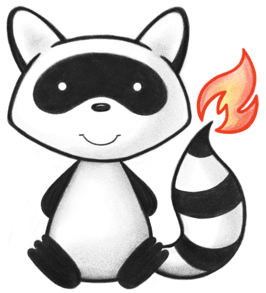
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Money Type: An amount of economic utility in some recognized currency. 051 */ 052@DatatypeDef(name="Money") 053public class Money extends DataType implements ICompositeType { 054 055 /** 056 * Numerical value (with implicit precision). 057 */ 058 @Child(name = "value", type = {DecimalType.class}, order=0, min=0, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Numerical value (with implicit precision)", formalDefinition="Numerical value (with implicit precision)." ) 060 protected DecimalType value; 061 062 /** 063 * ISO 4217 Currency Code. 064 */ 065 @Child(name = "currency", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="ISO 4217 Currency Code", formalDefinition="ISO 4217 Currency Code." ) 067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/currencies") 068 protected CodeType currency; 069 070 private static final long serialVersionUID = -484637938L; 071 072 /** 073 * Constructor 074 */ 075 public Money() { 076 super(); 077 } 078 079 /** 080 * @return {@link #value} (Numerical value (with implicit precision).). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 081 */ 082 public DecimalType getValueElement() { 083 if (this.value == null) 084 if (Configuration.errorOnAutoCreate()) 085 throw new Error("Attempt to auto-create Money.value"); 086 else if (Configuration.doAutoCreate()) 087 this.value = new DecimalType(); // bb 088 return this.value; 089 } 090 091 public boolean hasValueElement() { 092 return this.value != null && !this.value.isEmpty(); 093 } 094 095 public boolean hasValue() { 096 return this.value != null && !this.value.isEmpty(); 097 } 098 099 /** 100 * @param value {@link #value} (Numerical value (with implicit precision).). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 101 */ 102 public Money setValueElement(DecimalType value) { 103 this.value = value; 104 return this; 105 } 106 107 /** 108 * @return Numerical value (with implicit precision). 109 */ 110 public BigDecimal getValue() { 111 return this.value == null ? null : this.value.getValue(); 112 } 113 114 /** 115 * @param value Numerical value (with implicit precision). 116 */ 117 public Money setValue(BigDecimal value) { 118 if (value == null) 119 this.value = null; 120 else { 121 if (this.value == null) 122 this.value = new DecimalType(); 123 this.value.setValue(value); 124 } 125 return this; 126 } 127 128 /** 129 * @param value Numerical value (with implicit precision). 130 */ 131 public Money setValue(long value) { 132 this.value = new DecimalType(); 133 this.value.setValue(value); 134 return this; 135 } 136 137 /** 138 * @param value Numerical value (with implicit precision). 139 */ 140 public Money setValue(double value) { 141 this.value = new DecimalType(); 142 this.value.setValue(value); 143 return this; 144 } 145 146 /** 147 * @return {@link #currency} (ISO 4217 Currency Code.). This is the underlying object with id, value and extensions. The accessor "getCurrency" gives direct access to the value 148 */ 149 public CodeType getCurrencyElement() { 150 if (this.currency == null) 151 if (Configuration.errorOnAutoCreate()) 152 throw new Error("Attempt to auto-create Money.currency"); 153 else if (Configuration.doAutoCreate()) 154 this.currency = new CodeType(); // bb 155 return this.currency; 156 } 157 158 public boolean hasCurrencyElement() { 159 return this.currency != null && !this.currency.isEmpty(); 160 } 161 162 public boolean hasCurrency() { 163 return this.currency != null && !this.currency.isEmpty(); 164 } 165 166 /** 167 * @param value {@link #currency} (ISO 4217 Currency Code.). This is the underlying object with id, value and extensions. The accessor "getCurrency" gives direct access to the value 168 */ 169 public Money setCurrencyElement(CodeType value) { 170 this.currency = value; 171 return this; 172 } 173 174 /** 175 * @return ISO 4217 Currency Code. 176 */ 177 public String getCurrency() { 178 return this.currency == null ? null : this.currency.getValue(); 179 } 180 181 /** 182 * @param value ISO 4217 Currency Code. 183 */ 184 public Money setCurrency(String value) { 185 if (Utilities.noString(value)) 186 this.currency = null; 187 else { 188 if (this.currency == null) 189 this.currency = new CodeType(); 190 this.currency.setValue(value); 191 } 192 return this; 193 } 194 195 protected void listChildren(List<Property> children) { 196 super.listChildren(children); 197 children.add(new Property("value", "decimal", "Numerical value (with implicit precision).", 0, 1, value)); 198 children.add(new Property("currency", "code", "ISO 4217 Currency Code.", 0, 1, currency)); 199 } 200 201 @Override 202 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 203 switch (_hash) { 204 case 111972721: /*value*/ return new Property("value", "decimal", "Numerical value (with implicit precision).", 0, 1, value); 205 case 575402001: /*currency*/ return new Property("currency", "code", "ISO 4217 Currency Code.", 0, 1, currency); 206 default: return super.getNamedProperty(_hash, _name, _checkValid); 207 } 208 209 } 210 211 @Override 212 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 213 switch (hash) { 214 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DecimalType 215 case 575402001: /*currency*/ return this.currency == null ? new Base[0] : new Base[] {this.currency}; // CodeType 216 default: return super.getProperty(hash, name, checkValid); 217 } 218 219 } 220 221 @Override 222 public Base setProperty(int hash, String name, Base value) throws FHIRException { 223 switch (hash) { 224 case 111972721: // value 225 this.value = TypeConvertor.castToDecimal(value); // DecimalType 226 return value; 227 case 575402001: // currency 228 this.currency = TypeConvertor.castToCode(value); // CodeType 229 return value; 230 default: return super.setProperty(hash, name, value); 231 } 232 233 } 234 235 @Override 236 public Base setProperty(String name, Base value) throws FHIRException { 237 if (name.equals("value")) { 238 this.value = TypeConvertor.castToDecimal(value); // DecimalType 239 } else if (name.equals("currency")) { 240 this.currency = TypeConvertor.castToCode(value); // CodeType 241 } else 242 return super.setProperty(name, value); 243 return value; 244 } 245 246 @Override 247 public void removeChild(String name, Base value) throws FHIRException { 248 if (name.equals("value")) { 249 this.value = null; 250 } else if (name.equals("currency")) { 251 this.currency = null; 252 } else 253 super.removeChild(name, value); 254 255 } 256 257 @Override 258 public Base makeProperty(int hash, String name) throws FHIRException { 259 switch (hash) { 260 case 111972721: return getValueElement(); 261 case 575402001: return getCurrencyElement(); 262 default: return super.makeProperty(hash, name); 263 } 264 265 } 266 267 @Override 268 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 269 switch (hash) { 270 case 111972721: /*value*/ return new String[] {"decimal"}; 271 case 575402001: /*currency*/ return new String[] {"code"}; 272 default: return super.getTypesForProperty(hash, name); 273 } 274 275 } 276 277 @Override 278 public Base addChild(String name) throws FHIRException { 279 if (name.equals("value")) { 280 throw new FHIRException("Cannot call addChild on a singleton property Money.value"); 281 } 282 else if (name.equals("currency")) { 283 throw new FHIRException("Cannot call addChild on a singleton property Money.currency"); 284 } 285 else 286 return super.addChild(name); 287 } 288 289 public String fhirType() { 290 return "Money"; 291 292 } 293 294 public Money copy() { 295 Money dst = new Money(); 296 copyValues(dst); 297 return dst; 298 } 299 300 public void copyValues(Money dst) { 301 super.copyValues(dst); 302 dst.value = value == null ? null : value.copy(); 303 dst.currency = currency == null ? null : currency.copy(); 304 } 305 306 protected Money typedCopy() { 307 return copy(); 308 } 309 310 @Override 311 public boolean equalsDeep(Base other_) { 312 if (!super.equalsDeep(other_)) 313 return false; 314 if (!(other_ instanceof Money)) 315 return false; 316 Money o = (Money) other_; 317 return compareDeep(value, o.value, true) && compareDeep(currency, o.currency, true); 318 } 319 320 @Override 321 public boolean equalsShallow(Base other_) { 322 if (!super.equalsShallow(other_)) 323 return false; 324 if (!(other_ instanceof Money)) 325 return false; 326 Money o = (Money) other_; 327 return compareValues(value, o.value, true) && compareValues(currency, o.currency, true); 328 } 329 330 public boolean isEmpty() { 331 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, currency); 332 } 333 334 335} 336