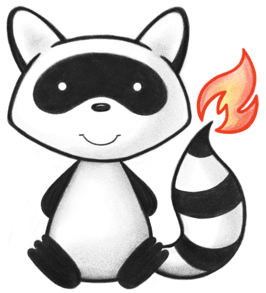
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Measurements and simple assertions made about a patient, device or other subject. 052 */ 053@ResourceDef(name="Observation", profile="http://hl7.org/fhir/StructureDefinition/Observation") 054public class Observation extends DomainResource { 055 056 public enum TriggeredBytype { 057 /** 058 * Performance of one or more other tests depending on the results of the initial test. This may include collection of additional specimen. While a new ServiceRequest is not required to perform the additional test, where it is still needed (e.g., requesting another laboratory to perform the reflex test), the Observation.basedOn would reference the new ServiceRequest that requested the additional test to be performed as well as the original ServiceRequest to reflect the one that provided the authorization. 059 */ 060 REFLEX, 061 /** 062 * Performance of the same test again with the same parameters/settings/solution. 063 */ 064 REPEAT, 065 /** 066 * Performance of the same test but with different parameters/settings/solution. 067 */ 068 RERUN, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static TriggeredBytype fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("reflex".equals(codeString)) 077 return REFLEX; 078 if ("repeat".equals(codeString)) 079 return REPEAT; 080 if ("re-run".equals(codeString)) 081 return RERUN; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown TriggeredBytype code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case REFLEX: return "reflex"; 090 case REPEAT: return "repeat"; 091 case RERUN: return "re-run"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case REFLEX: return "http://hl7.org/fhir/observation-triggeredbytype"; 099 case REPEAT: return "http://hl7.org/fhir/observation-triggeredbytype"; 100 case RERUN: return "http://hl7.org/fhir/observation-triggeredbytype"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case REFLEX: return "Performance of one or more other tests depending on the results of the initial test. This may include collection of additional specimen. While a new ServiceRequest is not required to perform the additional test, where it is still needed (e.g., requesting another laboratory to perform the reflex test), the Observation.basedOn would reference the new ServiceRequest that requested the additional test to be performed as well as the original ServiceRequest to reflect the one that provided the authorization."; 108 case REPEAT: return "Performance of the same test again with the same parameters/settings/solution."; 109 case RERUN: return "Performance of the same test but with different parameters/settings/solution."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case REFLEX: return "Reflex"; 117 case REPEAT: return "Repeat (per policy)"; 118 case RERUN: return "Re-run (per policy)"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class TriggeredBytypeEnumFactory implements EnumFactory<TriggeredBytype> { 126 public TriggeredBytype fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("reflex".equals(codeString)) 131 return TriggeredBytype.REFLEX; 132 if ("repeat".equals(codeString)) 133 return TriggeredBytype.REPEAT; 134 if ("re-run".equals(codeString)) 135 return TriggeredBytype.RERUN; 136 throw new IllegalArgumentException("Unknown TriggeredBytype code '"+codeString+"'"); 137 } 138 public Enumeration<TriggeredBytype> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.NULL, code); 146 if ("reflex".equals(codeString)) 147 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.REFLEX, code); 148 if ("repeat".equals(codeString)) 149 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.REPEAT, code); 150 if ("re-run".equals(codeString)) 151 return new Enumeration<TriggeredBytype>(this, TriggeredBytype.RERUN, code); 152 throw new FHIRException("Unknown TriggeredBytype code '"+codeString+"'"); 153 } 154 public String toCode(TriggeredBytype code) { 155 if (code == TriggeredBytype.REFLEX) 156 return "reflex"; 157 if (code == TriggeredBytype.REPEAT) 158 return "repeat"; 159 if (code == TriggeredBytype.RERUN) 160 return "re-run"; 161 return "?"; 162 } 163 public String toSystem(TriggeredBytype code) { 164 return code.getSystem(); 165 } 166 } 167 168 @Block() 169 public static class ObservationTriggeredByComponent extends BackboneElement implements IBaseBackboneElement { 170 /** 171 * Reference to the triggering observation. 172 */ 173 @Child(name = "observation", type = {Observation.class}, order=1, min=1, max=1, modifier=false, summary=true) 174 @Description(shortDefinition="Triggering observation", formalDefinition="Reference to the triggering observation." ) 175 protected Reference observation; 176 177 /** 178 * The type of trigger. 179Reflex | Repeat | Re-run. 180 */ 181 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 182 @Description(shortDefinition="reflex | repeat | re-run", formalDefinition="The type of trigger.\nReflex | Repeat | Re-run." ) 183 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-triggeredbytype") 184 protected Enumeration<TriggeredBytype> type; 185 186 /** 187 * Provides the reason why this observation was performed as a result of the observation(s) referenced. 188 */ 189 @Child(name = "reason", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Reason that the observation was triggered", formalDefinition="Provides the reason why this observation was performed as a result of the observation(s) referenced." ) 191 protected StringType reason; 192 193 private static final long serialVersionUID = -737822241L; 194 195 /** 196 * Constructor 197 */ 198 public ObservationTriggeredByComponent() { 199 super(); 200 } 201 202 /** 203 * Constructor 204 */ 205 public ObservationTriggeredByComponent(Reference observation, TriggeredBytype type) { 206 super(); 207 this.setObservation(observation); 208 this.setType(type); 209 } 210 211 /** 212 * @return {@link #observation} (Reference to the triggering observation.) 213 */ 214 public Reference getObservation() { 215 if (this.observation == null) 216 if (Configuration.errorOnAutoCreate()) 217 throw new Error("Attempt to auto-create ObservationTriggeredByComponent.observation"); 218 else if (Configuration.doAutoCreate()) 219 this.observation = new Reference(); // cc 220 return this.observation; 221 } 222 223 public boolean hasObservation() { 224 return this.observation != null && !this.observation.isEmpty(); 225 } 226 227 /** 228 * @param value {@link #observation} (Reference to the triggering observation.) 229 */ 230 public ObservationTriggeredByComponent setObservation(Reference value) { 231 this.observation = value; 232 return this; 233 } 234 235 /** 236 * @return {@link #type} (The type of trigger. 237Reflex | Repeat | Re-run.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 238 */ 239 public Enumeration<TriggeredBytype> getTypeElement() { 240 if (this.type == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create ObservationTriggeredByComponent.type"); 243 else if (Configuration.doAutoCreate()) 244 this.type = new Enumeration<TriggeredBytype>(new TriggeredBytypeEnumFactory()); // bb 245 return this.type; 246 } 247 248 public boolean hasTypeElement() { 249 return this.type != null && !this.type.isEmpty(); 250 } 251 252 public boolean hasType() { 253 return this.type != null && !this.type.isEmpty(); 254 } 255 256 /** 257 * @param value {@link #type} (The type of trigger. 258Reflex | Repeat | Re-run.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 259 */ 260 public ObservationTriggeredByComponent setTypeElement(Enumeration<TriggeredBytype> value) { 261 this.type = value; 262 return this; 263 } 264 265 /** 266 * @return The type of trigger. 267Reflex | Repeat | Re-run. 268 */ 269 public TriggeredBytype getType() { 270 return this.type == null ? null : this.type.getValue(); 271 } 272 273 /** 274 * @param value The type of trigger. 275Reflex | Repeat | Re-run. 276 */ 277 public ObservationTriggeredByComponent setType(TriggeredBytype value) { 278 if (this.type == null) 279 this.type = new Enumeration<TriggeredBytype>(new TriggeredBytypeEnumFactory()); 280 this.type.setValue(value); 281 return this; 282 } 283 284 /** 285 * @return {@link #reason} (Provides the reason why this observation was performed as a result of the observation(s) referenced.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 286 */ 287 public StringType getReasonElement() { 288 if (this.reason == null) 289 if (Configuration.errorOnAutoCreate()) 290 throw new Error("Attempt to auto-create ObservationTriggeredByComponent.reason"); 291 else if (Configuration.doAutoCreate()) 292 this.reason = new StringType(); // bb 293 return this.reason; 294 } 295 296 public boolean hasReasonElement() { 297 return this.reason != null && !this.reason.isEmpty(); 298 } 299 300 public boolean hasReason() { 301 return this.reason != null && !this.reason.isEmpty(); 302 } 303 304 /** 305 * @param value {@link #reason} (Provides the reason why this observation was performed as a result of the observation(s) referenced.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 306 */ 307 public ObservationTriggeredByComponent setReasonElement(StringType value) { 308 this.reason = value; 309 return this; 310 } 311 312 /** 313 * @return Provides the reason why this observation was performed as a result of the observation(s) referenced. 314 */ 315 public String getReason() { 316 return this.reason == null ? null : this.reason.getValue(); 317 } 318 319 /** 320 * @param value Provides the reason why this observation was performed as a result of the observation(s) referenced. 321 */ 322 public ObservationTriggeredByComponent setReason(String value) { 323 if (Utilities.noString(value)) 324 this.reason = null; 325 else { 326 if (this.reason == null) 327 this.reason = new StringType(); 328 this.reason.setValue(value); 329 } 330 return this; 331 } 332 333 protected void listChildren(List<Property> children) { 334 super.listChildren(children); 335 children.add(new Property("observation", "Reference(Observation)", "Reference to the triggering observation.", 0, 1, observation)); 336 children.add(new Property("type", "code", "The type of trigger.\nReflex | Repeat | Re-run.", 0, 1, type)); 337 children.add(new Property("reason", "string", "Provides the reason why this observation was performed as a result of the observation(s) referenced.", 0, 1, reason)); 338 } 339 340 @Override 341 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 342 switch (_hash) { 343 case 122345516: /*observation*/ return new Property("observation", "Reference(Observation)", "Reference to the triggering observation.", 0, 1, observation); 344 case 3575610: /*type*/ return new Property("type", "code", "The type of trigger.\nReflex | Repeat | Re-run.", 0, 1, type); 345 case -934964668: /*reason*/ return new Property("reason", "string", "Provides the reason why this observation was performed as a result of the observation(s) referenced.", 0, 1, reason); 346 default: return super.getNamedProperty(_hash, _name, _checkValid); 347 } 348 349 } 350 351 @Override 352 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 353 switch (hash) { 354 case 122345516: /*observation*/ return this.observation == null ? new Base[0] : new Base[] {this.observation}; // Reference 355 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TriggeredBytype> 356 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // StringType 357 default: return super.getProperty(hash, name, checkValid); 358 } 359 360 } 361 362 @Override 363 public Base setProperty(int hash, String name, Base value) throws FHIRException { 364 switch (hash) { 365 case 122345516: // observation 366 this.observation = TypeConvertor.castToReference(value); // Reference 367 return value; 368 case 3575610: // type 369 value = new TriggeredBytypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 370 this.type = (Enumeration) value; // Enumeration<TriggeredBytype> 371 return value; 372 case -934964668: // reason 373 this.reason = TypeConvertor.castToString(value); // StringType 374 return value; 375 default: return super.setProperty(hash, name, value); 376 } 377 378 } 379 380 @Override 381 public Base setProperty(String name, Base value) throws FHIRException { 382 if (name.equals("observation")) { 383 this.observation = TypeConvertor.castToReference(value); // Reference 384 } else if (name.equals("type")) { 385 value = new TriggeredBytypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 386 this.type = (Enumeration) value; // Enumeration<TriggeredBytype> 387 } else if (name.equals("reason")) { 388 this.reason = TypeConvertor.castToString(value); // StringType 389 } else 390 return super.setProperty(name, value); 391 return value; 392 } 393 394 @Override 395 public Base makeProperty(int hash, String name) throws FHIRException { 396 switch (hash) { 397 case 122345516: return getObservation(); 398 case 3575610: return getTypeElement(); 399 case -934964668: return getReasonElement(); 400 default: return super.makeProperty(hash, name); 401 } 402 403 } 404 405 @Override 406 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 407 switch (hash) { 408 case 122345516: /*observation*/ return new String[] {"Reference"}; 409 case 3575610: /*type*/ return new String[] {"code"}; 410 case -934964668: /*reason*/ return new String[] {"string"}; 411 default: return super.getTypesForProperty(hash, name); 412 } 413 414 } 415 416 @Override 417 public Base addChild(String name) throws FHIRException { 418 if (name.equals("observation")) { 419 this.observation = new Reference(); 420 return this.observation; 421 } 422 else if (name.equals("type")) { 423 throw new FHIRException("Cannot call addChild on a singleton property Observation.triggeredBy.type"); 424 } 425 else if (name.equals("reason")) { 426 throw new FHIRException("Cannot call addChild on a singleton property Observation.triggeredBy.reason"); 427 } 428 else 429 return super.addChild(name); 430 } 431 432 public ObservationTriggeredByComponent copy() { 433 ObservationTriggeredByComponent dst = new ObservationTriggeredByComponent(); 434 copyValues(dst); 435 return dst; 436 } 437 438 public void copyValues(ObservationTriggeredByComponent dst) { 439 super.copyValues(dst); 440 dst.observation = observation == null ? null : observation.copy(); 441 dst.type = type == null ? null : type.copy(); 442 dst.reason = reason == null ? null : reason.copy(); 443 } 444 445 @Override 446 public boolean equalsDeep(Base other_) { 447 if (!super.equalsDeep(other_)) 448 return false; 449 if (!(other_ instanceof ObservationTriggeredByComponent)) 450 return false; 451 ObservationTriggeredByComponent o = (ObservationTriggeredByComponent) other_; 452 return compareDeep(observation, o.observation, true) && compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true) 453 ; 454 } 455 456 @Override 457 public boolean equalsShallow(Base other_) { 458 if (!super.equalsShallow(other_)) 459 return false; 460 if (!(other_ instanceof ObservationTriggeredByComponent)) 461 return false; 462 ObservationTriggeredByComponent o = (ObservationTriggeredByComponent) other_; 463 return compareValues(type, o.type, true) && compareValues(reason, o.reason, true); 464 } 465 466 public boolean isEmpty() { 467 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(observation, type, reason 468 ); 469 } 470 471 public String fhirType() { 472 return "Observation.triggeredBy"; 473 474 } 475 476 } 477 478 @Block() 479 public static class ObservationReferenceRangeComponent extends BackboneElement implements IBaseBackboneElement { 480 /** 481 * The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3). 482 */ 483 @Child(name = "low", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 484 @Description(shortDefinition="Low Range, if relevant", formalDefinition="The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3)." ) 485 protected Quantity low; 486 487 /** 488 * The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3). 489 */ 490 @Child(name = "high", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 491 @Description(shortDefinition="High Range, if relevant", formalDefinition="The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3)." ) 492 protected Quantity high; 493 494 /** 495 * The value of the normal value of the reference range. 496 */ 497 @Child(name = "normalValue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 498 @Description(shortDefinition="Normal value, if relevant", formalDefinition="The value of the normal value of the reference range." ) 499 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-referencerange-normalvalue") 500 protected CodeableConcept normalValue; 501 502 /** 503 * Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range. 504 */ 505 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 506 @Description(shortDefinition="Reference range qualifier", formalDefinition="Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range." ) 507 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-meaning") 508 protected CodeableConcept type; 509 510 /** 511 * Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an "AND" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used. 512 */ 513 @Child(name = "appliesTo", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 514 @Description(shortDefinition="Reference range population", formalDefinition="Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used." ) 515 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-appliesto") 516 protected List<CodeableConcept> appliesTo; 517 518 /** 519 * The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so. 520 */ 521 @Child(name = "age", type = {Range.class}, order=6, min=0, max=1, modifier=false, summary=false) 522 @Description(shortDefinition="Applicable age range, if relevant", formalDefinition="The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so." ) 523 protected Range age; 524 525 /** 526 * Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals". 527 */ 528 @Child(name = "text", type = {MarkdownType.class}, order=7, min=0, max=1, modifier=false, summary=false) 529 @Description(shortDefinition="Text based reference range in an observation", formalDefinition="Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\"." ) 530 protected MarkdownType text; 531 532 private static final long serialVersionUID = 470232080L; 533 534 /** 535 * Constructor 536 */ 537 public ObservationReferenceRangeComponent() { 538 super(); 539 } 540 541 /** 542 * @return {@link #low} (The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).) 543 */ 544 public Quantity getLow() { 545 if (this.low == null) 546 if (Configuration.errorOnAutoCreate()) 547 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.low"); 548 else if (Configuration.doAutoCreate()) 549 this.low = new Quantity(); // cc 550 return this.low; 551 } 552 553 public boolean hasLow() { 554 return this.low != null && !this.low.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #low} (The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).) 559 */ 560 public ObservationReferenceRangeComponent setLow(Quantity value) { 561 this.low = value; 562 return this; 563 } 564 565 /** 566 * @return {@link #high} (The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).) 567 */ 568 public Quantity getHigh() { 569 if (this.high == null) 570 if (Configuration.errorOnAutoCreate()) 571 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.high"); 572 else if (Configuration.doAutoCreate()) 573 this.high = new Quantity(); // cc 574 return this.high; 575 } 576 577 public boolean hasHigh() { 578 return this.high != null && !this.high.isEmpty(); 579 } 580 581 /** 582 * @param value {@link #high} (The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).) 583 */ 584 public ObservationReferenceRangeComponent setHigh(Quantity value) { 585 this.high = value; 586 return this; 587 } 588 589 /** 590 * @return {@link #normalValue} (The value of the normal value of the reference range.) 591 */ 592 public CodeableConcept getNormalValue() { 593 if (this.normalValue == null) 594 if (Configuration.errorOnAutoCreate()) 595 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.normalValue"); 596 else if (Configuration.doAutoCreate()) 597 this.normalValue = new CodeableConcept(); // cc 598 return this.normalValue; 599 } 600 601 public boolean hasNormalValue() { 602 return this.normalValue != null && !this.normalValue.isEmpty(); 603 } 604 605 /** 606 * @param value {@link #normalValue} (The value of the normal value of the reference range.) 607 */ 608 public ObservationReferenceRangeComponent setNormalValue(CodeableConcept value) { 609 this.normalValue = value; 610 return this; 611 } 612 613 /** 614 * @return {@link #type} (Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.) 615 */ 616 public CodeableConcept getType() { 617 if (this.type == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.type"); 620 else if (Configuration.doAutoCreate()) 621 this.type = new CodeableConcept(); // cc 622 return this.type; 623 } 624 625 public boolean hasType() { 626 return this.type != null && !this.type.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #type} (Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.) 631 */ 632 public ObservationReferenceRangeComponent setType(CodeableConcept value) { 633 this.type = value; 634 return this; 635 } 636 637 /** 638 * @return {@link #appliesTo} (Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an "AND" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.) 639 */ 640 public List<CodeableConcept> getAppliesTo() { 641 if (this.appliesTo == null) 642 this.appliesTo = new ArrayList<CodeableConcept>(); 643 return this.appliesTo; 644 } 645 646 /** 647 * @return Returns a reference to <code>this</code> for easy method chaining 648 */ 649 public ObservationReferenceRangeComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 650 this.appliesTo = theAppliesTo; 651 return this; 652 } 653 654 public boolean hasAppliesTo() { 655 if (this.appliesTo == null) 656 return false; 657 for (CodeableConcept item : this.appliesTo) 658 if (!item.isEmpty()) 659 return true; 660 return false; 661 } 662 663 public CodeableConcept addAppliesTo() { //3 664 CodeableConcept t = new CodeableConcept(); 665 if (this.appliesTo == null) 666 this.appliesTo = new ArrayList<CodeableConcept>(); 667 this.appliesTo.add(t); 668 return t; 669 } 670 671 public ObservationReferenceRangeComponent addAppliesTo(CodeableConcept t) { //3 672 if (t == null) 673 return this; 674 if (this.appliesTo == null) 675 this.appliesTo = new ArrayList<CodeableConcept>(); 676 this.appliesTo.add(t); 677 return this; 678 } 679 680 /** 681 * @return The first repetition of repeating field {@link #appliesTo}, creating it if it does not already exist {3} 682 */ 683 public CodeableConcept getAppliesToFirstRep() { 684 if (getAppliesTo().isEmpty()) { 685 addAppliesTo(); 686 } 687 return getAppliesTo().get(0); 688 } 689 690 /** 691 * @return {@link #age} (The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.) 692 */ 693 public Range getAge() { 694 if (this.age == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.age"); 697 else if (Configuration.doAutoCreate()) 698 this.age = new Range(); // cc 699 return this.age; 700 } 701 702 public boolean hasAge() { 703 return this.age != null && !this.age.isEmpty(); 704 } 705 706 /** 707 * @param value {@link #age} (The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.) 708 */ 709 public ObservationReferenceRangeComponent setAge(Range value) { 710 this.age = value; 711 return this; 712 } 713 714 /** 715 * @return {@link #text} (Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals".). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 716 */ 717 public MarkdownType getTextElement() { 718 if (this.text == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.text"); 721 else if (Configuration.doAutoCreate()) 722 this.text = new MarkdownType(); // bb 723 return this.text; 724 } 725 726 public boolean hasTextElement() { 727 return this.text != null && !this.text.isEmpty(); 728 } 729 730 public boolean hasText() { 731 return this.text != null && !this.text.isEmpty(); 732 } 733 734 /** 735 * @param value {@link #text} (Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals".). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 736 */ 737 public ObservationReferenceRangeComponent setTextElement(MarkdownType value) { 738 this.text = value; 739 return this; 740 } 741 742 /** 743 * @return Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals". 744 */ 745 public String getText() { 746 return this.text == null ? null : this.text.getValue(); 747 } 748 749 /** 750 * @param value Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of "normals". 751 */ 752 public ObservationReferenceRangeComponent setText(String value) { 753 if (Utilities.noString(value)) 754 this.text = null; 755 else { 756 if (this.text == null) 757 this.text = new MarkdownType(); 758 this.text.setValue(value); 759 } 760 return this; 761 } 762 763 protected void listChildren(List<Property> children) { 764 super.listChildren(children); 765 children.add(new Property("low", "Quantity", "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 0, 1, low)); 766 children.add(new Property("high", "Quantity", "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 0, 1, high)); 767 children.add(new Property("normalValue", "CodeableConcept", "The value of the normal value of the reference range.", 0, 1, normalValue)); 768 children.add(new Property("type", "CodeableConcept", "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 0, 1, type)); 769 children.add(new Property("appliesTo", "CodeableConcept", "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 0, java.lang.Integer.MAX_VALUE, appliesTo)); 770 children.add(new Property("age", "Range", "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 0, 1, age)); 771 children.add(new Property("text", "markdown", "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 0, 1, text)); 772 } 773 774 @Override 775 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 776 switch (_hash) { 777 case 107348: /*low*/ return new Property("low", "Quantity", "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 0, 1, low); 778 case 3202466: /*high*/ return new Property("high", "Quantity", "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 0, 1, high); 779 case -270017334: /*normalValue*/ return new Property("normalValue", "CodeableConcept", "The value of the normal value of the reference range.", 0, 1, normalValue); 780 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 0, 1, type); 781 case -2089924569: /*appliesTo*/ return new Property("appliesTo", "CodeableConcept", "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 0, java.lang.Integer.MAX_VALUE, appliesTo); 782 case 96511: /*age*/ return new Property("age", "Range", "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 0, 1, age); 783 case 3556653: /*text*/ return new Property("text", "markdown", "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 0, 1, text); 784 default: return super.getNamedProperty(_hash, _name, _checkValid); 785 } 786 787 } 788 789 @Override 790 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 791 switch (hash) { 792 case 107348: /*low*/ return this.low == null ? new Base[0] : new Base[] {this.low}; // Quantity 793 case 3202466: /*high*/ return this.high == null ? new Base[0] : new Base[] {this.high}; // Quantity 794 case -270017334: /*normalValue*/ return this.normalValue == null ? new Base[0] : new Base[] {this.normalValue}; // CodeableConcept 795 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 796 case -2089924569: /*appliesTo*/ return this.appliesTo == null ? new Base[0] : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 797 case 96511: /*age*/ return this.age == null ? new Base[0] : new Base[] {this.age}; // Range 798 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // MarkdownType 799 default: return super.getProperty(hash, name, checkValid); 800 } 801 802 } 803 804 @Override 805 public Base setProperty(int hash, String name, Base value) throws FHIRException { 806 switch (hash) { 807 case 107348: // low 808 this.low = TypeConvertor.castToQuantity(value); // Quantity 809 return value; 810 case 3202466: // high 811 this.high = TypeConvertor.castToQuantity(value); // Quantity 812 return value; 813 case -270017334: // normalValue 814 this.normalValue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 815 return value; 816 case 3575610: // type 817 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 818 return value; 819 case -2089924569: // appliesTo 820 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 821 return value; 822 case 96511: // age 823 this.age = TypeConvertor.castToRange(value); // Range 824 return value; 825 case 3556653: // text 826 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 827 return value; 828 default: return super.setProperty(hash, name, value); 829 } 830 831 } 832 833 @Override 834 public Base setProperty(String name, Base value) throws FHIRException { 835 if (name.equals("low")) { 836 this.low = TypeConvertor.castToQuantity(value); // Quantity 837 } else if (name.equals("high")) { 838 this.high = TypeConvertor.castToQuantity(value); // Quantity 839 } else if (name.equals("normalValue")) { 840 this.normalValue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 841 } else if (name.equals("type")) { 842 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 843 } else if (name.equals("appliesTo")) { 844 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); 845 } else if (name.equals("age")) { 846 this.age = TypeConvertor.castToRange(value); // Range 847 } else if (name.equals("text")) { 848 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 849 } else 850 return super.setProperty(name, value); 851 return value; 852 } 853 854 @Override 855 public Base makeProperty(int hash, String name) throws FHIRException { 856 switch (hash) { 857 case 107348: return getLow(); 858 case 3202466: return getHigh(); 859 case -270017334: return getNormalValue(); 860 case 3575610: return getType(); 861 case -2089924569: return addAppliesTo(); 862 case 96511: return getAge(); 863 case 3556653: return getTextElement(); 864 default: return super.makeProperty(hash, name); 865 } 866 867 } 868 869 @Override 870 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 871 switch (hash) { 872 case 107348: /*low*/ return new String[] {"Quantity"}; 873 case 3202466: /*high*/ return new String[] {"Quantity"}; 874 case -270017334: /*normalValue*/ return new String[] {"CodeableConcept"}; 875 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 876 case -2089924569: /*appliesTo*/ return new String[] {"CodeableConcept"}; 877 case 96511: /*age*/ return new String[] {"Range"}; 878 case 3556653: /*text*/ return new String[] {"markdown"}; 879 default: return super.getTypesForProperty(hash, name); 880 } 881 882 } 883 884 @Override 885 public Base addChild(String name) throws FHIRException { 886 if (name.equals("low")) { 887 this.low = new Quantity(); 888 return this.low; 889 } 890 else if (name.equals("high")) { 891 this.high = new Quantity(); 892 return this.high; 893 } 894 else if (name.equals("normalValue")) { 895 this.normalValue = new CodeableConcept(); 896 return this.normalValue; 897 } 898 else if (name.equals("type")) { 899 this.type = new CodeableConcept(); 900 return this.type; 901 } 902 else if (name.equals("appliesTo")) { 903 return addAppliesTo(); 904 } 905 else if (name.equals("age")) { 906 this.age = new Range(); 907 return this.age; 908 } 909 else if (name.equals("text")) { 910 throw new FHIRException("Cannot call addChild on a singleton property Observation.referenceRange.text"); 911 } 912 else 913 return super.addChild(name); 914 } 915 916 public ObservationReferenceRangeComponent copy() { 917 ObservationReferenceRangeComponent dst = new ObservationReferenceRangeComponent(); 918 copyValues(dst); 919 return dst; 920 } 921 922 public void copyValues(ObservationReferenceRangeComponent dst) { 923 super.copyValues(dst); 924 dst.low = low == null ? null : low.copy(); 925 dst.high = high == null ? null : high.copy(); 926 dst.normalValue = normalValue == null ? null : normalValue.copy(); 927 dst.type = type == null ? null : type.copy(); 928 if (appliesTo != null) { 929 dst.appliesTo = new ArrayList<CodeableConcept>(); 930 for (CodeableConcept i : appliesTo) 931 dst.appliesTo.add(i.copy()); 932 }; 933 dst.age = age == null ? null : age.copy(); 934 dst.text = text == null ? null : text.copy(); 935 } 936 937 @Override 938 public boolean equalsDeep(Base other_) { 939 if (!super.equalsDeep(other_)) 940 return false; 941 if (!(other_ instanceof ObservationReferenceRangeComponent)) 942 return false; 943 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 944 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true) && compareDeep(normalValue, o.normalValue, true) 945 && compareDeep(type, o.type, true) && compareDeep(appliesTo, o.appliesTo, true) && compareDeep(age, o.age, true) 946 && compareDeep(text, o.text, true); 947 } 948 949 @Override 950 public boolean equalsShallow(Base other_) { 951 if (!super.equalsShallow(other_)) 952 return false; 953 if (!(other_ instanceof ObservationReferenceRangeComponent)) 954 return false; 955 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 956 return compareValues(text, o.text, true); 957 } 958 959 public boolean isEmpty() { 960 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(low, high, normalValue, type 961 , appliesTo, age, text); 962 } 963 964 public String fhirType() { 965 return "Observation.referenceRange"; 966 967 } 968 969 } 970 971 @Block() 972 public static class ObservationComponentComponent extends BackboneElement implements IBaseBackboneElement { 973 /** 974 * Describes what was observed. Sometimes this is called the observation "code". 975 */ 976 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 977 @Description(shortDefinition="Type of component observation (code / type)", formalDefinition="Describes what was observed. Sometimes this is called the observation \"code\"." ) 978 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 979 protected CodeableConcept code; 980 981 /** 982 * The information determined as a result of making the observation, if the information has a simple value. 983 */ 984 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, Period.class, Attachment.class, MolecularSequence.class}, order=2, min=0, max=1, modifier=false, summary=true) 985 @Description(shortDefinition="Actual component result", formalDefinition="The information determined as a result of making the observation, if the information has a simple value." ) 986 protected DataType value; 987 988 /** 989 * Provides a reason why the expected value in the element Observation.component.value[x] is missing. 990 */ 991 @Child(name = "dataAbsentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 992 @Description(shortDefinition="Why the component result is missing", formalDefinition="Provides a reason why the expected value in the element Observation.component.value[x] is missing." ) 993 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/data-absent-reason") 994 protected CodeableConcept dataAbsentReason; 995 996 /** 997 * A categorical assessment of an observation value. For example, high, low, normal. 998 */ 999 @Child(name = "interpretation", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1000 @Description(shortDefinition="High, low, normal, etc", formalDefinition="A categorical assessment of an observation value. For example, high, low, normal." ) 1001 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-interpretation") 1002 protected List<CodeableConcept> interpretation; 1003 1004 /** 1005 * Guidance on how to interpret the value by comparison to a normal or recommended range. 1006 */ 1007 @Child(name = "referenceRange", type = {ObservationReferenceRangeComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1008 @Description(shortDefinition="Provides guide for interpretation of component result", formalDefinition="Guidance on how to interpret the value by comparison to a normal or recommended range." ) 1009 protected List<ObservationReferenceRangeComponent> referenceRange; 1010 1011 private static final long serialVersionUID = -1771757751L; 1012 1013 /** 1014 * Constructor 1015 */ 1016 public ObservationComponentComponent() { 1017 super(); 1018 } 1019 1020 /** 1021 * Constructor 1022 */ 1023 public ObservationComponentComponent(CodeableConcept code) { 1024 super(); 1025 this.setCode(code); 1026 } 1027 1028 /** 1029 * @return {@link #code} (Describes what was observed. Sometimes this is called the observation "code".) 1030 */ 1031 public CodeableConcept getCode() { 1032 if (this.code == null) 1033 if (Configuration.errorOnAutoCreate()) 1034 throw new Error("Attempt to auto-create ObservationComponentComponent.code"); 1035 else if (Configuration.doAutoCreate()) 1036 this.code = new CodeableConcept(); // cc 1037 return this.code; 1038 } 1039 1040 public boolean hasCode() { 1041 return this.code != null && !this.code.isEmpty(); 1042 } 1043 1044 /** 1045 * @param value {@link #code} (Describes what was observed. Sometimes this is called the observation "code".) 1046 */ 1047 public ObservationComponentComponent setCode(CodeableConcept value) { 1048 this.code = value; 1049 return this; 1050 } 1051 1052 /** 1053 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1054 */ 1055 public DataType getValue() { 1056 return this.value; 1057 } 1058 1059 /** 1060 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1061 */ 1062 public Quantity getValueQuantity() throws FHIRException { 1063 if (this.value == null) 1064 this.value = new Quantity(); 1065 if (!(this.value instanceof Quantity)) 1066 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1067 return (Quantity) this.value; 1068 } 1069 1070 public boolean hasValueQuantity() { 1071 return this != null && this.value instanceof Quantity; 1072 } 1073 1074 /** 1075 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1076 */ 1077 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1078 if (this.value == null) 1079 this.value = new CodeableConcept(); 1080 if (!(this.value instanceof CodeableConcept)) 1081 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1082 return (CodeableConcept) this.value; 1083 } 1084 1085 public boolean hasValueCodeableConcept() { 1086 return this != null && this.value instanceof CodeableConcept; 1087 } 1088 1089 /** 1090 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1091 */ 1092 public StringType getValueStringType() throws FHIRException { 1093 if (this.value == null) 1094 this.value = new StringType(); 1095 if (!(this.value instanceof StringType)) 1096 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1097 return (StringType) this.value; 1098 } 1099 1100 public boolean hasValueStringType() { 1101 return this != null && this.value instanceof StringType; 1102 } 1103 1104 /** 1105 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1106 */ 1107 public BooleanType getValueBooleanType() throws FHIRException { 1108 if (this.value == null) 1109 this.value = new BooleanType(); 1110 if (!(this.value instanceof BooleanType)) 1111 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1112 return (BooleanType) this.value; 1113 } 1114 1115 public boolean hasValueBooleanType() { 1116 return this != null && this.value instanceof BooleanType; 1117 } 1118 1119 /** 1120 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1121 */ 1122 public IntegerType getValueIntegerType() throws FHIRException { 1123 if (this.value == null) 1124 this.value = new IntegerType(); 1125 if (!(this.value instanceof IntegerType)) 1126 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 1127 return (IntegerType) this.value; 1128 } 1129 1130 public boolean hasValueIntegerType() { 1131 return this != null && this.value instanceof IntegerType; 1132 } 1133 1134 /** 1135 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1136 */ 1137 public Range getValueRange() throws FHIRException { 1138 if (this.value == null) 1139 this.value = new Range(); 1140 if (!(this.value instanceof Range)) 1141 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1142 return (Range) this.value; 1143 } 1144 1145 public boolean hasValueRange() { 1146 return this != null && this.value instanceof Range; 1147 } 1148 1149 /** 1150 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1151 */ 1152 public Ratio getValueRatio() throws FHIRException { 1153 if (this.value == null) 1154 this.value = new Ratio(); 1155 if (!(this.value instanceof Ratio)) 1156 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 1157 return (Ratio) this.value; 1158 } 1159 1160 public boolean hasValueRatio() { 1161 return this != null && this.value instanceof Ratio; 1162 } 1163 1164 /** 1165 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1166 */ 1167 public SampledData getValueSampledData() throws FHIRException { 1168 if (this.value == null) 1169 this.value = new SampledData(); 1170 if (!(this.value instanceof SampledData)) 1171 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 1172 return (SampledData) this.value; 1173 } 1174 1175 public boolean hasValueSampledData() { 1176 return this != null && this.value instanceof SampledData; 1177 } 1178 1179 /** 1180 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1181 */ 1182 public TimeType getValueTimeType() throws FHIRException { 1183 if (this.value == null) 1184 this.value = new TimeType(); 1185 if (!(this.value instanceof TimeType)) 1186 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1187 return (TimeType) this.value; 1188 } 1189 1190 public boolean hasValueTimeType() { 1191 return this != null && this.value instanceof TimeType; 1192 } 1193 1194 /** 1195 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1196 */ 1197 public DateTimeType getValueDateTimeType() throws FHIRException { 1198 if (this.value == null) 1199 this.value = new DateTimeType(); 1200 if (!(this.value instanceof DateTimeType)) 1201 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1202 return (DateTimeType) this.value; 1203 } 1204 1205 public boolean hasValueDateTimeType() { 1206 return this != null && this.value instanceof DateTimeType; 1207 } 1208 1209 /** 1210 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1211 */ 1212 public Period getValuePeriod() throws FHIRException { 1213 if (this.value == null) 1214 this.value = new Period(); 1215 if (!(this.value instanceof Period)) 1216 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 1217 return (Period) this.value; 1218 } 1219 1220 public boolean hasValuePeriod() { 1221 return this != null && this.value instanceof Period; 1222 } 1223 1224 /** 1225 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1226 */ 1227 public Attachment getValueAttachment() throws FHIRException { 1228 if (this.value == null) 1229 this.value = new Attachment(); 1230 if (!(this.value instanceof Attachment)) 1231 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1232 return (Attachment) this.value; 1233 } 1234 1235 public boolean hasValueAttachment() { 1236 return this != null && this.value instanceof Attachment; 1237 } 1238 1239 /** 1240 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1241 */ 1242 public Reference getValueReference() throws FHIRException { 1243 if (this.value == null) 1244 this.value = new Reference(); 1245 if (!(this.value instanceof Reference)) 1246 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1247 return (Reference) this.value; 1248 } 1249 1250 public boolean hasValueReference() { 1251 return this != null && this.value instanceof Reference; 1252 } 1253 1254 public boolean hasValue() { 1255 return this.value != null && !this.value.isEmpty(); 1256 } 1257 1258 /** 1259 * @param value {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1260 */ 1261 public ObservationComponentComponent setValue(DataType value) { 1262 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Ratio || value instanceof SampledData || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period || value instanceof Attachment || value instanceof Reference)) 1263 throw new FHIRException("Not the right type for Observation.component.value[x]: "+value.fhirType()); 1264 this.value = value; 1265 return this; 1266 } 1267 1268 /** 1269 * @return {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.component.value[x] is missing.) 1270 */ 1271 public CodeableConcept getDataAbsentReason() { 1272 if (this.dataAbsentReason == null) 1273 if (Configuration.errorOnAutoCreate()) 1274 throw new Error("Attempt to auto-create ObservationComponentComponent.dataAbsentReason"); 1275 else if (Configuration.doAutoCreate()) 1276 this.dataAbsentReason = new CodeableConcept(); // cc 1277 return this.dataAbsentReason; 1278 } 1279 1280 public boolean hasDataAbsentReason() { 1281 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.component.value[x] is missing.) 1286 */ 1287 public ObservationComponentComponent setDataAbsentReason(CodeableConcept value) { 1288 this.dataAbsentReason = value; 1289 return this; 1290 } 1291 1292 /** 1293 * @return {@link #interpretation} (A categorical assessment of an observation value. For example, high, low, normal.) 1294 */ 1295 public List<CodeableConcept> getInterpretation() { 1296 if (this.interpretation == null) 1297 this.interpretation = new ArrayList<CodeableConcept>(); 1298 return this.interpretation; 1299 } 1300 1301 /** 1302 * @return Returns a reference to <code>this</code> for easy method chaining 1303 */ 1304 public ObservationComponentComponent setInterpretation(List<CodeableConcept> theInterpretation) { 1305 this.interpretation = theInterpretation; 1306 return this; 1307 } 1308 1309 public boolean hasInterpretation() { 1310 if (this.interpretation == null) 1311 return false; 1312 for (CodeableConcept item : this.interpretation) 1313 if (!item.isEmpty()) 1314 return true; 1315 return false; 1316 } 1317 1318 public CodeableConcept addInterpretation() { //3 1319 CodeableConcept t = new CodeableConcept(); 1320 if (this.interpretation == null) 1321 this.interpretation = new ArrayList<CodeableConcept>(); 1322 this.interpretation.add(t); 1323 return t; 1324 } 1325 1326 public ObservationComponentComponent addInterpretation(CodeableConcept t) { //3 1327 if (t == null) 1328 return this; 1329 if (this.interpretation == null) 1330 this.interpretation = new ArrayList<CodeableConcept>(); 1331 this.interpretation.add(t); 1332 return this; 1333 } 1334 1335 /** 1336 * @return The first repetition of repeating field {@link #interpretation}, creating it if it does not already exist {3} 1337 */ 1338 public CodeableConcept getInterpretationFirstRep() { 1339 if (getInterpretation().isEmpty()) { 1340 addInterpretation(); 1341 } 1342 return getInterpretation().get(0); 1343 } 1344 1345 /** 1346 * @return {@link #referenceRange} (Guidance on how to interpret the value by comparison to a normal or recommended range.) 1347 */ 1348 public List<ObservationReferenceRangeComponent> getReferenceRange() { 1349 if (this.referenceRange == null) 1350 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1351 return this.referenceRange; 1352 } 1353 1354 /** 1355 * @return Returns a reference to <code>this</code> for easy method chaining 1356 */ 1357 public ObservationComponentComponent setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 1358 this.referenceRange = theReferenceRange; 1359 return this; 1360 } 1361 1362 public boolean hasReferenceRange() { 1363 if (this.referenceRange == null) 1364 return false; 1365 for (ObservationReferenceRangeComponent item : this.referenceRange) 1366 if (!item.isEmpty()) 1367 return true; 1368 return false; 1369 } 1370 1371 public ObservationReferenceRangeComponent addReferenceRange() { //3 1372 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 1373 if (this.referenceRange == null) 1374 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1375 this.referenceRange.add(t); 1376 return t; 1377 } 1378 1379 public ObservationComponentComponent addReferenceRange(ObservationReferenceRangeComponent t) { //3 1380 if (t == null) 1381 return this; 1382 if (this.referenceRange == null) 1383 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1384 this.referenceRange.add(t); 1385 return this; 1386 } 1387 1388 /** 1389 * @return The first repetition of repeating field {@link #referenceRange}, creating it if it does not already exist {3} 1390 */ 1391 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 1392 if (getReferenceRange().isEmpty()) { 1393 addReferenceRange(); 1394 } 1395 return getReferenceRange().get(0); 1396 } 1397 1398 protected void listChildren(List<Property> children) { 1399 super.listChildren(children); 1400 children.add(new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code)); 1401 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value)); 1402 children.add(new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, dataAbsentReason)); 1403 children.add(new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation)); 1404 children.add(new Property("referenceRange", "@Observation.referenceRange", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange)); 1405 } 1406 1407 @Override 1408 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1409 switch (_hash) { 1410 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code); 1411 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1412 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1413 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1414 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1415 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1416 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1417 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1418 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1419 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1420 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1421 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1422 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1423 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1424 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1425 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1426 case 1034315687: /*dataAbsentReason*/ return new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, dataAbsentReason); 1427 case -297950712: /*interpretation*/ return new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation); 1428 case -1912545102: /*referenceRange*/ return new Property("referenceRange", "@Observation.referenceRange", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange); 1429 default: return super.getNamedProperty(_hash, _name, _checkValid); 1430 } 1431 1432 } 1433 1434 @Override 1435 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1436 switch (hash) { 1437 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1438 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1439 case 1034315687: /*dataAbsentReason*/ return this.dataAbsentReason == null ? new Base[0] : new Base[] {this.dataAbsentReason}; // CodeableConcept 1440 case -297950712: /*interpretation*/ return this.interpretation == null ? new Base[0] : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 1441 case -1912545102: /*referenceRange*/ return this.referenceRange == null ? new Base[0] : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 1442 default: return super.getProperty(hash, name, checkValid); 1443 } 1444 1445 } 1446 1447 @Override 1448 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1449 switch (hash) { 1450 case 3059181: // code 1451 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1452 return value; 1453 case 111972721: // value 1454 this.value = TypeConvertor.castToType(value); // DataType 1455 return value; 1456 case 1034315687: // dataAbsentReason 1457 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1458 return value; 1459 case -297950712: // interpretation 1460 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1461 return value; 1462 case -1912545102: // referenceRange 1463 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 1464 return value; 1465 default: return super.setProperty(hash, name, value); 1466 } 1467 1468 } 1469 1470 @Override 1471 public Base setProperty(String name, Base value) throws FHIRException { 1472 if (name.equals("code")) { 1473 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1474 } else if (name.equals("value[x]")) { 1475 this.value = TypeConvertor.castToType(value); // DataType 1476 } else if (name.equals("dataAbsentReason")) { 1477 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1478 } else if (name.equals("interpretation")) { 1479 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); 1480 } else if (name.equals("referenceRange")) { 1481 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 1482 } else 1483 return super.setProperty(name, value); 1484 return value; 1485 } 1486 1487 @Override 1488 public Base makeProperty(int hash, String name) throws FHIRException { 1489 switch (hash) { 1490 case 3059181: return getCode(); 1491 case -1410166417: return getValue(); 1492 case 111972721: return getValue(); 1493 case 1034315687: return getDataAbsentReason(); 1494 case -297950712: return addInterpretation(); 1495 case -1912545102: return addReferenceRange(); 1496 default: return super.makeProperty(hash, name); 1497 } 1498 1499 } 1500 1501 @Override 1502 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1503 switch (hash) { 1504 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1505 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Ratio", "SampledData", "time", "dateTime", "Period", "Attachment", "Reference"}; 1506 case 1034315687: /*dataAbsentReason*/ return new String[] {"CodeableConcept"}; 1507 case -297950712: /*interpretation*/ return new String[] {"CodeableConcept"}; 1508 case -1912545102: /*referenceRange*/ return new String[] {"@Observation.referenceRange"}; 1509 default: return super.getTypesForProperty(hash, name); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base addChild(String name) throws FHIRException { 1516 if (name.equals("code")) { 1517 this.code = new CodeableConcept(); 1518 return this.code; 1519 } 1520 else if (name.equals("valueQuantity")) { 1521 this.value = new Quantity(); 1522 return this.value; 1523 } 1524 else if (name.equals("valueCodeableConcept")) { 1525 this.value = new CodeableConcept(); 1526 return this.value; 1527 } 1528 else if (name.equals("valueString")) { 1529 this.value = new StringType(); 1530 return this.value; 1531 } 1532 else if (name.equals("valueBoolean")) { 1533 this.value = new BooleanType(); 1534 return this.value; 1535 } 1536 else if (name.equals("valueInteger")) { 1537 this.value = new IntegerType(); 1538 return this.value; 1539 } 1540 else if (name.equals("valueRange")) { 1541 this.value = new Range(); 1542 return this.value; 1543 } 1544 else if (name.equals("valueRatio")) { 1545 this.value = new Ratio(); 1546 return this.value; 1547 } 1548 else if (name.equals("valueSampledData")) { 1549 this.value = new SampledData(); 1550 return this.value; 1551 } 1552 else if (name.equals("valueTime")) { 1553 this.value = new TimeType(); 1554 return this.value; 1555 } 1556 else if (name.equals("valueDateTime")) { 1557 this.value = new DateTimeType(); 1558 return this.value; 1559 } 1560 else if (name.equals("valuePeriod")) { 1561 this.value = new Period(); 1562 return this.value; 1563 } 1564 else if (name.equals("valueAttachment")) { 1565 this.value = new Attachment(); 1566 return this.value; 1567 } 1568 else if (name.equals("valueReference")) { 1569 this.value = new Reference(); 1570 return this.value; 1571 } 1572 else if (name.equals("dataAbsentReason")) { 1573 this.dataAbsentReason = new CodeableConcept(); 1574 return this.dataAbsentReason; 1575 } 1576 else if (name.equals("interpretation")) { 1577 return addInterpretation(); 1578 } 1579 else if (name.equals("referenceRange")) { 1580 return addReferenceRange(); 1581 } 1582 else 1583 return super.addChild(name); 1584 } 1585 1586 public ObservationComponentComponent copy() { 1587 ObservationComponentComponent dst = new ObservationComponentComponent(); 1588 copyValues(dst); 1589 return dst; 1590 } 1591 1592 public void copyValues(ObservationComponentComponent dst) { 1593 super.copyValues(dst); 1594 dst.code = code == null ? null : code.copy(); 1595 dst.value = value == null ? null : value.copy(); 1596 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 1597 if (interpretation != null) { 1598 dst.interpretation = new ArrayList<CodeableConcept>(); 1599 for (CodeableConcept i : interpretation) 1600 dst.interpretation.add(i.copy()); 1601 }; 1602 if (referenceRange != null) { 1603 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1604 for (ObservationReferenceRangeComponent i : referenceRange) 1605 dst.referenceRange.add(i.copy()); 1606 }; 1607 } 1608 1609 @Override 1610 public boolean equalsDeep(Base other_) { 1611 if (!super.equalsDeep(other_)) 1612 return false; 1613 if (!(other_ instanceof ObservationComponentComponent)) 1614 return false; 1615 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1616 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 1617 && compareDeep(interpretation, o.interpretation, true) && compareDeep(referenceRange, o.referenceRange, true) 1618 ; 1619 } 1620 1621 @Override 1622 public boolean equalsShallow(Base other_) { 1623 if (!super.equalsShallow(other_)) 1624 return false; 1625 if (!(other_ instanceof ObservationComponentComponent)) 1626 return false; 1627 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1628 return true; 1629 } 1630 1631 public boolean isEmpty() { 1632 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, dataAbsentReason 1633 , interpretation, referenceRange); 1634 } 1635 1636 public String fhirType() { 1637 return "Observation.component"; 1638 1639 } 1640 1641 } 1642 1643 /** 1644 * A unique identifier assigned to this observation. 1645 */ 1646 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1647 @Description(shortDefinition="Business Identifier for observation", formalDefinition="A unique identifier assigned to this observation." ) 1648 protected List<Identifier> identifier; 1649 1650 /** 1651 * The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance. 1652 */ 1653 @Child(name = "instantiates", type = {CanonicalType.class, ObservationDefinition.class}, order=1, min=0, max=1, modifier=false, summary=true) 1654 @Description(shortDefinition="Instantiates FHIR ObservationDefinition", formalDefinition="The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance." ) 1655 protected DataType instantiates; 1656 1657 /** 1658 * A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed. 1659 */ 1660 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1661 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed." ) 1662 protected List<Reference> basedOn; 1663 1664 /** 1665 * Identifies the observation(s) that triggered the performance of this observation. 1666 */ 1667 @Child(name = "triggeredBy", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1668 @Description(shortDefinition="Triggering observation(s)", formalDefinition="Identifies the observation(s) that triggered the performance of this observation." ) 1669 protected List<ObservationTriggeredByComponent> triggeredBy; 1670 1671 /** 1672 * A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure. 1673 */ 1674 @Child(name = "partOf", type = {MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Procedure.class, Immunization.class, ImagingStudy.class, GenomicStudy.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1675 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure." ) 1676 protected List<Reference> partOf; 1677 1678 /** 1679 * The status of the result value. 1680 */ 1681 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 1682 @Description(shortDefinition="registered | preliminary | final | amended +", formalDefinition="The status of the result value." ) 1683 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-status") 1684 protected Enumeration<ObservationStatus> status; 1685 1686 /** 1687 * A code that classifies the general type of observation being made. 1688 */ 1689 @Child(name = "category", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1690 @Description(shortDefinition="Classification of type of observation", formalDefinition="A code that classifies the general type of observation being made." ) 1691 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-category") 1692 protected List<CodeableConcept> category; 1693 1694 /** 1695 * Describes what was observed. Sometimes this is called the observation "name". 1696 */ 1697 @Child(name = "code", type = {CodeableConcept.class}, order=7, min=1, max=1, modifier=false, summary=true) 1698 @Description(shortDefinition="Type of observation (code / type)", formalDefinition="Describes what was observed. Sometimes this is called the observation \"name\"." ) 1699 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1700 protected CodeableConcept code; 1701 1702 /** 1703 * The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation. 1704 */ 1705 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class, Organization.class, Procedure.class, Practitioner.class, Medication.class, Substance.class, BiologicallyDerivedProduct.class, NutritionProduct.class}, order=8, min=0, max=1, modifier=false, summary=true) 1706 @Description(shortDefinition="Who and/or what the observation is about", formalDefinition="The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation." ) 1707 protected Reference subject; 1708 1709 /** 1710 * The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus. 1711 */ 1712 @Child(name = "focus", type = {Reference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1713 @Description(shortDefinition="What the observation is about, when it is not about the subject of record", formalDefinition="The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus." ) 1714 protected List<Reference> focus; 1715 1716 /** 1717 * The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made. 1718 */ 1719 @Child(name = "encounter", type = {Encounter.class}, order=10, min=0, max=1, modifier=false, summary=true) 1720 @Description(shortDefinition="Healthcare event during which this observation is made", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made." ) 1721 protected Reference encounter; 1722 1723 /** 1724 * The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself. 1725 */ 1726 @Child(name = "effective", type = {DateTimeType.class, Period.class, Timing.class, InstantType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1727 @Description(shortDefinition="Clinically relevant time/time-period for observation", formalDefinition="The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself." ) 1728 protected DataType effective; 1729 1730 /** 1731 * The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified. 1732 */ 1733 @Child(name = "issued", type = {InstantType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1734 @Description(shortDefinition="Date/Time this version was made available", formalDefinition="The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified." ) 1735 protected InstantType issued; 1736 1737 /** 1738 * Who was responsible for asserting the observed value as "true". 1739 */ 1740 @Child(name = "performer", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, RelatedPerson.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1741 @Description(shortDefinition="Who is responsible for the observation", formalDefinition="Who was responsible for asserting the observed value as \"true\"." ) 1742 protected List<Reference> performer; 1743 1744 /** 1745 * The information determined as a result of making the observation, if the information has a simple value. 1746 */ 1747 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, Period.class, Attachment.class, MolecularSequence.class}, order=14, min=0, max=1, modifier=false, summary=true) 1748 @Description(shortDefinition="Actual result", formalDefinition="The information determined as a result of making the observation, if the information has a simple value." ) 1749 protected DataType value; 1750 1751 /** 1752 * Provides a reason why the expected value in the element Observation.value[x] is missing. 1753 */ 1754 @Child(name = "dataAbsentReason", type = {CodeableConcept.class}, order=15, min=0, max=1, modifier=false, summary=false) 1755 @Description(shortDefinition="Why the result is missing", formalDefinition="Provides a reason why the expected value in the element Observation.value[x] is missing." ) 1756 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/data-absent-reason") 1757 protected CodeableConcept dataAbsentReason; 1758 1759 /** 1760 * A categorical assessment of an observation value. For example, high, low, normal. 1761 */ 1762 @Child(name = "interpretation", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1763 @Description(shortDefinition="High, low, normal, etc", formalDefinition="A categorical assessment of an observation value. For example, high, low, normal." ) 1764 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-interpretation") 1765 protected List<CodeableConcept> interpretation; 1766 1767 /** 1768 * Comments about the observation or the results. 1769 */ 1770 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1771 @Description(shortDefinition="Comments about the observation", formalDefinition="Comments about the observation or the results." ) 1772 protected List<Annotation> note; 1773 1774 /** 1775 * Indicates the site on the subject's body where the observation was made (i.e. the target site). 1776 */ 1777 @Child(name = "bodySite", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=false) 1778 @Description(shortDefinition="Observed body part", formalDefinition="Indicates the site on the subject's body where the observation was made (i.e. the target site)." ) 1779 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1780 protected CodeableConcept bodySite; 1781 1782 /** 1783 * Indicates the body structure on the subject's body where the observation was made (i.e. the target site). 1784 */ 1785 @Child(name = "bodyStructure", type = {BodyStructure.class}, order=19, min=0, max=1, modifier=false, summary=false) 1786 @Description(shortDefinition="Observed body structure", formalDefinition="Indicates the body structure on the subject's body where the observation was made (i.e. the target site)." ) 1787 protected Reference bodyStructure; 1788 1789 /** 1790 * Indicates the mechanism used to perform the observation. 1791 */ 1792 @Child(name = "method", type = {CodeableConcept.class}, order=20, min=0, max=1, modifier=false, summary=false) 1793 @Description(shortDefinition="How it was done", formalDefinition="Indicates the mechanism used to perform the observation." ) 1794 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-methods") 1795 protected CodeableConcept method; 1796 1797 /** 1798 * The specimen that was used when this observation was made. 1799 */ 1800 @Child(name = "specimen", type = {Specimen.class, Group.class}, order=21, min=0, max=1, modifier=false, summary=false) 1801 @Description(shortDefinition="Specimen used for this observation", formalDefinition="The specimen that was used when this observation was made." ) 1802 protected Reference specimen; 1803 1804 /** 1805 * A reference to the device that generates the measurements or the device settings for the device. 1806 */ 1807 @Child(name = "device", type = {Device.class, DeviceMetric.class}, order=22, min=0, max=1, modifier=false, summary=false) 1808 @Description(shortDefinition="A reference to the device that generates the measurements or the device settings for the device", formalDefinition="A reference to the device that generates the measurements or the device settings for the device." ) 1809 protected Reference device; 1810 1811 /** 1812 * Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an "OR". In other words, to represent two distinct target populations, two `referenceRange` elements would be used. 1813 */ 1814 @Child(name = "referenceRange", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1815 @Description(shortDefinition="Provides guide for interpretation", formalDefinition="Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used." ) 1816 protected List<ObservationReferenceRangeComponent> referenceRange; 1817 1818 /** 1819 * This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group. 1820 */ 1821 @Child(name = "hasMember", type = {Observation.class, QuestionnaireResponse.class, MolecularSequence.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1822 @Description(shortDefinition="Related resource that belongs to the Observation group", formalDefinition="This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group." ) 1823 protected List<Reference> hasMember; 1824 1825 /** 1826 * The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image. 1827 */ 1828 @Child(name = "derivedFrom", type = {DocumentReference.class, ImagingStudy.class, ImagingSelection.class, QuestionnaireResponse.class, Observation.class, MolecularSequence.class, GenomicStudy.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1829 @Description(shortDefinition="Related resource from which the observation is made", formalDefinition="The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image." ) 1830 protected List<Reference> derivedFrom; 1831 1832 /** 1833 * Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations. 1834 */ 1835 @Child(name = "component", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1836 @Description(shortDefinition="Component results", formalDefinition="Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations." ) 1837 protected List<ObservationComponentComponent> component; 1838 1839 private static final long serialVersionUID = -44103660L; 1840 1841 /** 1842 * Constructor 1843 */ 1844 public Observation() { 1845 super(); 1846 } 1847 1848 /** 1849 * Constructor 1850 */ 1851 public Observation(ObservationStatus status, CodeableConcept code) { 1852 super(); 1853 this.setStatus(status); 1854 this.setCode(code); 1855 } 1856 1857 /** 1858 * @return {@link #identifier} (A unique identifier assigned to this observation.) 1859 */ 1860 public List<Identifier> getIdentifier() { 1861 if (this.identifier == null) 1862 this.identifier = new ArrayList<Identifier>(); 1863 return this.identifier; 1864 } 1865 1866 /** 1867 * @return Returns a reference to <code>this</code> for easy method chaining 1868 */ 1869 public Observation setIdentifier(List<Identifier> theIdentifier) { 1870 this.identifier = theIdentifier; 1871 return this; 1872 } 1873 1874 public boolean hasIdentifier() { 1875 if (this.identifier == null) 1876 return false; 1877 for (Identifier item : this.identifier) 1878 if (!item.isEmpty()) 1879 return true; 1880 return false; 1881 } 1882 1883 public Identifier addIdentifier() { //3 1884 Identifier t = new Identifier(); 1885 if (this.identifier == null) 1886 this.identifier = new ArrayList<Identifier>(); 1887 this.identifier.add(t); 1888 return t; 1889 } 1890 1891 public Observation addIdentifier(Identifier t) { //3 1892 if (t == null) 1893 return this; 1894 if (this.identifier == null) 1895 this.identifier = new ArrayList<Identifier>(); 1896 this.identifier.add(t); 1897 return this; 1898 } 1899 1900 /** 1901 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1902 */ 1903 public Identifier getIdentifierFirstRep() { 1904 if (getIdentifier().isEmpty()) { 1905 addIdentifier(); 1906 } 1907 return getIdentifier().get(0); 1908 } 1909 1910 /** 1911 * @return {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 1912 */ 1913 public DataType getInstantiates() { 1914 return this.instantiates; 1915 } 1916 1917 /** 1918 * @return {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 1919 */ 1920 public CanonicalType getInstantiatesCanonicalType() throws FHIRException { 1921 if (this.instantiates == null) 1922 this.instantiates = new CanonicalType(); 1923 if (!(this.instantiates instanceof CanonicalType)) 1924 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.instantiates.getClass().getName()+" was encountered"); 1925 return (CanonicalType) this.instantiates; 1926 } 1927 1928 public boolean hasInstantiatesCanonicalType() { 1929 return this != null && this.instantiates instanceof CanonicalType; 1930 } 1931 1932 /** 1933 * @return {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 1934 */ 1935 public Reference getInstantiatesReference() throws FHIRException { 1936 if (this.instantiates == null) 1937 this.instantiates = new Reference(); 1938 if (!(this.instantiates instanceof Reference)) 1939 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.instantiates.getClass().getName()+" was encountered"); 1940 return (Reference) this.instantiates; 1941 } 1942 1943 public boolean hasInstantiatesReference() { 1944 return this != null && this.instantiates instanceof Reference; 1945 } 1946 1947 public boolean hasInstantiates() { 1948 return this.instantiates != null && !this.instantiates.isEmpty(); 1949 } 1950 1951 /** 1952 * @param value {@link #instantiates} (The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.) 1953 */ 1954 public Observation setInstantiates(DataType value) { 1955 if (value != null && !(value instanceof CanonicalType || value instanceof Reference)) 1956 throw new FHIRException("Not the right type for Observation.instantiates[x]: "+value.fhirType()); 1957 this.instantiates = value; 1958 return this; 1959 } 1960 1961 /** 1962 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.) 1963 */ 1964 public List<Reference> getBasedOn() { 1965 if (this.basedOn == null) 1966 this.basedOn = new ArrayList<Reference>(); 1967 return this.basedOn; 1968 } 1969 1970 /** 1971 * @return Returns a reference to <code>this</code> for easy method chaining 1972 */ 1973 public Observation setBasedOn(List<Reference> theBasedOn) { 1974 this.basedOn = theBasedOn; 1975 return this; 1976 } 1977 1978 public boolean hasBasedOn() { 1979 if (this.basedOn == null) 1980 return false; 1981 for (Reference item : this.basedOn) 1982 if (!item.isEmpty()) 1983 return true; 1984 return false; 1985 } 1986 1987 public Reference addBasedOn() { //3 1988 Reference t = new Reference(); 1989 if (this.basedOn == null) 1990 this.basedOn = new ArrayList<Reference>(); 1991 this.basedOn.add(t); 1992 return t; 1993 } 1994 1995 public Observation addBasedOn(Reference t) { //3 1996 if (t == null) 1997 return this; 1998 if (this.basedOn == null) 1999 this.basedOn = new ArrayList<Reference>(); 2000 this.basedOn.add(t); 2001 return this; 2002 } 2003 2004 /** 2005 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 2006 */ 2007 public Reference getBasedOnFirstRep() { 2008 if (getBasedOn().isEmpty()) { 2009 addBasedOn(); 2010 } 2011 return getBasedOn().get(0); 2012 } 2013 2014 /** 2015 * @return {@link #triggeredBy} (Identifies the observation(s) that triggered the performance of this observation.) 2016 */ 2017 public List<ObservationTriggeredByComponent> getTriggeredBy() { 2018 if (this.triggeredBy == null) 2019 this.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 2020 return this.triggeredBy; 2021 } 2022 2023 /** 2024 * @return Returns a reference to <code>this</code> for easy method chaining 2025 */ 2026 public Observation setTriggeredBy(List<ObservationTriggeredByComponent> theTriggeredBy) { 2027 this.triggeredBy = theTriggeredBy; 2028 return this; 2029 } 2030 2031 public boolean hasTriggeredBy() { 2032 if (this.triggeredBy == null) 2033 return false; 2034 for (ObservationTriggeredByComponent item : this.triggeredBy) 2035 if (!item.isEmpty()) 2036 return true; 2037 return false; 2038 } 2039 2040 public ObservationTriggeredByComponent addTriggeredBy() { //3 2041 ObservationTriggeredByComponent t = new ObservationTriggeredByComponent(); 2042 if (this.triggeredBy == null) 2043 this.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 2044 this.triggeredBy.add(t); 2045 return t; 2046 } 2047 2048 public Observation addTriggeredBy(ObservationTriggeredByComponent t) { //3 2049 if (t == null) 2050 return this; 2051 if (this.triggeredBy == null) 2052 this.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 2053 this.triggeredBy.add(t); 2054 return this; 2055 } 2056 2057 /** 2058 * @return The first repetition of repeating field {@link #triggeredBy}, creating it if it does not already exist {3} 2059 */ 2060 public ObservationTriggeredByComponent getTriggeredByFirstRep() { 2061 if (getTriggeredBy().isEmpty()) { 2062 addTriggeredBy(); 2063 } 2064 return getTriggeredBy().get(0); 2065 } 2066 2067 /** 2068 * @return {@link #partOf} (A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.) 2069 */ 2070 public List<Reference> getPartOf() { 2071 if (this.partOf == null) 2072 this.partOf = new ArrayList<Reference>(); 2073 return this.partOf; 2074 } 2075 2076 /** 2077 * @return Returns a reference to <code>this</code> for easy method chaining 2078 */ 2079 public Observation setPartOf(List<Reference> thePartOf) { 2080 this.partOf = thePartOf; 2081 return this; 2082 } 2083 2084 public boolean hasPartOf() { 2085 if (this.partOf == null) 2086 return false; 2087 for (Reference item : this.partOf) 2088 if (!item.isEmpty()) 2089 return true; 2090 return false; 2091 } 2092 2093 public Reference addPartOf() { //3 2094 Reference t = new Reference(); 2095 if (this.partOf == null) 2096 this.partOf = new ArrayList<Reference>(); 2097 this.partOf.add(t); 2098 return t; 2099 } 2100 2101 public Observation addPartOf(Reference t) { //3 2102 if (t == null) 2103 return this; 2104 if (this.partOf == null) 2105 this.partOf = new ArrayList<Reference>(); 2106 this.partOf.add(t); 2107 return this; 2108 } 2109 2110 /** 2111 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 2112 */ 2113 public Reference getPartOfFirstRep() { 2114 if (getPartOf().isEmpty()) { 2115 addPartOf(); 2116 } 2117 return getPartOf().get(0); 2118 } 2119 2120 /** 2121 * @return {@link #status} (The status of the result value.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2122 */ 2123 public Enumeration<ObservationStatus> getStatusElement() { 2124 if (this.status == null) 2125 if (Configuration.errorOnAutoCreate()) 2126 throw new Error("Attempt to auto-create Observation.status"); 2127 else if (Configuration.doAutoCreate()) 2128 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); // bb 2129 return this.status; 2130 } 2131 2132 public boolean hasStatusElement() { 2133 return this.status != null && !this.status.isEmpty(); 2134 } 2135 2136 public boolean hasStatus() { 2137 return this.status != null && !this.status.isEmpty(); 2138 } 2139 2140 /** 2141 * @param value {@link #status} (The status of the result value.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2142 */ 2143 public Observation setStatusElement(Enumeration<ObservationStatus> value) { 2144 this.status = value; 2145 return this; 2146 } 2147 2148 /** 2149 * @return The status of the result value. 2150 */ 2151 public ObservationStatus getStatus() { 2152 return this.status == null ? null : this.status.getValue(); 2153 } 2154 2155 /** 2156 * @param value The status of the result value. 2157 */ 2158 public Observation setStatus(ObservationStatus value) { 2159 if (this.status == null) 2160 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); 2161 this.status.setValue(value); 2162 return this; 2163 } 2164 2165 /** 2166 * @return {@link #category} (A code that classifies the general type of observation being made.) 2167 */ 2168 public List<CodeableConcept> getCategory() { 2169 if (this.category == null) 2170 this.category = new ArrayList<CodeableConcept>(); 2171 return this.category; 2172 } 2173 2174 /** 2175 * @return Returns a reference to <code>this</code> for easy method chaining 2176 */ 2177 public Observation setCategory(List<CodeableConcept> theCategory) { 2178 this.category = theCategory; 2179 return this; 2180 } 2181 2182 public boolean hasCategory() { 2183 if (this.category == null) 2184 return false; 2185 for (CodeableConcept item : this.category) 2186 if (!item.isEmpty()) 2187 return true; 2188 return false; 2189 } 2190 2191 public CodeableConcept addCategory() { //3 2192 CodeableConcept t = new CodeableConcept(); 2193 if (this.category == null) 2194 this.category = new ArrayList<CodeableConcept>(); 2195 this.category.add(t); 2196 return t; 2197 } 2198 2199 public Observation addCategory(CodeableConcept t) { //3 2200 if (t == null) 2201 return this; 2202 if (this.category == null) 2203 this.category = new ArrayList<CodeableConcept>(); 2204 this.category.add(t); 2205 return this; 2206 } 2207 2208 /** 2209 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2210 */ 2211 public CodeableConcept getCategoryFirstRep() { 2212 if (getCategory().isEmpty()) { 2213 addCategory(); 2214 } 2215 return getCategory().get(0); 2216 } 2217 2218 /** 2219 * @return {@link #code} (Describes what was observed. Sometimes this is called the observation "name".) 2220 */ 2221 public CodeableConcept getCode() { 2222 if (this.code == null) 2223 if (Configuration.errorOnAutoCreate()) 2224 throw new Error("Attempt to auto-create Observation.code"); 2225 else if (Configuration.doAutoCreate()) 2226 this.code = new CodeableConcept(); // cc 2227 return this.code; 2228 } 2229 2230 public boolean hasCode() { 2231 return this.code != null && !this.code.isEmpty(); 2232 } 2233 2234 /** 2235 * @param value {@link #code} (Describes what was observed. Sometimes this is called the observation "name".) 2236 */ 2237 public Observation setCode(CodeableConcept value) { 2238 this.code = value; 2239 return this; 2240 } 2241 2242 /** 2243 * @return {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.) 2244 */ 2245 public Reference getSubject() { 2246 if (this.subject == null) 2247 if (Configuration.errorOnAutoCreate()) 2248 throw new Error("Attempt to auto-create Observation.subject"); 2249 else if (Configuration.doAutoCreate()) 2250 this.subject = new Reference(); // cc 2251 return this.subject; 2252 } 2253 2254 public boolean hasSubject() { 2255 return this.subject != null && !this.subject.isEmpty(); 2256 } 2257 2258 /** 2259 * @param value {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.) 2260 */ 2261 public Observation setSubject(Reference value) { 2262 this.subject = value; 2263 return this; 2264 } 2265 2266 /** 2267 * @return {@link #focus} (The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.) 2268 */ 2269 public List<Reference> getFocus() { 2270 if (this.focus == null) 2271 this.focus = new ArrayList<Reference>(); 2272 return this.focus; 2273 } 2274 2275 /** 2276 * @return Returns a reference to <code>this</code> for easy method chaining 2277 */ 2278 public Observation setFocus(List<Reference> theFocus) { 2279 this.focus = theFocus; 2280 return this; 2281 } 2282 2283 public boolean hasFocus() { 2284 if (this.focus == null) 2285 return false; 2286 for (Reference item : this.focus) 2287 if (!item.isEmpty()) 2288 return true; 2289 return false; 2290 } 2291 2292 public Reference addFocus() { //3 2293 Reference t = new Reference(); 2294 if (this.focus == null) 2295 this.focus = new ArrayList<Reference>(); 2296 this.focus.add(t); 2297 return t; 2298 } 2299 2300 public Observation addFocus(Reference t) { //3 2301 if (t == null) 2302 return this; 2303 if (this.focus == null) 2304 this.focus = new ArrayList<Reference>(); 2305 this.focus.add(t); 2306 return this; 2307 } 2308 2309 /** 2310 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 2311 */ 2312 public Reference getFocusFirstRep() { 2313 if (getFocus().isEmpty()) { 2314 addFocus(); 2315 } 2316 return getFocus().get(0); 2317 } 2318 2319 /** 2320 * @return {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2321 */ 2322 public Reference getEncounter() { 2323 if (this.encounter == null) 2324 if (Configuration.errorOnAutoCreate()) 2325 throw new Error("Attempt to auto-create Observation.encounter"); 2326 else if (Configuration.doAutoCreate()) 2327 this.encounter = new Reference(); // cc 2328 return this.encounter; 2329 } 2330 2331 public boolean hasEncounter() { 2332 return this.encounter != null && !this.encounter.isEmpty(); 2333 } 2334 2335 /** 2336 * @param value {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2337 */ 2338 public Observation setEncounter(Reference value) { 2339 this.encounter = value; 2340 return this; 2341 } 2342 2343 /** 2344 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2345 */ 2346 public DataType getEffective() { 2347 return this.effective; 2348 } 2349 2350 /** 2351 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2352 */ 2353 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 2354 if (this.effective == null) 2355 this.effective = new DateTimeType(); 2356 if (!(this.effective instanceof DateTimeType)) 2357 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 2358 return (DateTimeType) this.effective; 2359 } 2360 2361 public boolean hasEffectiveDateTimeType() { 2362 return this != null && this.effective instanceof DateTimeType; 2363 } 2364 2365 /** 2366 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2367 */ 2368 public Period getEffectivePeriod() throws FHIRException { 2369 if (this.effective == null) 2370 this.effective = new Period(); 2371 if (!(this.effective instanceof Period)) 2372 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 2373 return (Period) this.effective; 2374 } 2375 2376 public boolean hasEffectivePeriod() { 2377 return this != null && this.effective instanceof Period; 2378 } 2379 2380 /** 2381 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2382 */ 2383 public Timing getEffectiveTiming() throws FHIRException { 2384 if (this.effective == null) 2385 this.effective = new Timing(); 2386 if (!(this.effective instanceof Timing)) 2387 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.effective.getClass().getName()+" was encountered"); 2388 return (Timing) this.effective; 2389 } 2390 2391 public boolean hasEffectiveTiming() { 2392 return this != null && this.effective instanceof Timing; 2393 } 2394 2395 /** 2396 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2397 */ 2398 public InstantType getEffectiveInstantType() throws FHIRException { 2399 if (this.effective == null) 2400 this.effective = new InstantType(); 2401 if (!(this.effective instanceof InstantType)) 2402 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.effective.getClass().getName()+" was encountered"); 2403 return (InstantType) this.effective; 2404 } 2405 2406 public boolean hasEffectiveInstantType() { 2407 return this != null && this.effective instanceof InstantType; 2408 } 2409 2410 public boolean hasEffective() { 2411 return this.effective != null && !this.effective.isEmpty(); 2412 } 2413 2414 /** 2415 * @param value {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2416 */ 2417 public Observation setEffective(DataType value) { 2418 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing || value instanceof InstantType)) 2419 throw new FHIRException("Not the right type for Observation.effective[x]: "+value.fhirType()); 2420 this.effective = value; 2421 return this; 2422 } 2423 2424 /** 2425 * @return {@link #issued} (The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2426 */ 2427 public InstantType getIssuedElement() { 2428 if (this.issued == null) 2429 if (Configuration.errorOnAutoCreate()) 2430 throw new Error("Attempt to auto-create Observation.issued"); 2431 else if (Configuration.doAutoCreate()) 2432 this.issued = new InstantType(); // bb 2433 return this.issued; 2434 } 2435 2436 public boolean hasIssuedElement() { 2437 return this.issued != null && !this.issued.isEmpty(); 2438 } 2439 2440 public boolean hasIssued() { 2441 return this.issued != null && !this.issued.isEmpty(); 2442 } 2443 2444 /** 2445 * @param value {@link #issued} (The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2446 */ 2447 public Observation setIssuedElement(InstantType value) { 2448 this.issued = value; 2449 return this; 2450 } 2451 2452 /** 2453 * @return The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified. 2454 */ 2455 public Date getIssued() { 2456 return this.issued == null ? null : this.issued.getValue(); 2457 } 2458 2459 /** 2460 * @param value The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified. 2461 */ 2462 public Observation setIssued(Date value) { 2463 if (value == null) 2464 this.issued = null; 2465 else { 2466 if (this.issued == null) 2467 this.issued = new InstantType(); 2468 this.issued.setValue(value); 2469 } 2470 return this; 2471 } 2472 2473 /** 2474 * @return {@link #performer} (Who was responsible for asserting the observed value as "true".) 2475 */ 2476 public List<Reference> getPerformer() { 2477 if (this.performer == null) 2478 this.performer = new ArrayList<Reference>(); 2479 return this.performer; 2480 } 2481 2482 /** 2483 * @return Returns a reference to <code>this</code> for easy method chaining 2484 */ 2485 public Observation setPerformer(List<Reference> thePerformer) { 2486 this.performer = thePerformer; 2487 return this; 2488 } 2489 2490 public boolean hasPerformer() { 2491 if (this.performer == null) 2492 return false; 2493 for (Reference item : this.performer) 2494 if (!item.isEmpty()) 2495 return true; 2496 return false; 2497 } 2498 2499 public Reference addPerformer() { //3 2500 Reference t = new Reference(); 2501 if (this.performer == null) 2502 this.performer = new ArrayList<Reference>(); 2503 this.performer.add(t); 2504 return t; 2505 } 2506 2507 public Observation addPerformer(Reference t) { //3 2508 if (t == null) 2509 return this; 2510 if (this.performer == null) 2511 this.performer = new ArrayList<Reference>(); 2512 this.performer.add(t); 2513 return this; 2514 } 2515 2516 /** 2517 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2518 */ 2519 public Reference getPerformerFirstRep() { 2520 if (getPerformer().isEmpty()) { 2521 addPerformer(); 2522 } 2523 return getPerformer().get(0); 2524 } 2525 2526 /** 2527 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2528 */ 2529 public DataType getValue() { 2530 return this.value; 2531 } 2532 2533 /** 2534 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2535 */ 2536 public Quantity getValueQuantity() throws FHIRException { 2537 if (this.value == null) 2538 this.value = new Quantity(); 2539 if (!(this.value instanceof Quantity)) 2540 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2541 return (Quantity) this.value; 2542 } 2543 2544 public boolean hasValueQuantity() { 2545 return this != null && this.value instanceof Quantity; 2546 } 2547 2548 /** 2549 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2550 */ 2551 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2552 if (this.value == null) 2553 this.value = new CodeableConcept(); 2554 if (!(this.value instanceof CodeableConcept)) 2555 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2556 return (CodeableConcept) this.value; 2557 } 2558 2559 public boolean hasValueCodeableConcept() { 2560 return this != null && this.value instanceof CodeableConcept; 2561 } 2562 2563 /** 2564 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2565 */ 2566 public StringType getValueStringType() throws FHIRException { 2567 if (this.value == null) 2568 this.value = new StringType(); 2569 if (!(this.value instanceof StringType)) 2570 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2571 return (StringType) this.value; 2572 } 2573 2574 public boolean hasValueStringType() { 2575 return this != null && this.value instanceof StringType; 2576 } 2577 2578 /** 2579 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2580 */ 2581 public BooleanType getValueBooleanType() throws FHIRException { 2582 if (this.value == null) 2583 this.value = new BooleanType(); 2584 if (!(this.value instanceof BooleanType)) 2585 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2586 return (BooleanType) this.value; 2587 } 2588 2589 public boolean hasValueBooleanType() { 2590 return this != null && this.value instanceof BooleanType; 2591 } 2592 2593 /** 2594 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2595 */ 2596 public IntegerType getValueIntegerType() throws FHIRException { 2597 if (this.value == null) 2598 this.value = new IntegerType(); 2599 if (!(this.value instanceof IntegerType)) 2600 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2601 return (IntegerType) this.value; 2602 } 2603 2604 public boolean hasValueIntegerType() { 2605 return this != null && this.value instanceof IntegerType; 2606 } 2607 2608 /** 2609 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2610 */ 2611 public Range getValueRange() throws FHIRException { 2612 if (this.value == null) 2613 this.value = new Range(); 2614 if (!(this.value instanceof Range)) 2615 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2616 return (Range) this.value; 2617 } 2618 2619 public boolean hasValueRange() { 2620 return this != null && this.value instanceof Range; 2621 } 2622 2623 /** 2624 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2625 */ 2626 public Ratio getValueRatio() throws FHIRException { 2627 if (this.value == null) 2628 this.value = new Ratio(); 2629 if (!(this.value instanceof Ratio)) 2630 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 2631 return (Ratio) this.value; 2632 } 2633 2634 public boolean hasValueRatio() { 2635 return this != null && this.value instanceof Ratio; 2636 } 2637 2638 /** 2639 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2640 */ 2641 public SampledData getValueSampledData() throws FHIRException { 2642 if (this.value == null) 2643 this.value = new SampledData(); 2644 if (!(this.value instanceof SampledData)) 2645 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 2646 return (SampledData) this.value; 2647 } 2648 2649 public boolean hasValueSampledData() { 2650 return this != null && this.value instanceof SampledData; 2651 } 2652 2653 /** 2654 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2655 */ 2656 public TimeType getValueTimeType() throws FHIRException { 2657 if (this.value == null) 2658 this.value = new TimeType(); 2659 if (!(this.value instanceof TimeType)) 2660 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2661 return (TimeType) this.value; 2662 } 2663 2664 public boolean hasValueTimeType() { 2665 return this != null && this.value instanceof TimeType; 2666 } 2667 2668 /** 2669 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2670 */ 2671 public DateTimeType getValueDateTimeType() throws FHIRException { 2672 if (this.value == null) 2673 this.value = new DateTimeType(); 2674 if (!(this.value instanceof DateTimeType)) 2675 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2676 return (DateTimeType) this.value; 2677 } 2678 2679 public boolean hasValueDateTimeType() { 2680 return this != null && this.value instanceof DateTimeType; 2681 } 2682 2683 /** 2684 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2685 */ 2686 public Period getValuePeriod() throws FHIRException { 2687 if (this.value == null) 2688 this.value = new Period(); 2689 if (!(this.value instanceof Period)) 2690 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 2691 return (Period) this.value; 2692 } 2693 2694 public boolean hasValuePeriod() { 2695 return this != null && this.value instanceof Period; 2696 } 2697 2698 /** 2699 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2700 */ 2701 public Attachment getValueAttachment() throws FHIRException { 2702 if (this.value == null) 2703 this.value = new Attachment(); 2704 if (!(this.value instanceof Attachment)) 2705 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 2706 return (Attachment) this.value; 2707 } 2708 2709 public boolean hasValueAttachment() { 2710 return this != null && this.value instanceof Attachment; 2711 } 2712 2713 /** 2714 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2715 */ 2716 public Reference getValueReference() throws FHIRException { 2717 if (this.value == null) 2718 this.value = new Reference(); 2719 if (!(this.value instanceof Reference)) 2720 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 2721 return (Reference) this.value; 2722 } 2723 2724 public boolean hasValueReference() { 2725 return this != null && this.value instanceof Reference; 2726 } 2727 2728 public boolean hasValue() { 2729 return this.value != null && !this.value.isEmpty(); 2730 } 2731 2732 /** 2733 * @param value {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2734 */ 2735 public Observation setValue(DataType value) { 2736 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Ratio || value instanceof SampledData || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period || value instanceof Attachment || value instanceof Reference)) 2737 throw new FHIRException("Not the right type for Observation.value[x]: "+value.fhirType()); 2738 this.value = value; 2739 return this; 2740 } 2741 2742 /** 2743 * @return {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 2744 */ 2745 public CodeableConcept getDataAbsentReason() { 2746 if (this.dataAbsentReason == null) 2747 if (Configuration.errorOnAutoCreate()) 2748 throw new Error("Attempt to auto-create Observation.dataAbsentReason"); 2749 else if (Configuration.doAutoCreate()) 2750 this.dataAbsentReason = new CodeableConcept(); // cc 2751 return this.dataAbsentReason; 2752 } 2753 2754 public boolean hasDataAbsentReason() { 2755 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 2756 } 2757 2758 /** 2759 * @param value {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 2760 */ 2761 public Observation setDataAbsentReason(CodeableConcept value) { 2762 this.dataAbsentReason = value; 2763 return this; 2764 } 2765 2766 /** 2767 * @return {@link #interpretation} (A categorical assessment of an observation value. For example, high, low, normal.) 2768 */ 2769 public List<CodeableConcept> getInterpretation() { 2770 if (this.interpretation == null) 2771 this.interpretation = new ArrayList<CodeableConcept>(); 2772 return this.interpretation; 2773 } 2774 2775 /** 2776 * @return Returns a reference to <code>this</code> for easy method chaining 2777 */ 2778 public Observation setInterpretation(List<CodeableConcept> theInterpretation) { 2779 this.interpretation = theInterpretation; 2780 return this; 2781 } 2782 2783 public boolean hasInterpretation() { 2784 if (this.interpretation == null) 2785 return false; 2786 for (CodeableConcept item : this.interpretation) 2787 if (!item.isEmpty()) 2788 return true; 2789 return false; 2790 } 2791 2792 public CodeableConcept addInterpretation() { //3 2793 CodeableConcept t = new CodeableConcept(); 2794 if (this.interpretation == null) 2795 this.interpretation = new ArrayList<CodeableConcept>(); 2796 this.interpretation.add(t); 2797 return t; 2798 } 2799 2800 public Observation addInterpretation(CodeableConcept t) { //3 2801 if (t == null) 2802 return this; 2803 if (this.interpretation == null) 2804 this.interpretation = new ArrayList<CodeableConcept>(); 2805 this.interpretation.add(t); 2806 return this; 2807 } 2808 2809 /** 2810 * @return The first repetition of repeating field {@link #interpretation}, creating it if it does not already exist {3} 2811 */ 2812 public CodeableConcept getInterpretationFirstRep() { 2813 if (getInterpretation().isEmpty()) { 2814 addInterpretation(); 2815 } 2816 return getInterpretation().get(0); 2817 } 2818 2819 /** 2820 * @return {@link #note} (Comments about the observation or the results.) 2821 */ 2822 public List<Annotation> getNote() { 2823 if (this.note == null) 2824 this.note = new ArrayList<Annotation>(); 2825 return this.note; 2826 } 2827 2828 /** 2829 * @return Returns a reference to <code>this</code> for easy method chaining 2830 */ 2831 public Observation setNote(List<Annotation> theNote) { 2832 this.note = theNote; 2833 return this; 2834 } 2835 2836 public boolean hasNote() { 2837 if (this.note == null) 2838 return false; 2839 for (Annotation item : this.note) 2840 if (!item.isEmpty()) 2841 return true; 2842 return false; 2843 } 2844 2845 public Annotation addNote() { //3 2846 Annotation t = new Annotation(); 2847 if (this.note == null) 2848 this.note = new ArrayList<Annotation>(); 2849 this.note.add(t); 2850 return t; 2851 } 2852 2853 public Observation addNote(Annotation t) { //3 2854 if (t == null) 2855 return this; 2856 if (this.note == null) 2857 this.note = new ArrayList<Annotation>(); 2858 this.note.add(t); 2859 return this; 2860 } 2861 2862 /** 2863 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2864 */ 2865 public Annotation getNoteFirstRep() { 2866 if (getNote().isEmpty()) { 2867 addNote(); 2868 } 2869 return getNote().get(0); 2870 } 2871 2872 /** 2873 * @return {@link #bodySite} (Indicates the site on the subject's body where the observation was made (i.e. the target site).) 2874 */ 2875 public CodeableConcept getBodySite() { 2876 if (this.bodySite == null) 2877 if (Configuration.errorOnAutoCreate()) 2878 throw new Error("Attempt to auto-create Observation.bodySite"); 2879 else if (Configuration.doAutoCreate()) 2880 this.bodySite = new CodeableConcept(); // cc 2881 return this.bodySite; 2882 } 2883 2884 public boolean hasBodySite() { 2885 return this.bodySite != null && !this.bodySite.isEmpty(); 2886 } 2887 2888 /** 2889 * @param value {@link #bodySite} (Indicates the site on the subject's body where the observation was made (i.e. the target site).) 2890 */ 2891 public Observation setBodySite(CodeableConcept value) { 2892 this.bodySite = value; 2893 return this; 2894 } 2895 2896 /** 2897 * @return {@link #bodyStructure} (Indicates the body structure on the subject's body where the observation was made (i.e. the target site).) 2898 */ 2899 public Reference getBodyStructure() { 2900 if (this.bodyStructure == null) 2901 if (Configuration.errorOnAutoCreate()) 2902 throw new Error("Attempt to auto-create Observation.bodyStructure"); 2903 else if (Configuration.doAutoCreate()) 2904 this.bodyStructure = new Reference(); // cc 2905 return this.bodyStructure; 2906 } 2907 2908 public boolean hasBodyStructure() { 2909 return this.bodyStructure != null && !this.bodyStructure.isEmpty(); 2910 } 2911 2912 /** 2913 * @param value {@link #bodyStructure} (Indicates the body structure on the subject's body where the observation was made (i.e. the target site).) 2914 */ 2915 public Observation setBodyStructure(Reference value) { 2916 this.bodyStructure = value; 2917 return this; 2918 } 2919 2920 /** 2921 * @return {@link #method} (Indicates the mechanism used to perform the observation.) 2922 */ 2923 public CodeableConcept getMethod() { 2924 if (this.method == null) 2925 if (Configuration.errorOnAutoCreate()) 2926 throw new Error("Attempt to auto-create Observation.method"); 2927 else if (Configuration.doAutoCreate()) 2928 this.method = new CodeableConcept(); // cc 2929 return this.method; 2930 } 2931 2932 public boolean hasMethod() { 2933 return this.method != null && !this.method.isEmpty(); 2934 } 2935 2936 /** 2937 * @param value {@link #method} (Indicates the mechanism used to perform the observation.) 2938 */ 2939 public Observation setMethod(CodeableConcept value) { 2940 this.method = value; 2941 return this; 2942 } 2943 2944 /** 2945 * @return {@link #specimen} (The specimen that was used when this observation was made.) 2946 */ 2947 public Reference getSpecimen() { 2948 if (this.specimen == null) 2949 if (Configuration.errorOnAutoCreate()) 2950 throw new Error("Attempt to auto-create Observation.specimen"); 2951 else if (Configuration.doAutoCreate()) 2952 this.specimen = new Reference(); // cc 2953 return this.specimen; 2954 } 2955 2956 public boolean hasSpecimen() { 2957 return this.specimen != null && !this.specimen.isEmpty(); 2958 } 2959 2960 /** 2961 * @param value {@link #specimen} (The specimen that was used when this observation was made.) 2962 */ 2963 public Observation setSpecimen(Reference value) { 2964 this.specimen = value; 2965 return this; 2966 } 2967 2968 /** 2969 * @return {@link #device} (A reference to the device that generates the measurements or the device settings for the device.) 2970 */ 2971 public Reference getDevice() { 2972 if (this.device == null) 2973 if (Configuration.errorOnAutoCreate()) 2974 throw new Error("Attempt to auto-create Observation.device"); 2975 else if (Configuration.doAutoCreate()) 2976 this.device = new Reference(); // cc 2977 return this.device; 2978 } 2979 2980 public boolean hasDevice() { 2981 return this.device != null && !this.device.isEmpty(); 2982 } 2983 2984 /** 2985 * @param value {@link #device} (A reference to the device that generates the measurements or the device settings for the device.) 2986 */ 2987 public Observation setDevice(Reference value) { 2988 this.device = value; 2989 return this; 2990 } 2991 2992 /** 2993 * @return {@link #referenceRange} (Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an "OR". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.) 2994 */ 2995 public List<ObservationReferenceRangeComponent> getReferenceRange() { 2996 if (this.referenceRange == null) 2997 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2998 return this.referenceRange; 2999 } 3000 3001 /** 3002 * @return Returns a reference to <code>this</code> for easy method chaining 3003 */ 3004 public Observation setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 3005 this.referenceRange = theReferenceRange; 3006 return this; 3007 } 3008 3009 public boolean hasReferenceRange() { 3010 if (this.referenceRange == null) 3011 return false; 3012 for (ObservationReferenceRangeComponent item : this.referenceRange) 3013 if (!item.isEmpty()) 3014 return true; 3015 return false; 3016 } 3017 3018 public ObservationReferenceRangeComponent addReferenceRange() { //3 3019 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 3020 if (this.referenceRange == null) 3021 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3022 this.referenceRange.add(t); 3023 return t; 3024 } 3025 3026 public Observation addReferenceRange(ObservationReferenceRangeComponent t) { //3 3027 if (t == null) 3028 return this; 3029 if (this.referenceRange == null) 3030 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3031 this.referenceRange.add(t); 3032 return this; 3033 } 3034 3035 /** 3036 * @return The first repetition of repeating field {@link #referenceRange}, creating it if it does not already exist {3} 3037 */ 3038 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 3039 if (getReferenceRange().isEmpty()) { 3040 addReferenceRange(); 3041 } 3042 return getReferenceRange().get(0); 3043 } 3044 3045 /** 3046 * @return {@link #hasMember} (This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.) 3047 */ 3048 public List<Reference> getHasMember() { 3049 if (this.hasMember == null) 3050 this.hasMember = new ArrayList<Reference>(); 3051 return this.hasMember; 3052 } 3053 3054 /** 3055 * @return Returns a reference to <code>this</code> for easy method chaining 3056 */ 3057 public Observation setHasMember(List<Reference> theHasMember) { 3058 this.hasMember = theHasMember; 3059 return this; 3060 } 3061 3062 public boolean hasHasMember() { 3063 if (this.hasMember == null) 3064 return false; 3065 for (Reference item : this.hasMember) 3066 if (!item.isEmpty()) 3067 return true; 3068 return false; 3069 } 3070 3071 public Reference addHasMember() { //3 3072 Reference t = new Reference(); 3073 if (this.hasMember == null) 3074 this.hasMember = new ArrayList<Reference>(); 3075 this.hasMember.add(t); 3076 return t; 3077 } 3078 3079 public Observation addHasMember(Reference t) { //3 3080 if (t == null) 3081 return this; 3082 if (this.hasMember == null) 3083 this.hasMember = new ArrayList<Reference>(); 3084 this.hasMember.add(t); 3085 return this; 3086 } 3087 3088 /** 3089 * @return The first repetition of repeating field {@link #hasMember}, creating it if it does not already exist {3} 3090 */ 3091 public Reference getHasMemberFirstRep() { 3092 if (getHasMember().isEmpty()) { 3093 addHasMember(); 3094 } 3095 return getHasMember().get(0); 3096 } 3097 3098 /** 3099 * @return {@link #derivedFrom} (The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.) 3100 */ 3101 public List<Reference> getDerivedFrom() { 3102 if (this.derivedFrom == null) 3103 this.derivedFrom = new ArrayList<Reference>(); 3104 return this.derivedFrom; 3105 } 3106 3107 /** 3108 * @return Returns a reference to <code>this</code> for easy method chaining 3109 */ 3110 public Observation setDerivedFrom(List<Reference> theDerivedFrom) { 3111 this.derivedFrom = theDerivedFrom; 3112 return this; 3113 } 3114 3115 public boolean hasDerivedFrom() { 3116 if (this.derivedFrom == null) 3117 return false; 3118 for (Reference item : this.derivedFrom) 3119 if (!item.isEmpty()) 3120 return true; 3121 return false; 3122 } 3123 3124 public Reference addDerivedFrom() { //3 3125 Reference t = new Reference(); 3126 if (this.derivedFrom == null) 3127 this.derivedFrom = new ArrayList<Reference>(); 3128 this.derivedFrom.add(t); 3129 return t; 3130 } 3131 3132 public Observation addDerivedFrom(Reference t) { //3 3133 if (t == null) 3134 return this; 3135 if (this.derivedFrom == null) 3136 this.derivedFrom = new ArrayList<Reference>(); 3137 this.derivedFrom.add(t); 3138 return this; 3139 } 3140 3141 /** 3142 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 3143 */ 3144 public Reference getDerivedFromFirstRep() { 3145 if (getDerivedFrom().isEmpty()) { 3146 addDerivedFrom(); 3147 } 3148 return getDerivedFrom().get(0); 3149 } 3150 3151 /** 3152 * @return {@link #component} (Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.) 3153 */ 3154 public List<ObservationComponentComponent> getComponent() { 3155 if (this.component == null) 3156 this.component = new ArrayList<ObservationComponentComponent>(); 3157 return this.component; 3158 } 3159 3160 /** 3161 * @return Returns a reference to <code>this</code> for easy method chaining 3162 */ 3163 public Observation setComponent(List<ObservationComponentComponent> theComponent) { 3164 this.component = theComponent; 3165 return this; 3166 } 3167 3168 public boolean hasComponent() { 3169 if (this.component == null) 3170 return false; 3171 for (ObservationComponentComponent item : this.component) 3172 if (!item.isEmpty()) 3173 return true; 3174 return false; 3175 } 3176 3177 public ObservationComponentComponent addComponent() { //3 3178 ObservationComponentComponent t = new ObservationComponentComponent(); 3179 if (this.component == null) 3180 this.component = new ArrayList<ObservationComponentComponent>(); 3181 this.component.add(t); 3182 return t; 3183 } 3184 3185 public Observation addComponent(ObservationComponentComponent t) { //3 3186 if (t == null) 3187 return this; 3188 if (this.component == null) 3189 this.component = new ArrayList<ObservationComponentComponent>(); 3190 this.component.add(t); 3191 return this; 3192 } 3193 3194 /** 3195 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 3196 */ 3197 public ObservationComponentComponent getComponentFirstRep() { 3198 if (getComponent().isEmpty()) { 3199 addComponent(); 3200 } 3201 return getComponent().get(0); 3202 } 3203 3204 protected void listChildren(List<Property> children) { 3205 super.listChildren(children); 3206 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3207 children.add(new Property("instantiates[x]", "canonical(ObservationDefinition)|Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates)); 3208 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3209 children.add(new Property("triggeredBy", "", "Identifies the observation(s) that triggered the performance of this observation.", 0, java.lang.Integer.MAX_VALUE, triggeredBy)); 3210 children.add(new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy|GenomicStudy)", "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 0, java.lang.Integer.MAX_VALUE, partOf)); 3211 children.add(new Property("status", "code", "The status of the result value.", 0, 1, status)); 3212 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, category)); 3213 children.add(new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 3214 children.add(new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|BiologicallyDerivedProduct|NutritionProduct)", "The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 0, 1, subject)); 3215 children.add(new Property("focus", "Reference(Any)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus)); 3216 children.add(new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 0, 1, encounter)); 3217 children.add(new Property("effective[x]", "dateTime|Period|Timing|instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective)); 3218 children.add(new Property("issued", "instant", "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 0, 1, issued)); 3219 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, performer)); 3220 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value)); 3221 children.add(new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason)); 3222 children.add(new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation)); 3223 children.add(new Property("note", "Annotation", "Comments about the observation or the results.", 0, java.lang.Integer.MAX_VALUE, note)); 3224 children.add(new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodySite)); 3225 children.add(new Property("bodyStructure", "Reference(BodyStructure)", "Indicates the body structure on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodyStructure)); 3226 children.add(new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 0, 1, method)); 3227 children.add(new Property("specimen", "Reference(Specimen|Group)", "The specimen that was used when this observation was made.", 0, 1, specimen)); 3228 children.add(new Property("device", "Reference(Device|DeviceMetric)", "A reference to the device that generates the measurements or the device settings for the device.", 0, 1, device)); 3229 children.add(new Property("referenceRange", "", "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 0, java.lang.Integer.MAX_VALUE, referenceRange)); 3230 children.add(new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember)); 3231 children.add(new Property("derivedFrom", "Reference(DocumentReference|ImagingStudy|ImagingSelection|QuestionnaireResponse|Observation|MolecularSequence|GenomicStudy)", "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 3232 children.add(new Property("component", "", "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 0, java.lang.Integer.MAX_VALUE, component)); 3233 } 3234 3235 @Override 3236 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3237 switch (_hash) { 3238 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier); 3239 case -1926387433: /*instantiates[x]*/ return new Property("instantiates[x]", "canonical(ObservationDefinition)|Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3240 case -246883639: /*instantiates*/ return new Property("instantiates[x]", "canonical(ObservationDefinition)|Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3241 case 8911915: /*instantiatesCanonical*/ return new Property("instantiates[x]", "canonical(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3242 case -1744595326: /*instantiatesReference*/ return new Property("instantiates[x]", "Reference(ObservationDefinition)", "The reference to a FHIR ObservationDefinition resource that provides the definition that is adhered to in whole or in part by this Observation instance.", 0, 1, instantiates); 3243 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3244 case -680451314: /*triggeredBy*/ return new Property("triggeredBy", "", "Identifies the observation(s) that triggered the performance of this observation.", 0, java.lang.Integer.MAX_VALUE, triggeredBy); 3245 case -995410646: /*partOf*/ return new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy|GenomicStudy)", "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 0, java.lang.Integer.MAX_VALUE, partOf); 3246 case -892481550: /*status*/ return new Property("status", "code", "The status of the result value.", 0, 1, status); 3247 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, category); 3248 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code); 3249 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|BiologicallyDerivedProduct|NutritionProduct)", "The patient, or group of patients, location, device, organization, procedure or practitioner this observation is about and into whose or what record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 0, 1, subject); 3250 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus); 3251 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 0, 1, encounter); 3252 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period|Timing|instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3253 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period|Timing|instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3254 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3255 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "Period", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3256 case -285872943: /*effectiveTiming*/ return new Property("effective[x]", "Timing", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3257 case -1295730118: /*effectiveInstant*/ return new Property("effective[x]", "instant", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 3258 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 0, 1, issued); 3259 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, performer); 3260 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3261 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period|Attachment|Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3262 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3263 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3264 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3265 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3266 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3267 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3268 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3269 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3270 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3271 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3272 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3273 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3274 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(MolecularSequence)", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 3275 case 1034315687: /*dataAbsentReason*/ return new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason); 3276 case -297950712: /*interpretation*/ return new Property("interpretation", "CodeableConcept", "A categorical assessment of an observation value. For example, high, low, normal.", 0, java.lang.Integer.MAX_VALUE, interpretation); 3277 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments about the observation or the results.", 0, java.lang.Integer.MAX_VALUE, note); 3278 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodySite); 3279 case -1001731599: /*bodyStructure*/ return new Property("bodyStructure", "Reference(BodyStructure)", "Indicates the body structure on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodyStructure); 3280 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 0, 1, method); 3281 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen|Group)", "The specimen that was used when this observation was made.", 0, 1, specimen); 3282 case -1335157162: /*device*/ return new Property("device", "Reference(Device|DeviceMetric)", "A reference to the device that generates the measurements or the device settings for the device.", 0, 1, device); 3283 case -1912545102: /*referenceRange*/ return new Property("referenceRange", "", "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 0, java.lang.Integer.MAX_VALUE, referenceRange); 3284 case -458019372: /*hasMember*/ return new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember); 3285 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(DocumentReference|ImagingStudy|ImagingSelection|QuestionnaireResponse|Observation|MolecularSequence|GenomicStudy)", "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 3286 case -1399907075: /*component*/ return new Property("component", "", "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 0, java.lang.Integer.MAX_VALUE, component); 3287 default: return super.getNamedProperty(_hash, _name, _checkValid); 3288 } 3289 3290 } 3291 3292 @Override 3293 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3294 switch (hash) { 3295 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3296 case -246883639: /*instantiates*/ return this.instantiates == null ? new Base[0] : new Base[] {this.instantiates}; // DataType 3297 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3298 case -680451314: /*triggeredBy*/ return this.triggeredBy == null ? new Base[0] : this.triggeredBy.toArray(new Base[this.triggeredBy.size()]); // ObservationTriggeredByComponent 3299 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 3300 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ObservationStatus> 3301 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3302 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3303 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3304 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 3305 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3306 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // DataType 3307 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 3308 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 3309 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3310 case 1034315687: /*dataAbsentReason*/ return this.dataAbsentReason == null ? new Base[0] : new Base[] {this.dataAbsentReason}; // CodeableConcept 3311 case -297950712: /*interpretation*/ return this.interpretation == null ? new Base[0] : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 3312 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3313 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 3314 case -1001731599: /*bodyStructure*/ return this.bodyStructure == null ? new Base[0] : new Base[] {this.bodyStructure}; // Reference 3315 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 3316 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : new Base[] {this.specimen}; // Reference 3317 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 3318 case -1912545102: /*referenceRange*/ return this.referenceRange == null ? new Base[0] : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 3319 case -458019372: /*hasMember*/ return this.hasMember == null ? new Base[0] : this.hasMember.toArray(new Base[this.hasMember.size()]); // Reference 3320 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 3321 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ObservationComponentComponent 3322 default: return super.getProperty(hash, name, checkValid); 3323 } 3324 3325 } 3326 3327 @Override 3328 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3329 switch (hash) { 3330 case -1618432855: // identifier 3331 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3332 return value; 3333 case -246883639: // instantiates 3334 this.instantiates = TypeConvertor.castToType(value); // DataType 3335 return value; 3336 case -332612366: // basedOn 3337 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 3338 return value; 3339 case -680451314: // triggeredBy 3340 this.getTriggeredBy().add((ObservationTriggeredByComponent) value); // ObservationTriggeredByComponent 3341 return value; 3342 case -995410646: // partOf 3343 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 3344 return value; 3345 case -892481550: // status 3346 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3347 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3348 return value; 3349 case 50511102: // category 3350 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3351 return value; 3352 case 3059181: // code 3353 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3354 return value; 3355 case -1867885268: // subject 3356 this.subject = TypeConvertor.castToReference(value); // Reference 3357 return value; 3358 case 97604824: // focus 3359 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 3360 return value; 3361 case 1524132147: // encounter 3362 this.encounter = TypeConvertor.castToReference(value); // Reference 3363 return value; 3364 case -1468651097: // effective 3365 this.effective = TypeConvertor.castToType(value); // DataType 3366 return value; 3367 case -1179159893: // issued 3368 this.issued = TypeConvertor.castToInstant(value); // InstantType 3369 return value; 3370 case 481140686: // performer 3371 this.getPerformer().add(TypeConvertor.castToReference(value)); // Reference 3372 return value; 3373 case 111972721: // value 3374 this.value = TypeConvertor.castToType(value); // DataType 3375 return value; 3376 case 1034315687: // dataAbsentReason 3377 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3378 return value; 3379 case -297950712: // interpretation 3380 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3381 return value; 3382 case 3387378: // note 3383 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3384 return value; 3385 case 1702620169: // bodySite 3386 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3387 return value; 3388 case -1001731599: // bodyStructure 3389 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 3390 return value; 3391 case -1077554975: // method 3392 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3393 return value; 3394 case -2132868344: // specimen 3395 this.specimen = TypeConvertor.castToReference(value); // Reference 3396 return value; 3397 case -1335157162: // device 3398 this.device = TypeConvertor.castToReference(value); // Reference 3399 return value; 3400 case -1912545102: // referenceRange 3401 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 3402 return value; 3403 case -458019372: // hasMember 3404 this.getHasMember().add(TypeConvertor.castToReference(value)); // Reference 3405 return value; 3406 case 1077922663: // derivedFrom 3407 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 3408 return value; 3409 case -1399907075: // component 3410 this.getComponent().add((ObservationComponentComponent) value); // ObservationComponentComponent 3411 return value; 3412 default: return super.setProperty(hash, name, value); 3413 } 3414 3415 } 3416 3417 @Override 3418 public Base setProperty(String name, Base value) throws FHIRException { 3419 if (name.equals("identifier")) { 3420 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3421 } else if (name.equals("instantiates[x]")) { 3422 this.instantiates = TypeConvertor.castToType(value); // DataType 3423 } else if (name.equals("basedOn")) { 3424 this.getBasedOn().add(TypeConvertor.castToReference(value)); 3425 } else if (name.equals("triggeredBy")) { 3426 this.getTriggeredBy().add((ObservationTriggeredByComponent) value); 3427 } else if (name.equals("partOf")) { 3428 this.getPartOf().add(TypeConvertor.castToReference(value)); 3429 } else if (name.equals("status")) { 3430 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3431 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3432 } else if (name.equals("category")) { 3433 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3434 } else if (name.equals("code")) { 3435 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3436 } else if (name.equals("subject")) { 3437 this.subject = TypeConvertor.castToReference(value); // Reference 3438 } else if (name.equals("focus")) { 3439 this.getFocus().add(TypeConvertor.castToReference(value)); 3440 } else if (name.equals("encounter")) { 3441 this.encounter = TypeConvertor.castToReference(value); // Reference 3442 } else if (name.equals("effective[x]")) { 3443 this.effective = TypeConvertor.castToType(value); // DataType 3444 } else if (name.equals("issued")) { 3445 this.issued = TypeConvertor.castToInstant(value); // InstantType 3446 } else if (name.equals("performer")) { 3447 this.getPerformer().add(TypeConvertor.castToReference(value)); 3448 } else if (name.equals("value[x]")) { 3449 this.value = TypeConvertor.castToType(value); // DataType 3450 } else if (name.equals("dataAbsentReason")) { 3451 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3452 } else if (name.equals("interpretation")) { 3453 this.getInterpretation().add(TypeConvertor.castToCodeableConcept(value)); 3454 } else if (name.equals("note")) { 3455 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3456 } else if (name.equals("bodySite")) { 3457 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3458 } else if (name.equals("bodyStructure")) { 3459 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 3460 } else if (name.equals("method")) { 3461 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3462 } else if (name.equals("specimen")) { 3463 this.specimen = TypeConvertor.castToReference(value); // Reference 3464 } else if (name.equals("device")) { 3465 this.device = TypeConvertor.castToReference(value); // Reference 3466 } else if (name.equals("referenceRange")) { 3467 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 3468 } else if (name.equals("hasMember")) { 3469 this.getHasMember().add(TypeConvertor.castToReference(value)); 3470 } else if (name.equals("derivedFrom")) { 3471 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 3472 } else if (name.equals("component")) { 3473 this.getComponent().add((ObservationComponentComponent) value); 3474 } else 3475 return super.setProperty(name, value); 3476 return value; 3477 } 3478 3479 @Override 3480 public Base makeProperty(int hash, String name) throws FHIRException { 3481 switch (hash) { 3482 case -1618432855: return addIdentifier(); 3483 case -1926387433: return getInstantiates(); 3484 case -246883639: return getInstantiates(); 3485 case -332612366: return addBasedOn(); 3486 case -680451314: return addTriggeredBy(); 3487 case -995410646: return addPartOf(); 3488 case -892481550: return getStatusElement(); 3489 case 50511102: return addCategory(); 3490 case 3059181: return getCode(); 3491 case -1867885268: return getSubject(); 3492 case 97604824: return addFocus(); 3493 case 1524132147: return getEncounter(); 3494 case 247104889: return getEffective(); 3495 case -1468651097: return getEffective(); 3496 case -1179159893: return getIssuedElement(); 3497 case 481140686: return addPerformer(); 3498 case -1410166417: return getValue(); 3499 case 111972721: return getValue(); 3500 case 1034315687: return getDataAbsentReason(); 3501 case -297950712: return addInterpretation(); 3502 case 3387378: return addNote(); 3503 case 1702620169: return getBodySite(); 3504 case -1001731599: return getBodyStructure(); 3505 case -1077554975: return getMethod(); 3506 case -2132868344: return getSpecimen(); 3507 case -1335157162: return getDevice(); 3508 case -1912545102: return addReferenceRange(); 3509 case -458019372: return addHasMember(); 3510 case 1077922663: return addDerivedFrom(); 3511 case -1399907075: return addComponent(); 3512 default: return super.makeProperty(hash, name); 3513 } 3514 3515 } 3516 3517 @Override 3518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3519 switch (hash) { 3520 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3521 case -246883639: /*instantiates*/ return new String[] {"canonical", "Reference"}; 3522 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3523 case -680451314: /*triggeredBy*/ return new String[] {}; 3524 case -995410646: /*partOf*/ return new String[] {"Reference"}; 3525 case -892481550: /*status*/ return new String[] {"code"}; 3526 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3527 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3528 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3529 case 97604824: /*focus*/ return new String[] {"Reference"}; 3530 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3531 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period", "Timing", "instant"}; 3532 case -1179159893: /*issued*/ return new String[] {"instant"}; 3533 case 481140686: /*performer*/ return new String[] {"Reference"}; 3534 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Ratio", "SampledData", "time", "dateTime", "Period", "Attachment", "Reference"}; 3535 case 1034315687: /*dataAbsentReason*/ return new String[] {"CodeableConcept"}; 3536 case -297950712: /*interpretation*/ return new String[] {"CodeableConcept"}; 3537 case 3387378: /*note*/ return new String[] {"Annotation"}; 3538 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 3539 case -1001731599: /*bodyStructure*/ return new String[] {"Reference"}; 3540 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 3541 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 3542 case -1335157162: /*device*/ return new String[] {"Reference"}; 3543 case -1912545102: /*referenceRange*/ return new String[] {}; 3544 case -458019372: /*hasMember*/ return new String[] {"Reference"}; 3545 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 3546 case -1399907075: /*component*/ return new String[] {}; 3547 default: return super.getTypesForProperty(hash, name); 3548 } 3549 3550 } 3551 3552 @Override 3553 public Base addChild(String name) throws FHIRException { 3554 if (name.equals("identifier")) { 3555 return addIdentifier(); 3556 } 3557 else if (name.equals("instantiatesCanonical")) { 3558 this.instantiates = new CanonicalType(); 3559 return this.instantiates; 3560 } 3561 else if (name.equals("instantiatesReference")) { 3562 this.instantiates = new Reference(); 3563 return this.instantiates; 3564 } 3565 else if (name.equals("basedOn")) { 3566 return addBasedOn(); 3567 } 3568 else if (name.equals("triggeredBy")) { 3569 return addTriggeredBy(); 3570 } 3571 else if (name.equals("partOf")) { 3572 return addPartOf(); 3573 } 3574 else if (name.equals("status")) { 3575 throw new FHIRException("Cannot call addChild on a singleton property Observation.status"); 3576 } 3577 else if (name.equals("category")) { 3578 return addCategory(); 3579 } 3580 else if (name.equals("code")) { 3581 this.code = new CodeableConcept(); 3582 return this.code; 3583 } 3584 else if (name.equals("subject")) { 3585 this.subject = new Reference(); 3586 return this.subject; 3587 } 3588 else if (name.equals("focus")) { 3589 return addFocus(); 3590 } 3591 else if (name.equals("encounter")) { 3592 this.encounter = new Reference(); 3593 return this.encounter; 3594 } 3595 else if (name.equals("effectiveDateTime")) { 3596 this.effective = new DateTimeType(); 3597 return this.effective; 3598 } 3599 else if (name.equals("effectivePeriod")) { 3600 this.effective = new Period(); 3601 return this.effective; 3602 } 3603 else if (name.equals("effectiveTiming")) { 3604 this.effective = new Timing(); 3605 return this.effective; 3606 } 3607 else if (name.equals("effectiveInstant")) { 3608 this.effective = new InstantType(); 3609 return this.effective; 3610 } 3611 else if (name.equals("issued")) { 3612 throw new FHIRException("Cannot call addChild on a singleton property Observation.issued"); 3613 } 3614 else if (name.equals("performer")) { 3615 return addPerformer(); 3616 } 3617 else if (name.equals("valueQuantity")) { 3618 this.value = new Quantity(); 3619 return this.value; 3620 } 3621 else if (name.equals("valueCodeableConcept")) { 3622 this.value = new CodeableConcept(); 3623 return this.value; 3624 } 3625 else if (name.equals("valueString")) { 3626 this.value = new StringType(); 3627 return this.value; 3628 } 3629 else if (name.equals("valueBoolean")) { 3630 this.value = new BooleanType(); 3631 return this.value; 3632 } 3633 else if (name.equals("valueInteger")) { 3634 this.value = new IntegerType(); 3635 return this.value; 3636 } 3637 else if (name.equals("valueRange")) { 3638 this.value = new Range(); 3639 return this.value; 3640 } 3641 else if (name.equals("valueRatio")) { 3642 this.value = new Ratio(); 3643 return this.value; 3644 } 3645 else if (name.equals("valueSampledData")) { 3646 this.value = new SampledData(); 3647 return this.value; 3648 } 3649 else if (name.equals("valueTime")) { 3650 this.value = new TimeType(); 3651 return this.value; 3652 } 3653 else if (name.equals("valueDateTime")) { 3654 this.value = new DateTimeType(); 3655 return this.value; 3656 } 3657 else if (name.equals("valuePeriod")) { 3658 this.value = new Period(); 3659 return this.value; 3660 } 3661 else if (name.equals("valueAttachment")) { 3662 this.value = new Attachment(); 3663 return this.value; 3664 } 3665 else if (name.equals("valueReference")) { 3666 this.value = new Reference(); 3667 return this.value; 3668 } 3669 else if (name.equals("dataAbsentReason")) { 3670 this.dataAbsentReason = new CodeableConcept(); 3671 return this.dataAbsentReason; 3672 } 3673 else if (name.equals("interpretation")) { 3674 return addInterpretation(); 3675 } 3676 else if (name.equals("note")) { 3677 return addNote(); 3678 } 3679 else if (name.equals("bodySite")) { 3680 this.bodySite = new CodeableConcept(); 3681 return this.bodySite; 3682 } 3683 else if (name.equals("bodyStructure")) { 3684 this.bodyStructure = new Reference(); 3685 return this.bodyStructure; 3686 } 3687 else if (name.equals("method")) { 3688 this.method = new CodeableConcept(); 3689 return this.method; 3690 } 3691 else if (name.equals("specimen")) { 3692 this.specimen = new Reference(); 3693 return this.specimen; 3694 } 3695 else if (name.equals("device")) { 3696 this.device = new Reference(); 3697 return this.device; 3698 } 3699 else if (name.equals("referenceRange")) { 3700 return addReferenceRange(); 3701 } 3702 else if (name.equals("hasMember")) { 3703 return addHasMember(); 3704 } 3705 else if (name.equals("derivedFrom")) { 3706 return addDerivedFrom(); 3707 } 3708 else if (name.equals("component")) { 3709 return addComponent(); 3710 } 3711 else 3712 return super.addChild(name); 3713 } 3714 3715 public String fhirType() { 3716 return "Observation"; 3717 3718 } 3719 3720 public Observation copy() { 3721 Observation dst = new Observation(); 3722 copyValues(dst); 3723 return dst; 3724 } 3725 3726 public void copyValues(Observation dst) { 3727 super.copyValues(dst); 3728 if (identifier != null) { 3729 dst.identifier = new ArrayList<Identifier>(); 3730 for (Identifier i : identifier) 3731 dst.identifier.add(i.copy()); 3732 }; 3733 dst.instantiates = instantiates == null ? null : instantiates.copy(); 3734 if (basedOn != null) { 3735 dst.basedOn = new ArrayList<Reference>(); 3736 for (Reference i : basedOn) 3737 dst.basedOn.add(i.copy()); 3738 }; 3739 if (triggeredBy != null) { 3740 dst.triggeredBy = new ArrayList<ObservationTriggeredByComponent>(); 3741 for (ObservationTriggeredByComponent i : triggeredBy) 3742 dst.triggeredBy.add(i.copy()); 3743 }; 3744 if (partOf != null) { 3745 dst.partOf = new ArrayList<Reference>(); 3746 for (Reference i : partOf) 3747 dst.partOf.add(i.copy()); 3748 }; 3749 dst.status = status == null ? null : status.copy(); 3750 if (category != null) { 3751 dst.category = new ArrayList<CodeableConcept>(); 3752 for (CodeableConcept i : category) 3753 dst.category.add(i.copy()); 3754 }; 3755 dst.code = code == null ? null : code.copy(); 3756 dst.subject = subject == null ? null : subject.copy(); 3757 if (focus != null) { 3758 dst.focus = new ArrayList<Reference>(); 3759 for (Reference i : focus) 3760 dst.focus.add(i.copy()); 3761 }; 3762 dst.encounter = encounter == null ? null : encounter.copy(); 3763 dst.effective = effective == null ? null : effective.copy(); 3764 dst.issued = issued == null ? null : issued.copy(); 3765 if (performer != null) { 3766 dst.performer = new ArrayList<Reference>(); 3767 for (Reference i : performer) 3768 dst.performer.add(i.copy()); 3769 }; 3770 dst.value = value == null ? null : value.copy(); 3771 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 3772 if (interpretation != null) { 3773 dst.interpretation = new ArrayList<CodeableConcept>(); 3774 for (CodeableConcept i : interpretation) 3775 dst.interpretation.add(i.copy()); 3776 }; 3777 if (note != null) { 3778 dst.note = new ArrayList<Annotation>(); 3779 for (Annotation i : note) 3780 dst.note.add(i.copy()); 3781 }; 3782 dst.bodySite = bodySite == null ? null : bodySite.copy(); 3783 dst.bodyStructure = bodyStructure == null ? null : bodyStructure.copy(); 3784 dst.method = method == null ? null : method.copy(); 3785 dst.specimen = specimen == null ? null : specimen.copy(); 3786 dst.device = device == null ? null : device.copy(); 3787 if (referenceRange != null) { 3788 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3789 for (ObservationReferenceRangeComponent i : referenceRange) 3790 dst.referenceRange.add(i.copy()); 3791 }; 3792 if (hasMember != null) { 3793 dst.hasMember = new ArrayList<Reference>(); 3794 for (Reference i : hasMember) 3795 dst.hasMember.add(i.copy()); 3796 }; 3797 if (derivedFrom != null) { 3798 dst.derivedFrom = new ArrayList<Reference>(); 3799 for (Reference i : derivedFrom) 3800 dst.derivedFrom.add(i.copy()); 3801 }; 3802 if (component != null) { 3803 dst.component = new ArrayList<ObservationComponentComponent>(); 3804 for (ObservationComponentComponent i : component) 3805 dst.component.add(i.copy()); 3806 }; 3807 } 3808 3809 protected Observation typedCopy() { 3810 return copy(); 3811 } 3812 3813 @Override 3814 public boolean equalsDeep(Base other_) { 3815 if (!super.equalsDeep(other_)) 3816 return false; 3817 if (!(other_ instanceof Observation)) 3818 return false; 3819 Observation o = (Observation) other_; 3820 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiates, o.instantiates, true) 3821 && compareDeep(basedOn, o.basedOn, true) && compareDeep(triggeredBy, o.triggeredBy, true) && compareDeep(partOf, o.partOf, true) 3822 && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 3823 && compareDeep(subject, o.subject, true) && compareDeep(focus, o.focus, true) && compareDeep(encounter, o.encounter, true) 3824 && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) 3825 && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 3826 && compareDeep(interpretation, o.interpretation, true) && compareDeep(note, o.note, true) && compareDeep(bodySite, o.bodySite, true) 3827 && compareDeep(bodyStructure, o.bodyStructure, true) && compareDeep(method, o.method, true) && compareDeep(specimen, o.specimen, true) 3828 && compareDeep(device, o.device, true) && compareDeep(referenceRange, o.referenceRange, true) && compareDeep(hasMember, o.hasMember, true) 3829 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(component, o.component, true); 3830 } 3831 3832 @Override 3833 public boolean equalsShallow(Base other_) { 3834 if (!super.equalsShallow(other_)) 3835 return false; 3836 if (!(other_ instanceof Observation)) 3837 return false; 3838 Observation o = (Observation) other_; 3839 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true); 3840 } 3841 3842 public boolean isEmpty() { 3843 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiates, basedOn 3844 , triggeredBy, partOf, status, category, code, subject, focus, encounter, effective 3845 , issued, performer, value, dataAbsentReason, interpretation, note, bodySite, bodyStructure 3846 , method, specimen, device, referenceRange, hasMember, derivedFrom, component 3847 ); 3848 } 3849 3850 @Override 3851 public ResourceType getResourceType() { 3852 return ResourceType.Observation; 3853 } 3854 3855 /** 3856 * Search parameter: <b>based-on</b> 3857 * <p> 3858 * Description: <b>Reference to the service request.</b><br> 3859 * Type: <b>reference</b><br> 3860 * Path: <b>Observation.basedOn</b><br> 3861 * </p> 3862 */ 3863 @SearchParamDefinition(name="based-on", path="Observation.basedOn", description="Reference to the service request.", type="reference", target={CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class } ) 3864 public static final String SP_BASED_ON = "based-on"; 3865 /** 3866 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3867 * <p> 3868 * Description: <b>Reference to the service request.</b><br> 3869 * Type: <b>reference</b><br> 3870 * Path: <b>Observation.basedOn</b><br> 3871 * </p> 3872 */ 3873 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3874 3875/** 3876 * Constant for fluent queries to be used to add include statements. Specifies 3877 * the path value of "<b>Observation:based-on</b>". 3878 */ 3879 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Observation:based-on").toLocked(); 3880 3881 /** 3882 * Search parameter: <b>category</b> 3883 * <p> 3884 * Description: <b>The classification of the type of observation</b><br> 3885 * Type: <b>token</b><br> 3886 * Path: <b>Observation.category</b><br> 3887 * </p> 3888 */ 3889 @SearchParamDefinition(name="category", path="Observation.category", description="The classification of the type of observation", type="token" ) 3890 public static final String SP_CATEGORY = "category"; 3891 /** 3892 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3893 * <p> 3894 * Description: <b>The classification of the type of observation</b><br> 3895 * Type: <b>token</b><br> 3896 * Path: <b>Observation.category</b><br> 3897 * </p> 3898 */ 3899 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3900 3901 /** 3902 * Search parameter: <b>code-value-concept</b> 3903 * <p> 3904 * Description: <b>Code and coded value parameter pair</b><br> 3905 * Type: <b>composite</b><br> 3906 * Path: <b>Observation</b><br> 3907 * </p> 3908 */ 3909 @SearchParamDefinition(name="code-value-concept", path="Observation", description="Code and coded value parameter pair", type="composite", compositeOf={"code", "value-concept"} ) 3910 public static final String SP_CODE_VALUE_CONCEPT = "code-value-concept"; 3911 /** 3912 * <b>Fluent Client</b> search parameter constant for <b>code-value-concept</b> 3913 * <p> 3914 * Description: <b>Code and coded value parameter pair</b><br> 3915 * Type: <b>composite</b><br> 3916 * Path: <b>Observation</b><br> 3917 * </p> 3918 */ 3919 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CODE_VALUE_CONCEPT); 3920 3921 /** 3922 * Search parameter: <b>code-value-date</b> 3923 * <p> 3924 * Description: <b>Code and date/time value parameter pair</b><br> 3925 * Type: <b>composite</b><br> 3926 * Path: <b>Observation</b><br> 3927 * </p> 3928 */ 3929 @SearchParamDefinition(name="code-value-date", path="Observation", description="Code and date/time value parameter pair", type="composite", compositeOf={"code", "value-date"} ) 3930 public static final String SP_CODE_VALUE_DATE = "code-value-date"; 3931 /** 3932 * <b>Fluent Client</b> search parameter constant for <b>code-value-date</b> 3933 * <p> 3934 * Description: <b>Code and date/time value parameter pair</b><br> 3935 * Type: <b>composite</b><br> 3936 * Path: <b>Observation</b><br> 3937 * </p> 3938 */ 3939 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam> CODE_VALUE_DATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam>(SP_CODE_VALUE_DATE); 3940 3941 /** 3942 * Search parameter: <b>code-value-quantity</b> 3943 * <p> 3944 * Description: <b>Code and quantity value parameter pair</b><br> 3945 * Type: <b>composite</b><br> 3946 * Path: <b>Observation</b><br> 3947 * </p> 3948 */ 3949 @SearchParamDefinition(name="code-value-quantity", path="Observation", description="Code and quantity value parameter pair", type="composite", compositeOf={"code", "value-quantity"} ) 3950 public static final String SP_CODE_VALUE_QUANTITY = "code-value-quantity"; 3951 /** 3952 * <b>Fluent Client</b> search parameter constant for <b>code-value-quantity</b> 3953 * <p> 3954 * Description: <b>Code and quantity value parameter pair</b><br> 3955 * Type: <b>composite</b><br> 3956 * Path: <b>Observation</b><br> 3957 * </p> 3958 */ 3959 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CODE_VALUE_QUANTITY); 3960 3961 /** 3962 * Search parameter: <b>combo-code-value-concept</b> 3963 * <p> 3964 * Description: <b>Code and coded value parameter pair, including in components</b><br> 3965 * Type: <b>composite</b><br> 3966 * Path: <b>Observation | Observation.component</b><br> 3967 * </p> 3968 */ 3969 @SearchParamDefinition(name="combo-code-value-concept", path="Observation | Observation.component", description="Code and coded value parameter pair, including in components", type="composite", compositeOf={"combo-code", "combo-value-concept"} ) 3970 public static final String SP_COMBO_CODE_VALUE_CONCEPT = "combo-code-value-concept"; 3971 /** 3972 * <b>Fluent Client</b> search parameter constant for <b>combo-code-value-concept</b> 3973 * <p> 3974 * Description: <b>Code and coded value parameter pair, including in components</b><br> 3975 * Type: <b>composite</b><br> 3976 * Path: <b>Observation | Observation.component</b><br> 3977 * </p> 3978 */ 3979 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMBO_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_COMBO_CODE_VALUE_CONCEPT); 3980 3981 /** 3982 * Search parameter: <b>combo-code-value-quantity</b> 3983 * <p> 3984 * Description: <b>Code and quantity value parameter pair, including in components</b><br> 3985 * Type: <b>composite</b><br> 3986 * Path: <b>Observation | Observation.component</b><br> 3987 * </p> 3988 */ 3989 @SearchParamDefinition(name="combo-code-value-quantity", path="Observation | Observation.component", description="Code and quantity value parameter pair, including in components", type="composite", compositeOf={"combo-code", "combo-value-quantity"} ) 3990 public static final String SP_COMBO_CODE_VALUE_QUANTITY = "combo-code-value-quantity"; 3991 /** 3992 * <b>Fluent Client</b> search parameter constant for <b>combo-code-value-quantity</b> 3993 * <p> 3994 * Description: <b>Code and quantity value parameter pair, including in components</b><br> 3995 * Type: <b>composite</b><br> 3996 * Path: <b>Observation | Observation.component</b><br> 3997 * </p> 3998 */ 3999 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMBO_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_COMBO_CODE_VALUE_QUANTITY); 4000 4001 /** 4002 * Search parameter: <b>combo-code</b> 4003 * <p> 4004 * Description: <b>The code of the observation type or component type</b><br> 4005 * Type: <b>token</b><br> 4006 * Path: <b>Observation.code | Observation.component.code</b><br> 4007 * </p> 4008 */ 4009 @SearchParamDefinition(name="combo-code", path="Observation.code | Observation.component.code", description="The code of the observation type or component type", type="token" ) 4010 public static final String SP_COMBO_CODE = "combo-code"; 4011 /** 4012 * <b>Fluent Client</b> search parameter constant for <b>combo-code</b> 4013 * <p> 4014 * Description: <b>The code of the observation type or component type</b><br> 4015 * Type: <b>token</b><br> 4016 * Path: <b>Observation.code | Observation.component.code</b><br> 4017 * </p> 4018 */ 4019 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_CODE); 4020 4021 /** 4022 * Search parameter: <b>combo-data-absent-reason</b> 4023 * <p> 4024 * Description: <b>The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4025 * Type: <b>token</b><br> 4026 * Path: <b>Observation.dataAbsentReason | Observation.component.dataAbsentReason</b><br> 4027 * </p> 4028 */ 4029 @SearchParamDefinition(name="combo-data-absent-reason", path="Observation.dataAbsentReason | Observation.component.dataAbsentReason", description="The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.", type="token" ) 4030 public static final String SP_COMBO_DATA_ABSENT_REASON = "combo-data-absent-reason"; 4031 /** 4032 * <b>Fluent Client</b> search parameter constant for <b>combo-data-absent-reason</b> 4033 * <p> 4034 * Description: <b>The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4035 * Type: <b>token</b><br> 4036 * Path: <b>Observation.dataAbsentReason | Observation.component.dataAbsentReason</b><br> 4037 * </p> 4038 */ 4039 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_DATA_ABSENT_REASON); 4040 4041 /** 4042 * Search parameter: <b>combo-value-concept</b> 4043 * <p> 4044 * Description: <b>The value or component value of the observation, if the value is a CodeableConcept</b><br> 4045 * Type: <b>token</b><br> 4046 * Path: <b>Observation.value.ofType(CodeableConcept) | Observation.component.value.ofType(CodeableConcept)</b><br> 4047 * </p> 4048 */ 4049 @SearchParamDefinition(name="combo-value-concept", path="Observation.value.ofType(CodeableConcept) | Observation.component.value.ofType(CodeableConcept)", description="The value or component value of the observation, if the value is a CodeableConcept", type="token" ) 4050 public static final String SP_COMBO_VALUE_CONCEPT = "combo-value-concept"; 4051 /** 4052 * <b>Fluent Client</b> search parameter constant for <b>combo-value-concept</b> 4053 * <p> 4054 * Description: <b>The value or component value of the observation, if the value is a CodeableConcept</b><br> 4055 * Type: <b>token</b><br> 4056 * Path: <b>Observation.value.ofType(CodeableConcept) | Observation.component.value.ofType(CodeableConcept)</b><br> 4057 * </p> 4058 */ 4059 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_VALUE_CONCEPT); 4060 4061 /** 4062 * Search parameter: <b>combo-value-quantity</b> 4063 * <p> 4064 * Description: <b>The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4065 * Type: <b>quantity</b><br> 4066 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData) | Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4067 * </p> 4068 */ 4069 @SearchParamDefinition(name="combo-value-quantity", path="Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData) | Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)", description="The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 4070 public static final String SP_COMBO_VALUE_QUANTITY = "combo-value-quantity"; 4071 /** 4072 * <b>Fluent Client</b> search parameter constant for <b>combo-value-quantity</b> 4073 * <p> 4074 * Description: <b>The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4075 * Type: <b>quantity</b><br> 4076 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData) | Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4077 * </p> 4078 */ 4079 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMBO_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_COMBO_VALUE_QUANTITY); 4080 4081 /** 4082 * Search parameter: <b>component-code-value-concept</b> 4083 * <p> 4084 * Description: <b>Component code and component coded value parameter pair</b><br> 4085 * Type: <b>composite</b><br> 4086 * Path: <b>Observation.component</b><br> 4087 * </p> 4088 */ 4089 @SearchParamDefinition(name="component-code-value-concept", path="Observation.component", description="Component code and component coded value parameter pair", type="composite", compositeOf={"component-code", "component-value-concept"} ) 4090 public static final String SP_COMPONENT_CODE_VALUE_CONCEPT = "component-code-value-concept"; 4091 /** 4092 * <b>Fluent Client</b> search parameter constant for <b>component-code-value-concept</b> 4093 * <p> 4094 * Description: <b>Component code and component coded value parameter pair</b><br> 4095 * Type: <b>composite</b><br> 4096 * Path: <b>Observation.component</b><br> 4097 * </p> 4098 */ 4099 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMPONENT_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_COMPONENT_CODE_VALUE_CONCEPT); 4100 4101 /** 4102 * Search parameter: <b>component-code-value-quantity</b> 4103 * <p> 4104 * Description: <b>Component code and component quantity value parameter pair</b><br> 4105 * Type: <b>composite</b><br> 4106 * Path: <b>Observation.component</b><br> 4107 * </p> 4108 */ 4109 @SearchParamDefinition(name="component-code-value-quantity", path="Observation.component", description="Component code and component quantity value parameter pair", type="composite", compositeOf={"component-code", "component-value-quantity"} ) 4110 public static final String SP_COMPONENT_CODE_VALUE_QUANTITY = "component-code-value-quantity"; 4111 /** 4112 * <b>Fluent Client</b> search parameter constant for <b>component-code-value-quantity</b> 4113 * <p> 4114 * Description: <b>Component code and component quantity value parameter pair</b><br> 4115 * Type: <b>composite</b><br> 4116 * Path: <b>Observation.component</b><br> 4117 * </p> 4118 */ 4119 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMPONENT_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_COMPONENT_CODE_VALUE_QUANTITY); 4120 4121 /** 4122 * Search parameter: <b>component-code</b> 4123 * <p> 4124 * Description: <b>The component code of the observation type</b><br> 4125 * Type: <b>token</b><br> 4126 * Path: <b>Observation.component.code</b><br> 4127 * </p> 4128 */ 4129 @SearchParamDefinition(name="component-code", path="Observation.component.code", description="The component code of the observation type", type="token" ) 4130 public static final String SP_COMPONENT_CODE = "component-code"; 4131 /** 4132 * <b>Fluent Client</b> search parameter constant for <b>component-code</b> 4133 * <p> 4134 * Description: <b>The component code of the observation type</b><br> 4135 * Type: <b>token</b><br> 4136 * Path: <b>Observation.component.code</b><br> 4137 * </p> 4138 */ 4139 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_CODE); 4140 4141 /** 4142 * Search parameter: <b>component-data-absent-reason</b> 4143 * <p> 4144 * Description: <b>The reason why the expected value in the element Observation.component.value[x] is missing.</b><br> 4145 * Type: <b>token</b><br> 4146 * Path: <b>Observation.component.dataAbsentReason</b><br> 4147 * </p> 4148 */ 4149 @SearchParamDefinition(name="component-data-absent-reason", path="Observation.component.dataAbsentReason", description="The reason why the expected value in the element Observation.component.value[x] is missing.", type="token" ) 4150 public static final String SP_COMPONENT_DATA_ABSENT_REASON = "component-data-absent-reason"; 4151 /** 4152 * <b>Fluent Client</b> search parameter constant for <b>component-data-absent-reason</b> 4153 * <p> 4154 * Description: <b>The reason why the expected value in the element Observation.component.value[x] is missing.</b><br> 4155 * Type: <b>token</b><br> 4156 * Path: <b>Observation.component.dataAbsentReason</b><br> 4157 * </p> 4158 */ 4159 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_DATA_ABSENT_REASON); 4160 4161 /** 4162 * Search parameter: <b>component-value-canonical</b> 4163 * <p> 4164 * Description: <b>URL contained in valueCanonical.</b><br> 4165 * Type: <b>uri</b><br> 4166 * Path: <b>Observation.component.value.ofType(canonical)</b><br> 4167 * </p> 4168 */ 4169 @SearchParamDefinition(name="component-value-canonical", path="Observation.component.value.ofType(canonical)", description="URL contained in valueCanonical.", type="uri" ) 4170 public static final String SP_COMPONENT_VALUE_CANONICAL = "component-value-canonical"; 4171 /** 4172 * <b>Fluent Client</b> search parameter constant for <b>component-value-canonical</b> 4173 * <p> 4174 * Description: <b>URL contained in valueCanonical.</b><br> 4175 * Type: <b>uri</b><br> 4176 * Path: <b>Observation.component.value.ofType(canonical)</b><br> 4177 * </p> 4178 */ 4179 public static final ca.uhn.fhir.rest.gclient.UriClientParam COMPONENT_VALUE_CANONICAL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_COMPONENT_VALUE_CANONICAL); 4180 4181 /** 4182 * Search parameter: <b>component-value-concept</b> 4183 * <p> 4184 * Description: <b>The value of the component observation, if the value is a CodeableConcept</b><br> 4185 * Type: <b>token</b><br> 4186 * Path: <b>Observation.component.value.ofType(CodeableConcept)</b><br> 4187 * </p> 4188 */ 4189 @SearchParamDefinition(name="component-value-concept", path="Observation.component.value.ofType(CodeableConcept)", description="The value of the component observation, if the value is a CodeableConcept", type="token" ) 4190 public static final String SP_COMPONENT_VALUE_CONCEPT = "component-value-concept"; 4191 /** 4192 * <b>Fluent Client</b> search parameter constant for <b>component-value-concept</b> 4193 * <p> 4194 * Description: <b>The value of the component observation, if the value is a CodeableConcept</b><br> 4195 * Type: <b>token</b><br> 4196 * Path: <b>Observation.component.value.ofType(CodeableConcept)</b><br> 4197 * </p> 4198 */ 4199 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_VALUE_CONCEPT); 4200 4201 /** 4202 * Search parameter: <b>component-value-quantity</b> 4203 * <p> 4204 * Description: <b>The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4205 * Type: <b>quantity</b><br> 4206 * Path: <b>Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4207 * </p> 4208 */ 4209 @SearchParamDefinition(name="component-value-quantity", path="Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)", description="The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 4210 public static final String SP_COMPONENT_VALUE_QUANTITY = "component-value-quantity"; 4211 /** 4212 * <b>Fluent Client</b> search parameter constant for <b>component-value-quantity</b> 4213 * <p> 4214 * Description: <b>The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4215 * Type: <b>quantity</b><br> 4216 * Path: <b>Observation.component.value.ofType(Quantity) | Observation.component.value.ofType(SampledData)</b><br> 4217 * </p> 4218 */ 4219 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMPONENT_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_COMPONENT_VALUE_QUANTITY); 4220 4221 /** 4222 * Search parameter: <b>component-value-reference</b> 4223 * <p> 4224 * Description: <b>Reference contained in valueReference.</b><br> 4225 * Type: <b>reference</b><br> 4226 * Path: <b>Observation.component.value.ofType(Reference)</b><br> 4227 * </p> 4228 */ 4229 @SearchParamDefinition(name="component-value-reference", path="Observation.component.value.ofType(Reference)", description="Reference contained in valueReference.", type="reference", target={MolecularSequence.class } ) 4230 public static final String SP_COMPONENT_VALUE_REFERENCE = "component-value-reference"; 4231 /** 4232 * <b>Fluent Client</b> search parameter constant for <b>component-value-reference</b> 4233 * <p> 4234 * Description: <b>Reference contained in valueReference.</b><br> 4235 * Type: <b>reference</b><br> 4236 * Path: <b>Observation.component.value.ofType(Reference)</b><br> 4237 * </p> 4238 */ 4239 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT_VALUE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPONENT_VALUE_REFERENCE); 4240 4241/** 4242 * Constant for fluent queries to be used to add include statements. Specifies 4243 * the path value of "<b>Observation:component-value-reference</b>". 4244 */ 4245 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT_VALUE_REFERENCE = new ca.uhn.fhir.model.api.Include("Observation:component-value-reference").toLocked(); 4246 4247 /** 4248 * Search parameter: <b>data-absent-reason</b> 4249 * <p> 4250 * Description: <b>The reason why the expected value in the element Observation.value[x] is missing.</b><br> 4251 * Type: <b>token</b><br> 4252 * Path: <b>Observation.dataAbsentReason</b><br> 4253 * </p> 4254 */ 4255 @SearchParamDefinition(name="data-absent-reason", path="Observation.dataAbsentReason", description="The reason why the expected value in the element Observation.value[x] is missing.", type="token" ) 4256 public static final String SP_DATA_ABSENT_REASON = "data-absent-reason"; 4257 /** 4258 * <b>Fluent Client</b> search parameter constant for <b>data-absent-reason</b> 4259 * <p> 4260 * Description: <b>The reason why the expected value in the element Observation.value[x] is missing.</b><br> 4261 * Type: <b>token</b><br> 4262 * Path: <b>Observation.dataAbsentReason</b><br> 4263 * </p> 4264 */ 4265 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DATA_ABSENT_REASON); 4266 4267 /** 4268 * Search parameter: <b>derived-from</b> 4269 * <p> 4270 * Description: <b>Related measurements the observation is made from</b><br> 4271 * Type: <b>reference</b><br> 4272 * Path: <b>Observation.derivedFrom</b><br> 4273 * </p> 4274 */ 4275 @SearchParamDefinition(name="derived-from", path="Observation.derivedFrom", description="Related measurements the observation is made from", type="reference", target={DocumentReference.class, GenomicStudy.class, ImagingSelection.class, ImagingStudy.class, MolecularSequence.class, Observation.class, QuestionnaireResponse.class } ) 4276 public static final String SP_DERIVED_FROM = "derived-from"; 4277 /** 4278 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4279 * <p> 4280 * Description: <b>Related measurements the observation is made from</b><br> 4281 * Type: <b>reference</b><br> 4282 * Path: <b>Observation.derivedFrom</b><br> 4283 * </p> 4284 */ 4285 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4286 4287/** 4288 * Constant for fluent queries to be used to add include statements. Specifies 4289 * the path value of "<b>Observation:derived-from</b>". 4290 */ 4291 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Observation:derived-from").toLocked(); 4292 4293 /** 4294 * Search parameter: <b>device</b> 4295 * <p> 4296 * Description: <b>The Device that generated the observation data.</b><br> 4297 * Type: <b>reference</b><br> 4298 * Path: <b>Observation.device</b><br> 4299 * </p> 4300 */ 4301 @SearchParamDefinition(name="device", path="Observation.device", description="The Device that generated the observation data.", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class, DeviceMetric.class } ) 4302 public static final String SP_DEVICE = "device"; 4303 /** 4304 * <b>Fluent Client</b> search parameter constant for <b>device</b> 4305 * <p> 4306 * Description: <b>The Device that generated the observation data.</b><br> 4307 * Type: <b>reference</b><br> 4308 * Path: <b>Observation.device</b><br> 4309 * </p> 4310 */ 4311 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 4312 4313/** 4314 * Constant for fluent queries to be used to add include statements. Specifies 4315 * the path value of "<b>Observation:device</b>". 4316 */ 4317 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("Observation:device").toLocked(); 4318 4319 /** 4320 * Search parameter: <b>focus</b> 4321 * <p> 4322 * Description: <b>The focus of an observation when the focus is not the patient of record.</b><br> 4323 * Type: <b>reference</b><br> 4324 * Path: <b>Observation.focus</b><br> 4325 * </p> 4326 */ 4327 @SearchParamDefinition(name="focus", path="Observation.focus", description="The focus of an observation when the focus is not the patient of record.", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4328 public static final String SP_FOCUS = "focus"; 4329 /** 4330 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 4331 * <p> 4332 * Description: <b>The focus of an observation when the focus is not the patient of record.</b><br> 4333 * Type: <b>reference</b><br> 4334 * Path: <b>Observation.focus</b><br> 4335 * </p> 4336 */ 4337 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 4338 4339/** 4340 * Constant for fluent queries to be used to add include statements. Specifies 4341 * the path value of "<b>Observation:focus</b>". 4342 */ 4343 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("Observation:focus").toLocked(); 4344 4345 /** 4346 * Search parameter: <b>has-member</b> 4347 * <p> 4348 * Description: <b>Related resource that belongs to the Observation group</b><br> 4349 * Type: <b>reference</b><br> 4350 * Path: <b>Observation.hasMember</b><br> 4351 * </p> 4352 */ 4353 @SearchParamDefinition(name="has-member", path="Observation.hasMember", description="Related resource that belongs to the Observation group", type="reference", target={MolecularSequence.class, Observation.class, QuestionnaireResponse.class } ) 4354 public static final String SP_HAS_MEMBER = "has-member"; 4355 /** 4356 * <b>Fluent Client</b> search parameter constant for <b>has-member</b> 4357 * <p> 4358 * Description: <b>Related resource that belongs to the Observation group</b><br> 4359 * Type: <b>reference</b><br> 4360 * Path: <b>Observation.hasMember</b><br> 4361 * </p> 4362 */ 4363 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam HAS_MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_HAS_MEMBER); 4364 4365/** 4366 * Constant for fluent queries to be used to add include statements. Specifies 4367 * the path value of "<b>Observation:has-member</b>". 4368 */ 4369 public static final ca.uhn.fhir.model.api.Include INCLUDE_HAS_MEMBER = new ca.uhn.fhir.model.api.Include("Observation:has-member").toLocked(); 4370 4371 /** 4372 * Search parameter: <b>method</b> 4373 * <p> 4374 * Description: <b>The method used for the observation</b><br> 4375 * Type: <b>token</b><br> 4376 * Path: <b>Observation.method</b><br> 4377 * </p> 4378 */ 4379 @SearchParamDefinition(name="method", path="Observation.method", description="The method used for the observation", type="token" ) 4380 public static final String SP_METHOD = "method"; 4381 /** 4382 * <b>Fluent Client</b> search parameter constant for <b>method</b> 4383 * <p> 4384 * Description: <b>The method used for the observation</b><br> 4385 * Type: <b>token</b><br> 4386 * Path: <b>Observation.method</b><br> 4387 * </p> 4388 */ 4389 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_METHOD); 4390 4391 /** 4392 * Search parameter: <b>part-of</b> 4393 * <p> 4394 * Description: <b>Part of referenced event</b><br> 4395 * Type: <b>reference</b><br> 4396 * Path: <b>Observation.partOf</b><br> 4397 * </p> 4398 */ 4399 @SearchParamDefinition(name="part-of", path="Observation.partOf", description="Part of referenced event", type="reference", target={GenomicStudy.class, ImagingStudy.class, Immunization.class, MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Procedure.class } ) 4400 public static final String SP_PART_OF = "part-of"; 4401 /** 4402 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4403 * <p> 4404 * Description: <b>Part of referenced event</b><br> 4405 * Type: <b>reference</b><br> 4406 * Path: <b>Observation.partOf</b><br> 4407 * </p> 4408 */ 4409 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 4410 4411/** 4412 * Constant for fluent queries to be used to add include statements. Specifies 4413 * the path value of "<b>Observation:part-of</b>". 4414 */ 4415 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Observation:part-of").toLocked(); 4416 4417 /** 4418 * Search parameter: <b>performer</b> 4419 * <p> 4420 * Description: <b>Who performed the observation</b><br> 4421 * Type: <b>reference</b><br> 4422 * Path: <b>Observation.performer</b><br> 4423 * </p> 4424 */ 4425 @SearchParamDefinition(name="performer", path="Observation.performer", description="Who performed the observation", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 4426 public static final String SP_PERFORMER = "performer"; 4427 /** 4428 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4429 * <p> 4430 * Description: <b>Who performed the observation</b><br> 4431 * Type: <b>reference</b><br> 4432 * Path: <b>Observation.performer</b><br> 4433 * </p> 4434 */ 4435 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 4436 4437/** 4438 * Constant for fluent queries to be used to add include statements. Specifies 4439 * the path value of "<b>Observation:performer</b>". 4440 */ 4441 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("Observation:performer").toLocked(); 4442 4443 /** 4444 * Search parameter: <b>specimen</b> 4445 * <p> 4446 * Description: <b>Specimen used for this observation</b><br> 4447 * Type: <b>reference</b><br> 4448 * Path: <b>Observation.specimen</b><br> 4449 * </p> 4450 */ 4451 @SearchParamDefinition(name="specimen", path="Observation.specimen", description="Specimen used for this observation", type="reference", target={Group.class, Specimen.class } ) 4452 public static final String SP_SPECIMEN = "specimen"; 4453 /** 4454 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 4455 * <p> 4456 * Description: <b>Specimen used for this observation</b><br> 4457 * Type: <b>reference</b><br> 4458 * Path: <b>Observation.specimen</b><br> 4459 * </p> 4460 */ 4461 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 4462 4463/** 4464 * Constant for fluent queries to be used to add include statements. Specifies 4465 * the path value of "<b>Observation:specimen</b>". 4466 */ 4467 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("Observation:specimen").toLocked(); 4468 4469 /** 4470 * Search parameter: <b>status</b> 4471 * <p> 4472 * Description: <b>The status of the observation</b><br> 4473 * Type: <b>token</b><br> 4474 * Path: <b>Observation.status</b><br> 4475 * </p> 4476 */ 4477 @SearchParamDefinition(name="status", path="Observation.status", description="The status of the observation", type="token" ) 4478 public static final String SP_STATUS = "status"; 4479 /** 4480 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4481 * <p> 4482 * Description: <b>The status of the observation</b><br> 4483 * Type: <b>token</b><br> 4484 * Path: <b>Observation.status</b><br> 4485 * </p> 4486 */ 4487 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4488 4489 /** 4490 * Search parameter: <b>subject</b> 4491 * <p> 4492 * Description: <b>The subject that the observation is about</b><br> 4493 * Type: <b>reference</b><br> 4494 * Path: <b>Observation.subject</b><br> 4495 * </p> 4496 */ 4497 @SearchParamDefinition(name="subject", path="Observation.subject", description="The subject that the observation is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={BiologicallyDerivedProduct.class, Device.class, Group.class, Location.class, Medication.class, NutritionProduct.class, Organization.class, Patient.class, Practitioner.class, Procedure.class, Substance.class } ) 4498 public static final String SP_SUBJECT = "subject"; 4499 /** 4500 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4501 * <p> 4502 * Description: <b>The subject that the observation is about</b><br> 4503 * Type: <b>reference</b><br> 4504 * Path: <b>Observation.subject</b><br> 4505 * </p> 4506 */ 4507 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4508 4509/** 4510 * Constant for fluent queries to be used to add include statements. Specifies 4511 * the path value of "<b>Observation:subject</b>". 4512 */ 4513 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Observation:subject").toLocked(); 4514 4515 /** 4516 * Search parameter: <b>value-canonical</b> 4517 * <p> 4518 * Description: <b>URL contained in valueCanonical.</b><br> 4519 * Type: <b>uri</b><br> 4520 * Path: <b>Observation.value.ofType(canonical)</b><br> 4521 * </p> 4522 */ 4523 @SearchParamDefinition(name="value-canonical", path="Observation.value.ofType(canonical)", description="URL contained in valueCanonical.", type="uri" ) 4524 public static final String SP_VALUE_CANONICAL = "value-canonical"; 4525 /** 4526 * <b>Fluent Client</b> search parameter constant for <b>value-canonical</b> 4527 * <p> 4528 * Description: <b>URL contained in valueCanonical.</b><br> 4529 * Type: <b>uri</b><br> 4530 * Path: <b>Observation.value.ofType(canonical)</b><br> 4531 * </p> 4532 */ 4533 public static final ca.uhn.fhir.rest.gclient.UriClientParam VALUE_CANONICAL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_VALUE_CANONICAL); 4534 4535 /** 4536 * Search parameter: <b>value-concept</b> 4537 * <p> 4538 * Description: <b>The value of the observation, if the value is a CodeableConcept</b><br> 4539 * Type: <b>token</b><br> 4540 * Path: <b>Observation.value.ofType(CodeableConcept)</b><br> 4541 * </p> 4542 */ 4543 @SearchParamDefinition(name="value-concept", path="Observation.value.ofType(CodeableConcept)", description="The value of the observation, if the value is a CodeableConcept", type="token" ) 4544 public static final String SP_VALUE_CONCEPT = "value-concept"; 4545 /** 4546 * <b>Fluent Client</b> search parameter constant for <b>value-concept</b> 4547 * <p> 4548 * Description: <b>The value of the observation, if the value is a CodeableConcept</b><br> 4549 * Type: <b>token</b><br> 4550 * Path: <b>Observation.value.ofType(CodeableConcept)</b><br> 4551 * </p> 4552 */ 4553 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VALUE_CONCEPT); 4554 4555 /** 4556 * Search parameter: <b>value-date</b> 4557 * <p> 4558 * Description: <b>The value of the observation, if the value is a date or period of time</b><br> 4559 * Type: <b>date</b><br> 4560 * Path: <b>Observation.value.ofType(dateTime) | Observation.value.ofType(Period)</b><br> 4561 * </p> 4562 */ 4563 @SearchParamDefinition(name="value-date", path="Observation.value.ofType(dateTime) | Observation.value.ofType(Period)", description="The value of the observation, if the value is a date or period of time", type="date" ) 4564 public static final String SP_VALUE_DATE = "value-date"; 4565 /** 4566 * <b>Fluent Client</b> search parameter constant for <b>value-date</b> 4567 * <p> 4568 * Description: <b>The value of the observation, if the value is a date or period of time</b><br> 4569 * Type: <b>date</b><br> 4570 * Path: <b>Observation.value.ofType(dateTime) | Observation.value.ofType(Period)</b><br> 4571 * </p> 4572 */ 4573 public static final ca.uhn.fhir.rest.gclient.DateClientParam VALUE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_VALUE_DATE); 4574 4575 /** 4576 * Search parameter: <b>value-markdown</b> 4577 * <p> 4578 * Description: <b>The value of the observation, if the value is a string, and also searches in CodeableConcept.text</b><br> 4579 * Type: <b>string</b><br> 4580 * Path: <b>Observation.value.ofType(markdown) | Observation.value.ofType(CodeableConcept).text</b><br> 4581 * </p> 4582 */ 4583 @SearchParamDefinition(name="value-markdown", path="Observation.value.ofType(markdown) | Observation.value.ofType(CodeableConcept).text", description="The value of the observation, if the value is a string, and also searches in CodeableConcept.text", type="string" ) 4584 public static final String SP_VALUE_MARKDOWN = "value-markdown"; 4585 /** 4586 * <b>Fluent Client</b> search parameter constant for <b>value-markdown</b> 4587 * <p> 4588 * Description: <b>The value of the observation, if the value is a string, and also searches in CodeableConcept.text</b><br> 4589 * Type: <b>string</b><br> 4590 * Path: <b>Observation.value.ofType(markdown) | Observation.value.ofType(CodeableConcept).text</b><br> 4591 * </p> 4592 */ 4593 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE_MARKDOWN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VALUE_MARKDOWN); 4594 4595 /** 4596 * Search parameter: <b>value-quantity</b> 4597 * <p> 4598 * Description: <b>The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4599 * Type: <b>quantity</b><br> 4600 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData)</b><br> 4601 * </p> 4602 */ 4603 @SearchParamDefinition(name="value-quantity", path="Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData)", description="The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 4604 public static final String SP_VALUE_QUANTITY = "value-quantity"; 4605 /** 4606 * <b>Fluent Client</b> search parameter constant for <b>value-quantity</b> 4607 * <p> 4608 * Description: <b>The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4609 * Type: <b>quantity</b><br> 4610 * Path: <b>Observation.value.ofType(Quantity) | Observation.value.ofType(SampledData)</b><br> 4611 * </p> 4612 */ 4613 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_VALUE_QUANTITY); 4614 4615 /** 4616 * Search parameter: <b>value-reference</b> 4617 * <p> 4618 * Description: <b>Reference contained in valueReference.</b><br> 4619 * Type: <b>reference</b><br> 4620 * Path: <b>Observation.value.ofType(Reference)</b><br> 4621 * </p> 4622 */ 4623 @SearchParamDefinition(name="value-reference", path="Observation.value.ofType(Reference)", description="Reference contained in valueReference.", type="reference", target={MolecularSequence.class } ) 4624 public static final String SP_VALUE_REFERENCE = "value-reference"; 4625 /** 4626 * <b>Fluent Client</b> search parameter constant for <b>value-reference</b> 4627 * <p> 4628 * Description: <b>Reference contained in valueReference.</b><br> 4629 * Type: <b>reference</b><br> 4630 * Path: <b>Observation.value.ofType(Reference)</b><br> 4631 * </p> 4632 */ 4633 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam VALUE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_VALUE_REFERENCE); 4634 4635/** 4636 * Constant for fluent queries to be used to add include statements. Specifies 4637 * the path value of "<b>Observation:value-reference</b>". 4638 */ 4639 public static final ca.uhn.fhir.model.api.Include INCLUDE_VALUE_REFERENCE = new ca.uhn.fhir.model.api.Include("Observation:value-reference").toLocked(); 4640 4641 /** 4642 * Search parameter: <b>code</b> 4643 * <p> 4644 * Description: <b>Multiple Resources: 4645 4646* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4647* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4648* [AuditEvent](auditevent.html): More specific code for the event 4649* [Basic](basic.html): Kind of Resource 4650* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4651* [Condition](condition.html): Code for the condition 4652* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4653* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4654* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4655* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4656* [ImagingSelection](imagingselection.html): The imaging selection status 4657* [List](list.html): What the purpose of this list is 4658* [Medication](medication.html): Returns medications for a specific code 4659* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4660* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4661* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4662* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4663* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4664* [Observation](observation.html): The code of the observation type 4665* [Procedure](procedure.html): A code to identify a procedure 4666* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4667* [Task](task.html): Search by task code 4668</b><br> 4669 * Type: <b>token</b><br> 4670 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4671 * </p> 4672 */ 4673 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 4674 public static final String SP_CODE = "code"; 4675 /** 4676 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4677 * <p> 4678 * Description: <b>Multiple Resources: 4679 4680* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 4681* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 4682* [AuditEvent](auditevent.html): More specific code for the event 4683* [Basic](basic.html): Kind of Resource 4684* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 4685* [Condition](condition.html): Code for the condition 4686* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 4687* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 4688* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 4689* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 4690* [ImagingSelection](imagingselection.html): The imaging selection status 4691* [List](list.html): What the purpose of this list is 4692* [Medication](medication.html): Returns medications for a specific code 4693* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4694* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4695* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4696* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4697* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4698* [Observation](observation.html): The code of the observation type 4699* [Procedure](procedure.html): A code to identify a procedure 4700* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4701* [Task](task.html): Search by task code 4702</b><br> 4703 * Type: <b>token</b><br> 4704 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4705 * </p> 4706 */ 4707 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4708 4709 /** 4710 * Search parameter: <b>date</b> 4711 * <p> 4712 * Description: <b>Multiple Resources: 4713 4714* [AdverseEvent](adverseevent.html): When the event occurred 4715* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4716* [Appointment](appointment.html): Appointment date/time. 4717* [AuditEvent](auditevent.html): Time when the event was recorded 4718* [CarePlan](careplan.html): Time period plan covers 4719* [CareTeam](careteam.html): A date within the coverage time period. 4720* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4721* [Composition](composition.html): Composition editing time 4722* [Consent](consent.html): When consent was agreed to 4723* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4724* [DocumentReference](documentreference.html): When this document reference was created 4725* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4726* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4727* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4728* [Flag](flag.html): Time period when flag is active 4729* [Immunization](immunization.html): Vaccination (non)-Administration Date 4730* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4731* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4732* [Invoice](invoice.html): Invoice date / posting date 4733* [List](list.html): When the list was prepared 4734* [MeasureReport](measurereport.html): The date of the measure report 4735* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4736* [Observation](observation.html): Clinically relevant time/time-period for observation 4737* [Procedure](procedure.html): When the procedure occurred or is occurring 4738* [ResearchSubject](researchsubject.html): Start and end of participation 4739* [RiskAssessment](riskassessment.html): When was assessment made? 4740* [SupplyRequest](supplyrequest.html): When the request was made 4741</b><br> 4742 * Type: <b>date</b><br> 4743 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4744 * </p> 4745 */ 4746 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4747 public static final String SP_DATE = "date"; 4748 /** 4749 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4750 * <p> 4751 * Description: <b>Multiple Resources: 4752 4753* [AdverseEvent](adverseevent.html): When the event occurred 4754* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4755* [Appointment](appointment.html): Appointment date/time. 4756* [AuditEvent](auditevent.html): Time when the event was recorded 4757* [CarePlan](careplan.html): Time period plan covers 4758* [CareTeam](careteam.html): A date within the coverage time period. 4759* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4760* [Composition](composition.html): Composition editing time 4761* [Consent](consent.html): When consent was agreed to 4762* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4763* [DocumentReference](documentreference.html): When this document reference was created 4764* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4765* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4766* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4767* [Flag](flag.html): Time period when flag is active 4768* [Immunization](immunization.html): Vaccination (non)-Administration Date 4769* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4770* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4771* [Invoice](invoice.html): Invoice date / posting date 4772* [List](list.html): When the list was prepared 4773* [MeasureReport](measurereport.html): The date of the measure report 4774* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4775* [Observation](observation.html): Clinically relevant time/time-period for observation 4776* [Procedure](procedure.html): When the procedure occurred or is occurring 4777* [ResearchSubject](researchsubject.html): Start and end of participation 4778* [RiskAssessment](riskassessment.html): When was assessment made? 4779* [SupplyRequest](supplyrequest.html): When the request was made 4780</b><br> 4781 * Type: <b>date</b><br> 4782 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4783 * </p> 4784 */ 4785 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4786 4787 /** 4788 * Search parameter: <b>encounter</b> 4789 * <p> 4790 * Description: <b>Multiple Resources: 4791 4792* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 4793* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 4794* [ChargeItem](chargeitem.html): Encounter associated with event 4795* [Claim](claim.html): Encounters associated with a billed line item 4796* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 4797* [Communication](communication.html): The Encounter during which this Communication was created 4798* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 4799* [Composition](composition.html): Context of the Composition 4800* [Condition](condition.html): The Encounter during which this Condition was created 4801* [DeviceRequest](devicerequest.html): Encounter during which request was created 4802* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 4803* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 4804* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 4805* [Flag](flag.html): Alert relevant during encounter 4806* [ImagingStudy](imagingstudy.html): The context of the study 4807* [List](list.html): Context in which list created 4808* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 4809* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 4810* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 4811* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 4812* [Observation](observation.html): Encounter related to the observation 4813* [Procedure](procedure.html): The Encounter during which this Procedure was created 4814* [Provenance](provenance.html): Encounter related to the Provenance 4815* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 4816* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 4817* [RiskAssessment](riskassessment.html): Where was assessment performed? 4818* [ServiceRequest](servicerequest.html): An encounter in which this request is made 4819* [Task](task.html): Search by encounter 4820* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 4821</b><br> 4822 * Type: <b>reference</b><br> 4823 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 4824 * </p> 4825 */ 4826 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 4827 public static final String SP_ENCOUNTER = "encounter"; 4828 /** 4829 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4830 * <p> 4831 * Description: <b>Multiple Resources: 4832 4833* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 4834* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 4835* [ChargeItem](chargeitem.html): Encounter associated with event 4836* [Claim](claim.html): Encounters associated with a billed line item 4837* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 4838* [Communication](communication.html): The Encounter during which this Communication was created 4839* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 4840* [Composition](composition.html): Context of the Composition 4841* [Condition](condition.html): The Encounter during which this Condition was created 4842* [DeviceRequest](devicerequest.html): Encounter during which request was created 4843* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 4844* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 4845* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 4846* [Flag](flag.html): Alert relevant during encounter 4847* [ImagingStudy](imagingstudy.html): The context of the study 4848* [List](list.html): Context in which list created 4849* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 4850* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 4851* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 4852* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 4853* [Observation](observation.html): Encounter related to the observation 4854* [Procedure](procedure.html): The Encounter during which this Procedure was created 4855* [Provenance](provenance.html): Encounter related to the Provenance 4856* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 4857* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 4858* [RiskAssessment](riskassessment.html): Where was assessment performed? 4859* [ServiceRequest](servicerequest.html): An encounter in which this request is made 4860* [Task](task.html): Search by encounter 4861* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 4862</b><br> 4863 * Type: <b>reference</b><br> 4864 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 4865 * </p> 4866 */ 4867 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4868 4869/** 4870 * Constant for fluent queries to be used to add include statements. Specifies 4871 * the path value of "<b>Observation:encounter</b>". 4872 */ 4873 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Observation:encounter").toLocked(); 4874 4875 /** 4876 * Search parameter: <b>identifier</b> 4877 * <p> 4878 * Description: <b>Multiple Resources: 4879 4880* [Account](account.html): Account number 4881* [AdverseEvent](adverseevent.html): Business identifier for the event 4882* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4883* [Appointment](appointment.html): An Identifier of the Appointment 4884* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4885* [Basic](basic.html): Business identifier 4886* [BodyStructure](bodystructure.html): Bodystructure identifier 4887* [CarePlan](careplan.html): External Ids for this plan 4888* [CareTeam](careteam.html): External Ids for this team 4889* [ChargeItem](chargeitem.html): Business Identifier for item 4890* [Claim](claim.html): The primary identifier of the financial resource 4891* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4892* [ClinicalImpression](clinicalimpression.html): Business identifier 4893* [Communication](communication.html): Unique identifier 4894* [CommunicationRequest](communicationrequest.html): Unique identifier 4895* [Composition](composition.html): Version-independent identifier for the Composition 4896* [Condition](condition.html): A unique identifier of the condition record 4897* [Consent](consent.html): Identifier for this record (external references) 4898* [Contract](contract.html): The identity of the contract 4899* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4900* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4901* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4902* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4903* [DeviceRequest](devicerequest.html): Business identifier for request/order 4904* [DeviceUsage](deviceusage.html): Search by identifier 4905* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4906* [DocumentReference](documentreference.html): Identifier of the attachment binary 4907* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4908* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4909* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4910* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4911* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4912* [Flag](flag.html): Business identifier 4913* [Goal](goal.html): External Ids for this goal 4914* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4915* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4916* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4917* [Immunization](immunization.html): Business identifier 4918* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4919* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4920* [Invoice](invoice.html): Business Identifier for item 4921* [List](list.html): Business identifier 4922* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4923* [Medication](medication.html): Returns medications with this external identifier 4924* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4925* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4926* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4927* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4928* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4929* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4930* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4931* [Observation](observation.html): The unique id for a particular observation 4932* [Person](person.html): A person Identifier 4933* [Procedure](procedure.html): A unique identifier for a procedure 4934* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4935* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4936* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4937* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4938* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4939* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4940* [Specimen](specimen.html): The unique identifier associated with the specimen 4941* [SupplyDelivery](supplydelivery.html): External identifier 4942* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4943* [Task](task.html): Search for a task instance by its business identifier 4944* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4945</b><br> 4946 * Type: <b>token</b><br> 4947 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4948 * </p> 4949 */ 4950 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4951 public static final String SP_IDENTIFIER = "identifier"; 4952 /** 4953 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4954 * <p> 4955 * Description: <b>Multiple Resources: 4956 4957* [Account](account.html): Account number 4958* [AdverseEvent](adverseevent.html): Business identifier for the event 4959* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4960* [Appointment](appointment.html): An Identifier of the Appointment 4961* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4962* [Basic](basic.html): Business identifier 4963* [BodyStructure](bodystructure.html): Bodystructure identifier 4964* [CarePlan](careplan.html): External Ids for this plan 4965* [CareTeam](careteam.html): External Ids for this team 4966* [ChargeItem](chargeitem.html): Business Identifier for item 4967* [Claim](claim.html): The primary identifier of the financial resource 4968* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4969* [ClinicalImpression](clinicalimpression.html): Business identifier 4970* [Communication](communication.html): Unique identifier 4971* [CommunicationRequest](communicationrequest.html): Unique identifier 4972* [Composition](composition.html): Version-independent identifier for the Composition 4973* [Condition](condition.html): A unique identifier of the condition record 4974* [Consent](consent.html): Identifier for this record (external references) 4975* [Contract](contract.html): The identity of the contract 4976* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4977* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4978* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4979* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4980* [DeviceRequest](devicerequest.html): Business identifier for request/order 4981* [DeviceUsage](deviceusage.html): Search by identifier 4982* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4983* [DocumentReference](documentreference.html): Identifier of the attachment binary 4984* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4985* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4986* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4987* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4988* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4989* [Flag](flag.html): Business identifier 4990* [Goal](goal.html): External Ids for this goal 4991* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4992* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4993* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4994* [Immunization](immunization.html): Business identifier 4995* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4996* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4997* [Invoice](invoice.html): Business Identifier for item 4998* [List](list.html): Business identifier 4999* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 5000* [Medication](medication.html): Returns medications with this external identifier 5001* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 5002* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 5003* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 5004* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 5005* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 5006* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 5007* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 5008* [Observation](observation.html): The unique id for a particular observation 5009* [Person](person.html): A person Identifier 5010* [Procedure](procedure.html): A unique identifier for a procedure 5011* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 5012* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 5013* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 5014* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 5015* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 5016* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 5017* [Specimen](specimen.html): The unique identifier associated with the specimen 5018* [SupplyDelivery](supplydelivery.html): External identifier 5019* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 5020* [Task](task.html): Search for a task instance by its business identifier 5021* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 5022</b><br> 5023 * Type: <b>token</b><br> 5024 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 5025 * </p> 5026 */ 5027 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5028 5029 /** 5030 * Search parameter: <b>patient</b> 5031 * <p> 5032 * Description: <b>Multiple Resources: 5033 5034* [Account](account.html): The entity that caused the expenses 5035* [AdverseEvent](adverseevent.html): Subject impacted by event 5036* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 5037* [Appointment](appointment.html): One of the individuals of the appointment is this patient 5038* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 5039* [AuditEvent](auditevent.html): Where the activity involved patient data 5040* [Basic](basic.html): Identifies the focus of this resource 5041* [BodyStructure](bodystructure.html): Who this is about 5042* [CarePlan](careplan.html): Who the care plan is for 5043* [CareTeam](careteam.html): Who care team is for 5044* [ChargeItem](chargeitem.html): Individual service was done for/to 5045* [Claim](claim.html): Patient receiving the products or services 5046* [ClaimResponse](claimresponse.html): The subject of care 5047* [ClinicalImpression](clinicalimpression.html): Patient assessed 5048* [Communication](communication.html): Focus of message 5049* [CommunicationRequest](communicationrequest.html): Focus of message 5050* [Composition](composition.html): Who and/or what the composition is about 5051* [Condition](condition.html): Who has the condition? 5052* [Consent](consent.html): Who the consent applies to 5053* [Contract](contract.html): The identity of the subject of the contract (if a patient) 5054* [Coverage](coverage.html): Retrieve coverages for a patient 5055* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 5056* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 5057* [DetectedIssue](detectedissue.html): Associated patient 5058* [DeviceRequest](devicerequest.html): Individual the service is ordered for 5059* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 5060* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 5061* [DocumentReference](documentreference.html): Who/what is the subject of the document 5062* [Encounter](encounter.html): The patient present at the encounter 5063* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 5064* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 5065* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 5066* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 5067* [Flag](flag.html): The identity of a subject to list flags for 5068* [Goal](goal.html): Who this goal is intended for 5069* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 5070* [ImagingSelection](imagingselection.html): Who the study is about 5071* [ImagingStudy](imagingstudy.html): Who the study is about 5072* [Immunization](immunization.html): The patient for the vaccination record 5073* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 5074* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 5075* [Invoice](invoice.html): Recipient(s) of goods and services 5076* [List](list.html): If all resources have the same subject 5077* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 5078* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 5079* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 5080* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 5081* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 5082* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 5083* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 5084* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 5085* [Observation](observation.html): The subject that the observation is about (if patient) 5086* [Person](person.html): The Person links to this Patient 5087* [Procedure](procedure.html): Search by subject - a patient 5088* [Provenance](provenance.html): Where the activity involved patient data 5089* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 5090* [RelatedPerson](relatedperson.html): The patient this related person is related to 5091* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 5092* [ResearchSubject](researchsubject.html): Who or what is part of study 5093* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 5094* [ServiceRequest](servicerequest.html): Search by subject - a patient 5095* [Specimen](specimen.html): The patient the specimen comes from 5096* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 5097* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 5098* [Task](task.html): Search by patient 5099* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 5100</b><br> 5101 * Type: <b>reference</b><br> 5102 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 5103 * </p> 5104 */ 5105 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 5106 public static final String SP_PATIENT = "patient"; 5107 /** 5108 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5109 * <p> 5110 * Description: <b>Multiple Resources: 5111 5112* [Account](account.html): The entity that caused the expenses 5113* [AdverseEvent](adverseevent.html): Subject impacted by event 5114* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 5115* [Appointment](appointment.html): One of the individuals of the appointment is this patient 5116* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 5117* [AuditEvent](auditevent.html): Where the activity involved patient data 5118* [Basic](basic.html): Identifies the focus of this resource 5119* [BodyStructure](bodystructure.html): Who this is about 5120* [CarePlan](careplan.html): Who the care plan is for 5121* [CareTeam](careteam.html): Who care team is for 5122* [ChargeItem](chargeitem.html): Individual service was done for/to 5123* [Claim](claim.html): Patient receiving the products or services 5124* [ClaimResponse](claimresponse.html): The subject of care 5125* [ClinicalImpression](clinicalimpression.html): Patient assessed 5126* [Communication](communication.html): Focus of message 5127* [CommunicationRequest](communicationrequest.html): Focus of message 5128* [Composition](composition.html): Who and/or what the composition is about 5129* [Condition](condition.html): Who has the condition? 5130* [Consent](consent.html): Who the consent applies to 5131* [Contract](contract.html): The identity of the subject of the contract (if a patient) 5132* [Coverage](coverage.html): Retrieve coverages for a patient 5133* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 5134* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 5135* [DetectedIssue](detectedissue.html): Associated patient 5136* [DeviceRequest](devicerequest.html): Individual the service is ordered for 5137* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 5138* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 5139* [DocumentReference](documentreference.html): Who/what is the subject of the document 5140* [Encounter](encounter.html): The patient present at the encounter 5141* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 5142* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 5143* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 5144* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 5145* [Flag](flag.html): The identity of a subject to list flags for 5146* [Goal](goal.html): Who this goal is intended for 5147* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 5148* [ImagingSelection](imagingselection.html): Who the study is about 5149* [ImagingStudy](imagingstudy.html): Who the study is about 5150* [Immunization](immunization.html): The patient for the vaccination record 5151* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 5152* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 5153* [Invoice](invoice.html): Recipient(s) of goods and services 5154* [List](list.html): If all resources have the same subject 5155* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 5156* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 5157* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 5158* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 5159* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 5160* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 5161* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 5162* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 5163* [Observation](observation.html): The subject that the observation is about (if patient) 5164* [Person](person.html): The Person links to this Patient 5165* [Procedure](procedure.html): Search by subject - a patient 5166* [Provenance](provenance.html): Where the activity involved patient data 5167* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 5168* [RelatedPerson](relatedperson.html): The patient this related person is related to 5169* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 5170* [ResearchSubject](researchsubject.html): Who or what is part of study 5171* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 5172* [ServiceRequest](servicerequest.html): Search by subject - a patient 5173* [Specimen](specimen.html): The patient the specimen comes from 5174* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 5175* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 5176* [Task](task.html): Search by patient 5177* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 5178</b><br> 5179 * Type: <b>reference</b><br> 5180 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 5181 * </p> 5182 */ 5183 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 5184 5185/** 5186 * Constant for fluent queries to be used to add include statements. Specifies 5187 * the path value of "<b>Observation:patient</b>". 5188 */ 5189 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Observation:patient").toLocked(); 5190 5191 5192} 5193