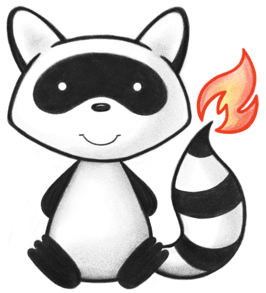
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 052 */ 053@ResourceDef(name="NamingSystem", profile="http://hl7.org/fhir/StructureDefinition/NamingSystem") 054public class NamingSystem extends MetadataResource { 055 056 public enum NamingSystemIdentifierType { 057 /** 058 * An ISO object identifier; e.g. 1.2.3.4.5. 059 */ 060 OID, 061 /** 062 * A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11. 063 */ 064 UUID, 065 /** 066 * A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org. 067 */ 068 URI, 069 /** 070 * An IRI string that can be prepended to the code to obtain a concept IRI for RDF applications. This should be a valid, absolute IRI as defined in RFC 3987. See rdf.html#iri-stem for details on how this value may be used. 071 */ 072 IRISTEM, 073 /** 074 * A short string published by HL7 for use in the V2 family of standsrds to idenfify a code system in the V12 coded data types CWE, CNE, and CF. The code values are also published by HL7 at http://www.hl7.org/Special/committees/vocab/table_0396/index.cfm 075 */ 076 V2CSMNEMONIC, 077 /** 078 * Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC. 079 */ 080 OTHER, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static NamingSystemIdentifierType fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("oid".equals(codeString)) 089 return OID; 090 if ("uuid".equals(codeString)) 091 return UUID; 092 if ("uri".equals(codeString)) 093 return URI; 094 if ("iri-stem".equals(codeString)) 095 return IRISTEM; 096 if ("v2csmnemonic".equals(codeString)) 097 return V2CSMNEMONIC; 098 if ("other".equals(codeString)) 099 return OTHER; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case OID: return "oid"; 108 case UUID: return "uuid"; 109 case URI: return "uri"; 110 case IRISTEM: return "iri-stem"; 111 case V2CSMNEMONIC: return "v2csmnemonic"; 112 case OTHER: return "other"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getSystem() { 118 switch (this) { 119 case OID: return "http://hl7.org/fhir/namingsystem-identifier-type"; 120 case UUID: return "http://hl7.org/fhir/namingsystem-identifier-type"; 121 case URI: return "http://hl7.org/fhir/namingsystem-identifier-type"; 122 case IRISTEM: return "http://hl7.org/fhir/namingsystem-identifier-type"; 123 case V2CSMNEMONIC: return "http://hl7.org/fhir/namingsystem-identifier-type"; 124 case OTHER: return "http://hl7.org/fhir/namingsystem-identifier-type"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDefinition() { 130 switch (this) { 131 case OID: return "An ISO object identifier; e.g. 1.2.3.4.5."; 132 case UUID: return "A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11."; 133 case URI: return "A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org."; 134 case IRISTEM: return "An IRI string that can be prepended to the code to obtain a concept IRI for RDF applications. This should be a valid, absolute IRI as defined in RFC 3987. See rdf.html#iri-stem for details on how this value may be used."; 135 case V2CSMNEMONIC: return "A short string published by HL7 for use in the V2 family of standsrds to idenfify a code system in the V12 coded data types CWE, CNE, and CF. The code values are also published by HL7 at http://www.hl7.org/Special/committees/vocab/table_0396/index.cfm"; 136 case OTHER: return "Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC."; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getDisplay() { 142 switch (this) { 143 case OID: return "OID"; 144 case UUID: return "UUID"; 145 case URI: return "URI"; 146 case IRISTEM: return "IRI stem"; 147 case V2CSMNEMONIC: return "V2CSMNemonic"; 148 case OTHER: return "Other"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 } 154 155 public static class NamingSystemIdentifierTypeEnumFactory implements EnumFactory<NamingSystemIdentifierType> { 156 public NamingSystemIdentifierType fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("oid".equals(codeString)) 161 return NamingSystemIdentifierType.OID; 162 if ("uuid".equals(codeString)) 163 return NamingSystemIdentifierType.UUID; 164 if ("uri".equals(codeString)) 165 return NamingSystemIdentifierType.URI; 166 if ("iri-stem".equals(codeString)) 167 return NamingSystemIdentifierType.IRISTEM; 168 if ("v2csmnemonic".equals(codeString)) 169 return NamingSystemIdentifierType.V2CSMNEMONIC; 170 if ("other".equals(codeString)) 171 return NamingSystemIdentifierType.OTHER; 172 throw new IllegalArgumentException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 173 } 174 public Enumeration<NamingSystemIdentifierType> fromType(PrimitiveType<?> code) throws FHIRException { 175 if (code == null) 176 return null; 177 if (code.isEmpty()) 178 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 179 String codeString = ((PrimitiveType) code).asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 182 if ("oid".equals(codeString)) 183 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OID, code); 184 if ("uuid".equals(codeString)) 185 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.UUID, code); 186 if ("uri".equals(codeString)) 187 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.URI, code); 188 if ("iri-stem".equals(codeString)) 189 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.IRISTEM, code); 190 if ("v2csmnemonic".equals(codeString)) 191 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.V2CSMNEMONIC, code); 192 if ("other".equals(codeString)) 193 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OTHER, code); 194 throw new FHIRException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 195 } 196 public String toCode(NamingSystemIdentifierType code) { 197 if (code == NamingSystemIdentifierType.NULL) 198 return null; 199 if (code == NamingSystemIdentifierType.OID) 200 return "oid"; 201 if (code == NamingSystemIdentifierType.UUID) 202 return "uuid"; 203 if (code == NamingSystemIdentifierType.URI) 204 return "uri"; 205 if (code == NamingSystemIdentifierType.IRISTEM) 206 return "iri-stem"; 207 if (code == NamingSystemIdentifierType.V2CSMNEMONIC) 208 return "v2csmnemonic"; 209 if (code == NamingSystemIdentifierType.OTHER) 210 return "other"; 211 return "?"; 212 } 213 public String toSystem(NamingSystemIdentifierType code) { 214 return code.getSystem(); 215 } 216 } 217 218 public enum NamingSystemType { 219 /** 220 * The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc. 221 */ 222 CODESYSTEM, 223 /** 224 * The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.). 225 */ 226 IDENTIFIER, 227 /** 228 * The naming system is used as the root for other identifiers and naming systems. 229 */ 230 ROOT, 231 /** 232 * added to help the parsers with the generic types 233 */ 234 NULL; 235 public static NamingSystemType fromCode(String codeString) throws FHIRException { 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("codesystem".equals(codeString)) 239 return CODESYSTEM; 240 if ("identifier".equals(codeString)) 241 return IDENTIFIER; 242 if ("root".equals(codeString)) 243 return ROOT; 244 if (Configuration.isAcceptInvalidEnums()) 245 return null; 246 else 247 throw new FHIRException("Unknown NamingSystemType code '"+codeString+"'"); 248 } 249 public String toCode() { 250 switch (this) { 251 case CODESYSTEM: return "codesystem"; 252 case IDENTIFIER: return "identifier"; 253 case ROOT: return "root"; 254 case NULL: return null; 255 default: return "?"; 256 } 257 } 258 public String getSystem() { 259 switch (this) { 260 case CODESYSTEM: return "http://hl7.org/fhir/namingsystem-type"; 261 case IDENTIFIER: return "http://hl7.org/fhir/namingsystem-type"; 262 case ROOT: return "http://hl7.org/fhir/namingsystem-type"; 263 case NULL: return null; 264 default: return "?"; 265 } 266 } 267 public String getDefinition() { 268 switch (this) { 269 case CODESYSTEM: return "The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc."; 270 case IDENTIFIER: return "The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.)."; 271 case ROOT: return "The naming system is used as the root for other identifiers and naming systems."; 272 case NULL: return null; 273 default: return "?"; 274 } 275 } 276 public String getDisplay() { 277 switch (this) { 278 case CODESYSTEM: return "Code System"; 279 case IDENTIFIER: return "Identifier"; 280 case ROOT: return "Root"; 281 case NULL: return null; 282 default: return "?"; 283 } 284 } 285 } 286 287 public static class NamingSystemTypeEnumFactory implements EnumFactory<NamingSystemType> { 288 public NamingSystemType fromCode(String codeString) throws IllegalArgumentException { 289 if (codeString == null || "".equals(codeString)) 290 if (codeString == null || "".equals(codeString)) 291 return null; 292 if ("codesystem".equals(codeString)) 293 return NamingSystemType.CODESYSTEM; 294 if ("identifier".equals(codeString)) 295 return NamingSystemType.IDENTIFIER; 296 if ("root".equals(codeString)) 297 return NamingSystemType.ROOT; 298 throw new IllegalArgumentException("Unknown NamingSystemType code '"+codeString+"'"); 299 } 300 public Enumeration<NamingSystemType> fromType(PrimitiveType<?> code) throws FHIRException { 301 if (code == null) 302 return null; 303 if (code.isEmpty()) 304 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 305 String codeString = ((PrimitiveType) code).asStringValue(); 306 if (codeString == null || "".equals(codeString)) 307 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 308 if ("codesystem".equals(codeString)) 309 return new Enumeration<NamingSystemType>(this, NamingSystemType.CODESYSTEM, code); 310 if ("identifier".equals(codeString)) 311 return new Enumeration<NamingSystemType>(this, NamingSystemType.IDENTIFIER, code); 312 if ("root".equals(codeString)) 313 return new Enumeration<NamingSystemType>(this, NamingSystemType.ROOT, code); 314 throw new FHIRException("Unknown NamingSystemType code '"+codeString+"'"); 315 } 316 public String toCode(NamingSystemType code) { 317 if (code == NamingSystemType.NULL) 318 return null; 319 if (code == NamingSystemType.NULL) 320 return null; 321 if (code == NamingSystemType.CODESYSTEM) 322 return "codesystem"; 323 if (code == NamingSystemType.IDENTIFIER) 324 return "identifier"; 325 if (code == NamingSystemType.ROOT) 326 return "root"; 327 return "?"; 328 } 329 public String toSystem(NamingSystemType code) { 330 return code.getSystem(); 331 } 332 } 333 334 @Block() 335 public static class NamingSystemUniqueIdComponent extends BackboneElement implements IBaseBackboneElement { 336 /** 337 * Identifies the unique identifier scheme used for this particular identifier. 338 */ 339 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 340 @Description(shortDefinition="oid | uuid | uri | iri-stem | v2csmnemonic | other", formalDefinition="Identifies the unique identifier scheme used for this particular identifier." ) 341 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-identifier-type") 342 protected Enumeration<NamingSystemIdentifierType> type; 343 344 /** 345 * The string that should be sent over the wire to identify the code system or identifier system. 346 */ 347 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 348 @Description(shortDefinition="The unique identifier", formalDefinition="The string that should be sent over the wire to identify the code system or identifier system." ) 349 protected StringType value; 350 351 /** 352 * Indicates whether this identifier is the "preferred" identifier of this type. 353 */ 354 @Child(name = "preferred", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 355 @Description(shortDefinition="Is this the id that should be used for this type", formalDefinition="Indicates whether this identifier is the \"preferred\" identifier of this type." ) 356 protected BooleanType preferred; 357 358 /** 359 * Notes about the past or intended usage of this identifier. 360 */ 361 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 362 @Description(shortDefinition="Notes about identifier usage", formalDefinition="Notes about the past or intended usage of this identifier." ) 363 protected StringType comment; 364 365 /** 366 * Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic. 367 */ 368 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=false) 369 @Description(shortDefinition="When is identifier valid?", formalDefinition="Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic." ) 370 protected Period period; 371 372 /** 373 * Indicates whether this identifier ie endorsed by the official owner of the associated naming system. 374 */ 375 @Child(name = "authoritative", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=false) 376 @Description(shortDefinition="Whether the identifier is authoritative", formalDefinition="Indicates whether this identifier ie endorsed by the official owner of the associated naming system." ) 377 protected BooleanType authoritative; 378 379 private static final long serialVersionUID = -166953751L; 380 381 /** 382 * Constructor 383 */ 384 public NamingSystemUniqueIdComponent() { 385 super(); 386 } 387 388 /** 389 * Constructor 390 */ 391 public NamingSystemUniqueIdComponent(NamingSystemIdentifierType type, String value) { 392 super(); 393 this.setType(type); 394 this.setValue(value); 395 } 396 397 /** 398 * @return {@link #type} (Identifies the unique identifier scheme used for this particular identifier.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 399 */ 400 public Enumeration<NamingSystemIdentifierType> getTypeElement() { 401 if (this.type == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.type"); 404 else if (Configuration.doAutoCreate()) 405 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); // bb 406 return this.type; 407 } 408 409 public boolean hasTypeElement() { 410 return this.type != null && !this.type.isEmpty(); 411 } 412 413 public boolean hasType() { 414 return this.type != null && !this.type.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #type} (Identifies the unique identifier scheme used for this particular identifier.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 419 */ 420 public NamingSystemUniqueIdComponent setTypeElement(Enumeration<NamingSystemIdentifierType> value) { 421 this.type = value; 422 return this; 423 } 424 425 /** 426 * @return Identifies the unique identifier scheme used for this particular identifier. 427 */ 428 public NamingSystemIdentifierType getType() { 429 return this.type == null ? null : this.type.getValue(); 430 } 431 432 /** 433 * @param value Identifies the unique identifier scheme used for this particular identifier. 434 */ 435 public NamingSystemUniqueIdComponent setType(NamingSystemIdentifierType value) { 436 if (this.type == null) 437 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); 438 this.type.setValue(value); 439 return this; 440 } 441 442 /** 443 * @return {@link #value} (The string that should be sent over the wire to identify the code system or identifier system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 444 */ 445 public StringType getValueElement() { 446 if (this.value == null) 447 if (Configuration.errorOnAutoCreate()) 448 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.value"); 449 else if (Configuration.doAutoCreate()) 450 this.value = new StringType(); // bb 451 return this.value; 452 } 453 454 public boolean hasValueElement() { 455 return this.value != null && !this.value.isEmpty(); 456 } 457 458 public boolean hasValue() { 459 return this.value != null && !this.value.isEmpty(); 460 } 461 462 /** 463 * @param value {@link #value} (The string that should be sent over the wire to identify the code system or identifier system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 464 */ 465 public NamingSystemUniqueIdComponent setValueElement(StringType value) { 466 this.value = value; 467 return this; 468 } 469 470 /** 471 * @return The string that should be sent over the wire to identify the code system or identifier system. 472 */ 473 public String getValue() { 474 return this.value == null ? null : this.value.getValue(); 475 } 476 477 /** 478 * @param value The string that should be sent over the wire to identify the code system or identifier system. 479 */ 480 public NamingSystemUniqueIdComponent setValue(String value) { 481 if (this.value == null) 482 this.value = new StringType(); 483 this.value.setValue(value); 484 return this; 485 } 486 487 /** 488 * @return {@link #preferred} (Indicates whether this identifier is the "preferred" identifier of this type.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 489 */ 490 public BooleanType getPreferredElement() { 491 if (this.preferred == null) 492 if (Configuration.errorOnAutoCreate()) 493 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.preferred"); 494 else if (Configuration.doAutoCreate()) 495 this.preferred = new BooleanType(); // bb 496 return this.preferred; 497 } 498 499 public boolean hasPreferredElement() { 500 return this.preferred != null && !this.preferred.isEmpty(); 501 } 502 503 public boolean hasPreferred() { 504 return this.preferred != null && !this.preferred.isEmpty(); 505 } 506 507 /** 508 * @param value {@link #preferred} (Indicates whether this identifier is the "preferred" identifier of this type.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 509 */ 510 public NamingSystemUniqueIdComponent setPreferredElement(BooleanType value) { 511 this.preferred = value; 512 return this; 513 } 514 515 /** 516 * @return Indicates whether this identifier is the "preferred" identifier of this type. 517 */ 518 public boolean getPreferred() { 519 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 520 } 521 522 /** 523 * @param value Indicates whether this identifier is the "preferred" identifier of this type. 524 */ 525 public NamingSystemUniqueIdComponent setPreferred(boolean value) { 526 if (this.preferred == null) 527 this.preferred = new BooleanType(); 528 this.preferred.setValue(value); 529 return this; 530 } 531 532 /** 533 * @return {@link #comment} (Notes about the past or intended usage of this identifier.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 534 */ 535 public StringType getCommentElement() { 536 if (this.comment == null) 537 if (Configuration.errorOnAutoCreate()) 538 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.comment"); 539 else if (Configuration.doAutoCreate()) 540 this.comment = new StringType(); // bb 541 return this.comment; 542 } 543 544 public boolean hasCommentElement() { 545 return this.comment != null && !this.comment.isEmpty(); 546 } 547 548 public boolean hasComment() { 549 return this.comment != null && !this.comment.isEmpty(); 550 } 551 552 /** 553 * @param value {@link #comment} (Notes about the past or intended usage of this identifier.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 554 */ 555 public NamingSystemUniqueIdComponent setCommentElement(StringType value) { 556 this.comment = value; 557 return this; 558 } 559 560 /** 561 * @return Notes about the past or intended usage of this identifier. 562 */ 563 public String getComment() { 564 return this.comment == null ? null : this.comment.getValue(); 565 } 566 567 /** 568 * @param value Notes about the past or intended usage of this identifier. 569 */ 570 public NamingSystemUniqueIdComponent setComment(String value) { 571 if (Utilities.noString(value)) 572 this.comment = null; 573 else { 574 if (this.comment == null) 575 this.comment = new StringType(); 576 this.comment.setValue(value); 577 } 578 return this; 579 } 580 581 /** 582 * @return {@link #period} (Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.) 583 */ 584 public Period getPeriod() { 585 if (this.period == null) 586 if (Configuration.errorOnAutoCreate()) 587 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.period"); 588 else if (Configuration.doAutoCreate()) 589 this.period = new Period(); // cc 590 return this.period; 591 } 592 593 public boolean hasPeriod() { 594 return this.period != null && !this.period.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #period} (Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.) 599 */ 600 public NamingSystemUniqueIdComponent setPeriod(Period value) { 601 this.period = value; 602 return this; 603 } 604 605 /** 606 * @return {@link #authoritative} (Indicates whether this identifier ie endorsed by the official owner of the associated naming system.). This is the underlying object with id, value and extensions. The accessor "getAuthoritative" gives direct access to the value 607 */ 608 public BooleanType getAuthoritativeElement() { 609 if (this.authoritative == null) 610 if (Configuration.errorOnAutoCreate()) 611 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.authoritative"); 612 else if (Configuration.doAutoCreate()) 613 this.authoritative = new BooleanType(); // bb 614 return this.authoritative; 615 } 616 617 public boolean hasAuthoritativeElement() { 618 return this.authoritative != null && !this.authoritative.isEmpty(); 619 } 620 621 public boolean hasAuthoritative() { 622 return this.authoritative != null && !this.authoritative.isEmpty(); 623 } 624 625 /** 626 * @param value {@link #authoritative} (Indicates whether this identifier ie endorsed by the official owner of the associated naming system.). This is the underlying object with id, value and extensions. The accessor "getAuthoritative" gives direct access to the value 627 */ 628 public NamingSystemUniqueIdComponent setAuthoritativeElement(BooleanType value) { 629 this.authoritative = value; 630 return this; 631 } 632 633 /** 634 * @return Indicates whether this identifier ie endorsed by the official owner of the associated naming system. 635 */ 636 public boolean getAuthoritative() { 637 return this.authoritative == null || this.authoritative.isEmpty() ? false : this.authoritative.getValue(); 638 } 639 640 /** 641 * @param value Indicates whether this identifier ie endorsed by the official owner of the associated naming system. 642 */ 643 public NamingSystemUniqueIdComponent setAuthoritative(boolean value) { 644 if (this.authoritative == null) 645 this.authoritative = new BooleanType(); 646 this.authoritative.setValue(value); 647 return this; 648 } 649 650 protected void listChildren(List<Property> children) { 651 super.listChildren(children); 652 children.add(new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type)); 653 children.add(new Property("value", "string", "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, value)); 654 children.add(new Property("preferred", "boolean", "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred)); 655 children.add(new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, comment)); 656 children.add(new Property("period", "Period", "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 0, 1, period)); 657 children.add(new Property("authoritative", "boolean", "Indicates whether this identifier ie endorsed by the official owner of the associated naming system.", 0, 1, authoritative)); 658 } 659 660 @Override 661 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 662 switch (_hash) { 663 case 3575610: /*type*/ return new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type); 664 case 111972721: /*value*/ return new Property("value", "string", "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, value); 665 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred); 666 case 950398559: /*comment*/ return new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, comment); 667 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 0, 1, period); 668 case -1557344881: /*authoritative*/ return new Property("authoritative", "boolean", "Indicates whether this identifier ie endorsed by the official owner of the associated naming system.", 0, 1, authoritative); 669 default: return super.getNamedProperty(_hash, _name, _checkValid); 670 } 671 672 } 673 674 @Override 675 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 676 switch (hash) { 677 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<NamingSystemIdentifierType> 678 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 679 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 680 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 681 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 682 case -1557344881: /*authoritative*/ return this.authoritative == null ? new Base[0] : new Base[] {this.authoritative}; // BooleanType 683 default: return super.getProperty(hash, name, checkValid); 684 } 685 686 } 687 688 @Override 689 public Base setProperty(int hash, String name, Base value) throws FHIRException { 690 switch (hash) { 691 case 3575610: // type 692 value = new NamingSystemIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 693 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 694 return value; 695 case 111972721: // value 696 this.value = TypeConvertor.castToString(value); // StringType 697 return value; 698 case -1294005119: // preferred 699 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 700 return value; 701 case 950398559: // comment 702 this.comment = TypeConvertor.castToString(value); // StringType 703 return value; 704 case -991726143: // period 705 this.period = TypeConvertor.castToPeriod(value); // Period 706 return value; 707 case -1557344881: // authoritative 708 this.authoritative = TypeConvertor.castToBoolean(value); // BooleanType 709 return value; 710 default: return super.setProperty(hash, name, value); 711 } 712 713 } 714 715 @Override 716 public Base setProperty(String name, Base value) throws FHIRException { 717 if (name.equals("type")) { 718 value = new NamingSystemIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 719 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 720 } else if (name.equals("value")) { 721 this.value = TypeConvertor.castToString(value); // StringType 722 } else if (name.equals("preferred")) { 723 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 724 } else if (name.equals("comment")) { 725 this.comment = TypeConvertor.castToString(value); // StringType 726 } else if (name.equals("period")) { 727 this.period = TypeConvertor.castToPeriod(value); // Period 728 } else if (name.equals("authoritative")) { 729 this.authoritative = TypeConvertor.castToBoolean(value); // BooleanType 730 } else 731 return super.setProperty(name, value); 732 return value; 733 } 734 735 @Override 736 public void removeChild(String name, Base value) throws FHIRException { 737 if (name.equals("type")) { 738 value = new NamingSystemIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 739 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 740 } else if (name.equals("value")) { 741 this.value = null; 742 } else if (name.equals("preferred")) { 743 this.preferred = null; 744 } else if (name.equals("comment")) { 745 this.comment = null; 746 } else if (name.equals("period")) { 747 this.period = null; 748 } else if (name.equals("authoritative")) { 749 this.authoritative = null; 750 } else 751 super.removeChild(name, value); 752 753 } 754 755 @Override 756 public Base makeProperty(int hash, String name) throws FHIRException { 757 switch (hash) { 758 case 3575610: return getTypeElement(); 759 case 111972721: return getValueElement(); 760 case -1294005119: return getPreferredElement(); 761 case 950398559: return getCommentElement(); 762 case -991726143: return getPeriod(); 763 case -1557344881: return getAuthoritativeElement(); 764 default: return super.makeProperty(hash, name); 765 } 766 767 } 768 769 @Override 770 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 771 switch (hash) { 772 case 3575610: /*type*/ return new String[] {"code"}; 773 case 111972721: /*value*/ return new String[] {"string"}; 774 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 775 case 950398559: /*comment*/ return new String[] {"string"}; 776 case -991726143: /*period*/ return new String[] {"Period"}; 777 case -1557344881: /*authoritative*/ return new String[] {"boolean"}; 778 default: return super.getTypesForProperty(hash, name); 779 } 780 781 } 782 783 @Override 784 public Base addChild(String name) throws FHIRException { 785 if (name.equals("type")) { 786 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.type"); 787 } 788 else if (name.equals("value")) { 789 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.value"); 790 } 791 else if (name.equals("preferred")) { 792 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.preferred"); 793 } 794 else if (name.equals("comment")) { 795 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.comment"); 796 } 797 else if (name.equals("period")) { 798 this.period = new Period(); 799 return this.period; 800 } 801 else if (name.equals("authoritative")) { 802 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.uniqueId.authoritative"); 803 } 804 else 805 return super.addChild(name); 806 } 807 808 public NamingSystemUniqueIdComponent copy() { 809 NamingSystemUniqueIdComponent dst = new NamingSystemUniqueIdComponent(); 810 copyValues(dst); 811 return dst; 812 } 813 814 public void copyValues(NamingSystemUniqueIdComponent dst) { 815 super.copyValues(dst); 816 dst.type = type == null ? null : type.copy(); 817 dst.value = value == null ? null : value.copy(); 818 dst.preferred = preferred == null ? null : preferred.copy(); 819 dst.comment = comment == null ? null : comment.copy(); 820 dst.period = period == null ? null : period.copy(); 821 dst.authoritative = authoritative == null ? null : authoritative.copy(); 822 } 823 824 @Override 825 public boolean equalsDeep(Base other_) { 826 if (!super.equalsDeep(other_)) 827 return false; 828 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 829 return false; 830 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 831 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(preferred, o.preferred, true) 832 && compareDeep(comment, o.comment, true) && compareDeep(period, o.period, true) && compareDeep(authoritative, o.authoritative, true) 833 ; 834 } 835 836 @Override 837 public boolean equalsShallow(Base other_) { 838 if (!super.equalsShallow(other_)) 839 return false; 840 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 841 return false; 842 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 843 return compareValues(type, o.type, true) && compareValues(value, o.value, true) && compareValues(preferred, o.preferred, true) 844 && compareValues(comment, o.comment, true) && compareValues(authoritative, o.authoritative, true); 845 } 846 847 public boolean isEmpty() { 848 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, preferred, comment 849 , period, authoritative); 850 } 851 852 public String fhirType() { 853 return "NamingSystem.uniqueId"; 854 855 } 856 857 } 858 859 /** 860 * An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers. 861 */ 862 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 863 @Description(shortDefinition="Canonical identifier for this naming system, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers." ) 864 protected UriType url; 865 866 /** 867 * A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance. 868 */ 869 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 870 @Description(shortDefinition="Additional identifier for the naming system (business identifier)", formalDefinition="A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 871 protected List<Identifier> identifier; 872 873 /** 874 * The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 875 */ 876 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 877 @Description(shortDefinition="Business version of the naming system", formalDefinition="The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 878 protected StringType version; 879 880 /** 881 * Indicates the mechanism used to compare versions to determine which NamingSystem is more current. 882 */ 883 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 884 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which NamingSystem is more current." ) 885 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 886 protected DataType versionAlgorithm; 887 888 /** 889 * A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 890 */ 891 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 892 @Description(shortDefinition="Name for this naming system (computer friendly)", formalDefinition="A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 893 protected StringType name; 894 895 /** 896 * A short, descriptive, user-friendly title for the naming system. 897 */ 898 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 899 @Description(shortDefinition="Title for this naming system (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the naming system." ) 900 protected StringType title; 901 902 /** 903 * The status of this naming system. Enables tracking the life-cycle of the content. 904 */ 905 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 906 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this naming system. Enables tracking the life-cycle of the content." ) 907 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 908 protected Enumeration<PublicationStatus> status; 909 910 /** 911 * Indicates the purpose for the naming system - what kinds of things does it make unique? 912 */ 913 @Child(name = "kind", type = {CodeType.class}, order=7, min=1, max=1, modifier=false, summary=true) 914 @Description(shortDefinition="codesystem | identifier | root", formalDefinition="Indicates the purpose for the naming system - what kinds of things does it make unique?" ) 915 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-type") 916 protected Enumeration<NamingSystemType> kind; 917 918 /** 919 * A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 920 */ 921 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 922 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 923 protected BooleanType experimental; 924 925 /** 926 * The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 927 */ 928 @Child(name = "date", type = {DateTimeType.class}, order=9, min=1, max=1, modifier=false, summary=true) 929 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes." ) 930 protected DateTimeType date; 931 932 /** 933 * The name of the organization or individual responsible for the release and ongoing maintenance of the naming system. 934 */ 935 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 936 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the naming system." ) 937 protected StringType publisher; 938 939 /** 940 * Contact details to assist a user in finding and communicating with the publisher. 941 */ 942 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 943 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 944 protected List<ContactDetail> contact; 945 946 /** 947 * The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 948 */ 949 @Child(name = "responsible", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 950 @Description(shortDefinition="Who maintains system namespace?", formalDefinition="The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision." ) 951 protected StringType responsible; 952 953 /** 954 * Categorizes a naming system for easier search by grouping related naming systems. 955 */ 956 @Child(name = "type", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 957 @Description(shortDefinition="e.g. driver, provider, patient, bank etc", formalDefinition="Categorizes a naming system for easier search by grouping related naming systems." ) 958 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-identifier-system-type") 959 protected CodeableConcept type; 960 961 /** 962 * A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 963 */ 964 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 965 @Description(shortDefinition="Natural language description of the naming system", formalDefinition="A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc." ) 966 protected MarkdownType description; 967 968 /** 969 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances. 970 */ 971 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 972 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances." ) 973 protected List<UsageContext> useContext; 974 975 /** 976 * A legal or geographic region in which the naming system is intended to be used. 977 */ 978 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 979 @Description(shortDefinition="Intended jurisdiction for naming system (if applicable)", formalDefinition="A legal or geographic region in which the naming system is intended to be used." ) 980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 981 protected List<CodeableConcept> jurisdiction; 982 983 /** 984 * Explanation of why this naming system is needed and why it has been designed as it has. 985 */ 986 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 987 @Description(shortDefinition="Why this naming system is defined", formalDefinition="Explanation of why this naming system is needed and why it has been designed as it has." ) 988 protected MarkdownType purpose; 989 990 /** 991 * A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system. 992 */ 993 @Child(name = "copyright", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 994 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system." ) 995 protected MarkdownType copyright; 996 997 /** 998 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 999 */ 1000 @Child(name = "copyrightLabel", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 1001 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1002 protected StringType copyrightLabel; 1003 1004 /** 1005 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1006 */ 1007 @Child(name = "approvalDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=false) 1008 @Description(shortDefinition="When the NamingSystem was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 1009 protected DateType approvalDate; 1010 1011 /** 1012 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1013 */ 1014 @Child(name = "lastReviewDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1015 @Description(shortDefinition="When the NamingSystem was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 1016 protected DateType lastReviewDate; 1017 1018 /** 1019 * The period during which the NamingSystem content was or is planned to be in active use. 1020 */ 1021 @Child(name = "effectivePeriod", type = {Period.class}, order=22, min=0, max=1, modifier=false, summary=true) 1022 @Description(shortDefinition="When the NamingSystem is expected to be used", formalDefinition="The period during which the NamingSystem content was or is planned to be in active use." ) 1023 protected Period effectivePeriod; 1024 1025 /** 1026 * Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching. 1027 */ 1028 @Child(name = "topic", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1029 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching." ) 1030 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 1031 protected List<CodeableConcept> topic; 1032 1033 /** 1034 * An individiual or organization primarily involved in the creation and maintenance of the NamingSystem. 1035 */ 1036 @Child(name = "author", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1037 @Description(shortDefinition="Who authored the CodeSystem", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the NamingSystem." ) 1038 protected List<ContactDetail> author; 1039 1040 /** 1041 * An individual or organization primarily responsible for internal coherence of the NamingSystem. 1042 */ 1043 @Child(name = "editor", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1044 @Description(shortDefinition="Who edited the NamingSystem", formalDefinition="An individual or organization primarily responsible for internal coherence of the NamingSystem." ) 1045 protected List<ContactDetail> editor; 1046 1047 /** 1048 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem. 1049 */ 1050 @Child(name = "reviewer", type = {ContactDetail.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1051 @Description(shortDefinition="Who reviewed the NamingSystem", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem." ) 1052 protected List<ContactDetail> reviewer; 1053 1054 /** 1055 * An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting. 1056 */ 1057 @Child(name = "endorser", type = {ContactDetail.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1058 @Description(shortDefinition="Who endorsed the NamingSystem", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting." ) 1059 protected List<ContactDetail> endorser; 1060 1061 /** 1062 * Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts. 1063 */ 1064 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1065 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts." ) 1066 protected List<RelatedArtifact> relatedArtifact; 1067 1068 /** 1069 * Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 1070 */ 1071 @Child(name = "usage", type = {StringType.class}, order=29, min=0, max=1, modifier=false, summary=false) 1072 @Description(shortDefinition="How/where is it used", formalDefinition="Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc." ) 1073 protected StringType usage; 1074 1075 /** 1076 * Indicates how the system may be identified when referenced in electronic exchange. 1077 */ 1078 @Child(name = "uniqueId", type = {}, order=30, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1079 @Description(shortDefinition="Unique identifiers used for system", formalDefinition="Indicates how the system may be identified when referenced in electronic exchange." ) 1080 protected List<NamingSystemUniqueIdComponent> uniqueId; 1081 1082 private static final long serialVersionUID = -1403308569L; 1083 1084 /** 1085 * Constructor 1086 */ 1087 public NamingSystem() { 1088 super(); 1089 } 1090 1091 /** 1092 * Constructor 1093 */ 1094 public NamingSystem(String name, PublicationStatus status, NamingSystemType kind, Date date, NamingSystemUniqueIdComponent uniqueId) { 1095 super(); 1096 this.setName(name); 1097 this.setStatus(status); 1098 this.setKind(kind); 1099 this.setDate(date); 1100 this.addUniqueId(uniqueId); 1101 } 1102 1103 /** 1104 * @return {@link #url} (An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1105 */ 1106 public UriType getUrlElement() { 1107 if (this.url == null) 1108 if (Configuration.errorOnAutoCreate()) 1109 throw new Error("Attempt to auto-create NamingSystem.url"); 1110 else if (Configuration.doAutoCreate()) 1111 this.url = new UriType(); // bb 1112 return this.url; 1113 } 1114 1115 public boolean hasUrlElement() { 1116 return this.url != null && !this.url.isEmpty(); 1117 } 1118 1119 public boolean hasUrl() { 1120 return this.url != null && !this.url.isEmpty(); 1121 } 1122 1123 /** 1124 * @param value {@link #url} (An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1125 */ 1126 public NamingSystem setUrlElement(UriType value) { 1127 this.url = value; 1128 return this; 1129 } 1130 1131 /** 1132 * @return An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers. 1133 */ 1134 public String getUrl() { 1135 return this.url == null ? null : this.url.getValue(); 1136 } 1137 1138 /** 1139 * @param value An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers. 1140 */ 1141 public NamingSystem setUrl(String value) { 1142 if (Utilities.noString(value)) 1143 this.url = null; 1144 else { 1145 if (this.url == null) 1146 this.url = new UriType(); 1147 this.url.setValue(value); 1148 } 1149 return this; 1150 } 1151 1152 /** 1153 * @return {@link #identifier} (A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1154 */ 1155 public List<Identifier> getIdentifier() { 1156 if (this.identifier == null) 1157 this.identifier = new ArrayList<Identifier>(); 1158 return this.identifier; 1159 } 1160 1161 /** 1162 * @return Returns a reference to <code>this</code> for easy method chaining 1163 */ 1164 public NamingSystem setIdentifier(List<Identifier> theIdentifier) { 1165 this.identifier = theIdentifier; 1166 return this; 1167 } 1168 1169 public boolean hasIdentifier() { 1170 if (this.identifier == null) 1171 return false; 1172 for (Identifier item : this.identifier) 1173 if (!item.isEmpty()) 1174 return true; 1175 return false; 1176 } 1177 1178 public Identifier addIdentifier() { //3 1179 Identifier t = new Identifier(); 1180 if (this.identifier == null) 1181 this.identifier = new ArrayList<Identifier>(); 1182 this.identifier.add(t); 1183 return t; 1184 } 1185 1186 public NamingSystem addIdentifier(Identifier t) { //3 1187 if (t == null) 1188 return this; 1189 if (this.identifier == null) 1190 this.identifier = new ArrayList<Identifier>(); 1191 this.identifier.add(t); 1192 return this; 1193 } 1194 1195 /** 1196 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1197 */ 1198 public Identifier getIdentifierFirstRep() { 1199 if (getIdentifier().isEmpty()) { 1200 addIdentifier(); 1201 } 1202 return getIdentifier().get(0); 1203 } 1204 1205 /** 1206 * @return {@link #version} (The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1207 */ 1208 public StringType getVersionElement() { 1209 if (this.version == null) 1210 if (Configuration.errorOnAutoCreate()) 1211 throw new Error("Attempt to auto-create NamingSystem.version"); 1212 else if (Configuration.doAutoCreate()) 1213 this.version = new StringType(); // bb 1214 return this.version; 1215 } 1216 1217 public boolean hasVersionElement() { 1218 return this.version != null && !this.version.isEmpty(); 1219 } 1220 1221 public boolean hasVersion() { 1222 return this.version != null && !this.version.isEmpty(); 1223 } 1224 1225 /** 1226 * @param value {@link #version} (The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1227 */ 1228 public NamingSystem setVersionElement(StringType value) { 1229 this.version = value; 1230 return this; 1231 } 1232 1233 /** 1234 * @return The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1235 */ 1236 public String getVersion() { 1237 return this.version == null ? null : this.version.getValue(); 1238 } 1239 1240 /** 1241 * @param value The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1242 */ 1243 public NamingSystem setVersion(String value) { 1244 if (Utilities.noString(value)) 1245 this.version = null; 1246 else { 1247 if (this.version == null) 1248 this.version = new StringType(); 1249 this.version.setValue(value); 1250 } 1251 return this; 1252 } 1253 1254 /** 1255 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1256 */ 1257 public DataType getVersionAlgorithm() { 1258 return this.versionAlgorithm; 1259 } 1260 1261 /** 1262 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1263 */ 1264 public StringType getVersionAlgorithmStringType() throws FHIRException { 1265 if (this.versionAlgorithm == null) 1266 this.versionAlgorithm = new StringType(); 1267 if (!(this.versionAlgorithm instanceof StringType)) 1268 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1269 return (StringType) this.versionAlgorithm; 1270 } 1271 1272 public boolean hasVersionAlgorithmStringType() { 1273 return this.versionAlgorithm instanceof StringType; 1274 } 1275 1276 /** 1277 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1278 */ 1279 public Coding getVersionAlgorithmCoding() throws FHIRException { 1280 if (this.versionAlgorithm == null) 1281 this.versionAlgorithm = new Coding(); 1282 if (!(this.versionAlgorithm instanceof Coding)) 1283 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1284 return (Coding) this.versionAlgorithm; 1285 } 1286 1287 public boolean hasVersionAlgorithmCoding() { 1288 return this.versionAlgorithm instanceof Coding; 1289 } 1290 1291 public boolean hasVersionAlgorithm() { 1292 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1293 } 1294 1295 /** 1296 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which NamingSystem is more current.) 1297 */ 1298 public NamingSystem setVersionAlgorithm(DataType value) { 1299 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1300 throw new FHIRException("Not the right type for NamingSystem.versionAlgorithm[x]: "+value.fhirType()); 1301 this.versionAlgorithm = value; 1302 return this; 1303 } 1304 1305 /** 1306 * @return {@link #name} (A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1307 */ 1308 public StringType getNameElement() { 1309 if (this.name == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create NamingSystem.name"); 1312 else if (Configuration.doAutoCreate()) 1313 this.name = new StringType(); // bb 1314 return this.name; 1315 } 1316 1317 public boolean hasNameElement() { 1318 return this.name != null && !this.name.isEmpty(); 1319 } 1320 1321 public boolean hasName() { 1322 return this.name != null && !this.name.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #name} (A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1327 */ 1328 public NamingSystem setNameElement(StringType value) { 1329 this.name = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1335 */ 1336 public String getName() { 1337 return this.name == null ? null : this.name.getValue(); 1338 } 1339 1340 /** 1341 * @param value A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1342 */ 1343 public NamingSystem setName(String value) { 1344 if (this.name == null) 1345 this.name = new StringType(); 1346 this.name.setValue(value); 1347 return this; 1348 } 1349 1350 /** 1351 * @return {@link #title} (A short, descriptive, user-friendly title for the naming system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1352 */ 1353 public StringType getTitleElement() { 1354 if (this.title == null) 1355 if (Configuration.errorOnAutoCreate()) 1356 throw new Error("Attempt to auto-create NamingSystem.title"); 1357 else if (Configuration.doAutoCreate()) 1358 this.title = new StringType(); // bb 1359 return this.title; 1360 } 1361 1362 public boolean hasTitleElement() { 1363 return this.title != null && !this.title.isEmpty(); 1364 } 1365 1366 public boolean hasTitle() { 1367 return this.title != null && !this.title.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #title} (A short, descriptive, user-friendly title for the naming system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1372 */ 1373 public NamingSystem setTitleElement(StringType value) { 1374 this.title = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return A short, descriptive, user-friendly title for the naming system. 1380 */ 1381 public String getTitle() { 1382 return this.title == null ? null : this.title.getValue(); 1383 } 1384 1385 /** 1386 * @param value A short, descriptive, user-friendly title for the naming system. 1387 */ 1388 public NamingSystem setTitle(String value) { 1389 if (Utilities.noString(value)) 1390 this.title = null; 1391 else { 1392 if (this.title == null) 1393 this.title = new StringType(); 1394 this.title.setValue(value); 1395 } 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #status} (The status of this naming system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1401 */ 1402 public Enumeration<PublicationStatus> getStatusElement() { 1403 if (this.status == null) 1404 if (Configuration.errorOnAutoCreate()) 1405 throw new Error("Attempt to auto-create NamingSystem.status"); 1406 else if (Configuration.doAutoCreate()) 1407 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1408 return this.status; 1409 } 1410 1411 public boolean hasStatusElement() { 1412 return this.status != null && !this.status.isEmpty(); 1413 } 1414 1415 public boolean hasStatus() { 1416 return this.status != null && !this.status.isEmpty(); 1417 } 1418 1419 /** 1420 * @param value {@link #status} (The status of this naming system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1421 */ 1422 public NamingSystem setStatusElement(Enumeration<PublicationStatus> value) { 1423 this.status = value; 1424 return this; 1425 } 1426 1427 /** 1428 * @return The status of this naming system. Enables tracking the life-cycle of the content. 1429 */ 1430 public PublicationStatus getStatus() { 1431 return this.status == null ? null : this.status.getValue(); 1432 } 1433 1434 /** 1435 * @param value The status of this naming system. Enables tracking the life-cycle of the content. 1436 */ 1437 public NamingSystem setStatus(PublicationStatus value) { 1438 if (this.status == null) 1439 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1440 this.status.setValue(value); 1441 return this; 1442 } 1443 1444 /** 1445 * @return {@link #kind} (Indicates the purpose for the naming system - what kinds of things does it make unique?). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1446 */ 1447 public Enumeration<NamingSystemType> getKindElement() { 1448 if (this.kind == null) 1449 if (Configuration.errorOnAutoCreate()) 1450 throw new Error("Attempt to auto-create NamingSystem.kind"); 1451 else if (Configuration.doAutoCreate()) 1452 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); // bb 1453 return this.kind; 1454 } 1455 1456 public boolean hasKindElement() { 1457 return this.kind != null && !this.kind.isEmpty(); 1458 } 1459 1460 public boolean hasKind() { 1461 return this.kind != null && !this.kind.isEmpty(); 1462 } 1463 1464 /** 1465 * @param value {@link #kind} (Indicates the purpose for the naming system - what kinds of things does it make unique?). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1466 */ 1467 public NamingSystem setKindElement(Enumeration<NamingSystemType> value) { 1468 this.kind = value; 1469 return this; 1470 } 1471 1472 /** 1473 * @return Indicates the purpose for the naming system - what kinds of things does it make unique? 1474 */ 1475 public NamingSystemType getKind() { 1476 return this.kind == null ? null : this.kind.getValue(); 1477 } 1478 1479 /** 1480 * @param value Indicates the purpose for the naming system - what kinds of things does it make unique? 1481 */ 1482 public NamingSystem setKind(NamingSystemType value) { 1483 if (this.kind == null) 1484 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); 1485 this.kind.setValue(value); 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #experimental} (A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1491 */ 1492 public BooleanType getExperimentalElement() { 1493 if (this.experimental == null) 1494 if (Configuration.errorOnAutoCreate()) 1495 throw new Error("Attempt to auto-create NamingSystem.experimental"); 1496 else if (Configuration.doAutoCreate()) 1497 this.experimental = new BooleanType(); // bb 1498 return this.experimental; 1499 } 1500 1501 public boolean hasExperimentalElement() { 1502 return this.experimental != null && !this.experimental.isEmpty(); 1503 } 1504 1505 public boolean hasExperimental() { 1506 return this.experimental != null && !this.experimental.isEmpty(); 1507 } 1508 1509 /** 1510 * @param value {@link #experimental} (A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1511 */ 1512 public NamingSystem setExperimentalElement(BooleanType value) { 1513 this.experimental = value; 1514 return this; 1515 } 1516 1517 /** 1518 * @return A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1519 */ 1520 public boolean getExperimental() { 1521 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1522 } 1523 1524 /** 1525 * @param value A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1526 */ 1527 public NamingSystem setExperimental(boolean value) { 1528 if (this.experimental == null) 1529 this.experimental = new BooleanType(); 1530 this.experimental.setValue(value); 1531 return this; 1532 } 1533 1534 /** 1535 * @return {@link #date} (The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1536 */ 1537 public DateTimeType getDateElement() { 1538 if (this.date == null) 1539 if (Configuration.errorOnAutoCreate()) 1540 throw new Error("Attempt to auto-create NamingSystem.date"); 1541 else if (Configuration.doAutoCreate()) 1542 this.date = new DateTimeType(); // bb 1543 return this.date; 1544 } 1545 1546 public boolean hasDateElement() { 1547 return this.date != null && !this.date.isEmpty(); 1548 } 1549 1550 public boolean hasDate() { 1551 return this.date != null && !this.date.isEmpty(); 1552 } 1553 1554 /** 1555 * @param value {@link #date} (The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1556 */ 1557 public NamingSystem setDateElement(DateTimeType value) { 1558 this.date = value; 1559 return this; 1560 } 1561 1562 /** 1563 * @return The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 1564 */ 1565 public Date getDate() { 1566 return this.date == null ? null : this.date.getValue(); 1567 } 1568 1569 /** 1570 * @param value The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 1571 */ 1572 public NamingSystem setDate(Date value) { 1573 if (this.date == null) 1574 this.date = new DateTimeType(); 1575 this.date.setValue(value); 1576 return this; 1577 } 1578 1579 /** 1580 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1581 */ 1582 public StringType getPublisherElement() { 1583 if (this.publisher == null) 1584 if (Configuration.errorOnAutoCreate()) 1585 throw new Error("Attempt to auto-create NamingSystem.publisher"); 1586 else if (Configuration.doAutoCreate()) 1587 this.publisher = new StringType(); // bb 1588 return this.publisher; 1589 } 1590 1591 public boolean hasPublisherElement() { 1592 return this.publisher != null && !this.publisher.isEmpty(); 1593 } 1594 1595 public boolean hasPublisher() { 1596 return this.publisher != null && !this.publisher.isEmpty(); 1597 } 1598 1599 /** 1600 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1601 */ 1602 public NamingSystem setPublisherElement(StringType value) { 1603 this.publisher = value; 1604 return this; 1605 } 1606 1607 /** 1608 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the naming system. 1609 */ 1610 public String getPublisher() { 1611 return this.publisher == null ? null : this.publisher.getValue(); 1612 } 1613 1614 /** 1615 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the naming system. 1616 */ 1617 public NamingSystem setPublisher(String value) { 1618 if (Utilities.noString(value)) 1619 this.publisher = null; 1620 else { 1621 if (this.publisher == null) 1622 this.publisher = new StringType(); 1623 this.publisher.setValue(value); 1624 } 1625 return this; 1626 } 1627 1628 /** 1629 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1630 */ 1631 public List<ContactDetail> getContact() { 1632 if (this.contact == null) 1633 this.contact = new ArrayList<ContactDetail>(); 1634 return this.contact; 1635 } 1636 1637 /** 1638 * @return Returns a reference to <code>this</code> for easy method chaining 1639 */ 1640 public NamingSystem setContact(List<ContactDetail> theContact) { 1641 this.contact = theContact; 1642 return this; 1643 } 1644 1645 public boolean hasContact() { 1646 if (this.contact == null) 1647 return false; 1648 for (ContactDetail item : this.contact) 1649 if (!item.isEmpty()) 1650 return true; 1651 return false; 1652 } 1653 1654 public ContactDetail addContact() { //3 1655 ContactDetail t = new ContactDetail(); 1656 if (this.contact == null) 1657 this.contact = new ArrayList<ContactDetail>(); 1658 this.contact.add(t); 1659 return t; 1660 } 1661 1662 public NamingSystem addContact(ContactDetail t) { //3 1663 if (t == null) 1664 return this; 1665 if (this.contact == null) 1666 this.contact = new ArrayList<ContactDetail>(); 1667 this.contact.add(t); 1668 return this; 1669 } 1670 1671 /** 1672 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1673 */ 1674 public ContactDetail getContactFirstRep() { 1675 if (getContact().isEmpty()) { 1676 addContact(); 1677 } 1678 return getContact().get(0); 1679 } 1680 1681 /** 1682 * @return {@link #responsible} (The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1683 */ 1684 public StringType getResponsibleElement() { 1685 if (this.responsible == null) 1686 if (Configuration.errorOnAutoCreate()) 1687 throw new Error("Attempt to auto-create NamingSystem.responsible"); 1688 else if (Configuration.doAutoCreate()) 1689 this.responsible = new StringType(); // bb 1690 return this.responsible; 1691 } 1692 1693 public boolean hasResponsibleElement() { 1694 return this.responsible != null && !this.responsible.isEmpty(); 1695 } 1696 1697 public boolean hasResponsible() { 1698 return this.responsible != null && !this.responsible.isEmpty(); 1699 } 1700 1701 /** 1702 * @param value {@link #responsible} (The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1703 */ 1704 public NamingSystem setResponsibleElement(StringType value) { 1705 this.responsible = value; 1706 return this; 1707 } 1708 1709 /** 1710 * @return The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 1711 */ 1712 public String getResponsible() { 1713 return this.responsible == null ? null : this.responsible.getValue(); 1714 } 1715 1716 /** 1717 * @param value The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 1718 */ 1719 public NamingSystem setResponsible(String value) { 1720 if (Utilities.noString(value)) 1721 this.responsible = null; 1722 else { 1723 if (this.responsible == null) 1724 this.responsible = new StringType(); 1725 this.responsible.setValue(value); 1726 } 1727 return this; 1728 } 1729 1730 /** 1731 * @return {@link #type} (Categorizes a naming system for easier search by grouping related naming systems.) 1732 */ 1733 public CodeableConcept getType() { 1734 if (this.type == null) 1735 if (Configuration.errorOnAutoCreate()) 1736 throw new Error("Attempt to auto-create NamingSystem.type"); 1737 else if (Configuration.doAutoCreate()) 1738 this.type = new CodeableConcept(); // cc 1739 return this.type; 1740 } 1741 1742 public boolean hasType() { 1743 return this.type != null && !this.type.isEmpty(); 1744 } 1745 1746 /** 1747 * @param value {@link #type} (Categorizes a naming system for easier search by grouping related naming systems.) 1748 */ 1749 public NamingSystem setType(CodeableConcept value) { 1750 this.type = value; 1751 return this; 1752 } 1753 1754 /** 1755 * @return {@link #description} (A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1756 */ 1757 public MarkdownType getDescriptionElement() { 1758 if (this.description == null) 1759 if (Configuration.errorOnAutoCreate()) 1760 throw new Error("Attempt to auto-create NamingSystem.description"); 1761 else if (Configuration.doAutoCreate()) 1762 this.description = new MarkdownType(); // bb 1763 return this.description; 1764 } 1765 1766 public boolean hasDescriptionElement() { 1767 return this.description != null && !this.description.isEmpty(); 1768 } 1769 1770 public boolean hasDescription() { 1771 return this.description != null && !this.description.isEmpty(); 1772 } 1773 1774 /** 1775 * @param value {@link #description} (A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1776 */ 1777 public NamingSystem setDescriptionElement(MarkdownType value) { 1778 this.description = value; 1779 return this; 1780 } 1781 1782 /** 1783 * @return A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 1784 */ 1785 public String getDescription() { 1786 return this.description == null ? null : this.description.getValue(); 1787 } 1788 1789 /** 1790 * @param value A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 1791 */ 1792 public NamingSystem setDescription(String value) { 1793 if (Utilities.noString(value)) 1794 this.description = null; 1795 else { 1796 if (this.description == null) 1797 this.description = new MarkdownType(); 1798 this.description.setValue(value); 1799 } 1800 return this; 1801 } 1802 1803 /** 1804 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.) 1805 */ 1806 public List<UsageContext> getUseContext() { 1807 if (this.useContext == null) 1808 this.useContext = new ArrayList<UsageContext>(); 1809 return this.useContext; 1810 } 1811 1812 /** 1813 * @return Returns a reference to <code>this</code> for easy method chaining 1814 */ 1815 public NamingSystem setUseContext(List<UsageContext> theUseContext) { 1816 this.useContext = theUseContext; 1817 return this; 1818 } 1819 1820 public boolean hasUseContext() { 1821 if (this.useContext == null) 1822 return false; 1823 for (UsageContext item : this.useContext) 1824 if (!item.isEmpty()) 1825 return true; 1826 return false; 1827 } 1828 1829 public UsageContext addUseContext() { //3 1830 UsageContext t = new UsageContext(); 1831 if (this.useContext == null) 1832 this.useContext = new ArrayList<UsageContext>(); 1833 this.useContext.add(t); 1834 return t; 1835 } 1836 1837 public NamingSystem addUseContext(UsageContext t) { //3 1838 if (t == null) 1839 return this; 1840 if (this.useContext == null) 1841 this.useContext = new ArrayList<UsageContext>(); 1842 this.useContext.add(t); 1843 return this; 1844 } 1845 1846 /** 1847 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1848 */ 1849 public UsageContext getUseContextFirstRep() { 1850 if (getUseContext().isEmpty()) { 1851 addUseContext(); 1852 } 1853 return getUseContext().get(0); 1854 } 1855 1856 /** 1857 * @return {@link #jurisdiction} (A legal or geographic region in which the naming system is intended to be used.) 1858 */ 1859 public List<CodeableConcept> getJurisdiction() { 1860 if (this.jurisdiction == null) 1861 this.jurisdiction = new ArrayList<CodeableConcept>(); 1862 return this.jurisdiction; 1863 } 1864 1865 /** 1866 * @return Returns a reference to <code>this</code> for easy method chaining 1867 */ 1868 public NamingSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 1869 this.jurisdiction = theJurisdiction; 1870 return this; 1871 } 1872 1873 public boolean hasJurisdiction() { 1874 if (this.jurisdiction == null) 1875 return false; 1876 for (CodeableConcept item : this.jurisdiction) 1877 if (!item.isEmpty()) 1878 return true; 1879 return false; 1880 } 1881 1882 public CodeableConcept addJurisdiction() { //3 1883 CodeableConcept t = new CodeableConcept(); 1884 if (this.jurisdiction == null) 1885 this.jurisdiction = new ArrayList<CodeableConcept>(); 1886 this.jurisdiction.add(t); 1887 return t; 1888 } 1889 1890 public NamingSystem addJurisdiction(CodeableConcept t) { //3 1891 if (t == null) 1892 return this; 1893 if (this.jurisdiction == null) 1894 this.jurisdiction = new ArrayList<CodeableConcept>(); 1895 this.jurisdiction.add(t); 1896 return this; 1897 } 1898 1899 /** 1900 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1901 */ 1902 public CodeableConcept getJurisdictionFirstRep() { 1903 if (getJurisdiction().isEmpty()) { 1904 addJurisdiction(); 1905 } 1906 return getJurisdiction().get(0); 1907 } 1908 1909 /** 1910 * @return {@link #purpose} (Explanation of why this naming system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1911 */ 1912 public MarkdownType getPurposeElement() { 1913 if (this.purpose == null) 1914 if (Configuration.errorOnAutoCreate()) 1915 throw new Error("Attempt to auto-create NamingSystem.purpose"); 1916 else if (Configuration.doAutoCreate()) 1917 this.purpose = new MarkdownType(); // bb 1918 return this.purpose; 1919 } 1920 1921 public boolean hasPurposeElement() { 1922 return this.purpose != null && !this.purpose.isEmpty(); 1923 } 1924 1925 public boolean hasPurpose() { 1926 return this.purpose != null && !this.purpose.isEmpty(); 1927 } 1928 1929 /** 1930 * @param value {@link #purpose} (Explanation of why this naming system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1931 */ 1932 public NamingSystem setPurposeElement(MarkdownType value) { 1933 this.purpose = value; 1934 return this; 1935 } 1936 1937 /** 1938 * @return Explanation of why this naming system is needed and why it has been designed as it has. 1939 */ 1940 public String getPurpose() { 1941 return this.purpose == null ? null : this.purpose.getValue(); 1942 } 1943 1944 /** 1945 * @param value Explanation of why this naming system is needed and why it has been designed as it has. 1946 */ 1947 public NamingSystem setPurpose(String value) { 1948 if (Utilities.noString(value)) 1949 this.purpose = null; 1950 else { 1951 if (this.purpose == null) 1952 this.purpose = new MarkdownType(); 1953 this.purpose.setValue(value); 1954 } 1955 return this; 1956 } 1957 1958 /** 1959 * @return {@link #copyright} (A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1960 */ 1961 public MarkdownType getCopyrightElement() { 1962 if (this.copyright == null) 1963 if (Configuration.errorOnAutoCreate()) 1964 throw new Error("Attempt to auto-create NamingSystem.copyright"); 1965 else if (Configuration.doAutoCreate()) 1966 this.copyright = new MarkdownType(); // bb 1967 return this.copyright; 1968 } 1969 1970 public boolean hasCopyrightElement() { 1971 return this.copyright != null && !this.copyright.isEmpty(); 1972 } 1973 1974 public boolean hasCopyright() { 1975 return this.copyright != null && !this.copyright.isEmpty(); 1976 } 1977 1978 /** 1979 * @param value {@link #copyright} (A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1980 */ 1981 public NamingSystem setCopyrightElement(MarkdownType value) { 1982 this.copyright = value; 1983 return this; 1984 } 1985 1986 /** 1987 * @return A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system. 1988 */ 1989 public String getCopyright() { 1990 return this.copyright == null ? null : this.copyright.getValue(); 1991 } 1992 1993 /** 1994 * @param value A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system. 1995 */ 1996 public NamingSystem setCopyright(String value) { 1997 if (Utilities.noString(value)) 1998 this.copyright = null; 1999 else { 2000 if (this.copyright == null) 2001 this.copyright = new MarkdownType(); 2002 this.copyright.setValue(value); 2003 } 2004 return this; 2005 } 2006 2007 /** 2008 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2009 */ 2010 public StringType getCopyrightLabelElement() { 2011 if (this.copyrightLabel == null) 2012 if (Configuration.errorOnAutoCreate()) 2013 throw new Error("Attempt to auto-create NamingSystem.copyrightLabel"); 2014 else if (Configuration.doAutoCreate()) 2015 this.copyrightLabel = new StringType(); // bb 2016 return this.copyrightLabel; 2017 } 2018 2019 public boolean hasCopyrightLabelElement() { 2020 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2021 } 2022 2023 public boolean hasCopyrightLabel() { 2024 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2025 } 2026 2027 /** 2028 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2029 */ 2030 public NamingSystem setCopyrightLabelElement(StringType value) { 2031 this.copyrightLabel = value; 2032 return this; 2033 } 2034 2035 /** 2036 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2037 */ 2038 public String getCopyrightLabel() { 2039 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2040 } 2041 2042 /** 2043 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2044 */ 2045 public NamingSystem setCopyrightLabel(String value) { 2046 if (Utilities.noString(value)) 2047 this.copyrightLabel = null; 2048 else { 2049 if (this.copyrightLabel == null) 2050 this.copyrightLabel = new StringType(); 2051 this.copyrightLabel.setValue(value); 2052 } 2053 return this; 2054 } 2055 2056 /** 2057 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2058 */ 2059 public DateType getApprovalDateElement() { 2060 if (this.approvalDate == null) 2061 if (Configuration.errorOnAutoCreate()) 2062 throw new Error("Attempt to auto-create NamingSystem.approvalDate"); 2063 else if (Configuration.doAutoCreate()) 2064 this.approvalDate = new DateType(); // bb 2065 return this.approvalDate; 2066 } 2067 2068 public boolean hasApprovalDateElement() { 2069 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2070 } 2071 2072 public boolean hasApprovalDate() { 2073 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2074 } 2075 2076 /** 2077 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2078 */ 2079 public NamingSystem setApprovalDateElement(DateType value) { 2080 this.approvalDate = value; 2081 return this; 2082 } 2083 2084 /** 2085 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2086 */ 2087 public Date getApprovalDate() { 2088 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2089 } 2090 2091 /** 2092 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2093 */ 2094 public NamingSystem setApprovalDate(Date value) { 2095 if (value == null) 2096 this.approvalDate = null; 2097 else { 2098 if (this.approvalDate == null) 2099 this.approvalDate = new DateType(); 2100 this.approvalDate.setValue(value); 2101 } 2102 return this; 2103 } 2104 2105 /** 2106 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2107 */ 2108 public DateType getLastReviewDateElement() { 2109 if (this.lastReviewDate == null) 2110 if (Configuration.errorOnAutoCreate()) 2111 throw new Error("Attempt to auto-create NamingSystem.lastReviewDate"); 2112 else if (Configuration.doAutoCreate()) 2113 this.lastReviewDate = new DateType(); // bb 2114 return this.lastReviewDate; 2115 } 2116 2117 public boolean hasLastReviewDateElement() { 2118 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2119 } 2120 2121 public boolean hasLastReviewDate() { 2122 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2123 } 2124 2125 /** 2126 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2127 */ 2128 public NamingSystem setLastReviewDateElement(DateType value) { 2129 this.lastReviewDate = value; 2130 return this; 2131 } 2132 2133 /** 2134 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 2135 */ 2136 public Date getLastReviewDate() { 2137 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2138 } 2139 2140 /** 2141 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 2142 */ 2143 public NamingSystem setLastReviewDate(Date value) { 2144 if (value == null) 2145 this.lastReviewDate = null; 2146 else { 2147 if (this.lastReviewDate == null) 2148 this.lastReviewDate = new DateType(); 2149 this.lastReviewDate.setValue(value); 2150 } 2151 return this; 2152 } 2153 2154 /** 2155 * @return {@link #effectivePeriod} (The period during which the NamingSystem content was or is planned to be in active use.) 2156 */ 2157 public Period getEffectivePeriod() { 2158 if (this.effectivePeriod == null) 2159 if (Configuration.errorOnAutoCreate()) 2160 throw new Error("Attempt to auto-create NamingSystem.effectivePeriod"); 2161 else if (Configuration.doAutoCreate()) 2162 this.effectivePeriod = new Period(); // cc 2163 return this.effectivePeriod; 2164 } 2165 2166 public boolean hasEffectivePeriod() { 2167 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2168 } 2169 2170 /** 2171 * @param value {@link #effectivePeriod} (The period during which the NamingSystem content was or is planned to be in active use.) 2172 */ 2173 public NamingSystem setEffectivePeriod(Period value) { 2174 this.effectivePeriod = value; 2175 return this; 2176 } 2177 2178 /** 2179 * @return {@link #topic} (Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching.) 2180 */ 2181 public List<CodeableConcept> getTopic() { 2182 if (this.topic == null) 2183 this.topic = new ArrayList<CodeableConcept>(); 2184 return this.topic; 2185 } 2186 2187 /** 2188 * @return Returns a reference to <code>this</code> for easy method chaining 2189 */ 2190 public NamingSystem setTopic(List<CodeableConcept> theTopic) { 2191 this.topic = theTopic; 2192 return this; 2193 } 2194 2195 public boolean hasTopic() { 2196 if (this.topic == null) 2197 return false; 2198 for (CodeableConcept item : this.topic) 2199 if (!item.isEmpty()) 2200 return true; 2201 return false; 2202 } 2203 2204 public CodeableConcept addTopic() { //3 2205 CodeableConcept t = new CodeableConcept(); 2206 if (this.topic == null) 2207 this.topic = new ArrayList<CodeableConcept>(); 2208 this.topic.add(t); 2209 return t; 2210 } 2211 2212 public NamingSystem addTopic(CodeableConcept t) { //3 2213 if (t == null) 2214 return this; 2215 if (this.topic == null) 2216 this.topic = new ArrayList<CodeableConcept>(); 2217 this.topic.add(t); 2218 return this; 2219 } 2220 2221 /** 2222 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 2223 */ 2224 public CodeableConcept getTopicFirstRep() { 2225 if (getTopic().isEmpty()) { 2226 addTopic(); 2227 } 2228 return getTopic().get(0); 2229 } 2230 2231 /** 2232 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the NamingSystem.) 2233 */ 2234 public List<ContactDetail> getAuthor() { 2235 if (this.author == null) 2236 this.author = new ArrayList<ContactDetail>(); 2237 return this.author; 2238 } 2239 2240 /** 2241 * @return Returns a reference to <code>this</code> for easy method chaining 2242 */ 2243 public NamingSystem setAuthor(List<ContactDetail> theAuthor) { 2244 this.author = theAuthor; 2245 return this; 2246 } 2247 2248 public boolean hasAuthor() { 2249 if (this.author == null) 2250 return false; 2251 for (ContactDetail item : this.author) 2252 if (!item.isEmpty()) 2253 return true; 2254 return false; 2255 } 2256 2257 public ContactDetail addAuthor() { //3 2258 ContactDetail t = new ContactDetail(); 2259 if (this.author == null) 2260 this.author = new ArrayList<ContactDetail>(); 2261 this.author.add(t); 2262 return t; 2263 } 2264 2265 public NamingSystem addAuthor(ContactDetail t) { //3 2266 if (t == null) 2267 return this; 2268 if (this.author == null) 2269 this.author = new ArrayList<ContactDetail>(); 2270 this.author.add(t); 2271 return this; 2272 } 2273 2274 /** 2275 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 2276 */ 2277 public ContactDetail getAuthorFirstRep() { 2278 if (getAuthor().isEmpty()) { 2279 addAuthor(); 2280 } 2281 return getAuthor().get(0); 2282 } 2283 2284 /** 2285 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the NamingSystem.) 2286 */ 2287 public List<ContactDetail> getEditor() { 2288 if (this.editor == null) 2289 this.editor = new ArrayList<ContactDetail>(); 2290 return this.editor; 2291 } 2292 2293 /** 2294 * @return Returns a reference to <code>this</code> for easy method chaining 2295 */ 2296 public NamingSystem setEditor(List<ContactDetail> theEditor) { 2297 this.editor = theEditor; 2298 return this; 2299 } 2300 2301 public boolean hasEditor() { 2302 if (this.editor == null) 2303 return false; 2304 for (ContactDetail item : this.editor) 2305 if (!item.isEmpty()) 2306 return true; 2307 return false; 2308 } 2309 2310 public ContactDetail addEditor() { //3 2311 ContactDetail t = new ContactDetail(); 2312 if (this.editor == null) 2313 this.editor = new ArrayList<ContactDetail>(); 2314 this.editor.add(t); 2315 return t; 2316 } 2317 2318 public NamingSystem addEditor(ContactDetail t) { //3 2319 if (t == null) 2320 return this; 2321 if (this.editor == null) 2322 this.editor = new ArrayList<ContactDetail>(); 2323 this.editor.add(t); 2324 return this; 2325 } 2326 2327 /** 2328 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 2329 */ 2330 public ContactDetail getEditorFirstRep() { 2331 if (getEditor().isEmpty()) { 2332 addEditor(); 2333 } 2334 return getEditor().get(0); 2335 } 2336 2337 /** 2338 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem.) 2339 */ 2340 public List<ContactDetail> getReviewer() { 2341 if (this.reviewer == null) 2342 this.reviewer = new ArrayList<ContactDetail>(); 2343 return this.reviewer; 2344 } 2345 2346 /** 2347 * @return Returns a reference to <code>this</code> for easy method chaining 2348 */ 2349 public NamingSystem setReviewer(List<ContactDetail> theReviewer) { 2350 this.reviewer = theReviewer; 2351 return this; 2352 } 2353 2354 public boolean hasReviewer() { 2355 if (this.reviewer == null) 2356 return false; 2357 for (ContactDetail item : this.reviewer) 2358 if (!item.isEmpty()) 2359 return true; 2360 return false; 2361 } 2362 2363 public ContactDetail addReviewer() { //3 2364 ContactDetail t = new ContactDetail(); 2365 if (this.reviewer == null) 2366 this.reviewer = new ArrayList<ContactDetail>(); 2367 this.reviewer.add(t); 2368 return t; 2369 } 2370 2371 public NamingSystem addReviewer(ContactDetail t) { //3 2372 if (t == null) 2373 return this; 2374 if (this.reviewer == null) 2375 this.reviewer = new ArrayList<ContactDetail>(); 2376 this.reviewer.add(t); 2377 return this; 2378 } 2379 2380 /** 2381 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 2382 */ 2383 public ContactDetail getReviewerFirstRep() { 2384 if (getReviewer().isEmpty()) { 2385 addReviewer(); 2386 } 2387 return getReviewer().get(0); 2388 } 2389 2390 /** 2391 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting.) 2392 */ 2393 public List<ContactDetail> getEndorser() { 2394 if (this.endorser == null) 2395 this.endorser = new ArrayList<ContactDetail>(); 2396 return this.endorser; 2397 } 2398 2399 /** 2400 * @return Returns a reference to <code>this</code> for easy method chaining 2401 */ 2402 public NamingSystem setEndorser(List<ContactDetail> theEndorser) { 2403 this.endorser = theEndorser; 2404 return this; 2405 } 2406 2407 public boolean hasEndorser() { 2408 if (this.endorser == null) 2409 return false; 2410 for (ContactDetail item : this.endorser) 2411 if (!item.isEmpty()) 2412 return true; 2413 return false; 2414 } 2415 2416 public ContactDetail addEndorser() { //3 2417 ContactDetail t = new ContactDetail(); 2418 if (this.endorser == null) 2419 this.endorser = new ArrayList<ContactDetail>(); 2420 this.endorser.add(t); 2421 return t; 2422 } 2423 2424 public NamingSystem addEndorser(ContactDetail t) { //3 2425 if (t == null) 2426 return this; 2427 if (this.endorser == null) 2428 this.endorser = new ArrayList<ContactDetail>(); 2429 this.endorser.add(t); 2430 return this; 2431 } 2432 2433 /** 2434 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 2435 */ 2436 public ContactDetail getEndorserFirstRep() { 2437 if (getEndorser().isEmpty()) { 2438 addEndorser(); 2439 } 2440 return getEndorser().get(0); 2441 } 2442 2443 /** 2444 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 2445 */ 2446 public List<RelatedArtifact> getRelatedArtifact() { 2447 if (this.relatedArtifact == null) 2448 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2449 return this.relatedArtifact; 2450 } 2451 2452 /** 2453 * @return Returns a reference to <code>this</code> for easy method chaining 2454 */ 2455 public NamingSystem setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2456 this.relatedArtifact = theRelatedArtifact; 2457 return this; 2458 } 2459 2460 public boolean hasRelatedArtifact() { 2461 if (this.relatedArtifact == null) 2462 return false; 2463 for (RelatedArtifact item : this.relatedArtifact) 2464 if (!item.isEmpty()) 2465 return true; 2466 return false; 2467 } 2468 2469 public RelatedArtifact addRelatedArtifact() { //3 2470 RelatedArtifact t = new RelatedArtifact(); 2471 if (this.relatedArtifact == null) 2472 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2473 this.relatedArtifact.add(t); 2474 return t; 2475 } 2476 2477 public NamingSystem addRelatedArtifact(RelatedArtifact t) { //3 2478 if (t == null) 2479 return this; 2480 if (this.relatedArtifact == null) 2481 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2482 this.relatedArtifact.add(t); 2483 return this; 2484 } 2485 2486 /** 2487 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 2488 */ 2489 public RelatedArtifact getRelatedArtifactFirstRep() { 2490 if (getRelatedArtifact().isEmpty()) { 2491 addRelatedArtifact(); 2492 } 2493 return getRelatedArtifact().get(0); 2494 } 2495 2496 /** 2497 * @return {@link #usage} (Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2498 */ 2499 public StringType getUsageElement() { 2500 if (this.usage == null) 2501 if (Configuration.errorOnAutoCreate()) 2502 throw new Error("Attempt to auto-create NamingSystem.usage"); 2503 else if (Configuration.doAutoCreate()) 2504 this.usage = new StringType(); // bb 2505 return this.usage; 2506 } 2507 2508 public boolean hasUsageElement() { 2509 return this.usage != null && !this.usage.isEmpty(); 2510 } 2511 2512 public boolean hasUsage() { 2513 return this.usage != null && !this.usage.isEmpty(); 2514 } 2515 2516 /** 2517 * @param value {@link #usage} (Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2518 */ 2519 public NamingSystem setUsageElement(StringType value) { 2520 this.usage = value; 2521 return this; 2522 } 2523 2524 /** 2525 * @return Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 2526 */ 2527 public String getUsage() { 2528 return this.usage == null ? null : this.usage.getValue(); 2529 } 2530 2531 /** 2532 * @param value Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 2533 */ 2534 public NamingSystem setUsage(String value) { 2535 if (Utilities.noString(value)) 2536 this.usage = null; 2537 else { 2538 if (this.usage == null) 2539 this.usage = new StringType(); 2540 this.usage.setValue(value); 2541 } 2542 return this; 2543 } 2544 2545 /** 2546 * @return {@link #uniqueId} (Indicates how the system may be identified when referenced in electronic exchange.) 2547 */ 2548 public List<NamingSystemUniqueIdComponent> getUniqueId() { 2549 if (this.uniqueId == null) 2550 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2551 return this.uniqueId; 2552 } 2553 2554 /** 2555 * @return Returns a reference to <code>this</code> for easy method chaining 2556 */ 2557 public NamingSystem setUniqueId(List<NamingSystemUniqueIdComponent> theUniqueId) { 2558 this.uniqueId = theUniqueId; 2559 return this; 2560 } 2561 2562 public boolean hasUniqueId() { 2563 if (this.uniqueId == null) 2564 return false; 2565 for (NamingSystemUniqueIdComponent item : this.uniqueId) 2566 if (!item.isEmpty()) 2567 return true; 2568 return false; 2569 } 2570 2571 public NamingSystemUniqueIdComponent addUniqueId() { //3 2572 NamingSystemUniqueIdComponent t = new NamingSystemUniqueIdComponent(); 2573 if (this.uniqueId == null) 2574 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2575 this.uniqueId.add(t); 2576 return t; 2577 } 2578 2579 public NamingSystem addUniqueId(NamingSystemUniqueIdComponent t) { //3 2580 if (t == null) 2581 return this; 2582 if (this.uniqueId == null) 2583 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2584 this.uniqueId.add(t); 2585 return this; 2586 } 2587 2588 /** 2589 * @return The first repetition of repeating field {@link #uniqueId}, creating it if it does not already exist {3} 2590 */ 2591 public NamingSystemUniqueIdComponent getUniqueIdFirstRep() { 2592 if (getUniqueId().isEmpty()) { 2593 addUniqueId(); 2594 } 2595 return getUniqueId().get(0); 2596 } 2597 2598 protected void listChildren(List<Property> children) { 2599 super.listChildren(children); 2600 children.add(new Property("url", "uri", "An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.", 0, 1, url)); 2601 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2602 children.add(new Property("version", "string", "The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2603 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm)); 2604 children.add(new Property("name", "string", "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2605 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the naming system.", 0, 1, title)); 2606 children.add(new Property("status", "code", "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status)); 2607 children.add(new Property("kind", "code", "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind)); 2608 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2609 children.add(new Property("date", "dateTime", "The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 0, 1, date)); 2610 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.", 0, 1, publisher)); 2611 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2612 children.add(new Property("responsible", "string", "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 0, 1, responsible)); 2613 children.add(new Property("type", "CodeableConcept", "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type)); 2614 children.add(new Property("description", "markdown", "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1, description)); 2615 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2616 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the naming system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2617 children.add(new Property("purpose", "markdown", "Explanation of why this naming system is needed and why it has been designed as it has.", 0, 1, purpose)); 2618 children.add(new Property("copyright", "markdown", "A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.", 0, 1, copyright)); 2619 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2620 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 2621 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 2622 children.add(new Property("effectivePeriod", "Period", "The period during which the NamingSystem content was or is planned to be in active use.", 0, 1, effectivePeriod)); 2623 children.add(new Property("topic", "CodeableConcept", "Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 2624 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, author)); 2625 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, editor)); 2626 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 2627 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 2628 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 2629 children.add(new Property("usage", "string", "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 0, 1, usage)); 2630 children.add(new Property("uniqueId", "", "Indicates how the system may be identified when referenced in electronic exchange.", 0, java.lang.Integer.MAX_VALUE, uniqueId)); 2631 } 2632 2633 @Override 2634 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2635 switch (_hash) { 2636 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this naming system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this naming system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the naming system is stored on different servers.", 0, 1, url); 2637 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this naming system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2638 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the naming system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the naming system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2639 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2640 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2641 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2642 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which NamingSystem is more current.", 0, 1, versionAlgorithm); 2643 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2644 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the naming system.", 0, 1, title); 2645 case -892481550: /*status*/ return new Property("status", "code", "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status); 2646 case 3292052: /*kind*/ return new Property("kind", "code", "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind); 2647 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this naming system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2648 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the naming system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 0, 1, date); 2649 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the naming system.", 0, 1, publisher); 2650 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2651 case 1847674614: /*responsible*/ return new Property("responsible", "string", "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 0, 1, responsible); 2652 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type); 2653 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1, description); 2654 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2655 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the naming system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2656 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this naming system is needed and why it has been designed as it has.", 0, 1, purpose); 2657 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the naming system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the naming system.", 0, 1, copyright); 2658 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2659 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 2660 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 2661 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the NamingSystem content was or is planned to be in active use.", 0, 1, effectivePeriod); 2662 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptions related to the content of the NamingSystem. Topics provide a high-level categorization as well as keywords for the NamingSystem that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 2663 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, author); 2664 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, editor); 2665 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the NamingSystem.", 0, java.lang.Integer.MAX_VALUE, reviewer); 2666 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the NamingSystem for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 2667 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 2668 case 111574433: /*usage*/ return new Property("usage", "string", "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 0, 1, usage); 2669 case -294460212: /*uniqueId*/ return new Property("uniqueId", "", "Indicates how the system may be identified when referenced in electronic exchange.", 0, java.lang.Integer.MAX_VALUE, uniqueId); 2670 default: return super.getNamedProperty(_hash, _name, _checkValid); 2671 } 2672 2673 } 2674 2675 @Override 2676 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2677 switch (hash) { 2678 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2679 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2680 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2681 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2682 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2683 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2684 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2685 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<NamingSystemType> 2686 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2687 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2688 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2689 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2690 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // StringType 2691 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2692 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2693 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2694 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2695 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2696 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2697 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2698 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 2699 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 2700 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 2701 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 2702 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 2703 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 2704 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 2705 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 2706 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2707 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 2708 case -294460212: /*uniqueId*/ return this.uniqueId == null ? new Base[0] : this.uniqueId.toArray(new Base[this.uniqueId.size()]); // NamingSystemUniqueIdComponent 2709 default: return super.getProperty(hash, name, checkValid); 2710 } 2711 2712 } 2713 2714 @Override 2715 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2716 switch (hash) { 2717 case 116079: // url 2718 this.url = TypeConvertor.castToUri(value); // UriType 2719 return value; 2720 case -1618432855: // identifier 2721 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2722 return value; 2723 case 351608024: // version 2724 this.version = TypeConvertor.castToString(value); // StringType 2725 return value; 2726 case 1508158071: // versionAlgorithm 2727 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2728 return value; 2729 case 3373707: // name 2730 this.name = TypeConvertor.castToString(value); // StringType 2731 return value; 2732 case 110371416: // title 2733 this.title = TypeConvertor.castToString(value); // StringType 2734 return value; 2735 case -892481550: // status 2736 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2737 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2738 return value; 2739 case 3292052: // kind 2740 value = new NamingSystemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2741 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 2742 return value; 2743 case -404562712: // experimental 2744 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2745 return value; 2746 case 3076014: // date 2747 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2748 return value; 2749 case 1447404028: // publisher 2750 this.publisher = TypeConvertor.castToString(value); // StringType 2751 return value; 2752 case 951526432: // contact 2753 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2754 return value; 2755 case 1847674614: // responsible 2756 this.responsible = TypeConvertor.castToString(value); // StringType 2757 return value; 2758 case 3575610: // type 2759 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2760 return value; 2761 case -1724546052: // description 2762 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2763 return value; 2764 case -669707736: // useContext 2765 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2766 return value; 2767 case -507075711: // jurisdiction 2768 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2769 return value; 2770 case -220463842: // purpose 2771 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2772 return value; 2773 case 1522889671: // copyright 2774 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2775 return value; 2776 case 765157229: // copyrightLabel 2777 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2778 return value; 2779 case 223539345: // approvalDate 2780 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2781 return value; 2782 case -1687512484: // lastReviewDate 2783 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2784 return value; 2785 case -403934648: // effectivePeriod 2786 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 2787 return value; 2788 case 110546223: // topic 2789 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2790 return value; 2791 case -1406328437: // author 2792 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2793 return value; 2794 case -1307827859: // editor 2795 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2796 return value; 2797 case -261190139: // reviewer 2798 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2799 return value; 2800 case 1740277666: // endorser 2801 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2802 return value; 2803 case 666807069: // relatedArtifact 2804 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 2805 return value; 2806 case 111574433: // usage 2807 this.usage = TypeConvertor.castToString(value); // StringType 2808 return value; 2809 case -294460212: // uniqueId 2810 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); // NamingSystemUniqueIdComponent 2811 return value; 2812 default: return super.setProperty(hash, name, value); 2813 } 2814 2815 } 2816 2817 @Override 2818 public Base setProperty(String name, Base value) throws FHIRException { 2819 if (name.equals("url")) { 2820 this.url = TypeConvertor.castToUri(value); // UriType 2821 } else if (name.equals("identifier")) { 2822 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2823 } else if (name.equals("version")) { 2824 this.version = TypeConvertor.castToString(value); // StringType 2825 } else if (name.equals("versionAlgorithm[x]")) { 2826 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2827 } else if (name.equals("name")) { 2828 this.name = TypeConvertor.castToString(value); // StringType 2829 } else if (name.equals("title")) { 2830 this.title = TypeConvertor.castToString(value); // StringType 2831 } else if (name.equals("status")) { 2832 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2833 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2834 } else if (name.equals("kind")) { 2835 value = new NamingSystemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2836 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 2837 } else if (name.equals("experimental")) { 2838 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2839 } else if (name.equals("date")) { 2840 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2841 } else if (name.equals("publisher")) { 2842 this.publisher = TypeConvertor.castToString(value); // StringType 2843 } else if (name.equals("contact")) { 2844 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2845 } else if (name.equals("responsible")) { 2846 this.responsible = TypeConvertor.castToString(value); // StringType 2847 } else if (name.equals("type")) { 2848 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2849 } else if (name.equals("description")) { 2850 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2851 } else if (name.equals("useContext")) { 2852 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2853 } else if (name.equals("jurisdiction")) { 2854 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2855 } else if (name.equals("purpose")) { 2856 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2857 } else if (name.equals("copyright")) { 2858 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2859 } else if (name.equals("copyrightLabel")) { 2860 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2861 } else if (name.equals("approvalDate")) { 2862 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2863 } else if (name.equals("lastReviewDate")) { 2864 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2865 } else if (name.equals("effectivePeriod")) { 2866 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 2867 } else if (name.equals("topic")) { 2868 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 2869 } else if (name.equals("author")) { 2870 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 2871 } else if (name.equals("editor")) { 2872 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 2873 } else if (name.equals("reviewer")) { 2874 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 2875 } else if (name.equals("endorser")) { 2876 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 2877 } else if (name.equals("relatedArtifact")) { 2878 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 2879 } else if (name.equals("usage")) { 2880 this.usage = TypeConvertor.castToString(value); // StringType 2881 } else if (name.equals("uniqueId")) { 2882 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); 2883 } else 2884 return super.setProperty(name, value); 2885 return value; 2886 } 2887 2888 @Override 2889 public void removeChild(String name, Base value) throws FHIRException { 2890 if (name.equals("url")) { 2891 this.url = null; 2892 } else if (name.equals("identifier")) { 2893 this.getIdentifier().remove(value); 2894 } else if (name.equals("version")) { 2895 this.version = null; 2896 } else if (name.equals("versionAlgorithm[x]")) { 2897 this.versionAlgorithm = null; 2898 } else if (name.equals("name")) { 2899 this.name = null; 2900 } else if (name.equals("title")) { 2901 this.title = null; 2902 } else if (name.equals("status")) { 2903 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2904 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2905 } else if (name.equals("kind")) { 2906 value = new NamingSystemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2907 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 2908 } else if (name.equals("experimental")) { 2909 this.experimental = null; 2910 } else if (name.equals("date")) { 2911 this.date = null; 2912 } else if (name.equals("publisher")) { 2913 this.publisher = null; 2914 } else if (name.equals("contact")) { 2915 this.getContact().remove(value); 2916 } else if (name.equals("responsible")) { 2917 this.responsible = null; 2918 } else if (name.equals("type")) { 2919 this.type = null; 2920 } else if (name.equals("description")) { 2921 this.description = null; 2922 } else if (name.equals("useContext")) { 2923 this.getUseContext().remove(value); 2924 } else if (name.equals("jurisdiction")) { 2925 this.getJurisdiction().remove(value); 2926 } else if (name.equals("purpose")) { 2927 this.purpose = null; 2928 } else if (name.equals("copyright")) { 2929 this.copyright = null; 2930 } else if (name.equals("copyrightLabel")) { 2931 this.copyrightLabel = null; 2932 } else if (name.equals("approvalDate")) { 2933 this.approvalDate = null; 2934 } else if (name.equals("lastReviewDate")) { 2935 this.lastReviewDate = null; 2936 } else if (name.equals("effectivePeriod")) { 2937 this.effectivePeriod = null; 2938 } else if (name.equals("topic")) { 2939 this.getTopic().remove(value); 2940 } else if (name.equals("author")) { 2941 this.getAuthor().remove(value); 2942 } else if (name.equals("editor")) { 2943 this.getEditor().remove(value); 2944 } else if (name.equals("reviewer")) { 2945 this.getReviewer().remove(value); 2946 } else if (name.equals("endorser")) { 2947 this.getEndorser().remove(value); 2948 } else if (name.equals("relatedArtifact")) { 2949 this.getRelatedArtifact().remove(value); 2950 } else if (name.equals("usage")) { 2951 this.usage = null; 2952 } else if (name.equals("uniqueId")) { 2953 this.getUniqueId().remove((NamingSystemUniqueIdComponent) value); 2954 } else 2955 super.removeChild(name, value); 2956 2957 } 2958 2959 @Override 2960 public Base makeProperty(int hash, String name) throws FHIRException { 2961 switch (hash) { 2962 case 116079: return getUrlElement(); 2963 case -1618432855: return addIdentifier(); 2964 case 351608024: return getVersionElement(); 2965 case -115699031: return getVersionAlgorithm(); 2966 case 1508158071: return getVersionAlgorithm(); 2967 case 3373707: return getNameElement(); 2968 case 110371416: return getTitleElement(); 2969 case -892481550: return getStatusElement(); 2970 case 3292052: return getKindElement(); 2971 case -404562712: return getExperimentalElement(); 2972 case 3076014: return getDateElement(); 2973 case 1447404028: return getPublisherElement(); 2974 case 951526432: return addContact(); 2975 case 1847674614: return getResponsibleElement(); 2976 case 3575610: return getType(); 2977 case -1724546052: return getDescriptionElement(); 2978 case -669707736: return addUseContext(); 2979 case -507075711: return addJurisdiction(); 2980 case -220463842: return getPurposeElement(); 2981 case 1522889671: return getCopyrightElement(); 2982 case 765157229: return getCopyrightLabelElement(); 2983 case 223539345: return getApprovalDateElement(); 2984 case -1687512484: return getLastReviewDateElement(); 2985 case -403934648: return getEffectivePeriod(); 2986 case 110546223: return addTopic(); 2987 case -1406328437: return addAuthor(); 2988 case -1307827859: return addEditor(); 2989 case -261190139: return addReviewer(); 2990 case 1740277666: return addEndorser(); 2991 case 666807069: return addRelatedArtifact(); 2992 case 111574433: return getUsageElement(); 2993 case -294460212: return addUniqueId(); 2994 default: return super.makeProperty(hash, name); 2995 } 2996 2997 } 2998 2999 @Override 3000 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3001 switch (hash) { 3002 case 116079: /*url*/ return new String[] {"uri"}; 3003 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3004 case 351608024: /*version*/ return new String[] {"string"}; 3005 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3006 case 3373707: /*name*/ return new String[] {"string"}; 3007 case 110371416: /*title*/ return new String[] {"string"}; 3008 case -892481550: /*status*/ return new String[] {"code"}; 3009 case 3292052: /*kind*/ return new String[] {"code"}; 3010 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3011 case 3076014: /*date*/ return new String[] {"dateTime"}; 3012 case 1447404028: /*publisher*/ return new String[] {"string"}; 3013 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3014 case 1847674614: /*responsible*/ return new String[] {"string"}; 3015 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3016 case -1724546052: /*description*/ return new String[] {"markdown"}; 3017 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3018 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3019 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3020 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3021 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 3022 case 223539345: /*approvalDate*/ return new String[] {"date"}; 3023 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 3024 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 3025 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 3026 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 3027 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 3028 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 3029 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 3030 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 3031 case 111574433: /*usage*/ return new String[] {"string"}; 3032 case -294460212: /*uniqueId*/ return new String[] {}; 3033 default: return super.getTypesForProperty(hash, name); 3034 } 3035 3036 } 3037 3038 @Override 3039 public Base addChild(String name) throws FHIRException { 3040 if (name.equals("url")) { 3041 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.url"); 3042 } 3043 else if (name.equals("identifier")) { 3044 return addIdentifier(); 3045 } 3046 else if (name.equals("version")) { 3047 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.version"); 3048 } 3049 else if (name.equals("versionAlgorithmString")) { 3050 this.versionAlgorithm = new StringType(); 3051 return this.versionAlgorithm; 3052 } 3053 else if (name.equals("versionAlgorithmCoding")) { 3054 this.versionAlgorithm = new Coding(); 3055 return this.versionAlgorithm; 3056 } 3057 else if (name.equals("name")) { 3058 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 3059 } 3060 else if (name.equals("title")) { 3061 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.title"); 3062 } 3063 else if (name.equals("status")) { 3064 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.status"); 3065 } 3066 else if (name.equals("kind")) { 3067 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.kind"); 3068 } 3069 else if (name.equals("experimental")) { 3070 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.experimental"); 3071 } 3072 else if (name.equals("date")) { 3073 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.date"); 3074 } 3075 else if (name.equals("publisher")) { 3076 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.publisher"); 3077 } 3078 else if (name.equals("contact")) { 3079 return addContact(); 3080 } 3081 else if (name.equals("responsible")) { 3082 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.responsible"); 3083 } 3084 else if (name.equals("type")) { 3085 this.type = new CodeableConcept(); 3086 return this.type; 3087 } 3088 else if (name.equals("description")) { 3089 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.description"); 3090 } 3091 else if (name.equals("useContext")) { 3092 return addUseContext(); 3093 } 3094 else if (name.equals("jurisdiction")) { 3095 return addJurisdiction(); 3096 } 3097 else if (name.equals("purpose")) { 3098 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.purpose"); 3099 } 3100 else if (name.equals("copyright")) { 3101 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.copyright"); 3102 } 3103 else if (name.equals("copyrightLabel")) { 3104 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.copyrightLabel"); 3105 } 3106 else if (name.equals("approvalDate")) { 3107 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.approvalDate"); 3108 } 3109 else if (name.equals("lastReviewDate")) { 3110 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.lastReviewDate"); 3111 } 3112 else if (name.equals("effectivePeriod")) { 3113 this.effectivePeriod = new Period(); 3114 return this.effectivePeriod; 3115 } 3116 else if (name.equals("topic")) { 3117 return addTopic(); 3118 } 3119 else if (name.equals("author")) { 3120 return addAuthor(); 3121 } 3122 else if (name.equals("editor")) { 3123 return addEditor(); 3124 } 3125 else if (name.equals("reviewer")) { 3126 return addReviewer(); 3127 } 3128 else if (name.equals("endorser")) { 3129 return addEndorser(); 3130 } 3131 else if (name.equals("relatedArtifact")) { 3132 return addRelatedArtifact(); 3133 } 3134 else if (name.equals("usage")) { 3135 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.usage"); 3136 } 3137 else if (name.equals("uniqueId")) { 3138 return addUniqueId(); 3139 } 3140 else 3141 return super.addChild(name); 3142 } 3143 3144 public String fhirType() { 3145 return "NamingSystem"; 3146 3147 } 3148 3149 public NamingSystem copy() { 3150 NamingSystem dst = new NamingSystem(); 3151 copyValues(dst); 3152 return dst; 3153 } 3154 3155 public void copyValues(NamingSystem dst) { 3156 super.copyValues(dst); 3157 dst.url = url == null ? null : url.copy(); 3158 if (identifier != null) { 3159 dst.identifier = new ArrayList<Identifier>(); 3160 for (Identifier i : identifier) 3161 dst.identifier.add(i.copy()); 3162 }; 3163 dst.version = version == null ? null : version.copy(); 3164 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 3165 dst.name = name == null ? null : name.copy(); 3166 dst.title = title == null ? null : title.copy(); 3167 dst.status = status == null ? null : status.copy(); 3168 dst.kind = kind == null ? null : kind.copy(); 3169 dst.experimental = experimental == null ? null : experimental.copy(); 3170 dst.date = date == null ? null : date.copy(); 3171 dst.publisher = publisher == null ? null : publisher.copy(); 3172 if (contact != null) { 3173 dst.contact = new ArrayList<ContactDetail>(); 3174 for (ContactDetail i : contact) 3175 dst.contact.add(i.copy()); 3176 }; 3177 dst.responsible = responsible == null ? null : responsible.copy(); 3178 dst.type = type == null ? null : type.copy(); 3179 dst.description = description == null ? null : description.copy(); 3180 if (useContext != null) { 3181 dst.useContext = new ArrayList<UsageContext>(); 3182 for (UsageContext i : useContext) 3183 dst.useContext.add(i.copy()); 3184 }; 3185 if (jurisdiction != null) { 3186 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3187 for (CodeableConcept i : jurisdiction) 3188 dst.jurisdiction.add(i.copy()); 3189 }; 3190 dst.purpose = purpose == null ? null : purpose.copy(); 3191 dst.copyright = copyright == null ? null : copyright.copy(); 3192 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 3193 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 3194 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 3195 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 3196 if (topic != null) { 3197 dst.topic = new ArrayList<CodeableConcept>(); 3198 for (CodeableConcept i : topic) 3199 dst.topic.add(i.copy()); 3200 }; 3201 if (author != null) { 3202 dst.author = new ArrayList<ContactDetail>(); 3203 for (ContactDetail i : author) 3204 dst.author.add(i.copy()); 3205 }; 3206 if (editor != null) { 3207 dst.editor = new ArrayList<ContactDetail>(); 3208 for (ContactDetail i : editor) 3209 dst.editor.add(i.copy()); 3210 }; 3211 if (reviewer != null) { 3212 dst.reviewer = new ArrayList<ContactDetail>(); 3213 for (ContactDetail i : reviewer) 3214 dst.reviewer.add(i.copy()); 3215 }; 3216 if (endorser != null) { 3217 dst.endorser = new ArrayList<ContactDetail>(); 3218 for (ContactDetail i : endorser) 3219 dst.endorser.add(i.copy()); 3220 }; 3221 if (relatedArtifact != null) { 3222 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 3223 for (RelatedArtifact i : relatedArtifact) 3224 dst.relatedArtifact.add(i.copy()); 3225 }; 3226 dst.usage = usage == null ? null : usage.copy(); 3227 if (uniqueId != null) { 3228 dst.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 3229 for (NamingSystemUniqueIdComponent i : uniqueId) 3230 dst.uniqueId.add(i.copy()); 3231 }; 3232 } 3233 3234 protected NamingSystem typedCopy() { 3235 return copy(); 3236 } 3237 3238 @Override 3239 public boolean equalsDeep(Base other_) { 3240 if (!super.equalsDeep(other_)) 3241 return false; 3242 if (!(other_ instanceof NamingSystem)) 3243 return false; 3244 NamingSystem o = (NamingSystem) other_; 3245 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 3246 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 3247 && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) && compareDeep(experimental, o.experimental, true) 3248 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 3249 && compareDeep(responsible, o.responsible, true) && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 3250 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 3251 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 3252 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 3253 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 3254 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 3255 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(usage, o.usage, true) && compareDeep(uniqueId, o.uniqueId, true) 3256 ; 3257 } 3258 3259 @Override 3260 public boolean equalsShallow(Base other_) { 3261 if (!super.equalsShallow(other_)) 3262 return false; 3263 if (!(other_ instanceof NamingSystem)) 3264 return false; 3265 NamingSystem o = (NamingSystem) other_; 3266 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 3267 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(kind, o.kind, true) 3268 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 3269 && compareValues(responsible, o.responsible, true) && compareValues(description, o.description, true) 3270 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 3271 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 3272 && compareValues(usage, o.usage, true); 3273 } 3274 3275 public boolean isEmpty() { 3276 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 3277 , versionAlgorithm, name, title, status, kind, experimental, date, publisher 3278 , contact, responsible, type, description, useContext, jurisdiction, purpose, copyright 3279 , copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor 3280 , reviewer, endorser, relatedArtifact, usage, uniqueId); 3281 } 3282 3283 @Override 3284 public ResourceType getResourceType() { 3285 return ResourceType.NamingSystem; 3286 } 3287 3288 /** 3289 * Search parameter: <b>context-quantity</b> 3290 * <p> 3291 * Description: <b>Multiple Resources: 3292 3293* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3294* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3295* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3296* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3297* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3298* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3299* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3300* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3301* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3302* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3303* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3304* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3305* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3306* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3307* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3308* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3309* [Library](library.html): A quantity- or range-valued use context assigned to the library 3310* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3311* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3312* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3313* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3314* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3315* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3316* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3317* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3318* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3319* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3320* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3321* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3322* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3323</b><br> 3324 * Type: <b>quantity</b><br> 3325 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3326 * </p> 3327 */ 3328 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 3329 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3330 /** 3331 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3332 * <p> 3333 * Description: <b>Multiple Resources: 3334 3335* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3336* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3337* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3338* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3339* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3340* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3341* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3342* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3343* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3344* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3345* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3346* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3347* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3348* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3349* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3350* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3351* [Library](library.html): A quantity- or range-valued use context assigned to the library 3352* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3353* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3354* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3355* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3356* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3357* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3358* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3359* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3360* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3361* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3362* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3363* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3364* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3365</b><br> 3366 * Type: <b>quantity</b><br> 3367 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3368 * </p> 3369 */ 3370 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3371 3372 /** 3373 * Search parameter: <b>context-type-quantity</b> 3374 * <p> 3375 * Description: <b>Multiple Resources: 3376 3377* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3378* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3379* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3380* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3381* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3382* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3383* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3384* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3385* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3386* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3387* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3388* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3389* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3390* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3391* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3392* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3393* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3394* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3395* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3396* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3397* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3398* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3399* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3400* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3401* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3402* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3403* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3404* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3405* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3406* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3407</b><br> 3408 * Type: <b>composite</b><br> 3409 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3410 * </p> 3411 */ 3412 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3413 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3414 /** 3415 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3416 * <p> 3417 * Description: <b>Multiple Resources: 3418 3419* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3420* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3421* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3422* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3423* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3424* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3425* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3426* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3427* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3428* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3429* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3430* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3431* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3432* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3433* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3434* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3435* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3436* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3437* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3438* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3439* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3440* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3441* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3442* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3443* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3444* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3445* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3446* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3447* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3448* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3449</b><br> 3450 * Type: <b>composite</b><br> 3451 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3452 * </p> 3453 */ 3454 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3455 3456 /** 3457 * Search parameter: <b>context-type-value</b> 3458 * <p> 3459 * Description: <b>Multiple Resources: 3460 3461* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3462* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3463* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3464* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3465* [Citation](citation.html): A use context type and value assigned to the citation 3466* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3467* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3468* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3469* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3470* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3471* [Evidence](evidence.html): A use context type and value assigned to the evidence 3472* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3473* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3474* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3475* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3476* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3477* [Library](library.html): A use context type and value assigned to the library 3478* [Measure](measure.html): A use context type and value assigned to the measure 3479* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3480* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3481* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3482* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3483* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3484* [Requirements](requirements.html): A use context type and value assigned to the requirements 3485* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3486* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3487* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3488* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3489* [TestScript](testscript.html): A use context type and value assigned to the test script 3490* [ValueSet](valueset.html): A use context type and value assigned to the value set 3491</b><br> 3492 * Type: <b>composite</b><br> 3493 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3494 * </p> 3495 */ 3496 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3497 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3498 /** 3499 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3500 * <p> 3501 * Description: <b>Multiple Resources: 3502 3503* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3504* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3505* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3506* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3507* [Citation](citation.html): A use context type and value assigned to the citation 3508* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3509* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3510* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3511* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3512* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3513* [Evidence](evidence.html): A use context type and value assigned to the evidence 3514* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3515* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3516* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3517* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3518* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3519* [Library](library.html): A use context type and value assigned to the library 3520* [Measure](measure.html): A use context type and value assigned to the measure 3521* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3522* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3523* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3524* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3525* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3526* [Requirements](requirements.html): A use context type and value assigned to the requirements 3527* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3528* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3529* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3530* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3531* [TestScript](testscript.html): A use context type and value assigned to the test script 3532* [ValueSet](valueset.html): A use context type and value assigned to the value set 3533</b><br> 3534 * Type: <b>composite</b><br> 3535 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3536 * </p> 3537 */ 3538 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3539 3540 /** 3541 * Search parameter: <b>context-type</b> 3542 * <p> 3543 * Description: <b>Multiple Resources: 3544 3545* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3546* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3547* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3548* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3549* [Citation](citation.html): A type of use context assigned to the citation 3550* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3551* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3552* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3553* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3554* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3555* [Evidence](evidence.html): A type of use context assigned to the evidence 3556* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3557* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3558* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3559* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3560* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3561* [Library](library.html): A type of use context assigned to the library 3562* [Measure](measure.html): A type of use context assigned to the measure 3563* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3564* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3565* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3566* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3567* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3568* [Requirements](requirements.html): A type of use context assigned to the requirements 3569* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3570* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3571* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3572* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3573* [TestScript](testscript.html): A type of use context assigned to the test script 3574* [ValueSet](valueset.html): A type of use context assigned to the value set 3575</b><br> 3576 * Type: <b>token</b><br> 3577 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3578 * </p> 3579 */ 3580 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3581 public static final String SP_CONTEXT_TYPE = "context-type"; 3582 /** 3583 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3584 * <p> 3585 * Description: <b>Multiple Resources: 3586 3587* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3588* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3589* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3590* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3591* [Citation](citation.html): A type of use context assigned to the citation 3592* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3593* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3594* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3595* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3596* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3597* [Evidence](evidence.html): A type of use context assigned to the evidence 3598* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3599* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3600* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3601* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3602* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3603* [Library](library.html): A type of use context assigned to the library 3604* [Measure](measure.html): A type of use context assigned to the measure 3605* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3606* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3607* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3608* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3609* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3610* [Requirements](requirements.html): A type of use context assigned to the requirements 3611* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3612* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3613* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3614* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3615* [TestScript](testscript.html): A type of use context assigned to the test script 3616* [ValueSet](valueset.html): A type of use context assigned to the value set 3617</b><br> 3618 * Type: <b>token</b><br> 3619 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3620 * </p> 3621 */ 3622 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3623 3624 /** 3625 * Search parameter: <b>context</b> 3626 * <p> 3627 * Description: <b>Multiple Resources: 3628 3629* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3630* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3631* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3632* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3633* [Citation](citation.html): A use context assigned to the citation 3634* [CodeSystem](codesystem.html): A use context assigned to the code system 3635* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3636* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3637* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3638* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3639* [Evidence](evidence.html): A use context assigned to the evidence 3640* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3641* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3642* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3643* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3644* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3645* [Library](library.html): A use context assigned to the library 3646* [Measure](measure.html): A use context assigned to the measure 3647* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3648* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3649* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3650* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3651* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3652* [Requirements](requirements.html): A use context assigned to the requirements 3653* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3654* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3655* [StructureMap](structuremap.html): A use context assigned to the structure map 3656* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3657* [TestScript](testscript.html): A use context assigned to the test script 3658* [ValueSet](valueset.html): A use context assigned to the value set 3659</b><br> 3660 * Type: <b>token</b><br> 3661 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3662 * </p> 3663 */ 3664 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3665 public static final String SP_CONTEXT = "context"; 3666 /** 3667 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3668 * <p> 3669 * Description: <b>Multiple Resources: 3670 3671* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3672* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3673* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3674* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3675* [Citation](citation.html): A use context assigned to the citation 3676* [CodeSystem](codesystem.html): A use context assigned to the code system 3677* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3678* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3679* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3680* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3681* [Evidence](evidence.html): A use context assigned to the evidence 3682* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3683* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3684* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3685* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3686* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3687* [Library](library.html): A use context assigned to the library 3688* [Measure](measure.html): A use context assigned to the measure 3689* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3690* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3691* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3692* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3693* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3694* [Requirements](requirements.html): A use context assigned to the requirements 3695* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3696* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3697* [StructureMap](structuremap.html): A use context assigned to the structure map 3698* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3699* [TestScript](testscript.html): A use context assigned to the test script 3700* [ValueSet](valueset.html): A use context assigned to the value set 3701</b><br> 3702 * Type: <b>token</b><br> 3703 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3704 * </p> 3705 */ 3706 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3707 3708 /** 3709 * Search parameter: <b>date</b> 3710 * <p> 3711 * Description: <b>Multiple Resources: 3712 3713* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3714* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3715* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3716* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3717* [Citation](citation.html): The citation publication date 3718* [CodeSystem](codesystem.html): The code system publication date 3719* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3720* [ConceptMap](conceptmap.html): The concept map publication date 3721* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3722* [EventDefinition](eventdefinition.html): The event definition publication date 3723* [Evidence](evidence.html): The evidence publication date 3724* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3725* [ExampleScenario](examplescenario.html): The example scenario publication date 3726* [GraphDefinition](graphdefinition.html): The graph definition publication date 3727* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3728* [Library](library.html): The library publication date 3729* [Measure](measure.html): The measure publication date 3730* [MessageDefinition](messagedefinition.html): The message definition publication date 3731* [NamingSystem](namingsystem.html): The naming system publication date 3732* [OperationDefinition](operationdefinition.html): The operation definition publication date 3733* [PlanDefinition](plandefinition.html): The plan definition publication date 3734* [Questionnaire](questionnaire.html): The questionnaire publication date 3735* [Requirements](requirements.html): The requirements publication date 3736* [SearchParameter](searchparameter.html): The search parameter publication date 3737* [StructureDefinition](structuredefinition.html): The structure definition publication date 3738* [StructureMap](structuremap.html): The structure map publication date 3739* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3740* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3741* [TestScript](testscript.html): The test script publication date 3742* [ValueSet](valueset.html): The value set publication date 3743</b><br> 3744 * Type: <b>date</b><br> 3745 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3746 * </p> 3747 */ 3748 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3749 public static final String SP_DATE = "date"; 3750 /** 3751 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3752 * <p> 3753 * Description: <b>Multiple Resources: 3754 3755* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3756* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3757* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3758* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3759* [Citation](citation.html): The citation publication date 3760* [CodeSystem](codesystem.html): The code system publication date 3761* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3762* [ConceptMap](conceptmap.html): The concept map publication date 3763* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3764* [EventDefinition](eventdefinition.html): The event definition publication date 3765* [Evidence](evidence.html): The evidence publication date 3766* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3767* [ExampleScenario](examplescenario.html): The example scenario publication date 3768* [GraphDefinition](graphdefinition.html): The graph definition publication date 3769* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3770* [Library](library.html): The library publication date 3771* [Measure](measure.html): The measure publication date 3772* [MessageDefinition](messagedefinition.html): The message definition publication date 3773* [NamingSystem](namingsystem.html): The naming system publication date 3774* [OperationDefinition](operationdefinition.html): The operation definition publication date 3775* [PlanDefinition](plandefinition.html): The plan definition publication date 3776* [Questionnaire](questionnaire.html): The questionnaire publication date 3777* [Requirements](requirements.html): The requirements publication date 3778* [SearchParameter](searchparameter.html): The search parameter publication date 3779* [StructureDefinition](structuredefinition.html): The structure definition publication date 3780* [StructureMap](structuremap.html): The structure map publication date 3781* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3782* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3783* [TestScript](testscript.html): The test script publication date 3784* [ValueSet](valueset.html): The value set publication date 3785</b><br> 3786 * Type: <b>date</b><br> 3787 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3788 * </p> 3789 */ 3790 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3791 3792 /** 3793 * Search parameter: <b>description</b> 3794 * <p> 3795 * Description: <b>Multiple Resources: 3796 3797* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3798* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3799* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3800* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3801* [Citation](citation.html): The description of the citation 3802* [CodeSystem](codesystem.html): The description of the code system 3803* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3804* [ConceptMap](conceptmap.html): The description of the concept map 3805* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3806* [EventDefinition](eventdefinition.html): The description of the event definition 3807* [Evidence](evidence.html): The description of the evidence 3808* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3809* [GraphDefinition](graphdefinition.html): The description of the graph definition 3810* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3811* [Library](library.html): The description of the library 3812* [Measure](measure.html): The description of the measure 3813* [MessageDefinition](messagedefinition.html): The description of the message definition 3814* [NamingSystem](namingsystem.html): The description of the naming system 3815* [OperationDefinition](operationdefinition.html): The description of the operation definition 3816* [PlanDefinition](plandefinition.html): The description of the plan definition 3817* [Questionnaire](questionnaire.html): The description of the questionnaire 3818* [Requirements](requirements.html): The description of the requirements 3819* [SearchParameter](searchparameter.html): The description of the search parameter 3820* [StructureDefinition](structuredefinition.html): The description of the structure definition 3821* [StructureMap](structuremap.html): The description of the structure map 3822* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3823* [TestScript](testscript.html): The description of the test script 3824* [ValueSet](valueset.html): The description of the value set 3825</b><br> 3826 * Type: <b>string</b><br> 3827 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3828 * </p> 3829 */ 3830 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3831 public static final String SP_DESCRIPTION = "description"; 3832 /** 3833 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3834 * <p> 3835 * Description: <b>Multiple Resources: 3836 3837* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3838* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3839* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3840* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3841* [Citation](citation.html): The description of the citation 3842* [CodeSystem](codesystem.html): The description of the code system 3843* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3844* [ConceptMap](conceptmap.html): The description of the concept map 3845* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3846* [EventDefinition](eventdefinition.html): The description of the event definition 3847* [Evidence](evidence.html): The description of the evidence 3848* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3849* [GraphDefinition](graphdefinition.html): The description of the graph definition 3850* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3851* [Library](library.html): The description of the library 3852* [Measure](measure.html): The description of the measure 3853* [MessageDefinition](messagedefinition.html): The description of the message definition 3854* [NamingSystem](namingsystem.html): The description of the naming system 3855* [OperationDefinition](operationdefinition.html): The description of the operation definition 3856* [PlanDefinition](plandefinition.html): The description of the plan definition 3857* [Questionnaire](questionnaire.html): The description of the questionnaire 3858* [Requirements](requirements.html): The description of the requirements 3859* [SearchParameter](searchparameter.html): The description of the search parameter 3860* [StructureDefinition](structuredefinition.html): The description of the structure definition 3861* [StructureMap](structuremap.html): The description of the structure map 3862* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3863* [TestScript](testscript.html): The description of the test script 3864* [ValueSet](valueset.html): The description of the value set 3865</b><br> 3866 * Type: <b>string</b><br> 3867 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3868 * </p> 3869 */ 3870 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3871 3872 /** 3873 * Search parameter: <b>identifier</b> 3874 * <p> 3875 * Description: <b>Multiple Resources: 3876 3877* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3878* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3879* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3880* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3881* [Citation](citation.html): External identifier for the citation 3882* [CodeSystem](codesystem.html): External identifier for the code system 3883* [ConceptMap](conceptmap.html): External identifier for the concept map 3884* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3885* [EventDefinition](eventdefinition.html): External identifier for the event definition 3886* [Evidence](evidence.html): External identifier for the evidence 3887* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3888* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3889* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3890* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3891* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3892* [Library](library.html): External identifier for the library 3893* [Measure](measure.html): External identifier for the measure 3894* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3895* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3896* [NamingSystem](namingsystem.html): External identifier for the naming system 3897* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3898* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3899* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3900* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3901* [Requirements](requirements.html): External identifier for the requirements 3902* [SearchParameter](searchparameter.html): External identifier for the search parameter 3903* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3904* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3905* [StructureMap](structuremap.html): External identifier for the structure map 3906* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3907* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3908* [TestPlan](testplan.html): An identifier for the test plan 3909* [TestScript](testscript.html): External identifier for the test script 3910* [ValueSet](valueset.html): External identifier for the value set 3911</b><br> 3912 * Type: <b>token</b><br> 3913 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3914 * </p> 3915 */ 3916 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3917 public static final String SP_IDENTIFIER = "identifier"; 3918 /** 3919 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3920 * <p> 3921 * Description: <b>Multiple Resources: 3922 3923* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3924* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3925* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3926* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3927* [Citation](citation.html): External identifier for the citation 3928* [CodeSystem](codesystem.html): External identifier for the code system 3929* [ConceptMap](conceptmap.html): External identifier for the concept map 3930* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3931* [EventDefinition](eventdefinition.html): External identifier for the event definition 3932* [Evidence](evidence.html): External identifier for the evidence 3933* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3934* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3935* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3936* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3937* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3938* [Library](library.html): External identifier for the library 3939* [Measure](measure.html): External identifier for the measure 3940* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3941* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3942* [NamingSystem](namingsystem.html): External identifier for the naming system 3943* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3944* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3945* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3946* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3947* [Requirements](requirements.html): External identifier for the requirements 3948* [SearchParameter](searchparameter.html): External identifier for the search parameter 3949* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3950* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3951* [StructureMap](structuremap.html): External identifier for the structure map 3952* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3953* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3954* [TestPlan](testplan.html): An identifier for the test plan 3955* [TestScript](testscript.html): External identifier for the test script 3956* [ValueSet](valueset.html): External identifier for the value set 3957</b><br> 3958 * Type: <b>token</b><br> 3959 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3960 * </p> 3961 */ 3962 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3963 3964 /** 3965 * Search parameter: <b>jurisdiction</b> 3966 * <p> 3967 * Description: <b>Multiple Resources: 3968 3969* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3970* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3971* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3972* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3973* [Citation](citation.html): Intended jurisdiction for the citation 3974* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3975* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3976* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3977* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3978* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3979* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3980* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3981* [Library](library.html): Intended jurisdiction for the library 3982* [Measure](measure.html): Intended jurisdiction for the measure 3983* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3984* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3985* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3986* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3987* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3988* [Requirements](requirements.html): Intended jurisdiction for the requirements 3989* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3990* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3991* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3992* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3993* [TestScript](testscript.html): Intended jurisdiction for the test script 3994* [ValueSet](valueset.html): Intended jurisdiction for the value set 3995</b><br> 3996 * Type: <b>token</b><br> 3997 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3998 * </p> 3999 */ 4000 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 4001 public static final String SP_JURISDICTION = "jurisdiction"; 4002 /** 4003 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4004 * <p> 4005 * Description: <b>Multiple Resources: 4006 4007* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4008* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4009* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4010* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4011* [Citation](citation.html): Intended jurisdiction for the citation 4012* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4013* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4014* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4015* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4016* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4017* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4018* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4019* [Library](library.html): Intended jurisdiction for the library 4020* [Measure](measure.html): Intended jurisdiction for the measure 4021* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4022* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4023* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4024* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4025* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4026* [Requirements](requirements.html): Intended jurisdiction for the requirements 4027* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4028* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4029* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4030* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4031* [TestScript](testscript.html): Intended jurisdiction for the test script 4032* [ValueSet](valueset.html): Intended jurisdiction for the value set 4033</b><br> 4034 * Type: <b>token</b><br> 4035 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4036 * </p> 4037 */ 4038 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4039 4040 /** 4041 * Search parameter: <b>name</b> 4042 * <p> 4043 * Description: <b>Multiple Resources: 4044 4045* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4046* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4047* [Citation](citation.html): Computationally friendly name of the citation 4048* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4049* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4050* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4051* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4052* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4053* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4054* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4055* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4056* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4057* [Library](library.html): Computationally friendly name of the library 4058* [Measure](measure.html): Computationally friendly name of the measure 4059* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4060* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4061* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4062* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4063* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4064* [Requirements](requirements.html): Computationally friendly name of the requirements 4065* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4066* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4067* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4068* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4069* [TestScript](testscript.html): Computationally friendly name of the test script 4070* [ValueSet](valueset.html): Computationally friendly name of the value set 4071</b><br> 4072 * Type: <b>string</b><br> 4073 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4074 * </p> 4075 */ 4076 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 4077 public static final String SP_NAME = "name"; 4078 /** 4079 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4080 * <p> 4081 * Description: <b>Multiple Resources: 4082 4083* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4084* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4085* [Citation](citation.html): Computationally friendly name of the citation 4086* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4087* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4088* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4089* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4090* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4091* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4092* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4093* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4094* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4095* [Library](library.html): Computationally friendly name of the library 4096* [Measure](measure.html): Computationally friendly name of the measure 4097* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4098* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4099* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4100* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4101* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4102* [Requirements](requirements.html): Computationally friendly name of the requirements 4103* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4104* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4105* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4106* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4107* [TestScript](testscript.html): Computationally friendly name of the test script 4108* [ValueSet](valueset.html): Computationally friendly name of the value set 4109</b><br> 4110 * Type: <b>string</b><br> 4111 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4112 * </p> 4113 */ 4114 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4115 4116 /** 4117 * Search parameter: <b>publisher</b> 4118 * <p> 4119 * Description: <b>Multiple Resources: 4120 4121* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4122* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4123* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4124* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4125* [Citation](citation.html): Name of the publisher of the citation 4126* [CodeSystem](codesystem.html): Name of the publisher of the code system 4127* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4128* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4129* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4130* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4131* [Evidence](evidence.html): Name of the publisher of the evidence 4132* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4133* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4134* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4135* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4136* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4137* [Library](library.html): Name of the publisher of the library 4138* [Measure](measure.html): Name of the publisher of the measure 4139* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4140* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4141* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4142* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4143* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4144* [Requirements](requirements.html): Name of the publisher of the requirements 4145* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4146* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4147* [StructureMap](structuremap.html): Name of the publisher of the structure map 4148* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4149* [TestScript](testscript.html): Name of the publisher of the test script 4150* [ValueSet](valueset.html): Name of the publisher of the value set 4151</b><br> 4152 * Type: <b>string</b><br> 4153 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4154 * </p> 4155 */ 4156 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 4157 public static final String SP_PUBLISHER = "publisher"; 4158 /** 4159 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4160 * <p> 4161 * Description: <b>Multiple Resources: 4162 4163* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4164* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4165* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4166* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4167* [Citation](citation.html): Name of the publisher of the citation 4168* [CodeSystem](codesystem.html): Name of the publisher of the code system 4169* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4170* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4171* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4172* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4173* [Evidence](evidence.html): Name of the publisher of the evidence 4174* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4175* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4176* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4177* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4178* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4179* [Library](library.html): Name of the publisher of the library 4180* [Measure](measure.html): Name of the publisher of the measure 4181* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4182* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4183* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4184* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4185* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4186* [Requirements](requirements.html): Name of the publisher of the requirements 4187* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4188* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4189* [StructureMap](structuremap.html): Name of the publisher of the structure map 4190* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4191* [TestScript](testscript.html): Name of the publisher of the test script 4192* [ValueSet](valueset.html): Name of the publisher of the value set 4193</b><br> 4194 * Type: <b>string</b><br> 4195 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4196 * </p> 4197 */ 4198 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4199 4200 /** 4201 * Search parameter: <b>status</b> 4202 * <p> 4203 * Description: <b>Multiple Resources: 4204 4205* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4206* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4207* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4208* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4209* [Citation](citation.html): The current status of the citation 4210* [CodeSystem](codesystem.html): The current status of the code system 4211* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4212* [ConceptMap](conceptmap.html): The current status of the concept map 4213* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4214* [EventDefinition](eventdefinition.html): The current status of the event definition 4215* [Evidence](evidence.html): The current status of the evidence 4216* [EvidenceReport](evidencereport.html): The current status of the evidence report 4217* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4218* [ExampleScenario](examplescenario.html): The current status of the example scenario 4219* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4220* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4221* [Library](library.html): The current status of the library 4222* [Measure](measure.html): The current status of the measure 4223* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4224* [MessageDefinition](messagedefinition.html): The current status of the message definition 4225* [NamingSystem](namingsystem.html): The current status of the naming system 4226* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4227* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4228* [PlanDefinition](plandefinition.html): The current status of the plan definition 4229* [Questionnaire](questionnaire.html): The current status of the questionnaire 4230* [Requirements](requirements.html): The current status of the requirements 4231* [SearchParameter](searchparameter.html): The current status of the search parameter 4232* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4233* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4234* [StructureMap](structuremap.html): The current status of the structure map 4235* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4236* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4237* [TestPlan](testplan.html): The current status of the test plan 4238* [TestScript](testscript.html): The current status of the test script 4239* [ValueSet](valueset.html): The current status of the value set 4240</b><br> 4241 * Type: <b>token</b><br> 4242 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4243 * </p> 4244 */ 4245 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4246 public static final String SP_STATUS = "status"; 4247 /** 4248 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4249 * <p> 4250 * Description: <b>Multiple Resources: 4251 4252* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4253* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4254* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4255* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4256* [Citation](citation.html): The current status of the citation 4257* [CodeSystem](codesystem.html): The current status of the code system 4258* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4259* [ConceptMap](conceptmap.html): The current status of the concept map 4260* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4261* [EventDefinition](eventdefinition.html): The current status of the event definition 4262* [Evidence](evidence.html): The current status of the evidence 4263* [EvidenceReport](evidencereport.html): The current status of the evidence report 4264* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4265* [ExampleScenario](examplescenario.html): The current status of the example scenario 4266* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4267* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4268* [Library](library.html): The current status of the library 4269* [Measure](measure.html): The current status of the measure 4270* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4271* [MessageDefinition](messagedefinition.html): The current status of the message definition 4272* [NamingSystem](namingsystem.html): The current status of the naming system 4273* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4274* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4275* [PlanDefinition](plandefinition.html): The current status of the plan definition 4276* [Questionnaire](questionnaire.html): The current status of the questionnaire 4277* [Requirements](requirements.html): The current status of the requirements 4278* [SearchParameter](searchparameter.html): The current status of the search parameter 4279* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4280* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4281* [StructureMap](structuremap.html): The current status of the structure map 4282* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4283* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4284* [TestPlan](testplan.html): The current status of the test plan 4285* [TestScript](testscript.html): The current status of the test script 4286* [ValueSet](valueset.html): The current status of the value set 4287</b><br> 4288 * Type: <b>token</b><br> 4289 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4290 * </p> 4291 */ 4292 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4293 4294 /** 4295 * Search parameter: <b>url</b> 4296 * <p> 4297 * Description: <b>Multiple Resources: 4298 4299* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4300* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4301* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4302* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4303* [Citation](citation.html): The uri that identifies the citation 4304* [CodeSystem](codesystem.html): The uri that identifies the code system 4305* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4306* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4307* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4308* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4309* [Evidence](evidence.html): The uri that identifies the evidence 4310* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4311* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4312* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4313* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4314* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4315* [Library](library.html): The uri that identifies the library 4316* [Measure](measure.html): The uri that identifies the measure 4317* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4318* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4319* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4320* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4321* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4322* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4323* [Requirements](requirements.html): The uri that identifies the requirements 4324* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4325* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4326* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4327* [StructureMap](structuremap.html): The uri that identifies the structure map 4328* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4329* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4330* [TestPlan](testplan.html): The uri that identifies the test plan 4331* [TestScript](testscript.html): The uri that identifies the test script 4332* [ValueSet](valueset.html): The uri that identifies the value set 4333</b><br> 4334 * Type: <b>uri</b><br> 4335 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4336 * </p> 4337 */ 4338 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4339 public static final String SP_URL = "url"; 4340 /** 4341 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4342 * <p> 4343 * Description: <b>Multiple Resources: 4344 4345* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4346* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4347* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4348* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4349* [Citation](citation.html): The uri that identifies the citation 4350* [CodeSystem](codesystem.html): The uri that identifies the code system 4351* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4352* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4353* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4354* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4355* [Evidence](evidence.html): The uri that identifies the evidence 4356* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4357* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4358* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4359* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4360* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4361* [Library](library.html): The uri that identifies the library 4362* [Measure](measure.html): The uri that identifies the measure 4363* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4364* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4365* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4366* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4367* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4368* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4369* [Requirements](requirements.html): The uri that identifies the requirements 4370* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4371* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4372* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4373* [StructureMap](structuremap.html): The uri that identifies the structure map 4374* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4375* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4376* [TestPlan](testplan.html): The uri that identifies the test plan 4377* [TestScript](testscript.html): The uri that identifies the test script 4378* [ValueSet](valueset.html): The uri that identifies the value set 4379</b><br> 4380 * Type: <b>uri</b><br> 4381 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4382 * </p> 4383 */ 4384 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4385 4386 /** 4387 * Search parameter: <b>version</b> 4388 * <p> 4389 * Description: <b>Multiple Resources: 4390 4391* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4392* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4393* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4394* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4395* [Citation](citation.html): The business version of the citation 4396* [CodeSystem](codesystem.html): The business version of the code system 4397* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4398* [ConceptMap](conceptmap.html): The business version of the concept map 4399* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4400* [EventDefinition](eventdefinition.html): The business version of the event definition 4401* [Evidence](evidence.html): The business version of the evidence 4402* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4403* [ExampleScenario](examplescenario.html): The business version of the example scenario 4404* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4405* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4406* [Library](library.html): The business version of the library 4407* [Measure](measure.html): The business version of the measure 4408* [MessageDefinition](messagedefinition.html): The business version of the message definition 4409* [NamingSystem](namingsystem.html): The business version of the naming system 4410* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4411* [PlanDefinition](plandefinition.html): The business version of the plan definition 4412* [Questionnaire](questionnaire.html): The business version of the questionnaire 4413* [Requirements](requirements.html): The business version of the requirements 4414* [SearchParameter](searchparameter.html): The business version of the search parameter 4415* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4416* [StructureMap](structuremap.html): The business version of the structure map 4417* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4418* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4419* [TestScript](testscript.html): The business version of the test script 4420* [ValueSet](valueset.html): The business version of the value set 4421</b><br> 4422 * Type: <b>token</b><br> 4423 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4424 * </p> 4425 */ 4426 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4427 public static final String SP_VERSION = "version"; 4428 /** 4429 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4430 * <p> 4431 * Description: <b>Multiple Resources: 4432 4433* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4434* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4435* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4436* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4437* [Citation](citation.html): The business version of the citation 4438* [CodeSystem](codesystem.html): The business version of the code system 4439* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4440* [ConceptMap](conceptmap.html): The business version of the concept map 4441* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4442* [EventDefinition](eventdefinition.html): The business version of the event definition 4443* [Evidence](evidence.html): The business version of the evidence 4444* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4445* [ExampleScenario](examplescenario.html): The business version of the example scenario 4446* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4447* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4448* [Library](library.html): The business version of the library 4449* [Measure](measure.html): The business version of the measure 4450* [MessageDefinition](messagedefinition.html): The business version of the message definition 4451* [NamingSystem](namingsystem.html): The business version of the naming system 4452* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4453* [PlanDefinition](plandefinition.html): The business version of the plan definition 4454* [Questionnaire](questionnaire.html): The business version of the questionnaire 4455* [Requirements](requirements.html): The business version of the requirements 4456* [SearchParameter](searchparameter.html): The business version of the search parameter 4457* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4458* [StructureMap](structuremap.html): The business version of the structure map 4459* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4460* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4461* [TestScript](testscript.html): The business version of the test script 4462* [ValueSet](valueset.html): The business version of the value set 4463</b><br> 4464 * Type: <b>token</b><br> 4465 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4466 * </p> 4467 */ 4468 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4469 4470 /** 4471 * Search parameter: <b>derived-from</b> 4472 * <p> 4473 * Description: <b>Multiple Resources: 4474 4475* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4476* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 4477* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 4478* [EventDefinition](eventdefinition.html): What resource is being referenced 4479* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4480* [Library](library.html): What resource is being referenced 4481* [Measure](measure.html): What resource is being referenced 4482* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 4483* [PlanDefinition](plandefinition.html): What resource is being referenced 4484* [ValueSet](valueset.html): A resource that the ValueSet is derived from 4485</b><br> 4486 * Type: <b>reference</b><br> 4487 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 4488 * </p> 4489 */ 4490 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4491 public static final String SP_DERIVED_FROM = "derived-from"; 4492 /** 4493 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4494 * <p> 4495 * Description: <b>Multiple Resources: 4496 4497* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4498* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 4499* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 4500* [EventDefinition](eventdefinition.html): What resource is being referenced 4501* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4502* [Library](library.html): What resource is being referenced 4503* [Measure](measure.html): What resource is being referenced 4504* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 4505* [PlanDefinition](plandefinition.html): What resource is being referenced 4506* [ValueSet](valueset.html): A resource that the ValueSet is derived from 4507</b><br> 4508 * Type: <b>reference</b><br> 4509 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 4510 * </p> 4511 */ 4512 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4513 4514/** 4515 * Constant for fluent queries to be used to add include statements. Specifies 4516 * the path value of "<b>NamingSystem:derived-from</b>". 4517 */ 4518 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("NamingSystem:derived-from").toLocked(); 4519 4520 /** 4521 * Search parameter: <b>effective</b> 4522 * <p> 4523 * Description: <b>Multiple Resources: 4524 4525* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4526* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4527* [Citation](citation.html): The time during which the citation is intended to be in use 4528* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4529* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4530* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4531* [Library](library.html): The time during which the library is intended to be in use 4532* [Measure](measure.html): The time during which the measure is intended to be in use 4533* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4534* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4535* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4536* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4537</b><br> 4538 * Type: <b>date</b><br> 4539 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4540 * </p> 4541 */ 4542 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 4543 public static final String SP_EFFECTIVE = "effective"; 4544 /** 4545 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4546 * <p> 4547 * Description: <b>Multiple Resources: 4548 4549* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4550* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4551* [Citation](citation.html): The time during which the citation is intended to be in use 4552* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4553* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4554* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4555* [Library](library.html): The time during which the library is intended to be in use 4556* [Measure](measure.html): The time during which the measure is intended to be in use 4557* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4558* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4559* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4560* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4561</b><br> 4562 * Type: <b>date</b><br> 4563 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4564 * </p> 4565 */ 4566 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 4567 4568 /** 4569 * Search parameter: <b>predecessor</b> 4570 * <p> 4571 * Description: <b>Multiple Resources: 4572 4573* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4574* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 4575* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 4576* [EventDefinition](eventdefinition.html): What resource is being referenced 4577* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4578* [Library](library.html): What resource is being referenced 4579* [Measure](measure.html): What resource is being referenced 4580* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 4581* [PlanDefinition](plandefinition.html): What resource is being referenced 4582* [ValueSet](valueset.html): The predecessor of the ValueSet 4583</b><br> 4584 * Type: <b>reference</b><br> 4585 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 4586 * </p> 4587 */ 4588 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4589 public static final String SP_PREDECESSOR = "predecessor"; 4590 /** 4591 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 4592 * <p> 4593 * Description: <b>Multiple Resources: 4594 4595* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4596* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 4597* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 4598* [EventDefinition](eventdefinition.html): What resource is being referenced 4599* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4600* [Library](library.html): What resource is being referenced 4601* [Measure](measure.html): What resource is being referenced 4602* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 4603* [PlanDefinition](plandefinition.html): What resource is being referenced 4604* [ValueSet](valueset.html): The predecessor of the ValueSet 4605</b><br> 4606 * Type: <b>reference</b><br> 4607 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 4608 * </p> 4609 */ 4610 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 4611 4612/** 4613 * Constant for fluent queries to be used to add include statements. Specifies 4614 * the path value of "<b>NamingSystem:predecessor</b>". 4615 */ 4616 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("NamingSystem:predecessor").toLocked(); 4617 4618 /** 4619 * Search parameter: <b>topic</b> 4620 * <p> 4621 * Description: <b>Multiple Resources: 4622 4623* [ActivityDefinition](activitydefinition.html): Topics associated with the module 4624* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 4625* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 4626* [EventDefinition](eventdefinition.html): Topics associated with the module 4627* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 4628* [Library](library.html): Topics associated with the module 4629* [Measure](measure.html): Topics associated with the measure 4630* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4631* [PlanDefinition](plandefinition.html): Topics associated with the module 4632* [ValueSet](valueset.html): Topics associated with the ValueSet 4633</b><br> 4634 * Type: <b>token</b><br> 4635 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4636 * </p> 4637 */ 4638 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 4639 public static final String SP_TOPIC = "topic"; 4640 /** 4641 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 4642 * <p> 4643 * Description: <b>Multiple Resources: 4644 4645* [ActivityDefinition](activitydefinition.html): Topics associated with the module 4646* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 4647* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 4648* [EventDefinition](eventdefinition.html): Topics associated with the module 4649* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 4650* [Library](library.html): Topics associated with the module 4651* [Measure](measure.html): Topics associated with the measure 4652* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4653* [PlanDefinition](plandefinition.html): Topics associated with the module 4654* [ValueSet](valueset.html): Topics associated with the ValueSet 4655</b><br> 4656 * Type: <b>token</b><br> 4657 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4658 * </p> 4659 */ 4660 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 4661 4662 /** 4663 * Search parameter: <b>contact</b> 4664 * <p> 4665 * Description: <b>Name of an individual to contact</b><br> 4666 * Type: <b>string</b><br> 4667 * Path: <b>NamingSystem.contact.name</b><br> 4668 * </p> 4669 */ 4670 @SearchParamDefinition(name="contact", path="NamingSystem.contact.name", description="Name of an individual to contact", type="string" ) 4671 public static final String SP_CONTACT = "contact"; 4672 /** 4673 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 4674 * <p> 4675 * Description: <b>Name of an individual to contact</b><br> 4676 * Type: <b>string</b><br> 4677 * Path: <b>NamingSystem.contact.name</b><br> 4678 * </p> 4679 */ 4680 public static final ca.uhn.fhir.rest.gclient.StringClientParam CONTACT = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_CONTACT); 4681 4682 /** 4683 * Search parameter: <b>id-type</b> 4684 * <p> 4685 * Description: <b>oid | uuid | uri | other</b><br> 4686 * Type: <b>token</b><br> 4687 * Path: <b>NamingSystem.uniqueId.type</b><br> 4688 * </p> 4689 */ 4690 @SearchParamDefinition(name="id-type", path="NamingSystem.uniqueId.type", description="oid | uuid | uri | other", type="token" ) 4691 public static final String SP_ID_TYPE = "id-type"; 4692 /** 4693 * <b>Fluent Client</b> search parameter constant for <b>id-type</b> 4694 * <p> 4695 * Description: <b>oid | uuid | uri | other</b><br> 4696 * Type: <b>token</b><br> 4697 * Path: <b>NamingSystem.uniqueId.type</b><br> 4698 * </p> 4699 */ 4700 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ID_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ID_TYPE); 4701 4702 /** 4703 * Search parameter: <b>kind</b> 4704 * <p> 4705 * Description: <b>codesystem | identifier | root</b><br> 4706 * Type: <b>token</b><br> 4707 * Path: <b>NamingSystem.kind</b><br> 4708 * </p> 4709 */ 4710 @SearchParamDefinition(name="kind", path="NamingSystem.kind", description="codesystem | identifier | root", type="token" ) 4711 public static final String SP_KIND = "kind"; 4712 /** 4713 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 4714 * <p> 4715 * Description: <b>codesystem | identifier | root</b><br> 4716 * Type: <b>token</b><br> 4717 * Path: <b>NamingSystem.kind</b><br> 4718 * </p> 4719 */ 4720 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 4721 4722 /** 4723 * Search parameter: <b>period</b> 4724 * <p> 4725 * Description: <b>When is identifier valid?</b><br> 4726 * Type: <b>date</b><br> 4727 * Path: <b>NamingSystem.uniqueId.period</b><br> 4728 * </p> 4729 */ 4730 @SearchParamDefinition(name="period", path="NamingSystem.uniqueId.period", description="When is identifier valid?", type="date" ) 4731 public static final String SP_PERIOD = "period"; 4732 /** 4733 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4734 * <p> 4735 * Description: <b>When is identifier valid?</b><br> 4736 * Type: <b>date</b><br> 4737 * Path: <b>NamingSystem.uniqueId.period</b><br> 4738 * </p> 4739 */ 4740 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 4741 4742 /** 4743 * Search parameter: <b>responsible</b> 4744 * <p> 4745 * Description: <b>Who maintains system namespace?</b><br> 4746 * Type: <b>string</b><br> 4747 * Path: <b>NamingSystem.responsible</b><br> 4748 * </p> 4749 */ 4750 @SearchParamDefinition(name="responsible", path="NamingSystem.responsible", description="Who maintains system namespace?", type="string" ) 4751 public static final String SP_RESPONSIBLE = "responsible"; 4752 /** 4753 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 4754 * <p> 4755 * Description: <b>Who maintains system namespace?</b><br> 4756 * Type: <b>string</b><br> 4757 * Path: <b>NamingSystem.responsible</b><br> 4758 * </p> 4759 */ 4760 public static final ca.uhn.fhir.rest.gclient.StringClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_RESPONSIBLE); 4761 4762 /** 4763 * Search parameter: <b>telecom</b> 4764 * <p> 4765 * Description: <b>Contact details for individual or organization</b><br> 4766 * Type: <b>token</b><br> 4767 * Path: <b>NamingSystem.contact.telecom</b><br> 4768 * </p> 4769 */ 4770 @SearchParamDefinition(name="telecom", path="NamingSystem.contact.telecom", description="Contact details for individual or organization", type="token" ) 4771 public static final String SP_TELECOM = "telecom"; 4772 /** 4773 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 4774 * <p> 4775 * Description: <b>Contact details for individual or organization</b><br> 4776 * Type: <b>token</b><br> 4777 * Path: <b>NamingSystem.contact.telecom</b><br> 4778 * </p> 4779 */ 4780 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 4781 4782 /** 4783 * Search parameter: <b>type</b> 4784 * <p> 4785 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 4786 * Type: <b>token</b><br> 4787 * Path: <b>NamingSystem.type</b><br> 4788 * </p> 4789 */ 4790 @SearchParamDefinition(name="type", path="NamingSystem.type", description="e.g. driver, provider, patient, bank etc.", type="token" ) 4791 public static final String SP_TYPE = "type"; 4792 /** 4793 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4794 * <p> 4795 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 4796 * Type: <b>token</b><br> 4797 * Path: <b>NamingSystem.type</b><br> 4798 * </p> 4799 */ 4800 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4801 4802 /** 4803 * Search parameter: <b>value</b> 4804 * <p> 4805 * Description: <b>The unique identifier</b><br> 4806 * Type: <b>string</b><br> 4807 * Path: <b>NamingSystem.uniqueId.value</b><br> 4808 * </p> 4809 */ 4810 @SearchParamDefinition(name="value", path="NamingSystem.uniqueId.value", description="The unique identifier", type="string" ) 4811 public static final String SP_VALUE = "value"; 4812 /** 4813 * <b>Fluent Client</b> search parameter constant for <b>value</b> 4814 * <p> 4815 * Description: <b>The unique identifier</b><br> 4816 * Type: <b>string</b><br> 4817 * Path: <b>NamingSystem.uniqueId.value</b><br> 4818 * </p> 4819 */ 4820 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VALUE); 4821 4822// Manual code (from Configuration.txt): 4823 public boolean supportsCopyright() { 4824 return true; 4825 } 4826 4827// end addition 4828 4829} 4830