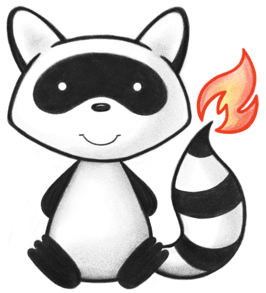
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.xhtml.NodeType; 038import org.hl7.fhir.utilities.xhtml.XhtmlNode; 039import org.hl7.fhir.utilities.Utilities; 040import org.hl7.fhir.r5.model.Enumerations.*; 041import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.instance.model.api.ICompositeType; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.DatatypeDef; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050import org.hl7.fhir.instance.model.api.INarrative; 051/** 052 * Narrative Type: A human-readable summary of the resource conveying the essential clinical and business information for the resource. 053 */ 054@DatatypeDef(name="Narrative") 055public class Narrative extends BaseNarrative implements INarrative { 056 057 public enum NarrativeStatus { 058 /** 059 * The contents of the narrative are entirely generated from the core elements in the content. 060 */ 061 GENERATED, 062 /** 063 * The contents of the narrative are entirely generated from the core elements in the content and some of the content is generated from extensions. The narrative SHALL reflect the impact of all modifier extensions. 064 */ 065 EXTENSIONS, 066 /** 067 * The contents of the narrative may contain additional information not found in the structured data. Note that there is no computable way to determine what the extra information is, other than by human inspection. 068 */ 069 ADDITIONAL, 070 /** 071 * The contents of the narrative are some equivalent of \"No human-readable text provided in this case\". 072 */ 073 EMPTY, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 public static NarrativeStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("generated".equals(codeString)) 082 return GENERATED; 083 if ("extensions".equals(codeString)) 084 return EXTENSIONS; 085 if ("additional".equals(codeString)) 086 return ADDITIONAL; 087 if ("empty".equals(codeString)) 088 return EMPTY; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown NarrativeStatus code '"+codeString+"'"); 093 } 094 public String toCode() { 095 switch (this) { 096 case GENERATED: return "generated"; 097 case EXTENSIONS: return "extensions"; 098 case ADDITIONAL: return "additional"; 099 case EMPTY: return "empty"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getSystem() { 105 switch (this) { 106 case GENERATED: return "http://hl7.org/fhir/narrative-status"; 107 case EXTENSIONS: return "http://hl7.org/fhir/narrative-status"; 108 case ADDITIONAL: return "http://hl7.org/fhir/narrative-status"; 109 case EMPTY: return "http://hl7.org/fhir/narrative-status"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDefinition() { 115 switch (this) { 116 case GENERATED: return "The contents of the narrative are entirely generated from the core elements in the content."; 117 case EXTENSIONS: return "The contents of the narrative are entirely generated from the core elements in the content and some of the content is generated from extensions. The narrative SHALL reflect the impact of all modifier extensions."; 118 case ADDITIONAL: return "The contents of the narrative may contain additional information not found in the structured data. Note that there is no computable way to determine what the extra information is, other than by human inspection."; 119 case EMPTY: return "The contents of the narrative are some equivalent of \"No human-readable text provided in this case\"."; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDisplay() { 125 switch (this) { 126 case GENERATED: return "Generated"; 127 case EXTENSIONS: return "Extensions"; 128 case ADDITIONAL: return "Additional"; 129 case EMPTY: return "Empty"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 } 135 136 public static class NarrativeStatusEnumFactory implements EnumFactory<NarrativeStatus> { 137 public NarrativeStatus fromCode(String codeString) throws IllegalArgumentException { 138 if (codeString == null || "".equals(codeString)) 139 if (codeString == null || "".equals(codeString)) 140 return null; 141 if ("generated".equals(codeString)) 142 return NarrativeStatus.GENERATED; 143 if ("extensions".equals(codeString)) 144 return NarrativeStatus.EXTENSIONS; 145 if ("additional".equals(codeString)) 146 return NarrativeStatus.ADDITIONAL; 147 if ("empty".equals(codeString)) 148 return NarrativeStatus.EMPTY; 149 throw new IllegalArgumentException("Unknown NarrativeStatus code '"+codeString+"'"); 150 } 151 public Enumeration<NarrativeStatus> fromType(PrimitiveType<?> code) throws FHIRException { 152 if (code == null) 153 return null; 154 if (code.isEmpty()) 155 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.NULL, code); 156 String codeString = ((PrimitiveType) code).asStringValue(); 157 if (codeString == null || "".equals(codeString)) 158 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.NULL, code); 159 if ("generated".equals(codeString)) 160 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.GENERATED, code); 161 if ("extensions".equals(codeString)) 162 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EXTENSIONS, code); 163 if ("additional".equals(codeString)) 164 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.ADDITIONAL, code); 165 if ("empty".equals(codeString)) 166 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EMPTY, code); 167 throw new FHIRException("Unknown NarrativeStatus code '"+codeString+"'"); 168 } 169 public String toCode(NarrativeStatus code) { 170 if (code == NarrativeStatus.NULL) 171 return null; 172 if (code == NarrativeStatus.GENERATED) 173 return "generated"; 174 if (code == NarrativeStatus.EXTENSIONS) 175 return "extensions"; 176 if (code == NarrativeStatus.ADDITIONAL) 177 return "additional"; 178 if (code == NarrativeStatus.EMPTY) 179 return "empty"; 180 return "?"; 181 } 182 public String toSystem(NarrativeStatus code) { 183 return code.getSystem(); 184 } 185 } 186 187 /** 188 * The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data. 189 */ 190 @Child(name = "status", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="generated | extensions | additional | empty", formalDefinition="The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data." ) 192 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/narrative-status") 193 protected Enumeration<NarrativeStatus> status; 194 195 /** 196 * The actual narrative content, a stripped down version of XHTML. 197 */ 198 @Child(name = "div", type = {XhtmlType.class}, order=1, min=1, max=1, modifier=false, summary=false) 199 @Description(shortDefinition="Limited xhtml content", formalDefinition="The actual narrative content, a stripped down version of XHTML." ) 200 protected XhtmlNode div; 201 202 private static final long serialVersionUID = 1463852859L; 203 204 /** 205 * Constructor 206 */ 207 public Narrative() { 208 super(); 209 } 210 211 /** 212 * Constructor 213 */ 214 public Narrative(NarrativeStatus status, XhtmlNode div) { 215 super(); 216 this.setStatus(status); 217 this.setDiv(div); 218 } 219 220 /** 221 * @return {@link #status} (The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 222 */ 223 public Enumeration<NarrativeStatus> getStatusElement() { 224 if (this.status == null) 225 if (Configuration.errorOnAutoCreate()) 226 throw new Error("Attempt to auto-create Narrative.status"); 227 else if (Configuration.doAutoCreate()) 228 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); // bb 229 return this.status; 230 } 231 232 public boolean hasStatusElement() { 233 return this.status != null && !this.status.isEmpty(); 234 } 235 236 public boolean hasStatus() { 237 return this.status != null && !this.status.isEmpty(); 238 } 239 240 /** 241 * @param value {@link #status} (The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 242 */ 243 public Narrative setStatusElement(Enumeration<NarrativeStatus> value) { 244 this.status = value; 245 return this; 246 } 247 248 /** 249 * @return The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data. 250 */ 251 public NarrativeStatus getStatus() { 252 return this.status == null ? null : this.status.getValue(); 253 } 254 255 /** 256 * @param value The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data. 257 */ 258 public Narrative setStatus(NarrativeStatus value) { 259 if (this.status == null) 260 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); 261 this.status.setValue(value); 262 return this; 263 } 264 265 /** 266 * @return {@link #div} (The actual narrative content, a stripped down version of XHTML.) 267 */ 268 public XhtmlNode getDiv() { 269 if (this.div == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create Narrative.div"); 272 else if (Configuration.doAutoCreate()) 273 this.div = new XhtmlNode(NodeType.Element, "div"); // cc.1 274 return this.div; 275 } 276 277 public boolean hasDiv() { 278 return this.div != null && !this.div.isEmpty(); 279 } 280 281 /** 282 * @param value {@link #div} (The actual narrative content, a stripped down version of XHTML.) 283 */ 284 public Narrative setDiv(XhtmlNode value) { 285 this.div = value; 286 return this; 287 } 288 289 protected void listChildren(List<Property> children) { 290 super.listChildren(children); 291 children.add(new Property("status", "code", "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.", 0, 1, status)); 292 children.add(new Property("div", "xhtml", "The actual narrative content, a stripped down version of XHTML", 0, 1, new XhtmlType(this))); 293 } 294 295 @Override 296 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 297 switch (_hash) { 298 case -892481550: /*status*/ return new Property("status", "code", "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.", 0, 1, status); 299 default: return super.getNamedProperty(_hash, _name, _checkValid); 300 } 301 302 } 303 304 @Override 305 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 306 switch (hash) { 307 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<NarrativeStatus> 308 case 99473: /*div*/ return this.div == null ? new Base[0] : new Base[] {new XhtmlType(this)}; // XhtmlNode 309 default: return super.getProperty(hash, name, checkValid); 310 } 311 312 } 313 314 @Override 315 public Base setProperty(int hash, String name, Base value) throws FHIRException { 316 switch (hash) { 317 case -892481550: // status 318 value = new NarrativeStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 319 this.status = (Enumeration) value; // Enumeration<NarrativeStatus> 320 return value; 321 case 99473: // div 322 this.div = TypeConvertor.castToXhtml(value); // XhtmlNode 323 ((XhtmlType) value).setPlace(this); 324 return value; 325 default: return super.setProperty(hash, name, value); 326 } 327 328 } 329 330 @Override 331 public Base setProperty(String name, Base value) throws FHIRException { 332 if (name.equals("status")) { 333 value = new NarrativeStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 334 this.status = (Enumeration) value; // Enumeration<NarrativeStatus> 335 } else if (name.equals("div")) { 336 this.div = TypeConvertor.castToXhtml(value); // XhtmlNode 337 } else 338 return super.setProperty(name, value); 339 return value; 340 } 341 342 @Override 343 public void removeChild(String name, Base value) throws FHIRException { 344 if (name.equals("status")) { 345 value = new NarrativeStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 346 this.status = (Enumeration) value; // Enumeration<NarrativeStatus> 347 } else if (name.equals("div")) { 348 this.div = null; 349 } else 350 super.removeChild(name, value); 351 352 } 353 354 @Override 355 public Base makeProperty(int hash, String name) throws FHIRException { 356 switch (hash) { 357 case -892481550: return getStatusElement(); 358 case 99473: /*div*/ 359 if (div == null) 360 div = new XhtmlNode(NodeType.Element, "div"); 361 return new StringType(new org.hl7.fhir.utilities.xhtml.XhtmlComposer(true).composeEx(this.div)); 362 default: return super.makeProperty(hash, name); 363 } 364 365 } 366 367 @Override 368 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 369 switch (hash) { 370 case -892481550: /*status*/ return new String[] {"code"}; 371 case 99473: /*div*/ return new String[] {"xhtml"}; 372 default: return super.getTypesForProperty(hash, name); 373 } 374 375 } 376 377 @Override 378 public Base addChild(String name) throws FHIRException { 379 if (name.equals("status")) { 380 throw new FHIRException("Cannot call addChild on a singleton property Narrative.status"); 381 } 382 else 383 return super.addChild(name); 384 } 385 386 public String fhirType() { 387 return "Narrative"; 388 389 } 390 391 public Narrative copy() { 392 Narrative dst = new Narrative(); 393 copyValues(dst); 394 return dst; 395 } 396 397 public void copyValues(Narrative dst) { 398 super.copyValues(dst); 399 dst.status = status == null ? null : status.copy(); 400 dst.div = div == null ? null : div.copy(); 401 } 402 403 protected Narrative typedCopy() { 404 return copy(); 405 } 406 407 @Override 408 public boolean equalsDeep(Base other_) { 409 if (!super.equalsDeep(other_)) 410 return false; 411 if (!(other_ instanceof Narrative)) 412 return false; 413 Narrative o = (Narrative) other_; 414 return compareDeep(status, o.status, true) && compareDeep(div, o.div, true); 415 } 416 417 @Override 418 public boolean equalsShallow(Base other_) { 419 if (!super.equalsShallow(other_)) 420 return false; 421 if (!(other_ instanceof Narrative)) 422 return false; 423 Narrative o = (Narrative) other_; 424 return compareValues(status, o.status, true); 425 } 426 427 public boolean isEmpty() { 428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, div); 429 } 430 431 432} 433