonical"}; 1907 case -1429847026: /*destination*/ return new String[] {}; 1908 case -905962955: /*sender*/ return new String[] {"Reference"}; 1909 case -1406328437: /*author*/ return new String[] {"Reference"}; 1910 case -896505829: /*source*/ return new String[] {}; 1911 case 1847674614: /*responsible*/ return new String[] {"Reference"}; 1912 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1913 case -340323263: /*response*/ return new String[] {}; 1914 case 97604824: /*focus*/ return new String[] {"Reference"}; 1915 case -1014418093: /*definition*/ return new String[] {"canonical"}; 1916 default: return super.getTypesForProperty(hash, name); 1917 } 1918 1919 } 1920 1921 @Override 1922 public Base addChild(String name) throws FHIRException { 1923 if (name.equals("eventCoding")) { 1924 this.event = new Coding(); 1925 return this.event; 1926 } 1927 else if (name.equals("eventCanonical")) { 1928 this.event = new CanonicalType(); 1929 return this.event; 1930 } 1931 else if (name.equals("destination")) { 1932 return addDestination(); 1933 } 1934 else if (name.equals("sender")) { 1935 this.sender = new Reference(); 1936 return this.sender; 1937 } 1938 else if (name.equals("author")) { 1939 this.author = new Reference(); 1940 return this.author; 1941 } 1942 else if (name.equals("source")) { 1943 this.source = new MessageSourceComponent(); 1944 return this.source; 1945 } 1946 else if (name.equals("responsible")) { 1947 this.responsible = new Reference(); 1948 return this.responsible; 1949 } 1950 else if (name.equals("reason")) { 1951 this.reason = new CodeableConcept(); 1952 return this.reason; 1953 } 1954 else if (name.equals("response")) { 1955 this.response = new MessageHeaderResponseComponent(); 1956 return this.response; 1957 } 1958 else if (name.equals("focus")) { 1959 return addFocus(); 1960 } 1961 else if (name.equals("definition")) { 1962 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.definition"); 1963 } 1964 else 1965 return super.addChild(name); 1966 } 1967 1968 public String fhirType() { 1969 return "MessageHeader"; 1970 1971 } 1972 1973 public MessageHeader copy() { 1974 MessageHeader dst = new MessageHeader(); 1975 copyValues(dst); 1976 return dst; 1977 } 1978 1979 public void copyValues(MessageHeader dst) { 1980 super.copyValues(dst); 1981 dst.event = event == null ? null : event.copy(); 1982 if (destination != null) { 1983 dst.destination = new ArrayList<MessageDestinationComponent>(); 1984 for (MessageDestinationComponent i : destination) 1985 dst.destination.add(i.copy()); 1986 }; 1987 dst.sender = sender == null ? null : sender.copy(); 1988 dst.author = author == null ? null : author.copy(); 1989 dst.source = source == null ? null : source.copy(); 1990 dst.responsible = responsible == null ? null : responsible.copy(); 1991 dst.reason = reason == null ? null : reason.copy(); 1992 dst.response = response == null ? null : response.copy(); 1993 if (focus != null) { 1994 dst.focus = new ArrayList<Reference>(); 1995 for (Reference i : focus) 1996 dst.focus.add(i.copy()); 1997 }; 1998 dst.definition = definition == null ? null : definition.copy(); 1999 } 2000 2001 protected MessageHeader typedCopy() { 2002 return copy(); 2003 } 2004 2005 @Override 2006 public boolean equalsDeep(Base other_) { 2007 if (!super.equalsDeep(other_)) 2008 return false; 2009 if (!(other_ instanceof MessageHeader)) 2010 return false; 2011 MessageHeader o = (MessageHeader) other_; 2012 return compareDeep(event, o.event, true) && compareDeep(destination, o.destination, true) && compareDeep(sender, o.sender, true) 2013 && compareDeep(author, o.author, true) && compareDeep(source, o.source, true) && compareDeep(responsible, o.responsible, true) 2014 && compareDeep(reason, o.reason, true) && compareDeep(response, o.response, true) && compareDeep(focus, o.focus, true) 2015 && compareDeep(definition, o.definition, true); 2016 } 2017 2018 @Override 2019 public boolean equalsShallow(Base other_) { 2020 if (!super.equalsShallow(other_)) 2021 return false; 2022 if (!(other_ instanceof MessageHeader)) 2023 return false; 2024 MessageHeader o = (MessageHeader) other_; 2025 return compareValues(definition, o.definition, true); 2026 } 2027 2028 public boolean isEmpty() { 2029 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(event, destination, sender 2030 , author, source, responsible, reason, response, focus, definition); 2031 } 2032 2033 @Override 2034 public ResourceType getResourceType() { 2035 return ResourceType.MessageHeader; 2036 } 2037 2038 /** 2039 * Search parameter: <b>author</b> 2040 * <p> 2041 * Description: <b>The source of the decision</b><br> 2042 * Type: <b>reference</b><br> 2043 * Path: <b>MessageHeader.author</b><br> 2044 * </p> 2045 */ 2046 @SearchParamDefinition(name="author", path="MessageHeader.author", description="The source of the decision", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Practitioner.class, PractitionerRole.class } ) 2047 public static final String SP_AUTHOR = "author"; 2048 /** 2049 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2050 * <p> 2051 * Description: <b>The source of the decision</b><br> 2052 * Type: <b>reference</b><br> 2053 * Path: <b>MessageHeader.author</b><br> 2054 * </p> 2055 */ 2056 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2057 2058/** 2059 * Constant for fluent queries to be used to add include statements. Specifies 2060 * the path value of "<b>MessageHeader:author</b>". 2061 */ 2062 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("MessageHeader:author").toLocked(); 2063 2064 /** 2065 * Search parameter: <b>code</b> 2066 * <p> 2067 * Description: <b>ok | transient-error | fatal-error</b><br> 2068 * Type: <b>token</b><br> 2069 * Path: <b>MessageHeader.response.code</b><br> 2070 * </p> 2071 */ 2072 @SearchParamDefinition(name="code", path="MessageHeader.response.code", description="ok | transient-error | fatal-error", type="token" ) 2073 public static final String SP_CODE = "code"; 2074 /** 2075 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2076 * <p> 2077 * Description: <b>ok | transient-error | fatal-error</b><br> 2078 * Type: <b>token</b><br> 2079 * Path: <b>MessageHeader.response.code</b><br> 2080 * </p> 2081 */ 2082 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2083 2084 /** 2085 * Search parameter: <b>destination</b> 2086 * <p> 2087 * Description: <b>Name of system</b><br> 2088 * Type: <b>string</b><br> 2089 * Path: <b>MessageHeader.destination.name</b><br> 2090 * </p> 2091 */ 2092 @SearchParamDefinition(name="destination", path="MessageHeader.destination.name", description="Name of system", type="string" ) 2093 public static final String SP_DESTINATION = "destination"; 2094 /** 2095 * <b>Fluent Client</b> search parameter constant for <b>destination</b> 2096 * <p> 2097 * Description: <b>Name of system</b><br> 2098 * Type: <b>string</b><br> 2099 * Path: <b>MessageHeader.destination.name</b><br> 2100 * </p> 2101 */ 2102 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESTINATION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESTINATION); 2103 2104 /** 2105 * Search parameter: <b>event</b> 2106 * <p> 2107 * Description: <b>Code for the event this message represents or link to event definition</b><br> 2108 * Type: <b>token</b><br> 2109 * Path: <b>MessageHeader.event.ofType(Coding) | MessageHeader.event.ofType(canonical)</b><br> 2110 * </p> 2111 */ 2112 @SearchParamDefinition(name="event", path="MessageHeader.event.ofType(Coding) | MessageHeader.event.ofType(canonical)", description="Code for the event this message represents or link to event definition", type="token" ) 2113 public static final String SP_EVENT = "event"; 2114 /** 2115 * <b>Fluent Client</b> search parameter constant for <b>event</b> 2116 * <p> 2117 * Description: <b>Code for the event this message represents or link to event definition</b><br> 2118 * Type: <b>token</b><br> 2119 * Path: <b>MessageHeader.event.ofType(Coding) | MessageHeader.event.ofType(canonical)</b><br> 2120 * </p> 2121 */ 2122 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 2123 2124 /** 2125 * Search parameter: <b>focus</b> 2126 * <p> 2127 * Description: <b>The actual content of the message</b><br> 2128 * Type: <b>reference</b><br> 2129 * Path: <b>MessageHeader.focus</b><br> 2130 * </p> 2131 */ 2132 @SearchParamDefinition(name="focus", path="MessageHeader.focus", description="The actual content of the message", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2133 public static final String SP_FOCUS = "focus"; 2134 /** 2135 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 2136 * <p> 2137 * Description: <b>The actual content of the message</b><br> 2138 * Type: <b>reference</b><br> 2139 * Path: <b>MessageHeader.focus</b><br> 2140 * </p> 2141 */ 2142 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 2143 2144/** 2145 * Constant for fluent queries to be used to add include statements. Specifies 2146 * the path value of "<b>MessageHeader:focus</b>". 2147 */ 2148 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("MessageHeader:focus").toLocked(); 2149 2150 /** 2151 * Search parameter: <b>receiver</b> 2152 * <p> 2153 * Description: <b>Intended "real-world" recipient for the data</b><br> 2154 * Type: <b>reference</b><br> 2155 * Path: <b>MessageHeader.destination.receiver</b><br> 2156 * </p> 2157 */ 2158 @SearchParamDefinition(name="receiver", path="MessageHeader.destination.receiver", description="Intended \"real-world\" recipient for the data", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 2159 public static final String SP_RECEIVER = "receiver"; 2160 /** 2161 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 2162 * <p> 2163 * Description: <b>Intended "real-world" recipient for the data</b><br> 2164 * Type: <b>reference</b><br> 2165 * Path: <b>MessageHeader.destination.receiver</b><br> 2166 * </p> 2167 */ 2168 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECEIVER); 2169 2170/** 2171 * Constant for fluent queries to be used to add include statements. Specifies 2172 * the path value of "<b>MessageHeader:receiver</b>". 2173 */ 2174 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include("MessageHeader:receiver").toLocked(); 2175 2176 /** 2177 * Search parameter: <b>response-id</b> 2178 * <p> 2179 * Description: <b>Id of original message</b><br> 2180 * Type: <b>token</b><br> 2181 * Path: <b>MessageHeader.response.identifier</b><br> 2182 * </p> 2183 */ 2184 @SearchParamDefinition(name="response-id", path="MessageHeader.response.identifier", description="Id of original message", type="token" ) 2185 public static final String SP_RESPONSE_ID = "response-id"; 2186 /** 2187 * <b>Fluent Client</b> search parameter constant for <b>response-id</b> 2188 * <p> 2189 * Description: <b>Id of original message</b><br> 2190 * Type: <b>token</b><br> 2191 * Path: <b>MessageHeader.response.identifier</b><br> 2192 * </p> 2193 */ 2194 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESPONSE_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESPONSE_ID); 2195 2196 /** 2197 * Search parameter: <b>responsible</b> 2198 * <p> 2199 * Description: <b>Final responsibility for event</b><br> 2200 * Type: <b>reference</b><br> 2201 * Path: <b>MessageHeader.responsible</b><br> 2202 * </p> 2203 */ 2204 @SearchParamDefinition(name="responsible", path="MessageHeader.responsible", description="Final responsibility for event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 2205 public static final String SP_RESPONSIBLE = "responsible"; 2206 /** 2207 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 2208 * <p> 2209 * Description: <b>Final responsibility for event</b><br> 2210 * Type: <b>reference</b><br> 2211 * Path: <b>MessageHeader.responsible</b><br> 2212 * </p> 2213 */ 2214 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESPONSIBLE); 2215 2216/** 2217 * Constant for fluent queries to be used to add include statements. Specifies 2218 * the path value of "<b>MessageHeader:responsible</b>". 2219 */ 2220 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSIBLE = new ca.uhn.fhir.model.api.Include("MessageHeader:responsible").toLocked(); 2221 2222 /** 2223 * Search parameter: <b>sender</b> 2224 * <p> 2225 * Description: <b>Real world sender of the message</b><br> 2226 * Type: <b>reference</b><br> 2227 * Path: <b>MessageHeader.sender</b><br> 2228 * </p> 2229 */ 2230 @SearchParamDefinition(name="sender", path="MessageHeader.sender", description="Real world sender of the message", type="reference", target={Device.class, Organization.class, Practitioner.class, PractitionerRole.class } ) 2231 public static final String SP_SENDER = "sender"; 2232 /** 2233 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 2234 * <p> 2235 * Description: <b>Real world sender of the message</b><br> 2236 * Type: <b>reference</b><br> 2237 * Path: <b>MessageHeader.sender</b><br> 2238 * </p> 2239 */ 2240 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SENDER); 2241 2242/** 2243 * Constant for fluent queries to be used to add include statements. Specifies 2244 * the path value of "<b>MessageHeader:sender</b>". 2245 */ 2246 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include("MessageHeader:sender").toLocked(); 2247 2248 /** 2249 * Search parameter: <b>source</b> 2250 * <p> 2251 * Description: <b>Name of system</b><br> 2252 * Type: <b>string</b><br> 2253 * Path: <b>MessageHeader.source.name</b><br> 2254 * </p> 2255 */ 2256 @SearchParamDefinition(name="source", path="MessageHeader.source.name", description="Name of system", type="string" ) 2257 public static final String SP_SOURCE = "source"; 2258 /** 2259 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2260 * <p> 2261 * Description: <b>Name of system</b><br> 2262 * Type: <b>string</b><br> 2263 * Path: <b>MessageHeader.source.name</b><br> 2264 * </p> 2265 */ 2266 public static final ca.uhn.fhir.rest.gclient.StringClientParam SOURCE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SOURCE); 2267 2268 /** 2269 * Search parameter: <b>target</b> 2270 * <p> 2271 * Description: <b>Particular delivery destination within the destination</b><br> 2272 * Type: <b>reference</b><br> 2273 * Path: <b>MessageHeader.destination.target</b><br> 2274 * </p> 2275 */ 2276 @SearchParamDefinition(name="target", path="MessageHeader.destination.target", description="Particular delivery destination within the destination", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 2277 public static final String SP_TARGET = "target"; 2278 /** 2279 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2280 * <p> 2281 * Description: <b>Particular delivery destination within the destination</b><br> 2282 * Type: <b>reference</b><br> 2283 * Path: <b>MessageHeader.destination.target</b><br> 2284 * </p> 2285 */ 2286 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET); 2287 2288/** 2289 * Constant for fluent queries to be used to add include statements. Specifies 2290 * the path value of "<b>MessageHeader:target</b>". 2291 */ 2292 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include("MessageHeader:target").toLocked(); 2293 2294 2295} 2296