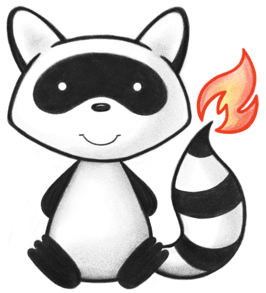
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 052 */ 053@ResourceDef(name="NutritionOrder", profile="http://hl7.org/fhir/StructureDefinition/NutritionOrder") 054public class NutritionOrder extends DomainResource { 055 056 @Block() 057 public static class NutritionOrderOralDietComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 062 @Description(shortDefinition="Type of oral diet or diet restrictions that describe what can be consumed orally", formalDefinition="The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diet-type") 064 protected List<CodeableConcept> type; 065 066 /** 067 * Schedule information for an oral diet. 068 */ 069 @Child(name = "schedule", type = {}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Scheduling information for oral diets", formalDefinition="Schedule information for an oral diet." ) 071 protected OralDietScheduleComponent schedule; 072 073 /** 074 * Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet. 075 */ 076 @Child(name = "nutrient", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 077 @Description(shortDefinition="Required nutrient modifications", formalDefinition="Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet." ) 078 protected List<NutritionOrderOralDietNutrientComponent> nutrient; 079 080 /** 081 * Class that describes any texture modifications required for the patient to safely consume various types of solid foods. 082 */ 083 @Child(name = "texture", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 084 @Description(shortDefinition="Required texture modifications", formalDefinition="Class that describes any texture modifications required for the patient to safely consume various types of solid foods." ) 085 protected List<NutritionOrderOralDietTextureComponent> texture; 086 087 /** 088 * The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient. 089 */ 090 @Child(name = "fluidConsistencyType", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 091 @Description(shortDefinition="The required consistency of fluids and liquids provided to the patient", formalDefinition="The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient." ) 092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consistency-type") 093 protected List<CodeableConcept> fluidConsistencyType; 094 095 /** 096 * Free text or additional instructions or information pertaining to the oral diet. 097 */ 098 @Child(name = "instruction", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 099 @Description(shortDefinition="Instructions or additional information about the oral diet", formalDefinition="Free text or additional instructions or information pertaining to the oral diet." ) 100 protected StringType instruction; 101 102 private static final long serialVersionUID = -1779061880L; 103 104 /** 105 * Constructor 106 */ 107 public NutritionOrderOralDietComponent() { 108 super(); 109 } 110 111 /** 112 * @return {@link #type} (The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.) 113 */ 114 public List<CodeableConcept> getType() { 115 if (this.type == null) 116 this.type = new ArrayList<CodeableConcept>(); 117 return this.type; 118 } 119 120 /** 121 * @return Returns a reference to <code>this</code> for easy method chaining 122 */ 123 public NutritionOrderOralDietComponent setType(List<CodeableConcept> theType) { 124 this.type = theType; 125 return this; 126 } 127 128 public boolean hasType() { 129 if (this.type == null) 130 return false; 131 for (CodeableConcept item : this.type) 132 if (!item.isEmpty()) 133 return true; 134 return false; 135 } 136 137 public CodeableConcept addType() { //3 138 CodeableConcept t = new CodeableConcept(); 139 if (this.type == null) 140 this.type = new ArrayList<CodeableConcept>(); 141 this.type.add(t); 142 return t; 143 } 144 145 public NutritionOrderOralDietComponent addType(CodeableConcept t) { //3 146 if (t == null) 147 return this; 148 if (this.type == null) 149 this.type = new ArrayList<CodeableConcept>(); 150 this.type.add(t); 151 return this; 152 } 153 154 /** 155 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 156 */ 157 public CodeableConcept getTypeFirstRep() { 158 if (getType().isEmpty()) { 159 addType(); 160 } 161 return getType().get(0); 162 } 163 164 /** 165 * @return {@link #schedule} (Schedule information for an oral diet.) 166 */ 167 public OralDietScheduleComponent getSchedule() { 168 if (this.schedule == null) 169 if (Configuration.errorOnAutoCreate()) 170 throw new Error("Attempt to auto-create NutritionOrderOralDietComponent.schedule"); 171 else if (Configuration.doAutoCreate()) 172 this.schedule = new OralDietScheduleComponent(); // cc 173 return this.schedule; 174 } 175 176 public boolean hasSchedule() { 177 return this.schedule != null && !this.schedule.isEmpty(); 178 } 179 180 /** 181 * @param value {@link #schedule} (Schedule information for an oral diet.) 182 */ 183 public NutritionOrderOralDietComponent setSchedule(OralDietScheduleComponent value) { 184 this.schedule = value; 185 return this; 186 } 187 188 /** 189 * @return {@link #nutrient} (Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.) 190 */ 191 public List<NutritionOrderOralDietNutrientComponent> getNutrient() { 192 if (this.nutrient == null) 193 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 194 return this.nutrient; 195 } 196 197 /** 198 * @return Returns a reference to <code>this</code> for easy method chaining 199 */ 200 public NutritionOrderOralDietComponent setNutrient(List<NutritionOrderOralDietNutrientComponent> theNutrient) { 201 this.nutrient = theNutrient; 202 return this; 203 } 204 205 public boolean hasNutrient() { 206 if (this.nutrient == null) 207 return false; 208 for (NutritionOrderOralDietNutrientComponent item : this.nutrient) 209 if (!item.isEmpty()) 210 return true; 211 return false; 212 } 213 214 public NutritionOrderOralDietNutrientComponent addNutrient() { //3 215 NutritionOrderOralDietNutrientComponent t = new NutritionOrderOralDietNutrientComponent(); 216 if (this.nutrient == null) 217 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 218 this.nutrient.add(t); 219 return t; 220 } 221 222 public NutritionOrderOralDietComponent addNutrient(NutritionOrderOralDietNutrientComponent t) { //3 223 if (t == null) 224 return this; 225 if (this.nutrient == null) 226 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 227 this.nutrient.add(t); 228 return this; 229 } 230 231 /** 232 * @return The first repetition of repeating field {@link #nutrient}, creating it if it does not already exist {3} 233 */ 234 public NutritionOrderOralDietNutrientComponent getNutrientFirstRep() { 235 if (getNutrient().isEmpty()) { 236 addNutrient(); 237 } 238 return getNutrient().get(0); 239 } 240 241 /** 242 * @return {@link #texture} (Class that describes any texture modifications required for the patient to safely consume various types of solid foods.) 243 */ 244 public List<NutritionOrderOralDietTextureComponent> getTexture() { 245 if (this.texture == null) 246 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 247 return this.texture; 248 } 249 250 /** 251 * @return Returns a reference to <code>this</code> for easy method chaining 252 */ 253 public NutritionOrderOralDietComponent setTexture(List<NutritionOrderOralDietTextureComponent> theTexture) { 254 this.texture = theTexture; 255 return this; 256 } 257 258 public boolean hasTexture() { 259 if (this.texture == null) 260 return false; 261 for (NutritionOrderOralDietTextureComponent item : this.texture) 262 if (!item.isEmpty()) 263 return true; 264 return false; 265 } 266 267 public NutritionOrderOralDietTextureComponent addTexture() { //3 268 NutritionOrderOralDietTextureComponent t = new NutritionOrderOralDietTextureComponent(); 269 if (this.texture == null) 270 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 271 this.texture.add(t); 272 return t; 273 } 274 275 public NutritionOrderOralDietComponent addTexture(NutritionOrderOralDietTextureComponent t) { //3 276 if (t == null) 277 return this; 278 if (this.texture == null) 279 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 280 this.texture.add(t); 281 return this; 282 } 283 284 /** 285 * @return The first repetition of repeating field {@link #texture}, creating it if it does not already exist {3} 286 */ 287 public NutritionOrderOralDietTextureComponent getTextureFirstRep() { 288 if (getTexture().isEmpty()) { 289 addTexture(); 290 } 291 return getTexture().get(0); 292 } 293 294 /** 295 * @return {@link #fluidConsistencyType} (The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.) 296 */ 297 public List<CodeableConcept> getFluidConsistencyType() { 298 if (this.fluidConsistencyType == null) 299 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 300 return this.fluidConsistencyType; 301 } 302 303 /** 304 * @return Returns a reference to <code>this</code> for easy method chaining 305 */ 306 public NutritionOrderOralDietComponent setFluidConsistencyType(List<CodeableConcept> theFluidConsistencyType) { 307 this.fluidConsistencyType = theFluidConsistencyType; 308 return this; 309 } 310 311 public boolean hasFluidConsistencyType() { 312 if (this.fluidConsistencyType == null) 313 return false; 314 for (CodeableConcept item : this.fluidConsistencyType) 315 if (!item.isEmpty()) 316 return true; 317 return false; 318 } 319 320 public CodeableConcept addFluidConsistencyType() { //3 321 CodeableConcept t = new CodeableConcept(); 322 if (this.fluidConsistencyType == null) 323 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 324 this.fluidConsistencyType.add(t); 325 return t; 326 } 327 328 public NutritionOrderOralDietComponent addFluidConsistencyType(CodeableConcept t) { //3 329 if (t == null) 330 return this; 331 if (this.fluidConsistencyType == null) 332 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 333 this.fluidConsistencyType.add(t); 334 return this; 335 } 336 337 /** 338 * @return The first repetition of repeating field {@link #fluidConsistencyType}, creating it if it does not already exist {3} 339 */ 340 public CodeableConcept getFluidConsistencyTypeFirstRep() { 341 if (getFluidConsistencyType().isEmpty()) { 342 addFluidConsistencyType(); 343 } 344 return getFluidConsistencyType().get(0); 345 } 346 347 /** 348 * @return {@link #instruction} (Free text or additional instructions or information pertaining to the oral diet.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 349 */ 350 public StringType getInstructionElement() { 351 if (this.instruction == null) 352 if (Configuration.errorOnAutoCreate()) 353 throw new Error("Attempt to auto-create NutritionOrderOralDietComponent.instruction"); 354 else if (Configuration.doAutoCreate()) 355 this.instruction = new StringType(); // bb 356 return this.instruction; 357 } 358 359 public boolean hasInstructionElement() { 360 return this.instruction != null && !this.instruction.isEmpty(); 361 } 362 363 public boolean hasInstruction() { 364 return this.instruction != null && !this.instruction.isEmpty(); 365 } 366 367 /** 368 * @param value {@link #instruction} (Free text or additional instructions or information pertaining to the oral diet.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 369 */ 370 public NutritionOrderOralDietComponent setInstructionElement(StringType value) { 371 this.instruction = value; 372 return this; 373 } 374 375 /** 376 * @return Free text or additional instructions or information pertaining to the oral diet. 377 */ 378 public String getInstruction() { 379 return this.instruction == null ? null : this.instruction.getValue(); 380 } 381 382 /** 383 * @param value Free text or additional instructions or information pertaining to the oral diet. 384 */ 385 public NutritionOrderOralDietComponent setInstruction(String value) { 386 if (Utilities.noString(value)) 387 this.instruction = null; 388 else { 389 if (this.instruction == null) 390 this.instruction = new StringType(); 391 this.instruction.setValue(value); 392 } 393 return this; 394 } 395 396 protected void listChildren(List<Property> children) { 397 super.listChildren(children); 398 children.add(new Property("type", "CodeableConcept", "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, java.lang.Integer.MAX_VALUE, type)); 399 children.add(new Property("schedule", "", "Schedule information for an oral diet.", 0, 1, schedule)); 400 children.add(new Property("nutrient", "", "Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.", 0, java.lang.Integer.MAX_VALUE, nutrient)); 401 children.add(new Property("texture", "", "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 0, java.lang.Integer.MAX_VALUE, texture)); 402 children.add(new Property("fluidConsistencyType", "CodeableConcept", "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType)); 403 children.add(new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral diet.", 0, 1, instruction)); 404 } 405 406 @Override 407 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 408 switch (_hash) { 409 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, java.lang.Integer.MAX_VALUE, type); 410 case -697920873: /*schedule*/ return new Property("schedule", "", "Schedule information for an oral diet.", 0, 1, schedule); 411 case -1671151641: /*nutrient*/ return new Property("nutrient", "", "Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.", 0, java.lang.Integer.MAX_VALUE, nutrient); 412 case -1417816805: /*texture*/ return new Property("texture", "", "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 0, java.lang.Integer.MAX_VALUE, texture); 413 case -525105592: /*fluidConsistencyType*/ return new Property("fluidConsistencyType", "CodeableConcept", "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType); 414 case 301526158: /*instruction*/ return new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral diet.", 0, 1, instruction); 415 default: return super.getNamedProperty(_hash, _name, _checkValid); 416 } 417 418 } 419 420 @Override 421 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 422 switch (hash) { 423 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 424 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : new Base[] {this.schedule}; // OralDietScheduleComponent 425 case -1671151641: /*nutrient*/ return this.nutrient == null ? new Base[0] : this.nutrient.toArray(new Base[this.nutrient.size()]); // NutritionOrderOralDietNutrientComponent 426 case -1417816805: /*texture*/ return this.texture == null ? new Base[0] : this.texture.toArray(new Base[this.texture.size()]); // NutritionOrderOralDietTextureComponent 427 case -525105592: /*fluidConsistencyType*/ return this.fluidConsistencyType == null ? new Base[0] : this.fluidConsistencyType.toArray(new Base[this.fluidConsistencyType.size()]); // CodeableConcept 428 case 301526158: /*instruction*/ return this.instruction == null ? new Base[0] : new Base[] {this.instruction}; // StringType 429 default: return super.getProperty(hash, name, checkValid); 430 } 431 432 } 433 434 @Override 435 public Base setProperty(int hash, String name, Base value) throws FHIRException { 436 switch (hash) { 437 case 3575610: // type 438 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 439 return value; 440 case -697920873: // schedule 441 this.schedule = (OralDietScheduleComponent) value; // OralDietScheduleComponent 442 return value; 443 case -1671151641: // nutrient 444 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); // NutritionOrderOralDietNutrientComponent 445 return value; 446 case -1417816805: // texture 447 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); // NutritionOrderOralDietTextureComponent 448 return value; 449 case -525105592: // fluidConsistencyType 450 this.getFluidConsistencyType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 451 return value; 452 case 301526158: // instruction 453 this.instruction = TypeConvertor.castToString(value); // StringType 454 return value; 455 default: return super.setProperty(hash, name, value); 456 } 457 458 } 459 460 @Override 461 public Base setProperty(String name, Base value) throws FHIRException { 462 if (name.equals("type")) { 463 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 464 } else if (name.equals("schedule")) { 465 this.schedule = (OralDietScheduleComponent) value; // OralDietScheduleComponent 466 } else if (name.equals("nutrient")) { 467 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); 468 } else if (name.equals("texture")) { 469 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); 470 } else if (name.equals("fluidConsistencyType")) { 471 this.getFluidConsistencyType().add(TypeConvertor.castToCodeableConcept(value)); 472 } else if (name.equals("instruction")) { 473 this.instruction = TypeConvertor.castToString(value); // StringType 474 } else 475 return super.setProperty(name, value); 476 return value; 477 } 478 479 @Override 480 public void removeChild(String name, Base value) throws FHIRException { 481 if (name.equals("type")) { 482 this.getType().remove(value); 483 } else if (name.equals("schedule")) { 484 this.schedule = (OralDietScheduleComponent) value; // OralDietScheduleComponent 485 } else if (name.equals("nutrient")) { 486 this.getNutrient().remove((NutritionOrderOralDietNutrientComponent) value); 487 } else if (name.equals("texture")) { 488 this.getTexture().remove((NutritionOrderOralDietTextureComponent) value); 489 } else if (name.equals("fluidConsistencyType")) { 490 this.getFluidConsistencyType().remove(value); 491 } else if (name.equals("instruction")) { 492 this.instruction = null; 493 } else 494 super.removeChild(name, value); 495 496 } 497 498 @Override 499 public Base makeProperty(int hash, String name) throws FHIRException { 500 switch (hash) { 501 case 3575610: return addType(); 502 case -697920873: return getSchedule(); 503 case -1671151641: return addNutrient(); 504 case -1417816805: return addTexture(); 505 case -525105592: return addFluidConsistencyType(); 506 case 301526158: return getInstructionElement(); 507 default: return super.makeProperty(hash, name); 508 } 509 510 } 511 512 @Override 513 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 514 switch (hash) { 515 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 516 case -697920873: /*schedule*/ return new String[] {}; 517 case -1671151641: /*nutrient*/ return new String[] {}; 518 case -1417816805: /*texture*/ return new String[] {}; 519 case -525105592: /*fluidConsistencyType*/ return new String[] {"CodeableConcept"}; 520 case 301526158: /*instruction*/ return new String[] {"string"}; 521 default: return super.getTypesForProperty(hash, name); 522 } 523 524 } 525 526 @Override 527 public Base addChild(String name) throws FHIRException { 528 if (name.equals("type")) { 529 return addType(); 530 } 531 else if (name.equals("schedule")) { 532 this.schedule = new OralDietScheduleComponent(); 533 return this.schedule; 534 } 535 else if (name.equals("nutrient")) { 536 return addNutrient(); 537 } 538 else if (name.equals("texture")) { 539 return addTexture(); 540 } 541 else if (name.equals("fluidConsistencyType")) { 542 return addFluidConsistencyType(); 543 } 544 else if (name.equals("instruction")) { 545 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.oralDiet.instruction"); 546 } 547 else 548 return super.addChild(name); 549 } 550 551 public NutritionOrderOralDietComponent copy() { 552 NutritionOrderOralDietComponent dst = new NutritionOrderOralDietComponent(); 553 copyValues(dst); 554 return dst; 555 } 556 557 public void copyValues(NutritionOrderOralDietComponent dst) { 558 super.copyValues(dst); 559 if (type != null) { 560 dst.type = new ArrayList<CodeableConcept>(); 561 for (CodeableConcept i : type) 562 dst.type.add(i.copy()); 563 }; 564 dst.schedule = schedule == null ? null : schedule.copy(); 565 if (nutrient != null) { 566 dst.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 567 for (NutritionOrderOralDietNutrientComponent i : nutrient) 568 dst.nutrient.add(i.copy()); 569 }; 570 if (texture != null) { 571 dst.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 572 for (NutritionOrderOralDietTextureComponent i : texture) 573 dst.texture.add(i.copy()); 574 }; 575 if (fluidConsistencyType != null) { 576 dst.fluidConsistencyType = new ArrayList<CodeableConcept>(); 577 for (CodeableConcept i : fluidConsistencyType) 578 dst.fluidConsistencyType.add(i.copy()); 579 }; 580 dst.instruction = instruction == null ? null : instruction.copy(); 581 } 582 583 @Override 584 public boolean equalsDeep(Base other_) { 585 if (!super.equalsDeep(other_)) 586 return false; 587 if (!(other_ instanceof NutritionOrderOralDietComponent)) 588 return false; 589 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other_; 590 return compareDeep(type, o.type, true) && compareDeep(schedule, o.schedule, true) && compareDeep(nutrient, o.nutrient, true) 591 && compareDeep(texture, o.texture, true) && compareDeep(fluidConsistencyType, o.fluidConsistencyType, true) 592 && compareDeep(instruction, o.instruction, true); 593 } 594 595 @Override 596 public boolean equalsShallow(Base other_) { 597 if (!super.equalsShallow(other_)) 598 return false; 599 if (!(other_ instanceof NutritionOrderOralDietComponent)) 600 return false; 601 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other_; 602 return compareValues(instruction, o.instruction, true); 603 } 604 605 public boolean isEmpty() { 606 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, schedule, nutrient 607 , texture, fluidConsistencyType, instruction); 608 } 609 610 public String fhirType() { 611 return "NutritionOrder.oralDiet"; 612 613 } 614 615 } 616 617 @Block() 618 public static class OralDietScheduleComponent extends BackboneElement implements IBaseBackboneElement { 619 /** 620 * The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present. 621 */ 622 @Child(name = "timing", type = {Timing.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 623 @Description(shortDefinition="Scheduled frequency of diet", formalDefinition="The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present." ) 624 protected List<Timing> timing; 625 626 /** 627 * Indicates whether the product is only taken when needed within a specific dosing schedule. 628 */ 629 @Child(name = "asNeeded", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 630 @Description(shortDefinition="Take 'as needed'", formalDefinition="Indicates whether the product is only taken when needed within a specific dosing schedule." ) 631 protected BooleanType asNeeded; 632 633 /** 634 * Indicates whether the product is only taken based on a precondition for taking the product. 635 */ 636 @Child(name = "asNeededFor", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 637 @Description(shortDefinition="Take 'as needed' for x", formalDefinition="Indicates whether the product is only taken based on a precondition for taking the product." ) 638 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 639 protected CodeableConcept asNeededFor; 640 641 private static final long serialVersionUID = -1051458478L; 642 643 /** 644 * Constructor 645 */ 646 public OralDietScheduleComponent() { 647 super(); 648 } 649 650 /** 651 * @return {@link #timing} (The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.) 652 */ 653 public List<Timing> getTiming() { 654 if (this.timing == null) 655 this.timing = new ArrayList<Timing>(); 656 return this.timing; 657 } 658 659 /** 660 * @return Returns a reference to <code>this</code> for easy method chaining 661 */ 662 public OralDietScheduleComponent setTiming(List<Timing> theTiming) { 663 this.timing = theTiming; 664 return this; 665 } 666 667 public boolean hasTiming() { 668 if (this.timing == null) 669 return false; 670 for (Timing item : this.timing) 671 if (!item.isEmpty()) 672 return true; 673 return false; 674 } 675 676 public Timing addTiming() { //3 677 Timing t = new Timing(); 678 if (this.timing == null) 679 this.timing = new ArrayList<Timing>(); 680 this.timing.add(t); 681 return t; 682 } 683 684 public OralDietScheduleComponent addTiming(Timing t) { //3 685 if (t == null) 686 return this; 687 if (this.timing == null) 688 this.timing = new ArrayList<Timing>(); 689 this.timing.add(t); 690 return this; 691 } 692 693 /** 694 * @return The first repetition of repeating field {@link #timing}, creating it if it does not already exist {3} 695 */ 696 public Timing getTimingFirstRep() { 697 if (getTiming().isEmpty()) { 698 addTiming(); 699 } 700 return getTiming().get(0); 701 } 702 703 /** 704 * @return {@link #asNeeded} (Indicates whether the product is only taken when needed within a specific dosing schedule.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 705 */ 706 public BooleanType getAsNeededElement() { 707 if (this.asNeeded == null) 708 if (Configuration.errorOnAutoCreate()) 709 throw new Error("Attempt to auto-create OralDietScheduleComponent.asNeeded"); 710 else if (Configuration.doAutoCreate()) 711 this.asNeeded = new BooleanType(); // bb 712 return this.asNeeded; 713 } 714 715 public boolean hasAsNeededElement() { 716 return this.asNeeded != null && !this.asNeeded.isEmpty(); 717 } 718 719 public boolean hasAsNeeded() { 720 return this.asNeeded != null && !this.asNeeded.isEmpty(); 721 } 722 723 /** 724 * @param value {@link #asNeeded} (Indicates whether the product is only taken when needed within a specific dosing schedule.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 725 */ 726 public OralDietScheduleComponent setAsNeededElement(BooleanType value) { 727 this.asNeeded = value; 728 return this; 729 } 730 731 /** 732 * @return Indicates whether the product is only taken when needed within a specific dosing schedule. 733 */ 734 public boolean getAsNeeded() { 735 return this.asNeeded == null || this.asNeeded.isEmpty() ? false : this.asNeeded.getValue(); 736 } 737 738 /** 739 * @param value Indicates whether the product is only taken when needed within a specific dosing schedule. 740 */ 741 public OralDietScheduleComponent setAsNeeded(boolean value) { 742 if (this.asNeeded == null) 743 this.asNeeded = new BooleanType(); 744 this.asNeeded.setValue(value); 745 return this; 746 } 747 748 /** 749 * @return {@link #asNeededFor} (Indicates whether the product is only taken based on a precondition for taking the product.) 750 */ 751 public CodeableConcept getAsNeededFor() { 752 if (this.asNeededFor == null) 753 if (Configuration.errorOnAutoCreate()) 754 throw new Error("Attempt to auto-create OralDietScheduleComponent.asNeededFor"); 755 else if (Configuration.doAutoCreate()) 756 this.asNeededFor = new CodeableConcept(); // cc 757 return this.asNeededFor; 758 } 759 760 public boolean hasAsNeededFor() { 761 return this.asNeededFor != null && !this.asNeededFor.isEmpty(); 762 } 763 764 /** 765 * @param value {@link #asNeededFor} (Indicates whether the product is only taken based on a precondition for taking the product.) 766 */ 767 public OralDietScheduleComponent setAsNeededFor(CodeableConcept value) { 768 this.asNeededFor = value; 769 return this; 770 } 771 772 protected void listChildren(List<Property> children) { 773 super.listChildren(children); 774 children.add(new Property("timing", "Timing", "The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, timing)); 775 children.add(new Property("asNeeded", "boolean", "Indicates whether the product is only taken when needed within a specific dosing schedule.", 0, 1, asNeeded)); 776 children.add(new Property("asNeededFor", "CodeableConcept", "Indicates whether the product is only taken based on a precondition for taking the product.", 0, 1, asNeededFor)); 777 } 778 779 @Override 780 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 781 switch (_hash) { 782 case -873664438: /*timing*/ return new Property("timing", "Timing", "The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, timing); 783 case -1432923513: /*asNeeded*/ return new Property("asNeeded", "boolean", "Indicates whether the product is only taken when needed within a specific dosing schedule.", 0, 1, asNeeded); 784 case -544350014: /*asNeededFor*/ return new Property("asNeededFor", "CodeableConcept", "Indicates whether the product is only taken based on a precondition for taking the product.", 0, 1, asNeededFor); 785 default: return super.getNamedProperty(_hash, _name, _checkValid); 786 } 787 788 } 789 790 @Override 791 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 792 switch (hash) { 793 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : this.timing.toArray(new Base[this.timing.size()]); // Timing 794 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // BooleanType 795 case -544350014: /*asNeededFor*/ return this.asNeededFor == null ? new Base[0] : new Base[] {this.asNeededFor}; // CodeableConcept 796 default: return super.getProperty(hash, name, checkValid); 797 } 798 799 } 800 801 @Override 802 public Base setProperty(int hash, String name, Base value) throws FHIRException { 803 switch (hash) { 804 case -873664438: // timing 805 this.getTiming().add(TypeConvertor.castToTiming(value)); // Timing 806 return value; 807 case -1432923513: // asNeeded 808 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 809 return value; 810 case -544350014: // asNeededFor 811 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 812 return value; 813 default: return super.setProperty(hash, name, value); 814 } 815 816 } 817 818 @Override 819 public Base setProperty(String name, Base value) throws FHIRException { 820 if (name.equals("timing")) { 821 this.getTiming().add(TypeConvertor.castToTiming(value)); 822 } else if (name.equals("asNeeded")) { 823 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 824 } else if (name.equals("asNeededFor")) { 825 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 826 } else 827 return super.setProperty(name, value); 828 return value; 829 } 830 831 @Override 832 public void removeChild(String name, Base value) throws FHIRException { 833 if (name.equals("timing")) { 834 this.getTiming().remove(value); 835 } else if (name.equals("asNeeded")) { 836 this.asNeeded = null; 837 } else if (name.equals("asNeededFor")) { 838 this.asNeededFor = null; 839 } else 840 super.removeChild(name, value); 841 842 } 843 844 @Override 845 public Base makeProperty(int hash, String name) throws FHIRException { 846 switch (hash) { 847 case -873664438: return addTiming(); 848 case -1432923513: return getAsNeededElement(); 849 case -544350014: return getAsNeededFor(); 850 default: return super.makeProperty(hash, name); 851 } 852 853 } 854 855 @Override 856 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 857 switch (hash) { 858 case -873664438: /*timing*/ return new String[] {"Timing"}; 859 case -1432923513: /*asNeeded*/ return new String[] {"boolean"}; 860 case -544350014: /*asNeededFor*/ return new String[] {"CodeableConcept"}; 861 default: return super.getTypesForProperty(hash, name); 862 } 863 864 } 865 866 @Override 867 public Base addChild(String name) throws FHIRException { 868 if (name.equals("timing")) { 869 return addTiming(); 870 } 871 else if (name.equals("asNeeded")) { 872 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.oralDiet.schedule.asNeeded"); 873 } 874 else if (name.equals("asNeededFor")) { 875 this.asNeededFor = new CodeableConcept(); 876 return this.asNeededFor; 877 } 878 else 879 return super.addChild(name); 880 } 881 882 public OralDietScheduleComponent copy() { 883 OralDietScheduleComponent dst = new OralDietScheduleComponent(); 884 copyValues(dst); 885 return dst; 886 } 887 888 public void copyValues(OralDietScheduleComponent dst) { 889 super.copyValues(dst); 890 if (timing != null) { 891 dst.timing = new ArrayList<Timing>(); 892 for (Timing i : timing) 893 dst.timing.add(i.copy()); 894 }; 895 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 896 dst.asNeededFor = asNeededFor == null ? null : asNeededFor.copy(); 897 } 898 899 @Override 900 public boolean equalsDeep(Base other_) { 901 if (!super.equalsDeep(other_)) 902 return false; 903 if (!(other_ instanceof OralDietScheduleComponent)) 904 return false; 905 OralDietScheduleComponent o = (OralDietScheduleComponent) other_; 906 return compareDeep(timing, o.timing, true) && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(asNeededFor, o.asNeededFor, true) 907 ; 908 } 909 910 @Override 911 public boolean equalsShallow(Base other_) { 912 if (!super.equalsShallow(other_)) 913 return false; 914 if (!(other_ instanceof OralDietScheduleComponent)) 915 return false; 916 OralDietScheduleComponent o = (OralDietScheduleComponent) other_; 917 return compareValues(asNeeded, o.asNeeded, true); 918 } 919 920 public boolean isEmpty() { 921 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(timing, asNeeded, asNeededFor 922 ); 923 } 924 925 public String fhirType() { 926 return "NutritionOrder.oralDiet.schedule"; 927 928 } 929 930 } 931 932 @Block() 933 public static class NutritionOrderOralDietNutrientComponent extends BackboneElement implements IBaseBackboneElement { 934 /** 935 * The nutrient that is being modified such as carbohydrate or sodium. 936 */ 937 @Child(name = "modifier", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 938 @Description(shortDefinition="Type of nutrient that is being modified", formalDefinition="The nutrient that is being modified such as carbohydrate or sodium." ) 939 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/nutrient-code") 940 protected CodeableConcept modifier; 941 942 /** 943 * The quantity of the specified nutrient to include in diet. 944 */ 945 @Child(name = "amount", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 946 @Description(shortDefinition="Quantity of the specified nutrient", formalDefinition="The quantity of the specified nutrient to include in diet." ) 947 protected Quantity amount; 948 949 private static final long serialVersionUID = 1042462093L; 950 951 /** 952 * Constructor 953 */ 954 public NutritionOrderOralDietNutrientComponent() { 955 super(); 956 } 957 958 /** 959 * @return {@link #modifier} (The nutrient that is being modified such as carbohydrate or sodium.) 960 */ 961 public CodeableConcept getModifier() { 962 if (this.modifier == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.modifier"); 965 else if (Configuration.doAutoCreate()) 966 this.modifier = new CodeableConcept(); // cc 967 return this.modifier; 968 } 969 970 public boolean hasModifier() { 971 return this.modifier != null && !this.modifier.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #modifier} (The nutrient that is being modified such as carbohydrate or sodium.) 976 */ 977 public NutritionOrderOralDietNutrientComponent setModifier(CodeableConcept value) { 978 this.modifier = value; 979 return this; 980 } 981 982 /** 983 * @return {@link #amount} (The quantity of the specified nutrient to include in diet.) 984 */ 985 public Quantity getAmount() { 986 if (this.amount == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.amount"); 989 else if (Configuration.doAutoCreate()) 990 this.amount = new Quantity(); // cc 991 return this.amount; 992 } 993 994 public boolean hasAmount() { 995 return this.amount != null && !this.amount.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #amount} (The quantity of the specified nutrient to include in diet.) 1000 */ 1001 public NutritionOrderOralDietNutrientComponent setAmount(Quantity value) { 1002 this.amount = value; 1003 return this; 1004 } 1005 1006 protected void listChildren(List<Property> children) { 1007 super.listChildren(children); 1008 children.add(new Property("modifier", "CodeableConcept", "The nutrient that is being modified such as carbohydrate or sodium.", 0, 1, modifier)); 1009 children.add(new Property("amount", "Quantity", "The quantity of the specified nutrient to include in diet.", 0, 1, amount)); 1010 } 1011 1012 @Override 1013 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1014 switch (_hash) { 1015 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "The nutrient that is being modified such as carbohydrate or sodium.", 0, 1, modifier); 1016 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "The quantity of the specified nutrient to include in diet.", 0, 1, amount); 1017 default: return super.getNamedProperty(_hash, _name, _checkValid); 1018 } 1019 1020 } 1021 1022 @Override 1023 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1024 switch (hash) { 1025 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : new Base[] {this.modifier}; // CodeableConcept 1026 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Quantity 1027 default: return super.getProperty(hash, name, checkValid); 1028 } 1029 1030 } 1031 1032 @Override 1033 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1034 switch (hash) { 1035 case -615513385: // modifier 1036 this.modifier = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1037 return value; 1038 case -1413853096: // amount 1039 this.amount = TypeConvertor.castToQuantity(value); // Quantity 1040 return value; 1041 default: return super.setProperty(hash, name, value); 1042 } 1043 1044 } 1045 1046 @Override 1047 public Base setProperty(String name, Base value) throws FHIRException { 1048 if (name.equals("modifier")) { 1049 this.modifier = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1050 } else if (name.equals("amount")) { 1051 this.amount = TypeConvertor.castToQuantity(value); // Quantity 1052 } else 1053 return super.setProperty(name, value); 1054 return value; 1055 } 1056 1057 @Override 1058 public void removeChild(String name, Base value) throws FHIRException { 1059 if (name.equals("modifier")) { 1060 this.modifier = null; 1061 } else if (name.equals("amount")) { 1062 this.amount = null; 1063 } else 1064 super.removeChild(name, value); 1065 1066 } 1067 1068 @Override 1069 public Base makeProperty(int hash, String name) throws FHIRException { 1070 switch (hash) { 1071 case -615513385: return getModifier(); 1072 case -1413853096: return getAmount(); 1073 default: return super.makeProperty(hash, name); 1074 } 1075 1076 } 1077 1078 @Override 1079 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1080 switch (hash) { 1081 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 1082 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 1083 default: return super.getTypesForProperty(hash, name); 1084 } 1085 1086 } 1087 1088 @Override 1089 public Base addChild(String name) throws FHIRException { 1090 if (name.equals("modifier")) { 1091 this.modifier = new CodeableConcept(); 1092 return this.modifier; 1093 } 1094 else if (name.equals("amount")) { 1095 this.amount = new Quantity(); 1096 return this.amount; 1097 } 1098 else 1099 return super.addChild(name); 1100 } 1101 1102 public NutritionOrderOralDietNutrientComponent copy() { 1103 NutritionOrderOralDietNutrientComponent dst = new NutritionOrderOralDietNutrientComponent(); 1104 copyValues(dst); 1105 return dst; 1106 } 1107 1108 public void copyValues(NutritionOrderOralDietNutrientComponent dst) { 1109 super.copyValues(dst); 1110 dst.modifier = modifier == null ? null : modifier.copy(); 1111 dst.amount = amount == null ? null : amount.copy(); 1112 } 1113 1114 @Override 1115 public boolean equalsDeep(Base other_) { 1116 if (!super.equalsDeep(other_)) 1117 return false; 1118 if (!(other_ instanceof NutritionOrderOralDietNutrientComponent)) 1119 return false; 1120 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other_; 1121 return compareDeep(modifier, o.modifier, true) && compareDeep(amount, o.amount, true); 1122 } 1123 1124 @Override 1125 public boolean equalsShallow(Base other_) { 1126 if (!super.equalsShallow(other_)) 1127 return false; 1128 if (!(other_ instanceof NutritionOrderOralDietNutrientComponent)) 1129 return false; 1130 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other_; 1131 return true; 1132 } 1133 1134 public boolean isEmpty() { 1135 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifier, amount); 1136 } 1137 1138 public String fhirType() { 1139 return "NutritionOrder.oralDiet.nutrient"; 1140 1141 } 1142 1143 } 1144 1145 @Block() 1146 public static class NutritionOrderOralDietTextureComponent extends BackboneElement implements IBaseBackboneElement { 1147 /** 1148 * Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed. 1149 */ 1150 @Child(name = "modifier", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1151 @Description(shortDefinition="Code to indicate how to alter the texture of the foods, e.g. pureed", formalDefinition="Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed." ) 1152 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/texture-code") 1153 protected CodeableConcept modifier; 1154 1155 /** 1156 * The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types. 1157 */ 1158 @Child(name = "foodType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1159 @Description(shortDefinition="Concepts that are used to identify an entity that is ingested for nutritional purposes", formalDefinition="The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types." ) 1160 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/modified-foodtype") 1161 protected CodeableConcept foodType; 1162 1163 private static final long serialVersionUID = -56402817L; 1164 1165 /** 1166 * Constructor 1167 */ 1168 public NutritionOrderOralDietTextureComponent() { 1169 super(); 1170 } 1171 1172 /** 1173 * @return {@link #modifier} (Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.) 1174 */ 1175 public CodeableConcept getModifier() { 1176 if (this.modifier == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.modifier"); 1179 else if (Configuration.doAutoCreate()) 1180 this.modifier = new CodeableConcept(); // cc 1181 return this.modifier; 1182 } 1183 1184 public boolean hasModifier() { 1185 return this.modifier != null && !this.modifier.isEmpty(); 1186 } 1187 1188 /** 1189 * @param value {@link #modifier} (Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.) 1190 */ 1191 public NutritionOrderOralDietTextureComponent setModifier(CodeableConcept value) { 1192 this.modifier = value; 1193 return this; 1194 } 1195 1196 /** 1197 * @return {@link #foodType} (The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.) 1198 */ 1199 public CodeableConcept getFoodType() { 1200 if (this.foodType == null) 1201 if (Configuration.errorOnAutoCreate()) 1202 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.foodType"); 1203 else if (Configuration.doAutoCreate()) 1204 this.foodType = new CodeableConcept(); // cc 1205 return this.foodType; 1206 } 1207 1208 public boolean hasFoodType() { 1209 return this.foodType != null && !this.foodType.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #foodType} (The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.) 1214 */ 1215 public NutritionOrderOralDietTextureComponent setFoodType(CodeableConcept value) { 1216 this.foodType = value; 1217 return this; 1218 } 1219 1220 protected void listChildren(List<Property> children) { 1221 super.listChildren(children); 1222 children.add(new Property("modifier", "CodeableConcept", "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 0, 1, modifier)); 1223 children.add(new Property("foodType", "CodeableConcept", "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 0, 1, foodType)); 1224 } 1225 1226 @Override 1227 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1228 switch (_hash) { 1229 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 0, 1, modifier); 1230 case 379498680: /*foodType*/ return new Property("foodType", "CodeableConcept", "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 0, 1, foodType); 1231 default: return super.getNamedProperty(_hash, _name, _checkValid); 1232 } 1233 1234 } 1235 1236 @Override 1237 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1238 switch (hash) { 1239 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : new Base[] {this.modifier}; // CodeableConcept 1240 case 379498680: /*foodType*/ return this.foodType == null ? new Base[0] : new Base[] {this.foodType}; // CodeableConcept 1241 default: return super.getProperty(hash, name, checkValid); 1242 } 1243 1244 } 1245 1246 @Override 1247 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1248 switch (hash) { 1249 case -615513385: // modifier 1250 this.modifier = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1251 return value; 1252 case 379498680: // foodType 1253 this.foodType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1254 return value; 1255 default: return super.setProperty(hash, name, value); 1256 } 1257 1258 } 1259 1260 @Override 1261 public Base setProperty(String name, Base value) throws FHIRException { 1262 if (name.equals("modifier")) { 1263 this.modifier = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1264 } else if (name.equals("foodType")) { 1265 this.foodType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1266 } else 1267 return super.setProperty(name, value); 1268 return value; 1269 } 1270 1271 @Override 1272 public void removeChild(String name, Base value) throws FHIRException { 1273 if (name.equals("modifier")) { 1274 this.modifier = null; 1275 } else if (name.equals("foodType")) { 1276 this.foodType = null; 1277 } else 1278 super.removeChild(name, value); 1279 1280 } 1281 1282 @Override 1283 public Base makeProperty(int hash, String name) throws FHIRException { 1284 switch (hash) { 1285 case -615513385: return getModifier(); 1286 case 379498680: return getFoodType(); 1287 default: return super.makeProperty(hash, name); 1288 } 1289 1290 } 1291 1292 @Override 1293 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1294 switch (hash) { 1295 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 1296 case 379498680: /*foodType*/ return new String[] {"CodeableConcept"}; 1297 default: return super.getTypesForProperty(hash, name); 1298 } 1299 1300 } 1301 1302 @Override 1303 public Base addChild(String name) throws FHIRException { 1304 if (name.equals("modifier")) { 1305 this.modifier = new CodeableConcept(); 1306 return this.modifier; 1307 } 1308 else if (name.equals("foodType")) { 1309 this.foodType = new CodeableConcept(); 1310 return this.foodType; 1311 } 1312 else 1313 return super.addChild(name); 1314 } 1315 1316 public NutritionOrderOralDietTextureComponent copy() { 1317 NutritionOrderOralDietTextureComponent dst = new NutritionOrderOralDietTextureComponent(); 1318 copyValues(dst); 1319 return dst; 1320 } 1321 1322 public void copyValues(NutritionOrderOralDietTextureComponent dst) { 1323 super.copyValues(dst); 1324 dst.modifier = modifier == null ? null : modifier.copy(); 1325 dst.foodType = foodType == null ? null : foodType.copy(); 1326 } 1327 1328 @Override 1329 public boolean equalsDeep(Base other_) { 1330 if (!super.equalsDeep(other_)) 1331 return false; 1332 if (!(other_ instanceof NutritionOrderOralDietTextureComponent)) 1333 return false; 1334 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other_; 1335 return compareDeep(modifier, o.modifier, true) && compareDeep(foodType, o.foodType, true); 1336 } 1337 1338 @Override 1339 public boolean equalsShallow(Base other_) { 1340 if (!super.equalsShallow(other_)) 1341 return false; 1342 if (!(other_ instanceof NutritionOrderOralDietTextureComponent)) 1343 return false; 1344 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other_; 1345 return true; 1346 } 1347 1348 public boolean isEmpty() { 1349 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifier, foodType); 1350 } 1351 1352 public String fhirType() { 1353 return "NutritionOrder.oralDiet.texture"; 1354 1355 } 1356 1357 } 1358 1359 @Block() 1360 public static class NutritionOrderSupplementComponent extends BackboneElement implements IBaseBackboneElement { 1361 /** 1362 * The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement. 1363 */ 1364 @Child(name = "type", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 1365 @Description(shortDefinition="Type of supplement product requested", formalDefinition="The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement." ) 1366 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplement-type") 1367 protected CodeableReference type; 1368 1369 /** 1370 * The product or brand name of the nutritional supplement such as "Acme Protein Shake". 1371 */ 1372 @Child(name = "productName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1373 @Description(shortDefinition="Product or brand name of the nutritional supplement", formalDefinition="The product or brand name of the nutritional supplement such as \"Acme Protein Shake\"." ) 1374 protected StringType productName; 1375 1376 /** 1377 * Schedule information for a supplement. 1378 */ 1379 @Child(name = "schedule", type = {}, order=3, min=0, max=1, modifier=false, summary=false) 1380 @Description(shortDefinition="Scheduling information for supplements", formalDefinition="Schedule information for a supplement." ) 1381 protected SupplementScheduleComponent schedule; 1382 1383 /** 1384 * The amount of the nutritional supplement to be given. 1385 */ 1386 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 1387 @Description(shortDefinition="Amount of the nutritional supplement", formalDefinition="The amount of the nutritional supplement to be given." ) 1388 protected Quantity quantity; 1389 1390 /** 1391 * Free text or additional instructions or information pertaining to the oral supplement. 1392 */ 1393 @Child(name = "instruction", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1394 @Description(shortDefinition="Instructions or additional information about the oral supplement", formalDefinition="Free text or additional instructions or information pertaining to the oral supplement." ) 1395 protected StringType instruction; 1396 1397 private static final long serialVersionUID = 648731928L; 1398 1399 /** 1400 * Constructor 1401 */ 1402 public NutritionOrderSupplementComponent() { 1403 super(); 1404 } 1405 1406 /** 1407 * @return {@link #type} (The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.) 1408 */ 1409 public CodeableReference getType() { 1410 if (this.type == null) 1411 if (Configuration.errorOnAutoCreate()) 1412 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.type"); 1413 else if (Configuration.doAutoCreate()) 1414 this.type = new CodeableReference(); // cc 1415 return this.type; 1416 } 1417 1418 public boolean hasType() { 1419 return this.type != null && !this.type.isEmpty(); 1420 } 1421 1422 /** 1423 * @param value {@link #type} (The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.) 1424 */ 1425 public NutritionOrderSupplementComponent setType(CodeableReference value) { 1426 this.type = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return {@link #productName} (The product or brand name of the nutritional supplement such as "Acme Protein Shake".). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 1432 */ 1433 public StringType getProductNameElement() { 1434 if (this.productName == null) 1435 if (Configuration.errorOnAutoCreate()) 1436 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.productName"); 1437 else if (Configuration.doAutoCreate()) 1438 this.productName = new StringType(); // bb 1439 return this.productName; 1440 } 1441 1442 public boolean hasProductNameElement() { 1443 return this.productName != null && !this.productName.isEmpty(); 1444 } 1445 1446 public boolean hasProductName() { 1447 return this.productName != null && !this.productName.isEmpty(); 1448 } 1449 1450 /** 1451 * @param value {@link #productName} (The product or brand name of the nutritional supplement such as "Acme Protein Shake".). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 1452 */ 1453 public NutritionOrderSupplementComponent setProductNameElement(StringType value) { 1454 this.productName = value; 1455 return this; 1456 } 1457 1458 /** 1459 * @return The product or brand name of the nutritional supplement such as "Acme Protein Shake". 1460 */ 1461 public String getProductName() { 1462 return this.productName == null ? null : this.productName.getValue(); 1463 } 1464 1465 /** 1466 * @param value The product or brand name of the nutritional supplement such as "Acme Protein Shake". 1467 */ 1468 public NutritionOrderSupplementComponent setProductName(String value) { 1469 if (Utilities.noString(value)) 1470 this.productName = null; 1471 else { 1472 if (this.productName == null) 1473 this.productName = new StringType(); 1474 this.productName.setValue(value); 1475 } 1476 return this; 1477 } 1478 1479 /** 1480 * @return {@link #schedule} (Schedule information for a supplement.) 1481 */ 1482 public SupplementScheduleComponent getSchedule() { 1483 if (this.schedule == null) 1484 if (Configuration.errorOnAutoCreate()) 1485 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.schedule"); 1486 else if (Configuration.doAutoCreate()) 1487 this.schedule = new SupplementScheduleComponent(); // cc 1488 return this.schedule; 1489 } 1490 1491 public boolean hasSchedule() { 1492 return this.schedule != null && !this.schedule.isEmpty(); 1493 } 1494 1495 /** 1496 * @param value {@link #schedule} (Schedule information for a supplement.) 1497 */ 1498 public NutritionOrderSupplementComponent setSchedule(SupplementScheduleComponent value) { 1499 this.schedule = value; 1500 return this; 1501 } 1502 1503 /** 1504 * @return {@link #quantity} (The amount of the nutritional supplement to be given.) 1505 */ 1506 public Quantity getQuantity() { 1507 if (this.quantity == null) 1508 if (Configuration.errorOnAutoCreate()) 1509 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.quantity"); 1510 else if (Configuration.doAutoCreate()) 1511 this.quantity = new Quantity(); // cc 1512 return this.quantity; 1513 } 1514 1515 public boolean hasQuantity() { 1516 return this.quantity != null && !this.quantity.isEmpty(); 1517 } 1518 1519 /** 1520 * @param value {@link #quantity} (The amount of the nutritional supplement to be given.) 1521 */ 1522 public NutritionOrderSupplementComponent setQuantity(Quantity value) { 1523 this.quantity = value; 1524 return this; 1525 } 1526 1527 /** 1528 * @return {@link #instruction} (Free text or additional instructions or information pertaining to the oral supplement.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 1529 */ 1530 public StringType getInstructionElement() { 1531 if (this.instruction == null) 1532 if (Configuration.errorOnAutoCreate()) 1533 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.instruction"); 1534 else if (Configuration.doAutoCreate()) 1535 this.instruction = new StringType(); // bb 1536 return this.instruction; 1537 } 1538 1539 public boolean hasInstructionElement() { 1540 return this.instruction != null && !this.instruction.isEmpty(); 1541 } 1542 1543 public boolean hasInstruction() { 1544 return this.instruction != null && !this.instruction.isEmpty(); 1545 } 1546 1547 /** 1548 * @param value {@link #instruction} (Free text or additional instructions or information pertaining to the oral supplement.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 1549 */ 1550 public NutritionOrderSupplementComponent setInstructionElement(StringType value) { 1551 this.instruction = value; 1552 return this; 1553 } 1554 1555 /** 1556 * @return Free text or additional instructions or information pertaining to the oral supplement. 1557 */ 1558 public String getInstruction() { 1559 return this.instruction == null ? null : this.instruction.getValue(); 1560 } 1561 1562 /** 1563 * @param value Free text or additional instructions or information pertaining to the oral supplement. 1564 */ 1565 public NutritionOrderSupplementComponent setInstruction(String value) { 1566 if (Utilities.noString(value)) 1567 this.instruction = null; 1568 else { 1569 if (this.instruction == null) 1570 this.instruction = new StringType(); 1571 this.instruction.setValue(value); 1572 } 1573 return this; 1574 } 1575 1576 protected void listChildren(List<Property> children) { 1577 super.listChildren(children); 1578 children.add(new Property("type", "CodeableReference(NutritionProduct)", "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 0, 1, type)); 1579 children.add(new Property("productName", "string", "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1, productName)); 1580 children.add(new Property("schedule", "", "Schedule information for a supplement.", 0, 1, schedule)); 1581 children.add(new Property("quantity", "Quantity", "The amount of the nutritional supplement to be given.", 0, 1, quantity)); 1582 children.add(new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1, instruction)); 1583 } 1584 1585 @Override 1586 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1587 switch (_hash) { 1588 case 3575610: /*type*/ return new Property("type", "CodeableReference(NutritionProduct)", "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 0, 1, type); 1589 case -1491817446: /*productName*/ return new Property("productName", "string", "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1, productName); 1590 case -697920873: /*schedule*/ return new Property("schedule", "", "Schedule information for a supplement.", 0, 1, schedule); 1591 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of the nutritional supplement to be given.", 0, 1, quantity); 1592 case 301526158: /*instruction*/ return new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1, instruction); 1593 default: return super.getNamedProperty(_hash, _name, _checkValid); 1594 } 1595 1596 } 1597 1598 @Override 1599 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1600 switch (hash) { 1601 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableReference 1602 case -1491817446: /*productName*/ return this.productName == null ? new Base[0] : new Base[] {this.productName}; // StringType 1603 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : new Base[] {this.schedule}; // SupplementScheduleComponent 1604 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1605 case 301526158: /*instruction*/ return this.instruction == null ? new Base[0] : new Base[] {this.instruction}; // StringType 1606 default: return super.getProperty(hash, name, checkValid); 1607 } 1608 1609 } 1610 1611 @Override 1612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1613 switch (hash) { 1614 case 3575610: // type 1615 this.type = TypeConvertor.castToCodeableReference(value); // CodeableReference 1616 return value; 1617 case -1491817446: // productName 1618 this.productName = TypeConvertor.castToString(value); // StringType 1619 return value; 1620 case -697920873: // schedule 1621 this.schedule = (SupplementScheduleComponent) value; // SupplementScheduleComponent 1622 return value; 1623 case -1285004149: // quantity 1624 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1625 return value; 1626 case 301526158: // instruction 1627 this.instruction = TypeConvertor.castToString(value); // StringType 1628 return value; 1629 default: return super.setProperty(hash, name, value); 1630 } 1631 1632 } 1633 1634 @Override 1635 public Base setProperty(String name, Base value) throws FHIRException { 1636 if (name.equals("type")) { 1637 this.type = TypeConvertor.castToCodeableReference(value); // CodeableReference 1638 } else if (name.equals("productName")) { 1639 this.productName = TypeConvertor.castToString(value); // StringType 1640 } else if (name.equals("schedule")) { 1641 this.schedule = (SupplementScheduleComponent) value; // SupplementScheduleComponent 1642 } else if (name.equals("quantity")) { 1643 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1644 } else if (name.equals("instruction")) { 1645 this.instruction = TypeConvertor.castToString(value); // StringType 1646 } else 1647 return super.setProperty(name, value); 1648 return value; 1649 } 1650 1651 @Override 1652 public void removeChild(String name, Base value) throws FHIRException { 1653 if (name.equals("type")) { 1654 this.type = null; 1655 } else if (name.equals("productName")) { 1656 this.productName = null; 1657 } else if (name.equals("schedule")) { 1658 this.schedule = (SupplementScheduleComponent) value; // SupplementScheduleComponent 1659 } else if (name.equals("quantity")) { 1660 this.quantity = null; 1661 } else if (name.equals("instruction")) { 1662 this.instruction = null; 1663 } else 1664 super.removeChild(name, value); 1665 1666 } 1667 1668 @Override 1669 public Base makeProperty(int hash, String name) throws FHIRException { 1670 switch (hash) { 1671 case 3575610: return getType(); 1672 case -1491817446: return getProductNameElement(); 1673 case -697920873: return getSchedule(); 1674 case -1285004149: return getQuantity(); 1675 case 301526158: return getInstructionElement(); 1676 default: return super.makeProperty(hash, name); 1677 } 1678 1679 } 1680 1681 @Override 1682 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1683 switch (hash) { 1684 case 3575610: /*type*/ return new String[] {"CodeableReference"}; 1685 case -1491817446: /*productName*/ return new String[] {"string"}; 1686 case -697920873: /*schedule*/ return new String[] {}; 1687 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1688 case 301526158: /*instruction*/ return new String[] {"string"}; 1689 default: return super.getTypesForProperty(hash, name); 1690 } 1691 1692 } 1693 1694 @Override 1695 public Base addChild(String name) throws FHIRException { 1696 if (name.equals("type")) { 1697 this.type = new CodeableReference(); 1698 return this.type; 1699 } 1700 else if (name.equals("productName")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.supplement.productName"); 1702 } 1703 else if (name.equals("schedule")) { 1704 this.schedule = new SupplementScheduleComponent(); 1705 return this.schedule; 1706 } 1707 else if (name.equals("quantity")) { 1708 this.quantity = new Quantity(); 1709 return this.quantity; 1710 } 1711 else if (name.equals("instruction")) { 1712 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.supplement.instruction"); 1713 } 1714 else 1715 return super.addChild(name); 1716 } 1717 1718 public NutritionOrderSupplementComponent copy() { 1719 NutritionOrderSupplementComponent dst = new NutritionOrderSupplementComponent(); 1720 copyValues(dst); 1721 return dst; 1722 } 1723 1724 public void copyValues(NutritionOrderSupplementComponent dst) { 1725 super.copyValues(dst); 1726 dst.type = type == null ? null : type.copy(); 1727 dst.productName = productName == null ? null : productName.copy(); 1728 dst.schedule = schedule == null ? null : schedule.copy(); 1729 dst.quantity = quantity == null ? null : quantity.copy(); 1730 dst.instruction = instruction == null ? null : instruction.copy(); 1731 } 1732 1733 @Override 1734 public boolean equalsDeep(Base other_) { 1735 if (!super.equalsDeep(other_)) 1736 return false; 1737 if (!(other_ instanceof NutritionOrderSupplementComponent)) 1738 return false; 1739 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other_; 1740 return compareDeep(type, o.type, true) && compareDeep(productName, o.productName, true) && compareDeep(schedule, o.schedule, true) 1741 && compareDeep(quantity, o.quantity, true) && compareDeep(instruction, o.instruction, true); 1742 } 1743 1744 @Override 1745 public boolean equalsShallow(Base other_) { 1746 if (!super.equalsShallow(other_)) 1747 return false; 1748 if (!(other_ instanceof NutritionOrderSupplementComponent)) 1749 return false; 1750 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other_; 1751 return compareValues(productName, o.productName, true) && compareValues(instruction, o.instruction, true) 1752 ; 1753 } 1754 1755 public boolean isEmpty() { 1756 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, productName, schedule 1757 , quantity, instruction); 1758 } 1759 1760 public String fhirType() { 1761 return "NutritionOrder.supplement"; 1762 1763 } 1764 1765 } 1766 1767 @Block() 1768 public static class SupplementScheduleComponent extends BackboneElement implements IBaseBackboneElement { 1769 /** 1770 * The time period and frequency at which the supplement should be given. The supplement should be given for the combination of all schedules if more than one schedule is present. 1771 */ 1772 @Child(name = "timing", type = {Timing.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1773 @Description(shortDefinition="Scheduled frequency of diet", formalDefinition="The time period and frequency at which the supplement should be given. The supplement should be given for the combination of all schedules if more than one schedule is present." ) 1774 protected List<Timing> timing; 1775 1776 /** 1777 * Indicates whether the supplement is only taken when needed within a specific dosing schedule. 1778 */ 1779 @Child(name = "asNeeded", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1780 @Description(shortDefinition="Take 'as needed'", formalDefinition="Indicates whether the supplement is only taken when needed within a specific dosing schedule." ) 1781 protected BooleanType asNeeded; 1782 1783 /** 1784 * Indicates whether the supplement is only taken based on a precondition for taking the supplement. 1785 */ 1786 @Child(name = "asNeededFor", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1787 @Description(shortDefinition="Take 'as needed' for x", formalDefinition="Indicates whether the supplement is only taken based on a precondition for taking the supplement." ) 1788 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 1789 protected CodeableConcept asNeededFor; 1790 1791 private static final long serialVersionUID = -1051458478L; 1792 1793 /** 1794 * Constructor 1795 */ 1796 public SupplementScheduleComponent() { 1797 super(); 1798 } 1799 1800 /** 1801 * @return {@link #timing} (The time period and frequency at which the supplement should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.) 1802 */ 1803 public List<Timing> getTiming() { 1804 if (this.timing == null) 1805 this.timing = new ArrayList<Timing>(); 1806 return this.timing; 1807 } 1808 1809 /** 1810 * @return Returns a reference to <code>this</code> for easy method chaining 1811 */ 1812 public SupplementScheduleComponent setTiming(List<Timing> theTiming) { 1813 this.timing = theTiming; 1814 return this; 1815 } 1816 1817 public boolean hasTiming() { 1818 if (this.timing == null) 1819 return false; 1820 for (Timing item : this.timing) 1821 if (!item.isEmpty()) 1822 return true; 1823 return false; 1824 } 1825 1826 public Timing addTiming() { //3 1827 Timing t = new Timing(); 1828 if (this.timing == null) 1829 this.timing = new ArrayList<Timing>(); 1830 this.timing.add(t); 1831 return t; 1832 } 1833 1834 public SupplementScheduleComponent addTiming(Timing t) { //3 1835 if (t == null) 1836 return this; 1837 if (this.timing == null) 1838 this.timing = new ArrayList<Timing>(); 1839 this.timing.add(t); 1840 return this; 1841 } 1842 1843 /** 1844 * @return The first repetition of repeating field {@link #timing}, creating it if it does not already exist {3} 1845 */ 1846 public Timing getTimingFirstRep() { 1847 if (getTiming().isEmpty()) { 1848 addTiming(); 1849 } 1850 return getTiming().get(0); 1851 } 1852 1853 /** 1854 * @return {@link #asNeeded} (Indicates whether the supplement is only taken when needed within a specific dosing schedule.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 1855 */ 1856 public BooleanType getAsNeededElement() { 1857 if (this.asNeeded == null) 1858 if (Configuration.errorOnAutoCreate()) 1859 throw new Error("Attempt to auto-create SupplementScheduleComponent.asNeeded"); 1860 else if (Configuration.doAutoCreate()) 1861 this.asNeeded = new BooleanType(); // bb 1862 return this.asNeeded; 1863 } 1864 1865 public boolean hasAsNeededElement() { 1866 return this.asNeeded != null && !this.asNeeded.isEmpty(); 1867 } 1868 1869 public boolean hasAsNeeded() { 1870 return this.asNeeded != null && !this.asNeeded.isEmpty(); 1871 } 1872 1873 /** 1874 * @param value {@link #asNeeded} (Indicates whether the supplement is only taken when needed within a specific dosing schedule.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 1875 */ 1876 public SupplementScheduleComponent setAsNeededElement(BooleanType value) { 1877 this.asNeeded = value; 1878 return this; 1879 } 1880 1881 /** 1882 * @return Indicates whether the supplement is only taken when needed within a specific dosing schedule. 1883 */ 1884 public boolean getAsNeeded() { 1885 return this.asNeeded == null || this.asNeeded.isEmpty() ? false : this.asNeeded.getValue(); 1886 } 1887 1888 /** 1889 * @param value Indicates whether the supplement is only taken when needed within a specific dosing schedule. 1890 */ 1891 public SupplementScheduleComponent setAsNeeded(boolean value) { 1892 if (this.asNeeded == null) 1893 this.asNeeded = new BooleanType(); 1894 this.asNeeded.setValue(value); 1895 return this; 1896 } 1897 1898 /** 1899 * @return {@link #asNeededFor} (Indicates whether the supplement is only taken based on a precondition for taking the supplement.) 1900 */ 1901 public CodeableConcept getAsNeededFor() { 1902 if (this.asNeededFor == null) 1903 if (Configuration.errorOnAutoCreate()) 1904 throw new Error("Attempt to auto-create SupplementScheduleComponent.asNeededFor"); 1905 else if (Configuration.doAutoCreate()) 1906 this.asNeededFor = new CodeableConcept(); // cc 1907 return this.asNeededFor; 1908 } 1909 1910 public boolean hasAsNeededFor() { 1911 return this.asNeededFor != null && !this.asNeededFor.isEmpty(); 1912 } 1913 1914 /** 1915 * @param value {@link #asNeededFor} (Indicates whether the supplement is only taken based on a precondition for taking the supplement.) 1916 */ 1917 public SupplementScheduleComponent setAsNeededFor(CodeableConcept value) { 1918 this.asNeededFor = value; 1919 return this; 1920 } 1921 1922 protected void listChildren(List<Property> children) { 1923 super.listChildren(children); 1924 children.add(new Property("timing", "Timing", "The time period and frequency at which the supplement should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, timing)); 1925 children.add(new Property("asNeeded", "boolean", "Indicates whether the supplement is only taken when needed within a specific dosing schedule.", 0, 1, asNeeded)); 1926 children.add(new Property("asNeededFor", "CodeableConcept", "Indicates whether the supplement is only taken based on a precondition for taking the supplement.", 0, 1, asNeededFor)); 1927 } 1928 1929 @Override 1930 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1931 switch (_hash) { 1932 case -873664438: /*timing*/ return new Property("timing", "Timing", "The time period and frequency at which the supplement should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, timing); 1933 case -1432923513: /*asNeeded*/ return new Property("asNeeded", "boolean", "Indicates whether the supplement is only taken when needed within a specific dosing schedule.", 0, 1, asNeeded); 1934 case -544350014: /*asNeededFor*/ return new Property("asNeededFor", "CodeableConcept", "Indicates whether the supplement is only taken based on a precondition for taking the supplement.", 0, 1, asNeededFor); 1935 default: return super.getNamedProperty(_hash, _name, _checkValid); 1936 } 1937 1938 } 1939 1940 @Override 1941 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1942 switch (hash) { 1943 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : this.timing.toArray(new Base[this.timing.size()]); // Timing 1944 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // BooleanType 1945 case -544350014: /*asNeededFor*/ return this.asNeededFor == null ? new Base[0] : new Base[] {this.asNeededFor}; // CodeableConcept 1946 default: return super.getProperty(hash, name, checkValid); 1947 } 1948 1949 } 1950 1951 @Override 1952 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1953 switch (hash) { 1954 case -873664438: // timing 1955 this.getTiming().add(TypeConvertor.castToTiming(value)); // Timing 1956 return value; 1957 case -1432923513: // asNeeded 1958 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 1959 return value; 1960 case -544350014: // asNeededFor 1961 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1962 return value; 1963 default: return super.setProperty(hash, name, value); 1964 } 1965 1966 } 1967 1968 @Override 1969 public Base setProperty(String name, Base value) throws FHIRException { 1970 if (name.equals("timing")) { 1971 this.getTiming().add(TypeConvertor.castToTiming(value)); 1972 } else if (name.equals("asNeeded")) { 1973 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 1974 } else if (name.equals("asNeededFor")) { 1975 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1976 } else 1977 return super.setProperty(name, value); 1978 return value; 1979 } 1980 1981 @Override 1982 public void removeChild(String name, Base value) throws FHIRException { 1983 if (name.equals("timing")) { 1984 this.getTiming().remove(value); 1985 } else if (name.equals("asNeeded")) { 1986 this.asNeeded = null; 1987 } else if (name.equals("asNeededFor")) { 1988 this.asNeededFor = null; 1989 } else 1990 super.removeChild(name, value); 1991 1992 } 1993 1994 @Override 1995 public Base makeProperty(int hash, String name) throws FHIRException { 1996 switch (hash) { 1997 case -873664438: return addTiming(); 1998 case -1432923513: return getAsNeededElement(); 1999 case -544350014: return getAsNeededFor(); 2000 default: return super.makeProperty(hash, name); 2001 } 2002 2003 } 2004 2005 @Override 2006 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2007 switch (hash) { 2008 case -873664438: /*timing*/ return new String[] {"Timing"}; 2009 case -1432923513: /*asNeeded*/ return new String[] {"boolean"}; 2010 case -544350014: /*asNeededFor*/ return new String[] {"CodeableConcept"}; 2011 default: return super.getTypesForProperty(hash, name); 2012 } 2013 2014 } 2015 2016 @Override 2017 public Base addChild(String name) throws FHIRException { 2018 if (name.equals("timing")) { 2019 return addTiming(); 2020 } 2021 else if (name.equals("asNeeded")) { 2022 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.supplement.schedule.asNeeded"); 2023 } 2024 else if (name.equals("asNeededFor")) { 2025 this.asNeededFor = new CodeableConcept(); 2026 return this.asNeededFor; 2027 } 2028 else 2029 return super.addChild(name); 2030 } 2031 2032 public SupplementScheduleComponent copy() { 2033 SupplementScheduleComponent dst = new SupplementScheduleComponent(); 2034 copyValues(dst); 2035 return dst; 2036 } 2037 2038 public void copyValues(SupplementScheduleComponent dst) { 2039 super.copyValues(dst); 2040 if (timing != null) { 2041 dst.timing = new ArrayList<Timing>(); 2042 for (Timing i : timing) 2043 dst.timing.add(i.copy()); 2044 }; 2045 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 2046 dst.asNeededFor = asNeededFor == null ? null : asNeededFor.copy(); 2047 } 2048 2049 @Override 2050 public boolean equalsDeep(Base other_) { 2051 if (!super.equalsDeep(other_)) 2052 return false; 2053 if (!(other_ instanceof SupplementScheduleComponent)) 2054 return false; 2055 SupplementScheduleComponent o = (SupplementScheduleComponent) other_; 2056 return compareDeep(timing, o.timing, true) && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(asNeededFor, o.asNeededFor, true) 2057 ; 2058 } 2059 2060 @Override 2061 public boolean equalsShallow(Base other_) { 2062 if (!super.equalsShallow(other_)) 2063 return false; 2064 if (!(other_ instanceof SupplementScheduleComponent)) 2065 return false; 2066 SupplementScheduleComponent o = (SupplementScheduleComponent) other_; 2067 return compareValues(asNeeded, o.asNeeded, true); 2068 } 2069 2070 public boolean isEmpty() { 2071 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(timing, asNeeded, asNeededFor 2072 ); 2073 } 2074 2075 public String fhirType() { 2076 return "NutritionOrder.supplement.schedule"; 2077 2078 } 2079 2080 } 2081 2082 @Block() 2083 public static class NutritionOrderEnteralFormulaComponent extends BackboneElement implements IBaseBackboneElement { 2084 /** 2085 * The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula. 2086 */ 2087 @Child(name = "baseFormulaType", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 2088 @Description(shortDefinition="Type of enteral or infant formula", formalDefinition="The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula." ) 2089 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/entformula-type") 2090 protected CodeableReference baseFormulaType; 2091 2092 /** 2093 * The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula". 2094 */ 2095 @Child(name = "baseFormulaProductName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2096 @Description(shortDefinition="Product or brand name of the enteral or infant formula", formalDefinition="The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\"." ) 2097 protected StringType baseFormulaProductName; 2098 2099 /** 2100 * The intended type of device that is to be used for the administration of the enteral formula. 2101 */ 2102 @Child(name = "deliveryDevice", type = {CodeableReference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2103 @Description(shortDefinition="Intended type of device for the administration", formalDefinition="The intended type of device that is to be used for the administration of the enteral formula." ) 2104 protected List<CodeableReference> deliveryDevice; 2105 2106 /** 2107 * Indicates modular components to be provided in addition or mixed with the base formula. 2108 */ 2109 @Child(name = "additive", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2110 @Description(shortDefinition="Components to add to the feeding", formalDefinition="Indicates modular components to be provided in addition or mixed with the base formula." ) 2111 protected List<NutritionOrderEnteralFormulaAdditiveComponent> additive; 2112 2113 /** 2114 * The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL. 2115 */ 2116 @Child(name = "caloricDensity", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 2117 @Description(shortDefinition="Amount of energy per specified volume that is required", formalDefinition="The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL." ) 2118 protected Quantity caloricDensity; 2119 2120 /** 2121 * The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube. 2122 */ 2123 @Child(name = "routeOfAdministration", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 2124 @Description(shortDefinition="How the formula should enter the patient's gastrointestinal tract", formalDefinition="The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube." ) 2125 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/enteral-route") 2126 protected CodeableConcept routeOfAdministration; 2127 2128 /** 2129 * Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours. 2130 */ 2131 @Child(name = "administration", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2132 @Description(shortDefinition="Formula feeding instruction as structured data", formalDefinition="Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours." ) 2133 protected List<NutritionOrderEnteralFormulaAdministrationComponent> administration; 2134 2135 /** 2136 * The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours. 2137 */ 2138 @Child(name = "maxVolumeToDeliver", type = {Quantity.class}, order=8, min=0, max=1, modifier=false, summary=false) 2139 @Description(shortDefinition="Upper limit on formula volume per unit of time", formalDefinition="The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours." ) 2140 protected Quantity maxVolumeToDeliver; 2141 2142 /** 2143 * Free text formula administration, feeding instructions or additional instructions or information. 2144 */ 2145 @Child(name = "administrationInstruction", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2146 @Description(shortDefinition="Formula feeding instructions expressed as text", formalDefinition="Free text formula administration, feeding instructions or additional instructions or information." ) 2147 protected MarkdownType administrationInstruction; 2148 2149 private static final long serialVersionUID = -694080614L; 2150 2151 /** 2152 * Constructor 2153 */ 2154 public NutritionOrderEnteralFormulaComponent() { 2155 super(); 2156 } 2157 2158 /** 2159 * @return {@link #baseFormulaType} (The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.) 2160 */ 2161 public CodeableReference getBaseFormulaType() { 2162 if (this.baseFormulaType == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaType"); 2165 else if (Configuration.doAutoCreate()) 2166 this.baseFormulaType = new CodeableReference(); // cc 2167 return this.baseFormulaType; 2168 } 2169 2170 public boolean hasBaseFormulaType() { 2171 return this.baseFormulaType != null && !this.baseFormulaType.isEmpty(); 2172 } 2173 2174 /** 2175 * @param value {@link #baseFormulaType} (The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.) 2176 */ 2177 public NutritionOrderEnteralFormulaComponent setBaseFormulaType(CodeableReference value) { 2178 this.baseFormulaType = value; 2179 return this; 2180 } 2181 2182 /** 2183 * @return {@link #baseFormulaProductName} (The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula".). This is the underlying object with id, value and extensions. The accessor "getBaseFormulaProductName" gives direct access to the value 2184 */ 2185 public StringType getBaseFormulaProductNameElement() { 2186 if (this.baseFormulaProductName == null) 2187 if (Configuration.errorOnAutoCreate()) 2188 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaProductName"); 2189 else if (Configuration.doAutoCreate()) 2190 this.baseFormulaProductName = new StringType(); // bb 2191 return this.baseFormulaProductName; 2192 } 2193 2194 public boolean hasBaseFormulaProductNameElement() { 2195 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 2196 } 2197 2198 public boolean hasBaseFormulaProductName() { 2199 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 2200 } 2201 2202 /** 2203 * @param value {@link #baseFormulaProductName} (The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula".). This is the underlying object with id, value and extensions. The accessor "getBaseFormulaProductName" gives direct access to the value 2204 */ 2205 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductNameElement(StringType value) { 2206 this.baseFormulaProductName = value; 2207 return this; 2208 } 2209 2210 /** 2211 * @return The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula". 2212 */ 2213 public String getBaseFormulaProductName() { 2214 return this.baseFormulaProductName == null ? null : this.baseFormulaProductName.getValue(); 2215 } 2216 2217 /** 2218 * @param value The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula". 2219 */ 2220 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductName(String value) { 2221 if (Utilities.noString(value)) 2222 this.baseFormulaProductName = null; 2223 else { 2224 if (this.baseFormulaProductName == null) 2225 this.baseFormulaProductName = new StringType(); 2226 this.baseFormulaProductName.setValue(value); 2227 } 2228 return this; 2229 } 2230 2231 /** 2232 * @return {@link #deliveryDevice} (The intended type of device that is to be used for the administration of the enteral formula.) 2233 */ 2234 public List<CodeableReference> getDeliveryDevice() { 2235 if (this.deliveryDevice == null) 2236 this.deliveryDevice = new ArrayList<CodeableReference>(); 2237 return this.deliveryDevice; 2238 } 2239 2240 /** 2241 * @return Returns a reference to <code>this</code> for easy method chaining 2242 */ 2243 public NutritionOrderEnteralFormulaComponent setDeliveryDevice(List<CodeableReference> theDeliveryDevice) { 2244 this.deliveryDevice = theDeliveryDevice; 2245 return this; 2246 } 2247 2248 public boolean hasDeliveryDevice() { 2249 if (this.deliveryDevice == null) 2250 return false; 2251 for (CodeableReference item : this.deliveryDevice) 2252 if (!item.isEmpty()) 2253 return true; 2254 return false; 2255 } 2256 2257 public CodeableReference addDeliveryDevice() { //3 2258 CodeableReference t = new CodeableReference(); 2259 if (this.deliveryDevice == null) 2260 this.deliveryDevice = new ArrayList<CodeableReference>(); 2261 this.deliveryDevice.add(t); 2262 return t; 2263 } 2264 2265 public NutritionOrderEnteralFormulaComponent addDeliveryDevice(CodeableReference t) { //3 2266 if (t == null) 2267 return this; 2268 if (this.deliveryDevice == null) 2269 this.deliveryDevice = new ArrayList<CodeableReference>(); 2270 this.deliveryDevice.add(t); 2271 return this; 2272 } 2273 2274 /** 2275 * @return The first repetition of repeating field {@link #deliveryDevice}, creating it if it does not already exist {3} 2276 */ 2277 public CodeableReference getDeliveryDeviceFirstRep() { 2278 if (getDeliveryDevice().isEmpty()) { 2279 addDeliveryDevice(); 2280 } 2281 return getDeliveryDevice().get(0); 2282 } 2283 2284 /** 2285 * @return {@link #additive} (Indicates modular components to be provided in addition or mixed with the base formula.) 2286 */ 2287 public List<NutritionOrderEnteralFormulaAdditiveComponent> getAdditive() { 2288 if (this.additive == null) 2289 this.additive = new ArrayList<NutritionOrderEnteralFormulaAdditiveComponent>(); 2290 return this.additive; 2291 } 2292 2293 /** 2294 * @return Returns a reference to <code>this</code> for easy method chaining 2295 */ 2296 public NutritionOrderEnteralFormulaComponent setAdditive(List<NutritionOrderEnteralFormulaAdditiveComponent> theAdditive) { 2297 this.additive = theAdditive; 2298 return this; 2299 } 2300 2301 public boolean hasAdditive() { 2302 if (this.additive == null) 2303 return false; 2304 for (NutritionOrderEnteralFormulaAdditiveComponent item : this.additive) 2305 if (!item.isEmpty()) 2306 return true; 2307 return false; 2308 } 2309 2310 public NutritionOrderEnteralFormulaAdditiveComponent addAdditive() { //3 2311 NutritionOrderEnteralFormulaAdditiveComponent t = new NutritionOrderEnteralFormulaAdditiveComponent(); 2312 if (this.additive == null) 2313 this.additive = new ArrayList<NutritionOrderEnteralFormulaAdditiveComponent>(); 2314 this.additive.add(t); 2315 return t; 2316 } 2317 2318 public NutritionOrderEnteralFormulaComponent addAdditive(NutritionOrderEnteralFormulaAdditiveComponent t) { //3 2319 if (t == null) 2320 return this; 2321 if (this.additive == null) 2322 this.additive = new ArrayList<NutritionOrderEnteralFormulaAdditiveComponent>(); 2323 this.additive.add(t); 2324 return this; 2325 } 2326 2327 /** 2328 * @return The first repetition of repeating field {@link #additive}, creating it if it does not already exist {3} 2329 */ 2330 public NutritionOrderEnteralFormulaAdditiveComponent getAdditiveFirstRep() { 2331 if (getAdditive().isEmpty()) { 2332 addAdditive(); 2333 } 2334 return getAdditive().get(0); 2335 } 2336 2337 /** 2338 * @return {@link #caloricDensity} (The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.) 2339 */ 2340 public Quantity getCaloricDensity() { 2341 if (this.caloricDensity == null) 2342 if (Configuration.errorOnAutoCreate()) 2343 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.caloricDensity"); 2344 else if (Configuration.doAutoCreate()) 2345 this.caloricDensity = new Quantity(); // cc 2346 return this.caloricDensity; 2347 } 2348 2349 public boolean hasCaloricDensity() { 2350 return this.caloricDensity != null && !this.caloricDensity.isEmpty(); 2351 } 2352 2353 /** 2354 * @param value {@link #caloricDensity} (The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.) 2355 */ 2356 public NutritionOrderEnteralFormulaComponent setCaloricDensity(Quantity value) { 2357 this.caloricDensity = value; 2358 return this; 2359 } 2360 2361 /** 2362 * @return {@link #routeOfAdministration} (The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.) 2363 */ 2364 public CodeableConcept getRouteOfAdministration() { 2365 if (this.routeOfAdministration == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.routeOfAdministration"); 2368 else if (Configuration.doAutoCreate()) 2369 this.routeOfAdministration = new CodeableConcept(); // cc 2370 return this.routeOfAdministration; 2371 } 2372 2373 public boolean hasRouteOfAdministration() { 2374 return this.routeOfAdministration != null && !this.routeOfAdministration.isEmpty(); 2375 } 2376 2377 /** 2378 * @param value {@link #routeOfAdministration} (The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.) 2379 */ 2380 public NutritionOrderEnteralFormulaComponent setRouteOfAdministration(CodeableConcept value) { 2381 this.routeOfAdministration = value; 2382 return this; 2383 } 2384 2385 /** 2386 * @return {@link #administration} (Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.) 2387 */ 2388 public List<NutritionOrderEnteralFormulaAdministrationComponent> getAdministration() { 2389 if (this.administration == null) 2390 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2391 return this.administration; 2392 } 2393 2394 /** 2395 * @return Returns a reference to <code>this</code> for easy method chaining 2396 */ 2397 public NutritionOrderEnteralFormulaComponent setAdministration(List<NutritionOrderEnteralFormulaAdministrationComponent> theAdministration) { 2398 this.administration = theAdministration; 2399 return this; 2400 } 2401 2402 public boolean hasAdministration() { 2403 if (this.administration == null) 2404 return false; 2405 for (NutritionOrderEnteralFormulaAdministrationComponent item : this.administration) 2406 if (!item.isEmpty()) 2407 return true; 2408 return false; 2409 } 2410 2411 public NutritionOrderEnteralFormulaAdministrationComponent addAdministration() { //3 2412 NutritionOrderEnteralFormulaAdministrationComponent t = new NutritionOrderEnteralFormulaAdministrationComponent(); 2413 if (this.administration == null) 2414 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2415 this.administration.add(t); 2416 return t; 2417 } 2418 2419 public NutritionOrderEnteralFormulaComponent addAdministration(NutritionOrderEnteralFormulaAdministrationComponent t) { //3 2420 if (t == null) 2421 return this; 2422 if (this.administration == null) 2423 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2424 this.administration.add(t); 2425 return this; 2426 } 2427 2428 /** 2429 * @return The first repetition of repeating field {@link #administration}, creating it if it does not already exist {3} 2430 */ 2431 public NutritionOrderEnteralFormulaAdministrationComponent getAdministrationFirstRep() { 2432 if (getAdministration().isEmpty()) { 2433 addAdministration(); 2434 } 2435 return getAdministration().get(0); 2436 } 2437 2438 /** 2439 * @return {@link #maxVolumeToDeliver} (The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.) 2440 */ 2441 public Quantity getMaxVolumeToDeliver() { 2442 if (this.maxVolumeToDeliver == null) 2443 if (Configuration.errorOnAutoCreate()) 2444 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.maxVolumeToDeliver"); 2445 else if (Configuration.doAutoCreate()) 2446 this.maxVolumeToDeliver = new Quantity(); // cc 2447 return this.maxVolumeToDeliver; 2448 } 2449 2450 public boolean hasMaxVolumeToDeliver() { 2451 return this.maxVolumeToDeliver != null && !this.maxVolumeToDeliver.isEmpty(); 2452 } 2453 2454 /** 2455 * @param value {@link #maxVolumeToDeliver} (The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.) 2456 */ 2457 public NutritionOrderEnteralFormulaComponent setMaxVolumeToDeliver(Quantity value) { 2458 this.maxVolumeToDeliver = value; 2459 return this; 2460 } 2461 2462 /** 2463 * @return {@link #administrationInstruction} (Free text formula administration, feeding instructions or additional instructions or information.). This is the underlying object with id, value and extensions. The accessor "getAdministrationInstruction" gives direct access to the value 2464 */ 2465 public MarkdownType getAdministrationInstructionElement() { 2466 if (this.administrationInstruction == null) 2467 if (Configuration.errorOnAutoCreate()) 2468 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.administrationInstruction"); 2469 else if (Configuration.doAutoCreate()) 2470 this.administrationInstruction = new MarkdownType(); // bb 2471 return this.administrationInstruction; 2472 } 2473 2474 public boolean hasAdministrationInstructionElement() { 2475 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 2476 } 2477 2478 public boolean hasAdministrationInstruction() { 2479 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 2480 } 2481 2482 /** 2483 * @param value {@link #administrationInstruction} (Free text formula administration, feeding instructions or additional instructions or information.). This is the underlying object with id, value and extensions. The accessor "getAdministrationInstruction" gives direct access to the value 2484 */ 2485 public NutritionOrderEnteralFormulaComponent setAdministrationInstructionElement(MarkdownType value) { 2486 this.administrationInstruction = value; 2487 return this; 2488 } 2489 2490 /** 2491 * @return Free text formula administration, feeding instructions or additional instructions or information. 2492 */ 2493 public String getAdministrationInstruction() { 2494 return this.administrationInstruction == null ? null : this.administrationInstruction.getValue(); 2495 } 2496 2497 /** 2498 * @param value Free text formula administration, feeding instructions or additional instructions or information. 2499 */ 2500 public NutritionOrderEnteralFormulaComponent setAdministrationInstruction(String value) { 2501 if (Utilities.noString(value)) 2502 this.administrationInstruction = null; 2503 else { 2504 if (this.administrationInstruction == null) 2505 this.administrationInstruction = new MarkdownType(); 2506 this.administrationInstruction.setValue(value); 2507 } 2508 return this; 2509 } 2510 2511 protected void listChildren(List<Property> children) { 2512 super.listChildren(children); 2513 children.add(new Property("baseFormulaType", "CodeableReference(NutritionProduct)", "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 0, 1, baseFormulaType)); 2514 children.add(new Property("baseFormulaProductName", "string", "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 0, 1, baseFormulaProductName)); 2515 children.add(new Property("deliveryDevice", "CodeableReference(DeviceDefinition)", "The intended type of device that is to be used for the administration of the enteral formula.", 0, java.lang.Integer.MAX_VALUE, deliveryDevice)); 2516 children.add(new Property("additive", "", "Indicates modular components to be provided in addition or mixed with the base formula.", 0, java.lang.Integer.MAX_VALUE, additive)); 2517 children.add(new Property("caloricDensity", "Quantity", "The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.", 0, 1, caloricDensity)); 2518 children.add(new Property("routeOfAdministration", "CodeableConcept", "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 0, 1, routeOfAdministration)); 2519 children.add(new Property("administration", "", "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 0, java.lang.Integer.MAX_VALUE, administration)); 2520 children.add(new Property("maxVolumeToDeliver", "Quantity", "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 0, 1, maxVolumeToDeliver)); 2521 children.add(new Property("administrationInstruction", "markdown", "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1, administrationInstruction)); 2522 } 2523 2524 @Override 2525 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2526 switch (_hash) { 2527 case -138930641: /*baseFormulaType*/ return new Property("baseFormulaType", "CodeableReference(NutritionProduct)", "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 0, 1, baseFormulaType); 2528 case -1267705979: /*baseFormulaProductName*/ return new Property("baseFormulaProductName", "string", "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 0, 1, baseFormulaProductName); 2529 case 2060803946: /*deliveryDevice*/ return new Property("deliveryDevice", "CodeableReference(DeviceDefinition)", "The intended type of device that is to be used for the administration of the enteral formula.", 0, java.lang.Integer.MAX_VALUE, deliveryDevice); 2530 case -1226589236: /*additive*/ return new Property("additive", "", "Indicates modular components to be provided in addition or mixed with the base formula.", 0, java.lang.Integer.MAX_VALUE, additive); 2531 case 186983261: /*caloricDensity*/ return new Property("caloricDensity", "Quantity", "The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.", 0, 1, caloricDensity); 2532 case 1742084734: /*routeOfAdministration*/ return new Property("routeOfAdministration", "CodeableConcept", "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 0, 1, routeOfAdministration); 2533 case 1255702622: /*administration*/ return new Property("administration", "", "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 0, java.lang.Integer.MAX_VALUE, administration); 2534 case 2017924652: /*maxVolumeToDeliver*/ return new Property("maxVolumeToDeliver", "Quantity", "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 0, 1, maxVolumeToDeliver); 2535 case 427085136: /*administrationInstruction*/ return new Property("administrationInstruction", "markdown", "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1, administrationInstruction); 2536 default: return super.getNamedProperty(_hash, _name, _checkValid); 2537 } 2538 2539 } 2540 2541 @Override 2542 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2543 switch (hash) { 2544 case -138930641: /*baseFormulaType*/ return this.baseFormulaType == null ? new Base[0] : new Base[] {this.baseFormulaType}; // CodeableReference 2545 case -1267705979: /*baseFormulaProductName*/ return this.baseFormulaProductName == null ? new Base[0] : new Base[] {this.baseFormulaProductName}; // StringType 2546 case 2060803946: /*deliveryDevice*/ return this.deliveryDevice == null ? new Base[0] : this.deliveryDevice.toArray(new Base[this.deliveryDevice.size()]); // CodeableReference 2547 case -1226589236: /*additive*/ return this.additive == null ? new Base[0] : this.additive.toArray(new Base[this.additive.size()]); // NutritionOrderEnteralFormulaAdditiveComponent 2548 case 186983261: /*caloricDensity*/ return this.caloricDensity == null ? new Base[0] : new Base[] {this.caloricDensity}; // Quantity 2549 case 1742084734: /*routeOfAdministration*/ return this.routeOfAdministration == null ? new Base[0] : new Base[] {this.routeOfAdministration}; // CodeableConcept 2550 case 1255702622: /*administration*/ return this.administration == null ? new Base[0] : this.administration.toArray(new Base[this.administration.size()]); // NutritionOrderEnteralFormulaAdministrationComponent 2551 case 2017924652: /*maxVolumeToDeliver*/ return this.maxVolumeToDeliver == null ? new Base[0] : new Base[] {this.maxVolumeToDeliver}; // Quantity 2552 case 427085136: /*administrationInstruction*/ return this.administrationInstruction == null ? new Base[0] : new Base[] {this.administrationInstruction}; // MarkdownType 2553 default: return super.getProperty(hash, name, checkValid); 2554 } 2555 2556 } 2557 2558 @Override 2559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2560 switch (hash) { 2561 case -138930641: // baseFormulaType 2562 this.baseFormulaType = TypeConvertor.castToCodeableReference(value); // CodeableReference 2563 return value; 2564 case -1267705979: // baseFormulaProductName 2565 this.baseFormulaProductName = TypeConvertor.castToString(value); // StringType 2566 return value; 2567 case 2060803946: // deliveryDevice 2568 this.getDeliveryDevice().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2569 return value; 2570 case -1226589236: // additive 2571 this.getAdditive().add((NutritionOrderEnteralFormulaAdditiveComponent) value); // NutritionOrderEnteralFormulaAdditiveComponent 2572 return value; 2573 case 186983261: // caloricDensity 2574 this.caloricDensity = TypeConvertor.castToQuantity(value); // Quantity 2575 return value; 2576 case 1742084734: // routeOfAdministration 2577 this.routeOfAdministration = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2578 return value; 2579 case 1255702622: // administration 2580 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); // NutritionOrderEnteralFormulaAdministrationComponent 2581 return value; 2582 case 2017924652: // maxVolumeToDeliver 2583 this.maxVolumeToDeliver = TypeConvertor.castToQuantity(value); // Quantity 2584 return value; 2585 case 427085136: // administrationInstruction 2586 this.administrationInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 2587 return value; 2588 default: return super.setProperty(hash, name, value); 2589 } 2590 2591 } 2592 2593 @Override 2594 public Base setProperty(String name, Base value) throws FHIRException { 2595 if (name.equals("baseFormulaType")) { 2596 this.baseFormulaType = TypeConvertor.castToCodeableReference(value); // CodeableReference 2597 } else if (name.equals("baseFormulaProductName")) { 2598 this.baseFormulaProductName = TypeConvertor.castToString(value); // StringType 2599 } else if (name.equals("deliveryDevice")) { 2600 this.getDeliveryDevice().add(TypeConvertor.castToCodeableReference(value)); 2601 } else if (name.equals("additive")) { 2602 this.getAdditive().add((NutritionOrderEnteralFormulaAdditiveComponent) value); 2603 } else if (name.equals("caloricDensity")) { 2604 this.caloricDensity = TypeConvertor.castToQuantity(value); // Quantity 2605 } else if (name.equals("routeOfAdministration")) { 2606 this.routeOfAdministration = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2607 } else if (name.equals("administration")) { 2608 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); 2609 } else if (name.equals("maxVolumeToDeliver")) { 2610 this.maxVolumeToDeliver = TypeConvertor.castToQuantity(value); // Quantity 2611 } else if (name.equals("administrationInstruction")) { 2612 this.administrationInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 2613 } else 2614 return super.setProperty(name, value); 2615 return value; 2616 } 2617 2618 @Override 2619 public void removeChild(String name, Base value) throws FHIRException { 2620 if (name.equals("baseFormulaType")) { 2621 this.baseFormulaType = null; 2622 } else if (name.equals("baseFormulaProductName")) { 2623 this.baseFormulaProductName = null; 2624 } else if (name.equals("deliveryDevice")) { 2625 this.getDeliveryDevice().remove(value); 2626 } else if (name.equals("additive")) { 2627 this.getAdditive().remove((NutritionOrderEnteralFormulaAdditiveComponent) value); 2628 } else if (name.equals("caloricDensity")) { 2629 this.caloricDensity = null; 2630 } else if (name.equals("routeOfAdministration")) { 2631 this.routeOfAdministration = null; 2632 } else if (name.equals("administration")) { 2633 this.getAdministration().remove((NutritionOrderEnteralFormulaAdministrationComponent) value); 2634 } else if (name.equals("maxVolumeToDeliver")) { 2635 this.maxVolumeToDeliver = null; 2636 } else if (name.equals("administrationInstruction")) { 2637 this.administrationInstruction = null; 2638 } else 2639 super.removeChild(name, value); 2640 2641 } 2642 2643 @Override 2644 public Base makeProperty(int hash, String name) throws FHIRException { 2645 switch (hash) { 2646 case -138930641: return getBaseFormulaType(); 2647 case -1267705979: return getBaseFormulaProductNameElement(); 2648 case 2060803946: return addDeliveryDevice(); 2649 case -1226589236: return addAdditive(); 2650 case 186983261: return getCaloricDensity(); 2651 case 1742084734: return getRouteOfAdministration(); 2652 case 1255702622: return addAdministration(); 2653 case 2017924652: return getMaxVolumeToDeliver(); 2654 case 427085136: return getAdministrationInstructionElement(); 2655 default: return super.makeProperty(hash, name); 2656 } 2657 2658 } 2659 2660 @Override 2661 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2662 switch (hash) { 2663 case -138930641: /*baseFormulaType*/ return new String[] {"CodeableReference"}; 2664 case -1267705979: /*baseFormulaProductName*/ return new String[] {"string"}; 2665 case 2060803946: /*deliveryDevice*/ return new String[] {"CodeableReference"}; 2666 case -1226589236: /*additive*/ return new String[] {}; 2667 case 186983261: /*caloricDensity*/ return new String[] {"Quantity"}; 2668 case 1742084734: /*routeOfAdministration*/ return new String[] {"CodeableConcept"}; 2669 case 1255702622: /*administration*/ return new String[] {}; 2670 case 2017924652: /*maxVolumeToDeliver*/ return new String[] {"Quantity"}; 2671 case 427085136: /*administrationInstruction*/ return new String[] {"markdown"}; 2672 default: return super.getTypesForProperty(hash, name); 2673 } 2674 2675 } 2676 2677 @Override 2678 public Base addChild(String name) throws FHIRException { 2679 if (name.equals("baseFormulaType")) { 2680 this.baseFormulaType = new CodeableReference(); 2681 return this.baseFormulaType; 2682 } 2683 else if (name.equals("baseFormulaProductName")) { 2684 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.enteralFormula.baseFormulaProductName"); 2685 } 2686 else if (name.equals("deliveryDevice")) { 2687 return addDeliveryDevice(); 2688 } 2689 else if (name.equals("additive")) { 2690 return addAdditive(); 2691 } 2692 else if (name.equals("caloricDensity")) { 2693 this.caloricDensity = new Quantity(); 2694 return this.caloricDensity; 2695 } 2696 else if (name.equals("routeOfAdministration")) { 2697 this.routeOfAdministration = new CodeableConcept(); 2698 return this.routeOfAdministration; 2699 } 2700 else if (name.equals("administration")) { 2701 return addAdministration(); 2702 } 2703 else if (name.equals("maxVolumeToDeliver")) { 2704 this.maxVolumeToDeliver = new Quantity(); 2705 return this.maxVolumeToDeliver; 2706 } 2707 else if (name.equals("administrationInstruction")) { 2708 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.enteralFormula.administrationInstruction"); 2709 } 2710 else 2711 return super.addChild(name); 2712 } 2713 2714 public NutritionOrderEnteralFormulaComponent copy() { 2715 NutritionOrderEnteralFormulaComponent dst = new NutritionOrderEnteralFormulaComponent(); 2716 copyValues(dst); 2717 return dst; 2718 } 2719 2720 public void copyValues(NutritionOrderEnteralFormulaComponent dst) { 2721 super.copyValues(dst); 2722 dst.baseFormulaType = baseFormulaType == null ? null : baseFormulaType.copy(); 2723 dst.baseFormulaProductName = baseFormulaProductName == null ? null : baseFormulaProductName.copy(); 2724 if (deliveryDevice != null) { 2725 dst.deliveryDevice = new ArrayList<CodeableReference>(); 2726 for (CodeableReference i : deliveryDevice) 2727 dst.deliveryDevice.add(i.copy()); 2728 }; 2729 if (additive != null) { 2730 dst.additive = new ArrayList<NutritionOrderEnteralFormulaAdditiveComponent>(); 2731 for (NutritionOrderEnteralFormulaAdditiveComponent i : additive) 2732 dst.additive.add(i.copy()); 2733 }; 2734 dst.caloricDensity = caloricDensity == null ? null : caloricDensity.copy(); 2735 dst.routeOfAdministration = routeOfAdministration == null ? null : routeOfAdministration.copy(); 2736 if (administration != null) { 2737 dst.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2738 for (NutritionOrderEnteralFormulaAdministrationComponent i : administration) 2739 dst.administration.add(i.copy()); 2740 }; 2741 dst.maxVolumeToDeliver = maxVolumeToDeliver == null ? null : maxVolumeToDeliver.copy(); 2742 dst.administrationInstruction = administrationInstruction == null ? null : administrationInstruction.copy(); 2743 } 2744 2745 @Override 2746 public boolean equalsDeep(Base other_) { 2747 if (!super.equalsDeep(other_)) 2748 return false; 2749 if (!(other_ instanceof NutritionOrderEnteralFormulaComponent)) 2750 return false; 2751 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other_; 2752 return compareDeep(baseFormulaType, o.baseFormulaType, true) && compareDeep(baseFormulaProductName, o.baseFormulaProductName, true) 2753 && compareDeep(deliveryDevice, o.deliveryDevice, true) && compareDeep(additive, o.additive, true) 2754 && compareDeep(caloricDensity, o.caloricDensity, true) && compareDeep(routeOfAdministration, o.routeOfAdministration, true) 2755 && compareDeep(administration, o.administration, true) && compareDeep(maxVolumeToDeliver, o.maxVolumeToDeliver, true) 2756 && compareDeep(administrationInstruction, o.administrationInstruction, true); 2757 } 2758 2759 @Override 2760 public boolean equalsShallow(Base other_) { 2761 if (!super.equalsShallow(other_)) 2762 return false; 2763 if (!(other_ instanceof NutritionOrderEnteralFormulaComponent)) 2764 return false; 2765 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other_; 2766 return compareValues(baseFormulaProductName, o.baseFormulaProductName, true) && compareValues(administrationInstruction, o.administrationInstruction, true) 2767 ; 2768 } 2769 2770 public boolean isEmpty() { 2771 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(baseFormulaType, baseFormulaProductName 2772 , deliveryDevice, additive, caloricDensity, routeOfAdministration, administration 2773 , maxVolumeToDeliver, administrationInstruction); 2774 } 2775 2776 public String fhirType() { 2777 return "NutritionOrder.enteralFormula"; 2778 2779 } 2780 2781 } 2782 2783 @Block() 2784 public static class NutritionOrderEnteralFormulaAdditiveComponent extends BackboneElement implements IBaseBackboneElement { 2785 /** 2786 * Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula. 2787 */ 2788 @Child(name = "type", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=false) 2789 @Description(shortDefinition="Type of modular component to add to the feeding", formalDefinition="Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula." ) 2790 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/entformula-additive") 2791 protected CodeableReference type; 2792 2793 /** 2794 * The product or brand name of the type of modular component to be added to the formula. 2795 */ 2796 @Child(name = "productName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2797 @Description(shortDefinition="Product or brand name of the modular additive", formalDefinition="The product or brand name of the type of modular component to be added to the formula." ) 2798 protected StringType productName; 2799 2800 /** 2801 * The amount of additive to be given in addition or to be mixed in with the base formula. 2802 */ 2803 @Child(name = "quantity", type = {Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 2804 @Description(shortDefinition="Amount of additive to be given or mixed in", formalDefinition="The amount of additive to be given in addition or to be mixed in with the base formula." ) 2805 protected Quantity quantity; 2806 2807 private static final long serialVersionUID = 2072791035L; 2808 2809 /** 2810 * Constructor 2811 */ 2812 public NutritionOrderEnteralFormulaAdditiveComponent() { 2813 super(); 2814 } 2815 2816 /** 2817 * @return {@link #type} (Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.) 2818 */ 2819 public CodeableReference getType() { 2820 if (this.type == null) 2821 if (Configuration.errorOnAutoCreate()) 2822 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdditiveComponent.type"); 2823 else if (Configuration.doAutoCreate()) 2824 this.type = new CodeableReference(); // cc 2825 return this.type; 2826 } 2827 2828 public boolean hasType() { 2829 return this.type != null && !this.type.isEmpty(); 2830 } 2831 2832 /** 2833 * @param value {@link #type} (Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.) 2834 */ 2835 public NutritionOrderEnteralFormulaAdditiveComponent setType(CodeableReference value) { 2836 this.type = value; 2837 return this; 2838 } 2839 2840 /** 2841 * @return {@link #productName} (The product or brand name of the type of modular component to be added to the formula.). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 2842 */ 2843 public StringType getProductNameElement() { 2844 if (this.productName == null) 2845 if (Configuration.errorOnAutoCreate()) 2846 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdditiveComponent.productName"); 2847 else if (Configuration.doAutoCreate()) 2848 this.productName = new StringType(); // bb 2849 return this.productName; 2850 } 2851 2852 public boolean hasProductNameElement() { 2853 return this.productName != null && !this.productName.isEmpty(); 2854 } 2855 2856 public boolean hasProductName() { 2857 return this.productName != null && !this.productName.isEmpty(); 2858 } 2859 2860 /** 2861 * @param value {@link #productName} (The product or brand name of the type of modular component to be added to the formula.). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 2862 */ 2863 public NutritionOrderEnteralFormulaAdditiveComponent setProductNameElement(StringType value) { 2864 this.productName = value; 2865 return this; 2866 } 2867 2868 /** 2869 * @return The product or brand name of the type of modular component to be added to the formula. 2870 */ 2871 public String getProductName() { 2872 return this.productName == null ? null : this.productName.getValue(); 2873 } 2874 2875 /** 2876 * @param value The product or brand name of the type of modular component to be added to the formula. 2877 */ 2878 public NutritionOrderEnteralFormulaAdditiveComponent setProductName(String value) { 2879 if (Utilities.noString(value)) 2880 this.productName = null; 2881 else { 2882 if (this.productName == null) 2883 this.productName = new StringType(); 2884 this.productName.setValue(value); 2885 } 2886 return this; 2887 } 2888 2889 /** 2890 * @return {@link #quantity} (The amount of additive to be given in addition or to be mixed in with the base formula.) 2891 */ 2892 public Quantity getQuantity() { 2893 if (this.quantity == null) 2894 if (Configuration.errorOnAutoCreate()) 2895 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdditiveComponent.quantity"); 2896 else if (Configuration.doAutoCreate()) 2897 this.quantity = new Quantity(); // cc 2898 return this.quantity; 2899 } 2900 2901 public boolean hasQuantity() { 2902 return this.quantity != null && !this.quantity.isEmpty(); 2903 } 2904 2905 /** 2906 * @param value {@link #quantity} (The amount of additive to be given in addition or to be mixed in with the base formula.) 2907 */ 2908 public NutritionOrderEnteralFormulaAdditiveComponent setQuantity(Quantity value) { 2909 this.quantity = value; 2910 return this; 2911 } 2912 2913 protected void listChildren(List<Property> children) { 2914 super.listChildren(children); 2915 children.add(new Property("type", "CodeableReference(NutritionProduct)", "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 0, 1, type)); 2916 children.add(new Property("productName", "string", "The product or brand name of the type of modular component to be added to the formula.", 0, 1, productName)); 2917 children.add(new Property("quantity", "Quantity", "The amount of additive to be given in addition or to be mixed in with the base formula.", 0, 1, quantity)); 2918 } 2919 2920 @Override 2921 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2922 switch (_hash) { 2923 case 3575610: /*type*/ return new Property("type", "CodeableReference(NutritionProduct)", "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 0, 1, type); 2924 case -1491817446: /*productName*/ return new Property("productName", "string", "The product or brand name of the type of modular component to be added to the formula.", 0, 1, productName); 2925 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of additive to be given in addition or to be mixed in with the base formula.", 0, 1, quantity); 2926 default: return super.getNamedProperty(_hash, _name, _checkValid); 2927 } 2928 2929 } 2930 2931 @Override 2932 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2933 switch (hash) { 2934 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableReference 2935 case -1491817446: /*productName*/ return this.productName == null ? new Base[0] : new Base[] {this.productName}; // StringType 2936 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 2937 default: return super.getProperty(hash, name, checkValid); 2938 } 2939 2940 } 2941 2942 @Override 2943 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2944 switch (hash) { 2945 case 3575610: // type 2946 this.type = TypeConvertor.castToCodeableReference(value); // CodeableReference 2947 return value; 2948 case -1491817446: // productName 2949 this.productName = TypeConvertor.castToString(value); // StringType 2950 return value; 2951 case -1285004149: // quantity 2952 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2953 return value; 2954 default: return super.setProperty(hash, name, value); 2955 } 2956 2957 } 2958 2959 @Override 2960 public Base setProperty(String name, Base value) throws FHIRException { 2961 if (name.equals("type")) { 2962 this.type = TypeConvertor.castToCodeableReference(value); // CodeableReference 2963 } else if (name.equals("productName")) { 2964 this.productName = TypeConvertor.castToString(value); // StringType 2965 } else if (name.equals("quantity")) { 2966 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2967 } else 2968 return super.setProperty(name, value); 2969 return value; 2970 } 2971 2972 @Override 2973 public void removeChild(String name, Base value) throws FHIRException { 2974 if (name.equals("type")) { 2975 this.type = null; 2976 } else if (name.equals("productName")) { 2977 this.productName = null; 2978 } else if (name.equals("quantity")) { 2979 this.quantity = null; 2980 } else 2981 super.removeChild(name, value); 2982 2983 } 2984 2985 @Override 2986 public Base makeProperty(int hash, String name) throws FHIRException { 2987 switch (hash) { 2988 case 3575610: return getType(); 2989 case -1491817446: return getProductNameElement(); 2990 case -1285004149: return getQuantity(); 2991 default: return super.makeProperty(hash, name); 2992 } 2993 2994 } 2995 2996 @Override 2997 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2998 switch (hash) { 2999 case 3575610: /*type*/ return new String[] {"CodeableReference"}; 3000 case -1491817446: /*productName*/ return new String[] {"string"}; 3001 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 3002 default: return super.getTypesForProperty(hash, name); 3003 } 3004 3005 } 3006 3007 @Override 3008 public Base addChild(String name) throws FHIRException { 3009 if (name.equals("type")) { 3010 this.type = new CodeableReference(); 3011 return this.type; 3012 } 3013 else if (name.equals("productName")) { 3014 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.enteralFormula.additive.productName"); 3015 } 3016 else if (name.equals("quantity")) { 3017 this.quantity = new Quantity(); 3018 return this.quantity; 3019 } 3020 else 3021 return super.addChild(name); 3022 } 3023 3024 public NutritionOrderEnteralFormulaAdditiveComponent copy() { 3025 NutritionOrderEnteralFormulaAdditiveComponent dst = new NutritionOrderEnteralFormulaAdditiveComponent(); 3026 copyValues(dst); 3027 return dst; 3028 } 3029 3030 public void copyValues(NutritionOrderEnteralFormulaAdditiveComponent dst) { 3031 super.copyValues(dst); 3032 dst.type = type == null ? null : type.copy(); 3033 dst.productName = productName == null ? null : productName.copy(); 3034 dst.quantity = quantity == null ? null : quantity.copy(); 3035 } 3036 3037 @Override 3038 public boolean equalsDeep(Base other_) { 3039 if (!super.equalsDeep(other_)) 3040 return false; 3041 if (!(other_ instanceof NutritionOrderEnteralFormulaAdditiveComponent)) 3042 return false; 3043 NutritionOrderEnteralFormulaAdditiveComponent o = (NutritionOrderEnteralFormulaAdditiveComponent) other_; 3044 return compareDeep(type, o.type, true) && compareDeep(productName, o.productName, true) && compareDeep(quantity, o.quantity, true) 3045 ; 3046 } 3047 3048 @Override 3049 public boolean equalsShallow(Base other_) { 3050 if (!super.equalsShallow(other_)) 3051 return false; 3052 if (!(other_ instanceof NutritionOrderEnteralFormulaAdditiveComponent)) 3053 return false; 3054 NutritionOrderEnteralFormulaAdditiveComponent o = (NutritionOrderEnteralFormulaAdditiveComponent) other_; 3055 return compareValues(productName, o.productName, true); 3056 } 3057 3058 public boolean isEmpty() { 3059 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, productName, quantity 3060 ); 3061 } 3062 3063 public String fhirType() { 3064 return "NutritionOrder.enteralFormula.additive"; 3065 3066 } 3067 3068 } 3069 3070 @Block() 3071 public static class NutritionOrderEnteralFormulaAdministrationComponent extends BackboneElement implements IBaseBackboneElement { 3072 /** 3073 * Schedule information for an enteral formula. 3074 */ 3075 @Child(name = "schedule", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 3076 @Description(shortDefinition="Scheduling information for enteral formula products", formalDefinition="Schedule information for an enteral formula." ) 3077 protected EnteralFormulaScheduleComponent schedule; 3078 3079 /** 3080 * The volume of formula to provide to the patient per the specified administration schedule. 3081 */ 3082 @Child(name = "quantity", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 3083 @Description(shortDefinition="The volume of formula to provide", formalDefinition="The volume of formula to provide to the patient per the specified administration schedule." ) 3084 protected Quantity quantity; 3085 3086 /** 3087 * The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule. 3088 */ 3089 @Child(name = "rate", type = {Quantity.class, Ratio.class}, order=3, min=0, max=1, modifier=false, summary=false) 3090 @Description(shortDefinition="Speed with which the formula is provided per period of time", formalDefinition="The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule." ) 3091 protected DataType rate; 3092 3093 private static final long serialVersionUID = 42374218L; 3094 3095 /** 3096 * Constructor 3097 */ 3098 public NutritionOrderEnteralFormulaAdministrationComponent() { 3099 super(); 3100 } 3101 3102 /** 3103 * @return {@link #schedule} (Schedule information for an enteral formula.) 3104 */ 3105 public EnteralFormulaScheduleComponent getSchedule() { 3106 if (this.schedule == null) 3107 if (Configuration.errorOnAutoCreate()) 3108 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.schedule"); 3109 else if (Configuration.doAutoCreate()) 3110 this.schedule = new EnteralFormulaScheduleComponent(); // cc 3111 return this.schedule; 3112 } 3113 3114 public boolean hasSchedule() { 3115 return this.schedule != null && !this.schedule.isEmpty(); 3116 } 3117 3118 /** 3119 * @param value {@link #schedule} (Schedule information for an enteral formula.) 3120 */ 3121 public NutritionOrderEnteralFormulaAdministrationComponent setSchedule(EnteralFormulaScheduleComponent value) { 3122 this.schedule = value; 3123 return this; 3124 } 3125 3126 /** 3127 * @return {@link #quantity} (The volume of formula to provide to the patient per the specified administration schedule.) 3128 */ 3129 public Quantity getQuantity() { 3130 if (this.quantity == null) 3131 if (Configuration.errorOnAutoCreate()) 3132 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.quantity"); 3133 else if (Configuration.doAutoCreate()) 3134 this.quantity = new Quantity(); // cc 3135 return this.quantity; 3136 } 3137 3138 public boolean hasQuantity() { 3139 return this.quantity != null && !this.quantity.isEmpty(); 3140 } 3141 3142 /** 3143 * @param value {@link #quantity} (The volume of formula to provide to the patient per the specified administration schedule.) 3144 */ 3145 public NutritionOrderEnteralFormulaAdministrationComponent setQuantity(Quantity value) { 3146 this.quantity = value; 3147 return this; 3148 } 3149 3150 /** 3151 * @return {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 3152 */ 3153 public DataType getRate() { 3154 return this.rate; 3155 } 3156 3157 /** 3158 * @return {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 3159 */ 3160 public Quantity getRateQuantity() throws FHIRException { 3161 if (this.rate == null) 3162 this.rate = new Quantity(); 3163 if (!(this.rate instanceof Quantity)) 3164 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.rate.getClass().getName()+" was encountered"); 3165 return (Quantity) this.rate; 3166 } 3167 3168 public boolean hasRateQuantity() { 3169 return this != null && this.rate instanceof Quantity; 3170 } 3171 3172 /** 3173 * @return {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 3174 */ 3175 public Ratio getRateRatio() throws FHIRException { 3176 if (this.rate == null) 3177 this.rate = new Ratio(); 3178 if (!(this.rate instanceof Ratio)) 3179 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.rate.getClass().getName()+" was encountered"); 3180 return (Ratio) this.rate; 3181 } 3182 3183 public boolean hasRateRatio() { 3184 return this != null && this.rate instanceof Ratio; 3185 } 3186 3187 public boolean hasRate() { 3188 return this.rate != null && !this.rate.isEmpty(); 3189 } 3190 3191 /** 3192 * @param value {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 3193 */ 3194 public NutritionOrderEnteralFormulaAdministrationComponent setRate(DataType value) { 3195 if (value != null && !(value instanceof Quantity || value instanceof Ratio)) 3196 throw new FHIRException("Not the right type for NutritionOrder.enteralFormula.administration.rate[x]: "+value.fhirType()); 3197 this.rate = value; 3198 return this; 3199 } 3200 3201 protected void listChildren(List<Property> children) { 3202 super.listChildren(children); 3203 children.add(new Property("schedule", "", "Schedule information for an enteral formula.", 0, 1, schedule)); 3204 children.add(new Property("quantity", "Quantity", "The volume of formula to provide to the patient per the specified administration schedule.", 0, 1, quantity)); 3205 children.add(new Property("rate[x]", "Quantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate)); 3206 } 3207 3208 @Override 3209 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3210 switch (_hash) { 3211 case -697920873: /*schedule*/ return new Property("schedule", "", "Schedule information for an enteral formula.", 0, 1, schedule); 3212 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The volume of formula to provide to the patient per the specified administration schedule.", 0, 1, quantity); 3213 case 983460768: /*rate[x]*/ return new Property("rate[x]", "Quantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 3214 case 3493088: /*rate*/ return new Property("rate[x]", "Quantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 3215 case -1085459061: /*rateQuantity*/ return new Property("rate[x]", "Quantity", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 3216 case 204021515: /*rateRatio*/ return new Property("rate[x]", "Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 3217 default: return super.getNamedProperty(_hash, _name, _checkValid); 3218 } 3219 3220 } 3221 3222 @Override 3223 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3224 switch (hash) { 3225 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : new Base[] {this.schedule}; // EnteralFormulaScheduleComponent 3226 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 3227 case 3493088: /*rate*/ return this.rate == null ? new Base[0] : new Base[] {this.rate}; // DataType 3228 default: return super.getProperty(hash, name, checkValid); 3229 } 3230 3231 } 3232 3233 @Override 3234 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3235 switch (hash) { 3236 case -697920873: // schedule 3237 this.schedule = (EnteralFormulaScheduleComponent) value; // EnteralFormulaScheduleComponent 3238 return value; 3239 case -1285004149: // quantity 3240 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 3241 return value; 3242 case 3493088: // rate 3243 this.rate = TypeConvertor.castToType(value); // DataType 3244 return value; 3245 default: return super.setProperty(hash, name, value); 3246 } 3247 3248 } 3249 3250 @Override 3251 public Base setProperty(String name, Base value) throws FHIRException { 3252 if (name.equals("schedule")) { 3253 this.schedule = (EnteralFormulaScheduleComponent) value; // EnteralFormulaScheduleComponent 3254 } else if (name.equals("quantity")) { 3255 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 3256 } else if (name.equals("rate[x]")) { 3257 this.rate = TypeConvertor.castToType(value); // DataType 3258 } else 3259 return super.setProperty(name, value); 3260 return value; 3261 } 3262 3263 @Override 3264 public void removeChild(String name, Base value) throws FHIRException { 3265 if (name.equals("schedule")) { 3266 this.schedule = (EnteralFormulaScheduleComponent) value; // EnteralFormulaScheduleComponent 3267 } else if (name.equals("quantity")) { 3268 this.quantity = null; 3269 } else if (name.equals("rate[x]")) { 3270 this.rate = null; 3271 } else 3272 super.removeChild(name, value); 3273 3274 } 3275 3276 @Override 3277 public Base makeProperty(int hash, String name) throws FHIRException { 3278 switch (hash) { 3279 case -697920873: return getSchedule(); 3280 case -1285004149: return getQuantity(); 3281 case 983460768: return getRate(); 3282 case 3493088: return getRate(); 3283 default: return super.makeProperty(hash, name); 3284 } 3285 3286 } 3287 3288 @Override 3289 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3290 switch (hash) { 3291 case -697920873: /*schedule*/ return new String[] {}; 3292 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 3293 case 3493088: /*rate*/ return new String[] {"Quantity", "Ratio"}; 3294 default: return super.getTypesForProperty(hash, name); 3295 } 3296 3297 } 3298 3299 @Override 3300 public Base addChild(String name) throws FHIRException { 3301 if (name.equals("schedule")) { 3302 this.schedule = new EnteralFormulaScheduleComponent(); 3303 return this.schedule; 3304 } 3305 else if (name.equals("quantity")) { 3306 this.quantity = new Quantity(); 3307 return this.quantity; 3308 } 3309 else if (name.equals("rateQuantity")) { 3310 this.rate = new Quantity(); 3311 return this.rate; 3312 } 3313 else if (name.equals("rateRatio")) { 3314 this.rate = new Ratio(); 3315 return this.rate; 3316 } 3317 else 3318 return super.addChild(name); 3319 } 3320 3321 public NutritionOrderEnteralFormulaAdministrationComponent copy() { 3322 NutritionOrderEnteralFormulaAdministrationComponent dst = new NutritionOrderEnteralFormulaAdministrationComponent(); 3323 copyValues(dst); 3324 return dst; 3325 } 3326 3327 public void copyValues(NutritionOrderEnteralFormulaAdministrationComponent dst) { 3328 super.copyValues(dst); 3329 dst.schedule = schedule == null ? null : schedule.copy(); 3330 dst.quantity = quantity == null ? null : quantity.copy(); 3331 dst.rate = rate == null ? null : rate.copy(); 3332 } 3333 3334 @Override 3335 public boolean equalsDeep(Base other_) { 3336 if (!super.equalsDeep(other_)) 3337 return false; 3338 if (!(other_ instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 3339 return false; 3340 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other_; 3341 return compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) && compareDeep(rate, o.rate, true) 3342 ; 3343 } 3344 3345 @Override 3346 public boolean equalsShallow(Base other_) { 3347 if (!super.equalsShallow(other_)) 3348 return false; 3349 if (!(other_ instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 3350 return false; 3351 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other_; 3352 return true; 3353 } 3354 3355 public boolean isEmpty() { 3356 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(schedule, quantity, rate 3357 ); 3358 } 3359 3360 public String fhirType() { 3361 return "NutritionOrder.enteralFormula.administration"; 3362 3363 } 3364 3365 } 3366 3367 @Block() 3368 public static class EnteralFormulaScheduleComponent extends BackboneElement implements IBaseBackboneElement { 3369 /** 3370 * The time period and frequency at which the enteral formula should be given. The enteral formula should be given for the combination of all schedules if more than one schedule is present. 3371 */ 3372 @Child(name = "timing", type = {Timing.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3373 @Description(shortDefinition="Scheduled frequency of enteral formula", formalDefinition="The time period and frequency at which the enteral formula should be given. The enteral formula should be given for the combination of all schedules if more than one schedule is present." ) 3374 protected List<Timing> timing; 3375 3376 /** 3377 * Indicates whether the enteral formula is only taken when needed within a specific dosing schedule. 3378 */ 3379 @Child(name = "asNeeded", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3380 @Description(shortDefinition="Take 'as needed'", formalDefinition="Indicates whether the enteral formula is only taken when needed within a specific dosing schedule." ) 3381 protected BooleanType asNeeded; 3382 3383 /** 3384 * Indicates whether the enteral formula is only taken based on a precondition for taking the enteral formula. 3385 */ 3386 @Child(name = "asNeededFor", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 3387 @Description(shortDefinition="Take 'as needed' for x", formalDefinition="Indicates whether the enteral formula is only taken based on a precondition for taking the enteral formula." ) 3388 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 3389 protected CodeableConcept asNeededFor; 3390 3391 private static final long serialVersionUID = -1051458478L; 3392 3393 /** 3394 * Constructor 3395 */ 3396 public EnteralFormulaScheduleComponent() { 3397 super(); 3398 } 3399 3400 /** 3401 * @return {@link #timing} (The time period and frequency at which the enteral formula should be given. The enteral formula should be given for the combination of all schedules if more than one schedule is present.) 3402 */ 3403 public List<Timing> getTiming() { 3404 if (this.timing == null) 3405 this.timing = new ArrayList<Timing>(); 3406 return this.timing; 3407 } 3408 3409 /** 3410 * @return Returns a reference to <code>this</code> for easy method chaining 3411 */ 3412 public EnteralFormulaScheduleComponent setTiming(List<Timing> theTiming) { 3413 this.timing = theTiming; 3414 return this; 3415 } 3416 3417 public boolean hasTiming() { 3418 if (this.timing == null) 3419 return false; 3420 for (Timing item : this.timing) 3421 if (!item.isEmpty()) 3422 return true; 3423 return false; 3424 } 3425 3426 public Timing addTiming() { //3 3427 Timing t = new Timing(); 3428 if (this.timing == null) 3429 this.timing = new ArrayList<Timing>(); 3430 this.timing.add(t); 3431 return t; 3432 } 3433 3434 public EnteralFormulaScheduleComponent addTiming(Timing t) { //3 3435 if (t == null) 3436 return this; 3437 if (this.timing == null) 3438 this.timing = new ArrayList<Timing>(); 3439 this.timing.add(t); 3440 return this; 3441 } 3442 3443 /** 3444 * @return The first repetition of repeating field {@link #timing}, creating it if it does not already exist {3} 3445 */ 3446 public Timing getTimingFirstRep() { 3447 if (getTiming().isEmpty()) { 3448 addTiming(); 3449 } 3450 return getTiming().get(0); 3451 } 3452 3453 /** 3454 * @return {@link #asNeeded} (Indicates whether the enteral formula is only taken when needed within a specific dosing schedule.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 3455 */ 3456 public BooleanType getAsNeededElement() { 3457 if (this.asNeeded == null) 3458 if (Configuration.errorOnAutoCreate()) 3459 throw new Error("Attempt to auto-create EnteralFormulaScheduleComponent.asNeeded"); 3460 else if (Configuration.doAutoCreate()) 3461 this.asNeeded = new BooleanType(); // bb 3462 return this.asNeeded; 3463 } 3464 3465 public boolean hasAsNeededElement() { 3466 return this.asNeeded != null && !this.asNeeded.isEmpty(); 3467 } 3468 3469 public boolean hasAsNeeded() { 3470 return this.asNeeded != null && !this.asNeeded.isEmpty(); 3471 } 3472 3473 /** 3474 * @param value {@link #asNeeded} (Indicates whether the enteral formula is only taken when needed within a specific dosing schedule.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 3475 */ 3476 public EnteralFormulaScheduleComponent setAsNeededElement(BooleanType value) { 3477 this.asNeeded = value; 3478 return this; 3479 } 3480 3481 /** 3482 * @return Indicates whether the enteral formula is only taken when needed within a specific dosing schedule. 3483 */ 3484 public boolean getAsNeeded() { 3485 return this.asNeeded == null || this.asNeeded.isEmpty() ? false : this.asNeeded.getValue(); 3486 } 3487 3488 /** 3489 * @param value Indicates whether the enteral formula is only taken when needed within a specific dosing schedule. 3490 */ 3491 public EnteralFormulaScheduleComponent setAsNeeded(boolean value) { 3492 if (this.asNeeded == null) 3493 this.asNeeded = new BooleanType(); 3494 this.asNeeded.setValue(value); 3495 return this; 3496 } 3497 3498 /** 3499 * @return {@link #asNeededFor} (Indicates whether the enteral formula is only taken based on a precondition for taking the enteral formula.) 3500 */ 3501 public CodeableConcept getAsNeededFor() { 3502 if (this.asNeededFor == null) 3503 if (Configuration.errorOnAutoCreate()) 3504 throw new Error("Attempt to auto-create EnteralFormulaScheduleComponent.asNeededFor"); 3505 else if (Configuration.doAutoCreate()) 3506 this.asNeededFor = new CodeableConcept(); // cc 3507 return this.asNeededFor; 3508 } 3509 3510 public boolean hasAsNeededFor() { 3511 return this.asNeededFor != null && !this.asNeededFor.isEmpty(); 3512 } 3513 3514 /** 3515 * @param value {@link #asNeededFor} (Indicates whether the enteral formula is only taken based on a precondition for taking the enteral formula.) 3516 */ 3517 public EnteralFormulaScheduleComponent setAsNeededFor(CodeableConcept value) { 3518 this.asNeededFor = value; 3519 return this; 3520 } 3521 3522 protected void listChildren(List<Property> children) { 3523 super.listChildren(children); 3524 children.add(new Property("timing", "Timing", "The time period and frequency at which the enteral formula should be given. The enteral formula should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, timing)); 3525 children.add(new Property("asNeeded", "boolean", "Indicates whether the enteral formula is only taken when needed within a specific dosing schedule.", 0, 1, asNeeded)); 3526 children.add(new Property("asNeededFor", "CodeableConcept", "Indicates whether the enteral formula is only taken based on a precondition for taking the enteral formula.", 0, 1, asNeededFor)); 3527 } 3528 3529 @Override 3530 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3531 switch (_hash) { 3532 case -873664438: /*timing*/ return new Property("timing", "Timing", "The time period and frequency at which the enteral formula should be given. The enteral formula should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, timing); 3533 case -1432923513: /*asNeeded*/ return new Property("asNeeded", "boolean", "Indicates whether the enteral formula is only taken when needed within a specific dosing schedule.", 0, 1, asNeeded); 3534 case -544350014: /*asNeededFor*/ return new Property("asNeededFor", "CodeableConcept", "Indicates whether the enteral formula is only taken based on a precondition for taking the enteral formula.", 0, 1, asNeededFor); 3535 default: return super.getNamedProperty(_hash, _name, _checkValid); 3536 } 3537 3538 } 3539 3540 @Override 3541 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3542 switch (hash) { 3543 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : this.timing.toArray(new Base[this.timing.size()]); // Timing 3544 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // BooleanType 3545 case -544350014: /*asNeededFor*/ return this.asNeededFor == null ? new Base[0] : new Base[] {this.asNeededFor}; // CodeableConcept 3546 default: return super.getProperty(hash, name, checkValid); 3547 } 3548 3549 } 3550 3551 @Override 3552 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3553 switch (hash) { 3554 case -873664438: // timing 3555 this.getTiming().add(TypeConvertor.castToTiming(value)); // Timing 3556 return value; 3557 case -1432923513: // asNeeded 3558 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 3559 return value; 3560 case -544350014: // asNeededFor 3561 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3562 return value; 3563 default: return super.setProperty(hash, name, value); 3564 } 3565 3566 } 3567 3568 @Override 3569 public Base setProperty(String name, Base value) throws FHIRException { 3570 if (name.equals("timing")) { 3571 this.getTiming().add(TypeConvertor.castToTiming(value)); 3572 } else if (name.equals("asNeeded")) { 3573 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 3574 } else if (name.equals("asNeededFor")) { 3575 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3576 } else 3577 return super.setProperty(name, value); 3578 return value; 3579 } 3580 3581 @Override 3582 public void removeChild(String name, Base value) throws FHIRException { 3583 if (name.equals("timing")) { 3584 this.getTiming().remove(value); 3585 } else if (name.equals("asNeeded")) { 3586 this.asNeeded = null; 3587 } else if (name.equals("asNeededFor")) { 3588 this.asNeededFor = null; 3589 } else 3590 super.removeChild(name, value); 3591 3592 } 3593 3594 @Override 3595 public Base makeProperty(int hash, String name) throws FHIRException { 3596 switch (hash) { 3597 case -873664438: return addTiming(); 3598 case -1432923513: return getAsNeededElement(); 3599 case -544350014: return getAsNeededFor(); 3600 default: return super.makeProperty(hash, name); 3601 } 3602 3603 } 3604 3605 @Override 3606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3607 switch (hash) { 3608 case -873664438: /*timing*/ return new String[] {"Timing"}; 3609 case -1432923513: /*asNeeded*/ return new String[] {"boolean"}; 3610 case -544350014: /*asNeededFor*/ return new String[] {"CodeableConcept"}; 3611 default: return super.getTypesForProperty(hash, name); 3612 } 3613 3614 } 3615 3616 @Override 3617 public Base addChild(String name) throws FHIRException { 3618 if (name.equals("timing")) { 3619 return addTiming(); 3620 } 3621 else if (name.equals("asNeeded")) { 3622 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.enteralFormula.administration.schedule.asNeeded"); 3623 } 3624 else if (name.equals("asNeededFor")) { 3625 this.asNeededFor = new CodeableConcept(); 3626 return this.asNeededFor; 3627 } 3628 else 3629 return super.addChild(name); 3630 } 3631 3632 public EnteralFormulaScheduleComponent copy() { 3633 EnteralFormulaScheduleComponent dst = new EnteralFormulaScheduleComponent(); 3634 copyValues(dst); 3635 return dst; 3636 } 3637 3638 public void copyValues(EnteralFormulaScheduleComponent dst) { 3639 super.copyValues(dst); 3640 if (timing != null) { 3641 dst.timing = new ArrayList<Timing>(); 3642 for (Timing i : timing) 3643 dst.timing.add(i.copy()); 3644 }; 3645 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 3646 dst.asNeededFor = asNeededFor == null ? null : asNeededFor.copy(); 3647 } 3648 3649 @Override 3650 public boolean equalsDeep(Base other_) { 3651 if (!super.equalsDeep(other_)) 3652 return false; 3653 if (!(other_ instanceof EnteralFormulaScheduleComponent)) 3654 return false; 3655 EnteralFormulaScheduleComponent o = (EnteralFormulaScheduleComponent) other_; 3656 return compareDeep(timing, o.timing, true) && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(asNeededFor, o.asNeededFor, true) 3657 ; 3658 } 3659 3660 @Override 3661 public boolean equalsShallow(Base other_) { 3662 if (!super.equalsShallow(other_)) 3663 return false; 3664 if (!(other_ instanceof EnteralFormulaScheduleComponent)) 3665 return false; 3666 EnteralFormulaScheduleComponent o = (EnteralFormulaScheduleComponent) other_; 3667 return compareValues(asNeeded, o.asNeeded, true); 3668 } 3669 3670 public boolean isEmpty() { 3671 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(timing, asNeeded, asNeededFor 3672 ); 3673 } 3674 3675 public String fhirType() { 3676 return "NutritionOrder.enteralFormula.administration.schedule"; 3677 3678 } 3679 3680 } 3681 3682 /** 3683 * Identifiers assigned to this order by the order sender or by the order receiver. 3684 */ 3685 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3686 @Description(shortDefinition="Identifiers assigned to this order", formalDefinition="Identifiers assigned to this order by the order sender or by the order receiver." ) 3687 protected List<Identifier> identifier; 3688 3689 /** 3690 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder. 3691 */ 3692 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3693 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder." ) 3694 protected List<CanonicalType> instantiatesCanonical; 3695 3696 /** 3697 * The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder. 3698 */ 3699 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3700 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder." ) 3701 protected List<UriType> instantiatesUri; 3702 3703 /** 3704 * The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder. 3705 */ 3706 @Child(name = "instantiates", type = {UriType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3707 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder." ) 3708 protected List<UriType> instantiates; 3709 3710 /** 3711 * A plan or request that is fulfilled in whole or in part by this nutrition order. 3712 */ 3713 @Child(name = "basedOn", type = {CarePlan.class, NutritionOrder.class, ServiceRequest.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3714 @Description(shortDefinition="What this order fulfills", formalDefinition="A plan or request that is fulfilled in whole or in part by this nutrition order." ) 3715 protected List<Reference> basedOn; 3716 3717 /** 3718 * A shared identifier common to all nutrition orders that were authorized more or less simultaneously by a single author, representing the composite or group identifier. 3719 */ 3720 @Child(name = "groupIdentifier", type = {Identifier.class}, order=5, min=0, max=1, modifier=false, summary=true) 3721 @Description(shortDefinition="Composite Request ID", formalDefinition="A shared identifier common to all nutrition orders that were authorized more or less simultaneously by a single author, representing the composite or group identifier." ) 3722 protected Identifier groupIdentifier; 3723 3724 /** 3725 * The workflow status of the nutrition order/request. 3726 */ 3727 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 3728 @Description(shortDefinition="draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition="The workflow status of the nutrition order/request." ) 3729 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 3730 protected Enumeration<RequestStatus> status; 3731 3732 /** 3733 * Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain. 3734 */ 3735 @Child(name = "intent", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 3736 @Description(shortDefinition="proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain." ) 3737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 3738 protected Enumeration<RequestIntent> intent; 3739 3740 /** 3741 * Indicates how quickly the Nutrition Order should be addressed with respect to other requests. 3742 */ 3743 @Child(name = "priority", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=false) 3744 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the Nutrition Order should be addressed with respect to other requests." ) 3745 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 3746 protected Enumeration<RequestPriority> priority; 3747 3748 /** 3749 * The person or set of individuals who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding. 3750 */ 3751 @Child(name = "subject", type = {Patient.class, Group.class}, order=9, min=1, max=1, modifier=false, summary=true) 3752 @Description(shortDefinition="Who requires the diet, formula or nutritional supplement", formalDefinition="The person or set of individuals who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding." ) 3753 protected Reference subject; 3754 3755 /** 3756 * An encounter that provides additional information about the healthcare context in which this request is made. 3757 */ 3758 @Child(name = "encounter", type = {Encounter.class}, order=10, min=0, max=1, modifier=false, summary=false) 3759 @Description(shortDefinition="The encounter associated with this nutrition order", formalDefinition="An encounter that provides additional information about the healthcare context in which this request is made." ) 3760 protected Reference encounter; 3761 3762 /** 3763 * Information to support fulfilling (i.e. dispensing or administering) of the nutrition, for example, patient height and weight). 3764 */ 3765 @Child(name = "supportingInformation", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3766 @Description(shortDefinition="Information to support fulfilling of the nutrition order", formalDefinition="Information to support fulfilling (i.e. dispensing or administering) of the nutrition, for example, patient height and weight)." ) 3767 protected List<Reference> supportingInformation; 3768 3769 /** 3770 * The date and time that this nutrition order was requested. 3771 */ 3772 @Child(name = "dateTime", type = {DateTimeType.class}, order=12, min=1, max=1, modifier=false, summary=true) 3773 @Description(shortDefinition="Date and time the nutrition order was requested", formalDefinition="The date and time that this nutrition order was requested." ) 3774 protected DateTimeType dateTime; 3775 3776 /** 3777 * The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings. 3778 */ 3779 @Child(name = "orderer", type = {Practitioner.class, PractitionerRole.class}, order=13, min=0, max=1, modifier=false, summary=true) 3780 @Description(shortDefinition="Who ordered the diet, formula or nutritional supplement", formalDefinition="The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings." ) 3781 protected Reference orderer; 3782 3783 /** 3784 * The specified desired performer of the nutrition order. 3785 */ 3786 @Child(name = "performer", type = {CodeableReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3787 @Description(shortDefinition="Who is desired to perform the administration of what is being ordered", formalDefinition="The specified desired performer of the nutrition order." ) 3788 protected List<CodeableReference> performer; 3789 3790 /** 3791 * A link to a record of allergies or intolerances which should be included in the nutrition order. 3792 */ 3793 @Child(name = "allergyIntolerance", type = {AllergyIntolerance.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3794 @Description(shortDefinition="List of the patient's food and nutrition-related allergies and intolerances", formalDefinition="A link to a record of allergies or intolerances which should be included in the nutrition order." ) 3795 protected List<Reference> allergyIntolerance; 3796 3797 /** 3798 * This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings. 3799 */ 3800 @Child(name = "foodPreferenceModifier", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3801 @Description(shortDefinition="Order-specific modifier about the type of food that should be given", formalDefinition="This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings." ) 3802 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-diet") 3803 protected List<CodeableConcept> foodPreferenceModifier; 3804 3805 /** 3806 * This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings. 3807 */ 3808 @Child(name = "excludeFoodModifier", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3809 @Description(shortDefinition="Order-specific modifier about the type of food that should not be given", formalDefinition="This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings." ) 3810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/food-type") 3811 protected List<CodeableConcept> excludeFoodModifier; 3812 3813 /** 3814 * This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item. 3815 */ 3816 @Child(name = "outsideFoodAllowed", type = {BooleanType.class}, order=18, min=0, max=1, modifier=false, summary=false) 3817 @Description(shortDefinition="Capture when a food item is brought in by the patient and/or family", formalDefinition="This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item." ) 3818 protected BooleanType outsideFoodAllowed; 3819 3820 /** 3821 * Diet given orally in contrast to enteral (tube) feeding. 3822 */ 3823 @Child(name = "oralDiet", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 3824 @Description(shortDefinition="Oral diet components", formalDefinition="Diet given orally in contrast to enteral (tube) feeding." ) 3825 protected NutritionOrderOralDietComponent oralDiet; 3826 3827 /** 3828 * Oral nutritional products given in order to add further nutritional value to the patient's diet. 3829 */ 3830 @Child(name = "supplement", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3831 @Description(shortDefinition="Supplement components", formalDefinition="Oral nutritional products given in order to add further nutritional value to the patient's diet." ) 3832 protected List<NutritionOrderSupplementComponent> supplement; 3833 3834 /** 3835 * Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity. 3836 */ 3837 @Child(name = "enteralFormula", type = {}, order=21, min=0, max=1, modifier=false, summary=false) 3838 @Description(shortDefinition="Enteral formula components", formalDefinition="Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity." ) 3839 protected NutritionOrderEnteralFormulaComponent enteralFormula; 3840 3841 /** 3842 * Comments made about the nutrition order by the requester, performer, subject or other participants. 3843 */ 3844 @Child(name = "note", type = {Annotation.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3845 @Description(shortDefinition="Comments", formalDefinition="Comments made about the nutrition order by the requester, performer, subject or other participants." ) 3846 protected List<Annotation> note; 3847 3848 private static final long serialVersionUID = -659986813L; 3849 3850 /** 3851 * Constructor 3852 */ 3853 public NutritionOrder() { 3854 super(); 3855 } 3856 3857 /** 3858 * Constructor 3859 */ 3860 public NutritionOrder(RequestStatus status, RequestIntent intent, Reference subject, Date dateTime) { 3861 super(); 3862 this.setStatus(status); 3863 this.setIntent(intent); 3864 this.setSubject(subject); 3865 this.setDateTime(dateTime); 3866 } 3867 3868 /** 3869 * @return {@link #identifier} (Identifiers assigned to this order by the order sender or by the order receiver.) 3870 */ 3871 public List<Identifier> getIdentifier() { 3872 if (this.identifier == null) 3873 this.identifier = new ArrayList<Identifier>(); 3874 return this.identifier; 3875 } 3876 3877 /** 3878 * @return Returns a reference to <code>this</code> for easy method chaining 3879 */ 3880 public NutritionOrder setIdentifier(List<Identifier> theIdentifier) { 3881 this.identifier = theIdentifier; 3882 return this; 3883 } 3884 3885 public boolean hasIdentifier() { 3886 if (this.identifier == null) 3887 return false; 3888 for (Identifier item : this.identifier) 3889 if (!item.isEmpty()) 3890 return true; 3891 return false; 3892 } 3893 3894 public Identifier addIdentifier() { //3 3895 Identifier t = new Identifier(); 3896 if (this.identifier == null) 3897 this.identifier = new ArrayList<Identifier>(); 3898 this.identifier.add(t); 3899 return t; 3900 } 3901 3902 public NutritionOrder addIdentifier(Identifier t) { //3 3903 if (t == null) 3904 return this; 3905 if (this.identifier == null) 3906 this.identifier = new ArrayList<Identifier>(); 3907 this.identifier.add(t); 3908 return this; 3909 } 3910 3911 /** 3912 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3913 */ 3914 public Identifier getIdentifierFirstRep() { 3915 if (getIdentifier().isEmpty()) { 3916 addIdentifier(); 3917 } 3918 return getIdentifier().get(0); 3919 } 3920 3921 /** 3922 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 3923 */ 3924 public List<CanonicalType> getInstantiatesCanonical() { 3925 if (this.instantiatesCanonical == null) 3926 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3927 return this.instantiatesCanonical; 3928 } 3929 3930 /** 3931 * @return Returns a reference to <code>this</code> for easy method chaining 3932 */ 3933 public NutritionOrder setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 3934 this.instantiatesCanonical = theInstantiatesCanonical; 3935 return this; 3936 } 3937 3938 public boolean hasInstantiatesCanonical() { 3939 if (this.instantiatesCanonical == null) 3940 return false; 3941 for (CanonicalType item : this.instantiatesCanonical) 3942 if (!item.isEmpty()) 3943 return true; 3944 return false; 3945 } 3946 3947 /** 3948 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 3949 */ 3950 public CanonicalType addInstantiatesCanonicalElement() {//2 3951 CanonicalType t = new CanonicalType(); 3952 if (this.instantiatesCanonical == null) 3953 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3954 this.instantiatesCanonical.add(t); 3955 return t; 3956 } 3957 3958 /** 3959 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 3960 */ 3961 public NutritionOrder addInstantiatesCanonical(String value) { //1 3962 CanonicalType t = new CanonicalType(); 3963 t.setValue(value); 3964 if (this.instantiatesCanonical == null) 3965 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3966 this.instantiatesCanonical.add(t); 3967 return this; 3968 } 3969 3970 /** 3971 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 3972 */ 3973 public boolean hasInstantiatesCanonical(String value) { 3974 if (this.instantiatesCanonical == null) 3975 return false; 3976 for (CanonicalType v : this.instantiatesCanonical) 3977 if (v.getValue().equals(value)) // canonical 3978 return true; 3979 return false; 3980 } 3981 3982 /** 3983 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 3984 */ 3985 public List<UriType> getInstantiatesUri() { 3986 if (this.instantiatesUri == null) 3987 this.instantiatesUri = new ArrayList<UriType>(); 3988 return this.instantiatesUri; 3989 } 3990 3991 /** 3992 * @return Returns a reference to <code>this</code> for easy method chaining 3993 */ 3994 public NutritionOrder setInstantiatesUri(List<UriType> theInstantiatesUri) { 3995 this.instantiatesUri = theInstantiatesUri; 3996 return this; 3997 } 3998 3999 public boolean hasInstantiatesUri() { 4000 if (this.instantiatesUri == null) 4001 return false; 4002 for (UriType item : this.instantiatesUri) 4003 if (!item.isEmpty()) 4004 return true; 4005 return false; 4006 } 4007 4008 /** 4009 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 4010 */ 4011 public UriType addInstantiatesUriElement() {//2 4012 UriType t = new UriType(); 4013 if (this.instantiatesUri == null) 4014 this.instantiatesUri = new ArrayList<UriType>(); 4015 this.instantiatesUri.add(t); 4016 return t; 4017 } 4018 4019 /** 4020 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 4021 */ 4022 public NutritionOrder addInstantiatesUri(String value) { //1 4023 UriType t = new UriType(); 4024 t.setValue(value); 4025 if (this.instantiatesUri == null) 4026 this.instantiatesUri = new ArrayList<UriType>(); 4027 this.instantiatesUri.add(t); 4028 return this; 4029 } 4030 4031 /** 4032 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 4033 */ 4034 public boolean hasInstantiatesUri(String value) { 4035 if (this.instantiatesUri == null) 4036 return false; 4037 for (UriType v : this.instantiatesUri) 4038 if (v.getValue().equals(value)) // uri 4039 return true; 4040 return false; 4041 } 4042 4043 /** 4044 * @return {@link #instantiates} (The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 4045 */ 4046 public List<UriType> getInstantiates() { 4047 if (this.instantiates == null) 4048 this.instantiates = new ArrayList<UriType>(); 4049 return this.instantiates; 4050 } 4051 4052 /** 4053 * @return Returns a reference to <code>this</code> for easy method chaining 4054 */ 4055 public NutritionOrder setInstantiates(List<UriType> theInstantiates) { 4056 this.instantiates = theInstantiates; 4057 return this; 4058 } 4059 4060 public boolean hasInstantiates() { 4061 if (this.instantiates == null) 4062 return false; 4063 for (UriType item : this.instantiates) 4064 if (!item.isEmpty()) 4065 return true; 4066 return false; 4067 } 4068 4069 /** 4070 * @return {@link #instantiates} (The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 4071 */ 4072 public UriType addInstantiatesElement() {//2 4073 UriType t = new UriType(); 4074 if (this.instantiates == null) 4075 this.instantiates = new ArrayList<UriType>(); 4076 this.instantiates.add(t); 4077 return t; 4078 } 4079 4080 /** 4081 * @param value {@link #instantiates} (The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 4082 */ 4083 public NutritionOrder addInstantiates(String value) { //1 4084 UriType t = new UriType(); 4085 t.setValue(value); 4086 if (this.instantiates == null) 4087 this.instantiates = new ArrayList<UriType>(); 4088 this.instantiates.add(t); 4089 return this; 4090 } 4091 4092 /** 4093 * @param value {@link #instantiates} (The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.) 4094 */ 4095 public boolean hasInstantiates(String value) { 4096 if (this.instantiates == null) 4097 return false; 4098 for (UriType v : this.instantiates) 4099 if (v.getValue().equals(value)) // uri 4100 return true; 4101 return false; 4102 } 4103 4104 /** 4105 * @return {@link #basedOn} (A plan or request that is fulfilled in whole or in part by this nutrition order.) 4106 */ 4107 public List<Reference> getBasedOn() { 4108 if (this.basedOn == null) 4109 this.basedOn = new ArrayList<Reference>(); 4110 return this.basedOn; 4111 } 4112 4113 /** 4114 * @return Returns a reference to <code>this</code> for easy method chaining 4115 */ 4116 public NutritionOrder setBasedOn(List<Reference> theBasedOn) { 4117 this.basedOn = theBasedOn; 4118 return this; 4119 } 4120 4121 public boolean hasBasedOn() { 4122 if (this.basedOn == null) 4123 return false; 4124 for (Reference item : this.basedOn) 4125 if (!item.isEmpty()) 4126 return true; 4127 return false; 4128 } 4129 4130 public Reference addBasedOn() { //3 4131 Reference t = new Reference(); 4132 if (this.basedOn == null) 4133 this.basedOn = new ArrayList<Reference>(); 4134 this.basedOn.add(t); 4135 return t; 4136 } 4137 4138 public NutritionOrder addBasedOn(Reference t) { //3 4139 if (t == null) 4140 return this; 4141 if (this.basedOn == null) 4142 this.basedOn = new ArrayList<Reference>(); 4143 this.basedOn.add(t); 4144 return this; 4145 } 4146 4147 /** 4148 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 4149 */ 4150 public Reference getBasedOnFirstRep() { 4151 if (getBasedOn().isEmpty()) { 4152 addBasedOn(); 4153 } 4154 return getBasedOn().get(0); 4155 } 4156 4157 /** 4158 * @return {@link #groupIdentifier} (A shared identifier common to all nutrition orders that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 4159 */ 4160 public Identifier getGroupIdentifier() { 4161 if (this.groupIdentifier == null) 4162 if (Configuration.errorOnAutoCreate()) 4163 throw new Error("Attempt to auto-create NutritionOrder.groupIdentifier"); 4164 else if (Configuration.doAutoCreate()) 4165 this.groupIdentifier = new Identifier(); // cc 4166 return this.groupIdentifier; 4167 } 4168 4169 public boolean hasGroupIdentifier() { 4170 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 4171 } 4172 4173 /** 4174 * @param value {@link #groupIdentifier} (A shared identifier common to all nutrition orders that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 4175 */ 4176 public NutritionOrder setGroupIdentifier(Identifier value) { 4177 this.groupIdentifier = value; 4178 return this; 4179 } 4180 4181 /** 4182 * @return {@link #status} (The workflow status of the nutrition order/request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4183 */ 4184 public Enumeration<RequestStatus> getStatusElement() { 4185 if (this.status == null) 4186 if (Configuration.errorOnAutoCreate()) 4187 throw new Error("Attempt to auto-create NutritionOrder.status"); 4188 else if (Configuration.doAutoCreate()) 4189 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 4190 return this.status; 4191 } 4192 4193 public boolean hasStatusElement() { 4194 return this.status != null && !this.status.isEmpty(); 4195 } 4196 4197 public boolean hasStatus() { 4198 return this.status != null && !this.status.isEmpty(); 4199 } 4200 4201 /** 4202 * @param value {@link #status} (The workflow status of the nutrition order/request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4203 */ 4204 public NutritionOrder setStatusElement(Enumeration<RequestStatus> value) { 4205 this.status = value; 4206 return this; 4207 } 4208 4209 /** 4210 * @return The workflow status of the nutrition order/request. 4211 */ 4212 public RequestStatus getStatus() { 4213 return this.status == null ? null : this.status.getValue(); 4214 } 4215 4216 /** 4217 * @param value The workflow status of the nutrition order/request. 4218 */ 4219 public NutritionOrder setStatus(RequestStatus value) { 4220 if (this.status == null) 4221 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 4222 this.status.setValue(value); 4223 return this; 4224 } 4225 4226 /** 4227 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4228 */ 4229 public Enumeration<RequestIntent> getIntentElement() { 4230 if (this.intent == null) 4231 if (Configuration.errorOnAutoCreate()) 4232 throw new Error("Attempt to auto-create NutritionOrder.intent"); 4233 else if (Configuration.doAutoCreate()) 4234 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 4235 return this.intent; 4236 } 4237 4238 public boolean hasIntentElement() { 4239 return this.intent != null && !this.intent.isEmpty(); 4240 } 4241 4242 public boolean hasIntent() { 4243 return this.intent != null && !this.intent.isEmpty(); 4244 } 4245 4246 /** 4247 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4248 */ 4249 public NutritionOrder setIntentElement(Enumeration<RequestIntent> value) { 4250 this.intent = value; 4251 return this; 4252 } 4253 4254 /** 4255 * @return Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain. 4256 */ 4257 public RequestIntent getIntent() { 4258 return this.intent == null ? null : this.intent.getValue(); 4259 } 4260 4261 /** 4262 * @param value Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain. 4263 */ 4264 public NutritionOrder setIntent(RequestIntent value) { 4265 if (this.intent == null) 4266 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 4267 this.intent.setValue(value); 4268 return this; 4269 } 4270 4271 /** 4272 * @return {@link #priority} (Indicates how quickly the Nutrition Order should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4273 */ 4274 public Enumeration<RequestPriority> getPriorityElement() { 4275 if (this.priority == null) 4276 if (Configuration.errorOnAutoCreate()) 4277 throw new Error("Attempt to auto-create NutritionOrder.priority"); 4278 else if (Configuration.doAutoCreate()) 4279 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 4280 return this.priority; 4281 } 4282 4283 public boolean hasPriorityElement() { 4284 return this.priority != null && !this.priority.isEmpty(); 4285 } 4286 4287 public boolean hasPriority() { 4288 return this.priority != null && !this.priority.isEmpty(); 4289 } 4290 4291 /** 4292 * @param value {@link #priority} (Indicates how quickly the Nutrition Order should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4293 */ 4294 public NutritionOrder setPriorityElement(Enumeration<RequestPriority> value) { 4295 this.priority = value; 4296 return this; 4297 } 4298 4299 /** 4300 * @return Indicates how quickly the Nutrition Order should be addressed with respect to other requests. 4301 */ 4302 public RequestPriority getPriority() { 4303 return this.priority == null ? null : this.priority.getValue(); 4304 } 4305 4306 /** 4307 * @param value Indicates how quickly the Nutrition Order should be addressed with respect to other requests. 4308 */ 4309 public NutritionOrder setPriority(RequestPriority value) { 4310 if (value == null) 4311 this.priority = null; 4312 else { 4313 if (this.priority == null) 4314 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 4315 this.priority.setValue(value); 4316 } 4317 return this; 4318 } 4319 4320 /** 4321 * @return {@link #subject} (The person or set of individuals who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.) 4322 */ 4323 public Reference getSubject() { 4324 if (this.subject == null) 4325 if (Configuration.errorOnAutoCreate()) 4326 throw new Error("Attempt to auto-create NutritionOrder.subject"); 4327 else if (Configuration.doAutoCreate()) 4328 this.subject = new Reference(); // cc 4329 return this.subject; 4330 } 4331 4332 public boolean hasSubject() { 4333 return this.subject != null && !this.subject.isEmpty(); 4334 } 4335 4336 /** 4337 * @param value {@link #subject} (The person or set of individuals who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.) 4338 */ 4339 public NutritionOrder setSubject(Reference value) { 4340 this.subject = value; 4341 return this; 4342 } 4343 4344 /** 4345 * @return {@link #encounter} (An encounter that provides additional information about the healthcare context in which this request is made.) 4346 */ 4347 public Reference getEncounter() { 4348 if (this.encounter == null) 4349 if (Configuration.errorOnAutoCreate()) 4350 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 4351 else if (Configuration.doAutoCreate()) 4352 this.encounter = new Reference(); // cc 4353 return this.encounter; 4354 } 4355 4356 public boolean hasEncounter() { 4357 return this.encounter != null && !this.encounter.isEmpty(); 4358 } 4359 4360 /** 4361 * @param value {@link #encounter} (An encounter that provides additional information about the healthcare context in which this request is made.) 4362 */ 4363 public NutritionOrder setEncounter(Reference value) { 4364 this.encounter = value; 4365 return this; 4366 } 4367 4368 /** 4369 * @return {@link #supportingInformation} (Information to support fulfilling (i.e. dispensing or administering) of the nutrition, for example, patient height and weight).) 4370 */ 4371 public List<Reference> getSupportingInformation() { 4372 if (this.supportingInformation == null) 4373 this.supportingInformation = new ArrayList<Reference>(); 4374 return this.supportingInformation; 4375 } 4376 4377 /** 4378 * @return Returns a reference to <code>this</code> for easy method chaining 4379 */ 4380 public NutritionOrder setSupportingInformation(List<Reference> theSupportingInformation) { 4381 this.supportingInformation = theSupportingInformation; 4382 return this; 4383 } 4384 4385 public boolean hasSupportingInformation() { 4386 if (this.supportingInformation == null) 4387 return false; 4388 for (Reference item : this.supportingInformation) 4389 if (!item.isEmpty()) 4390 return true; 4391 return false; 4392 } 4393 4394 public Reference addSupportingInformation() { //3 4395 Reference t = new Reference(); 4396 if (this.supportingInformation == null) 4397 this.supportingInformation = new ArrayList<Reference>(); 4398 this.supportingInformation.add(t); 4399 return t; 4400 } 4401 4402 public NutritionOrder addSupportingInformation(Reference t) { //3 4403 if (t == null) 4404 return this; 4405 if (this.supportingInformation == null) 4406 this.supportingInformation = new ArrayList<Reference>(); 4407 this.supportingInformation.add(t); 4408 return this; 4409 } 4410 4411 /** 4412 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 4413 */ 4414 public Reference getSupportingInformationFirstRep() { 4415 if (getSupportingInformation().isEmpty()) { 4416 addSupportingInformation(); 4417 } 4418 return getSupportingInformation().get(0); 4419 } 4420 4421 /** 4422 * @return {@link #dateTime} (The date and time that this nutrition order was requested.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 4423 */ 4424 public DateTimeType getDateTimeElement() { 4425 if (this.dateTime == null) 4426 if (Configuration.errorOnAutoCreate()) 4427 throw new Error("Attempt to auto-create NutritionOrder.dateTime"); 4428 else if (Configuration.doAutoCreate()) 4429 this.dateTime = new DateTimeType(); // bb 4430 return this.dateTime; 4431 } 4432 4433 public boolean hasDateTimeElement() { 4434 return this.dateTime != null && !this.dateTime.isEmpty(); 4435 } 4436 4437 public boolean hasDateTime() { 4438 return this.dateTime != null && !this.dateTime.isEmpty(); 4439 } 4440 4441 /** 4442 * @param value {@link #dateTime} (The date and time that this nutrition order was requested.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 4443 */ 4444 public NutritionOrder setDateTimeElement(DateTimeType value) { 4445 this.dateTime = value; 4446 return this; 4447 } 4448 4449 /** 4450 * @return The date and time that this nutrition order was requested. 4451 */ 4452 public Date getDateTime() { 4453 return this.dateTime == null ? null : this.dateTime.getValue(); 4454 } 4455 4456 /** 4457 * @param value The date and time that this nutrition order was requested. 4458 */ 4459 public NutritionOrder setDateTime(Date value) { 4460 if (this.dateTime == null) 4461 this.dateTime = new DateTimeType(); 4462 this.dateTime.setValue(value); 4463 return this; 4464 } 4465 4466 /** 4467 * @return {@link #orderer} (The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.) 4468 */ 4469 public Reference getOrderer() { 4470 if (this.orderer == null) 4471 if (Configuration.errorOnAutoCreate()) 4472 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 4473 else if (Configuration.doAutoCreate()) 4474 this.orderer = new Reference(); // cc 4475 return this.orderer; 4476 } 4477 4478 public boolean hasOrderer() { 4479 return this.orderer != null && !this.orderer.isEmpty(); 4480 } 4481 4482 /** 4483 * @param value {@link #orderer} (The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.) 4484 */ 4485 public NutritionOrder setOrderer(Reference value) { 4486 this.orderer = value; 4487 return this; 4488 } 4489 4490 /** 4491 * @return {@link #performer} (The specified desired performer of the nutrition order.) 4492 */ 4493 public List<CodeableReference> getPerformer() { 4494 if (this.performer == null) 4495 this.performer = new ArrayList<CodeableReference>(); 4496 return this.performer; 4497 } 4498 4499 /** 4500 * @return Returns a reference to <code>this</code> for easy method chaining 4501 */ 4502 public NutritionOrder setPerformer(List<CodeableReference> thePerformer) { 4503 this.performer = thePerformer; 4504 return this; 4505 } 4506 4507 public boolean hasPerformer() { 4508 if (this.performer == null) 4509 return false; 4510 for (CodeableReference item : this.performer) 4511 if (!item.isEmpty()) 4512 return true; 4513 return false; 4514 } 4515 4516 public CodeableReference addPerformer() { //3 4517 CodeableReference t = new CodeableReference(); 4518 if (this.performer == null) 4519 this.performer = new ArrayList<CodeableReference>(); 4520 this.performer.add(t); 4521 return t; 4522 } 4523 4524 public NutritionOrder addPerformer(CodeableReference t) { //3 4525 if (t == null) 4526 return this; 4527 if (this.performer == null) 4528 this.performer = new ArrayList<CodeableReference>(); 4529 this.performer.add(t); 4530 return this; 4531 } 4532 4533 /** 4534 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 4535 */ 4536 public CodeableReference getPerformerFirstRep() { 4537 if (getPerformer().isEmpty()) { 4538 addPerformer(); 4539 } 4540 return getPerformer().get(0); 4541 } 4542 4543 /** 4544 * @return {@link #allergyIntolerance} (A link to a record of allergies or intolerances which should be included in the nutrition order.) 4545 */ 4546 public List<Reference> getAllergyIntolerance() { 4547 if (this.allergyIntolerance == null) 4548 this.allergyIntolerance = new ArrayList<Reference>(); 4549 return this.allergyIntolerance; 4550 } 4551 4552 /** 4553 * @return Returns a reference to <code>this</code> for easy method chaining 4554 */ 4555 public NutritionOrder setAllergyIntolerance(List<Reference> theAllergyIntolerance) { 4556 this.allergyIntolerance = theAllergyIntolerance; 4557 return this; 4558 } 4559 4560 public boolean hasAllergyIntolerance() { 4561 if (this.allergyIntolerance == null) 4562 return false; 4563 for (Reference item : this.allergyIntolerance) 4564 if (!item.isEmpty()) 4565 return true; 4566 return false; 4567 } 4568 4569 public Reference addAllergyIntolerance() { //3 4570 Reference t = new Reference(); 4571 if (this.allergyIntolerance == null) 4572 this.allergyIntolerance = new ArrayList<Reference>(); 4573 this.allergyIntolerance.add(t); 4574 return t; 4575 } 4576 4577 public NutritionOrder addAllergyIntolerance(Reference t) { //3 4578 if (t == null) 4579 return this; 4580 if (this.allergyIntolerance == null) 4581 this.allergyIntolerance = new ArrayList<Reference>(); 4582 this.allergyIntolerance.add(t); 4583 return this; 4584 } 4585 4586 /** 4587 * @return The first repetition of repeating field {@link #allergyIntolerance}, creating it if it does not already exist {3} 4588 */ 4589 public Reference getAllergyIntoleranceFirstRep() { 4590 if (getAllergyIntolerance().isEmpty()) { 4591 addAllergyIntolerance(); 4592 } 4593 return getAllergyIntolerance().get(0); 4594 } 4595 4596 /** 4597 * @return {@link #foodPreferenceModifier} (This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.) 4598 */ 4599 public List<CodeableConcept> getFoodPreferenceModifier() { 4600 if (this.foodPreferenceModifier == null) 4601 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 4602 return this.foodPreferenceModifier; 4603 } 4604 4605 /** 4606 * @return Returns a reference to <code>this</code> for easy method chaining 4607 */ 4608 public NutritionOrder setFoodPreferenceModifier(List<CodeableConcept> theFoodPreferenceModifier) { 4609 this.foodPreferenceModifier = theFoodPreferenceModifier; 4610 return this; 4611 } 4612 4613 public boolean hasFoodPreferenceModifier() { 4614 if (this.foodPreferenceModifier == null) 4615 return false; 4616 for (CodeableConcept item : this.foodPreferenceModifier) 4617 if (!item.isEmpty()) 4618 return true; 4619 return false; 4620 } 4621 4622 public CodeableConcept addFoodPreferenceModifier() { //3 4623 CodeableConcept t = new CodeableConcept(); 4624 if (this.foodPreferenceModifier == null) 4625 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 4626 this.foodPreferenceModifier.add(t); 4627 return t; 4628 } 4629 4630 public NutritionOrder addFoodPreferenceModifier(CodeableConcept t) { //3 4631 if (t == null) 4632 return this; 4633 if (this.foodPreferenceModifier == null) 4634 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 4635 this.foodPreferenceModifier.add(t); 4636 return this; 4637 } 4638 4639 /** 4640 * @return The first repetition of repeating field {@link #foodPreferenceModifier}, creating it if it does not already exist {3} 4641 */ 4642 public CodeableConcept getFoodPreferenceModifierFirstRep() { 4643 if (getFoodPreferenceModifier().isEmpty()) { 4644 addFoodPreferenceModifier(); 4645 } 4646 return getFoodPreferenceModifier().get(0); 4647 } 4648 4649 /** 4650 * @return {@link #excludeFoodModifier} (This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.) 4651 */ 4652 public List<CodeableConcept> getExcludeFoodModifier() { 4653 if (this.excludeFoodModifier == null) 4654 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 4655 return this.excludeFoodModifier; 4656 } 4657 4658 /** 4659 * @return Returns a reference to <code>this</code> for easy method chaining 4660 */ 4661 public NutritionOrder setExcludeFoodModifier(List<CodeableConcept> theExcludeFoodModifier) { 4662 this.excludeFoodModifier = theExcludeFoodModifier; 4663 return this; 4664 } 4665 4666 public boolean hasExcludeFoodModifier() { 4667 if (this.excludeFoodModifier == null) 4668 return false; 4669 for (CodeableConcept item : this.excludeFoodModifier) 4670 if (!item.isEmpty()) 4671 return true; 4672 return false; 4673 } 4674 4675 public CodeableConcept addExcludeFoodModifier() { //3 4676 CodeableConcept t = new CodeableConcept(); 4677 if (this.excludeFoodModifier == null) 4678 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 4679 this.excludeFoodModifier.add(t); 4680 return t; 4681 } 4682 4683 public NutritionOrder addExcludeFoodModifier(CodeableConcept t) { //3 4684 if (t == null) 4685 return this; 4686 if (this.excludeFoodModifier == null) 4687 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 4688 this.excludeFoodModifier.add(t); 4689 return this; 4690 } 4691 4692 /** 4693 * @return The first repetition of repeating field {@link #excludeFoodModifier}, creating it if it does not already exist {3} 4694 */ 4695 public CodeableConcept getExcludeFoodModifierFirstRep() { 4696 if (getExcludeFoodModifier().isEmpty()) { 4697 addExcludeFoodModifier(); 4698 } 4699 return getExcludeFoodModifier().get(0); 4700 } 4701 4702 /** 4703 * @return {@link #outsideFoodAllowed} (This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item.). This is the underlying object with id, value and extensions. The accessor "getOutsideFoodAllowed" gives direct access to the value 4704 */ 4705 public BooleanType getOutsideFoodAllowedElement() { 4706 if (this.outsideFoodAllowed == null) 4707 if (Configuration.errorOnAutoCreate()) 4708 throw new Error("Attempt to auto-create NutritionOrder.outsideFoodAllowed"); 4709 else if (Configuration.doAutoCreate()) 4710 this.outsideFoodAllowed = new BooleanType(); // bb 4711 return this.outsideFoodAllowed; 4712 } 4713 4714 public boolean hasOutsideFoodAllowedElement() { 4715 return this.outsideFoodAllowed != null && !this.outsideFoodAllowed.isEmpty(); 4716 } 4717 4718 public boolean hasOutsideFoodAllowed() { 4719 return this.outsideFoodAllowed != null && !this.outsideFoodAllowed.isEmpty(); 4720 } 4721 4722 /** 4723 * @param value {@link #outsideFoodAllowed} (This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item.). This is the underlying object with id, value and extensions. The accessor "getOutsideFoodAllowed" gives direct access to the value 4724 */ 4725 public NutritionOrder setOutsideFoodAllowedElement(BooleanType value) { 4726 this.outsideFoodAllowed = value; 4727 return this; 4728 } 4729 4730 /** 4731 * @return This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item. 4732 */ 4733 public boolean getOutsideFoodAllowed() { 4734 return this.outsideFoodAllowed == null || this.outsideFoodAllowed.isEmpty() ? false : this.outsideFoodAllowed.getValue(); 4735 } 4736 4737 /** 4738 * @param value This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item. 4739 */ 4740 public NutritionOrder setOutsideFoodAllowed(boolean value) { 4741 if (this.outsideFoodAllowed == null) 4742 this.outsideFoodAllowed = new BooleanType(); 4743 this.outsideFoodAllowed.setValue(value); 4744 return this; 4745 } 4746 4747 /** 4748 * @return {@link #oralDiet} (Diet given orally in contrast to enteral (tube) feeding.) 4749 */ 4750 public NutritionOrderOralDietComponent getOralDiet() { 4751 if (this.oralDiet == null) 4752 if (Configuration.errorOnAutoCreate()) 4753 throw new Error("Attempt to auto-create NutritionOrder.oralDiet"); 4754 else if (Configuration.doAutoCreate()) 4755 this.oralDiet = new NutritionOrderOralDietComponent(); // cc 4756 return this.oralDiet; 4757 } 4758 4759 public boolean hasOralDiet() { 4760 return this.oralDiet != null && !this.oralDiet.isEmpty(); 4761 } 4762 4763 /** 4764 * @param value {@link #oralDiet} (Diet given orally in contrast to enteral (tube) feeding.) 4765 */ 4766 public NutritionOrder setOralDiet(NutritionOrderOralDietComponent value) { 4767 this.oralDiet = value; 4768 return this; 4769 } 4770 4771 /** 4772 * @return {@link #supplement} (Oral nutritional products given in order to add further nutritional value to the patient's diet.) 4773 */ 4774 public List<NutritionOrderSupplementComponent> getSupplement() { 4775 if (this.supplement == null) 4776 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 4777 return this.supplement; 4778 } 4779 4780 /** 4781 * @return Returns a reference to <code>this</code> for easy method chaining 4782 */ 4783 public NutritionOrder setSupplement(List<NutritionOrderSupplementComponent> theSupplement) { 4784 this.supplement = theSupplement; 4785 return this; 4786 } 4787 4788 public boolean hasSupplement() { 4789 if (this.supplement == null) 4790 return false; 4791 for (NutritionOrderSupplementComponent item : this.supplement) 4792 if (!item.isEmpty()) 4793 return true; 4794 return false; 4795 } 4796 4797 public NutritionOrderSupplementComponent addSupplement() { //3 4798 NutritionOrderSupplementComponent t = new NutritionOrderSupplementComponent(); 4799 if (this.supplement == null) 4800 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 4801 this.supplement.add(t); 4802 return t; 4803 } 4804 4805 public NutritionOrder addSupplement(NutritionOrderSupplementComponent t) { //3 4806 if (t == null) 4807 return this; 4808 if (this.supplement == null) 4809 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 4810 this.supplement.add(t); 4811 return this; 4812 } 4813 4814 /** 4815 * @return The first repetition of repeating field {@link #supplement}, creating it if it does not already exist {3} 4816 */ 4817 public NutritionOrderSupplementComponent getSupplementFirstRep() { 4818 if (getSupplement().isEmpty()) { 4819 addSupplement(); 4820 } 4821 return getSupplement().get(0); 4822 } 4823 4824 /** 4825 * @return {@link #enteralFormula} (Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.) 4826 */ 4827 public NutritionOrderEnteralFormulaComponent getEnteralFormula() { 4828 if (this.enteralFormula == null) 4829 if (Configuration.errorOnAutoCreate()) 4830 throw new Error("Attempt to auto-create NutritionOrder.enteralFormula"); 4831 else if (Configuration.doAutoCreate()) 4832 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); // cc 4833 return this.enteralFormula; 4834 } 4835 4836 public boolean hasEnteralFormula() { 4837 return this.enteralFormula != null && !this.enteralFormula.isEmpty(); 4838 } 4839 4840 /** 4841 * @param value {@link #enteralFormula} (Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.) 4842 */ 4843 public NutritionOrder setEnteralFormula(NutritionOrderEnteralFormulaComponent value) { 4844 this.enteralFormula = value; 4845 return this; 4846 } 4847 4848 /** 4849 * @return {@link #note} (Comments made about the nutrition order by the requester, performer, subject or other participants.) 4850 */ 4851 public List<Annotation> getNote() { 4852 if (this.note == null) 4853 this.note = new ArrayList<Annotation>(); 4854 return this.note; 4855 } 4856 4857 /** 4858 * @return Returns a reference to <code>this</code> for easy method chaining 4859 */ 4860 public NutritionOrder setNote(List<Annotation> theNote) { 4861 this.note = theNote; 4862 return this; 4863 } 4864 4865 public boolean hasNote() { 4866 if (this.note == null) 4867 return false; 4868 for (Annotation item : this.note) 4869 if (!item.isEmpty()) 4870 return true; 4871 return false; 4872 } 4873 4874 public Annotation addNote() { //3 4875 Annotation t = new Annotation(); 4876 if (this.note == null) 4877 this.note = new ArrayList<Annotation>(); 4878 this.note.add(t); 4879 return t; 4880 } 4881 4882 public NutritionOrder addNote(Annotation t) { //3 4883 if (t == null) 4884 return this; 4885 if (this.note == null) 4886 this.note = new ArrayList<Annotation>(); 4887 this.note.add(t); 4888 return this; 4889 } 4890 4891 /** 4892 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 4893 */ 4894 public Annotation getNoteFirstRep() { 4895 if (getNote().isEmpty()) { 4896 addNote(); 4897 } 4898 return getNote().get(0); 4899 } 4900 4901 protected void listChildren(List<Property> children) { 4902 super.listChildren(children); 4903 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order by the order sender or by the order receiver.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4904 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 4905 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 4906 children.add(new Property("instantiates", "uri", "The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 0, java.lang.Integer.MAX_VALUE, instantiates)); 4907 children.add(new Property("basedOn", "Reference(CarePlan|NutritionOrder|ServiceRequest)", "A plan or request that is fulfilled in whole or in part by this nutrition order.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 4908 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to all nutrition orders that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, groupIdentifier)); 4909 children.add(new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 1, status)); 4910 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain.", 0, 1, intent)); 4911 children.add(new Property("priority", "code", "Indicates how quickly the Nutrition Order should be addressed with respect to other requests.", 0, 1, priority)); 4912 children.add(new Property("subject", "Reference(Patient|Group)", "The person or set of individuals who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 0, 1, subject)); 4913 children.add(new Property("encounter", "Reference(Encounter)", "An encounter that provides additional information about the healthcare context in which this request is made.", 0, 1, encounter)); 4914 children.add(new Property("supportingInformation", "Reference(Any)", "Information to support fulfilling (i.e. dispensing or administering) of the nutrition, for example, patient height and weight).", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 4915 children.add(new Property("dateTime", "dateTime", "The date and time that this nutrition order was requested.", 0, 1, dateTime)); 4916 children.add(new Property("orderer", "Reference(Practitioner|PractitionerRole)", "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 0, 1, orderer)); 4917 children.add(new Property("performer", "CodeableReference(CareTeam|Practitioner|PractitionerRole|RelatedPerson|Patient|Organization)", "The specified desired performer of the nutrition order.", 0, java.lang.Integer.MAX_VALUE, performer)); 4918 children.add(new Property("allergyIntolerance", "Reference(AllergyIntolerance)", "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, java.lang.Integer.MAX_VALUE, allergyIntolerance)); 4919 children.add(new Property("foodPreferenceModifier", "CodeableConcept", "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier)); 4920 children.add(new Property("excludeFoodModifier", "CodeableConcept", "This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier)); 4921 children.add(new Property("outsideFoodAllowed", "boolean", "This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item.", 0, 1, outsideFoodAllowed)); 4922 children.add(new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 1, oralDiet)); 4923 children.add(new Property("supplement", "", "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, java.lang.Integer.MAX_VALUE, supplement)); 4924 children.add(new Property("enteralFormula", "", "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 0, 1, enteralFormula)); 4925 children.add(new Property("note", "Annotation", "Comments made about the nutrition order by the requester, performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 4926 } 4927 4928 @Override 4929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4930 switch (_hash) { 4931 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order by the order sender or by the order receiver.", 0, java.lang.Integer.MAX_VALUE, identifier); 4932 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 4933 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 4934 case -246883639: /*instantiates*/ return new Property("instantiates", "uri", "The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 0, java.lang.Integer.MAX_VALUE, instantiates); 4935 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|NutritionOrder|ServiceRequest)", "A plan or request that is fulfilled in whole or in part by this nutrition order.", 0, java.lang.Integer.MAX_VALUE, basedOn); 4936 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to all nutrition orders that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, groupIdentifier); 4937 case -892481550: /*status*/ return new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 1, status); 4938 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain.", 0, 1, intent); 4939 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the Nutrition Order should be addressed with respect to other requests.", 0, 1, priority); 4940 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person or set of individuals who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 0, 1, subject); 4941 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "An encounter that provides additional information about the healthcare context in which this request is made.", 0, 1, encounter); 4942 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Information to support fulfilling (i.e. dispensing or administering) of the nutrition, for example, patient height and weight).", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 4943 case 1792749467: /*dateTime*/ return new Property("dateTime", "dateTime", "The date and time that this nutrition order was requested.", 0, 1, dateTime); 4944 case -1207109509: /*orderer*/ return new Property("orderer", "Reference(Practitioner|PractitionerRole)", "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 0, 1, orderer); 4945 case 481140686: /*performer*/ return new Property("performer", "CodeableReference(CareTeam|Practitioner|PractitionerRole|RelatedPerson|Patient|Organization)", "The specified desired performer of the nutrition order.", 0, java.lang.Integer.MAX_VALUE, performer); 4946 case -120164120: /*allergyIntolerance*/ return new Property("allergyIntolerance", "Reference(AllergyIntolerance)", "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, java.lang.Integer.MAX_VALUE, allergyIntolerance); 4947 case 659473872: /*foodPreferenceModifier*/ return new Property("foodPreferenceModifier", "CodeableConcept", "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier); 4948 case 1760260175: /*excludeFoodModifier*/ return new Property("excludeFoodModifier", "CodeableConcept", "This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier); 4949 case 833777797: /*outsideFoodAllowed*/ return new Property("outsideFoodAllowed", "boolean", "This modifier is used to convey whether a food item is allowed to be brought in by the patient and/or family. If set to true, indicates that the receiving system does not need to supply the food item.", 0, 1, outsideFoodAllowed); 4950 case 1153521250: /*oralDiet*/ return new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 1, oralDiet); 4951 case -711993159: /*supplement*/ return new Property("supplement", "", "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, java.lang.Integer.MAX_VALUE, supplement); 4952 case -671083805: /*enteralFormula*/ return new Property("enteralFormula", "", "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 0, 1, enteralFormula); 4953 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the nutrition order by the requester, performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 4954 default: return super.getNamedProperty(_hash, _name, _checkValid); 4955 } 4956 4957 } 4958 4959 @Override 4960 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4961 switch (hash) { 4962 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4963 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 4964 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 4965 case -246883639: /*instantiates*/ return this.instantiates == null ? new Base[0] : this.instantiates.toArray(new Base[this.instantiates.size()]); // UriType 4966 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4967 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 4968 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 4969 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 4970 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 4971 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 4972 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 4973 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 4974 case 1792749467: /*dateTime*/ return this.dateTime == null ? new Base[0] : new Base[] {this.dateTime}; // DateTimeType 4975 case -1207109509: /*orderer*/ return this.orderer == null ? new Base[0] : new Base[] {this.orderer}; // Reference 4976 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // CodeableReference 4977 case -120164120: /*allergyIntolerance*/ return this.allergyIntolerance == null ? new Base[0] : this.allergyIntolerance.toArray(new Base[this.allergyIntolerance.size()]); // Reference 4978 case 659473872: /*foodPreferenceModifier*/ return this.foodPreferenceModifier == null ? new Base[0] : this.foodPreferenceModifier.toArray(new Base[this.foodPreferenceModifier.size()]); // CodeableConcept 4979 case 1760260175: /*excludeFoodModifier*/ return this.excludeFoodModifier == null ? new Base[0] : this.excludeFoodModifier.toArray(new Base[this.excludeFoodModifier.size()]); // CodeableConcept 4980 case 833777797: /*outsideFoodAllowed*/ return this.outsideFoodAllowed == null ? new Base[0] : new Base[] {this.outsideFoodAllowed}; // BooleanType 4981 case 1153521250: /*oralDiet*/ return this.oralDiet == null ? new Base[0] : new Base[] {this.oralDiet}; // NutritionOrderOralDietComponent 4982 case -711993159: /*supplement*/ return this.supplement == null ? new Base[0] : this.supplement.toArray(new Base[this.supplement.size()]); // NutritionOrderSupplementComponent 4983 case -671083805: /*enteralFormula*/ return this.enteralFormula == null ? new Base[0] : new Base[] {this.enteralFormula}; // NutritionOrderEnteralFormulaComponent 4984 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4985 default: return super.getProperty(hash, name, checkValid); 4986 } 4987 4988 } 4989 4990 @Override 4991 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4992 switch (hash) { 4993 case -1618432855: // identifier 4994 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4995 return value; 4996 case 8911915: // instantiatesCanonical 4997 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4998 return value; 4999 case -1926393373: // instantiatesUri 5000 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 5001 return value; 5002 case -246883639: // instantiates 5003 this.getInstantiates().add(TypeConvertor.castToUri(value)); // UriType 5004 return value; 5005 case -332612366: // basedOn 5006 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 5007 return value; 5008 case -445338488: // groupIdentifier 5009 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 5010 return value; 5011 case -892481550: // status 5012 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5013 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5014 return value; 5015 case -1183762788: // intent 5016 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5017 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5018 return value; 5019 case -1165461084: // priority 5020 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5021 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5022 return value; 5023 case -1867885268: // subject 5024 this.subject = TypeConvertor.castToReference(value); // Reference 5025 return value; 5026 case 1524132147: // encounter 5027 this.encounter = TypeConvertor.castToReference(value); // Reference 5028 return value; 5029 case -1248768647: // supportingInformation 5030 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 5031 return value; 5032 case 1792749467: // dateTime 5033 this.dateTime = TypeConvertor.castToDateTime(value); // DateTimeType 5034 return value; 5035 case -1207109509: // orderer 5036 this.orderer = TypeConvertor.castToReference(value); // Reference 5037 return value; 5038 case 481140686: // performer 5039 this.getPerformer().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5040 return value; 5041 case -120164120: // allergyIntolerance 5042 this.getAllergyIntolerance().add(TypeConvertor.castToReference(value)); // Reference 5043 return value; 5044 case 659473872: // foodPreferenceModifier 5045 this.getFoodPreferenceModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5046 return value; 5047 case 1760260175: // excludeFoodModifier 5048 this.getExcludeFoodModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5049 return value; 5050 case 833777797: // outsideFoodAllowed 5051 this.outsideFoodAllowed = TypeConvertor.castToBoolean(value); // BooleanType 5052 return value; 5053 case 1153521250: // oralDiet 5054 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 5055 return value; 5056 case -711993159: // supplement 5057 this.getSupplement().add((NutritionOrderSupplementComponent) value); // NutritionOrderSupplementComponent 5058 return value; 5059 case -671083805: // enteralFormula 5060 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 5061 return value; 5062 case 3387378: // note 5063 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 5064 return value; 5065 default: return super.setProperty(hash, name, value); 5066 } 5067 5068 } 5069 5070 @Override 5071 public Base setProperty(String name, Base value) throws FHIRException { 5072 if (name.equals("identifier")) { 5073 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5074 } else if (name.equals("instantiatesCanonical")) { 5075 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 5076 } else if (name.equals("instantiatesUri")) { 5077 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 5078 } else if (name.equals("instantiates")) { 5079 this.getInstantiates().add(TypeConvertor.castToUri(value)); 5080 } else if (name.equals("basedOn")) { 5081 this.getBasedOn().add(TypeConvertor.castToReference(value)); 5082 } else if (name.equals("groupIdentifier")) { 5083 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 5084 } else if (name.equals("status")) { 5085 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5086 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5087 } else if (name.equals("intent")) { 5088 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5089 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5090 } else if (name.equals("priority")) { 5091 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5092 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5093 } else if (name.equals("subject")) { 5094 this.subject = TypeConvertor.castToReference(value); // Reference 5095 } else if (name.equals("encounter")) { 5096 this.encounter = TypeConvertor.castToReference(value); // Reference 5097 } else if (name.equals("supportingInformation")) { 5098 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 5099 } else if (name.equals("dateTime")) { 5100 this.dateTime = TypeConvertor.castToDateTime(value); // DateTimeType 5101 } else if (name.equals("orderer")) { 5102 this.orderer = TypeConvertor.castToReference(value); // Reference 5103 } else if (name.equals("performer")) { 5104 this.getPerformer().add(TypeConvertor.castToCodeableReference(value)); 5105 } else if (name.equals("allergyIntolerance")) { 5106 this.getAllergyIntolerance().add(TypeConvertor.castToReference(value)); 5107 } else if (name.equals("foodPreferenceModifier")) { 5108 this.getFoodPreferenceModifier().add(TypeConvertor.castToCodeableConcept(value)); 5109 } else if (name.equals("excludeFoodModifier")) { 5110 this.getExcludeFoodModifier().add(TypeConvertor.castToCodeableConcept(value)); 5111 } else if (name.equals("outsideFoodAllowed")) { 5112 this.outsideFoodAllowed = TypeConvertor.castToBoolean(value); // BooleanType 5113 } else if (name.equals("oralDiet")) { 5114 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 5115 } else if (name.equals("supplement")) { 5116 this.getSupplement().add((NutritionOrderSupplementComponent) value); 5117 } else if (name.equals("enteralFormula")) { 5118 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 5119 } else if (name.equals("note")) { 5120 this.getNote().add(TypeConvertor.castToAnnotation(value)); 5121 } else 5122 return super.setProperty(name, value); 5123 return value; 5124 } 5125 5126 @Override 5127 public void removeChild(String name, Base value) throws FHIRException { 5128 if (name.equals("identifier")) { 5129 this.getIdentifier().remove(value); 5130 } else if (name.equals("instantiatesCanonical")) { 5131 this.getInstantiatesCanonical().remove(value); 5132 } else if (name.equals("instantiatesUri")) { 5133 this.getInstantiatesUri().remove(value); 5134 } else if (name.equals("instantiates")) { 5135 this.getInstantiates().remove(value); 5136 } else if (name.equals("basedOn")) { 5137 this.getBasedOn().remove(value); 5138 } else if (name.equals("groupIdentifier")) { 5139 this.groupIdentifier = null; 5140 } else if (name.equals("status")) { 5141 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5142 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5143 } else if (name.equals("intent")) { 5144 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5145 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5146 } else if (name.equals("priority")) { 5147 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5148 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5149 } else if (name.equals("subject")) { 5150 this.subject = null; 5151 } else if (name.equals("encounter")) { 5152 this.encounter = null; 5153 } else if (name.equals("supportingInformation")) { 5154 this.getSupportingInformation().remove(value); 5155 } else if (name.equals("dateTime")) { 5156 this.dateTime = null; 5157 } else if (name.equals("orderer")) { 5158 this.orderer = null; 5159 } else if (name.equals("performer")) { 5160 this.getPerformer().remove(value); 5161 } else if (name.equals("allergyIntolerance")) { 5162 this.getAllergyIntolerance().remove(value); 5163 } else if (name.equals("foodPreferenceModifier")) { 5164 this.getFoodPreferenceModifier().remove(value); 5165 } else if (name.equals("excludeFoodModifier")) { 5166 this.getExcludeFoodModifier().remove(value); 5167 } else if (name.equals("outsideFoodAllowed")) { 5168 this.outsideFoodAllowed = null; 5169 } else if (name.equals("oralDiet")) { 5170 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 5171 } else if (name.equals("supplement")) { 5172 this.getSupplement().remove((NutritionOrderSupplementComponent) value); 5173 } else if (name.equals("enteralFormula")) { 5174 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 5175 } else if (name.equals("note")) { 5176 this.getNote().remove(value); 5177 } else 5178 super.removeChild(name, value); 5179 5180 } 5181 5182 @Override 5183 public Base makeProperty(int hash, String name) throws FHIRException { 5184 switch (hash) { 5185 case -1618432855: return addIdentifier(); 5186 case 8911915: return addInstantiatesCanonicalElement(); 5187 case -1926393373: return addInstantiatesUriElement(); 5188 case -246883639: return addInstantiatesElement(); 5189 case -332612366: return addBasedOn(); 5190 case -445338488: return getGroupIdentifier(); 5191 case -892481550: return getStatusElement(); 5192 case -1183762788: return getIntentElement(); 5193 case -1165461084: return getPriorityElement(); 5194 case -1867885268: return getSubject(); 5195 case 1524132147: return getEncounter(); 5196 case -1248768647: return addSupportingInformation(); 5197 case 1792749467: return getDateTimeElement(); 5198 case -1207109509: return getOrderer(); 5199 case 481140686: return addPerformer(); 5200 case -120164120: return addAllergyIntolerance(); 5201 case 659473872: return addFoodPreferenceModifier(); 5202 case 1760260175: return addExcludeFoodModifier(); 5203 case 833777797: return getOutsideFoodAllowedElement(); 5204 case 1153521250: return getOralDiet(); 5205 case -711993159: return addSupplement(); 5206 case -671083805: return getEnteralFormula(); 5207 case 3387378: return addNote(); 5208 default: return super.makeProperty(hash, name); 5209 } 5210 5211 } 5212 5213 @Override 5214 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5215 switch (hash) { 5216 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5217 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 5218 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 5219 case -246883639: /*instantiates*/ return new String[] {"uri"}; 5220 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 5221 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 5222 case -892481550: /*status*/ return new String[] {"code"}; 5223 case -1183762788: /*intent*/ return new String[] {"code"}; 5224 case -1165461084: /*priority*/ return new String[] {"code"}; 5225 case -1867885268: /*subject*/ return new String[] {"Reference"}; 5226 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5227 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 5228 case 1792749467: /*dateTime*/ return new String[] {"dateTime"}; 5229 case -1207109509: /*orderer*/ return new String[] {"Reference"}; 5230 case 481140686: /*performer*/ return new String[] {"CodeableReference"}; 5231 case -120164120: /*allergyIntolerance*/ return new String[] {"Reference"}; 5232 case 659473872: /*foodPreferenceModifier*/ return new String[] {"CodeableConcept"}; 5233 case 1760260175: /*excludeFoodModifier*/ return new String[] {"CodeableConcept"}; 5234 case 833777797: /*outsideFoodAllowed*/ return new String[] {"boolean"}; 5235 case 1153521250: /*oralDiet*/ return new String[] {}; 5236 case -711993159: /*supplement*/ return new String[] {}; 5237 case -671083805: /*enteralFormula*/ return new String[] {}; 5238 case 3387378: /*note*/ return new String[] {"Annotation"}; 5239 default: return super.getTypesForProperty(hash, name); 5240 } 5241 5242 } 5243 5244 @Override 5245 public Base addChild(String name) throws FHIRException { 5246 if (name.equals("identifier")) { 5247 return addIdentifier(); 5248 } 5249 else if (name.equals("instantiatesCanonical")) { 5250 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instantiatesCanonical"); 5251 } 5252 else if (name.equals("instantiatesUri")) { 5253 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instantiatesUri"); 5254 } 5255 else if (name.equals("instantiates")) { 5256 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instantiates"); 5257 } 5258 else if (name.equals("basedOn")) { 5259 return addBasedOn(); 5260 } 5261 else if (name.equals("groupIdentifier")) { 5262 this.groupIdentifier = new Identifier(); 5263 return this.groupIdentifier; 5264 } 5265 else if (name.equals("status")) { 5266 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.status"); 5267 } 5268 else if (name.equals("intent")) { 5269 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.intent"); 5270 } 5271 else if (name.equals("priority")) { 5272 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.priority"); 5273 } 5274 else if (name.equals("subject")) { 5275 this.subject = new Reference(); 5276 return this.subject; 5277 } 5278 else if (name.equals("encounter")) { 5279 this.encounter = new Reference(); 5280 return this.encounter; 5281 } 5282 else if (name.equals("supportingInformation")) { 5283 return addSupportingInformation(); 5284 } 5285 else if (name.equals("dateTime")) { 5286 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.dateTime"); 5287 } 5288 else if (name.equals("orderer")) { 5289 this.orderer = new Reference(); 5290 return this.orderer; 5291 } 5292 else if (name.equals("performer")) { 5293 return addPerformer(); 5294 } 5295 else if (name.equals("allergyIntolerance")) { 5296 return addAllergyIntolerance(); 5297 } 5298 else if (name.equals("foodPreferenceModifier")) { 5299 return addFoodPreferenceModifier(); 5300 } 5301 else if (name.equals("excludeFoodModifier")) { 5302 return addExcludeFoodModifier(); 5303 } 5304 else if (name.equals("outsideFoodAllowed")) { 5305 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.outsideFoodAllowed"); 5306 } 5307 else if (name.equals("oralDiet")) { 5308 this.oralDiet = new NutritionOrderOralDietComponent(); 5309 return this.oralDiet; 5310 } 5311 else if (name.equals("supplement")) { 5312 return addSupplement(); 5313 } 5314 else if (name.equals("enteralFormula")) { 5315 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); 5316 return this.enteralFormula; 5317 } 5318 else if (name.equals("note")) { 5319 return addNote(); 5320 } 5321 else 5322 return super.addChild(name); 5323 } 5324 5325 public String fhirType() { 5326 return "NutritionOrder"; 5327 5328 } 5329 5330 public NutritionOrder copy() { 5331 NutritionOrder dst = new NutritionOrder(); 5332 copyValues(dst); 5333 return dst; 5334 } 5335 5336 public void copyValues(NutritionOrder dst) { 5337 super.copyValues(dst); 5338 if (identifier != null) { 5339 dst.identifier = new ArrayList<Identifier>(); 5340 for (Identifier i : identifier) 5341 dst.identifier.add(i.copy()); 5342 }; 5343 if (instantiatesCanonical != null) { 5344 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 5345 for (CanonicalType i : instantiatesCanonical) 5346 dst.instantiatesCanonical.add(i.copy()); 5347 }; 5348 if (instantiatesUri != null) { 5349 dst.instantiatesUri = new ArrayList<UriType>(); 5350 for (UriType i : instantiatesUri) 5351 dst.instantiatesUri.add(i.copy()); 5352 }; 5353 if (instantiates != null) { 5354 dst.instantiates = new ArrayList<UriType>(); 5355 for (UriType i : instantiates) 5356 dst.instantiates.add(i.copy()); 5357 }; 5358 if (basedOn != null) { 5359 dst.basedOn = new ArrayList<Reference>(); 5360 for (Reference i : basedOn) 5361 dst.basedOn.add(i.copy()); 5362 }; 5363 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 5364 dst.status = status == null ? null : status.copy(); 5365 dst.intent = intent == null ? null : intent.copy(); 5366 dst.priority = priority == null ? null : priority.copy(); 5367 dst.subject = subject == null ? null : subject.copy(); 5368 dst.encounter = encounter == null ? null : encounter.copy(); 5369 if (supportingInformation != null) { 5370 dst.supportingInformation = new ArrayList<Reference>(); 5371 for (Reference i : supportingInformation) 5372 dst.supportingInformation.add(i.copy()); 5373 }; 5374 dst.dateTime = dateTime == null ? null : dateTime.copy(); 5375 dst.orderer = orderer == null ? null : orderer.copy(); 5376 if (performer != null) { 5377 dst.performer = new ArrayList<CodeableReference>(); 5378 for (CodeableReference i : performer) 5379 dst.performer.add(i.copy()); 5380 }; 5381 if (allergyIntolerance != null) { 5382 dst.allergyIntolerance = new ArrayList<Reference>(); 5383 for (Reference i : allergyIntolerance) 5384 dst.allergyIntolerance.add(i.copy()); 5385 }; 5386 if (foodPreferenceModifier != null) { 5387 dst.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 5388 for (CodeableConcept i : foodPreferenceModifier) 5389 dst.foodPreferenceModifier.add(i.copy()); 5390 }; 5391 if (excludeFoodModifier != null) { 5392 dst.excludeFoodModifier = new ArrayList<CodeableConcept>(); 5393 for (CodeableConcept i : excludeFoodModifier) 5394 dst.excludeFoodModifier.add(i.copy()); 5395 }; 5396 dst.outsideFoodAllowed = outsideFoodAllowed == null ? null : outsideFoodAllowed.copy(); 5397 dst.oralDiet = oralDiet == null ? null : oralDiet.copy(); 5398 if (supplement != null) { 5399 dst.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 5400 for (NutritionOrderSupplementComponent i : supplement) 5401 dst.supplement.add(i.copy()); 5402 }; 5403 dst.enteralFormula = enteralFormula == null ? null : enteralFormula.copy(); 5404 if (note != null) { 5405 dst.note = new ArrayList<Annotation>(); 5406 for (Annotation i : note) 5407 dst.note.add(i.copy()); 5408 }; 5409 } 5410 5411 protected NutritionOrder typedCopy() { 5412 return copy(); 5413 } 5414 5415 @Override 5416 public boolean equalsDeep(Base other_) { 5417 if (!super.equalsDeep(other_)) 5418 return false; 5419 if (!(other_ instanceof NutritionOrder)) 5420 return false; 5421 NutritionOrder o = (NutritionOrder) other_; 5422 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 5423 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(instantiates, o.instantiates, true) 5424 && compareDeep(basedOn, o.basedOn, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 5425 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 5426 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) && compareDeep(supportingInformation, o.supportingInformation, true) 5427 && compareDeep(dateTime, o.dateTime, true) && compareDeep(orderer, o.orderer, true) && compareDeep(performer, o.performer, true) 5428 && compareDeep(allergyIntolerance, o.allergyIntolerance, true) && compareDeep(foodPreferenceModifier, o.foodPreferenceModifier, true) 5429 && compareDeep(excludeFoodModifier, o.excludeFoodModifier, true) && compareDeep(outsideFoodAllowed, o.outsideFoodAllowed, true) 5430 && compareDeep(oralDiet, o.oralDiet, true) && compareDeep(supplement, o.supplement, true) && compareDeep(enteralFormula, o.enteralFormula, true) 5431 && compareDeep(note, o.note, true); 5432 } 5433 5434 @Override 5435 public boolean equalsShallow(Base other_) { 5436 if (!super.equalsShallow(other_)) 5437 return false; 5438 if (!(other_ instanceof NutritionOrder)) 5439 return false; 5440 NutritionOrder o = (NutritionOrder) other_; 5441 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 5442 && compareValues(instantiates, o.instantiates, true) && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) 5443 && compareValues(priority, o.priority, true) && compareValues(dateTime, o.dateTime, true) && compareValues(outsideFoodAllowed, o.outsideFoodAllowed, true) 5444 ; 5445 } 5446 5447 public boolean isEmpty() { 5448 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 5449 , instantiatesUri, instantiates, basedOn, groupIdentifier, status, intent, priority 5450 , subject, encounter, supportingInformation, dateTime, orderer, performer, allergyIntolerance 5451 , foodPreferenceModifier, excludeFoodModifier, outsideFoodAllowed, oralDiet, supplement 5452 , enteralFormula, note); 5453 } 5454 5455 @Override 5456 public ResourceType getResourceType() { 5457 return ResourceType.NutritionOrder; 5458 } 5459 5460 /** 5461 * Search parameter: <b>additive</b> 5462 * <p> 5463 * Description: <b>Type of module component to add to the feeding</b><br> 5464 * Type: <b>token</b><br> 5465 * Path: <b>NutritionOrder.enteralFormula.additive.type.concept</b><br> 5466 * </p> 5467 */ 5468 @SearchParamDefinition(name="additive", path="NutritionOrder.enteralFormula.additive.type.concept", description="Type of module component to add to the feeding", type="token" ) 5469 public static final String SP_ADDITIVE = "additive"; 5470 /** 5471 * <b>Fluent Client</b> search parameter constant for <b>additive</b> 5472 * <p> 5473 * Description: <b>Type of module component to add to the feeding</b><br> 5474 * Type: <b>token</b><br> 5475 * Path: <b>NutritionOrder.enteralFormula.additive.type.concept</b><br> 5476 * </p> 5477 */ 5478 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDITIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDITIVE); 5479 5480 /** 5481 * Search parameter: <b>datetime</b> 5482 * <p> 5483 * Description: <b>Return nutrition orders requested on this date</b><br> 5484 * Type: <b>date</b><br> 5485 * Path: <b>NutritionOrder.dateTime</b><br> 5486 * </p> 5487 */ 5488 @SearchParamDefinition(name="datetime", path="NutritionOrder.dateTime", description="Return nutrition orders requested on this date", type="date" ) 5489 public static final String SP_DATETIME = "datetime"; 5490 /** 5491 * <b>Fluent Client</b> search parameter constant for <b>datetime</b> 5492 * <p> 5493 * Description: <b>Return nutrition orders requested on this date</b><br> 5494 * Type: <b>date</b><br> 5495 * Path: <b>NutritionOrder.dateTime</b><br> 5496 * </p> 5497 */ 5498 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATETIME = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATETIME); 5499 5500 /** 5501 * Search parameter: <b>formula</b> 5502 * <p> 5503 * Description: <b>Type of enteral or infant formula</b><br> 5504 * Type: <b>token</b><br> 5505 * Path: <b>NutritionOrder.enteralFormula.baseFormulaType.concept</b><br> 5506 * </p> 5507 */ 5508 @SearchParamDefinition(name="formula", path="NutritionOrder.enteralFormula.baseFormulaType.concept", description="Type of enteral or infant formula", type="token" ) 5509 public static final String SP_FORMULA = "formula"; 5510 /** 5511 * <b>Fluent Client</b> search parameter constant for <b>formula</b> 5512 * <p> 5513 * Description: <b>Type of enteral or infant formula</b><br> 5514 * Type: <b>token</b><br> 5515 * Path: <b>NutritionOrder.enteralFormula.baseFormulaType.concept</b><br> 5516 * </p> 5517 */ 5518 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMULA = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORMULA); 5519 5520 /** 5521 * Search parameter: <b>group-identifier</b> 5522 * <p> 5523 * Description: <b>Composite Request ID</b><br> 5524 * Type: <b>token</b><br> 5525 * Path: <b>NutritionOrder.groupIdentifier</b><br> 5526 * </p> 5527 */ 5528 @SearchParamDefinition(name="group-identifier", path="NutritionOrder.groupIdentifier", description="Composite Request ID", type="token" ) 5529 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 5530 /** 5531 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 5532 * <p> 5533 * Description: <b>Composite Request ID</b><br> 5534 * Type: <b>token</b><br> 5535 * Path: <b>NutritionOrder.groupIdentifier</b><br> 5536 * </p> 5537 */ 5538 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 5539 5540 /** 5541 * Search parameter: <b>oraldiet</b> 5542 * <p> 5543 * Description: <b>Type of diet that can be consumed orally (i.e., take via the mouth).</b><br> 5544 * Type: <b>token</b><br> 5545 * Path: <b>NutritionOrder.oralDiet.type</b><br> 5546 * </p> 5547 */ 5548 @SearchParamDefinition(name="oraldiet", path="NutritionOrder.oralDiet.type", description="Type of diet that can be consumed orally (i.e., take via the mouth).", type="token" ) 5549 public static final String SP_ORALDIET = "oraldiet"; 5550 /** 5551 * <b>Fluent Client</b> search parameter constant for <b>oraldiet</b> 5552 * <p> 5553 * Description: <b>Type of diet that can be consumed orally (i.e., take via the mouth).</b><br> 5554 * Type: <b>token</b><br> 5555 * Path: <b>NutritionOrder.oralDiet.type</b><br> 5556 * </p> 5557 */ 5558 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ORALDIET = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ORALDIET); 5559 5560 /** 5561 * Search parameter: <b>provider</b> 5562 * <p> 5563 * Description: <b>The identity of the provider who placed the nutrition order</b><br> 5564 * Type: <b>reference</b><br> 5565 * Path: <b>NutritionOrder.orderer</b><br> 5566 * </p> 5567 */ 5568 @SearchParamDefinition(name="provider", path="NutritionOrder.orderer", description="The identity of the provider who placed the nutrition order", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class, PractitionerRole.class } ) 5569 public static final String SP_PROVIDER = "provider"; 5570 /** 5571 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 5572 * <p> 5573 * Description: <b>The identity of the provider who placed the nutrition order</b><br> 5574 * Type: <b>reference</b><br> 5575 * Path: <b>NutritionOrder.orderer</b><br> 5576 * </p> 5577 */ 5578 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 5579 5580/** 5581 * Constant for fluent queries to be used to add include statements. Specifies 5582 * the path value of "<b>NutritionOrder:provider</b>". 5583 */ 5584 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("NutritionOrder:provider").toLocked(); 5585 5586 /** 5587 * Search parameter: <b>status</b> 5588 * <p> 5589 * Description: <b>Status of the nutrition order.</b><br> 5590 * Type: <b>token</b><br> 5591 * Path: <b>NutritionOrder.status</b><br> 5592 * </p> 5593 */ 5594 @SearchParamDefinition(name="status", path="NutritionOrder.status", description="Status of the nutrition order.", type="token" ) 5595 public static final String SP_STATUS = "status"; 5596 /** 5597 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5598 * <p> 5599 * Description: <b>Status of the nutrition order.</b><br> 5600 * Type: <b>token</b><br> 5601 * Path: <b>NutritionOrder.status</b><br> 5602 * </p> 5603 */ 5604 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5605 5606 /** 5607 * Search parameter: <b>subject</b> 5608 * <p> 5609 * Description: <b>The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement</b><br> 5610 * Type: <b>reference</b><br> 5611 * Path: <b>NutritionOrder.subject</b><br> 5612 * </p> 5613 */ 5614 @SearchParamDefinition(name="subject", path="NutritionOrder.subject", description="The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement", type="reference", target={Group.class, Patient.class } ) 5615 public static final String SP_SUBJECT = "subject"; 5616 /** 5617 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5618 * <p> 5619 * Description: <b>The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement</b><br> 5620 * Type: <b>reference</b><br> 5621 * Path: <b>NutritionOrder.subject</b><br> 5622 * </p> 5623 */ 5624 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 5625 5626/** 5627 * Constant for fluent queries to be used to add include statements. Specifies 5628 * the path value of "<b>NutritionOrder:subject</b>". 5629 */ 5630 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("NutritionOrder:subject").toLocked(); 5631 5632 /** 5633 * Search parameter: <b>supplement</b> 5634 * <p> 5635 * Description: <b>Type of supplement product requested</b><br> 5636 * Type: <b>token</b><br> 5637 * Path: <b>NutritionOrder.supplement.type.concept</b><br> 5638 * </p> 5639 */ 5640 @SearchParamDefinition(name="supplement", path="NutritionOrder.supplement.type.concept", description="Type of supplement product requested", type="token" ) 5641 public static final String SP_SUPPLEMENT = "supplement"; 5642 /** 5643 * <b>Fluent Client</b> search parameter constant for <b>supplement</b> 5644 * <p> 5645 * Description: <b>Type of supplement product requested</b><br> 5646 * Type: <b>token</b><br> 5647 * Path: <b>NutritionOrder.supplement.type.concept</b><br> 5648 * </p> 5649 */ 5650 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUPPLEMENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUPPLEMENT); 5651 5652 /** 5653 * Search parameter: <b>encounter</b> 5654 * <p> 5655 * Description: <b>Multiple Resources: 5656 5657* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 5658* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 5659* [ChargeItem](chargeitem.html): Encounter associated with event 5660* [Claim](claim.html): Encounters associated with a billed line item 5661* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 5662* [Communication](communication.html): The Encounter during which this Communication was created 5663* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 5664* [Composition](composition.html): Context of the Composition 5665* [Condition](condition.html): The Encounter during which this Condition was created 5666* [DeviceRequest](devicerequest.html): Encounter during which request was created 5667* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 5668* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 5669* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 5670* [Flag](flag.html): Alert relevant during encounter 5671* [ImagingStudy](imagingstudy.html): The context of the study 5672* [List](list.html): Context in which list created 5673* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 5674* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 5675* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 5676* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 5677* [Observation](observation.html): Encounter related to the observation 5678* [Procedure](procedure.html): The Encounter during which this Procedure was created 5679* [Provenance](provenance.html): Encounter related to the Provenance 5680* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 5681* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 5682* [RiskAssessment](riskassessment.html): Where was assessment performed? 5683* [ServiceRequest](servicerequest.html): An encounter in which this request is made 5684* [Task](task.html): Search by encounter 5685* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 5686</b><br> 5687 * Type: <b>reference</b><br> 5688 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 5689 * </p> 5690 */ 5691 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 5692 public static final String SP_ENCOUNTER = "encounter"; 5693 /** 5694 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5695 * <p> 5696 * Description: <b>Multiple Resources: 5697 5698* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 5699* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 5700* [ChargeItem](chargeitem.html): Encounter associated with event 5701* [Claim](claim.html): Encounters associated with a billed line item 5702* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 5703* [Communication](communication.html): The Encounter during which this Communication was created 5704* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 5705* [Composition](composition.html): Context of the Composition 5706* [Condition](condition.html): The Encounter during which this Condition was created 5707* [DeviceRequest](devicerequest.html): Encounter during which request was created 5708* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 5709* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 5710* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 5711* [Flag](flag.html): Alert relevant during encounter 5712* [ImagingStudy](imagingstudy.html): The context of the study 5713* [List](list.html): Context in which list created 5714* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 5715* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 5716* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 5717* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 5718* [Observation](observation.html): Encounter related to the observation 5719* [Procedure](procedure.html): The Encounter during which this Procedure was created 5720* [Provenance](provenance.html): Encounter related to the Provenance 5721* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 5722* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 5723* [RiskAssessment](riskassessment.html): Where was assessment performed? 5724* [ServiceRequest](servicerequest.html): An encounter in which this request is made 5725* [Task](task.html): Search by encounter 5726* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 5727</b><br> 5728 * Type: <b>reference</b><br> 5729 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 5730 * </p> 5731 */ 5732 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 5733 5734/** 5735 * Constant for fluent queries to be used to add include statements. Specifies 5736 * the path value of "<b>NutritionOrder:encounter</b>". 5737 */ 5738 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("NutritionOrder:encounter").toLocked(); 5739 5740 /** 5741 * Search parameter: <b>identifier</b> 5742 * <p> 5743 * Description: <b>Multiple Resources: 5744 5745* [Account](account.html): Account number 5746* [AdverseEvent](adverseevent.html): Business identifier for the event 5747* [AllergyIntolerance](allergyintolerance.html): External ids for this item 5748* [Appointment](appointment.html): An Identifier of the Appointment 5749* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 5750* [Basic](basic.html): Business identifier 5751* [BodyStructure](bodystructure.html): Bodystructure identifier 5752* [CarePlan](careplan.html): External Ids for this plan 5753* [CareTeam](careteam.html): External Ids for this team 5754* [ChargeItem](chargeitem.html): Business Identifier for item 5755* [Claim](claim.html): The primary identifier of the financial resource 5756* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 5757* [ClinicalImpression](clinicalimpression.html): Business identifier 5758* [Communication](communication.html): Unique identifier 5759* [CommunicationRequest](communicationrequest.html): Unique identifier 5760* [Composition](composition.html): Version-independent identifier for the Composition 5761* [Condition](condition.html): A unique identifier of the condition record 5762* [Consent](consent.html): Identifier for this record (external references) 5763* [Contract](contract.html): The identity of the contract 5764* [Coverage](coverage.html): The primary identifier of the insured and the coverage 5765* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 5766* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 5767* [DetectedIssue](detectedissue.html): Unique id for the detected issue 5768* [DeviceRequest](devicerequest.html): Business identifier for request/order 5769* [DeviceUsage](deviceusage.html): Search by identifier 5770* [DiagnosticReport](diagnosticreport.html): An identifier for the report 5771* [DocumentReference](documentreference.html): Identifier of the attachment binary 5772* [Encounter](encounter.html): Identifier(s) by which this encounter is known 5773* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 5774* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 5775* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 5776* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 5777* [Flag](flag.html): Business identifier 5778* [Goal](goal.html): External Ids for this goal 5779* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 5780* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 5781* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 5782* [Immunization](immunization.html): Business identifier 5783* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 5784* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 5785* [Invoice](invoice.html): Business Identifier for item 5786* [List](list.html): Business identifier 5787* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 5788* [Medication](medication.html): Returns medications with this external identifier 5789* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 5790* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 5791* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 5792* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 5793* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 5794* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 5795* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 5796* [Observation](observation.html): The unique id for a particular observation 5797* [Person](person.html): A person Identifier 5798* [Procedure](procedure.html): A unique identifier for a procedure 5799* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 5800* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 5801* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 5802* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 5803* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 5804* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 5805* [Specimen](specimen.html): The unique identifier associated with the specimen 5806* [SupplyDelivery](supplydelivery.html): External identifier 5807* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 5808* [Task](task.html): Search for a task instance by its business identifier 5809* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 5810</b><br> 5811 * Type: <b>token</b><br> 5812 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 5813 * </p> 5814 */ 5815 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 5816 public static final String SP_IDENTIFIER = "identifier"; 5817 /** 5818 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5819 * <p> 5820 * Description: <b>Multiple Resources: 5821 5822* [Account](account.html): Account number 5823* [AdverseEvent](adverseevent.html): Business identifier for the event 5824* [AllergyIntolerance](allergyintolerance.html): External ids for this item 5825* [Appointment](appointment.html): An Identifier of the Appointment 5826* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 5827* [Basic](basic.html): Business identifier 5828* [BodyStructure](bodystructure.html): Bodystructure identifier 5829* [CarePlan](careplan.html): External Ids for this plan 5830* [CareTeam](careteam.html): External Ids for this team 5831* [ChargeItem](chargeitem.html): Business Identifier for item 5832* [Claim](claim.html): The primary identifier of the financial resource 5833* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 5834* [ClinicalImpression](clinicalimpression.html): Business identifier 5835* [Communication](communication.html): Unique identifier 5836* [CommunicationRequest](communicationrequest.html): Unique identifier 5837* [Composition](composition.html): Version-independent identifier for the Composition 5838* [Condition](condition.html): A unique identifier of the condition record 5839* [Consent](consent.html): Identifier for this record (external references) 5840* [Contract](contract.html): The identity of the contract 5841* [Coverage](coverage.html): The primary identifier of the insured and the coverage 5842* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 5843* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 5844* [DetectedIssue](detectedissue.html): Unique id for the detected issue 5845* [DeviceRequest](devicerequest.html): Business identifier for request/order 5846* [DeviceUsage](deviceusage.html): Search by identifier 5847* [DiagnosticReport](diagnosticreport.html): An identifier for the report 5848* [DocumentReference](documentreference.html): Identifier of the attachment binary 5849* [Encounter](encounter.html): Identifier(s) by which this encounter is known 5850* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 5851* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 5852* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 5853* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 5854* [Flag](flag.html): Business identifier 5855* [Goal](goal.html): External Ids for this goal 5856* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 5857* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 5858* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 5859* [Immunization](immunization.html): Business identifier 5860* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 5861* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 5862* [Invoice](invoice.html): Business Identifier for item 5863* [List](list.html): Business identifier 5864* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 5865* [Medication](medication.html): Returns medications with this external identifier 5866* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 5867* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 5868* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 5869* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 5870* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 5871* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 5872* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 5873* [Observation](observation.html): The unique id for a particular observation 5874* [Person](person.html): A person Identifier 5875* [Procedure](procedure.html): A unique identifier for a procedure 5876* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 5877* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 5878* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 5879* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 5880* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 5881* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 5882* [Specimen](specimen.html): The unique identifier associated with the specimen 5883* [SupplyDelivery](supplydelivery.html): External identifier 5884* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 5885* [Task](task.html): Search for a task instance by its business identifier 5886* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 5887</b><br> 5888 * Type: <b>token</b><br> 5889 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 5890 * </p> 5891 */ 5892 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5893 5894 /** 5895 * Search parameter: <b>patient</b> 5896 * <p> 5897 * Description: <b>Multiple Resources: 5898 5899* [Account](account.html): The entity that caused the expenses 5900* [AdverseEvent](adverseevent.html): Subject impacted by event 5901* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 5902* [Appointment](appointment.html): One of the individuals of the appointment is this patient 5903* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 5904* [AuditEvent](auditevent.html): Where the activity involved patient data 5905* [Basic](basic.html): Identifies the focus of this resource 5906* [BodyStructure](bodystructure.html): Who this is about 5907* [CarePlan](careplan.html): Who the care plan is for 5908* [CareTeam](careteam.html): Who care team is for 5909* [ChargeItem](chargeitem.html): Individual service was done for/to 5910* [Claim](claim.html): Patient receiving the products or services 5911* [ClaimResponse](claimresponse.html): The subject of care 5912* [ClinicalImpression](clinicalimpression.html): Patient assessed 5913* [Communication](communication.html): Focus of message 5914* [CommunicationRequest](communicationrequest.html): Focus of message 5915* [Composition](composition.html): Who and/or what the composition is about 5916* [Condition](condition.html): Who has the condition? 5917* [Consent](consent.html): Who the consent applies to 5918* [Contract](contract.html): The identity of the subject of the contract (if a patient) 5919* [Coverage](coverage.html): Retrieve coverages for a patient 5920* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 5921* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 5922* [DetectedIssue](detectedissue.html): Associated patient 5923* [DeviceRequest](devicerequest.html): Individual the service is ordered for 5924* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 5925* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 5926* [DocumentReference](documentreference.html): Who/what is the subject of the document 5927* [Encounter](encounter.html): The patient present at the encounter 5928* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 5929* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 5930* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 5931* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 5932* [Flag](flag.html): The identity of a subject to list flags for 5933* [Goal](goal.html): Who this goal is intended for 5934* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 5935* [ImagingSelection](imagingselection.html): Who the study is about 5936* [ImagingStudy](imagingstudy.html): Who the study is about 5937* [Immunization](immunization.html): The patient for the vaccination record 5938* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 5939* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 5940* [Invoice](invoice.html): Recipient(s) of goods and services 5941* [List](list.html): If all resources have the same subject 5942* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 5943* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 5944* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 5945* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 5946* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 5947* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 5948* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 5949* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 5950* [Observation](observation.html): The subject that the observation is about (if patient) 5951* [Person](person.html): The Person links to this Patient 5952* [Procedure](procedure.html): Search by subject - a patient 5953* [Provenance](provenance.html): Where the activity involved patient data 5954* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 5955* [RelatedPerson](relatedperson.html): The patient this related person is related to 5956* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 5957* [ResearchSubject](researchsubject.html): Who or what is part of study 5958* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 5959* [ServiceRequest](servicerequest.html): Search by subject - a patient 5960* [Specimen](specimen.html): The patient the specimen comes from 5961* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 5962* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 5963* [Task](task.html): Search by patient 5964* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 5965</b><br> 5966 * Type: <b>reference</b><br> 5967 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 5968 * </p> 5969 */ 5970 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 5971 public static final String SP_PATIENT = "patient"; 5972 /** 5973 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5974 * <p> 5975 * Description: <b>Multiple Resources: 5976 5977* [Account](account.html): The entity that caused the expenses 5978* [AdverseEvent](adverseevent.html): Subject impacted by event 5979* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 5980* [Appointment](appointment.html): One of the individuals of the appointment is this patient 5981* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 5982* [AuditEvent](auditevent.html): Where the activity involved patient data 5983* [Basic](basic.html): Identifies the focus of this resource 5984* [BodyStructure](bodystructure.html): Who this is about 5985* [CarePlan](careplan.html): Who the care plan is for 5986* [CareTeam](careteam.html): Who care team is for 5987* [ChargeItem](chargeitem.html): Individual service was done for/to 5988* [Claim](claim.html): Patient receiving the products or services 5989* [ClaimResponse](claimresponse.html): The subject of care 5990* [ClinicalImpression](clinicalimpression.html): Patient assessed 5991* [Communication](communication.html): Focus of message 5992* [CommunicationRequest](communicationrequest.html): Focus of message 5993* [Composition](composition.html): Who and/or what the composition is about 5994* [Condition](condition.html): Who has the condition? 5995* [Consent](consent.html): Who the consent applies to 5996* [Contract](contract.html): The identity of the subject of the contract (if a patient) 5997* [Coverage](coverage.html): Retrieve coverages for a patient 5998* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 5999* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 6000* [DetectedIssue](detectedissue.html): Associated patient 6001* [DeviceRequest](devicerequest.html): Individual the service is ordered for 6002* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 6003* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 6004* [DocumentReference](documentreference.html): Who/what is the subject of the document 6005* [Encounter](encounter.html): The patient present at the encounter 6006* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 6007* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 6008* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 6009* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 6010* [Flag](flag.html): The identity of a subject to list flags for 6011* [Goal](goal.html): Who this goal is intended for 6012* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 6013* [ImagingSelection](imagingselection.html): Who the study is about 6014* [ImagingStudy](imagingstudy.html): Who the study is about 6015* [Immunization](immunization.html): The patient for the vaccination record 6016* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 6017* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 6018* [Invoice](invoice.html): Recipient(s) of goods and services 6019* [List](list.html): If all resources have the same subject 6020* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 6021* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 6022* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 6023* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 6024* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 6025* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 6026* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 6027* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 6028* [Observation](observation.html): The subject that the observation is about (if patient) 6029* [Person](person.html): The Person links to this Patient 6030* [Procedure](procedure.html): Search by subject - a patient 6031* [Provenance](provenance.html): Where the activity involved patient data 6032* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 6033* [RelatedPerson](relatedperson.html): The patient this related person is related to 6034* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 6035* [ResearchSubject](researchsubject.html): Who or what is part of study 6036* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 6037* [ServiceRequest](servicerequest.html): Search by subject - a patient 6038* [Specimen](specimen.html): The patient the specimen comes from 6039* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 6040* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 6041* [Task](task.html): Search by patient 6042* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 6043</b><br> 6044 * Type: <b>reference</b><br> 6045 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 6046 * </p> 6047 */ 6048 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 6049 6050/** 6051 * Constant for fluent queries to be used to add include statements. Specifies 6052 * the path value of "<b>NutritionOrder:patient</b>". 6053 */ 6054 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("NutritionOrder:patient").toLocked(); 6055 6056 6057} 6058