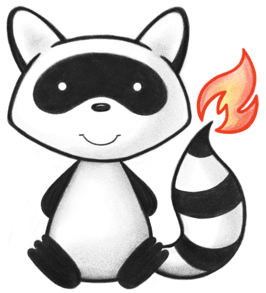
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Representation of a molecular sequence. 052 */ 053@ResourceDef(name="MolecularSequence", profile="http://hl7.org/fhir/StructureDefinition/MolecularSequence") 054public class MolecularSequence extends DomainResource { 055 056 public enum OrientationType { 057 /** 058 * Sense orientation of reference sequence. 059 */ 060 SENSE, 061 /** 062 * Antisense orientation of reference sequence. 063 */ 064 ANTISENSE, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static OrientationType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("sense".equals(codeString)) 073 return SENSE; 074 if ("antisense".equals(codeString)) 075 return ANTISENSE; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown OrientationType code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case SENSE: return "sense"; 084 case ANTISENSE: return "antisense"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case SENSE: return "http://hl7.org/fhir/orientation-type"; 092 case ANTISENSE: return "http://hl7.org/fhir/orientation-type"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case SENSE: return "Sense orientation of reference sequence."; 100 case ANTISENSE: return "Antisense orientation of reference sequence."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case SENSE: return "Sense orientation of referenceSeq"; 108 case ANTISENSE: return "Antisense orientation of referenceSeq"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class OrientationTypeEnumFactory implements EnumFactory<OrientationType> { 116 public OrientationType fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("sense".equals(codeString)) 121 return OrientationType.SENSE; 122 if ("antisense".equals(codeString)) 123 return OrientationType.ANTISENSE; 124 throw new IllegalArgumentException("Unknown OrientationType code '"+codeString+"'"); 125 } 126 public Enumeration<OrientationType> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<OrientationType>(this, OrientationType.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<OrientationType>(this, OrientationType.NULL, code); 134 if ("sense".equals(codeString)) 135 return new Enumeration<OrientationType>(this, OrientationType.SENSE, code); 136 if ("antisense".equals(codeString)) 137 return new Enumeration<OrientationType>(this, OrientationType.ANTISENSE, code); 138 throw new FHIRException("Unknown OrientationType code '"+codeString+"'"); 139 } 140 public String toCode(OrientationType code) { 141 if (code == OrientationType.NULL) 142 return null; 143 if (code == OrientationType.SENSE) 144 return "sense"; 145 if (code == OrientationType.ANTISENSE) 146 return "antisense"; 147 return "?"; 148 } 149 public String toSystem(OrientationType code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum SequenceType { 155 /** 156 * Amino acid sequence. 157 */ 158 AA, 159 /** 160 * DNA Sequence. 161 */ 162 DNA, 163 /** 164 * RNA Sequence. 165 */ 166 RNA, 167 /** 168 * added to help the parsers with the generic types 169 */ 170 NULL; 171 public static SequenceType fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("aa".equals(codeString)) 175 return AA; 176 if ("dna".equals(codeString)) 177 return DNA; 178 if ("rna".equals(codeString)) 179 return RNA; 180 if (Configuration.isAcceptInvalidEnums()) 181 return null; 182 else 183 throw new FHIRException("Unknown SequenceType code '"+codeString+"'"); 184 } 185 public String toCode() { 186 switch (this) { 187 case AA: return "aa"; 188 case DNA: return "dna"; 189 case RNA: return "rna"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case AA: return "http://hl7.org/fhir/sequence-type"; 197 case DNA: return "http://hl7.org/fhir/sequence-type"; 198 case RNA: return "http://hl7.org/fhir/sequence-type"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getDefinition() { 204 switch (this) { 205 case AA: return "Amino acid sequence."; 206 case DNA: return "DNA Sequence."; 207 case RNA: return "RNA Sequence."; 208 case NULL: return null; 209 default: return "?"; 210 } 211 } 212 public String getDisplay() { 213 switch (this) { 214 case AA: return "AA Sequence"; 215 case DNA: return "DNA Sequence"; 216 case RNA: return "RNA Sequence"; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 } 222 223 public static class SequenceTypeEnumFactory implements EnumFactory<SequenceType> { 224 public SequenceType fromCode(String codeString) throws IllegalArgumentException { 225 if (codeString == null || "".equals(codeString)) 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("aa".equals(codeString)) 229 return SequenceType.AA; 230 if ("dna".equals(codeString)) 231 return SequenceType.DNA; 232 if ("rna".equals(codeString)) 233 return SequenceType.RNA; 234 throw new IllegalArgumentException("Unknown SequenceType code '"+codeString+"'"); 235 } 236 public Enumeration<SequenceType> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<SequenceType>(this, SequenceType.NULL, code); 241 String codeString = ((PrimitiveType) code).asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<SequenceType>(this, SequenceType.NULL, code); 244 if ("aa".equals(codeString)) 245 return new Enumeration<SequenceType>(this, SequenceType.AA, code); 246 if ("dna".equals(codeString)) 247 return new Enumeration<SequenceType>(this, SequenceType.DNA, code); 248 if ("rna".equals(codeString)) 249 return new Enumeration<SequenceType>(this, SequenceType.RNA, code); 250 throw new FHIRException("Unknown SequenceType code '"+codeString+"'"); 251 } 252 public String toCode(SequenceType code) { 253 if (code == SequenceType.NULL) 254 return null; 255 if (code == SequenceType.AA) 256 return "aa"; 257 if (code == SequenceType.DNA) 258 return "dna"; 259 if (code == SequenceType.RNA) 260 return "rna"; 261 return "?"; 262 } 263 public String toSystem(SequenceType code) { 264 return code.getSystem(); 265 } 266 } 267 268 public enum StrandType { 269 /** 270 * Watson strand of starting sequence. 271 */ 272 WATSON, 273 /** 274 * Crick strand of starting sequence. 275 */ 276 CRICK, 277 /** 278 * added to help the parsers with the generic types 279 */ 280 NULL; 281 public static StrandType fromCode(String codeString) throws FHIRException { 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("watson".equals(codeString)) 285 return WATSON; 286 if ("crick".equals(codeString)) 287 return CRICK; 288 if (Configuration.isAcceptInvalidEnums()) 289 return null; 290 else 291 throw new FHIRException("Unknown StrandType code '"+codeString+"'"); 292 } 293 public String toCode() { 294 switch (this) { 295 case WATSON: return "watson"; 296 case CRICK: return "crick"; 297 case NULL: return null; 298 default: return "?"; 299 } 300 } 301 public String getSystem() { 302 switch (this) { 303 case WATSON: return "http://hl7.org/fhir/strand-type"; 304 case CRICK: return "http://hl7.org/fhir/strand-type"; 305 case NULL: return null; 306 default: return "?"; 307 } 308 } 309 public String getDefinition() { 310 switch (this) { 311 case WATSON: return "Watson strand of starting sequence."; 312 case CRICK: return "Crick strand of starting sequence."; 313 case NULL: return null; 314 default: return "?"; 315 } 316 } 317 public String getDisplay() { 318 switch (this) { 319 case WATSON: return "Watson strand of starting sequence"; 320 case CRICK: return "Crick strand of starting sequence"; 321 case NULL: return null; 322 default: return "?"; 323 } 324 } 325 } 326 327 public static class StrandTypeEnumFactory implements EnumFactory<StrandType> { 328 public StrandType fromCode(String codeString) throws IllegalArgumentException { 329 if (codeString == null || "".equals(codeString)) 330 if (codeString == null || "".equals(codeString)) 331 return null; 332 if ("watson".equals(codeString)) 333 return StrandType.WATSON; 334 if ("crick".equals(codeString)) 335 return StrandType.CRICK; 336 throw new IllegalArgumentException("Unknown StrandType code '"+codeString+"'"); 337 } 338 public Enumeration<StrandType> fromType(PrimitiveType<?> code) throws FHIRException { 339 if (code == null) 340 return null; 341 if (code.isEmpty()) 342 return new Enumeration<StrandType>(this, StrandType.NULL, code); 343 String codeString = ((PrimitiveType) code).asStringValue(); 344 if (codeString == null || "".equals(codeString)) 345 return new Enumeration<StrandType>(this, StrandType.NULL, code); 346 if ("watson".equals(codeString)) 347 return new Enumeration<StrandType>(this, StrandType.WATSON, code); 348 if ("crick".equals(codeString)) 349 return new Enumeration<StrandType>(this, StrandType.CRICK, code); 350 throw new FHIRException("Unknown StrandType code '"+codeString+"'"); 351 } 352 public String toCode(StrandType code) { 353 if (code == StrandType.NULL) 354 return null; 355 if (code == StrandType.WATSON) 356 return "watson"; 357 if (code == StrandType.CRICK) 358 return "crick"; 359 return "?"; 360 } 361 public String toSystem(StrandType code) { 362 return code.getSystem(); 363 } 364 } 365 366 @Block() 367 public static class MolecularSequenceRelativeComponent extends BackboneElement implements IBaseBackboneElement { 368 /** 369 * These are different ways of identifying nucleotides or amino acids within a sequence. Different databases and file types may use different systems. For detail definitions, see https://loinc.org/92822-6/ for more detail. 370 */ 371 @Child(name = "coordinateSystem", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 372 @Description(shortDefinition="Ways of identifying nucleotides or amino acids within a sequence", formalDefinition="These are different ways of identifying nucleotides or amino acids within a sequence. Different databases and file types may use different systems. For detail definitions, see https://loinc.org/92822-6/ for more detail." ) 373 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://loinc.org/LL5323-2/") 374 protected CodeableConcept coordinateSystem; 375 376 /** 377 * Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together. 378 */ 379 @Child(name = "ordinalPosition", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=false) 380 @Description(shortDefinition="Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together", formalDefinition="Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together." ) 381 protected IntegerType ordinalPosition; 382 383 /** 384 * Indicates the nucleotide range in the composed sequence when multiple 'relative' elements are used together. 385 */ 386 @Child(name = "sequenceRange", type = {Range.class}, order=3, min=0, max=1, modifier=false, summary=false) 387 @Description(shortDefinition="Indicates the nucleotide range in the composed sequence when multiple 'relative' elements are used together", formalDefinition="Indicates the nucleotide range in the composed sequence when multiple 'relative' elements are used together." ) 388 protected Range sequenceRange; 389 390 /** 391 * A sequence that is used as a starting sequence to describe variants that are present in a sequence analyzed. 392 */ 393 @Child(name = "startingSequence", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 394 @Description(shortDefinition="A sequence used as starting sequence", formalDefinition="A sequence that is used as a starting sequence to describe variants that are present in a sequence analyzed." ) 395 protected MolecularSequenceRelativeStartingSequenceComponent startingSequence; 396 397 /** 398 * Changes in sequence from the starting sequence. 399 */ 400 @Child(name = "edit", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 401 @Description(shortDefinition="Changes in sequence from the starting sequence", formalDefinition="Changes in sequence from the starting sequence." ) 402 protected List<MolecularSequenceRelativeEditComponent> edit; 403 404 private static final long serialVersionUID = -1455983973L; 405 406 /** 407 * Constructor 408 */ 409 public MolecularSequenceRelativeComponent() { 410 super(); 411 } 412 413 /** 414 * Constructor 415 */ 416 public MolecularSequenceRelativeComponent(CodeableConcept coordinateSystem) { 417 super(); 418 this.setCoordinateSystem(coordinateSystem); 419 } 420 421 /** 422 * @return {@link #coordinateSystem} (These are different ways of identifying nucleotides or amino acids within a sequence. Different databases and file types may use different systems. For detail definitions, see https://loinc.org/92822-6/ for more detail.) 423 */ 424 public CodeableConcept getCoordinateSystem() { 425 if (this.coordinateSystem == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create MolecularSequenceRelativeComponent.coordinateSystem"); 428 else if (Configuration.doAutoCreate()) 429 this.coordinateSystem = new CodeableConcept(); // cc 430 return this.coordinateSystem; 431 } 432 433 public boolean hasCoordinateSystem() { 434 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 435 } 436 437 /** 438 * @param value {@link #coordinateSystem} (These are different ways of identifying nucleotides or amino acids within a sequence. Different databases and file types may use different systems. For detail definitions, see https://loinc.org/92822-6/ for more detail.) 439 */ 440 public MolecularSequenceRelativeComponent setCoordinateSystem(CodeableConcept value) { 441 this.coordinateSystem = value; 442 return this; 443 } 444 445 /** 446 * @return {@link #ordinalPosition} (Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together.). This is the underlying object with id, value and extensions. The accessor "getOrdinalPosition" gives direct access to the value 447 */ 448 public IntegerType getOrdinalPositionElement() { 449 if (this.ordinalPosition == null) 450 if (Configuration.errorOnAutoCreate()) 451 throw new Error("Attempt to auto-create MolecularSequenceRelativeComponent.ordinalPosition"); 452 else if (Configuration.doAutoCreate()) 453 this.ordinalPosition = new IntegerType(); // bb 454 return this.ordinalPosition; 455 } 456 457 public boolean hasOrdinalPositionElement() { 458 return this.ordinalPosition != null && !this.ordinalPosition.isEmpty(); 459 } 460 461 public boolean hasOrdinalPosition() { 462 return this.ordinalPosition != null && !this.ordinalPosition.isEmpty(); 463 } 464 465 /** 466 * @param value {@link #ordinalPosition} (Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together.). This is the underlying object with id, value and extensions. The accessor "getOrdinalPosition" gives direct access to the value 467 */ 468 public MolecularSequenceRelativeComponent setOrdinalPositionElement(IntegerType value) { 469 this.ordinalPosition = value; 470 return this; 471 } 472 473 /** 474 * @return Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together. 475 */ 476 public int getOrdinalPosition() { 477 return this.ordinalPosition == null || this.ordinalPosition.isEmpty() ? 0 : this.ordinalPosition.getValue(); 478 } 479 480 /** 481 * @param value Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together. 482 */ 483 public MolecularSequenceRelativeComponent setOrdinalPosition(int value) { 484 if (this.ordinalPosition == null) 485 this.ordinalPosition = new IntegerType(); 486 this.ordinalPosition.setValue(value); 487 return this; 488 } 489 490 /** 491 * @return {@link #sequenceRange} (Indicates the nucleotide range in the composed sequence when multiple 'relative' elements are used together.) 492 */ 493 public Range getSequenceRange() { 494 if (this.sequenceRange == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create MolecularSequenceRelativeComponent.sequenceRange"); 497 else if (Configuration.doAutoCreate()) 498 this.sequenceRange = new Range(); // cc 499 return this.sequenceRange; 500 } 501 502 public boolean hasSequenceRange() { 503 return this.sequenceRange != null && !this.sequenceRange.isEmpty(); 504 } 505 506 /** 507 * @param value {@link #sequenceRange} (Indicates the nucleotide range in the composed sequence when multiple 'relative' elements are used together.) 508 */ 509 public MolecularSequenceRelativeComponent setSequenceRange(Range value) { 510 this.sequenceRange = value; 511 return this; 512 } 513 514 /** 515 * @return {@link #startingSequence} (A sequence that is used as a starting sequence to describe variants that are present in a sequence analyzed.) 516 */ 517 public MolecularSequenceRelativeStartingSequenceComponent getStartingSequence() { 518 if (this.startingSequence == null) 519 if (Configuration.errorOnAutoCreate()) 520 throw new Error("Attempt to auto-create MolecularSequenceRelativeComponent.startingSequence"); 521 else if (Configuration.doAutoCreate()) 522 this.startingSequence = new MolecularSequenceRelativeStartingSequenceComponent(); // cc 523 return this.startingSequence; 524 } 525 526 public boolean hasStartingSequence() { 527 return this.startingSequence != null && !this.startingSequence.isEmpty(); 528 } 529 530 /** 531 * @param value {@link #startingSequence} (A sequence that is used as a starting sequence to describe variants that are present in a sequence analyzed.) 532 */ 533 public MolecularSequenceRelativeComponent setStartingSequence(MolecularSequenceRelativeStartingSequenceComponent value) { 534 this.startingSequence = value; 535 return this; 536 } 537 538 /** 539 * @return {@link #edit} (Changes in sequence from the starting sequence.) 540 */ 541 public List<MolecularSequenceRelativeEditComponent> getEdit() { 542 if (this.edit == null) 543 this.edit = new ArrayList<MolecularSequenceRelativeEditComponent>(); 544 return this.edit; 545 } 546 547 /** 548 * @return Returns a reference to <code>this</code> for easy method chaining 549 */ 550 public MolecularSequenceRelativeComponent setEdit(List<MolecularSequenceRelativeEditComponent> theEdit) { 551 this.edit = theEdit; 552 return this; 553 } 554 555 public boolean hasEdit() { 556 if (this.edit == null) 557 return false; 558 for (MolecularSequenceRelativeEditComponent item : this.edit) 559 if (!item.isEmpty()) 560 return true; 561 return false; 562 } 563 564 public MolecularSequenceRelativeEditComponent addEdit() { //3 565 MolecularSequenceRelativeEditComponent t = new MolecularSequenceRelativeEditComponent(); 566 if (this.edit == null) 567 this.edit = new ArrayList<MolecularSequenceRelativeEditComponent>(); 568 this.edit.add(t); 569 return t; 570 } 571 572 public MolecularSequenceRelativeComponent addEdit(MolecularSequenceRelativeEditComponent t) { //3 573 if (t == null) 574 return this; 575 if (this.edit == null) 576 this.edit = new ArrayList<MolecularSequenceRelativeEditComponent>(); 577 this.edit.add(t); 578 return this; 579 } 580 581 /** 582 * @return The first repetition of repeating field {@link #edit}, creating it if it does not already exist {3} 583 */ 584 public MolecularSequenceRelativeEditComponent getEditFirstRep() { 585 if (getEdit().isEmpty()) { 586 addEdit(); 587 } 588 return getEdit().get(0); 589 } 590 591 protected void listChildren(List<Property> children) { 592 super.listChildren(children); 593 children.add(new Property("coordinateSystem", "CodeableConcept", "These are different ways of identifying nucleotides or amino acids within a sequence. Different databases and file types may use different systems. For detail definitions, see https://loinc.org/92822-6/ for more detail.", 0, 1, coordinateSystem)); 594 children.add(new Property("ordinalPosition", "integer", "Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together.", 0, 1, ordinalPosition)); 595 children.add(new Property("sequenceRange", "Range", "Indicates the nucleotide range in the composed sequence when multiple 'relative' elements are used together.", 0, 1, sequenceRange)); 596 children.add(new Property("startingSequence", "", "A sequence that is used as a starting sequence to describe variants that are present in a sequence analyzed.", 0, 1, startingSequence)); 597 children.add(new Property("edit", "", "Changes in sequence from the starting sequence.", 0, java.lang.Integer.MAX_VALUE, edit)); 598 } 599 600 @Override 601 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 602 switch (_hash) { 603 case 354212295: /*coordinateSystem*/ return new Property("coordinateSystem", "CodeableConcept", "These are different ways of identifying nucleotides or amino acids within a sequence. Different databases and file types may use different systems. For detail definitions, see https://loinc.org/92822-6/ for more detail.", 0, 1, coordinateSystem); 604 case 626439866: /*ordinalPosition*/ return new Property("ordinalPosition", "integer", "Indicates the order in which the sequence should be considered when putting multiple 'relative' elements together.", 0, 1, ordinalPosition); 605 case -733314564: /*sequenceRange*/ return new Property("sequenceRange", "Range", "Indicates the nucleotide range in the composed sequence when multiple 'relative' elements are used together.", 0, 1, sequenceRange); 606 case 1493400609: /*startingSequence*/ return new Property("startingSequence", "", "A sequence that is used as a starting sequence to describe variants that are present in a sequence analyzed.", 0, 1, startingSequence); 607 case 3108362: /*edit*/ return new Property("edit", "", "Changes in sequence from the starting sequence.", 0, java.lang.Integer.MAX_VALUE, edit); 608 default: return super.getNamedProperty(_hash, _name, _checkValid); 609 } 610 611 } 612 613 @Override 614 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 615 switch (hash) { 616 case 354212295: /*coordinateSystem*/ return this.coordinateSystem == null ? new Base[0] : new Base[] {this.coordinateSystem}; // CodeableConcept 617 case 626439866: /*ordinalPosition*/ return this.ordinalPosition == null ? new Base[0] : new Base[] {this.ordinalPosition}; // IntegerType 618 case -733314564: /*sequenceRange*/ return this.sequenceRange == null ? new Base[0] : new Base[] {this.sequenceRange}; // Range 619 case 1493400609: /*startingSequence*/ return this.startingSequence == null ? new Base[0] : new Base[] {this.startingSequence}; // MolecularSequenceRelativeStartingSequenceComponent 620 case 3108362: /*edit*/ return this.edit == null ? new Base[0] : this.edit.toArray(new Base[this.edit.size()]); // MolecularSequenceRelativeEditComponent 621 default: return super.getProperty(hash, name, checkValid); 622 } 623 624 } 625 626 @Override 627 public Base setProperty(int hash, String name, Base value) throws FHIRException { 628 switch (hash) { 629 case 354212295: // coordinateSystem 630 this.coordinateSystem = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 631 return value; 632 case 626439866: // ordinalPosition 633 this.ordinalPosition = TypeConvertor.castToInteger(value); // IntegerType 634 return value; 635 case -733314564: // sequenceRange 636 this.sequenceRange = TypeConvertor.castToRange(value); // Range 637 return value; 638 case 1493400609: // startingSequence 639 this.startingSequence = (MolecularSequenceRelativeStartingSequenceComponent) value; // MolecularSequenceRelativeStartingSequenceComponent 640 return value; 641 case 3108362: // edit 642 this.getEdit().add((MolecularSequenceRelativeEditComponent) value); // MolecularSequenceRelativeEditComponent 643 return value; 644 default: return super.setProperty(hash, name, value); 645 } 646 647 } 648 649 @Override 650 public Base setProperty(String name, Base value) throws FHIRException { 651 if (name.equals("coordinateSystem")) { 652 this.coordinateSystem = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 653 } else if (name.equals("ordinalPosition")) { 654 this.ordinalPosition = TypeConvertor.castToInteger(value); // IntegerType 655 } else if (name.equals("sequenceRange")) { 656 this.sequenceRange = TypeConvertor.castToRange(value); // Range 657 } else if (name.equals("startingSequence")) { 658 this.startingSequence = (MolecularSequenceRelativeStartingSequenceComponent) value; // MolecularSequenceRelativeStartingSequenceComponent 659 } else if (name.equals("edit")) { 660 this.getEdit().add((MolecularSequenceRelativeEditComponent) value); 661 } else 662 return super.setProperty(name, value); 663 return value; 664 } 665 666 @Override 667 public void removeChild(String name, Base value) throws FHIRException { 668 if (name.equals("coordinateSystem")) { 669 this.coordinateSystem = null; 670 } else if (name.equals("ordinalPosition")) { 671 this.ordinalPosition = null; 672 } else if (name.equals("sequenceRange")) { 673 this.sequenceRange = null; 674 } else if (name.equals("startingSequence")) { 675 this.startingSequence = (MolecularSequenceRelativeStartingSequenceComponent) value; // MolecularSequenceRelativeStartingSequenceComponent 676 } else if (name.equals("edit")) { 677 this.getEdit().remove((MolecularSequenceRelativeEditComponent) value); 678 } else 679 super.removeChild(name, value); 680 681 } 682 683 @Override 684 public Base makeProperty(int hash, String name) throws FHIRException { 685 switch (hash) { 686 case 354212295: return getCoordinateSystem(); 687 case 626439866: return getOrdinalPositionElement(); 688 case -733314564: return getSequenceRange(); 689 case 1493400609: return getStartingSequence(); 690 case 3108362: return addEdit(); 691 default: return super.makeProperty(hash, name); 692 } 693 694 } 695 696 @Override 697 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 698 switch (hash) { 699 case 354212295: /*coordinateSystem*/ return new String[] {"CodeableConcept"}; 700 case 626439866: /*ordinalPosition*/ return new String[] {"integer"}; 701 case -733314564: /*sequenceRange*/ return new String[] {"Range"}; 702 case 1493400609: /*startingSequence*/ return new String[] {}; 703 case 3108362: /*edit*/ return new String[] {}; 704 default: return super.getTypesForProperty(hash, name); 705 } 706 707 } 708 709 @Override 710 public Base addChild(String name) throws FHIRException { 711 if (name.equals("coordinateSystem")) { 712 this.coordinateSystem = new CodeableConcept(); 713 return this.coordinateSystem; 714 } 715 else if (name.equals("ordinalPosition")) { 716 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.ordinalPosition"); 717 } 718 else if (name.equals("sequenceRange")) { 719 this.sequenceRange = new Range(); 720 return this.sequenceRange; 721 } 722 else if (name.equals("startingSequence")) { 723 this.startingSequence = new MolecularSequenceRelativeStartingSequenceComponent(); 724 return this.startingSequence; 725 } 726 else if (name.equals("edit")) { 727 return addEdit(); 728 } 729 else 730 return super.addChild(name); 731 } 732 733 public MolecularSequenceRelativeComponent copy() { 734 MolecularSequenceRelativeComponent dst = new MolecularSequenceRelativeComponent(); 735 copyValues(dst); 736 return dst; 737 } 738 739 public void copyValues(MolecularSequenceRelativeComponent dst) { 740 super.copyValues(dst); 741 dst.coordinateSystem = coordinateSystem == null ? null : coordinateSystem.copy(); 742 dst.ordinalPosition = ordinalPosition == null ? null : ordinalPosition.copy(); 743 dst.sequenceRange = sequenceRange == null ? null : sequenceRange.copy(); 744 dst.startingSequence = startingSequence == null ? null : startingSequence.copy(); 745 if (edit != null) { 746 dst.edit = new ArrayList<MolecularSequenceRelativeEditComponent>(); 747 for (MolecularSequenceRelativeEditComponent i : edit) 748 dst.edit.add(i.copy()); 749 }; 750 } 751 752 @Override 753 public boolean equalsDeep(Base other_) { 754 if (!super.equalsDeep(other_)) 755 return false; 756 if (!(other_ instanceof MolecularSequenceRelativeComponent)) 757 return false; 758 MolecularSequenceRelativeComponent o = (MolecularSequenceRelativeComponent) other_; 759 return compareDeep(coordinateSystem, o.coordinateSystem, true) && compareDeep(ordinalPosition, o.ordinalPosition, true) 760 && compareDeep(sequenceRange, o.sequenceRange, true) && compareDeep(startingSequence, o.startingSequence, true) 761 && compareDeep(edit, o.edit, true); 762 } 763 764 @Override 765 public boolean equalsShallow(Base other_) { 766 if (!super.equalsShallow(other_)) 767 return false; 768 if (!(other_ instanceof MolecularSequenceRelativeComponent)) 769 return false; 770 MolecularSequenceRelativeComponent o = (MolecularSequenceRelativeComponent) other_; 771 return compareValues(ordinalPosition, o.ordinalPosition, true); 772 } 773 774 public boolean isEmpty() { 775 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coordinateSystem, ordinalPosition 776 , sequenceRange, startingSequence, edit); 777 } 778 779 public String fhirType() { 780 return "MolecularSequence.relative"; 781 782 } 783 784 } 785 786 @Block() 787 public static class MolecularSequenceRelativeStartingSequenceComponent extends BackboneElement implements IBaseBackboneElement { 788 /** 789 * The genome assembly used for starting sequence, e.g. GRCh38. 790 */ 791 @Child(name = "genomeAssembly", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 792 @Description(shortDefinition="The genome assembly used for starting sequence, e.g. GRCh38", formalDefinition="The genome assembly used for starting sequence, e.g. GRCh38." ) 793 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://loinc.org/LL1040-6/") 794 protected CodeableConcept genomeAssembly; 795 796 /** 797 * Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)). 798 */ 799 @Child(name = "chromosome", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 800 @Description(shortDefinition="Chromosome Identifier", formalDefinition="Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340))." ) 801 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://loinc.org/LL2938-0/") 802 protected CodeableConcept chromosome; 803 804 /** 805 * The reference sequence that represents the starting sequence. 806 */ 807 @Child(name = "sequence", type = {CodeableConcept.class, StringType.class, MolecularSequence.class}, order=3, min=0, max=1, modifier=false, summary=true) 808 @Description(shortDefinition="The reference sequence that represents the starting sequence", formalDefinition="The reference sequence that represents the starting sequence." ) 809 protected DataType sequence; 810 811 /** 812 * Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem. 813 */ 814 @Child(name = "windowStart", type = {IntegerType.class}, order=4, min=0, max=1, modifier=false, summary=true) 815 @Description(shortDefinition="Start position of the window on the starting sequence", formalDefinition="Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem." ) 816 protected IntegerType windowStart; 817 818 /** 819 * End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem. 820 */ 821 @Child(name = "windowEnd", type = {IntegerType.class}, order=5, min=0, max=1, modifier=false, summary=true) 822 @Description(shortDefinition="End position of the window on the starting sequence", formalDefinition="End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem." ) 823 protected IntegerType windowEnd; 824 825 /** 826 * A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the "sense" strand, and the opposite complementary strand is the "antisense" strand. 827 */ 828 @Child(name = "orientation", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 829 @Description(shortDefinition="sense | antisense", formalDefinition="A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand." ) 830 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/orientation-type") 831 protected Enumeration<OrientationType> orientation; 832 833 /** 834 * An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm. 835 */ 836 @Child(name = "strand", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 837 @Description(shortDefinition="watson | crick", formalDefinition="An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm." ) 838 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/strand-type") 839 protected Enumeration<StrandType> strand; 840 841 private static final long serialVersionUID = 502438613L; 842 843 /** 844 * Constructor 845 */ 846 public MolecularSequenceRelativeStartingSequenceComponent() { 847 super(); 848 } 849 850 /** 851 * @return {@link #genomeAssembly} (The genome assembly used for starting sequence, e.g. GRCh38.) 852 */ 853 public CodeableConcept getGenomeAssembly() { 854 if (this.genomeAssembly == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create MolecularSequenceRelativeStartingSequenceComponent.genomeAssembly"); 857 else if (Configuration.doAutoCreate()) 858 this.genomeAssembly = new CodeableConcept(); // cc 859 return this.genomeAssembly; 860 } 861 862 public boolean hasGenomeAssembly() { 863 return this.genomeAssembly != null && !this.genomeAssembly.isEmpty(); 864 } 865 866 /** 867 * @param value {@link #genomeAssembly} (The genome assembly used for starting sequence, e.g. GRCh38.) 868 */ 869 public MolecularSequenceRelativeStartingSequenceComponent setGenomeAssembly(CodeableConcept value) { 870 this.genomeAssembly = value; 871 return this; 872 } 873 874 /** 875 * @return {@link #chromosome} (Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 876 */ 877 public CodeableConcept getChromosome() { 878 if (this.chromosome == null) 879 if (Configuration.errorOnAutoCreate()) 880 throw new Error("Attempt to auto-create MolecularSequenceRelativeStartingSequenceComponent.chromosome"); 881 else if (Configuration.doAutoCreate()) 882 this.chromosome = new CodeableConcept(); // cc 883 return this.chromosome; 884 } 885 886 public boolean hasChromosome() { 887 return this.chromosome != null && !this.chromosome.isEmpty(); 888 } 889 890 /** 891 * @param value {@link #chromosome} (Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 892 */ 893 public MolecularSequenceRelativeStartingSequenceComponent setChromosome(CodeableConcept value) { 894 this.chromosome = value; 895 return this; 896 } 897 898 /** 899 * @return {@link #sequence} (The reference sequence that represents the starting sequence.) 900 */ 901 public DataType getSequence() { 902 return this.sequence; 903 } 904 905 /** 906 * @return {@link #sequence} (The reference sequence that represents the starting sequence.) 907 */ 908 public CodeableConcept getSequenceCodeableConcept() throws FHIRException { 909 if (this.sequence == null) 910 this.sequence = new CodeableConcept(); 911 if (!(this.sequence instanceof CodeableConcept)) 912 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.sequence.getClass().getName()+" was encountered"); 913 return (CodeableConcept) this.sequence; 914 } 915 916 public boolean hasSequenceCodeableConcept() { 917 return this.sequence instanceof CodeableConcept; 918 } 919 920 /** 921 * @return {@link #sequence} (The reference sequence that represents the starting sequence.) 922 */ 923 public StringType getSequenceStringType() throws FHIRException { 924 if (this.sequence == null) 925 this.sequence = new StringType(); 926 if (!(this.sequence instanceof StringType)) 927 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.sequence.getClass().getName()+" was encountered"); 928 return (StringType) this.sequence; 929 } 930 931 public boolean hasSequenceStringType() { 932 return this.sequence instanceof StringType; 933 } 934 935 /** 936 * @return {@link #sequence} (The reference sequence that represents the starting sequence.) 937 */ 938 public Reference getSequenceReference() throws FHIRException { 939 if (this.sequence == null) 940 this.sequence = new Reference(); 941 if (!(this.sequence instanceof Reference)) 942 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.sequence.getClass().getName()+" was encountered"); 943 return (Reference) this.sequence; 944 } 945 946 public boolean hasSequenceReference() { 947 return this.sequence instanceof Reference; 948 } 949 950 public boolean hasSequence() { 951 return this.sequence != null && !this.sequence.isEmpty(); 952 } 953 954 /** 955 * @param value {@link #sequence} (The reference sequence that represents the starting sequence.) 956 */ 957 public MolecularSequenceRelativeStartingSequenceComponent setSequence(DataType value) { 958 if (value != null && !(value instanceof CodeableConcept || value instanceof StringType || value instanceof Reference)) 959 throw new FHIRException("Not the right type for MolecularSequence.relative.startingSequence.sequence[x]: "+value.fhirType()); 960 this.sequence = value; 961 return this; 962 } 963 964 /** 965 * @return {@link #windowStart} (Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.). This is the underlying object with id, value and extensions. The accessor "getWindowStart" gives direct access to the value 966 */ 967 public IntegerType getWindowStartElement() { 968 if (this.windowStart == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create MolecularSequenceRelativeStartingSequenceComponent.windowStart"); 971 else if (Configuration.doAutoCreate()) 972 this.windowStart = new IntegerType(); // bb 973 return this.windowStart; 974 } 975 976 public boolean hasWindowStartElement() { 977 return this.windowStart != null && !this.windowStart.isEmpty(); 978 } 979 980 public boolean hasWindowStart() { 981 return this.windowStart != null && !this.windowStart.isEmpty(); 982 } 983 984 /** 985 * @param value {@link #windowStart} (Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.). This is the underlying object with id, value and extensions. The accessor "getWindowStart" gives direct access to the value 986 */ 987 public MolecularSequenceRelativeStartingSequenceComponent setWindowStartElement(IntegerType value) { 988 this.windowStart = value; 989 return this; 990 } 991 992 /** 993 * @return Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem. 994 */ 995 public int getWindowStart() { 996 return this.windowStart == null || this.windowStart.isEmpty() ? 0 : this.windowStart.getValue(); 997 } 998 999 /** 1000 * @param value Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem. 1001 */ 1002 public MolecularSequenceRelativeStartingSequenceComponent setWindowStart(int value) { 1003 if (this.windowStart == null) 1004 this.windowStart = new IntegerType(); 1005 this.windowStart.setValue(value); 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #windowEnd} (End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.). This is the underlying object with id, value and extensions. The accessor "getWindowEnd" gives direct access to the value 1011 */ 1012 public IntegerType getWindowEndElement() { 1013 if (this.windowEnd == null) 1014 if (Configuration.errorOnAutoCreate()) 1015 throw new Error("Attempt to auto-create MolecularSequenceRelativeStartingSequenceComponent.windowEnd"); 1016 else if (Configuration.doAutoCreate()) 1017 this.windowEnd = new IntegerType(); // bb 1018 return this.windowEnd; 1019 } 1020 1021 public boolean hasWindowEndElement() { 1022 return this.windowEnd != null && !this.windowEnd.isEmpty(); 1023 } 1024 1025 public boolean hasWindowEnd() { 1026 return this.windowEnd != null && !this.windowEnd.isEmpty(); 1027 } 1028 1029 /** 1030 * @param value {@link #windowEnd} (End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.). This is the underlying object with id, value and extensions. The accessor "getWindowEnd" gives direct access to the value 1031 */ 1032 public MolecularSequenceRelativeStartingSequenceComponent setWindowEndElement(IntegerType value) { 1033 this.windowEnd = value; 1034 return this; 1035 } 1036 1037 /** 1038 * @return End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem. 1039 */ 1040 public int getWindowEnd() { 1041 return this.windowEnd == null || this.windowEnd.isEmpty() ? 0 : this.windowEnd.getValue(); 1042 } 1043 1044 /** 1045 * @param value End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem. 1046 */ 1047 public MolecularSequenceRelativeStartingSequenceComponent setWindowEnd(int value) { 1048 if (this.windowEnd == null) 1049 this.windowEnd = new IntegerType(); 1050 this.windowEnd.setValue(value); 1051 return this; 1052 } 1053 1054 /** 1055 * @return {@link #orientation} (A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the "sense" strand, and the opposite complementary strand is the "antisense" strand.). This is the underlying object with id, value and extensions. The accessor "getOrientation" gives direct access to the value 1056 */ 1057 public Enumeration<OrientationType> getOrientationElement() { 1058 if (this.orientation == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create MolecularSequenceRelativeStartingSequenceComponent.orientation"); 1061 else if (Configuration.doAutoCreate()) 1062 this.orientation = new Enumeration<OrientationType>(new OrientationTypeEnumFactory()); // bb 1063 return this.orientation; 1064 } 1065 1066 public boolean hasOrientationElement() { 1067 return this.orientation != null && !this.orientation.isEmpty(); 1068 } 1069 1070 public boolean hasOrientation() { 1071 return this.orientation != null && !this.orientation.isEmpty(); 1072 } 1073 1074 /** 1075 * @param value {@link #orientation} (A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the "sense" strand, and the opposite complementary strand is the "antisense" strand.). This is the underlying object with id, value and extensions. The accessor "getOrientation" gives direct access to the value 1076 */ 1077 public MolecularSequenceRelativeStartingSequenceComponent setOrientationElement(Enumeration<OrientationType> value) { 1078 this.orientation = value; 1079 return this; 1080 } 1081 1082 /** 1083 * @return A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the "sense" strand, and the opposite complementary strand is the "antisense" strand. 1084 */ 1085 public OrientationType getOrientation() { 1086 return this.orientation == null ? null : this.orientation.getValue(); 1087 } 1088 1089 /** 1090 * @param value A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the "sense" strand, and the opposite complementary strand is the "antisense" strand. 1091 */ 1092 public MolecularSequenceRelativeStartingSequenceComponent setOrientation(OrientationType value) { 1093 if (value == null) 1094 this.orientation = null; 1095 else { 1096 if (this.orientation == null) 1097 this.orientation = new Enumeration<OrientationType>(new OrientationTypeEnumFactory()); 1098 this.orientation.setValue(value); 1099 } 1100 return this; 1101 } 1102 1103 /** 1104 * @return {@link #strand} (An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.). This is the underlying object with id, value and extensions. The accessor "getStrand" gives direct access to the value 1105 */ 1106 public Enumeration<StrandType> getStrandElement() { 1107 if (this.strand == null) 1108 if (Configuration.errorOnAutoCreate()) 1109 throw new Error("Attempt to auto-create MolecularSequenceRelativeStartingSequenceComponent.strand"); 1110 else if (Configuration.doAutoCreate()) 1111 this.strand = new Enumeration<StrandType>(new StrandTypeEnumFactory()); // bb 1112 return this.strand; 1113 } 1114 1115 public boolean hasStrandElement() { 1116 return this.strand != null && !this.strand.isEmpty(); 1117 } 1118 1119 public boolean hasStrand() { 1120 return this.strand != null && !this.strand.isEmpty(); 1121 } 1122 1123 /** 1124 * @param value {@link #strand} (An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.). This is the underlying object with id, value and extensions. The accessor "getStrand" gives direct access to the value 1125 */ 1126 public MolecularSequenceRelativeStartingSequenceComponent setStrandElement(Enumeration<StrandType> value) { 1127 this.strand = value; 1128 return this; 1129 } 1130 1131 /** 1132 * @return An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm. 1133 */ 1134 public StrandType getStrand() { 1135 return this.strand == null ? null : this.strand.getValue(); 1136 } 1137 1138 /** 1139 * @param value An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm. 1140 */ 1141 public MolecularSequenceRelativeStartingSequenceComponent setStrand(StrandType value) { 1142 if (value == null) 1143 this.strand = null; 1144 else { 1145 if (this.strand == null) 1146 this.strand = new Enumeration<StrandType>(new StrandTypeEnumFactory()); 1147 this.strand.setValue(value); 1148 } 1149 return this; 1150 } 1151 1152 protected void listChildren(List<Property> children) { 1153 super.listChildren(children); 1154 children.add(new Property("genomeAssembly", "CodeableConcept", "The genome assembly used for starting sequence, e.g. GRCh38.", 0, 1, genomeAssembly)); 1155 children.add(new Property("chromosome", "CodeableConcept", "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 0, 1, chromosome)); 1156 children.add(new Property("sequence[x]", "CodeableConcept|string|Reference(MolecularSequence)", "The reference sequence that represents the starting sequence.", 0, 1, sequence)); 1157 children.add(new Property("windowStart", "integer", "Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.", 0, 1, windowStart)); 1158 children.add(new Property("windowEnd", "integer", "End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.", 0, 1, windowEnd)); 1159 children.add(new Property("orientation", "code", "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.", 0, 1, orientation)); 1160 children.add(new Property("strand", "code", "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.", 0, 1, strand)); 1161 } 1162 1163 @Override 1164 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1165 switch (_hash) { 1166 case 1196021757: /*genomeAssembly*/ return new Property("genomeAssembly", "CodeableConcept", "The genome assembly used for starting sequence, e.g. GRCh38.", 0, 1, genomeAssembly); 1167 case -1499470472: /*chromosome*/ return new Property("chromosome", "CodeableConcept", "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 0, 1, chromosome); 1168 case -805222113: /*sequence[x]*/ return new Property("sequence[x]", "CodeableConcept|string|Reference(MolecularSequence)", "The reference sequence that represents the starting sequence.", 0, 1, sequence); 1169 case 1349547969: /*sequence*/ return new Property("sequence[x]", "CodeableConcept|string|Reference(MolecularSequence)", "The reference sequence that represents the starting sequence.", 0, 1, sequence); 1170 case 1508480416: /*sequenceCodeableConcept*/ return new Property("sequence[x]", "CodeableConcept", "The reference sequence that represents the starting sequence.", 0, 1, sequence); 1171 case -1211617486: /*sequenceString*/ return new Property("sequence[x]", "string", "The reference sequence that represents the starting sequence.", 0, 1, sequence); 1172 case -1127149430: /*sequenceReference*/ return new Property("sequence[x]", "Reference(MolecularSequence)", "The reference sequence that represents the starting sequence.", 0, 1, sequence); 1173 case 1903685202: /*windowStart*/ return new Property("windowStart", "integer", "Start position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.", 0, 1, windowStart); 1174 case -217026869: /*windowEnd*/ return new Property("windowEnd", "integer", "End position of the window on the starting sequence. This value should honor the rules of the coordinateSystem.", 0, 1, windowEnd); 1175 case -1439500848: /*orientation*/ return new Property("orientation", "code", "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.", 0, 1, orientation); 1176 case -891993594: /*strand*/ return new Property("strand", "code", "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.", 0, 1, strand); 1177 default: return super.getNamedProperty(_hash, _name, _checkValid); 1178 } 1179 1180 } 1181 1182 @Override 1183 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1184 switch (hash) { 1185 case 1196021757: /*genomeAssembly*/ return this.genomeAssembly == null ? new Base[0] : new Base[] {this.genomeAssembly}; // CodeableConcept 1186 case -1499470472: /*chromosome*/ return this.chromosome == null ? new Base[0] : new Base[] {this.chromosome}; // CodeableConcept 1187 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // DataType 1188 case 1903685202: /*windowStart*/ return this.windowStart == null ? new Base[0] : new Base[] {this.windowStart}; // IntegerType 1189 case -217026869: /*windowEnd*/ return this.windowEnd == null ? new Base[0] : new Base[] {this.windowEnd}; // IntegerType 1190 case -1439500848: /*orientation*/ return this.orientation == null ? new Base[0] : new Base[] {this.orientation}; // Enumeration<OrientationType> 1191 case -891993594: /*strand*/ return this.strand == null ? new Base[0] : new Base[] {this.strand}; // Enumeration<StrandType> 1192 default: return super.getProperty(hash, name, checkValid); 1193 } 1194 1195 } 1196 1197 @Override 1198 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1199 switch (hash) { 1200 case 1196021757: // genomeAssembly 1201 this.genomeAssembly = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1202 return value; 1203 case -1499470472: // chromosome 1204 this.chromosome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1205 return value; 1206 case 1349547969: // sequence 1207 this.sequence = TypeConvertor.castToType(value); // DataType 1208 return value; 1209 case 1903685202: // windowStart 1210 this.windowStart = TypeConvertor.castToInteger(value); // IntegerType 1211 return value; 1212 case -217026869: // windowEnd 1213 this.windowEnd = TypeConvertor.castToInteger(value); // IntegerType 1214 return value; 1215 case -1439500848: // orientation 1216 value = new OrientationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1217 this.orientation = (Enumeration) value; // Enumeration<OrientationType> 1218 return value; 1219 case -891993594: // strand 1220 value = new StrandTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1221 this.strand = (Enumeration) value; // Enumeration<StrandType> 1222 return value; 1223 default: return super.setProperty(hash, name, value); 1224 } 1225 1226 } 1227 1228 @Override 1229 public Base setProperty(String name, Base value) throws FHIRException { 1230 if (name.equals("genomeAssembly")) { 1231 this.genomeAssembly = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1232 } else if (name.equals("chromosome")) { 1233 this.chromosome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1234 } else if (name.equals("sequence[x]")) { 1235 this.sequence = TypeConvertor.castToType(value); // DataType 1236 } else if (name.equals("windowStart")) { 1237 this.windowStart = TypeConvertor.castToInteger(value); // IntegerType 1238 } else if (name.equals("windowEnd")) { 1239 this.windowEnd = TypeConvertor.castToInteger(value); // IntegerType 1240 } else if (name.equals("orientation")) { 1241 value = new OrientationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1242 this.orientation = (Enumeration) value; // Enumeration<OrientationType> 1243 } else if (name.equals("strand")) { 1244 value = new StrandTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1245 this.strand = (Enumeration) value; // Enumeration<StrandType> 1246 } else 1247 return super.setProperty(name, value); 1248 return value; 1249 } 1250 1251 @Override 1252 public void removeChild(String name, Base value) throws FHIRException { 1253 if (name.equals("genomeAssembly")) { 1254 this.genomeAssembly = null; 1255 } else if (name.equals("chromosome")) { 1256 this.chromosome = null; 1257 } else if (name.equals("sequence[x]")) { 1258 this.sequence = null; 1259 } else if (name.equals("windowStart")) { 1260 this.windowStart = null; 1261 } else if (name.equals("windowEnd")) { 1262 this.windowEnd = null; 1263 } else if (name.equals("orientation")) { 1264 value = new OrientationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1265 this.orientation = (Enumeration) value; // Enumeration<OrientationType> 1266 } else if (name.equals("strand")) { 1267 value = new StrandTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1268 this.strand = (Enumeration) value; // Enumeration<StrandType> 1269 } else 1270 super.removeChild(name, value); 1271 1272 } 1273 1274 @Override 1275 public Base makeProperty(int hash, String name) throws FHIRException { 1276 switch (hash) { 1277 case 1196021757: return getGenomeAssembly(); 1278 case -1499470472: return getChromosome(); 1279 case -805222113: return getSequence(); 1280 case 1349547969: return getSequence(); 1281 case 1903685202: return getWindowStartElement(); 1282 case -217026869: return getWindowEndElement(); 1283 case -1439500848: return getOrientationElement(); 1284 case -891993594: return getStrandElement(); 1285 default: return super.makeProperty(hash, name); 1286 } 1287 1288 } 1289 1290 @Override 1291 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1292 switch (hash) { 1293 case 1196021757: /*genomeAssembly*/ return new String[] {"CodeableConcept"}; 1294 case -1499470472: /*chromosome*/ return new String[] {"CodeableConcept"}; 1295 case 1349547969: /*sequence*/ return new String[] {"CodeableConcept", "string", "Reference"}; 1296 case 1903685202: /*windowStart*/ return new String[] {"integer"}; 1297 case -217026869: /*windowEnd*/ return new String[] {"integer"}; 1298 case -1439500848: /*orientation*/ return new String[] {"code"}; 1299 case -891993594: /*strand*/ return new String[] {"code"}; 1300 default: return super.getTypesForProperty(hash, name); 1301 } 1302 1303 } 1304 1305 @Override 1306 public Base addChild(String name) throws FHIRException { 1307 if (name.equals("genomeAssembly")) { 1308 this.genomeAssembly = new CodeableConcept(); 1309 return this.genomeAssembly; 1310 } 1311 else if (name.equals("chromosome")) { 1312 this.chromosome = new CodeableConcept(); 1313 return this.chromosome; 1314 } 1315 else if (name.equals("sequenceCodeableConcept")) { 1316 this.sequence = new CodeableConcept(); 1317 return this.sequence; 1318 } 1319 else if (name.equals("sequenceString")) { 1320 this.sequence = new StringType(); 1321 return this.sequence; 1322 } 1323 else if (name.equals("sequenceReference")) { 1324 this.sequence = new Reference(); 1325 return this.sequence; 1326 } 1327 else if (name.equals("windowStart")) { 1328 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.startingSequence.windowStart"); 1329 } 1330 else if (name.equals("windowEnd")) { 1331 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.startingSequence.windowEnd"); 1332 } 1333 else if (name.equals("orientation")) { 1334 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.startingSequence.orientation"); 1335 } 1336 else if (name.equals("strand")) { 1337 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.startingSequence.strand"); 1338 } 1339 else 1340 return super.addChild(name); 1341 } 1342 1343 public MolecularSequenceRelativeStartingSequenceComponent copy() { 1344 MolecularSequenceRelativeStartingSequenceComponent dst = new MolecularSequenceRelativeStartingSequenceComponent(); 1345 copyValues(dst); 1346 return dst; 1347 } 1348 1349 public void copyValues(MolecularSequenceRelativeStartingSequenceComponent dst) { 1350 super.copyValues(dst); 1351 dst.genomeAssembly = genomeAssembly == null ? null : genomeAssembly.copy(); 1352 dst.chromosome = chromosome == null ? null : chromosome.copy(); 1353 dst.sequence = sequence == null ? null : sequence.copy(); 1354 dst.windowStart = windowStart == null ? null : windowStart.copy(); 1355 dst.windowEnd = windowEnd == null ? null : windowEnd.copy(); 1356 dst.orientation = orientation == null ? null : orientation.copy(); 1357 dst.strand = strand == null ? null : strand.copy(); 1358 } 1359 1360 @Override 1361 public boolean equalsDeep(Base other_) { 1362 if (!super.equalsDeep(other_)) 1363 return false; 1364 if (!(other_ instanceof MolecularSequenceRelativeStartingSequenceComponent)) 1365 return false; 1366 MolecularSequenceRelativeStartingSequenceComponent o = (MolecularSequenceRelativeStartingSequenceComponent) other_; 1367 return compareDeep(genomeAssembly, o.genomeAssembly, true) && compareDeep(chromosome, o.chromosome, true) 1368 && compareDeep(sequence, o.sequence, true) && compareDeep(windowStart, o.windowStart, true) && compareDeep(windowEnd, o.windowEnd, true) 1369 && compareDeep(orientation, o.orientation, true) && compareDeep(strand, o.strand, true); 1370 } 1371 1372 @Override 1373 public boolean equalsShallow(Base other_) { 1374 if (!super.equalsShallow(other_)) 1375 return false; 1376 if (!(other_ instanceof MolecularSequenceRelativeStartingSequenceComponent)) 1377 return false; 1378 MolecularSequenceRelativeStartingSequenceComponent o = (MolecularSequenceRelativeStartingSequenceComponent) other_; 1379 return compareValues(windowStart, o.windowStart, true) && compareValues(windowEnd, o.windowEnd, true) 1380 && compareValues(orientation, o.orientation, true) && compareValues(strand, o.strand, true); 1381 } 1382 1383 public boolean isEmpty() { 1384 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(genomeAssembly, chromosome 1385 , sequence, windowStart, windowEnd, orientation, strand); 1386 } 1387 1388 public String fhirType() { 1389 return "MolecularSequence.relative.startingSequence"; 1390 1391 } 1392 1393 } 1394 1395 @Block() 1396 public static class MolecularSequenceRelativeEditComponent extends BackboneElement implements IBaseBackboneElement { 1397 /** 1398 * Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1399 */ 1400 @Child(name = "start", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1401 @Description(shortDefinition="Start position of the edit on the starting sequence", formalDefinition="Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive." ) 1402 protected IntegerType start; 1403 1404 /** 1405 * End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1406 */ 1407 @Child(name = "end", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1408 @Description(shortDefinition="End position of the edit on the starting sequence", formalDefinition="End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position." ) 1409 protected IntegerType end; 1410 1411 /** 1412 * Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1413 */ 1414 @Child(name = "replacementSequence", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1415 @Description(shortDefinition="Allele that was observed", formalDefinition="Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end." ) 1416 protected StringType replacementSequence; 1417 1418 /** 1419 * Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1420 */ 1421 @Child(name = "replacedSequence", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1422 @Description(shortDefinition="Allele in the starting sequence", formalDefinition="Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end." ) 1423 protected StringType replacedSequence; 1424 1425 private static final long serialVersionUID = 550127909L; 1426 1427 /** 1428 * Constructor 1429 */ 1430 public MolecularSequenceRelativeEditComponent() { 1431 super(); 1432 } 1433 1434 /** 1435 * @return {@link #start} (Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1436 */ 1437 public IntegerType getStartElement() { 1438 if (this.start == null) 1439 if (Configuration.errorOnAutoCreate()) 1440 throw new Error("Attempt to auto-create MolecularSequenceRelativeEditComponent.start"); 1441 else if (Configuration.doAutoCreate()) 1442 this.start = new IntegerType(); // bb 1443 return this.start; 1444 } 1445 1446 public boolean hasStartElement() { 1447 return this.start != null && !this.start.isEmpty(); 1448 } 1449 1450 public boolean hasStart() { 1451 return this.start != null && !this.start.isEmpty(); 1452 } 1453 1454 /** 1455 * @param value {@link #start} (Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1456 */ 1457 public MolecularSequenceRelativeEditComponent setStartElement(IntegerType value) { 1458 this.start = value; 1459 return this; 1460 } 1461 1462 /** 1463 * @return Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1464 */ 1465 public int getStart() { 1466 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 1467 } 1468 1469 /** 1470 * @param value Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1471 */ 1472 public MolecularSequenceRelativeEditComponent setStart(int value) { 1473 if (this.start == null) 1474 this.start = new IntegerType(); 1475 this.start.setValue(value); 1476 return this; 1477 } 1478 1479 /** 1480 * @return {@link #end} (End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1481 */ 1482 public IntegerType getEndElement() { 1483 if (this.end == null) 1484 if (Configuration.errorOnAutoCreate()) 1485 throw new Error("Attempt to auto-create MolecularSequenceRelativeEditComponent.end"); 1486 else if (Configuration.doAutoCreate()) 1487 this.end = new IntegerType(); // bb 1488 return this.end; 1489 } 1490 1491 public boolean hasEndElement() { 1492 return this.end != null && !this.end.isEmpty(); 1493 } 1494 1495 public boolean hasEnd() { 1496 return this.end != null && !this.end.isEmpty(); 1497 } 1498 1499 /** 1500 * @param value {@link #end} (End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1501 */ 1502 public MolecularSequenceRelativeEditComponent setEndElement(IntegerType value) { 1503 this.end = value; 1504 return this; 1505 } 1506 1507 /** 1508 * @return End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1509 */ 1510 public int getEnd() { 1511 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 1512 } 1513 1514 /** 1515 * @param value End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1516 */ 1517 public MolecularSequenceRelativeEditComponent setEnd(int value) { 1518 if (this.end == null) 1519 this.end = new IntegerType(); 1520 this.end.setValue(value); 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #replacementSequence} (Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReplacementSequence" gives direct access to the value 1526 */ 1527 public StringType getReplacementSequenceElement() { 1528 if (this.replacementSequence == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create MolecularSequenceRelativeEditComponent.replacementSequence"); 1531 else if (Configuration.doAutoCreate()) 1532 this.replacementSequence = new StringType(); // bb 1533 return this.replacementSequence; 1534 } 1535 1536 public boolean hasReplacementSequenceElement() { 1537 return this.replacementSequence != null && !this.replacementSequence.isEmpty(); 1538 } 1539 1540 public boolean hasReplacementSequence() { 1541 return this.replacementSequence != null && !this.replacementSequence.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #replacementSequence} (Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReplacementSequence" gives direct access to the value 1546 */ 1547 public MolecularSequenceRelativeEditComponent setReplacementSequenceElement(StringType value) { 1548 this.replacementSequence = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1554 */ 1555 public String getReplacementSequence() { 1556 return this.replacementSequence == null ? null : this.replacementSequence.getValue(); 1557 } 1558 1559 /** 1560 * @param value Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1561 */ 1562 public MolecularSequenceRelativeEditComponent setReplacementSequence(String value) { 1563 if (Utilities.noString(value)) 1564 this.replacementSequence = null; 1565 else { 1566 if (this.replacementSequence == null) 1567 this.replacementSequence = new StringType(); 1568 this.replacementSequence.setValue(value); 1569 } 1570 return this; 1571 } 1572 1573 /** 1574 * @return {@link #replacedSequence} (Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReplacedSequence" gives direct access to the value 1575 */ 1576 public StringType getReplacedSequenceElement() { 1577 if (this.replacedSequence == null) 1578 if (Configuration.errorOnAutoCreate()) 1579 throw new Error("Attempt to auto-create MolecularSequenceRelativeEditComponent.replacedSequence"); 1580 else if (Configuration.doAutoCreate()) 1581 this.replacedSequence = new StringType(); // bb 1582 return this.replacedSequence; 1583 } 1584 1585 public boolean hasReplacedSequenceElement() { 1586 return this.replacedSequence != null && !this.replacedSequence.isEmpty(); 1587 } 1588 1589 public boolean hasReplacedSequence() { 1590 return this.replacedSequence != null && !this.replacedSequence.isEmpty(); 1591 } 1592 1593 /** 1594 * @param value {@link #replacedSequence} (Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReplacedSequence" gives direct access to the value 1595 */ 1596 public MolecularSequenceRelativeEditComponent setReplacedSequenceElement(StringType value) { 1597 this.replacedSequence = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1603 */ 1604 public String getReplacedSequence() { 1605 return this.replacedSequence == null ? null : this.replacedSequence.getValue(); 1606 } 1607 1608 /** 1609 * @param value Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1610 */ 1611 public MolecularSequenceRelativeEditComponent setReplacedSequence(String value) { 1612 if (Utilities.noString(value)) 1613 this.replacedSequence = null; 1614 else { 1615 if (this.replacedSequence == null) 1616 this.replacedSequence = new StringType(); 1617 this.replacedSequence.setValue(value); 1618 } 1619 return this; 1620 } 1621 1622 protected void listChildren(List<Property> children) { 1623 super.listChildren(children); 1624 children.add(new Property("start", "integer", "Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start)); 1625 children.add(new Property("end", "integer", "End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end)); 1626 children.add(new Property("replacementSequence", "string", "Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, replacementSequence)); 1627 children.add(new Property("replacedSequence", "string", "Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, replacedSequence)); 1628 } 1629 1630 @Override 1631 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1632 switch (_hash) { 1633 case 109757538: /*start*/ return new Property("start", "integer", "Start position of the edit on the starting sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start); 1634 case 100571: /*end*/ return new Property("end", "integer", "End position of the edit on the starting sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end); 1635 case -1784940557: /*replacementSequence*/ return new Property("replacementSequence", "string", "Allele that was observed. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, replacementSequence); 1636 case 1972719633: /*replacedSequence*/ return new Property("replacedSequence", "string", "Allele in the starting sequence. Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the starting sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, replacedSequence); 1637 default: return super.getNamedProperty(_hash, _name, _checkValid); 1638 } 1639 1640 } 1641 1642 @Override 1643 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1644 switch (hash) { 1645 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // IntegerType 1646 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // IntegerType 1647 case -1784940557: /*replacementSequence*/ return this.replacementSequence == null ? new Base[0] : new Base[] {this.replacementSequence}; // StringType 1648 case 1972719633: /*replacedSequence*/ return this.replacedSequence == null ? new Base[0] : new Base[] {this.replacedSequence}; // StringType 1649 default: return super.getProperty(hash, name, checkValid); 1650 } 1651 1652 } 1653 1654 @Override 1655 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1656 switch (hash) { 1657 case 109757538: // start 1658 this.start = TypeConvertor.castToInteger(value); // IntegerType 1659 return value; 1660 case 100571: // end 1661 this.end = TypeConvertor.castToInteger(value); // IntegerType 1662 return value; 1663 case -1784940557: // replacementSequence 1664 this.replacementSequence = TypeConvertor.castToString(value); // StringType 1665 return value; 1666 case 1972719633: // replacedSequence 1667 this.replacedSequence = TypeConvertor.castToString(value); // StringType 1668 return value; 1669 default: return super.setProperty(hash, name, value); 1670 } 1671 1672 } 1673 1674 @Override 1675 public Base setProperty(String name, Base value) throws FHIRException { 1676 if (name.equals("start")) { 1677 this.start = TypeConvertor.castToInteger(value); // IntegerType 1678 } else if (name.equals("end")) { 1679 this.end = TypeConvertor.castToInteger(value); // IntegerType 1680 } else if (name.equals("replacementSequence")) { 1681 this.replacementSequence = TypeConvertor.castToString(value); // StringType 1682 } else if (name.equals("replacedSequence")) { 1683 this.replacedSequence = TypeConvertor.castToString(value); // StringType 1684 } else 1685 return super.setProperty(name, value); 1686 return value; 1687 } 1688 1689 @Override 1690 public void removeChild(String name, Base value) throws FHIRException { 1691 if (name.equals("start")) { 1692 this.start = null; 1693 } else if (name.equals("end")) { 1694 this.end = null; 1695 } else if (name.equals("replacementSequence")) { 1696 this.replacementSequence = null; 1697 } else if (name.equals("replacedSequence")) { 1698 this.replacedSequence = null; 1699 } else 1700 super.removeChild(name, value); 1701 1702 } 1703 1704 @Override 1705 public Base makeProperty(int hash, String name) throws FHIRException { 1706 switch (hash) { 1707 case 109757538: return getStartElement(); 1708 case 100571: return getEndElement(); 1709 case -1784940557: return getReplacementSequenceElement(); 1710 case 1972719633: return getReplacedSequenceElement(); 1711 default: return super.makeProperty(hash, name); 1712 } 1713 1714 } 1715 1716 @Override 1717 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1718 switch (hash) { 1719 case 109757538: /*start*/ return new String[] {"integer"}; 1720 case 100571: /*end*/ return new String[] {"integer"}; 1721 case -1784940557: /*replacementSequence*/ return new String[] {"string"}; 1722 case 1972719633: /*replacedSequence*/ return new String[] {"string"}; 1723 default: return super.getTypesForProperty(hash, name); 1724 } 1725 1726 } 1727 1728 @Override 1729 public Base addChild(String name) throws FHIRException { 1730 if (name.equals("start")) { 1731 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.edit.start"); 1732 } 1733 else if (name.equals("end")) { 1734 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.edit.end"); 1735 } 1736 else if (name.equals("replacementSequence")) { 1737 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.edit.replacementSequence"); 1738 } 1739 else if (name.equals("replacedSequence")) { 1740 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.relative.edit.replacedSequence"); 1741 } 1742 else 1743 return super.addChild(name); 1744 } 1745 1746 public MolecularSequenceRelativeEditComponent copy() { 1747 MolecularSequenceRelativeEditComponent dst = new MolecularSequenceRelativeEditComponent(); 1748 copyValues(dst); 1749 return dst; 1750 } 1751 1752 public void copyValues(MolecularSequenceRelativeEditComponent dst) { 1753 super.copyValues(dst); 1754 dst.start = start == null ? null : start.copy(); 1755 dst.end = end == null ? null : end.copy(); 1756 dst.replacementSequence = replacementSequence == null ? null : replacementSequence.copy(); 1757 dst.replacedSequence = replacedSequence == null ? null : replacedSequence.copy(); 1758 } 1759 1760 @Override 1761 public boolean equalsDeep(Base other_) { 1762 if (!super.equalsDeep(other_)) 1763 return false; 1764 if (!(other_ instanceof MolecularSequenceRelativeEditComponent)) 1765 return false; 1766 MolecularSequenceRelativeEditComponent o = (MolecularSequenceRelativeEditComponent) other_; 1767 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(replacementSequence, o.replacementSequence, true) 1768 && compareDeep(replacedSequence, o.replacedSequence, true); 1769 } 1770 1771 @Override 1772 public boolean equalsShallow(Base other_) { 1773 if (!super.equalsShallow(other_)) 1774 return false; 1775 if (!(other_ instanceof MolecularSequenceRelativeEditComponent)) 1776 return false; 1777 MolecularSequenceRelativeEditComponent o = (MolecularSequenceRelativeEditComponent) other_; 1778 return compareValues(start, o.start, true) && compareValues(end, o.end, true) && compareValues(replacementSequence, o.replacementSequence, true) 1779 && compareValues(replacedSequence, o.replacedSequence, true); 1780 } 1781 1782 public boolean isEmpty() { 1783 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end, replacementSequence 1784 , replacedSequence); 1785 } 1786 1787 public String fhirType() { 1788 return "MolecularSequence.relative.edit"; 1789 1790 } 1791 1792 } 1793 1794 /** 1795 * A unique identifier for this particular sequence instance. 1796 */ 1797 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1798 @Description(shortDefinition="Unique ID for this particular sequence", formalDefinition="A unique identifier for this particular sequence instance." ) 1799 protected List<Identifier> identifier; 1800 1801 /** 1802 * Amino Acid Sequence/ DNA Sequence / RNA Sequence. 1803 */ 1804 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1805 @Description(shortDefinition="aa | dna | rna", formalDefinition="Amino Acid Sequence/ DNA Sequence / RNA Sequence." ) 1806 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-type") 1807 protected Enumeration<SequenceType> type; 1808 1809 /** 1810 * Indicates the subject this sequence is associated too. 1811 */ 1812 @Child(name = "subject", type = {Patient.class, Group.class, Substance.class, BiologicallyDerivedProduct.class, NutritionProduct.class}, order=2, min=0, max=1, modifier=false, summary=true) 1813 @Description(shortDefinition="Subject this sequence is associated too", formalDefinition="Indicates the subject this sequence is associated too." ) 1814 protected Reference subject; 1815 1816 /** 1817 * The actual focus of a molecular sequence when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the subject would be the child (proband) and the focus would be the parent. 1818 */ 1819 @Child(name = "focus", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1820 @Description(shortDefinition="What the molecular sequence is about, when it is not about the subject of record", formalDefinition="The actual focus of a molecular sequence when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the subject would be the child (proband) and the focus would be the parent." ) 1821 protected List<Reference> focus; 1822 1823 /** 1824 * Specimen used for sequencing. 1825 */ 1826 @Child(name = "specimen", type = {Specimen.class}, order=4, min=0, max=1, modifier=false, summary=true) 1827 @Description(shortDefinition="Specimen used for sequencing", formalDefinition="Specimen used for sequencing." ) 1828 protected Reference specimen; 1829 1830 /** 1831 * The method for sequencing, for example, chip information. 1832 */ 1833 @Child(name = "device", type = {Device.class}, order=5, min=0, max=1, modifier=false, summary=true) 1834 @Description(shortDefinition="The method for sequencing", formalDefinition="The method for sequencing, for example, chip information." ) 1835 protected Reference device; 1836 1837 /** 1838 * The organization or lab that should be responsible for this result. 1839 */ 1840 @Child(name = "performer", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=true) 1841 @Description(shortDefinition="Who should be responsible for test result", formalDefinition="The organization or lab that should be responsible for this result." ) 1842 protected Reference performer; 1843 1844 /** 1845 * Sequence that was observed. 1846 */ 1847 @Child(name = "literal", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1848 @Description(shortDefinition="Sequence that was observed", formalDefinition="Sequence that was observed." ) 1849 protected StringType literal; 1850 1851 /** 1852 * Sequence that was observed as file content. Can be an actual file contents, or referenced by a URL to an external system. 1853 */ 1854 @Child(name = "formatted", type = {Attachment.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1855 @Description(shortDefinition="Embedded file or a link (URL) which contains content to represent the sequence", formalDefinition="Sequence that was observed as file content. Can be an actual file contents, or referenced by a URL to an external system." ) 1856 protected List<Attachment> formatted; 1857 1858 /** 1859 * A sequence defined relative to another sequence. 1860 */ 1861 @Child(name = "relative", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1862 @Description(shortDefinition="A sequence defined relative to another sequence", formalDefinition="A sequence defined relative to another sequence." ) 1863 protected List<MolecularSequenceRelativeComponent> relative; 1864 1865 private static final long serialVersionUID = 611205496L; 1866 1867 /** 1868 * Constructor 1869 */ 1870 public MolecularSequence() { 1871 super(); 1872 } 1873 1874 /** 1875 * @return {@link #identifier} (A unique identifier for this particular sequence instance.) 1876 */ 1877 public List<Identifier> getIdentifier() { 1878 if (this.identifier == null) 1879 this.identifier = new ArrayList<Identifier>(); 1880 return this.identifier; 1881 } 1882 1883 /** 1884 * @return Returns a reference to <code>this</code> for easy method chaining 1885 */ 1886 public MolecularSequence setIdentifier(List<Identifier> theIdentifier) { 1887 this.identifier = theIdentifier; 1888 return this; 1889 } 1890 1891 public boolean hasIdentifier() { 1892 if (this.identifier == null) 1893 return false; 1894 for (Identifier item : this.identifier) 1895 if (!item.isEmpty()) 1896 return true; 1897 return false; 1898 } 1899 1900 public Identifier addIdentifier() { //3 1901 Identifier t = new Identifier(); 1902 if (this.identifier == null) 1903 this.identifier = new ArrayList<Identifier>(); 1904 this.identifier.add(t); 1905 return t; 1906 } 1907 1908 public MolecularSequence addIdentifier(Identifier t) { //3 1909 if (t == null) 1910 return this; 1911 if (this.identifier == null) 1912 this.identifier = new ArrayList<Identifier>(); 1913 this.identifier.add(t); 1914 return this; 1915 } 1916 1917 /** 1918 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1919 */ 1920 public Identifier getIdentifierFirstRep() { 1921 if (getIdentifier().isEmpty()) { 1922 addIdentifier(); 1923 } 1924 return getIdentifier().get(0); 1925 } 1926 1927 /** 1928 * @return {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1929 */ 1930 public Enumeration<SequenceType> getTypeElement() { 1931 if (this.type == null) 1932 if (Configuration.errorOnAutoCreate()) 1933 throw new Error("Attempt to auto-create MolecularSequence.type"); 1934 else if (Configuration.doAutoCreate()) 1935 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); // bb 1936 return this.type; 1937 } 1938 1939 public boolean hasTypeElement() { 1940 return this.type != null && !this.type.isEmpty(); 1941 } 1942 1943 public boolean hasType() { 1944 return this.type != null && !this.type.isEmpty(); 1945 } 1946 1947 /** 1948 * @param value {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1949 */ 1950 public MolecularSequence setTypeElement(Enumeration<SequenceType> value) { 1951 this.type = value; 1952 return this; 1953 } 1954 1955 /** 1956 * @return Amino Acid Sequence/ DNA Sequence / RNA Sequence. 1957 */ 1958 public SequenceType getType() { 1959 return this.type == null ? null : this.type.getValue(); 1960 } 1961 1962 /** 1963 * @param value Amino Acid Sequence/ DNA Sequence / RNA Sequence. 1964 */ 1965 public MolecularSequence setType(SequenceType value) { 1966 if (value == null) 1967 this.type = null; 1968 else { 1969 if (this.type == null) 1970 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); 1971 this.type.setValue(value); 1972 } 1973 return this; 1974 } 1975 1976 /** 1977 * @return {@link #subject} (Indicates the subject this sequence is associated too.) 1978 */ 1979 public Reference getSubject() { 1980 if (this.subject == null) 1981 if (Configuration.errorOnAutoCreate()) 1982 throw new Error("Attempt to auto-create MolecularSequence.subject"); 1983 else if (Configuration.doAutoCreate()) 1984 this.subject = new Reference(); // cc 1985 return this.subject; 1986 } 1987 1988 public boolean hasSubject() { 1989 return this.subject != null && !this.subject.isEmpty(); 1990 } 1991 1992 /** 1993 * @param value {@link #subject} (Indicates the subject this sequence is associated too.) 1994 */ 1995 public MolecularSequence setSubject(Reference value) { 1996 this.subject = value; 1997 return this; 1998 } 1999 2000 /** 2001 * @return {@link #focus} (The actual focus of a molecular sequence when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the subject would be the child (proband) and the focus would be the parent.) 2002 */ 2003 public List<Reference> getFocus() { 2004 if (this.focus == null) 2005 this.focus = new ArrayList<Reference>(); 2006 return this.focus; 2007 } 2008 2009 /** 2010 * @return Returns a reference to <code>this</code> for easy method chaining 2011 */ 2012 public MolecularSequence setFocus(List<Reference> theFocus) { 2013 this.focus = theFocus; 2014 return this; 2015 } 2016 2017 public boolean hasFocus() { 2018 if (this.focus == null) 2019 return false; 2020 for (Reference item : this.focus) 2021 if (!item.isEmpty()) 2022 return true; 2023 return false; 2024 } 2025 2026 public Reference addFocus() { //3 2027 Reference t = new Reference(); 2028 if (this.focus == null) 2029 this.focus = new ArrayList<Reference>(); 2030 this.focus.add(t); 2031 return t; 2032 } 2033 2034 public MolecularSequence addFocus(Reference t) { //3 2035 if (t == null) 2036 return this; 2037 if (this.focus == null) 2038 this.focus = new ArrayList<Reference>(); 2039 this.focus.add(t); 2040 return this; 2041 } 2042 2043 /** 2044 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 2045 */ 2046 public Reference getFocusFirstRep() { 2047 if (getFocus().isEmpty()) { 2048 addFocus(); 2049 } 2050 return getFocus().get(0); 2051 } 2052 2053 /** 2054 * @return {@link #specimen} (Specimen used for sequencing.) 2055 */ 2056 public Reference getSpecimen() { 2057 if (this.specimen == null) 2058 if (Configuration.errorOnAutoCreate()) 2059 throw new Error("Attempt to auto-create MolecularSequence.specimen"); 2060 else if (Configuration.doAutoCreate()) 2061 this.specimen = new Reference(); // cc 2062 return this.specimen; 2063 } 2064 2065 public boolean hasSpecimen() { 2066 return this.specimen != null && !this.specimen.isEmpty(); 2067 } 2068 2069 /** 2070 * @param value {@link #specimen} (Specimen used for sequencing.) 2071 */ 2072 public MolecularSequence setSpecimen(Reference value) { 2073 this.specimen = value; 2074 return this; 2075 } 2076 2077 /** 2078 * @return {@link #device} (The method for sequencing, for example, chip information.) 2079 */ 2080 public Reference getDevice() { 2081 if (this.device == null) 2082 if (Configuration.errorOnAutoCreate()) 2083 throw new Error("Attempt to auto-create MolecularSequence.device"); 2084 else if (Configuration.doAutoCreate()) 2085 this.device = new Reference(); // cc 2086 return this.device; 2087 } 2088 2089 public boolean hasDevice() { 2090 return this.device != null && !this.device.isEmpty(); 2091 } 2092 2093 /** 2094 * @param value {@link #device} (The method for sequencing, for example, chip information.) 2095 */ 2096 public MolecularSequence setDevice(Reference value) { 2097 this.device = value; 2098 return this; 2099 } 2100 2101 /** 2102 * @return {@link #performer} (The organization or lab that should be responsible for this result.) 2103 */ 2104 public Reference getPerformer() { 2105 if (this.performer == null) 2106 if (Configuration.errorOnAutoCreate()) 2107 throw new Error("Attempt to auto-create MolecularSequence.performer"); 2108 else if (Configuration.doAutoCreate()) 2109 this.performer = new Reference(); // cc 2110 return this.performer; 2111 } 2112 2113 public boolean hasPerformer() { 2114 return this.performer != null && !this.performer.isEmpty(); 2115 } 2116 2117 /** 2118 * @param value {@link #performer} (The organization or lab that should be responsible for this result.) 2119 */ 2120 public MolecularSequence setPerformer(Reference value) { 2121 this.performer = value; 2122 return this; 2123 } 2124 2125 /** 2126 * @return {@link #literal} (Sequence that was observed.). This is the underlying object with id, value and extensions. The accessor "getLiteral" gives direct access to the value 2127 */ 2128 public StringType getLiteralElement() { 2129 if (this.literal == null) 2130 if (Configuration.errorOnAutoCreate()) 2131 throw new Error("Attempt to auto-create MolecularSequence.literal"); 2132 else if (Configuration.doAutoCreate()) 2133 this.literal = new StringType(); // bb 2134 return this.literal; 2135 } 2136 2137 public boolean hasLiteralElement() { 2138 return this.literal != null && !this.literal.isEmpty(); 2139 } 2140 2141 public boolean hasLiteral() { 2142 return this.literal != null && !this.literal.isEmpty(); 2143 } 2144 2145 /** 2146 * @param value {@link #literal} (Sequence that was observed.). This is the underlying object with id, value and extensions. The accessor "getLiteral" gives direct access to the value 2147 */ 2148 public MolecularSequence setLiteralElement(StringType value) { 2149 this.literal = value; 2150 return this; 2151 } 2152 2153 /** 2154 * @return Sequence that was observed. 2155 */ 2156 public String getLiteral() { 2157 return this.literal == null ? null : this.literal.getValue(); 2158 } 2159 2160 /** 2161 * @param value Sequence that was observed. 2162 */ 2163 public MolecularSequence setLiteral(String value) { 2164 if (Utilities.noString(value)) 2165 this.literal = null; 2166 else { 2167 if (this.literal == null) 2168 this.literal = new StringType(); 2169 this.literal.setValue(value); 2170 } 2171 return this; 2172 } 2173 2174 /** 2175 * @return {@link #formatted} (Sequence that was observed as file content. Can be an actual file contents, or referenced by a URL to an external system.) 2176 */ 2177 public List<Attachment> getFormatted() { 2178 if (this.formatted == null) 2179 this.formatted = new ArrayList<Attachment>(); 2180 return this.formatted; 2181 } 2182 2183 /** 2184 * @return Returns a reference to <code>this</code> for easy method chaining 2185 */ 2186 public MolecularSequence setFormatted(List<Attachment> theFormatted) { 2187 this.formatted = theFormatted; 2188 return this; 2189 } 2190 2191 public boolean hasFormatted() { 2192 if (this.formatted == null) 2193 return false; 2194 for (Attachment item : this.formatted) 2195 if (!item.isEmpty()) 2196 return true; 2197 return false; 2198 } 2199 2200 public Attachment addFormatted() { //3 2201 Attachment t = new Attachment(); 2202 if (this.formatted == null) 2203 this.formatted = new ArrayList<Attachment>(); 2204 this.formatted.add(t); 2205 return t; 2206 } 2207 2208 public MolecularSequence addFormatted(Attachment t) { //3 2209 if (t == null) 2210 return this; 2211 if (this.formatted == null) 2212 this.formatted = new ArrayList<Attachment>(); 2213 this.formatted.add(t); 2214 return this; 2215 } 2216 2217 /** 2218 * @return The first repetition of repeating field {@link #formatted}, creating it if it does not already exist {3} 2219 */ 2220 public Attachment getFormattedFirstRep() { 2221 if (getFormatted().isEmpty()) { 2222 addFormatted(); 2223 } 2224 return getFormatted().get(0); 2225 } 2226 2227 /** 2228 * @return {@link #relative} (A sequence defined relative to another sequence.) 2229 */ 2230 public List<MolecularSequenceRelativeComponent> getRelative() { 2231 if (this.relative == null) 2232 this.relative = new ArrayList<MolecularSequenceRelativeComponent>(); 2233 return this.relative; 2234 } 2235 2236 /** 2237 * @return Returns a reference to <code>this</code> for easy method chaining 2238 */ 2239 public MolecularSequence setRelative(List<MolecularSequenceRelativeComponent> theRelative) { 2240 this.relative = theRelative; 2241 return this; 2242 } 2243 2244 public boolean hasRelative() { 2245 if (this.relative == null) 2246 return false; 2247 for (MolecularSequenceRelativeComponent item : this.relative) 2248 if (!item.isEmpty()) 2249 return true; 2250 return false; 2251 } 2252 2253 public MolecularSequenceRelativeComponent addRelative() { //3 2254 MolecularSequenceRelativeComponent t = new MolecularSequenceRelativeComponent(); 2255 if (this.relative == null) 2256 this.relative = new ArrayList<MolecularSequenceRelativeComponent>(); 2257 this.relative.add(t); 2258 return t; 2259 } 2260 2261 public MolecularSequence addRelative(MolecularSequenceRelativeComponent t) { //3 2262 if (t == null) 2263 return this; 2264 if (this.relative == null) 2265 this.relative = new ArrayList<MolecularSequenceRelativeComponent>(); 2266 this.relative.add(t); 2267 return this; 2268 } 2269 2270 /** 2271 * @return The first repetition of repeating field {@link #relative}, creating it if it does not already exist {3} 2272 */ 2273 public MolecularSequenceRelativeComponent getRelativeFirstRep() { 2274 if (getRelative().isEmpty()) { 2275 addRelative(); 2276 } 2277 return getRelative().get(0); 2278 } 2279 2280 protected void listChildren(List<Property> children) { 2281 super.listChildren(children); 2282 children.add(new Property("identifier", "Identifier", "A unique identifier for this particular sequence instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2283 children.add(new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type)); 2284 children.add(new Property("subject", "Reference(Patient|Group|Substance|BiologicallyDerivedProduct|NutritionProduct)", "Indicates the subject this sequence is associated too.", 0, 1, subject)); 2285 children.add(new Property("focus", "Reference(Any)", "The actual focus of a molecular sequence when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the subject would be the child (proband) and the focus would be the parent.", 0, java.lang.Integer.MAX_VALUE, focus)); 2286 children.add(new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen)); 2287 children.add(new Property("device", "Reference(Device)", "The method for sequencing, for example, chip information.", 0, 1, device)); 2288 children.add(new Property("performer", "Reference(Organization)", "The organization or lab that should be responsible for this result.", 0, 1, performer)); 2289 children.add(new Property("literal", "string", "Sequence that was observed.", 0, 1, literal)); 2290 children.add(new Property("formatted", "Attachment", "Sequence that was observed as file content. Can be an actual file contents, or referenced by a URL to an external system.", 0, java.lang.Integer.MAX_VALUE, formatted)); 2291 children.add(new Property("relative", "", "A sequence defined relative to another sequence.", 0, java.lang.Integer.MAX_VALUE, relative)); 2292 } 2293 2294 @Override 2295 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2296 switch (_hash) { 2297 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier for this particular sequence instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2298 case 3575610: /*type*/ return new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type); 2299 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Substance|BiologicallyDerivedProduct|NutritionProduct)", "Indicates the subject this sequence is associated too.", 0, 1, subject); 2300 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The actual focus of a molecular sequence when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the subject would be the child (proband) and the focus would be the parent.", 0, java.lang.Integer.MAX_VALUE, focus); 2301 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen); 2302 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "The method for sequencing, for example, chip information.", 0, 1, device); 2303 case 481140686: /*performer*/ return new Property("performer", "Reference(Organization)", "The organization or lab that should be responsible for this result.", 0, 1, performer); 2304 case 182460591: /*literal*/ return new Property("literal", "string", "Sequence that was observed.", 0, 1, literal); 2305 case 1811591356: /*formatted*/ return new Property("formatted", "Attachment", "Sequence that was observed as file content. Can be an actual file contents, or referenced by a URL to an external system.", 0, java.lang.Integer.MAX_VALUE, formatted); 2306 case -554435892: /*relative*/ return new Property("relative", "", "A sequence defined relative to another sequence.", 0, java.lang.Integer.MAX_VALUE, relative); 2307 default: return super.getNamedProperty(_hash, _name, _checkValid); 2308 } 2309 2310 } 2311 2312 @Override 2313 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2314 switch (hash) { 2315 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2316 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SequenceType> 2317 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2318 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 2319 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : new Base[] {this.specimen}; // Reference 2320 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 2321 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 2322 case 182460591: /*literal*/ return this.literal == null ? new Base[0] : new Base[] {this.literal}; // StringType 2323 case 1811591356: /*formatted*/ return this.formatted == null ? new Base[0] : this.formatted.toArray(new Base[this.formatted.size()]); // Attachment 2324 case -554435892: /*relative*/ return this.relative == null ? new Base[0] : this.relative.toArray(new Base[this.relative.size()]); // MolecularSequenceRelativeComponent 2325 default: return super.getProperty(hash, name, checkValid); 2326 } 2327 2328 } 2329 2330 @Override 2331 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2332 switch (hash) { 2333 case -1618432855: // identifier 2334 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2335 return value; 2336 case 3575610: // type 2337 value = new SequenceTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2338 this.type = (Enumeration) value; // Enumeration<SequenceType> 2339 return value; 2340 case -1867885268: // subject 2341 this.subject = TypeConvertor.castToReference(value); // Reference 2342 return value; 2343 case 97604824: // focus 2344 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 2345 return value; 2346 case -2132868344: // specimen 2347 this.specimen = TypeConvertor.castToReference(value); // Reference 2348 return value; 2349 case -1335157162: // device 2350 this.device = TypeConvertor.castToReference(value); // Reference 2351 return value; 2352 case 481140686: // performer 2353 this.performer = TypeConvertor.castToReference(value); // Reference 2354 return value; 2355 case 182460591: // literal 2356 this.literal = TypeConvertor.castToString(value); // StringType 2357 return value; 2358 case 1811591356: // formatted 2359 this.getFormatted().add(TypeConvertor.castToAttachment(value)); // Attachment 2360 return value; 2361 case -554435892: // relative 2362 this.getRelative().add((MolecularSequenceRelativeComponent) value); // MolecularSequenceRelativeComponent 2363 return value; 2364 default: return super.setProperty(hash, name, value); 2365 } 2366 2367 } 2368 2369 @Override 2370 public Base setProperty(String name, Base value) throws FHIRException { 2371 if (name.equals("identifier")) { 2372 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2373 } else if (name.equals("type")) { 2374 value = new SequenceTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2375 this.type = (Enumeration) value; // Enumeration<SequenceType> 2376 } else if (name.equals("subject")) { 2377 this.subject = TypeConvertor.castToReference(value); // Reference 2378 } else if (name.equals("focus")) { 2379 this.getFocus().add(TypeConvertor.castToReference(value)); 2380 } else if (name.equals("specimen")) { 2381 this.specimen = TypeConvertor.castToReference(value); // Reference 2382 } else if (name.equals("device")) { 2383 this.device = TypeConvertor.castToReference(value); // Reference 2384 } else if (name.equals("performer")) { 2385 this.performer = TypeConvertor.castToReference(value); // Reference 2386 } else if (name.equals("literal")) { 2387 this.literal = TypeConvertor.castToString(value); // StringType 2388 } else if (name.equals("formatted")) { 2389 this.getFormatted().add(TypeConvertor.castToAttachment(value)); 2390 } else if (name.equals("relative")) { 2391 this.getRelative().add((MolecularSequenceRelativeComponent) value); 2392 } else 2393 return super.setProperty(name, value); 2394 return value; 2395 } 2396 2397 @Override 2398 public void removeChild(String name, Base value) throws FHIRException { 2399 if (name.equals("identifier")) { 2400 this.getIdentifier().remove(value); 2401 } else if (name.equals("type")) { 2402 value = new SequenceTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2403 this.type = (Enumeration) value; // Enumeration<SequenceType> 2404 } else if (name.equals("subject")) { 2405 this.subject = null; 2406 } else if (name.equals("focus")) { 2407 this.getFocus().remove(value); 2408 } else if (name.equals("specimen")) { 2409 this.specimen = null; 2410 } else if (name.equals("device")) { 2411 this.device = null; 2412 } else if (name.equals("performer")) { 2413 this.performer = null; 2414 } else if (name.equals("literal")) { 2415 this.literal = null; 2416 } else if (name.equals("formatted")) { 2417 this.getFormatted().remove(value); 2418 } else if (name.equals("relative")) { 2419 this.getRelative().remove((MolecularSequenceRelativeComponent) value); 2420 } else 2421 super.removeChild(name, value); 2422 2423 } 2424 2425 @Override 2426 public Base makeProperty(int hash, String name) throws FHIRException { 2427 switch (hash) { 2428 case -1618432855: return addIdentifier(); 2429 case 3575610: return getTypeElement(); 2430 case -1867885268: return getSubject(); 2431 case 97604824: return addFocus(); 2432 case -2132868344: return getSpecimen(); 2433 case -1335157162: return getDevice(); 2434 case 481140686: return getPerformer(); 2435 case 182460591: return getLiteralElement(); 2436 case 1811591356: return addFormatted(); 2437 case -554435892: return addRelative(); 2438 default: return super.makeProperty(hash, name); 2439 } 2440 2441 } 2442 2443 @Override 2444 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2445 switch (hash) { 2446 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2447 case 3575610: /*type*/ return new String[] {"code"}; 2448 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2449 case 97604824: /*focus*/ return new String[] {"Reference"}; 2450 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 2451 case -1335157162: /*device*/ return new String[] {"Reference"}; 2452 case 481140686: /*performer*/ return new String[] {"Reference"}; 2453 case 182460591: /*literal*/ return new String[] {"string"}; 2454 case 1811591356: /*formatted*/ return new String[] {"Attachment"}; 2455 case -554435892: /*relative*/ return new String[] {}; 2456 default: return super.getTypesForProperty(hash, name); 2457 } 2458 2459 } 2460 2461 @Override 2462 public Base addChild(String name) throws FHIRException { 2463 if (name.equals("identifier")) { 2464 return addIdentifier(); 2465 } 2466 else if (name.equals("type")) { 2467 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.type"); 2468 } 2469 else if (name.equals("subject")) { 2470 this.subject = new Reference(); 2471 return this.subject; 2472 } 2473 else if (name.equals("focus")) { 2474 return addFocus(); 2475 } 2476 else if (name.equals("specimen")) { 2477 this.specimen = new Reference(); 2478 return this.specimen; 2479 } 2480 else if (name.equals("device")) { 2481 this.device = new Reference(); 2482 return this.device; 2483 } 2484 else if (name.equals("performer")) { 2485 this.performer = new Reference(); 2486 return this.performer; 2487 } 2488 else if (name.equals("literal")) { 2489 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.literal"); 2490 } 2491 else if (name.equals("formatted")) { 2492 return addFormatted(); 2493 } 2494 else if (name.equals("relative")) { 2495 return addRelative(); 2496 } 2497 else 2498 return super.addChild(name); 2499 } 2500 2501 public String fhirType() { 2502 return "MolecularSequence"; 2503 2504 } 2505 2506 public MolecularSequence copy() { 2507 MolecularSequence dst = new MolecularSequence(); 2508 copyValues(dst); 2509 return dst; 2510 } 2511 2512 public void copyValues(MolecularSequence dst) { 2513 super.copyValues(dst); 2514 if (identifier != null) { 2515 dst.identifier = new ArrayList<Identifier>(); 2516 for (Identifier i : identifier) 2517 dst.identifier.add(i.copy()); 2518 }; 2519 dst.type = type == null ? null : type.copy(); 2520 dst.subject = subject == null ? null : subject.copy(); 2521 if (focus != null) { 2522 dst.focus = new ArrayList<Reference>(); 2523 for (Reference i : focus) 2524 dst.focus.add(i.copy()); 2525 }; 2526 dst.specimen = specimen == null ? null : specimen.copy(); 2527 dst.device = device == null ? null : device.copy(); 2528 dst.performer = performer == null ? null : performer.copy(); 2529 dst.literal = literal == null ? null : literal.copy(); 2530 if (formatted != null) { 2531 dst.formatted = new ArrayList<Attachment>(); 2532 for (Attachment i : formatted) 2533 dst.formatted.add(i.copy()); 2534 }; 2535 if (relative != null) { 2536 dst.relative = new ArrayList<MolecularSequenceRelativeComponent>(); 2537 for (MolecularSequenceRelativeComponent i : relative) 2538 dst.relative.add(i.copy()); 2539 }; 2540 } 2541 2542 protected MolecularSequence typedCopy() { 2543 return copy(); 2544 } 2545 2546 @Override 2547 public boolean equalsDeep(Base other_) { 2548 if (!super.equalsDeep(other_)) 2549 return false; 2550 if (!(other_ instanceof MolecularSequence)) 2551 return false; 2552 MolecularSequence o = (MolecularSequence) other_; 2553 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 2554 && compareDeep(focus, o.focus, true) && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 2555 && compareDeep(performer, o.performer, true) && compareDeep(literal, o.literal, true) && compareDeep(formatted, o.formatted, true) 2556 && compareDeep(relative, o.relative, true); 2557 } 2558 2559 @Override 2560 public boolean equalsShallow(Base other_) { 2561 if (!super.equalsShallow(other_)) 2562 return false; 2563 if (!(other_ instanceof MolecularSequence)) 2564 return false; 2565 MolecularSequence o = (MolecularSequence) other_; 2566 return compareValues(type, o.type, true) && compareValues(literal, o.literal, true); 2567 } 2568 2569 public boolean isEmpty() { 2570 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, subject 2571 , focus, specimen, device, performer, literal, formatted, relative); 2572 } 2573 2574 @Override 2575 public ResourceType getResourceType() { 2576 return ResourceType.MolecularSequence; 2577 } 2578 2579 /** 2580 * Search parameter: <b>focus</b> 2581 * <p> 2582 * Description: <b>What the molecular sequence is about, when it is not about the subject of record</b><br> 2583 * Type: <b>reference</b><br> 2584 * Path: <b>MolecularSequence.focus</b><br> 2585 * </p> 2586 */ 2587 @SearchParamDefinition(name="focus", path="MolecularSequence.focus", description="What the molecular sequence is about, when it is not about the subject of record", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2588 public static final String SP_FOCUS = "focus"; 2589 /** 2590 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 2591 * <p> 2592 * Description: <b>What the molecular sequence is about, when it is not about the subject of record</b><br> 2593 * Type: <b>reference</b><br> 2594 * Path: <b>MolecularSequence.focus</b><br> 2595 * </p> 2596 */ 2597 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 2598 2599/** 2600 * Constant for fluent queries to be used to add include statements. Specifies 2601 * the path value of "<b>MolecularSequence:focus</b>". 2602 */ 2603 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("MolecularSequence:focus").toLocked(); 2604 2605 /** 2606 * Search parameter: <b>subject</b> 2607 * <p> 2608 * Description: <b>The subject that the sequence is about</b><br> 2609 * Type: <b>reference</b><br> 2610 * Path: <b>MolecularSequence.subject</b><br> 2611 * </p> 2612 */ 2613 @SearchParamDefinition(name="subject", path="MolecularSequence.subject", description="The subject that the sequence is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={BiologicallyDerivedProduct.class, Group.class, NutritionProduct.class, Patient.class, Substance.class } ) 2614 public static final String SP_SUBJECT = "subject"; 2615 /** 2616 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2617 * <p> 2618 * Description: <b>The subject that the sequence is about</b><br> 2619 * Type: <b>reference</b><br> 2620 * Path: <b>MolecularSequence.subject</b><br> 2621 * </p> 2622 */ 2623 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2624 2625/** 2626 * Constant for fluent queries to be used to add include statements. Specifies 2627 * the path value of "<b>MolecularSequence:subject</b>". 2628 */ 2629 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MolecularSequence:subject").toLocked(); 2630 2631 /** 2632 * Search parameter: <b>identifier</b> 2633 * <p> 2634 * Description: <b>Multiple Resources: 2635 2636* [Account](account.html): Account number 2637* [AdverseEvent](adverseevent.html): Business identifier for the event 2638* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2639* [Appointment](appointment.html): An Identifier of the Appointment 2640* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2641* [Basic](basic.html): Business identifier 2642* [BodyStructure](bodystructure.html): Bodystructure identifier 2643* [CarePlan](careplan.html): External Ids for this plan 2644* [CareTeam](careteam.html): External Ids for this team 2645* [ChargeItem](chargeitem.html): Business Identifier for item 2646* [Claim](claim.html): The primary identifier of the financial resource 2647* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2648* [ClinicalImpression](clinicalimpression.html): Business identifier 2649* [Communication](communication.html): Unique identifier 2650* [CommunicationRequest](communicationrequest.html): Unique identifier 2651* [Composition](composition.html): Version-independent identifier for the Composition 2652* [Condition](condition.html): A unique identifier of the condition record 2653* [Consent](consent.html): Identifier for this record (external references) 2654* [Contract](contract.html): The identity of the contract 2655* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2656* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2657* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2658* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2659* [DeviceRequest](devicerequest.html): Business identifier for request/order 2660* [DeviceUsage](deviceusage.html): Search by identifier 2661* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2662* [DocumentReference](documentreference.html): Identifier of the attachment binary 2663* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2664* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2665* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2666* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2667* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2668* [Flag](flag.html): Business identifier 2669* [Goal](goal.html): External Ids for this goal 2670* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2671* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2672* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2673* [Immunization](immunization.html): Business identifier 2674* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2675* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2676* [Invoice](invoice.html): Business Identifier for item 2677* [List](list.html): Business identifier 2678* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2679* [Medication](medication.html): Returns medications with this external identifier 2680* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2681* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2682* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2683* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2684* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2685* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2686* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2687* [Observation](observation.html): The unique id for a particular observation 2688* [Person](person.html): A person Identifier 2689* [Procedure](procedure.html): A unique identifier for a procedure 2690* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2691* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2692* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2693* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2694* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2695* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2696* [Specimen](specimen.html): The unique identifier associated with the specimen 2697* [SupplyDelivery](supplydelivery.html): External identifier 2698* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2699* [Task](task.html): Search for a task instance by its business identifier 2700* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2701</b><br> 2702 * Type: <b>token</b><br> 2703 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2704 * </p> 2705 */ 2706 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2707 public static final String SP_IDENTIFIER = "identifier"; 2708 /** 2709 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2710 * <p> 2711 * Description: <b>Multiple Resources: 2712 2713* [Account](account.html): Account number 2714* [AdverseEvent](adverseevent.html): Business identifier for the event 2715* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2716* [Appointment](appointment.html): An Identifier of the Appointment 2717* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2718* [Basic](basic.html): Business identifier 2719* [BodyStructure](bodystructure.html): Bodystructure identifier 2720* [CarePlan](careplan.html): External Ids for this plan 2721* [CareTeam](careteam.html): External Ids for this team 2722* [ChargeItem](chargeitem.html): Business Identifier for item 2723* [Claim](claim.html): The primary identifier of the financial resource 2724* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2725* [ClinicalImpression](clinicalimpression.html): Business identifier 2726* [Communication](communication.html): Unique identifier 2727* [CommunicationRequest](communicationrequest.html): Unique identifier 2728* [Composition](composition.html): Version-independent identifier for the Composition 2729* [Condition](condition.html): A unique identifier of the condition record 2730* [Consent](consent.html): Identifier for this record (external references) 2731* [Contract](contract.html): The identity of the contract 2732* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2733* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2734* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2735* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2736* [DeviceRequest](devicerequest.html): Business identifier for request/order 2737* [DeviceUsage](deviceusage.html): Search by identifier 2738* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2739* [DocumentReference](documentreference.html): Identifier of the attachment binary 2740* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2741* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2742* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2743* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2744* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2745* [Flag](flag.html): Business identifier 2746* [Goal](goal.html): External Ids for this goal 2747* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2748* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2749* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2750* [Immunization](immunization.html): Business identifier 2751* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2752* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2753* [Invoice](invoice.html): Business Identifier for item 2754* [List](list.html): Business identifier 2755* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2756* [Medication](medication.html): Returns medications with this external identifier 2757* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2758* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2759* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2760* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2761* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2762* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2763* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2764* [Observation](observation.html): The unique id for a particular observation 2765* [Person](person.html): A person Identifier 2766* [Procedure](procedure.html): A unique identifier for a procedure 2767* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2768* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2769* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2770* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2771* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2772* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2773* [Specimen](specimen.html): The unique identifier associated with the specimen 2774* [SupplyDelivery](supplydelivery.html): External identifier 2775* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2776* [Task](task.html): Search for a task instance by its business identifier 2777* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2778</b><br> 2779 * Type: <b>token</b><br> 2780 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2781 * </p> 2782 */ 2783 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2784 2785 /** 2786 * Search parameter: <b>patient</b> 2787 * <p> 2788 * Description: <b>Multiple Resources: 2789 2790* [Account](account.html): The entity that caused the expenses 2791* [AdverseEvent](adverseevent.html): Subject impacted by event 2792* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2793* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2794* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2795* [AuditEvent](auditevent.html): Where the activity involved patient data 2796* [Basic](basic.html): Identifies the focus of this resource 2797* [BodyStructure](bodystructure.html): Who this is about 2798* [CarePlan](careplan.html): Who the care plan is for 2799* [CareTeam](careteam.html): Who care team is for 2800* [ChargeItem](chargeitem.html): Individual service was done for/to 2801* [Claim](claim.html): Patient receiving the products or services 2802* [ClaimResponse](claimresponse.html): The subject of care 2803* [ClinicalImpression](clinicalimpression.html): Patient assessed 2804* [Communication](communication.html): Focus of message 2805* [CommunicationRequest](communicationrequest.html): Focus of message 2806* [Composition](composition.html): Who and/or what the composition is about 2807* [Condition](condition.html): Who has the condition? 2808* [Consent](consent.html): Who the consent applies to 2809* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2810* [Coverage](coverage.html): Retrieve coverages for a patient 2811* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2812* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2813* [DetectedIssue](detectedissue.html): Associated patient 2814* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2815* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2816* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2817* [DocumentReference](documentreference.html): Who/what is the subject of the document 2818* [Encounter](encounter.html): The patient present at the encounter 2819* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2820* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2821* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2822* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2823* [Flag](flag.html): The identity of a subject to list flags for 2824* [Goal](goal.html): Who this goal is intended for 2825* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2826* [ImagingSelection](imagingselection.html): Who the study is about 2827* [ImagingStudy](imagingstudy.html): Who the study is about 2828* [Immunization](immunization.html): The patient for the vaccination record 2829* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2830* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2831* [Invoice](invoice.html): Recipient(s) of goods and services 2832* [List](list.html): If all resources have the same subject 2833* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2834* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2835* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2836* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2837* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2838* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2839* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2840* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2841* [Observation](observation.html): The subject that the observation is about (if patient) 2842* [Person](person.html): The Person links to this Patient 2843* [Procedure](procedure.html): Search by subject - a patient 2844* [Provenance](provenance.html): Where the activity involved patient data 2845* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2846* [RelatedPerson](relatedperson.html): The patient this related person is related to 2847* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2848* [ResearchSubject](researchsubject.html): Who or what is part of study 2849* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2850* [ServiceRequest](servicerequest.html): Search by subject - a patient 2851* [Specimen](specimen.html): The patient the specimen comes from 2852* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2853* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2854* [Task](task.html): Search by patient 2855* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2856</b><br> 2857 * Type: <b>reference</b><br> 2858 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2859 * </p> 2860 */ 2861 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2862 public static final String SP_PATIENT = "patient"; 2863 /** 2864 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2865 * <p> 2866 * Description: <b>Multiple Resources: 2867 2868* [Account](account.html): The entity that caused the expenses 2869* [AdverseEvent](adverseevent.html): Subject impacted by event 2870* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2871* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2872* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2873* [AuditEvent](auditevent.html): Where the activity involved patient data 2874* [Basic](basic.html): Identifies the focus of this resource 2875* [BodyStructure](bodystructure.html): Who this is about 2876* [CarePlan](careplan.html): Who the care plan is for 2877* [CareTeam](careteam.html): Who care team is for 2878* [ChargeItem](chargeitem.html): Individual service was done for/to 2879* [Claim](claim.html): Patient receiving the products or services 2880* [ClaimResponse](claimresponse.html): The subject of care 2881* [ClinicalImpression](clinicalimpression.html): Patient assessed 2882* [Communication](communication.html): Focus of message 2883* [CommunicationRequest](communicationrequest.html): Focus of message 2884* [Composition](composition.html): Who and/or what the composition is about 2885* [Condition](condition.html): Who has the condition? 2886* [Consent](consent.html): Who the consent applies to 2887* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2888* [Coverage](coverage.html): Retrieve coverages for a patient 2889* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2890* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2891* [DetectedIssue](detectedissue.html): Associated patient 2892* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2893* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2894* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2895* [DocumentReference](documentreference.html): Who/what is the subject of the document 2896* [Encounter](encounter.html): The patient present at the encounter 2897* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2898* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2899* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2900* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2901* [Flag](flag.html): The identity of a subject to list flags for 2902* [Goal](goal.html): Who this goal is intended for 2903* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2904* [ImagingSelection](imagingselection.html): Who the study is about 2905* [ImagingStudy](imagingstudy.html): Who the study is about 2906* [Immunization](immunization.html): The patient for the vaccination record 2907* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2908* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2909* [Invoice](invoice.html): Recipient(s) of goods and services 2910* [List](list.html): If all resources have the same subject 2911* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2912* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2913* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2914* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2915* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2916* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2917* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2918* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2919* [Observation](observation.html): The subject that the observation is about (if patient) 2920* [Person](person.html): The Person links to this Patient 2921* [Procedure](procedure.html): Search by subject - a patient 2922* [Provenance](provenance.html): Where the activity involved patient data 2923* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2924* [RelatedPerson](relatedperson.html): The patient this related person is related to 2925* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2926* [ResearchSubject](researchsubject.html): Who or what is part of study 2927* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2928* [ServiceRequest](servicerequest.html): Search by subject - a patient 2929* [Specimen](specimen.html): The patient the specimen comes from 2930* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2931* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2932* [Task](task.html): Search by patient 2933* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2934</b><br> 2935 * Type: <b>reference</b><br> 2936 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2937 * </p> 2938 */ 2939 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2940 2941/** 2942 * Constant for fluent queries to be used to add include statements. Specifies 2943 * the path value of "<b>MolecularSequence:patient</b>". 2944 */ 2945 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MolecularSequence:patient").toLocked(); 2946 2947 /** 2948 * Search parameter: <b>type</b> 2949 * <p> 2950 * Description: <b>Multiple Resources: 2951 2952* [Account](account.html): E.g. patient, expense, depreciation 2953* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2954* [Composition](composition.html): Kind of composition (LOINC if possible) 2955* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2956* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2957* [Encounter](encounter.html): Specific type of encounter 2958* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2959* [Invoice](invoice.html): Type of Invoice 2960* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2961* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2962* [Specimen](specimen.html): The specimen type 2963</b><br> 2964 * Type: <b>token</b><br> 2965 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2966 * </p> 2967 */ 2968 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 2969 public static final String SP_TYPE = "type"; 2970 /** 2971 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2972 * <p> 2973 * Description: <b>Multiple Resources: 2974 2975* [Account](account.html): E.g. patient, expense, depreciation 2976* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2977* [Composition](composition.html): Kind of composition (LOINC if possible) 2978* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2979* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2980* [Encounter](encounter.html): Specific type of encounter 2981* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2982* [Invoice](invoice.html): Type of Invoice 2983* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2984* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2985* [Specimen](specimen.html): The specimen type 2986</b><br> 2987 * Type: <b>token</b><br> 2988 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2989 * </p> 2990 */ 2991 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2992 2993 2994} 2995