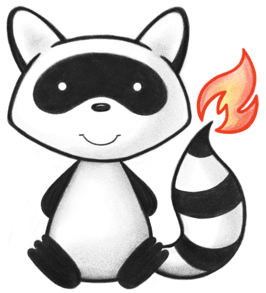
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Common Interface declaration for conformance and knowledge artifact resources. 051 */ 052public abstract class MetadataResource extends CanonicalResource { 053 054 private static final long serialVersionUID = 0L; 055 056 /** 057 * Constructor 058 */ 059 public MetadataResource() { 060 super(); 061 } 062 063 /** 064 * How many allowed for this property by the implementation 065 */ 066 public int getApprovalDateMax() { 067 return 1; 068 } 069 /** 070 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 071 */ 072 public abstract DateType getApprovalDateElement(); 073 074 public abstract boolean hasApprovalDateElement(); 075 public abstract boolean hasApprovalDate(); 076 077 /** 078 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 079 */ 080 public abstract MetadataResource setApprovalDateElement(DateType value); 081 /** 082 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 083 */ 084 public abstract Date getApprovalDate(); 085 /** 086 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 087 */ 088 public abstract MetadataResource setApprovalDate(Date value); 089 /** 090 * How many allowed for this property by the implementation 091 */ 092 public int getLastReviewDateMax() { 093 return 1; 094 } 095 /** 096 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 097 */ 098 public abstract DateType getLastReviewDateElement(); 099 100 public abstract boolean hasLastReviewDateElement(); 101 public abstract boolean hasLastReviewDate(); 102 103 /** 104 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 105 */ 106 public abstract MetadataResource setLastReviewDateElement(DateType value); 107 /** 108 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 109 */ 110 public abstract Date getLastReviewDate(); 111 /** 112 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 113 */ 114 public abstract MetadataResource setLastReviewDate(Date value); 115 /** 116 * How many allowed for this property by the implementation 117 */ 118 public int getEffectivePeriodMax() { 119 return 1; 120 } 121 /** 122 * @return {@link #effectivePeriod} (The period during which the metadata resource content was or is planned to be in active use.) 123 */ 124 public abstract Period getEffectivePeriod(); 125 public abstract boolean hasEffectivePeriod(); 126 /** 127 * @param value {@link #effectivePeriod} (The period during which the metadata resource content was or is planned to be in active use.) 128 */ 129 public abstract MetadataResource setEffectivePeriod(Period value); 130 131 /** 132 * How many allowed for this property by the implementation 133 */ 134 public int getTopicMax() { 135 return Integer.MAX_VALUE; 136 } 137 /** 138 * @return {@link #topic} (Descriptive topics related to the content of the metadata resource. Topics provide a high-level categorization as well as keywords for the metadata resource that can be useful for filtering and searching.) 139 */ 140 public abstract List<CodeableConcept> getTopic(); 141 /** 142 * @return Returns a reference to <code>this</code> for easy method chaining 143 */ 144 public abstract MetadataResource setTopic(List<CodeableConcept> theTopic); 145 public abstract boolean hasTopic(); 146 147 public abstract CodeableConcept addTopic(); //3 148 public abstract MetadataResource addTopic(CodeableConcept t); //3 149 /** 150 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {1} 151 */ 152 public abstract CodeableConcept getTopicFirstRep(); 153 /** 154 * How many allowed for this property by the implementation 155 */ 156 public int getAuthorMax() { 157 return Integer.MAX_VALUE; 158 } 159 /** 160 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the metadata resource.) 161 */ 162 public abstract List<ContactDetail> getAuthor(); 163 /** 164 * @return Returns a reference to <code>this</code> for easy method chaining 165 */ 166 public abstract MetadataResource setAuthor(List<ContactDetail> theAuthor); 167 public abstract boolean hasAuthor(); 168 169 public abstract ContactDetail addAuthor(); //3 170 public abstract MetadataResource addAuthor(ContactDetail t); //3 171 /** 172 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {1} 173 */ 174 public abstract ContactDetail getAuthorFirstRep(); 175 /** 176 * How many allowed for this property by the implementation 177 */ 178 public int getEditorMax() { 179 return Integer.MAX_VALUE; 180 } 181 /** 182 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the metadata resource.) 183 */ 184 public abstract List<ContactDetail> getEditor(); 185 /** 186 * @return Returns a reference to <code>this</code> for easy method chaining 187 */ 188 public abstract MetadataResource setEditor(List<ContactDetail> theEditor); 189 public abstract boolean hasEditor(); 190 191 public abstract ContactDetail addEditor(); //3 192 public abstract MetadataResource addEditor(ContactDetail t); //3 193 /** 194 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {1} 195 */ 196 public abstract ContactDetail getEditorFirstRep(); 197 /** 198 * How many allowed for this property by the implementation 199 */ 200 public int getReviewerMax() { 201 return Integer.MAX_VALUE; 202 } 203 /** 204 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the metadata resource.) 205 */ 206 public abstract List<ContactDetail> getReviewer(); 207 /** 208 * @return Returns a reference to <code>this</code> for easy method chaining 209 */ 210 public abstract MetadataResource setReviewer(List<ContactDetail> theReviewer); 211 public abstract boolean hasReviewer(); 212 213 public abstract ContactDetail addReviewer(); //3 214 public abstract MetadataResource addReviewer(ContactDetail t); //3 215 /** 216 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {1} 217 */ 218 public abstract ContactDetail getReviewerFirstRep(); 219 /** 220 * How many allowed for this property by the implementation 221 */ 222 public int getEndorserMax() { 223 return Integer.MAX_VALUE; 224 } 225 /** 226 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the metadata resource for use in some setting.) 227 */ 228 public abstract List<ContactDetail> getEndorser(); 229 /** 230 * @return Returns a reference to <code>this</code> for easy method chaining 231 */ 232 public abstract MetadataResource setEndorser(List<ContactDetail> theEndorser); 233 public abstract boolean hasEndorser(); 234 235 public abstract ContactDetail addEndorser(); //3 236 public abstract MetadataResource addEndorser(ContactDetail t); //3 237 /** 238 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {1} 239 */ 240 public abstract ContactDetail getEndorserFirstRep(); 241 /** 242 * How many allowed for this property by the implementation 243 */ 244 public int getRelatedArtifactMax() { 245 return Integer.MAX_VALUE; 246 } 247 /** 248 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 249 */ 250 public abstract List<RelatedArtifact> getRelatedArtifact(); 251 /** 252 * @return Returns a reference to <code>this</code> for easy method chaining 253 */ 254 public abstract MetadataResource setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact); 255 public abstract boolean hasRelatedArtifact(); 256 257 public abstract RelatedArtifact addRelatedArtifact(); //3 258 public abstract MetadataResource addRelatedArtifact(RelatedArtifact t); //3 259 /** 260 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {1} 261 */ 262 public abstract RelatedArtifact getRelatedArtifactFirstRep(); 263 protected void listChildren(List<Property> children) { 264 super.listChildren(children); 265 } 266 267 @Override 268 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 269 switch (_hash) { 270 default: return super.getNamedProperty(_hash, _name, _checkValid); 271 } 272 273 } 274 275 @Override 276 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 277 switch (hash) { 278 default: return super.getProperty(hash, name, checkValid); 279 } 280 281 } 282 283 @Override 284 public Base setProperty(int hash, String name, Base value) throws FHIRException { 285 switch (hash) { 286 default: return super.setProperty(hash, name, value); 287 } 288 289 } 290 291 @Override 292 public Base setProperty(String name, Base value) throws FHIRException { 293 return super.setProperty(name, value); 294 } 295 296 @Override 297 public void removeChild(String name, Base value) throws FHIRException { 298 super.removeChild(name, value); 299 } 300 301 @Override 302 public Base makeProperty(int hash, String name) throws FHIRException { 303 switch (hash) { 304 default: return super.makeProperty(hash, name); 305 } 306 307 } 308 309 @Override 310 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 311 switch (hash) { 312 default: return super.getTypesForProperty(hash, name); 313 } 314 315 } 316 317 @Override 318 public Base addChild(String name) throws FHIRException { 319 return super.addChild(name); 320 } 321 322 public String fhirType() { 323 return "MetadataResource"; 324 325 } 326 327 public abstract MetadataResource copy(); 328 329 public void copyValues(MetadataResource dst) { 330 super.copyValues(dst); 331 } 332 333 @Override 334 public boolean equalsDeep(Base other_) { 335 if (!super.equalsDeep(other_)) 336 return false; 337 if (!(other_ instanceof MetadataResource)) 338 return false; 339 MetadataResource o = (MetadataResource) other_; 340 return true; 341 } 342 343 @Override 344 public boolean equalsShallow(Base other_) { 345 if (!super.equalsShallow(other_)) 346 return false; 347 if (!(other_ instanceof MetadataResource)) 348 return false; 349 MetadataResource o = (MetadataResource) other_; 350 return true; 351 } 352 353 public boolean isEmpty() { 354 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(); 355 } 356 357 358} 359