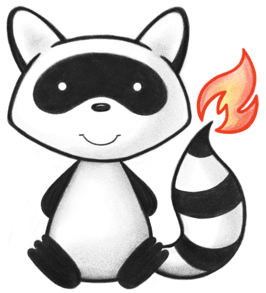
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A food or supplement that is consumed by patients. 052 */ 053@ResourceDef(name="NutritionProduct", profile="http://hl7.org/fhir/StructureDefinition/NutritionProduct") 054public class NutritionProduct extends DomainResource { 055 056 public enum NutritionProductStatus { 057 /** 058 * The product can be used. 059 */ 060 ACTIVE, 061 /** 062 * The product is not expected or allowed to be used. 063 */ 064 INACTIVE, 065 /** 066 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static NutritionProductStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown NutritionProductStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/nutritionproduct-status"; 099 case INACTIVE: return "http://hl7.org/fhir/nutritionproduct-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/nutritionproduct-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The product can be used."; 108 case INACTIVE: return "The product is not expected or allowed to be used."; 109 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class NutritionProductStatusEnumFactory implements EnumFactory<NutritionProductStatus> { 126 public NutritionProductStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return NutritionProductStatus.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return NutritionProductStatus.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return NutritionProductStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown NutritionProductStatus code '"+codeString+"'"); 137 } 138 public Enumeration<NutritionProductStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<NutritionProductStatus>(this, NutritionProductStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<NutritionProductStatus>(this, NutritionProductStatus.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<NutritionProductStatus>(this, NutritionProductStatus.ACTIVE, code); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<NutritionProductStatus>(this, NutritionProductStatus.INACTIVE, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<NutritionProductStatus>(this, NutritionProductStatus.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown NutritionProductStatus code '"+codeString+"'"); 153 } 154 public String toCode(NutritionProductStatus code) { 155 if (code == NutritionProductStatus.NULL) 156 return null; 157 if (code == NutritionProductStatus.ACTIVE) 158 return "active"; 159 if (code == NutritionProductStatus.INACTIVE) 160 return "inactive"; 161 if (code == NutritionProductStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(NutritionProductStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class NutritionProductNutrientComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The (relevant) nutrients in the product. 174 */ 175 @Child(name = "item", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="The (relevant) nutrients in the product", formalDefinition="The (relevant) nutrients in the product." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/nutrition-product-nutrient") 178 protected CodeableReference item; 179 180 /** 181 * The amount of nutrient expressed in one or more units: X per pack / per serving / per dose. 182 */ 183 @Child(name = "amount", type = {Ratio.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 184 @Description(shortDefinition="The amount of nutrient expressed in one or more units: X per pack / per serving / per dose", formalDefinition="The amount of nutrient expressed in one or more units: X per pack / per serving / per dose." ) 185 protected List<Ratio> amount; 186 187 private static final long serialVersionUID = -776277304L; 188 189 /** 190 * Constructor 191 */ 192 public NutritionProductNutrientComponent() { 193 super(); 194 } 195 196 /** 197 * @return {@link #item} (The (relevant) nutrients in the product.) 198 */ 199 public CodeableReference getItem() { 200 if (this.item == null) 201 if (Configuration.errorOnAutoCreate()) 202 throw new Error("Attempt to auto-create NutritionProductNutrientComponent.item"); 203 else if (Configuration.doAutoCreate()) 204 this.item = new CodeableReference(); // cc 205 return this.item; 206 } 207 208 public boolean hasItem() { 209 return this.item != null && !this.item.isEmpty(); 210 } 211 212 /** 213 * @param value {@link #item} (The (relevant) nutrients in the product.) 214 */ 215 public NutritionProductNutrientComponent setItem(CodeableReference value) { 216 this.item = value; 217 return this; 218 } 219 220 /** 221 * @return {@link #amount} (The amount of nutrient expressed in one or more units: X per pack / per serving / per dose.) 222 */ 223 public List<Ratio> getAmount() { 224 if (this.amount == null) 225 this.amount = new ArrayList<Ratio>(); 226 return this.amount; 227 } 228 229 /** 230 * @return Returns a reference to <code>this</code> for easy method chaining 231 */ 232 public NutritionProductNutrientComponent setAmount(List<Ratio> theAmount) { 233 this.amount = theAmount; 234 return this; 235 } 236 237 public boolean hasAmount() { 238 if (this.amount == null) 239 return false; 240 for (Ratio item : this.amount) 241 if (!item.isEmpty()) 242 return true; 243 return false; 244 } 245 246 public Ratio addAmount() { //3 247 Ratio t = new Ratio(); 248 if (this.amount == null) 249 this.amount = new ArrayList<Ratio>(); 250 this.amount.add(t); 251 return t; 252 } 253 254 public NutritionProductNutrientComponent addAmount(Ratio t) { //3 255 if (t == null) 256 return this; 257 if (this.amount == null) 258 this.amount = new ArrayList<Ratio>(); 259 this.amount.add(t); 260 return this; 261 } 262 263 /** 264 * @return The first repetition of repeating field {@link #amount}, creating it if it does not already exist {3} 265 */ 266 public Ratio getAmountFirstRep() { 267 if (getAmount().isEmpty()) { 268 addAmount(); 269 } 270 return getAmount().get(0); 271 } 272 273 protected void listChildren(List<Property> children) { 274 super.listChildren(children); 275 children.add(new Property("item", "CodeableReference(Substance)", "The (relevant) nutrients in the product.", 0, 1, item)); 276 children.add(new Property("amount", "Ratio", "The amount of nutrient expressed in one or more units: X per pack / per serving / per dose.", 0, java.lang.Integer.MAX_VALUE, amount)); 277 } 278 279 @Override 280 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 281 switch (_hash) { 282 case 3242771: /*item*/ return new Property("item", "CodeableReference(Substance)", "The (relevant) nutrients in the product.", 0, 1, item); 283 case -1413853096: /*amount*/ return new Property("amount", "Ratio", "The amount of nutrient expressed in one or more units: X per pack / per serving / per dose.", 0, java.lang.Integer.MAX_VALUE, amount); 284 default: return super.getNamedProperty(_hash, _name, _checkValid); 285 } 286 287 } 288 289 @Override 290 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 291 switch (hash) { 292 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 293 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : this.amount.toArray(new Base[this.amount.size()]); // Ratio 294 default: return super.getProperty(hash, name, checkValid); 295 } 296 297 } 298 299 @Override 300 public Base setProperty(int hash, String name, Base value) throws FHIRException { 301 switch (hash) { 302 case 3242771: // item 303 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 304 return value; 305 case -1413853096: // amount 306 this.getAmount().add(TypeConvertor.castToRatio(value)); // Ratio 307 return value; 308 default: return super.setProperty(hash, name, value); 309 } 310 311 } 312 313 @Override 314 public Base setProperty(String name, Base value) throws FHIRException { 315 if (name.equals("item")) { 316 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 317 } else if (name.equals("amount")) { 318 this.getAmount().add(TypeConvertor.castToRatio(value)); 319 } else 320 return super.setProperty(name, value); 321 return value; 322 } 323 324 @Override 325 public void removeChild(String name, Base value) throws FHIRException { 326 if (name.equals("item")) { 327 this.item = null; 328 } else if (name.equals("amount")) { 329 this.getAmount().remove(value); 330 } else 331 super.removeChild(name, value); 332 333 } 334 335 @Override 336 public Base makeProperty(int hash, String name) throws FHIRException { 337 switch (hash) { 338 case 3242771: return getItem(); 339 case -1413853096: return addAmount(); 340 default: return super.makeProperty(hash, name); 341 } 342 343 } 344 345 @Override 346 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 347 switch (hash) { 348 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 349 case -1413853096: /*amount*/ return new String[] {"Ratio"}; 350 default: return super.getTypesForProperty(hash, name); 351 } 352 353 } 354 355 @Override 356 public Base addChild(String name) throws FHIRException { 357 if (name.equals("item")) { 358 this.item = new CodeableReference(); 359 return this.item; 360 } 361 else if (name.equals("amount")) { 362 return addAmount(); 363 } 364 else 365 return super.addChild(name); 366 } 367 368 public NutritionProductNutrientComponent copy() { 369 NutritionProductNutrientComponent dst = new NutritionProductNutrientComponent(); 370 copyValues(dst); 371 return dst; 372 } 373 374 public void copyValues(NutritionProductNutrientComponent dst) { 375 super.copyValues(dst); 376 dst.item = item == null ? null : item.copy(); 377 if (amount != null) { 378 dst.amount = new ArrayList<Ratio>(); 379 for (Ratio i : amount) 380 dst.amount.add(i.copy()); 381 }; 382 } 383 384 @Override 385 public boolean equalsDeep(Base other_) { 386 if (!super.equalsDeep(other_)) 387 return false; 388 if (!(other_ instanceof NutritionProductNutrientComponent)) 389 return false; 390 NutritionProductNutrientComponent o = (NutritionProductNutrientComponent) other_; 391 return compareDeep(item, o.item, true) && compareDeep(amount, o.amount, true); 392 } 393 394 @Override 395 public boolean equalsShallow(Base other_) { 396 if (!super.equalsShallow(other_)) 397 return false; 398 if (!(other_ instanceof NutritionProductNutrientComponent)) 399 return false; 400 NutritionProductNutrientComponent o = (NutritionProductNutrientComponent) other_; 401 return true; 402 } 403 404 public boolean isEmpty() { 405 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, amount); 406 } 407 408 public String fhirType() { 409 return "NutritionProduct.nutrient"; 410 411 } 412 413 } 414 415 @Block() 416 public static class NutritionProductIngredientComponent extends BackboneElement implements IBaseBackboneElement { 417 /** 418 * The ingredient contained in the product. 419 */ 420 @Child(name = "item", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=true) 421 @Description(shortDefinition="The ingredient contained in the product", formalDefinition="The ingredient contained in the product." ) 422 protected CodeableReference item; 423 424 /** 425 * The amount of ingredient that is in the product. 426 */ 427 @Child(name = "amount", type = {Ratio.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 428 @Description(shortDefinition="The amount of ingredient that is in the product", formalDefinition="The amount of ingredient that is in the product." ) 429 protected List<Ratio> amount; 430 431 private static final long serialVersionUID = -776277304L; 432 433 /** 434 * Constructor 435 */ 436 public NutritionProductIngredientComponent() { 437 super(); 438 } 439 440 /** 441 * Constructor 442 */ 443 public NutritionProductIngredientComponent(CodeableReference item) { 444 super(); 445 this.setItem(item); 446 } 447 448 /** 449 * @return {@link #item} (The ingredient contained in the product.) 450 */ 451 public CodeableReference getItem() { 452 if (this.item == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create NutritionProductIngredientComponent.item"); 455 else if (Configuration.doAutoCreate()) 456 this.item = new CodeableReference(); // cc 457 return this.item; 458 } 459 460 public boolean hasItem() { 461 return this.item != null && !this.item.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #item} (The ingredient contained in the product.) 466 */ 467 public NutritionProductIngredientComponent setItem(CodeableReference value) { 468 this.item = value; 469 return this; 470 } 471 472 /** 473 * @return {@link #amount} (The amount of ingredient that is in the product.) 474 */ 475 public List<Ratio> getAmount() { 476 if (this.amount == null) 477 this.amount = new ArrayList<Ratio>(); 478 return this.amount; 479 } 480 481 /** 482 * @return Returns a reference to <code>this</code> for easy method chaining 483 */ 484 public NutritionProductIngredientComponent setAmount(List<Ratio> theAmount) { 485 this.amount = theAmount; 486 return this; 487 } 488 489 public boolean hasAmount() { 490 if (this.amount == null) 491 return false; 492 for (Ratio item : this.amount) 493 if (!item.isEmpty()) 494 return true; 495 return false; 496 } 497 498 public Ratio addAmount() { //3 499 Ratio t = new Ratio(); 500 if (this.amount == null) 501 this.amount = new ArrayList<Ratio>(); 502 this.amount.add(t); 503 return t; 504 } 505 506 public NutritionProductIngredientComponent addAmount(Ratio t) { //3 507 if (t == null) 508 return this; 509 if (this.amount == null) 510 this.amount = new ArrayList<Ratio>(); 511 this.amount.add(t); 512 return this; 513 } 514 515 /** 516 * @return The first repetition of repeating field {@link #amount}, creating it if it does not already exist {3} 517 */ 518 public Ratio getAmountFirstRep() { 519 if (getAmount().isEmpty()) { 520 addAmount(); 521 } 522 return getAmount().get(0); 523 } 524 525 protected void listChildren(List<Property> children) { 526 super.listChildren(children); 527 children.add(new Property("item", "CodeableReference(NutritionProduct)", "The ingredient contained in the product.", 0, 1, item)); 528 children.add(new Property("amount", "Ratio", "The amount of ingredient that is in the product.", 0, java.lang.Integer.MAX_VALUE, amount)); 529 } 530 531 @Override 532 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 533 switch (_hash) { 534 case 3242771: /*item*/ return new Property("item", "CodeableReference(NutritionProduct)", "The ingredient contained in the product.", 0, 1, item); 535 case -1413853096: /*amount*/ return new Property("amount", "Ratio", "The amount of ingredient that is in the product.", 0, java.lang.Integer.MAX_VALUE, amount); 536 default: return super.getNamedProperty(_hash, _name, _checkValid); 537 } 538 539 } 540 541 @Override 542 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 543 switch (hash) { 544 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 545 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : this.amount.toArray(new Base[this.amount.size()]); // Ratio 546 default: return super.getProperty(hash, name, checkValid); 547 } 548 549 } 550 551 @Override 552 public Base setProperty(int hash, String name, Base value) throws FHIRException { 553 switch (hash) { 554 case 3242771: // item 555 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 556 return value; 557 case -1413853096: // amount 558 this.getAmount().add(TypeConvertor.castToRatio(value)); // Ratio 559 return value; 560 default: return super.setProperty(hash, name, value); 561 } 562 563 } 564 565 @Override 566 public Base setProperty(String name, Base value) throws FHIRException { 567 if (name.equals("item")) { 568 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 569 } else if (name.equals("amount")) { 570 this.getAmount().add(TypeConvertor.castToRatio(value)); 571 } else 572 return super.setProperty(name, value); 573 return value; 574 } 575 576 @Override 577 public void removeChild(String name, Base value) throws FHIRException { 578 if (name.equals("item")) { 579 this.item = null; 580 } else if (name.equals("amount")) { 581 this.getAmount().remove(value); 582 } else 583 super.removeChild(name, value); 584 585 } 586 587 @Override 588 public Base makeProperty(int hash, String name) throws FHIRException { 589 switch (hash) { 590 case 3242771: return getItem(); 591 case -1413853096: return addAmount(); 592 default: return super.makeProperty(hash, name); 593 } 594 595 } 596 597 @Override 598 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 599 switch (hash) { 600 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 601 case -1413853096: /*amount*/ return new String[] {"Ratio"}; 602 default: return super.getTypesForProperty(hash, name); 603 } 604 605 } 606 607 @Override 608 public Base addChild(String name) throws FHIRException { 609 if (name.equals("item")) { 610 this.item = new CodeableReference(); 611 return this.item; 612 } 613 else if (name.equals("amount")) { 614 return addAmount(); 615 } 616 else 617 return super.addChild(name); 618 } 619 620 public NutritionProductIngredientComponent copy() { 621 NutritionProductIngredientComponent dst = new NutritionProductIngredientComponent(); 622 copyValues(dst); 623 return dst; 624 } 625 626 public void copyValues(NutritionProductIngredientComponent dst) { 627 super.copyValues(dst); 628 dst.item = item == null ? null : item.copy(); 629 if (amount != null) { 630 dst.amount = new ArrayList<Ratio>(); 631 for (Ratio i : amount) 632 dst.amount.add(i.copy()); 633 }; 634 } 635 636 @Override 637 public boolean equalsDeep(Base other_) { 638 if (!super.equalsDeep(other_)) 639 return false; 640 if (!(other_ instanceof NutritionProductIngredientComponent)) 641 return false; 642 NutritionProductIngredientComponent o = (NutritionProductIngredientComponent) other_; 643 return compareDeep(item, o.item, true) && compareDeep(amount, o.amount, true); 644 } 645 646 @Override 647 public boolean equalsShallow(Base other_) { 648 if (!super.equalsShallow(other_)) 649 return false; 650 if (!(other_ instanceof NutritionProductIngredientComponent)) 651 return false; 652 NutritionProductIngredientComponent o = (NutritionProductIngredientComponent) other_; 653 return true; 654 } 655 656 public boolean isEmpty() { 657 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, amount); 658 } 659 660 public String fhirType() { 661 return "NutritionProduct.ingredient"; 662 663 } 664 665 } 666 667 @Block() 668 public static class NutritionProductCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 669 /** 670 * A code specifying which characteristic of the product is being described (for example, colour, shape). 671 */ 672 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 673 @Description(shortDefinition="Code specifying the type of characteristic", formalDefinition="A code specifying which characteristic of the product is being described (for example, colour, shape)." ) 674 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measurement-property") 675 protected CodeableConcept type; 676 677 /** 678 * The actual characteristic value corresponding to the type. 679 */ 680 @Child(name = "value", type = {CodeableConcept.class, StringType.class, Quantity.class, Base64BinaryType.class, Attachment.class, BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 681 @Description(shortDefinition="The value of the characteristic", formalDefinition="The actual characteristic value corresponding to the type." ) 682 protected DataType value; 683 684 private static final long serialVersionUID = -1659186716L; 685 686 /** 687 * Constructor 688 */ 689 public NutritionProductCharacteristicComponent() { 690 super(); 691 } 692 693 /** 694 * Constructor 695 */ 696 public NutritionProductCharacteristicComponent(CodeableConcept type, DataType value) { 697 super(); 698 this.setType(type); 699 this.setValue(value); 700 } 701 702 /** 703 * @return {@link #type} (A code specifying which characteristic of the product is being described (for example, colour, shape).) 704 */ 705 public CodeableConcept getType() { 706 if (this.type == null) 707 if (Configuration.errorOnAutoCreate()) 708 throw new Error("Attempt to auto-create NutritionProductCharacteristicComponent.type"); 709 else if (Configuration.doAutoCreate()) 710 this.type = new CodeableConcept(); // cc 711 return this.type; 712 } 713 714 public boolean hasType() { 715 return this.type != null && !this.type.isEmpty(); 716 } 717 718 /** 719 * @param value {@link #type} (A code specifying which characteristic of the product is being described (for example, colour, shape).) 720 */ 721 public NutritionProductCharacteristicComponent setType(CodeableConcept value) { 722 this.type = value; 723 return this; 724 } 725 726 /** 727 * @return {@link #value} (The actual characteristic value corresponding to the type.) 728 */ 729 public DataType getValue() { 730 return this.value; 731 } 732 733 /** 734 * @return {@link #value} (The actual characteristic value corresponding to the type.) 735 */ 736 public CodeableConcept getValueCodeableConcept() throws FHIRException { 737 if (this.value == null) 738 this.value = new CodeableConcept(); 739 if (!(this.value instanceof CodeableConcept)) 740 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 741 return (CodeableConcept) this.value; 742 } 743 744 public boolean hasValueCodeableConcept() { 745 return this != null && this.value instanceof CodeableConcept; 746 } 747 748 /** 749 * @return {@link #value} (The actual characteristic value corresponding to the type.) 750 */ 751 public StringType getValueStringType() throws FHIRException { 752 if (this.value == null) 753 this.value = new StringType(); 754 if (!(this.value instanceof StringType)) 755 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 756 return (StringType) this.value; 757 } 758 759 public boolean hasValueStringType() { 760 return this != null && this.value instanceof StringType; 761 } 762 763 /** 764 * @return {@link #value} (The actual characteristic value corresponding to the type.) 765 */ 766 public Quantity getValueQuantity() throws FHIRException { 767 if (this.value == null) 768 this.value = new Quantity(); 769 if (!(this.value instanceof Quantity)) 770 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 771 return (Quantity) this.value; 772 } 773 774 public boolean hasValueQuantity() { 775 return this != null && this.value instanceof Quantity; 776 } 777 778 /** 779 * @return {@link #value} (The actual characteristic value corresponding to the type.) 780 */ 781 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 782 if (this.value == null) 783 this.value = new Base64BinaryType(); 784 if (!(this.value instanceof Base64BinaryType)) 785 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 786 return (Base64BinaryType) this.value; 787 } 788 789 public boolean hasValueBase64BinaryType() { 790 return this != null && this.value instanceof Base64BinaryType; 791 } 792 793 /** 794 * @return {@link #value} (The actual characteristic value corresponding to the type.) 795 */ 796 public Attachment getValueAttachment() throws FHIRException { 797 if (this.value == null) 798 this.value = new Attachment(); 799 if (!(this.value instanceof Attachment)) 800 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 801 return (Attachment) this.value; 802 } 803 804 public boolean hasValueAttachment() { 805 return this != null && this.value instanceof Attachment; 806 } 807 808 /** 809 * @return {@link #value} (The actual characteristic value corresponding to the type.) 810 */ 811 public BooleanType getValueBooleanType() throws FHIRException { 812 if (this.value == null) 813 this.value = new BooleanType(); 814 if (!(this.value instanceof BooleanType)) 815 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 816 return (BooleanType) this.value; 817 } 818 819 public boolean hasValueBooleanType() { 820 return this != null && this.value instanceof BooleanType; 821 } 822 823 public boolean hasValue() { 824 return this.value != null && !this.value.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #value} (The actual characteristic value corresponding to the type.) 829 */ 830 public NutritionProductCharacteristicComponent setValue(DataType value) { 831 if (value != null && !(value instanceof CodeableConcept || value instanceof StringType || value instanceof Quantity || value instanceof Base64BinaryType || value instanceof Attachment || value instanceof BooleanType)) 832 throw new FHIRException("Not the right type for NutritionProduct.characteristic.value[x]: "+value.fhirType()); 833 this.value = value; 834 return this; 835 } 836 837 protected void listChildren(List<Property> children) { 838 super.listChildren(children); 839 children.add(new Property("type", "CodeableConcept", "A code specifying which characteristic of the product is being described (for example, colour, shape).", 0, 1, type)); 840 children.add(new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment|boolean", "The actual characteristic value corresponding to the type.", 0, 1, value)); 841 } 842 843 @Override 844 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 845 switch (_hash) { 846 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code specifying which characteristic of the product is being described (for example, colour, shape).", 0, 1, type); 847 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment|boolean", "The actual characteristic value corresponding to the type.", 0, 1, value); 848 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment|boolean", "The actual characteristic value corresponding to the type.", 0, 1, value); 849 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The actual characteristic value corresponding to the type.", 0, 1, value); 850 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The actual characteristic value corresponding to the type.", 0, 1, value); 851 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The actual characteristic value corresponding to the type.", 0, 1, value); 852 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The actual characteristic value corresponding to the type.", 0, 1, value); 853 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The actual characteristic value corresponding to the type.", 0, 1, value); 854 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The actual characteristic value corresponding to the type.", 0, 1, value); 855 default: return super.getNamedProperty(_hash, _name, _checkValid); 856 } 857 858 } 859 860 @Override 861 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 862 switch (hash) { 863 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 864 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 865 default: return super.getProperty(hash, name, checkValid); 866 } 867 868 } 869 870 @Override 871 public Base setProperty(int hash, String name, Base value) throws FHIRException { 872 switch (hash) { 873 case 3575610: // type 874 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 875 return value; 876 case 111972721: // value 877 this.value = TypeConvertor.castToType(value); // DataType 878 return value; 879 default: return super.setProperty(hash, name, value); 880 } 881 882 } 883 884 @Override 885 public Base setProperty(String name, Base value) throws FHIRException { 886 if (name.equals("type")) { 887 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 888 } else if (name.equals("value[x]")) { 889 this.value = TypeConvertor.castToType(value); // DataType 890 } else 891 return super.setProperty(name, value); 892 return value; 893 } 894 895 @Override 896 public void removeChild(String name, Base value) throws FHIRException { 897 if (name.equals("type")) { 898 this.type = null; 899 } else if (name.equals("value[x]")) { 900 this.value = null; 901 } else 902 super.removeChild(name, value); 903 904 } 905 906 @Override 907 public Base makeProperty(int hash, String name) throws FHIRException { 908 switch (hash) { 909 case 3575610: return getType(); 910 case -1410166417: return getValue(); 911 case 111972721: return getValue(); 912 default: return super.makeProperty(hash, name); 913 } 914 915 } 916 917 @Override 918 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 919 switch (hash) { 920 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 921 case 111972721: /*value*/ return new String[] {"CodeableConcept", "string", "Quantity", "base64Binary", "Attachment", "boolean"}; 922 default: return super.getTypesForProperty(hash, name); 923 } 924 925 } 926 927 @Override 928 public Base addChild(String name) throws FHIRException { 929 if (name.equals("type")) { 930 this.type = new CodeableConcept(); 931 return this.type; 932 } 933 else if (name.equals("valueCodeableConcept")) { 934 this.value = new CodeableConcept(); 935 return this.value; 936 } 937 else if (name.equals("valueString")) { 938 this.value = new StringType(); 939 return this.value; 940 } 941 else if (name.equals("valueQuantity")) { 942 this.value = new Quantity(); 943 return this.value; 944 } 945 else if (name.equals("valueBase64Binary")) { 946 this.value = new Base64BinaryType(); 947 return this.value; 948 } 949 else if (name.equals("valueAttachment")) { 950 this.value = new Attachment(); 951 return this.value; 952 } 953 else if (name.equals("valueBoolean")) { 954 this.value = new BooleanType(); 955 return this.value; 956 } 957 else 958 return super.addChild(name); 959 } 960 961 public NutritionProductCharacteristicComponent copy() { 962 NutritionProductCharacteristicComponent dst = new NutritionProductCharacteristicComponent(); 963 copyValues(dst); 964 return dst; 965 } 966 967 public void copyValues(NutritionProductCharacteristicComponent dst) { 968 super.copyValues(dst); 969 dst.type = type == null ? null : type.copy(); 970 dst.value = value == null ? null : value.copy(); 971 } 972 973 @Override 974 public boolean equalsDeep(Base other_) { 975 if (!super.equalsDeep(other_)) 976 return false; 977 if (!(other_ instanceof NutritionProductCharacteristicComponent)) 978 return false; 979 NutritionProductCharacteristicComponent o = (NutritionProductCharacteristicComponent) other_; 980 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 981 } 982 983 @Override 984 public boolean equalsShallow(Base other_) { 985 if (!super.equalsShallow(other_)) 986 return false; 987 if (!(other_ instanceof NutritionProductCharacteristicComponent)) 988 return false; 989 NutritionProductCharacteristicComponent o = (NutritionProductCharacteristicComponent) other_; 990 return true; 991 } 992 993 public boolean isEmpty() { 994 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 995 } 996 997 public String fhirType() { 998 return "NutritionProduct.characteristic"; 999 1000 } 1001 1002 } 1003 1004 @Block() 1005 public static class NutritionProductInstanceComponent extends BackboneElement implements IBaseBackboneElement { 1006 /** 1007 * The amount of items or instances that the resource considers, for instance when referring to 2 identical units together. 1008 */ 1009 @Child(name = "quantity", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 1010 @Description(shortDefinition="The amount of items or instances", formalDefinition="The amount of items or instances that the resource considers, for instance when referring to 2 identical units together." ) 1011 protected Quantity quantity; 1012 1013 /** 1014 * The identifier for the physical instance, typically a serial number or manufacturer number. 1015 */ 1016 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1017 @Description(shortDefinition="The identifier for the physical instance, typically a serial number or manufacturer number", formalDefinition="The identifier for the physical instance, typically a serial number or manufacturer number." ) 1018 protected List<Identifier> identifier; 1019 1020 /** 1021 * The name for the specific product. 1022 */ 1023 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1024 @Description(shortDefinition="The name for the specific product", formalDefinition="The name for the specific product." ) 1025 protected StringType name; 1026 1027 /** 1028 * The identification of the batch or lot of the product. 1029 */ 1030 @Child(name = "lotNumber", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1031 @Description(shortDefinition="The identification of the batch or lot of the product", formalDefinition="The identification of the batch or lot of the product." ) 1032 protected StringType lotNumber; 1033 1034 /** 1035 * The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed. 1036 */ 1037 @Child(name = "expiry", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1038 @Description(shortDefinition="The expiry date or date and time for the product", formalDefinition="The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed." ) 1039 protected DateTimeType expiry; 1040 1041 /** 1042 * The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed. 1043 */ 1044 @Child(name = "useBy", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1045 @Description(shortDefinition="The date until which the product is expected to be good for consumption", formalDefinition="The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed." ) 1046 protected DateTimeType useBy; 1047 1048 /** 1049 * An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled. 1050 */ 1051 @Child(name = "biologicalSourceEvent", type = {Identifier.class}, order=7, min=0, max=1, modifier=false, summary=false) 1052 @Description(shortDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled", formalDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled." ) 1053 protected Identifier biologicalSourceEvent; 1054 1055 private static final long serialVersionUID = -954985011L; 1056 1057 /** 1058 * Constructor 1059 */ 1060 public NutritionProductInstanceComponent() { 1061 super(); 1062 } 1063 1064 /** 1065 * @return {@link #quantity} (The amount of items or instances that the resource considers, for instance when referring to 2 identical units together.) 1066 */ 1067 public Quantity getQuantity() { 1068 if (this.quantity == null) 1069 if (Configuration.errorOnAutoCreate()) 1070 throw new Error("Attempt to auto-create NutritionProductInstanceComponent.quantity"); 1071 else if (Configuration.doAutoCreate()) 1072 this.quantity = new Quantity(); // cc 1073 return this.quantity; 1074 } 1075 1076 public boolean hasQuantity() { 1077 return this.quantity != null && !this.quantity.isEmpty(); 1078 } 1079 1080 /** 1081 * @param value {@link #quantity} (The amount of items or instances that the resource considers, for instance when referring to 2 identical units together.) 1082 */ 1083 public NutritionProductInstanceComponent setQuantity(Quantity value) { 1084 this.quantity = value; 1085 return this; 1086 } 1087 1088 /** 1089 * @return {@link #identifier} (The identifier for the physical instance, typically a serial number or manufacturer number.) 1090 */ 1091 public List<Identifier> getIdentifier() { 1092 if (this.identifier == null) 1093 this.identifier = new ArrayList<Identifier>(); 1094 return this.identifier; 1095 } 1096 1097 /** 1098 * @return Returns a reference to <code>this</code> for easy method chaining 1099 */ 1100 public NutritionProductInstanceComponent setIdentifier(List<Identifier> theIdentifier) { 1101 this.identifier = theIdentifier; 1102 return this; 1103 } 1104 1105 public boolean hasIdentifier() { 1106 if (this.identifier == null) 1107 return false; 1108 for (Identifier item : this.identifier) 1109 if (!item.isEmpty()) 1110 return true; 1111 return false; 1112 } 1113 1114 public Identifier addIdentifier() { //3 1115 Identifier t = new Identifier(); 1116 if (this.identifier == null) 1117 this.identifier = new ArrayList<Identifier>(); 1118 this.identifier.add(t); 1119 return t; 1120 } 1121 1122 public NutritionProductInstanceComponent addIdentifier(Identifier t) { //3 1123 if (t == null) 1124 return this; 1125 if (this.identifier == null) 1126 this.identifier = new ArrayList<Identifier>(); 1127 this.identifier.add(t); 1128 return this; 1129 } 1130 1131 /** 1132 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1133 */ 1134 public Identifier getIdentifierFirstRep() { 1135 if (getIdentifier().isEmpty()) { 1136 addIdentifier(); 1137 } 1138 return getIdentifier().get(0); 1139 } 1140 1141 /** 1142 * @return {@link #name} (The name for the specific product.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1143 */ 1144 public StringType getNameElement() { 1145 if (this.name == null) 1146 if (Configuration.errorOnAutoCreate()) 1147 throw new Error("Attempt to auto-create NutritionProductInstanceComponent.name"); 1148 else if (Configuration.doAutoCreate()) 1149 this.name = new StringType(); // bb 1150 return this.name; 1151 } 1152 1153 public boolean hasNameElement() { 1154 return this.name != null && !this.name.isEmpty(); 1155 } 1156 1157 public boolean hasName() { 1158 return this.name != null && !this.name.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #name} (The name for the specific product.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1163 */ 1164 public NutritionProductInstanceComponent setNameElement(StringType value) { 1165 this.name = value; 1166 return this; 1167 } 1168 1169 /** 1170 * @return The name for the specific product. 1171 */ 1172 public String getName() { 1173 return this.name == null ? null : this.name.getValue(); 1174 } 1175 1176 /** 1177 * @param value The name for the specific product. 1178 */ 1179 public NutritionProductInstanceComponent setName(String value) { 1180 if (Utilities.noString(value)) 1181 this.name = null; 1182 else { 1183 if (this.name == null) 1184 this.name = new StringType(); 1185 this.name.setValue(value); 1186 } 1187 return this; 1188 } 1189 1190 /** 1191 * @return {@link #lotNumber} (The identification of the batch or lot of the product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1192 */ 1193 public StringType getLotNumberElement() { 1194 if (this.lotNumber == null) 1195 if (Configuration.errorOnAutoCreate()) 1196 throw new Error("Attempt to auto-create NutritionProductInstanceComponent.lotNumber"); 1197 else if (Configuration.doAutoCreate()) 1198 this.lotNumber = new StringType(); // bb 1199 return this.lotNumber; 1200 } 1201 1202 public boolean hasLotNumberElement() { 1203 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1204 } 1205 1206 public boolean hasLotNumber() { 1207 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1208 } 1209 1210 /** 1211 * @param value {@link #lotNumber} (The identification of the batch or lot of the product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1212 */ 1213 public NutritionProductInstanceComponent setLotNumberElement(StringType value) { 1214 this.lotNumber = value; 1215 return this; 1216 } 1217 1218 /** 1219 * @return The identification of the batch or lot of the product. 1220 */ 1221 public String getLotNumber() { 1222 return this.lotNumber == null ? null : this.lotNumber.getValue(); 1223 } 1224 1225 /** 1226 * @param value The identification of the batch or lot of the product. 1227 */ 1228 public NutritionProductInstanceComponent setLotNumber(String value) { 1229 if (Utilities.noString(value)) 1230 this.lotNumber = null; 1231 else { 1232 if (this.lotNumber == null) 1233 this.lotNumber = new StringType(); 1234 this.lotNumber.setValue(value); 1235 } 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #expiry} (The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 1241 */ 1242 public DateTimeType getExpiryElement() { 1243 if (this.expiry == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create NutritionProductInstanceComponent.expiry"); 1246 else if (Configuration.doAutoCreate()) 1247 this.expiry = new DateTimeType(); // bb 1248 return this.expiry; 1249 } 1250 1251 public boolean hasExpiryElement() { 1252 return this.expiry != null && !this.expiry.isEmpty(); 1253 } 1254 1255 public boolean hasExpiry() { 1256 return this.expiry != null && !this.expiry.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #expiry} (The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 1261 */ 1262 public NutritionProductInstanceComponent setExpiryElement(DateTimeType value) { 1263 this.expiry = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed. 1269 */ 1270 public Date getExpiry() { 1271 return this.expiry == null ? null : this.expiry.getValue(); 1272 } 1273 1274 /** 1275 * @param value The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed. 1276 */ 1277 public NutritionProductInstanceComponent setExpiry(Date value) { 1278 if (value == null) 1279 this.expiry = null; 1280 else { 1281 if (this.expiry == null) 1282 this.expiry = new DateTimeType(); 1283 this.expiry.setValue(value); 1284 } 1285 return this; 1286 } 1287 1288 /** 1289 * @return {@link #useBy} (The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.). This is the underlying object with id, value and extensions. The accessor "getUseBy" gives direct access to the value 1290 */ 1291 public DateTimeType getUseByElement() { 1292 if (this.useBy == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create NutritionProductInstanceComponent.useBy"); 1295 else if (Configuration.doAutoCreate()) 1296 this.useBy = new DateTimeType(); // bb 1297 return this.useBy; 1298 } 1299 1300 public boolean hasUseByElement() { 1301 return this.useBy != null && !this.useBy.isEmpty(); 1302 } 1303 1304 public boolean hasUseBy() { 1305 return this.useBy != null && !this.useBy.isEmpty(); 1306 } 1307 1308 /** 1309 * @param value {@link #useBy} (The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.). This is the underlying object with id, value and extensions. The accessor "getUseBy" gives direct access to the value 1310 */ 1311 public NutritionProductInstanceComponent setUseByElement(DateTimeType value) { 1312 this.useBy = value; 1313 return this; 1314 } 1315 1316 /** 1317 * @return The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed. 1318 */ 1319 public Date getUseBy() { 1320 return this.useBy == null ? null : this.useBy.getValue(); 1321 } 1322 1323 /** 1324 * @param value The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed. 1325 */ 1326 public NutritionProductInstanceComponent setUseBy(Date value) { 1327 if (value == null) 1328 this.useBy = null; 1329 else { 1330 if (this.useBy == null) 1331 this.useBy = new DateTimeType(); 1332 this.useBy.setValue(value); 1333 } 1334 return this; 1335 } 1336 1337 /** 1338 * @return {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 1339 */ 1340 public Identifier getBiologicalSourceEvent() { 1341 if (this.biologicalSourceEvent == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create NutritionProductInstanceComponent.biologicalSourceEvent"); 1344 else if (Configuration.doAutoCreate()) 1345 this.biologicalSourceEvent = new Identifier(); // cc 1346 return this.biologicalSourceEvent; 1347 } 1348 1349 public boolean hasBiologicalSourceEvent() { 1350 return this.biologicalSourceEvent != null && !this.biologicalSourceEvent.isEmpty(); 1351 } 1352 1353 /** 1354 * @param value {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 1355 */ 1356 public NutritionProductInstanceComponent setBiologicalSourceEvent(Identifier value) { 1357 this.biologicalSourceEvent = value; 1358 return this; 1359 } 1360 1361 protected void listChildren(List<Property> children) { 1362 super.listChildren(children); 1363 children.add(new Property("quantity", "Quantity", "The amount of items or instances that the resource considers, for instance when referring to 2 identical units together.", 0, 1, quantity)); 1364 children.add(new Property("identifier", "Identifier", "The identifier for the physical instance, typically a serial number or manufacturer number.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1365 children.add(new Property("name", "string", "The name for the specific product.", 0, 1, name)); 1366 children.add(new Property("lotNumber", "string", "The identification of the batch or lot of the product.", 0, 1, lotNumber)); 1367 children.add(new Property("expiry", "dateTime", "The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.", 0, 1, expiry)); 1368 children.add(new Property("useBy", "dateTime", "The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.", 0, 1, useBy)); 1369 children.add(new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent)); 1370 } 1371 1372 @Override 1373 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1374 switch (_hash) { 1375 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of items or instances that the resource considers, for instance when referring to 2 identical units together.", 0, 1, quantity); 1376 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier for the physical instance, typically a serial number or manufacturer number.", 0, java.lang.Integer.MAX_VALUE, identifier); 1377 case 3373707: /*name*/ return new Property("name", "string", "The name for the specific product.", 0, 1, name); 1378 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "The identification of the batch or lot of the product.", 0, 1, lotNumber); 1379 case -1289159373: /*expiry*/ return new Property("expiry", "dateTime", "The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.", 0, 1, expiry); 1380 case 111577150: /*useBy*/ return new Property("useBy", "dateTime", "The time after which the product is no longer expected to be in proper condition, or its use is not advised or not allowed.", 0, 1, useBy); 1381 case -654468482: /*biologicalSourceEvent*/ return new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent); 1382 default: return super.getNamedProperty(_hash, _name, _checkValid); 1383 } 1384 1385 } 1386 1387 @Override 1388 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1389 switch (hash) { 1390 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1391 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1392 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1393 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 1394 case -1289159373: /*expiry*/ return this.expiry == null ? new Base[0] : new Base[] {this.expiry}; // DateTimeType 1395 case 111577150: /*useBy*/ return this.useBy == null ? new Base[0] : new Base[] {this.useBy}; // DateTimeType 1396 case -654468482: /*biologicalSourceEvent*/ return this.biologicalSourceEvent == null ? new Base[0] : new Base[] {this.biologicalSourceEvent}; // Identifier 1397 default: return super.getProperty(hash, name, checkValid); 1398 } 1399 1400 } 1401 1402 @Override 1403 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1404 switch (hash) { 1405 case -1285004149: // quantity 1406 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1407 return value; 1408 case -1618432855: // identifier 1409 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1410 return value; 1411 case 3373707: // name 1412 this.name = TypeConvertor.castToString(value); // StringType 1413 return value; 1414 case 462547450: // lotNumber 1415 this.lotNumber = TypeConvertor.castToString(value); // StringType 1416 return value; 1417 case -1289159373: // expiry 1418 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 1419 return value; 1420 case 111577150: // useBy 1421 this.useBy = TypeConvertor.castToDateTime(value); // DateTimeType 1422 return value; 1423 case -654468482: // biologicalSourceEvent 1424 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 1425 return value; 1426 default: return super.setProperty(hash, name, value); 1427 } 1428 1429 } 1430 1431 @Override 1432 public Base setProperty(String name, Base value) throws FHIRException { 1433 if (name.equals("quantity")) { 1434 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1435 } else if (name.equals("identifier")) { 1436 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1437 } else if (name.equals("name")) { 1438 this.name = TypeConvertor.castToString(value); // StringType 1439 } else if (name.equals("lotNumber")) { 1440 this.lotNumber = TypeConvertor.castToString(value); // StringType 1441 } else if (name.equals("expiry")) { 1442 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 1443 } else if (name.equals("useBy")) { 1444 this.useBy = TypeConvertor.castToDateTime(value); // DateTimeType 1445 } else if (name.equals("biologicalSourceEvent")) { 1446 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 1447 } else 1448 return super.setProperty(name, value); 1449 return value; 1450 } 1451 1452 @Override 1453 public void removeChild(String name, Base value) throws FHIRException { 1454 if (name.equals("quantity")) { 1455 this.quantity = null; 1456 } else if (name.equals("identifier")) { 1457 this.getIdentifier().remove(value); 1458 } else if (name.equals("name")) { 1459 this.name = null; 1460 } else if (name.equals("lotNumber")) { 1461 this.lotNumber = null; 1462 } else if (name.equals("expiry")) { 1463 this.expiry = null; 1464 } else if (name.equals("useBy")) { 1465 this.useBy = null; 1466 } else if (name.equals("biologicalSourceEvent")) { 1467 this.biologicalSourceEvent = null; 1468 } else 1469 super.removeChild(name, value); 1470 1471 } 1472 1473 @Override 1474 public Base makeProperty(int hash, String name) throws FHIRException { 1475 switch (hash) { 1476 case -1285004149: return getQuantity(); 1477 case -1618432855: return addIdentifier(); 1478 case 3373707: return getNameElement(); 1479 case 462547450: return getLotNumberElement(); 1480 case -1289159373: return getExpiryElement(); 1481 case 111577150: return getUseByElement(); 1482 case -654468482: return getBiologicalSourceEvent(); 1483 default: return super.makeProperty(hash, name); 1484 } 1485 1486 } 1487 1488 @Override 1489 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1490 switch (hash) { 1491 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1492 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1493 case 3373707: /*name*/ return new String[] {"string"}; 1494 case 462547450: /*lotNumber*/ return new String[] {"string"}; 1495 case -1289159373: /*expiry*/ return new String[] {"dateTime"}; 1496 case 111577150: /*useBy*/ return new String[] {"dateTime"}; 1497 case -654468482: /*biologicalSourceEvent*/ return new String[] {"Identifier"}; 1498 default: return super.getTypesForProperty(hash, name); 1499 } 1500 1501 } 1502 1503 @Override 1504 public Base addChild(String name) throws FHIRException { 1505 if (name.equals("quantity")) { 1506 this.quantity = new Quantity(); 1507 return this.quantity; 1508 } 1509 else if (name.equals("identifier")) { 1510 return addIdentifier(); 1511 } 1512 else if (name.equals("name")) { 1513 throw new FHIRException("Cannot call addChild on a singleton property NutritionProduct.instance.name"); 1514 } 1515 else if (name.equals("lotNumber")) { 1516 throw new FHIRException("Cannot call addChild on a singleton property NutritionProduct.instance.lotNumber"); 1517 } 1518 else if (name.equals("expiry")) { 1519 throw new FHIRException("Cannot call addChild on a singleton property NutritionProduct.instance.expiry"); 1520 } 1521 else if (name.equals("useBy")) { 1522 throw new FHIRException("Cannot call addChild on a singleton property NutritionProduct.instance.useBy"); 1523 } 1524 else if (name.equals("biologicalSourceEvent")) { 1525 this.biologicalSourceEvent = new Identifier(); 1526 return this.biologicalSourceEvent; 1527 } 1528 else 1529 return super.addChild(name); 1530 } 1531 1532 public NutritionProductInstanceComponent copy() { 1533 NutritionProductInstanceComponent dst = new NutritionProductInstanceComponent(); 1534 copyValues(dst); 1535 return dst; 1536 } 1537 1538 public void copyValues(NutritionProductInstanceComponent dst) { 1539 super.copyValues(dst); 1540 dst.quantity = quantity == null ? null : quantity.copy(); 1541 if (identifier != null) { 1542 dst.identifier = new ArrayList<Identifier>(); 1543 for (Identifier i : identifier) 1544 dst.identifier.add(i.copy()); 1545 }; 1546 dst.name = name == null ? null : name.copy(); 1547 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 1548 dst.expiry = expiry == null ? null : expiry.copy(); 1549 dst.useBy = useBy == null ? null : useBy.copy(); 1550 dst.biologicalSourceEvent = biologicalSourceEvent == null ? null : biologicalSourceEvent.copy(); 1551 } 1552 1553 @Override 1554 public boolean equalsDeep(Base other_) { 1555 if (!super.equalsDeep(other_)) 1556 return false; 1557 if (!(other_ instanceof NutritionProductInstanceComponent)) 1558 return false; 1559 NutritionProductInstanceComponent o = (NutritionProductInstanceComponent) other_; 1560 return compareDeep(quantity, o.quantity, true) && compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 1561 && compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expiry, o.expiry, true) && compareDeep(useBy, o.useBy, true) 1562 && compareDeep(biologicalSourceEvent, o.biologicalSourceEvent, true); 1563 } 1564 1565 @Override 1566 public boolean equalsShallow(Base other_) { 1567 if (!super.equalsShallow(other_)) 1568 return false; 1569 if (!(other_ instanceof NutritionProductInstanceComponent)) 1570 return false; 1571 NutritionProductInstanceComponent o = (NutritionProductInstanceComponent) other_; 1572 return compareValues(name, o.name, true) && compareValues(lotNumber, o.lotNumber, true) && compareValues(expiry, o.expiry, true) 1573 && compareValues(useBy, o.useBy, true); 1574 } 1575 1576 public boolean isEmpty() { 1577 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, identifier, name 1578 , lotNumber, expiry, useBy, biologicalSourceEvent); 1579 } 1580 1581 public String fhirType() { 1582 return "NutritionProduct.instance"; 1583 1584 } 1585 1586 } 1587 1588 /** 1589 * The code assigned to the product, for example a USDA NDB number, a USDA FDC ID number, or a Langual code. 1590 */ 1591 @Child(name = "code", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 1592 @Description(shortDefinition="A code that can identify the detailed nutrients and ingredients in a specific food product", formalDefinition="The code assigned to the product, for example a USDA NDB number, a USDA FDC ID number, or a Langual code." ) 1593 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/edible-substance-type") 1594 protected CodeableConcept code; 1595 1596 /** 1597 * The current state of the product. 1598 */ 1599 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1600 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="The current state of the product." ) 1601 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/nutritionproduct-status") 1602 protected Enumeration<NutritionProductStatus> status; 1603 1604 /** 1605 * Nutrition products can have different classifications - according to its nutritional properties, preparation methods, etc. 1606 */ 1607 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1608 @Description(shortDefinition="Broad product groups or categories used to classify the product, such as Legume and Legume Products, Beverages, or Beef Products", formalDefinition="Nutrition products can have different classifications - according to its nutritional properties, preparation methods, etc." ) 1609 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/nutrition-product-category") 1610 protected List<CodeableConcept> category; 1611 1612 /** 1613 * The organisation (manufacturer, representative or legal authorization holder) that is responsible for the device. 1614 */ 1615 @Child(name = "manufacturer", type = {Organization.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1616 @Description(shortDefinition="Manufacturer, representative or officially responsible for the product", formalDefinition="The organisation (manufacturer, representative or legal authorization holder) that is responsible for the device." ) 1617 protected List<Reference> manufacturer; 1618 1619 /** 1620 * The product's nutritional information expressed by the nutrients. 1621 */ 1622 @Child(name = "nutrient", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1623 @Description(shortDefinition="The product's nutritional information expressed by the nutrients", formalDefinition="The product's nutritional information expressed by the nutrients." ) 1624 protected List<NutritionProductNutrientComponent> nutrient; 1625 1626 /** 1627 * Ingredients contained in this product. 1628 */ 1629 @Child(name = "ingredient", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1630 @Description(shortDefinition="Ingredients contained in this product", formalDefinition="Ingredients contained in this product." ) 1631 protected List<NutritionProductIngredientComponent> ingredient; 1632 1633 /** 1634 * Allergens that are known or suspected to be a part of this nutrition product. 1635 */ 1636 @Child(name = "knownAllergen", type = {CodeableReference.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1637 @Description(shortDefinition="Known or suspected allergens that are a part of this product", formalDefinition="Allergens that are known or suspected to be a part of this nutrition product." ) 1638 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergen-class") 1639 protected List<CodeableReference> knownAllergen; 1640 1641 /** 1642 * Specifies descriptive properties of the nutrition product. 1643 */ 1644 @Child(name = "characteristic", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1645 @Description(shortDefinition="Specifies descriptive properties of the nutrition product", formalDefinition="Specifies descriptive properties of the nutrition product." ) 1646 protected List<NutritionProductCharacteristicComponent> characteristic; 1647 1648 /** 1649 * Conveys instance-level information about this product item. One or several physical, countable instances or occurrences of the product. 1650 */ 1651 @Child(name = "instance", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1652 @Description(shortDefinition="One or several physical instances or occurrences of the nutrition product", formalDefinition="Conveys instance-level information about this product item. One or several physical, countable instances or occurrences of the product." ) 1653 protected List<NutritionProductInstanceComponent> instance; 1654 1655 /** 1656 * Comments made about the product. 1657 */ 1658 @Child(name = "note", type = {Annotation.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1659 @Description(shortDefinition="Comments made about the product", formalDefinition="Comments made about the product." ) 1660 protected List<Annotation> note; 1661 1662 private static final long serialVersionUID = 182320595L; 1663 1664 /** 1665 * Constructor 1666 */ 1667 public NutritionProduct() { 1668 super(); 1669 } 1670 1671 /** 1672 * Constructor 1673 */ 1674 public NutritionProduct(NutritionProductStatus status) { 1675 super(); 1676 this.setStatus(status); 1677 } 1678 1679 /** 1680 * @return {@link #code} (The code assigned to the product, for example a USDA NDB number, a USDA FDC ID number, or a Langual code.) 1681 */ 1682 public CodeableConcept getCode() { 1683 if (this.code == null) 1684 if (Configuration.errorOnAutoCreate()) 1685 throw new Error("Attempt to auto-create NutritionProduct.code"); 1686 else if (Configuration.doAutoCreate()) 1687 this.code = new CodeableConcept(); // cc 1688 return this.code; 1689 } 1690 1691 public boolean hasCode() { 1692 return this.code != null && !this.code.isEmpty(); 1693 } 1694 1695 /** 1696 * @param value {@link #code} (The code assigned to the product, for example a USDA NDB number, a USDA FDC ID number, or a Langual code.) 1697 */ 1698 public NutritionProduct setCode(CodeableConcept value) { 1699 this.code = value; 1700 return this; 1701 } 1702 1703 /** 1704 * @return {@link #status} (The current state of the product.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1705 */ 1706 public Enumeration<NutritionProductStatus> getStatusElement() { 1707 if (this.status == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create NutritionProduct.status"); 1710 else if (Configuration.doAutoCreate()) 1711 this.status = new Enumeration<NutritionProductStatus>(new NutritionProductStatusEnumFactory()); // bb 1712 return this.status; 1713 } 1714 1715 public boolean hasStatusElement() { 1716 return this.status != null && !this.status.isEmpty(); 1717 } 1718 1719 public boolean hasStatus() { 1720 return this.status != null && !this.status.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #status} (The current state of the product.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1725 */ 1726 public NutritionProduct setStatusElement(Enumeration<NutritionProductStatus> value) { 1727 this.status = value; 1728 return this; 1729 } 1730 1731 /** 1732 * @return The current state of the product. 1733 */ 1734 public NutritionProductStatus getStatus() { 1735 return this.status == null ? null : this.status.getValue(); 1736 } 1737 1738 /** 1739 * @param value The current state of the product. 1740 */ 1741 public NutritionProduct setStatus(NutritionProductStatus value) { 1742 if (this.status == null) 1743 this.status = new Enumeration<NutritionProductStatus>(new NutritionProductStatusEnumFactory()); 1744 this.status.setValue(value); 1745 return this; 1746 } 1747 1748 /** 1749 * @return {@link #category} (Nutrition products can have different classifications - according to its nutritional properties, preparation methods, etc.) 1750 */ 1751 public List<CodeableConcept> getCategory() { 1752 if (this.category == null) 1753 this.category = new ArrayList<CodeableConcept>(); 1754 return this.category; 1755 } 1756 1757 /** 1758 * @return Returns a reference to <code>this</code> for easy method chaining 1759 */ 1760 public NutritionProduct setCategory(List<CodeableConcept> theCategory) { 1761 this.category = theCategory; 1762 return this; 1763 } 1764 1765 public boolean hasCategory() { 1766 if (this.category == null) 1767 return false; 1768 for (CodeableConcept item : this.category) 1769 if (!item.isEmpty()) 1770 return true; 1771 return false; 1772 } 1773 1774 public CodeableConcept addCategory() { //3 1775 CodeableConcept t = new CodeableConcept(); 1776 if (this.category == null) 1777 this.category = new ArrayList<CodeableConcept>(); 1778 this.category.add(t); 1779 return t; 1780 } 1781 1782 public NutritionProduct addCategory(CodeableConcept t) { //3 1783 if (t == null) 1784 return this; 1785 if (this.category == null) 1786 this.category = new ArrayList<CodeableConcept>(); 1787 this.category.add(t); 1788 return this; 1789 } 1790 1791 /** 1792 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1793 */ 1794 public CodeableConcept getCategoryFirstRep() { 1795 if (getCategory().isEmpty()) { 1796 addCategory(); 1797 } 1798 return getCategory().get(0); 1799 } 1800 1801 /** 1802 * @return {@link #manufacturer} (The organisation (manufacturer, representative or legal authorization holder) that is responsible for the device.) 1803 */ 1804 public List<Reference> getManufacturer() { 1805 if (this.manufacturer == null) 1806 this.manufacturer = new ArrayList<Reference>(); 1807 return this.manufacturer; 1808 } 1809 1810 /** 1811 * @return Returns a reference to <code>this</code> for easy method chaining 1812 */ 1813 public NutritionProduct setManufacturer(List<Reference> theManufacturer) { 1814 this.manufacturer = theManufacturer; 1815 return this; 1816 } 1817 1818 public boolean hasManufacturer() { 1819 if (this.manufacturer == null) 1820 return false; 1821 for (Reference item : this.manufacturer) 1822 if (!item.isEmpty()) 1823 return true; 1824 return false; 1825 } 1826 1827 public Reference addManufacturer() { //3 1828 Reference t = new Reference(); 1829 if (this.manufacturer == null) 1830 this.manufacturer = new ArrayList<Reference>(); 1831 this.manufacturer.add(t); 1832 return t; 1833 } 1834 1835 public NutritionProduct addManufacturer(Reference t) { //3 1836 if (t == null) 1837 return this; 1838 if (this.manufacturer == null) 1839 this.manufacturer = new ArrayList<Reference>(); 1840 this.manufacturer.add(t); 1841 return this; 1842 } 1843 1844 /** 1845 * @return The first repetition of repeating field {@link #manufacturer}, creating it if it does not already exist {3} 1846 */ 1847 public Reference getManufacturerFirstRep() { 1848 if (getManufacturer().isEmpty()) { 1849 addManufacturer(); 1850 } 1851 return getManufacturer().get(0); 1852 } 1853 1854 /** 1855 * @return {@link #nutrient} (The product's nutritional information expressed by the nutrients.) 1856 */ 1857 public List<NutritionProductNutrientComponent> getNutrient() { 1858 if (this.nutrient == null) 1859 this.nutrient = new ArrayList<NutritionProductNutrientComponent>(); 1860 return this.nutrient; 1861 } 1862 1863 /** 1864 * @return Returns a reference to <code>this</code> for easy method chaining 1865 */ 1866 public NutritionProduct setNutrient(List<NutritionProductNutrientComponent> theNutrient) { 1867 this.nutrient = theNutrient; 1868 return this; 1869 } 1870 1871 public boolean hasNutrient() { 1872 if (this.nutrient == null) 1873 return false; 1874 for (NutritionProductNutrientComponent item : this.nutrient) 1875 if (!item.isEmpty()) 1876 return true; 1877 return false; 1878 } 1879 1880 public NutritionProductNutrientComponent addNutrient() { //3 1881 NutritionProductNutrientComponent t = new NutritionProductNutrientComponent(); 1882 if (this.nutrient == null) 1883 this.nutrient = new ArrayList<NutritionProductNutrientComponent>(); 1884 this.nutrient.add(t); 1885 return t; 1886 } 1887 1888 public NutritionProduct addNutrient(NutritionProductNutrientComponent t) { //3 1889 if (t == null) 1890 return this; 1891 if (this.nutrient == null) 1892 this.nutrient = new ArrayList<NutritionProductNutrientComponent>(); 1893 this.nutrient.add(t); 1894 return this; 1895 } 1896 1897 /** 1898 * @return The first repetition of repeating field {@link #nutrient}, creating it if it does not already exist {3} 1899 */ 1900 public NutritionProductNutrientComponent getNutrientFirstRep() { 1901 if (getNutrient().isEmpty()) { 1902 addNutrient(); 1903 } 1904 return getNutrient().get(0); 1905 } 1906 1907 /** 1908 * @return {@link #ingredient} (Ingredients contained in this product.) 1909 */ 1910 public List<NutritionProductIngredientComponent> getIngredient() { 1911 if (this.ingredient == null) 1912 this.ingredient = new ArrayList<NutritionProductIngredientComponent>(); 1913 return this.ingredient; 1914 } 1915 1916 /** 1917 * @return Returns a reference to <code>this</code> for easy method chaining 1918 */ 1919 public NutritionProduct setIngredient(List<NutritionProductIngredientComponent> theIngredient) { 1920 this.ingredient = theIngredient; 1921 return this; 1922 } 1923 1924 public boolean hasIngredient() { 1925 if (this.ingredient == null) 1926 return false; 1927 for (NutritionProductIngredientComponent item : this.ingredient) 1928 if (!item.isEmpty()) 1929 return true; 1930 return false; 1931 } 1932 1933 public NutritionProductIngredientComponent addIngredient() { //3 1934 NutritionProductIngredientComponent t = new NutritionProductIngredientComponent(); 1935 if (this.ingredient == null) 1936 this.ingredient = new ArrayList<NutritionProductIngredientComponent>(); 1937 this.ingredient.add(t); 1938 return t; 1939 } 1940 1941 public NutritionProduct addIngredient(NutritionProductIngredientComponent t) { //3 1942 if (t == null) 1943 return this; 1944 if (this.ingredient == null) 1945 this.ingredient = new ArrayList<NutritionProductIngredientComponent>(); 1946 this.ingredient.add(t); 1947 return this; 1948 } 1949 1950 /** 1951 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 1952 */ 1953 public NutritionProductIngredientComponent getIngredientFirstRep() { 1954 if (getIngredient().isEmpty()) { 1955 addIngredient(); 1956 } 1957 return getIngredient().get(0); 1958 } 1959 1960 /** 1961 * @return {@link #knownAllergen} (Allergens that are known or suspected to be a part of this nutrition product.) 1962 */ 1963 public List<CodeableReference> getKnownAllergen() { 1964 if (this.knownAllergen == null) 1965 this.knownAllergen = new ArrayList<CodeableReference>(); 1966 return this.knownAllergen; 1967 } 1968 1969 /** 1970 * @return Returns a reference to <code>this</code> for easy method chaining 1971 */ 1972 public NutritionProduct setKnownAllergen(List<CodeableReference> theKnownAllergen) { 1973 this.knownAllergen = theKnownAllergen; 1974 return this; 1975 } 1976 1977 public boolean hasKnownAllergen() { 1978 if (this.knownAllergen == null) 1979 return false; 1980 for (CodeableReference item : this.knownAllergen) 1981 if (!item.isEmpty()) 1982 return true; 1983 return false; 1984 } 1985 1986 public CodeableReference addKnownAllergen() { //3 1987 CodeableReference t = new CodeableReference(); 1988 if (this.knownAllergen == null) 1989 this.knownAllergen = new ArrayList<CodeableReference>(); 1990 this.knownAllergen.add(t); 1991 return t; 1992 } 1993 1994 public NutritionProduct addKnownAllergen(CodeableReference t) { //3 1995 if (t == null) 1996 return this; 1997 if (this.knownAllergen == null) 1998 this.knownAllergen = new ArrayList<CodeableReference>(); 1999 this.knownAllergen.add(t); 2000 return this; 2001 } 2002 2003 /** 2004 * @return The first repetition of repeating field {@link #knownAllergen}, creating it if it does not already exist {3} 2005 */ 2006 public CodeableReference getKnownAllergenFirstRep() { 2007 if (getKnownAllergen().isEmpty()) { 2008 addKnownAllergen(); 2009 } 2010 return getKnownAllergen().get(0); 2011 } 2012 2013 /** 2014 * @return {@link #characteristic} (Specifies descriptive properties of the nutrition product.) 2015 */ 2016 public List<NutritionProductCharacteristicComponent> getCharacteristic() { 2017 if (this.characteristic == null) 2018 this.characteristic = new ArrayList<NutritionProductCharacteristicComponent>(); 2019 return this.characteristic; 2020 } 2021 2022 /** 2023 * @return Returns a reference to <code>this</code> for easy method chaining 2024 */ 2025 public NutritionProduct setCharacteristic(List<NutritionProductCharacteristicComponent> theCharacteristic) { 2026 this.characteristic = theCharacteristic; 2027 return this; 2028 } 2029 2030 public boolean hasCharacteristic() { 2031 if (this.characteristic == null) 2032 return false; 2033 for (NutritionProductCharacteristicComponent item : this.characteristic) 2034 if (!item.isEmpty()) 2035 return true; 2036 return false; 2037 } 2038 2039 public NutritionProductCharacteristicComponent addCharacteristic() { //3 2040 NutritionProductCharacteristicComponent t = new NutritionProductCharacteristicComponent(); 2041 if (this.characteristic == null) 2042 this.characteristic = new ArrayList<NutritionProductCharacteristicComponent>(); 2043 this.characteristic.add(t); 2044 return t; 2045 } 2046 2047 public NutritionProduct addCharacteristic(NutritionProductCharacteristicComponent t) { //3 2048 if (t == null) 2049 return this; 2050 if (this.characteristic == null) 2051 this.characteristic = new ArrayList<NutritionProductCharacteristicComponent>(); 2052 this.characteristic.add(t); 2053 return this; 2054 } 2055 2056 /** 2057 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 2058 */ 2059 public NutritionProductCharacteristicComponent getCharacteristicFirstRep() { 2060 if (getCharacteristic().isEmpty()) { 2061 addCharacteristic(); 2062 } 2063 return getCharacteristic().get(0); 2064 } 2065 2066 /** 2067 * @return {@link #instance} (Conveys instance-level information about this product item. One or several physical, countable instances or occurrences of the product.) 2068 */ 2069 public List<NutritionProductInstanceComponent> getInstance() { 2070 if (this.instance == null) 2071 this.instance = new ArrayList<NutritionProductInstanceComponent>(); 2072 return this.instance; 2073 } 2074 2075 /** 2076 * @return Returns a reference to <code>this</code> for easy method chaining 2077 */ 2078 public NutritionProduct setInstance(List<NutritionProductInstanceComponent> theInstance) { 2079 this.instance = theInstance; 2080 return this; 2081 } 2082 2083 public boolean hasInstance() { 2084 if (this.instance == null) 2085 return false; 2086 for (NutritionProductInstanceComponent item : this.instance) 2087 if (!item.isEmpty()) 2088 return true; 2089 return false; 2090 } 2091 2092 public NutritionProductInstanceComponent addInstance() { //3 2093 NutritionProductInstanceComponent t = new NutritionProductInstanceComponent(); 2094 if (this.instance == null) 2095 this.instance = new ArrayList<NutritionProductInstanceComponent>(); 2096 this.instance.add(t); 2097 return t; 2098 } 2099 2100 public NutritionProduct addInstance(NutritionProductInstanceComponent t) { //3 2101 if (t == null) 2102 return this; 2103 if (this.instance == null) 2104 this.instance = new ArrayList<NutritionProductInstanceComponent>(); 2105 this.instance.add(t); 2106 return this; 2107 } 2108 2109 /** 2110 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist {3} 2111 */ 2112 public NutritionProductInstanceComponent getInstanceFirstRep() { 2113 if (getInstance().isEmpty()) { 2114 addInstance(); 2115 } 2116 return getInstance().get(0); 2117 } 2118 2119 /** 2120 * @return {@link #note} (Comments made about the product.) 2121 */ 2122 public List<Annotation> getNote() { 2123 if (this.note == null) 2124 this.note = new ArrayList<Annotation>(); 2125 return this.note; 2126 } 2127 2128 /** 2129 * @return Returns a reference to <code>this</code> for easy method chaining 2130 */ 2131 public NutritionProduct setNote(List<Annotation> theNote) { 2132 this.note = theNote; 2133 return this; 2134 } 2135 2136 public boolean hasNote() { 2137 if (this.note == null) 2138 return false; 2139 for (Annotation item : this.note) 2140 if (!item.isEmpty()) 2141 return true; 2142 return false; 2143 } 2144 2145 public Annotation addNote() { //3 2146 Annotation t = new Annotation(); 2147 if (this.note == null) 2148 this.note = new ArrayList<Annotation>(); 2149 this.note.add(t); 2150 return t; 2151 } 2152 2153 public NutritionProduct addNote(Annotation t) { //3 2154 if (t == null) 2155 return this; 2156 if (this.note == null) 2157 this.note = new ArrayList<Annotation>(); 2158 this.note.add(t); 2159 return this; 2160 } 2161 2162 /** 2163 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2164 */ 2165 public Annotation getNoteFirstRep() { 2166 if (getNote().isEmpty()) { 2167 addNote(); 2168 } 2169 return getNote().get(0); 2170 } 2171 2172 protected void listChildren(List<Property> children) { 2173 super.listChildren(children); 2174 children.add(new Property("code", "CodeableConcept", "The code assigned to the product, for example a USDA NDB number, a USDA FDC ID number, or a Langual code.", 0, 1, code)); 2175 children.add(new Property("status", "code", "The current state of the product.", 0, 1, status)); 2176 children.add(new Property("category", "CodeableConcept", "Nutrition products can have different classifications - according to its nutritional properties, preparation methods, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 2177 children.add(new Property("manufacturer", "Reference(Organization)", "The organisation (manufacturer, representative or legal authorization holder) that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, manufacturer)); 2178 children.add(new Property("nutrient", "", "The product's nutritional information expressed by the nutrients.", 0, java.lang.Integer.MAX_VALUE, nutrient)); 2179 children.add(new Property("ingredient", "", "Ingredients contained in this product.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 2180 children.add(new Property("knownAllergen", "CodeableReference(Substance)", "Allergens that are known or suspected to be a part of this nutrition product.", 0, java.lang.Integer.MAX_VALUE, knownAllergen)); 2181 children.add(new Property("characteristic", "", "Specifies descriptive properties of the nutrition product.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 2182 children.add(new Property("instance", "", "Conveys instance-level information about this product item. One or several physical, countable instances or occurrences of the product.", 0, java.lang.Integer.MAX_VALUE, instance)); 2183 children.add(new Property("note", "Annotation", "Comments made about the product.", 0, java.lang.Integer.MAX_VALUE, note)); 2184 } 2185 2186 @Override 2187 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2188 switch (_hash) { 2189 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The code assigned to the product, for example a USDA NDB number, a USDA FDC ID number, or a Langual code.", 0, 1, code); 2190 case -892481550: /*status*/ return new Property("status", "code", "The current state of the product.", 0, 1, status); 2191 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Nutrition products can have different classifications - according to its nutritional properties, preparation methods, etc.", 0, java.lang.Integer.MAX_VALUE, category); 2192 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "The organisation (manufacturer, representative or legal authorization holder) that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 2193 case -1671151641: /*nutrient*/ return new Property("nutrient", "", "The product's nutritional information expressed by the nutrients.", 0, java.lang.Integer.MAX_VALUE, nutrient); 2194 case -206409263: /*ingredient*/ return new Property("ingredient", "", "Ingredients contained in this product.", 0, java.lang.Integer.MAX_VALUE, ingredient); 2195 case 1093336805: /*knownAllergen*/ return new Property("knownAllergen", "CodeableReference(Substance)", "Allergens that are known or suspected to be a part of this nutrition product.", 0, java.lang.Integer.MAX_VALUE, knownAllergen); 2196 case 366313883: /*characteristic*/ return new Property("characteristic", "", "Specifies descriptive properties of the nutrition product.", 0, java.lang.Integer.MAX_VALUE, characteristic); 2197 case 555127957: /*instance*/ return new Property("instance", "", "Conveys instance-level information about this product item. One or several physical, countable instances or occurrences of the product.", 0, java.lang.Integer.MAX_VALUE, instance); 2198 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the product.", 0, java.lang.Integer.MAX_VALUE, note); 2199 default: return super.getNamedProperty(_hash, _name, _checkValid); 2200 } 2201 2202 } 2203 2204 @Override 2205 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2206 switch (hash) { 2207 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2208 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<NutritionProductStatus> 2209 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2210 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 2211 case -1671151641: /*nutrient*/ return this.nutrient == null ? new Base[0] : this.nutrient.toArray(new Base[this.nutrient.size()]); // NutritionProductNutrientComponent 2212 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // NutritionProductIngredientComponent 2213 case 1093336805: /*knownAllergen*/ return this.knownAllergen == null ? new Base[0] : this.knownAllergen.toArray(new Base[this.knownAllergen.size()]); // CodeableReference 2214 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // NutritionProductCharacteristicComponent 2215 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // NutritionProductInstanceComponent 2216 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2217 default: return super.getProperty(hash, name, checkValid); 2218 } 2219 2220 } 2221 2222 @Override 2223 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2224 switch (hash) { 2225 case 3059181: // code 2226 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2227 return value; 2228 case -892481550: // status 2229 value = new NutritionProductStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2230 this.status = (Enumeration) value; // Enumeration<NutritionProductStatus> 2231 return value; 2232 case 50511102: // category 2233 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2234 return value; 2235 case -1969347631: // manufacturer 2236 this.getManufacturer().add(TypeConvertor.castToReference(value)); // Reference 2237 return value; 2238 case -1671151641: // nutrient 2239 this.getNutrient().add((NutritionProductNutrientComponent) value); // NutritionProductNutrientComponent 2240 return value; 2241 case -206409263: // ingredient 2242 this.getIngredient().add((NutritionProductIngredientComponent) value); // NutritionProductIngredientComponent 2243 return value; 2244 case 1093336805: // knownAllergen 2245 this.getKnownAllergen().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2246 return value; 2247 case 366313883: // characteristic 2248 this.getCharacteristic().add((NutritionProductCharacteristicComponent) value); // NutritionProductCharacteristicComponent 2249 return value; 2250 case 555127957: // instance 2251 this.getInstance().add((NutritionProductInstanceComponent) value); // NutritionProductInstanceComponent 2252 return value; 2253 case 3387378: // note 2254 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2255 return value; 2256 default: return super.setProperty(hash, name, value); 2257 } 2258 2259 } 2260 2261 @Override 2262 public Base setProperty(String name, Base value) throws FHIRException { 2263 if (name.equals("code")) { 2264 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2265 } else if (name.equals("status")) { 2266 value = new NutritionProductStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2267 this.status = (Enumeration) value; // Enumeration<NutritionProductStatus> 2268 } else if (name.equals("category")) { 2269 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2270 } else if (name.equals("manufacturer")) { 2271 this.getManufacturer().add(TypeConvertor.castToReference(value)); 2272 } else if (name.equals("nutrient")) { 2273 this.getNutrient().add((NutritionProductNutrientComponent) value); 2274 } else if (name.equals("ingredient")) { 2275 this.getIngredient().add((NutritionProductIngredientComponent) value); 2276 } else if (name.equals("knownAllergen")) { 2277 this.getKnownAllergen().add(TypeConvertor.castToCodeableReference(value)); 2278 } else if (name.equals("characteristic")) { 2279 this.getCharacteristic().add((NutritionProductCharacteristicComponent) value); 2280 } else if (name.equals("instance")) { 2281 this.getInstance().add((NutritionProductInstanceComponent) value); 2282 } else if (name.equals("note")) { 2283 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2284 } else 2285 return super.setProperty(name, value); 2286 return value; 2287 } 2288 2289 @Override 2290 public void removeChild(String name, Base value) throws FHIRException { 2291 if (name.equals("code")) { 2292 this.code = null; 2293 } else if (name.equals("status")) { 2294 value = new NutritionProductStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2295 this.status = (Enumeration) value; // Enumeration<NutritionProductStatus> 2296 } else if (name.equals("category")) { 2297 this.getCategory().remove(value); 2298 } else if (name.equals("manufacturer")) { 2299 this.getManufacturer().remove(value); 2300 } else if (name.equals("nutrient")) { 2301 this.getNutrient().remove((NutritionProductNutrientComponent) value); 2302 } else if (name.equals("ingredient")) { 2303 this.getIngredient().remove((NutritionProductIngredientComponent) value); 2304 } else if (name.equals("knownAllergen")) { 2305 this.getKnownAllergen().remove(value); 2306 } else if (name.equals("characteristic")) { 2307 this.getCharacteristic().remove((NutritionProductCharacteristicComponent) value); 2308 } else if (name.equals("instance")) { 2309 this.getInstance().remove((NutritionProductInstanceComponent) value); 2310 } else if (name.equals("note")) { 2311 this.getNote().remove(value); 2312 } else 2313 super.removeChild(name, value); 2314 2315 } 2316 2317 @Override 2318 public Base makeProperty(int hash, String name) throws FHIRException { 2319 switch (hash) { 2320 case 3059181: return getCode(); 2321 case -892481550: return getStatusElement(); 2322 case 50511102: return addCategory(); 2323 case -1969347631: return addManufacturer(); 2324 case -1671151641: return addNutrient(); 2325 case -206409263: return addIngredient(); 2326 case 1093336805: return addKnownAllergen(); 2327 case 366313883: return addCharacteristic(); 2328 case 555127957: return addInstance(); 2329 case 3387378: return addNote(); 2330 default: return super.makeProperty(hash, name); 2331 } 2332 2333 } 2334 2335 @Override 2336 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2337 switch (hash) { 2338 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2339 case -892481550: /*status*/ return new String[] {"code"}; 2340 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2341 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 2342 case -1671151641: /*nutrient*/ return new String[] {}; 2343 case -206409263: /*ingredient*/ return new String[] {}; 2344 case 1093336805: /*knownAllergen*/ return new String[] {"CodeableReference"}; 2345 case 366313883: /*characteristic*/ return new String[] {}; 2346 case 555127957: /*instance*/ return new String[] {}; 2347 case 3387378: /*note*/ return new String[] {"Annotation"}; 2348 default: return super.getTypesForProperty(hash, name); 2349 } 2350 2351 } 2352 2353 @Override 2354 public Base addChild(String name) throws FHIRException { 2355 if (name.equals("code")) { 2356 this.code = new CodeableConcept(); 2357 return this.code; 2358 } 2359 else if (name.equals("status")) { 2360 throw new FHIRException("Cannot call addChild on a singleton property NutritionProduct.status"); 2361 } 2362 else if (name.equals("category")) { 2363 return addCategory(); 2364 } 2365 else if (name.equals("manufacturer")) { 2366 return addManufacturer(); 2367 } 2368 else if (name.equals("nutrient")) { 2369 return addNutrient(); 2370 } 2371 else if (name.equals("ingredient")) { 2372 return addIngredient(); 2373 } 2374 else if (name.equals("knownAllergen")) { 2375 return addKnownAllergen(); 2376 } 2377 else if (name.equals("characteristic")) { 2378 return addCharacteristic(); 2379 } 2380 else if (name.equals("instance")) { 2381 return addInstance(); 2382 } 2383 else if (name.equals("note")) { 2384 return addNote(); 2385 } 2386 else 2387 return super.addChild(name); 2388 } 2389 2390 public String fhirType() { 2391 return "NutritionProduct"; 2392 2393 } 2394 2395 public NutritionProduct copy() { 2396 NutritionProduct dst = new NutritionProduct(); 2397 copyValues(dst); 2398 return dst; 2399 } 2400 2401 public void copyValues(NutritionProduct dst) { 2402 super.copyValues(dst); 2403 dst.code = code == null ? null : code.copy(); 2404 dst.status = status == null ? null : status.copy(); 2405 if (category != null) { 2406 dst.category = new ArrayList<CodeableConcept>(); 2407 for (CodeableConcept i : category) 2408 dst.category.add(i.copy()); 2409 }; 2410 if (manufacturer != null) { 2411 dst.manufacturer = new ArrayList<Reference>(); 2412 for (Reference i : manufacturer) 2413 dst.manufacturer.add(i.copy()); 2414 }; 2415 if (nutrient != null) { 2416 dst.nutrient = new ArrayList<NutritionProductNutrientComponent>(); 2417 for (NutritionProductNutrientComponent i : nutrient) 2418 dst.nutrient.add(i.copy()); 2419 }; 2420 if (ingredient != null) { 2421 dst.ingredient = new ArrayList<NutritionProductIngredientComponent>(); 2422 for (NutritionProductIngredientComponent i : ingredient) 2423 dst.ingredient.add(i.copy()); 2424 }; 2425 if (knownAllergen != null) { 2426 dst.knownAllergen = new ArrayList<CodeableReference>(); 2427 for (CodeableReference i : knownAllergen) 2428 dst.knownAllergen.add(i.copy()); 2429 }; 2430 if (characteristic != null) { 2431 dst.characteristic = new ArrayList<NutritionProductCharacteristicComponent>(); 2432 for (NutritionProductCharacteristicComponent i : characteristic) 2433 dst.characteristic.add(i.copy()); 2434 }; 2435 if (instance != null) { 2436 dst.instance = new ArrayList<NutritionProductInstanceComponent>(); 2437 for (NutritionProductInstanceComponent i : instance) 2438 dst.instance.add(i.copy()); 2439 }; 2440 if (note != null) { 2441 dst.note = new ArrayList<Annotation>(); 2442 for (Annotation i : note) 2443 dst.note.add(i.copy()); 2444 }; 2445 } 2446 2447 protected NutritionProduct typedCopy() { 2448 return copy(); 2449 } 2450 2451 @Override 2452 public boolean equalsDeep(Base other_) { 2453 if (!super.equalsDeep(other_)) 2454 return false; 2455 if (!(other_ instanceof NutritionProduct)) 2456 return false; 2457 NutritionProduct o = (NutritionProduct) other_; 2458 return compareDeep(code, o.code, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 2459 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(nutrient, o.nutrient, true) && compareDeep(ingredient, o.ingredient, true) 2460 && compareDeep(knownAllergen, o.knownAllergen, true) && compareDeep(characteristic, o.characteristic, true) 2461 && compareDeep(instance, o.instance, true) && compareDeep(note, o.note, true); 2462 } 2463 2464 @Override 2465 public boolean equalsShallow(Base other_) { 2466 if (!super.equalsShallow(other_)) 2467 return false; 2468 if (!(other_ instanceof NutritionProduct)) 2469 return false; 2470 NutritionProduct o = (NutritionProduct) other_; 2471 return compareValues(status, o.status, true); 2472 } 2473 2474 public boolean isEmpty() { 2475 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, status, category, manufacturer 2476 , nutrient, ingredient, knownAllergen, characteristic, instance, note); 2477 } 2478 2479 @Override 2480 public ResourceType getResourceType() { 2481 return ResourceType.NutritionProduct; 2482 } 2483 2484 /** 2485 * Search parameter: <b>code</b> 2486 * <p> 2487 * Description: <b>The code identifying a specific nutrition product</b><br> 2488 * Type: <b>token</b><br> 2489 * Path: <b>NutritionProduct.code</b><br> 2490 * </p> 2491 */ 2492 @SearchParamDefinition(name="code", path="NutritionProduct.code", description="The code identifying a specific nutrition product", type="token" ) 2493 public static final String SP_CODE = "code"; 2494 /** 2495 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2496 * <p> 2497 * Description: <b>The code identifying a specific nutrition product</b><br> 2498 * Type: <b>token</b><br> 2499 * Path: <b>NutritionProduct.code</b><br> 2500 * </p> 2501 */ 2502 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2503 2504 /** 2505 * Search parameter: <b>identifier</b> 2506 * <p> 2507 * Description: <b>The identifier for the physical instance, typically a serial number</b><br> 2508 * Type: <b>token</b><br> 2509 * Path: <b>NutritionProduct.instance.identifier</b><br> 2510 * </p> 2511 */ 2512 @SearchParamDefinition(name="identifier", path="NutritionProduct.instance.identifier", description="The identifier for the physical instance, typically a serial number", type="token" ) 2513 public static final String SP_IDENTIFIER = "identifier"; 2514 /** 2515 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2516 * <p> 2517 * Description: <b>The identifier for the physical instance, typically a serial number</b><br> 2518 * Type: <b>token</b><br> 2519 * Path: <b>NutritionProduct.instance.identifier</b><br> 2520 * </p> 2521 */ 2522 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2523 2524 /** 2525 * Search parameter: <b>lot-number</b> 2526 * <p> 2527 * Description: <b>The lot number</b><br> 2528 * Type: <b>token</b><br> 2529 * Path: <b>NutritionProduct.instance.lotNumber</b><br> 2530 * </p> 2531 */ 2532 @SearchParamDefinition(name="lot-number", path="NutritionProduct.instance.lotNumber", description="The lot number", type="token" ) 2533 public static final String SP_LOT_NUMBER = "lot-number"; 2534 /** 2535 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 2536 * <p> 2537 * Description: <b>The lot number</b><br> 2538 * Type: <b>token</b><br> 2539 * Path: <b>NutritionProduct.instance.lotNumber</b><br> 2540 * </p> 2541 */ 2542 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LOT_NUMBER); 2543 2544 /** 2545 * Search parameter: <b>serial-number</b> 2546 * <p> 2547 * Description: <b>The serial number</b><br> 2548 * Type: <b>token</b><br> 2549 * Path: <b>NutritionProduct.instance.identifier</b><br> 2550 * </p> 2551 */ 2552 @SearchParamDefinition(name="serial-number", path="NutritionProduct.instance.identifier", description="The serial number", type="token" ) 2553 public static final String SP_SERIAL_NUMBER = "serial-number"; 2554 /** 2555 * <b>Fluent Client</b> search parameter constant for <b>serial-number</b> 2556 * <p> 2557 * Description: <b>The serial number</b><br> 2558 * Type: <b>token</b><br> 2559 * Path: <b>NutritionProduct.instance.identifier</b><br> 2560 * </p> 2561 */ 2562 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIAL_NUMBER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERIAL_NUMBER); 2563 2564 /** 2565 * Search parameter: <b>status</b> 2566 * <p> 2567 * Description: <b>The broad product group or category of the nutrition product</b><br> 2568 * Type: <b>token</b><br> 2569 * Path: <b>NutritionProduct.category</b><br> 2570 * </p> 2571 */ 2572 @SearchParamDefinition(name="status", path="NutritionProduct.category", description="The broad product group or category of the nutrition product", type="token" ) 2573 public static final String SP_STATUS = "status"; 2574 /** 2575 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2576 * <p> 2577 * Description: <b>The broad product group or category of the nutrition product</b><br> 2578 * Type: <b>token</b><br> 2579 * Path: <b>NutritionProduct.category</b><br> 2580 * </p> 2581 */ 2582 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2583 2584 2585} 2586