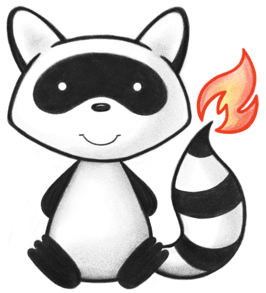
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import org.hl7.fhir.instance.model.api.IBaseMetaType; 049/** 050 * Meta Type: The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource. 051 */ 052@DatatypeDef(name="Meta") 053public class Meta extends DataType implements IBaseMetaType { 054 055 /** 056 * The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted. 057 */ 058 @Child(name = "versionId", type = {IdType.class}, order=0, min=0, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Version specific identifier", formalDefinition="The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted." ) 060 protected IdType versionId; 061 062 /** 063 * When the resource last changed - e.g. when the version changed. 064 */ 065 @Child(name = "lastUpdated", type = {InstantType.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="When the resource version last changed", formalDefinition="When the resource last changed - e.g. when the version changed." ) 067 protected InstantType lastUpdated; 068 069 /** 070 * A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc. 071 */ 072 @Child(name = "source", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 073 @Description(shortDefinition="Identifies where the resource comes from", formalDefinition="A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc." ) 074 protected UriType source; 075 076 /** 077 * A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url). 078 */ 079 @Child(name = "profile", type = {CanonicalType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 080 @Description(shortDefinition="Profiles this resource claims to conform to", formalDefinition="A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url)." ) 081 protected List<CanonicalType> profile; 082 083 /** 084 * Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure. 085 */ 086 @Child(name = "security", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 087 @Description(shortDefinition="Security Labels applied to this resource", formalDefinition="Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure." ) 088 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 089 protected List<Coding> security; 090 091 /** 092 * Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource. 093 */ 094 @Child(name = "tag", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 095 @Description(shortDefinition="Tags applied to this resource", formalDefinition="Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource." ) 096 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/common-tags") 097 protected List<Coding> tag; 098 099 private static final long serialVersionUID = -1386695622L; 100 101 /** 102 * Constructor 103 */ 104 public Meta() { 105 super(); 106 } 107 108 /** 109 * @return {@link #versionId} (The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted.). This is the underlying object with id, value and extensions. The accessor "getVersionId" gives direct access to the value 110 */ 111 public IdType getVersionIdElement() { 112 if (this.versionId == null) 113 if (Configuration.errorOnAutoCreate()) 114 throw new Error("Attempt to auto-create Meta.versionId"); 115 else if (Configuration.doAutoCreate()) 116 this.versionId = new IdType(); // bb 117 return this.versionId; 118 } 119 120 public boolean hasVersionIdElement() { 121 return this.versionId != null && !this.versionId.isEmpty(); 122 } 123 124 public boolean hasVersionId() { 125 return this.versionId != null && !this.versionId.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #versionId} (The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted.). This is the underlying object with id, value and extensions. The accessor "getVersionId" gives direct access to the value 130 */ 131 public Meta setVersionIdElement(IdType value) { 132 this.versionId = value; 133 return this; 134 } 135 136 /** 137 * @return The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted. 138 */ 139 public String getVersionId() { 140 return this.versionId == null ? null : this.versionId.getValue(); 141 } 142 143 /** 144 * @param value The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted. 145 */ 146 public Meta setVersionId(String value) { 147 if (Utilities.noString(value)) 148 this.versionId = null; 149 else { 150 if (this.versionId == null) 151 this.versionId = new IdType(); 152 this.versionId.setValue(value); 153 } 154 return this; 155 } 156 157 /** 158 * @return {@link #lastUpdated} (When the resource last changed - e.g. when the version changed.). This is the underlying object with id, value and extensions. The accessor "getLastUpdated" gives direct access to the value 159 */ 160 public InstantType getLastUpdatedElement() { 161 if (this.lastUpdated == null) 162 if (Configuration.errorOnAutoCreate()) 163 throw new Error("Attempt to auto-create Meta.lastUpdated"); 164 else if (Configuration.doAutoCreate()) 165 this.lastUpdated = new InstantType(); // bb 166 return this.lastUpdated; 167 } 168 169 public boolean hasLastUpdatedElement() { 170 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 171 } 172 173 public boolean hasLastUpdated() { 174 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #lastUpdated} (When the resource last changed - e.g. when the version changed.). This is the underlying object with id, value and extensions. The accessor "getLastUpdated" gives direct access to the value 179 */ 180 public Meta setLastUpdatedElement(InstantType value) { 181 this.lastUpdated = value; 182 return this; 183 } 184 185 /** 186 * @return When the resource last changed - e.g. when the version changed. 187 */ 188 public Date getLastUpdated() { 189 return this.lastUpdated == null ? null : this.lastUpdated.getValue(); 190 } 191 192 /** 193 * @param value When the resource last changed - e.g. when the version changed. 194 */ 195 public Meta setLastUpdated(Date value) { 196 if (value == null) 197 this.lastUpdated = null; 198 else { 199 if (this.lastUpdated == null) 200 this.lastUpdated = new InstantType(); 201 this.lastUpdated.setValue(value); 202 } 203 return this; 204 } 205 206 /** 207 * @return {@link #source} (A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 208 */ 209 public UriType getSourceElement() { 210 if (this.source == null) 211 if (Configuration.errorOnAutoCreate()) 212 throw new Error("Attempt to auto-create Meta.source"); 213 else if (Configuration.doAutoCreate()) 214 this.source = new UriType(); // bb 215 return this.source; 216 } 217 218 public boolean hasSourceElement() { 219 return this.source != null && !this.source.isEmpty(); 220 } 221 222 public boolean hasSource() { 223 return this.source != null && !this.source.isEmpty(); 224 } 225 226 /** 227 * @param value {@link #source} (A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 228 */ 229 public Meta setSourceElement(UriType value) { 230 this.source = value; 231 return this; 232 } 233 234 /** 235 * @return A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc. 236 */ 237 public String getSource() { 238 return this.source == null ? null : this.source.getValue(); 239 } 240 241 /** 242 * @param value A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc. 243 */ 244 public Meta setSource(String value) { 245 if (Utilities.noString(value)) 246 this.source = null; 247 else { 248 if (this.source == null) 249 this.source = new UriType(); 250 this.source.setValue(value); 251 } 252 return this; 253 } 254 255 /** 256 * @return {@link #profile} (A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url).) 257 */ 258 public List<CanonicalType> getProfile() { 259 if (this.profile == null) 260 this.profile = new ArrayList<CanonicalType>(); 261 return this.profile; 262 } 263 264 /** 265 * @return Returns a reference to <code>this</code> for easy method chaining 266 */ 267 public Meta setProfile(List<CanonicalType> theProfile) { 268 this.profile = theProfile; 269 return this; 270 } 271 272 public boolean hasProfile() { 273 if (this.profile == null) 274 return false; 275 for (CanonicalType item : this.profile) 276 if (!item.isEmpty()) 277 return true; 278 return false; 279 } 280 281 /** 282 * @return {@link #profile} (A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url).) 283 */ 284 public CanonicalType addProfileElement() {//2 285 CanonicalType t = new CanonicalType(); 286 if (this.profile == null) 287 this.profile = new ArrayList<CanonicalType>(); 288 this.profile.add(t); 289 return t; 290 } 291 292 /** 293 * @param value {@link #profile} (A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url).) 294 */ 295 public Meta addProfile(String value) { //1 296 CanonicalType t = new CanonicalType(); 297 t.setValue(value); 298 if (this.profile == null) 299 this.profile = new ArrayList<CanonicalType>(); 300 this.profile.add(t); 301 return this; 302 } 303 304 /** 305 * @param value {@link #profile} (A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url).) 306 */ 307 public boolean hasProfile(String value) { 308 if (this.profile == null) 309 return false; 310 for (CanonicalType v : this.profile) 311 if (v.getValue().equals(value)) // canonical 312 return true; 313 return false; 314 } 315 316 /** 317 * @return {@link #security} (Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.) 318 */ 319 public List<Coding> getSecurity() { 320 if (this.security == null) 321 this.security = new ArrayList<Coding>(); 322 return this.security; 323 } 324 325 /** 326 * @return Returns a reference to <code>this</code> for easy method chaining 327 */ 328 public Meta setSecurity(List<Coding> theSecurity) { 329 this.security = theSecurity; 330 return this; 331 } 332 333 public boolean hasSecurity() { 334 if (this.security == null) 335 return false; 336 for (Coding item : this.security) 337 if (!item.isEmpty()) 338 return true; 339 return false; 340 } 341 342 public Coding addSecurity() { //3 343 Coding t = new Coding(); 344 if (this.security == null) 345 this.security = new ArrayList<Coding>(); 346 this.security.add(t); 347 return t; 348 } 349 350 public Meta addSecurity(Coding t) { //3 351 if (t == null) 352 return this; 353 if (this.security == null) 354 this.security = new ArrayList<Coding>(); 355 this.security.add(t); 356 return this; 357 } 358 359 /** 360 * @return The first repetition of repeating field {@link #security}, creating it if it does not already exist {3} 361 */ 362 public Coding getSecurityFirstRep() { 363 if (getSecurity().isEmpty()) { 364 addSecurity(); 365 } 366 return getSecurity().get(0); 367 } 368 369 /** 370 * @return {@link #tag} (Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.) 371 */ 372 public List<Coding> getTag() { 373 if (this.tag == null) 374 this.tag = new ArrayList<Coding>(); 375 return this.tag; 376 } 377 378 /** 379 * @return Returns a reference to <code>this</code> for easy method chaining 380 */ 381 public Meta setTag(List<Coding> theTag) { 382 this.tag = theTag; 383 return this; 384 } 385 386 public boolean hasTag() { 387 if (this.tag == null) 388 return false; 389 for (Coding item : this.tag) 390 if (!item.isEmpty()) 391 return true; 392 return false; 393 } 394 395 public Coding addTag() { //3 396 Coding t = new Coding(); 397 if (this.tag == null) 398 this.tag = new ArrayList<Coding>(); 399 this.tag.add(t); 400 return t; 401 } 402 403 public Meta addTag(Coding t) { //3 404 if (t == null) 405 return this; 406 if (this.tag == null) 407 this.tag = new ArrayList<Coding>(); 408 this.tag.add(t); 409 return this; 410 } 411 412 /** 413 * @return The first repetition of repeating field {@link #tag}, creating it if it does not already exist {3} 414 */ 415 public Coding getTagFirstRep() { 416 if (getTag().isEmpty()) { 417 addTag(); 418 } 419 return getTag().get(0); 420 } 421 422 protected void listChildren(List<Property> children) { 423 super.listChildren(children); 424 children.add(new Property("versionId", "id", "The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted.", 0, 1, versionId)); 425 children.add(new Property("lastUpdated", "instant", "When the resource last changed - e.g. when the version changed.", 0, 1, lastUpdated)); 426 children.add(new Property("source", "uri", "A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc.", 0, 1, source)); 427 children.add(new Property("profile", "canonical(StructureDefinition)", "A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url).", 0, java.lang.Integer.MAX_VALUE, profile)); 428 children.add(new Property("security", "Coding", "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.", 0, java.lang.Integer.MAX_VALUE, security)); 429 children.add(new Property("tag", "Coding", "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.", 0, java.lang.Integer.MAX_VALUE, tag)); 430 } 431 432 @Override 433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 434 switch (_hash) { 435 case -1407102957: /*versionId*/ return new Property("versionId", "id", "The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted.", 0, 1, versionId); 436 case 1649733957: /*lastUpdated*/ return new Property("lastUpdated", "instant", "When the resource last changed - e.g. when the version changed.", 0, 1, lastUpdated); 437 case -896505829: /*source*/ return new Property("source", "uri", "A uri that identifies the source system of the resource. This provides a minimal amount of [Provenance](provenance.html#) information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc.", 0, 1, source); 438 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "A list of profiles (references to [StructureDefinition](structuredefinition.html#) resources) that this resource claims to conform to. The URL is a reference to [StructureDefinition.url](structuredefinition-definitions.html#StructureDefinition.url).", 0, java.lang.Integer.MAX_VALUE, profile); 439 case 949122880: /*security*/ return new Property("security", "Coding", "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.", 0, java.lang.Integer.MAX_VALUE, security); 440 case 114586: /*tag*/ return new Property("tag", "Coding", "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.", 0, java.lang.Integer.MAX_VALUE, tag); 441 default: return super.getNamedProperty(_hash, _name, _checkValid); 442 } 443 444 } 445 446 @Override 447 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 448 switch (hash) { 449 case -1407102957: /*versionId*/ return this.versionId == null ? new Base[0] : new Base[] {this.versionId}; // IdType 450 case 1649733957: /*lastUpdated*/ return this.lastUpdated == null ? new Base[0] : new Base[] {this.lastUpdated}; // InstantType 451 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // UriType 452 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 453 case 949122880: /*security*/ return this.security == null ? new Base[0] : this.security.toArray(new Base[this.security.size()]); // Coding 454 case 114586: /*tag*/ return this.tag == null ? new Base[0] : this.tag.toArray(new Base[this.tag.size()]); // Coding 455 default: return super.getProperty(hash, name, checkValid); 456 } 457 458 } 459 460 @Override 461 public Base setProperty(int hash, String name, Base value) throws FHIRException { 462 switch (hash) { 463 case -1407102957: // versionId 464 this.versionId = TypeConvertor.castToId(value); // IdType 465 return value; 466 case 1649733957: // lastUpdated 467 this.lastUpdated = TypeConvertor.castToInstant(value); // InstantType 468 return value; 469 case -896505829: // source 470 this.source = TypeConvertor.castToUri(value); // UriType 471 return value; 472 case -309425751: // profile 473 this.getProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 474 return value; 475 case 949122880: // security 476 this.getSecurity().add(TypeConvertor.castToCoding(value)); // Coding 477 return value; 478 case 114586: // tag 479 this.getTag().add(TypeConvertor.castToCoding(value)); // Coding 480 return value; 481 default: return super.setProperty(hash, name, value); 482 } 483 484 } 485 486 @Override 487 public Base setProperty(String name, Base value) throws FHIRException { 488 if (name.equals("versionId")) { 489 this.versionId = TypeConvertor.castToId(value); // IdType 490 } else if (name.equals("lastUpdated")) { 491 this.lastUpdated = TypeConvertor.castToInstant(value); // InstantType 492 } else if (name.equals("source")) { 493 this.source = TypeConvertor.castToUri(value); // UriType 494 } else if (name.equals("profile")) { 495 this.getProfile().add(TypeConvertor.castToCanonical(value)); 496 } else if (name.equals("security")) { 497 this.getSecurity().add(TypeConvertor.castToCoding(value)); 498 } else if (name.equals("tag")) { 499 this.getTag().add(TypeConvertor.castToCoding(value)); 500 } else 501 return super.setProperty(name, value); 502 return value; 503 } 504 505 @Override 506 public void removeChild(String name, Base value) throws FHIRException { 507 if (name.equals("versionId")) { 508 this.versionId = null; 509 } else if (name.equals("lastUpdated")) { 510 this.lastUpdated = null; 511 } else if (name.equals("source")) { 512 this.source = null; 513 } else if (name.equals("profile")) { 514 this.getProfile().remove(value); 515 } else if (name.equals("security")) { 516 this.getSecurity().remove(value); 517 } else if (name.equals("tag")) { 518 this.getTag().remove(value); 519 } else 520 super.removeChild(name, value); 521 522 } 523 524 @Override 525 public Base makeProperty(int hash, String name) throws FHIRException { 526 switch (hash) { 527 case -1407102957: return getVersionIdElement(); 528 case 1649733957: return getLastUpdatedElement(); 529 case -896505829: return getSourceElement(); 530 case -309425751: return addProfileElement(); 531 case 949122880: return addSecurity(); 532 case 114586: return addTag(); 533 default: return super.makeProperty(hash, name); 534 } 535 536 } 537 538 @Override 539 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 540 switch (hash) { 541 case -1407102957: /*versionId*/ return new String[] {"id"}; 542 case 1649733957: /*lastUpdated*/ return new String[] {"instant"}; 543 case -896505829: /*source*/ return new String[] {"uri"}; 544 case -309425751: /*profile*/ return new String[] {"canonical"}; 545 case 949122880: /*security*/ return new String[] {"Coding"}; 546 case 114586: /*tag*/ return new String[] {"Coding"}; 547 default: return super.getTypesForProperty(hash, name); 548 } 549 550 } 551 552 @Override 553 public Base addChild(String name) throws FHIRException { 554 if (name.equals("versionId")) { 555 throw new FHIRException("Cannot call addChild on a singleton property Meta.versionId"); 556 } 557 else if (name.equals("lastUpdated")) { 558 throw new FHIRException("Cannot call addChild on a singleton property Meta.lastUpdated"); 559 } 560 else if (name.equals("source")) { 561 throw new FHIRException("Cannot call addChild on a singleton property Meta.source"); 562 } 563 else if (name.equals("profile")) { 564 throw new FHIRException("Cannot call addChild on a singleton property Meta.profile"); 565 } 566 else if (name.equals("security")) { 567 return addSecurity(); 568 } 569 else if (name.equals("tag")) { 570 return addTag(); 571 } 572 else 573 return super.addChild(name); 574 } 575 576 public String fhirType() { 577 return "Meta"; 578 579 } 580 581 public Meta copy() { 582 Meta dst = new Meta(); 583 copyValues(dst); 584 return dst; 585 } 586 587 public void copyValues(Meta dst) { 588 super.copyValues(dst); 589 dst.versionId = versionId == null ? null : versionId.copy(); 590 dst.lastUpdated = lastUpdated == null ? null : lastUpdated.copy(); 591 dst.source = source == null ? null : source.copy(); 592 if (profile != null) { 593 dst.profile = new ArrayList<CanonicalType>(); 594 for (CanonicalType i : profile) 595 dst.profile.add(i.copy()); 596 }; 597 if (security != null) { 598 dst.security = new ArrayList<Coding>(); 599 for (Coding i : security) 600 dst.security.add(i.copy()); 601 }; 602 if (tag != null) { 603 dst.tag = new ArrayList<Coding>(); 604 for (Coding i : tag) 605 dst.tag.add(i.copy()); 606 }; 607 } 608 609 protected Meta typedCopy() { 610 return copy(); 611 } 612 613 @Override 614 public boolean equalsDeep(Base other_) { 615 if (!super.equalsDeep(other_)) 616 return false; 617 if (!(other_ instanceof Meta)) 618 return false; 619 Meta o = (Meta) other_; 620 return compareDeep(versionId, o.versionId, true) && compareDeep(lastUpdated, o.lastUpdated, true) 621 && compareDeep(source, o.source, true) && compareDeep(profile, o.profile, true) && compareDeep(security, o.security, true) 622 && compareDeep(tag, o.tag, true); 623 } 624 625 @Override 626 public boolean equalsShallow(Base other_) { 627 if (!super.equalsShallow(other_)) 628 return false; 629 if (!(other_ instanceof Meta)) 630 return false; 631 Meta o = (Meta) other_; 632 return compareValues(versionId, o.versionId, true) && compareValues(lastUpdated, o.lastUpdated, true) 633 && compareValues(source, o.source, true) && compareValues(profile, o.profile, true); 634 } 635 636 public boolean isEmpty() { 637 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(versionId, lastUpdated, source 638 , profile, security, tag); 639 } 640 641// Manual code (from Configuration.txt): 642/** 643 * Convenience method which adds a tag 644 * 645 * @param theSystem The code system 646 * @param theCode The code 647 * @param theDisplay The display name 648 * @return Returns a reference to <code>this</code> for easy chaining 649 */ 650 public Meta addTag(String theSystem, String theCode, String theDisplay) { 651 addTag().setSystem(theSystem).setCode(theCode).setDisplay(theDisplay); 652 return this; 653 } 654 655 /** 656 * Returns the first tag (if any) that has the given system and code, or returns 657 * <code>null</code> if none 658 */ 659 public Coding getTag(String theSystem, String theCode) { 660 for (Coding next : getTag()) { 661 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 662 return next; 663 } 664 } 665 return null; 666 } 667 668 /** 669 * Returns the first security label (if any) that has the given system and code, or returns 670 * <code>null</code> if none 671 */ 672 public Coding getSecurity(String theSystem, String theCode) { 673 for (Coding next : getSecurity()) { 674 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 675 return next; 676 } 677 } 678 return null; 679 } 680// end addition 681 682} 683