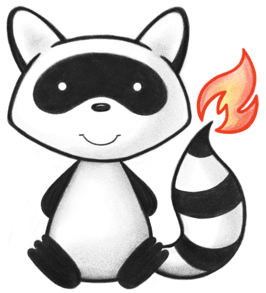
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Measurements and simple assertions made about a patient, device or other subject. 052 */ 053@ResourceDef(name="Observation", profile="http://hl7.org/fhir/StructureDefinition/Observation") 054public class Observation extends DomainResource { 055 056 public enum TriggeredBytype { 057 /** 058 * Performance of one or more other tests depending on the results of the initial test. This may include collection of additional specimen. While a new ServiceRequest is not required to perform the additional test, where it is still needed (e.g., requesting another laboratory to perform the reflex test), the Observation.basedOn would reference the new ServiceRequest that requested the additional test to be performed as well as the original ServiceRequest to reflect the one that provided the authorization. 059 */ 060 REFLEX, 061 /** 062 * Performance of the same test again with the same parameters/settings/solution. 063 */ 064 REPEAT, 065 /** 066 * Performance of the same test but with different parameters/settings/solution. 067 */ 068 RERUN, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static TriggeredBytype fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("reflex".equals(codeString)) 077 return REFLEX; 078 if ("repeat".equals(codeString)) 079 return REPEAT; 080 if ("re-run".equals(codeString)) 081 return RERUN; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown TriggeredBytype code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case REFLEX: return "reflex"; 090 case REPEAT: return "repeat"; 091 case RERUN: return "re-run"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case REFLEX: return "http://hl7.org/fhir/observation-triggeredbytype"; 099 case REPEAT: return "http://hl7.org/fhir/observation-triggeredbytype"; 100 case RERUN: return "http://hl7.org/fhir/observation-triggeredbytype"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case REFLEX: return "Performance of one or more other tests depending on the results of the initial test. This may include collection of additional specimen. While a new ServiceRequest is not required to perform the additional test, where it is still needed (e.g., requesting another laboratory to perform the reflex test), the Observation.basedOn would reference the new ServiceRequest that requested the additional test to be performed as well as the original ServiceRequest to reflect the one that provided the authorization."; 108 case REPEAT: return "Performance of the same test again with the same parameters/settings/solution."; 109 case RERUN: return "Performance of the same test but with different parameters/settings/solution."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case REFLEX: return "Reflex"; 117 case REPEAT: return "Repeat (per policy)"; 118 case RERUN: return "Re-run (per policy)"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class TriggeredBytypeEnumFactory implements EnumFactory<TriggeredBytype> { 126 public TriggeredBytype fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("reflex".equals(codeString)) 131 return TriggeredBytype.REFLEX; 132 if ("repeat".equals(codeString)) 133 return TriggeredBytype.REPEAT; 134 if ("re-run".equals(codeString)) 135 return TriggeredBytype.RERUN; 136 throw new IllegalArgumentException("Unknown TriggeredBytype code '"+codeString+"'"); 137 } 138 public Enumeration<TriggeredBytype> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.NULL, code); 146 if ("reflex".equals(codeString)) 147 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.REFLEX, code); 148 if ("repeat".equals(codeString)) 149 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.REPEAT, code); 150 if ("re-run".equals(codeString)) 151 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.RERUN, code); 152 throw new FHIRException("Unknown TriggeredBytype code '"+codeString+"'"); 153 } 154 public String toCode(TriggeredBytype code) { 155 if (code == TriggeredBytype.NULL) 156 return null; 157 if (code == TriggeredBytype.REFLEX) 158 return "reflex"; 159 if (code == TriggeredBytype.REPEAT) 160 return "repeat"; 161 if (code == TriggeredBytype.RERUN) 162 return "re-run"; 163 return "?"; 164 } 165 public String toSystem(TriggeredBytype code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class ObservationTriggeredByComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * Reference to the triggering observation. 174 */ 175 @Child(name = "observation", type = {Observation.class}, order=1, min=1, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="Triggering observation", formalDefinition="Reference to the triggering observation." ) 177 protected Reference observation; 178 179 /** 180 * The type of trigger. 181Reflex | Repeat | Re-run. 182 */ 183 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 184 @Description(shortDefinition="reflex | repeat | re-run", formalDefinition="The type of trigger.\nReflex | Repeat | Re-run." ) 185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-triggeredbytype") 186 protected Enumeration<TriggeredBytype> type; 187 188 /** 189 * Provides the reason why this observation was performed as a result of the observation(s) referenced. 190 */ 191 @Child(name = "reason", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 192 @Description(shortDefinition="Reason that the observation was triggered", formalDefinition="Provides the reason why this observation was performed as a result of the observation(s) referenced." ) 193 protected StringType reason; 194 195 private static final long serialVersionUID = -737822241L; 196 197 /** 198 * Constructor 199 */ 200 public ObservationTriggeredByComponent() { 201 super(); 202 } 203 204 /** 205 * Constructor 206 */ 207 public ObservationTriggeredByComponent(Reference observation, TriggeredBytype type) { 208 super(); 209 this.setObservation(observation); 210 this.setType(type); 211 } 212 213 /** 214 * @return {@link #observation} (Reference to the triggering observation.) 215 */ 216 public Reference getObservation() { 217 if (this.observation == null) 218 if (Configuration.errorOnAutoCreate()) 219 throw new Error("Attempt to auto-create ObservationTriggeredByComponent.observation"); 220 else if (Configuration.doAutoCreate()) 221 this.observation = new Reference(); // cc 222 return this.observation; 223 } 224 225 public boolean hasObservation() { 226 return this.observation != null && !this.observation.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #observation} (Reference to the triggering observation.) 231 */ 232 public ObservationTriggeredByComponent setObservation(Reference value) { 233 this.observation = value; 234 return this; 235 } 236 237 /** 238 * @return {@link #type} (The type of trigger. 239Reflex | Repeat | Re-run.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 240 */ 241 public Enumeration<TriggeredBytype> getTypeElement() { 242 if (this.type == null) 243 if (Configuration.errorOnAutoCreate()) 244 throw new Error("Attempt to auto-create ObservationTriggeredByComponent.type"); 245 else if (Configuration.doAutoCreate()) 246 this.type = new Enumeration<TriggeredBytype>(new TriggeredBytypeEnumFactory()); // bb 247 return this.type; 248 } 249 250 public boolean hasTypeElement() { 251 return this.type != null && !this.type.isEmpty(); 252 } 253 254 public boolean hasType() { 255 return this.type != null && !this.type.isEmpty(); 256 } 257 258 /** 259 * @param value {@link #type} (The type of trigger. 260Reflex | Repeat | Re-run.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 261 */ 262 public ObservationTriggeredByComponent setTypeElement(Enumeration<TriggeredBytype> value) { 263 this.type = value; 264 return this; 265 } 266 267 /** 268 * @return The type of trigger. 269Reflex | Repeat | Re-run. 270 */ 271 public TriggeredBytype getType() { 272 return this.type == null ? null : this.type.getValue(); 273 } 274 275 /** 276 * @param value The type of trigger. 277Reflex | Repeat | Re-run. 278 */ 279 public ObservationTriggeredByComponent setType(TriggeredBytype value) { 280 if (this.type == null) 281 this.type = new Enumeration<TriggeredBytype>(new TriggeredBytypeEnumFactory()); 282 this.type.setValue(value); 283 return this; 284 } 285 286 /** 287 * @return {@link #reason} (Provides the reason why this observation was performed as a result of the observation(s) referenced.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 288 */ 289 public StringType getReasonElement() { 290 if (this.reason == null) 291 if (Configuration.errorOnAutoCreate()) 292 throw new Error("Attempt to auto-create ObservationTriggeredByComponent.reason"); 293 else if (Configuration.doAutoCreate()) 294 this.reason = new StringType(); // bb 295 return this.reason; 296 } 297 298 public boolean hasReasonElement() { 299 return this.reason != null && !this.reason.isEmpty(); 300 } 301 302 public boolean hasReason() { 303 return this.reason != null && !this.reason.isEmpty(); 304 } 305 306 /** 307 * @param value {@link #reason} (Provides the reason why this observation was performed as a result of the observation(s) referenced.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 308 */ 309 public ObservationTriggeredByComponent setReasonElement(StringType value) { 310 this.reason = value; 311 return this; 312 } 313 314 /** 315 * @return Provides the reason why this observation was performed as a result of the observation(s) referenced. 316 */ 317 public String getReason() { 318 return this.reason == null ? null : this.reason.getValue(); 319 } 320 321 /** 322 * @param value Provides the reason why this observation was performed as a result of the observation(s) referenced. 323 */ 324 public ObservationTriggeredByComponent setReason(String value) { 325 if (Utilities.noString(value)) 326 this.reason = null; 327 else { 328 if (this.reason == null) 329 this.reason = new StringType(); 330 this.reason.setValue(value); 331 } 332 return this; 333 } 334 335 protected void listChildren(List<Property> children) { 336 super.listChildren(children); 337 children.add(new Property("observation", "Reference(Observation)", "Reference to the triggering observation.", 0, 1, observation)); 338 children.add(new Property("type", "code", "The type of trigger.\nReflex | Repeat | Re-run.", 0, 1, type)); 339 children.add(new Property("reason", "string", "Provides the reason why this observation was performed as a result of the observation(s) referenced.", 0, 1, reason)); 340 } 341 342 @Override 343 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 344 switch (_hash) { 345 case 122345516: /*observation*/ return new Property("observation", "Reference(Observation)", "Reference to the triggering observation.", 0, 1, observation); 346 case 3575610: /*type*/ return new Property("type", "code", "The type of trigger.\nReflex | Repeat | Re-run.", 0, 1, type); 347 case -934964668: /*reason*/ return new Property("reason", "string", "Provides the reason why this observation was performed as a result of the observation(s) referenced.", 0, 1, reason); 348 default: return super.getNamedProperty(_hash, _name, _checkValid); 349 } 350 351 } 352 353 @Override 354 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 355 switch (hash) { 356 case 122345516: /*observation*/ return this.observation == null ? new Base[0] : new Base[] {this.observation}; // Reference 357 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TriggeredBytype> 358 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // StringType 359 default: return super.getProperty(hash, name, checkValid); 360 } 361 362 } 363 364 @Override 365 public Base setProperty(int hash, String name, Base value) throws FHIRException { 366 switch (hash) { 367 case 122345516: // observation 368 this.observation = TypeConvertor.castToReference(value); // Reference 369 return value; 370 case 3575610: // type 371 value = new TriggeredBytypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 372 this.type = (Enumeration) value; // Enumeration<TriggeredBytype> 373 return value; 374 case -934964668: // reason 375 this.reason = TypeConvertor.castToString(value); // StringType 376 return value; 377 default: return super.setProperty(hash, name, value); 378 } 379 380 } 381 382 @Override 383 public Base setProperty(String name, Base value) throws FHIRException { 384 if (name.equals("observation")) { 385 this.observation = TypeConvertor.castToReference(value); // Reference 386 } else if (name.equals("type")) { 387 value = new TriggeredBytypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 388 this.type = (Enumeration) value; // Enumeration<TriggeredBytype> 389 } else if (name.equals("reason")) { 390 this.reason = TypeConvertor.castToString(value); // StringType 391 } else 392 return super.setProperty(name, value); 393 return value; 394 } 395 396 @Override 397 public void removeChild(String name, Base value) throws FHIRException { 398 if (name.equals("observation")) { 399 this.observation = null; 400 } else if (name.equals("type")) { 401 value = new TriggeredBytypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 402 this.type = (Enumeration) value; // Enumeration<TriggeredBytype> 403 } else if (name.equals("reason")) { 404 this.reason = null; 405 } else 406 super.removeChild(name, value); 407 408 } 409 410 @Override 411 public Base makeProperty(int hash, String name) throws FHIRException { 412 switch (hash) { 413 case 122345516: return getObservation(); 414 case 3575610: return getTypeElement(); 415 case -934964668: return getReasonElement(); 416 default: return super.makeProperty(hash, name); 417 } 418 419 } 420 421 @Override 422 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 423 switch (hash) { 424 case 122345516: /*observation*/ return new String[] {"Reference"}; 425 case 3575610: /*type*/ return new String[] {"code"}; 426 case -934964668: /*reason*/ return new String[] {"string"}; 427 default: return super.getTypesForProperty(hash, name); 428 } 429 430 } 431 432 @Override 433 public Base addChild(String name) throws FHIRException { 434 if (name.equals("observation")) { 435 this.observation = new Reference(); 436 return this.observation; 437 } 438 else if (name.equals("type")) { 439 throw new FHIRException("Cannot call addChild on a singleton property Observation.triggeredBy.type"); 440 } 441 else if (name.equals("reason")) { 442 throw new FHIRException("Cannot call addChild on a singleton property Observation.triggeredBy.reason"); 443 } 444 else 445 return super.addChild(name); 446 } 447 448 public ObservationTriggeredByComponent copy() { 449 ObservationTriggeredByComponent dst = new ObservationTriggeredByComponent(); 450 copyValues(dst); 451 return dst; 452 } 453 454 public void copyValues(ObservationTriggeredByComponent dst) { 455 super.copyValues(dst); 456 dst.observation = observation == null ? null : observation.copy(); 457 dst.type = type == null ? null : type.copy(); 458 dst.reason = reason == null ? null : reason.copy(); 459 } 460 461 @Override 462 public boolean equalsDeep(Base other_) { 463 if (!super.equalsDeep(other_)) 464 return false; 465 if (!(other_ instanceof ObservationTriggeredByComponent)) 466 return false; 467 ObservationTriggeredByComponent o = (ObservationTriggeredByComponent) other_; 468 return compareDeep(observation, o.observation, true) && compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true) 469 ; 470 } 471 472 @Override 473 public boolean equalsShallow(Base other_) { 474 if (!super.equalsShallow(other_)) 475 return false; 476 if (!(other_ instanceof ObservationTriggeredByComponent)) 477 return false; 478 ObservationTriggeredByComponent o = (ObservationTriggeredByComponent) other_; 479 return compareValues(type, o.type, true) && compareValues(reason, o.reason, true); 480 } 481 482 public boolean isEmpty() { 483 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(observation, type, reason 484 ); 485 } 486 487 public String fhirType() { 488 return "Observation.triggeredBy"; 489 490 } 491 492 } 493 494 @Block() 495 public static class ObservationReferenceRangeComponent extends BackboneElement implements IBaseBackboneElement { 496 /** 497 * The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3). 498 */ 499 @Child(name = "low", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 500 @Description(shortDefinition="Low Range, if relevant", formalDefinition="The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3)." ) 501 protected Quantity low; 502 503 /** 504 * The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3). 505 */ 506 @Child(name = "high", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 507 @Description(shortDefinition="High Range, if relevant", formalDefinition="The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3)." ) 508 protected Quantity high; 509 510 /** 511 * The value of the normal value of the reference range. 512 */ 513 @Child(name = "normalValue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 514 @Description(shortDefinition="Normal value, if relevant", formalDefinition="The value of the normal value of the reference range." ) 515 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-referencerange-normalvalue") 516 protected CodeableConcept normalValue; 517 518 /** 519 * Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range. 520 */ 521 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 522 @Description(shortDefinition="Reference range qualifier", formalDefinition="Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range." ) 523 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-meaning") 524 protected CodeableConcept type; 525 526 /** 527 * Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an "AND" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used. 528 */ 529 @Child(name = "appliesTo", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 530 @Description(shortDefinition="Reference range population", formalDefinition="Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used." ) 531 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-appliesto") 532 protected List<CodeableConcept> appliesTo; 533 534 /** 535 * The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so. 536 */ 537 @Child(name = "age", type = {Range.class}, order=6, min=0, max=1, modifier=false, summary=false) 538 @Description(shortDefinition="Applicable age range, if relevant", formalDefinition="The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so." ) 539 protected Range age; 540 541 /** 542 * Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals". 543 */ 544 @Child(name = "text", type = {MarkdownType.class}, order=7, min=0, max=1, modifier=false, summary=false) 545 @Description(shortDefinition="Text based reference range in an observation", formalDefinition="Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\"." ) 546 protected MarkdownType text; 547 548 private static final long serialVersionUID = 470232080L; 549 550 /** 551 * Constructor 552 */ 553 public ObservationReferenceRangeComponent() { 554 super(); 555 } 556 557 /** 558 * @return {@link #low} (The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).) 559 */ 560 public Quantity getLow() { 561 if (this.low == null) 562 if (Configuration.errorOnAutoCreate()) 563 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.low"); 564 else if (Configuration.doAutoCreate()) 565 this.low = new Quantity(); // cc 566 return this.low; 567 } 568 569 public boolean hasLow() { 570 return this.low != null && !this.low.isEmpty(); 571 } 572 573 /** 574 * @param value {@link #low} (The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).) 575 */ 576 public ObservationReferenceRangeComponent setLow(Quantity value) { 577 this.low = value; 578 return this; 579 } 580 581 /** 582 * @return {@link #high} (The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).) 583 */ 584 public Quantity getHigh() { 585 if (this.high == null) 586 if (Configuration.errorOnAutoCreate()) 587 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.high"); 588 else if (Configuration.doAutoCreate()) 589 this.high = new Quantity(); // cc 590 return this.high; 591 } 592 593 public boolean hasHigh() { 594 return this.high != null && !this.high.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #high} (The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).) 599 */ 600 public ObservationReferenceRangeComponent setHigh(Quantity value) { 601 this.high = value; 602 return this; 603 } 604 605 /** 606 * @return {@link #normalValue} (The value of the normal value of the reference range.) 607 */ 608 public CodeableConcept getNormalValue() { 609 if (this.normalValue == null) 610 if (Configuration.errorOnAutoCreate()) 611 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.normalValue"); 612 else if (Configuration.doAutoCreate()) 613 this.normalValue = new CodeableConcept(); // cc 614 return this.normalValue; 615 } 616 617 public boolean hasNormalValue() { 618 return this.normalValue != null && !this.normalValue.isEmpty(); 619 } 620 621 /** 622 * @param value {@link #normalValue} (The value of the normal value of the reference range.) 623 */ 624 public ObservationReferenceRangeComponent setNormalValue(CodeableConcept value) { 625 this.normalValue = value; 626 return this; 627 } 628 629 /** 630 * @return {@link #type} (Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.) 631 */ 632 public CodeableConcept getType() { 633 if (this.type == null) 634 if (Configuration.errorOnAutoCreate()) 635 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.type"); 636 else if (Configuration.doAutoCreate()) 637 this.type = new CodeableConcept(); // cc 638 return this.type; 639 } 640 641 public boolean hasType() { 642 return this.type != null && !this.type.isEmpty(); 643 } 644 645 /** 646 * @param value {@link #type} (Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.) 647 */ 648 public ObservationReferenceRangeComponent setType(CodeableConcept value) { 649 this.type = value; 650 return this; 651 } 652 653 /** 654 * @return {@link #appliesTo} (Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an "AND" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.) 655 */ 656 public List<CodeableConcept> getAppliesTo() { 657 if (this.appliesTo == null) 658 this.appliesTo = new ArrayList<CodeableConcept>(); 659 return this.appliesTo; 660 } 661 662 /** 663 * @return Returns a reference to <code>this</code> for easy method chaining 664 */ 665 public ObservationReferenceRangeComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 666 this.appliesTo = theAppliesTo; 667 return this; 668 } 669 670 public boolean hasAppliesTo() { 671 if (this.appliesTo == null) 672 return false; 673 for (CodeableConcept item : this.appliesTo) 674 if (!item.isEmpty()) 675 return true; 676 return false; 677 } 678 679 public CodeableConcept addAppliesTo() { //3 680 CodeableConcept t = new CodeableConcept(); 681 if (this.appliesTo == null) 682 this.appliesTo = new ArrayList<CodeableConcept>(); 683 this.appliesTo.add(t); 684 return t; 685 } 686 687 public ObservationReferenceRangeComponent addAppliesTo(CodeableConcept t) { //3 688 if (t == null) 689 return this; 690 if (this.appliesTo == null) 691 this.appliesTo = new ArrayList<CodeableConcept>(); 692 this.appliesTo.add(t); 693 return this; 694 } 695 696 /** 697 * @return The first repetition of repeating field {@link #appliesTo}, creating it if it does not already exist {3} 698 */ 699 public CodeableConcept getAppliesToFirstRep() { 700 if (getAppliesTo().isEmpty()) { 701 addAppliesTo(); 702 } 703 return getAppliesTo().get(0); 704 } 705 706 /** 707 * @return {@link #age} (The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.) 708 */ 709 public Range getAge() { 710 if (this.age == null) 711 if (Configuration.errorOnAutoCreate()) 712 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.age"); 713 else if (Configuration.doAutoCreate()) 714 this.age = new Range(); // cc 715 return this.age; 716 } 717 718 public boolean hasAge() { 719 return this.age != null && !this.age.isEmpty(); 720 } 721 722 /** 723 * @param value {@link #age} (The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.) 724 */ 725 public ObservationReferenceRangeComponent setAge(Range value) { 726 this.age = value; 727 return this; 728 } 729 730 /** 731 * @return {@link #text} (Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals".). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 732 */ 733 public MarkdownType getTextElement() { 734 if (this.text == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.text"); 737 else if (Configuration.doAutoCreate()) 738 this.text = new MarkdownType(); // bb 739 return this.text; 740 } 741 742 public boolean hasTextElement() { 743 return this.text != null && !this.text.isEmpty(); 744 } 745 746 public boolean hasText() { 747 return this.text != null && !this.text.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #text} (Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals".). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 752 */ 753 public ObservationReferenceRangeComponent setTextElement(MarkdownType value) { 754 this.text = value; 755 return this; 756 } 757 758 /** 759 * @return Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals". 760 */ 761 public String getText() { 762 return this.text == null ? null : this.text.getValue(); 763 } 764 765 /** 766 * @param value Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals". 767 */ 768 public ObservationReferenceRangeComponent setText(String value) { 769 if (Utilities.noString(value)) 770 this.text = null; 771 else { 772 if (this.text == null) 773 this.text = new MarkdownType(); 774 this.text.setValue(value); 775 } 776 return this; 777 } 778 779 protected void listChildren(List<Property> children) { 780 super.listChildren(children); 781 children.add(new Property("low", "Quantity", "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 0, 1, low)); 782 children.add(new Property("high", "Quantity", "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 0, 1, high)); 783 children.add(new Property("normalValue", "CodeableConcept", "The value of the normal value of the reference range.", 0, 1, normalValue)); 784 children.add(new Property("type", "CodeableConcept", "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 0, 1, type)); 785 children.add(new Property("appliesTo", "CodeableConcept", "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 0, java.lang.Integer.MAX_VALUE, appliesTo)); 786 children.add(new Property("age", "Range", "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 0, 1, age)); 787 children.add(new Property("text", "markdown", "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 0, 1, text)); 788 } 789 790 @Override 791 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 792 switch (_hash) { 793 case 107348: /*low*/ return new Property("low", "Quantity", "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 0, 1, low); 794 case 3202466: /*high*/ return new Property("high", "Quantity", "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 0, 1, high); 795 case -270017334: /*normalValue*/ return new Property("normalValue", "CodeableConcept", "The value of the normal value of the reference range.", 0, 1, normalValue); 796 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 0, 1, type); 797 case -2089924569: /*appliesTo*/ return new Property("appliesTo", "CodeableConcept", "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 0, java.lang.Integer.MAX_VALUE, appliesTo); 798 case 96511: /*age*/ return new Property("age", "Range", "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 0, 1, age); 799 case 3556653: /*text*/ return new Property("text", "markdown", "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 0, 1, text); 800 default: return super.getNamedProperty(_hash, _name, _checkValid); 801 } 802 803 } 804 805 @Override 806 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 807 switch (hash) { 808 case 107348: /*low*/ return this.low == null ? new Base[0] : new Base[] {this.low}; // Quantity 809 case 3202466: /*high*/ return this.high == null ? new Base[0] : new Base[] {this.high}; // Quantity 810 case -270017334: /*normalValue*/ return this.normalValue == null ? new Base[0] : new Base[] {this.normalValue}; // CodeableConcept 811 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 812 case -2089924569: /*appliesTo*/ return this.appliesTo == null ? new Base[0] : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 813 case 96511: /*age*/ return this.age == null ? new Base[0] : new Base[] {this.age}; // Range 814 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // MarkdownType 815 default: return super.getProperty(hash, name, checkValid); 816 } 817 818 } 819 820 @Override 821 public Base setProperty(int hash, String name, Base value) throws FHIRException { 822 switch (hash) { 823 case 107348: // low 824 this.low = TypeConvertor.castToQuantity(value); // Quantity 825 return value; 826 case 3202466: // high 827 this.high = TypeConvertor.castToQuantity(value); // Quantity 828 return value; 829 case -270017334: // normalValue 830 this.normalValue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 831 return value; 832 case 3575610: // type 833 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 834 return value; 835 case -2089924569: // appliesTo 836 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 837 return value; 838 case 96511: // age 839 this.age = TypeConvertor.castToRange(value); // Range 840 return value; 841 case 3556653: // text 842 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 843 return value; 844 default: return super.setProperty(hash, name, value); 845 } 846 847 } 848 849 @Override 850 public Base setProperty(String name, Base value) throws FHIRException { 851 if (name.equals("low")) { 852 this.low = TypeConvertor.castToQuantity(value); // Quantity 853 } else if (name.equals("high")) { 854 this.high = TypeConvertor.castToQuantity(value); // Quantity 855 } else if (name.equals("normalValue")) { 856 this.normalValue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 857 } else if (name.equals("type")) { 858 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 859 } else if (name.equals("appliesTo")) { 860 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); 861 } else if (name.equals("age")) { 862 this.age = TypeConvertor.castToRange(value); // Range 863 } else if (name.equals("text")) { 864 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 865 } else 866 return super.setProperty(name, value); 867 return value; 868 } 869 870 @Override 871 public void removeChild(String name, Base value) throws FHIRException { 872 if (name.equals("low")) { 873 this.low = null; 874 } else if (name.equals("high")) { 875 this.high = null; 876 } else if (name.equals("normalValue")) { 877 this.normalValue = null; 878 } else if (name.equals("type")) { 879 this.type = null; 880 } else if (name.equals("appliesTo")) { 881 this.getAppliesTo().remove(value); 882 } else if (name.equals("age")) { 883 this.age = null; 884 } else if (name.equals("text")) { 885 this.text = null; 886 } else 887 super.removeChild(name, value); 888 889 } 890 891 @Override 892 public Base makeProperty(int hash, String name) throws FHIRException { 893 switch (hash) { 894 case 107348: return getLow(); 895 case 3202466: return getHigh(); 896 case -270017334: return getNormalValue(); 897 case 3575610: return getType(); 898 case -2089924569: return addAppliesTo(); 899 case 96511: return getAge(); 900 case 3556653: return getTextElement(); 901 default: return super.makeProperty(hash, name); 902 } 903 904 } 905 906 @Override 907 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 908 switch (hash) { 909 case 107348: /*low*/ return new String[] {"Quantity"}; 910 case 3202466: /*high*/ return new String[] {"Quantity"}; 911 case -270017334: /*normalValue*/ return new String[] {"CodeableConcept"}; 912 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 913 case -2089924569: /*appliesTo*/ return new String[] {"CodeableConcept"}; 914 case 96511: /*age*/ return new String[] {"Range"}; 915 case 3556653: /*text*/ return new String[] {"markdown"}; 916 default: return super.getTypesForProperty(hash, name); 917 } 918 919 } 920 921 @Override 922 public Base addChild(String name) throws FHIRException { 923 if (name.equals("low")) { 924 this.low = new Quantity(); 925 return this.low; 926 } 927 else if (name.equals("high")) { 928 this.high = new Quantity(); 929 return this.high; 930 } 931 else if (name.equals("normalValue")) { 932 this.normalValue = new CodeableConcept(); 933 return this.normalValue; 934 } 935 else if (name.equals("type")) { 936 this.type = new CodeableConcept(); 937 return this.type; 938 } 939 else if (name.equals("appliesTo")) { 940 return addAppliesTo(); 941 } 942 else if (name.equals("age")) { 943 this.age = new Range(); 944 return this.age; 945 } 946 else if (name.equals("text")) { 947 throw new FHIRException("Cannot call addChild on a singleton property Observation.referenceRange.text"); 948 } 949 else 950 return super.addChild(name); 951 } 952 953 public ObservationReferenceRangeComponent copy() { 954 ObservationReferenceRangeComponent dst = new ObservationReferenceRangeComponent(); 955 copyValues(dst); 956 return dst; 957 } 958 959 public void copyValues(ObservationReferenceRangeComponent dst) { 960 super.copyValues(dst); 961 dst.low = low == null ? null : low.copy(); 962 dst.high = high == null ? null : high.copy(); 963 dst.normalValue = normalValue == null ? null : normalValue.copy(); 964 dst.type = type == null ? null : type.copy(); 965 if (appliesTo != null) { 966 dst.appliesTo = new ArrayList<CodeableConcept>(); 967 for (CodeableConcept i : appliesTo) 968 dst.appliesTo.add(i.copy()); 969 }; 970 dst.age = age == null ? null : age.copy(); 971 dst.text = text == null ? null : text.copy(); 972 } 973 974 @Override 975 public boolean equalsDeep(Base other_) { 976 if (!super.equalsDeep(other_)) 977 return false; 978 if (!(other_ instanceof ObservationReferenceRangeComponent)) 979 return false; 980 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 981 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true) && compareDeep(normalValue, o.normalValue, true) 982 && compareDeep(type, o.type, true) && compareDeep(appliesTo, o.appliesTo, true) && compareDeep(age, o.age, true) 983 && compareDeep(text, o.text, true); 984 } 985 986 @Override 987 public boolean equalsShallow(Base other_) { 988 if (!super.equalsShallow(other_)) 989 return false; 990 if (!(other_ instanceof ObservationReferenceRangeComponent)) 991 return false; 992 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 993 return compareValues(text, o.text, true); 994 } 995 996 public boolean isEmpty() { 997 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(low, high, normalValue, type 998 , appliesTo, age, text); 999 } 1000 1001 public String fhirType() { 1002 return "Observation.referenceRange"; 1003 1004 } 1005 1006 } 1007 1008 @Block() 1009 public static class ObservationComponentComponent extends BackboneElement implements IBaseBackboneElement { 1010 /** 1011 * Describes what was observed. Sometimes this is called the observation "code". 1012 */ 1013 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1014 @Description(shortDefinition="Type of component observation (code / type)", formalDefinition="Describes what was observed. Sometimes this is called the observation \"code\"." ) 1015 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1016 protected CodeableConcept code; 1017 1018 /** 1019 * The information determined as a result of making the observation, if the information has a simple value. 1020 */ 1021 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, Period.class, Attachment.class, MolecularSequence.class}, order=2, min=0, max=1, modifier=false, summary=true) 1022 @Description(shortDefinition="Actual component result", formalDefinition="The information determined as a result of making the observation, if the information has a simple value." ) 1023 protected DataType value; 1024 1025 /** 1026 * Provides a reason why the expected value in the element Observation.component.value[x] is missing. 1027 */ 1028 @Child(name = "dataAbsentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1029 @Description(shortDefinition="Why the component result is missing", formalDefinition="Provides a reason why the expected value in the element Observation.component.value[x] is missing." ) 1030 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/data-absent-reason") 1031 protected CodeableConcept dataAbsentReason; 1032 1033 /** 1034 * A categorical assessment of an observation value. For example, high, low, normal. 1035 */ 1036 @Child(name = "interpretation", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1037 @Description(shortDefinition="High, low, normal, etc", formalDefinition="A categorical assessment of an observation value. For example, high, low, normal." ) 1038 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-interpretation") 1039 protected List<CodeableConcept> interpretation; 1040 1041 /** 1042 * Guidance on how to interpret the value by comparison to a normal or recommended range. 1043 */ 1044 @Child(name = "referenceRange", type = {ObservationReferenceRangeComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1045 @Description(shortDefinition="Provides guide for interpretation of component result", formalDefinition="Guidance on how to interpret the value by comparison to a normal or recommended range." ) 1046 protected List<ObservationReferenceRangeComponent> referenceRange; 1047 1048 private static final long serialVersionUID = -1771757751L; 1049 1050 /** 1051 * Constructor 1052 */ 1053 public ObservationComponentComponent() { 1054 super(); 1055 } 1056 1057 /** 1058 * Constructor 1059 */ 1060 public ObservationComponentComponent(CodeableConcept code) { 1061 super(); 1062 this.setCode(code); 1063 } 1064 1065 /** 1066 * @return {@link #code} (Describes what was observed. Sometimes this is called the observation "code".) 1067 */ 1068 public CodeableConcept getCode() { 1069 if (this.code == null) 1070 if (Configuration.errorOnAutoCreate()) 1071 throw new Error("Attempt to auto-create ObservationComponentComponent.code"); 1072 else if (Configuration.doAutoCreate()) 1073 this.code = new CodeableConcept(); // cc 1074 return this.code; 1075 } 1076 1077 public boolean hasCode() { 1078 return this.code != null && !this.code.isEmpty(); 1079 } 1080 1081 /** 1082 * @param value {@link #code} (Describes what was observed. Sometimes this is called the observation "code".) 1083 */ 1084 public ObservationComponentComponent setCode(CodeableConcept value) { 1085 this.code = value; 1086 return this; 1087 } 1088 1089 /** 1090 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1091 */ 1092 public DataType getValue() { 1093 return this.value; 1094 } 1095 1096 /** 1097 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1098 */ 1099 public Quantity getValueQuantity() throws FHIRException { 1100 if (this.value == null) 1101 this.value = new Quantity(); 1102 if (!(this.value instanceof Quantity)) 1103 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1104 return (Quantity) this.value; 1105 } 1106 1107 public boolean hasValueQuantity() { 1108 return this.value instanceof Quantity; 1109 } 1110 1111 /** 1112 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1113 */ 1114 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1115 if (this.value == null) 1116 this.value = new CodeableConcept(); 1117 if (!(this.value instanceof CodeableConcept)) 1118 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1119 return (CodeableConcept) this.value; 1120 } 1121 1122 public boolean hasValueCodeableConcept() { 1123 return this.value instanceof CodeableConcept; 1124 } 1125 1126 /** 1127 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1128 */ 1129 public StringType getValueStringType() throws FHIRException { 1130 if (this.value == null) 1131 this.value = new StringType(); 1132 if (!(this.value instanceof StringType)) 1133 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1134 return (StringType) this.value; 1135 } 1136 1137 public boolean hasValueStringType() { 1138 return this.value instanceof StringType; 1139 } 1140 1141 /** 1142 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1143 */ 1144 public BooleanType getValueBooleanType() throws FHIRException { 1145 if (this.value == null) 1146 this.value = new BooleanType(); 1147 if (!(this.value instanceof BooleanType)) 1148 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1149 return (BooleanType) this.value; 1150 } 1151 1152 public boolean hasValueBooleanType() { 1153 return this.value instanceof BooleanType; 1154 } 1155 1156 /** 1157 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1158 */ 1159 public IntegerType getValueIntegerType() throws FHIRException { 1160 if (this.value == null) 1161 this.value = new IntegerType(); 1162 if (!(this.value instanceof IntegerType)) 1163 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 1164 return (IntegerType) this.value; 1165 } 1166 1167 public boolean hasValueIntegerType() { 1168 return this.value instanceof IntegerType; 1169 } 1170 1171 /** 1172 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1173 */ 1174 public Range getValueRange() throws FHIRException { 1175 if (this.value == null) 1176 this.value = new Range(); 1177 if (!(this.value instanceof Range)) 1178 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1179 return (Range) this.value; 1180 } 1181 1182 public boolean hasValueRange() { 1183 return this.value instanceof Range; 1184 } 1185 1186 /** 1187 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1188 */ 1189 public Ratio getValueRatio() throws FHIRException { 1190 if (this.value == null) 1191 this.value = new Ratio(); 1192 if (!(this.value instanceof Ratio)) 1193 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 1194 return (Ratio) this.value; 1195 } 1196 1197 public boolean hasValueRatio() { 1198 return this.value instanceof Ratio; 1199 } 1200 1201 /** 1202 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1203 */ 1204 public SampledData getValueSampledData() throws FHIRException { 1205 if (this.value == null) 1206 this.value = new SampledData(); 1207 if (!(this.value instanceof SampledData)) 1208 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 1209 return (SampledData) this.value; 1210 } 1211 1212 public boolean hasValueSampledData() { 1213 return this.value instanceof SampledData; 1214 } 1215 1216 /** 1217 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1218 */ 1219 public TimeType getValueTimeType() throws FHIRException { 1220 if (this.value == null) 1221 this.value = new TimeType(); 1222 if (!(this.value instanceof TimeType)) 1223 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1224 return (TimeType) this.value; 1225 } 1226 1227 public boolean hasValueTimeType() { 1228 return this.value instanceof TimeType; 1229 } 1230 1231 /** 1232 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1233 */ 1234 public DateTimeType getValueDateTimeType() throws FHIRException { 1235 if (this.value == null) 1236 this.value = new DateTimeType(); 1237 if (!(this.value instanceof DateTimeType)) 1238 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1239 return (DateTimeType) this.value; 1240 } 1241 1242 public boolean hasValueDateTimeType() { 1243 return this.value instanceof DateTimeType; 1244 } 1245 1246 /** 1247 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1248 */ 1249 public Period getValuePeriod() throws FHIRException { 1250 if (this.value == null) 1251 this.value = new Period(); 1252 if (!(this.value instanceof Period)) 1253 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 1254 return (Period) this.value; 1255 } 1256 1257 public boolean hasValuePeriod() { 1258 return this.value instanceof Period; 1259 } 1260 1261 /** 1262 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1263 */ 1264 public Attachment getValueAttachment() throws FHIRException { 1265 if (this.value == null) 1266 this.value = new Attachment(); 1267 if (!(this.value instanceof Attachment)) 1268 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1269 return (Attachment) this.value; 1270 } 1271 1272 public boolean hasValueAttachment() { 1273 return this.value instanceof Attachment; 1274 } 1275 1276 /** 1277 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1278 */ 1279 public Reference getValueReference() throws FHIRException { 1280 if (this.value == null) 1281 this.value = new Reference(); 1282 if (!(this.value instanceof Reference)) 1283 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1284 return (Reference) this.value; 1285 } 1286 1287 public boolean hasValueReference() { 1288 return this.value instanceof Reference; 1289 } 1290 1291 public boolean hasValue() { 1292 return this.value != null && !this.value.isEmpty(); 1293 } 1294 1295 /** 1296 * @param value {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1297 */ 1298 public ObservationComponentComponent setValue(DataType value) { 1299 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Ratio || value instanceof SampledData || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period || value instanceof Attachment || value instanceof Reference)) 1300 throw new FHIRException("Not the right type for Observation.component.value[x]: "+value.fhirType()); 1301 this.value = value; 1302 return this; 1303 } 1304 1305 /** 1306 * @return {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.component.value[x] is missing.) 1307 */ 1308 public CodeableConcept getDataAbsentReason() { 1309 if (this.dataAbsentReason == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create ObservationComponentComponent.dataAbsentReason"); 1312 else if (Configuration.doAutoCreate()) 1313 this.dataAbsentReason = new CodeableConcept(); // cc 1314 return this.dataAbsentReason; 1315 } 1316 1317 public boolean hasDataAbsentReason() { 1318 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.component.value[x] is missing.) 1323 */ 1324 public ObservationComponentComponent setDataAbsentReason(CodeableConcept value) { 1325 this.dataAbsentReason = value; 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #interpretation} (A categorical assessment of an observation value. For example, high, low, normal.) 1331 */ 1332 public List<CodeableConcept> getInterpretation() { 1333 if (this.interpretation == null) 1334 this.interpretation = new ArrayList<CodeableConcept>(); 1335 return this.interpretation; 1336 } 1337 1338 /** 1339 * @return Returns a reference to <code>this</code> for easy method chaining 1340 */ 1341 public ObservationComponentComponent setInterpretation(List<CodeableConcept> theInterpretation) { 1342 this.interpretation = theInterpretation; 1343 return this; 1344 } 1345 1346 public boolean hasInterpretation() { 1347 if (this.interpretation == null) 1348 return false; 1349 for (CodeableConcept item : this.interpretation) 1350 if (!item.isEmpty()) 1351 return true; 1352 return false; 1353 } 1354 1355 public CodeableConcept addInterpretation() { //3 1356 CodeableConcept t = new CodeableConcept(); 1357 if (this.interpretation == null) 1358 this.interpretation = new ArrayList<CodeableConcept>(); 1359 this.interpretation.add(t); 1360 return t; 1361 } 1362 1363 public ObservationComponentComponent addInterpretation(CodeableConcept t) { //3 1364 if (t == null) 1365 return this; 1366 if (this.interpretation == null) 1367 this.interpretation = new ArrayList<CodeableConcept>(); 1368 this.interpretation.add(t); 1369 return this; 1370 } 1371 1372 /** 1373 * @return The first repetition of repeating field {@link #interpretation}, creating it if it does not already exist {3} 1374 */ 1375 public CodeableConcept getInterpretationFirstRep() { 1376 if (getInterpretation().isEmpty()) { 1377 addInterpretation(); 1378 } 1379 return getInterpretation().get(0); 1380 } 1381 1382 /** 1383 * @return {@link #referenceRange} (Guidance on how to interpret the value by comparison to a normal or recommended range.) 1384 */ 1385 public List<ObservationReferenceRangeComponent> getReferenceRange() { 1386 if (this.referenceRange == null) 1387 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1388 return this.referenceRange; 1389 } 1390 1391 /** 1392 * @return Returns a reference to <code>this</code> for easy method chaining 1393 */ 1394 public ObservationComponentComponent setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 1395 this.referenceRange = theReferenceRange; 1396 return this; 1397 } 1398 1399 public boolean hasReferenceRange() { 1400 if (this.referenceRange == null) 1401 return false; 1402 for (ObservationReferenceRangeComponent item : this.referenceRange) 1403 if (!item.isEmpty()) 1404 return true; 1405 return false; 1406 } 1407 1408 public ObservationReferenceRangeComponent addReferenceRange() { //3 1409 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 1410 if (this.referenceRange == null) 1411 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1412 this.referenceRange.add(t); 1413 return t; 1414 } 1415 1416 public ObservationComponentComponent addReferenceRange(ObservationReferenceRangeComponent t) { //3 1417 if (t == null) 1418 return this; 1419 if (this.referenceRange == null) 1420 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1421 this.referenceRange.add(t); 1422 return this; 1423 } 1424 1425 /** 1426 * @return The first repetition of repeating field {@link #referenceRange}, creating it if it does not already exist {3} 1427 */ 1428 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 1429 if (getReferenceRange().isEmpty()) { 1430 addReferenceRange(); 1431 } 1432 return getReferenceRange().get(0); 1433 } 1434 1435 protected void listChildren(List<Property> children) { 1436 super.listChildren(children); 1437 children.add(new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code)); 1438 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value)); 1439 children.add(new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, dataAbsentReason)); 1440 children.add(new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation)); 1441 children.add(new Property("referenceRange", "@Observation.referenceRange", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange)); 1442 } 1443 1444 @Override 1445 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1446 switch (_hash) { 1447 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code); 1448 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1449 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1450 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1451 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1452 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1453 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1454 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1455 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1456 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1457 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1458 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1459 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1460 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1461 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1462 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1463 case 1034315687: /*dataAbsentReason*/ return new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, dataAbsentReason); 1464 case -297950712: /*interpretation*/ return new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation); 1465 case -1912545102: /*referenceRange*/ return new Property("referenceRange", "@Observation.referenceRange", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange); 1466 default: return super.getNamedProperty(_hash, _name, _checkValid); 1467 } 1468 1469 } 1470 1471 @Override 1472 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1473 switch (hash) { 1474 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1475 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1476 case 1034315687: /*dataAbsentReason*/ return this.dataAbsentReason == null ? new Base[0] : new Base[] {this.dataAbsentReason}; // CodeableConcept 1477 case -297950712: /*interpretation*/ return this.interpretation == null ? new Base[0] : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 1478 case -1912545102: /*referenceRange*/ return this.referenceRange == null ? new Base[0] : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 1479 default: return super.getProperty(hash, name, checkValid); 1480 } 1481 1482 } 1483 1484 @Override 1485 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1486 switch (hash) { 1487 case 3059181: // code 1488 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1489 return value; 1490 case 111972721: // value 1491 this.value = TypeConvertor.castToType(value); // DataType 1492 return value; 1493 case 1034315687: // dataAbsentReason 1494 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1495 return value; 1496 case -297950712: // interpretation 1497 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1498 return value; 1499 case -1912545102: // referenceRange 1500 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 1501 return value; 1502 default: return super.setProperty(hash, name, value); 1503 } 1504 1505 } 1506 1507 @Override 1508 public Base setProperty(String name, Base value) throws FHIRException { 1509 if (name.equals("code")) { 1510 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1511 } else if (name.equals("value[x]")) { 1512 this.value = TypeConvertor.castToType(value); // DataType 1513 } else if (name.equals("dataAbsentReason")) { 1514 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1515 } else if (name.equals("interpretation")) { 1516 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); 1517 } else if (name.equals("referenceRange")) { 1518 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 1519 } else 1520 return super.setProperty(name, value); 1521 return value; 1522 } 1523 1524 @Override 1525 public void removeChild(String name, Base value) throws FHIRException { 1526 if (name.equals("code")) { 1527 this.code = null; 1528 } else if (name.equals("value[x]")) { 1529 this.value = null; 1530 } else if (name.equals("dataAbsentReason")) { 1531 this.dataAbsentReason = null; 1532 } else if (name.equals("interpretation")) { 1533 this.getInterpretation().remove(value); 1534 } else if (name.equals("referenceRange")) { 1535 this.getReferenceRange().remove((ObservationReferenceRangeComponent) value); 1536 } else 1537 super.removeChild(name, value); 1538 1539 } 1540 1541 @Override 1542 public Base makeProperty(int hash, String name) throws FHIRException { 1543 switch (hash) { 1544 case 3059181: return getCode(); 1545 case -1410166417: return getValue(); 1546 case 111972721: return getValue(); 1547 case 1034315687: return getDataAbsentReason(); 1548 case -297950712: return addInterpretation(); 1549 case -1912545102: return addReferenceRange(); 1550 default: return super.makeProperty(hash, name); 1551 } 1552 1553 } 1554 1555 @Override 1556 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1557 switch (hash) { 1558 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1559 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Ratio", "SampledData", "time", "dateTime", "Period", "Attachment", "Reference"}; 1560 case 1034315687: /*dataAbsentReason*/ return new String[] {"CodeableConcept"}; 1561 case -297950712: /*interpretation*/ return new String[] {"CodeableConcept"}; 1562 case -1912545102: /*referenceRange*/ return new String[] {"@Observation.referenceRange"}; 1563 default: return super.getTypesForProperty(hash, name); 1564 } 1565 1566 } 1567 1568 @Override 1569 public Base addChild(String name) throws FHIRException { 1570 if (name.equals("code")) { 1571 this.code = new CodeableConcept(); 1572 return this.code; 1573 } 1574 else if (name.equals("valueQuantity")) { 1575 this.value = new Quantity(); 1576 return this.value; 1577 } 1578 else if (name.equals("valueCodeableConcept")) { 1579 this.value = new CodeableConcept(); 1580 return this.value; 1581 } 1582 else if (name.equals("valueString")) { 1583 this.value = new StringType(); 1584 return this.value; 1585 } 1586 else if (name.equals("valueBoolean")) { 1587 this.value = new BooleanType(); 1588 return this.value; 1589 } 1590 else if (name.equals("valueInteger")) { 1591 this.value = new IntegerType(); 1592 return this.value; 1593 } 1594 else if (name.equals("valueRange")) { 1595 this.value = new Range(); 1596 return this.value; 1597 } 1598 else if (name.equals("valueRatio")) { 1599 this.value = new Ratio(); 1600 return this.value; 1601 } 1602 else if (name.equals("valueSampledData")) { 1603 this.value = new SampledData(); 1604 return this.value; 1605 } 1606 else if (name.equals("valueTime")) { 1607 this.value = new TimeType(); 1608 return this.value; 1609 } 1610 else if (name.equals("valueDateTime")) { 1611 this.value = new DateTimeType(); 1612 return this.value; 1613 } 1614 else if (name.equals("valuePeriod")) { 1615 this.value = new Period(); 1616 return this.value; 1617 } 1618 else if (name.equals("valueAttachment")) { 1619 this.value = new Attachment(); 1620 return this.value; 1621 } 1622 else if (name.equals("valueReference")) { 1623 this.value = new Reference(); 1624 return this.value; 1625 } 1626 else if (name.equals("dataAbsentReason")) { 1627 this.dataAbsentReason = new CodeableConcept(); 1628 return this.dataAbsentReason; 1629 } 1630 else if (name.equals("interpretation")) { 1631 return addInterpretation(); 1632 } 1633 else if (name.equals("referenceRange")) { 1634 return addReferenceRange(); 1635 } 1636 else 1637 return super.addChild(name); 1638 } 1639 1640 public ObservationComponentComponent copy() { 1641 ObservationComponentComponent dst = new ObservationComponentComponent(); 1642 copyValues(dst); 1643 return dst; 1644 } 1645 1646 public void copyValues(ObservationComponentComponent dst) { 1647 super.copyValues(dst); 1648 dst.code = code == null ? null : code.copy(); 1649 dst.value = value == null ? null : value.copy(); 1650 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 1651 if (interpretation != null) { 1652 dst.interpretation = new ArrayList<CodeableConcept>(); 1653 for (CodeableConcept i : interpretation) 1654 dst.interpretation.add(i.copy()); 1655 }; 1656 if (referenceRange != null) { 1657 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1658 for (ObservationReferenceRangeComponent i : referenceRange) 1659 dst.referenceRange.add(i.copy()); 1660 }; 1661 } 1662 1663 @Override 1664 public boolean equalsDeep(Base other_) { 1665 if (!super.equalsDeep(other_)) 1666 return false; 1667 if (!(other_ instanceof ObservationComponentComponent)) 1668 return false; 1669 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1670 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 1671 && compareDeep(interpretation, o.interpretation, true) && compareDeep(referenceRange, o.referenceRange, true) 1672 ; 1673 } 1674 1675 @Override 1676 public boolean equalsShallow(Base other_) { 1677 if (!super.equalsShallow(other_)) 1678 return false; 1679 if (!(other_ instanceof ObservationComponentComponent)) 1680 return false; 1681 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1682 return true; 1683 } 1684 1685 public boolean isEmpty() { 1686 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, dataAbsentReason 1687 , interpretation, referenceRange); 1688 } 1689 1690 public String fhirType() { 1691 return "Observation.component"; 1692 1693 } 1694 1695 } 1696 1697 /** 1698 * A unique identifier assigned to this observation. 1699 */ 1700 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1701 @Description(shortDefinition="Business Identifier for observation", formalDefinition="A unique identifier assigned to this observation." ) 1702 protected List<Identifier> identifier; 1703 1704 /** 1705 * The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance. 1706 */ 1707 @Child(name = "instantiates", type = {CanonicalType.class, ObservationDefinition.class}, order=1, min=0, max=1, modifier=false, summary=true) 1708 @Description(shortDefinition="Instantiates FHIR ObservationDefinition", formalDefinition="The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance." ) 1709 protected DataType instantiates; 1710 1711 /** 1712 * A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed. 1713 */ 1714 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1715 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed." ) 1716 protected List<Reference> basedOn; 1717 1718 /** 1719 * Identifies the observation(s) that triggered the performance of this observation. 1720 */ 1721 @Child(name = "triggeredBy", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1722 @Description(shortDefinition="Triggering observation(s)", formalDefinition="Identifies the observation(s) that triggered the performance of this observation." ) 1723 protected List<ObservationTriggeredByComponent> triggeredBy; 1724 1725 /** 1726 * A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure. 1727 */ 1728 @Child(name = "partOf", type = {MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Procedure.class, Immunization.class, ImagingStudy.class, GenomicStudy.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1729 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure." ) 1730 protected List<Reference> partOf; 1731 1732 /** 1733 * The status of the result value. 1734 */ 1735 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 1736 @Description(shortDefinition="registered | preliminary | final | amended +", formalDefinition="The status of the result value." ) 1737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-status") 1738 protected Enumeration<ObservationStatus> status; 1739 1740 /** 1741 * A code that classifies the general type of observation being made. 1742 */ 1743 @Child(name = "category", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1744 @Description(shortDefinition="Classification of type of observation", formalDefinition="A code that classifies the general type of observation being made." ) 1745 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-category") 1746 protected List<CodeableConcept> category; 1747 1748 /** 1749 * Describes what was observed. Sometimes this is called the observation "name". 1750 */ 1751 @Child(name = "code", type = {CodeableConcept.class}, order=7, min=1, max=1, modifier=false, summary=true) 1752 @Description(shortDefinition="Type of observation (code / type)", formalDefinition="Describes what was observed. Sometimes this is called the observation \"name\"." ) 1753 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1754 protected CodeableConcept code; 1755 1756 /** 1757 * The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation. 1758 */ 1759 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class, Organization.class, Procedure.class, Practitioner.class, Medication.class, Substance.class, BiologicallyDerivedProduct.class, NutritionProduct.class}, order=8, min=0, max=1, modifier=false, summary=true) 1760 @Description(shortDefinition="Who and/or what the observation is about", formalDefinition="The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation." ) 1761 protected Reference subject; 1762 1763 /** 1764 * The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus. 1765 */ 1766 @Child(name = "focus", type = {Reference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1767 @Description(shortDefinition="What the observation is about, when it is not about the subject of record", formalDefinition="The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus." ) 1768 protected List<Reference> focus; 1769 1770 /** 1771 * The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made. 1772 */ 1773 @Child(name = "encounter", type = {Encounter.class}, order=10, min=0, max=1, modifier=false, summary=true) 1774 @Description(shortDefinition="Healthcare event during which this observation is made", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made." ) 1775 protected Reference encounter; 1776 1777 /** 1778 * The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself. 1779 */ 1780 @Child(name = "effective", type = {DateTimeType.class, Period.class, Timing.class, InstantType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1781 @Description(shortDefinition="Clinically relevant time/time-period for observation", formalDefinition="The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself." ) 1782 protected DataType effective; 1783 1784 /** 1785 * The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified. 1786 */ 1787 @Child(name = "issued", type = {InstantType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1788 @Description(shortDefinition="Date/Time this version was made available", formalDefinition="The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified." ) 1789 protected InstantType issued; 1790 1791 /** 1792 * Who was responsible for asserting the observed value as "true". 1793 */ 1794 @Child(name = "performer", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, RelatedPerson.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1795 @Description(shortDefinition="Who is responsible for the observation", formalDefinition="Who was responsible for asserting the observed value as \"true\"." ) 1796 protected List<Reference> performer; 1797 1798 /** 1799 * The information determined as a result of making the observation, if the information has a simple value. 1800 */ 1801 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, Period.class, Attachment.class, MolecularSequence.class}, order=14, min=0, max=1, modifier=false, summary=true) 1802 @Description(shortDefinition="Actual result", formalDefinition="The information determined as a result of making the observation, if the information has a simple value." ) 1803 protected DataType value; 1804 1805 /** 1806 * Provides a reason why the expected value in the element Observation.value[x] is missing. 1807 */ 1808 @Child(name = "dataAbsentReason", type = {CodeableConcept.class}, order=15, min=0, max=1, modifier=false, summary=false) 1809 @Description(shortDefinition="Why the result is missing", formalDefinition="Provides a reason why the expected value in the element Observation.value[x] is missing." ) 1810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/data-absent-reason") 1811 protected CodeableConcept dataAbsentReason; 1812 1813 /** 1814 * A categorical assessment of an observation value. For example, high, low, normal. 1815 */ 1816 @Child(name = "interpretation", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1817 @Description(shortDefinition="High, low, normal, etc", formalDefinition="A categorical assessment of an observation value. For example, high, low, normal." ) 1818 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-interpretation") 1819 protected List<CodeableConcept> interpretation; 1820 1821 /** 1822 * Comments about the observation or the results. 1823 */ 1824 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1825 @Description(shortDefinition="Comments about the observation", formalDefinition="Comments about the observation or the results." ) 1826 protected List<Annotation> note; 1827 1828 /** 1829 * Indicates the site on the subject's body where the observation was made (i.e. the target site). 1830 */ 1831 @Child(name = "bodySite", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=false) 1832 @Description(shortDefinition="Observed body part", formalDefinition="Indicates the site on the subject's body where the observation was made (i.e. the target site)." ) 1833 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1834 protected CodeableConcept bodySite; 1835 1836 /** 1837 * Indicates the body structure on the subject's body where the observation was made (i.e. the target site). 1838 */ 1839 @Child(name = "bodyStructure", type = {BodyStructure.class}, order=19, min=0, max=1, modifier=false, summary=false) 1840 @Description(shortDefinition="Observed body structure", formalDefinition="Indicates the body structure on the subject's body where the observation was made (i.e. the target site)." ) 1841 protected Reference bodyStructure; 1842 1843 /** 1844 * Indicates the mechanism used to perform the observation. 1845 */ 1846 @Child(name = "method", type = {CodeableConcept.class}, order=20, min=0, max=1, modifier=false, summary=false) 1847 @Description(shortDefinition="How it was done", formalDefinition="Indicates the mechanism used to perform the observation." ) 1848 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-methods") 1849 protected CodeableConcept method; 1850 1851 /** 1852 * The specimen that was used when this observation was made. 1853 */ 1854 @Child(name = "specimen", type = {Specimen.class, Group.class}, order=21, min=0, max=1, modifier=false, summary=false) 1855 @Description(shortDefinition="Specimen used for this observation", formalDefinition="The specimen that was used when this observation was made." ) 1856 protected Reference specimen; 1857 1858 /** 1859 * A reference to the device that generates the measurements or the device settings for the device. 1860 */ 1861 @Child(name = "device", type = {Device.class, DeviceMetric.class}, order=22, min=0, max=1, modifier=false, summary=false) 1862 @Description(shortDefinition="A reference to the device that generates the measurements or the device settings for the device", formalDefinition="A reference to the device that generates the measurements or the device settings for the device." ) 1863 protected Reference device; 1864 1865 /** 1866 * Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an "OR". In other words, to represent two distinct target populations, two `referenceRange` elements would be used. 1867 */ 1868 @Child(name = "referenceRange", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1869 @Description(shortDefinition="Provides guide for interpretation", formalDefinition="Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used." ) 1870 protected List<ObservationReferenceRangeComponent> referenceRange; 1871 1872 /** 1873 * This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group. 1874 */ 1875 @Child(name = "hasMember", type = {Observation.class, QuestionnaireResponse.class, MolecularSequence.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1876 @Description(shortDefinition="Related resource that belongs to the Observation group", formalDefinition="This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group." ) 1877 protected List<Reference> hasMember; 1878 1879 /** 1880 * The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image. 1881 */ 1882 @Child(name = "derivedFrom", type = {DocumentReference.class, ImagingStudy.class, ImagingSelection.class, QuestionnaireResponse.class, Observation.class, MolecularSequence.class, GenomicStudy.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1883 @Description(shortDefinition="Related resource from which the observation is made", formalDefinition="The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image." ) 1884 protected List<Reference> derivedFrom; 1885 1886 /** 1887 * Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations. 1888 */ 1889 @Child(name = "component", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1890 @Description(shortDefinition="Component results", formalDefinition="Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations." ) 1891 protected List<ObservationComponentComponent> component; 1892 1893 private static final long serialVersionUID = -44103660L; 1894 1895 /** 1896 * Constructor 1897 */ 1898 public Observation() { 1899 super(); 1900 } 1901 1902 /** 1903 * Constructor 1904 */ 1905 public Observation(ObservationStatus status, CodeableConcept code) { 1906 super(); 1907 this.setStatus(status); 1908 this.setCode(code); 1909 } 1910 1911 /** 1912 * @return {@link #identifier} (A unique identifier assigned to this observation.) 1913 */ 1914 public List<Identifier> getIdentifier() { 1915 if (this.identifier == null) 1916 this.identifier = new ArrayList<Identifier>(); 1917 return this.identifier; 1918 } 1919 1920 /** 1921 * @return Returns a reference to <code>this</code> for easy method chaining 1922 */ 1923 public Observation setIdentifier(List<Identifier> theIdentifier) { 1924 this.identifier = theIdentifier; 1925 return this; 1926 } 1927 1928 public boolean hasIdentifier() { 1929 if (this.identifier == null) 1930 return false; 1931 for (Identifier item : this.identifier) 1932 if (!item.isEmpty()) 1933 return true; 1934 return false; 1935 } 1936 1937 public Identifier addIdentifier() { //3 1938 Identifier t = new Identifier(); 1939 if (this.identifier == null) 1940 this.identifier = new ArrayList<Identifier>(); 1941 this.identifier.add(t); 1942 return t; 1943 } 1944 1945 public Observation addIdentifier(Identifier t) { //3 1946 if (t == null) 1947 return this; 1948 if (this.identifier == null) 1949 this.identifier = new ArrayList<Identifier>(); 1950 this.identifier.add(t); 1951 return this; 1952 } 1953 1954 /** 1955 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1956 */ 1957 public Identifier getIdentifierFirstRep() { 1958 if (getIdentifier().isEmpty()) { 1959 addIdentifier(); 1960 } 1961 return getIdentifier().get(0); 1962 } 1963 1964 /** 1965 * @return {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 1966 */ 1967 public DataType getInstantiates() { 1968 return this.instantiates; 1969 } 1970 1971 /** 1972 * @return {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 1973 */ 1974 public CanonicalType getInstantiatesCanonicalType() throws FHIRException { 1975 if (this.instantiates == null) 1976 this.instantiates = new CanonicalType(); 1977 if (!(this.instantiates instanceof CanonicalType)) 1978 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.instantiates.getClass().getName()+" was encountered"); 1979 return (CanonicalType) this.instantiates; 1980 } 1981 1982 public boolean hasInstantiatesCanonicalType() { 1983 return this.instantiates instanceof CanonicalType; 1984 } 1985 1986 /** 1987 * @return {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 1988 */ 1989 public Reference getInstantiatesReference() throws FHIRException { 1990 if (this.instantiates == null) 1991 this.instantiates = new Reference(); 1992 if (!(this.instantiates instanceof Reference)) 1993 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.instantiates.getClass().getName()+" was encountered"); 1994 return (Reference) this.instantiates; 1995 } 1996 1997 public boolean hasInstantiatesReference() { 1998 return this.instantiates instanceof Reference; 1999 } 2000 2001 public boolean hasInstantiates() { 2002 return this.instantiates != null && !this.instantiates.isEmpty(); 2003 } 2004 2005 /** 2006 * @param value {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 2007 */ 2008 public Observation setInstantiates(DataType value) { 2009 if (value != null && !(value instanceof CanonicalType || value instanceof Reference)) 2010 throw new FHIRException("Not the right type for Observation.instantiates[x]: "+value.fhirType()); 2011 this.instantiates = value; 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.) 2017 */ 2018 public List<Reference> getBasedOn() { 2019 if (this.basedOn == null) 2020 this.basedOn = new ArrayList<Reference>(); 2021 return this.basedOn; 2022 } 2023 2024 /** 2025 * @return Returns a reference to <code>this</code> for easy method chaining 2026 */ 2027 public Observation setBasedOn(List<Reference> theBasedOn) { 2028 this.basedOn = theBasedOn; 2029 return this; 2030 } 2031 2032 public boolean hasBasedOn() { 2033 if (this.basedOn == null) 2034 return false; 2035 for (Reference item : this.basedOn) 2036 if (!item.isEmpty()) 2037 return true; 2038 return false; 2039 } 2040 2041 public Reference addBasedOn() { //3 2042 Reference t = new Reference(); 2043 if (this.basedOn == null) 2044 this.basedOn = new ArrayList<Reference>(); 2045 this.basedOn.add(t); 2046 return t; 2047 } 2048 2049 public Observation addBasedOn(Reference t) { //3 2050 if (t == null) 2051 return this; 2052 if (this.basedOn == null) 2053 this.basedOn = new ArrayList<Reference>(); 2054 this.basedOn.add(t); 2055 return this; 2056 } 2057 2058 /** 2059 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 2060 */ 2061 public Reference getBasedOnFirstRep() { 2062 if (getBasedOn().isEmpty()) { 2063 addBasedOn(); 2064 } 2065 return getBasedOn().get(0); 2066 } 2067 2068 /** 2069 * @return {@link #triggeredBy} (Identifies the observation(s) that triggered the performance of this observation.) 2070 */ 2071 public List<ObservationTriggeredByComponent> getTriggeredBy() { 2072 if (this.triggeredBy == null) 2073 this.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 2074 return this.triggeredBy; 2075 } 2076 2077 /** 2078 * @return Returns a reference to <code>this</code> for easy method chaining 2079 */ 2080 public Observation setTriggeredBy(List<ObservationTriggeredByComponent> theTriggeredBy) { 2081 this.triggeredBy = theTriggeredBy; 2082 return this; 2083 } 2084 2085 public boolean hasTriggeredBy() { 2086 if (this.triggeredBy == null) 2087 return false; 2088 for (ObservationTriggeredByComponent item : this.triggeredBy) 2089 if (!item.isEmpty()) 2090 return true; 2091 return false; 2092 } 2093 2094 public ObservationTriggeredByComponent addTriggeredBy() { //3 2095 ObservationTriggeredByComponent t = new ObservationTriggeredByComponent(); 2096 if (this.triggeredBy == null) 2097 this.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 2098 this.triggeredBy.add(t); 2099 return t; 2100 } 2101 2102 public Observation addTriggeredBy(ObservationTriggeredByComponent t) { //3 2103 if (t == null) 2104 return this; 2105 if (this.triggeredBy == null) 2106 this.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 2107 this.triggeredBy.add(t); 2108 return this; 2109 } 2110 2111 /** 2112 * @return The first repetition of repeating field {@link #triggeredBy}, creating it if it does not already exist {3} 2113 */ 2114 public ObservationTriggeredByComponent getTriggeredByFirstRep() { 2115 if (getTriggeredBy().isEmpty()) { 2116 addTriggeredBy(); 2117 } 2118 return getTriggeredBy().get(0); 2119 } 2120 2121 /** 2122 * @return {@link #partOf} (A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.) 2123 */ 2124 public List<Reference> getPartOf() { 2125 if (this.partOf == null) 2126 this.partOf = new ArrayList<Reference>(); 2127 return this.partOf; 2128 } 2129 2130 /** 2131 * @return Returns a reference to <code>this</code> for easy method chaining 2132 */ 2133 public Observation setPartOf(List<Reference> thePartOf) { 2134 this.partOf = thePartOf; 2135 return this; 2136 } 2137 2138 public boolean hasPartOf() { 2139 if (this.partOf == null) 2140 return false; 2141 for (Reference item : this.partOf) 2142 if (!item.isEmpty()) 2143 return true; 2144 return false; 2145 } 2146 2147 public Reference addPartOf() { //3 2148 Reference t = new Reference(); 2149 if (this.partOf == null) 2150 this.partOf = new ArrayList<Reference>(); 2151 this.partOf.add(t); 2152 return t; 2153 } 2154 2155 public Observation addPartOf(Reference t) { //3 2156 if (t == null) 2157 return this; 2158 if (this.partOf == null) 2159 this.partOf = new ArrayList<Reference>(); 2160 this.partOf.add(t); 2161 return this; 2162 } 2163 2164 /** 2165 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 2166 */ 2167 public Reference getPartOfFirstRep() { 2168 if (getPartOf().isEmpty()) { 2169 addPartOf(); 2170 } 2171 return getPartOf().get(0); 2172 } 2173 2174 /** 2175 * @return {@link #status} (The status of the result value.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2176 */ 2177 public Enumeration<ObservationStatus> getStatusElement() { 2178 if (this.status == null) 2179 if (Configuration.errorOnAutoCreate()) 2180 throw new Error("Attempt to auto-create Observation.status"); 2181 else if (Configuration.doAutoCreate()) 2182 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); // bb 2183 return this.status; 2184 } 2185 2186 public boolean hasStatusElement() { 2187 return this.status != null && !this.status.isEmpty(); 2188 } 2189 2190 public boolean hasStatus() { 2191 return this.status != null && !this.status.isEmpty(); 2192 } 2193 2194 /** 2195 * @param value {@link #status} (The status of the result value.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2196 */ 2197 public Observation setStatusElement(Enumeration<ObservationStatus> value) { 2198 this.status = value; 2199 return this; 2200 } 2201 2202 /** 2203 * @return The status of the result value. 2204 */ 2205 public ObservationStatus getStatus() { 2206 return this.status == null ? null : this.status.getValue(); 2207 } 2208 2209 /** 2210 * @param value The status of the result value. 2211 */ 2212 public Observation setStatus(ObservationStatus value) { 2213 if (this.status == null) 2214 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); 2215 this.status.setValue(value); 2216 return this; 2217 } 2218 2219 /** 2220 * @return {@link #category} (A code that classifies the general type of observation being made.) 2221 */ 2222 public List<CodeableConcept> getCategory() { 2223 if (this.category == null) 2224 this.category = new ArrayList<CodeableConcept>(); 2225 return this.category; 2226 } 2227 2228 /** 2229 * @return Returns a reference to <code>this</code> for easy method chaining 2230 */ 2231 public Observation setCategory(List<CodeableConcept> theCategory) { 2232 this.category = theCategory; 2233 return this; 2234 } 2235 2236 public boolean hasCategory() { 2237 if (this.category == null) 2238 return false; 2239 for (CodeableConcept item : this.category) 2240 if (!item.isEmpty()) 2241 return true; 2242 return false; 2243 } 2244 2245 public CodeableConcept addCategory() { //3 2246 CodeableConcept t = new CodeableConcept(); 2247 if (this.category == null) 2248 this.category = new ArrayList<CodeableConcept>(); 2249 this.category.add(t); 2250 return t; 2251 } 2252 2253 public Observation addCategory(CodeableConcept t) { //3 2254 if (t == null) 2255 return this; 2256 if (this.category == null) 2257 this.category = new ArrayList<CodeableConcept>(); 2258 this.category.add(t); 2259 return this; 2260 } 2261 2262 /** 2263 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2264 */ 2265 public CodeableConcept getCategoryFirstRep() { 2266 if (getCategory().isEmpty()) { 2267 addCategory(); 2268 } 2269 return getCategory().get(0); 2270 } 2271 2272 /** 2273 * @return {@link #code} (Describes what was observed. Sometimes this is called the observation "name".) 2274 */ 2275 public CodeableConcept getCode() { 2276 if (this.code == null) 2277 if (Configuration.errorOnAutoCreate()) 2278 throw new Error("Attempt to auto-create Observation.code"); 2279 else if (Configuration.doAutoCreate()) 2280 this.code = new CodeableConcept(); // cc 2281 return this.code; 2282 } 2283 2284 public boolean hasCode() { 2285 return this.code != null && !this.code.isEmpty(); 2286 } 2287 2288 /** 2289 * @param value {@link #code} (Describes what was observed. Sometimes this is called the observation "name".) 2290 */ 2291 public Observation setCode(CodeableConcept value) { 2292 this.code = value; 2293 return this; 2294 } 2295 2296 /** 2297 * @return {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.) 2298 */ 2299 public Reference getSubject() { 2300 if (this.subject == null) 2301 if (Configuration.errorOnAutoCreate()) 2302 throw new Error("Attempt to auto-create Observation.subject"); 2303 else if (Configuration.doAutoCreate()) 2304 this.subject = new Reference(); // cc 2305 return this.subject; 2306 } 2307 2308 public boolean hasSubject() { 2309 return this.subject != null && !this.subject.isEmpty(); 2310 } 2311 2312 /** 2313 * @param value {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.) 2314 */ 2315 public Observation setSubject(Reference value) { 2316 this.subject = value; 2317 return this; 2318 } 2319 2320 /** 2321 * @return {@link #focus} (The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.) 2322 */ 2323 public List<Reference> getFocus() { 2324 if (this.focus == null) 2325 this.focus = new ArrayList<Reference>(); 2326 return this.focus; 2327 } 2328 2329 /** 2330 * @return Returns a reference to <code>this</code> for easy method chaining 2331 */ 2332 public Observation setFocus(List<Reference> theFocus) { 2333 this.focus = theFocus; 2334 return this; 2335 } 2336 2337 public boolean hasFocus() { 2338 if (this.focus == null) 2339 return false; 2340 for (Reference item : this.focus) 2341 if (!item.isEmpty()) 2342 return true; 2343 return false; 2344 } 2345 2346 public Reference addFocus() { //3 2347 Reference t = new Reference(); 2348 if (this.focus == null) 2349 this.focus = new ArrayList<Reference>(); 2350 this.focus.add(t); 2351 return t; 2352 } 2353 2354 public Observation addFocus(Reference t) { //3 2355 if (t == null) 2356 return this; 2357 if (this.focus == null) 2358 this.focus = new ArrayList<Reference>(); 2359 this.focus.add(t); 2360 return this; 2361 } 2362 2363 /** 2364 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 2365 */ 2366 public Reference getFocusFirstRep() { 2367 if (getFocus().isEmpty()) { 2368 addFocus(); 2369 } 2370 return getFocus().get(0); 2371 } 2372 2373 /** 2374 * @return {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2375 */ 2376 public Reference getEncounter() { 2377 if (this.encounter == null) 2378 if (Configuration.errorOnAutoCreate()) 2379 throw new Error("Attempt to auto-create Observation.encounter"); 2380 else if (Configuration.doAutoCreate()) 2381 this.encounter = new Reference(); // cc 2382 return this.encounter; 2383 } 2384 2385 public boolean hasEncounter() { 2386 return this.encounter != null && !this.encounter.isEmpty(); 2387 } 2388 2389 /** 2390 * @param value {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2391 */ 2392 public Observation setEncounter(Reference value) { 2393 this.encounter = value; 2394 return this; 2395 } 2396 2397 /** 2398 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2399 */ 2400 public DataType getEffective() { 2401 return this.effective; 2402 } 2403 2404 /** 2405 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2406 */ 2407 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 2408 if (this.effective == null) 2409 this.effective = new DateTimeType(); 2410 if (!(this.effective instanceof DateTimeType)) 2411 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 2412 return (DateTimeType) this.effective; 2413 } 2414 2415 public boolean hasEffectiveDateTimeType() { 2416 return this.effective instanceof DateTimeType; 2417 } 2418 2419 /** 2420 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2421 */ 2422 public Period getEffectivePeriod() throws FHIRException { 2423 if (this.effective == null) 2424 this.effective = new Period(); 2425 if (!(this.effective instanceof Period)) 2426 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 2427 return (Period) this.effective; 2428 } 2429 2430 public boolean hasEffectivePeriod() { 2431 return this.effective instanceof Period; 2432 } 2433 2434 /** 2435 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2436 */ 2437 public Timing getEffectiveTiming() throws FHIRException { 2438 if (this.effective == null) 2439 this.effective = new Timing(); 2440 if (!(this.effective instanceof Timing)) 2441 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.effective.getClass().getName()+" was encountered"); 2442 return (Timing) this.effective; 2443 } 2444 2445 public boolean hasEffectiveTiming() { 2446 return this.effective instanceof Timing; 2447 } 2448 2449 /** 2450 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2451 */ 2452 public InstantType getEffectiveInstantType() throws FHIRException { 2453 if (this.effective == null) 2454 this.effective = new InstantType(); 2455 if (!(this.effective instanceof InstantType)) 2456 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.effective.getClass().getName()+" was encountered"); 2457 return (InstantType) this.effective; 2458 } 2459 2460 public boolean hasEffectiveInstantType() { 2461 return this.effective instanceof InstantType; 2462 } 2463 2464 public boolean hasEffective() { 2465 return this.effective != null && !this.effective.isEmpty(); 2466 } 2467 2468 /** 2469 * @param value {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2470 */ 2471 public Observation setEffective(DataType value) { 2472 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing || value instanceof InstantType)) 2473 throw new FHIRException("Not the right type for Observation.effective[x]: "+value.fhirType()); 2474 this.effective = value; 2475 return this; 2476 } 2477 2478 /** 2479 * @return {@link #issued} (The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2480 */ 2481 public InstantType getIssuedElement() { 2482 if (this.issued == null) 2483 if (Configuration.errorOnAutoCreate()) 2484 throw new Error("Attempt to auto-create Observation.issued"); 2485 else if (Configuration.doAutoCreate()) 2486 this.issued = new InstantType(); // bb 2487 return this.issued; 2488 } 2489 2490 public boolean hasIssuedElement() { 2491 return this.issued != null && !this.issued.isEmpty(); 2492 } 2493 2494 public boolean hasIssued() { 2495 return this.issued != null && !this.issued.isEmpty(); 2496 } 2497 2498 /** 2499 * @param value {@link #issued} (The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2500 */ 2501 public Observation setIssuedElement(InstantType value) { 2502 this.issued = value; 2503 return this; 2504 } 2505 2506 /** 2507 * @return The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified. 2508 */ 2509 public Date getIssued() { 2510 return this.issued == null ? null : this.issued.getValue(); 2511 } 2512 2513 /** 2514 * @param value The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified. 2515 */ 2516 public Observation setIssued(Date value) { 2517 if (value == null) 2518 this.issued = null; 2519 else { 2520 if (this.issued == null) 2521 this.issued = new InstantType(); 2522 this.issued.setValue(value); 2523 } 2524 return this; 2525 } 2526 2527 /** 2528 * @return {@link #performer} (Who was responsible for asserting the observed value as "true".) 2529 */ 2530 public List<Reference> getPerformer() { 2531 if (this.performer == null) 2532 this.performer = new ArrayList<Reference>(); 2533 return this.performer; 2534 } 2535 2536 /** 2537 * @return Returns a reference to <code>this</code> for easy method chaining 2538 */ 2539 public Observation setPerformer(List<Reference> thePerformer) { 2540 this.performer = thePerformer; 2541 return this; 2542 } 2543 2544 public boolean hasPerformer() { 2545 if (this.performer == null) 2546 return false; 2547 for (Reference item : this.performer) 2548 if (!item.isEmpty()) 2549 return true; 2550 return false; 2551 } 2552 2553 public Reference addPerformer() { //3 2554 Reference t = new Reference(); 2555 if (this.performer == null) 2556 this.performer = new ArrayList<Reference>(); 2557 this.performer.add(t); 2558 return t; 2559 } 2560 2561 public Observation addPerformer(Reference t) { //3 2562 if (t == null) 2563 return this; 2564 if (this.performer == null) 2565 this.performer = new ArrayList<Reference>(); 2566 this.performer.add(t); 2567 return this; 2568 } 2569 2570 /** 2571 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2572 */ 2573 public Reference getPerformerFirstRep() { 2574 if (getPerformer().isEmpty()) { 2575 addPerformer(); 2576 } 2577 return getPerformer().get(0); 2578 } 2579 2580 /** 2581 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2582 */ 2583 public DataType getValue() { 2584 return this.value; 2585 } 2586 2587 /** 2588 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2589 */ 2590 public Quantity getValueQuantity() throws FHIRException { 2591 if (this.value == null) 2592 this.value = new Quantity(); 2593 if (!(this.value instanceof Quantity)) 2594 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2595 return (Quantity) this.value; 2596 } 2597 2598 public boolean hasValueQuantity() { 2599 return this.value instanceof Quantity; 2600 } 2601 2602 /** 2603 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2604 */ 2605 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2606 if (this.value == null) 2607 this.value = new CodeableConcept(); 2608 if (!(this.value instanceof CodeableConcept)) 2609 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2610 return (CodeableConcept) this.value; 2611 } 2612 2613 public boolean hasValueCodeableConcept() { 2614 return this.value instanceof CodeableConcept; 2615 } 2616 2617 /** 2618 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2619 */ 2620 public StringType getValueStringType() throws FHIRException { 2621 if (this.value == null) 2622 this.value = new StringType(); 2623 if (!(this.value instanceof StringType)) 2624 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2625 return (StringType) this.value; 2626 } 2627 2628 public boolean hasValueStringType() { 2629 return this.value instanceof StringType; 2630 } 2631 2632 /** 2633 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2634 */ 2635 public BooleanType getValueBooleanType() throws FHIRException { 2636 if (this.value == null) 2637 this.value = new BooleanType(); 2638 if (!(this.value instanceof BooleanType)) 2639 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2640 return (BooleanType) this.value; 2641 } 2642 2643 public boolean hasValueBooleanType() { 2644 return this.value instanceof BooleanType; 2645 } 2646 2647 /** 2648 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2649 */ 2650 public IntegerType getValueIntegerType() throws FHIRException { 2651 if (this.value == null) 2652 this.value = new IntegerType(); 2653 if (!(this.value instanceof IntegerType)) 2654 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2655 return (IntegerType) this.value; 2656 } 2657 2658 public boolean hasValueIntegerType() { 2659 return this.value instanceof IntegerType; 2660 } 2661 2662 /** 2663 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2664 */ 2665 public Range getValueRange() throws FHIRException { 2666 if (this.value == null) 2667 this.value = new Range(); 2668 if (!(this.value instanceof Range)) 2669 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2670 return (Range) this.value; 2671 } 2672 2673 public boolean hasValueRange() { 2674 return this.value instanceof Range; 2675 } 2676 2677 /** 2678 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2679 */ 2680 public Ratio getValueRatio() throws FHIRException { 2681 if (this.value == null) 2682 this.value = new Ratio(); 2683 if (!(this.value instanceof Ratio)) 2684 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 2685 return (Ratio) this.value; 2686 } 2687 2688 public boolean hasValueRatio() { 2689 return this.value instanceof Ratio; 2690 } 2691 2692 /** 2693 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2694 */ 2695 public SampledData getValueSampledData() throws FHIRException { 2696 if (this.value == null) 2697 this.value = new SampledData(); 2698 if (!(this.value instanceof SampledData)) 2699 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 2700 return (SampledData) this.value; 2701 } 2702 2703 public boolean hasValueSampledData() { 2704 return this.value instanceof SampledData; 2705 } 2706 2707 /** 2708 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2709 */ 2710 public TimeType getValueTimeType() throws FHIRException { 2711 if (this.value == null) 2712 this.value = new TimeType(); 2713 if (!(this.value instanceof TimeType)) 2714 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2715 return (TimeType) this.value; 2716 } 2717 2718 public boolean hasValueTimeType() { 2719 return this.value instanceof TimeType; 2720 } 2721 2722 /** 2723 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2724 */ 2725 public DateTimeType getValueDateTimeType() throws FHIRException { 2726 if (this.value == null) 2727 this.value = new DateTimeType(); 2728 if (!(this.value instanceof DateTimeType)) 2729 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2730 return (DateTimeType) this.value; 2731 } 2732 2733 public boolean hasValueDateTimeType() { 2734 return this.value instanceof DateTimeType; 2735 } 2736 2737 /** 2738 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2739 */ 2740 public Period getValuePeriod() throws FHIRException { 2741 if (this.value == null) 2742 this.value = new Period(); 2743 if (!(this.value instanceof Period)) 2744 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 2745 return (Period) this.value; 2746 } 2747 2748 public boolean hasValuePeriod() { 2749 return this.value instanceof Period; 2750 } 2751 2752 /** 2753 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2754 */ 2755 public Attachment getValueAttachment() throws FHIRException { 2756 if (this.value == null) 2757 this.value = new Attachment(); 2758 if (!(this.value instanceof Attachment)) 2759 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 2760 return (Attachment) this.value; 2761 } 2762 2763 public boolean hasValueAttachment() { 2764 return this.value instanceof Attachment; 2765 } 2766 2767 /** 2768 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2769 */ 2770 public Reference getValueReference() throws FHIRException { 2771 if (this.value == null) 2772 this.value = new Reference(); 2773 if (!(this.value instanceof Reference)) 2774 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 2775 return (Reference) this.value; 2776 } 2777 2778 public boolean hasValueReference() { 2779 return this.value instanceof Reference; 2780 } 2781 2782 public boolean hasValue() { 2783 return this.value != null && !this.value.isEmpty(); 2784 } 2785 2786 /** 2787 * @param value {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2788 */ 2789 public Observation setValue(DataType value) { 2790 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Ratio || value instanceof SampledData || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period || value instanceof Attachment || value instanceof Reference)) 2791 throw new FHIRException("Not the right type for Observation.value[x]: "+value.fhirType()); 2792 this.value = value; 2793 return this; 2794 } 2795 2796 /** 2797 * @return {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 2798 */ 2799 public CodeableConcept getDataAbsentReason() { 2800 if (this.dataAbsentReason == null) 2801 if (Configuration.errorOnAutoCreate()) 2802 throw new Error("Attempt to auto-create Observation.dataAbsentReason"); 2803 else if (Configuration.doAutoCreate()) 2804 this.dataAbsentReason = new CodeableConcept(); // cc 2805 return this.dataAbsentReason; 2806 } 2807 2808 public boolean hasDataAbsentReason() { 2809 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 2810 } 2811 2812 /** 2813 * @param value {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 2814 */ 2815 public Observation setDataAbsentReason(CodeableConcept value) { 2816 this.dataAbsentReason = value; 2817 return this; 2818 } 2819 2820 /** 2821 * @return {@link #interpretation} (A categorical assessment of an observation value. For example, high, low, normal.) 2822 */ 2823 public List<CodeableConcept> getInterpretation() { 2824 if (this.interpretation == null) 2825 this.interpretation = new ArrayList<CodeableConcept>(); 2826 return this.interpretation; 2827 } 2828 2829 /** 2830 * @return Returns a reference to <code>this</code> for easy method chaining 2831 */ 2832 public Observation setInterpretation(List<CodeableConcept> theInterpretation) { 2833 this.interpretation = theInterpretation; 2834 return this; 2835 } 2836 2837 public boolean hasInterpretation() { 2838 if (this.interpretation == null) 2839 return false; 2840 for (CodeableConcept item : this.interpretation) 2841 if (!item.isEmpty()) 2842 return true; 2843 return false; 2844 } 2845 2846 public CodeableConcept addInterpretation() { //3 2847 CodeableConcept t = new CodeableConcept(); 2848 if (this.interpretation == null) 2849 this.interpretation = new ArrayList<CodeableConcept>(); 2850 this.interpretation.add(t); 2851 return t; 2852 } 2853 2854 public Observation addInterpretation(CodeableConcept t) { //3 2855 if (t == null) 2856 return this; 2857 if (this.interpretation == null) 2858 this.interpretation = new ArrayList<CodeableConcept>(); 2859 this.interpretation.add(t); 2860 return this; 2861 } 2862 2863 /** 2864 * @return The first repetition of repeating field {@link #interpretation}, creating it if it does not already exist {3} 2865 */ 2866 public CodeableConcept getInterpretationFirstRep() { 2867 if (getInterpretation().isEmpty()) { 2868 addInterpretation(); 2869 } 2870 return getInterpretation().get(0); 2871 } 2872 2873 /** 2874 * @return {@link #note} (Comments about the observation or the results.) 2875 */ 2876 public List<Annotation> getNote() { 2877 if (this.note == null) 2878 this.note = new ArrayList<Annotation>(); 2879 return this.note; 2880 } 2881 2882 /** 2883 * @return Returns a reference to <code>this</code> for easy method chaining 2884 */ 2885 public Observation setNote(List<Annotation> theNote) { 2886 this.note = theNote; 2887 return this; 2888 } 2889 2890 public boolean hasNote() { 2891 if (this.note == null) 2892 return false; 2893 for (Annotation item : this.note) 2894 if (!item.isEmpty()) 2895 return true; 2896 return false; 2897 } 2898 2899 public Annotation addNote() { //3 2900 Annotation t = new Annotation(); 2901 if (this.note == null) 2902 this.note = new ArrayList<Annotation>(); 2903 this.note.add(t); 2904 return t; 2905 } 2906 2907 public Observation addNote(Annotation t) { //3 2908 if (t == null) 2909 return this; 2910 if (this.note == null) 2911 this.note = new ArrayList<Annotation>(); 2912 this.note.add(t); 2913 return this; 2914 } 2915 2916 /** 2917 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2918 */ 2919 public Annotation getNoteFirstRep() { 2920 if (getNote().isEmpty()) { 2921 addNote(); 2922 } 2923 return getNote().get(0); 2924 } 2925 2926 /** 2927 * @return {@link #bodySite} (Indicates the site on the subject's body where the observation was made (i.e. the target site).) 2928 */ 2929 public CodeableConcept getBodySite() { 2930 if (this.bodySite == null) 2931 if (Configuration.errorOnAutoCreate()) 2932 throw new Error("Attempt to auto-create Observation.bodySite"); 2933 else if (Configuration.doAutoCreate()) 2934 this.bodySite = new CodeableConcept(); // cc 2935 return this.bodySite; 2936 } 2937 2938 public boolean hasBodySite() { 2939 return this.bodySite != null && !this.bodySite.isEmpty(); 2940 } 2941 2942 /** 2943 * @param value {@link #bodySite} (Indicates the site on the subject's body where the observation was made (i.e. the target site).) 2944 */ 2945 public Observation setBodySite(CodeableConcept value) { 2946 this.bodySite = value; 2947 return this; 2948 } 2949 2950 /** 2951 * @return {@link #bodyStructure} (Indicates the body structure on the subject's body where the observation was made (i.e. the target site).) 2952 */ 2953 public Reference getBodyStructure() { 2954 if (this.bodyStructure == null) 2955 if (Configuration.errorOnAutoCreate()) 2956 throw new Error("Attempt to auto-create Observation.bodyStructure"); 2957 else if (Configuration.doAutoCreate()) 2958 this.bodyStructure = new Reference(); // cc 2959 return this.bodyStructure; 2960 } 2961 2962 public boolean hasBodyStructure() { 2963 return this.bodyStructure != null && !this.bodyStructure.isEmpty(); 2964 } 2965 2966 /** 2967 * @param value {@link #bodyStructure} (Indicates the body structure on the subject's body where the observation was made (i.e. the target site).) 2968 */ 2969 public Observation setBodyStructure(Reference value) { 2970 this.bodyStructure = value; 2971 return this; 2972 } 2973 2974 /** 2975 * @return {@link #method} (Indicates the mechanism used to perform the observation.) 2976 */ 2977 public CodeableConcept getMethod() { 2978 if (this.method == null) 2979 if (Configuration.errorOnAutoCreate()) 2980 throw new Error("Attempt to auto-create Observation.method"); 2981 else if (Configuration.doAutoCreate()) 2982 this.method = new CodeableConcept(); // cc 2983 return this.method; 2984 } 2985 2986 public boolean hasMethod() { 2987 return this.method != null && !this.method.isEmpty(); 2988 } 2989 2990 /** 2991 * @param value {@link #method} (Indicates the mechanism used to perform the observation.) 2992 */ 2993 public Observation setMethod(CodeableConcept value) { 2994 this.method = value; 2995 return this; 2996 } 2997 2998 /** 2999 * @return {@link #specimen} (The specimen that was used when this observation was made.) 3000 */ 3001 public Reference getSpecimen() { 3002 if (this.specimen == null) 3003 if (Configuration.errorOnAutoCreate()) 3004 throw new Error("Attempt to auto-create Observation.specimen"); 3005 else if (Configuration.doAutoCreate()) 3006 this.specimen = new Reference(); // cc 3007 return this.specimen; 3008 } 3009 3010 public boolean hasSpecimen() { 3011 return this.specimen != null && !this.specimen.isEmpty(); 3012 } 3013 3014 /** 3015 * @param value {@link #specimen} (The specimen that was used when this observation was made.) 3016 */ 3017 public Observation setSpecimen(Reference value) { 3018 this.specimen = value; 3019 return this; 3020 } 3021 3022 /** 3023 * @return {@link #device} (A reference to the device that generates the measurements or the device settings for the device.) 3024 */ 3025 public Reference getDevice() { 3026 if (this.device == null) 3027 if (Configuration.errorOnAutoCreate()) 3028 throw new Error("Attempt to auto-create Observation.device"); 3029 else if (Configuration.doAutoCreate()) 3030 this.device = new Reference(); // cc 3031 return this.device; 3032 } 3033 3034 public boolean hasDevice() { 3035 return this.device != null && !this.device.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #device} (A reference to the device that generates the measurements or the device settings for the device.) 3040 */ 3041 public Observation setDevice(Reference value) { 3042 this.device = value; 3043 return this; 3044 } 3045 3046 /** 3047 * @return {@link #referenceRange} (Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an "OR". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.) 3048 */ 3049 public List<ObservationReferenceRangeComponent> getReferenceRange() { 3050 if (this.referenceRange == null) 3051 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3052 return this.referenceRange; 3053 } 3054 3055 /** 3056 * @return Returns a reference to <code>this</code> for easy method chaining 3057 */ 3058 public Observation setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 3059 this.referenceRange = theReferenceRange; 3060 return this; 3061 } 3062 3063 public boolean hasReferenceRange() { 3064 if (this.referenceRange == null) 3065 return false; 3066 for (ObservationReferenceRangeComponent item : this.referenceRange) 3067 if (!item.isEmpty()) 3068 return true; 3069 return false; 3070 } 3071 3072 public ObservationReferenceRangeComponent addReferenceRange() { //3 3073 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 3074 if (this.referenceRange == null) 3075 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3076 this.referenceRange.add(t); 3077 return t; 3078 } 3079 3080 public Observation addReferenceRange(ObservationReferenceRangeComponent t) { //3 3081 if (t == null) 3082 return this; 3083 if (this.referenceRange == null) 3084 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3085 this.referenceRange.add(t); 3086 return this; 3087 } 3088 3089 /** 3090 * @return The first repetition of repeating field {@link #referenceRange}, creating it if it does not already exist {3} 3091 */ 3092 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 3093 if (getReferenceRange().isEmpty()) { 3094 addReferenceRange(); 3095 } 3096 return getReferenceRange().get(0); 3097 } 3098 3099 /** 3100 * @return {@link #hasMember} (This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.) 3101 */ 3102 public List<Reference> getHasMember() { 3103 if (this.hasMember == null) 3104 this.hasMember = new ArrayList<Reference>(); 3105 return this.hasMember; 3106 } 3107 3108 /** 3109 * @return Returns a reference to <code>this</code> for easy method chaining 3110 */ 3111 public Observation setHasMember(List<Reference> theHasMember) { 3112 this.hasMember = theHasMember; 3113 return this; 3114 } 3115 3116 public boolean hasHasMember() { 3117 if (this.hasMember == null) 3118 return false; 3119 for (Reference item : this.hasMember) 3120 if (!item.isEmpty()) 3121 return true; 3122 return false; 3123 } 3124 3125 public Reference addHasMember() { //3 3126 Reference t = new Reference(); 3127 if (this.hasMember == null) 3128 this.hasMember = new ArrayList<Reference>(); 3129 this.hasMember.add(t); 3130 return t; 3131 } 3132 3133 public Observation addHasMember(Reference t) { //3 3134 if (t == null) 3135 return this; 3136 if (this.hasMember == null) 3137 this.hasMember = new ArrayList<Reference>(); 3138 this.hasMember.add(t); 3139 return this; 3140 } 3141 3142 /** 3143 * @return The first repetition of repeating field {@link #hasMember}, creating it if it does not already exist {3} 3144 */ 3145 public Reference getHasMemberFirstRep() { 3146 if (getHasMember().isEmpty()) { 3147 addHasMember(); 3148 } 3149 return getHasMember().get(0); 3150 } 3151 3152 /** 3153 * @return {@link #derivedFrom} (The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.) 3154 */ 3155 public List<Reference> getDerivedFrom() { 3156 if (this.derivedFrom == null) 3157 this.derivedFrom = new ArrayList<Reference>(); 3158 return this.derivedFrom; 3159 } 3160 3161 /** 3162 * @return Returns a reference to <code>this</code> for easy method chaining 3163 */ 3164 public Observation setDerivedFrom(List<Reference> theDerivedFrom) { 3165 this.derivedFrom = theDerivedFrom; 3166 return this; 3167 } 3168 3169 public boolean hasDerivedFrom() { 3170 if (this.derivedFrom == null) 3171 return false; 3172 for (Reference item : this.derivedFrom) 3173 if (!item.isEmpty()) 3174 return true; 3175 return false; 3176 } 3177 3178 public Reference addDerivedFrom() { //3 3179 Reference t = new Reference(); 3180 if (this.derivedFrom == null) 3181 this.derivedFrom = new ArrayList<Reference>(); 3182 this.derivedFrom.add(t); 3183 return t; 3184 } 3185 3186 public Observation addDerivedFrom(Reference t) { //3 3187 if (t == null) 3188 return this; 3189 if (this.derivedFrom == null) 3190 this.derivedFrom = new ArrayList<Reference>(); 3191 this.derivedFrom.add(t); 3192 return this; 3193 } 3194 3195 /** 3196 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 3197 */ 3198 public Reference getDerivedFromFirstRep() { 3199 if (getDerivedFrom().isEmpty()) { 3200 addDerivedFrom(); 3201 } 3202 return getDerivedFrom().get(0); 3203 } 3204 3205 /** 3206 * @return {@link #component} (Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.) 3207 */ 3208 public List<ObservationComponentComponent> getComponent() { 3209 if (this.component == null) 3210 this.component = new ArrayList<ObservationComponentComponent>(); 3211 return this.component; 3212 } 3213 3214 /** 3215 * @return Returns a reference to <code>this</code> for easy method chaining 3216 */ 3217 public Observation setComponent(List<ObservationComponentComponent> theComponent) { 3218 this.component = theComponent; 3219 return this; 3220 } 3221 3222 public boolean hasComponent() { 3223 if (this.component == null) 3224 return false; 3225 for (ObservationComponentComponent item : this.component) 3226 if (!item.isEmpty()) 3227 return true; 3228 return false; 3229 } 3230 3231 public ObservationComponentComponent addComponent() { //3 3232 ObservationComponentComponent t = new ObservationComponentComponent(); 3233 if (this.component == null) 3234 this.component = new ArrayList<ObservationComponentComponent>(); 3235 this.component.add(t); 3236 return t; 3237 } 3238 3239 public Observation addComponent(ObservationComponentComponent t) { //3 3240 if (t == null) 3241 return this; 3242 if (this.component == null) 3243 this.component = new ArrayList<ObservationComponentComponent>(); 3244 this.component.add(t); 3245 return this; 3246 } 3247 3248 /** 3249 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 3250 */ 3251 public ObservationComponentComponent getComponentFirstRep() { 3252 if (getComponent().isEmpty()) { 3253 addComponent(); 3254 } 3255 return getComponent().get(0); 3256 } 3257 3258 protected void listChildren(List<Property> children) { 3259 super.listChildren(children); 3260 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3261 children.add(new Property("instantiates[x]", "canonical(ObservationDefinition)|Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates)); 3262 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3263 children.add(new Property("triggeredBy", "", "Identifies the observation(s) that triggered the performance of this observation.", 0, java.lang.Integer.MAX_VALUE, triggeredBy)); 3264 children.add(new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy|GenomicStudy)", "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 0, java.lang.Integer.MAX_VALUE, partOf)); 3265 children.add(new Property("status", "code", "The status of the result value.", 0, 1, status)); 3266 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, category)); 3267 children.add(new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 3268 children.add(new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|BiologicallyDerivedProduct|NutritionProduct)", "The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 0, 1, subject)); 3269 children.add(new Property("focus", "Reference(Any)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus)); 3270 children.add(new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 0, 1, encounter)); 3271 children.add(new Property("effective[x]", "dateTime|Period|Timing|instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective)); 3272 children.add(new Property("issued", "instant", "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 0, 1, issued)); 3273 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, performer)); 3274 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value)); 3275 children.add(new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason)); 3276 children.add(new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation)); 3277 children.add(new Property("note", "Annotation", "Comments about the observation or the results.", 0, java.lang.Integer.MAX_VALUE, note)); 3278 children.add(new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodySite)); 3279 children.add(new Property("bodyStructure", "Reference(BodyStructure)", "Indicates the body structure on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodyStructure)); 3280 children.add(new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 0, 1, method)); 3281 children.add(new Property("specimen", "Reference(Specimen|Group)", "The specimen that was used when this observation was made.", 0, 1, specimen)); 3282 children.add(new Property("device", "Reference(Device|DeviceMetric)", "A reference to the device that generates the measurements or the device settings for the device.", 0, 1, device)); 3283 children.add(new Property("referenceRange", "", "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 0, java.lang.Integer.MAX_VALUE, referenceRange)); 3284 children.add(new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember)); 3285 children.add(new Property("derivedFrom", "Reference(DocumentReference|ImagingStudy|ImagingSelection|QuestionnaireResponse|Observation|MolecularSequence|GenomicStudy)", "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 3286 children.add(new Property("component", "", "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 0, java.lang.Integer.MAX_VALUE, component)); 3287 } 3288 3289 @Override 3290 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3291 switch (_hash) { 3292 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier); 3293 case -1926387433: /*instantiates[x]*/ return new Property("instantiates[x]", "canonical(ObservationDefinition)|Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3294 case -246883639: /*instantiates*/ return new Property("instantiates[x]", "canonical(ObservationDefinition)|Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3295 case 8911915: /*instantiatesCanonical*/ return new Property("instantiates[x]", "canonical(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3296 case -1744595326: /*instantiatesReference*/ return new Property("instantiates[x]", "Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3297 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3298 case -680451314: /*triggeredBy*/ return new Property("triggeredBy", "", "Identifies the observation(s) that triggered the performance of this observation.", 0, java.lang.Integer.MAX_VALUE, triggeredBy); 3299 case -995410646: /*partOf*/ return new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy|GenomicStudy)", "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 0, java.lang.Integer.MAX_VALUE, partOf); 3300 case -892481550: /*status*/ return new Property("status", "code", "The status of the result value.", 0, 1, status); 3301 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, category); 3302 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code); 3303 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|BiologicallyDerivedProduct|NutritionProduct)", "The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 0, 1, subject); 3304 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus); 3305 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 0, 1, encounter); 3306 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period|Timing|instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3307 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period|Timing|instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3308 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3309 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "Period", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3310 case -285872943: /*effectiveTiming*/ return new Property("effective[x]", "Timing", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3311 case -1295730118: /*effectiveInstant*/ return new Property("effective[x]", "instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3312 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 0, 1, issued); 3313 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, performer); 3314 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3315 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3316 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3317 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3318 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3319 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3320 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3321 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3322 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3323 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3324 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3325 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3326 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3327 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3328 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3329 case 1034315687: /*dataAbsentReason*/ return new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason); 3330 case -297950712: /*interpretation*/ return new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation); 3331 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments about the observation or the results.", 0, java.lang.Integer.MAX_VALUE, note); 3332 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodySite); 3333 case -1001731599: /*bodyStructure*/ return new Property("bodyStructure", "Reference(BodyStructure)", "Indicates the body structure on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodyStructure); 3334 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 0, 1, method); 3335 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen|Group)", "The specimen that was used when this observation was made.", 0, 1, specimen); 3336 case -1335157162: /*device*/ return new Property("device", "Reference(Device|DeviceMetric)", "A reference to the device that generates the measurements or the device settings for the device.", 0, 1, device); 3337 case -1912545102: /*referenceRange*/ return new Property("referenceRange", "", "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 0, java.lang.Integer.MAX_VALUE, referenceRange); 3338 case -458019372: /*hasMember*/ return new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember); 3339 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(DocumentReference|ImagingStudy|ImagingSelection|QuestionnaireResponse|Observation|MolecularSequence|GenomicStudy)", "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 3340 case -1399907075: /*component*/ return new Property("component", "", "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 0, java.lang.Integer.MAX_VALUE, component); 3341 default: return super.getNamedProperty(_hash, _name, _checkValid); 3342 } 3343 3344 } 3345 3346 @Override 3347 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3348 switch (hash) { 3349 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3350 case -246883639: /*instantiates*/ return this.instantiates == null ? new Base[0] : new Base[] {this.instantiates}; // DataType 3351 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3352 case -680451314: /*triggeredBy*/ return this.triggeredBy == null ? new Base[0] : this.triggeredBy.toArray(new Base[this.triggeredBy.size()]); // ObservationTriggeredByComponent 3353 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 3354 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ObservationStatus> 3355 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3356 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3357 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3358 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 3359 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3360 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // DataType 3361 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 3362 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 3363 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3364 case 1034315687: /*dataAbsentReason*/ return this.dataAbsentReason == null ? new Base[0] : new Base[] {this.dataAbsentReason}; // CodeableConcept 3365 case -297950712: /*interpretation*/ return this.interpretation == null ? new Base[0] : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 3366 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3367 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 3368 case -1001731599: /*bodyStructure*/ return this.bodyStructure == null ? new Base[0] : new Base[] {this.bodyStructure}; // Reference 3369 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 3370 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : new Base[] {this.specimen}; // Reference 3371 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 3372 case -1912545102: /*referenceRange*/ return this.referenceRange == null ? new Base[0] : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 3373 case -458019372: /*hasMember*/ return this.hasMember == null ? new Base[0] : this.hasMember.toArray(new Base[this.hasMember.size()]); // Reference 3374 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 3375 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ObservationComponentComponent 3376 default: return super.getProperty(hash, name, checkValid); 3377 } 3378 3379 } 3380 3381 @Override 3382 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3383 switch (hash) { 3384 case -1618432855: // identifier 3385 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3386 return value; 3387 case -246883639: // instantiates 3388 this.instantiates = TypeConvertor.castToType(value); // DataType 3389 return value; 3390 case -332612366: // basedOn 3391 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 3392 return value; 3393 case -680451314: // triggeredBy 3394 this.getTriggeredBy().add((ObservationTriggeredByComponent) value); // ObservationTriggeredByComponent 3395 return value; 3396 case -995410646: // partOf 3397 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 3398 return value; 3399 case -892481550: // status 3400 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3401 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3402 return value; 3403 case 50511102: // category 3404 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3405 return value; 3406 case 3059181: // code 3407 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3408 return value; 3409 case -1867885268: // subject 3410 this.subject = TypeConvertor.castToReference(value); // Reference 3411 return value; 3412 case 97604824: // focus 3413 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 3414 return value; 3415 case 1524132147: // encounter 3416 this.encounter = TypeConvertor.castToReference(value); // Reference 3417 return value; 3418 case -1468651097: // effective 3419 this.effective = TypeConvertor.castToType(value); // DataType 3420 return value; 3421 case -1179159893: // issued 3422 this.issued = TypeConvertor.castToInstant(value); // InstantType 3423 return value; 3424 case 481140686: // performer 3425 this.getPerformer().add(TypeConvertor.castToReference(value)); // Reference 3426 return value; 3427 case 111972721: // value 3428 this.value = TypeConvertor.castToType(value); // DataType 3429 return value; 3430 case 1034315687: // dataAbsentReason 3431 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3432 return value; 3433 case -297950712: // interpretation 3434 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3435 return value; 3436 case 3387378: // note 3437 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3438 return value; 3439 case 1702620169: // bodySite 3440 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3441 return value; 3442 case -1001731599: // bodyStructure 3443 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 3444 return value; 3445 case -1077554975: // method 3446 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3447 return value; 3448 case -2132868344: // specimen 3449 this.specimen = TypeConvertor.castToReference(value); // Reference 3450 return value; 3451 case -1335157162: // device 3452 this.device = TypeConvertor.castToReference(value); // Reference 3453 return value; 3454 case -1912545102: // referenceRange 3455 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 3456 return value; 3457 case -458019372: // hasMember 3458 this.getHasMember().add(TypeConvertor.castToReference(value)); // Reference 3459 return value; 3460 case 1077922663: // derivedFrom 3461 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 3462 return value; 3463 case -1399907075: // component 3464 this.getComponent().add((ObservationComponentComponent) value); // ObservationComponentComponent 3465 return value; 3466 default: return super.setProperty(hash, name, value); 3467 } 3468 3469 } 3470 3471 @Override 3472 public Base setProperty(String name, Base value) throws FHIRException { 3473 if (name.equals("identifier")) { 3474 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3475 } else if (name.equals("instantiates[x]")) { 3476 this.instantiates = TypeConvertor.castToType(value); // DataType 3477 } else if (name.equals("basedOn")) { 3478 this.getBasedOn().add(TypeConvertor.castToReference(value)); 3479 } else if (name.equals("triggeredBy")) { 3480 this.getTriggeredBy().add((ObservationTriggeredByComponent) value); 3481 } else if (name.equals("partOf")) { 3482 this.getPartOf().add(TypeConvertor.castToReference(value)); 3483 } else if (name.equals("status")) { 3484 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3485 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3486 } else if (name.equals("category")) { 3487 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3488 } else if (name.equals("code")) { 3489 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3490 } else if (name.equals("subject")) { 3491 this.subject = TypeConvertor.castToReference(value); // Reference 3492 } else if (name.equals("focus")) { 3493 this.getFocus().add(TypeConvertor.castToReference(value)); 3494 } else if (name.equals("encounter")) { 3495 this.encounter = TypeConvertor.castToReference(value); // Reference 3496 } else if (name.equals("effective[x]")) { 3497 this.effective = TypeConvertor.castToType(value); // DataType 3498 } else if (name.equals("issued")) { 3499 this.issued = TypeConvertor.castToInstant(value); // InstantType 3500 } else if (name.equals("performer")) { 3501 this.getPerformer().add(TypeConvertor.castToReference(value)); 3502 } else if (name.equals("value[x]")) { 3503 this.value = TypeConvertor.castToType(value); // DataType 3504 } else if (name.equals("dataAbsentReason")) { 3505 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3506 } else if (name.equals("interpretation")) { 3507 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); 3508 } else if (name.equals("note")) { 3509 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3510 } else if (name.equals("bodySite")) { 3511 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3512 } else if (name.equals("bodyStructure")) { 3513 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 3514 } else if (name.equals("method")) { 3515 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3516 } else if (name.equals("specimen")) { 3517 this.specimen = TypeConvertor.castToReference(value); // Reference 3518 } else if (name.equals("device")) { 3519 this.device = TypeConvertor.castToReference(value); // Reference 3520 } else if (name.equals("referenceRange")) { 3521 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 3522 } else if (name.equals("hasMember")) { 3523 this.getHasMember().add(TypeConvertor.castToReference(value)); 3524 } else if (name.equals("derivedFrom")) { 3525 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 3526 } else if (name.equals("component")) { 3527 this.getComponent().add((ObservationComponentComponent) value); 3528 } else 3529 return super.setProperty(name, value); 3530 return value; 3531 } 3532 3533 @Override 3534 public void removeChild(String name, Base value) throws FHIRException { 3535 if (name.equals("identifier")) { 3536 this.getIdentifier().remove(value); 3537 } else if (name.equals("instantiates[x]")) { 3538 this.instantiates = null; 3539 } else if (name.equals("basedOn")) { 3540 this.getBasedOn().remove(value); 3541 } else if (name.equals("triggeredBy")) { 3542 this.getTriggeredBy().remove((ObservationTriggeredByComponent) value); 3543 } else if (name.equals("partOf")) { 3544 this.getPartOf().remove(value); 3545 } else if (name.equals("status")) { 3546 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3547 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3548 } else if (name.equals("category")) { 3549 this.getCategory().remove(value); 3550 } else if (name.equals("code")) { 3551 this.code = null; 3552 } else if (name.equals("subject")) { 3553 this.subject = null; 3554 } else if (name.equals("focus")) { 3555 this.getFocus().remove(value); 3556 } else if (name.equals("encounter")) { 3557 this.encounter = null; 3558 } else if (name.equals("effective[x]")) { 3559 this.effective = null; 3560 } else if (name.equals("issued")) { 3561 this.issued = null; 3562 } else if (name.equals("performer")) { 3563 this.getPerformer().remove(value); 3564 } else if (name.equals("value[x]")) { 3565 this.value = null; 3566 } else if (name.equals("dataAbsentReason")) { 3567 this.dataAbsentReason = null; 3568 } else if (name.equals("interpretation")) { 3569 this.getInterpretation().remove(value); 3570 } else if (name.equals("note")) { 3571 this.getNote().remove(value); 3572 } else if (name.equals("bodySite")) { 3573 this.bodySite = null; 3574 } else if (name.equals("bodyStructure")) { 3575 this.bodyStructure = null; 3576 } else if (name.equals("method")) { 3577 this.method = null; 3578 } else if (name.equals("specimen")) { 3579 this.specimen = null; 3580 } else if (name.equals("device")) { 3581 this.device = null; 3582 } else if (name.equals("referenceRange")) { 3583 this.getReferenceRange().remove((ObservationReferenceRangeComponent) value); 3584 } else if (name.equals("hasMember")) { 3585 this.getHasMember().remove(value); 3586 } else if (name.equals("derivedFrom")) { 3587 this.getDerivedFrom().remove(value); 3588 } else if (name.equals("component")) { 3589 this.getComponent().remove((ObservationComponentComponent) value); 3590 } else 3591 super.removeChild(name, value); 3592 3593 } 3594 3595 @Override 3596 public Base makeProperty(int hash, String name) throws FHIRException { 3597 switch (hash) { 3598 case -1618432855: return addIdentifier(); 3599 case -1926387433: return getInstantiates(); 3600 case -246883639: return getInstantiates(); 3601 case -332612366: return addBasedOn(); 3602 case -680451314: return addTriggeredBy(); 3603 case -995410646: return addPartOf(); 3604 case -892481550: return getStatusElement(); 3605 case 50511102: return addCategory(); 3606 case 3059181: return getCode(); 3607 case -1867885268: return getSubject(); 3608 case 97604824: return addFocus(); 3609 case 1524132147: return getEncounter(); 3610 case 247104889: return getEffective(); 3611 case -1468651097: return getEffective(); 3612 case -1179159893: return getIssuedElement(); 3613 case 481140686: return addPerformer(); 3614 case -1410166417: return getValue(); 3615 case 111972721: return getValue(); 3616 case 1034315687: return getDataAbsentReason(); 3617 case -297950712: return addInterpretation(); 3618 case 3387378: return addNote(); 3619 case 1702620169: return getBodySite(); 3620 case -1001731599: return getBodyStructure(); 3621 case -1077554975: return getMethod(); 3622 case -2132868344: return getSpecimen(); 3623 case -1335157162: return getDevice(); 3624 case -1912545102: return addReferenceRange(); 3625 case -458019372: return addHasMember(); 3626 case 1077922663: return addDerivedFrom(); 3627 case -1399907075: return addComponent(); 3628 default: return super.makeProperty(hash, name); 3629 } 3630 3631 } 3632 3633 @Override 3634 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3635 switch (hash) { 3636 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3637 case -246883639: /*instantiates*/ return new String[] {"canonical", "Reference"}; 3638 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3639 case -680451314: /*triggeredBy*/ return new String[] {}; 3640 case -995410646: /*partOf*/ return new String[] {"Reference"}; 3641 case -892481550: /*status*/ return new String[] {"code"}; 3642 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3643 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3644 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3645 case 97604824: /*focus*/ return new String[] {"Reference"}; 3646 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3647 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period", "Timing", "instant"}; 3648 case -1179159893: /*issued*/ return new String[] {"instant"}; 3649 case 481140686: /*performer*/ return new String[] {"Reference"}; 3650 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Ratio", "SampledData", "time", "dateTime", "Period", "Attachment", "Reference"}; 3651 case 1034315687: /*dataAbsentReason*/ return new String[] {"CodeableConcept"}; 3652 case -297950712: /*interpretation*/ return new String[] {"CodeableConcept"}; 3653 case 3387378: /*note*/ return new String[] {"Annotation"}; 3654 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 3655 case -1001731599: /*bodyStructure*/ return new String[] {"Reference"}; 3656 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 3657 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 3658 case -1335157162: /*device*/ return new String[] {"Reference"}; 3659 case -1912545102: /*referenceRange*/ return new String[] {}; 3660 case -458019372: /*hasMember*/ return new String[] {"Reference"}; 3661 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 3662 case -1399907075: /*component*/ return new String[] {}; 3663 default: return super.getTypesForProperty(hash, name); 3664 } 3665 3666 } 3667 3668 @Override 3669 public Base addChild(String name) throws FHIRException { 3670 if (name.equals("identifier")) { 3671 return addIdentifier(); 3672 } 3673 else if (name.equals("instantiatesCanonical")) { 3674 this.instantiates = new CanonicalType(); 3675 return this.instantiates; 3676 } 3677 else if (name.equals("instantiatesReference")) { 3678 this.instantiates = new Reference(); 3679 return this.instantiates; 3680 } 3681 else if (name.equals("basedOn")) { 3682 return addBasedOn(); 3683 } 3684 else if (name.equals("triggeredBy")) { 3685 return addTriggeredBy(); 3686 } 3687 else if (name.equals("partOf")) { 3688 return addPartOf(); 3689 } 3690 else if (name.equals("status")) { 3691 throw new FHIRException("Cannot call addChild on a singleton property Observation.status"); 3692 } 3693 else if (name.equals("category")) { 3694 return addCategory(); 3695 } 3696 else if (name.equals("code")) { 3697 this.code = new CodeableConcept(); 3698 return this.code; 3699 } 3700 else if (name.equals("subject")) { 3701 this.subject = new Reference(); 3702 return this.subject; 3703 } 3704 else if (name.equals("focus")) { 3705 return addFocus(); 3706 } 3707 else if (name.equals("encounter")) { 3708 this.encounter = new Reference(); 3709 return this.encounter; 3710 } 3711 else if (name.equals("effectiveDateTime")) { 3712 this.effective = new DateTimeType(); 3713 return this.effective; 3714 } 3715 else if (name.equals("effectivePeriod")) { 3716 this.effective = new Period(); 3717 return this.effective; 3718 } 3719 else if (name.equals("effectiveTiming")) { 3720 this.effective = new Timing(); 3721 return this.effective; 3722 } 3723 else if (name.equals("effectiveInstant")) { 3724 this.effective = new InstantType(); 3725 return this.effective; 3726 } 3727 else if (name.equals("issued")) { 3728 throw new FHIRException("Cannot call addChild on a singleton property Observation.issued"); 3729 } 3730 else if (name.equals("performer")) { 3731 return addPerformer(); 3732 } 3733 else if (name.equals("valueQuantity")) { 3734 this.value = new Quantity(); 3735 return this.value; 3736 } 3737 else if (name.equals("valueCodeableConcept")) { 3738 this.value = new CodeableConcept(); 3739 return this.value; 3740 } 3741 else if (name.equals("valueString")) { 3742 this.value = new StringType(); 3743 return this.value; 3744 } 3745 else if (name.equals("valueBoolean")) { 3746 this.value = new BooleanType(); 3747 return this.value; 3748 } 3749 else if (name.equals("valueInteger")) { 3750 this.value = new IntegerType(); 3751 return this.value; 3752 } 3753 else if (name.equals("valueRange")) { 3754 this.value = new Range(); 3755 return this.value; 3756 } 3757 else if (name.equals("valueRatio")) { 3758 this.value = new Ratio(); 3759 return this.value; 3760 } 3761 else if (name.equals("valueSampledData")) { 3762 this.value = new SampledData(); 3763 return this.value; 3764 } 3765 else if (name.equals("valueTime")) { 3766 this.value = new TimeType(); 3767 return this.value; 3768 } 3769 else if (name.equals("valueDateTime")) { 3770 this.value = new DateTimeType(); 3771 return this.value; 3772 } 3773 else if (name.equals("valuePeriod")) { 3774 this.value = new Period(); 3775 return this.value; 3776 } 3777 else if (name.equals("valueAttachment")) { 3778 this.value = new Attachment(); 3779 return this.value; 3780 } 3781 else if (name.equals("valueReference")) { 3782 this.value = new Reference(); 3783 return this.value; 3784 } 3785 else if (name.equals("dataAbsentReason")) { 3786 this.dataAbsentReason = new CodeableConcept(); 3787 return this.dataAbsentReason; 3788 } 3789 else if (name.equals("interpretation")) { 3790 return addInterpretation(); 3791 } 3792 else if (name.equals("note")) { 3793 return addNote(); 3794 } 3795 else if (name.equals("bodySite")) { 3796 this.bodySite = new CodeableConcept(); 3797 return this.bodySite; 3798 } 3799 else if (name.equals("bodyStructure")) { 3800 this.bodyStructure = new Reference(); 3801 return this.bodyStructure; 3802 } 3803 else if (name.equals("method")) { 3804 this.method = new CodeableConcept(); 3805 return this.method; 3806 } 3807 else if (name.equals("specimen")) { 3808 this.specimen = new Reference(); 3809 return this.specimen; 3810 } 3811 else if (name.equals("device")) { 3812 this.device = new Reference(); 3813 return this.device; 3814 } 3815 else if (name.equals("referenceRange")) { 3816 return addReferenceRange(); 3817 } 3818 else if (name.equals("hasMember")) { 3819 return addHasMember(); 3820 } 3821 else if (name.equals("derivedFrom")) { 3822 return addDerivedFrom(); 3823 } 3824 else if (name.equals("component")) { 3825 return addComponent(); 3826 } 3827 else 3828 return super.addChild(name); 3829 } 3830 3831 public String fhirType() { 3832 return "Observation"; 3833 3834 } 3835 3836 public Observation copy() { 3837 Observation dst = new Observation(); 3838 copyValues(dst); 3839 return dst; 3840 } 3841 3842 public void copyValues(Observation dst) { 3843 super.copyValues(dst); 3844 if (identifier != null) { 3845 dst.identifier = new ArrayList<Identifier>(); 3846 for (Identifier i : identifier) 3847 dst.identifier.add(i.copy()); 3848 }; 3849 dst.instantiates = instantiates == null ? null : instantiates.copy(); 3850 if (basedOn != null) { 3851 dst.basedOn = new ArrayList<Reference>(); 3852 for (Reference i : basedOn) 3853 dst.basedOn.add(i.copy()); 3854 }; 3855 if (triggeredBy != null) { 3856 dst.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 3857 for (ObservationTriggeredByComponent i : triggeredBy) 3858 dst.triggeredBy.add(i.copy()); 3859 }; 3860 if (partOf != null) { 3861 dst.partOf = new ArrayList<Reference>(); 3862 for (Reference i : partOf) 3863 dst.partOf.add(i.copy()); 3864 }; 3865 dst.status = status == null ? null : status.copy(); 3866 if (category != null) { 3867 dst.category = new ArrayList<CodeableConcept>(); 3868 for (CodeableConcept i : category) 3869 dst.category.add(i.copy()); 3870 }; 3871 dst.code = code == null ? null : code.copy(); 3872 dst.subject = subject == null ? null : subject.copy(); 3873 if (focus != null) { 3874 dst.focus = new ArrayList<Reference>(); 3875 for (Reference i : focus) 3876 dst.focus.add(i.copy()); 3877 }; 3878 dst.encounter = encounter == null ? null : encounter.copy(); 3879 dst.effective = effective == null ? null : effective.copy(); 3880 dst.issued = issued == null ? null : issued.copy(); 3881 if (performer != null) { 3882 dst.performer = new ArrayList<Reference>(); 3883 for (Reference i : performer) 3884 dst.performer.add(i.copy()); 3885 }; 3886 dst.value = value == null ? null : value.copy(); 3887 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 3888 if (interpretation != null) { 3889 dst.interpretation = new ArrayList<CodeableConcept>(); 3890 for (CodeableConcept i : interpretation) 3891 dst.interpretation.add(i.copy()); 3892 }; 3893 if (note != null) { 3894 dst.note = new ArrayList<Annotation>(); 3895 for (Annotation i : note) 3896 dst.note.add(i.copy()); 3897 }; 3898 dst.bodySite = bodySite == null ? null : bodySite.copy(); 3899 dst.bodyStructure = bodyStructure == null ? null : bodyStructure.copy(); 3900 dst.method = method == null ? null : method.copy(); 3901 dst.specimen = specimen == null ? null : specimen.copy(); 3902 dst.device = device == null ? null : device.copy(); 3903 if (referenceRange != null) { 3904 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3905 for (ObservationReferenceRangeComponent i : referenceRange) 3906 dst.referenceRange.add(i.copy()); 3907 }; 3908 if (hasMember != null) { 3909 dst.hasMember = new ArrayList<Reference>(); 3910 for (Reference i : hasMember) 3911 dst.hasMember.add(i.copy()); 3912 }; 3913 if (derivedFrom != null) { 3914 dst.derivedFrom = new ArrayList<Reference>(); 3915 for (Reference i : derivedFrom) 3916 dst.derivedFrom.add(i.copy()); 3917 }; 3918 if (component != null) { 3919 dst.component = new ArrayList<ObservationComponentComponent>(); 3920 for (ObservationComponentComponent i : component) 3921 dst.component.add(i.copy()); 3922 }; 3923 } 3924 3925 protected Observation typedCopy() { 3926 return copy(); 3927 } 3928 3929 @Override 3930 public boolean equalsDeep(Base other_) { 3931 if (!super.equalsDeep(other_)) 3932 return false; 3933 if (!(other_ instanceof Observation)) 3934 return false; 3935 Observation o = (Observation) other_; 3936 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiates, o.instantiates, true) 3937 && compareDeep(basedOn, o.basedOn, true) && compareDeep(triggeredBy, o.triggeredBy, true) && compareDeep(partOf, o.partOf, true) 3938 && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 3939 && compareDeep(subject, o.subject, true) && compareDeep(focus, o.focus, true) && compareDeep(encounter, o.encounter, true) 3940 && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) 3941 && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 3942 && compareDeep(interpretation, o.interpretation, true) && compareDeep(note, o.note, true) && compareDeep(bodySite, o.bodySite, true) 3943 && compareDeep(bodyStructure, o.bodyStructure, true) && compareDeep(method, o.method, true) && compareDeep(specimen, o.specimen, true) 3944 && compareDeep(device, o.device, true) && compareDeep(referenceRange, o.referenceRange, true) && compareDeep(hasMember, o.hasMember, true) 3945 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(component, o.component, true); 3946 } 3947 3948 @Override 3949 public boolean equalsShallow(Base other_) { 3950 if (!super.equalsShallow(other_)) 3951 return false; 3952 if (!(other_ instanceof Observation)) 3953 return false; 3954 Observation o = (Observation) other_; 3955 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true); 3956 } 3957 3958 public boolean isEmpty() { 3959 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiates, basedOn 3960 , triggeredBy, partOf, status, category, code, subject, focus, encounter, effective 3961 , issued, performer, value, dataAbsentReason, interpretation, note, bodySite, bodyStructure 3962 , method, specimen, device, referenceRange, hasMember, derivedFrom, component 3963 ); 3964 } 3965 3966 @Override 3967 public ResourceType getResourceType() { 3968 return ResourceType.Observation; 3969 } 3970 3971 /** 3972 * Search parameter: <b>based-on</b> 3973 * <p> 3974 * Description: <b>Reference to the service request.</b><br> 3975 * Type: <b>reference</b><br> 3976 * Path: <b>Observation.basedOn</b><br> 3977 * </p> 3978 */ 3979 @SearchParamDefinition(name="based-on", path="Observation.basedOn", description="Reference to the service request.", type="reference", target={CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class } ) 3980 public static final String SP_BASED_ON = "based-on"; 3981 /** 3982 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3983 * <p> 3984 * Description: <b>Reference to the service request.</b><br> 3985 * Type: <b>reference</b><br> 3986 * Path: <b>Observation.basedOn</b><br> 3987 * </p> 3988 */ 3989 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3990 3991/** 3992 * Constant for fluent queries to be used to add include statements. Specifies 3993 * the path value of "<b>Observation:based-on</b>". 3994 */ 3995 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Observation:based-on").toLocked(); 3996 3997 /** 3998 * Search parameter: <b>category</b> 3999 * <p> 4000 * Description: <b>The classification of the type of observation</b><br> 4001 * Type: <b>token</b><br> 4002 * Path: <b>Observation.category</b><br> 4003 * </p> 4004 */ 4005 @SearchParamDefinition(name="category", path="Observation.category", description="The classification of the type of observation", type="token" ) 4006 public static final String SP_CATEGORY = "category"; 4007 /** 4008 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4009 * <p> 4010 * Description: <b>The classification of the type of observation</b><br> 4011 * Type: <b>token</b><br> 4012 * Path: <b>Observation.category</b><br> 4013 * </p> 4014 */ 4015 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4016 4017 /** 4018 * Search parameter: <b>code-value-concept</b> 4019 * <p> 4020 * Description: <b>Code and coded value parameter pair</b><br> 4021 * Type: <b>composite</b><br> 4022 * Path: <b>Observation</b><br> 4023 * </p> 4024 */ 4025 @SearchParamDefinition(name="code-value-concept", path="Observation", description="Code and coded value parameter pair", type="composite", compositeOf={"code", "value-concept"} ) 4026 public static final String SP_CODE_VALUE_CONCEPT = "code-value-concept"; 4027 /** 4028 * <b>Fluent Client</b> search parameter constant for <b>code-value-concept</b> 4029 * <p> 4030 * Description: <b>Code and coded value parameter pair</b><br> 4031 * Type: <b>composite</b><br> 4032 * Path: <b>Observation</b><br> 4033 * </p> 4034 */ 4035 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CODE_VALUE_CONCEPT); 4036 4037 /** 4038 * Search parameter: <b>code-value-date</b> 4039 * <p> 4040 * Description: <b>Code and date/time value parameter pair</b><br> 4041 * Type: <b>composite</b><br> 4042 * Path: <b>Observation</b><br> 4043 * </p> 4044 */ 4045 @SearchParamDefinition(name="code-value-date", path="Observation", description="Code and date/time value parameter pair", type="composite", compositeOf={"code", "value-date"} ) 4046 public static final String SP_CODE_VALUE_DATE = "code-value-date"; 4047 /** 4048 * <b>Fluent Client</b> search parameter constant for <b>code-value-date</b> 4049 * <p> 4050 * Description: <b>Code and date/time value parameter pair</b><br> 4051 * Type: <b>composite</b><br> 4052 * Path: <b>Observation</b><br> 4053 * </p> 4054 */ 4055 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam> CODE_VALUE_DATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam>(SP_CODE_VALUE_DATE); 4056 4057 /** 4058 * Search parameter: <b>code-value-quantity</b> 4059 * <p> 4060 * Description: <b>Code and quantity value parameter pair</b><br> 4061 * Type: <b>composite</b><br> 4062 * Path: <b>Observation</b><br> 4063 * </p> 4064 */ 4065 @SearchParamDefinition(name="code-value-quantity", path="Observation", description="Code and quantity value parameter pair", type="composite", compositeOf={"code", "value-quantity"} ) 4066 public static final String SP_CODE_VALUE_QUANTITY = "code-value-quantity"; 4067 /** 4068 * <b>Fluent Client</b> search parameter constant for <b>code-value-quantity</b> 4069 * <p> 4070 * Description: <b>Code and quantity value parameter pair</b><br> 4071 * Type: <b>composite</b><br> 4072 * Path: <b>Observation</b><br> 4073 * </p> 4074 */ 4075 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CODE_VALUE_QUANTITY); 4076 4077 /** 4078 * Search parameter: <b>combo-code-value-concept</b> 4079 * <p> 4080 * Description: <b>Code and coded value parameter pair, including in components</b><br> 4081 * Type: <b>composite</b><br> 4082 * Path: <b>Observation | Observation.component</b><br> 4083 * </p> 4084 */ 4085 @SearchParamDefinition(name="combo-code-value-concept", path="Observation | Observation.component", description="Code and coded value parameter pair, including in components", type="composite", compositeOf={"combo-code", "combo-value-concept"} ) 4086 public static final String SP_COMBO_CODE_VALUE_CONCEPT = "combo-code-value-concept"; 4087 /** 4088 * <b>Fluent Client</b> search parameter constant for <b>combo-code-value-concept</b> 4089 * <p> 4090 * Description: <b>Code and coded value parameter pair, including in components</b><br> 4091 * Type: <b>composite</b><br> 4092 * Path: <b>Observation | Observation.component</b><br> 4093 * </p> 4094 */ 4095 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMBO_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_COMBO_CODE_VALUE_CONCEPT); 4096 4097 /** 4098 * Search parameter: <b>combo-code-value-quantity</b> 4099 * <p> 4100 * Description: <b>Code and quantity value parameter pair, including in components</b><br> 4101 * Type: <b>composite</b><br> 4102 * Path: <b>Observation | Observation.component</b><br> 4103 * </p> 4104 */ 4105 @SearchParamDefinition(name="combo-code-value-quantity", path="Observation | Observation.component", description="Code and quantity value parameter pair, including in components", type="composite", compositeOf={"combo-code", "combo-value-quantity"} ) 4106 public static final String SP_COMBO_CODE_VALUE_QUANTITY = "combo-code-value-quantity"; 4107 /** 4108 * <b>Fluent Client</b> search parameter constant for <b>combo-code-value-quantity</b> 4109 * <p> 4110 * Description: <b>Code and quantity value parameter pair, including in components</b><br> 4111 * Type: <b>composite</b><br> 4112 * Path: <b>Observation | Observation.component</b><br> 4113 * </p> 4114 */ 4115 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMBO_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_COMBO_CODE_VALUE_QUANTITY); 4116 4117 /** 4118 * Search parameter: <b>combo-code</b> 4119 * <p> 4120 * Description: <b>The code of the observation type or component type</b><br> 4121 * Type: <b>token</b><br> 4122 * Path: <b>Observation.code | Observation.component.code</b><br> 4123 * </p> 4124 */ 4125 @SearchParamDefinition(name="combo-code", path="Observation.code | Observation.component.code", description="The code of the observation type or component type", type="token" ) 4126 public static final String SP_COMBO_CODE = "combo-code"; 4127 /** 4128 * <b>Fluent Client</b> search parameter constant for <b>combo-code</b> 4129 * <p> 4130 * Description: <b>The code of the observation type or component type</b><br> 4131 * Type: <b>token</b><br> 4132 * Path: <b>Observation.code | Observation.component.code</b><br> 4133 * </p> 4134 */ 4135 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_CODE); 4136 4137 /** 4138 * Search parameter: <b>combo-data-absent-reason</b> 4139 * <p> 4140 * Description: <b>The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4141 * Type: <b>token</b><br> 4142 * Path: <b>Observation.dataAbsentReason | Observation.component.dataAbsentReason</b><br> 4143 * </p> 4144 */ 4145 @SearchParamDefinition(name="combo-data-absent-reason", path="Observation.dataAbsentReason | Observation.component.dataAbsentReason", description="The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.", type="token" ) 4146 public static final String SP_COMBO_DATA_ABSENT_REASON = "combo-data-absent-reason"; 4147 /** 4148 * <b>Fluent Client</b> search parameter constant for <b>combo-data-absent-reason</b> 4149 * <p> 4150 * Description: <b>The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4151 * Type: <b>token</b><br> 4152 * Path: <b>Observation.dataAbsentReason | Observation.component.dataAbsentReason</b><br> 4153 * </p> 4154 */ 4155 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_DATA_ABSENT_REASON); 4156 4157 /** 4158 * Search parameter: <b>combo-value-concept</b> 4159 * <p> 4160 * Description: <b>The value or component value of the observation, if the value is a CodeableConcept</b><br> 4161 * Type: <b>token</b><br> 4162 * Path: <b>Observation.value.ofType(CodeableConcept) | Observation.component.value.ofType(CodeableConcept)</b><br> 4163 * </p> 4164 */ 4165 @SearchParamDefinition(name="combo-value-concept", path="Observation.value.ofType(CodeableConcept) | Observation.component.value.ofType(CodeableConcept)", description="The value or component value of the observation, if the value is a CodeableConcept", type="token" ) 4166 public static final String SP_COMBO_VALUE_CONCEPT = "combo-value-concept"; 4167 /** 4168 * <b>Fluent Client</b> search parameter constant for <b>combo-value-concept</b> 4169 * <p> 4170 * Description: <b>The value or component value of the observation, if the value is a CodeableConcept</b><br> 4171 * Type: <b>token</b><br> 4172 * Path: <b>Observation.value.ofType(CodeableConcept) | Observation.component.value.ofType(CodeableConcept)</b><br> 4173 * </p> 4174 */ 4175 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_VALUE_CONCEPT); 4176 4177 /** 4178 * Search parameter: <b>combo-value-quantity</b> 4179 * <p> 4180 * Description: <b>The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4181 * Type: <b>quantity</b><br> 4182 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData) | Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4183 * </p> 4184 */ 4185 @SearchParamDefinition(name="combo-value-quantity", path="Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData) | Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)", description="The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 4186 public static final String SP_COMBO_VALUE_QUANTITY = "combo-value-quantity"; 4187 /** 4188 * <b>Fluent Client</b> search parameter constant for <b>combo-value-quantity</b> 4189 * <p> 4190 * Description: <b>The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4191 * Type: <b>quantity</b><br> 4192 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData) | Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4193 * </p> 4194 */ 4195 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMBO_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_COMBO_VALUE_QUANTITY); 4196 4197 /** 4198 * Search parameter: <b>component-code-value-concept</b> 4199 * <p> 4200 * Description: <b>Component code and component coded value parameter pair</b><br> 4201 * Type: <b>composite</b><br> 4202 * Path: <b>Observation.component</b><br> 4203 * </p> 4204 */ 4205 @SearchParamDefinition(name="component-code-value-concept", path="Observation.component", description="Component code and component coded value parameter pair", type="composite", compositeOf={"component-code", "component-value-concept"} ) 4206 public static final String SP_COMPONENT_CODE_VALUE_CONCEPT = "component-code-value-concept"; 4207 /** 4208 * <b>Fluent Client</b> search parameter constant for <b>component-code-value-concept</b> 4209 * <p> 4210 * Description: <b>Component code and component coded value parameter pair</b><br> 4211 * Type: <b>composite</b><br> 4212 * Path: <b>Observation.component</b><br> 4213 * </p> 4214 */ 4215 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMPONENT_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_COMPONENT_CODE_VALUE_CONCEPT); 4216 4217 /** 4218 * Search parameter: <b>component-code-value-quantity</b> 4219 * <p> 4220 * Description: <b>Component code and component quantity value parameter pair</b><br> 4221 * Type: <b>composite</b><br> 4222 * Path: <b>Observation.component</b><br> 4223 * </p> 4224 */ 4225 @SearchParamDefinition(name="component-code-value-quantity", path="Observation.component", description="Component code and component quantity value parameter pair", type="composite", compositeOf={"component-code", "component-value-quantity"} ) 4226 public static final String SP_COMPONENT_CODE_VALUE_QUANTITY = "component-code-value-quantity"; 4227 /** 4228 * <b>Fluent Client</b> search parameter constant for <b>component-code-value-quantity</b> 4229 * <p> 4230 * Description: <b>Component code and component quantity value parameter pair</b><br> 4231 * Type: <b>composite</b><br> 4232 * Path: <b>Observation.component</b><br> 4233 * </p> 4234 */ 4235 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMPONENT_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_COMPONENT_CODE_VALUE_QUANTITY); 4236 4237 /** 4238 * Search parameter: <b>component-code</b> 4239 * <p> 4240 * Description: <b>The component code of the observation type</b><br> 4241 * Type: <b>token</b><br> 4242 * Path: <b>Observation.component.code</b><br> 4243 * </p> 4244 */ 4245 @SearchParamDefinition(name="component-code", path="Observation.component.code", description="The component code of the observation type", type="token" ) 4246 public static final String SP_COMPONENT_CODE = "component-code"; 4247 /** 4248 * <b>Fluent Client</b> search parameter constant for <b>component-code</b> 4249 * <p> 4250 * Description: <b>The component code of the observation type</b><br> 4251 * Type: <b>token</b><br> 4252 * Path: <b>Observation.component.code</b><br> 4253 * </p> 4254 */ 4255 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_CODE); 4256 4257 /** 4258 * Search parameter: <b>component-data-absent-reason</b> 4259 * <p> 4260 * Description: <b>The reason why the expected value in the element Observation.component.value[x] is missing.</b><br> 4261 * Type: <b>token</b><br> 4262 * Path: <b>Observation.component.dataAbsentReason</b><br> 4263 * </p> 4264 */ 4265 @SearchParamDefinition(name="component-data-absent-reason", path="Observation.component.dataAbsentReason", description="The reason why the expected value in the element Observation.component.value[x] is missing.", type="token" ) 4266 public static final String SP_COMPONENT_DATA_ABSENT_REASON = "component-data-absent-reason"; 4267 /** 4268 * <b>Fluent Client</b> search parameter constant for <b>component-data-absent-reason</b> 4269 * <p> 4270 * Description: <b>The reason why the expected value in the element Observation.component.value[x] is missing.</b><br> 4271 * Type: <b>token</b><br> 4272 * Path: <b>Observation.component.dataAbsentReason</b><br> 4273 * </p> 4274 */ 4275 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_DATA_ABSENT_REASON); 4276 4277 /** 4278 * Search parameter: <b>component-value-canonical</b> 4279 * <p> 4280 * Description: <b>URL contained in valueCanonical.</b><br> 4281 * Type: <b>uri</b><br> 4282 * Path: <b>Observation.component.value.ofType(canonical)</b><br> 4283 * </p> 4284 */ 4285 @SearchParamDefinition(name="component-value-canonical", path="Observation.component.value.ofType(canonical)", description="URL contained in valueCanonical.", type="uri" ) 4286 public static final String SP_COMPONENT_VALUE_CANONICAL = "component-value-canonical"; 4287 /** 4288 * <b>Fluent Client</b> search parameter constant for <b>component-value-canonical</b> 4289 * <p> 4290 * Description: <b>URL contained in valueCanonical.</b><br> 4291 * Type: <b>uri</b><br> 4292 * Path: <b>Observation.component.value.ofType(canonical)</b><br> 4293 * </p> 4294 */ 4295 public static final ca.uhn.fhir.rest.gclient.UriClientParam COMPONENT_VALUE_CANONICAL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_COMPONENT_VALUE_CANONICAL); 4296 4297 /** 4298 * Search parameter: <b>component-value-concept</b> 4299 * <p> 4300 * Description: <b>The value of the component observation, if the value is a CodeableConcept</b><br> 4301 * Type: <b>token</b><br> 4302 * Path: <b>Observation.component.value.ofType(CodeableConcept)</b><br> 4303 * </p> 4304 */ 4305 @SearchParamDefinition(name="component-value-concept", path="Observation.component.value.ofType(CodeableConcept)", description="The value of the component observation, if the value is a CodeableConcept", type="token" ) 4306 public static final String SP_COMPONENT_VALUE_CONCEPT = "component-value-concept"; 4307 /** 4308 * <b>Fluent Client</b> search parameter constant for <b>component-value-concept</b> 4309 * <p> 4310 * Description: <b>The value of the component observation, if the value is a CodeableConcept</b><br> 4311 * Type: <b>token</b><br> 4312 * Path: <b>Observation.component.value.ofType(CodeableConcept)</b><br> 4313 * </p> 4314 */ 4315 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_VALUE_CONCEPT); 4316 4317 /** 4318 * Search parameter: <b>component-value-quantity</b> 4319 * <p> 4320 * Description: <b>The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4321 * Type: <b>quantity</b><br> 4322 * Path: <b>Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4323 * </p> 4324 */ 4325 @SearchParamDefinition(name="component-value-quantity", path="Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)", description="The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 4326 public static final String SP_COMPONENT_VALUE_QUANTITY = "component-value-quantity"; 4327 /** 4328 * <b>Fluent Client</b> search parameter constant for <b>component-value-quantity</b> 4329 * <p> 4330 * Description: <b>The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4331 * Type: <b>quantity</b><br> 4332 * Path: <b>Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4333 * </p> 4334 */ 4335 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMPONENT_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_COMPONENT_VALUE_QUANTITY); 4336 4337 /** 4338 * Search parameter: <b>component-value-reference</b> 4339 * <p> 4340 * Description: <b>Reference contained in valueReference.</b><br> 4341 * Type: <b>reference</b><br> 4342 * Path: <b>Observation.component.value.ofType(Reference)</b><br> 4343 * </p> 4344 */ 4345 @SearchParamDefinition(name="component-value-reference", path="Observation.component.value.ofType(Reference)", description="Reference contained in valueReference.", type="reference", target={MolecularSequence.class } ) 4346 public static final String SP_COMPONENT_VALUE_REFERENCE = "component-value-reference"; 4347 /** 4348 * <b>Fluent Client</b> search parameter constant for <b>component-value-reference</b> 4349 * <p> 4350 * Description: <b>Reference contained in valueReference.</b><br> 4351 * Type: <b>reference</b><br> 4352 * Path: <b>Observation.component.value.ofType(Reference)</b><br> 4353 * </p> 4354 */ 4355 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT_VALUE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPONENT_VALUE_REFERENCE); 4356 4357/** 4358 * Constant for fluent queries to be used to add include statements. Specifies 4359 * the path value of "<b>Observation:component-value-reference</b>". 4360 */ 4361 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT_VALUE_REFERENCE = new ca.uhn.fhir.model.api.Include("Observation:component-value-reference").toLocked(); 4362 4363 /** 4364 * Search parameter: <b>data-absent-reason</b> 4365 * <p> 4366 * Description: <b>The reason why the expected value in the element Observation.value[x] is missing.</b><br> 4367 * Type: <b>token</b><br> 4368 * Path: <b>Observation.dataAbsentReason</b><br> 4369 * </p> 4370 */ 4371 @SearchParamDefinition(name="data-absent-reason", path="Observation.dataAbsentReason", description="The reason why the expected value in the element Observation.value[x] is missing.", type="token" ) 4372 public static final String SP_DATA_ABSENT_REASON = "data-absent-reason"; 4373 /** 4374 * <b>Fluent Client</b> search parameter constant for <b>data-absent-reason</b> 4375 * <p> 4376 * Description: <b>The reason why the expected value in the element Observation.value[x] is missing.</b><br> 4377 * Type: <b>token</b><br> 4378 * Path: <b>Observation.dataAbsentReason</b><br> 4379 * </p> 4380 */ 4381 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DATA_ABSENT_REASON); 4382 4383 /** 4384 * Search parameter: <b>derived-from</b> 4385 * <p> 4386 * Description: <b>Related measurements the observation is made from</b><br> 4387 * Type: <b>reference</b><br> 4388 * Path: <b>Observation.derivedFrom</b><br> 4389 * </p> 4390 */ 4391 @SearchParamDefinition(name="derived-from", path="Observation.derivedFrom", description="Related measurements the observation is made from", type="reference", target={DocumentReference.class, GenomicStudy.class, ImagingSelection.class, ImagingStudy.class, MolecularSequence.class, Observation.class, QuestionnaireResponse.class } ) 4392 public static final String SP_DERIVED_FROM = "derived-from"; 4393 /** 4394 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4395 * <p> 4396 * Description: <b>Related measurements the observation is made from</b><br> 4397 * Type: <b>reference</b><br> 4398 * Path: <b>Observation.derivedFrom</b><br> 4399 * </p> 4400 */ 4401 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4402 4403/** 4404 * Constant for fluent queries to be used to add include statements. Specifies 4405 * the path value of "<b>Observation:derived-from</b>". 4406 */ 4407 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Observation:derived-from").toLocked(); 4408 4409 /** 4410 * Search parameter: <b>device</b> 4411 * <p> 4412 * Description: <b>The Device that generated the observation data.</b><br> 4413 * Type: <b>reference</b><br> 4414 * Path: <b>Observation.device</b><br> 4415 * </p> 4416 */ 4417 @SearchParamDefinition(name="device", path="Observation.device", description="The Device that generated the observation data.", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class, DeviceMetric.class } ) 4418 public static final String SP_DEVICE = "device"; 4419 /** 4420 * <b>Fluent Client</b> search parameter constant for <b>device</b> 4421 * <p> 4422 * Description: <b>The Device that generated the observation data.</b><br> 4423 * Type: <b>reference</b><br> 4424 * Path: <b>Observation.device</b><br> 4425 * </p> 4426 */ 4427 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 4428 4429/** 4430 * Constant for fluent queries to be used to add include statements. Specifies 4431 * the path value of "<b>Observation:device</b>". 4432 */ 4433 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("Observation:device").toLocked(); 4434 4435 /** 4436 * Search parameter: <b>focus</b> 4437 * <p> 4438 * Description: <b>The focus of an observation when the focus is not the patient of record.</b><br> 4439 * Type: <b>reference</b><br> 4440 * Path: <b>Observation.focus</b><br> 4441 * </p> 4442 */ 4443 @SearchParamDefinition(name="focus", path="Observation.focus", description="The focus of an observation when the focus is not the patient of record.", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4444 public static final String SP_FOCUS = "focus"; 4445 /** 4446 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 4447 * <p> 4448 * Description: <b>The focus of an observation when the focus is not the patient of record.</b><br> 4449 * Type: <b>reference</b><br> 4450 * Path: <b>Observation.focus</b><br> 4451 * </p> 4452 */ 4453 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 4454 4455/** 4456 * Constant for fluent queries to be used to add include statements. Specifies 4457 * the path value of "<b>Observation:focus</b>". 4458 */ 4459 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("Observation:focus").toLocked(); 4460 4461 /** 4462 * Search parameter: <b>has-member</b> 4463 * <p> 4464 * Description: <b>Related resource that belongs to the Observation group</b><br> 4465 * Type: <b>reference</b><br> 4466 * Path: <b>Observation.hasMember</b><br> 4467 * </p> 4468 */ 4469 @SearchParamDefinition(name="has-member", path="Observation.hasMember", description="Related resource that belongs to the Observation group", type="reference", target={MolecularSequence.class, Observation.class, QuestionnaireResponse.class } ) 4470 public static final String SP_HAS_MEMBER = "has-member"; 4471 /** 4472 * <b>Fluent Client</b> search parameter constant for <b>has-member</b> 4473 * <p> 4474 * Description: <b>Related resource that belongs to the Observation group</b><br> 4475 * Type: <b>reference</b><br> 4476 * Path: <b>Observation.hasMember</b><br> 4477 * </p> 4478 */ 4479 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam HAS_MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_HAS_MEMBER); 4480 4481/** 4482 * Constant for fluent queries to be used to add include statements. Specifies 4483 * the path value of "<b>Observation:has-member</b>". 4484 */ 4485 public static final ca.uhn.fhir.model.api.Include INCLUDE_HAS_MEMBER = new ca.uhn.fhir.model.api.Include("Observation:has-member").toLocked(); 4486 4487 /** 4488 * Search parameter: <b>method</b> 4489 * <p> 4490 * Description: <b>The method used for the observation</b><br> 4491 * Type: <b>token</b><br> 4492 * Path: <b>Observation.method</b><br> 4493 * </p> 4494 */ 4495 @SearchParamDefinition(name="method", path="Observation.method", description="The method used for the observation", type="token" ) 4496 public static final String SP_METHOD = "method"; 4497 /** 4498 * <b>Fluent Client</b> search parameter constant for <b>method</b> 4499 * <p> 4500 * Description: <b>The method used for the observation</b><br> 4501 * Type: <b>token</b><br> 4502 * Path: <b>Observation.method</b><br> 4503 * </p> 4504 */ 4505 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_METHOD); 4506 4507 /** 4508 * Search parameter: <b>part-of</b> 4509 * <p> 4510 * Description: <b>Part of referenced event</b><br> 4511 * Type: <b>reference</b><br> 4512 * Path: <b>Observation.partOf</b><br> 4513 * </p> 4514 */ 4515 @SearchParamDefinition(name="part-of", path="Observation.partOf", description="Part of referenced event", type="reference", target={GenomicStudy.class, ImagingStudy.class, Immunization.class, MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Procedure.class } ) 4516 public static final String SP_PART_OF = "part-of"; 4517 /** 4518 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4519 * <p> 4520 * Description: <b>Part of referenced event</b><br> 4521 * Type: <b>reference</b><br> 4522 * Path: <b>Observation.partOf</b><br> 4523 * </p> 4524 */ 4525 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 4526 4527/** 4528 * Constant for fluent queries to be used to add include statements. Specifies 4529 * the path value of "<b>Observation:part-of</b>". 4530 */ 4531 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Observation:part-of").toLocked(); 4532 4533 /** 4534 * Search parameter: <b>performer</b> 4535 * <p> 4536 * Description: <b>Who performed the observation</b><br> 4537 * Type: <b>reference</b><br> 4538 * Path: <b>Observation.performer</b><br> 4539 * </p> 4540 */ 4541 @SearchParamDefinition(name="performer", path="Observation.performer", description="Who performed the observation", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 4542 public static final String SP_PERFORMER = "performer"; 4543 /** 4544 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4545 * <p> 4546 * Description: <b>Who performed the observation</b><br> 4547 * Type: <b>reference</b><br> 4548 * Path: <b>Observation.performer</b><br> 4549 * </p> 4550 */ 4551 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 4552 4553/** 4554 * Constant for fluent queries to be used to add include statements. Specifies 4555 * the path value of "<b>Observation:performer</b>". 4556 */ 4557 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("Observation:performer").toLocked(); 4558 4559 /** 4560 * Search parameter: <b>specimen</b> 4561 * <p> 4562 * Description: <b>Specimen used for this observation</b><br> 4563 * Type: <b>reference</b><br> 4564 * Path: <b>Observation.specimen</b><br> 4565 * </p> 4566 */ 4567 @SearchParamDefinition(name="specimen", path="Observation.specimen", description="Specimen used for this observation", type="reference", target={Group.class, Specimen.class } ) 4568 public static final String SP_SPECIMEN = "specimen"; 4569 /** 4570 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 4571 * <p> 4572 * Description: <b>Specimen used for this observation</b><br> 4573 * Type: <b>reference</b><br> 4574 * Path: <b>Observation.specimen</b><br> 4575 * </p> 4576 */ 4577 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 4578 4579/** 4580 * Constant for fluent queries to be used to add include statements. Specifies 4581 * the path value of "<b>Observation:specimen</b>". 4582 */ 4583 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("Observation:specimen").toLocked(); 4584 4585 /** 4586 * Search parameter: <b>status</b> 4587 * <p> 4588 * Description: <b>The status of the observation</b><br> 4589 * Type: <b>token</b><br> 4590 * Path: <b>Observation.status</b><br> 4591 * </p> 4592 */ 4593 @SearchParamDefinition(name="status", path="Observation.status", description="The status of the observation", type="token" ) 4594 public static final String SP_STATUS = "status"; 4595 /** 4596 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4597 * <p> 4598 * Description: <b>The status of the observation</b><br> 4599 * Type: <b>token</b><br> 4600 * Path: <b>Observation.status</b><br> 4601 * </p> 4602 */ 4603 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4604 4605 /** 4606 * Search parameter: <b>subject</b> 4607 * <p> 4608 * Description: <b>The subject that the observation is about</b><br> 4609 * Type: <b>reference</b><br> 4610 * Path: <b>Observation.subject</b><br> 4611 * </p> 4612 */ 4613 @SearchParamDefinition(name="subject", path="Observation.subject", description="The subject that the observation is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={BiologicallyDerivedProduct.class, Device.class, Group.class, Location.class, Medication.class, NutritionProduct.class, Organization.class, Patient.class, Practitioner.class, Procedure.class, Substance.class } ) 4614 public static final String SP_SUBJECT = "subject"; 4615 /** 4616 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4617 * <p> 4618 * Description: <b>The subject that the observation is about</b><br> 4619 * Type: <b>reference</b><br> 4620 * Path: <b>Observation.subject</b><br> 4621 * </p> 4622 */ 4623 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4624 4625/** 4626 * Constant for fluent queries to be used to add include statements. Specifies 4627 * the path value of "<b>Observation:subject</b>". 4628 */ 4629 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Observation:subject").toLocked(); 4630 4631 /** 4632 * Search parameter: <b>value-canonical</b> 4633 * <p> 4634 * Description: <b>URL contained in valueCanonical.</b><br> 4635 * Type: <b>uri</b><br> 4636 * Path: <b>Observation.value.ofType(canonical)</b><br> 4637 * </p> 4638 */ 4639 @SearchParamDefinition(name="value-canonical", path="Observation.value.ofType(canonical)", description="URL contained in valueCanonical.", type="uri" ) 4640 public static final String SP_VALUE_CANONICAL = "value-canonical"; 4641 /** 4642 * <b>Fluent Client</b> search parameter constant for <b>value-canonical</b> 4643 * <p> 4644 * Description: <b>URL contained in valueCanonical.</b><br> 4645 * Type: <b>uri</b><br> 4646 * Path: <b>Observation.value.ofType(canonical)</b><br> 4647 * </p> 4648 */ 4649 public static final ca.uhn.fhir.rest.gclient.UriClientParam VALUE_CANONICAL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_VALUE_CANONICAL); 4650 4651 /** 4652 * Search parameter: <b>value-concept</b> 4653 * <p> 4654 * Description: <b>The value of the observation, if the value is a CodeableConcept</b><br> 4655 * Type: <b>token</b><br> 4656 * Path: <b>Observation.value.ofType(CodeableConcept)</b><br> 4657 * </p> 4658 */ 4659 @SearchParamDefinition(name="value-concept", path="Observation.value.ofType(CodeableConcept)", description="The value of the observation, if the value is a CodeableConcept", type="token" ) 4660 public static final String SP_VALUE_CONCEPT = "value-concept"; 4661 /** 4662 * <b>Fluent Client</b> search parameter constant for <b>value-concept</b> 4663 * <p> 4664 * Description: <b>The value of the observation, if the value is a CodeableConcept</b><br> 4665 * Type: <b>token</b><br> 4666 * Path: <b>Observation.value.ofType(CodeableConcept)</b><br> 4667 * </p> 4668 */ 4669 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VALUE_CONCEPT); 4670 4671 /** 4672 * Search parameter: <b>value-date</b> 4673 * <p> 4674 * Description: <b>The value of the observation, if the value is a date or period of time</b><br> 4675 * Type: <b>date</b><br> 4676 * Path: <b>Observation.value.ofType(dateTime) | Observation.value.ofType(Period)</b><br> 4677 * </p> 4678 */ 4679 @SearchParamDefinition(name="value-date", path="Observation.value.ofType(dateTime) | Observation.value.ofType(Period)", description="The value of the observation, if the value is a date or period of time", type="date" ) 4680 public static final String SP_VALUE_DATE = "value-date"; 4681 /** 4682 * <b>Fluent Client</b> search parameter constant for <b>value-date</b> 4683 * <p> 4684 * Description: <b>The value of the observation, if the value is a date or period of time</b><br> 4685 * Type: <b>date</b><br> 4686 * Path: <b>Observation.value.ofType(dateTime) | Observation.value.ofType(Period)</b><br> 4687 * </p> 4688 */ 4689 public static final ca.uhn.fhir.rest.gclient.DateClientParam VALUE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_VALUE_DATE); 4690 4691 /** 4692 * Search parameter: <b>value-markdown</b> 4693 * <p> 4694 * Description: <b>The value of the observation, if the value is a string, and also searches in CodeableConcept.text</b><br> 4695 * Type: <b>string</b><br> 4696 * Path: <b>Observation.value.ofType(markdown) | Observation.value.ofType(CodeableConcept).text</b><br> 4697 * </p> 4698 */ 4699 @SearchParamDefinition(name="value-markdown", path="Observation.value.ofType(markdown) | Observation.value.ofType(CodeableConcept).text", description="The value of the observation, if the value is a string, and also searches in CodeableConcept.text", type="string" ) 4700 public static final String SP_VALUE_MARKDOWN = "value-markdown"; 4701 /** 4702 * <b>Fluent Client</b> search parameter constant for <b>value-markdown</b> 4703 * <p> 4704 * Description: <b>The value of the observation, if the value is a string, and also searches in CodeableConcept.text</b><br> 4705 * Type: <b>string</b><br> 4706 * Path: <b>Observation.value.ofType(markdown) | Observation.value.ofType(CodeableConcept).text</b><br> 4707 * </p> 4708 */ 4709 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE_MARKDOWN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VALUE_MARKDOWN); 4710 4711 /** 4712 * Search parameter: <b>value-quantity</b> 4713 * <p> 4714 * Description: <b>The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4715 * Type: <b>quantity</b><br> 4716 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData)</b><br> 4717 * </p> 4718 */ 4719 @SearchParamDefinition(name="value-quantity", path="Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData)", description="The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 4720 public static final String SP_VALUE_QUANTITY = "value-quantity"; 4721 /** 4722 * <b>Fluent Client</b> search parameter constant for <b>value-quantity</b> 4723 * <p> 4724 * Description: <b>The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4725 * Type: <b>quantity</b><br> 4726 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData)</b><br> 4727 * </p> 4728 */ 4729 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_VALUE_QUANTITY); 4730 4731 /** 4732 * Search parameter: <b>value-reference</b> 4733 * <p> 4734 * Description: <b>Reference contained in valueReference.</b><br> 4735 * Type: <b>reference</b><br> 4736 * Path: <b>Observation.value.ofType(Reference)</b><br> 4737 * </p> 4738 */ 4739 @SearchParamDefinition(name="value-reference", path="Observation.value.ofType(Reference)", description="Reference contained in valueReference.", type="reference", target={MolecularSequence.class } ) 4740 public static final String SP_VALUE_REFERENCE = "value-reference"; 4741 /** 4742 * <b>Fluent Client</b> search parameter constant for <b>value-reference</b> 4743 * <p> 4744 * Description: <b>Reference contained in valueReference.</b><br> 4745 * Type: <b>reference</b><br> 4746 * Path: <b>Observation.value.ofType(Reference)</b><br> 4747 * </p> 4748 */ 4749 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam VALUE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_VALUE_REFERENCE); 4750 4751/** 4752 * Constant for fluent queries to be used to add include statements. Specifies 4753 * the path value of "<b>Observation:value-reference</b>". 4754 */ 4755 public static final ca.uhn.fhir.model.api.Include INCLUDE_VALUE_REFERENCE = new ca.uhn.fhir.model.api.Include("Observation:value-reference").toLocked(); 4756 4757 /** 4758 * Search parameter: <b>code</b> 4759 * <p> 4760 * Description: <b>Multiple Resources: 4761 4762* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4763* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4764* [AuditEvent](auditevent.html): More specific code for the event 4765* [Basic](basic.html): Kind of Resource 4766* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4767* [Condition](condition.html): Code for the condition 4768* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4769* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4770* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4771* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4772* [ImagingSelection](imagingselection.html): The imaging selection status 4773* [List](list.html): What the purpose of this list is 4774* [Medication](medication.html): Returns medications for a specific code 4775* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4776* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4777* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4778* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4779* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4780* [Observation](observation.html): The code of the observation type 4781* [Procedure](procedure.html): A code to identify a procedure 4782* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4783* [Task](task.html): Search by task code 4784</b><br> 4785 * Type: <b>token</b><br> 4786 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4787 * </p> 4788 */ 4789 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 4790 public static final String SP_CODE = "code"; 4791 /** 4792 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4793 * <p> 4794 * Description: <b>Multiple Resources: 4795 4796* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4797* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4798* [AuditEvent](auditevent.html): More specific code for the event 4799* [Basic](basic.html): Kind of Resource 4800* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4801* [Condition](condition.html): Code for the condition 4802* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4803* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4804* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4805* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4806* [ImagingSelection](imagingselection.html): The imaging selection status 4807* [List](list.html): What the purpose of this list is 4808* [Medication](medication.html): Returns medications for a specific code 4809* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4810* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4811* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4812* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4813* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4814* [Observation](observation.html): The code of the observation type 4815* [Procedure](procedure.html): A code to identify a procedure 4816* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4817* [Task](task.html): Search by task code 4818</b><br> 4819 * Type: <b>token</b><br> 4820 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4821 * </p> 4822 */ 4823 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4824 4825 /** 4826 * Search parameter: <b>date</b> 4827 * <p> 4828 * Description: <b>Multiple Resources: 4829 4830* [AdverseEvent](adverseevent.html): When the event occurred 4831* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4832* [Appointment](appointment.html): Appointment date/time. 4833* [AuditEvent](auditevent.html): Time when the event was recorded 4834* [CarePlan](careplan.html): Time period plan covers 4835* [CareTeam](careteam.html): A date within the coverage time period. 4836* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4837* [Composition](composition.html): Composition editing time 4838* [Consent](consent.html): When consent was agreed to 4839* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4840* [DocumentReference](documentreference.html): When this document reference was created 4841* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4842* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4843* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4844* [Flag](flag.html): Time period when flag is active 4845* [Immunization](immunization.html): Vaccination (non)-Administration Date 4846* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4847* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4848* [Invoice](invoice.html): Invoice date / posting date 4849* [List](list.html): When the list was prepared 4850* [MeasureReport](measurereport.html): The date of the measure report 4851* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4852* [Observation](observation.html): Clinically relevant time/time-period for observation 4853* [Procedure](procedure.html): When the procedure occurred or is occurring 4854* [ResearchSubject](researchsubject.html): Start and end of participation 4855* [RiskAssessment](riskassessment.html): When was assessment made? 4856* [SupplyRequest](supplyrequest.html): When the request was made 4857</b><br> 4858 * Type: <b>date</b><br> 4859 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4860 * </p> 4861 */ 4862 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4863 public static final String SP_DATE = "date"; 4864 /** 4865 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4866 * <p> 4867 * Description: <b>Multiple Resources: 4868 4869* [AdverseEvent](adverseevent.html): When the event occurred 4870* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4871* [Appointment](appointment.html): Appointment date/time. 4872* [AuditEvent](auditevent.html): Time when the event was recorded 4873* [CarePlan](careplan.html): Time period plan covers 4874* [CareTeam](careteam.html): A date within the coverage time period. 4875* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4876* [Composition](composition.html): Composition editing time 4877* [Consent](consent.html): When consent was agreed to 4878* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4879* [DocumentReference](documentreference.html): When this document reference was created 4880* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4881* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4882* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4883* [Flag](flag.html): Time period when flag is active 4884* [Immunization](immunization.html): Vaccination (non)-Administration Date 4885* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4886* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4887* [Invoice](invoice.html): Invoice date / posting date 4888* [List](list.html): When the list was prepared 4889* [MeasureReport](measurereport.html): The date of the measure report 4890* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4891* [Observation](observation.html): Clinically relevant time/time-period for observation 4892* [Procedure](procedure.html): When the procedure occurred or is occurring 4893* [ResearchSubject](researchsubject.html): Start and end of participation 4894* [RiskAssessment](riskassessment.html): When was assessment made? 4895* [SupplyRequest](supplyrequest.html): When the request was made 4896</b><br> 4897 * Type: <b>date</b><br> 4898 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4899 * </p> 4900 */ 4901 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4902 4903 /** 4904 * Search parameter: <b>encounter</b> 4905 * <p> 4906 * Description: <b>Multiple Resources: 4907 4908* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 4909* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 4910* [ChargeItem](chargeitem.html): Encounter associated with event 4911* [Claim](claim.html): Encounters associated with a billed line item 4912* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 4913* [Communication](communication.html): The Encounter during which this Communication was created 4914* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 4915* [Composition](composition.html): Context of the Composition 4916* [Condition](condition.html): The Encounter during which this Condition was created 4917* [DeviceRequest](devicerequest.html): Encounter during which request was created 4918* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 4919* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 4920* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 4921* [Flag](flag.html): Alert relevant during encounter 4922* [ImagingStudy](imagingstudy.html): The context of the study 4923* [List](list.html): Context in which list created 4924* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 4925* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 4926* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 4927* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 4928* [Observation](observation.html): Encounter related to the observation 4929* [Procedure](procedure.html): The Encounter during which this Procedure was created 4930* [Provenance](provenance.html): Encounter related to the Provenance 4931* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 4932* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 4933* [RiskAssessment](riskassessment.html): Where was assessment performed? 4934* [ServiceRequest](servicerequest.html): An encounter in which this request is made 4935* [Task](task.html): Search by encounter 4936* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 4937</b><br> 4938 * Type: <b>reference</b><br> 4939 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 4940 * </p> 4941 */ 4942 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 4943 public static final String SP_ENCOUNTER = "encounter"; 4944 /** 4945 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4946 * <p> 4947 * Description: <b>Multiple Resources: 4948 4949* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 4950* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 4951* [ChargeItem](chargeitem.html): Encounter associated with event 4952* [Claim](claim.html): Encounters associated with a billed line item 4953* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 4954* [Communication](communication.html): The Encounter during which this Communication was created 4955* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 4956* [Composition](composition.html): Context of the Composition 4957* [Condition](condition.html): The Encounter during which this Condition was created 4958* [DeviceRequest](devicerequest.html): Encounter during which request was created 4959* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 4960* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 4961* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 4962* [Flag](flag.html): Alert relevant during encounter 4963* [ImagingStudy](imagingstudy.html): The context of the study 4964* [List](list.html): Context in which list created 4965* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 4966* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 4967* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 4968* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 4969* [Observation](observation.html): Encounter related to the observation 4970* [Procedure](procedure.html): The Encounter during which this Procedure was created 4971* [Provenance](provenance.html): Encounter related to the Provenance 4972* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 4973* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 4974* [RiskAssessment](riskassessment.html): Where was assessment performed? 4975* [ServiceRequest](servicerequest.html): An encounter in which this request is made 4976* [Task](task.html): Search by encounter 4977* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 4978</b><br> 4979 * Type: <b>reference</b><br> 4980 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 4981 * </p> 4982 */ 4983 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4984 4985/** 4986 * Constant for fluent queries to be used to add include statements. Specifies 4987 * the path value of "<b>Observation:encounter</b>". 4988 */ 4989 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Observation:encounter").toLocked(); 4990 4991 /** 4992 * Search parameter: <b>identifier</b> 4993 * <p> 4994 * Description: <b>Multiple Resources: 4995 4996* [Account](account.html): Account number 4997* [AdverseEvent](adverseevent.html): Business identifier for the event 4998* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4999* [Appointment](appointment.html): An Identifier of the Appointment 5000* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 5001* [Basic](basic.html): Business identifier 5002* [BodyStructure](bodystructure.html): Bodystructure identifier 5003* [CarePlan](careplan.html): External Ids for this plan 5004* [CareTeam](careteam.html): External Ids for this team 5005* [ChargeItem](chargeitem.html): Business Identifier for item 5006* [Claim](claim.html): The primary identifier of the financial resource 5007* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 5008* [ClinicalImpression](clinicalimpression.html): Business identifier 5009* [Communication](communication.html): Unique identifier 5010* [CommunicationRequest](communicationrequest.html): Unique identifier 5011* [Composition](composition.html): Version-independent identifier for the Composition 5012* [Condition](condition.html): A unique identifier of the condition record 5013* [Consent](consent.html): Identifier for this record (external references) 5014* [Contract](contract.html): The identity of the contract 5015* [Coverage](coverage.html): The primary identifier of the insured and the coverage 5016* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 5017* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 5018* [DetectedIssue](detectedissue.html): Unique id for the detected issue 5019* [DeviceRequest](devicerequest.html): Business identifier for request/order 5020* [DeviceUsage](deviceusage.html): Search by identifier 5021* [DiagnosticReport](diagnosticreport.html): An identifier for the report 5022* [DocumentReference](documentreference.html): Identifier of the attachment binary 5023* [Encounter](encounter.html): Identifier(s) by which this encounter is known 5024* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 5025* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 5026* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 5027* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 5028* [Flag](flag.html): Business identifier 5029* [Goal](goal.html): External Ids for this goal 5030* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 5031* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 5032* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 5033* [Immunization](immunization.html): Business identifier 5034* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 5035* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 5036* [Invoice](invoice.html): Business Identifier for item 5037* [List](list.html): Business identifier 5038* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 5039* [Medication](medication.html): Returns medications with this external identifier 5040* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 5041* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 5042* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 5043* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 5044* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 5045* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 5046* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 5047* [Observation](observation.html): The unique id for a particular observation 5048* [Person](person.html): A person Identifier 5049* [Procedure](procedure.html): A unique identifier for a procedure 5050* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 5051* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 5052* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 5053* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 5054* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 5055* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 5056* [Specimen](specimen.html): The unique identifier associated with the specimen 5057* [SupplyDelivery](supplydelivery.html): External identifier 5058* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 5059* [Task](task.html): Search for a task instance by its business identifier 5060* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 5061</b><br> 5062 * Type: <b>token</b><br> 5063 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 5064 * </p> 5065 */ 5066 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 5067 public static final String SP_IDENTIFIER = "identifier"; 5068 /** 5069 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5070 * <p> 5071 * Description: <b>Multiple Resources: 5072 5073* [Account](account.html): Account number 5074* [AdverseEvent](adverseevent.html): Business identifier for the event 5075* [AllergyIntolerance](allergyintolerance.html): External ids for this item 5076* [Appointment](appointment.html): An Identifier of the Appointment 5077* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 5078* [Basic](basic.html): Business identifier 5079* [BodyStructure](bodystructure.html): Bodystructure identifier 5080* [CarePlan](careplan.html): External Ids for this plan 5081* [CareTeam](careteam.html): External Ids for this team 5082* [ChargeItem](chargeitem.html): Business Identifier for item 5083* [Claim](claim.html): The primary identifier of the financial resource 5084* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 5085* [ClinicalImpression](clinicalimpression.html): Business identifier 5086* [Communication](communication.html): Unique identifier 5087* [CommunicationRequest](communicationrequest.html): Unique identifier 5088* [Composition](composition.html): Version-independent identifier for the Composition 5089* [Condition](condition.html): A unique identifier of the condition record 5090* [Consent](consent.html): Identifier for this record (external references) 5091* [Contract](contract.html): The identity of the contract 5092* [Coverage](coverage.html): The primary identifier of the insured and the coverage 5093* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 5094* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 5095* [DetectedIssue](detectedissue.html): Unique id for the detected issue 5096* [DeviceRequest](devicerequest.html): Business identifier for request/order 5097* [DeviceUsage](deviceusage.html): Search by identifier 5098* [DiagnosticReport](diagnosticreport.html): An identifier for the report 5099* [DocumentReference](documentreference.html): Identifier of the attachment binary 5100* [Encounter](encounter.html): Identifier(s) by which this encounter is known 5101* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 5102* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 5103* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 5104* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 5105* [Flag](flag.html): Business identifier 5106* [Goal](goal.html): External Ids for this goal 5107* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 5108* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 5109* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 5110* [Immunization](immunization.html): Business identifier 5111* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 5112* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 5113* [Invoice](invoice.html): Business Identifier for item 5114* [List](list.html): Business identifier 5115* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 5116* [Medication](medication.html): Returns medications with this external identifier 5117* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 5118* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 5119* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 5120* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 5121* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 5122* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 5123* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 5124* [Observation](observation.html): The unique id for a particular observation 5125* [Person](person.html): A person Identifier 5126* [Procedure](procedure.html): A unique identifier for a procedure 5127* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 5128* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 5129* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 5130* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 5131* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 5132* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 5133* [Specimen](specimen.html): The unique identifier associated with the specimen 5134* [SupplyDelivery](supplydelivery.html): External identifier 5135* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 5136* [Task](task.html): Search for a task instance by its business identifier 5137* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 5138</b><br> 5139 * Type: <b>token</b><br> 5140 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 5141 * </p> 5142 */ 5143 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5144 5145 /** 5146 * Search parameter: <b>patient</b> 5147 * <p> 5148 * Description: <b>Multiple Resources: 5149 5150* [Account](account.html): The entity that caused the expenses 5151* [AdverseEvent](adverseevent.html): Subject impacted by event 5152* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 5153* [Appointment](appointment.html): One of the individuals of the appointment is this patient 5154* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 5155* [AuditEvent](auditevent.html): Where the activity involved patient data 5156* [Basic](basic.html): Identifies the focus of this resource 5157* [BodyStructure](bodystructure.html): Who this is about 5158* [CarePlan](careplan.html): Who the care plan is for 5159* [CareTeam](careteam.html): Who care team is for 5160* [ChargeItem](chargeitem.html): Individual service was done for/to 5161* [Claim](claim.html): Patient receiving the products or services 5162* [ClaimResponse](claimresponse.html): The subject of care 5163* [ClinicalImpression](clinicalimpression.html): Patient assessed 5164* [Communication](communication.html): Focus of message 5165* [CommunicationRequest](communicationrequest.html): Focus of message 5166* [Composition](composition.html): Who and/or what the composition is about 5167* [Condition](condition.html): Who has the condition? 5168* [Consent](consent.html): Who the consent applies to 5169* [Contract](contract.html): The identity of the subject of the contract (if a patient) 5170* [Coverage](coverage.html): Retrieve coverages for a patient 5171* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 5172* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 5173* [DetectedIssue](detectedissue.html): Associated patient 5174* [DeviceRequest](devicerequest.html): Individual the service is ordered for 5175* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 5176* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 5177* [DocumentReference](documentreference.html): Who/what is the subject of the document 5178* [Encounter](encounter.html): The patient present at the encounter 5179* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 5180* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 5181* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 5182* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 5183* [Flag](flag.html): The identity of a subject to list flags for 5184* [Goal](goal.html): Who this goal is intended for 5185* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 5186* [ImagingSelection](imagingselection.html): Who the study is about 5187* [ImagingStudy](imagingstudy.html): Who the study is about 5188* [Immunization](immunization.html): The patient for the vaccination record 5189* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 5190* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 5191* [Invoice](invoice.html): Recipient(s) of goods and services 5192* [List](list.html): If all resources have the same subject 5193* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 5194* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 5195* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 5196* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 5197* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 5198* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 5199* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 5200* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 5201* [Observation](observation.html): The subject that the observation is about (if patient) 5202* [Person](person.html): The Person links to this Patient 5203* [Procedure](procedure.html): Search by subject - a patient 5204* [Provenance](provenance.html): Where the activity involved patient data 5205* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 5206* [RelatedPerson](relatedperson.html): The patient this related person is related to 5207* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 5208* [ResearchSubject](researchsubject.html): Who or what is part of study 5209* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 5210* [ServiceRequest](servicerequest.html): Search by subject - a patient 5211* [Specimen](specimen.html): The patient the specimen comes from 5212* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 5213* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 5214* [Task](task.html): Search by patient 5215* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 5216</b><br> 5217 * Type: <b>reference</b><br> 5218 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 5219 * </p> 5220 */ 5221 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 5222 public static final String SP_PATIENT = "patient"; 5223 /** 5224 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5225 * <p> 5226 * Description: <b>Multiple Resources: 5227 5228* [Account](account.html): The entity that caused the expenses 5229* [AdverseEvent](adverseevent.html): Subject impacted by event 5230* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 5231* [Appointment](appointment.html): One of the individuals of the appointment is this patient 5232* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 5233* [AuditEvent](auditevent.html): Where the activity involved patient data 5234* [Basic](basic.html): Identifies the focus of this resource 5235* [BodyStructure](bodystructure.html): Who this is about 5236* [CarePlan](careplan.html): Who the care plan is for 5237* [CareTeam](careteam.html): Who care team is for 5238* [ChargeItem](chargeitem.html): Individual service was done for/to 5239* [Claim](claim.html): Patient receiving the products or services 5240* [ClaimResponse](claimresponse.html): The subject of care 5241* [ClinicalImpression](clinicalimpression.html): Patient assessed 5242* [Communication](communication.html): Focus of message 5243* [CommunicationRequest](communicationrequest.html): Focus of message 5244* [Composition](composition.html): Who and/or what the composition is about 5245* [Condition](condition.html): Who has the condition? 5246* [Consent](consent.html): Who the consent applies to 5247* [Contract](contract.html): The identity of the subject of the contract (if a patient) 5248* [Coverage](coverage.html): Retrieve coverages for a patient 5249* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 5250* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 5251* [DetectedIssue](detectedissue.html): Associated patient 5252* [DeviceRequest](devicerequest.html): Individual the service is ordered for 5253* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 5254* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 5255* [DocumentReference](documentreference.html): Who/what is the subject of the document 5256* [Encounter](encounter.html): The patient present at the encounter 5257* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 5258* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 5259* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 5260* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 5261* [Flag](flag.html): The identity of a subject to list flags for 5262* [Goal](goal.html): Who this goal is intended for 5263* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 5264* [ImagingSelection](imagingselection.html): Who the study is about 5265* [ImagingStudy](imagingstudy.html): Who the study is about 5266* [Immunization](immunization.html): The patient for the vaccination record 5267* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 5268* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 5269* [Invoice](invoice.html): Recipient(s) of goods and services 5270* [List](list.html): If all resources have the same subject 5271* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 5272* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 5273* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 5274* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 5275* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 5276* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 5277* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 5278* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 5279* [Observation](observation.html): The subject that the observation is about (if patient) 5280* [Person](person.html): The Person links to this Patient 5281* [Procedure](procedure.html): Search by subject - a patient 5282* [Provenance](provenance.html): Where the activity involved patient data 5283* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 5284* [RelatedPerson](relatedperson.html): The patient this related person is related to 5285* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 5286* [ResearchSubject](researchsubject.html): Who or what is part of study 5287* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 5288* [ServiceRequest](servicerequest.html): Search by subject - a patient 5289* [Specimen](specimen.html): The patient the specimen comes from 5290* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 5291* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 5292* [Task](task.html): Search by patient 5293* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 5294</b><br> 5295 * Type: <b>reference</b><br> 5296 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 5297 * </p> 5298 */ 5299 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 5300 5301/** 5302 * Constant for fluent queries to be used to add include statements. Specifies 5303 * the path value of "<b>Observation:patient</b>". 5304 */ 5305 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Observation:patient").toLocked(); 5306 5307 5308} 5309