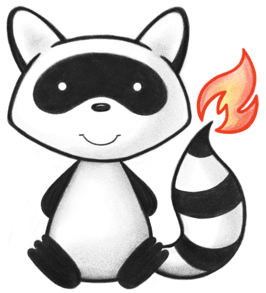
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service. 052 */ 053@ResourceDef(name="ObservationDefinition", profile="http://hl7.org/fhir/StructureDefinition/ObservationDefinition") 054public class ObservationDefinition extends DomainResource { 055 056 public enum ObservationDataType { 057 /** 058 * A measured amount. 059 */ 060 QUANTITY, 061 /** 062 * A coded concept from a reference terminology and/or text. 063 */ 064 CODEABLECONCEPT, 065 /** 066 * A sequence of Unicode characters. 067 */ 068 STRING, 069 /** 070 * true or false. 071 */ 072 BOOLEAN, 073 /** 074 * A signed integer. 075 */ 076 INTEGER, 077 /** 078 * A set of values bounded by low and high. 079 */ 080 RANGE, 081 /** 082 * A ratio of two Quantity values - a numerator and a denominator. 083 */ 084 RATIO, 085 /** 086 * A series of measurements taken by a device. 087 */ 088 SAMPLEDDATA, 089 /** 090 * A time during the day, in the format hh:mm:ss. 091 */ 092 TIME, 093 /** 094 * A date, date-time or partial date (e.g. just year or year + month) as used in human communication. 095 */ 096 DATETIME, 097 /** 098 * A time range defined by start and end date/time. 099 */ 100 PERIOD, 101 /** 102 * added to help the parsers with the generic types 103 */ 104 NULL; 105 public static ObservationDataType fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("Quantity".equals(codeString)) 109 return QUANTITY; 110 if ("CodeableConcept".equals(codeString)) 111 return CODEABLECONCEPT; 112 if ("string".equals(codeString)) 113 return STRING; 114 if ("boolean".equals(codeString)) 115 return BOOLEAN; 116 if ("integer".equals(codeString)) 117 return INTEGER; 118 if ("Range".equals(codeString)) 119 return RANGE; 120 if ("Ratio".equals(codeString)) 121 return RATIO; 122 if ("SampledData".equals(codeString)) 123 return SAMPLEDDATA; 124 if ("time".equals(codeString)) 125 return TIME; 126 if ("dateTime".equals(codeString)) 127 return DATETIME; 128 if ("Period".equals(codeString)) 129 return PERIOD; 130 if (Configuration.isAcceptInvalidEnums()) 131 return null; 132 else 133 throw new FHIRException("Unknown ObservationDataType code '"+codeString+"'"); 134 } 135 public String toCode() { 136 switch (this) { 137 case QUANTITY: return "Quantity"; 138 case CODEABLECONCEPT: return "CodeableConcept"; 139 case STRING: return "string"; 140 case BOOLEAN: return "boolean"; 141 case INTEGER: return "integer"; 142 case RANGE: return "Range"; 143 case RATIO: return "Ratio"; 144 case SAMPLEDDATA: return "SampledData"; 145 case TIME: return "time"; 146 case DATETIME: return "dateTime"; 147 case PERIOD: return "Period"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 public String getSystem() { 153 switch (this) { 154 case QUANTITY: return "http://hl7.org/fhir/permitted-data-type"; 155 case CODEABLECONCEPT: return "http://hl7.org/fhir/permitted-data-type"; 156 case STRING: return "http://hl7.org/fhir/permitted-data-type"; 157 case BOOLEAN: return "http://hl7.org/fhir/permitted-data-type"; 158 case INTEGER: return "http://hl7.org/fhir/permitted-data-type"; 159 case RANGE: return "http://hl7.org/fhir/permitted-data-type"; 160 case RATIO: return "http://hl7.org/fhir/permitted-data-type"; 161 case SAMPLEDDATA: return "http://hl7.org/fhir/permitted-data-type"; 162 case TIME: return "http://hl7.org/fhir/permitted-data-type"; 163 case DATETIME: return "http://hl7.org/fhir/permitted-data-type"; 164 case PERIOD: return "http://hl7.org/fhir/permitted-data-type"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 public String getDefinition() { 170 switch (this) { 171 case QUANTITY: return "A measured amount."; 172 case CODEABLECONCEPT: return "A coded concept from a reference terminology and/or text."; 173 case STRING: return "A sequence of Unicode characters."; 174 case BOOLEAN: return "true or false."; 175 case INTEGER: return "A signed integer."; 176 case RANGE: return "A set of values bounded by low and high."; 177 case RATIO: return "A ratio of two Quantity values - a numerator and a denominator."; 178 case SAMPLEDDATA: return "A series of measurements taken by a device."; 179 case TIME: return "A time during the day, in the format hh:mm:ss."; 180 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month) as used in human communication."; 181 case PERIOD: return "A time range defined by start and end date/time."; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case QUANTITY: return "Quantity"; 189 case CODEABLECONCEPT: return "CodeableConcept"; 190 case STRING: return "string"; 191 case BOOLEAN: return "boolean"; 192 case INTEGER: return "integer"; 193 case RANGE: return "Range"; 194 case RATIO: return "Ratio"; 195 case SAMPLEDDATA: return "SampledData"; 196 case TIME: return "time"; 197 case DATETIME: return "dateTime"; 198 case PERIOD: return "Period"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 } 204 205 public static class ObservationDataTypeEnumFactory implements EnumFactory<ObservationDataType> { 206 public ObservationDataType fromCode(String codeString) throws IllegalArgumentException { 207 if (codeString == null || "".equals(codeString)) 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("Quantity".equals(codeString)) 211 return ObservationDataType.QUANTITY; 212 if ("CodeableConcept".equals(codeString)) 213 return ObservationDataType.CODEABLECONCEPT; 214 if ("string".equals(codeString)) 215 return ObservationDataType.STRING; 216 if ("boolean".equals(codeString)) 217 return ObservationDataType.BOOLEAN; 218 if ("integer".equals(codeString)) 219 return ObservationDataType.INTEGER; 220 if ("Range".equals(codeString)) 221 return ObservationDataType.RANGE; 222 if ("Ratio".equals(codeString)) 223 return ObservationDataType.RATIO; 224 if ("SampledData".equals(codeString)) 225 return ObservationDataType.SAMPLEDDATA; 226 if ("time".equals(codeString)) 227 return ObservationDataType.TIME; 228 if ("dateTime".equals(codeString)) 229 return ObservationDataType.DATETIME; 230 if ("Period".equals(codeString)) 231 return ObservationDataType.PERIOD; 232 throw new IllegalArgumentException("Unknown ObservationDataType code '"+codeString+"'"); 233 } 234 public Enumeration<ObservationDataType> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<ObservationDataType>(this, ObservationDataType.NULL, code); 239 String codeString = ((PrimitiveType) code).asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<ObservationDataType>(this, ObservationDataType.NULL, code); 242 if ("Quantity".equals(codeString)) 243 return new Enumeration<ObservationDataType>(this, ObservationDataType.QUANTITY, code); 244 if ("CodeableConcept".equals(codeString)) 245 return new Enumeration<ObservationDataType>(this, ObservationDataType.CODEABLECONCEPT, code); 246 if ("string".equals(codeString)) 247 return new Enumeration<ObservationDataType>(this, ObservationDataType.STRING, code); 248 if ("boolean".equals(codeString)) 249 return new Enumeration<ObservationDataType>(this, ObservationDataType.BOOLEAN, code); 250 if ("integer".equals(codeString)) 251 return new Enumeration<ObservationDataType>(this, ObservationDataType.INTEGER, code); 252 if ("Range".equals(codeString)) 253 return new Enumeration<ObservationDataType>(this, ObservationDataType.RANGE, code); 254 if ("Ratio".equals(codeString)) 255 return new Enumeration<ObservationDataType>(this, ObservationDataType.RATIO, code); 256 if ("SampledData".equals(codeString)) 257 return new Enumeration<ObservationDataType>(this, ObservationDataType.SAMPLEDDATA, code); 258 if ("time".equals(codeString)) 259 return new Enumeration<ObservationDataType>(this, ObservationDataType.TIME, code); 260 if ("dateTime".equals(codeString)) 261 return new Enumeration<ObservationDataType>(this, ObservationDataType.DATETIME, code); 262 if ("Period".equals(codeString)) 263 return new Enumeration<ObservationDataType>(this, ObservationDataType.PERIOD, code); 264 throw new FHIRException("Unknown ObservationDataType code '"+codeString+"'"); 265 } 266 public String toCode(ObservationDataType code) { 267 if (code == ObservationDataType.QUANTITY) 268 return "Quantity"; 269 if (code == ObservationDataType.CODEABLECONCEPT) 270 return "CodeableConcept"; 271 if (code == ObservationDataType.STRING) 272 return "string"; 273 if (code == ObservationDataType.BOOLEAN) 274 return "boolean"; 275 if (code == ObservationDataType.INTEGER) 276 return "integer"; 277 if (code == ObservationDataType.RANGE) 278 return "Range"; 279 if (code == ObservationDataType.RATIO) 280 return "Ratio"; 281 if (code == ObservationDataType.SAMPLEDDATA) 282 return "SampledData"; 283 if (code == ObservationDataType.TIME) 284 return "time"; 285 if (code == ObservationDataType.DATETIME) 286 return "dateTime"; 287 if (code == ObservationDataType.PERIOD) 288 return "Period"; 289 return "?"; 290 } 291 public String toSystem(ObservationDataType code) { 292 return code.getSystem(); 293 } 294 } 295 296 public enum ObservationRangeCategory { 297 /** 298 * Reference (Normal) Range for Ordinal and Continuous Observations. 299 */ 300 REFERENCE, 301 /** 302 * Critical Range for Ordinal and Continuous Observations. Results outside this range are critical. 303 */ 304 CRITICAL, 305 /** 306 * Absolute Range for Ordinal and Continuous Observations. Results outside this range are not possible. 307 */ 308 ABSOLUTE, 309 /** 310 * added to help the parsers with the generic types 311 */ 312 NULL; 313 public static ObservationRangeCategory fromCode(String codeString) throws FHIRException { 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("reference".equals(codeString)) 317 return REFERENCE; 318 if ("critical".equals(codeString)) 319 return CRITICAL; 320 if ("absolute".equals(codeString)) 321 return ABSOLUTE; 322 if (Configuration.isAcceptInvalidEnums()) 323 return null; 324 else 325 throw new FHIRException("Unknown ObservationRangeCategory code '"+codeString+"'"); 326 } 327 public String toCode() { 328 switch (this) { 329 case REFERENCE: return "reference"; 330 case CRITICAL: return "critical"; 331 case ABSOLUTE: return "absolute"; 332 case NULL: return null; 333 default: return "?"; 334 } 335 } 336 public String getSystem() { 337 switch (this) { 338 case REFERENCE: return "http://hl7.org/fhir/observation-range-category"; 339 case CRITICAL: return "http://hl7.org/fhir/observation-range-category"; 340 case ABSOLUTE: return "http://hl7.org/fhir/observation-range-category"; 341 case NULL: return null; 342 default: return "?"; 343 } 344 } 345 public String getDefinition() { 346 switch (this) { 347 case REFERENCE: return "Reference (Normal) Range for Ordinal and Continuous Observations."; 348 case CRITICAL: return "Critical Range for Ordinal and Continuous Observations. Results outside this range are critical."; 349 case ABSOLUTE: return "Absolute Range for Ordinal and Continuous Observations. Results outside this range are not possible."; 350 case NULL: return null; 351 default: return "?"; 352 } 353 } 354 public String getDisplay() { 355 switch (this) { 356 case REFERENCE: return "reference range"; 357 case CRITICAL: return "critical range"; 358 case ABSOLUTE: return "absolute range"; 359 case NULL: return null; 360 default: return "?"; 361 } 362 } 363 } 364 365 public static class ObservationRangeCategoryEnumFactory implements EnumFactory<ObservationRangeCategory> { 366 public ObservationRangeCategory fromCode(String codeString) throws IllegalArgumentException { 367 if (codeString == null || "".equals(codeString)) 368 if (codeString == null || "".equals(codeString)) 369 return null; 370 if ("reference".equals(codeString)) 371 return ObservationRangeCategory.REFERENCE; 372 if ("critical".equals(codeString)) 373 return ObservationRangeCategory.CRITICAL; 374 if ("absolute".equals(codeString)) 375 return ObservationRangeCategory.ABSOLUTE; 376 throw new IllegalArgumentException("Unknown ObservationRangeCategory code '"+codeString+"'"); 377 } 378 public Enumeration<ObservationRangeCategory> fromType(PrimitiveType<?> code) throws FHIRException { 379 if (code == null) 380 return null; 381 if (code.isEmpty()) 382 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.NULL, code); 383 String codeString = ((PrimitiveType) code).asStringValue(); 384 if (codeString == null || "".equals(codeString)) 385 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.NULL, code); 386 if ("reference".equals(codeString)) 387 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.REFERENCE, code); 388 if ("critical".equals(codeString)) 389 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.CRITICAL, code); 390 if ("absolute".equals(codeString)) 391 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.ABSOLUTE, code); 392 throw new FHIRException("Unknown ObservationRangeCategory code '"+codeString+"'"); 393 } 394 public String toCode(ObservationRangeCategory code) { 395 if (code == ObservationRangeCategory.REFERENCE) 396 return "reference"; 397 if (code == ObservationRangeCategory.CRITICAL) 398 return "critical"; 399 if (code == ObservationRangeCategory.ABSOLUTE) 400 return "absolute"; 401 return "?"; 402 } 403 public String toSystem(ObservationRangeCategory code) { 404 return code.getSystem(); 405 } 406 } 407 408 @Block() 409 public static class ObservationDefinitionQualifiedValueComponent extends BackboneElement implements IBaseBackboneElement { 410 /** 411 * A concept defining the context for this set of qualified values. 412 */ 413 @Child(name = "context", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 414 @Description(shortDefinition="Context qualifier for the set of qualified values", formalDefinition="A concept defining the context for this set of qualified values." ) 415 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-meaning") 416 protected CodeableConcept context; 417 418 /** 419 * The target population this set of qualified values applies to. 420 */ 421 @Child(name = "appliesTo", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 422 @Description(shortDefinition="Targetted population for the set of qualified values", formalDefinition="The target population this set of qualified values applies to." ) 423 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-appliesto") 424 protected List<CodeableConcept> appliesTo; 425 426 /** 427 * The gender this set of qualified values applies to. 428 */ 429 @Child(name = "gender", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 430 @Description(shortDefinition="male | female | other | unknown", formalDefinition="The gender this set of qualified values applies to." ) 431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 432 protected Enumeration<AdministrativeGender> gender; 433 434 /** 435 * The age range this set of qualified values applies to. 436 */ 437 @Child(name = "age", type = {Range.class}, order=4, min=0, max=1, modifier=false, summary=false) 438 @Description(shortDefinition="Applicable age range for the set of qualified values", formalDefinition="The age range this set of qualified values applies to." ) 439 protected Range age; 440 441 /** 442 * The gestational age this set of qualified values applies to. 443 */ 444 @Child(name = "gestationalAge", type = {Range.class}, order=5, min=0, max=1, modifier=false, summary=false) 445 @Description(shortDefinition="Applicable gestational age range for the set of qualified values", formalDefinition="The gestational age this set of qualified values applies to." ) 446 protected Range gestationalAge; 447 448 /** 449 * Text based condition for which the the set of qualified values is valid. 450 */ 451 @Child(name = "condition", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 452 @Description(shortDefinition="Condition associated with the set of qualified values", formalDefinition="Text based condition for which the the set of qualified values is valid." ) 453 protected StringType condition; 454 455 /** 456 * The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values. 457 */ 458 @Child(name = "rangeCategory", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 459 @Description(shortDefinition="reference | critical | absolute", formalDefinition="The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values." ) 460 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-range-category") 461 protected Enumeration<ObservationRangeCategory> rangeCategory; 462 463 /** 464 * The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values. 465 */ 466 @Child(name = "range", type = {Range.class}, order=8, min=0, max=1, modifier=false, summary=false) 467 @Description(shortDefinition="The range for continuous or ordinal observations", formalDefinition="The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values." ) 468 protected Range range; 469 470 /** 471 * The set of valid coded results for qualitative observations that match the criteria of this set of qualified values. 472 */ 473 @Child(name = "validCodedValueSet", type = {CanonicalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 474 @Description(shortDefinition="Value set of valid coded values as part of this set of qualified values", formalDefinition="The set of valid coded results for qualitative observations that match the criteria of this set of qualified values." ) 475 protected CanonicalType validCodedValueSet; 476 477 /** 478 * The set of normal coded results for qualitative observations that match the criteria of this set of qualified values. 479 */ 480 @Child(name = "normalCodedValueSet", type = {CanonicalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 481 @Description(shortDefinition="Value set of normal coded values as part of this set of qualified values", formalDefinition="The set of normal coded results for qualitative observations that match the criteria of this set of qualified values." ) 482 protected CanonicalType normalCodedValueSet; 483 484 /** 485 * The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values. 486 */ 487 @Child(name = "abnormalCodedValueSet", type = {CanonicalType.class}, order=11, min=0, max=1, modifier=false, summary=false) 488 @Description(shortDefinition="Value set of abnormal coded values as part of this set of qualified values", formalDefinition="The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values." ) 489 protected CanonicalType abnormalCodedValueSet; 490 491 /** 492 * The set of critical coded results for qualitative observations that match the criteria of this set of qualified values. 493 */ 494 @Child(name = "criticalCodedValueSet", type = {CanonicalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 495 @Description(shortDefinition="Value set of critical coded values as part of this set of qualified values", formalDefinition="The set of critical coded results for qualitative observations that match the criteria of this set of qualified values." ) 496 protected CanonicalType criticalCodedValueSet; 497 498 private static final long serialVersionUID = -538666361L; 499 500 /** 501 * Constructor 502 */ 503 public ObservationDefinitionQualifiedValueComponent() { 504 super(); 505 } 506 507 /** 508 * @return {@link #context} (A concept defining the context for this set of qualified values.) 509 */ 510 public CodeableConcept getContext() { 511 if (this.context == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.context"); 514 else if (Configuration.doAutoCreate()) 515 this.context = new CodeableConcept(); // cc 516 return this.context; 517 } 518 519 public boolean hasContext() { 520 return this.context != null && !this.context.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #context} (A concept defining the context for this set of qualified values.) 525 */ 526 public ObservationDefinitionQualifiedValueComponent setContext(CodeableConcept value) { 527 this.context = value; 528 return this; 529 } 530 531 /** 532 * @return {@link #appliesTo} (The target population this set of qualified values applies to.) 533 */ 534 public List<CodeableConcept> getAppliesTo() { 535 if (this.appliesTo == null) 536 this.appliesTo = new ArrayList<CodeableConcept>(); 537 return this.appliesTo; 538 } 539 540 /** 541 * @return Returns a reference to <code>this</code> for easy method chaining 542 */ 543 public ObservationDefinitionQualifiedValueComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 544 this.appliesTo = theAppliesTo; 545 return this; 546 } 547 548 public boolean hasAppliesTo() { 549 if (this.appliesTo == null) 550 return false; 551 for (CodeableConcept item : this.appliesTo) 552 if (!item.isEmpty()) 553 return true; 554 return false; 555 } 556 557 public CodeableConcept addAppliesTo() { //3 558 CodeableConcept t = new CodeableConcept(); 559 if (this.appliesTo == null) 560 this.appliesTo = new ArrayList<CodeableConcept>(); 561 this.appliesTo.add(t); 562 return t; 563 } 564 565 public ObservationDefinitionQualifiedValueComponent addAppliesTo(CodeableConcept t) { //3 566 if (t == null) 567 return this; 568 if (this.appliesTo == null) 569 this.appliesTo = new ArrayList<CodeableConcept>(); 570 this.appliesTo.add(t); 571 return this; 572 } 573 574 /** 575 * @return The first repetition of repeating field {@link #appliesTo}, creating it if it does not already exist {3} 576 */ 577 public CodeableConcept getAppliesToFirstRep() { 578 if (getAppliesTo().isEmpty()) { 579 addAppliesTo(); 580 } 581 return getAppliesTo().get(0); 582 } 583 584 /** 585 * @return {@link #gender} (The gender this set of qualified values applies to.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 586 */ 587 public Enumeration<AdministrativeGender> getGenderElement() { 588 if (this.gender == null) 589 if (Configuration.errorOnAutoCreate()) 590 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.gender"); 591 else if (Configuration.doAutoCreate()) 592 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 593 return this.gender; 594 } 595 596 public boolean hasGenderElement() { 597 return this.gender != null && !this.gender.isEmpty(); 598 } 599 600 public boolean hasGender() { 601 return this.gender != null && !this.gender.isEmpty(); 602 } 603 604 /** 605 * @param value {@link #gender} (The gender this set of qualified values applies to.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 606 */ 607 public ObservationDefinitionQualifiedValueComponent setGenderElement(Enumeration<AdministrativeGender> value) { 608 this.gender = value; 609 return this; 610 } 611 612 /** 613 * @return The gender this set of qualified values applies to. 614 */ 615 public AdministrativeGender getGender() { 616 return this.gender == null ? null : this.gender.getValue(); 617 } 618 619 /** 620 * @param value The gender this set of qualified values applies to. 621 */ 622 public ObservationDefinitionQualifiedValueComponent setGender(AdministrativeGender value) { 623 if (value == null) 624 this.gender = null; 625 else { 626 if (this.gender == null) 627 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 628 this.gender.setValue(value); 629 } 630 return this; 631 } 632 633 /** 634 * @return {@link #age} (The age range this set of qualified values applies to.) 635 */ 636 public Range getAge() { 637 if (this.age == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.age"); 640 else if (Configuration.doAutoCreate()) 641 this.age = new Range(); // cc 642 return this.age; 643 } 644 645 public boolean hasAge() { 646 return this.age != null && !this.age.isEmpty(); 647 } 648 649 /** 650 * @param value {@link #age} (The age range this set of qualified values applies to.) 651 */ 652 public ObservationDefinitionQualifiedValueComponent setAge(Range value) { 653 this.age = value; 654 return this; 655 } 656 657 /** 658 * @return {@link #gestationalAge} (The gestational age this set of qualified values applies to.) 659 */ 660 public Range getGestationalAge() { 661 if (this.gestationalAge == null) 662 if (Configuration.errorOnAutoCreate()) 663 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.gestationalAge"); 664 else if (Configuration.doAutoCreate()) 665 this.gestationalAge = new Range(); // cc 666 return this.gestationalAge; 667 } 668 669 public boolean hasGestationalAge() { 670 return this.gestationalAge != null && !this.gestationalAge.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #gestationalAge} (The gestational age this set of qualified values applies to.) 675 */ 676 public ObservationDefinitionQualifiedValueComponent setGestationalAge(Range value) { 677 this.gestationalAge = value; 678 return this; 679 } 680 681 /** 682 * @return {@link #condition} (Text based condition for which the the set of qualified values is valid.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 683 */ 684 public StringType getConditionElement() { 685 if (this.condition == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.condition"); 688 else if (Configuration.doAutoCreate()) 689 this.condition = new StringType(); // bb 690 return this.condition; 691 } 692 693 public boolean hasConditionElement() { 694 return this.condition != null && !this.condition.isEmpty(); 695 } 696 697 public boolean hasCondition() { 698 return this.condition != null && !this.condition.isEmpty(); 699 } 700 701 /** 702 * @param value {@link #condition} (Text based condition for which the the set of qualified values is valid.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 703 */ 704 public ObservationDefinitionQualifiedValueComponent setConditionElement(StringType value) { 705 this.condition = value; 706 return this; 707 } 708 709 /** 710 * @return Text based condition for which the the set of qualified values is valid. 711 */ 712 public String getCondition() { 713 return this.condition == null ? null : this.condition.getValue(); 714 } 715 716 /** 717 * @param value Text based condition for which the the set of qualified values is valid. 718 */ 719 public ObservationDefinitionQualifiedValueComponent setCondition(String value) { 720 if (Utilities.noString(value)) 721 this.condition = null; 722 else { 723 if (this.condition == null) 724 this.condition = new StringType(); 725 this.condition.setValue(value); 726 } 727 return this; 728 } 729 730 /** 731 * @return {@link #rangeCategory} (The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getRangeCategory" gives direct access to the value 732 */ 733 public Enumeration<ObservationRangeCategory> getRangeCategoryElement() { 734 if (this.rangeCategory == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.rangeCategory"); 737 else if (Configuration.doAutoCreate()) 738 this.rangeCategory = new Enumeration<ObservationRangeCategory>(new ObservationRangeCategoryEnumFactory()); // bb 739 return this.rangeCategory; 740 } 741 742 public boolean hasRangeCategoryElement() { 743 return this.rangeCategory != null && !this.rangeCategory.isEmpty(); 744 } 745 746 public boolean hasRangeCategory() { 747 return this.rangeCategory != null && !this.rangeCategory.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #rangeCategory} (The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getRangeCategory" gives direct access to the value 752 */ 753 public ObservationDefinitionQualifiedValueComponent setRangeCategoryElement(Enumeration<ObservationRangeCategory> value) { 754 this.rangeCategory = value; 755 return this; 756 } 757 758 /** 759 * @return The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values. 760 */ 761 public ObservationRangeCategory getRangeCategory() { 762 return this.rangeCategory == null ? null : this.rangeCategory.getValue(); 763 } 764 765 /** 766 * @param value The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values. 767 */ 768 public ObservationDefinitionQualifiedValueComponent setRangeCategory(ObservationRangeCategory value) { 769 if (value == null) 770 this.rangeCategory = null; 771 else { 772 if (this.rangeCategory == null) 773 this.rangeCategory = new Enumeration<ObservationRangeCategory>(new ObservationRangeCategoryEnumFactory()); 774 this.rangeCategory.setValue(value); 775 } 776 return this; 777 } 778 779 /** 780 * @return {@link #range} (The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.) 781 */ 782 public Range getRange() { 783 if (this.range == null) 784 if (Configuration.errorOnAutoCreate()) 785 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.range"); 786 else if (Configuration.doAutoCreate()) 787 this.range = new Range(); // cc 788 return this.range; 789 } 790 791 public boolean hasRange() { 792 return this.range != null && !this.range.isEmpty(); 793 } 794 795 /** 796 * @param value {@link #range} (The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.) 797 */ 798 public ObservationDefinitionQualifiedValueComponent setRange(Range value) { 799 this.range = value; 800 return this; 801 } 802 803 /** 804 * @return {@link #validCodedValueSet} (The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getValidCodedValueSet" gives direct access to the value 805 */ 806 public CanonicalType getValidCodedValueSetElement() { 807 if (this.validCodedValueSet == null) 808 if (Configuration.errorOnAutoCreate()) 809 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.validCodedValueSet"); 810 else if (Configuration.doAutoCreate()) 811 this.validCodedValueSet = new CanonicalType(); // bb 812 return this.validCodedValueSet; 813 } 814 815 public boolean hasValidCodedValueSetElement() { 816 return this.validCodedValueSet != null && !this.validCodedValueSet.isEmpty(); 817 } 818 819 public boolean hasValidCodedValueSet() { 820 return this.validCodedValueSet != null && !this.validCodedValueSet.isEmpty(); 821 } 822 823 /** 824 * @param value {@link #validCodedValueSet} (The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getValidCodedValueSet" gives direct access to the value 825 */ 826 public ObservationDefinitionQualifiedValueComponent setValidCodedValueSetElement(CanonicalType value) { 827 this.validCodedValueSet = value; 828 return this; 829 } 830 831 /** 832 * @return The set of valid coded results for qualitative observations that match the criteria of this set of qualified values. 833 */ 834 public String getValidCodedValueSet() { 835 return this.validCodedValueSet == null ? null : this.validCodedValueSet.getValue(); 836 } 837 838 /** 839 * @param value The set of valid coded results for qualitative observations that match the criteria of this set of qualified values. 840 */ 841 public ObservationDefinitionQualifiedValueComponent setValidCodedValueSet(String value) { 842 if (Utilities.noString(value)) 843 this.validCodedValueSet = null; 844 else { 845 if (this.validCodedValueSet == null) 846 this.validCodedValueSet = new CanonicalType(); 847 this.validCodedValueSet.setValue(value); 848 } 849 return this; 850 } 851 852 /** 853 * @return {@link #normalCodedValueSet} (The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getNormalCodedValueSet" gives direct access to the value 854 */ 855 public CanonicalType getNormalCodedValueSetElement() { 856 if (this.normalCodedValueSet == null) 857 if (Configuration.errorOnAutoCreate()) 858 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.normalCodedValueSet"); 859 else if (Configuration.doAutoCreate()) 860 this.normalCodedValueSet = new CanonicalType(); // bb 861 return this.normalCodedValueSet; 862 } 863 864 public boolean hasNormalCodedValueSetElement() { 865 return this.normalCodedValueSet != null && !this.normalCodedValueSet.isEmpty(); 866 } 867 868 public boolean hasNormalCodedValueSet() { 869 return this.normalCodedValueSet != null && !this.normalCodedValueSet.isEmpty(); 870 } 871 872 /** 873 * @param value {@link #normalCodedValueSet} (The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getNormalCodedValueSet" gives direct access to the value 874 */ 875 public ObservationDefinitionQualifiedValueComponent setNormalCodedValueSetElement(CanonicalType value) { 876 this.normalCodedValueSet = value; 877 return this; 878 } 879 880 /** 881 * @return The set of normal coded results for qualitative observations that match the criteria of this set of qualified values. 882 */ 883 public String getNormalCodedValueSet() { 884 return this.normalCodedValueSet == null ? null : this.normalCodedValueSet.getValue(); 885 } 886 887 /** 888 * @param value The set of normal coded results for qualitative observations that match the criteria of this set of qualified values. 889 */ 890 public ObservationDefinitionQualifiedValueComponent setNormalCodedValueSet(String value) { 891 if (Utilities.noString(value)) 892 this.normalCodedValueSet = null; 893 else { 894 if (this.normalCodedValueSet == null) 895 this.normalCodedValueSet = new CanonicalType(); 896 this.normalCodedValueSet.setValue(value); 897 } 898 return this; 899 } 900 901 /** 902 * @return {@link #abnormalCodedValueSet} (The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getAbnormalCodedValueSet" gives direct access to the value 903 */ 904 public CanonicalType getAbnormalCodedValueSetElement() { 905 if (this.abnormalCodedValueSet == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.abnormalCodedValueSet"); 908 else if (Configuration.doAutoCreate()) 909 this.abnormalCodedValueSet = new CanonicalType(); // bb 910 return this.abnormalCodedValueSet; 911 } 912 913 public boolean hasAbnormalCodedValueSetElement() { 914 return this.abnormalCodedValueSet != null && !this.abnormalCodedValueSet.isEmpty(); 915 } 916 917 public boolean hasAbnormalCodedValueSet() { 918 return this.abnormalCodedValueSet != null && !this.abnormalCodedValueSet.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #abnormalCodedValueSet} (The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getAbnormalCodedValueSet" gives direct access to the value 923 */ 924 public ObservationDefinitionQualifiedValueComponent setAbnormalCodedValueSetElement(CanonicalType value) { 925 this.abnormalCodedValueSet = value; 926 return this; 927 } 928 929 /** 930 * @return The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values. 931 */ 932 public String getAbnormalCodedValueSet() { 933 return this.abnormalCodedValueSet == null ? null : this.abnormalCodedValueSet.getValue(); 934 } 935 936 /** 937 * @param value The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values. 938 */ 939 public ObservationDefinitionQualifiedValueComponent setAbnormalCodedValueSet(String value) { 940 if (Utilities.noString(value)) 941 this.abnormalCodedValueSet = null; 942 else { 943 if (this.abnormalCodedValueSet == null) 944 this.abnormalCodedValueSet = new CanonicalType(); 945 this.abnormalCodedValueSet.setValue(value); 946 } 947 return this; 948 } 949 950 /** 951 * @return {@link #criticalCodedValueSet} (The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getCriticalCodedValueSet" gives direct access to the value 952 */ 953 public CanonicalType getCriticalCodedValueSetElement() { 954 if (this.criticalCodedValueSet == null) 955 if (Configuration.errorOnAutoCreate()) 956 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.criticalCodedValueSet"); 957 else if (Configuration.doAutoCreate()) 958 this.criticalCodedValueSet = new CanonicalType(); // bb 959 return this.criticalCodedValueSet; 960 } 961 962 public boolean hasCriticalCodedValueSetElement() { 963 return this.criticalCodedValueSet != null && !this.criticalCodedValueSet.isEmpty(); 964 } 965 966 public boolean hasCriticalCodedValueSet() { 967 return this.criticalCodedValueSet != null && !this.criticalCodedValueSet.isEmpty(); 968 } 969 970 /** 971 * @param value {@link #criticalCodedValueSet} (The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getCriticalCodedValueSet" gives direct access to the value 972 */ 973 public ObservationDefinitionQualifiedValueComponent setCriticalCodedValueSetElement(CanonicalType value) { 974 this.criticalCodedValueSet = value; 975 return this; 976 } 977 978 /** 979 * @return The set of critical coded results for qualitative observations that match the criteria of this set of qualified values. 980 */ 981 public String getCriticalCodedValueSet() { 982 return this.criticalCodedValueSet == null ? null : this.criticalCodedValueSet.getValue(); 983 } 984 985 /** 986 * @param value The set of critical coded results for qualitative observations that match the criteria of this set of qualified values. 987 */ 988 public ObservationDefinitionQualifiedValueComponent setCriticalCodedValueSet(String value) { 989 if (Utilities.noString(value)) 990 this.criticalCodedValueSet = null; 991 else { 992 if (this.criticalCodedValueSet == null) 993 this.criticalCodedValueSet = new CanonicalType(); 994 this.criticalCodedValueSet.setValue(value); 995 } 996 return this; 997 } 998 999 protected void listChildren(List<Property> children) { 1000 super.listChildren(children); 1001 children.add(new Property("context", "CodeableConcept", "A concept defining the context for this set of qualified values.", 0, 1, context)); 1002 children.add(new Property("appliesTo", "CodeableConcept", "The target population this set of qualified values applies to.", 0, java.lang.Integer.MAX_VALUE, appliesTo)); 1003 children.add(new Property("gender", "code", "The gender this set of qualified values applies to.", 0, 1, gender)); 1004 children.add(new Property("age", "Range", "The age range this set of qualified values applies to.", 0, 1, age)); 1005 children.add(new Property("gestationalAge", "Range", "The gestational age this set of qualified values applies to.", 0, 1, gestationalAge)); 1006 children.add(new Property("condition", "string", "Text based condition for which the the set of qualified values is valid.", 0, 1, condition)); 1007 children.add(new Property("rangeCategory", "code", "The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, rangeCategory)); 1008 children.add(new Property("range", "Range", "The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, range)); 1009 children.add(new Property("validCodedValueSet", "canonical(ValueSet)", "The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, validCodedValueSet)); 1010 children.add(new Property("normalCodedValueSet", "canonical(ValueSet)", "The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, normalCodedValueSet)); 1011 children.add(new Property("abnormalCodedValueSet", "canonical(ValueSet)", "The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, abnormalCodedValueSet)); 1012 children.add(new Property("criticalCodedValueSet", "canonical(ValueSet)", "The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, criticalCodedValueSet)); 1013 } 1014 1015 @Override 1016 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1017 switch (_hash) { 1018 case 951530927: /*context*/ return new Property("context", "CodeableConcept", "A concept defining the context for this set of qualified values.", 0, 1, context); 1019 case -2089924569: /*appliesTo*/ return new Property("appliesTo", "CodeableConcept", "The target population this set of qualified values applies to.", 0, java.lang.Integer.MAX_VALUE, appliesTo); 1020 case -1249512767: /*gender*/ return new Property("gender", "code", "The gender this set of qualified values applies to.", 0, 1, gender); 1021 case 96511: /*age*/ return new Property("age", "Range", "The age range this set of qualified values applies to.", 0, 1, age); 1022 case -241217538: /*gestationalAge*/ return new Property("gestationalAge", "Range", "The gestational age this set of qualified values applies to.", 0, 1, gestationalAge); 1023 case -861311717: /*condition*/ return new Property("condition", "string", "Text based condition for which the the set of qualified values is valid.", 0, 1, condition); 1024 case -363410085: /*rangeCategory*/ return new Property("rangeCategory", "code", "The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, rangeCategory); 1025 case 108280125: /*range*/ return new Property("range", "Range", "The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, range); 1026 case 1374640076: /*validCodedValueSet*/ return new Property("validCodedValueSet", "canonical(ValueSet)", "The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, validCodedValueSet); 1027 case -837500735: /*normalCodedValueSet*/ return new Property("normalCodedValueSet", "canonical(ValueSet)", "The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, normalCodedValueSet); 1028 case 1073600256: /*abnormalCodedValueSet*/ return new Property("abnormalCodedValueSet", "canonical(ValueSet)", "The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, abnormalCodedValueSet); 1029 case 2568457: /*criticalCodedValueSet*/ return new Property("criticalCodedValueSet", "canonical(ValueSet)", "The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, criticalCodedValueSet); 1030 default: return super.getNamedProperty(_hash, _name, _checkValid); 1031 } 1032 1033 } 1034 1035 @Override 1036 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1037 switch (hash) { 1038 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // CodeableConcept 1039 case -2089924569: /*appliesTo*/ return this.appliesTo == null ? new Base[0] : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 1040 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1041 case 96511: /*age*/ return this.age == null ? new Base[0] : new Base[] {this.age}; // Range 1042 case -241217538: /*gestationalAge*/ return this.gestationalAge == null ? new Base[0] : new Base[] {this.gestationalAge}; // Range 1043 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // StringType 1044 case -363410085: /*rangeCategory*/ return this.rangeCategory == null ? new Base[0] : new Base[] {this.rangeCategory}; // Enumeration<ObservationRangeCategory> 1045 case 108280125: /*range*/ return this.range == null ? new Base[0] : new Base[] {this.range}; // Range 1046 case 1374640076: /*validCodedValueSet*/ return this.validCodedValueSet == null ? new Base[0] : new Base[] {this.validCodedValueSet}; // CanonicalType 1047 case -837500735: /*normalCodedValueSet*/ return this.normalCodedValueSet == null ? new Base[0] : new Base[] {this.normalCodedValueSet}; // CanonicalType 1048 case 1073600256: /*abnormalCodedValueSet*/ return this.abnormalCodedValueSet == null ? new Base[0] : new Base[] {this.abnormalCodedValueSet}; // CanonicalType 1049 case 2568457: /*criticalCodedValueSet*/ return this.criticalCodedValueSet == null ? new Base[0] : new Base[] {this.criticalCodedValueSet}; // CanonicalType 1050 default: return super.getProperty(hash, name, checkValid); 1051 } 1052 1053 } 1054 1055 @Override 1056 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1057 switch (hash) { 1058 case 951530927: // context 1059 this.context = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1060 return value; 1061 case -2089924569: // appliesTo 1062 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1063 return value; 1064 case -1249512767: // gender 1065 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1066 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1067 return value; 1068 case 96511: // age 1069 this.age = TypeConvertor.castToRange(value); // Range 1070 return value; 1071 case -241217538: // gestationalAge 1072 this.gestationalAge = TypeConvertor.castToRange(value); // Range 1073 return value; 1074 case -861311717: // condition 1075 this.condition = TypeConvertor.castToString(value); // StringType 1076 return value; 1077 case -363410085: // rangeCategory 1078 value = new ObservationRangeCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1079 this.rangeCategory = (Enumeration) value; // Enumeration<ObservationRangeCategory> 1080 return value; 1081 case 108280125: // range 1082 this.range = TypeConvertor.castToRange(value); // Range 1083 return value; 1084 case 1374640076: // validCodedValueSet 1085 this.validCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1086 return value; 1087 case -837500735: // normalCodedValueSet 1088 this.normalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1089 return value; 1090 case 1073600256: // abnormalCodedValueSet 1091 this.abnormalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1092 return value; 1093 case 2568457: // criticalCodedValueSet 1094 this.criticalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1095 return value; 1096 default: return super.setProperty(hash, name, value); 1097 } 1098 1099 } 1100 1101 @Override 1102 public Base setProperty(String name, Base value) throws FHIRException { 1103 if (name.equals("context")) { 1104 this.context = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1105 } else if (name.equals("appliesTo")) { 1106 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); 1107 } else if (name.equals("gender")) { 1108 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1109 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1110 } else if (name.equals("age")) { 1111 this.age = TypeConvertor.castToRange(value); // Range 1112 } else if (name.equals("gestationalAge")) { 1113 this.gestationalAge = TypeConvertor.castToRange(value); // Range 1114 } else if (name.equals("condition")) { 1115 this.condition = TypeConvertor.castToString(value); // StringType 1116 } else if (name.equals("rangeCategory")) { 1117 value = new ObservationRangeCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1118 this.rangeCategory = (Enumeration) value; // Enumeration<ObservationRangeCategory> 1119 } else if (name.equals("range")) { 1120 this.range = TypeConvertor.castToRange(value); // Range 1121 } else if (name.equals("validCodedValueSet")) { 1122 this.validCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1123 } else if (name.equals("normalCodedValueSet")) { 1124 this.normalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1125 } else if (name.equals("abnormalCodedValueSet")) { 1126 this.abnormalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1127 } else if (name.equals("criticalCodedValueSet")) { 1128 this.criticalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1129 } else 1130 return super.setProperty(name, value); 1131 return value; 1132 } 1133 1134 @Override 1135 public Base makeProperty(int hash, String name) throws FHIRException { 1136 switch (hash) { 1137 case 951530927: return getContext(); 1138 case -2089924569: return addAppliesTo(); 1139 case -1249512767: return getGenderElement(); 1140 case 96511: return getAge(); 1141 case -241217538: return getGestationalAge(); 1142 case -861311717: return getConditionElement(); 1143 case -363410085: return getRangeCategoryElement(); 1144 case 108280125: return getRange(); 1145 case 1374640076: return getValidCodedValueSetElement(); 1146 case -837500735: return getNormalCodedValueSetElement(); 1147 case 1073600256: return getAbnormalCodedValueSetElement(); 1148 case 2568457: return getCriticalCodedValueSetElement(); 1149 default: return super.makeProperty(hash, name); 1150 } 1151 1152 } 1153 1154 @Override 1155 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1156 switch (hash) { 1157 case 951530927: /*context*/ return new String[] {"CodeableConcept"}; 1158 case -2089924569: /*appliesTo*/ return new String[] {"CodeableConcept"}; 1159 case -1249512767: /*gender*/ return new String[] {"code"}; 1160 case 96511: /*age*/ return new String[] {"Range"}; 1161 case -241217538: /*gestationalAge*/ return new String[] {"Range"}; 1162 case -861311717: /*condition*/ return new String[] {"string"}; 1163 case -363410085: /*rangeCategory*/ return new String[] {"code"}; 1164 case 108280125: /*range*/ return new String[] {"Range"}; 1165 case 1374640076: /*validCodedValueSet*/ return new String[] {"canonical"}; 1166 case -837500735: /*normalCodedValueSet*/ return new String[] {"canonical"}; 1167 case 1073600256: /*abnormalCodedValueSet*/ return new String[] {"canonical"}; 1168 case 2568457: /*criticalCodedValueSet*/ return new String[] {"canonical"}; 1169 default: return super.getTypesForProperty(hash, name); 1170 } 1171 1172 } 1173 1174 @Override 1175 public Base addChild(String name) throws FHIRException { 1176 if (name.equals("context")) { 1177 this.context = new CodeableConcept(); 1178 return this.context; 1179 } 1180 else if (name.equals("appliesTo")) { 1181 return addAppliesTo(); 1182 } 1183 else if (name.equals("gender")) { 1184 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.gender"); 1185 } 1186 else if (name.equals("age")) { 1187 this.age = new Range(); 1188 return this.age; 1189 } 1190 else if (name.equals("gestationalAge")) { 1191 this.gestationalAge = new Range(); 1192 return this.gestationalAge; 1193 } 1194 else if (name.equals("condition")) { 1195 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.condition"); 1196 } 1197 else if (name.equals("rangeCategory")) { 1198 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.rangeCategory"); 1199 } 1200 else if (name.equals("range")) { 1201 this.range = new Range(); 1202 return this.range; 1203 } 1204 else if (name.equals("validCodedValueSet")) { 1205 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.validCodedValueSet"); 1206 } 1207 else if (name.equals("normalCodedValueSet")) { 1208 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.normalCodedValueSet"); 1209 } 1210 else if (name.equals("abnormalCodedValueSet")) { 1211 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.abnormalCodedValueSet"); 1212 } 1213 else if (name.equals("criticalCodedValueSet")) { 1214 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.criticalCodedValueSet"); 1215 } 1216 else 1217 return super.addChild(name); 1218 } 1219 1220 public ObservationDefinitionQualifiedValueComponent copy() { 1221 ObservationDefinitionQualifiedValueComponent dst = new ObservationDefinitionQualifiedValueComponent(); 1222 copyValues(dst); 1223 return dst; 1224 } 1225 1226 public void copyValues(ObservationDefinitionQualifiedValueComponent dst) { 1227 super.copyValues(dst); 1228 dst.context = context == null ? null : context.copy(); 1229 if (appliesTo != null) { 1230 dst.appliesTo = new ArrayList<CodeableConcept>(); 1231 for (CodeableConcept i : appliesTo) 1232 dst.appliesTo.add(i.copy()); 1233 }; 1234 dst.gender = gender == null ? null : gender.copy(); 1235 dst.age = age == null ? null : age.copy(); 1236 dst.gestationalAge = gestationalAge == null ? null : gestationalAge.copy(); 1237 dst.condition = condition == null ? null : condition.copy(); 1238 dst.rangeCategory = rangeCategory == null ? null : rangeCategory.copy(); 1239 dst.range = range == null ? null : range.copy(); 1240 dst.validCodedValueSet = validCodedValueSet == null ? null : validCodedValueSet.copy(); 1241 dst.normalCodedValueSet = normalCodedValueSet == null ? null : normalCodedValueSet.copy(); 1242 dst.abnormalCodedValueSet = abnormalCodedValueSet == null ? null : abnormalCodedValueSet.copy(); 1243 dst.criticalCodedValueSet = criticalCodedValueSet == null ? null : criticalCodedValueSet.copy(); 1244 } 1245 1246 @Override 1247 public boolean equalsDeep(Base other_) { 1248 if (!super.equalsDeep(other_)) 1249 return false; 1250 if (!(other_ instanceof ObservationDefinitionQualifiedValueComponent)) 1251 return false; 1252 ObservationDefinitionQualifiedValueComponent o = (ObservationDefinitionQualifiedValueComponent) other_; 1253 return compareDeep(context, o.context, true) && compareDeep(appliesTo, o.appliesTo, true) && compareDeep(gender, o.gender, true) 1254 && compareDeep(age, o.age, true) && compareDeep(gestationalAge, o.gestationalAge, true) && compareDeep(condition, o.condition, true) 1255 && compareDeep(rangeCategory, o.rangeCategory, true) && compareDeep(range, o.range, true) && compareDeep(validCodedValueSet, o.validCodedValueSet, true) 1256 && compareDeep(normalCodedValueSet, o.normalCodedValueSet, true) && compareDeep(abnormalCodedValueSet, o.abnormalCodedValueSet, true) 1257 && compareDeep(criticalCodedValueSet, o.criticalCodedValueSet, true); 1258 } 1259 1260 @Override 1261 public boolean equalsShallow(Base other_) { 1262 if (!super.equalsShallow(other_)) 1263 return false; 1264 if (!(other_ instanceof ObservationDefinitionQualifiedValueComponent)) 1265 return false; 1266 ObservationDefinitionQualifiedValueComponent o = (ObservationDefinitionQualifiedValueComponent) other_; 1267 return compareValues(gender, o.gender, true) && compareValues(condition, o.condition, true) && compareValues(rangeCategory, o.rangeCategory, true) 1268 && compareValues(validCodedValueSet, o.validCodedValueSet, true) && compareValues(normalCodedValueSet, o.normalCodedValueSet, true) 1269 && compareValues(abnormalCodedValueSet, o.abnormalCodedValueSet, true) && compareValues(criticalCodedValueSet, o.criticalCodedValueSet, true) 1270 ; 1271 } 1272 1273 public boolean isEmpty() { 1274 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, appliesTo, gender 1275 , age, gestationalAge, condition, rangeCategory, range, validCodedValueSet, normalCodedValueSet 1276 , abnormalCodedValueSet, criticalCodedValueSet); 1277 } 1278 1279 public String fhirType() { 1280 return "ObservationDefinition.qualifiedValue"; 1281 1282 } 1283 1284 } 1285 1286 @Block() 1287 public static class ObservationDefinitionComponentComponent extends BackboneElement implements IBaseBackboneElement { 1288 /** 1289 * Describes what will be observed. 1290 */ 1291 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1292 @Description(shortDefinition="Type of observation", formalDefinition="Describes what will be observed." ) 1293 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1294 protected CodeableConcept code; 1295 1296 /** 1297 * The data types allowed for the value element of the instance of this component observations. 1298 */ 1299 @Child(name = "permittedDataType", type = {CodeType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1300 @Description(shortDefinition="Quantity | CodeableConcept | string | boolean | integer | Range | Ratio | SampledData | time | dateTime | Period", formalDefinition="The data types allowed for the value element of the instance of this component observations." ) 1301 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/permitted-data-type") 1302 protected List<Enumeration<ObservationDataType>> permittedDataType; 1303 1304 /** 1305 * Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition. 1306 */ 1307 @Child(name = "permittedUnit", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1308 @Description(shortDefinition="Unit for quantitative results", formalDefinition="Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition." ) 1309 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ucum-units") 1310 protected List<Coding> permittedUnit; 1311 1312 /** 1313 * A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations. 1314 */ 1315 @Child(name = "qualifiedValue", type = {ObservationDefinitionQualifiedValueComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1316 @Description(shortDefinition="Set of qualified values for observation results", formalDefinition="A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations." ) 1317 protected List<ObservationDefinitionQualifiedValueComponent> qualifiedValue; 1318 1319 private static final long serialVersionUID = 2106051085L; 1320 1321 /** 1322 * Constructor 1323 */ 1324 public ObservationDefinitionComponentComponent() { 1325 super(); 1326 } 1327 1328 /** 1329 * Constructor 1330 */ 1331 public ObservationDefinitionComponentComponent(CodeableConcept code) { 1332 super(); 1333 this.setCode(code); 1334 } 1335 1336 /** 1337 * @return {@link #code} (Describes what will be observed.) 1338 */ 1339 public CodeableConcept getCode() { 1340 if (this.code == null) 1341 if (Configuration.errorOnAutoCreate()) 1342 throw new Error("Attempt to auto-create ObservationDefinitionComponentComponent.code"); 1343 else if (Configuration.doAutoCreate()) 1344 this.code = new CodeableConcept(); // cc 1345 return this.code; 1346 } 1347 1348 public boolean hasCode() { 1349 return this.code != null && !this.code.isEmpty(); 1350 } 1351 1352 /** 1353 * @param value {@link #code} (Describes what will be observed.) 1354 */ 1355 public ObservationDefinitionComponentComponent setCode(CodeableConcept value) { 1356 this.code = value; 1357 return this; 1358 } 1359 1360 /** 1361 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1362 */ 1363 public List<Enumeration<ObservationDataType>> getPermittedDataType() { 1364 if (this.permittedDataType == null) 1365 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1366 return this.permittedDataType; 1367 } 1368 1369 /** 1370 * @return Returns a reference to <code>this</code> for easy method chaining 1371 */ 1372 public ObservationDefinitionComponentComponent setPermittedDataType(List<Enumeration<ObservationDataType>> thePermittedDataType) { 1373 this.permittedDataType = thePermittedDataType; 1374 return this; 1375 } 1376 1377 public boolean hasPermittedDataType() { 1378 if (this.permittedDataType == null) 1379 return false; 1380 for (Enumeration<ObservationDataType> item : this.permittedDataType) 1381 if (!item.isEmpty()) 1382 return true; 1383 return false; 1384 } 1385 1386 /** 1387 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1388 */ 1389 public Enumeration<ObservationDataType> addPermittedDataTypeElement() {//2 1390 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 1391 if (this.permittedDataType == null) 1392 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1393 this.permittedDataType.add(t); 1394 return t; 1395 } 1396 1397 /** 1398 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1399 */ 1400 public ObservationDefinitionComponentComponent addPermittedDataType(ObservationDataType value) { //1 1401 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 1402 t.setValue(value); 1403 if (this.permittedDataType == null) 1404 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1405 this.permittedDataType.add(t); 1406 return this; 1407 } 1408 1409 /** 1410 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1411 */ 1412 public boolean hasPermittedDataType(ObservationDataType value) { 1413 if (this.permittedDataType == null) 1414 return false; 1415 for (Enumeration<ObservationDataType> v : this.permittedDataType) 1416 if (v.getValue().equals(value)) // code 1417 return true; 1418 return false; 1419 } 1420 1421 /** 1422 * @return {@link #permittedUnit} (Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.) 1423 */ 1424 public List<Coding> getPermittedUnit() { 1425 if (this.permittedUnit == null) 1426 this.permittedUnit = new ArrayList<Coding>(); 1427 return this.permittedUnit; 1428 } 1429 1430 /** 1431 * @return Returns a reference to <code>this</code> for easy method chaining 1432 */ 1433 public ObservationDefinitionComponentComponent setPermittedUnit(List<Coding> thePermittedUnit) { 1434 this.permittedUnit = thePermittedUnit; 1435 return this; 1436 } 1437 1438 public boolean hasPermittedUnit() { 1439 if (this.permittedUnit == null) 1440 return false; 1441 for (Coding item : this.permittedUnit) 1442 if (!item.isEmpty()) 1443 return true; 1444 return false; 1445 } 1446 1447 public Coding addPermittedUnit() { //3 1448 Coding t = new Coding(); 1449 if (this.permittedUnit == null) 1450 this.permittedUnit = new ArrayList<Coding>(); 1451 this.permittedUnit.add(t); 1452 return t; 1453 } 1454 1455 public ObservationDefinitionComponentComponent addPermittedUnit(Coding t) { //3 1456 if (t == null) 1457 return this; 1458 if (this.permittedUnit == null) 1459 this.permittedUnit = new ArrayList<Coding>(); 1460 this.permittedUnit.add(t); 1461 return this; 1462 } 1463 1464 /** 1465 * @return The first repetition of repeating field {@link #permittedUnit}, creating it if it does not already exist {3} 1466 */ 1467 public Coding getPermittedUnitFirstRep() { 1468 if (getPermittedUnit().isEmpty()) { 1469 addPermittedUnit(); 1470 } 1471 return getPermittedUnit().get(0); 1472 } 1473 1474 /** 1475 * @return {@link #qualifiedValue} (A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.) 1476 */ 1477 public List<ObservationDefinitionQualifiedValueComponent> getQualifiedValue() { 1478 if (this.qualifiedValue == null) 1479 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1480 return this.qualifiedValue; 1481 } 1482 1483 /** 1484 * @return Returns a reference to <code>this</code> for easy method chaining 1485 */ 1486 public ObservationDefinitionComponentComponent setQualifiedValue(List<ObservationDefinitionQualifiedValueComponent> theQualifiedValue) { 1487 this.qualifiedValue = theQualifiedValue; 1488 return this; 1489 } 1490 1491 public boolean hasQualifiedValue() { 1492 if (this.qualifiedValue == null) 1493 return false; 1494 for (ObservationDefinitionQualifiedValueComponent item : this.qualifiedValue) 1495 if (!item.isEmpty()) 1496 return true; 1497 return false; 1498 } 1499 1500 public ObservationDefinitionQualifiedValueComponent addQualifiedValue() { //3 1501 ObservationDefinitionQualifiedValueComponent t = new ObservationDefinitionQualifiedValueComponent(); 1502 if (this.qualifiedValue == null) 1503 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1504 this.qualifiedValue.add(t); 1505 return t; 1506 } 1507 1508 public ObservationDefinitionComponentComponent addQualifiedValue(ObservationDefinitionQualifiedValueComponent t) { //3 1509 if (t == null) 1510 return this; 1511 if (this.qualifiedValue == null) 1512 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1513 this.qualifiedValue.add(t); 1514 return this; 1515 } 1516 1517 /** 1518 * @return The first repetition of repeating field {@link #qualifiedValue}, creating it if it does not already exist {3} 1519 */ 1520 public ObservationDefinitionQualifiedValueComponent getQualifiedValueFirstRep() { 1521 if (getQualifiedValue().isEmpty()) { 1522 addQualifiedValue(); 1523 } 1524 return getQualifiedValue().get(0); 1525 } 1526 1527 protected void listChildren(List<Property> children) { 1528 super.listChildren(children); 1529 children.add(new Property("code", "CodeableConcept", "Describes what will be observed.", 0, 1, code)); 1530 children.add(new Property("permittedDataType", "code", "The data types allowed for the value element of the instance of this component observations.", 0, java.lang.Integer.MAX_VALUE, permittedDataType)); 1531 children.add(new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit)); 1532 children.add(new Property("qualifiedValue", "@ObservationDefinition.qualifiedValue", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue)); 1533 } 1534 1535 @Override 1536 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1537 switch (_hash) { 1538 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what will be observed.", 0, 1, code); 1539 case -99492804: /*permittedDataType*/ return new Property("permittedDataType", "code", "The data types allowed for the value element of the instance of this component observations.", 0, java.lang.Integer.MAX_VALUE, permittedDataType); 1540 case 1073054652: /*permittedUnit*/ return new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit); 1541 case -558517707: /*qualifiedValue*/ return new Property("qualifiedValue", "@ObservationDefinition.qualifiedValue", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue); 1542 default: return super.getNamedProperty(_hash, _name, _checkValid); 1543 } 1544 1545 } 1546 1547 @Override 1548 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1549 switch (hash) { 1550 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1551 case -99492804: /*permittedDataType*/ return this.permittedDataType == null ? new Base[0] : this.permittedDataType.toArray(new Base[this.permittedDataType.size()]); // Enumeration<ObservationDataType> 1552 case 1073054652: /*permittedUnit*/ return this.permittedUnit == null ? new Base[0] : this.permittedUnit.toArray(new Base[this.permittedUnit.size()]); // Coding 1553 case -558517707: /*qualifiedValue*/ return this.qualifiedValue == null ? new Base[0] : this.qualifiedValue.toArray(new Base[this.qualifiedValue.size()]); // ObservationDefinitionQualifiedValueComponent 1554 default: return super.getProperty(hash, name, checkValid); 1555 } 1556 1557 } 1558 1559 @Override 1560 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1561 switch (hash) { 1562 case 3059181: // code 1563 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1564 return value; 1565 case -99492804: // permittedDataType 1566 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1567 this.getPermittedDataType().add((Enumeration) value); // Enumeration<ObservationDataType> 1568 return value; 1569 case 1073054652: // permittedUnit 1570 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); // Coding 1571 return value; 1572 case -558517707: // qualifiedValue 1573 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); // ObservationDefinitionQualifiedValueComponent 1574 return value; 1575 default: return super.setProperty(hash, name, value); 1576 } 1577 1578 } 1579 1580 @Override 1581 public Base setProperty(String name, Base value) throws FHIRException { 1582 if (name.equals("code")) { 1583 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1584 } else if (name.equals("permittedDataType")) { 1585 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1586 this.getPermittedDataType().add((Enumeration) value); 1587 } else if (name.equals("permittedUnit")) { 1588 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); 1589 } else if (name.equals("qualifiedValue")) { 1590 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); 1591 } else 1592 return super.setProperty(name, value); 1593 return value; 1594 } 1595 1596 @Override 1597 public Base makeProperty(int hash, String name) throws FHIRException { 1598 switch (hash) { 1599 case 3059181: return getCode(); 1600 case -99492804: return addPermittedDataTypeElement(); 1601 case 1073054652: return addPermittedUnit(); 1602 case -558517707: return addQualifiedValue(); 1603 default: return super.makeProperty(hash, name); 1604 } 1605 1606 } 1607 1608 @Override 1609 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1610 switch (hash) { 1611 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1612 case -99492804: /*permittedDataType*/ return new String[] {"code"}; 1613 case 1073054652: /*permittedUnit*/ return new String[] {"Coding"}; 1614 case -558517707: /*qualifiedValue*/ return new String[] {"@ObservationDefinition.qualifiedValue"}; 1615 default: return super.getTypesForProperty(hash, name); 1616 } 1617 1618 } 1619 1620 @Override 1621 public Base addChild(String name) throws FHIRException { 1622 if (name.equals("code")) { 1623 this.code = new CodeableConcept(); 1624 return this.code; 1625 } 1626 else if (name.equals("permittedDataType")) { 1627 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.component.permittedDataType"); 1628 } 1629 else if (name.equals("permittedUnit")) { 1630 return addPermittedUnit(); 1631 } 1632 else if (name.equals("qualifiedValue")) { 1633 return addQualifiedValue(); 1634 } 1635 else 1636 return super.addChild(name); 1637 } 1638 1639 public ObservationDefinitionComponentComponent copy() { 1640 ObservationDefinitionComponentComponent dst = new ObservationDefinitionComponentComponent(); 1641 copyValues(dst); 1642 return dst; 1643 } 1644 1645 public void copyValues(ObservationDefinitionComponentComponent dst) { 1646 super.copyValues(dst); 1647 dst.code = code == null ? null : code.copy(); 1648 if (permittedDataType != null) { 1649 dst.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1650 for (Enumeration<ObservationDataType> i : permittedDataType) 1651 dst.permittedDataType.add(i.copy()); 1652 }; 1653 if (permittedUnit != null) { 1654 dst.permittedUnit = new ArrayList<Coding>(); 1655 for (Coding i : permittedUnit) 1656 dst.permittedUnit.add(i.copy()); 1657 }; 1658 if (qualifiedValue != null) { 1659 dst.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1660 for (ObservationDefinitionQualifiedValueComponent i : qualifiedValue) 1661 dst.qualifiedValue.add(i.copy()); 1662 }; 1663 } 1664 1665 @Override 1666 public boolean equalsDeep(Base other_) { 1667 if (!super.equalsDeep(other_)) 1668 return false; 1669 if (!(other_ instanceof ObservationDefinitionComponentComponent)) 1670 return false; 1671 ObservationDefinitionComponentComponent o = (ObservationDefinitionComponentComponent) other_; 1672 return compareDeep(code, o.code, true) && compareDeep(permittedDataType, o.permittedDataType, true) 1673 && compareDeep(permittedUnit, o.permittedUnit, true) && compareDeep(qualifiedValue, o.qualifiedValue, true) 1674 ; 1675 } 1676 1677 @Override 1678 public boolean equalsShallow(Base other_) { 1679 if (!super.equalsShallow(other_)) 1680 return false; 1681 if (!(other_ instanceof ObservationDefinitionComponentComponent)) 1682 return false; 1683 ObservationDefinitionComponentComponent o = (ObservationDefinitionComponentComponent) other_; 1684 return compareValues(permittedDataType, o.permittedDataType, true); 1685 } 1686 1687 public boolean isEmpty() { 1688 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, permittedDataType, permittedUnit 1689 , qualifiedValue); 1690 } 1691 1692 public String fhirType() { 1693 return "ObservationDefinition.component"; 1694 1695 } 1696 1697 } 1698 1699 /** 1700 * An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions. 1701 */ 1702 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1703 @Description(shortDefinition="Logical canonical URL to reference this ObservationDefinition (globally unique)", formalDefinition="An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions." ) 1704 protected UriType url; 1705 1706 /** 1707 * Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server. 1708 */ 1709 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1710 @Description(shortDefinition="Business identifier of the ObservationDefinition", formalDefinition="Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server." ) 1711 protected Identifier identifier; 1712 1713 /** 1714 * The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 1715 */ 1716 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1717 @Description(shortDefinition="Business version of the ObservationDefinition", formalDefinition="The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable." ) 1718 protected StringType version; 1719 1720 /** 1721 * Indicates the mechanism used to compare versions to determine which is more current. 1722 */ 1723 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1724 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1725 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1726 protected DataType versionAlgorithm; 1727 1728 /** 1729 * A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1730 */ 1731 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1732 @Description(shortDefinition="Name for this ObservationDefinition (computer friendly)", formalDefinition="A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1733 protected StringType name; 1734 1735 /** 1736 * A short, descriptive, user-friendly title for the ObservationDefinition. 1737 */ 1738 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1739 @Description(shortDefinition="Name for this ObservationDefinition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the ObservationDefinition." ) 1740 protected StringType title; 1741 1742 /** 1743 * The current state of the ObservationDefinition. 1744 */ 1745 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 1746 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of the ObservationDefinition." ) 1747 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1748 protected Enumeration<PublicationStatus> status; 1749 1750 /** 1751 * A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1752 */ 1753 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1754 @Description(shortDefinition="If for testing purposes, not real usage", formalDefinition="A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage." ) 1755 protected BooleanType experimental; 1756 1757 /** 1758 * The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes. 1759 */ 1760 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1761 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes." ) 1762 protected DateTimeType date; 1763 1764 /** 1765 * Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact. 1766 */ 1767 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1768 @Description(shortDefinition="The name of the individual or organization that published the ObservationDefinition", formalDefinition="Helps establish the \"authority/credibility\" of the ObservationDefinition. May also allow for contact." ) 1769 protected StringType publisher; 1770 1771 /** 1772 * Contact details to assist a user in finding and communicating with the publisher. 1773 */ 1774 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1775 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1776 protected List<ContactDetail> contact; 1777 1778 /** 1779 * A free text natural language description of the ObservationDefinition from the consumer's perspective. 1780 */ 1781 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1782 @Description(shortDefinition="Natural language description of the ObservationDefinition", formalDefinition="A free text natural language description of the ObservationDefinition from the consumer's perspective." ) 1783 protected MarkdownType description; 1784 1785 /** 1786 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances. 1787 */ 1788 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1789 @Description(shortDefinition="Content intends to support these contexts", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances." ) 1790 protected List<UsageContext> useContext; 1791 1792 /** 1793 * A jurisdiction in which the ObservationDefinition is intended to be used. 1794 */ 1795 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1796 @Description(shortDefinition="Intended jurisdiction for this ObservationDefinition (if applicable)", formalDefinition="A jurisdiction in which the ObservationDefinition is intended to be used." ) 1797 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1798 protected List<CodeableConcept> jurisdiction; 1799 1800 /** 1801 * Explains why this ObservationDefinition is needed and why it has been designed as it has. 1802 */ 1803 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1804 @Description(shortDefinition="Why this ObservationDefinition is defined", formalDefinition="Explains why this ObservationDefinition is needed and why it has been designed as it has." ) 1805 protected MarkdownType purpose; 1806 1807 /** 1808 * Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition. 1809 */ 1810 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1811 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition." ) 1812 protected MarkdownType copyright; 1813 1814 /** 1815 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1816 */ 1817 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1818 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1819 protected StringType copyrightLabel; 1820 1821 /** 1822 * The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1823 */ 1824 @Child(name = "approvalDate", type = {DateType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1825 @Description(shortDefinition="When ObservationDefinition was approved by publisher", formalDefinition="The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 1826 protected DateType approvalDate; 1827 1828 /** 1829 * The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 1830 */ 1831 @Child(name = "lastReviewDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 1832 @Description(shortDefinition="Date on which the asset content was last reviewed by the publisher", formalDefinition="The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date." ) 1833 protected DateType lastReviewDate; 1834 1835 /** 1836 * The period during which the ObservationDefinition content was or is planned to be effective. 1837 */ 1838 @Child(name = "effectivePeriod", type = {Period.class}, order=19, min=0, max=1, modifier=false, summary=true) 1839 @Description(shortDefinition="The effective date range for the ObservationDefinition", formalDefinition="The period during which the ObservationDefinition content was or is planned to be effective." ) 1840 protected Period effectivePeriod; 1841 1842 /** 1843 * The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition. 1844 */ 1845 @Child(name = "derivedFromCanonical", type = {CanonicalType.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1846 @Description(shortDefinition="Based on FHIR definition of another observation", formalDefinition="The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition." ) 1847 protected List<CanonicalType> derivedFromCanonical; 1848 1849 /** 1850 * The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition. 1851 */ 1852 @Child(name = "derivedFromUri", type = {UriType.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1853 @Description(shortDefinition="Based on external definition", formalDefinition="The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition." ) 1854 protected List<UriType> derivedFromUri; 1855 1856 /** 1857 * A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition. 1858 */ 1859 @Child(name = "subject", type = {CodeableConcept.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1860 @Description(shortDefinition="Type of subject for the defined observation", formalDefinition="A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition." ) 1861 protected List<CodeableConcept> subject; 1862 1863 /** 1864 * The type of individual/organization/device that is expected to act upon instances of this definition. 1865 */ 1866 @Child(name = "performerType", type = {CodeableConcept.class}, order=23, min=0, max=1, modifier=false, summary=true) 1867 @Description(shortDefinition="Desired kind of performer for such kind of observation", formalDefinition="The type of individual/organization/device that is expected to act upon instances of this definition." ) 1868 protected CodeableConcept performerType; 1869 1870 /** 1871 * A code that classifies the general type of observation. 1872 */ 1873 @Child(name = "category", type = {CodeableConcept.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1874 @Description(shortDefinition="General type of observation", formalDefinition="A code that classifies the general type of observation." ) 1875 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-category") 1876 protected List<CodeableConcept> category; 1877 1878 /** 1879 * Describes what will be observed. Sometimes this is called the observation "name". 1880 */ 1881 @Child(name = "code", type = {CodeableConcept.class}, order=25, min=1, max=1, modifier=false, summary=true) 1882 @Description(shortDefinition="Type of observation", formalDefinition="Describes what will be observed. Sometimes this is called the observation \"name\"." ) 1883 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1884 protected CodeableConcept code; 1885 1886 /** 1887 * The data types allowed for the value element of the instance observations conforming to this ObservationDefinition. 1888 */ 1889 @Child(name = "permittedDataType", type = {CodeType.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1890 @Description(shortDefinition="Quantity | CodeableConcept | string | boolean | integer | Range | Ratio | SampledData | time | dateTime | Period", formalDefinition="The data types allowed for the value element of the instance observations conforming to this ObservationDefinition." ) 1891 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/permitted-data-type") 1892 protected List<Enumeration<ObservationDataType>> permittedDataType; 1893 1894 /** 1895 * Multiple results allowed for observations conforming to this ObservationDefinition. 1896 */ 1897 @Child(name = "multipleResultsAllowed", type = {BooleanType.class}, order=27, min=0, max=1, modifier=false, summary=false) 1898 @Description(shortDefinition="Multiple results allowed for conforming observations", formalDefinition="Multiple results allowed for observations conforming to this ObservationDefinition." ) 1899 protected BooleanType multipleResultsAllowed; 1900 1901 /** 1902 * The site on the subject's body where the observation is to be made. 1903 */ 1904 @Child(name = "bodySite", type = {CodeableConcept.class}, order=28, min=0, max=1, modifier=false, summary=false) 1905 @Description(shortDefinition="Body part to be observed", formalDefinition="The site on the subject's body where the observation is to be made." ) 1906 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1907 protected CodeableConcept bodySite; 1908 1909 /** 1910 * The method or technique used to perform the observation. 1911 */ 1912 @Child(name = "method", type = {CodeableConcept.class}, order=29, min=0, max=1, modifier=false, summary=false) 1913 @Description(shortDefinition="Method used to produce the observation", formalDefinition="The method or technique used to perform the observation." ) 1914 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-methods") 1915 protected CodeableConcept method; 1916 1917 /** 1918 * The kind of specimen that this type of observation is produced on. 1919 */ 1920 @Child(name = "specimen", type = {SpecimenDefinition.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1921 @Description(shortDefinition="Kind of specimen used by this type of observation", formalDefinition="The kind of specimen that this type of observation is produced on." ) 1922 protected List<Reference> specimen; 1923 1924 /** 1925 * The measurement model of device or actual device used to produce observations of this type. 1926 */ 1927 @Child(name = "device", type = {DeviceDefinition.class, Device.class}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1928 @Description(shortDefinition="Measurement device or model of device", formalDefinition="The measurement model of device or actual device used to produce observations of this type." ) 1929 protected List<Reference> device; 1930 1931 /** 1932 * The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition. 1933 */ 1934 @Child(name = "preferredReportName", type = {StringType.class}, order=32, min=0, max=1, modifier=false, summary=false) 1935 @Description(shortDefinition="The preferred name to be used when reporting the observation results", formalDefinition="The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition." ) 1936 protected StringType preferredReportName; 1937 1938 /** 1939 * Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition. 1940 */ 1941 @Child(name = "permittedUnit", type = {Coding.class}, order=33, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1942 @Description(shortDefinition="Unit for quantitative results", formalDefinition="Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition." ) 1943 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ucum-units") 1944 protected List<Coding> permittedUnit; 1945 1946 /** 1947 * A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations. 1948 */ 1949 @Child(name = "qualifiedValue", type = {}, order=34, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1950 @Description(shortDefinition="Set of qualified values for observation results", formalDefinition="A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations." ) 1951 protected List<ObservationDefinitionQualifiedValueComponent> qualifiedValue; 1952 1953 /** 1954 * This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group. 1955 */ 1956 @Child(name = "hasMember", type = {ObservationDefinition.class, Questionnaire.class}, order=35, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1957 @Description(shortDefinition="Definitions of related resources belonging to this kind of observation group", formalDefinition="This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group." ) 1958 protected List<Reference> hasMember; 1959 1960 /** 1961 * Some observations have multiple component observations, expressed as separate code value pairs. 1962 */ 1963 @Child(name = "component", type = {}, order=36, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1964 @Description(shortDefinition="Component results", formalDefinition="Some observations have multiple component observations, expressed as separate code value pairs." ) 1965 protected List<ObservationDefinitionComponentComponent> component; 1966 1967 private static final long serialVersionUID = -415480292L; 1968 1969 /** 1970 * Constructor 1971 */ 1972 public ObservationDefinition() { 1973 super(); 1974 } 1975 1976 /** 1977 * Constructor 1978 */ 1979 public ObservationDefinition(PublicationStatus status, CodeableConcept code) { 1980 super(); 1981 this.setStatus(status); 1982 this.setCode(code); 1983 } 1984 1985 /** 1986 * @return {@link #url} (An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1987 */ 1988 public UriType getUrlElement() { 1989 if (this.url == null) 1990 if (Configuration.errorOnAutoCreate()) 1991 throw new Error("Attempt to auto-create ObservationDefinition.url"); 1992 else if (Configuration.doAutoCreate()) 1993 this.url = new UriType(); // bb 1994 return this.url; 1995 } 1996 1997 public boolean hasUrlElement() { 1998 return this.url != null && !this.url.isEmpty(); 1999 } 2000 2001 public boolean hasUrl() { 2002 return this.url != null && !this.url.isEmpty(); 2003 } 2004 2005 /** 2006 * @param value {@link #url} (An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2007 */ 2008 public ObservationDefinition setUrlElement(UriType value) { 2009 this.url = value; 2010 return this; 2011 } 2012 2013 /** 2014 * @return An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions. 2015 */ 2016 public String getUrl() { 2017 return this.url == null ? null : this.url.getValue(); 2018 } 2019 2020 /** 2021 * @param value An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions. 2022 */ 2023 public ObservationDefinition setUrl(String value) { 2024 if (Utilities.noString(value)) 2025 this.url = null; 2026 else { 2027 if (this.url == null) 2028 this.url = new UriType(); 2029 this.url.setValue(value); 2030 } 2031 return this; 2032 } 2033 2034 /** 2035 * @return {@link #identifier} (Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 2036 */ 2037 public Identifier getIdentifier() { 2038 if (this.identifier == null) 2039 if (Configuration.errorOnAutoCreate()) 2040 throw new Error("Attempt to auto-create ObservationDefinition.identifier"); 2041 else if (Configuration.doAutoCreate()) 2042 this.identifier = new Identifier(); // cc 2043 return this.identifier; 2044 } 2045 2046 public boolean hasIdentifier() { 2047 return this.identifier != null && !this.identifier.isEmpty(); 2048 } 2049 2050 /** 2051 * @param value {@link #identifier} (Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 2052 */ 2053 public ObservationDefinition setIdentifier(Identifier value) { 2054 this.identifier = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return {@link #version} (The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2060 */ 2061 public StringType getVersionElement() { 2062 if (this.version == null) 2063 if (Configuration.errorOnAutoCreate()) 2064 throw new Error("Attempt to auto-create ObservationDefinition.version"); 2065 else if (Configuration.doAutoCreate()) 2066 this.version = new StringType(); // bb 2067 return this.version; 2068 } 2069 2070 public boolean hasVersionElement() { 2071 return this.version != null && !this.version.isEmpty(); 2072 } 2073 2074 public boolean hasVersion() { 2075 return this.version != null && !this.version.isEmpty(); 2076 } 2077 2078 /** 2079 * @param value {@link #version} (The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2080 */ 2081 public ObservationDefinition setVersionElement(StringType value) { 2082 this.version = value; 2083 return this; 2084 } 2085 2086 /** 2087 * @return The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2088 */ 2089 public String getVersion() { 2090 return this.version == null ? null : this.version.getValue(); 2091 } 2092 2093 /** 2094 * @param value The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2095 */ 2096 public ObservationDefinition setVersion(String value) { 2097 if (Utilities.noString(value)) 2098 this.version = null; 2099 else { 2100 if (this.version == null) 2101 this.version = new StringType(); 2102 this.version.setValue(value); 2103 } 2104 return this; 2105 } 2106 2107 /** 2108 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2109 */ 2110 public DataType getVersionAlgorithm() { 2111 return this.versionAlgorithm; 2112 } 2113 2114 /** 2115 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2116 */ 2117 public StringType getVersionAlgorithmStringType() throws FHIRException { 2118 if (this.versionAlgorithm == null) 2119 this.versionAlgorithm = new StringType(); 2120 if (!(this.versionAlgorithm instanceof StringType)) 2121 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2122 return (StringType) this.versionAlgorithm; 2123 } 2124 2125 public boolean hasVersionAlgorithmStringType() { 2126 return this != null && this.versionAlgorithm instanceof StringType; 2127 } 2128 2129 /** 2130 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2131 */ 2132 public Coding getVersionAlgorithmCoding() throws FHIRException { 2133 if (this.versionAlgorithm == null) 2134 this.versionAlgorithm = new Coding(); 2135 if (!(this.versionAlgorithm instanceof Coding)) 2136 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2137 return (Coding) this.versionAlgorithm; 2138 } 2139 2140 public boolean hasVersionAlgorithmCoding() { 2141 return this != null && this.versionAlgorithm instanceof Coding; 2142 } 2143 2144 public boolean hasVersionAlgorithm() { 2145 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2146 } 2147 2148 /** 2149 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2150 */ 2151 public ObservationDefinition setVersionAlgorithm(DataType value) { 2152 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2153 throw new FHIRException("Not the right type for ObservationDefinition.versionAlgorithm[x]: "+value.fhirType()); 2154 this.versionAlgorithm = value; 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #name} (A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2160 */ 2161 public StringType getNameElement() { 2162 if (this.name == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create ObservationDefinition.name"); 2165 else if (Configuration.doAutoCreate()) 2166 this.name = new StringType(); // bb 2167 return this.name; 2168 } 2169 2170 public boolean hasNameElement() { 2171 return this.name != null && !this.name.isEmpty(); 2172 } 2173 2174 public boolean hasName() { 2175 return this.name != null && !this.name.isEmpty(); 2176 } 2177 2178 /** 2179 * @param value {@link #name} (A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2180 */ 2181 public ObservationDefinition setNameElement(StringType value) { 2182 this.name = value; 2183 return this; 2184 } 2185 2186 /** 2187 * @return A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2188 */ 2189 public String getName() { 2190 return this.name == null ? null : this.name.getValue(); 2191 } 2192 2193 /** 2194 * @param value A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2195 */ 2196 public ObservationDefinition setName(String value) { 2197 if (Utilities.noString(value)) 2198 this.name = null; 2199 else { 2200 if (this.name == null) 2201 this.name = new StringType(); 2202 this.name.setValue(value); 2203 } 2204 return this; 2205 } 2206 2207 /** 2208 * @return {@link #title} (A short, descriptive, user-friendly title for the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2209 */ 2210 public StringType getTitleElement() { 2211 if (this.title == null) 2212 if (Configuration.errorOnAutoCreate()) 2213 throw new Error("Attempt to auto-create ObservationDefinition.title"); 2214 else if (Configuration.doAutoCreate()) 2215 this.title = new StringType(); // bb 2216 return this.title; 2217 } 2218 2219 public boolean hasTitleElement() { 2220 return this.title != null && !this.title.isEmpty(); 2221 } 2222 2223 public boolean hasTitle() { 2224 return this.title != null && !this.title.isEmpty(); 2225 } 2226 2227 /** 2228 * @param value {@link #title} (A short, descriptive, user-friendly title for the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2229 */ 2230 public ObservationDefinition setTitleElement(StringType value) { 2231 this.title = value; 2232 return this; 2233 } 2234 2235 /** 2236 * @return A short, descriptive, user-friendly title for the ObservationDefinition. 2237 */ 2238 public String getTitle() { 2239 return this.title == null ? null : this.title.getValue(); 2240 } 2241 2242 /** 2243 * @param value A short, descriptive, user-friendly title for the ObservationDefinition. 2244 */ 2245 public ObservationDefinition setTitle(String value) { 2246 if (Utilities.noString(value)) 2247 this.title = null; 2248 else { 2249 if (this.title == null) 2250 this.title = new StringType(); 2251 this.title.setValue(value); 2252 } 2253 return this; 2254 } 2255 2256 /** 2257 * @return {@link #status} (The current state of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2258 */ 2259 public Enumeration<PublicationStatus> getStatusElement() { 2260 if (this.status == null) 2261 if (Configuration.errorOnAutoCreate()) 2262 throw new Error("Attempt to auto-create ObservationDefinition.status"); 2263 else if (Configuration.doAutoCreate()) 2264 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2265 return this.status; 2266 } 2267 2268 public boolean hasStatusElement() { 2269 return this.status != null && !this.status.isEmpty(); 2270 } 2271 2272 public boolean hasStatus() { 2273 return this.status != null && !this.status.isEmpty(); 2274 } 2275 2276 /** 2277 * @param value {@link #status} (The current state of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2278 */ 2279 public ObservationDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2280 this.status = value; 2281 return this; 2282 } 2283 2284 /** 2285 * @return The current state of the ObservationDefinition. 2286 */ 2287 public PublicationStatus getStatus() { 2288 return this.status == null ? null : this.status.getValue(); 2289 } 2290 2291 /** 2292 * @param value The current state of the ObservationDefinition. 2293 */ 2294 public ObservationDefinition setStatus(PublicationStatus value) { 2295 if (this.status == null) 2296 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2297 this.status.setValue(value); 2298 return this; 2299 } 2300 2301 /** 2302 * @return {@link #experimental} (A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2303 */ 2304 public BooleanType getExperimentalElement() { 2305 if (this.experimental == null) 2306 if (Configuration.errorOnAutoCreate()) 2307 throw new Error("Attempt to auto-create ObservationDefinition.experimental"); 2308 else if (Configuration.doAutoCreate()) 2309 this.experimental = new BooleanType(); // bb 2310 return this.experimental; 2311 } 2312 2313 public boolean hasExperimentalElement() { 2314 return this.experimental != null && !this.experimental.isEmpty(); 2315 } 2316 2317 public boolean hasExperimental() { 2318 return this.experimental != null && !this.experimental.isEmpty(); 2319 } 2320 2321 /** 2322 * @param value {@link #experimental} (A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2323 */ 2324 public ObservationDefinition setExperimentalElement(BooleanType value) { 2325 this.experimental = value; 2326 return this; 2327 } 2328 2329 /** 2330 * @return A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2331 */ 2332 public boolean getExperimental() { 2333 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2334 } 2335 2336 /** 2337 * @param value A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2338 */ 2339 public ObservationDefinition setExperimental(boolean value) { 2340 if (this.experimental == null) 2341 this.experimental = new BooleanType(); 2342 this.experimental.setValue(value); 2343 return this; 2344 } 2345 2346 /** 2347 * @return {@link #date} (The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2348 */ 2349 public DateTimeType getDateElement() { 2350 if (this.date == null) 2351 if (Configuration.errorOnAutoCreate()) 2352 throw new Error("Attempt to auto-create ObservationDefinition.date"); 2353 else if (Configuration.doAutoCreate()) 2354 this.date = new DateTimeType(); // bb 2355 return this.date; 2356 } 2357 2358 public boolean hasDateElement() { 2359 return this.date != null && !this.date.isEmpty(); 2360 } 2361 2362 public boolean hasDate() { 2363 return this.date != null && !this.date.isEmpty(); 2364 } 2365 2366 /** 2367 * @param value {@link #date} (The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2368 */ 2369 public ObservationDefinition setDateElement(DateTimeType value) { 2370 this.date = value; 2371 return this; 2372 } 2373 2374 /** 2375 * @return The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes. 2376 */ 2377 public Date getDate() { 2378 return this.date == null ? null : this.date.getValue(); 2379 } 2380 2381 /** 2382 * @param value The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes. 2383 */ 2384 public ObservationDefinition setDate(Date value) { 2385 if (value == null) 2386 this.date = null; 2387 else { 2388 if (this.date == null) 2389 this.date = new DateTimeType(); 2390 this.date.setValue(value); 2391 } 2392 return this; 2393 } 2394 2395 /** 2396 * @return {@link #publisher} (Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2397 */ 2398 public StringType getPublisherElement() { 2399 if (this.publisher == null) 2400 if (Configuration.errorOnAutoCreate()) 2401 throw new Error("Attempt to auto-create ObservationDefinition.publisher"); 2402 else if (Configuration.doAutoCreate()) 2403 this.publisher = new StringType(); // bb 2404 return this.publisher; 2405 } 2406 2407 public boolean hasPublisherElement() { 2408 return this.publisher != null && !this.publisher.isEmpty(); 2409 } 2410 2411 public boolean hasPublisher() { 2412 return this.publisher != null && !this.publisher.isEmpty(); 2413 } 2414 2415 /** 2416 * @param value {@link #publisher} (Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2417 */ 2418 public ObservationDefinition setPublisherElement(StringType value) { 2419 this.publisher = value; 2420 return this; 2421 } 2422 2423 /** 2424 * @return Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact. 2425 */ 2426 public String getPublisher() { 2427 return this.publisher == null ? null : this.publisher.getValue(); 2428 } 2429 2430 /** 2431 * @param value Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact. 2432 */ 2433 public ObservationDefinition setPublisher(String value) { 2434 if (Utilities.noString(value)) 2435 this.publisher = null; 2436 else { 2437 if (this.publisher == null) 2438 this.publisher = new StringType(); 2439 this.publisher.setValue(value); 2440 } 2441 return this; 2442 } 2443 2444 /** 2445 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2446 */ 2447 public List<ContactDetail> getContact() { 2448 if (this.contact == null) 2449 this.contact = new ArrayList<ContactDetail>(); 2450 return this.contact; 2451 } 2452 2453 /** 2454 * @return Returns a reference to <code>this</code> for easy method chaining 2455 */ 2456 public ObservationDefinition setContact(List<ContactDetail> theContact) { 2457 this.contact = theContact; 2458 return this; 2459 } 2460 2461 public boolean hasContact() { 2462 if (this.contact == null) 2463 return false; 2464 for (ContactDetail item : this.contact) 2465 if (!item.isEmpty()) 2466 return true; 2467 return false; 2468 } 2469 2470 public ContactDetail addContact() { //3 2471 ContactDetail t = new ContactDetail(); 2472 if (this.contact == null) 2473 this.contact = new ArrayList<ContactDetail>(); 2474 this.contact.add(t); 2475 return t; 2476 } 2477 2478 public ObservationDefinition addContact(ContactDetail t) { //3 2479 if (t == null) 2480 return this; 2481 if (this.contact == null) 2482 this.contact = new ArrayList<ContactDetail>(); 2483 this.contact.add(t); 2484 return this; 2485 } 2486 2487 /** 2488 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2489 */ 2490 public ContactDetail getContactFirstRep() { 2491 if (getContact().isEmpty()) { 2492 addContact(); 2493 } 2494 return getContact().get(0); 2495 } 2496 2497 /** 2498 * @return {@link #description} (A free text natural language description of the ObservationDefinition from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2499 */ 2500 public MarkdownType getDescriptionElement() { 2501 if (this.description == null) 2502 if (Configuration.errorOnAutoCreate()) 2503 throw new Error("Attempt to auto-create ObservationDefinition.description"); 2504 else if (Configuration.doAutoCreate()) 2505 this.description = new MarkdownType(); // bb 2506 return this.description; 2507 } 2508 2509 public boolean hasDescriptionElement() { 2510 return this.description != null && !this.description.isEmpty(); 2511 } 2512 2513 public boolean hasDescription() { 2514 return this.description != null && !this.description.isEmpty(); 2515 } 2516 2517 /** 2518 * @param value {@link #description} (A free text natural language description of the ObservationDefinition from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2519 */ 2520 public ObservationDefinition setDescriptionElement(MarkdownType value) { 2521 this.description = value; 2522 return this; 2523 } 2524 2525 /** 2526 * @return A free text natural language description of the ObservationDefinition from the consumer's perspective. 2527 */ 2528 public String getDescription() { 2529 return this.description == null ? null : this.description.getValue(); 2530 } 2531 2532 /** 2533 * @param value A free text natural language description of the ObservationDefinition from the consumer's perspective. 2534 */ 2535 public ObservationDefinition setDescription(String value) { 2536 if (Utilities.noString(value)) 2537 this.description = null; 2538 else { 2539 if (this.description == null) 2540 this.description = new MarkdownType(); 2541 this.description.setValue(value); 2542 } 2543 return this; 2544 } 2545 2546 /** 2547 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances.) 2548 */ 2549 public List<UsageContext> getUseContext() { 2550 if (this.useContext == null) 2551 this.useContext = new ArrayList<UsageContext>(); 2552 return this.useContext; 2553 } 2554 2555 /** 2556 * @return Returns a reference to <code>this</code> for easy method chaining 2557 */ 2558 public ObservationDefinition setUseContext(List<UsageContext> theUseContext) { 2559 this.useContext = theUseContext; 2560 return this; 2561 } 2562 2563 public boolean hasUseContext() { 2564 if (this.useContext == null) 2565 return false; 2566 for (UsageContext item : this.useContext) 2567 if (!item.isEmpty()) 2568 return true; 2569 return false; 2570 } 2571 2572 public UsageContext addUseContext() { //3 2573 UsageContext t = new UsageContext(); 2574 if (this.useContext == null) 2575 this.useContext = new ArrayList<UsageContext>(); 2576 this.useContext.add(t); 2577 return t; 2578 } 2579 2580 public ObservationDefinition addUseContext(UsageContext t) { //3 2581 if (t == null) 2582 return this; 2583 if (this.useContext == null) 2584 this.useContext = new ArrayList<UsageContext>(); 2585 this.useContext.add(t); 2586 return this; 2587 } 2588 2589 /** 2590 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2591 */ 2592 public UsageContext getUseContextFirstRep() { 2593 if (getUseContext().isEmpty()) { 2594 addUseContext(); 2595 } 2596 return getUseContext().get(0); 2597 } 2598 2599 /** 2600 * @return {@link #jurisdiction} (A jurisdiction in which the ObservationDefinition is intended to be used.) 2601 */ 2602 public List<CodeableConcept> getJurisdiction() { 2603 if (this.jurisdiction == null) 2604 this.jurisdiction = new ArrayList<CodeableConcept>(); 2605 return this.jurisdiction; 2606 } 2607 2608 /** 2609 * @return Returns a reference to <code>this</code> for easy method chaining 2610 */ 2611 public ObservationDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2612 this.jurisdiction = theJurisdiction; 2613 return this; 2614 } 2615 2616 public boolean hasJurisdiction() { 2617 if (this.jurisdiction == null) 2618 return false; 2619 for (CodeableConcept item : this.jurisdiction) 2620 if (!item.isEmpty()) 2621 return true; 2622 return false; 2623 } 2624 2625 public CodeableConcept addJurisdiction() { //3 2626 CodeableConcept t = new CodeableConcept(); 2627 if (this.jurisdiction == null) 2628 this.jurisdiction = new ArrayList<CodeableConcept>(); 2629 this.jurisdiction.add(t); 2630 return t; 2631 } 2632 2633 public ObservationDefinition addJurisdiction(CodeableConcept t) { //3 2634 if (t == null) 2635 return this; 2636 if (this.jurisdiction == null) 2637 this.jurisdiction = new ArrayList<CodeableConcept>(); 2638 this.jurisdiction.add(t); 2639 return this; 2640 } 2641 2642 /** 2643 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2644 */ 2645 public CodeableConcept getJurisdictionFirstRep() { 2646 if (getJurisdiction().isEmpty()) { 2647 addJurisdiction(); 2648 } 2649 return getJurisdiction().get(0); 2650 } 2651 2652 /** 2653 * @return {@link #purpose} (Explains why this ObservationDefinition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2654 */ 2655 public MarkdownType getPurposeElement() { 2656 if (this.purpose == null) 2657 if (Configuration.errorOnAutoCreate()) 2658 throw new Error("Attempt to auto-create ObservationDefinition.purpose"); 2659 else if (Configuration.doAutoCreate()) 2660 this.purpose = new MarkdownType(); // bb 2661 return this.purpose; 2662 } 2663 2664 public boolean hasPurposeElement() { 2665 return this.purpose != null && !this.purpose.isEmpty(); 2666 } 2667 2668 public boolean hasPurpose() { 2669 return this.purpose != null && !this.purpose.isEmpty(); 2670 } 2671 2672 /** 2673 * @param value {@link #purpose} (Explains why this ObservationDefinition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2674 */ 2675 public ObservationDefinition setPurposeElement(MarkdownType value) { 2676 this.purpose = value; 2677 return this; 2678 } 2679 2680 /** 2681 * @return Explains why this ObservationDefinition is needed and why it has been designed as it has. 2682 */ 2683 public String getPurpose() { 2684 return this.purpose == null ? null : this.purpose.getValue(); 2685 } 2686 2687 /** 2688 * @param value Explains why this ObservationDefinition is needed and why it has been designed as it has. 2689 */ 2690 public ObservationDefinition setPurpose(String value) { 2691 if (Utilities.noString(value)) 2692 this.purpose = null; 2693 else { 2694 if (this.purpose == null) 2695 this.purpose = new MarkdownType(); 2696 this.purpose.setValue(value); 2697 } 2698 return this; 2699 } 2700 2701 /** 2702 * @return {@link #copyright} (Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2703 */ 2704 public MarkdownType getCopyrightElement() { 2705 if (this.copyright == null) 2706 if (Configuration.errorOnAutoCreate()) 2707 throw new Error("Attempt to auto-create ObservationDefinition.copyright"); 2708 else if (Configuration.doAutoCreate()) 2709 this.copyright = new MarkdownType(); // bb 2710 return this.copyright; 2711 } 2712 2713 public boolean hasCopyrightElement() { 2714 return this.copyright != null && !this.copyright.isEmpty(); 2715 } 2716 2717 public boolean hasCopyright() { 2718 return this.copyright != null && !this.copyright.isEmpty(); 2719 } 2720 2721 /** 2722 * @param value {@link #copyright} (Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2723 */ 2724 public ObservationDefinition setCopyrightElement(MarkdownType value) { 2725 this.copyright = value; 2726 return this; 2727 } 2728 2729 /** 2730 * @return Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition. 2731 */ 2732 public String getCopyright() { 2733 return this.copyright == null ? null : this.copyright.getValue(); 2734 } 2735 2736 /** 2737 * @param value Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition. 2738 */ 2739 public ObservationDefinition setCopyright(String value) { 2740 if (Utilities.noString(value)) 2741 this.copyright = null; 2742 else { 2743 if (this.copyright == null) 2744 this.copyright = new MarkdownType(); 2745 this.copyright.setValue(value); 2746 } 2747 return this; 2748 } 2749 2750 /** 2751 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2752 */ 2753 public StringType getCopyrightLabelElement() { 2754 if (this.copyrightLabel == null) 2755 if (Configuration.errorOnAutoCreate()) 2756 throw new Error("Attempt to auto-create ObservationDefinition.copyrightLabel"); 2757 else if (Configuration.doAutoCreate()) 2758 this.copyrightLabel = new StringType(); // bb 2759 return this.copyrightLabel; 2760 } 2761 2762 public boolean hasCopyrightLabelElement() { 2763 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2764 } 2765 2766 public boolean hasCopyrightLabel() { 2767 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2768 } 2769 2770 /** 2771 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2772 */ 2773 public ObservationDefinition setCopyrightLabelElement(StringType value) { 2774 this.copyrightLabel = value; 2775 return this; 2776 } 2777 2778 /** 2779 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2780 */ 2781 public String getCopyrightLabel() { 2782 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2783 } 2784 2785 /** 2786 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2787 */ 2788 public ObservationDefinition setCopyrightLabel(String value) { 2789 if (Utilities.noString(value)) 2790 this.copyrightLabel = null; 2791 else { 2792 if (this.copyrightLabel == null) 2793 this.copyrightLabel = new StringType(); 2794 this.copyrightLabel.setValue(value); 2795 } 2796 return this; 2797 } 2798 2799 /** 2800 * @return {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2801 */ 2802 public DateType getApprovalDateElement() { 2803 if (this.approvalDate == null) 2804 if (Configuration.errorOnAutoCreate()) 2805 throw new Error("Attempt to auto-create ObservationDefinition.approvalDate"); 2806 else if (Configuration.doAutoCreate()) 2807 this.approvalDate = new DateType(); // bb 2808 return this.approvalDate; 2809 } 2810 2811 public boolean hasApprovalDateElement() { 2812 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2813 } 2814 2815 public boolean hasApprovalDate() { 2816 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2817 } 2818 2819 /** 2820 * @param value {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2821 */ 2822 public ObservationDefinition setApprovalDateElement(DateType value) { 2823 this.approvalDate = value; 2824 return this; 2825 } 2826 2827 /** 2828 * @return The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2829 */ 2830 public Date getApprovalDate() { 2831 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2832 } 2833 2834 /** 2835 * @param value The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2836 */ 2837 public ObservationDefinition setApprovalDate(Date value) { 2838 if (value == null) 2839 this.approvalDate = null; 2840 else { 2841 if (this.approvalDate == null) 2842 this.approvalDate = new DateType(); 2843 this.approvalDate.setValue(value); 2844 } 2845 return this; 2846 } 2847 2848 /** 2849 * @return {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2850 */ 2851 public DateType getLastReviewDateElement() { 2852 if (this.lastReviewDate == null) 2853 if (Configuration.errorOnAutoCreate()) 2854 throw new Error("Attempt to auto-create ObservationDefinition.lastReviewDate"); 2855 else if (Configuration.doAutoCreate()) 2856 this.lastReviewDate = new DateType(); // bb 2857 return this.lastReviewDate; 2858 } 2859 2860 public boolean hasLastReviewDateElement() { 2861 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2862 } 2863 2864 public boolean hasLastReviewDate() { 2865 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2866 } 2867 2868 /** 2869 * @param value {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2870 */ 2871 public ObservationDefinition setLastReviewDateElement(DateType value) { 2872 this.lastReviewDate = value; 2873 return this; 2874 } 2875 2876 /** 2877 * @return The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 2878 */ 2879 public Date getLastReviewDate() { 2880 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2881 } 2882 2883 /** 2884 * @param value The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 2885 */ 2886 public ObservationDefinition setLastReviewDate(Date value) { 2887 if (value == null) 2888 this.lastReviewDate = null; 2889 else { 2890 if (this.lastReviewDate == null) 2891 this.lastReviewDate = new DateType(); 2892 this.lastReviewDate.setValue(value); 2893 } 2894 return this; 2895 } 2896 2897 /** 2898 * @return {@link #effectivePeriod} (The period during which the ObservationDefinition content was or is planned to be effective.) 2899 */ 2900 public Period getEffectivePeriod() { 2901 if (this.effectivePeriod == null) 2902 if (Configuration.errorOnAutoCreate()) 2903 throw new Error("Attempt to auto-create ObservationDefinition.effectivePeriod"); 2904 else if (Configuration.doAutoCreate()) 2905 this.effectivePeriod = new Period(); // cc 2906 return this.effectivePeriod; 2907 } 2908 2909 public boolean hasEffectivePeriod() { 2910 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2911 } 2912 2913 /** 2914 * @param value {@link #effectivePeriod} (The period during which the ObservationDefinition content was or is planned to be effective.) 2915 */ 2916 public ObservationDefinition setEffectivePeriod(Period value) { 2917 this.effectivePeriod = value; 2918 return this; 2919 } 2920 2921 /** 2922 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 2923 */ 2924 public List<CanonicalType> getDerivedFromCanonical() { 2925 if (this.derivedFromCanonical == null) 2926 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 2927 return this.derivedFromCanonical; 2928 } 2929 2930 /** 2931 * @return Returns a reference to <code>this</code> for easy method chaining 2932 */ 2933 public ObservationDefinition setDerivedFromCanonical(List<CanonicalType> theDerivedFromCanonical) { 2934 this.derivedFromCanonical = theDerivedFromCanonical; 2935 return this; 2936 } 2937 2938 public boolean hasDerivedFromCanonical() { 2939 if (this.derivedFromCanonical == null) 2940 return false; 2941 for (CanonicalType item : this.derivedFromCanonical) 2942 if (!item.isEmpty()) 2943 return true; 2944 return false; 2945 } 2946 2947 /** 2948 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 2949 */ 2950 public CanonicalType addDerivedFromCanonicalElement() {//2 2951 CanonicalType t = new CanonicalType(); 2952 if (this.derivedFromCanonical == null) 2953 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 2954 this.derivedFromCanonical.add(t); 2955 return t; 2956 } 2957 2958 /** 2959 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 2960 */ 2961 public ObservationDefinition addDerivedFromCanonical(String value) { //1 2962 CanonicalType t = new CanonicalType(); 2963 t.setValue(value); 2964 if (this.derivedFromCanonical == null) 2965 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 2966 this.derivedFromCanonical.add(t); 2967 return this; 2968 } 2969 2970 /** 2971 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 2972 */ 2973 public boolean hasDerivedFromCanonical(String value) { 2974 if (this.derivedFromCanonical == null) 2975 return false; 2976 for (CanonicalType v : this.derivedFromCanonical) 2977 if (v.getValue().equals(value)) // canonical 2978 return true; 2979 return false; 2980 } 2981 2982 /** 2983 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 2984 */ 2985 public List<UriType> getDerivedFromUri() { 2986 if (this.derivedFromUri == null) 2987 this.derivedFromUri = new ArrayList<UriType>(); 2988 return this.derivedFromUri; 2989 } 2990 2991 /** 2992 * @return Returns a reference to <code>this</code> for easy method chaining 2993 */ 2994 public ObservationDefinition setDerivedFromUri(List<UriType> theDerivedFromUri) { 2995 this.derivedFromUri = theDerivedFromUri; 2996 return this; 2997 } 2998 2999 public boolean hasDerivedFromUri() { 3000 if (this.derivedFromUri == null) 3001 return false; 3002 for (UriType item : this.derivedFromUri) 3003 if (!item.isEmpty()) 3004 return true; 3005 return false; 3006 } 3007 3008 /** 3009 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 3010 */ 3011 public UriType addDerivedFromUriElement() {//2 3012 UriType t = new UriType(); 3013 if (this.derivedFromUri == null) 3014 this.derivedFromUri = new ArrayList<UriType>(); 3015 this.derivedFromUri.add(t); 3016 return t; 3017 } 3018 3019 /** 3020 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 3021 */ 3022 public ObservationDefinition addDerivedFromUri(String value) { //1 3023 UriType t = new UriType(); 3024 t.setValue(value); 3025 if (this.derivedFromUri == null) 3026 this.derivedFromUri = new ArrayList<UriType>(); 3027 this.derivedFromUri.add(t); 3028 return this; 3029 } 3030 3031 /** 3032 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 3033 */ 3034 public boolean hasDerivedFromUri(String value) { 3035 if (this.derivedFromUri == null) 3036 return false; 3037 for (UriType v : this.derivedFromUri) 3038 if (v.getValue().equals(value)) // uri 3039 return true; 3040 return false; 3041 } 3042 3043 /** 3044 * @return {@link #subject} (A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition.) 3045 */ 3046 public List<CodeableConcept> getSubject() { 3047 if (this.subject == null) 3048 this.subject = new ArrayList<CodeableConcept>(); 3049 return this.subject; 3050 } 3051 3052 /** 3053 * @return Returns a reference to <code>this</code> for easy method chaining 3054 */ 3055 public ObservationDefinition setSubject(List<CodeableConcept> theSubject) { 3056 this.subject = theSubject; 3057 return this; 3058 } 3059 3060 public boolean hasSubject() { 3061 if (this.subject == null) 3062 return false; 3063 for (CodeableConcept item : this.subject) 3064 if (!item.isEmpty()) 3065 return true; 3066 return false; 3067 } 3068 3069 public CodeableConcept addSubject() { //3 3070 CodeableConcept t = new CodeableConcept(); 3071 if (this.subject == null) 3072 this.subject = new ArrayList<CodeableConcept>(); 3073 this.subject.add(t); 3074 return t; 3075 } 3076 3077 public ObservationDefinition addSubject(CodeableConcept t) { //3 3078 if (t == null) 3079 return this; 3080 if (this.subject == null) 3081 this.subject = new ArrayList<CodeableConcept>(); 3082 this.subject.add(t); 3083 return this; 3084 } 3085 3086 /** 3087 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 3088 */ 3089 public CodeableConcept getSubjectFirstRep() { 3090 if (getSubject().isEmpty()) { 3091 addSubject(); 3092 } 3093 return getSubject().get(0); 3094 } 3095 3096 /** 3097 * @return {@link #performerType} (The type of individual/organization/device that is expected to act upon instances of this definition.) 3098 */ 3099 public CodeableConcept getPerformerType() { 3100 if (this.performerType == null) 3101 if (Configuration.errorOnAutoCreate()) 3102 throw new Error("Attempt to auto-create ObservationDefinition.performerType"); 3103 else if (Configuration.doAutoCreate()) 3104 this.performerType = new CodeableConcept(); // cc 3105 return this.performerType; 3106 } 3107 3108 public boolean hasPerformerType() { 3109 return this.performerType != null && !this.performerType.isEmpty(); 3110 } 3111 3112 /** 3113 * @param value {@link #performerType} (The type of individual/organization/device that is expected to act upon instances of this definition.) 3114 */ 3115 public ObservationDefinition setPerformerType(CodeableConcept value) { 3116 this.performerType = value; 3117 return this; 3118 } 3119 3120 /** 3121 * @return {@link #category} (A code that classifies the general type of observation.) 3122 */ 3123 public List<CodeableConcept> getCategory() { 3124 if (this.category == null) 3125 this.category = new ArrayList<CodeableConcept>(); 3126 return this.category; 3127 } 3128 3129 /** 3130 * @return Returns a reference to <code>this</code> for easy method chaining 3131 */ 3132 public ObservationDefinition setCategory(List<CodeableConcept> theCategory) { 3133 this.category = theCategory; 3134 return this; 3135 } 3136 3137 public boolean hasCategory() { 3138 if (this.category == null) 3139 return false; 3140 for (CodeableConcept item : this.category) 3141 if (!item.isEmpty()) 3142 return true; 3143 return false; 3144 } 3145 3146 public CodeableConcept addCategory() { //3 3147 CodeableConcept t = new CodeableConcept(); 3148 if (this.category == null) 3149 this.category = new ArrayList<CodeableConcept>(); 3150 this.category.add(t); 3151 return t; 3152 } 3153 3154 public ObservationDefinition addCategory(CodeableConcept t) { //3 3155 if (t == null) 3156 return this; 3157 if (this.category == null) 3158 this.category = new ArrayList<CodeableConcept>(); 3159 this.category.add(t); 3160 return this; 3161 } 3162 3163 /** 3164 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 3165 */ 3166 public CodeableConcept getCategoryFirstRep() { 3167 if (getCategory().isEmpty()) { 3168 addCategory(); 3169 } 3170 return getCategory().get(0); 3171 } 3172 3173 /** 3174 * @return {@link #code} (Describes what will be observed. Sometimes this is called the observation "name".) 3175 */ 3176 public CodeableConcept getCode() { 3177 if (this.code == null) 3178 if (Configuration.errorOnAutoCreate()) 3179 throw new Error("Attempt to auto-create ObservationDefinition.code"); 3180 else if (Configuration.doAutoCreate()) 3181 this.code = new CodeableConcept(); // cc 3182 return this.code; 3183 } 3184 3185 public boolean hasCode() { 3186 return this.code != null && !this.code.isEmpty(); 3187 } 3188 3189 /** 3190 * @param value {@link #code} (Describes what will be observed. Sometimes this is called the observation "name".) 3191 */ 3192 public ObservationDefinition setCode(CodeableConcept value) { 3193 this.code = value; 3194 return this; 3195 } 3196 3197 /** 3198 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3199 */ 3200 public List<Enumeration<ObservationDataType>> getPermittedDataType() { 3201 if (this.permittedDataType == null) 3202 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 3203 return this.permittedDataType; 3204 } 3205 3206 /** 3207 * @return Returns a reference to <code>this</code> for easy method chaining 3208 */ 3209 public ObservationDefinition setPermittedDataType(List<Enumeration<ObservationDataType>> thePermittedDataType) { 3210 this.permittedDataType = thePermittedDataType; 3211 return this; 3212 } 3213 3214 public boolean hasPermittedDataType() { 3215 if (this.permittedDataType == null) 3216 return false; 3217 for (Enumeration<ObservationDataType> item : this.permittedDataType) 3218 if (!item.isEmpty()) 3219 return true; 3220 return false; 3221 } 3222 3223 /** 3224 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3225 */ 3226 public Enumeration<ObservationDataType> addPermittedDataTypeElement() {//2 3227 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 3228 if (this.permittedDataType == null) 3229 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 3230 this.permittedDataType.add(t); 3231 return t; 3232 } 3233 3234 /** 3235 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3236 */ 3237 public ObservationDefinition addPermittedDataType(ObservationDataType value) { //1 3238 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 3239 t.setValue(value); 3240 if (this.permittedDataType == null) 3241 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 3242 this.permittedDataType.add(t); 3243 return this; 3244 } 3245 3246 /** 3247 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3248 */ 3249 public boolean hasPermittedDataType(ObservationDataType value) { 3250 if (this.permittedDataType == null) 3251 return false; 3252 for (Enumeration<ObservationDataType> v : this.permittedDataType) 3253 if (v.getValue().equals(value)) // code 3254 return true; 3255 return false; 3256 } 3257 3258 /** 3259 * @return {@link #multipleResultsAllowed} (Multiple results allowed for observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getMultipleResultsAllowed" gives direct access to the value 3260 */ 3261 public BooleanType getMultipleResultsAllowedElement() { 3262 if (this.multipleResultsAllowed == null) 3263 if (Configuration.errorOnAutoCreate()) 3264 throw new Error("Attempt to auto-create ObservationDefinition.multipleResultsAllowed"); 3265 else if (Configuration.doAutoCreate()) 3266 this.multipleResultsAllowed = new BooleanType(); // bb 3267 return this.multipleResultsAllowed; 3268 } 3269 3270 public boolean hasMultipleResultsAllowedElement() { 3271 return this.multipleResultsAllowed != null && !this.multipleResultsAllowed.isEmpty(); 3272 } 3273 3274 public boolean hasMultipleResultsAllowed() { 3275 return this.multipleResultsAllowed != null && !this.multipleResultsAllowed.isEmpty(); 3276 } 3277 3278 /** 3279 * @param value {@link #multipleResultsAllowed} (Multiple results allowed for observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getMultipleResultsAllowed" gives direct access to the value 3280 */ 3281 public ObservationDefinition setMultipleResultsAllowedElement(BooleanType value) { 3282 this.multipleResultsAllowed = value; 3283 return this; 3284 } 3285 3286 /** 3287 * @return Multiple results allowed for observations conforming to this ObservationDefinition. 3288 */ 3289 public boolean getMultipleResultsAllowed() { 3290 return this.multipleResultsAllowed == null || this.multipleResultsAllowed.isEmpty() ? false : this.multipleResultsAllowed.getValue(); 3291 } 3292 3293 /** 3294 * @param value Multiple results allowed for observations conforming to this ObservationDefinition. 3295 */ 3296 public ObservationDefinition setMultipleResultsAllowed(boolean value) { 3297 if (this.multipleResultsAllowed == null) 3298 this.multipleResultsAllowed = new BooleanType(); 3299 this.multipleResultsAllowed.setValue(value); 3300 return this; 3301 } 3302 3303 /** 3304 * @return {@link #bodySite} (The site on the subject's body where the observation is to be made.) 3305 */ 3306 public CodeableConcept getBodySite() { 3307 if (this.bodySite == null) 3308 if (Configuration.errorOnAutoCreate()) 3309 throw new Error("Attempt to auto-create ObservationDefinition.bodySite"); 3310 else if (Configuration.doAutoCreate()) 3311 this.bodySite = new CodeableConcept(); // cc 3312 return this.bodySite; 3313 } 3314 3315 public boolean hasBodySite() { 3316 return this.bodySite != null && !this.bodySite.isEmpty(); 3317 } 3318 3319 /** 3320 * @param value {@link #bodySite} (The site on the subject's body where the observation is to be made.) 3321 */ 3322 public ObservationDefinition setBodySite(CodeableConcept value) { 3323 this.bodySite = value; 3324 return this; 3325 } 3326 3327 /** 3328 * @return {@link #method} (The method or technique used to perform the observation.) 3329 */ 3330 public CodeableConcept getMethod() { 3331 if (this.method == null) 3332 if (Configuration.errorOnAutoCreate()) 3333 throw new Error("Attempt to auto-create ObservationDefinition.method"); 3334 else if (Configuration.doAutoCreate()) 3335 this.method = new CodeableConcept(); // cc 3336 return this.method; 3337 } 3338 3339 public boolean hasMethod() { 3340 return this.method != null && !this.method.isEmpty(); 3341 } 3342 3343 /** 3344 * @param value {@link #method} (The method or technique used to perform the observation.) 3345 */ 3346 public ObservationDefinition setMethod(CodeableConcept value) { 3347 this.method = value; 3348 return this; 3349 } 3350 3351 /** 3352 * @return {@link #specimen} (The kind of specimen that this type of observation is produced on.) 3353 */ 3354 public List<Reference> getSpecimen() { 3355 if (this.specimen == null) 3356 this.specimen = new ArrayList<Reference>(); 3357 return this.specimen; 3358 } 3359 3360 /** 3361 * @return Returns a reference to <code>this</code> for easy method chaining 3362 */ 3363 public ObservationDefinition setSpecimen(List<Reference> theSpecimen) { 3364 this.specimen = theSpecimen; 3365 return this; 3366 } 3367 3368 public boolean hasSpecimen() { 3369 if (this.specimen == null) 3370 return false; 3371 for (Reference item : this.specimen) 3372 if (!item.isEmpty()) 3373 return true; 3374 return false; 3375 } 3376 3377 public Reference addSpecimen() { //3 3378 Reference t = new Reference(); 3379 if (this.specimen == null) 3380 this.specimen = new ArrayList<Reference>(); 3381 this.specimen.add(t); 3382 return t; 3383 } 3384 3385 public ObservationDefinition addSpecimen(Reference t) { //3 3386 if (t == null) 3387 return this; 3388 if (this.specimen == null) 3389 this.specimen = new ArrayList<Reference>(); 3390 this.specimen.add(t); 3391 return this; 3392 } 3393 3394 /** 3395 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist {3} 3396 */ 3397 public Reference getSpecimenFirstRep() { 3398 if (getSpecimen().isEmpty()) { 3399 addSpecimen(); 3400 } 3401 return getSpecimen().get(0); 3402 } 3403 3404 /** 3405 * @return {@link #device} (The measurement model of device or actual device used to produce observations of this type.) 3406 */ 3407 public List<Reference> getDevice() { 3408 if (this.device == null) 3409 this.device = new ArrayList<Reference>(); 3410 return this.device; 3411 } 3412 3413 /** 3414 * @return Returns a reference to <code>this</code> for easy method chaining 3415 */ 3416 public ObservationDefinition setDevice(List<Reference> theDevice) { 3417 this.device = theDevice; 3418 return this; 3419 } 3420 3421 public boolean hasDevice() { 3422 if (this.device == null) 3423 return false; 3424 for (Reference item : this.device) 3425 if (!item.isEmpty()) 3426 return true; 3427 return false; 3428 } 3429 3430 public Reference addDevice() { //3 3431 Reference t = new Reference(); 3432 if (this.device == null) 3433 this.device = new ArrayList<Reference>(); 3434 this.device.add(t); 3435 return t; 3436 } 3437 3438 public ObservationDefinition addDevice(Reference t) { //3 3439 if (t == null) 3440 return this; 3441 if (this.device == null) 3442 this.device = new ArrayList<Reference>(); 3443 this.device.add(t); 3444 return this; 3445 } 3446 3447 /** 3448 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 3449 */ 3450 public Reference getDeviceFirstRep() { 3451 if (getDevice().isEmpty()) { 3452 addDevice(); 3453 } 3454 return getDevice().get(0); 3455 } 3456 3457 /** 3458 * @return {@link #preferredReportName} (The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getPreferredReportName" gives direct access to the value 3459 */ 3460 public StringType getPreferredReportNameElement() { 3461 if (this.preferredReportName == null) 3462 if (Configuration.errorOnAutoCreate()) 3463 throw new Error("Attempt to auto-create ObservationDefinition.preferredReportName"); 3464 else if (Configuration.doAutoCreate()) 3465 this.preferredReportName = new StringType(); // bb 3466 return this.preferredReportName; 3467 } 3468 3469 public boolean hasPreferredReportNameElement() { 3470 return this.preferredReportName != null && !this.preferredReportName.isEmpty(); 3471 } 3472 3473 public boolean hasPreferredReportName() { 3474 return this.preferredReportName != null && !this.preferredReportName.isEmpty(); 3475 } 3476 3477 /** 3478 * @param value {@link #preferredReportName} (The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getPreferredReportName" gives direct access to the value 3479 */ 3480 public ObservationDefinition setPreferredReportNameElement(StringType value) { 3481 this.preferredReportName = value; 3482 return this; 3483 } 3484 3485 /** 3486 * @return The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition. 3487 */ 3488 public String getPreferredReportName() { 3489 return this.preferredReportName == null ? null : this.preferredReportName.getValue(); 3490 } 3491 3492 /** 3493 * @param value The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition. 3494 */ 3495 public ObservationDefinition setPreferredReportName(String value) { 3496 if (Utilities.noString(value)) 3497 this.preferredReportName = null; 3498 else { 3499 if (this.preferredReportName == null) 3500 this.preferredReportName = new StringType(); 3501 this.preferredReportName.setValue(value); 3502 } 3503 return this; 3504 } 3505 3506 /** 3507 * @return {@link #permittedUnit} (Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.) 3508 */ 3509 public List<Coding> getPermittedUnit() { 3510 if (this.permittedUnit == null) 3511 this.permittedUnit = new ArrayList<Coding>(); 3512 return this.permittedUnit; 3513 } 3514 3515 /** 3516 * @return Returns a reference to <code>this</code> for easy method chaining 3517 */ 3518 public ObservationDefinition setPermittedUnit(List<Coding> thePermittedUnit) { 3519 this.permittedUnit = thePermittedUnit; 3520 return this; 3521 } 3522 3523 public boolean hasPermittedUnit() { 3524 if (this.permittedUnit == null) 3525 return false; 3526 for (Coding item : this.permittedUnit) 3527 if (!item.isEmpty()) 3528 return true; 3529 return false; 3530 } 3531 3532 public Coding addPermittedUnit() { //3 3533 Coding t = new Coding(); 3534 if (this.permittedUnit == null) 3535 this.permittedUnit = new ArrayList<Coding>(); 3536 this.permittedUnit.add(t); 3537 return t; 3538 } 3539 3540 public ObservationDefinition addPermittedUnit(Coding t) { //3 3541 if (t == null) 3542 return this; 3543 if (this.permittedUnit == null) 3544 this.permittedUnit = new ArrayList<Coding>(); 3545 this.permittedUnit.add(t); 3546 return this; 3547 } 3548 3549 /** 3550 * @return The first repetition of repeating field {@link #permittedUnit}, creating it if it does not already exist {3} 3551 */ 3552 public Coding getPermittedUnitFirstRep() { 3553 if (getPermittedUnit().isEmpty()) { 3554 addPermittedUnit(); 3555 } 3556 return getPermittedUnit().get(0); 3557 } 3558 3559 /** 3560 * @return {@link #qualifiedValue} (A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.) 3561 */ 3562 public List<ObservationDefinitionQualifiedValueComponent> getQualifiedValue() { 3563 if (this.qualifiedValue == null) 3564 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 3565 return this.qualifiedValue; 3566 } 3567 3568 /** 3569 * @return Returns a reference to <code>this</code> for easy method chaining 3570 */ 3571 public ObservationDefinition setQualifiedValue(List<ObservationDefinitionQualifiedValueComponent> theQualifiedValue) { 3572 this.qualifiedValue = theQualifiedValue; 3573 return this; 3574 } 3575 3576 public boolean hasQualifiedValue() { 3577 if (this.qualifiedValue == null) 3578 return false; 3579 for (ObservationDefinitionQualifiedValueComponent item : this.qualifiedValue) 3580 if (!item.isEmpty()) 3581 return true; 3582 return false; 3583 } 3584 3585 public ObservationDefinitionQualifiedValueComponent addQualifiedValue() { //3 3586 ObservationDefinitionQualifiedValueComponent t = new ObservationDefinitionQualifiedValueComponent(); 3587 if (this.qualifiedValue == null) 3588 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 3589 this.qualifiedValue.add(t); 3590 return t; 3591 } 3592 3593 public ObservationDefinition addQualifiedValue(ObservationDefinitionQualifiedValueComponent t) { //3 3594 if (t == null) 3595 return this; 3596 if (this.qualifiedValue == null) 3597 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 3598 this.qualifiedValue.add(t); 3599 return this; 3600 } 3601 3602 /** 3603 * @return The first repetition of repeating field {@link #qualifiedValue}, creating it if it does not already exist {3} 3604 */ 3605 public ObservationDefinitionQualifiedValueComponent getQualifiedValueFirstRep() { 3606 if (getQualifiedValue().isEmpty()) { 3607 addQualifiedValue(); 3608 } 3609 return getQualifiedValue().get(0); 3610 } 3611 3612 /** 3613 * @return {@link #hasMember} (This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.) 3614 */ 3615 public List<Reference> getHasMember() { 3616 if (this.hasMember == null) 3617 this.hasMember = new ArrayList<Reference>(); 3618 return this.hasMember; 3619 } 3620 3621 /** 3622 * @return Returns a reference to <code>this</code> for easy method chaining 3623 */ 3624 public ObservationDefinition setHasMember(List<Reference> theHasMember) { 3625 this.hasMember = theHasMember; 3626 return this; 3627 } 3628 3629 public boolean hasHasMember() { 3630 if (this.hasMember == null) 3631 return false; 3632 for (Reference item : this.hasMember) 3633 if (!item.isEmpty()) 3634 return true; 3635 return false; 3636 } 3637 3638 public Reference addHasMember() { //3 3639 Reference t = new Reference(); 3640 if (this.hasMember == null) 3641 this.hasMember = new ArrayList<Reference>(); 3642 this.hasMember.add(t); 3643 return t; 3644 } 3645 3646 public ObservationDefinition addHasMember(Reference t) { //3 3647 if (t == null) 3648 return this; 3649 if (this.hasMember == null) 3650 this.hasMember = new ArrayList<Reference>(); 3651 this.hasMember.add(t); 3652 return this; 3653 } 3654 3655 /** 3656 * @return The first repetition of repeating field {@link #hasMember}, creating it if it does not already exist {3} 3657 */ 3658 public Reference getHasMemberFirstRep() { 3659 if (getHasMember().isEmpty()) { 3660 addHasMember(); 3661 } 3662 return getHasMember().get(0); 3663 } 3664 3665 /** 3666 * @return {@link #component} (Some observations have multiple component observations, expressed as separate code value pairs.) 3667 */ 3668 public List<ObservationDefinitionComponentComponent> getComponent() { 3669 if (this.component == null) 3670 this.component = new ArrayList<ObservationDefinitionComponentComponent>(); 3671 return this.component; 3672 } 3673 3674 /** 3675 * @return Returns a reference to <code>this</code> for easy method chaining 3676 */ 3677 public ObservationDefinition setComponent(List<ObservationDefinitionComponentComponent> theComponent) { 3678 this.component = theComponent; 3679 return this; 3680 } 3681 3682 public boolean hasComponent() { 3683 if (this.component == null) 3684 return false; 3685 for (ObservationDefinitionComponentComponent item : this.component) 3686 if (!item.isEmpty()) 3687 return true; 3688 return false; 3689 } 3690 3691 public ObservationDefinitionComponentComponent addComponent() { //3 3692 ObservationDefinitionComponentComponent t = new ObservationDefinitionComponentComponent(); 3693 if (this.component == null) 3694 this.component = new ArrayList<ObservationDefinitionComponentComponent>(); 3695 this.component.add(t); 3696 return t; 3697 } 3698 3699 public ObservationDefinition addComponent(ObservationDefinitionComponentComponent t) { //3 3700 if (t == null) 3701 return this; 3702 if (this.component == null) 3703 this.component = new ArrayList<ObservationDefinitionComponentComponent>(); 3704 this.component.add(t); 3705 return this; 3706 } 3707 3708 /** 3709 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 3710 */ 3711 public ObservationDefinitionComponentComponent getComponentFirstRep() { 3712 if (getComponent().isEmpty()) { 3713 addComponent(); 3714 } 3715 return getComponent().get(0); 3716 } 3717 3718 protected void listChildren(List<Property> children) { 3719 super.listChildren(children); 3720 children.add(new Property("url", "uri", "An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.", 0, 1, url)); 3721 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, 1, identifier)); 3722 children.add(new Property("version", "string", "The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version)); 3723 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3724 children.add(new Property("name", "string", "A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3725 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the ObservationDefinition.", 0, 1, title)); 3726 children.add(new Property("status", "code", "The current state of the ObservationDefinition.", 0, 1, status)); 3727 children.add(new Property("experimental", "boolean", "A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3728 children.add(new Property("date", "dateTime", "The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.", 0, 1, date)); 3729 children.add(new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the ObservationDefinition. May also allow for contact.", 0, 1, publisher)); 3730 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3731 children.add(new Property("description", "markdown", "A free text natural language description of the ObservationDefinition from the consumer's perspective.", 0, 1, description)); 3732 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3733 children.add(new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the ObservationDefinition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3734 children.add(new Property("purpose", "markdown", "Explains why this ObservationDefinition is needed and why it has been designed as it has.", 0, 1, purpose)); 3735 children.add(new Property("copyright", "markdown", "Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.", 0, 1, copyright)); 3736 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3737 children.add(new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3738 children.add(new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 3739 children.add(new Property("effectivePeriod", "Period", "The period during which the ObservationDefinition content was or is planned to be effective.", 0, 1, effectivePeriod)); 3740 children.add(new Property("derivedFromCanonical", "canonical(ObservationDefinition)", "The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromCanonical)); 3741 children.add(new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri)); 3742 children.add(new Property("subject", "CodeableConcept", "A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, subject)); 3743 children.add(new Property("performerType", "CodeableConcept", "The type of individual/organization/device that is expected to act upon instances of this definition.", 0, 1, performerType)); 3744 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of observation.", 0, java.lang.Integer.MAX_VALUE, category)); 3745 children.add(new Property("code", "CodeableConcept", "Describes what will be observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 3746 children.add(new Property("permittedDataType", "code", "The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedDataType)); 3747 children.add(new Property("multipleResultsAllowed", "boolean", "Multiple results allowed for observations conforming to this ObservationDefinition.", 0, 1, multipleResultsAllowed)); 3748 children.add(new Property("bodySite", "CodeableConcept", "The site on the subject's body where the observation is to be made.", 0, 1, bodySite)); 3749 children.add(new Property("method", "CodeableConcept", "The method or technique used to perform the observation.", 0, 1, method)); 3750 children.add(new Property("specimen", "Reference(SpecimenDefinition)", "The kind of specimen that this type of observation is produced on.", 0, java.lang.Integer.MAX_VALUE, specimen)); 3751 children.add(new Property("device", "Reference(DeviceDefinition|Device)", "The measurement model of device or actual device used to produce observations of this type.", 0, java.lang.Integer.MAX_VALUE, device)); 3752 children.add(new Property("preferredReportName", "string", "The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.", 0, 1, preferredReportName)); 3753 children.add(new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit)); 3754 children.add(new Property("qualifiedValue", "", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue)); 3755 children.add(new Property("hasMember", "Reference(ObservationDefinition|Questionnaire)", "This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember)); 3756 children.add(new Property("component", "", "Some observations have multiple component observations, expressed as separate code value pairs.", 0, java.lang.Integer.MAX_VALUE, component)); 3757 } 3758 3759 @Override 3760 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3761 switch (_hash) { 3762 case 116079: /*url*/ return new Property("url", "uri", "An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.", 0, 1, url); 3763 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, 1, identifier); 3764 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version); 3765 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3766 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3767 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3768 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3769 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3770 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the ObservationDefinition.", 0, 1, title); 3771 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ObservationDefinition.", 0, 1, status); 3772 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3773 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.", 0, 1, date); 3774 case 1447404028: /*publisher*/ return new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the ObservationDefinition. May also allow for contact.", 0, 1, publisher); 3775 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3776 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the ObservationDefinition from the consumer's perspective.", 0, 1, description); 3777 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3778 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the ObservationDefinition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3779 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explains why this ObservationDefinition is needed and why it has been designed as it has.", 0, 1, purpose); 3780 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.", 0, 1, copyright); 3781 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3782 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 3783 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate); 3784 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the ObservationDefinition content was or is planned to be effective.", 0, 1, effectivePeriod); 3785 case -978133683: /*derivedFromCanonical*/ return new Property("derivedFromCanonical", "canonical(ObservationDefinition)", "The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromCanonical); 3786 case -1076333435: /*derivedFromUri*/ return new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri); 3787 case -1867885268: /*subject*/ return new Property("subject", "CodeableConcept", "A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, subject); 3788 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "The type of individual/organization/device that is expected to act upon instances of this definition.", 0, 1, performerType); 3789 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of observation.", 0, java.lang.Integer.MAX_VALUE, category); 3790 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what will be observed. Sometimes this is called the observation \"name\".", 0, 1, code); 3791 case -99492804: /*permittedDataType*/ return new Property("permittedDataType", "code", "The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedDataType); 3792 case -2102414590: /*multipleResultsAllowed*/ return new Property("multipleResultsAllowed", "boolean", "Multiple results allowed for observations conforming to this ObservationDefinition.", 0, 1, multipleResultsAllowed); 3793 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "The site on the subject's body where the observation is to be made.", 0, 1, bodySite); 3794 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "The method or technique used to perform the observation.", 0, 1, method); 3795 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(SpecimenDefinition)", "The kind of specimen that this type of observation is produced on.", 0, java.lang.Integer.MAX_VALUE, specimen); 3796 case -1335157162: /*device*/ return new Property("device", "Reference(DeviceDefinition|Device)", "The measurement model of device or actual device used to produce observations of this type.", 0, java.lang.Integer.MAX_VALUE, device); 3797 case -1851030208: /*preferredReportName*/ return new Property("preferredReportName", "string", "The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.", 0, 1, preferredReportName); 3798 case 1073054652: /*permittedUnit*/ return new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit); 3799 case -558517707: /*qualifiedValue*/ return new Property("qualifiedValue", "", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue); 3800 case -458019372: /*hasMember*/ return new Property("hasMember", "Reference(ObservationDefinition|Questionnaire)", "This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember); 3801 case -1399907075: /*component*/ return new Property("component", "", "Some observations have multiple component observations, expressed as separate code value pairs.", 0, java.lang.Integer.MAX_VALUE, component); 3802 default: return super.getNamedProperty(_hash, _name, _checkValid); 3803 } 3804 3805 } 3806 3807 @Override 3808 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3809 switch (hash) { 3810 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3811 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3812 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3813 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3814 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3815 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3816 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3817 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3818 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3819 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3820 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3821 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3822 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3823 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3824 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3825 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3826 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3827 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 3828 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 3829 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 3830 case -978133683: /*derivedFromCanonical*/ return this.derivedFromCanonical == null ? new Base[0] : this.derivedFromCanonical.toArray(new Base[this.derivedFromCanonical.size()]); // CanonicalType 3831 case -1076333435: /*derivedFromUri*/ return this.derivedFromUri == null ? new Base[0] : this.derivedFromUri.toArray(new Base[this.derivedFromUri.size()]); // UriType 3832 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // CodeableConcept 3833 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 3834 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3835 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3836 case -99492804: /*permittedDataType*/ return this.permittedDataType == null ? new Base[0] : this.permittedDataType.toArray(new Base[this.permittedDataType.size()]); // Enumeration<ObservationDataType> 3837 case -2102414590: /*multipleResultsAllowed*/ return this.multipleResultsAllowed == null ? new Base[0] : new Base[] {this.multipleResultsAllowed}; // BooleanType 3838 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 3839 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 3840 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 3841 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 3842 case -1851030208: /*preferredReportName*/ return this.preferredReportName == null ? new Base[0] : new Base[] {this.preferredReportName}; // StringType 3843 case 1073054652: /*permittedUnit*/ return this.permittedUnit == null ? new Base[0] : this.permittedUnit.toArray(new Base[this.permittedUnit.size()]); // Coding 3844 case -558517707: /*qualifiedValue*/ return this.qualifiedValue == null ? new Base[0] : this.qualifiedValue.toArray(new Base[this.qualifiedValue.size()]); // ObservationDefinitionQualifiedValueComponent 3845 case -458019372: /*hasMember*/ return this.hasMember == null ? new Base[0] : this.hasMember.toArray(new Base[this.hasMember.size()]); // Reference 3846 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ObservationDefinitionComponentComponent 3847 default: return super.getProperty(hash, name, checkValid); 3848 } 3849 3850 } 3851 3852 @Override 3853 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3854 switch (hash) { 3855 case 116079: // url 3856 this.url = TypeConvertor.castToUri(value); // UriType 3857 return value; 3858 case -1618432855: // identifier 3859 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3860 return value; 3861 case 351608024: // version 3862 this.version = TypeConvertor.castToString(value); // StringType 3863 return value; 3864 case 1508158071: // versionAlgorithm 3865 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3866 return value; 3867 case 3373707: // name 3868 this.name = TypeConvertor.castToString(value); // StringType 3869 return value; 3870 case 110371416: // title 3871 this.title = TypeConvertor.castToString(value); // StringType 3872 return value; 3873 case -892481550: // status 3874 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3875 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3876 return value; 3877 case -404562712: // experimental 3878 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3879 return value; 3880 case 3076014: // date 3881 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3882 return value; 3883 case 1447404028: // publisher 3884 this.publisher = TypeConvertor.castToString(value); // StringType 3885 return value; 3886 case 951526432: // contact 3887 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3888 return value; 3889 case -1724546052: // description 3890 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3891 return value; 3892 case -669707736: // useContext 3893 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3894 return value; 3895 case -507075711: // jurisdiction 3896 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3897 return value; 3898 case -220463842: // purpose 3899 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3900 return value; 3901 case 1522889671: // copyright 3902 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3903 return value; 3904 case 765157229: // copyrightLabel 3905 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3906 return value; 3907 case 223539345: // approvalDate 3908 this.approvalDate = TypeConvertor.castToDate(value); // DateType 3909 return value; 3910 case -1687512484: // lastReviewDate 3911 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 3912 return value; 3913 case -403934648: // effectivePeriod 3914 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 3915 return value; 3916 case -978133683: // derivedFromCanonical 3917 this.getDerivedFromCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 3918 return value; 3919 case -1076333435: // derivedFromUri 3920 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); // UriType 3921 return value; 3922 case -1867885268: // subject 3923 this.getSubject().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3924 return value; 3925 case -901444568: // performerType 3926 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3927 return value; 3928 case 50511102: // category 3929 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3930 return value; 3931 case 3059181: // code 3932 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3933 return value; 3934 case -99492804: // permittedDataType 3935 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3936 this.getPermittedDataType().add((Enumeration) value); // Enumeration<ObservationDataType> 3937 return value; 3938 case -2102414590: // multipleResultsAllowed 3939 this.multipleResultsAllowed = TypeConvertor.castToBoolean(value); // BooleanType 3940 return value; 3941 case 1702620169: // bodySite 3942 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3943 return value; 3944 case -1077554975: // method 3945 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3946 return value; 3947 case -2132868344: // specimen 3948 this.getSpecimen().add(TypeConvertor.castToReference(value)); // Reference 3949 return value; 3950 case -1335157162: // device 3951 this.getDevice().add(TypeConvertor.castToReference(value)); // Reference 3952 return value; 3953 case -1851030208: // preferredReportName 3954 this.preferredReportName = TypeConvertor.castToString(value); // StringType 3955 return value; 3956 case 1073054652: // permittedUnit 3957 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); // Coding 3958 return value; 3959 case -558517707: // qualifiedValue 3960 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); // ObservationDefinitionQualifiedValueComponent 3961 return value; 3962 case -458019372: // hasMember 3963 this.getHasMember().add(TypeConvertor.castToReference(value)); // Reference 3964 return value; 3965 case -1399907075: // component 3966 this.getComponent().add((ObservationDefinitionComponentComponent) value); // ObservationDefinitionComponentComponent 3967 return value; 3968 default: return super.setProperty(hash, name, value); 3969 } 3970 3971 } 3972 3973 @Override 3974 public Base setProperty(String name, Base value) throws FHIRException { 3975 if (name.equals("url")) { 3976 this.url = TypeConvertor.castToUri(value); // UriType 3977 } else if (name.equals("identifier")) { 3978 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3979 } else if (name.equals("version")) { 3980 this.version = TypeConvertor.castToString(value); // StringType 3981 } else if (name.equals("versionAlgorithm[x]")) { 3982 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3983 } else if (name.equals("name")) { 3984 this.name = TypeConvertor.castToString(value); // StringType 3985 } else if (name.equals("title")) { 3986 this.title = TypeConvertor.castToString(value); // StringType 3987 } else if (name.equals("status")) { 3988 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3989 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3990 } else if (name.equals("experimental")) { 3991 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3992 } else if (name.equals("date")) { 3993 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3994 } else if (name.equals("publisher")) { 3995 this.publisher = TypeConvertor.castToString(value); // StringType 3996 } else if (name.equals("contact")) { 3997 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3998 } else if (name.equals("description")) { 3999 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4000 } else if (name.equals("useContext")) { 4001 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4002 } else if (name.equals("jurisdiction")) { 4003 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4004 } else if (name.equals("purpose")) { 4005 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4006 } else if (name.equals("copyright")) { 4007 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4008 } else if (name.equals("copyrightLabel")) { 4009 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4010 } else if (name.equals("approvalDate")) { 4011 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4012 } else if (name.equals("lastReviewDate")) { 4013 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4014 } else if (name.equals("effectivePeriod")) { 4015 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4016 } else if (name.equals("derivedFromCanonical")) { 4017 this.getDerivedFromCanonical().add(TypeConvertor.castToCanonical(value)); 4018 } else if (name.equals("derivedFromUri")) { 4019 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); 4020 } else if (name.equals("subject")) { 4021 this.getSubject().add(TypeConvertor.castToCodeableConcept(value)); 4022 } else if (name.equals("performerType")) { 4023 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4024 } else if (name.equals("category")) { 4025 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 4026 } else if (name.equals("code")) { 4027 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4028 } else if (name.equals("permittedDataType")) { 4029 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4030 this.getPermittedDataType().add((Enumeration) value); 4031 } else if (name.equals("multipleResultsAllowed")) { 4032 this.multipleResultsAllowed = TypeConvertor.castToBoolean(value); // BooleanType 4033 } else if (name.equals("bodySite")) { 4034 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4035 } else if (name.equals("method")) { 4036 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4037 } else if (name.equals("specimen")) { 4038 this.getSpecimen().add(TypeConvertor.castToReference(value)); 4039 } else if (name.equals("device")) { 4040 this.getDevice().add(TypeConvertor.castToReference(value)); 4041 } else if (name.equals("preferredReportName")) { 4042 this.preferredReportName = TypeConvertor.castToString(value); // StringType 4043 } else if (name.equals("permittedUnit")) { 4044 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); 4045 } else if (name.equals("qualifiedValue")) { 4046 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); 4047 } else if (name.equals("hasMember")) { 4048 this.getHasMember().add(TypeConvertor.castToReference(value)); 4049 } else if (name.equals("component")) { 4050 this.getComponent().add((ObservationDefinitionComponentComponent) value); 4051 } else 4052 return super.setProperty(name, value); 4053 return value; 4054 } 4055 4056 @Override 4057 public Base makeProperty(int hash, String name) throws FHIRException { 4058 switch (hash) { 4059 case 116079: return getUrlElement(); 4060 case -1618432855: return getIdentifier(); 4061 case 351608024: return getVersionElement(); 4062 case -115699031: return getVersionAlgorithm(); 4063 case 1508158071: return getVersionAlgorithm(); 4064 case 3373707: return getNameElement(); 4065 case 110371416: return getTitleElement(); 4066 case -892481550: return getStatusElement(); 4067 case -404562712: return getExperimentalElement(); 4068 case 3076014: return getDateElement(); 4069 case 1447404028: return getPublisherElement(); 4070 case 951526432: return addContact(); 4071 case -1724546052: return getDescriptionElement(); 4072 case -669707736: return addUseContext(); 4073 case -507075711: return addJurisdiction(); 4074 case -220463842: return getPurposeElement(); 4075 case 1522889671: return getCopyrightElement(); 4076 case 765157229: return getCopyrightLabelElement(); 4077 case 223539345: return getApprovalDateElement(); 4078 case -1687512484: return getLastReviewDateElement(); 4079 case -403934648: return getEffectivePeriod(); 4080 case -978133683: return addDerivedFromCanonicalElement(); 4081 case -1076333435: return addDerivedFromUriElement(); 4082 case -1867885268: return addSubject(); 4083 case -901444568: return getPerformerType(); 4084 case 50511102: return addCategory(); 4085 case 3059181: return getCode(); 4086 case -99492804: return addPermittedDataTypeElement(); 4087 case -2102414590: return getMultipleResultsAllowedElement(); 4088 case 1702620169: return getBodySite(); 4089 case -1077554975: return getMethod(); 4090 case -2132868344: return addSpecimen(); 4091 case -1335157162: return addDevice(); 4092 case -1851030208: return getPreferredReportNameElement(); 4093 case 1073054652: return addPermittedUnit(); 4094 case -558517707: return addQualifiedValue(); 4095 case -458019372: return addHasMember(); 4096 case -1399907075: return addComponent(); 4097 default: return super.makeProperty(hash, name); 4098 } 4099 4100 } 4101 4102 @Override 4103 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4104 switch (hash) { 4105 case 116079: /*url*/ return new String[] {"uri"}; 4106 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4107 case 351608024: /*version*/ return new String[] {"string"}; 4108 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 4109 case 3373707: /*name*/ return new String[] {"string"}; 4110 case 110371416: /*title*/ return new String[] {"string"}; 4111 case -892481550: /*status*/ return new String[] {"code"}; 4112 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4113 case 3076014: /*date*/ return new String[] {"dateTime"}; 4114 case 1447404028: /*publisher*/ return new String[] {"string"}; 4115 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4116 case -1724546052: /*description*/ return new String[] {"markdown"}; 4117 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4118 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4119 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4120 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4121 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 4122 case 223539345: /*approvalDate*/ return new String[] {"date"}; 4123 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 4124 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 4125 case -978133683: /*derivedFromCanonical*/ return new String[] {"canonical"}; 4126 case -1076333435: /*derivedFromUri*/ return new String[] {"uri"}; 4127 case -1867885268: /*subject*/ return new String[] {"CodeableConcept"}; 4128 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 4129 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4130 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 4131 case -99492804: /*permittedDataType*/ return new String[] {"code"}; 4132 case -2102414590: /*multipleResultsAllowed*/ return new String[] {"boolean"}; 4133 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 4134 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 4135 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 4136 case -1335157162: /*device*/ return new String[] {"Reference"}; 4137 case -1851030208: /*preferredReportName*/ return new String[] {"string"}; 4138 case 1073054652: /*permittedUnit*/ return new String[] {"Coding"}; 4139 case -558517707: /*qualifiedValue*/ return new String[] {}; 4140 case -458019372: /*hasMember*/ return new String[] {"Reference"}; 4141 case -1399907075: /*component*/ return new String[] {}; 4142 default: return super.getTypesForProperty(hash, name); 4143 } 4144 4145 } 4146 4147 @Override 4148 public Base addChild(String name) throws FHIRException { 4149 if (name.equals("url")) { 4150 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.url"); 4151 } 4152 else if (name.equals("identifier")) { 4153 this.identifier = new Identifier(); 4154 return this.identifier; 4155 } 4156 else if (name.equals("version")) { 4157 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.version"); 4158 } 4159 else if (name.equals("versionAlgorithmString")) { 4160 this.versionAlgorithm = new StringType(); 4161 return this.versionAlgorithm; 4162 } 4163 else if (name.equals("versionAlgorithmCoding")) { 4164 this.versionAlgorithm = new Coding(); 4165 return this.versionAlgorithm; 4166 } 4167 else if (name.equals("name")) { 4168 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.name"); 4169 } 4170 else if (name.equals("title")) { 4171 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.title"); 4172 } 4173 else if (name.equals("status")) { 4174 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.status"); 4175 } 4176 else if (name.equals("experimental")) { 4177 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.experimental"); 4178 } 4179 else if (name.equals("date")) { 4180 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.date"); 4181 } 4182 else if (name.equals("publisher")) { 4183 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.publisher"); 4184 } 4185 else if (name.equals("contact")) { 4186 return addContact(); 4187 } 4188 else if (name.equals("description")) { 4189 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.description"); 4190 } 4191 else if (name.equals("useContext")) { 4192 return addUseContext(); 4193 } 4194 else if (name.equals("jurisdiction")) { 4195 return addJurisdiction(); 4196 } 4197 else if (name.equals("purpose")) { 4198 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.purpose"); 4199 } 4200 else if (name.equals("copyright")) { 4201 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.copyright"); 4202 } 4203 else if (name.equals("copyrightLabel")) { 4204 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.copyrightLabel"); 4205 } 4206 else if (name.equals("approvalDate")) { 4207 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.approvalDate"); 4208 } 4209 else if (name.equals("lastReviewDate")) { 4210 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.lastReviewDate"); 4211 } 4212 else if (name.equals("effectivePeriod")) { 4213 this.effectivePeriod = new Period(); 4214 return this.effectivePeriod; 4215 } 4216 else if (name.equals("derivedFromCanonical")) { 4217 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.derivedFromCanonical"); 4218 } 4219 else if (name.equals("derivedFromUri")) { 4220 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.derivedFromUri"); 4221 } 4222 else if (name.equals("subject")) { 4223 return addSubject(); 4224 } 4225 else if (name.equals("performerType")) { 4226 this.performerType = new CodeableConcept(); 4227 return this.performerType; 4228 } 4229 else if (name.equals("category")) { 4230 return addCategory(); 4231 } 4232 else if (name.equals("code")) { 4233 this.code = new CodeableConcept(); 4234 return this.code; 4235 } 4236 else if (name.equals("permittedDataType")) { 4237 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.permittedDataType"); 4238 } 4239 else if (name.equals("multipleResultsAllowed")) { 4240 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.multipleResultsAllowed"); 4241 } 4242 else if (name.equals("bodySite")) { 4243 this.bodySite = new CodeableConcept(); 4244 return this.bodySite; 4245 } 4246 else if (name.equals("method")) { 4247 this.method = new CodeableConcept(); 4248 return this.method; 4249 } 4250 else if (name.equals("specimen")) { 4251 return addSpecimen(); 4252 } 4253 else if (name.equals("device")) { 4254 return addDevice(); 4255 } 4256 else if (name.equals("preferredReportName")) { 4257 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.preferredReportName"); 4258 } 4259 else if (name.equals("permittedUnit")) { 4260 return addPermittedUnit(); 4261 } 4262 else if (name.equals("qualifiedValue")) { 4263 return addQualifiedValue(); 4264 } 4265 else if (name.equals("hasMember")) { 4266 return addHasMember(); 4267 } 4268 else if (name.equals("component")) { 4269 return addComponent(); 4270 } 4271 else 4272 return super.addChild(name); 4273 } 4274 4275 public String fhirType() { 4276 return "ObservationDefinition"; 4277 4278 } 4279 4280 public ObservationDefinition copy() { 4281 ObservationDefinition dst = new ObservationDefinition(); 4282 copyValues(dst); 4283 return dst; 4284 } 4285 4286 public void copyValues(ObservationDefinition dst) { 4287 super.copyValues(dst); 4288 dst.url = url == null ? null : url.copy(); 4289 dst.identifier = identifier == null ? null : identifier.copy(); 4290 dst.version = version == null ? null : version.copy(); 4291 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4292 dst.name = name == null ? null : name.copy(); 4293 dst.title = title == null ? null : title.copy(); 4294 dst.status = status == null ? null : status.copy(); 4295 dst.experimental = experimental == null ? null : experimental.copy(); 4296 dst.date = date == null ? null : date.copy(); 4297 dst.publisher = publisher == null ? null : publisher.copy(); 4298 if (contact != null) { 4299 dst.contact = new ArrayList<ContactDetail>(); 4300 for (ContactDetail i : contact) 4301 dst.contact.add(i.copy()); 4302 }; 4303 dst.description = description == null ? null : description.copy(); 4304 if (useContext != null) { 4305 dst.useContext = new ArrayList<UsageContext>(); 4306 for (UsageContext i : useContext) 4307 dst.useContext.add(i.copy()); 4308 }; 4309 if (jurisdiction != null) { 4310 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4311 for (CodeableConcept i : jurisdiction) 4312 dst.jurisdiction.add(i.copy()); 4313 }; 4314 dst.purpose = purpose == null ? null : purpose.copy(); 4315 dst.copyright = copyright == null ? null : copyright.copy(); 4316 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4317 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4318 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4319 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4320 if (derivedFromCanonical != null) { 4321 dst.derivedFromCanonical = new ArrayList<CanonicalType>(); 4322 for (CanonicalType i : derivedFromCanonical) 4323 dst.derivedFromCanonical.add(i.copy()); 4324 }; 4325 if (derivedFromUri != null) { 4326 dst.derivedFromUri = new ArrayList<UriType>(); 4327 for (UriType i : derivedFromUri) 4328 dst.derivedFromUri.add(i.copy()); 4329 }; 4330 if (subject != null) { 4331 dst.subject = new ArrayList<CodeableConcept>(); 4332 for (CodeableConcept i : subject) 4333 dst.subject.add(i.copy()); 4334 }; 4335 dst.performerType = performerType == null ? null : performerType.copy(); 4336 if (category != null) { 4337 dst.category = new ArrayList<CodeableConcept>(); 4338 for (CodeableConcept i : category) 4339 dst.category.add(i.copy()); 4340 }; 4341 dst.code = code == null ? null : code.copy(); 4342 if (permittedDataType != null) { 4343 dst.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 4344 for (Enumeration<ObservationDataType> i : permittedDataType) 4345 dst.permittedDataType.add(i.copy()); 4346 }; 4347 dst.multipleResultsAllowed = multipleResultsAllowed == null ? null : multipleResultsAllowed.copy(); 4348 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4349 dst.method = method == null ? null : method.copy(); 4350 if (specimen != null) { 4351 dst.specimen = new ArrayList<Reference>(); 4352 for (Reference i : specimen) 4353 dst.specimen.add(i.copy()); 4354 }; 4355 if (device != null) { 4356 dst.device = new ArrayList<Reference>(); 4357 for (Reference i : device) 4358 dst.device.add(i.copy()); 4359 }; 4360 dst.preferredReportName = preferredReportName == null ? null : preferredReportName.copy(); 4361 if (permittedUnit != null) { 4362 dst.permittedUnit = new ArrayList<Coding>(); 4363 for (Coding i : permittedUnit) 4364 dst.permittedUnit.add(i.copy()); 4365 }; 4366 if (qualifiedValue != null) { 4367 dst.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 4368 for (ObservationDefinitionQualifiedValueComponent i : qualifiedValue) 4369 dst.qualifiedValue.add(i.copy()); 4370 }; 4371 if (hasMember != null) { 4372 dst.hasMember = new ArrayList<Reference>(); 4373 for (Reference i : hasMember) 4374 dst.hasMember.add(i.copy()); 4375 }; 4376 if (component != null) { 4377 dst.component = new ArrayList<ObservationDefinitionComponentComponent>(); 4378 for (ObservationDefinitionComponentComponent i : component) 4379 dst.component.add(i.copy()); 4380 }; 4381 } 4382 4383 protected ObservationDefinition typedCopy() { 4384 return copy(); 4385 } 4386 4387 @Override 4388 public boolean equalsDeep(Base other_) { 4389 if (!super.equalsDeep(other_)) 4390 return false; 4391 if (!(other_ instanceof ObservationDefinition)) 4392 return false; 4393 ObservationDefinition o = (ObservationDefinition) other_; 4394 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4395 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4396 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 4397 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 4398 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 4399 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 4400 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 4401 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(derivedFromCanonical, o.derivedFromCanonical, true) 4402 && compareDeep(derivedFromUri, o.derivedFromUri, true) && compareDeep(subject, o.subject, true) 4403 && compareDeep(performerType, o.performerType, true) && compareDeep(category, o.category, true) 4404 && compareDeep(code, o.code, true) && compareDeep(permittedDataType, o.permittedDataType, true) 4405 && compareDeep(multipleResultsAllowed, o.multipleResultsAllowed, true) && compareDeep(bodySite, o.bodySite, true) 4406 && compareDeep(method, o.method, true) && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 4407 && compareDeep(preferredReportName, o.preferredReportName, true) && compareDeep(permittedUnit, o.permittedUnit, true) 4408 && compareDeep(qualifiedValue, o.qualifiedValue, true) && compareDeep(hasMember, o.hasMember, true) 4409 && compareDeep(component, o.component, true); 4410 } 4411 4412 @Override 4413 public boolean equalsShallow(Base other_) { 4414 if (!super.equalsShallow(other_)) 4415 return false; 4416 if (!(other_ instanceof ObservationDefinition)) 4417 return false; 4418 ObservationDefinition o = (ObservationDefinition) other_; 4419 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4420 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 4421 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 4422 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 4423 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 4424 && compareValues(derivedFromCanonical, o.derivedFromCanonical, true) && compareValues(derivedFromUri, o.derivedFromUri, true) 4425 && compareValues(permittedDataType, o.permittedDataType, true) && compareValues(multipleResultsAllowed, o.multipleResultsAllowed, true) 4426 && compareValues(preferredReportName, o.preferredReportName, true); 4427 } 4428 4429 public boolean isEmpty() { 4430 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4431 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 4432 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, approvalDate 4433 , lastReviewDate, effectivePeriod, derivedFromCanonical, derivedFromUri, subject, performerType 4434 , category, code, permittedDataType, multipleResultsAllowed, bodySite, method, specimen 4435 , device, preferredReportName, permittedUnit, qualifiedValue, hasMember, component 4436 ); 4437 } 4438 4439 @Override 4440 public ResourceType getResourceType() { 4441 return ResourceType.ObservationDefinition; 4442 } 4443 4444 /** 4445 * Search parameter: <b>identifier</b> 4446 * <p> 4447 * Description: <b>Multiple Resources: 4448 4449* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4450* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4451* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4452* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4453* [Citation](citation.html): External identifier for the citation 4454* [CodeSystem](codesystem.html): External identifier for the code system 4455* [ConceptMap](conceptmap.html): External identifier for the concept map 4456* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4457* [EventDefinition](eventdefinition.html): External identifier for the event definition 4458* [Evidence](evidence.html): External identifier for the evidence 4459* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4460* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4461* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4462* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4463* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4464* [Library](library.html): External identifier for the library 4465* [Measure](measure.html): External identifier for the measure 4466* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4467* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4468* [NamingSystem](namingsystem.html): External identifier for the naming system 4469* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4470* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4471* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4472* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4473* [Requirements](requirements.html): External identifier for the requirements 4474* [SearchParameter](searchparameter.html): External identifier for the search parameter 4475* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4476* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4477* [StructureMap](structuremap.html): External identifier for the structure map 4478* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4479* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4480* [TestPlan](testplan.html): An identifier for the test plan 4481* [TestScript](testscript.html): External identifier for the test script 4482* [ValueSet](valueset.html): External identifier for the value set 4483</b><br> 4484 * Type: <b>token</b><br> 4485 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4486 * </p> 4487 */ 4488 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4489 public static final String SP_IDENTIFIER = "identifier"; 4490 /** 4491 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4492 * <p> 4493 * Description: <b>Multiple Resources: 4494 4495* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4496* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4497* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4498* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4499* [Citation](citation.html): External identifier for the citation 4500* [CodeSystem](codesystem.html): External identifier for the code system 4501* [ConceptMap](conceptmap.html): External identifier for the concept map 4502* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4503* [EventDefinition](eventdefinition.html): External identifier for the event definition 4504* [Evidence](evidence.html): External identifier for the evidence 4505* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4506* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4507* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4508* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4509* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4510* [Library](library.html): External identifier for the library 4511* [Measure](measure.html): External identifier for the measure 4512* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4513* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4514* [NamingSystem](namingsystem.html): External identifier for the naming system 4515* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4516* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4517* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4518* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4519* [Requirements](requirements.html): External identifier for the requirements 4520* [SearchParameter](searchparameter.html): External identifier for the search parameter 4521* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4522* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4523* [StructureMap](structuremap.html): External identifier for the structure map 4524* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4525* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4526* [TestPlan](testplan.html): An identifier for the test plan 4527* [TestScript](testscript.html): External identifier for the test script 4528* [ValueSet](valueset.html): External identifier for the value set 4529</b><br> 4530 * Type: <b>token</b><br> 4531 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4532 * </p> 4533 */ 4534 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4535 4536 /** 4537 * Search parameter: <b>status</b> 4538 * <p> 4539 * Description: <b>Multiple Resources: 4540 4541* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4542* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4543* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4544* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4545* [Citation](citation.html): The current status of the citation 4546* [CodeSystem](codesystem.html): The current status of the code system 4547* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4548* [ConceptMap](conceptmap.html): The current status of the concept map 4549* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4550* [EventDefinition](eventdefinition.html): The current status of the event definition 4551* [Evidence](evidence.html): The current status of the evidence 4552* [EvidenceReport](evidencereport.html): The current status of the evidence report 4553* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4554* [ExampleScenario](examplescenario.html): The current status of the example scenario 4555* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4556* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4557* [Library](library.html): The current status of the library 4558* [Measure](measure.html): The current status of the measure 4559* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4560* [MessageDefinition](messagedefinition.html): The current status of the message definition 4561* [NamingSystem](namingsystem.html): The current status of the naming system 4562* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4563* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4564* [PlanDefinition](plandefinition.html): The current status of the plan definition 4565* [Questionnaire](questionnaire.html): The current status of the questionnaire 4566* [Requirements](requirements.html): The current status of the requirements 4567* [SearchParameter](searchparameter.html): The current status of the search parameter 4568* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4569* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4570* [StructureMap](structuremap.html): The current status of the structure map 4571* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4572* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4573* [TestPlan](testplan.html): The current status of the test plan 4574* [TestScript](testscript.html): The current status of the test script 4575* [ValueSet](valueset.html): The current status of the value set 4576</b><br> 4577 * Type: <b>token</b><br> 4578 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4579 * </p> 4580 */ 4581 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4582 public static final String SP_STATUS = "status"; 4583 /** 4584 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4585 * <p> 4586 * Description: <b>Multiple Resources: 4587 4588* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4589* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4590* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4591* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4592* [Citation](citation.html): The current status of the citation 4593* [CodeSystem](codesystem.html): The current status of the code system 4594* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4595* [ConceptMap](conceptmap.html): The current status of the concept map 4596* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4597* [EventDefinition](eventdefinition.html): The current status of the event definition 4598* [Evidence](evidence.html): The current status of the evidence 4599* [EvidenceReport](evidencereport.html): The current status of the evidence report 4600* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4601* [ExampleScenario](examplescenario.html): The current status of the example scenario 4602* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4603* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4604* [Library](library.html): The current status of the library 4605* [Measure](measure.html): The current status of the measure 4606* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4607* [MessageDefinition](messagedefinition.html): The current status of the message definition 4608* [NamingSystem](namingsystem.html): The current status of the naming system 4609* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4610* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4611* [PlanDefinition](plandefinition.html): The current status of the plan definition 4612* [Questionnaire](questionnaire.html): The current status of the questionnaire 4613* [Requirements](requirements.html): The current status of the requirements 4614* [SearchParameter](searchparameter.html): The current status of the search parameter 4615* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4616* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4617* [StructureMap](structuremap.html): The current status of the structure map 4618* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4619* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4620* [TestPlan](testplan.html): The current status of the test plan 4621* [TestScript](testscript.html): The current status of the test script 4622* [ValueSet](valueset.html): The current status of the value set 4623</b><br> 4624 * Type: <b>token</b><br> 4625 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4626 * </p> 4627 */ 4628 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4629 4630 /** 4631 * Search parameter: <b>title</b> 4632 * <p> 4633 * Description: <b>Multiple Resources: 4634 4635* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4636* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4637* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4638* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4639* [Citation](citation.html): The human-friendly name of the citation 4640* [CodeSystem](codesystem.html): The human-friendly name of the code system 4641* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4642* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4643* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4644* [Evidence](evidence.html): The human-friendly name of the evidence 4645* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4646* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4647* [Library](library.html): The human-friendly name of the library 4648* [Measure](measure.html): The human-friendly name of the measure 4649* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4650* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4651* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4652* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4653* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4654* [Requirements](requirements.html): The human-friendly name of the requirements 4655* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4656* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4657* [StructureMap](structuremap.html): The human-friendly name of the structure map 4658* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4659* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4660* [TestScript](testscript.html): The human-friendly name of the test script 4661* [ValueSet](valueset.html): The human-friendly name of the value set 4662</b><br> 4663 * Type: <b>string</b><br> 4664 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4665 * </p> 4666 */ 4667 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4668 public static final String SP_TITLE = "title"; 4669 /** 4670 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4671 * <p> 4672 * Description: <b>Multiple Resources: 4673 4674* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4675* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4676* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4677* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4678* [Citation](citation.html): The human-friendly name of the citation 4679* [CodeSystem](codesystem.html): The human-friendly name of the code system 4680* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4681* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4682* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4683* [Evidence](evidence.html): The human-friendly name of the evidence 4684* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4685* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4686* [Library](library.html): The human-friendly name of the library 4687* [Measure](measure.html): The human-friendly name of the measure 4688* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4689* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4690* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4691* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4692* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4693* [Requirements](requirements.html): The human-friendly name of the requirements 4694* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4695* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4696* [StructureMap](structuremap.html): The human-friendly name of the structure map 4697* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4698* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4699* [TestScript](testscript.html): The human-friendly name of the test script 4700* [ValueSet](valueset.html): The human-friendly name of the value set 4701</b><br> 4702 * Type: <b>string</b><br> 4703 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4704 * </p> 4705 */ 4706 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4707 4708 /** 4709 * Search parameter: <b>url</b> 4710 * <p> 4711 * Description: <b>Multiple Resources: 4712 4713* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4714* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4715* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4716* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4717* [Citation](citation.html): The uri that identifies the citation 4718* [CodeSystem](codesystem.html): The uri that identifies the code system 4719* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4720* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4721* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4722* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4723* [Evidence](evidence.html): The uri that identifies the evidence 4724* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4725* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4726* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4727* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4728* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4729* [Library](library.html): The uri that identifies the library 4730* [Measure](measure.html): The uri that identifies the measure 4731* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4732* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4733* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4734* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4735* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4736* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4737* [Requirements](requirements.html): The uri that identifies the requirements 4738* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4739* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4740* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4741* [StructureMap](structuremap.html): The uri that identifies the structure map 4742* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4743* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4744* [TestPlan](testplan.html): The uri that identifies the test plan 4745* [TestScript](testscript.html): The uri that identifies the test script 4746* [ValueSet](valueset.html): The uri that identifies the value set 4747</b><br> 4748 * Type: <b>uri</b><br> 4749 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4750 * </p> 4751 */ 4752 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4753 public static final String SP_URL = "url"; 4754 /** 4755 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4756 * <p> 4757 * Description: <b>Multiple Resources: 4758 4759* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4760* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4761* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4762* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4763* [Citation](citation.html): The uri that identifies the citation 4764* [CodeSystem](codesystem.html): The uri that identifies the code system 4765* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4766* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4767* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4768* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4769* [Evidence](evidence.html): The uri that identifies the evidence 4770* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4771* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4772* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4773* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4774* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4775* [Library](library.html): The uri that identifies the library 4776* [Measure](measure.html): The uri that identifies the measure 4777* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4778* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4779* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4780* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4781* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4782* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4783* [Requirements](requirements.html): The uri that identifies the requirements 4784* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4785* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4786* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4787* [StructureMap](structuremap.html): The uri that identifies the structure map 4788* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4789* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4790* [TestPlan](testplan.html): The uri that identifies the test plan 4791* [TestScript](testscript.html): The uri that identifies the test script 4792* [ValueSet](valueset.html): The uri that identifies the value set 4793</b><br> 4794 * Type: <b>uri</b><br> 4795 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4796 * </p> 4797 */ 4798 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4799 4800 /** 4801 * Search parameter: <b>category</b> 4802 * <p> 4803 * Description: <b>Category (class) of observation</b><br> 4804 * Type: <b>token</b><br> 4805 * Path: <b>ObservationDefinition.category</b><br> 4806 * </p> 4807 */ 4808 @SearchParamDefinition(name="category", path="ObservationDefinition.category", description="Category (class) of observation", type="token" ) 4809 public static final String SP_CATEGORY = "category"; 4810 /** 4811 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4812 * <p> 4813 * Description: <b>Category (class) of observation</b><br> 4814 * Type: <b>token</b><br> 4815 * Path: <b>ObservationDefinition.category</b><br> 4816 * </p> 4817 */ 4818 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4819 4820 /** 4821 * Search parameter: <b>code</b> 4822 * <p> 4823 * Description: <b>Observation code</b><br> 4824 * Type: <b>token</b><br> 4825 * Path: <b>ObservationDefinition.code</b><br> 4826 * </p> 4827 */ 4828 @SearchParamDefinition(name="code", path="ObservationDefinition.code", description="Observation code", type="token" ) 4829 public static final String SP_CODE = "code"; 4830 /** 4831 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4832 * <p> 4833 * Description: <b>Observation code</b><br> 4834 * Type: <b>token</b><br> 4835 * Path: <b>ObservationDefinition.code</b><br> 4836 * </p> 4837 */ 4838 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4839 4840 /** 4841 * Search parameter: <b>experimental</b> 4842 * <p> 4843 * Description: <b>Not for genuine usage (true)</b><br> 4844 * Type: <b>token</b><br> 4845 * Path: <b>ObservationDefinition.experimental</b><br> 4846 * </p> 4847 */ 4848 @SearchParamDefinition(name="experimental", path="ObservationDefinition.experimental", description="Not for genuine usage (true)", type="token" ) 4849 public static final String SP_EXPERIMENTAL = "experimental"; 4850 /** 4851 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 4852 * <p> 4853 * Description: <b>Not for genuine usage (true)</b><br> 4854 * Type: <b>token</b><br> 4855 * Path: <b>ObservationDefinition.experimental</b><br> 4856 * </p> 4857 */ 4858 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 4859 4860 /** 4861 * Search parameter: <b>method</b> 4862 * <p> 4863 * Description: <b>Method of observation</b><br> 4864 * Type: <b>token</b><br> 4865 * Path: <b>ObservationDefinition.method</b><br> 4866 * </p> 4867 */ 4868 @SearchParamDefinition(name="method", path="ObservationDefinition.method", description="Method of observation", type="token" ) 4869 public static final String SP_METHOD = "method"; 4870 /** 4871 * <b>Fluent Client</b> search parameter constant for <b>method</b> 4872 * <p> 4873 * Description: <b>Method of observation</b><br> 4874 * Type: <b>token</b><br> 4875 * Path: <b>ObservationDefinition.method</b><br> 4876 * </p> 4877 */ 4878 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_METHOD); 4879 4880 4881} 4882