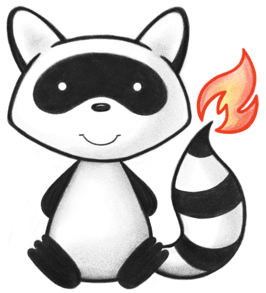
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * MonetaryComponent Type: Availability data for an {item}. 051 */ 052@DatatypeDef(name="MonetaryComponent") 053public class MonetaryComponent extends DataType implements ICompositeType { 054 055 public enum PriceComponentType { 056 /** 057 * the amount is the base price used for calculating the total price before applying surcharges, discount or taxes. 058 */ 059 BASE, 060 /** 061 * the amount is a surcharge applied on the base price. 062 */ 063 SURCHARGE, 064 /** 065 * the amount is a deduction applied on the base price. 066 */ 067 DEDUCTION, 068 /** 069 * the amount is a discount applied on the base price. 070 */ 071 DISCOUNT, 072 /** 073 * the amount is the tax component of the total price. 074 */ 075 TAX, 076 /** 077 * the amount is of informational character, it has not been applied in the calculation of the total price. 078 */ 079 INFORMATIONAL, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static PriceComponentType fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("base".equals(codeString)) 088 return BASE; 089 if ("surcharge".equals(codeString)) 090 return SURCHARGE; 091 if ("deduction".equals(codeString)) 092 return DEDUCTION; 093 if ("discount".equals(codeString)) 094 return DISCOUNT; 095 if ("tax".equals(codeString)) 096 return TAX; 097 if ("informational".equals(codeString)) 098 return INFORMATIONAL; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown PriceComponentType code '"+codeString+"'"); 103 } 104 public String toCode() { 105 switch (this) { 106 case BASE: return "base"; 107 case SURCHARGE: return "surcharge"; 108 case DEDUCTION: return "deduction"; 109 case DISCOUNT: return "discount"; 110 case TAX: return "tax"; 111 case INFORMATIONAL: return "informational"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getSystem() { 117 switch (this) { 118 case BASE: return "http://hl7.org/fhir/price-component-type"; 119 case SURCHARGE: return "http://hl7.org/fhir/price-component-type"; 120 case DEDUCTION: return "http://hl7.org/fhir/price-component-type"; 121 case DISCOUNT: return "http://hl7.org/fhir/price-component-type"; 122 case TAX: return "http://hl7.org/fhir/price-component-type"; 123 case INFORMATIONAL: return "http://hl7.org/fhir/price-component-type"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 public String getDefinition() { 129 switch (this) { 130 case BASE: return "the amount is the base price used for calculating the total price before applying surcharges, discount or taxes."; 131 case SURCHARGE: return "the amount is a surcharge applied on the base price."; 132 case DEDUCTION: return "the amount is a deduction applied on the base price."; 133 case DISCOUNT: return "the amount is a discount applied on the base price."; 134 case TAX: return "the amount is the tax component of the total price."; 135 case INFORMATIONAL: return "the amount is of informational character, it has not been applied in the calculation of the total price."; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 public String getDisplay() { 141 switch (this) { 142 case BASE: return "base price"; 143 case SURCHARGE: return "surcharge"; 144 case DEDUCTION: return "deduction"; 145 case DISCOUNT: return "discount"; 146 case TAX: return "tax"; 147 case INFORMATIONAL: return "informational"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 } 153 154 public static class PriceComponentTypeEnumFactory implements EnumFactory<PriceComponentType> { 155 public PriceComponentType fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("base".equals(codeString)) 160 return PriceComponentType.BASE; 161 if ("surcharge".equals(codeString)) 162 return PriceComponentType.SURCHARGE; 163 if ("deduction".equals(codeString)) 164 return PriceComponentType.DEDUCTION; 165 if ("discount".equals(codeString)) 166 return PriceComponentType.DISCOUNT; 167 if ("tax".equals(codeString)) 168 return PriceComponentType.TAX; 169 if ("informational".equals(codeString)) 170 return PriceComponentType.INFORMATIONAL; 171 throw new IllegalArgumentException("Unknown PriceComponentType code '"+codeString+"'"); 172 } 173 public Enumeration<PriceComponentType> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<PriceComponentType>(this, PriceComponentType.NULL, code); 178 String codeString = ((PrimitiveType) code).asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return new Enumeration<PriceComponentType>(this, PriceComponentType.NULL, code); 181 if ("base".equals(codeString)) 182 return new Enumeration<PriceComponentType>(this, PriceComponentType.BASE, code); 183 if ("surcharge".equals(codeString)) 184 return new Enumeration<PriceComponentType>(this, PriceComponentType.SURCHARGE, code); 185 if ("deduction".equals(codeString)) 186 return new Enumeration<PriceComponentType>(this, PriceComponentType.DEDUCTION, code); 187 if ("discount".equals(codeString)) 188 return new Enumeration<PriceComponentType>(this, PriceComponentType.DISCOUNT, code); 189 if ("tax".equals(codeString)) 190 return new Enumeration<PriceComponentType>(this, PriceComponentType.TAX, code); 191 if ("informational".equals(codeString)) 192 return new Enumeration<PriceComponentType>(this, PriceComponentType.INFORMATIONAL, code); 193 throw new FHIRException("Unknown PriceComponentType code '"+codeString+"'"); 194 } 195 public String toCode(PriceComponentType code) { 196 if (code == PriceComponentType.NULL) 197 return null; 198 if (code == PriceComponentType.BASE) 199 return "base"; 200 if (code == PriceComponentType.SURCHARGE) 201 return "surcharge"; 202 if (code == PriceComponentType.DEDUCTION) 203 return "deduction"; 204 if (code == PriceComponentType.DISCOUNT) 205 return "discount"; 206 if (code == PriceComponentType.TAX) 207 return "tax"; 208 if (code == PriceComponentType.INFORMATIONAL) 209 return "informational"; 210 return "?"; 211 } 212 public String toSystem(PriceComponentType code) { 213 return code.getSystem(); 214 } 215 } 216 217 /** 218 * base | surcharge | deduction | discount | tax | informational. 219 */ 220 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 221 @Description(shortDefinition="base | surcharge | deduction | discount | tax | informational", formalDefinition="base | surcharge | deduction | discount | tax | informational." ) 222 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/price-component-type") 223 protected Enumeration<PriceComponentType> type; 224 225 /** 226 * Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc. 227 */ 228 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 229 @Description(shortDefinition="Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", formalDefinition="Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc." ) 230 protected CodeableConcept code; 231 232 /** 233 * Factor used for calculating this component. 234 */ 235 @Child(name = "factor", type = {DecimalType.class}, order=2, min=0, max=1, modifier=false, summary=true) 236 @Description(shortDefinition="Factor used for calculating this component", formalDefinition="Factor used for calculating this component." ) 237 protected DecimalType factor; 238 239 /** 240 * Explicit value amount to be used. 241 */ 242 @Child(name = "amount", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=true) 243 @Description(shortDefinition="Explicit value amount to be used", formalDefinition="Explicit value amount to be used." ) 244 protected Money amount; 245 246 private static final long serialVersionUID = 576423679L; 247 248 /** 249 * Constructor 250 */ 251 public MonetaryComponent() { 252 super(); 253 } 254 255 /** 256 * Constructor 257 */ 258 public MonetaryComponent(PriceComponentType type) { 259 super(); 260 this.setType(type); 261 } 262 263 /** 264 * @return {@link #type} (base | surcharge | deduction | discount | tax | informational.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 265 */ 266 public Enumeration<PriceComponentType> getTypeElement() { 267 if (this.type == null) 268 if (Configuration.errorOnAutoCreate()) 269 throw new Error("Attempt to auto-create MonetaryComponent.type"); 270 else if (Configuration.doAutoCreate()) 271 this.type = new Enumeration<PriceComponentType>(new PriceComponentTypeEnumFactory()); // bb 272 return this.type; 273 } 274 275 public boolean hasTypeElement() { 276 return this.type != null && !this.type.isEmpty(); 277 } 278 279 public boolean hasType() { 280 return this.type != null && !this.type.isEmpty(); 281 } 282 283 /** 284 * @param value {@link #type} (base | surcharge | deduction | discount | tax | informational.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 285 */ 286 public MonetaryComponent setTypeElement(Enumeration<PriceComponentType> value) { 287 this.type = value; 288 return this; 289 } 290 291 /** 292 * @return base | surcharge | deduction | discount | tax | informational. 293 */ 294 public PriceComponentType getType() { 295 return this.type == null ? null : this.type.getValue(); 296 } 297 298 /** 299 * @param value base | surcharge | deduction | discount | tax | informational. 300 */ 301 public MonetaryComponent setType(PriceComponentType value) { 302 if (this.type == null) 303 this.type = new Enumeration<PriceComponentType>(new PriceComponentTypeEnumFactory()); 304 this.type.setValue(value); 305 return this; 306 } 307 308 /** 309 * @return {@link #code} (Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.) 310 */ 311 public CodeableConcept getCode() { 312 if (this.code == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create MonetaryComponent.code"); 315 else if (Configuration.doAutoCreate()) 316 this.code = new CodeableConcept(); // cc 317 return this.code; 318 } 319 320 public boolean hasCode() { 321 return this.code != null && !this.code.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #code} (Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.) 326 */ 327 public MonetaryComponent setCode(CodeableConcept value) { 328 this.code = value; 329 return this; 330 } 331 332 /** 333 * @return {@link #factor} (Factor used for calculating this component.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 334 */ 335 public DecimalType getFactorElement() { 336 if (this.factor == null) 337 if (Configuration.errorOnAutoCreate()) 338 throw new Error("Attempt to auto-create MonetaryComponent.factor"); 339 else if (Configuration.doAutoCreate()) 340 this.factor = new DecimalType(); // bb 341 return this.factor; 342 } 343 344 public boolean hasFactorElement() { 345 return this.factor != null && !this.factor.isEmpty(); 346 } 347 348 public boolean hasFactor() { 349 return this.factor != null && !this.factor.isEmpty(); 350 } 351 352 /** 353 * @param value {@link #factor} (Factor used for calculating this component.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 354 */ 355 public MonetaryComponent setFactorElement(DecimalType value) { 356 this.factor = value; 357 return this; 358 } 359 360 /** 361 * @return Factor used for calculating this component. 362 */ 363 public BigDecimal getFactor() { 364 return this.factor == null ? null : this.factor.getValue(); 365 } 366 367 /** 368 * @param value Factor used for calculating this component. 369 */ 370 public MonetaryComponent setFactor(BigDecimal value) { 371 if (value == null) 372 this.factor = null; 373 else { 374 if (this.factor == null) 375 this.factor = new DecimalType(); 376 this.factor.setValue(value); 377 } 378 return this; 379 } 380 381 /** 382 * @param value Factor used for calculating this component. 383 */ 384 public MonetaryComponent setFactor(long value) { 385 this.factor = new DecimalType(); 386 this.factor.setValue(value); 387 return this; 388 } 389 390 /** 391 * @param value Factor used for calculating this component. 392 */ 393 public MonetaryComponent setFactor(double value) { 394 this.factor = new DecimalType(); 395 this.factor.setValue(value); 396 return this; 397 } 398 399 /** 400 * @return {@link #amount} (Explicit value amount to be used.) 401 */ 402 public Money getAmount() { 403 if (this.amount == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create MonetaryComponent.amount"); 406 else if (Configuration.doAutoCreate()) 407 this.amount = new Money(); // cc 408 return this.amount; 409 } 410 411 public boolean hasAmount() { 412 return this.amount != null && !this.amount.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #amount} (Explicit value amount to be used.) 417 */ 418 public MonetaryComponent setAmount(Money value) { 419 this.amount = value; 420 return this; 421 } 422 423 protected void listChildren(List<Property> children) { 424 super.listChildren(children); 425 children.add(new Property("type", "code", "base | surcharge | deduction | discount | tax | informational.", 0, 1, type)); 426 children.add(new Property("code", "CodeableConcept", "Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 0, 1, code)); 427 children.add(new Property("factor", "decimal", "Factor used for calculating this component.", 0, 1, factor)); 428 children.add(new Property("amount", "Money", "Explicit value amount to be used.", 0, 1, amount)); 429 } 430 431 @Override 432 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 433 switch (_hash) { 434 case 3575610: /*type*/ return new Property("type", "code", "base | surcharge | deduction | discount | tax | informational.", 0, 1, type); 435 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 0, 1, code); 436 case -1282148017: /*factor*/ return new Property("factor", "decimal", "Factor used for calculating this component.", 0, 1, factor); 437 case -1413853096: /*amount*/ return new Property("amount", "Money", "Explicit value amount to be used.", 0, 1, amount); 438 default: return super.getNamedProperty(_hash, _name, _checkValid); 439 } 440 441 } 442 443 @Override 444 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 445 switch (hash) { 446 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<PriceComponentType> 447 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 448 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 449 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 450 default: return super.getProperty(hash, name, checkValid); 451 } 452 453 } 454 455 @Override 456 public Base setProperty(int hash, String name, Base value) throws FHIRException { 457 switch (hash) { 458 case 3575610: // type 459 value = new PriceComponentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 460 this.type = (Enumeration) value; // Enumeration<PriceComponentType> 461 return value; 462 case 3059181: // code 463 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 464 return value; 465 case -1282148017: // factor 466 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 467 return value; 468 case -1413853096: // amount 469 this.amount = TypeConvertor.castToMoney(value); // Money 470 return value; 471 default: return super.setProperty(hash, name, value); 472 } 473 474 } 475 476 @Override 477 public Base setProperty(String name, Base value) throws FHIRException { 478 if (name.equals("type")) { 479 value = new PriceComponentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 480 this.type = (Enumeration) value; // Enumeration<PriceComponentType> 481 } else if (name.equals("code")) { 482 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 483 } else if (name.equals("factor")) { 484 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 485 } else if (name.equals("amount")) { 486 this.amount = TypeConvertor.castToMoney(value); // Money 487 } else 488 return super.setProperty(name, value); 489 return value; 490 } 491 492 @Override 493 public void removeChild(String name, Base value) throws FHIRException { 494 if (name.equals("type")) { 495 value = new PriceComponentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 496 this.type = (Enumeration) value; // Enumeration<PriceComponentType> 497 } else if (name.equals("code")) { 498 this.code = null; 499 } else if (name.equals("factor")) { 500 this.factor = null; 501 } else if (name.equals("amount")) { 502 this.amount = null; 503 } else 504 super.removeChild(name, value); 505 506 } 507 508 @Override 509 public Base makeProperty(int hash, String name) throws FHIRException { 510 switch (hash) { 511 case 3575610: return getTypeElement(); 512 case 3059181: return getCode(); 513 case -1282148017: return getFactorElement(); 514 case -1413853096: return getAmount(); 515 default: return super.makeProperty(hash, name); 516 } 517 518 } 519 520 @Override 521 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 522 switch (hash) { 523 case 3575610: /*type*/ return new String[] {"code"}; 524 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 525 case -1282148017: /*factor*/ return new String[] {"decimal"}; 526 case -1413853096: /*amount*/ return new String[] {"Money"}; 527 default: return super.getTypesForProperty(hash, name); 528 } 529 530 } 531 532 @Override 533 public Base addChild(String name) throws FHIRException { 534 if (name.equals("type")) { 535 throw new FHIRException("Cannot call addChild on a singleton property MonetaryComponent.type"); 536 } 537 else if (name.equals("code")) { 538 this.code = new CodeableConcept(); 539 return this.code; 540 } 541 else if (name.equals("factor")) { 542 throw new FHIRException("Cannot call addChild on a singleton property MonetaryComponent.factor"); 543 } 544 else if (name.equals("amount")) { 545 this.amount = new Money(); 546 return this.amount; 547 } 548 else 549 return super.addChild(name); 550 } 551 552 public String fhirType() { 553 return "MonetaryComponent"; 554 555 } 556 557 public MonetaryComponent copy() { 558 MonetaryComponent dst = new MonetaryComponent(); 559 copyValues(dst); 560 return dst; 561 } 562 563 public void copyValues(MonetaryComponent dst) { 564 super.copyValues(dst); 565 dst.type = type == null ? null : type.copy(); 566 dst.code = code == null ? null : code.copy(); 567 dst.factor = factor == null ? null : factor.copy(); 568 dst.amount = amount == null ? null : amount.copy(); 569 } 570 571 protected MonetaryComponent typedCopy() { 572 return copy(); 573 } 574 575 @Override 576 public boolean equalsDeep(Base other_) { 577 if (!super.equalsDeep(other_)) 578 return false; 579 if (!(other_ instanceof MonetaryComponent)) 580 return false; 581 MonetaryComponent o = (MonetaryComponent) other_; 582 return compareDeep(type, o.type, true) && compareDeep(code, o.code, true) && compareDeep(factor, o.factor, true) 583 && compareDeep(amount, o.amount, true); 584 } 585 586 @Override 587 public boolean equalsShallow(Base other_) { 588 if (!super.equalsShallow(other_)) 589 return false; 590 if (!(other_ instanceof MonetaryComponent)) 591 return false; 592 MonetaryComponent o = (MonetaryComponent) other_; 593 return compareValues(type, o.type, true) && compareValues(factor, o.factor, true); 594 } 595 596 public boolean isEmpty() { 597 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, code, factor, amount 598 ); 599 } 600 601 602} 603