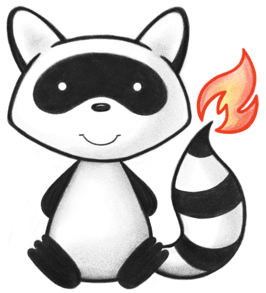
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 052 */ 053@ResourceDef(name="NamingSystem", profile="http://hl7.org/fhir/StructureDefinition/NamingSystem") 054public class NamingSystem extends MetadataResource { 055 056 public enum NamingSystemIdentifierType { 057 /** 058 * An ISO object identifier; e.g. 1.2.3.4.5. 059 */ 060 OID, 061 /** 062 * A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11. 063 */ 064 UUID, 065 /** 066 * A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org. 067 */ 068 URI, 069 /** 070 * An IRI string that can be prepended to the code to obtain a concept IRI for RDF applications. This should be a valid, absolute IRI as defined in RFC 3987. See rdf.html#iri-stem for details on how this value may be used. 071 */ 072 IRISTEM, 073 /** 074 * A short string published by HL7 for use in the V2 family of standsrds to idenfify a code system in the V12 coded data types CWE, CNE, and CF. The code values are also published by HL7 at http://www.hl7.org/Special/committees/vocab/table_0396/index.cfm 075 */ 076 V2CSMNEMONIC, 077 /** 078 * Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC. 079 */ 080 OTHER, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static NamingSystemIdentifierType fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("oid".equals(codeString)) 089 return OID; 090 if ("uuid".equals(codeString)) 091 return UUID; 092 if ("uri".equals(codeString)) 093 return URI; 094 if ("iri-stem".equals(codeString)) 095 return IRISTEM; 096 if ("v2csmnemonic".equals(codeString)) 097 return V2CSMNEMONIC; 098 if ("other".equals(codeString)) 099 return OTHER; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case OID: return "oid"; 108 case UUID: return "uuid"; 109 case URI: return "uri"; 110 case IRISTEM: return "iri-stem"; 111 case V2CSMNEMONIC: return "v2csmnemonic"; 112 case OTHER: return "other"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getSystem() { 118 switch (this) { 119 case OID: return "http://hl7.org/fhir/namingsystem-identifier-type"; 120 case UUID: return "http://hl7.org/fhir/namingsystem-identifier-type"; 121 case URI: return "http://hl7.org/fhir/namingsystem-identifier-type"; 122 case IRISTEM: return "http://hl7.org/fhir/namingsystem-identifier-type"; 123 case V2CSMNEMONIC: return "http://hl7.org/fhir/namingsystem-identifier-type"; 124 case OTHER: return "http://hl7.org/fhir/namingsystem-identifier-type"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDefinition() { 130 switch (this) { 131 case OID: return "An ISO object identifier; e.g. 1.2.3.4.5."; 132 case UUID: return "A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11."; 133 case URI: return "A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org."; 134 case IRISTEM: return "An IRI string that can be prepended to the code to obtain a concept IRI for RDF applications. This should be a valid, absolute IRI as defined in RFC 3987. See rdf.html#iri-stem for details on how this value may be used."; 135 case V2CSMNEMONIC: return "A short string published by HL7 for use in the V2 family of standsrds to idenfify a code system in the V12 coded data types CWE, CNE, and CF. The code values are also published by HL7 at http://www.hl7.org/Special/committees/vocab/table_0396/index.cfm"; 136 case OTHER: return "Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC."; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getDisplay() { 142 switch (this) { 143 case OID: return "OID"; 144 case UUID: return "UUID"; 145 case URI: return "URI"; 146 case IRISTEM: return "IRI stem"; 147 case V2CSMNEMONIC: return "V2CSMNemonic"; 148 case OTHER: return "Other"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 } 154 155 public static class NamingSystemIdentifierTypeEnumFactory implements EnumFactory<NamingSystemIdentifierType> { 156 public NamingSystemIdentifierType fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("oid".equals(codeString)) 161 return NamingSystemIdentifierType.OID; 162 if ("uuid".equals(codeString)) 163 return NamingSystemIdentifierType.UUID; 164 if ("uri".equals(codeString)) 165 return NamingSystemIdentifierType.URI; 166 if ("iri-stem".equals(codeString)) 167 return NamingSystemIdentifierType.IRISTEM; 168 if ("v2csmnemonic".equals(codeString)) 169 return NamingSystemIdentifierType.V2CSMNEMONIC; 170 if ("other".equals(codeString)) 171 return NamingSystemIdentifierType.OTHER; 172 throw new IllegalArgumentException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 173 } 174 public Enumeration<NamingSystemIdentifierType> fromType(PrimitiveType<?> code) throws FHIRException { 175 if (code == null) 176 return null; 177 if (code.isEmpty()) 178 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 179 String codeString = ((PrimitiveType) code).asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 182 if ("oid".equals(codeString)) 183 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OID, code); 184 if ("uuid".equals(codeString)) 185 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.UUID, code); 186 if ("uri".equals(codeString)) 187 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.URI, code); 188 if ("iri-stem".equals(codeString)) 189 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.IRISTEM, code); 190 if ("v2csmnemonic".equals(codeString)) 191 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.V2CSMNEMONIC, code); 192 if ("other".equals(codeString)) 193 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OTHER, code); 194 throw new FHIRException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 195 } 196 public String toCode(NamingSystemIdentifierType code) { 197 if (code == NamingSystemIdentifierType.OID) 198 return "oid"; 199 if (code == NamingSystemIdentifierType.UUID) 200 return "uuid"; 201 if (code == NamingSystemIdentifierType.URI) 202 return "uri"; 203 if (code == NamingSystemIdentifierType.IRISTEM) 204 return "iri-stem"; 205 if (code == NamingSystemIdentifierType.V2CSMNEMONIC) 206 return "v2csmnemonic"; 207 if (code == NamingSystemIdentifierType.OTHER) 208 return "other"; 209 return "?"; 210 } 211 public String toSystem(NamingSystemIdentifierType code) { 212 return code.getSystem(); 213 } 214 } 215 216 public enum NamingSystemType { 217 /** 218 * The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc. 219 */ 220 CODESYSTEM, 221 /** 222 * The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.). 223 */ 224 IDENTIFIER, 225 /** 226 * The naming system is used as the root for other identifiers and naming systems. 227 */ 228 ROOT, 229 /** 230 * added to help the parsers with the generic types 231 */ 232 NULL; 233 public static NamingSystemType fromCode(String codeString) throws FHIRException { 234 if (codeString == null || "".equals(codeString)) 235 return null; 236 if ("codesystem".equals(codeString)) 237 return CODESYSTEM; 238 if ("identifier".equals(codeString)) 239 return IDENTIFIER; 240 if ("root".equals(codeString)) 241 return ROOT; 242 if (Configuration.isAcceptInvalidEnums()) 243 return null; 244 else 245 throw new FHIRException("Unknown NamingSystemType code '"+codeString+"'"); 246 } 247 public String toCode() { 248 switch (this) { 249 case CODESYSTEM: return "codesystem"; 250 case IDENTIFIER: return "identifier"; 251 case ROOT: return "root"; 252 case NULL: return null; 253 default: return "?"; 254 } 255 } 256 public String getSystem() { 257 switch (this) { 258 case CODESYSTEM: return "http://hl7.org/fhir/namingsystem-type"; 259 case IDENTIFIER: return "http://hl7.org/fhir/namingsystem-type"; 260 case ROOT: return "http://hl7.org/fhir/namingsystem-type"; 261 case NULL: return null; 262 default: return "?"; 263 } 264 } 265 public String getDefinition() { 266 switch (this) { 267 case CODESYSTEM: return "The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc."; 268 case IDENTIFIER: return "The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.)."; 269 case ROOT: return "The naming system is used as the root for other identifiers and naming systems."; 270 case NULL: return null; 271 default: return "?"; 272 } 273 } 274 public String getDisplay() { 275 switch (this) { 276 case CODESYSTEM: return "Code System"; 277 case IDENTIFIER: return "Identifier"; 278 case ROOT: return "Root"; 279 case NULL: return null; 280 default: return "?"; 281 } 282 } 283 } 284 285 public static class NamingSystemTypeEnumFactory implements EnumFactory<NamingSystemType> { 286 public NamingSystemType fromCode(String codeString) throws IllegalArgumentException { 287 if (codeString == null || "".equals(codeString)) 288 if (codeString == null || "".equals(codeString)) 289 return null; 290 if ("codesystem".equals(codeString)) 291 return NamingSystemType.CODESYSTEM; 292 if ("identifier".equals(codeString)) 293 return NamingSystemType.IDENTIFIER; 294 if ("root".equals(codeString)) 295 return NamingSystemType.ROOT; 296 throw new IllegalArgumentException("Unknown NamingSystemType code '"+codeString+"'"); 297 } 298 public Enumeration<NamingSystemType> fromType(PrimitiveType<?> code) throws FHIRException { 299 if (code == null) 300 return null; 301 if (code.isEmpty()) 302 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 303 String codeString = ((PrimitiveType) code).asStringValue(); 304 if (codeString == null || "".equals(codeString)) 305 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 306 if ("codesystem".equals(codeString)) 307 return new Enumeration<NamingSystemType>(this, NamingSystemType.CODESYSTEM, code); 308 if ("identifier".equals(codeString)) 309 return new Enumeration<NamingSystemType>(this, NamingSystemType.IDENTIFIER, code); 310 if ("root".equals(codeString)) 311 return new Enumeration<NamingSystemType>(this, NamingSystemType.ROOT, code); 312 throw new FHIRException("Unknown NamingSystemType code '"+codeString+"'"); 313 } 314 public String toCode(NamingSystemType code) { 315 if (code == NamingSystemType.NULL) 316 return null; 317 if (code == NamingSystemType.CODESYSTEM) 318 return "codesystem"; 319 if (code == NamingSystemType.IDENTIFIER) 320 return "identifier"; 321 if (code == NamingSystemType.ROOT) 322 return "root"; 323 return "?"; 324 } 325 public String toSystem(NamingSystemType code) { 326 return code.getSystem(); 327 } 328 } 329 330 @Block() 331 public static class NamingSystemUniqueIdComponent extends BackboneElement implements IBaseBackboneElement { 332 /** 333 * Identifies the unique identifier scheme used for this particular identifier. 334 */ 335 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 336 @Description(shortDefinition="oid | uuid | uri | iri-stem | v2csmnemonic | other", formalDefinition="Identifies the unique identifier scheme used for this particular identifier." ) 337 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-identifier-type") 338 protected Enumeration<NamingSystemIdentifierType> type; 339 340 /** 341 * The string that should be sent over the wire to identify the code system or identifier system. 342 */ 343 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 344 @Description(shortDefinition="The unique identifier", formalDefinition="The string that should be sent over the wire to identify the code system or identifier system." ) 345 protected StringType value; 346 347 /** 348 * Indicates whether this identifier is the "preferred" identifier of this type. 349 */ 350 @Child(name = "preferred", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 351 @Description(shortDefinition="Is this the id that should be used for this type", formalDefinition="Indicates whether this identifier is the \"preferred\" identifier of this type." ) 352 protected BooleanType preferred; 353 354 /** 355 * Notes about the past or intended usage of this identifier. 356 */ 357 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 358 @Description(shortDefinition="Notes about identifier usage", formalDefinition="Notes about the past or intended usage of this identifier." ) 359 protected StringType comment; 360 361 /** 362 * Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic. 363 */ 364 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=false) 365 @Description(shortDefinition="When is identifier valid?", formalDefinition="Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic." ) 366 protected Period period; 367 368 /** 369 * Indicates whether this identifier ie endorsed by the official owner of the associated naming system. 370 */ 371 @Child(name = "authoritative", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=false) 372 @Description(shortDefinition="Whether the identifier is authoritative", formalDefinition="Indicates whether this identifier ie endorsed by the official owner of the associated naming system." ) 373 protected BooleanType authoritative; 374 375 private static final long serialVersionUID = -166953751L; 376 377 /** 378 * Constructor 379 */ 380 public NamingSystemUniqueIdComponent() { 381 super(); 382 } 383 384 /** 385 * Constructor 386 */ 387 public NamingSystemUniqueIdComponent(NamingSystemIdentifierType type, String value) { 388 super(); 389 this.setType(type); 390 this.setValue(value); 391 } 392 393 /** 394 * @return {@link #type} (Identifies the unique identifier scheme used for this particular identifier.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 395 */ 396 public Enumeration<NamingSystemIdentifierType> getTypeElement() { 397 if (this.type == null) 398 if (Configuration.errorOnAutoCreate()) 399 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.type"); 400 else if (Configuration.doAutoCreate()) 401 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); // bb 402 return this.type; 403 } 404 405 public boolean hasTypeElement() { 406 return this.type != null && !this.type.isEmpty(); 407 } 408 409 public boolean hasType() { 410 return this.type != null && !this.type.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #type} (Identifies the unique identifier scheme used for this particular identifier.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 415 */ 416 public NamingSystemUniqueIdComponent setTypeElement(Enumeration<NamingSystemIdentifierType> value) { 417 this.type = value; 418 return this; 419 } 420 421 /** 422 * @return Identifies the unique identifier scheme used for this particular identifier. 423 */ 424 public NamingSystemIdentifierType getType() { 425 return this.type == null ? null : this.type.getValue(); 426 } 427 428 /** 429 * @param value Identifies the unique identifier scheme used for this particular identifier. 430 */ 431 public NamingSystemUniqueIdComponent setType(NamingSystemIdentifierType value) { 432 if (this.type == null) 433 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); 434 this.type.setValue(value); 435 return this; 436 } 437 438 /** 439 * @return {@link #value} (The string that should be sent over the wire to identify the code system or identifier system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 440 */ 441 public StringType getValueElement() { 442 if (this.value == null) 443 if (Configuration.errorOnAutoCreate()) 444 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.value"); 445 else if (Configuration.doAutoCreate()) 446 this.value = new StringType(); // bb 447 return this.value; 448 } 449 450 public boolean hasValueElement() { 451 return this.value != null && !this.value.isEmpty(); 452 } 453 454 public boolean hasValue() { 455 return this.value != null && !this.value.isEmpty(); 456 } 457 458 /** 459 * @param value {@link #value} (The string that should be sent over the wire to identify the code system or identifier system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 460 */ 461 public NamingSystemUniqueIdComponent setValueElement(StringType value) { 462 this.value = value; 463 return this; 464 } 465 466 /** 467 * @return The string that should be sent over the wire to identify the code system or identifier system. 468 */ 469 public String getValue() { 470 return this.value == null ? null : this.value.getValue(); 471 } 472 473 /** 474 * @param value The string that should be sent over the wire to identify the code system or identifier system. 475 */ 476 public NamingSystemUniqueIdComponent setValue(String value) { 477 if (this.value == null) 478 this.value = new StringType(); 479 this.value.setValue(value); 480 return this; 481 } 482 483 /** 484 * @return {@link #preferred} (Indicates whether this identifier is the "preferred" identifier of this type.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 485 */ 486 public BooleanType getPreferredElement() { 487 if (this.preferred == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.preferred"); 490 else if (Configuration.doAutoCreate()) 491 this.preferred = new BooleanType(); // bb 492 return this.preferred; 493 } 494 495 public boolean hasPreferredElement() { 496 return this.preferred != null && !this.preferred.isEmpty(); 497 } 498 499 public boolean hasPreferred() { 500 return this.preferred != null && !this.preferred.isEmpty(); 501 } 502 503 /** 504 * @param value {@link #preferred} (Indicates whether this identifier is the "preferred" identifier of this type.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 505 */ 506 public NamingSystemUniqueIdComponent setPreferredElement(BooleanType value) { 507 this.preferred = value; 508 return this; 509 } 510 511 /** 512 * @return Indicates whether this identifier is the "preferred" identifier of this type. 513 */ 514 public boolean getPreferred() { 515 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 516 } 517 518 /** 519 * @param value Indicates whether this identifier is the "preferred" identifier of this type. 520 */ 521 public NamingSystemUniqueIdComponent setPreferred(boolean value) { 522 if (this.preferred == null) 523 this.preferred = new BooleanType(); 524 this.preferred.setValue(value); 525 return this; 526 } 527 528 /** 529 * @return {@link #comment} (Notes about the past or intended usage of this identifier.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 530 */ 531 public StringType getCommentElement() { 532 if (this.comment == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.comment"); 535 else if (Configuration.doAutoCreate()) 536 this.comment = new StringType(); // bb 537 return this.comment; 538 } 539 540 public boolean hasCommentElement() { 541 return this.comment != null && !this.comment.isEmpty(); 542 } 543 544 public boolean hasComment() { 545 return this.comment != null && !this.comment.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #comment} (Notes about the past or intended usage of this identifier.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 550 */ 551 public NamingSystemUniqueIdComponent setCommentElement(StringType value) { 552 this.comment = value; 553 return this; 554 } 555 556 /** 557 * @return Notes about the past or intended usage of this identifier. 558 */ 559 public String getComment() { 560 return this.comment == null ? null : this.comment.getValue(); 561 } 562 563 /** 564 * @param value Notes about the past or intended usage of this identifier. 565 */ 566 public NamingSystemUniqueIdComponent setComment(String value) { 567 if (Utilities.noString(value)) 568 this.comment = null; 569 else { 570 if (this.comment == null) 571 this.comment = new StringType(); 572 this.comment.setValue(value); 573 } 574 return this; 575 } 576 577 /** 578 * @return {@link #period} (Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.) 579 */ 580 public Period getPeriod() { 581 if (this.period == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.period"); 584 else if (Configuration.doAutoCreate()) 585 this.period = new Period(); // cc 586 return this.period; 587 } 588 589 public boolean hasPeriod() { 590 return this.period != null && !this.period.isEmpty(); 591 } 592 593 /** 594 * @param value {@link #period} (Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.) 595 */ 596 public NamingSystemUniqueIdComponent setPeriod(Period value) { 597 this.period = value; 598 return this; 599 } 600 601 /** 602 * @return {@link #authoritative} (Indicates whether this identifier ie endorsed by the official owner of the associated naming system.). This is the underlying object with id, value and extensions. The accessor "getAuthoritative" gives direct access to the value 603 */ 604 public BooleanType getAuthoritativeElement() { 605 if (this.authoritative == null) 606 if (Configuration.errorOnAutoCreate()) 607 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.authoritative"); 608 else if (Configuration.doAutoCreate()) 609 this.authoritative = new BooleanType(); // bb 610 return this.authoritative; 611 } 612 613 public boolean hasAuthoritativeElement() { 614 return this.authoritative != null && !this.authoritative.isEmpty(); 615 } 616 617 public boolean hasAuthoritative() { 618 return this.authoritative != null && !this.authoritative.isEmpty(); 619 } 620 621 /** 622 * @param value {@link #authoritative} (Indicates whether this identifier ie endorsed by the official owner of the associated naming system.). This is the underlying object with id, value and extensions. The accessor "getAuthoritative" gives direct access to the value 623 */ 624 public NamingSystemUniqueIdComponent setAuthoritativeElement(BooleanType value) { 625 this.authoritative = value; 626 return this; 627 } 628 629 /** 630 * @return Indicates whether this identifier ie endorsed by the official owner of the associated naming system. 631 */ 632 public boolean getAuthoritative() { 633 return this.authoritative == null || this.authoritative.isEmpty() ? false : this.authoritative.getValue(); 634 } 635 636 /** 637 * @param value Indicates whether this identifier ie endorsed by the official owner of the associated naming system. 638 */ 639 public NamingSystemUniqueIdComponent setAuthoritative(boolean value) { 640 if (this.authoritative == null) 641 this.authoritative = new BooleanType(); 642 this.authoritative.setValue(value); 643 return this; 644 } 645 646 protected void listChildren(List<Property> children) { 647 super.listChildren(children); 648 children.add(new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type)); 649 children.add(new Property("value", "string", "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, value)); 650 children.add(new Property("preferred", "boolean", "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred)); 651 children.add(new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, comment)); 652 children.add(new Property("period", "Period", "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 0, 1, period)); 653 children.add(new Property("authoritative", "boolean", "Indicates whether this identifier ie endorsed by the official owner of the associated naming system.", 0, 1, authoritative)); 654 } 655 656 @Override 657 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 658 switch (_hash) { 659 case 3575610: /*type*/ return new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type); 660 case 111972721: /*value*/ return new Property("value", "string", "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, value); 661 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred); 662 case 950398559: /*comment*/ return new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, comment); 663 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 0, 1, period); 664 case -1557344881: /*authoritative*/ return new Property("authoritative", "boolean", "Indicates whether this identifier ie endorsed by the official owner of the associated naming system.", 0, 1, authoritative); 665 default: return super.getNamedProperty(_hash, _name, _checkValid); 666 } 667 668 } 669 670 @Override 671 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 672 switch (hash) { 673 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<NamingSystemIdentifierType> 674 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 675 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 676 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 677 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 678 case -1557344881: /*authoritative*/ return this.authoritative == null ? new Base[0] : new Base[] {this.authoritative}; // BooleanType 679 default: return super.getProperty(hash, name, checkValid); 680 } 681 682 } 683 684 @Override 685 public Base setProperty(int hash, String name, Base value) throws FHIRException { 686 switch (hash) { 687 case 3575610: // type 688 value = new NamingSystemIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 689 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 690 return value; 691 case 111972721: // value 692 this.value = TypeConvertor.castToString(value); // StringType 693 return value; 694 case -1294005119: // preferred 695 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 696 return value; 697 case 950398559: // comment 698 this.comment = TypeConvertor.castToString(value); // StringType 699 return value; 700 case -991726143: // period 701 this.period = TypeConvertor.castToPeriod(value); // Period 702 return value; 703 case -1557344881: // authoritative 704 this.authoritative = TypeConvertor.castToBoolean(value); // BooleanType 705 return value; 706 default: return super.setProperty(hash, name, value); 707 } 708 709 } 710 711 @Override 712 public Base setProperty(String name, Base value) throws FHIRException { 713 if (name.equals("type")) { 714 value = new NamingSystemIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 715 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 716 } else if (name.equals("value")) { 717 this.value = TypeConvertor.castToString(value); // StringType 718 } else if (name.equals("preferred")) { 719 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 720 } else if (name.equals("comment")) { 721 this.comment = TypeConvertor.castToString(value); // StringType 722 } else if (name.equals("period")) { 723 this.period = TypeConvertor.castToPeriod(value); // Period 724 } else if (name.equals("authoritative")) { 725 this.authoritative = TypeConvertor.castToBoolean(value); // BooleanType 726 } else 727 return super.setProperty(name, value); 728 return value; 729 } 730 731 @Override 732 public void removeChild(String name, Base value) throws FHIRException { 733 if (name.equals("type")) { 734 value = new NamingSystemIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 735 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 736 } else if (name.equals("value")) { 737 this.value = null; 738 } else if (name.equals("preferred")) { 739 this.preferred = null; 740 } else if (name.equals("comment")) { 741 this.comment = null; 742 } else if (name.equals("period")) { 743 this.period = null; 744 } else if (name.equals("authoritative")) { 745 this.authoritative = null; 746 } else 747 super.removeChild(name, value); 748 749 } 750 751 @Override 752 public Base makeProperty(int hash, String name) throws FHIRException { 753 switch (hash) { 754 case 3575610: return getTypeElement(); 755 case 111972721: return getValueElement(); 756 case -1294005119: return getPreferredElement(); 757 case 950398559: return getCommentElement(); 758 case -991726143: return getPeriod(); 759 case -1557344881: return getAuthoritativeElement(); 760 default: return super.makeProperty(hash, name); 761 } 762 763 } 764 765 @Override 766 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 767 switch (hash) { 768 case 3575610: /*type*/ return new String[] {"code"}; 769 case 111972721: /*value*/ return new String[] {"string"}; 770 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 771 case 950398559: /*comment*/ return new String[] {"string"}; 772 case -991726143: /*period*/ return new String[] {"Period"}; 773 case -1557344881: /*authoritative*/ return new String[] {"boolean"}; 774 default: return super.getTypesForProperty(hash, name); 775 } 776 777 } 778 779 @Override 780 public Base addChild(String name) throws FHIRException { 781 if (name.equals("type")) { 782 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.type"); 783 } 784 else if (name.equals("value")) { 785 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.value"); 786 } 787 else if (name.equals("preferred")) { 788 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.preferred"); 789 } 790 else if (name.equals("comment")) { 791 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.comment"); 792 } 793 else if (name.equals("period")) { 794 this.period = new Period(); 795 return this.period; 796 } 797 else if (name.equals("authoritative")) { 798 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.authoritative"); 799 } 800 else 801 return super.addChild(name); 802 } 803 804 public NamingSystemUniqueIdComponent copy() { 805 NamingSystemUniqueIdComponent dst = new NamingSystemUniqueIdComponent(); 806 copyValues(dst); 807 return dst; 808 } 809 810 public void copyValues(NamingSystemUniqueIdComponent dst) { 811 super.copyValues(dst); 812 dst.type = type == null ? null : type.copy(); 813 dst.value = value == null ? null : value.copy(); 814 dst.preferred = preferred == null ? null : preferred.copy(); 815 dst.comment = comment == null ? null : comment.copy(); 816 dst.period = period == null ? null : period.copy(); 817 dst.authoritative = authoritative == null ? null : authoritative.copy(); 818 } 819 820 @Override 821 public boolean equalsDeep(Base other_) { 822 if (!super.equalsDeep(other_)) 823 return false; 824 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 825 return false; 826 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 827 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(preferred, o.preferred, true) 828 && compareDeep(comment, o.comment, true) && compareDeep(period, o.period, true) && compareDeep(authoritative, o.authoritative, true) 829 ; 830 } 831 832 @Override 833 public boolean equalsShallow(Base other_) { 834 if (!super.equalsShallow(other_)) 835 return false; 836 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 837 return false; 838 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 839 return compareValues(type, o.type, true) && compareValues(value, o.value, true) && compareValues(preferred, o.preferred, true) 840 && compareValues(comment, o.comment, true) && compareValues(authoritative, o.authoritative, true); 841 } 842 843 public boolean isEmpty() { 844 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, preferred, comment 845 , period, authoritative); 846 } 847 848 public String fhirType() { 849 return "NamingSystem.uniqueId"; 850 851 } 852 853 } 854 855 /** 856 * An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers. 857 */ 858 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 859 @Description(shortDefinition="Canonical identifier for this naming system, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers." ) 860 protected UriType url; 861 862 /** 863 * A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance. 864 */ 865 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 866 @Description(shortDefinition="Additional identifier for the naming system (business identifier)", formalDefinition="A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 867 protected List<Identifier> identifier; 868 869 /** 870 * The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 871 */ 872 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 873 @Description(shortDefinition="Business version of the naming system", formalDefinition="The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 874 protected StringType version; 875 876 /** 877 * Indicates the mechanism used to compare versions to determine which NamingSystem is more current. 878 */ 879 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 880 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which NamingSystem is more current." ) 881 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 882 protected DataType versionAlgorithm; 883 884 /** 885 * A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 886 */ 887 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 888 @Description(shortDefinition="Name for this naming system (computer friendly)", formalDefinition="A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 889 protected StringType name; 890 891 /** 892 * A short, descriptive, user-friendly title for the naming system. 893 */ 894 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 895 @Description(shortDefinition="Title for this naming system (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the naming system." ) 896 protected StringType title; 897 898 /** 899 * The status of this naming system. Enables tracking the life-cycle of the content. 900 */ 901 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 902 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this naming system. Enables tracking the life-cycle of the content." ) 903 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 904 protected Enumeration<PublicationStatus> status; 905 906 /** 907 * Indicates the purpose for the naming system - what kinds of things does it make unique? 908 */ 909 @Child(name = "kind", type = {CodeType.class}, order=7, min=1, max=1, modifier=false, summary=true) 910 @Description(shortDefinition="codesystem | identifier | root", formalDefinition="Indicates the purpose for the naming system - what kinds of things does it make unique?" ) 911 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-type") 912 protected Enumeration<NamingSystemType> kind; 913 914 /** 915 * A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 916 */ 917 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 918 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 919 protected BooleanType experimental; 920 921 /** 922 * The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 923 */ 924 @Child(name = "date", type = {DateTimeType.class}, order=9, min=1, max=1, modifier=false, summary=true) 925 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes." ) 926 protected DateTimeType date; 927 928 /** 929 * The name of the organization or individual responsible for the release and ongoing maintenance of the naming system. 930 */ 931 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 932 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the naming system." ) 933 protected StringType publisher; 934 935 /** 936 * Contact details to assist a user in finding and communicating with the publisher. 937 */ 938 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 939 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 940 protected List<ContactDetail> contact; 941 942 /** 943 * The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 944 */ 945 @Child(name = "responsible", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 946 @Description(shortDefinition="Who maintains system namespace?", formalDefinition="The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision." ) 947 protected StringType responsible; 948 949 /** 950 * Categorizes a naming system for easier search by grouping related naming systems. 951 */ 952 @Child(name = "type", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 953 @Description(shortDefinition="e.g. driver, provider, patient, bank etc", formalDefinition="Categorizes a naming system for easier search by grouping related naming systems." ) 954 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-identifier-system-type") 955 protected CodeableConcept type; 956 957 /** 958 * A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 959 */ 960 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 961 @Description(shortDefinition="Natural language description of the naming system", formalDefinition="A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc." ) 962 protected MarkdownType description; 963 964 /** 965 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances. 966 */ 967 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 968 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances." ) 969 protected List<UsageContext> useContext; 970 971 /** 972 * A legal or geographic region in which the naming system is intended to be used. 973 */ 974 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 975 @Description(shortDefinition="Intended jurisdiction for naming system (if applicable)", formalDefinition="A legal or geographic region in which the naming system is intended to be used." ) 976 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 977 protected List<CodeableConcept> jurisdiction; 978 979 /** 980 * Explanation of why this naming system is needed and why it has been designed as it has. 981 */ 982 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 983 @Description(shortDefinition="Why this naming system is defined", formalDefinition="Explanation of why this naming system is needed and why it has been designed as it has." ) 984 protected MarkdownType purpose; 985 986 /** 987 * A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system. 988 */ 989 @Child(name = "copyright", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 990 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system." ) 991 protected MarkdownType copyright; 992 993 /** 994 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 995 */ 996 @Child(name = "copyrightLabel", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 997 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 998 protected StringType copyrightLabel; 999 1000 /** 1001 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1002 */ 1003 @Child(name = "approvalDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=false) 1004 @Description(shortDefinition="When the NamingSystem was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 1005 protected DateType approvalDate; 1006 1007 /** 1008 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1009 */ 1010 @Child(name = "lastReviewDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1011 @Description(shortDefinition="When the NamingSystem was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 1012 protected DateType lastReviewDate; 1013 1014 /** 1015 * The period during which the NamingSystem content was or is planned to be in active use. 1016 */ 1017 @Child(name = "effectivePeriod", type = {Period.class}, order=22, min=0, max=1, modifier=false, summary=true) 1018 @Description(shortDefinition="When the NamingSystem is expected to be used", formalDefinition="The period during which the NamingSystem content was or is planned to be in active use." ) 1019 protected Period effectivePeriod; 1020 1021 /** 1022 * Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching. 1023 */ 1024 @Child(name = "topic", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1025 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching." ) 1026 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 1027 protected List<CodeableConcept> topic; 1028 1029 /** 1030 * An individiual or organization primarily involved in the creation and maintenance of the NamingSystem. 1031 */ 1032 @Child(name = "author", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1033 @Description(shortDefinition="Who authored the CodeSystem", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the NamingSystem." ) 1034 protected List<ContactDetail> author; 1035 1036 /** 1037 * An individual or organization primarily responsible for internal coherence of the NamingSystem. 1038 */ 1039 @Child(name = "editor", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1040 @Description(shortDefinition="Who edited the NamingSystem", formalDefinition="An individual or organization primarily responsible for internal coherence of the NamingSystem." ) 1041 protected List<ContactDetail> editor; 1042 1043 /** 1044 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem. 1045 */ 1046 @Child(name = "reviewer", type = {ContactDetail.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1047 @Description(shortDefinition="Who reviewed the NamingSystem", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem." ) 1048 protected List<ContactDetail> reviewer; 1049 1050 /** 1051 * An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting. 1052 */ 1053 @Child(name = "endorser", type = {ContactDetail.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1054 @Description(shortDefinition="Who endorsed the NamingSystem", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting." ) 1055 protected List<ContactDetail> endorser; 1056 1057 /** 1058 * Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts. 1059 */ 1060 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1061 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts." ) 1062 protected List<RelatedArtifact> relatedArtifact; 1063 1064 /** 1065 * Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 1066 */ 1067 @Child(name = "usage", type = {StringType.class}, order=29, min=0, max=1, modifier=false, summary=false) 1068 @Description(shortDefinition="How/where is it used", formalDefinition="Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc." ) 1069 protected StringType usage; 1070 1071 /** 1072 * Indicates how the system may be identified when referenced in electronic exchange. 1073 */ 1074 @Child(name = "uniqueId", type = {}, order=30, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1075 @Description(shortDefinition="Unique identifiers used for system", formalDefinition="Indicates how the system may be identified when referenced in electronic exchange." ) 1076 protected List<NamingSystemUniqueIdComponent> uniqueId; 1077 1078 private static final long serialVersionUID = -1403308569L; 1079 1080 /** 1081 * Constructor 1082 */ 1083 public NamingSystem() { 1084 super(); 1085 } 1086 1087 /** 1088 * Constructor 1089 */ 1090 public NamingSystem(String name, PublicationStatus status, NamingSystemType kind, Date date, NamingSystemUniqueIdComponent uniqueId) { 1091 super(); 1092 this.setName(name); 1093 this.setStatus(status); 1094 this.setKind(kind); 1095 this.setDate(date); 1096 this.addUniqueId(uniqueId); 1097 } 1098 1099 /** 1100 * @return {@link #url} (An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1101 */ 1102 public UriType getUrlElement() { 1103 if (this.url == null) 1104 if (Configuration.errorOnAutoCreate()) 1105 throw new Error("Attempt to auto-create NamingSystem.url"); 1106 else if (Configuration.doAutoCreate()) 1107 this.url = new UriType(); // bb 1108 return this.url; 1109 } 1110 1111 public boolean hasUrlElement() { 1112 return this.url != null && !this.url.isEmpty(); 1113 } 1114 1115 public boolean hasUrl() { 1116 return this.url != null && !this.url.isEmpty(); 1117 } 1118 1119 /** 1120 * @param value {@link #url} (An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1121 */ 1122 public NamingSystem setUrlElement(UriType value) { 1123 this.url = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers. 1129 */ 1130 public String getUrl() { 1131 return this.url == null ? null : this.url.getValue(); 1132 } 1133 1134 /** 1135 * @param value An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers. 1136 */ 1137 public NamingSystem setUrl(String value) { 1138 if (Utilities.noString(value)) 1139 this.url = null; 1140 else { 1141 if (this.url == null) 1142 this.url = new UriType(); 1143 this.url.setValue(value); 1144 } 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #identifier} (A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1150 */ 1151 public List<Identifier> getIdentifier() { 1152 if (this.identifier == null) 1153 this.identifier = new ArrayList<Identifier>(); 1154 return this.identifier; 1155 } 1156 1157 /** 1158 * @return Returns a reference to <code>this</code> for easy method chaining 1159 */ 1160 public NamingSystem setIdentifier(List<Identifier> theIdentifier) { 1161 this.identifier = theIdentifier; 1162 return this; 1163 } 1164 1165 public boolean hasIdentifier() { 1166 if (this.identifier == null) 1167 return false; 1168 for (Identifier item : this.identifier) 1169 if (!item.isEmpty()) 1170 return true; 1171 return false; 1172 } 1173 1174 public Identifier addIdentifier() { //3 1175 Identifier t = new Identifier(); 1176 if (this.identifier == null) 1177 this.identifier = new ArrayList<Identifier>(); 1178 this.identifier.add(t); 1179 return t; 1180 } 1181 1182 public NamingSystem addIdentifier(Identifier t) { //3 1183 if (t == null) 1184 return this; 1185 if (this.identifier == null) 1186 this.identifier = new ArrayList<Identifier>(); 1187 this.identifier.add(t); 1188 return this; 1189 } 1190 1191 /** 1192 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1193 */ 1194 public Identifier getIdentifierFirstRep() { 1195 if (getIdentifier().isEmpty()) { 1196 addIdentifier(); 1197 } 1198 return getIdentifier().get(0); 1199 } 1200 1201 /** 1202 * @return {@link #version} (The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1203 */ 1204 public StringType getVersionElement() { 1205 if (this.version == null) 1206 if (Configuration.errorOnAutoCreate()) 1207 throw new Error("Attempt to auto-create NamingSystem.version"); 1208 else if (Configuration.doAutoCreate()) 1209 this.version = new StringType(); // bb 1210 return this.version; 1211 } 1212 1213 public boolean hasVersionElement() { 1214 return this.version != null && !this.version.isEmpty(); 1215 } 1216 1217 public boolean hasVersion() { 1218 return this.version != null && !this.version.isEmpty(); 1219 } 1220 1221 /** 1222 * @param value {@link #version} (The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1223 */ 1224 public NamingSystem setVersionElement(StringType value) { 1225 this.version = value; 1226 return this; 1227 } 1228 1229 /** 1230 * @return The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1231 */ 1232 public String getVersion() { 1233 return this.version == null ? null : this.version.getValue(); 1234 } 1235 1236 /** 1237 * @param value The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1238 */ 1239 public NamingSystem setVersion(String value) { 1240 if (Utilities.noString(value)) 1241 this.version = null; 1242 else { 1243 if (this.version == null) 1244 this.version = new StringType(); 1245 this.version.setValue(value); 1246 } 1247 return this; 1248 } 1249 1250 /** 1251 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1252 */ 1253 public DataType getVersionAlgorithm() { 1254 return this.versionAlgorithm; 1255 } 1256 1257 /** 1258 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1259 */ 1260 public StringType getVersionAlgorithmStringType() throws FHIRException { 1261 if (this.versionAlgorithm == null) 1262 this.versionAlgorithm = new StringType(); 1263 if (!(this.versionAlgorithm instanceof StringType)) 1264 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1265 return (StringType) this.versionAlgorithm; 1266 } 1267 1268 public boolean hasVersionAlgorithmStringType() { 1269 return this != null && this.versionAlgorithm instanceof StringType; 1270 } 1271 1272 /** 1273 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1274 */ 1275 public Coding getVersionAlgorithmCoding() throws FHIRException { 1276 if (this.versionAlgorithm == null) 1277 this.versionAlgorithm = new Coding(); 1278 if (!(this.versionAlgorithm instanceof Coding)) 1279 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1280 return (Coding) this.versionAlgorithm; 1281 } 1282 1283 public boolean hasVersionAlgorithmCoding() { 1284 return this != null && this.versionAlgorithm instanceof Coding; 1285 } 1286 1287 public boolean hasVersionAlgorithm() { 1288 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1289 } 1290 1291 /** 1292 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1293 */ 1294 public NamingSystem setVersionAlgorithm(DataType value) { 1295 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1296 throw new FHIRException("Not the right type for NamingSystem.versionAlgorithm[x]: "+value.fhirType()); 1297 this.versionAlgorithm = value; 1298 return this; 1299 } 1300 1301 /** 1302 * @return {@link #name} (A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1303 */ 1304 public StringType getNameElement() { 1305 if (this.name == null) 1306 if (Configuration.errorOnAutoCreate()) 1307 throw new Error("Attempt to auto-create NamingSystem.name"); 1308 else if (Configuration.doAutoCreate()) 1309 this.name = new StringType(); // bb 1310 return this.name; 1311 } 1312 1313 public boolean hasNameElement() { 1314 return this.name != null && !this.name.isEmpty(); 1315 } 1316 1317 public boolean hasName() { 1318 return this.name != null && !this.name.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #name} (A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1323 */ 1324 public NamingSystem setNameElement(StringType value) { 1325 this.name = value; 1326 return this; 1327 } 1328 1329 /** 1330 * @return A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1331 */ 1332 public String getName() { 1333 return this.name == null ? null : this.name.getValue(); 1334 } 1335 1336 /** 1337 * @param value A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1338 */ 1339 public NamingSystem setName(String value) { 1340 if (this.name == null) 1341 this.name = new StringType(); 1342 this.name.setValue(value); 1343 return this; 1344 } 1345 1346 /** 1347 * @return {@link #title} (A short, descriptive, user-friendly title for the naming system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1348 */ 1349 public StringType getTitleElement() { 1350 if (this.title == null) 1351 if (Configuration.errorOnAutoCreate()) 1352 throw new Error("Attempt to auto-create NamingSystem.title"); 1353 else if (Configuration.doAutoCreate()) 1354 this.title = new StringType(); // bb 1355 return this.title; 1356 } 1357 1358 public boolean hasTitleElement() { 1359 return this.title != null && !this.title.isEmpty(); 1360 } 1361 1362 public boolean hasTitle() { 1363 return this.title != null && !this.title.isEmpty(); 1364 } 1365 1366 /** 1367 * @param value {@link #title} (A short, descriptive, user-friendly title for the naming system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1368 */ 1369 public NamingSystem setTitleElement(StringType value) { 1370 this.title = value; 1371 return this; 1372 } 1373 1374 /** 1375 * @return A short, descriptive, user-friendly title for the naming system. 1376 */ 1377 public String getTitle() { 1378 return this.title == null ? null : this.title.getValue(); 1379 } 1380 1381 /** 1382 * @param value A short, descriptive, user-friendly title for the naming system. 1383 */ 1384 public NamingSystem setTitle(String value) { 1385 if (Utilities.noString(value)) 1386 this.title = null; 1387 else { 1388 if (this.title == null) 1389 this.title = new StringType(); 1390 this.title.setValue(value); 1391 } 1392 return this; 1393 } 1394 1395 /** 1396 * @return {@link #status} (The status of this naming system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1397 */ 1398 public Enumeration<PublicationStatus> getStatusElement() { 1399 if (this.status == null) 1400 if (Configuration.errorOnAutoCreate()) 1401 throw new Error("Attempt to auto-create NamingSystem.status"); 1402 else if (Configuration.doAutoCreate()) 1403 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1404 return this.status; 1405 } 1406 1407 public boolean hasStatusElement() { 1408 return this.status != null && !this.status.isEmpty(); 1409 } 1410 1411 public boolean hasStatus() { 1412 return this.status != null && !this.status.isEmpty(); 1413 } 1414 1415 /** 1416 * @param value {@link #status} (The status of this naming system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1417 */ 1418 public NamingSystem setStatusElement(Enumeration<PublicationStatus> value) { 1419 this.status = value; 1420 return this; 1421 } 1422 1423 /** 1424 * @return The status of this naming system. Enables tracking the life-cycle of the content. 1425 */ 1426 public PublicationStatus getStatus() { 1427 return this.status == null ? null : this.status.getValue(); 1428 } 1429 1430 /** 1431 * @param value The status of this naming system. Enables tracking the life-cycle of the content. 1432 */ 1433 public NamingSystem setStatus(PublicationStatus value) { 1434 if (this.status == null) 1435 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1436 this.status.setValue(value); 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #kind} (Indicates the purpose for the naming system - what kinds of things does it make unique?). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1442 */ 1443 public Enumeration<NamingSystemType> getKindElement() { 1444 if (this.kind == null) 1445 if (Configuration.errorOnAutoCreate()) 1446 throw new Error("Attempt to auto-create NamingSystem.kind"); 1447 else if (Configuration.doAutoCreate()) 1448 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); // bb 1449 return this.kind; 1450 } 1451 1452 public boolean hasKindElement() { 1453 return this.kind != null && !this.kind.isEmpty(); 1454 } 1455 1456 public boolean hasKind() { 1457 return this.kind != null && !this.kind.isEmpty(); 1458 } 1459 1460 /** 1461 * @param value {@link #kind} (Indicates the purpose for the naming system - what kinds of things does it make unique?). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1462 */ 1463 public NamingSystem setKindElement(Enumeration<NamingSystemType> value) { 1464 this.kind = value; 1465 return this; 1466 } 1467 1468 /** 1469 * @return Indicates the purpose for the naming system - what kinds of things does it make unique? 1470 */ 1471 public NamingSystemType getKind() { 1472 return this.kind == null ? null : this.kind.getValue(); 1473 } 1474 1475 /** 1476 * @param value Indicates the purpose for the naming system - what kinds of things does it make unique? 1477 */ 1478 public NamingSystem setKind(NamingSystemType value) { 1479 if (this.kind == null) 1480 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); 1481 this.kind.setValue(value); 1482 return this; 1483 } 1484 1485 /** 1486 * @return {@link #experimental} (A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1487 */ 1488 public BooleanType getExperimentalElement() { 1489 if (this.experimental == null) 1490 if (Configuration.errorOnAutoCreate()) 1491 throw new Error("Attempt to auto-create NamingSystem.experimental"); 1492 else if (Configuration.doAutoCreate()) 1493 this.experimental = new BooleanType(); // bb 1494 return this.experimental; 1495 } 1496 1497 public boolean hasExperimentalElement() { 1498 return this.experimental != null && !this.experimental.isEmpty(); 1499 } 1500 1501 public boolean hasExperimental() { 1502 return this.experimental != null && !this.experimental.isEmpty(); 1503 } 1504 1505 /** 1506 * @param value {@link #experimental} (A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1507 */ 1508 public NamingSystem setExperimentalElement(BooleanType value) { 1509 this.experimental = value; 1510 return this; 1511 } 1512 1513 /** 1514 * @return A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1515 */ 1516 public boolean getExperimental() { 1517 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1518 } 1519 1520 /** 1521 * @param value A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1522 */ 1523 public NamingSystem setExperimental(boolean value) { 1524 if (this.experimental == null) 1525 this.experimental = new BooleanType(); 1526 this.experimental.setValue(value); 1527 return this; 1528 } 1529 1530 /** 1531 * @return {@link #date} (The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1532 */ 1533 public DateTimeType getDateElement() { 1534 if (this.date == null) 1535 if (Configuration.errorOnAutoCreate()) 1536 throw new Error("Attempt to auto-create NamingSystem.date"); 1537 else if (Configuration.doAutoCreate()) 1538 this.date = new DateTimeType(); // bb 1539 return this.date; 1540 } 1541 1542 public boolean hasDateElement() { 1543 return this.date != null && !this.date.isEmpty(); 1544 } 1545 1546 public boolean hasDate() { 1547 return this.date != null && !this.date.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #date} (The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1552 */ 1553 public NamingSystem setDateElement(DateTimeType value) { 1554 this.date = value; 1555 return this; 1556 } 1557 1558 /** 1559 * @return The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 1560 */ 1561 public Date getDate() { 1562 return this.date == null ? null : this.date.getValue(); 1563 } 1564 1565 /** 1566 * @param value The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 1567 */ 1568 public NamingSystem setDate(Date value) { 1569 if (this.date == null) 1570 this.date = new DateTimeType(); 1571 this.date.setValue(value); 1572 return this; 1573 } 1574 1575 /** 1576 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1577 */ 1578 public StringType getPublisherElement() { 1579 if (this.publisher == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create NamingSystem.publisher"); 1582 else if (Configuration.doAutoCreate()) 1583 this.publisher = new StringType(); // bb 1584 return this.publisher; 1585 } 1586 1587 public boolean hasPublisherElement() { 1588 return this.publisher != null && !this.publisher.isEmpty(); 1589 } 1590 1591 public boolean hasPublisher() { 1592 return this.publisher != null && !this.publisher.isEmpty(); 1593 } 1594 1595 /** 1596 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1597 */ 1598 public NamingSystem setPublisherElement(StringType value) { 1599 this.publisher = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the naming system. 1605 */ 1606 public String getPublisher() { 1607 return this.publisher == null ? null : this.publisher.getValue(); 1608 } 1609 1610 /** 1611 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the naming system. 1612 */ 1613 public NamingSystem setPublisher(String value) { 1614 if (Utilities.noString(value)) 1615 this.publisher = null; 1616 else { 1617 if (this.publisher == null) 1618 this.publisher = new StringType(); 1619 this.publisher.setValue(value); 1620 } 1621 return this; 1622 } 1623 1624 /** 1625 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1626 */ 1627 public List<ContactDetail> getContact() { 1628 if (this.contact == null) 1629 this.contact = new ArrayList<ContactDetail>(); 1630 return this.contact; 1631 } 1632 1633 /** 1634 * @return Returns a reference to <code>this</code> for easy method chaining 1635 */ 1636 public NamingSystem setContact(List<ContactDetail> theContact) { 1637 this.contact = theContact; 1638 return this; 1639 } 1640 1641 public boolean hasContact() { 1642 if (this.contact == null) 1643 return false; 1644 for (ContactDetail item : this.contact) 1645 if (!item.isEmpty()) 1646 return true; 1647 return false; 1648 } 1649 1650 public ContactDetail addContact() { //3 1651 ContactDetail t = new ContactDetail(); 1652 if (this.contact == null) 1653 this.contact = new ArrayList<ContactDetail>(); 1654 this.contact.add(t); 1655 return t; 1656 } 1657 1658 public NamingSystem addContact(ContactDetail t) { //3 1659 if (t == null) 1660 return this; 1661 if (this.contact == null) 1662 this.contact = new ArrayList<ContactDetail>(); 1663 this.contact.add(t); 1664 return this; 1665 } 1666 1667 /** 1668 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1669 */ 1670 public ContactDetail getContactFirstRep() { 1671 if (getContact().isEmpty()) { 1672 addContact(); 1673 } 1674 return getContact().get(0); 1675 } 1676 1677 /** 1678 * @return {@link #responsible} (The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1679 */ 1680 public StringType getResponsibleElement() { 1681 if (this.responsible == null) 1682 if (Configuration.errorOnAutoCreate()) 1683 throw new Error("Attempt to auto-create NamingSystem.responsible"); 1684 else if (Configuration.doAutoCreate()) 1685 this.responsible = new StringType(); // bb 1686 return this.responsible; 1687 } 1688 1689 public boolean hasResponsibleElement() { 1690 return this.responsible != null && !this.responsible.isEmpty(); 1691 } 1692 1693 public boolean hasResponsible() { 1694 return this.responsible != null && !this.responsible.isEmpty(); 1695 } 1696 1697 /** 1698 * @param value {@link #responsible} (The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1699 */ 1700 public NamingSystem setResponsibleElement(StringType value) { 1701 this.responsible = value; 1702 return this; 1703 } 1704 1705 /** 1706 * @return The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 1707 */ 1708 public String getResponsible() { 1709 return this.responsible == null ? null : this.responsible.getValue(); 1710 } 1711 1712 /** 1713 * @param value The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 1714 */ 1715 public NamingSystem setResponsible(String value) { 1716 if (Utilities.noString(value)) 1717 this.responsible = null; 1718 else { 1719 if (this.responsible == null) 1720 this.responsible = new StringType(); 1721 this.responsible.setValue(value); 1722 } 1723 return this; 1724 } 1725 1726 /** 1727 * @return {@link #type} (Categorizes a naming system for easier search by grouping related naming systems.) 1728 */ 1729 public CodeableConcept getType() { 1730 if (this.type == null) 1731 if (Configuration.errorOnAutoCreate()) 1732 throw new Error("Attempt to auto-create NamingSystem.type"); 1733 else if (Configuration.doAutoCreate()) 1734 this.type = new CodeableConcept(); // cc 1735 return this.type; 1736 } 1737 1738 public boolean hasType() { 1739 return this.type != null && !this.type.isEmpty(); 1740 } 1741 1742 /** 1743 * @param value {@link #type} (Categorizes a naming system for easier search by grouping related naming systems.) 1744 */ 1745 public NamingSystem setType(CodeableConcept value) { 1746 this.type = value; 1747 return this; 1748 } 1749 1750 /** 1751 * @return {@link #description} (A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1752 */ 1753 public MarkdownType getDescriptionElement() { 1754 if (this.description == null) 1755 if (Configuration.errorOnAutoCreate()) 1756 throw new Error("Attempt to auto-create NamingSystem.description"); 1757 else if (Configuration.doAutoCreate()) 1758 this.description = new MarkdownType(); // bb 1759 return this.description; 1760 } 1761 1762 public boolean hasDescriptionElement() { 1763 return this.description != null && !this.description.isEmpty(); 1764 } 1765 1766 public boolean hasDescription() { 1767 return this.description != null && !this.description.isEmpty(); 1768 } 1769 1770 /** 1771 * @param value {@link #description} (A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1772 */ 1773 public NamingSystem setDescriptionElement(MarkdownType value) { 1774 this.description = value; 1775 return this; 1776 } 1777 1778 /** 1779 * @return A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 1780 */ 1781 public String getDescription() { 1782 return this.description == null ? null : this.description.getValue(); 1783 } 1784 1785 /** 1786 * @param value A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 1787 */ 1788 public NamingSystem setDescription(String value) { 1789 if (Utilities.noString(value)) 1790 this.description = null; 1791 else { 1792 if (this.description == null) 1793 this.description = new MarkdownType(); 1794 this.description.setValue(value); 1795 } 1796 return this; 1797 } 1798 1799 /** 1800 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.) 1801 */ 1802 public List<UsageContext> getUseContext() { 1803 if (this.useContext == null) 1804 this.useContext = new ArrayList<UsageContext>(); 1805 return this.useContext; 1806 } 1807 1808 /** 1809 * @return Returns a reference to <code>this</code> for easy method chaining 1810 */ 1811 public NamingSystem setUseContext(List<UsageContext> theUseContext) { 1812 this.useContext = theUseContext; 1813 return this; 1814 } 1815 1816 public boolean hasUseContext() { 1817 if (this.useContext == null) 1818 return false; 1819 for (UsageContext item : this.useContext) 1820 if (!item.isEmpty()) 1821 return true; 1822 return false; 1823 } 1824 1825 public UsageContext addUseContext() { //3 1826 UsageContext t = new UsageContext(); 1827 if (this.useContext == null) 1828 this.useContext = new ArrayList<UsageContext>(); 1829 this.useContext.add(t); 1830 return t; 1831 } 1832 1833 public NamingSystem addUseContext(UsageContext t) { //3 1834 if (t == null) 1835 return this; 1836 if (this.useContext == null) 1837 this.useContext = new ArrayList<UsageContext>(); 1838 this.useContext.add(t); 1839 return this; 1840 } 1841 1842 /** 1843 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1844 */ 1845 public UsageContext getUseContextFirstRep() { 1846 if (getUseContext().isEmpty()) { 1847 addUseContext(); 1848 } 1849 return getUseContext().get(0); 1850 } 1851 1852 /** 1853 * @return {@link #jurisdiction} (A legal or geographic region in which the naming system is intended to be used.) 1854 */ 1855 public List<CodeableConcept> getJurisdiction() { 1856 if (this.jurisdiction == null) 1857 this.jurisdiction = new ArrayList<CodeableConcept>(); 1858 return this.jurisdiction; 1859 } 1860 1861 /** 1862 * @return Returns a reference to <code>this</code> for easy method chaining 1863 */ 1864 public NamingSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 1865 this.jurisdiction = theJurisdiction; 1866 return this; 1867 } 1868 1869 public boolean hasJurisdiction() { 1870 if (this.jurisdiction == null) 1871 return false; 1872 for (CodeableConcept item : this.jurisdiction) 1873 if (!item.isEmpty()) 1874 return true; 1875 return false; 1876 } 1877 1878 public CodeableConcept addJurisdiction() { //3 1879 CodeableConcept t = new CodeableConcept(); 1880 if (this.jurisdiction == null) 1881 this.jurisdiction = new ArrayList<CodeableConcept>(); 1882 this.jurisdiction.add(t); 1883 return t; 1884 } 1885 1886 public NamingSystem addJurisdiction(CodeableConcept t) { //3 1887 if (t == null) 1888 return this; 1889 if (this.jurisdiction == null) 1890 this.jurisdiction = new ArrayList<CodeableConcept>(); 1891 this.jurisdiction.add(t); 1892 return this; 1893 } 1894 1895 /** 1896 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1897 */ 1898 public CodeableConcept getJurisdictionFirstRep() { 1899 if (getJurisdiction().isEmpty()) { 1900 addJurisdiction(); 1901 } 1902 return getJurisdiction().get(0); 1903 } 1904 1905 /** 1906 * @return {@link #purpose} (Explanation of why this naming system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1907 */ 1908 public MarkdownType getPurposeElement() { 1909 if (this.purpose == null) 1910 if (Configuration.errorOnAutoCreate()) 1911 throw new Error("Attempt to auto-create NamingSystem.purpose"); 1912 else if (Configuration.doAutoCreate()) 1913 this.purpose = new MarkdownType(); // bb 1914 return this.purpose; 1915 } 1916 1917 public boolean hasPurposeElement() { 1918 return this.purpose != null && !this.purpose.isEmpty(); 1919 } 1920 1921 public boolean hasPurpose() { 1922 return this.purpose != null && !this.purpose.isEmpty(); 1923 } 1924 1925 /** 1926 * @param value {@link #purpose} (Explanation of why this naming system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1927 */ 1928 public NamingSystem setPurposeElement(MarkdownType value) { 1929 this.purpose = value; 1930 return this; 1931 } 1932 1933 /** 1934 * @return Explanation of why this naming system is needed and why it has been designed as it has. 1935 */ 1936 public String getPurpose() { 1937 return this.purpose == null ? null : this.purpose.getValue(); 1938 } 1939 1940 /** 1941 * @param value Explanation of why this naming system is needed and why it has been designed as it has. 1942 */ 1943 public NamingSystem setPurpose(String value) { 1944 if (Utilities.noString(value)) 1945 this.purpose = null; 1946 else { 1947 if (this.purpose == null) 1948 this.purpose = new MarkdownType(); 1949 this.purpose.setValue(value); 1950 } 1951 return this; 1952 } 1953 1954 /** 1955 * @return {@link #copyright} (A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1956 */ 1957 public MarkdownType getCopyrightElement() { 1958 if (this.copyright == null) 1959 if (Configuration.errorOnAutoCreate()) 1960 throw new Error("Attempt to auto-create NamingSystem.copyright"); 1961 else if (Configuration.doAutoCreate()) 1962 this.copyright = new MarkdownType(); // bb 1963 return this.copyright; 1964 } 1965 1966 public boolean hasCopyrightElement() { 1967 return this.copyright != null && !this.copyright.isEmpty(); 1968 } 1969 1970 public boolean hasCopyright() { 1971 return this.copyright != null && !this.copyright.isEmpty(); 1972 } 1973 1974 /** 1975 * @param value {@link #copyright} (A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1976 */ 1977 public NamingSystem setCopyrightElement(MarkdownType value) { 1978 this.copyright = value; 1979 return this; 1980 } 1981 1982 /** 1983 * @return A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system. 1984 */ 1985 public String getCopyright() { 1986 return this.copyright == null ? null : this.copyright.getValue(); 1987 } 1988 1989 /** 1990 * @param value A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system. 1991 */ 1992 public NamingSystem setCopyright(String value) { 1993 if (Utilities.noString(value)) 1994 this.copyright = null; 1995 else { 1996 if (this.copyright == null) 1997 this.copyright = new MarkdownType(); 1998 this.copyright.setValue(value); 1999 } 2000 return this; 2001 } 2002 2003 /** 2004 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2005 */ 2006 public StringType getCopyrightLabelElement() { 2007 if (this.copyrightLabel == null) 2008 if (Configuration.errorOnAutoCreate()) 2009 throw new Error("Attempt to auto-create NamingSystem.copyrightLabel"); 2010 else if (Configuration.doAutoCreate()) 2011 this.copyrightLabel = new StringType(); // bb 2012 return this.copyrightLabel; 2013 } 2014 2015 public boolean hasCopyrightLabelElement() { 2016 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2017 } 2018 2019 public boolean hasCopyrightLabel() { 2020 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2021 } 2022 2023 /** 2024 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2025 */ 2026 public NamingSystem setCopyrightLabelElement(StringType value) { 2027 this.copyrightLabel = value; 2028 return this; 2029 } 2030 2031 /** 2032 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2033 */ 2034 public String getCopyrightLabel() { 2035 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2036 } 2037 2038 /** 2039 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2040 */ 2041 public NamingSystem setCopyrightLabel(String value) { 2042 if (Utilities.noString(value)) 2043 this.copyrightLabel = null; 2044 else { 2045 if (this.copyrightLabel == null) 2046 this.copyrightLabel = new StringType(); 2047 this.copyrightLabel.setValue(value); 2048 } 2049 return this; 2050 } 2051 2052 /** 2053 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2054 */ 2055 public DateType getApprovalDateElement() { 2056 if (this.approvalDate == null) 2057 if (Configuration.errorOnAutoCreate()) 2058 throw new Error("Attempt to auto-create NamingSystem.approvalDate"); 2059 else if (Configuration.doAutoCreate()) 2060 this.approvalDate = new DateType(); // bb 2061 return this.approvalDate; 2062 } 2063 2064 public boolean hasApprovalDateElement() { 2065 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2066 } 2067 2068 public boolean hasApprovalDate() { 2069 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2070 } 2071 2072 /** 2073 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2074 */ 2075 public NamingSystem setApprovalDateElement(DateType value) { 2076 this.approvalDate = value; 2077 return this; 2078 } 2079 2080 /** 2081 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2082 */ 2083 public Date getApprovalDate() { 2084 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2085 } 2086 2087 /** 2088 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2089 */ 2090 public NamingSystem setApprovalDate(Date value) { 2091 if (value == null) 2092 this.approvalDate = null; 2093 else { 2094 if (this.approvalDate == null) 2095 this.approvalDate = new DateType(); 2096 this.approvalDate.setValue(value); 2097 } 2098 return this; 2099 } 2100 2101 /** 2102 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2103 */ 2104 public DateType getLastReviewDateElement() { 2105 if (this.lastReviewDate == null) 2106 if (Configuration.errorOnAutoCreate()) 2107 throw new Error("Attempt to auto-create NamingSystem.lastReviewDate"); 2108 else if (Configuration.doAutoCreate()) 2109 this.lastReviewDate = new DateType(); // bb 2110 return this.lastReviewDate; 2111 } 2112 2113 public boolean hasLastReviewDateElement() { 2114 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2115 } 2116 2117 public boolean hasLastReviewDate() { 2118 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2119 } 2120 2121 /** 2122 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2123 */ 2124 public NamingSystem setLastReviewDateElement(DateType value) { 2125 this.lastReviewDate = value; 2126 return this; 2127 } 2128 2129 /** 2130 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 2131 */ 2132 public Date getLastReviewDate() { 2133 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2134 } 2135 2136 /** 2137 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 2138 */ 2139 public NamingSystem setLastReviewDate(Date value) { 2140 if (value == null) 2141 this.lastReviewDate = null; 2142 else { 2143 if (this.lastReviewDate == null) 2144 this.lastReviewDate = new DateType(); 2145 this.lastReviewDate.setValue(value); 2146 } 2147 return this; 2148 } 2149 2150 /** 2151 * @return {@link #effectivePeriod} (The period during which the NamingSystem content was or is planned to be in active use.) 2152 */ 2153 public Period getEffectivePeriod() { 2154 if (this.effectivePeriod == null) 2155 if (Configuration.errorOnAutoCreate()) 2156 throw new Error("Attempt to auto-create NamingSystem.effectivePeriod"); 2157 else if (Configuration.doAutoCreate()) 2158 this.effectivePeriod = new Period(); // cc 2159 return this.effectivePeriod; 2160 } 2161 2162 public boolean hasEffectivePeriod() { 2163 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2164 } 2165 2166 /** 2167 * @param value {@link #effectivePeriod} (The period during which the NamingSystem content was or is planned to be in active use.) 2168 */ 2169 public NamingSystem setEffectivePeriod(Period value) { 2170 this.effectivePeriod = value; 2171 return this; 2172 } 2173 2174 /** 2175 * @return {@link #topic} (Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching.) 2176 */ 2177 public List<CodeableConcept> getTopic() { 2178 if (this.topic == null) 2179 this.topic = new ArrayList<CodeableConcept>(); 2180 return this.topic; 2181 } 2182 2183 /** 2184 * @return Returns a reference to <code>this</code> for easy method chaining 2185 */ 2186 public NamingSystem setTopic(List<CodeableConcept> theTopic) { 2187 this.topic = theTopic; 2188 return this; 2189 } 2190 2191 public boolean hasTopic() { 2192 if (this.topic == null) 2193 return false; 2194 for (CodeableConcept item : this.topic) 2195 if (!item.isEmpty()) 2196 return true; 2197 return false; 2198 } 2199 2200 public CodeableConcept addTopic() { //3 2201 CodeableConcept t = new CodeableConcept(); 2202 if (this.topic == null) 2203 this.topic = new ArrayList<CodeableConcept>(); 2204 this.topic.add(t); 2205 return t; 2206 } 2207 2208 public NamingSystem addTopic(CodeableConcept t) { //3 2209 if (t == null) 2210 return this; 2211 if (this.topic == null) 2212 this.topic = new ArrayList<CodeableConcept>(); 2213 this.topic.add(t); 2214 return this; 2215 } 2216 2217 /** 2218 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 2219 */ 2220 public CodeableConcept getTopicFirstRep() { 2221 if (getTopic().isEmpty()) { 2222 addTopic(); 2223 } 2224 return getTopic().get(0); 2225 } 2226 2227 /** 2228 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the NamingSystem.) 2229 */ 2230 public List<ContactDetail> getAuthor() { 2231 if (this.author == null) 2232 this.author = new ArrayList<ContactDetail>(); 2233 return this.author; 2234 } 2235 2236 /** 2237 * @return Returns a reference to <code>this</code> for easy method chaining 2238 */ 2239 public NamingSystem setAuthor(List<ContactDetail> theAuthor) { 2240 this.author = theAuthor; 2241 return this; 2242 } 2243 2244 public boolean hasAuthor() { 2245 if (this.author == null) 2246 return false; 2247 for (ContactDetail item : this.author) 2248 if (!item.isEmpty()) 2249 return true; 2250 return false; 2251 } 2252 2253 public ContactDetail addAuthor() { //3 2254 ContactDetail t = new ContactDetail(); 2255 if (this.author == null) 2256 this.author = new ArrayList<ContactDetail>(); 2257 this.author.add(t); 2258 return t; 2259 } 2260 2261 public NamingSystem addAuthor(ContactDetail t) { //3 2262 if (t == null) 2263 return this; 2264 if (this.author == null) 2265 this.author = new ArrayList<ContactDetail>(); 2266 this.author.add(t); 2267 return this; 2268 } 2269 2270 /** 2271 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 2272 */ 2273 public ContactDetail getAuthorFirstRep() { 2274 if (getAuthor().isEmpty()) { 2275 addAuthor(); 2276 } 2277 return getAuthor().get(0); 2278 } 2279 2280 /** 2281 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the NamingSystem.) 2282 */ 2283 public List<ContactDetail> getEditor() { 2284 if (this.editor == null) 2285 this.editor = new ArrayList<ContactDetail>(); 2286 return this.editor; 2287 } 2288 2289 /** 2290 * @return Returns a reference to <code>this</code> for easy method chaining 2291 */ 2292 public NamingSystem setEditor(List<ContactDetail> theEditor) { 2293 this.editor = theEditor; 2294 return this; 2295 } 2296 2297 public boolean hasEditor() { 2298 if (this.editor == null) 2299 return false; 2300 for (ContactDetail item : this.editor) 2301 if (!item.isEmpty()) 2302 return true; 2303 return false; 2304 } 2305 2306 public ContactDetail addEditor() { //3 2307 ContactDetail t = new ContactDetail(); 2308 if (this.editor == null) 2309 this.editor = new ArrayList<ContactDetail>(); 2310 this.editor.add(t); 2311 return t; 2312 } 2313 2314 public NamingSystem addEditor(ContactDetail t) { //3 2315 if (t == null) 2316 return this; 2317 if (this.editor == null) 2318 this.editor = new ArrayList<ContactDetail>(); 2319 this.editor.add(t); 2320 return this; 2321 } 2322 2323 /** 2324 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 2325 */ 2326 public ContactDetail getEditorFirstRep() { 2327 if (getEditor().isEmpty()) { 2328 addEditor(); 2329 } 2330 return getEditor().get(0); 2331 } 2332 2333 /** 2334 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem.) 2335 */ 2336 public List<ContactDetail> getReviewer() { 2337 if (this.reviewer == null) 2338 this.reviewer = new ArrayList<ContactDetail>(); 2339 return this.reviewer; 2340 } 2341 2342 /** 2343 * @return Returns a reference to <code>this</code> for easy method chaining 2344 */ 2345 public NamingSystem setReviewer(List<ContactDetail> theReviewer) { 2346 this.reviewer = theReviewer; 2347 return this; 2348 } 2349 2350 public boolean hasReviewer() { 2351 if (this.reviewer == null) 2352 return false; 2353 for (ContactDetail item : this.reviewer) 2354 if (!item.isEmpty()) 2355 return true; 2356 return false; 2357 } 2358 2359 public ContactDetail addReviewer() { //3 2360 ContactDetail t = new ContactDetail(); 2361 if (this.reviewer == null) 2362 this.reviewer = new ArrayList<ContactDetail>(); 2363 this.reviewer.add(t); 2364 return t; 2365 } 2366 2367 public NamingSystem addReviewer(ContactDetail t) { //3 2368 if (t == null) 2369 return this; 2370 if (this.reviewer == null) 2371 this.reviewer = new ArrayList<ContactDetail>(); 2372 this.reviewer.add(t); 2373 return this; 2374 } 2375 2376 /** 2377 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 2378 */ 2379 public ContactDetail getReviewerFirstRep() { 2380 if (getReviewer().isEmpty()) { 2381 addReviewer(); 2382 } 2383 return getReviewer().get(0); 2384 } 2385 2386 /** 2387 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting.) 2388 */ 2389 public List<ContactDetail> getEndorser() { 2390 if (this.endorser == null) 2391 this.endorser = new ArrayList<ContactDetail>(); 2392 return this.endorser; 2393 } 2394 2395 /** 2396 * @return Returns a reference to <code>this</code> for easy method chaining 2397 */ 2398 public NamingSystem setEndorser(List<ContactDetail> theEndorser) { 2399 this.endorser = theEndorser; 2400 return this; 2401 } 2402 2403 public boolean hasEndorser() { 2404 if (this.endorser == null) 2405 return false; 2406 for (ContactDetail item : this.endorser) 2407 if (!item.isEmpty()) 2408 return true; 2409 return false; 2410 } 2411 2412 public ContactDetail addEndorser() { //3 2413 ContactDetail t = new ContactDetail(); 2414 if (this.endorser == null) 2415 this.endorser = new ArrayList<ContactDetail>(); 2416 this.endorser.add(t); 2417 return t; 2418 } 2419 2420 public NamingSystem addEndorser(ContactDetail t) { //3 2421 if (t == null) 2422 return this; 2423 if (this.endorser == null) 2424 this.endorser = new ArrayList<ContactDetail>(); 2425 this.endorser.add(t); 2426 return this; 2427 } 2428 2429 /** 2430 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 2431 */ 2432 public ContactDetail getEndorserFirstRep() { 2433 if (getEndorser().isEmpty()) { 2434 addEndorser(); 2435 } 2436 return getEndorser().get(0); 2437 } 2438 2439 /** 2440 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 2441 */ 2442 public List<RelatedArtifact> getRelatedArtifact() { 2443 if (this.relatedArtifact == null) 2444 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2445 return this.relatedArtifact; 2446 } 2447 2448 /** 2449 * @return Returns a reference to <code>this</code> for easy method chaining 2450 */ 2451 public NamingSystem setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2452 this.relatedArtifact = theRelatedArtifact; 2453 return this; 2454 } 2455 2456 public boolean hasRelatedArtifact() { 2457 if (this.relatedArtifact == null) 2458 return false; 2459 for (RelatedArtifact item : this.relatedArtifact) 2460 if (!item.isEmpty()) 2461 return true; 2462 return false; 2463 } 2464 2465 public RelatedArtifact addRelatedArtifact() { //3 2466 RelatedArtifact t = new RelatedArtifact(); 2467 if (this.relatedArtifact == null) 2468 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2469 this.relatedArtifact.add(t); 2470 return t; 2471 } 2472 2473 public NamingSystem addRelatedArtifact(RelatedArtifact t) { //3 2474 if (t == null) 2475 return this; 2476 if (this.relatedArtifact == null) 2477 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2478 this.relatedArtifact.add(t); 2479 return this; 2480 } 2481 2482 /** 2483 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 2484 */ 2485 public RelatedArtifact getRelatedArtifactFirstRep() { 2486 if (getRelatedArtifact().isEmpty()) { 2487 addRelatedArtifact(); 2488 } 2489 return getRelatedArtifact().get(0); 2490 } 2491 2492 /** 2493 * @return {@link #usage} (Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2494 */ 2495 public StringType getUsageElement() { 2496 if (this.usage == null) 2497 if (Configuration.errorOnAutoCreate()) 2498 throw new Error("Attempt to auto-create NamingSystem.usage"); 2499 else if (Configuration.doAutoCreate()) 2500 this.usage = new StringType(); // bb 2501 return this.usage; 2502 } 2503 2504 public boolean hasUsageElement() { 2505 return this.usage != null && !this.usage.isEmpty(); 2506 } 2507 2508 public boolean hasUsage() { 2509 return this.usage != null && !this.usage.isEmpty(); 2510 } 2511 2512 /** 2513 * @param value {@link #usage} (Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2514 */ 2515 public NamingSystem setUsageElement(StringType value) { 2516 this.usage = value; 2517 return this; 2518 } 2519 2520 /** 2521 * @return Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 2522 */ 2523 public String getUsage() { 2524 return this.usage == null ? null : this.usage.getValue(); 2525 } 2526 2527 /** 2528 * @param value Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 2529 */ 2530 public NamingSystem setUsage(String value) { 2531 if (Utilities.noString(value)) 2532 this.usage = null; 2533 else { 2534 if (this.usage == null) 2535 this.usage = new StringType(); 2536 this.usage.setValue(value); 2537 } 2538 return this; 2539 } 2540 2541 /** 2542 * @return {@link #uniqueId} (Indicates how the system may be identified when referenced in electronic exchange.) 2543 */ 2544 public List<NamingSystemUniqueIdComponent> getUniqueId() { 2545 if (this.uniqueId == null) 2546 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2547 return this.uniqueId; 2548 } 2549 2550 /** 2551 * @return Returns a reference to <code>this</code> for easy method chaining 2552 */ 2553 public NamingSystem setUniqueId(List<NamingSystemUniqueIdComponent> theUniqueId) { 2554 this.uniqueId = theUniqueId; 2555 return this; 2556 } 2557 2558 public boolean hasUniqueId() { 2559 if (this.uniqueId == null) 2560 return false; 2561 for (NamingSystemUniqueIdComponent item : this.uniqueId) 2562 if (!item.isEmpty()) 2563 return true; 2564 return false; 2565 } 2566 2567 public NamingSystemUniqueIdComponent addUniqueId() { //3 2568 NamingSystemUniqueIdComponent t = new NamingSystemUniqueIdComponent(); 2569 if (this.uniqueId == null) 2570 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2571 this.uniqueId.add(t); 2572 return t; 2573 } 2574 2575 public NamingSystem addUniqueId(NamingSystemUniqueIdComponent t) { //3 2576 if (t == null) 2577 return this; 2578 if (this.uniqueId == null) 2579 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2580 this.uniqueId.add(t); 2581 return this; 2582 } 2583 2584 /** 2585 * @return The first repetition of repeating field {@link #uniqueId}, creating it if it does not already exist {3} 2586 */ 2587 public NamingSystemUniqueIdComponent getUniqueIdFirstRep() { 2588 if (getUniqueId().isEmpty()) { 2589 addUniqueId(); 2590 } 2591 return getUniqueId().get(0); 2592 } 2593 2594 protected void listChildren(List<Property> children) { 2595 super.listChildren(children); 2596 children.add(new Property("url", "uri", "An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.", 0, 1, url)); 2597 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2598 children.add(new Property("version", "string", "The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2599 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm)); 2600 children.add(new Property("name", "string", "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2601 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the naming system.", 0, 1, title)); 2602 children.add(new Property("status", "code", "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status)); 2603 children.add(new Property("kind", "code", "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind)); 2604 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2605 children.add(new Property("date", "dateTime", "The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 0, 1, date)); 2606 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.", 0, 1, publisher)); 2607 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2608 children.add(new Property("responsible", "string", "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 0, 1, responsible)); 2609 children.add(new Property("type", "CodeableConcept", "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type)); 2610 children.add(new Property("description", "markdown", "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1, description)); 2611 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2612 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the naming system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2613 children.add(new Property("purpose", "markdown", "Explanation of why this naming system is needed and why it has been designed as it has.", 0, 1, purpose)); 2614 children.add(new Property("copyright", "markdown", "A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.", 0, 1, copyright)); 2615 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2616 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 2617 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 2618 children.add(new Property("effectivePeriod", "Period", "The period during which the NamingSystem content was or is planned to be in active use.", 0, 1, effectivePeriod)); 2619 children.add(new Property("topic", "CodeableConcept", "Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 2620 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, author)); 2621 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, editor)); 2622 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 2623 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 2624 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 2625 children.add(new Property("usage", "string", "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 0, 1, usage)); 2626 children.add(new Property("uniqueId", "", "Indicates how the system may be identified when referenced in electronic exchange.", 0, java.lang.Integer.MAX_VALUE, uniqueId)); 2627 } 2628 2629 @Override 2630 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2631 switch (_hash) { 2632 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.", 0, 1, url); 2633 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2634 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2635 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2636 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2637 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2638 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2639 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2640 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the naming system.", 0, 1, title); 2641 case -892481550: /*status*/ return new Property("status", "code", "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status); 2642 case 3292052: /*kind*/ return new Property("kind", "code", "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind); 2643 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2644 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 0, 1, date); 2645 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.", 0, 1, publisher); 2646 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2647 case 1847674614: /*responsible*/ return new Property("responsible", "string", "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 0, 1, responsible); 2648 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type); 2649 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1, description); 2650 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2651 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the naming system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2652 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this naming system is needed and why it has been designed as it has.", 0, 1, purpose); 2653 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.", 0, 1, copyright); 2654 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2655 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 2656 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 2657 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the NamingSystem content was or is planned to be in active use.", 0, 1, effectivePeriod); 2658 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 2659 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, author); 2660 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, editor); 2661 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, reviewer); 2662 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 2663 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 2664 case 111574433: /*usage*/ return new Property("usage", "string", "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 0, 1, usage); 2665 case -294460212: /*uniqueId*/ return new Property("uniqueId", "", "Indicates how the system may be identified when referenced in electronic exchange.", 0, java.lang.Integer.MAX_VALUE, uniqueId); 2666 default: return super.getNamedProperty(_hash, _name, _checkValid); 2667 } 2668 2669 } 2670 2671 @Override 2672 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2673 switch (hash) { 2674 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2675 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2676 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2677 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2678 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2679 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2680 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2681 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<NamingSystemType> 2682 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2683 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2684 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2685 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2686 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // StringType 2687 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2688 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2689 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2690 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2691 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2692 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2693 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2694 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 2695 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 2696 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 2697 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 2698 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 2699 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 2700 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 2701 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 2702 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2703 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 2704 case -294460212: /*uniqueId*/ return this.uniqueId == null ? new Base[0] : this.uniqueId.toArray(new Base[this.uniqueId.size()]); // NamingSystemUniqueIdComponent 2705 default: return super.getProperty(hash, name, checkValid); 2706 } 2707 2708 } 2709 2710 @Override 2711 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2712 switch (hash) { 2713 case 116079: // url 2714 this.url = TypeConvertor.castToUri(value); // UriType 2715 return value; 2716 case -1618432855: // identifier 2717 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2718 return value; 2719 case 351608024: // version 2720 this.version = TypeConvertor.castToString(value); // StringType 2721 return value; 2722 case 1508158071: // versionAlgorithm 2723 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2724 return value; 2725 case 3373707: // name 2726 this.name = TypeConvertor.castToString(value); // StringType 2727 return value; 2728 case 110371416: // title 2729 this.title = TypeConvertor.castToString(value); // StringType 2730 return value; 2731 case -892481550: // status 2732 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2733 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2734 return value; 2735 case 3292052: // kind 2736 value = new NamingSystemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2737 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 2738 return value; 2739 case -404562712: // experimental 2740 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2741 return value; 2742 case 3076014: // date 2743 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2744 return value; 2745 case 1447404028: // publisher 2746 this.publisher = TypeConvertor.castToString(value); // StringType 2747 return value; 2748 case 951526432: // contact 2749 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2750 return value; 2751 case 1847674614: // responsible 2752 this.responsible = TypeConvertor.castToString(value); // StringType 2753 return value; 2754 case 3575610: // type 2755 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2756 return value; 2757 case -1724546052: // description 2758 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2759 return value; 2760 case -669707736: // useContext 2761 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2762 return value; 2763 case -507075711: // jurisdiction 2764 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2765 return value; 2766 case -220463842: // purpose 2767 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2768 return value; 2769 case 1522889671: // copyright 2770 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2771 return value; 2772 case 765157229: // copyrightLabel 2773 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2774 return value; 2775 case 223539345: // approvalDate 2776 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2777 return value; 2778 case -1687512484: // lastReviewDate 2779 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2780 return value; 2781 case -403934648: // effectivePeriod 2782 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 2783 return value; 2784 case 110546223: // topic 2785 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2786 return value; 2787 case -1406328437: // author 2788 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2789 return value; 2790 case -1307827859: // editor 2791 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2792 return value; 2793 case -261190139: // reviewer 2794 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2795 return value; 2796 case 1740277666: // endorser 2797 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2798 return value; 2799 case 666807069: // relatedArtifact 2800 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 2801 return value; 2802 case 111574433: // usage 2803 this.usage = TypeConvertor.castToString(value); // StringType 2804 return value; 2805 case -294460212: // uniqueId 2806 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); // NamingSystemUniqueIdComponent 2807 return value; 2808 default: return super.setProperty(hash, name, value); 2809 } 2810 2811 } 2812 2813 @Override 2814 public Base setProperty(String name, Base value) throws FHIRException { 2815 if (name.equals("url")) { 2816 this.url = TypeConvertor.castToUri(value); // UriType 2817 } else if (name.equals("identifier")) { 2818 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2819 } else if (name.equals("version")) { 2820 this.version = TypeConvertor.castToString(value); // StringType 2821 } else if (name.equals("versionAlgorithm[x]")) { 2822 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2823 } else if (name.equals("name")) { 2824 this.name = TypeConvertor.castToString(value); // StringType 2825 } else if (name.equals("title")) { 2826 this.title = TypeConvertor.castToString(value); // StringType 2827 } else if (name.equals("status")) { 2828 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2829 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2830 } else if (name.equals("kind")) { 2831 value = new NamingSystemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2832 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 2833 } else if (name.equals("experimental")) { 2834 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2835 } else if (name.equals("date")) { 2836 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2837 } else if (name.equals("publisher")) { 2838 this.publisher = TypeConvertor.castToString(value); // StringType 2839 } else if (name.equals("contact")) { 2840 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2841 } else if (name.equals("responsible")) { 2842 this.responsible = TypeConvertor.castToString(value); // StringType 2843 } else if (name.equals("type")) { 2844 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2845 } else if (name.equals("description")) { 2846 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2847 } else if (name.equals("useContext")) { 2848 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2849 } else if (name.equals("jurisdiction")) { 2850 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2851 } else if (name.equals("purpose")) { 2852 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2853 } else if (name.equals("copyright")) { 2854 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2855 } else if (name.equals("copyrightLabel")) { 2856 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2857 } else if (name.equals("approvalDate")) { 2858 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2859 } else if (name.equals("lastReviewDate")) { 2860 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2861 } else if (name.equals("effectivePeriod")) { 2862 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 2863 } else if (name.equals("topic")) { 2864 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 2865 } else if (name.equals("author")) { 2866 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 2867 } else if (name.equals("editor")) { 2868 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 2869 } else if (name.equals("reviewer")) { 2870 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 2871 } else if (name.equals("endorser")) { 2872 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 2873 } else if (name.equals("relatedArtifact")) { 2874 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 2875 } else if (name.equals("usage")) { 2876 this.usage = TypeConvertor.castToString(value); // StringType 2877 } else if (name.equals("uniqueId")) { 2878 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); 2879 } else 2880 return super.setProperty(name, value); 2881 return value; 2882 } 2883 2884 @Override 2885 public void removeChild(String name, Base value) throws FHIRException { 2886 if (name.equals("url")) { 2887 this.url = null; 2888 } else if (name.equals("identifier")) { 2889 this.getIdentifier().remove(value); 2890 } else if (name.equals("version")) { 2891 this.version = null; 2892 } else if (name.equals("versionAlgorithm[x]")) { 2893 this.versionAlgorithm = null; 2894 } else if (name.equals("name")) { 2895 this.name = null; 2896 } else if (name.equals("title")) { 2897 this.title = null; 2898 } else if (name.equals("status")) { 2899 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2900 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2901 } else if (name.equals("kind")) { 2902 value = new NamingSystemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2903 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 2904 } else if (name.equals("experimental")) { 2905 this.experimental = null; 2906 } else if (name.equals("date")) { 2907 this.date = null; 2908 } else if (name.equals("publisher")) { 2909 this.publisher = null; 2910 } else if (name.equals("contact")) { 2911 this.getContact().remove(value); 2912 } else if (name.equals("responsible")) { 2913 this.responsible = null; 2914 } else if (name.equals("type")) { 2915 this.type = null; 2916 } else if (name.equals("description")) { 2917 this.description = null; 2918 } else if (name.equals("useContext")) { 2919 this.getUseContext().remove(value); 2920 } else if (name.equals("jurisdiction")) { 2921 this.getJurisdiction().remove(value); 2922 } else if (name.equals("purpose")) { 2923 this.purpose = null; 2924 } else if (name.equals("copyright")) { 2925 this.copyright = null; 2926 } else if (name.equals("copyrightLabel")) { 2927 this.copyrightLabel = null; 2928 } else if (name.equals("approvalDate")) { 2929 this.approvalDate = null; 2930 } else if (name.equals("lastReviewDate")) { 2931 this.lastReviewDate = null; 2932 } else if (name.equals("effectivePeriod")) { 2933 this.effectivePeriod = null; 2934 } else if (name.equals("topic")) { 2935 this.getTopic().remove(value); 2936 } else if (name.equals("author")) { 2937 this.getAuthor().remove(value); 2938 } else if (name.equals("editor")) { 2939 this.getEditor().remove(value); 2940 } else if (name.equals("reviewer")) { 2941 this.getReviewer().remove(value); 2942 } else if (name.equals("endorser")) { 2943 this.getEndorser().remove(value); 2944 } else if (name.equals("relatedArtifact")) { 2945 this.getRelatedArtifact().remove(value); 2946 } else if (name.equals("usage")) { 2947 this.usage = null; 2948 } else if (name.equals("uniqueId")) { 2949 this.getUniqueId().remove((NamingSystemUniqueIdComponent) value); 2950 } else 2951 super.removeChild(name, value); 2952 2953 } 2954 2955 @Override 2956 public Base makeProperty(int hash, String name) throws FHIRException { 2957 switch (hash) { 2958 case 116079: return getUrlElement(); 2959 case -1618432855: return addIdentifier(); 2960 case 351608024: return getVersionElement(); 2961 case -115699031: return getVersionAlgorithm(); 2962 case 1508158071: return getVersionAlgorithm(); 2963 case 3373707: return getNameElement(); 2964 case 110371416: return getTitleElement(); 2965 case -892481550: return getStatusElement(); 2966 case 3292052: return getKindElement(); 2967 case -404562712: return getExperimentalElement(); 2968 case 3076014: return getDateElement(); 2969 case 1447404028: return getPublisherElement(); 2970 case 951526432: return addContact(); 2971 case 1847674614: return getResponsibleElement(); 2972 case 3575610: return getType(); 2973 case -1724546052: return getDescriptionElement(); 2974 case -669707736: return addUseContext(); 2975 case -507075711: return addJurisdiction(); 2976 case -220463842: return getPurposeElement(); 2977 case 1522889671: return getCopyrightElement(); 2978 case 765157229: return getCopyrightLabelElement(); 2979 case 223539345: return getApprovalDateElement(); 2980 case -1687512484: return getLastReviewDateElement(); 2981 case -403934648: return getEffectivePeriod(); 2982 case 110546223: return addTopic(); 2983 case -1406328437: return addAuthor(); 2984 case -1307827859: return addEditor(); 2985 case -261190139: return addReviewer(); 2986 case 1740277666: return addEndorser(); 2987 case 666807069: return addRelatedArtifact(); 2988 case 111574433: return getUsageElement(); 2989 case -294460212: return addUniqueId(); 2990 default: return super.makeProperty(hash, name); 2991 } 2992 2993 } 2994 2995 @Override 2996 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2997 switch (hash) { 2998 case 116079: /*url*/ return new String[] {"uri"}; 2999 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3000 case 351608024: /*version*/ return new String[] {"string"}; 3001 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3002 case 3373707: /*name*/ return new String[] {"string"}; 3003 case 110371416: /*title*/ return new String[] {"string"}; 3004 case -892481550: /*status*/ return new String[] {"code"}; 3005 case 3292052: /*kind*/ return new String[] {"code"}; 3006 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3007 case 3076014: /*date*/ return new String[] {"dateTime"}; 3008 case 1447404028: /*publisher*/ return new String[] {"string"}; 3009 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3010 case 1847674614: /*responsible*/ return new String[] {"string"}; 3011 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3012 case -1724546052: /*description*/ return new String[] {"markdown"}; 3013 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3014 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3015 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3016 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3017 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 3018 case 223539345: /*approvalDate*/ return new String[] {"date"}; 3019 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 3020 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 3021 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 3022 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 3023 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 3024 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 3025 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 3026 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 3027 case 111574433: /*usage*/ return new String[] {"string"}; 3028 case -294460212: /*uniqueId*/ return new String[] {}; 3029 default: return super.getTypesForProperty(hash, name); 3030 } 3031 3032 } 3033 3034 @Override 3035 public Base addChild(String name) throws FHIRException { 3036 if (name.equals("url")) { 3037 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.url"); 3038 } 3039 else if (name.equals("identifier")) { 3040 return addIdentifier(); 3041 } 3042 else if (name.equals("version")) { 3043 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.version"); 3044 } 3045 else if (name.equals("versionAlgorithmString")) { 3046 this.versionAlgorithm = new StringType(); 3047 return this.versionAlgorithm; 3048 } 3049 else if (name.equals("versionAlgorithmCoding")) { 3050 this.versionAlgorithm = new Coding(); 3051 return this.versionAlgorithm; 3052 } 3053 else if (name.equals("name")) { 3054 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 3055 } 3056 else if (name.equals("title")) { 3057 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.title"); 3058 } 3059 else if (name.equals("status")) { 3060 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.status"); 3061 } 3062 else if (name.equals("kind")) { 3063 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.kind"); 3064 } 3065 else if (name.equals("experimental")) { 3066 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.experimental"); 3067 } 3068 else if (name.equals("date")) { 3069 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.date"); 3070 } 3071 else if (name.equals("publisher")) { 3072 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.publisher"); 3073 } 3074 else if (name.equals("contact")) { 3075 return addContact(); 3076 } 3077 else if (name.equals("responsible")) { 3078 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.responsible"); 3079 } 3080 else if (name.equals("type")) { 3081 this.type = new CodeableConcept(); 3082 return this.type; 3083 } 3084 else if (name.equals("description")) { 3085 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.description"); 3086 } 3087 else if (name.equals("useContext")) { 3088 return addUseContext(); 3089 } 3090 else if (name.equals("jurisdiction")) { 3091 return addJurisdiction(); 3092 } 3093 else if (name.equals("purpose")) { 3094 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.purpose"); 3095 } 3096 else if (name.equals("copyright")) { 3097 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.copyright"); 3098 } 3099 else if (name.equals("copyrightLabel")) { 3100 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.copyrightLabel"); 3101 } 3102 else if (name.equals("approvalDate")) { 3103 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.approvalDate"); 3104 } 3105 else if (name.equals("lastReviewDate")) { 3106 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.lastReviewDate"); 3107 } 3108 else if (name.equals("effectivePeriod")) { 3109 this.effectivePeriod = new Period(); 3110 return this.effectivePeriod; 3111 } 3112 else if (name.equals("topic")) { 3113 return addTopic(); 3114 } 3115 else if (name.equals("author")) { 3116 return addAuthor(); 3117 } 3118 else if (name.equals("editor")) { 3119 return addEditor(); 3120 } 3121 else if (name.equals("reviewer")) { 3122 return addReviewer(); 3123 } 3124 else if (name.equals("endorser")) { 3125 return addEndorser(); 3126 } 3127 else if (name.equals("relatedArtifact")) { 3128 return addRelatedArtifact(); 3129 } 3130 else if (name.equals("usage")) { 3131 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.usage"); 3132 } 3133 else if (name.equals("uniqueId")) { 3134 return addUniqueId(); 3135 } 3136 else 3137 return super.addChild(name); 3138 } 3139 3140 public String fhirType() { 3141 return "NamingSystem"; 3142 3143 } 3144 3145 public NamingSystem copy() { 3146 NamingSystem dst = new NamingSystem(); 3147 copyValues(dst); 3148 return dst; 3149 } 3150 3151 public void copyValues(NamingSystem dst) { 3152 super.copyValues(dst); 3153 dst.url = url == null ? null : url.copy(); 3154 if (identifier != null) { 3155 dst.identifier = new ArrayList<Identifier>(); 3156 for (Identifier i : identifier) 3157 dst.identifier.add(i.copy()); 3158 }; 3159 dst.version = version == null ? null : version.copy(); 3160 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 3161 dst.name = name == null ? null : name.copy(); 3162 dst.title = title == null ? null : title.copy(); 3163 dst.status = status == null ? null : status.copy(); 3164 dst.kind = kind == null ? null : kind.copy(); 3165 dst.experimental = experimental == null ? null : experimental.copy(); 3166 dst.date = date == null ? null : date.copy(); 3167 dst.publisher = publisher == null ? null : publisher.copy(); 3168 if (contact != null) { 3169 dst.contact = new ArrayList<ContactDetail>(); 3170 for (ContactDetail i : contact) 3171 dst.contact.add(i.copy()); 3172 }; 3173 dst.responsible = responsible == null ? null : responsible.copy(); 3174 dst.type = type == null ? null : type.copy(); 3175 dst.description = description == null ? null : description.copy(); 3176 if (useContext != null) { 3177 dst.useContext = new ArrayList<UsageContext>(); 3178 for (UsageContext i : useContext) 3179 dst.useContext.add(i.copy()); 3180 }; 3181 if (jurisdiction != null) { 3182 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3183 for (CodeableConcept i : jurisdiction) 3184 dst.jurisdiction.add(i.copy()); 3185 }; 3186 dst.purpose = purpose == null ? null : purpose.copy(); 3187 dst.copyright = copyright == null ? null : copyright.copy(); 3188 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 3189 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 3190 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 3191 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 3192 if (topic != null) { 3193 dst.topic = new ArrayList<CodeableConcept>(); 3194 for (CodeableConcept i : topic) 3195 dst.topic.add(i.copy()); 3196 }; 3197 if (author != null) { 3198 dst.author = new ArrayList<ContactDetail>(); 3199 for (ContactDetail i : author) 3200 dst.author.add(i.copy()); 3201 }; 3202 if (editor != null) { 3203 dst.editor = new ArrayList<ContactDetail>(); 3204 for (ContactDetail i : editor) 3205 dst.editor.add(i.copy()); 3206 }; 3207 if (reviewer != null) { 3208 dst.reviewer = new ArrayList<ContactDetail>(); 3209 for (ContactDetail i : reviewer) 3210 dst.reviewer.add(i.copy()); 3211 }; 3212 if (endorser != null) { 3213 dst.endorser = new ArrayList<ContactDetail>(); 3214 for (ContactDetail i : endorser) 3215 dst.endorser.add(i.copy()); 3216 }; 3217 if (relatedArtifact != null) { 3218 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 3219 for (RelatedArtifact i : relatedArtifact) 3220 dst.relatedArtifact.add(i.copy()); 3221 }; 3222 dst.usage = usage == null ? null : usage.copy(); 3223 if (uniqueId != null) { 3224 dst.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 3225 for (NamingSystemUniqueIdComponent i : uniqueId) 3226 dst.uniqueId.add(i.copy()); 3227 }; 3228 } 3229 3230 protected NamingSystem typedCopy() { 3231 return copy(); 3232 } 3233 3234 @Override 3235 public boolean equalsDeep(Base other_) { 3236 if (!super.equalsDeep(other_)) 3237 return false; 3238 if (!(other_ instanceof NamingSystem)) 3239 return false; 3240 NamingSystem o = (NamingSystem) other_; 3241 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 3242 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 3243 && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) && compareDeep(experimental, o.experimental, true) 3244 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 3245 && compareDeep(responsible, o.responsible, true) && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 3246 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 3247 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 3248 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 3249 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 3250 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 3251 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(usage, o.usage, true) && compareDeep(uniqueId, o.uniqueId, true) 3252 ; 3253 } 3254 3255 @Override 3256 public boolean equalsShallow(Base other_) { 3257 if (!super.equalsShallow(other_)) 3258 return false; 3259 if (!(other_ instanceof NamingSystem)) 3260 return false; 3261 NamingSystem o = (NamingSystem) other_; 3262 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 3263 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(kind, o.kind, true) 3264 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 3265 && compareValues(responsible, o.responsible, true) && compareValues(description, o.description, true) 3266 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 3267 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 3268 && compareValues(usage, o.usage, true); 3269 } 3270 3271 public boolean isEmpty() { 3272 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 3273 , versionAlgorithm, name, title, status, kind, experimental, date, publisher 3274 , contact, responsible, type, description, useContext, jurisdiction, purpose, copyright 3275 , copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor 3276 , reviewer, endorser, relatedArtifact, usage, uniqueId); 3277 } 3278 3279 @Override 3280 public ResourceType getResourceType() { 3281 return ResourceType.NamingSystem; 3282 } 3283 3284 /** 3285 * Search parameter: <b>context-quantity</b> 3286 * <p> 3287 * Description: <b>Multiple Resources: 3288 3289* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3290* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3291* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3292* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3293* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3294* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3295* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3296* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3297* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3298* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3299* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3300* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3301* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3302* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3303* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3304* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3305* [Library](library.html): A quantity- or range-valued use context assigned to the library 3306* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3307* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3308* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3309* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3310* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3311* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3312* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3313* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3314* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3315* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3316* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3317* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3318* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3319</b><br> 3320 * Type: <b>quantity</b><br> 3321 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3322 * </p> 3323 */ 3324 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 3325 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3326 /** 3327 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3328 * <p> 3329 * Description: <b>Multiple Resources: 3330 3331* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3332* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3333* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3334* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3335* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3336* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3337* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3338* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3339* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3340* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3341* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3342* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3343* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3344* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3345* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3346* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3347* [Library](library.html): A quantity- or range-valued use context assigned to the library 3348* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3349* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3350* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3351* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3352* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3353* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3354* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3355* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3356* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3357* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3358* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3359* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3360* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3361</b><br> 3362 * Type: <b>quantity</b><br> 3363 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3364 * </p> 3365 */ 3366 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3367 3368 /** 3369 * Search parameter: <b>context-type-quantity</b> 3370 * <p> 3371 * Description: <b>Multiple Resources: 3372 3373* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3374* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3375* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3376* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3377* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3378* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3379* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3380* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3381* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3382* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3383* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3384* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3385* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3386* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3387* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3388* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3389* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3390* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3391* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3392* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3393* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3394* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3395* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3396* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3397* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3398* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3399* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3400* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3401* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3402* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3403</b><br> 3404 * Type: <b>composite</b><br> 3405 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3406 * </p> 3407 */ 3408 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3409 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3410 /** 3411 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3412 * <p> 3413 * Description: <b>Multiple Resources: 3414 3415* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3416* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3417* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3418* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3419* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3420* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3421* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3422* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3423* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3424* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3425* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3426* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3427* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3428* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3429* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3430* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3431* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3432* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3433* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3434* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3435* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3436* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3437* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3438* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3439* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3440* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3441* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3442* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3443* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3444* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3445</b><br> 3446 * Type: <b>composite</b><br> 3447 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3448 * </p> 3449 */ 3450 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3451 3452 /** 3453 * Search parameter: <b>context-type-value</b> 3454 * <p> 3455 * Description: <b>Multiple Resources: 3456 3457* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3458* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3459* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3460* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3461* [Citation](citation.html): A use context type and value assigned to the citation 3462* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3463* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3464* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3465* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3466* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3467* [Evidence](evidence.html): A use context type and value assigned to the evidence 3468* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3469* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3470* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3471* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3472* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3473* [Library](library.html): A use context type and value assigned to the library 3474* [Measure](measure.html): A use context type and value assigned to the measure 3475* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3476* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3477* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3478* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3479* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3480* [Requirements](requirements.html): A use context type and value assigned to the requirements 3481* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3482* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3483* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3484* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3485* [TestScript](testscript.html): A use context type and value assigned to the test script 3486* [ValueSet](valueset.html): A use context type and value assigned to the value set 3487</b><br> 3488 * Type: <b>composite</b><br> 3489 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3490 * </p> 3491 */ 3492 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3493 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3494 /** 3495 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3496 * <p> 3497 * Description: <b>Multiple Resources: 3498 3499* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3500* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3501* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3502* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3503* [Citation](citation.html): A use context type and value assigned to the citation 3504* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3505* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3506* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3507* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3508* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3509* [Evidence](evidence.html): A use context type and value assigned to the evidence 3510* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3511* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3512* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3513* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3514* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3515* [Library](library.html): A use context type and value assigned to the library 3516* [Measure](measure.html): A use context type and value assigned to the measure 3517* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3518* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3519* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3520* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3521* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3522* [Requirements](requirements.html): A use context type and value assigned to the requirements 3523* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3524* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3525* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3526* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3527* [TestScript](testscript.html): A use context type and value assigned to the test script 3528* [ValueSet](valueset.html): A use context type and value assigned to the value set 3529</b><br> 3530 * Type: <b>composite</b><br> 3531 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3532 * </p> 3533 */ 3534 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3535 3536 /** 3537 * Search parameter: <b>context-type</b> 3538 * <p> 3539 * Description: <b>Multiple Resources: 3540 3541* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3542* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3543* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3544* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3545* [Citation](citation.html): A type of use context assigned to the citation 3546* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3547* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3548* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3549* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3550* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3551* [Evidence](evidence.html): A type of use context assigned to the evidence 3552* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3553* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3554* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3555* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3556* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3557* [Library](library.html): A type of use context assigned to the library 3558* [Measure](measure.html): A type of use context assigned to the measure 3559* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3560* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3561* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3562* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3563* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3564* [Requirements](requirements.html): A type of use context assigned to the requirements 3565* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3566* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3567* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3568* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3569* [TestScript](testscript.html): A type of use context assigned to the test script 3570* [ValueSet](valueset.html): A type of use context assigned to the value set 3571</b><br> 3572 * Type: <b>token</b><br> 3573 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3574 * </p> 3575 */ 3576 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3577 public static final String SP_CONTEXT_TYPE = "context-type"; 3578 /** 3579 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3580 * <p> 3581 * Description: <b>Multiple Resources: 3582 3583* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3584* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3585* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3586* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3587* [Citation](citation.html): A type of use context assigned to the citation 3588* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3589* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3590* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3591* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3592* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3593* [Evidence](evidence.html): A type of use context assigned to the evidence 3594* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3595* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3596* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3597* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3598* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3599* [Library](library.html): A type of use context assigned to the library 3600* [Measure](measure.html): A type of use context assigned to the measure 3601* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3602* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3603* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3604* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3605* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3606* [Requirements](requirements.html): A type of use context assigned to the requirements 3607* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3608* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3609* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3610* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3611* [TestScript](testscript.html): A type of use context assigned to the test script 3612* [ValueSet](valueset.html): A type of use context assigned to the value set 3613</b><br> 3614 * Type: <b>token</b><br> 3615 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3616 * </p> 3617 */ 3618 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3619 3620 /** 3621 * Search parameter: <b>context</b> 3622 * <p> 3623 * Description: <b>Multiple Resources: 3624 3625* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3626* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3627* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3628* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3629* [Citation](citation.html): A use context assigned to the citation 3630* [CodeSystem](codesystem.html): A use context assigned to the code system 3631* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3632* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3633* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3634* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3635* [Evidence](evidence.html): A use context assigned to the evidence 3636* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3637* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3638* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3639* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3640* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3641* [Library](library.html): A use context assigned to the library 3642* [Measure](measure.html): A use context assigned to the measure 3643* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3644* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3645* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3646* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3647* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3648* [Requirements](requirements.html): A use context assigned to the requirements 3649* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3650* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3651* [StructureMap](structuremap.html): A use context assigned to the structure map 3652* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3653* [TestScript](testscript.html): A use context assigned to the test script 3654* [ValueSet](valueset.html): A use context assigned to the value set 3655</b><br> 3656 * Type: <b>token</b><br> 3657 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3658 * </p> 3659 */ 3660 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3661 public static final String SP_CONTEXT = "context"; 3662 /** 3663 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3664 * <p> 3665 * Description: <b>Multiple Resources: 3666 3667* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3668* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3669* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3670* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3671* [Citation](citation.html): A use context assigned to the citation 3672* [CodeSystem](codesystem.html): A use context assigned to the code system 3673* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3674* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3675* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3676* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3677* [Evidence](evidence.html): A use context assigned to the evidence 3678* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3679* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3680* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3681* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3682* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3683* [Library](library.html): A use context assigned to the library 3684* [Measure](measure.html): A use context assigned to the measure 3685* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3686* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3687* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3688* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3689* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3690* [Requirements](requirements.html): A use context assigned to the requirements 3691* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3692* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3693* [StructureMap](structuremap.html): A use context assigned to the structure map 3694* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3695* [TestScript](testscript.html): A use context assigned to the test script 3696* [ValueSet](valueset.html): A use context assigned to the value set 3697</b><br> 3698 * Type: <b>token</b><br> 3699 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3700 * </p> 3701 */ 3702 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3703 3704 /** 3705 * Search parameter: <b>date</b> 3706 * <p> 3707 * Description: <b>Multiple Resources: 3708 3709* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3710* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3711* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3712* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3713* [Citation](citation.html): The citation publication date 3714* [CodeSystem](codesystem.html): The code system publication date 3715* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3716* [ConceptMap](conceptmap.html): The concept map publication date 3717* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3718* [EventDefinition](eventdefinition.html): The event definition publication date 3719* [Evidence](evidence.html): The evidence publication date 3720* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3721* [ExampleScenario](examplescenario.html): The example scenario publication date 3722* [GraphDefinition](graphdefinition.html): The graph definition publication date 3723* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3724* [Library](library.html): The library publication date 3725* [Measure](measure.html): The measure publication date 3726* [MessageDefinition](messagedefinition.html): The message definition publication date 3727* [NamingSystem](namingsystem.html): The naming system publication date 3728* [OperationDefinition](operationdefinition.html): The operation definition publication date 3729* [PlanDefinition](plandefinition.html): The plan definition publication date 3730* [Questionnaire](questionnaire.html): The questionnaire publication date 3731* [Requirements](requirements.html): The requirements publication date 3732* [SearchParameter](searchparameter.html): The search parameter publication date 3733* [StructureDefinition](structuredefinition.html): The structure definition publication date 3734* [StructureMap](structuremap.html): The structure map publication date 3735* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3736* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3737* [TestScript](testscript.html): The test script publication date 3738* [ValueSet](valueset.html): The value set publication date 3739</b><br> 3740 * Type: <b>date</b><br> 3741 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3742 * </p> 3743 */ 3744 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3745 public static final String SP_DATE = "date"; 3746 /** 3747 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3748 * <p> 3749 * Description: <b>Multiple Resources: 3750 3751* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3752* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3753* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3754* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3755* [Citation](citation.html): The citation publication date 3756* [CodeSystem](codesystem.html): The code system publication date 3757* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3758* [ConceptMap](conceptmap.html): The concept map publication date 3759* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3760* [EventDefinition](eventdefinition.html): The event definition publication date 3761* [Evidence](evidence.html): The evidence publication date 3762* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3763* [ExampleScenario](examplescenario.html): The example scenario publication date 3764* [GraphDefinition](graphdefinition.html): The graph definition publication date 3765* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3766* [Library](library.html): The library publication date 3767* [Measure](measure.html): The measure publication date 3768* [MessageDefinition](messagedefinition.html): The message definition publication date 3769* [NamingSystem](namingsystem.html): The naming system publication date 3770* [OperationDefinition](operationdefinition.html): The operation definition publication date 3771* [PlanDefinition](plandefinition.html): The plan definition publication date 3772* [Questionnaire](questionnaire.html): The questionnaire publication date 3773* [Requirements](requirements.html): The requirements publication date 3774* [SearchParameter](searchparameter.html): The search parameter publication date 3775* [StructureDefinition](structuredefinition.html): The structure definition publication date 3776* [StructureMap](structuremap.html): The structure map publication date 3777* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3778* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3779* [TestScript](testscript.html): The test script publication date 3780* [ValueSet](valueset.html): The value set publication date 3781</b><br> 3782 * Type: <b>date</b><br> 3783 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3784 * </p> 3785 */ 3786 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3787 3788 /** 3789 * Search parameter: <b>description</b> 3790 * <p> 3791 * Description: <b>Multiple Resources: 3792 3793* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3794* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3795* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3796* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3797* [Citation](citation.html): The description of the citation 3798* [CodeSystem](codesystem.html): The description of the code system 3799* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3800* [ConceptMap](conceptmap.html): The description of the concept map 3801* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3802* [EventDefinition](eventdefinition.html): The description of the event definition 3803* [Evidence](evidence.html): The description of the evidence 3804* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3805* [GraphDefinition](graphdefinition.html): The description of the graph definition 3806* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3807* [Library](library.html): The description of the library 3808* [Measure](measure.html): The description of the measure 3809* [MessageDefinition](messagedefinition.html): The description of the message definition 3810* [NamingSystem](namingsystem.html): The description of the naming system 3811* [OperationDefinition](operationdefinition.html): The description of the operation definition 3812* [PlanDefinition](plandefinition.html): The description of the plan definition 3813* [Questionnaire](questionnaire.html): The description of the questionnaire 3814* [Requirements](requirements.html): The description of the requirements 3815* [SearchParameter](searchparameter.html): The description of the search parameter 3816* [StructureDefinition](structuredefinition.html): The description of the structure definition 3817* [StructureMap](structuremap.html): The description of the structure map 3818* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3819* [TestScript](testscript.html): The description of the test script 3820* [ValueSet](valueset.html): The description of the value set 3821</b><br> 3822 * Type: <b>string</b><br> 3823 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3824 * </p> 3825 */ 3826 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3827 public static final String SP_DESCRIPTION = "description"; 3828 /** 3829 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3830 * <p> 3831 * Description: <b>Multiple Resources: 3832 3833* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3834* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3835* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3836* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3837* [Citation](citation.html): The description of the citation 3838* [CodeSystem](codesystem.html): The description of the code system 3839* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3840* [ConceptMap](conceptmap.html): The description of the concept map 3841* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3842* [EventDefinition](eventdefinition.html): The description of the event definition 3843* [Evidence](evidence.html): The description of the evidence 3844* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3845* [GraphDefinition](graphdefinition.html): The description of the graph definition 3846* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3847* [Library](library.html): The description of the library 3848* [Measure](measure.html): The description of the measure 3849* [MessageDefinition](messagedefinition.html): The description of the message definition 3850* [NamingSystem](namingsystem.html): The description of the naming system 3851* [OperationDefinition](operationdefinition.html): The description of the operation definition 3852* [PlanDefinition](plandefinition.html): The description of the plan definition 3853* [Questionnaire](questionnaire.html): The description of the questionnaire 3854* [Requirements](requirements.html): The description of the requirements 3855* [SearchParameter](searchparameter.html): The description of the search parameter 3856* [StructureDefinition](structuredefinition.html): The description of the structure definition 3857* [StructureMap](structuremap.html): The description of the structure map 3858* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3859* [TestScript](testscript.html): The description of the test script 3860* [ValueSet](valueset.html): The description of the value set 3861</b><br> 3862 * Type: <b>string</b><br> 3863 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3864 * </p> 3865 */ 3866 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3867 3868 /** 3869 * Search parameter: <b>identifier</b> 3870 * <p> 3871 * Description: <b>Multiple Resources: 3872 3873* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3874* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3875* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3876* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3877* [Citation](citation.html): External identifier for the citation 3878* [CodeSystem](codesystem.html): External identifier for the code system 3879* [ConceptMap](conceptmap.html): External identifier for the concept map 3880* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3881* [EventDefinition](eventdefinition.html): External identifier for the event definition 3882* [Evidence](evidence.html): External identifier for the evidence 3883* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3884* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3885* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3886* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3887* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3888* [Library](library.html): External identifier for the library 3889* [Measure](measure.html): External identifier for the measure 3890* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3891* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3892* [NamingSystem](namingsystem.html): External identifier for the naming system 3893* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3894* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3895* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3896* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3897* [Requirements](requirements.html): External identifier for the requirements 3898* [SearchParameter](searchparameter.html): External identifier for the search parameter 3899* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3900* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3901* [StructureMap](structuremap.html): External identifier for the structure map 3902* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3903* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3904* [TestPlan](testplan.html): An identifier for the test plan 3905* [TestScript](testscript.html): External identifier for the test script 3906* [ValueSet](valueset.html): External identifier for the value set 3907</b><br> 3908 * Type: <b>token</b><br> 3909 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3910 * </p> 3911 */ 3912 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3913 public static final String SP_IDENTIFIER = "identifier"; 3914 /** 3915 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3916 * <p> 3917 * Description: <b>Multiple Resources: 3918 3919* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3920* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3921* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3922* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3923* [Citation](citation.html): External identifier for the citation 3924* [CodeSystem](codesystem.html): External identifier for the code system 3925* [ConceptMap](conceptmap.html): External identifier for the concept map 3926* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3927* [EventDefinition](eventdefinition.html): External identifier for the event definition 3928* [Evidence](evidence.html): External identifier for the evidence 3929* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3930* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3931* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3932* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3933* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3934* [Library](library.html): External identifier for the library 3935* [Measure](measure.html): External identifier for the measure 3936* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3937* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3938* [NamingSystem](namingsystem.html): External identifier for the naming system 3939* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3940* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3941* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3942* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3943* [Requirements](requirements.html): External identifier for the requirements 3944* [SearchParameter](searchparameter.html): External identifier for the search parameter 3945* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3946* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3947* [StructureMap](structuremap.html): External identifier for the structure map 3948* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3949* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3950* [TestPlan](testplan.html): An identifier for the test plan 3951* [TestScript](testscript.html): External identifier for the test script 3952* [ValueSet](valueset.html): External identifier for the value set 3953</b><br> 3954 * Type: <b>token</b><br> 3955 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3956 * </p> 3957 */ 3958 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3959 3960 /** 3961 * Search parameter: <b>jurisdiction</b> 3962 * <p> 3963 * Description: <b>Multiple Resources: 3964 3965* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3966* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3967* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3968* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3969* [Citation](citation.html): Intended jurisdiction for the citation 3970* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3971* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3972* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3973* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3974* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3975* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3976* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3977* [Library](library.html): Intended jurisdiction for the library 3978* [Measure](measure.html): Intended jurisdiction for the measure 3979* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3980* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3981* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3982* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3983* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3984* [Requirements](requirements.html): Intended jurisdiction for the requirements 3985* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3986* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3987* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3988* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3989* [TestScript](testscript.html): Intended jurisdiction for the test script 3990* [ValueSet](valueset.html): Intended jurisdiction for the value set 3991</b><br> 3992 * Type: <b>token</b><br> 3993 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3994 * </p> 3995 */ 3996 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3997 public static final String SP_JURISDICTION = "jurisdiction"; 3998 /** 3999 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4000 * <p> 4001 * Description: <b>Multiple Resources: 4002 4003* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4004* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4005* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4006* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4007* [Citation](citation.html): Intended jurisdiction for the citation 4008* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4009* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4010* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4011* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4012* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4013* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4014* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4015* [Library](library.html): Intended jurisdiction for the library 4016* [Measure](measure.html): Intended jurisdiction for the measure 4017* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4018* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4019* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4020* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4021* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4022* [Requirements](requirements.html): Intended jurisdiction for the requirements 4023* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4024* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4025* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4026* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4027* [TestScript](testscript.html): Intended jurisdiction for the test script 4028* [ValueSet](valueset.html): Intended jurisdiction for the value set 4029</b><br> 4030 * Type: <b>token</b><br> 4031 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4032 * </p> 4033 */ 4034 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4035 4036 /** 4037 * Search parameter: <b>name</b> 4038 * <p> 4039 * Description: <b>Multiple Resources: 4040 4041* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4042* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4043* [Citation](citation.html): Computationally friendly name of the citation 4044* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4045* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4046* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4047* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4048* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4049* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4050* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4051* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4052* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4053* [Library](library.html): Computationally friendly name of the library 4054* [Measure](measure.html): Computationally friendly name of the measure 4055* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4056* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4057* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4058* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4059* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4060* [Requirements](requirements.html): Computationally friendly name of the requirements 4061* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4062* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4063* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4064* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4065* [TestScript](testscript.html): Computationally friendly name of the test script 4066* [ValueSet](valueset.html): Computationally friendly name of the value set 4067</b><br> 4068 * Type: <b>string</b><br> 4069 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4070 * </p> 4071 */ 4072 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 4073 public static final String SP_NAME = "name"; 4074 /** 4075 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4076 * <p> 4077 * Description: <b>Multiple Resources: 4078 4079* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4080* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4081* [Citation](citation.html): Computationally friendly name of the citation 4082* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4083* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4084* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4085* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4086* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4087* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4088* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4089* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4090* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4091* [Library](library.html): Computationally friendly name of the library 4092* [Measure](measure.html): Computationally friendly name of the measure 4093* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4094* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4095* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4096* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4097* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4098* [Requirements](requirements.html): Computationally friendly name of the requirements 4099* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4100* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4101* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4102* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4103* [TestScript](testscript.html): Computationally friendly name of the test script 4104* [ValueSet](valueset.html): Computationally friendly name of the value set 4105</b><br> 4106 * Type: <b>string</b><br> 4107 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4108 * </p> 4109 */ 4110 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4111 4112 /** 4113 * Search parameter: <b>publisher</b> 4114 * <p> 4115 * Description: <b>Multiple Resources: 4116 4117* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4118* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4119* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4120* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4121* [Citation](citation.html): Name of the publisher of the citation 4122* [CodeSystem](codesystem.html): Name of the publisher of the code system 4123* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4124* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4125* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4126* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4127* [Evidence](evidence.html): Name of the publisher of the evidence 4128* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4129* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4130* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4131* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4132* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4133* [Library](library.html): Name of the publisher of the library 4134* [Measure](measure.html): Name of the publisher of the measure 4135* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4136* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4137* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4138* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4139* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4140* [Requirements](requirements.html): Name of the publisher of the requirements 4141* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4142* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4143* [StructureMap](structuremap.html): Name of the publisher of the structure map 4144* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4145* [TestScript](testscript.html): Name of the publisher of the test script 4146* [ValueSet](valueset.html): Name of the publisher of the value set 4147</b><br> 4148 * Type: <b>string</b><br> 4149 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4150 * </p> 4151 */ 4152 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 4153 public static final String SP_PUBLISHER = "publisher"; 4154 /** 4155 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4156 * <p> 4157 * Description: <b>Multiple Resources: 4158 4159* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4160* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4161* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4162* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4163* [Citation](citation.html): Name of the publisher of the citation 4164* [CodeSystem](codesystem.html): Name of the publisher of the code system 4165* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4166* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4167* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4168* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4169* [Evidence](evidence.html): Name of the publisher of the evidence 4170* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4171* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4172* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4173* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4174* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4175* [Library](library.html): Name of the publisher of the library 4176* [Measure](measure.html): Name of the publisher of the measure 4177* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4178* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4179* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4180* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4181* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4182* [Requirements](requirements.html): Name of the publisher of the requirements 4183* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4184* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4185* [StructureMap](structuremap.html): Name of the publisher of the structure map 4186* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4187* [TestScript](testscript.html): Name of the publisher of the test script 4188* [ValueSet](valueset.html): Name of the publisher of the value set 4189</b><br> 4190 * Type: <b>string</b><br> 4191 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4192 * </p> 4193 */ 4194 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4195 4196 /** 4197 * Search parameter: <b>status</b> 4198 * <p> 4199 * Description: <b>Multiple Resources: 4200 4201* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4202* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4203* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4204* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4205* [Citation](citation.html): The current status of the citation 4206* [CodeSystem](codesystem.html): The current status of the code system 4207* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4208* [ConceptMap](conceptmap.html): The current status of the concept map 4209* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4210* [EventDefinition](eventdefinition.html): The current status of the event definition 4211* [Evidence](evidence.html): The current status of the evidence 4212* [EvidenceReport](evidencereport.html): The current status of the evidence report 4213* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4214* [ExampleScenario](examplescenario.html): The current status of the example scenario 4215* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4216* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4217* [Library](library.html): The current status of the library 4218* [Measure](measure.html): The current status of the measure 4219* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4220* [MessageDefinition](messagedefinition.html): The current status of the message definition 4221* [NamingSystem](namingsystem.html): The current status of the naming system 4222* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4223* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4224* [PlanDefinition](plandefinition.html): The current status of the plan definition 4225* [Questionnaire](questionnaire.html): The current status of the questionnaire 4226* [Requirements](requirements.html): The current status of the requirements 4227* [SearchParameter](searchparameter.html): The current status of the search parameter 4228* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4229* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4230* [StructureMap](structuremap.html): The current status of the structure map 4231* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4232* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4233* [TestPlan](testplan.html): The current status of the test plan 4234* [TestScript](testscript.html): The current status of the test script 4235* [ValueSet](valueset.html): The current status of the value set 4236</b><br> 4237 * Type: <b>token</b><br> 4238 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4239 * </p> 4240 */ 4241 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4242 public static final String SP_STATUS = "status"; 4243 /** 4244 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4245 * <p> 4246 * Description: <b>Multiple Resources: 4247 4248* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4249* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4250* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4251* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4252* [Citation](citation.html): The current status of the citation 4253* [CodeSystem](codesystem.html): The current status of the code system 4254* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4255* [ConceptMap](conceptmap.html): The current status of the concept map 4256* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4257* [EventDefinition](eventdefinition.html): The current status of the event definition 4258* [Evidence](evidence.html): The current status of the evidence 4259* [EvidenceReport](evidencereport.html): The current status of the evidence report 4260* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4261* [ExampleScenario](examplescenario.html): The current status of the example scenario 4262* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4263* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4264* [Library](library.html): The current status of the library 4265* [Measure](measure.html): The current status of the measure 4266* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4267* [MessageDefinition](messagedefinition.html): The current status of the message definition 4268* [NamingSystem](namingsystem.html): The current status of the naming system 4269* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4270* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4271* [PlanDefinition](plandefinition.html): The current status of the plan definition 4272* [Questionnaire](questionnaire.html): The current status of the questionnaire 4273* [Requirements](requirements.html): The current status of the requirements 4274* [SearchParameter](searchparameter.html): The current status of the search parameter 4275* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4276* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4277* [StructureMap](structuremap.html): The current status of the structure map 4278* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4279* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4280* [TestPlan](testplan.html): The current status of the test plan 4281* [TestScript](testscript.html): The current status of the test script 4282* [ValueSet](valueset.html): The current status of the value set 4283</b><br> 4284 * Type: <b>token</b><br> 4285 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4286 * </p> 4287 */ 4288 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4289 4290 /** 4291 * Search parameter: <b>url</b> 4292 * <p> 4293 * Description: <b>Multiple Resources: 4294 4295* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4296* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4297* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4298* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4299* [Citation](citation.html): The uri that identifies the citation 4300* [CodeSystem](codesystem.html): The uri that identifies the code system 4301* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4302* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4303* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4304* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4305* [Evidence](evidence.html): The uri that identifies the evidence 4306* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4307* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4308* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4309* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4310* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4311* [Library](library.html): The uri that identifies the library 4312* [Measure](measure.html): The uri that identifies the measure 4313* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4314* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4315* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4316* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4317* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4318* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4319* [Requirements](requirements.html): The uri that identifies the requirements 4320* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4321* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4322* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4323* [StructureMap](structuremap.html): The uri that identifies the structure map 4324* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4325* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4326* [TestPlan](testplan.html): The uri that identifies the test plan 4327* [TestScript](testscript.html): The uri that identifies the test script 4328* [ValueSet](valueset.html): The uri that identifies the value set 4329</b><br> 4330 * Type: <b>uri</b><br> 4331 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4332 * </p> 4333 */ 4334 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4335 public static final String SP_URL = "url"; 4336 /** 4337 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4338 * <p> 4339 * Description: <b>Multiple Resources: 4340 4341* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4342* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4343* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4344* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4345* [Citation](citation.html): The uri that identifies the citation 4346* [CodeSystem](codesystem.html): The uri that identifies the code system 4347* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4348* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4349* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4350* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4351* [Evidence](evidence.html): The uri that identifies the evidence 4352* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4353* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4354* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4355* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4356* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4357* [Library](library.html): The uri that identifies the library 4358* [Measure](measure.html): The uri that identifies the measure 4359* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4360* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4361* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4362* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4363* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4364* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4365* [Requirements](requirements.html): The uri that identifies the requirements 4366* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4367* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4368* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4369* [StructureMap](structuremap.html): The uri that identifies the structure map 4370* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4371* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4372* [TestPlan](testplan.html): The uri that identifies the test plan 4373* [TestScript](testscript.html): The uri that identifies the test script 4374* [ValueSet](valueset.html): The uri that identifies the value set 4375</b><br> 4376 * Type: <b>uri</b><br> 4377 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4378 * </p> 4379 */ 4380 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4381 4382 /** 4383 * Search parameter: <b>version</b> 4384 * <p> 4385 * Description: <b>Multiple Resources: 4386 4387* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4388* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4389* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4390* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4391* [Citation](citation.html): The business version of the citation 4392* [CodeSystem](codesystem.html): The business version of the code system 4393* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4394* [ConceptMap](conceptmap.html): The business version of the concept map 4395* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4396* [EventDefinition](eventdefinition.html): The business version of the event definition 4397* [Evidence](evidence.html): The business version of the evidence 4398* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4399* [ExampleScenario](examplescenario.html): The business version of the example scenario 4400* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4401* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4402* [Library](library.html): The business version of the library 4403* [Measure](measure.html): The business version of the measure 4404* [MessageDefinition](messagedefinition.html): The business version of the message definition 4405* [NamingSystem](namingsystem.html): The business version of the naming system 4406* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4407* [PlanDefinition](plandefinition.html): The business version of the plan definition 4408* [Questionnaire](questionnaire.html): The business version of the questionnaire 4409* [Requirements](requirements.html): The business version of the requirements 4410* [SearchParameter](searchparameter.html): The business version of the search parameter 4411* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4412* [StructureMap](structuremap.html): The business version of the structure map 4413* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4414* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4415* [TestScript](testscript.html): The business version of the test script 4416* [ValueSet](valueset.html): The business version of the value set 4417</b><br> 4418 * Type: <b>token</b><br> 4419 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4420 * </p> 4421 */ 4422 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4423 public static final String SP_VERSION = "version"; 4424 /** 4425 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4426 * <p> 4427 * Description: <b>Multiple Resources: 4428 4429* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4430* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4431* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4432* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4433* [Citation](citation.html): The business version of the citation 4434* [CodeSystem](codesystem.html): The business version of the code system 4435* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4436* [ConceptMap](conceptmap.html): The business version of the concept map 4437* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4438* [EventDefinition](eventdefinition.html): The business version of the event definition 4439* [Evidence](evidence.html): The business version of the evidence 4440* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4441* [ExampleScenario](examplescenario.html): The business version of the example scenario 4442* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4443* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4444* [Library](library.html): The business version of the library 4445* [Measure](measure.html): The business version of the measure 4446* [MessageDefinition](messagedefinition.html): The business version of the message definition 4447* [NamingSystem](namingsystem.html): The business version of the naming system 4448* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4449* [PlanDefinition](plandefinition.html): The business version of the plan definition 4450* [Questionnaire](questionnaire.html): The business version of the questionnaire 4451* [Requirements](requirements.html): The business version of the requirements 4452* [SearchParameter](searchparameter.html): The business version of the search parameter 4453* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4454* [StructureMap](structuremap.html): The business version of the structure map 4455* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4456* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4457* [TestScript](testscript.html): The business version of the test script 4458* [ValueSet](valueset.html): The business version of the value set 4459</b><br> 4460 * Type: <b>token</b><br> 4461 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4462 * </p> 4463 */ 4464 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4465 4466 /** 4467 * Search parameter: <b>derived-from</b> 4468 * <p> 4469 * Description: <b>Multiple Resources: 4470 4471* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4472* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 4473* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 4474* [EventDefinition](eventdefinition.html): What resource is being referenced 4475* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4476* [Library](library.html): What resource is being referenced 4477* [Measure](measure.html): What resource is being referenced 4478* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 4479* [PlanDefinition](plandefinition.html): What resource is being referenced 4480* [ValueSet](valueset.html): A resource that the ValueSet is derived from 4481</b><br> 4482 * Type: <b>reference</b><br> 4483 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 4484 * </p> 4485 */ 4486 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4487 public static final String SP_DERIVED_FROM = "derived-from"; 4488 /** 4489 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4490 * <p> 4491 * Description: <b>Multiple Resources: 4492 4493* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4494* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 4495* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 4496* [EventDefinition](eventdefinition.html): What resource is being referenced 4497* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4498* [Library](library.html): What resource is being referenced 4499* [Measure](measure.html): What resource is being referenced 4500* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 4501* [PlanDefinition](plandefinition.html): What resource is being referenced 4502* [ValueSet](valueset.html): A resource that the ValueSet is derived from 4503</b><br> 4504 * Type: <b>reference</b><br> 4505 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 4506 * </p> 4507 */ 4508 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4509 4510/** 4511 * Constant for fluent queries to be used to add include statements. Specifies 4512 * the path value of "<b>NamingSystem:derived-from</b>". 4513 */ 4514 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("NamingSystem:derived-from").toLocked(); 4515 4516 /** 4517 * Search parameter: <b>effective</b> 4518 * <p> 4519 * Description: <b>Multiple Resources: 4520 4521* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4522* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4523* [Citation](citation.html): The time during which the citation is intended to be in use 4524* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4525* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4526* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4527* [Library](library.html): The time during which the library is intended to be in use 4528* [Measure](measure.html): The time during which the measure is intended to be in use 4529* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4530* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4531* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4532* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4533</b><br> 4534 * Type: <b>date</b><br> 4535 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4536 * </p> 4537 */ 4538 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 4539 public static final String SP_EFFECTIVE = "effective"; 4540 /** 4541 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4542 * <p> 4543 * Description: <b>Multiple Resources: 4544 4545* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4546* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4547* [Citation](citation.html): The time during which the citation is intended to be in use 4548* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4549* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4550* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4551* [Library](library.html): The time during which the library is intended to be in use 4552* [Measure](measure.html): The time during which the measure is intended to be in use 4553* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4554* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4555* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4556* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4557</b><br> 4558 * Type: <b>date</b><br> 4559 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4560 * </p> 4561 */ 4562 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 4563 4564 /** 4565 * Search parameter: <b>predecessor</b> 4566 * <p> 4567 * Description: <b>Multiple Resources: 4568 4569* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4570* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 4571* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 4572* [EventDefinition](eventdefinition.html): What resource is being referenced 4573* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4574* [Library](library.html): What resource is being referenced 4575* [Measure](measure.html): What resource is being referenced 4576* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 4577* [PlanDefinition](plandefinition.html): What resource is being referenced 4578* [ValueSet](valueset.html): The predecessor of the ValueSet 4579</b><br> 4580 * Type: <b>reference</b><br> 4581 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 4582 * </p> 4583 */ 4584 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4585 public static final String SP_PREDECESSOR = "predecessor"; 4586 /** 4587 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 4588 * <p> 4589 * Description: <b>Multiple Resources: 4590 4591* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4592* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 4593* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 4594* [EventDefinition](eventdefinition.html): What resource is being referenced 4595* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4596* [Library](library.html): What resource is being referenced 4597* [Measure](measure.html): What resource is being referenced 4598* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 4599* [PlanDefinition](plandefinition.html): What resource is being referenced 4600* [ValueSet](valueset.html): The predecessor of the ValueSet 4601</b><br> 4602 * Type: <b>reference</b><br> 4603 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 4604 * </p> 4605 */ 4606 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 4607 4608/** 4609 * Constant for fluent queries to be used to add include statements. Specifies 4610 * the path value of "<b>NamingSystem:predecessor</b>". 4611 */ 4612 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("NamingSystem:predecessor").toLocked(); 4613 4614 /** 4615 * Search parameter: <b>topic</b> 4616 * <p> 4617 * Description: <b>Multiple Resources: 4618 4619* [ActivityDefinition](activitydefinition.html): Topics associated with the module 4620* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 4621* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 4622* [EventDefinition](eventdefinition.html): Topics associated with the module 4623* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 4624* [Library](library.html): Topics associated with the module 4625* [Measure](measure.html): Topics associated with the measure 4626* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4627* [PlanDefinition](plandefinition.html): Topics associated with the module 4628* [ValueSet](valueset.html): Topics associated with the ValueSet 4629</b><br> 4630 * Type: <b>token</b><br> 4631 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4632 * </p> 4633 */ 4634 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 4635 public static final String SP_TOPIC = "topic"; 4636 /** 4637 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 4638 * <p> 4639 * Description: <b>Multiple Resources: 4640 4641* [ActivityDefinition](activitydefinition.html): Topics associated with the module 4642* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 4643* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 4644* [EventDefinition](eventdefinition.html): Topics associated with the module 4645* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 4646* [Library](library.html): Topics associated with the module 4647* [Measure](measure.html): Topics associated with the measure 4648* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4649* [PlanDefinition](plandefinition.html): Topics associated with the module 4650* [ValueSet](valueset.html): Topics associated with the ValueSet 4651</b><br> 4652 * Type: <b>token</b><br> 4653 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4654 * </p> 4655 */ 4656 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 4657 4658 /** 4659 * Search parameter: <b>contact</b> 4660 * <p> 4661 * Description: <b>Name of an individual to contact</b><br> 4662 * Type: <b>string</b><br> 4663 * Path: <b>NamingSystem.contact.name</b><br> 4664 * </p> 4665 */ 4666 @SearchParamDefinition(name="contact", path="NamingSystem.contact.name", description="Name of an individual to contact", type="string" ) 4667 public static final String SP_CONTACT = "contact"; 4668 /** 4669 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 4670 * <p> 4671 * Description: <b>Name of an individual to contact</b><br> 4672 * Type: <b>string</b><br> 4673 * Path: <b>NamingSystem.contact.name</b><br> 4674 * </p> 4675 */ 4676 public static final ca.uhn.fhir.rest.gclient.StringClientParam CONTACT = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_CONTACT); 4677 4678 /** 4679 * Search parameter: <b>id-type</b> 4680 * <p> 4681 * Description: <b>oid | uuid | uri | other</b><br> 4682 * Type: <b>token</b><br> 4683 * Path: <b>NamingSystem.uniqueId.type</b><br> 4684 * </p> 4685 */ 4686 @SearchParamDefinition(name="id-type", path="NamingSystem.uniqueId.type", description="oid | uuid | uri | other", type="token" ) 4687 public static final String SP_ID_TYPE = "id-type"; 4688 /** 4689 * <b>Fluent Client</b> search parameter constant for <b>id-type</b> 4690 * <p> 4691 * Description: <b>oid | uuid | uri | other</b><br> 4692 * Type: <b>token</b><br> 4693 * Path: <b>NamingSystem.uniqueId.type</b><br> 4694 * </p> 4695 */ 4696 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ID_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ID_TYPE); 4697 4698 /** 4699 * Search parameter: <b>kind</b> 4700 * <p> 4701 * Description: <b>codesystem | identifier | root</b><br> 4702 * Type: <b>token</b><br> 4703 * Path: <b>NamingSystem.kind</b><br> 4704 * </p> 4705 */ 4706 @SearchParamDefinition(name="kind", path="NamingSystem.kind", description="codesystem | identifier | root", type="token" ) 4707 public static final String SP_KIND = "kind"; 4708 /** 4709 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 4710 * <p> 4711 * Description: <b>codesystem | identifier | root</b><br> 4712 * Type: <b>token</b><br> 4713 * Path: <b>NamingSystem.kind</b><br> 4714 * </p> 4715 */ 4716 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 4717 4718 /** 4719 * Search parameter: <b>period</b> 4720 * <p> 4721 * Description: <b>When is identifier valid?</b><br> 4722 * Type: <b>date</b><br> 4723 * Path: <b>NamingSystem.uniqueId.period</b><br> 4724 * </p> 4725 */ 4726 @SearchParamDefinition(name="period", path="NamingSystem.uniqueId.period", description="When is identifier valid?", type="date" ) 4727 public static final String SP_PERIOD = "period"; 4728 /** 4729 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4730 * <p> 4731 * Description: <b>When is identifier valid?</b><br> 4732 * Type: <b>date</b><br> 4733 * Path: <b>NamingSystem.uniqueId.period</b><br> 4734 * </p> 4735 */ 4736 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 4737 4738 /** 4739 * Search parameter: <b>responsible</b> 4740 * <p> 4741 * Description: <b>Who maintains system namespace?</b><br> 4742 * Type: <b>string</b><br> 4743 * Path: <b>NamingSystem.responsible</b><br> 4744 * </p> 4745 */ 4746 @SearchParamDefinition(name="responsible", path="NamingSystem.responsible", description="Who maintains system namespace?", type="string" ) 4747 public static final String SP_RESPONSIBLE = "responsible"; 4748 /** 4749 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 4750 * <p> 4751 * Description: <b>Who maintains system namespace?</b><br> 4752 * Type: <b>string</b><br> 4753 * Path: <b>NamingSystem.responsible</b><br> 4754 * </p> 4755 */ 4756 public static final ca.uhn.fhir.rest.gclient.StringClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_RESPONSIBLE); 4757 4758 /** 4759 * Search parameter: <b>telecom</b> 4760 * <p> 4761 * Description: <b>Contact details for individual or organization</b><br> 4762 * Type: <b>token</b><br> 4763 * Path: <b>NamingSystem.contact.telecom</b><br> 4764 * </p> 4765 */ 4766 @SearchParamDefinition(name="telecom", path="NamingSystem.contact.telecom", description="Contact details for individual or organization", type="token" ) 4767 public static final String SP_TELECOM = "telecom"; 4768 /** 4769 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 4770 * <p> 4771 * Description: <b>Contact details for individual or organization</b><br> 4772 * Type: <b>token</b><br> 4773 * Path: <b>NamingSystem.contact.telecom</b><br> 4774 * </p> 4775 */ 4776 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 4777 4778 /** 4779 * Search parameter: <b>type</b> 4780 * <p> 4781 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 4782 * Type: <b>token</b><br> 4783 * Path: <b>NamingSystem.type</b><br> 4784 * </p> 4785 */ 4786 @SearchParamDefinition(name="type", path="NamingSystem.type", description="e.g. driver, provider, patient, bank etc.", type="token" ) 4787 public static final String SP_TYPE = "type"; 4788 /** 4789 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4790 * <p> 4791 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 4792 * Type: <b>token</b><br> 4793 * Path: <b>NamingSystem.type</b><br> 4794 * </p> 4795 */ 4796 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4797 4798 /** 4799 * Search parameter: <b>value</b> 4800 * <p> 4801 * Description: <b>The unique identifier</b><br> 4802 * Type: <b>string</b><br> 4803 * Path: <b>NamingSystem.uniqueId.value</b><br> 4804 * </p> 4805 */ 4806 @SearchParamDefinition(name="value", path="NamingSystem.uniqueId.value", description="The unique identifier", type="string" ) 4807 public static final String SP_VALUE = "value"; 4808 /** 4809 * <b>Fluent Client</b> search parameter constant for <b>value</b> 4810 * <p> 4811 * Description: <b>The unique identifier</b><br> 4812 * Type: <b>string</b><br> 4813 * Path: <b>NamingSystem.uniqueId.value</b><br> 4814 * </p> 4815 */ 4816 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VALUE); 4817 4818// Manual code (from Configuration.txt): 4819 public boolean supportsCopyright() { 4820 return true; 4821 } 4822 4823// end addition 4824 4825} 4826