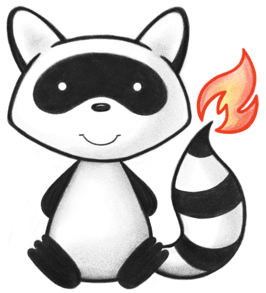
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of food or fluid that is being consumed by a patient. A NutritionIntake may indicate that the patient may be consuming the food or fluid now or has consumed the food or fluid in the past. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay or through an app that tracks food or fluids consumed. The consumption information may come from sources such as the patient's memory, from a nutrition label, or from a clinician documenting observed intake. 052 */ 053@ResourceDef(name="NutritionIntake", profile="http://hl7.org/fhir/StructureDefinition/NutritionIntake") 054public class NutritionIntake extends DomainResource { 055 056 @Block() 057 public static class NutritionIntakeConsumedItemComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Indicates what a category of item that was consumed: e.g., food, fluid, enteral, etc. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="The type of food or fluid product", formalDefinition="Indicates what a category of item that was consumed: e.g., food, fluid, enteral, etc." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/edible-substance-type") 064 protected CodeableConcept type; 065 066 /** 067 * Identifies the food or fluid product that was consumed. This is potentially a link to a resource representing the details of the food product (TBD) or a simple attribute carrying a code that identifies the food from a known list of foods. 068 */ 069 @Child(name = "nutritionProduct", type = {CodeableReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="Code that identifies the food or fluid product that was consumed", formalDefinition="Identifies the food or fluid product that was consumed. This is potentially a link to a resource representing the details of the food product (TBD) or a simple attribute carrying a code that identifies the food from a known list of foods." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/food-type") 072 protected CodeableReference nutritionProduct; 073 074 /** 075 * Scheduled frequency of consumption. 076 */ 077 @Child(name = "schedule", type = {Timing.class}, order=3, min=0, max=1, modifier=false, summary=false) 078 @Description(shortDefinition="Scheduled frequency of consumption", formalDefinition="Scheduled frequency of consumption." ) 079 protected Timing schedule; 080 081 /** 082 * Quantity of the specified food. 083 */ 084 @Child(name = "amount", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=true) 085 @Description(shortDefinition="Quantity of the specified food", formalDefinition="Quantity of the specified food." ) 086 protected Quantity amount; 087 088 /** 089 * Rate at which enteral feeding was administered. 090 */ 091 @Child(name = "rate", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=true) 092 @Description(shortDefinition="Rate at which enteral feeding was administered", formalDefinition="Rate at which enteral feeding was administered." ) 093 protected Quantity rate; 094 095 /** 096 * Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used. 097 */ 098 @Child(name = "notConsumed", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=false) 099 @Description(shortDefinition="Flag to indicate if the food or fluid item was refused or otherwise not consumed", formalDefinition="Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used." ) 100 protected BooleanType notConsumed; 101 102 /** 103 * Document the reason the food or fluid was not consumed, such as refused, held, etc. 104 */ 105 @Child(name = "notConsumedReason", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 106 @Description(shortDefinition="Reason food or fluid was not consumed", formalDefinition="Document the reason the food or fluid was not consumed, such as refused, held, etc." ) 107 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/not-consumed-reason") 108 protected CodeableConcept notConsumedReason; 109 110 private static final long serialVersionUID = -1625538068L; 111 112 /** 113 * Constructor 114 */ 115 public NutritionIntakeConsumedItemComponent() { 116 super(); 117 } 118 119 /** 120 * Constructor 121 */ 122 public NutritionIntakeConsumedItemComponent(CodeableConcept type, CodeableReference nutritionProduct) { 123 super(); 124 this.setType(type); 125 this.setNutritionProduct(nutritionProduct); 126 } 127 128 /** 129 * @return {@link #type} (Indicates what a category of item that was consumed: e.g., food, fluid, enteral, etc.) 130 */ 131 public CodeableConcept getType() { 132 if (this.type == null) 133 if (Configuration.errorOnAutoCreate()) 134 throw new Error("Attempt to auto-create NutritionIntakeConsumedItemComponent.type"); 135 else if (Configuration.doAutoCreate()) 136 this.type = new CodeableConcept(); // cc 137 return this.type; 138 } 139 140 public boolean hasType() { 141 return this.type != null && !this.type.isEmpty(); 142 } 143 144 /** 145 * @param value {@link #type} (Indicates what a category of item that was consumed: e.g., food, fluid, enteral, etc.) 146 */ 147 public NutritionIntakeConsumedItemComponent setType(CodeableConcept value) { 148 this.type = value; 149 return this; 150 } 151 152 /** 153 * @return {@link #nutritionProduct} (Identifies the food or fluid product that was consumed. This is potentially a link to a resource representing the details of the food product (TBD) or a simple attribute carrying a code that identifies the food from a known list of foods.) 154 */ 155 public CodeableReference getNutritionProduct() { 156 if (this.nutritionProduct == null) 157 if (Configuration.errorOnAutoCreate()) 158 throw new Error("Attempt to auto-create NutritionIntakeConsumedItemComponent.nutritionProduct"); 159 else if (Configuration.doAutoCreate()) 160 this.nutritionProduct = new CodeableReference(); // cc 161 return this.nutritionProduct; 162 } 163 164 public boolean hasNutritionProduct() { 165 return this.nutritionProduct != null && !this.nutritionProduct.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #nutritionProduct} (Identifies the food or fluid product that was consumed. This is potentially a link to a resource representing the details of the food product (TBD) or a simple attribute carrying a code that identifies the food from a known list of foods.) 170 */ 171 public NutritionIntakeConsumedItemComponent setNutritionProduct(CodeableReference value) { 172 this.nutritionProduct = value; 173 return this; 174 } 175 176 /** 177 * @return {@link #schedule} (Scheduled frequency of consumption.) 178 */ 179 public Timing getSchedule() { 180 if (this.schedule == null) 181 if (Configuration.errorOnAutoCreate()) 182 throw new Error("Attempt to auto-create NutritionIntakeConsumedItemComponent.schedule"); 183 else if (Configuration.doAutoCreate()) 184 this.schedule = new Timing(); // cc 185 return this.schedule; 186 } 187 188 public boolean hasSchedule() { 189 return this.schedule != null && !this.schedule.isEmpty(); 190 } 191 192 /** 193 * @param value {@link #schedule} (Scheduled frequency of consumption.) 194 */ 195 public NutritionIntakeConsumedItemComponent setSchedule(Timing value) { 196 this.schedule = value; 197 return this; 198 } 199 200 /** 201 * @return {@link #amount} (Quantity of the specified food.) 202 */ 203 public Quantity getAmount() { 204 if (this.amount == null) 205 if (Configuration.errorOnAutoCreate()) 206 throw new Error("Attempt to auto-create NutritionIntakeConsumedItemComponent.amount"); 207 else if (Configuration.doAutoCreate()) 208 this.amount = new Quantity(); // cc 209 return this.amount; 210 } 211 212 public boolean hasAmount() { 213 return this.amount != null && !this.amount.isEmpty(); 214 } 215 216 /** 217 * @param value {@link #amount} (Quantity of the specified food.) 218 */ 219 public NutritionIntakeConsumedItemComponent setAmount(Quantity value) { 220 this.amount = value; 221 return this; 222 } 223 224 /** 225 * @return {@link #rate} (Rate at which enteral feeding was administered.) 226 */ 227 public Quantity getRate() { 228 if (this.rate == null) 229 if (Configuration.errorOnAutoCreate()) 230 throw new Error("Attempt to auto-create NutritionIntakeConsumedItemComponent.rate"); 231 else if (Configuration.doAutoCreate()) 232 this.rate = new Quantity(); // cc 233 return this.rate; 234 } 235 236 public boolean hasRate() { 237 return this.rate != null && !this.rate.isEmpty(); 238 } 239 240 /** 241 * @param value {@link #rate} (Rate at which enteral feeding was administered.) 242 */ 243 public NutritionIntakeConsumedItemComponent setRate(Quantity value) { 244 this.rate = value; 245 return this; 246 } 247 248 /** 249 * @return {@link #notConsumed} (Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used.). This is the underlying object with id, value and extensions. The accessor "getNotConsumed" gives direct access to the value 250 */ 251 public BooleanType getNotConsumedElement() { 252 if (this.notConsumed == null) 253 if (Configuration.errorOnAutoCreate()) 254 throw new Error("Attempt to auto-create NutritionIntakeConsumedItemComponent.notConsumed"); 255 else if (Configuration.doAutoCreate()) 256 this.notConsumed = new BooleanType(); // bb 257 return this.notConsumed; 258 } 259 260 public boolean hasNotConsumedElement() { 261 return this.notConsumed != null && !this.notConsumed.isEmpty(); 262 } 263 264 public boolean hasNotConsumed() { 265 return this.notConsumed != null && !this.notConsumed.isEmpty(); 266 } 267 268 /** 269 * @param value {@link #notConsumed} (Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used.). This is the underlying object with id, value and extensions. The accessor "getNotConsumed" gives direct access to the value 270 */ 271 public NutritionIntakeConsumedItemComponent setNotConsumedElement(BooleanType value) { 272 this.notConsumed = value; 273 return this; 274 } 275 276 /** 277 * @return Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used. 278 */ 279 public boolean getNotConsumed() { 280 return this.notConsumed == null || this.notConsumed.isEmpty() ? false : this.notConsumed.getValue(); 281 } 282 283 /** 284 * @param value Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used. 285 */ 286 public NutritionIntakeConsumedItemComponent setNotConsumed(boolean value) { 287 if (this.notConsumed == null) 288 this.notConsumed = new BooleanType(); 289 this.notConsumed.setValue(value); 290 return this; 291 } 292 293 /** 294 * @return {@link #notConsumedReason} (Document the reason the food or fluid was not consumed, such as refused, held, etc.) 295 */ 296 public CodeableConcept getNotConsumedReason() { 297 if (this.notConsumedReason == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create NutritionIntakeConsumedItemComponent.notConsumedReason"); 300 else if (Configuration.doAutoCreate()) 301 this.notConsumedReason = new CodeableConcept(); // cc 302 return this.notConsumedReason; 303 } 304 305 public boolean hasNotConsumedReason() { 306 return this.notConsumedReason != null && !this.notConsumedReason.isEmpty(); 307 } 308 309 /** 310 * @param value {@link #notConsumedReason} (Document the reason the food or fluid was not consumed, such as refused, held, etc.) 311 */ 312 public NutritionIntakeConsumedItemComponent setNotConsumedReason(CodeableConcept value) { 313 this.notConsumedReason = value; 314 return this; 315 } 316 317 protected void listChildren(List<Property> children) { 318 super.listChildren(children); 319 children.add(new Property("type", "CodeableConcept", "Indicates what a category of item that was consumed: e.g., food, fluid, enteral, etc.", 0, 1, type)); 320 children.add(new Property("nutritionProduct", "CodeableReference(NutritionProduct)", "Identifies the food or fluid product that was consumed. This is potentially a link to a resource representing the details of the food product (TBD) or a simple attribute carrying a code that identifies the food from a known list of foods.", 0, 1, nutritionProduct)); 321 children.add(new Property("schedule", "Timing", "Scheduled frequency of consumption.", 0, 1, schedule)); 322 children.add(new Property("amount", "Quantity", "Quantity of the specified food.", 0, 1, amount)); 323 children.add(new Property("rate", "Quantity", "Rate at which enteral feeding was administered.", 0, 1, rate)); 324 children.add(new Property("notConsumed", "boolean", "Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used.", 0, 1, notConsumed)); 325 children.add(new Property("notConsumedReason", "CodeableConcept", "Document the reason the food or fluid was not consumed, such as refused, held, etc.", 0, 1, notConsumedReason)); 326 } 327 328 @Override 329 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 330 switch (_hash) { 331 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates what a category of item that was consumed: e.g., food, fluid, enteral, etc.", 0, 1, type); 332 case -1684132297: /*nutritionProduct*/ return new Property("nutritionProduct", "CodeableReference(NutritionProduct)", "Identifies the food or fluid product that was consumed. This is potentially a link to a resource representing the details of the food product (TBD) or a simple attribute carrying a code that identifies the food from a known list of foods.", 0, 1, nutritionProduct); 333 case -697920873: /*schedule*/ return new Property("schedule", "Timing", "Scheduled frequency of consumption.", 0, 1, schedule); 334 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "Quantity of the specified food.", 0, 1, amount); 335 case 3493088: /*rate*/ return new Property("rate", "Quantity", "Rate at which enteral feeding was administered.", 0, 1, rate); 336 case -148762661: /*notConsumed*/ return new Property("notConsumed", "boolean", "Indicator when a patient is in a setting where it is helpful to know if food was not consumed, such as it was refused, held (as in tube feedings), or otherwise not provided. If a consumption is being recorded from an app, such as MyFitnessPal, this indicator will likely not be used.", 0, 1, notConsumed); 337 case -440795649: /*notConsumedReason*/ return new Property("notConsumedReason", "CodeableConcept", "Document the reason the food or fluid was not consumed, such as refused, held, etc.", 0, 1, notConsumedReason); 338 default: return super.getNamedProperty(_hash, _name, _checkValid); 339 } 340 341 } 342 343 @Override 344 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 345 switch (hash) { 346 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 347 case -1684132297: /*nutritionProduct*/ return this.nutritionProduct == null ? new Base[0] : new Base[] {this.nutritionProduct}; // CodeableReference 348 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : new Base[] {this.schedule}; // Timing 349 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Quantity 350 case 3493088: /*rate*/ return this.rate == null ? new Base[0] : new Base[] {this.rate}; // Quantity 351 case -148762661: /*notConsumed*/ return this.notConsumed == null ? new Base[0] : new Base[] {this.notConsumed}; // BooleanType 352 case -440795649: /*notConsumedReason*/ return this.notConsumedReason == null ? new Base[0] : new Base[] {this.notConsumedReason}; // CodeableConcept 353 default: return super.getProperty(hash, name, checkValid); 354 } 355 356 } 357 358 @Override 359 public Base setProperty(int hash, String name, Base value) throws FHIRException { 360 switch (hash) { 361 case 3575610: // type 362 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 363 return value; 364 case -1684132297: // nutritionProduct 365 this.nutritionProduct = TypeConvertor.castToCodeableReference(value); // CodeableReference 366 return value; 367 case -697920873: // schedule 368 this.schedule = TypeConvertor.castToTiming(value); // Timing 369 return value; 370 case -1413853096: // amount 371 this.amount = TypeConvertor.castToQuantity(value); // Quantity 372 return value; 373 case 3493088: // rate 374 this.rate = TypeConvertor.castToQuantity(value); // Quantity 375 return value; 376 case -148762661: // notConsumed 377 this.notConsumed = TypeConvertor.castToBoolean(value); // BooleanType 378 return value; 379 case -440795649: // notConsumedReason 380 this.notConsumedReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 381 return value; 382 default: return super.setProperty(hash, name, value); 383 } 384 385 } 386 387 @Override 388 public Base setProperty(String name, Base value) throws FHIRException { 389 if (name.equals("type")) { 390 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 391 } else if (name.equals("nutritionProduct")) { 392 this.nutritionProduct = TypeConvertor.castToCodeableReference(value); // CodeableReference 393 } else if (name.equals("schedule")) { 394 this.schedule = TypeConvertor.castToTiming(value); // Timing 395 } else if (name.equals("amount")) { 396 this.amount = TypeConvertor.castToQuantity(value); // Quantity 397 } else if (name.equals("rate")) { 398 this.rate = TypeConvertor.castToQuantity(value); // Quantity 399 } else if (name.equals("notConsumed")) { 400 this.notConsumed = TypeConvertor.castToBoolean(value); // BooleanType 401 } else if (name.equals("notConsumedReason")) { 402 this.notConsumedReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 403 } else 404 return super.setProperty(name, value); 405 return value; 406 } 407 408 @Override 409 public void removeChild(String name, Base value) throws FHIRException { 410 if (name.equals("type")) { 411 this.type = null; 412 } else if (name.equals("nutritionProduct")) { 413 this.nutritionProduct = null; 414 } else if (name.equals("schedule")) { 415 this.schedule = null; 416 } else if (name.equals("amount")) { 417 this.amount = null; 418 } else if (name.equals("rate")) { 419 this.rate = null; 420 } else if (name.equals("notConsumed")) { 421 this.notConsumed = null; 422 } else if (name.equals("notConsumedReason")) { 423 this.notConsumedReason = null; 424 } else 425 super.removeChild(name, value); 426 427 } 428 429 @Override 430 public Base makeProperty(int hash, String name) throws FHIRException { 431 switch (hash) { 432 case 3575610: return getType(); 433 case -1684132297: return getNutritionProduct(); 434 case -697920873: return getSchedule(); 435 case -1413853096: return getAmount(); 436 case 3493088: return getRate(); 437 case -148762661: return getNotConsumedElement(); 438 case -440795649: return getNotConsumedReason(); 439 default: return super.makeProperty(hash, name); 440 } 441 442 } 443 444 @Override 445 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 446 switch (hash) { 447 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 448 case -1684132297: /*nutritionProduct*/ return new String[] {"CodeableReference"}; 449 case -697920873: /*schedule*/ return new String[] {"Timing"}; 450 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 451 case 3493088: /*rate*/ return new String[] {"Quantity"}; 452 case -148762661: /*notConsumed*/ return new String[] {"boolean"}; 453 case -440795649: /*notConsumedReason*/ return new String[] {"CodeableConcept"}; 454 default: return super.getTypesForProperty(hash, name); 455 } 456 457 } 458 459 @Override 460 public Base addChild(String name) throws FHIRException { 461 if (name.equals("type")) { 462 this.type = new CodeableConcept(); 463 return this.type; 464 } 465 else if (name.equals("nutritionProduct")) { 466 this.nutritionProduct = new CodeableReference(); 467 return this.nutritionProduct; 468 } 469 else if (name.equals("schedule")) { 470 this.schedule = new Timing(); 471 return this.schedule; 472 } 473 else if (name.equals("amount")) { 474 this.amount = new Quantity(); 475 return this.amount; 476 } 477 else if (name.equals("rate")) { 478 this.rate = new Quantity(); 479 return this.rate; 480 } 481 else if (name.equals("notConsumed")) { 482 throw new FHIRException("Cannot call addChild on a singleton property NutritionIntake.consumedItem.notConsumed"); 483 } 484 else if (name.equals("notConsumedReason")) { 485 this.notConsumedReason = new CodeableConcept(); 486 return this.notConsumedReason; 487 } 488 else 489 return super.addChild(name); 490 } 491 492 public NutritionIntakeConsumedItemComponent copy() { 493 NutritionIntakeConsumedItemComponent dst = new NutritionIntakeConsumedItemComponent(); 494 copyValues(dst); 495 return dst; 496 } 497 498 public void copyValues(NutritionIntakeConsumedItemComponent dst) { 499 super.copyValues(dst); 500 dst.type = type == null ? null : type.copy(); 501 dst.nutritionProduct = nutritionProduct == null ? null : nutritionProduct.copy(); 502 dst.schedule = schedule == null ? null : schedule.copy(); 503 dst.amount = amount == null ? null : amount.copy(); 504 dst.rate = rate == null ? null : rate.copy(); 505 dst.notConsumed = notConsumed == null ? null : notConsumed.copy(); 506 dst.notConsumedReason = notConsumedReason == null ? null : notConsumedReason.copy(); 507 } 508 509 @Override 510 public boolean equalsDeep(Base other_) { 511 if (!super.equalsDeep(other_)) 512 return false; 513 if (!(other_ instanceof NutritionIntakeConsumedItemComponent)) 514 return false; 515 NutritionIntakeConsumedItemComponent o = (NutritionIntakeConsumedItemComponent) other_; 516 return compareDeep(type, o.type, true) && compareDeep(nutritionProduct, o.nutritionProduct, true) 517 && compareDeep(schedule, o.schedule, true) && compareDeep(amount, o.amount, true) && compareDeep(rate, o.rate, true) 518 && compareDeep(notConsumed, o.notConsumed, true) && compareDeep(notConsumedReason, o.notConsumedReason, true) 519 ; 520 } 521 522 @Override 523 public boolean equalsShallow(Base other_) { 524 if (!super.equalsShallow(other_)) 525 return false; 526 if (!(other_ instanceof NutritionIntakeConsumedItemComponent)) 527 return false; 528 NutritionIntakeConsumedItemComponent o = (NutritionIntakeConsumedItemComponent) other_; 529 return compareValues(notConsumed, o.notConsumed, true); 530 } 531 532 public boolean isEmpty() { 533 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, nutritionProduct, schedule 534 , amount, rate, notConsumed, notConsumedReason); 535 } 536 537 public String fhirType() { 538 return "NutritionIntake.consumedItem"; 539 540 } 541 542 } 543 544 @Block() 545 public static class NutritionIntakeIngredientLabelComponent extends BackboneElement implements IBaseBackboneElement { 546 /** 547 * Total nutrient consumed. This could be a macronutrient (protein, fat, carbohydrate), or a vitamin and mineral. 548 */ 549 @Child(name = "nutrient", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=false) 550 @Description(shortDefinition="Total nutrient consumed", formalDefinition="Total nutrient consumed. This could be a macronutrient (protein, fat, carbohydrate), or a vitamin and mineral." ) 551 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/nutrient-code") 552 protected CodeableReference nutrient; 553 554 /** 555 * Total amount of nutrient consumed. 556 */ 557 @Child(name = "amount", type = {Quantity.class}, order=2, min=1, max=1, modifier=false, summary=false) 558 @Description(shortDefinition="Total amount of nutrient consumed", formalDefinition="Total amount of nutrient consumed." ) 559 protected Quantity amount; 560 561 private static final long serialVersionUID = -193123424L; 562 563 /** 564 * Constructor 565 */ 566 public NutritionIntakeIngredientLabelComponent() { 567 super(); 568 } 569 570 /** 571 * Constructor 572 */ 573 public NutritionIntakeIngredientLabelComponent(CodeableReference nutrient, Quantity amount) { 574 super(); 575 this.setNutrient(nutrient); 576 this.setAmount(amount); 577 } 578 579 /** 580 * @return {@link #nutrient} (Total nutrient consumed. This could be a macronutrient (protein, fat, carbohydrate), or a vitamin and mineral.) 581 */ 582 public CodeableReference getNutrient() { 583 if (this.nutrient == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create NutritionIntakeIngredientLabelComponent.nutrient"); 586 else if (Configuration.doAutoCreate()) 587 this.nutrient = new CodeableReference(); // cc 588 return this.nutrient; 589 } 590 591 public boolean hasNutrient() { 592 return this.nutrient != null && !this.nutrient.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #nutrient} (Total nutrient consumed. This could be a macronutrient (protein, fat, carbohydrate), or a vitamin and mineral.) 597 */ 598 public NutritionIntakeIngredientLabelComponent setNutrient(CodeableReference value) { 599 this.nutrient = value; 600 return this; 601 } 602 603 /** 604 * @return {@link #amount} (Total amount of nutrient consumed.) 605 */ 606 public Quantity getAmount() { 607 if (this.amount == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create NutritionIntakeIngredientLabelComponent.amount"); 610 else if (Configuration.doAutoCreate()) 611 this.amount = new Quantity(); // cc 612 return this.amount; 613 } 614 615 public boolean hasAmount() { 616 return this.amount != null && !this.amount.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #amount} (Total amount of nutrient consumed.) 621 */ 622 public NutritionIntakeIngredientLabelComponent setAmount(Quantity value) { 623 this.amount = value; 624 return this; 625 } 626 627 protected void listChildren(List<Property> children) { 628 super.listChildren(children); 629 children.add(new Property("nutrient", "CodeableReference(Substance)", "Total nutrient consumed. This could be a macronutrient (protein, fat, carbohydrate), or a vitamin and mineral.", 0, 1, nutrient)); 630 children.add(new Property("amount", "Quantity", "Total amount of nutrient consumed.", 0, 1, amount)); 631 } 632 633 @Override 634 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 635 switch (_hash) { 636 case -1671151641: /*nutrient*/ return new Property("nutrient", "CodeableReference(Substance)", "Total nutrient consumed. This could be a macronutrient (protein, fat, carbohydrate), or a vitamin and mineral.", 0, 1, nutrient); 637 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "Total amount of nutrient consumed.", 0, 1, amount); 638 default: return super.getNamedProperty(_hash, _name, _checkValid); 639 } 640 641 } 642 643 @Override 644 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 645 switch (hash) { 646 case -1671151641: /*nutrient*/ return this.nutrient == null ? new Base[0] : new Base[] {this.nutrient}; // CodeableReference 647 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Quantity 648 default: return super.getProperty(hash, name, checkValid); 649 } 650 651 } 652 653 @Override 654 public Base setProperty(int hash, String name, Base value) throws FHIRException { 655 switch (hash) { 656 case -1671151641: // nutrient 657 this.nutrient = TypeConvertor.castToCodeableReference(value); // CodeableReference 658 return value; 659 case -1413853096: // amount 660 this.amount = TypeConvertor.castToQuantity(value); // Quantity 661 return value; 662 default: return super.setProperty(hash, name, value); 663 } 664 665 } 666 667 @Override 668 public Base setProperty(String name, Base value) throws FHIRException { 669 if (name.equals("nutrient")) { 670 this.nutrient = TypeConvertor.castToCodeableReference(value); // CodeableReference 671 } else if (name.equals("amount")) { 672 this.amount = TypeConvertor.castToQuantity(value); // Quantity 673 } else 674 return super.setProperty(name, value); 675 return value; 676 } 677 678 @Override 679 public void removeChild(String name, Base value) throws FHIRException { 680 if (name.equals("nutrient")) { 681 this.nutrient = null; 682 } else if (name.equals("amount")) { 683 this.amount = null; 684 } else 685 super.removeChild(name, value); 686 687 } 688 689 @Override 690 public Base makeProperty(int hash, String name) throws FHIRException { 691 switch (hash) { 692 case -1671151641: return getNutrient(); 693 case -1413853096: return getAmount(); 694 default: return super.makeProperty(hash, name); 695 } 696 697 } 698 699 @Override 700 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 701 switch (hash) { 702 case -1671151641: /*nutrient*/ return new String[] {"CodeableReference"}; 703 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 704 default: return super.getTypesForProperty(hash, name); 705 } 706 707 } 708 709 @Override 710 public Base addChild(String name) throws FHIRException { 711 if (name.equals("nutrient")) { 712 this.nutrient = new CodeableReference(); 713 return this.nutrient; 714 } 715 else if (name.equals("amount")) { 716 this.amount = new Quantity(); 717 return this.amount; 718 } 719 else 720 return super.addChild(name); 721 } 722 723 public NutritionIntakeIngredientLabelComponent copy() { 724 NutritionIntakeIngredientLabelComponent dst = new NutritionIntakeIngredientLabelComponent(); 725 copyValues(dst); 726 return dst; 727 } 728 729 public void copyValues(NutritionIntakeIngredientLabelComponent dst) { 730 super.copyValues(dst); 731 dst.nutrient = nutrient == null ? null : nutrient.copy(); 732 dst.amount = amount == null ? null : amount.copy(); 733 } 734 735 @Override 736 public boolean equalsDeep(Base other_) { 737 if (!super.equalsDeep(other_)) 738 return false; 739 if (!(other_ instanceof NutritionIntakeIngredientLabelComponent)) 740 return false; 741 NutritionIntakeIngredientLabelComponent o = (NutritionIntakeIngredientLabelComponent) other_; 742 return compareDeep(nutrient, o.nutrient, true) && compareDeep(amount, o.amount, true); 743 } 744 745 @Override 746 public boolean equalsShallow(Base other_) { 747 if (!super.equalsShallow(other_)) 748 return false; 749 if (!(other_ instanceof NutritionIntakeIngredientLabelComponent)) 750 return false; 751 NutritionIntakeIngredientLabelComponent o = (NutritionIntakeIngredientLabelComponent) other_; 752 return true; 753 } 754 755 public boolean isEmpty() { 756 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(nutrient, amount); 757 } 758 759 public String fhirType() { 760 return "NutritionIntake.ingredientLabel"; 761 762 } 763 764 } 765 766 @Block() 767 public static class NutritionIntakePerformerComponent extends BackboneElement implements IBaseBackboneElement { 768 /** 769 * Type of performer. 770 */ 771 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 772 @Description(shortDefinition="Type of performer", formalDefinition="Type of performer." ) 773 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 774 protected CodeableConcept function; 775 776 /** 777 * Who performed the intake. 778 */ 779 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 780 @Description(shortDefinition="Who performed the intake", formalDefinition="Who performed the intake." ) 781 protected Reference actor; 782 783 private static final long serialVersionUID = -576943815L; 784 785 /** 786 * Constructor 787 */ 788 public NutritionIntakePerformerComponent() { 789 super(); 790 } 791 792 /** 793 * Constructor 794 */ 795 public NutritionIntakePerformerComponent(Reference actor) { 796 super(); 797 this.setActor(actor); 798 } 799 800 /** 801 * @return {@link #function} (Type of performer.) 802 */ 803 public CodeableConcept getFunction() { 804 if (this.function == null) 805 if (Configuration.errorOnAutoCreate()) 806 throw new Error("Attempt to auto-create NutritionIntakePerformerComponent.function"); 807 else if (Configuration.doAutoCreate()) 808 this.function = new CodeableConcept(); // cc 809 return this.function; 810 } 811 812 public boolean hasFunction() { 813 return this.function != null && !this.function.isEmpty(); 814 } 815 816 /** 817 * @param value {@link #function} (Type of performer.) 818 */ 819 public NutritionIntakePerformerComponent setFunction(CodeableConcept value) { 820 this.function = value; 821 return this; 822 } 823 824 /** 825 * @return {@link #actor} (Who performed the intake.) 826 */ 827 public Reference getActor() { 828 if (this.actor == null) 829 if (Configuration.errorOnAutoCreate()) 830 throw new Error("Attempt to auto-create NutritionIntakePerformerComponent.actor"); 831 else if (Configuration.doAutoCreate()) 832 this.actor = new Reference(); // cc 833 return this.actor; 834 } 835 836 public boolean hasActor() { 837 return this.actor != null && !this.actor.isEmpty(); 838 } 839 840 /** 841 * @param value {@link #actor} (Who performed the intake.) 842 */ 843 public NutritionIntakePerformerComponent setActor(Reference value) { 844 this.actor = value; 845 return this; 846 } 847 848 protected void listChildren(List<Property> children) { 849 super.listChildren(children); 850 children.add(new Property("function", "CodeableConcept", "Type of performer.", 0, 1, function)); 851 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Who performed the intake.", 0, 1, actor)); 852 } 853 854 @Override 855 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 856 switch (_hash) { 857 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Type of performer.", 0, 1, function); 858 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Who performed the intake.", 0, 1, actor); 859 default: return super.getNamedProperty(_hash, _name, _checkValid); 860 } 861 862 } 863 864 @Override 865 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 866 switch (hash) { 867 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 868 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 869 default: return super.getProperty(hash, name, checkValid); 870 } 871 872 } 873 874 @Override 875 public Base setProperty(int hash, String name, Base value) throws FHIRException { 876 switch (hash) { 877 case 1380938712: // function 878 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 879 return value; 880 case 92645877: // actor 881 this.actor = TypeConvertor.castToReference(value); // Reference 882 return value; 883 default: return super.setProperty(hash, name, value); 884 } 885 886 } 887 888 @Override 889 public Base setProperty(String name, Base value) throws FHIRException { 890 if (name.equals("function")) { 891 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 892 } else if (name.equals("actor")) { 893 this.actor = TypeConvertor.castToReference(value); // Reference 894 } else 895 return super.setProperty(name, value); 896 return value; 897 } 898 899 @Override 900 public void removeChild(String name, Base value) throws FHIRException { 901 if (name.equals("function")) { 902 this.function = null; 903 } else if (name.equals("actor")) { 904 this.actor = null; 905 } else 906 super.removeChild(name, value); 907 908 } 909 910 @Override 911 public Base makeProperty(int hash, String name) throws FHIRException { 912 switch (hash) { 913 case 1380938712: return getFunction(); 914 case 92645877: return getActor(); 915 default: return super.makeProperty(hash, name); 916 } 917 918 } 919 920 @Override 921 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 922 switch (hash) { 923 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 924 case 92645877: /*actor*/ return new String[] {"Reference"}; 925 default: return super.getTypesForProperty(hash, name); 926 } 927 928 } 929 930 @Override 931 public Base addChild(String name) throws FHIRException { 932 if (name.equals("function")) { 933 this.function = new CodeableConcept(); 934 return this.function; 935 } 936 else if (name.equals("actor")) { 937 this.actor = new Reference(); 938 return this.actor; 939 } 940 else 941 return super.addChild(name); 942 } 943 944 public NutritionIntakePerformerComponent copy() { 945 NutritionIntakePerformerComponent dst = new NutritionIntakePerformerComponent(); 946 copyValues(dst); 947 return dst; 948 } 949 950 public void copyValues(NutritionIntakePerformerComponent dst) { 951 super.copyValues(dst); 952 dst.function = function == null ? null : function.copy(); 953 dst.actor = actor == null ? null : actor.copy(); 954 } 955 956 @Override 957 public boolean equalsDeep(Base other_) { 958 if (!super.equalsDeep(other_)) 959 return false; 960 if (!(other_ instanceof NutritionIntakePerformerComponent)) 961 return false; 962 NutritionIntakePerformerComponent o = (NutritionIntakePerformerComponent) other_; 963 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 964 } 965 966 @Override 967 public boolean equalsShallow(Base other_) { 968 if (!super.equalsShallow(other_)) 969 return false; 970 if (!(other_ instanceof NutritionIntakePerformerComponent)) 971 return false; 972 NutritionIntakePerformerComponent o = (NutritionIntakePerformerComponent) other_; 973 return true; 974 } 975 976 public boolean isEmpty() { 977 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 978 } 979 980 public String fhirType() { 981 return "NutritionIntake.performer"; 982 983 } 984 985 } 986 987 /** 988 * Identifiers associated with this Nutrition Intake that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server. 989 */ 990 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 991 @Description(shortDefinition="External identifier", formalDefinition="Identifiers associated with this Nutrition Intake that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server." ) 992 protected List<Identifier> identifier; 993 994 /** 995 * Instantiates FHIR protocol or definition. 996 */ 997 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 998 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="Instantiates FHIR protocol or definition." ) 999 protected List<CanonicalType> instantiatesCanonical; 1000 1001 /** 1002 * Instantiates external protocol or definition. 1003 */ 1004 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1005 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="Instantiates external protocol or definition." ) 1006 protected List<UriType> instantiatesUri; 1007 1008 /** 1009 * A plan, proposal or order that is fulfilled in whole or in part by this event. 1010 */ 1011 @Child(name = "basedOn", type = {NutritionOrder.class, CarePlan.class, ServiceRequest.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1012 @Description(shortDefinition="Fulfils plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event." ) 1013 protected List<Reference> basedOn; 1014 1015 /** 1016 * A larger event of which this particular event is a component or step. 1017 */ 1018 @Child(name = "partOf", type = {NutritionIntake.class, Procedure.class, Observation.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1019 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 1020 protected List<Reference> partOf; 1021 1022 /** 1023 * A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed. 1024 */ 1025 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 1026 @Description(shortDefinition="preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition="A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed." ) 1027 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-status") 1028 protected Enumeration<EventStatus> status; 1029 1030 /** 1031 * Captures the reason for the current state of the NutritionIntake. 1032 */ 1033 @Child(name = "statusReason", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1034 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current state of the NutritionIntake." ) 1035 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinicalimpression-status-reason") 1036 protected List<CodeableConcept> statusReason; 1037 1038 /** 1039 * Overall type of nutrition intake. 1040 */ 1041 @Child(name = "code", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 1042 @Description(shortDefinition="Code representing an overall type of nutrition intake", formalDefinition="Overall type of nutrition intake." ) 1043 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diet-type") 1044 protected CodeableConcept code; 1045 1046 /** 1047 * The person, animal or group who is/was consuming the food or fluid. 1048 */ 1049 @Child(name = "subject", type = {Patient.class, Group.class}, order=8, min=1, max=1, modifier=false, summary=true) 1050 @Description(shortDefinition="Who is/was consuming the food or fluid", formalDefinition="The person, animal or group who is/was consuming the food or fluid." ) 1051 protected Reference subject; 1052 1053 /** 1054 * The encounter that establishes the context for this NutritionIntake. 1055 */ 1056 @Child(name = "encounter", type = {Encounter.class}, order=9, min=0, max=1, modifier=false, summary=true) 1057 @Description(shortDefinition="Encounter associated with NutritionIntake", formalDefinition="The encounter that establishes the context for this NutritionIntake." ) 1058 protected Reference encounter; 1059 1060 /** 1061 * The interval of time during which it is being asserted that the patient is/was consuming the food or fluid. 1062 */ 1063 @Child(name = "occurrence", type = {DateTimeType.class, Period.class}, order=10, min=0, max=1, modifier=false, summary=true) 1064 @Description(shortDefinition="The date/time or interval when the food or fluid is/was consumed", formalDefinition="The interval of time during which it is being asserted that the patient is/was consuming the food or fluid." ) 1065 protected DataType occurrence; 1066 1067 /** 1068 * The date when the Nutrition Intake was asserted by the information source. 1069 */ 1070 @Child(name = "recorded", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1071 @Description(shortDefinition="When the intake was recorded", formalDefinition="The date when the Nutrition Intake was asserted by the information source." ) 1072 protected DateTimeType recorded; 1073 1074 /** 1075 * The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources. 1076 */ 1077 @Child(name = "reported", type = {BooleanType.class, Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Organization.class}, order=12, min=0, max=1, modifier=false, summary=false) 1078 @Description(shortDefinition="Person or organization that provided the information about the consumption of this food or fluid", formalDefinition="The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources." ) 1079 protected DataType reported; 1080 1081 /** 1082 * What food or fluid product or item was consumed. 1083 */ 1084 @Child(name = "consumedItem", type = {}, order=13, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1085 @Description(shortDefinition="What food or fluid product or item was consumed", formalDefinition="What food or fluid product or item was consumed." ) 1086 protected List<NutritionIntakeConsumedItemComponent> consumedItem; 1087 1088 /** 1089 * Total nutrient amounts for the whole meal, product, serving, etc. 1090 */ 1091 @Child(name = "ingredientLabel", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1092 @Description(shortDefinition="Total nutrient for the whole meal, product, serving", formalDefinition="Total nutrient amounts for the whole meal, product, serving, etc." ) 1093 protected List<NutritionIntakeIngredientLabelComponent> ingredientLabel; 1094 1095 /** 1096 * Who performed the intake and how they were involved. 1097 */ 1098 @Child(name = "performer", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1099 @Description(shortDefinition="Who was performed in the intake", formalDefinition="Who performed the intake and how they were involved." ) 1100 protected List<NutritionIntakePerformerComponent> performer; 1101 1102 /** 1103 * Where the intake occurred. 1104 */ 1105 @Child(name = "location", type = {Location.class}, order=16, min=0, max=1, modifier=false, summary=false) 1106 @Description(shortDefinition="Where the intake occurred", formalDefinition="Where the intake occurred." ) 1107 protected Reference location; 1108 1109 /** 1110 * Allows linking the NutritionIntake to the underlying NutritionOrder, or to other information, such as AllergyIntolerance, that supports or is used to derive the NutritionIntake. 1111 */ 1112 @Child(name = "derivedFrom", type = {Reference.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1113 @Description(shortDefinition="Additional supporting information", formalDefinition="Allows linking the NutritionIntake to the underlying NutritionOrder, or to other information, such as AllergyIntolerance, that supports or is used to derive the NutritionIntake." ) 1114 protected List<Reference> derivedFrom; 1115 1116 /** 1117 * A reason, Condition or observation for why the food or fluid is /was consumed. 1118 */ 1119 @Child(name = "reason", type = {CodeableReference.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1120 @Description(shortDefinition="Reason for why the food or fluid is /was consumed", formalDefinition="A reason, Condition or observation for why the food or fluid is /was consumed." ) 1121 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 1122 protected List<CodeableReference> reason; 1123 1124 /** 1125 * Provides extra information about the Nutrition Intake that is not conveyed by the other attributes. 1126 */ 1127 @Child(name = "note", type = {Annotation.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1128 @Description(shortDefinition="Further information about the consumption", formalDefinition="Provides extra information about the Nutrition Intake that is not conveyed by the other attributes." ) 1129 protected List<Annotation> note; 1130 1131 private static final long serialVersionUID = 890692162L; 1132 1133 /** 1134 * Constructor 1135 */ 1136 public NutritionIntake() { 1137 super(); 1138 } 1139 1140 /** 1141 * Constructor 1142 */ 1143 public NutritionIntake(EventStatus status, Reference subject, NutritionIntakeConsumedItemComponent consumedItem) { 1144 super(); 1145 this.setStatus(status); 1146 this.setSubject(subject); 1147 this.addConsumedItem(consumedItem); 1148 } 1149 1150 /** 1151 * @return {@link #identifier} (Identifiers associated with this Nutrition Intake that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.) 1152 */ 1153 public List<Identifier> getIdentifier() { 1154 if (this.identifier == null) 1155 this.identifier = new ArrayList<Identifier>(); 1156 return this.identifier; 1157 } 1158 1159 /** 1160 * @return Returns a reference to <code>this</code> for easy method chaining 1161 */ 1162 public NutritionIntake setIdentifier(List<Identifier> theIdentifier) { 1163 this.identifier = theIdentifier; 1164 return this; 1165 } 1166 1167 public boolean hasIdentifier() { 1168 if (this.identifier == null) 1169 return false; 1170 for (Identifier item : this.identifier) 1171 if (!item.isEmpty()) 1172 return true; 1173 return false; 1174 } 1175 1176 public Identifier addIdentifier() { //3 1177 Identifier t = new Identifier(); 1178 if (this.identifier == null) 1179 this.identifier = new ArrayList<Identifier>(); 1180 this.identifier.add(t); 1181 return t; 1182 } 1183 1184 public NutritionIntake addIdentifier(Identifier t) { //3 1185 if (t == null) 1186 return this; 1187 if (this.identifier == null) 1188 this.identifier = new ArrayList<Identifier>(); 1189 this.identifier.add(t); 1190 return this; 1191 } 1192 1193 /** 1194 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1195 */ 1196 public Identifier getIdentifierFirstRep() { 1197 if (getIdentifier().isEmpty()) { 1198 addIdentifier(); 1199 } 1200 return getIdentifier().get(0); 1201 } 1202 1203 /** 1204 * @return {@link #instantiatesCanonical} (Instantiates FHIR protocol or definition.) 1205 */ 1206 public List<CanonicalType> getInstantiatesCanonical() { 1207 if (this.instantiatesCanonical == null) 1208 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1209 return this.instantiatesCanonical; 1210 } 1211 1212 /** 1213 * @return Returns a reference to <code>this</code> for easy method chaining 1214 */ 1215 public NutritionIntake setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1216 this.instantiatesCanonical = theInstantiatesCanonical; 1217 return this; 1218 } 1219 1220 public boolean hasInstantiatesCanonical() { 1221 if (this.instantiatesCanonical == null) 1222 return false; 1223 for (CanonicalType item : this.instantiatesCanonical) 1224 if (!item.isEmpty()) 1225 return true; 1226 return false; 1227 } 1228 1229 /** 1230 * @return {@link #instantiatesCanonical} (Instantiates FHIR protocol or definition.) 1231 */ 1232 public CanonicalType addInstantiatesCanonicalElement() {//2 1233 CanonicalType t = new CanonicalType(); 1234 if (this.instantiatesCanonical == null) 1235 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1236 this.instantiatesCanonical.add(t); 1237 return t; 1238 } 1239 1240 /** 1241 * @param value {@link #instantiatesCanonical} (Instantiates FHIR protocol or definition.) 1242 */ 1243 public NutritionIntake addInstantiatesCanonical(String value) { //1 1244 CanonicalType t = new CanonicalType(); 1245 t.setValue(value); 1246 if (this.instantiatesCanonical == null) 1247 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1248 this.instantiatesCanonical.add(t); 1249 return this; 1250 } 1251 1252 /** 1253 * @param value {@link #instantiatesCanonical} (Instantiates FHIR protocol or definition.) 1254 */ 1255 public boolean hasInstantiatesCanonical(String value) { 1256 if (this.instantiatesCanonical == null) 1257 return false; 1258 for (CanonicalType v : this.instantiatesCanonical) 1259 if (v.getValue().equals(value)) // canonical 1260 return true; 1261 return false; 1262 } 1263 1264 /** 1265 * @return {@link #instantiatesUri} (Instantiates external protocol or definition.) 1266 */ 1267 public List<UriType> getInstantiatesUri() { 1268 if (this.instantiatesUri == null) 1269 this.instantiatesUri = new ArrayList<UriType>(); 1270 return this.instantiatesUri; 1271 } 1272 1273 /** 1274 * @return Returns a reference to <code>this</code> for easy method chaining 1275 */ 1276 public NutritionIntake setInstantiatesUri(List<UriType> theInstantiatesUri) { 1277 this.instantiatesUri = theInstantiatesUri; 1278 return this; 1279 } 1280 1281 public boolean hasInstantiatesUri() { 1282 if (this.instantiatesUri == null) 1283 return false; 1284 for (UriType item : this.instantiatesUri) 1285 if (!item.isEmpty()) 1286 return true; 1287 return false; 1288 } 1289 1290 /** 1291 * @return {@link #instantiatesUri} (Instantiates external protocol or definition.) 1292 */ 1293 public UriType addInstantiatesUriElement() {//2 1294 UriType t = new UriType(); 1295 if (this.instantiatesUri == null) 1296 this.instantiatesUri = new ArrayList<UriType>(); 1297 this.instantiatesUri.add(t); 1298 return t; 1299 } 1300 1301 /** 1302 * @param value {@link #instantiatesUri} (Instantiates external protocol or definition.) 1303 */ 1304 public NutritionIntake addInstantiatesUri(String value) { //1 1305 UriType t = new UriType(); 1306 t.setValue(value); 1307 if (this.instantiatesUri == null) 1308 this.instantiatesUri = new ArrayList<UriType>(); 1309 this.instantiatesUri.add(t); 1310 return this; 1311 } 1312 1313 /** 1314 * @param value {@link #instantiatesUri} (Instantiates external protocol or definition.) 1315 */ 1316 public boolean hasInstantiatesUri(String value) { 1317 if (this.instantiatesUri == null) 1318 return false; 1319 for (UriType v : this.instantiatesUri) 1320 if (v.getValue().equals(value)) // uri 1321 return true; 1322 return false; 1323 } 1324 1325 /** 1326 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event.) 1327 */ 1328 public List<Reference> getBasedOn() { 1329 if (this.basedOn == null) 1330 this.basedOn = new ArrayList<Reference>(); 1331 return this.basedOn; 1332 } 1333 1334 /** 1335 * @return Returns a reference to <code>this</code> for easy method chaining 1336 */ 1337 public NutritionIntake setBasedOn(List<Reference> theBasedOn) { 1338 this.basedOn = theBasedOn; 1339 return this; 1340 } 1341 1342 public boolean hasBasedOn() { 1343 if (this.basedOn == null) 1344 return false; 1345 for (Reference item : this.basedOn) 1346 if (!item.isEmpty()) 1347 return true; 1348 return false; 1349 } 1350 1351 public Reference addBasedOn() { //3 1352 Reference t = new Reference(); 1353 if (this.basedOn == null) 1354 this.basedOn = new ArrayList<Reference>(); 1355 this.basedOn.add(t); 1356 return t; 1357 } 1358 1359 public NutritionIntake addBasedOn(Reference t) { //3 1360 if (t == null) 1361 return this; 1362 if (this.basedOn == null) 1363 this.basedOn = new ArrayList<Reference>(); 1364 this.basedOn.add(t); 1365 return this; 1366 } 1367 1368 /** 1369 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1370 */ 1371 public Reference getBasedOnFirstRep() { 1372 if (getBasedOn().isEmpty()) { 1373 addBasedOn(); 1374 } 1375 return getBasedOn().get(0); 1376 } 1377 1378 /** 1379 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 1380 */ 1381 public List<Reference> getPartOf() { 1382 if (this.partOf == null) 1383 this.partOf = new ArrayList<Reference>(); 1384 return this.partOf; 1385 } 1386 1387 /** 1388 * @return Returns a reference to <code>this</code> for easy method chaining 1389 */ 1390 public NutritionIntake setPartOf(List<Reference> thePartOf) { 1391 this.partOf = thePartOf; 1392 return this; 1393 } 1394 1395 public boolean hasPartOf() { 1396 if (this.partOf == null) 1397 return false; 1398 for (Reference item : this.partOf) 1399 if (!item.isEmpty()) 1400 return true; 1401 return false; 1402 } 1403 1404 public Reference addPartOf() { //3 1405 Reference t = new Reference(); 1406 if (this.partOf == null) 1407 this.partOf = new ArrayList<Reference>(); 1408 this.partOf.add(t); 1409 return t; 1410 } 1411 1412 public NutritionIntake addPartOf(Reference t) { //3 1413 if (t == null) 1414 return this; 1415 if (this.partOf == null) 1416 this.partOf = new ArrayList<Reference>(); 1417 this.partOf.add(t); 1418 return this; 1419 } 1420 1421 /** 1422 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 1423 */ 1424 public Reference getPartOfFirstRep() { 1425 if (getPartOf().isEmpty()) { 1426 addPartOf(); 1427 } 1428 return getPartOf().get(0); 1429 } 1430 1431 /** 1432 * @return {@link #status} (A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1433 */ 1434 public Enumeration<EventStatus> getStatusElement() { 1435 if (this.status == null) 1436 if (Configuration.errorOnAutoCreate()) 1437 throw new Error("Attempt to auto-create NutritionIntake.status"); 1438 else if (Configuration.doAutoCreate()) 1439 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); // bb 1440 return this.status; 1441 } 1442 1443 public boolean hasStatusElement() { 1444 return this.status != null && !this.status.isEmpty(); 1445 } 1446 1447 public boolean hasStatus() { 1448 return this.status != null && !this.status.isEmpty(); 1449 } 1450 1451 /** 1452 * @param value {@link #status} (A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1453 */ 1454 public NutritionIntake setStatusElement(Enumeration<EventStatus> value) { 1455 this.status = value; 1456 return this; 1457 } 1458 1459 /** 1460 * @return A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed. 1461 */ 1462 public EventStatus getStatus() { 1463 return this.status == null ? null : this.status.getValue(); 1464 } 1465 1466 /** 1467 * @param value A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed. 1468 */ 1469 public NutritionIntake setStatus(EventStatus value) { 1470 if (this.status == null) 1471 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); 1472 this.status.setValue(value); 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #statusReason} (Captures the reason for the current state of the NutritionIntake.) 1478 */ 1479 public List<CodeableConcept> getStatusReason() { 1480 if (this.statusReason == null) 1481 this.statusReason = new ArrayList<CodeableConcept>(); 1482 return this.statusReason; 1483 } 1484 1485 /** 1486 * @return Returns a reference to <code>this</code> for easy method chaining 1487 */ 1488 public NutritionIntake setStatusReason(List<CodeableConcept> theStatusReason) { 1489 this.statusReason = theStatusReason; 1490 return this; 1491 } 1492 1493 public boolean hasStatusReason() { 1494 if (this.statusReason == null) 1495 return false; 1496 for (CodeableConcept item : this.statusReason) 1497 if (!item.isEmpty()) 1498 return true; 1499 return false; 1500 } 1501 1502 public CodeableConcept addStatusReason() { //3 1503 CodeableConcept t = new CodeableConcept(); 1504 if (this.statusReason == null) 1505 this.statusReason = new ArrayList<CodeableConcept>(); 1506 this.statusReason.add(t); 1507 return t; 1508 } 1509 1510 public NutritionIntake addStatusReason(CodeableConcept t) { //3 1511 if (t == null) 1512 return this; 1513 if (this.statusReason == null) 1514 this.statusReason = new ArrayList<CodeableConcept>(); 1515 this.statusReason.add(t); 1516 return this; 1517 } 1518 1519 /** 1520 * @return The first repetition of repeating field {@link #statusReason}, creating it if it does not already exist {3} 1521 */ 1522 public CodeableConcept getStatusReasonFirstRep() { 1523 if (getStatusReason().isEmpty()) { 1524 addStatusReason(); 1525 } 1526 return getStatusReason().get(0); 1527 } 1528 1529 /** 1530 * @return {@link #code} (Overall type of nutrition intake.) 1531 */ 1532 public CodeableConcept getCode() { 1533 if (this.code == null) 1534 if (Configuration.errorOnAutoCreate()) 1535 throw new Error("Attempt to auto-create NutritionIntake.code"); 1536 else if (Configuration.doAutoCreate()) 1537 this.code = new CodeableConcept(); // cc 1538 return this.code; 1539 } 1540 1541 public boolean hasCode() { 1542 return this.code != null && !this.code.isEmpty(); 1543 } 1544 1545 /** 1546 * @param value {@link #code} (Overall type of nutrition intake.) 1547 */ 1548 public NutritionIntake setCode(CodeableConcept value) { 1549 this.code = value; 1550 return this; 1551 } 1552 1553 /** 1554 * @return {@link #subject} (The person, animal or group who is/was consuming the food or fluid.) 1555 */ 1556 public Reference getSubject() { 1557 if (this.subject == null) 1558 if (Configuration.errorOnAutoCreate()) 1559 throw new Error("Attempt to auto-create NutritionIntake.subject"); 1560 else if (Configuration.doAutoCreate()) 1561 this.subject = new Reference(); // cc 1562 return this.subject; 1563 } 1564 1565 public boolean hasSubject() { 1566 return this.subject != null && !this.subject.isEmpty(); 1567 } 1568 1569 /** 1570 * @param value {@link #subject} (The person, animal or group who is/was consuming the food or fluid.) 1571 */ 1572 public NutritionIntake setSubject(Reference value) { 1573 this.subject = value; 1574 return this; 1575 } 1576 1577 /** 1578 * @return {@link #encounter} (The encounter that establishes the context for this NutritionIntake.) 1579 */ 1580 public Reference getEncounter() { 1581 if (this.encounter == null) 1582 if (Configuration.errorOnAutoCreate()) 1583 throw new Error("Attempt to auto-create NutritionIntake.encounter"); 1584 else if (Configuration.doAutoCreate()) 1585 this.encounter = new Reference(); // cc 1586 return this.encounter; 1587 } 1588 1589 public boolean hasEncounter() { 1590 return this.encounter != null && !this.encounter.isEmpty(); 1591 } 1592 1593 /** 1594 * @param value {@link #encounter} (The encounter that establishes the context for this NutritionIntake.) 1595 */ 1596 public NutritionIntake setEncounter(Reference value) { 1597 this.encounter = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #occurrence} (The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.) 1603 */ 1604 public DataType getOccurrence() { 1605 return this.occurrence; 1606 } 1607 1608 /** 1609 * @return {@link #occurrence} (The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.) 1610 */ 1611 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1612 if (this.occurrence == null) 1613 this.occurrence = new DateTimeType(); 1614 if (!(this.occurrence instanceof DateTimeType)) 1615 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1616 return (DateTimeType) this.occurrence; 1617 } 1618 1619 public boolean hasOccurrenceDateTimeType() { 1620 return this != null && this.occurrence instanceof DateTimeType; 1621 } 1622 1623 /** 1624 * @return {@link #occurrence} (The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.) 1625 */ 1626 public Period getOccurrencePeriod() throws FHIRException { 1627 if (this.occurrence == null) 1628 this.occurrence = new Period(); 1629 if (!(this.occurrence instanceof Period)) 1630 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1631 return (Period) this.occurrence; 1632 } 1633 1634 public boolean hasOccurrencePeriod() { 1635 return this != null && this.occurrence instanceof Period; 1636 } 1637 1638 public boolean hasOccurrence() { 1639 return this.occurrence != null && !this.occurrence.isEmpty(); 1640 } 1641 1642 /** 1643 * @param value {@link #occurrence} (The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.) 1644 */ 1645 public NutritionIntake setOccurrence(DataType value) { 1646 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1647 throw new FHIRException("Not the right type for NutritionIntake.occurrence[x]: "+value.fhirType()); 1648 this.occurrence = value; 1649 return this; 1650 } 1651 1652 /** 1653 * @return {@link #recorded} (The date when the Nutrition Intake was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1654 */ 1655 public DateTimeType getRecordedElement() { 1656 if (this.recorded == null) 1657 if (Configuration.errorOnAutoCreate()) 1658 throw new Error("Attempt to auto-create NutritionIntake.recorded"); 1659 else if (Configuration.doAutoCreate()) 1660 this.recorded = new DateTimeType(); // bb 1661 return this.recorded; 1662 } 1663 1664 public boolean hasRecordedElement() { 1665 return this.recorded != null && !this.recorded.isEmpty(); 1666 } 1667 1668 public boolean hasRecorded() { 1669 return this.recorded != null && !this.recorded.isEmpty(); 1670 } 1671 1672 /** 1673 * @param value {@link #recorded} (The date when the Nutrition Intake was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1674 */ 1675 public NutritionIntake setRecordedElement(DateTimeType value) { 1676 this.recorded = value; 1677 return this; 1678 } 1679 1680 /** 1681 * @return The date when the Nutrition Intake was asserted by the information source. 1682 */ 1683 public Date getRecorded() { 1684 return this.recorded == null ? null : this.recorded.getValue(); 1685 } 1686 1687 /** 1688 * @param value The date when the Nutrition Intake was asserted by the information source. 1689 */ 1690 public NutritionIntake setRecorded(Date value) { 1691 if (value == null) 1692 this.recorded = null; 1693 else { 1694 if (this.recorded == null) 1695 this.recorded = new DateTimeType(); 1696 this.recorded.setValue(value); 1697 } 1698 return this; 1699 } 1700 1701 /** 1702 * @return {@link #reported} (The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.) 1703 */ 1704 public DataType getReported() { 1705 return this.reported; 1706 } 1707 1708 /** 1709 * @return {@link #reported} (The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.) 1710 */ 1711 public BooleanType getReportedBooleanType() throws FHIRException { 1712 if (this.reported == null) 1713 this.reported = new BooleanType(); 1714 if (!(this.reported instanceof BooleanType)) 1715 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.reported.getClass().getName()+" was encountered"); 1716 return (BooleanType) this.reported; 1717 } 1718 1719 public boolean hasReportedBooleanType() { 1720 return this != null && this.reported instanceof BooleanType; 1721 } 1722 1723 /** 1724 * @return {@link #reported} (The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.) 1725 */ 1726 public Reference getReportedReference() throws FHIRException { 1727 if (this.reported == null) 1728 this.reported = new Reference(); 1729 if (!(this.reported instanceof Reference)) 1730 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reported.getClass().getName()+" was encountered"); 1731 return (Reference) this.reported; 1732 } 1733 1734 public boolean hasReportedReference() { 1735 return this != null && this.reported instanceof Reference; 1736 } 1737 1738 public boolean hasReported() { 1739 return this.reported != null && !this.reported.isEmpty(); 1740 } 1741 1742 /** 1743 * @param value {@link #reported} (The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.) 1744 */ 1745 public NutritionIntake setReported(DataType value) { 1746 if (value != null && !(value instanceof BooleanType || value instanceof Reference)) 1747 throw new FHIRException("Not the right type for NutritionIntake.reported[x]: "+value.fhirType()); 1748 this.reported = value; 1749 return this; 1750 } 1751 1752 /** 1753 * @return {@link #consumedItem} (What food or fluid product or item was consumed.) 1754 */ 1755 public List<NutritionIntakeConsumedItemComponent> getConsumedItem() { 1756 if (this.consumedItem == null) 1757 this.consumedItem = new ArrayList<NutritionIntakeConsumedItemComponent>(); 1758 return this.consumedItem; 1759 } 1760 1761 /** 1762 * @return Returns a reference to <code>this</code> for easy method chaining 1763 */ 1764 public NutritionIntake setConsumedItem(List<NutritionIntakeConsumedItemComponent> theConsumedItem) { 1765 this.consumedItem = theConsumedItem; 1766 return this; 1767 } 1768 1769 public boolean hasConsumedItem() { 1770 if (this.consumedItem == null) 1771 return false; 1772 for (NutritionIntakeConsumedItemComponent item : this.consumedItem) 1773 if (!item.isEmpty()) 1774 return true; 1775 return false; 1776 } 1777 1778 public NutritionIntakeConsumedItemComponent addConsumedItem() { //3 1779 NutritionIntakeConsumedItemComponent t = new NutritionIntakeConsumedItemComponent(); 1780 if (this.consumedItem == null) 1781 this.consumedItem = new ArrayList<NutritionIntakeConsumedItemComponent>(); 1782 this.consumedItem.add(t); 1783 return t; 1784 } 1785 1786 public NutritionIntake addConsumedItem(NutritionIntakeConsumedItemComponent t) { //3 1787 if (t == null) 1788 return this; 1789 if (this.consumedItem == null) 1790 this.consumedItem = new ArrayList<NutritionIntakeConsumedItemComponent>(); 1791 this.consumedItem.add(t); 1792 return this; 1793 } 1794 1795 /** 1796 * @return The first repetition of repeating field {@link #consumedItem}, creating it if it does not already exist {3} 1797 */ 1798 public NutritionIntakeConsumedItemComponent getConsumedItemFirstRep() { 1799 if (getConsumedItem().isEmpty()) { 1800 addConsumedItem(); 1801 } 1802 return getConsumedItem().get(0); 1803 } 1804 1805 /** 1806 * @return {@link #ingredientLabel} (Total nutrient amounts for the whole meal, product, serving, etc.) 1807 */ 1808 public List<NutritionIntakeIngredientLabelComponent> getIngredientLabel() { 1809 if (this.ingredientLabel == null) 1810 this.ingredientLabel = new ArrayList<NutritionIntakeIngredientLabelComponent>(); 1811 return this.ingredientLabel; 1812 } 1813 1814 /** 1815 * @return Returns a reference to <code>this</code> for easy method chaining 1816 */ 1817 public NutritionIntake setIngredientLabel(List<NutritionIntakeIngredientLabelComponent> theIngredientLabel) { 1818 this.ingredientLabel = theIngredientLabel; 1819 return this; 1820 } 1821 1822 public boolean hasIngredientLabel() { 1823 if (this.ingredientLabel == null) 1824 return false; 1825 for (NutritionIntakeIngredientLabelComponent item : this.ingredientLabel) 1826 if (!item.isEmpty()) 1827 return true; 1828 return false; 1829 } 1830 1831 public NutritionIntakeIngredientLabelComponent addIngredientLabel() { //3 1832 NutritionIntakeIngredientLabelComponent t = new NutritionIntakeIngredientLabelComponent(); 1833 if (this.ingredientLabel == null) 1834 this.ingredientLabel = new ArrayList<NutritionIntakeIngredientLabelComponent>(); 1835 this.ingredientLabel.add(t); 1836 return t; 1837 } 1838 1839 public NutritionIntake addIngredientLabel(NutritionIntakeIngredientLabelComponent t) { //3 1840 if (t == null) 1841 return this; 1842 if (this.ingredientLabel == null) 1843 this.ingredientLabel = new ArrayList<NutritionIntakeIngredientLabelComponent>(); 1844 this.ingredientLabel.add(t); 1845 return this; 1846 } 1847 1848 /** 1849 * @return The first repetition of repeating field {@link #ingredientLabel}, creating it if it does not already exist {3} 1850 */ 1851 public NutritionIntakeIngredientLabelComponent getIngredientLabelFirstRep() { 1852 if (getIngredientLabel().isEmpty()) { 1853 addIngredientLabel(); 1854 } 1855 return getIngredientLabel().get(0); 1856 } 1857 1858 /** 1859 * @return {@link #performer} (Who performed the intake and how they were involved.) 1860 */ 1861 public List<NutritionIntakePerformerComponent> getPerformer() { 1862 if (this.performer == null) 1863 this.performer = new ArrayList<NutritionIntakePerformerComponent>(); 1864 return this.performer; 1865 } 1866 1867 /** 1868 * @return Returns a reference to <code>this</code> for easy method chaining 1869 */ 1870 public NutritionIntake setPerformer(List<NutritionIntakePerformerComponent> thePerformer) { 1871 this.performer = thePerformer; 1872 return this; 1873 } 1874 1875 public boolean hasPerformer() { 1876 if (this.performer == null) 1877 return false; 1878 for (NutritionIntakePerformerComponent item : this.performer) 1879 if (!item.isEmpty()) 1880 return true; 1881 return false; 1882 } 1883 1884 public NutritionIntakePerformerComponent addPerformer() { //3 1885 NutritionIntakePerformerComponent t = new NutritionIntakePerformerComponent(); 1886 if (this.performer == null) 1887 this.performer = new ArrayList<NutritionIntakePerformerComponent>(); 1888 this.performer.add(t); 1889 return t; 1890 } 1891 1892 public NutritionIntake addPerformer(NutritionIntakePerformerComponent t) { //3 1893 if (t == null) 1894 return this; 1895 if (this.performer == null) 1896 this.performer = new ArrayList<NutritionIntakePerformerComponent>(); 1897 this.performer.add(t); 1898 return this; 1899 } 1900 1901 /** 1902 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1903 */ 1904 public NutritionIntakePerformerComponent getPerformerFirstRep() { 1905 if (getPerformer().isEmpty()) { 1906 addPerformer(); 1907 } 1908 return getPerformer().get(0); 1909 } 1910 1911 /** 1912 * @return {@link #location} (Where the intake occurred.) 1913 */ 1914 public Reference getLocation() { 1915 if (this.location == null) 1916 if (Configuration.errorOnAutoCreate()) 1917 throw new Error("Attempt to auto-create NutritionIntake.location"); 1918 else if (Configuration.doAutoCreate()) 1919 this.location = new Reference(); // cc 1920 return this.location; 1921 } 1922 1923 public boolean hasLocation() { 1924 return this.location != null && !this.location.isEmpty(); 1925 } 1926 1927 /** 1928 * @param value {@link #location} (Where the intake occurred.) 1929 */ 1930 public NutritionIntake setLocation(Reference value) { 1931 this.location = value; 1932 return this; 1933 } 1934 1935 /** 1936 * @return {@link #derivedFrom} (Allows linking the NutritionIntake to the underlying NutritionOrder, or to other information, such as AllergyIntolerance, that supports or is used to derive the NutritionIntake.) 1937 */ 1938 public List<Reference> getDerivedFrom() { 1939 if (this.derivedFrom == null) 1940 this.derivedFrom = new ArrayList<Reference>(); 1941 return this.derivedFrom; 1942 } 1943 1944 /** 1945 * @return Returns a reference to <code>this</code> for easy method chaining 1946 */ 1947 public NutritionIntake setDerivedFrom(List<Reference> theDerivedFrom) { 1948 this.derivedFrom = theDerivedFrom; 1949 return this; 1950 } 1951 1952 public boolean hasDerivedFrom() { 1953 if (this.derivedFrom == null) 1954 return false; 1955 for (Reference item : this.derivedFrom) 1956 if (!item.isEmpty()) 1957 return true; 1958 return false; 1959 } 1960 1961 public Reference addDerivedFrom() { //3 1962 Reference t = new Reference(); 1963 if (this.derivedFrom == null) 1964 this.derivedFrom = new ArrayList<Reference>(); 1965 this.derivedFrom.add(t); 1966 return t; 1967 } 1968 1969 public NutritionIntake addDerivedFrom(Reference t) { //3 1970 if (t == null) 1971 return this; 1972 if (this.derivedFrom == null) 1973 this.derivedFrom = new ArrayList<Reference>(); 1974 this.derivedFrom.add(t); 1975 return this; 1976 } 1977 1978 /** 1979 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 1980 */ 1981 public Reference getDerivedFromFirstRep() { 1982 if (getDerivedFrom().isEmpty()) { 1983 addDerivedFrom(); 1984 } 1985 return getDerivedFrom().get(0); 1986 } 1987 1988 /** 1989 * @return {@link #reason} (A reason, Condition or observation for why the food or fluid is /was consumed.) 1990 */ 1991 public List<CodeableReference> getReason() { 1992 if (this.reason == null) 1993 this.reason = new ArrayList<CodeableReference>(); 1994 return this.reason; 1995 } 1996 1997 /** 1998 * @return Returns a reference to <code>this</code> for easy method chaining 1999 */ 2000 public NutritionIntake setReason(List<CodeableReference> theReason) { 2001 this.reason = theReason; 2002 return this; 2003 } 2004 2005 public boolean hasReason() { 2006 if (this.reason == null) 2007 return false; 2008 for (CodeableReference item : this.reason) 2009 if (!item.isEmpty()) 2010 return true; 2011 return false; 2012 } 2013 2014 public CodeableReference addReason() { //3 2015 CodeableReference t = new CodeableReference(); 2016 if (this.reason == null) 2017 this.reason = new ArrayList<CodeableReference>(); 2018 this.reason.add(t); 2019 return t; 2020 } 2021 2022 public NutritionIntake addReason(CodeableReference t) { //3 2023 if (t == null) 2024 return this; 2025 if (this.reason == null) 2026 this.reason = new ArrayList<CodeableReference>(); 2027 this.reason.add(t); 2028 return this; 2029 } 2030 2031 /** 2032 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2033 */ 2034 public CodeableReference getReasonFirstRep() { 2035 if (getReason().isEmpty()) { 2036 addReason(); 2037 } 2038 return getReason().get(0); 2039 } 2040 2041 /** 2042 * @return {@link #note} (Provides extra information about the Nutrition Intake that is not conveyed by the other attributes.) 2043 */ 2044 public List<Annotation> getNote() { 2045 if (this.note == null) 2046 this.note = new ArrayList<Annotation>(); 2047 return this.note; 2048 } 2049 2050 /** 2051 * @return Returns a reference to <code>this</code> for easy method chaining 2052 */ 2053 public NutritionIntake setNote(List<Annotation> theNote) { 2054 this.note = theNote; 2055 return this; 2056 } 2057 2058 public boolean hasNote() { 2059 if (this.note == null) 2060 return false; 2061 for (Annotation item : this.note) 2062 if (!item.isEmpty()) 2063 return true; 2064 return false; 2065 } 2066 2067 public Annotation addNote() { //3 2068 Annotation t = new Annotation(); 2069 if (this.note == null) 2070 this.note = new ArrayList<Annotation>(); 2071 this.note.add(t); 2072 return t; 2073 } 2074 2075 public NutritionIntake addNote(Annotation t) { //3 2076 if (t == null) 2077 return this; 2078 if (this.note == null) 2079 this.note = new ArrayList<Annotation>(); 2080 this.note.add(t); 2081 return this; 2082 } 2083 2084 /** 2085 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2086 */ 2087 public Annotation getNoteFirstRep() { 2088 if (getNote().isEmpty()) { 2089 addNote(); 2090 } 2091 return getNote().get(0); 2092 } 2093 2094 protected void listChildren(List<Property> children) { 2095 super.listChildren(children); 2096 children.add(new Property("identifier", "Identifier", "Identifiers associated with this Nutrition Intake that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2097 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition|ChargeItemDefinition|ClinicalUseDefinition|EventDefinition|Measure|MessageDefinition|ObservationDefinition|OperationDefinition|PlanDefinition|Questionnaire|Requirements|SubscriptionTopic|TestPlan|TestScript)", "Instantiates FHIR protocol or definition.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2098 children.add(new Property("instantiatesUri", "uri", "Instantiates external protocol or definition.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2099 children.add(new Property("basedOn", "Reference(NutritionOrder|CarePlan|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2100 children.add(new Property("partOf", "Reference(NutritionIntake|Procedure|Observation)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2101 children.add(new Property("status", "code", "A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed.", 0, 1, status)); 2102 children.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the NutritionIntake.", 0, java.lang.Integer.MAX_VALUE, statusReason)); 2103 children.add(new Property("code", "CodeableConcept", "Overall type of nutrition intake.", 0, 1, code)); 2104 children.add(new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was consuming the food or fluid.", 0, 1, subject)); 2105 children.add(new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this NutritionIntake.", 0, 1, encounter)); 2106 children.add(new Property("occurrence[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.", 0, 1, occurrence)); 2107 children.add(new Property("recorded", "dateTime", "The date when the Nutrition Intake was asserted by the information source.", 0, 1, recorded)); 2108 children.add(new Property("reported[x]", "boolean|Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.", 0, 1, reported)); 2109 children.add(new Property("consumedItem", "", "What food or fluid product or item was consumed.", 0, java.lang.Integer.MAX_VALUE, consumedItem)); 2110 children.add(new Property("ingredientLabel", "", "Total nutrient amounts for the whole meal, product, serving, etc.", 0, java.lang.Integer.MAX_VALUE, ingredientLabel)); 2111 children.add(new Property("performer", "", "Who performed the intake and how they were involved.", 0, java.lang.Integer.MAX_VALUE, performer)); 2112 children.add(new Property("location", "Reference(Location)", "Where the intake occurred.", 0, 1, location)); 2113 children.add(new Property("derivedFrom", "Reference(Any)", "Allows linking the NutritionIntake to the underlying NutritionOrder, or to other information, such as AllergyIntolerance, that supports or is used to derive the NutritionIntake.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 2114 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "A reason, Condition or observation for why the food or fluid is /was consumed.", 0, java.lang.Integer.MAX_VALUE, reason)); 2115 children.add(new Property("note", "Annotation", "Provides extra information about the Nutrition Intake that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2116 } 2117 2118 @Override 2119 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2120 switch (_hash) { 2121 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this Nutrition Intake that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 2122 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(ActivityDefinition|ChargeItemDefinition|ClinicalUseDefinition|EventDefinition|Measure|MessageDefinition|ObservationDefinition|OperationDefinition|PlanDefinition|Questionnaire|Requirements|SubscriptionTopic|TestPlan|TestScript)", "Instantiates FHIR protocol or definition.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2123 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "Instantiates external protocol or definition.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2124 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(NutritionOrder|CarePlan|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2125 case -995410646: /*partOf*/ return new Property("partOf", "Reference(NutritionIntake|Procedure|Observation)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 2126 case -892481550: /*status*/ return new Property("status", "code", "A code representing the patient or other source's judgment about the state of the intake that this assertion is about. Generally, this will be active or completed.", 0, 1, status); 2127 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the NutritionIntake.", 0, java.lang.Integer.MAX_VALUE, statusReason); 2128 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Overall type of nutrition intake.", 0, 1, code); 2129 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was consuming the food or fluid.", 0, 1, subject); 2130 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this NutritionIntake.", 0, 1, encounter); 2131 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.", 0, 1, occurrence); 2132 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.", 0, 1, occurrence); 2133 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.", 0, 1, occurrence); 2134 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The interval of time during which it is being asserted that the patient is/was consuming the food or fluid.", 0, 1, occurrence); 2135 case -799233872: /*recorded*/ return new Property("recorded", "dateTime", "The date when the Nutrition Intake was asserted by the information source.", 0, 1, recorded); 2136 case -241505587: /*reported[x]*/ return new Property("reported[x]", "boolean|Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.", 0, 1, reported); 2137 case -427039533: /*reported*/ return new Property("reported[x]", "boolean|Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.", 0, 1, reported); 2138 case 1219992533: /*reportedBoolean*/ return new Property("reported[x]", "boolean", "The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.", 0, 1, reported); 2139 case 1198143416: /*reportedReference*/ return new Property("reported[x]", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "The person or organization that provided the information about the consumption of this food or fluid. Note: Use derivedFrom when a NutritionIntake is derived from other resources.", 0, 1, reported); 2140 case -854114533: /*consumedItem*/ return new Property("consumedItem", "", "What food or fluid product or item was consumed.", 0, java.lang.Integer.MAX_VALUE, consumedItem); 2141 case -936536157: /*ingredientLabel*/ return new Property("ingredientLabel", "", "Total nutrient amounts for the whole meal, product, serving, etc.", 0, java.lang.Integer.MAX_VALUE, ingredientLabel); 2142 case 481140686: /*performer*/ return new Property("performer", "", "Who performed the intake and how they were involved.", 0, java.lang.Integer.MAX_VALUE, performer); 2143 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Where the intake occurred.", 0, 1, location); 2144 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(Any)", "Allows linking the NutritionIntake to the underlying NutritionOrder, or to other information, such as AllergyIntolerance, that supports or is used to derive the NutritionIntake.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 2145 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "A reason, Condition or observation for why the food or fluid is /was consumed.", 0, java.lang.Integer.MAX_VALUE, reason); 2146 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides extra information about the Nutrition Intake that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2147 default: return super.getNamedProperty(_hash, _name, _checkValid); 2148 } 2149 2150 } 2151 2152 @Override 2153 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2154 switch (hash) { 2155 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2156 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2157 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2158 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2159 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2160 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EventStatus> 2161 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 2162 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2163 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2164 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2165 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 2166 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // DateTimeType 2167 case -427039533: /*reported*/ return this.reported == null ? new Base[0] : new Base[] {this.reported}; // DataType 2168 case -854114533: /*consumedItem*/ return this.consumedItem == null ? new Base[0] : this.consumedItem.toArray(new Base[this.consumedItem.size()]); // NutritionIntakeConsumedItemComponent 2169 case -936536157: /*ingredientLabel*/ return this.ingredientLabel == null ? new Base[0] : this.ingredientLabel.toArray(new Base[this.ingredientLabel.size()]); // NutritionIntakeIngredientLabelComponent 2170 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // NutritionIntakePerformerComponent 2171 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2172 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 2173 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 2174 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2175 default: return super.getProperty(hash, name, checkValid); 2176 } 2177 2178 } 2179 2180 @Override 2181 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2182 switch (hash) { 2183 case -1618432855: // identifier 2184 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2185 return value; 2186 case 8911915: // instantiatesCanonical 2187 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2188 return value; 2189 case -1926393373: // instantiatesUri 2190 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 2191 return value; 2192 case -332612366: // basedOn 2193 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2194 return value; 2195 case -995410646: // partOf 2196 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 2197 return value; 2198 case -892481550: // status 2199 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2200 this.status = (Enumeration) value; // Enumeration<EventStatus> 2201 return value; 2202 case 2051346646: // statusReason 2203 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2204 return value; 2205 case 3059181: // code 2206 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2207 return value; 2208 case -1867885268: // subject 2209 this.subject = TypeConvertor.castToReference(value); // Reference 2210 return value; 2211 case 1524132147: // encounter 2212 this.encounter = TypeConvertor.castToReference(value); // Reference 2213 return value; 2214 case 1687874001: // occurrence 2215 this.occurrence = TypeConvertor.castToType(value); // DataType 2216 return value; 2217 case -799233872: // recorded 2218 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2219 return value; 2220 case -427039533: // reported 2221 this.reported = TypeConvertor.castToType(value); // DataType 2222 return value; 2223 case -854114533: // consumedItem 2224 this.getConsumedItem().add((NutritionIntakeConsumedItemComponent) value); // NutritionIntakeConsumedItemComponent 2225 return value; 2226 case -936536157: // ingredientLabel 2227 this.getIngredientLabel().add((NutritionIntakeIngredientLabelComponent) value); // NutritionIntakeIngredientLabelComponent 2228 return value; 2229 case 481140686: // performer 2230 this.getPerformer().add((NutritionIntakePerformerComponent) value); // NutritionIntakePerformerComponent 2231 return value; 2232 case 1901043637: // location 2233 this.location = TypeConvertor.castToReference(value); // Reference 2234 return value; 2235 case 1077922663: // derivedFrom 2236 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 2237 return value; 2238 case -934964668: // reason 2239 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2240 return value; 2241 case 3387378: // note 2242 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2243 return value; 2244 default: return super.setProperty(hash, name, value); 2245 } 2246 2247 } 2248 2249 @Override 2250 public Base setProperty(String name, Base value) throws FHIRException { 2251 if (name.equals("identifier")) { 2252 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2253 } else if (name.equals("instantiatesCanonical")) { 2254 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 2255 } else if (name.equals("instantiatesUri")) { 2256 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 2257 } else if (name.equals("basedOn")) { 2258 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2259 } else if (name.equals("partOf")) { 2260 this.getPartOf().add(TypeConvertor.castToReference(value)); 2261 } else if (name.equals("status")) { 2262 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2263 this.status = (Enumeration) value; // Enumeration<EventStatus> 2264 } else if (name.equals("statusReason")) { 2265 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); 2266 } else if (name.equals("code")) { 2267 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2268 } else if (name.equals("subject")) { 2269 this.subject = TypeConvertor.castToReference(value); // Reference 2270 } else if (name.equals("encounter")) { 2271 this.encounter = TypeConvertor.castToReference(value); // Reference 2272 } else if (name.equals("occurrence[x]")) { 2273 this.occurrence = TypeConvertor.castToType(value); // DataType 2274 } else if (name.equals("recorded")) { 2275 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2276 } else if (name.equals("reported[x]")) { 2277 this.reported = TypeConvertor.castToType(value); // DataType 2278 } else if (name.equals("consumedItem")) { 2279 this.getConsumedItem().add((NutritionIntakeConsumedItemComponent) value); 2280 } else if (name.equals("ingredientLabel")) { 2281 this.getIngredientLabel().add((NutritionIntakeIngredientLabelComponent) value); 2282 } else if (name.equals("performer")) { 2283 this.getPerformer().add((NutritionIntakePerformerComponent) value); 2284 } else if (name.equals("location")) { 2285 this.location = TypeConvertor.castToReference(value); // Reference 2286 } else if (name.equals("derivedFrom")) { 2287 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 2288 } else if (name.equals("reason")) { 2289 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 2290 } else if (name.equals("note")) { 2291 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2292 } else 2293 return super.setProperty(name, value); 2294 return value; 2295 } 2296 2297 @Override 2298 public void removeChild(String name, Base value) throws FHIRException { 2299 if (name.equals("identifier")) { 2300 this.getIdentifier().remove(value); 2301 } else if (name.equals("instantiatesCanonical")) { 2302 this.getInstantiatesCanonical().remove(value); 2303 } else if (name.equals("instantiatesUri")) { 2304 this.getInstantiatesUri().remove(value); 2305 } else if (name.equals("basedOn")) { 2306 this.getBasedOn().remove(value); 2307 } else if (name.equals("partOf")) { 2308 this.getPartOf().remove(value); 2309 } else if (name.equals("status")) { 2310 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2311 this.status = (Enumeration) value; // Enumeration<EventStatus> 2312 } else if (name.equals("statusReason")) { 2313 this.getStatusReason().remove(value); 2314 } else if (name.equals("code")) { 2315 this.code = null; 2316 } else if (name.equals("subject")) { 2317 this.subject = null; 2318 } else if (name.equals("encounter")) { 2319 this.encounter = null; 2320 } else if (name.equals("occurrence[x]")) { 2321 this.occurrence = null; 2322 } else if (name.equals("recorded")) { 2323 this.recorded = null; 2324 } else if (name.equals("reported[x]")) { 2325 this.reported = null; 2326 } else if (name.equals("consumedItem")) { 2327 this.getConsumedItem().remove((NutritionIntakeConsumedItemComponent) value); 2328 } else if (name.equals("ingredientLabel")) { 2329 this.getIngredientLabel().remove((NutritionIntakeIngredientLabelComponent) value); 2330 } else if (name.equals("performer")) { 2331 this.getPerformer().remove((NutritionIntakePerformerComponent) value); 2332 } else if (name.equals("location")) { 2333 this.location = null; 2334 } else if (name.equals("derivedFrom")) { 2335 this.getDerivedFrom().remove(value); 2336 } else if (name.equals("reason")) { 2337 this.getReason().remove(value); 2338 } else if (name.equals("note")) { 2339 this.getNote().remove(value); 2340 } else 2341 super.removeChild(name, value); 2342 2343 } 2344 2345 @Override 2346 public Base makeProperty(int hash, String name) throws FHIRException { 2347 switch (hash) { 2348 case -1618432855: return addIdentifier(); 2349 case 8911915: return addInstantiatesCanonicalElement(); 2350 case -1926393373: return addInstantiatesUriElement(); 2351 case -332612366: return addBasedOn(); 2352 case -995410646: return addPartOf(); 2353 case -892481550: return getStatusElement(); 2354 case 2051346646: return addStatusReason(); 2355 case 3059181: return getCode(); 2356 case -1867885268: return getSubject(); 2357 case 1524132147: return getEncounter(); 2358 case -2022646513: return getOccurrence(); 2359 case 1687874001: return getOccurrence(); 2360 case -799233872: return getRecordedElement(); 2361 case -241505587: return getReported(); 2362 case -427039533: return getReported(); 2363 case -854114533: return addConsumedItem(); 2364 case -936536157: return addIngredientLabel(); 2365 case 481140686: return addPerformer(); 2366 case 1901043637: return getLocation(); 2367 case 1077922663: return addDerivedFrom(); 2368 case -934964668: return addReason(); 2369 case 3387378: return addNote(); 2370 default: return super.makeProperty(hash, name); 2371 } 2372 2373 } 2374 2375 @Override 2376 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2377 switch (hash) { 2378 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2379 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 2380 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 2381 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2382 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2383 case -892481550: /*status*/ return new String[] {"code"}; 2384 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 2385 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2386 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2387 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2388 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period"}; 2389 case -799233872: /*recorded*/ return new String[] {"dateTime"}; 2390 case -427039533: /*reported*/ return new String[] {"boolean", "Reference"}; 2391 case -854114533: /*consumedItem*/ return new String[] {}; 2392 case -936536157: /*ingredientLabel*/ return new String[] {}; 2393 case 481140686: /*performer*/ return new String[] {}; 2394 case 1901043637: /*location*/ return new String[] {"Reference"}; 2395 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 2396 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 2397 case 3387378: /*note*/ return new String[] {"Annotation"}; 2398 default: return super.getTypesForProperty(hash, name); 2399 } 2400 2401 } 2402 2403 @Override 2404 public Base addChild(String name) throws FHIRException { 2405 if (name.equals("identifier")) { 2406 return addIdentifier(); 2407 } 2408 else if (name.equals("instantiatesCanonical")) { 2409 throw new FHIRException("Cannot call addChild on a singleton property NutritionIntake.instantiatesCanonical"); 2410 } 2411 else if (name.equals("instantiatesUri")) { 2412 throw new FHIRException("Cannot call addChild on a singleton property NutritionIntake.instantiatesUri"); 2413 } 2414 else if (name.equals("basedOn")) { 2415 return addBasedOn(); 2416 } 2417 else if (name.equals("partOf")) { 2418 return addPartOf(); 2419 } 2420 else if (name.equals("status")) { 2421 throw new FHIRException("Cannot call addChild on a singleton property NutritionIntake.status"); 2422 } 2423 else if (name.equals("statusReason")) { 2424 return addStatusReason(); 2425 } 2426 else if (name.equals("code")) { 2427 this.code = new CodeableConcept(); 2428 return this.code; 2429 } 2430 else if (name.equals("subject")) { 2431 this.subject = new Reference(); 2432 return this.subject; 2433 } 2434 else if (name.equals("encounter")) { 2435 this.encounter = new Reference(); 2436 return this.encounter; 2437 } 2438 else if (name.equals("occurrenceDateTime")) { 2439 this.occurrence = new DateTimeType(); 2440 return this.occurrence; 2441 } 2442 else if (name.equals("occurrencePeriod")) { 2443 this.occurrence = new Period(); 2444 return this.occurrence; 2445 } 2446 else if (name.equals("recorded")) { 2447 throw new FHIRException("Cannot call addChild on a singleton property NutritionIntake.recorded"); 2448 } 2449 else if (name.equals("reportedBoolean")) { 2450 this.reported = new BooleanType(); 2451 return this.reported; 2452 } 2453 else if (name.equals("reportedReference")) { 2454 this.reported = new Reference(); 2455 return this.reported; 2456 } 2457 else if (name.equals("consumedItem")) { 2458 return addConsumedItem(); 2459 } 2460 else if (name.equals("ingredientLabel")) { 2461 return addIngredientLabel(); 2462 } 2463 else if (name.equals("performer")) { 2464 return addPerformer(); 2465 } 2466 else if (name.equals("location")) { 2467 this.location = new Reference(); 2468 return this.location; 2469 } 2470 else if (name.equals("derivedFrom")) { 2471 return addDerivedFrom(); 2472 } 2473 else if (name.equals("reason")) { 2474 return addReason(); 2475 } 2476 else if (name.equals("note")) { 2477 return addNote(); 2478 } 2479 else 2480 return super.addChild(name); 2481 } 2482 2483 public String fhirType() { 2484 return "NutritionIntake"; 2485 2486 } 2487 2488 public NutritionIntake copy() { 2489 NutritionIntake dst = new NutritionIntake(); 2490 copyValues(dst); 2491 return dst; 2492 } 2493 2494 public void copyValues(NutritionIntake dst) { 2495 super.copyValues(dst); 2496 if (identifier != null) { 2497 dst.identifier = new ArrayList<Identifier>(); 2498 for (Identifier i : identifier) 2499 dst.identifier.add(i.copy()); 2500 }; 2501 if (instantiatesCanonical != null) { 2502 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 2503 for (CanonicalType i : instantiatesCanonical) 2504 dst.instantiatesCanonical.add(i.copy()); 2505 }; 2506 if (instantiatesUri != null) { 2507 dst.instantiatesUri = new ArrayList<UriType>(); 2508 for (UriType i : instantiatesUri) 2509 dst.instantiatesUri.add(i.copy()); 2510 }; 2511 if (basedOn != null) { 2512 dst.basedOn = new ArrayList<Reference>(); 2513 for (Reference i : basedOn) 2514 dst.basedOn.add(i.copy()); 2515 }; 2516 if (partOf != null) { 2517 dst.partOf = new ArrayList<Reference>(); 2518 for (Reference i : partOf) 2519 dst.partOf.add(i.copy()); 2520 }; 2521 dst.status = status == null ? null : status.copy(); 2522 if (statusReason != null) { 2523 dst.statusReason = new ArrayList<CodeableConcept>(); 2524 for (CodeableConcept i : statusReason) 2525 dst.statusReason.add(i.copy()); 2526 }; 2527 dst.code = code == null ? null : code.copy(); 2528 dst.subject = subject == null ? null : subject.copy(); 2529 dst.encounter = encounter == null ? null : encounter.copy(); 2530 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2531 dst.recorded = recorded == null ? null : recorded.copy(); 2532 dst.reported = reported == null ? null : reported.copy(); 2533 if (consumedItem != null) { 2534 dst.consumedItem = new ArrayList<NutritionIntakeConsumedItemComponent>(); 2535 for (NutritionIntakeConsumedItemComponent i : consumedItem) 2536 dst.consumedItem.add(i.copy()); 2537 }; 2538 if (ingredientLabel != null) { 2539 dst.ingredientLabel = new ArrayList<NutritionIntakeIngredientLabelComponent>(); 2540 for (NutritionIntakeIngredientLabelComponent i : ingredientLabel) 2541 dst.ingredientLabel.add(i.copy()); 2542 }; 2543 if (performer != null) { 2544 dst.performer = new ArrayList<NutritionIntakePerformerComponent>(); 2545 for (NutritionIntakePerformerComponent i : performer) 2546 dst.performer.add(i.copy()); 2547 }; 2548 dst.location = location == null ? null : location.copy(); 2549 if (derivedFrom != null) { 2550 dst.derivedFrom = new ArrayList<Reference>(); 2551 for (Reference i : derivedFrom) 2552 dst.derivedFrom.add(i.copy()); 2553 }; 2554 if (reason != null) { 2555 dst.reason = new ArrayList<CodeableReference>(); 2556 for (CodeableReference i : reason) 2557 dst.reason.add(i.copy()); 2558 }; 2559 if (note != null) { 2560 dst.note = new ArrayList<Annotation>(); 2561 for (Annotation i : note) 2562 dst.note.add(i.copy()); 2563 }; 2564 } 2565 2566 protected NutritionIntake typedCopy() { 2567 return copy(); 2568 } 2569 2570 @Override 2571 public boolean equalsDeep(Base other_) { 2572 if (!super.equalsDeep(other_)) 2573 return false; 2574 if (!(other_ instanceof NutritionIntake)) 2575 return false; 2576 NutritionIntake o = (NutritionIntake) other_; 2577 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 2578 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 2579 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 2580 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2581 && compareDeep(occurrence, o.occurrence, true) && compareDeep(recorded, o.recorded, true) && compareDeep(reported, o.reported, true) 2582 && compareDeep(consumedItem, o.consumedItem, true) && compareDeep(ingredientLabel, o.ingredientLabel, true) 2583 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) && compareDeep(derivedFrom, o.derivedFrom, true) 2584 && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true); 2585 } 2586 2587 @Override 2588 public boolean equalsShallow(Base other_) { 2589 if (!super.equalsShallow(other_)) 2590 return false; 2591 if (!(other_ instanceof NutritionIntake)) 2592 return false; 2593 NutritionIntake o = (NutritionIntake) other_; 2594 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 2595 && compareValues(status, o.status, true) && compareValues(recorded, o.recorded, true); 2596 } 2597 2598 public boolean isEmpty() { 2599 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 2600 , instantiatesUri, basedOn, partOf, status, statusReason, code, subject, encounter 2601 , occurrence, recorded, reported, consumedItem, ingredientLabel, performer, location 2602 , derivedFrom, reason, note); 2603 } 2604 2605 @Override 2606 public ResourceType getResourceType() { 2607 return ResourceType.NutritionIntake; 2608 } 2609 2610 /** 2611 * Search parameter: <b>nutrition</b> 2612 * <p> 2613 * Description: <b>Return intakes for a specific consumed item</b><br> 2614 * Type: <b>token</b><br> 2615 * Path: <b>NutritionIntake.consumedItem.nutritionProduct.concept</b><br> 2616 * </p> 2617 */ 2618 @SearchParamDefinition(name="nutrition", path="NutritionIntake.consumedItem.nutritionProduct.concept", description="Return intakes for a specific consumed item", type="token" ) 2619 public static final String SP_NUTRITION = "nutrition"; 2620 /** 2621 * <b>Fluent Client</b> search parameter constant for <b>nutrition</b> 2622 * <p> 2623 * Description: <b>Return intakes for a specific consumed item</b><br> 2624 * Type: <b>token</b><br> 2625 * Path: <b>NutritionIntake.consumedItem.nutritionProduct.concept</b><br> 2626 * </p> 2627 */ 2628 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NUTRITION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NUTRITION); 2629 2630 /** 2631 * Search parameter: <b>source</b> 2632 * <p> 2633 * Description: <b>Who or where the information in the statement came from</b><br> 2634 * Type: <b>reference</b><br> 2635 * Path: <b>(NutritionIntake.reported as Reference)</b><br> 2636 * </p> 2637 */ 2638 @SearchParamDefinition(name="source", path="(NutritionIntake.reported as Reference)", description="Who or where the information in the statement came from", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2639 public static final String SP_SOURCE = "source"; 2640 /** 2641 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2642 * <p> 2643 * Description: <b>Who or where the information in the statement came from</b><br> 2644 * Type: <b>reference</b><br> 2645 * Path: <b>(NutritionIntake.reported as Reference)</b><br> 2646 * </p> 2647 */ 2648 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 2649 2650/** 2651 * Constant for fluent queries to be used to add include statements. Specifies 2652 * the path value of "<b>NutritionIntake:source</b>". 2653 */ 2654 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("NutritionIntake:source").toLocked(); 2655 2656 /** 2657 * Search parameter: <b>status</b> 2658 * <p> 2659 * Description: <b>Return statements that match the given status</b><br> 2660 * Type: <b>token</b><br> 2661 * Path: <b>NutritionIntake.status</b><br> 2662 * </p> 2663 */ 2664 @SearchParamDefinition(name="status", path="NutritionIntake.status", description="Return statements that match the given status", type="token" ) 2665 public static final String SP_STATUS = "status"; 2666 /** 2667 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2668 * <p> 2669 * Description: <b>Return statements that match the given status</b><br> 2670 * Type: <b>token</b><br> 2671 * Path: <b>NutritionIntake.status</b><br> 2672 * </p> 2673 */ 2674 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2675 2676 /** 2677 * Search parameter: <b>subject</b> 2678 * <p> 2679 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 2680 * Type: <b>reference</b><br> 2681 * Path: <b>NutritionIntake.subject</b><br> 2682 * </p> 2683 */ 2684 @SearchParamDefinition(name="subject", path="NutritionIntake.subject", description="The identity of a patient, animal or group to list statements for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2685 public static final String SP_SUBJECT = "subject"; 2686 /** 2687 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2688 * <p> 2689 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 2690 * Type: <b>reference</b><br> 2691 * Path: <b>NutritionIntake.subject</b><br> 2692 * </p> 2693 */ 2694 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2695 2696/** 2697 * Constant for fluent queries to be used to add include statements. Specifies 2698 * the path value of "<b>NutritionIntake:subject</b>". 2699 */ 2700 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("NutritionIntake:subject").toLocked(); 2701 2702 /** 2703 * Search parameter: <b>code</b> 2704 * <p> 2705 * Description: <b>Multiple Resources: 2706 2707* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2708* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2709* [AuditEvent](auditevent.html): More specific code for the event 2710* [Basic](basic.html): Kind of Resource 2711* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2712* [Condition](condition.html): Code for the condition 2713* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2714* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2715* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2716* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2717* [ImagingSelection](imagingselection.html): The imaging selection status 2718* [List](list.html): What the purpose of this list is 2719* [Medication](medication.html): Returns medications for a specific code 2720* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2721* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2722* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2723* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2724* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2725* [Observation](observation.html): The code of the observation type 2726* [Procedure](procedure.html): A code to identify a procedure 2727* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2728* [Task](task.html): Search by task code 2729</b><br> 2730 * Type: <b>token</b><br> 2731 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2732 * </p> 2733 */ 2734 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2735 public static final String SP_CODE = "code"; 2736 /** 2737 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2738 * <p> 2739 * Description: <b>Multiple Resources: 2740 2741* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2742* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2743* [AuditEvent](auditevent.html): More specific code for the event 2744* [Basic](basic.html): Kind of Resource 2745* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2746* [Condition](condition.html): Code for the condition 2747* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2748* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2749* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2750* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2751* [ImagingSelection](imagingselection.html): The imaging selection status 2752* [List](list.html): What the purpose of this list is 2753* [Medication](medication.html): Returns medications for a specific code 2754* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2755* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2756* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2757* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2758* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2759* [Observation](observation.html): The code of the observation type 2760* [Procedure](procedure.html): A code to identify a procedure 2761* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2762* [Task](task.html): Search by task code 2763</b><br> 2764 * Type: <b>token</b><br> 2765 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2766 * </p> 2767 */ 2768 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2769 2770 /** 2771 * Search parameter: <b>date</b> 2772 * <p> 2773 * Description: <b>Multiple Resources: 2774 2775* [AdverseEvent](adverseevent.html): When the event occurred 2776* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2777* [Appointment](appointment.html): Appointment date/time. 2778* [AuditEvent](auditevent.html): Time when the event was recorded 2779* [CarePlan](careplan.html): Time period plan covers 2780* [CareTeam](careteam.html): A date within the coverage time period. 2781* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2782* [Composition](composition.html): Composition editing time 2783* [Consent](consent.html): When consent was agreed to 2784* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2785* [DocumentReference](documentreference.html): When this document reference was created 2786* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2787* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2788* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2789* [Flag](flag.html): Time period when flag is active 2790* [Immunization](immunization.html): Vaccination (non)-Administration Date 2791* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2792* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2793* [Invoice](invoice.html): Invoice date / posting date 2794* [List](list.html): When the list was prepared 2795* [MeasureReport](measurereport.html): The date of the measure report 2796* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2797* [Observation](observation.html): Clinically relevant time/time-period for observation 2798* [Procedure](procedure.html): When the procedure occurred or is occurring 2799* [ResearchSubject](researchsubject.html): Start and end of participation 2800* [RiskAssessment](riskassessment.html): When was assessment made? 2801* [SupplyRequest](supplyrequest.html): When the request was made 2802</b><br> 2803 * Type: <b>date</b><br> 2804 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2805 * </p> 2806 */ 2807 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 2808 public static final String SP_DATE = "date"; 2809 /** 2810 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2811 * <p> 2812 * Description: <b>Multiple Resources: 2813 2814* [AdverseEvent](adverseevent.html): When the event occurred 2815* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2816* [Appointment](appointment.html): Appointment date/time. 2817* [AuditEvent](auditevent.html): Time when the event was recorded 2818* [CarePlan](careplan.html): Time period plan covers 2819* [CareTeam](careteam.html): A date within the coverage time period. 2820* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2821* [Composition](composition.html): Composition editing time 2822* [Consent](consent.html): When consent was agreed to 2823* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2824* [DocumentReference](documentreference.html): When this document reference was created 2825* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2826* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2827* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2828* [Flag](flag.html): Time period when flag is active 2829* [Immunization](immunization.html): Vaccination (non)-Administration Date 2830* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2831* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2832* [Invoice](invoice.html): Invoice date / posting date 2833* [List](list.html): When the list was prepared 2834* [MeasureReport](measurereport.html): The date of the measure report 2835* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2836* [Observation](observation.html): Clinically relevant time/time-period for observation 2837* [Procedure](procedure.html): When the procedure occurred or is occurring 2838* [ResearchSubject](researchsubject.html): Start and end of participation 2839* [RiskAssessment](riskassessment.html): When was assessment made? 2840* [SupplyRequest](supplyrequest.html): When the request was made 2841</b><br> 2842 * Type: <b>date</b><br> 2843 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2844 * </p> 2845 */ 2846 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2847 2848 /** 2849 * Search parameter: <b>encounter</b> 2850 * <p> 2851 * Description: <b>Multiple Resources: 2852 2853* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2854* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2855* [ChargeItem](chargeitem.html): Encounter associated with event 2856* [Claim](claim.html): Encounters associated with a billed line item 2857* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2858* [Communication](communication.html): The Encounter during which this Communication was created 2859* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2860* [Composition](composition.html): Context of the Composition 2861* [Condition](condition.html): The Encounter during which this Condition was created 2862* [DeviceRequest](devicerequest.html): Encounter during which request was created 2863* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2864* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2865* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2866* [Flag](flag.html): Alert relevant during encounter 2867* [ImagingStudy](imagingstudy.html): The context of the study 2868* [List](list.html): Context in which list created 2869* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2870* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2871* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2872* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2873* [Observation](observation.html): Encounter related to the observation 2874* [Procedure](procedure.html): The Encounter during which this Procedure was created 2875* [Provenance](provenance.html): Encounter related to the Provenance 2876* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2877* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2878* [RiskAssessment](riskassessment.html): Where was assessment performed? 2879* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2880* [Task](task.html): Search by encounter 2881* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2882</b><br> 2883 * Type: <b>reference</b><br> 2884 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2885 * </p> 2886 */ 2887 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2888 public static final String SP_ENCOUNTER = "encounter"; 2889 /** 2890 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2891 * <p> 2892 * Description: <b>Multiple Resources: 2893 2894* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2895* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2896* [ChargeItem](chargeitem.html): Encounter associated with event 2897* [Claim](claim.html): Encounters associated with a billed line item 2898* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2899* [Communication](communication.html): The Encounter during which this Communication was created 2900* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2901* [Composition](composition.html): Context of the Composition 2902* [Condition](condition.html): The Encounter during which this Condition was created 2903* [DeviceRequest](devicerequest.html): Encounter during which request was created 2904* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2905* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2906* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2907* [Flag](flag.html): Alert relevant during encounter 2908* [ImagingStudy](imagingstudy.html): The context of the study 2909* [List](list.html): Context in which list created 2910* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2911* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2912* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2913* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2914* [Observation](observation.html): Encounter related to the observation 2915* [Procedure](procedure.html): The Encounter during which this Procedure was created 2916* [Provenance](provenance.html): Encounter related to the Provenance 2917* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2918* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2919* [RiskAssessment](riskassessment.html): Where was assessment performed? 2920* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2921* [Task](task.html): Search by encounter 2922* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2923</b><br> 2924 * Type: <b>reference</b><br> 2925 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2926 * </p> 2927 */ 2928 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2929 2930/** 2931 * Constant for fluent queries to be used to add include statements. Specifies 2932 * the path value of "<b>NutritionIntake:encounter</b>". 2933 */ 2934 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("NutritionIntake:encounter").toLocked(); 2935 2936 /** 2937 * Search parameter: <b>identifier</b> 2938 * <p> 2939 * Description: <b>Multiple Resources: 2940 2941* [Account](account.html): Account number 2942* [AdverseEvent](adverseevent.html): Business identifier for the event 2943* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2944* [Appointment](appointment.html): An Identifier of the Appointment 2945* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2946* [Basic](basic.html): Business identifier 2947* [BodyStructure](bodystructure.html): Bodystructure identifier 2948* [CarePlan](careplan.html): External Ids for this plan 2949* [CareTeam](careteam.html): External Ids for this team 2950* [ChargeItem](chargeitem.html): Business Identifier for item 2951* [Claim](claim.html): The primary identifier of the financial resource 2952* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2953* [ClinicalImpression](clinicalimpression.html): Business identifier 2954* [Communication](communication.html): Unique identifier 2955* [CommunicationRequest](communicationrequest.html): Unique identifier 2956* [Composition](composition.html): Version-independent identifier for the Composition 2957* [Condition](condition.html): A unique identifier of the condition record 2958* [Consent](consent.html): Identifier for this record (external references) 2959* [Contract](contract.html): The identity of the contract 2960* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2961* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2962* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2963* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2964* [DeviceRequest](devicerequest.html): Business identifier for request/order 2965* [DeviceUsage](deviceusage.html): Search by identifier 2966* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2967* [DocumentReference](documentreference.html): Identifier of the attachment binary 2968* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2969* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2970* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2971* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2972* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2973* [Flag](flag.html): Business identifier 2974* [Goal](goal.html): External Ids for this goal 2975* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2976* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2977* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2978* [Immunization](immunization.html): Business identifier 2979* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2980* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2981* [Invoice](invoice.html): Business Identifier for item 2982* [List](list.html): Business identifier 2983* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2984* [Medication](medication.html): Returns medications with this external identifier 2985* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2986* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2987* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2988* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2989* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2990* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2991* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2992* [Observation](observation.html): The unique id for a particular observation 2993* [Person](person.html): A person Identifier 2994* [Procedure](procedure.html): A unique identifier for a procedure 2995* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2996* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2997* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2998* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2999* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3000* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3001* [Specimen](specimen.html): The unique identifier associated with the specimen 3002* [SupplyDelivery](supplydelivery.html): External identifier 3003* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3004* [Task](task.html): Search for a task instance by its business identifier 3005* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3006</b><br> 3007 * Type: <b>token</b><br> 3008 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3009 * </p> 3010 */ 3011 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3012 public static final String SP_IDENTIFIER = "identifier"; 3013 /** 3014 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3015 * <p> 3016 * Description: <b>Multiple Resources: 3017 3018* [Account](account.html): Account number 3019* [AdverseEvent](adverseevent.html): Business identifier for the event 3020* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3021* [Appointment](appointment.html): An Identifier of the Appointment 3022* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3023* [Basic](basic.html): Business identifier 3024* [BodyStructure](bodystructure.html): Bodystructure identifier 3025* [CarePlan](careplan.html): External Ids for this plan 3026* [CareTeam](careteam.html): External Ids for this team 3027* [ChargeItem](chargeitem.html): Business Identifier for item 3028* [Claim](claim.html): The primary identifier of the financial resource 3029* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3030* [ClinicalImpression](clinicalimpression.html): Business identifier 3031* [Communication](communication.html): Unique identifier 3032* [CommunicationRequest](communicationrequest.html): Unique identifier 3033* [Composition](composition.html): Version-independent identifier for the Composition 3034* [Condition](condition.html): A unique identifier of the condition record 3035* [Consent](consent.html): Identifier for this record (external references) 3036* [Contract](contract.html): The identity of the contract 3037* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3038* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3039* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3040* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3041* [DeviceRequest](devicerequest.html): Business identifier for request/order 3042* [DeviceUsage](deviceusage.html): Search by identifier 3043* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3044* [DocumentReference](documentreference.html): Identifier of the attachment binary 3045* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3046* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3047* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3048* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3049* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3050* [Flag](flag.html): Business identifier 3051* [Goal](goal.html): External Ids for this goal 3052* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3053* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3054* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3055* [Immunization](immunization.html): Business identifier 3056* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3057* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3058* [Invoice](invoice.html): Business Identifier for item 3059* [List](list.html): Business identifier 3060* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3061* [Medication](medication.html): Returns medications with this external identifier 3062* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3063* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3064* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3065* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3066* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3067* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3068* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3069* [Observation](observation.html): The unique id for a particular observation 3070* [Person](person.html): A person Identifier 3071* [Procedure](procedure.html): A unique identifier for a procedure 3072* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3073* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3074* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3075* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3076* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3077* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3078* [Specimen](specimen.html): The unique identifier associated with the specimen 3079* [SupplyDelivery](supplydelivery.html): External identifier 3080* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3081* [Task](task.html): Search for a task instance by its business identifier 3082* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3083</b><br> 3084 * Type: <b>token</b><br> 3085 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3086 * </p> 3087 */ 3088 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3089 3090 /** 3091 * Search parameter: <b>patient</b> 3092 * <p> 3093 * Description: <b>Multiple Resources: 3094 3095* [Account](account.html): The entity that caused the expenses 3096* [AdverseEvent](adverseevent.html): Subject impacted by event 3097* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3098* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3099* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3100* [AuditEvent](auditevent.html): Where the activity involved patient data 3101* [Basic](basic.html): Identifies the focus of this resource 3102* [BodyStructure](bodystructure.html): Who this is about 3103* [CarePlan](careplan.html): Who the care plan is for 3104* [CareTeam](careteam.html): Who care team is for 3105* [ChargeItem](chargeitem.html): Individual service was done for/to 3106* [Claim](claim.html): Patient receiving the products or services 3107* [ClaimResponse](claimresponse.html): The subject of care 3108* [ClinicalImpression](clinicalimpression.html): Patient assessed 3109* [Communication](communication.html): Focus of message 3110* [CommunicationRequest](communicationrequest.html): Focus of message 3111* [Composition](composition.html): Who and/or what the composition is about 3112* [Condition](condition.html): Who has the condition? 3113* [Consent](consent.html): Who the consent applies to 3114* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3115* [Coverage](coverage.html): Retrieve coverages for a patient 3116* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3117* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3118* [DetectedIssue](detectedissue.html): Associated patient 3119* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3120* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3121* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3122* [DocumentReference](documentreference.html): Who/what is the subject of the document 3123* [Encounter](encounter.html): The patient present at the encounter 3124* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3125* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3126* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3127* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3128* [Flag](flag.html): The identity of a subject to list flags for 3129* [Goal](goal.html): Who this goal is intended for 3130* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3131* [ImagingSelection](imagingselection.html): Who the study is about 3132* [ImagingStudy](imagingstudy.html): Who the study is about 3133* [Immunization](immunization.html): The patient for the vaccination record 3134* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3135* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3136* [Invoice](invoice.html): Recipient(s) of goods and services 3137* [List](list.html): If all resources have the same subject 3138* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3139* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3140* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3141* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3142* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3143* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3144* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3145* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3146* [Observation](observation.html): The subject that the observation is about (if patient) 3147* [Person](person.html): The Person links to this Patient 3148* [Procedure](procedure.html): Search by subject - a patient 3149* [Provenance](provenance.html): Where the activity involved patient data 3150* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3151* [RelatedPerson](relatedperson.html): The patient this related person is related to 3152* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3153* [ResearchSubject](researchsubject.html): Who or what is part of study 3154* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3155* [ServiceRequest](servicerequest.html): Search by subject - a patient 3156* [Specimen](specimen.html): The patient the specimen comes from 3157* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3158* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3159* [Task](task.html): Search by patient 3160* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3161</b><br> 3162 * Type: <b>reference</b><br> 3163 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3164 * </p> 3165 */ 3166 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3167 public static final String SP_PATIENT = "patient"; 3168 /** 3169 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3170 * <p> 3171 * Description: <b>Multiple Resources: 3172 3173* [Account](account.html): The entity that caused the expenses 3174* [AdverseEvent](adverseevent.html): Subject impacted by event 3175* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3176* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3177* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3178* [AuditEvent](auditevent.html): Where the activity involved patient data 3179* [Basic](basic.html): Identifies the focus of this resource 3180* [BodyStructure](bodystructure.html): Who this is about 3181* [CarePlan](careplan.html): Who the care plan is for 3182* [CareTeam](careteam.html): Who care team is for 3183* [ChargeItem](chargeitem.html): Individual service was done for/to 3184* [Claim](claim.html): Patient receiving the products or services 3185* [ClaimResponse](claimresponse.html): The subject of care 3186* [ClinicalImpression](clinicalimpression.html): Patient assessed 3187* [Communication](communication.html): Focus of message 3188* [CommunicationRequest](communicationrequest.html): Focus of message 3189* [Composition](composition.html): Who and/or what the composition is about 3190* [Condition](condition.html): Who has the condition? 3191* [Consent](consent.html): Who the consent applies to 3192* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3193* [Coverage](coverage.html): Retrieve coverages for a patient 3194* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3195* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3196* [DetectedIssue](detectedissue.html): Associated patient 3197* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3198* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3199* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3200* [DocumentReference](documentreference.html): Who/what is the subject of the document 3201* [Encounter](encounter.html): The patient present at the encounter 3202* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3203* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3204* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3205* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3206* [Flag](flag.html): The identity of a subject to list flags for 3207* [Goal](goal.html): Who this goal is intended for 3208* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3209* [ImagingSelection](imagingselection.html): Who the study is about 3210* [ImagingStudy](imagingstudy.html): Who the study is about 3211* [Immunization](immunization.html): The patient for the vaccination record 3212* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3213* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3214* [Invoice](invoice.html): Recipient(s) of goods and services 3215* [List](list.html): If all resources have the same subject 3216* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3217* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3218* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3219* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3220* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3221* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3222* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3223* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3224* [Observation](observation.html): The subject that the observation is about (if patient) 3225* [Person](person.html): The Person links to this Patient 3226* [Procedure](procedure.html): Search by subject - a patient 3227* [Provenance](provenance.html): Where the activity involved patient data 3228* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3229* [RelatedPerson](relatedperson.html): The patient this related person is related to 3230* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3231* [ResearchSubject](researchsubject.html): Who or what is part of study 3232* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3233* [ServiceRequest](servicerequest.html): Search by subject - a patient 3234* [Specimen](specimen.html): The patient the specimen comes from 3235* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3236* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3237* [Task](task.html): Search by patient 3238* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3239</b><br> 3240 * Type: <b>reference</b><br> 3241 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3242 * </p> 3243 */ 3244 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3245 3246/** 3247 * Constant for fluent queries to be used to add include statements. Specifies 3248 * the path value of "<b>NutritionIntake:patient</b>". 3249 */ 3250 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("NutritionIntake:patient").toLocked(); 3251 3252 3253} 3254