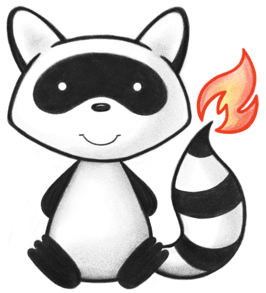
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service. 052 */ 053@ResourceDef(name="ObservationDefinition", profile="http://hl7.org/fhir/StructureDefinition/ObservationDefinition") 054public class ObservationDefinition extends DomainResource { 055 056 public enum ObservationDataType { 057 /** 058 * A measured amount. 059 */ 060 QUANTITY, 061 /** 062 * A coded concept from a reference terminology and/or text. 063 */ 064 CODEABLECONCEPT, 065 /** 066 * A sequence of Unicode characters. 067 */ 068 STRING, 069 /** 070 * true or false. 071 */ 072 BOOLEAN, 073 /** 074 * A signed integer. 075 */ 076 INTEGER, 077 /** 078 * A set of values bounded by low and high. 079 */ 080 RANGE, 081 /** 082 * A ratio of two Quantity values - a numerator and a denominator. 083 */ 084 RATIO, 085 /** 086 * A series of measurements taken by a device. 087 */ 088 SAMPLEDDATA, 089 /** 090 * A time during the day, in the format hh:mm:ss. 091 */ 092 TIME, 093 /** 094 * A date, date-time or partial date (e.g. just year or year + month) as used in human communication. 095 */ 096 DATETIME, 097 /** 098 * A time range defined by start and end date/time. 099 */ 100 PERIOD, 101 /** 102 * added to help the parsers with the generic types 103 */ 104 NULL; 105 public static ObservationDataType fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("Quantity".equals(codeString)) 109 return QUANTITY; 110 if ("CodeableConcept".equals(codeString)) 111 return CODEABLECONCEPT; 112 if ("string".equals(codeString)) 113 return STRING; 114 if ("boolean".equals(codeString)) 115 return BOOLEAN; 116 if ("integer".equals(codeString)) 117 return INTEGER; 118 if ("Range".equals(codeString)) 119 return RANGE; 120 if ("Ratio".equals(codeString)) 121 return RATIO; 122 if ("SampledData".equals(codeString)) 123 return SAMPLEDDATA; 124 if ("time".equals(codeString)) 125 return TIME; 126 if ("dateTime".equals(codeString)) 127 return DATETIME; 128 if ("Period".equals(codeString)) 129 return PERIOD; 130 if (Configuration.isAcceptInvalidEnums()) 131 return null; 132 else 133 throw new FHIRException("Unknown ObservationDataType code '"+codeString+"'"); 134 } 135 public String toCode() { 136 switch (this) { 137 case QUANTITY: return "Quantity"; 138 case CODEABLECONCEPT: return "CodeableConcept"; 139 case STRING: return "string"; 140 case BOOLEAN: return "boolean"; 141 case INTEGER: return "integer"; 142 case RANGE: return "Range"; 143 case RATIO: return "Ratio"; 144 case SAMPLEDDATA: return "SampledData"; 145 case TIME: return "time"; 146 case DATETIME: return "dateTime"; 147 case PERIOD: return "Period"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 public String getSystem() { 153 switch (this) { 154 case QUANTITY: return "http://hl7.org/fhir/permitted-data-type"; 155 case CODEABLECONCEPT: return "http://hl7.org/fhir/permitted-data-type"; 156 case STRING: return "http://hl7.org/fhir/permitted-data-type"; 157 case BOOLEAN: return "http://hl7.org/fhir/permitted-data-type"; 158 case INTEGER: return "http://hl7.org/fhir/permitted-data-type"; 159 case RANGE: return "http://hl7.org/fhir/permitted-data-type"; 160 case RATIO: return "http://hl7.org/fhir/permitted-data-type"; 161 case SAMPLEDDATA: return "http://hl7.org/fhir/permitted-data-type"; 162 case TIME: return "http://hl7.org/fhir/permitted-data-type"; 163 case DATETIME: return "http://hl7.org/fhir/permitted-data-type"; 164 case PERIOD: return "http://hl7.org/fhir/permitted-data-type"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 public String getDefinition() { 170 switch (this) { 171 case QUANTITY: return "A measured amount."; 172 case CODEABLECONCEPT: return "A coded concept from a reference terminology and/or text."; 173 case STRING: return "A sequence of Unicode characters."; 174 case BOOLEAN: return "true or false."; 175 case INTEGER: return "A signed integer."; 176 case RANGE: return "A set of values bounded by low and high."; 177 case RATIO: return "A ratio of two Quantity values - a numerator and a denominator."; 178 case SAMPLEDDATA: return "A series of measurements taken by a device."; 179 case TIME: return "A time during the day, in the format hh:mm:ss."; 180 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month) as used in human communication."; 181 case PERIOD: return "A time range defined by start and end date/time."; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case QUANTITY: return "Quantity"; 189 case CODEABLECONCEPT: return "CodeableConcept"; 190 case STRING: return "string"; 191 case BOOLEAN: return "boolean"; 192 case INTEGER: return "integer"; 193 case RANGE: return "Range"; 194 case RATIO: return "Ratio"; 195 case SAMPLEDDATA: return "SampledData"; 196 case TIME: return "time"; 197 case DATETIME: return "dateTime"; 198 case PERIOD: return "Period"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 } 204 205 public static class ObservationDataTypeEnumFactory implements EnumFactory<ObservationDataType> { 206 public ObservationDataType fromCode(String codeString) throws IllegalArgumentException { 207 if (codeString == null || "".equals(codeString)) 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("Quantity".equals(codeString)) 211 return ObservationDataType.QUANTITY; 212 if ("CodeableConcept".equals(codeString)) 213 return ObservationDataType.CODEABLECONCEPT; 214 if ("string".equals(codeString)) 215 return ObservationDataType.STRING; 216 if ("boolean".equals(codeString)) 217 return ObservationDataType.BOOLEAN; 218 if ("integer".equals(codeString)) 219 return ObservationDataType.INTEGER; 220 if ("Range".equals(codeString)) 221 return ObservationDataType.RANGE; 222 if ("Ratio".equals(codeString)) 223 return ObservationDataType.RATIO; 224 if ("SampledData".equals(codeString)) 225 return ObservationDataType.SAMPLEDDATA; 226 if ("time".equals(codeString)) 227 return ObservationDataType.TIME; 228 if ("dateTime".equals(codeString)) 229 return ObservationDataType.DATETIME; 230 if ("Period".equals(codeString)) 231 return ObservationDataType.PERIOD; 232 throw new IllegalArgumentException("Unknown ObservationDataType code '"+codeString+"'"); 233 } 234 public Enumeration<ObservationDataType> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<ObservationDataType>(this, ObservationDataType.NULL, code); 239 String codeString = ((PrimitiveType) code).asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<ObservationDataType>(this, ObservationDataType.NULL, code); 242 if ("Quantity".equals(codeString)) 243 return new Enumeration<ObservationDataType>(this, ObservationDataType.QUANTITY, code); 244 if ("CodeableConcept".equals(codeString)) 245 return new Enumeration<ObservationDataType>(this, ObservationDataType.CODEABLECONCEPT, code); 246 if ("string".equals(codeString)) 247 return new Enumeration<ObservationDataType>(this, ObservationDataType.STRING, code); 248 if ("boolean".equals(codeString)) 249 return new Enumeration<ObservationDataType>(this, ObservationDataType.BOOLEAN, code); 250 if ("integer".equals(codeString)) 251 return new Enumeration<ObservationDataType>(this, ObservationDataType.INTEGER, code); 252 if ("Range".equals(codeString)) 253 return new Enumeration<ObservationDataType>(this, ObservationDataType.RANGE, code); 254 if ("Ratio".equals(codeString)) 255 return new Enumeration<ObservationDataType>(this, ObservationDataType.RATIO, code); 256 if ("SampledData".equals(codeString)) 257 return new Enumeration<ObservationDataType>(this, ObservationDataType.SAMPLEDDATA, code); 258 if ("time".equals(codeString)) 259 return new Enumeration<ObservationDataType>(this, ObservationDataType.TIME, code); 260 if ("dateTime".equals(codeString)) 261 return new Enumeration<ObservationDataType>(this, ObservationDataType.DATETIME, code); 262 if ("Period".equals(codeString)) 263 return new Enumeration<ObservationDataType>(this, ObservationDataType.PERIOD, code); 264 throw new FHIRException("Unknown ObservationDataType code '"+codeString+"'"); 265 } 266 public String toCode(ObservationDataType code) { 267 if (code == ObservationDataType.NULL) 268 return null; 269 if (code == ObservationDataType.QUANTITY) 270 return "Quantity"; 271 if (code == ObservationDataType.CODEABLECONCEPT) 272 return "CodeableConcept"; 273 if (code == ObservationDataType.STRING) 274 return "string"; 275 if (code == ObservationDataType.BOOLEAN) 276 return "boolean"; 277 if (code == ObservationDataType.INTEGER) 278 return "integer"; 279 if (code == ObservationDataType.RANGE) 280 return "Range"; 281 if (code == ObservationDataType.RATIO) 282 return "Ratio"; 283 if (code == ObservationDataType.SAMPLEDDATA) 284 return "SampledData"; 285 if (code == ObservationDataType.TIME) 286 return "time"; 287 if (code == ObservationDataType.DATETIME) 288 return "dateTime"; 289 if (code == ObservationDataType.PERIOD) 290 return "Period"; 291 return "?"; 292 } 293 public String toSystem(ObservationDataType code) { 294 return code.getSystem(); 295 } 296 } 297 298 public enum ObservationRangeCategory { 299 /** 300 * Reference (Normal) Range for Ordinal and Continuous Observations. 301 */ 302 REFERENCE, 303 /** 304 * Critical Range for Ordinal and Continuous Observations. Results outside this range are critical. 305 */ 306 CRITICAL, 307 /** 308 * Absolute Range for Ordinal and Continuous Observations. Results outside this range are not possible. 309 */ 310 ABSOLUTE, 311 /** 312 * added to help the parsers with the generic types 313 */ 314 NULL; 315 public static ObservationRangeCategory fromCode(String codeString) throws FHIRException { 316 if (codeString == null || "".equals(codeString)) 317 return null; 318 if ("reference".equals(codeString)) 319 return REFERENCE; 320 if ("critical".equals(codeString)) 321 return CRITICAL; 322 if ("absolute".equals(codeString)) 323 return ABSOLUTE; 324 if (Configuration.isAcceptInvalidEnums()) 325 return null; 326 else 327 throw new FHIRException("Unknown ObservationRangeCategory code '"+codeString+"'"); 328 } 329 public String toCode() { 330 switch (this) { 331 case REFERENCE: return "reference"; 332 case CRITICAL: return "critical"; 333 case ABSOLUTE: return "absolute"; 334 case NULL: return null; 335 default: return "?"; 336 } 337 } 338 public String getSystem() { 339 switch (this) { 340 case REFERENCE: return "http://hl7.org/fhir/observation-range-category"; 341 case CRITICAL: return "http://hl7.org/fhir/observation-range-category"; 342 case ABSOLUTE: return "http://hl7.org/fhir/observation-range-category"; 343 case NULL: return null; 344 default: return "?"; 345 } 346 } 347 public String getDefinition() { 348 switch (this) { 349 case REFERENCE: return "Reference (Normal) Range for Ordinal and Continuous Observations."; 350 case CRITICAL: return "Critical Range for Ordinal and Continuous Observations. Results outside this range are critical."; 351 case ABSOLUTE: return "Absolute Range for Ordinal and Continuous Observations. Results outside this range are not possible."; 352 case NULL: return null; 353 default: return "?"; 354 } 355 } 356 public String getDisplay() { 357 switch (this) { 358 case REFERENCE: return "reference range"; 359 case CRITICAL: return "critical range"; 360 case ABSOLUTE: return "absolute range"; 361 case NULL: return null; 362 default: return "?"; 363 } 364 } 365 } 366 367 public static class ObservationRangeCategoryEnumFactory implements EnumFactory<ObservationRangeCategory> { 368 public ObservationRangeCategory fromCode(String codeString) throws IllegalArgumentException { 369 if (codeString == null || "".equals(codeString)) 370 if (codeString == null || "".equals(codeString)) 371 return null; 372 if ("reference".equals(codeString)) 373 return ObservationRangeCategory.REFERENCE; 374 if ("critical".equals(codeString)) 375 return ObservationRangeCategory.CRITICAL; 376 if ("absolute".equals(codeString)) 377 return ObservationRangeCategory.ABSOLUTE; 378 throw new IllegalArgumentException("Unknown ObservationRangeCategory code '"+codeString+"'"); 379 } 380 public Enumeration<ObservationRangeCategory> fromType(PrimitiveType<?> code) throws FHIRException { 381 if (code == null) 382 return null; 383 if (code.isEmpty()) 384 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.NULL, code); 385 String codeString = ((PrimitiveType) code).asStringValue(); 386 if (codeString == null || "".equals(codeString)) 387 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.NULL, code); 388 if ("reference".equals(codeString)) 389 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.REFERENCE, code); 390 if ("critical".equals(codeString)) 391 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.CRITICAL, code); 392 if ("absolute".equals(codeString)) 393 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.ABSOLUTE, code); 394 throw new FHIRException("Unknown ObservationRangeCategory code '"+codeString+"'"); 395 } 396 public String toCode(ObservationRangeCategory code) { 397 if (code == ObservationRangeCategory.NULL) 398 return null; 399 if (code == ObservationRangeCategory.REFERENCE) 400 return "reference"; 401 if (code == ObservationRangeCategory.CRITICAL) 402 return "critical"; 403 if (code == ObservationRangeCategory.ABSOLUTE) 404 return "absolute"; 405 return "?"; 406 } 407 public String toSystem(ObservationRangeCategory code) { 408 return code.getSystem(); 409 } 410 } 411 412 @Block() 413 public static class ObservationDefinitionQualifiedValueComponent extends BackboneElement implements IBaseBackboneElement { 414 /** 415 * A concept defining the context for this set of qualified values. 416 */ 417 @Child(name = "context", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 418 @Description(shortDefinition="Context qualifier for the set of qualified values", formalDefinition="A concept defining the context for this set of qualified values." ) 419 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-meaning") 420 protected CodeableConcept context; 421 422 /** 423 * The target population this set of qualified values applies to. 424 */ 425 @Child(name = "appliesTo", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 426 @Description(shortDefinition="Targetted population for the set of qualified values", formalDefinition="The target population this set of qualified values applies to." ) 427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-appliesto") 428 protected List<CodeableConcept> appliesTo; 429 430 /** 431 * The gender this set of qualified values applies to. 432 */ 433 @Child(name = "gender", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 434 @Description(shortDefinition="male | female | other | unknown", formalDefinition="The gender this set of qualified values applies to." ) 435 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 436 protected Enumeration<AdministrativeGender> gender; 437 438 /** 439 * The age range this set of qualified values applies to. 440 */ 441 @Child(name = "age", type = {Range.class}, order=4, min=0, max=1, modifier=false, summary=false) 442 @Description(shortDefinition="Applicable age range for the set of qualified values", formalDefinition="The age range this set of qualified values applies to." ) 443 protected Range age; 444 445 /** 446 * The gestational age this set of qualified values applies to. 447 */ 448 @Child(name = "gestationalAge", type = {Range.class}, order=5, min=0, max=1, modifier=false, summary=false) 449 @Description(shortDefinition="Applicable gestational age range for the set of qualified values", formalDefinition="The gestational age this set of qualified values applies to." ) 450 protected Range gestationalAge; 451 452 /** 453 * Text based condition for which the the set of qualified values is valid. 454 */ 455 @Child(name = "condition", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 456 @Description(shortDefinition="Condition associated with the set of qualified values", formalDefinition="Text based condition for which the the set of qualified values is valid." ) 457 protected StringType condition; 458 459 /** 460 * The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values. 461 */ 462 @Child(name = "rangeCategory", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 463 @Description(shortDefinition="reference | critical | absolute", formalDefinition="The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values." ) 464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-range-category") 465 protected Enumeration<ObservationRangeCategory> rangeCategory; 466 467 /** 468 * The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values. 469 */ 470 @Child(name = "range", type = {Range.class}, order=8, min=0, max=1, modifier=false, summary=false) 471 @Description(shortDefinition="The range for continuous or ordinal observations", formalDefinition="The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values." ) 472 protected Range range; 473 474 /** 475 * The set of valid coded results for qualitative observations that match the criteria of this set of qualified values. 476 */ 477 @Child(name = "validCodedValueSet", type = {CanonicalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 478 @Description(shortDefinition="Value set of valid coded values as part of this set of qualified values", formalDefinition="The set of valid coded results for qualitative observations that match the criteria of this set of qualified values." ) 479 protected CanonicalType validCodedValueSet; 480 481 /** 482 * The set of normal coded results for qualitative observations that match the criteria of this set of qualified values. 483 */ 484 @Child(name = "normalCodedValueSet", type = {CanonicalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 485 @Description(shortDefinition="Value set of normal coded values as part of this set of qualified values", formalDefinition="The set of normal coded results for qualitative observations that match the criteria of this set of qualified values." ) 486 protected CanonicalType normalCodedValueSet; 487 488 /** 489 * The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values. 490 */ 491 @Child(name = "abnormalCodedValueSet", type = {CanonicalType.class}, order=11, min=0, max=1, modifier=false, summary=false) 492 @Description(shortDefinition="Value set of abnormal coded values as part of this set of qualified values", formalDefinition="The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values." ) 493 protected CanonicalType abnormalCodedValueSet; 494 495 /** 496 * The set of critical coded results for qualitative observations that match the criteria of this set of qualified values. 497 */ 498 @Child(name = "criticalCodedValueSet", type = {CanonicalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 499 @Description(shortDefinition="Value set of critical coded values as part of this set of qualified values", formalDefinition="The set of critical coded results for qualitative observations that match the criteria of this set of qualified values." ) 500 protected CanonicalType criticalCodedValueSet; 501 502 private static final long serialVersionUID = -538666361L; 503 504 /** 505 * Constructor 506 */ 507 public ObservationDefinitionQualifiedValueComponent() { 508 super(); 509 } 510 511 /** 512 * @return {@link #context} (A concept defining the context for this set of qualified values.) 513 */ 514 public CodeableConcept getContext() { 515 if (this.context == null) 516 if (Configuration.errorOnAutoCreate()) 517 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.context"); 518 else if (Configuration.doAutoCreate()) 519 this.context = new CodeableConcept(); // cc 520 return this.context; 521 } 522 523 public boolean hasContext() { 524 return this.context != null && !this.context.isEmpty(); 525 } 526 527 /** 528 * @param value {@link #context} (A concept defining the context for this set of qualified values.) 529 */ 530 public ObservationDefinitionQualifiedValueComponent setContext(CodeableConcept value) { 531 this.context = value; 532 return this; 533 } 534 535 /** 536 * @return {@link #appliesTo} (The target population this set of qualified values applies to.) 537 */ 538 public List<CodeableConcept> getAppliesTo() { 539 if (this.appliesTo == null) 540 this.appliesTo = new ArrayList<CodeableConcept>(); 541 return this.appliesTo; 542 } 543 544 /** 545 * @return Returns a reference to <code>this</code> for easy method chaining 546 */ 547 public ObservationDefinitionQualifiedValueComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 548 this.appliesTo = theAppliesTo; 549 return this; 550 } 551 552 public boolean hasAppliesTo() { 553 if (this.appliesTo == null) 554 return false; 555 for (CodeableConcept item : this.appliesTo) 556 if (!item.isEmpty()) 557 return true; 558 return false; 559 } 560 561 public CodeableConcept addAppliesTo() { //3 562 CodeableConcept t = new CodeableConcept(); 563 if (this.appliesTo == null) 564 this.appliesTo = new ArrayList<CodeableConcept>(); 565 this.appliesTo.add(t); 566 return t; 567 } 568 569 public ObservationDefinitionQualifiedValueComponent addAppliesTo(CodeableConcept t) { //3 570 if (t == null) 571 return this; 572 if (this.appliesTo == null) 573 this.appliesTo = new ArrayList<CodeableConcept>(); 574 this.appliesTo.add(t); 575 return this; 576 } 577 578 /** 579 * @return The first repetition of repeating field {@link #appliesTo}, creating it if it does not already exist {3} 580 */ 581 public CodeableConcept getAppliesToFirstRep() { 582 if (getAppliesTo().isEmpty()) { 583 addAppliesTo(); 584 } 585 return getAppliesTo().get(0); 586 } 587 588 /** 589 * @return {@link #gender} (The gender this set of qualified values applies to.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 590 */ 591 public Enumeration<AdministrativeGender> getGenderElement() { 592 if (this.gender == null) 593 if (Configuration.errorOnAutoCreate()) 594 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.gender"); 595 else if (Configuration.doAutoCreate()) 596 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 597 return this.gender; 598 } 599 600 public boolean hasGenderElement() { 601 return this.gender != null && !this.gender.isEmpty(); 602 } 603 604 public boolean hasGender() { 605 return this.gender != null && !this.gender.isEmpty(); 606 } 607 608 /** 609 * @param value {@link #gender} (The gender this set of qualified values applies to.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 610 */ 611 public ObservationDefinitionQualifiedValueComponent setGenderElement(Enumeration<AdministrativeGender> value) { 612 this.gender = value; 613 return this; 614 } 615 616 /** 617 * @return The gender this set of qualified values applies to. 618 */ 619 public AdministrativeGender getGender() { 620 return this.gender == null ? null : this.gender.getValue(); 621 } 622 623 /** 624 * @param value The gender this set of qualified values applies to. 625 */ 626 public ObservationDefinitionQualifiedValueComponent setGender(AdministrativeGender value) { 627 if (value == null) 628 this.gender = null; 629 else { 630 if (this.gender == null) 631 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 632 this.gender.setValue(value); 633 } 634 return this; 635 } 636 637 /** 638 * @return {@link #age} (The age range this set of qualified values applies to.) 639 */ 640 public Range getAge() { 641 if (this.age == null) 642 if (Configuration.errorOnAutoCreate()) 643 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.age"); 644 else if (Configuration.doAutoCreate()) 645 this.age = new Range(); // cc 646 return this.age; 647 } 648 649 public boolean hasAge() { 650 return this.age != null && !this.age.isEmpty(); 651 } 652 653 /** 654 * @param value {@link #age} (The age range this set of qualified values applies to.) 655 */ 656 public ObservationDefinitionQualifiedValueComponent setAge(Range value) { 657 this.age = value; 658 return this; 659 } 660 661 /** 662 * @return {@link #gestationalAge} (The gestational age this set of qualified values applies to.) 663 */ 664 public Range getGestationalAge() { 665 if (this.gestationalAge == null) 666 if (Configuration.errorOnAutoCreate()) 667 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.gestationalAge"); 668 else if (Configuration.doAutoCreate()) 669 this.gestationalAge = new Range(); // cc 670 return this.gestationalAge; 671 } 672 673 public boolean hasGestationalAge() { 674 return this.gestationalAge != null && !this.gestationalAge.isEmpty(); 675 } 676 677 /** 678 * @param value {@link #gestationalAge} (The gestational age this set of qualified values applies to.) 679 */ 680 public ObservationDefinitionQualifiedValueComponent setGestationalAge(Range value) { 681 this.gestationalAge = value; 682 return this; 683 } 684 685 /** 686 * @return {@link #condition} (Text based condition for which the the set of qualified values is valid.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 687 */ 688 public StringType getConditionElement() { 689 if (this.condition == null) 690 if (Configuration.errorOnAutoCreate()) 691 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.condition"); 692 else if (Configuration.doAutoCreate()) 693 this.condition = new StringType(); // bb 694 return this.condition; 695 } 696 697 public boolean hasConditionElement() { 698 return this.condition != null && !this.condition.isEmpty(); 699 } 700 701 public boolean hasCondition() { 702 return this.condition != null && !this.condition.isEmpty(); 703 } 704 705 /** 706 * @param value {@link #condition} (Text based condition for which the the set of qualified values is valid.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 707 */ 708 public ObservationDefinitionQualifiedValueComponent setConditionElement(StringType value) { 709 this.condition = value; 710 return this; 711 } 712 713 /** 714 * @return Text based condition for which the the set of qualified values is valid. 715 */ 716 public String getCondition() { 717 return this.condition == null ? null : this.condition.getValue(); 718 } 719 720 /** 721 * @param value Text based condition for which the the set of qualified values is valid. 722 */ 723 public ObservationDefinitionQualifiedValueComponent setCondition(String value) { 724 if (Utilities.noString(value)) 725 this.condition = null; 726 else { 727 if (this.condition == null) 728 this.condition = new StringType(); 729 this.condition.setValue(value); 730 } 731 return this; 732 } 733 734 /** 735 * @return {@link #rangeCategory} (The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getRangeCategory" gives direct access to the value 736 */ 737 public Enumeration<ObservationRangeCategory> getRangeCategoryElement() { 738 if (this.rangeCategory == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.rangeCategory"); 741 else if (Configuration.doAutoCreate()) 742 this.rangeCategory = new Enumeration<ObservationRangeCategory>(new ObservationRangeCategoryEnumFactory()); // bb 743 return this.rangeCategory; 744 } 745 746 public boolean hasRangeCategoryElement() { 747 return this.rangeCategory != null && !this.rangeCategory.isEmpty(); 748 } 749 750 public boolean hasRangeCategory() { 751 return this.rangeCategory != null && !this.rangeCategory.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #rangeCategory} (The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getRangeCategory" gives direct access to the value 756 */ 757 public ObservationDefinitionQualifiedValueComponent setRangeCategoryElement(Enumeration<ObservationRangeCategory> value) { 758 this.rangeCategory = value; 759 return this; 760 } 761 762 /** 763 * @return The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values. 764 */ 765 public ObservationRangeCategory getRangeCategory() { 766 return this.rangeCategory == null ? null : this.rangeCategory.getValue(); 767 } 768 769 /** 770 * @param value The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values. 771 */ 772 public ObservationDefinitionQualifiedValueComponent setRangeCategory(ObservationRangeCategory value) { 773 if (value == null) 774 this.rangeCategory = null; 775 else { 776 if (this.rangeCategory == null) 777 this.rangeCategory = new Enumeration<ObservationRangeCategory>(new ObservationRangeCategoryEnumFactory()); 778 this.rangeCategory.setValue(value); 779 } 780 return this; 781 } 782 783 /** 784 * @return {@link #range} (The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.) 785 */ 786 public Range getRange() { 787 if (this.range == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.range"); 790 else if (Configuration.doAutoCreate()) 791 this.range = new Range(); // cc 792 return this.range; 793 } 794 795 public boolean hasRange() { 796 return this.range != null && !this.range.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #range} (The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.) 801 */ 802 public ObservationDefinitionQualifiedValueComponent setRange(Range value) { 803 this.range = value; 804 return this; 805 } 806 807 /** 808 * @return {@link #validCodedValueSet} (The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getValidCodedValueSet" gives direct access to the value 809 */ 810 public CanonicalType getValidCodedValueSetElement() { 811 if (this.validCodedValueSet == null) 812 if (Configuration.errorOnAutoCreate()) 813 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.validCodedValueSet"); 814 else if (Configuration.doAutoCreate()) 815 this.validCodedValueSet = new CanonicalType(); // bb 816 return this.validCodedValueSet; 817 } 818 819 public boolean hasValidCodedValueSetElement() { 820 return this.validCodedValueSet != null && !this.validCodedValueSet.isEmpty(); 821 } 822 823 public boolean hasValidCodedValueSet() { 824 return this.validCodedValueSet != null && !this.validCodedValueSet.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #validCodedValueSet} (The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getValidCodedValueSet" gives direct access to the value 829 */ 830 public ObservationDefinitionQualifiedValueComponent setValidCodedValueSetElement(CanonicalType value) { 831 this.validCodedValueSet = value; 832 return this; 833 } 834 835 /** 836 * @return The set of valid coded results for qualitative observations that match the criteria of this set of qualified values. 837 */ 838 public String getValidCodedValueSet() { 839 return this.validCodedValueSet == null ? null : this.validCodedValueSet.getValue(); 840 } 841 842 /** 843 * @param value The set of valid coded results for qualitative observations that match the criteria of this set of qualified values. 844 */ 845 public ObservationDefinitionQualifiedValueComponent setValidCodedValueSet(String value) { 846 if (Utilities.noString(value)) 847 this.validCodedValueSet = null; 848 else { 849 if (this.validCodedValueSet == null) 850 this.validCodedValueSet = new CanonicalType(); 851 this.validCodedValueSet.setValue(value); 852 } 853 return this; 854 } 855 856 /** 857 * @return {@link #normalCodedValueSet} (The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getNormalCodedValueSet" gives direct access to the value 858 */ 859 public CanonicalType getNormalCodedValueSetElement() { 860 if (this.normalCodedValueSet == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.normalCodedValueSet"); 863 else if (Configuration.doAutoCreate()) 864 this.normalCodedValueSet = new CanonicalType(); // bb 865 return this.normalCodedValueSet; 866 } 867 868 public boolean hasNormalCodedValueSetElement() { 869 return this.normalCodedValueSet != null && !this.normalCodedValueSet.isEmpty(); 870 } 871 872 public boolean hasNormalCodedValueSet() { 873 return this.normalCodedValueSet != null && !this.normalCodedValueSet.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #normalCodedValueSet} (The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getNormalCodedValueSet" gives direct access to the value 878 */ 879 public ObservationDefinitionQualifiedValueComponent setNormalCodedValueSetElement(CanonicalType value) { 880 this.normalCodedValueSet = value; 881 return this; 882 } 883 884 /** 885 * @return The set of normal coded results for qualitative observations that match the criteria of this set of qualified values. 886 */ 887 public String getNormalCodedValueSet() { 888 return this.normalCodedValueSet == null ? null : this.normalCodedValueSet.getValue(); 889 } 890 891 /** 892 * @param value The set of normal coded results for qualitative observations that match the criteria of this set of qualified values. 893 */ 894 public ObservationDefinitionQualifiedValueComponent setNormalCodedValueSet(String value) { 895 if (Utilities.noString(value)) 896 this.normalCodedValueSet = null; 897 else { 898 if (this.normalCodedValueSet == null) 899 this.normalCodedValueSet = new CanonicalType(); 900 this.normalCodedValueSet.setValue(value); 901 } 902 return this; 903 } 904 905 /** 906 * @return {@link #abnormalCodedValueSet} (The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getAbnormalCodedValueSet" gives direct access to the value 907 */ 908 public CanonicalType getAbnormalCodedValueSetElement() { 909 if (this.abnormalCodedValueSet == null) 910 if (Configuration.errorOnAutoCreate()) 911 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.abnormalCodedValueSet"); 912 else if (Configuration.doAutoCreate()) 913 this.abnormalCodedValueSet = new CanonicalType(); // bb 914 return this.abnormalCodedValueSet; 915 } 916 917 public boolean hasAbnormalCodedValueSetElement() { 918 return this.abnormalCodedValueSet != null && !this.abnormalCodedValueSet.isEmpty(); 919 } 920 921 public boolean hasAbnormalCodedValueSet() { 922 return this.abnormalCodedValueSet != null && !this.abnormalCodedValueSet.isEmpty(); 923 } 924 925 /** 926 * @param value {@link #abnormalCodedValueSet} (The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getAbnormalCodedValueSet" gives direct access to the value 927 */ 928 public ObservationDefinitionQualifiedValueComponent setAbnormalCodedValueSetElement(CanonicalType value) { 929 this.abnormalCodedValueSet = value; 930 return this; 931 } 932 933 /** 934 * @return The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values. 935 */ 936 public String getAbnormalCodedValueSet() { 937 return this.abnormalCodedValueSet == null ? null : this.abnormalCodedValueSet.getValue(); 938 } 939 940 /** 941 * @param value The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values. 942 */ 943 public ObservationDefinitionQualifiedValueComponent setAbnormalCodedValueSet(String value) { 944 if (Utilities.noString(value)) 945 this.abnormalCodedValueSet = null; 946 else { 947 if (this.abnormalCodedValueSet == null) 948 this.abnormalCodedValueSet = new CanonicalType(); 949 this.abnormalCodedValueSet.setValue(value); 950 } 951 return this; 952 } 953 954 /** 955 * @return {@link #criticalCodedValueSet} (The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getCriticalCodedValueSet" gives direct access to the value 956 */ 957 public CanonicalType getCriticalCodedValueSetElement() { 958 if (this.criticalCodedValueSet == null) 959 if (Configuration.errorOnAutoCreate()) 960 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedValueComponent.criticalCodedValueSet"); 961 else if (Configuration.doAutoCreate()) 962 this.criticalCodedValueSet = new CanonicalType(); // bb 963 return this.criticalCodedValueSet; 964 } 965 966 public boolean hasCriticalCodedValueSetElement() { 967 return this.criticalCodedValueSet != null && !this.criticalCodedValueSet.isEmpty(); 968 } 969 970 public boolean hasCriticalCodedValueSet() { 971 return this.criticalCodedValueSet != null && !this.criticalCodedValueSet.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #criticalCodedValueSet} (The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.). This is the underlying object with id, value and extensions. The accessor "getCriticalCodedValueSet" gives direct access to the value 976 */ 977 public ObservationDefinitionQualifiedValueComponent setCriticalCodedValueSetElement(CanonicalType value) { 978 this.criticalCodedValueSet = value; 979 return this; 980 } 981 982 /** 983 * @return The set of critical coded results for qualitative observations that match the criteria of this set of qualified values. 984 */ 985 public String getCriticalCodedValueSet() { 986 return this.criticalCodedValueSet == null ? null : this.criticalCodedValueSet.getValue(); 987 } 988 989 /** 990 * @param value The set of critical coded results for qualitative observations that match the criteria of this set of qualified values. 991 */ 992 public ObservationDefinitionQualifiedValueComponent setCriticalCodedValueSet(String value) { 993 if (Utilities.noString(value)) 994 this.criticalCodedValueSet = null; 995 else { 996 if (this.criticalCodedValueSet == null) 997 this.criticalCodedValueSet = new CanonicalType(); 998 this.criticalCodedValueSet.setValue(value); 999 } 1000 return this; 1001 } 1002 1003 protected void listChildren(List<Property> children) { 1004 super.listChildren(children); 1005 children.add(new Property("context", "CodeableConcept", "A concept defining the context for this set of qualified values.", 0, 1, context)); 1006 children.add(new Property("appliesTo", "CodeableConcept", "The target population this set of qualified values applies to.", 0, java.lang.Integer.MAX_VALUE, appliesTo)); 1007 children.add(new Property("gender", "code", "The gender this set of qualified values applies to.", 0, 1, gender)); 1008 children.add(new Property("age", "Range", "The age range this set of qualified values applies to.", 0, 1, age)); 1009 children.add(new Property("gestationalAge", "Range", "The gestational age this set of qualified values applies to.", 0, 1, gestationalAge)); 1010 children.add(new Property("condition", "string", "Text based condition for which the the set of qualified values is valid.", 0, 1, condition)); 1011 children.add(new Property("rangeCategory", "code", "The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, rangeCategory)); 1012 children.add(new Property("range", "Range", "The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, range)); 1013 children.add(new Property("validCodedValueSet", "canonical(ValueSet)", "The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, validCodedValueSet)); 1014 children.add(new Property("normalCodedValueSet", "canonical(ValueSet)", "The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, normalCodedValueSet)); 1015 children.add(new Property("abnormalCodedValueSet", "canonical(ValueSet)", "The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, abnormalCodedValueSet)); 1016 children.add(new Property("criticalCodedValueSet", "canonical(ValueSet)", "The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, criticalCodedValueSet)); 1017 } 1018 1019 @Override 1020 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1021 switch (_hash) { 1022 case 951530927: /*context*/ return new Property("context", "CodeableConcept", "A concept defining the context for this set of qualified values.", 0, 1, context); 1023 case -2089924569: /*appliesTo*/ return new Property("appliesTo", "CodeableConcept", "The target population this set of qualified values applies to.", 0, java.lang.Integer.MAX_VALUE, appliesTo); 1024 case -1249512767: /*gender*/ return new Property("gender", "code", "The gender this set of qualified values applies to.", 0, 1, gender); 1025 case 96511: /*age*/ return new Property("age", "Range", "The age range this set of qualified values applies to.", 0, 1, age); 1026 case -241217538: /*gestationalAge*/ return new Property("gestationalAge", "Range", "The gestational age this set of qualified values applies to.", 0, 1, gestationalAge); 1027 case -861311717: /*condition*/ return new Property("condition", "string", "Text based condition for which the the set of qualified values is valid.", 0, 1, condition); 1028 case -363410085: /*rangeCategory*/ return new Property("rangeCategory", "code", "The category of range of values for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, rangeCategory); 1029 case 108280125: /*range*/ return new Property("range", "Range", "The range of values defined for continuous or ordinal observations that match the criteria of this set of qualified values.", 0, 1, range); 1030 case 1374640076: /*validCodedValueSet*/ return new Property("validCodedValueSet", "canonical(ValueSet)", "The set of valid coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, validCodedValueSet); 1031 case -837500735: /*normalCodedValueSet*/ return new Property("normalCodedValueSet", "canonical(ValueSet)", "The set of normal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, normalCodedValueSet); 1032 case 1073600256: /*abnormalCodedValueSet*/ return new Property("abnormalCodedValueSet", "canonical(ValueSet)", "The set of abnormal coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, abnormalCodedValueSet); 1033 case 2568457: /*criticalCodedValueSet*/ return new Property("criticalCodedValueSet", "canonical(ValueSet)", "The set of critical coded results for qualitative observations that match the criteria of this set of qualified values.", 0, 1, criticalCodedValueSet); 1034 default: return super.getNamedProperty(_hash, _name, _checkValid); 1035 } 1036 1037 } 1038 1039 @Override 1040 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1041 switch (hash) { 1042 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // CodeableConcept 1043 case -2089924569: /*appliesTo*/ return this.appliesTo == null ? new Base[0] : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 1044 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1045 case 96511: /*age*/ return this.age == null ? new Base[0] : new Base[] {this.age}; // Range 1046 case -241217538: /*gestationalAge*/ return this.gestationalAge == null ? new Base[0] : new Base[] {this.gestationalAge}; // Range 1047 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // StringType 1048 case -363410085: /*rangeCategory*/ return this.rangeCategory == null ? new Base[0] : new Base[] {this.rangeCategory}; // Enumeration<ObservationRangeCategory> 1049 case 108280125: /*range*/ return this.range == null ? new Base[0] : new Base[] {this.range}; // Range 1050 case 1374640076: /*validCodedValueSet*/ return this.validCodedValueSet == null ? new Base[0] : new Base[] {this.validCodedValueSet}; // CanonicalType 1051 case -837500735: /*normalCodedValueSet*/ return this.normalCodedValueSet == null ? new Base[0] : new Base[] {this.normalCodedValueSet}; // CanonicalType 1052 case 1073600256: /*abnormalCodedValueSet*/ return this.abnormalCodedValueSet == null ? new Base[0] : new Base[] {this.abnormalCodedValueSet}; // CanonicalType 1053 case 2568457: /*criticalCodedValueSet*/ return this.criticalCodedValueSet == null ? new Base[0] : new Base[] {this.criticalCodedValueSet}; // CanonicalType 1054 default: return super.getProperty(hash, name, checkValid); 1055 } 1056 1057 } 1058 1059 @Override 1060 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1061 switch (hash) { 1062 case 951530927: // context 1063 this.context = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1064 return value; 1065 case -2089924569: // appliesTo 1066 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1067 return value; 1068 case -1249512767: // gender 1069 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1070 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1071 return value; 1072 case 96511: // age 1073 this.age = TypeConvertor.castToRange(value); // Range 1074 return value; 1075 case -241217538: // gestationalAge 1076 this.gestationalAge = TypeConvertor.castToRange(value); // Range 1077 return value; 1078 case -861311717: // condition 1079 this.condition = TypeConvertor.castToString(value); // StringType 1080 return value; 1081 case -363410085: // rangeCategory 1082 value = new ObservationRangeCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1083 this.rangeCategory = (Enumeration) value; // Enumeration<ObservationRangeCategory> 1084 return value; 1085 case 108280125: // range 1086 this.range = TypeConvertor.castToRange(value); // Range 1087 return value; 1088 case 1374640076: // validCodedValueSet 1089 this.validCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1090 return value; 1091 case -837500735: // normalCodedValueSet 1092 this.normalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1093 return value; 1094 case 1073600256: // abnormalCodedValueSet 1095 this.abnormalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1096 return value; 1097 case 2568457: // criticalCodedValueSet 1098 this.criticalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1099 return value; 1100 default: return super.setProperty(hash, name, value); 1101 } 1102 1103 } 1104 1105 @Override 1106 public Base setProperty(String name, Base value) throws FHIRException { 1107 if (name.equals("context")) { 1108 this.context = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1109 } else if (name.equals("appliesTo")) { 1110 this.getAppliesTo().add(TypeConvertor.castToCodeableConcept(value)); 1111 } else if (name.equals("gender")) { 1112 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1113 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1114 } else if (name.equals("age")) { 1115 this.age = TypeConvertor.castToRange(value); // Range 1116 } else if (name.equals("gestationalAge")) { 1117 this.gestationalAge = TypeConvertor.castToRange(value); // Range 1118 } else if (name.equals("condition")) { 1119 this.condition = TypeConvertor.castToString(value); // StringType 1120 } else if (name.equals("rangeCategory")) { 1121 value = new ObservationRangeCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1122 this.rangeCategory = (Enumeration) value; // Enumeration<ObservationRangeCategory> 1123 } else if (name.equals("range")) { 1124 this.range = TypeConvertor.castToRange(value); // Range 1125 } else if (name.equals("validCodedValueSet")) { 1126 this.validCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1127 } else if (name.equals("normalCodedValueSet")) { 1128 this.normalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1129 } else if (name.equals("abnormalCodedValueSet")) { 1130 this.abnormalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1131 } else if (name.equals("criticalCodedValueSet")) { 1132 this.criticalCodedValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1133 } else 1134 return super.setProperty(name, value); 1135 return value; 1136 } 1137 1138 @Override 1139 public void removeChild(String name, Base value) throws FHIRException { 1140 if (name.equals("context")) { 1141 this.context = null; 1142 } else if (name.equals("appliesTo")) { 1143 this.getAppliesTo().remove(value); 1144 } else if (name.equals("gender")) { 1145 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1146 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1147 } else if (name.equals("age")) { 1148 this.age = null; 1149 } else if (name.equals("gestationalAge")) { 1150 this.gestationalAge = null; 1151 } else if (name.equals("condition")) { 1152 this.condition = null; 1153 } else if (name.equals("rangeCategory")) { 1154 value = new ObservationRangeCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1155 this.rangeCategory = (Enumeration) value; // Enumeration<ObservationRangeCategory> 1156 } else if (name.equals("range")) { 1157 this.range = null; 1158 } else if (name.equals("validCodedValueSet")) { 1159 this.validCodedValueSet = null; 1160 } else if (name.equals("normalCodedValueSet")) { 1161 this.normalCodedValueSet = null; 1162 } else if (name.equals("abnormalCodedValueSet")) { 1163 this.abnormalCodedValueSet = null; 1164 } else if (name.equals("criticalCodedValueSet")) { 1165 this.criticalCodedValueSet = null; 1166 } else 1167 super.removeChild(name, value); 1168 1169 } 1170 1171 @Override 1172 public Base makeProperty(int hash, String name) throws FHIRException { 1173 switch (hash) { 1174 case 951530927: return getContext(); 1175 case -2089924569: return addAppliesTo(); 1176 case -1249512767: return getGenderElement(); 1177 case 96511: return getAge(); 1178 case -241217538: return getGestationalAge(); 1179 case -861311717: return getConditionElement(); 1180 case -363410085: return getRangeCategoryElement(); 1181 case 108280125: return getRange(); 1182 case 1374640076: return getValidCodedValueSetElement(); 1183 case -837500735: return getNormalCodedValueSetElement(); 1184 case 1073600256: return getAbnormalCodedValueSetElement(); 1185 case 2568457: return getCriticalCodedValueSetElement(); 1186 default: return super.makeProperty(hash, name); 1187 } 1188 1189 } 1190 1191 @Override 1192 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1193 switch (hash) { 1194 case 951530927: /*context*/ return new String[] {"CodeableConcept"}; 1195 case -2089924569: /*appliesTo*/ return new String[] {"CodeableConcept"}; 1196 case -1249512767: /*gender*/ return new String[] {"code"}; 1197 case 96511: /*age*/ return new String[] {"Range"}; 1198 case -241217538: /*gestationalAge*/ return new String[] {"Range"}; 1199 case -861311717: /*condition*/ return new String[] {"string"}; 1200 case -363410085: /*rangeCategory*/ return new String[] {"code"}; 1201 case 108280125: /*range*/ return new String[] {"Range"}; 1202 case 1374640076: /*validCodedValueSet*/ return new String[] {"canonical"}; 1203 case -837500735: /*normalCodedValueSet*/ return new String[] {"canonical"}; 1204 case 1073600256: /*abnormalCodedValueSet*/ return new String[] {"canonical"}; 1205 case 2568457: /*criticalCodedValueSet*/ return new String[] {"canonical"}; 1206 default: return super.getTypesForProperty(hash, name); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base addChild(String name) throws FHIRException { 1213 if (name.equals("context")) { 1214 this.context = new CodeableConcept(); 1215 return this.context; 1216 } 1217 else if (name.equals("appliesTo")) { 1218 return addAppliesTo(); 1219 } 1220 else if (name.equals("gender")) { 1221 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.gender"); 1222 } 1223 else if (name.equals("age")) { 1224 this.age = new Range(); 1225 return this.age; 1226 } 1227 else if (name.equals("gestationalAge")) { 1228 this.gestationalAge = new Range(); 1229 return this.gestationalAge; 1230 } 1231 else if (name.equals("condition")) { 1232 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.condition"); 1233 } 1234 else if (name.equals("rangeCategory")) { 1235 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.rangeCategory"); 1236 } 1237 else if (name.equals("range")) { 1238 this.range = new Range(); 1239 return this.range; 1240 } 1241 else if (name.equals("validCodedValueSet")) { 1242 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.validCodedValueSet"); 1243 } 1244 else if (name.equals("normalCodedValueSet")) { 1245 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.normalCodedValueSet"); 1246 } 1247 else if (name.equals("abnormalCodedValueSet")) { 1248 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.abnormalCodedValueSet"); 1249 } 1250 else if (name.equals("criticalCodedValueSet")) { 1251 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.qualifiedValue.criticalCodedValueSet"); 1252 } 1253 else 1254 return super.addChild(name); 1255 } 1256 1257 public ObservationDefinitionQualifiedValueComponent copy() { 1258 ObservationDefinitionQualifiedValueComponent dst = new ObservationDefinitionQualifiedValueComponent(); 1259 copyValues(dst); 1260 return dst; 1261 } 1262 1263 public void copyValues(ObservationDefinitionQualifiedValueComponent dst) { 1264 super.copyValues(dst); 1265 dst.context = context == null ? null : context.copy(); 1266 if (appliesTo != null) { 1267 dst.appliesTo = new ArrayList<CodeableConcept>(); 1268 for (CodeableConcept i : appliesTo) 1269 dst.appliesTo.add(i.copy()); 1270 }; 1271 dst.gender = gender == null ? null : gender.copy(); 1272 dst.age = age == null ? null : age.copy(); 1273 dst.gestationalAge = gestationalAge == null ? null : gestationalAge.copy(); 1274 dst.condition = condition == null ? null : condition.copy(); 1275 dst.rangeCategory = rangeCategory == null ? null : rangeCategory.copy(); 1276 dst.range = range == null ? null : range.copy(); 1277 dst.validCodedValueSet = validCodedValueSet == null ? null : validCodedValueSet.copy(); 1278 dst.normalCodedValueSet = normalCodedValueSet == null ? null : normalCodedValueSet.copy(); 1279 dst.abnormalCodedValueSet = abnormalCodedValueSet == null ? null : abnormalCodedValueSet.copy(); 1280 dst.criticalCodedValueSet = criticalCodedValueSet == null ? null : criticalCodedValueSet.copy(); 1281 } 1282 1283 @Override 1284 public boolean equalsDeep(Base other_) { 1285 if (!super.equalsDeep(other_)) 1286 return false; 1287 if (!(other_ instanceof ObservationDefinitionQualifiedValueComponent)) 1288 return false; 1289 ObservationDefinitionQualifiedValueComponent o = (ObservationDefinitionQualifiedValueComponent) other_; 1290 return compareDeep(context, o.context, true) && compareDeep(appliesTo, o.appliesTo, true) && compareDeep(gender, o.gender, true) 1291 && compareDeep(age, o.age, true) && compareDeep(gestationalAge, o.gestationalAge, true) && compareDeep(condition, o.condition, true) 1292 && compareDeep(rangeCategory, o.rangeCategory, true) && compareDeep(range, o.range, true) && compareDeep(validCodedValueSet, o.validCodedValueSet, true) 1293 && compareDeep(normalCodedValueSet, o.normalCodedValueSet, true) && compareDeep(abnormalCodedValueSet, o.abnormalCodedValueSet, true) 1294 && compareDeep(criticalCodedValueSet, o.criticalCodedValueSet, true); 1295 } 1296 1297 @Override 1298 public boolean equalsShallow(Base other_) { 1299 if (!super.equalsShallow(other_)) 1300 return false; 1301 if (!(other_ instanceof ObservationDefinitionQualifiedValueComponent)) 1302 return false; 1303 ObservationDefinitionQualifiedValueComponent o = (ObservationDefinitionQualifiedValueComponent) other_; 1304 return compareValues(gender, o.gender, true) && compareValues(condition, o.condition, true) && compareValues(rangeCategory, o.rangeCategory, true) 1305 && compareValues(validCodedValueSet, o.validCodedValueSet, true) && compareValues(normalCodedValueSet, o.normalCodedValueSet, true) 1306 && compareValues(abnormalCodedValueSet, o.abnormalCodedValueSet, true) && compareValues(criticalCodedValueSet, o.criticalCodedValueSet, true) 1307 ; 1308 } 1309 1310 public boolean isEmpty() { 1311 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, appliesTo, gender 1312 , age, gestationalAge, condition, rangeCategory, range, validCodedValueSet, normalCodedValueSet 1313 , abnormalCodedValueSet, criticalCodedValueSet); 1314 } 1315 1316 public String fhirType() { 1317 return "ObservationDefinition.qualifiedValue"; 1318 1319 } 1320 1321 } 1322 1323 @Block() 1324 public static class ObservationDefinitionComponentComponent extends BackboneElement implements IBaseBackboneElement { 1325 /** 1326 * Describes what will be observed. 1327 */ 1328 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1329 @Description(shortDefinition="Type of observation", formalDefinition="Describes what will be observed." ) 1330 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1331 protected CodeableConcept code; 1332 1333 /** 1334 * The data types allowed for the value element of the instance of this component observations. 1335 */ 1336 @Child(name = "permittedDataType", type = {CodeType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1337 @Description(shortDefinition="Quantity | CodeableConcept | string | boolean | integer | Range | Ratio | SampledData | time | dateTime | Period", formalDefinition="The data types allowed for the value element of the instance of this component observations." ) 1338 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/permitted-data-type") 1339 protected List<Enumeration<ObservationDataType>> permittedDataType; 1340 1341 /** 1342 * Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition. 1343 */ 1344 @Child(name = "permittedUnit", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1345 @Description(shortDefinition="Unit for quantitative results", formalDefinition="Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition." ) 1346 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ucum-units") 1347 protected List<Coding> permittedUnit; 1348 1349 /** 1350 * A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations. 1351 */ 1352 @Child(name = "qualifiedValue", type = {ObservationDefinitionQualifiedValueComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1353 @Description(shortDefinition="Set of qualified values for observation results", formalDefinition="A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations." ) 1354 protected List<ObservationDefinitionQualifiedValueComponent> qualifiedValue; 1355 1356 private static final long serialVersionUID = 2106051085L; 1357 1358 /** 1359 * Constructor 1360 */ 1361 public ObservationDefinitionComponentComponent() { 1362 super(); 1363 } 1364 1365 /** 1366 * Constructor 1367 */ 1368 public ObservationDefinitionComponentComponent(CodeableConcept code) { 1369 super(); 1370 this.setCode(code); 1371 } 1372 1373 /** 1374 * @return {@link #code} (Describes what will be observed.) 1375 */ 1376 public CodeableConcept getCode() { 1377 if (this.code == null) 1378 if (Configuration.errorOnAutoCreate()) 1379 throw new Error("Attempt to auto-create ObservationDefinitionComponentComponent.code"); 1380 else if (Configuration.doAutoCreate()) 1381 this.code = new CodeableConcept(); // cc 1382 return this.code; 1383 } 1384 1385 public boolean hasCode() { 1386 return this.code != null && !this.code.isEmpty(); 1387 } 1388 1389 /** 1390 * @param value {@link #code} (Describes what will be observed.) 1391 */ 1392 public ObservationDefinitionComponentComponent setCode(CodeableConcept value) { 1393 this.code = value; 1394 return this; 1395 } 1396 1397 /** 1398 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1399 */ 1400 public List<Enumeration<ObservationDataType>> getPermittedDataType() { 1401 if (this.permittedDataType == null) 1402 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1403 return this.permittedDataType; 1404 } 1405 1406 /** 1407 * @return Returns a reference to <code>this</code> for easy method chaining 1408 */ 1409 public ObservationDefinitionComponentComponent setPermittedDataType(List<Enumeration<ObservationDataType>> thePermittedDataType) { 1410 this.permittedDataType = thePermittedDataType; 1411 return this; 1412 } 1413 1414 public boolean hasPermittedDataType() { 1415 if (this.permittedDataType == null) 1416 return false; 1417 for (Enumeration<ObservationDataType> item : this.permittedDataType) 1418 if (!item.isEmpty()) 1419 return true; 1420 return false; 1421 } 1422 1423 /** 1424 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1425 */ 1426 public Enumeration<ObservationDataType> addPermittedDataTypeElement() {//2 1427 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 1428 if (this.permittedDataType == null) 1429 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1430 this.permittedDataType.add(t); 1431 return t; 1432 } 1433 1434 /** 1435 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1436 */ 1437 public ObservationDefinitionComponentComponent addPermittedDataType(ObservationDataType value) { //1 1438 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 1439 t.setValue(value); 1440 if (this.permittedDataType == null) 1441 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1442 this.permittedDataType.add(t); 1443 return this; 1444 } 1445 1446 /** 1447 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance of this component observations.) 1448 */ 1449 public boolean hasPermittedDataType(ObservationDataType value) { 1450 if (this.permittedDataType == null) 1451 return false; 1452 for (Enumeration<ObservationDataType> v : this.permittedDataType) 1453 if (v.getValue().equals(value)) // code 1454 return true; 1455 return false; 1456 } 1457 1458 /** 1459 * @return {@link #permittedUnit} (Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.) 1460 */ 1461 public List<Coding> getPermittedUnit() { 1462 if (this.permittedUnit == null) 1463 this.permittedUnit = new ArrayList<Coding>(); 1464 return this.permittedUnit; 1465 } 1466 1467 /** 1468 * @return Returns a reference to <code>this</code> for easy method chaining 1469 */ 1470 public ObservationDefinitionComponentComponent setPermittedUnit(List<Coding> thePermittedUnit) { 1471 this.permittedUnit = thePermittedUnit; 1472 return this; 1473 } 1474 1475 public boolean hasPermittedUnit() { 1476 if (this.permittedUnit == null) 1477 return false; 1478 for (Coding item : this.permittedUnit) 1479 if (!item.isEmpty()) 1480 return true; 1481 return false; 1482 } 1483 1484 public Coding addPermittedUnit() { //3 1485 Coding t = new Coding(); 1486 if (this.permittedUnit == null) 1487 this.permittedUnit = new ArrayList<Coding>(); 1488 this.permittedUnit.add(t); 1489 return t; 1490 } 1491 1492 public ObservationDefinitionComponentComponent addPermittedUnit(Coding t) { //3 1493 if (t == null) 1494 return this; 1495 if (this.permittedUnit == null) 1496 this.permittedUnit = new ArrayList<Coding>(); 1497 this.permittedUnit.add(t); 1498 return this; 1499 } 1500 1501 /** 1502 * @return The first repetition of repeating field {@link #permittedUnit}, creating it if it does not already exist {3} 1503 */ 1504 public Coding getPermittedUnitFirstRep() { 1505 if (getPermittedUnit().isEmpty()) { 1506 addPermittedUnit(); 1507 } 1508 return getPermittedUnit().get(0); 1509 } 1510 1511 /** 1512 * @return {@link #qualifiedValue} (A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.) 1513 */ 1514 public List<ObservationDefinitionQualifiedValueComponent> getQualifiedValue() { 1515 if (this.qualifiedValue == null) 1516 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1517 return this.qualifiedValue; 1518 } 1519 1520 /** 1521 * @return Returns a reference to <code>this</code> for easy method chaining 1522 */ 1523 public ObservationDefinitionComponentComponent setQualifiedValue(List<ObservationDefinitionQualifiedValueComponent> theQualifiedValue) { 1524 this.qualifiedValue = theQualifiedValue; 1525 return this; 1526 } 1527 1528 public boolean hasQualifiedValue() { 1529 if (this.qualifiedValue == null) 1530 return false; 1531 for (ObservationDefinitionQualifiedValueComponent item : this.qualifiedValue) 1532 if (!item.isEmpty()) 1533 return true; 1534 return false; 1535 } 1536 1537 public ObservationDefinitionQualifiedValueComponent addQualifiedValue() { //3 1538 ObservationDefinitionQualifiedValueComponent t = new ObservationDefinitionQualifiedValueComponent(); 1539 if (this.qualifiedValue == null) 1540 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1541 this.qualifiedValue.add(t); 1542 return t; 1543 } 1544 1545 public ObservationDefinitionComponentComponent addQualifiedValue(ObservationDefinitionQualifiedValueComponent t) { //3 1546 if (t == null) 1547 return this; 1548 if (this.qualifiedValue == null) 1549 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1550 this.qualifiedValue.add(t); 1551 return this; 1552 } 1553 1554 /** 1555 * @return The first repetition of repeating field {@link #qualifiedValue}, creating it if it does not already exist {3} 1556 */ 1557 public ObservationDefinitionQualifiedValueComponent getQualifiedValueFirstRep() { 1558 if (getQualifiedValue().isEmpty()) { 1559 addQualifiedValue(); 1560 } 1561 return getQualifiedValue().get(0); 1562 } 1563 1564 protected void listChildren(List<Property> children) { 1565 super.listChildren(children); 1566 children.add(new Property("code", "CodeableConcept", "Describes what will be observed.", 0, 1, code)); 1567 children.add(new Property("permittedDataType", "code", "The data types allowed for the value element of the instance of this component observations.", 0, java.lang.Integer.MAX_VALUE, permittedDataType)); 1568 children.add(new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit)); 1569 children.add(new Property("qualifiedValue", "@ObservationDefinition.qualifiedValue", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue)); 1570 } 1571 1572 @Override 1573 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1574 switch (_hash) { 1575 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what will be observed.", 0, 1, code); 1576 case -99492804: /*permittedDataType*/ return new Property("permittedDataType", "code", "The data types allowed for the value element of the instance of this component observations.", 0, java.lang.Integer.MAX_VALUE, permittedDataType); 1577 case 1073054652: /*permittedUnit*/ return new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit); 1578 case -558517707: /*qualifiedValue*/ return new Property("qualifiedValue", "@ObservationDefinition.qualifiedValue", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue); 1579 default: return super.getNamedProperty(_hash, _name, _checkValid); 1580 } 1581 1582 } 1583 1584 @Override 1585 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1586 switch (hash) { 1587 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1588 case -99492804: /*permittedDataType*/ return this.permittedDataType == null ? new Base[0] : this.permittedDataType.toArray(new Base[this.permittedDataType.size()]); // Enumeration<ObservationDataType> 1589 case 1073054652: /*permittedUnit*/ return this.permittedUnit == null ? new Base[0] : this.permittedUnit.toArray(new Base[this.permittedUnit.size()]); // Coding 1590 case -558517707: /*qualifiedValue*/ return this.qualifiedValue == null ? new Base[0] : this.qualifiedValue.toArray(new Base[this.qualifiedValue.size()]); // ObservationDefinitionQualifiedValueComponent 1591 default: return super.getProperty(hash, name, checkValid); 1592 } 1593 1594 } 1595 1596 @Override 1597 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1598 switch (hash) { 1599 case 3059181: // code 1600 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1601 return value; 1602 case -99492804: // permittedDataType 1603 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1604 this.getPermittedDataType().add((Enumeration) value); // Enumeration<ObservationDataType> 1605 return value; 1606 case 1073054652: // permittedUnit 1607 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); // Coding 1608 return value; 1609 case -558517707: // qualifiedValue 1610 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); // ObservationDefinitionQualifiedValueComponent 1611 return value; 1612 default: return super.setProperty(hash, name, value); 1613 } 1614 1615 } 1616 1617 @Override 1618 public Base setProperty(String name, Base value) throws FHIRException { 1619 if (name.equals("code")) { 1620 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1621 } else if (name.equals("permittedDataType")) { 1622 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1623 this.getPermittedDataType().add((Enumeration) value); 1624 } else if (name.equals("permittedUnit")) { 1625 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); 1626 } else if (name.equals("qualifiedValue")) { 1627 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); 1628 } else 1629 return super.setProperty(name, value); 1630 return value; 1631 } 1632 1633 @Override 1634 public void removeChild(String name, Base value) throws FHIRException { 1635 if (name.equals("code")) { 1636 this.code = null; 1637 } else if (name.equals("permittedDataType")) { 1638 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1639 this.getPermittedDataType().remove((Enumeration) value); 1640 } else if (name.equals("permittedUnit")) { 1641 this.getPermittedUnit().remove(value); 1642 } else if (name.equals("qualifiedValue")) { 1643 this.getQualifiedValue().remove((ObservationDefinitionQualifiedValueComponent) value); 1644 } else 1645 super.removeChild(name, value); 1646 1647 } 1648 1649 @Override 1650 public Base makeProperty(int hash, String name) throws FHIRException { 1651 switch (hash) { 1652 case 3059181: return getCode(); 1653 case -99492804: return addPermittedDataTypeElement(); 1654 case 1073054652: return addPermittedUnit(); 1655 case -558517707: return addQualifiedValue(); 1656 default: return super.makeProperty(hash, name); 1657 } 1658 1659 } 1660 1661 @Override 1662 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1663 switch (hash) { 1664 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1665 case -99492804: /*permittedDataType*/ return new String[] {"code"}; 1666 case 1073054652: /*permittedUnit*/ return new String[] {"Coding"}; 1667 case -558517707: /*qualifiedValue*/ return new String[] {"@ObservationDefinition.qualifiedValue"}; 1668 default: return super.getTypesForProperty(hash, name); 1669 } 1670 1671 } 1672 1673 @Override 1674 public Base addChild(String name) throws FHIRException { 1675 if (name.equals("code")) { 1676 this.code = new CodeableConcept(); 1677 return this.code; 1678 } 1679 else if (name.equals("permittedDataType")) { 1680 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.component.permittedDataType"); 1681 } 1682 else if (name.equals("permittedUnit")) { 1683 return addPermittedUnit(); 1684 } 1685 else if (name.equals("qualifiedValue")) { 1686 return addQualifiedValue(); 1687 } 1688 else 1689 return super.addChild(name); 1690 } 1691 1692 public ObservationDefinitionComponentComponent copy() { 1693 ObservationDefinitionComponentComponent dst = new ObservationDefinitionComponentComponent(); 1694 copyValues(dst); 1695 return dst; 1696 } 1697 1698 public void copyValues(ObservationDefinitionComponentComponent dst) { 1699 super.copyValues(dst); 1700 dst.code = code == null ? null : code.copy(); 1701 if (permittedDataType != null) { 1702 dst.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1703 for (Enumeration<ObservationDataType> i : permittedDataType) 1704 dst.permittedDataType.add(i.copy()); 1705 }; 1706 if (permittedUnit != null) { 1707 dst.permittedUnit = new ArrayList<Coding>(); 1708 for (Coding i : permittedUnit) 1709 dst.permittedUnit.add(i.copy()); 1710 }; 1711 if (qualifiedValue != null) { 1712 dst.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 1713 for (ObservationDefinitionQualifiedValueComponent i : qualifiedValue) 1714 dst.qualifiedValue.add(i.copy()); 1715 }; 1716 } 1717 1718 @Override 1719 public boolean equalsDeep(Base other_) { 1720 if (!super.equalsDeep(other_)) 1721 return false; 1722 if (!(other_ instanceof ObservationDefinitionComponentComponent)) 1723 return false; 1724 ObservationDefinitionComponentComponent o = (ObservationDefinitionComponentComponent) other_; 1725 return compareDeep(code, o.code, true) && compareDeep(permittedDataType, o.permittedDataType, true) 1726 && compareDeep(permittedUnit, o.permittedUnit, true) && compareDeep(qualifiedValue, o.qualifiedValue, true) 1727 ; 1728 } 1729 1730 @Override 1731 public boolean equalsShallow(Base other_) { 1732 if (!super.equalsShallow(other_)) 1733 return false; 1734 if (!(other_ instanceof ObservationDefinitionComponentComponent)) 1735 return false; 1736 ObservationDefinitionComponentComponent o = (ObservationDefinitionComponentComponent) other_; 1737 return compareValues(permittedDataType, o.permittedDataType, true); 1738 } 1739 1740 public boolean isEmpty() { 1741 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, permittedDataType, permittedUnit 1742 , qualifiedValue); 1743 } 1744 1745 public String fhirType() { 1746 return "ObservationDefinition.component"; 1747 1748 } 1749 1750 } 1751 1752 /** 1753 * An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions. 1754 */ 1755 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1756 @Description(shortDefinition="Logical canonical URL to reference this ObservationDefinition (globally unique)", formalDefinition="An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions." ) 1757 protected UriType url; 1758 1759 /** 1760 * Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server. 1761 */ 1762 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1763 @Description(shortDefinition="Business identifier of the ObservationDefinition", formalDefinition="Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server." ) 1764 protected Identifier identifier; 1765 1766 /** 1767 * The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 1768 */ 1769 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1770 @Description(shortDefinition="Business version of the ObservationDefinition", formalDefinition="The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable." ) 1771 protected StringType version; 1772 1773 /** 1774 * Indicates the mechanism used to compare versions to determine which is more current. 1775 */ 1776 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1777 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1778 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1779 protected DataType versionAlgorithm; 1780 1781 /** 1782 * A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1783 */ 1784 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1785 @Description(shortDefinition="Name for this ObservationDefinition (computer friendly)", formalDefinition="A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1786 protected StringType name; 1787 1788 /** 1789 * A short, descriptive, user-friendly title for the ObservationDefinition. 1790 */ 1791 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1792 @Description(shortDefinition="Name for this ObservationDefinition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the ObservationDefinition." ) 1793 protected StringType title; 1794 1795 /** 1796 * The current state of the ObservationDefinition. 1797 */ 1798 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 1799 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of the ObservationDefinition." ) 1800 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1801 protected Enumeration<PublicationStatus> status; 1802 1803 /** 1804 * A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1805 */ 1806 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1807 @Description(shortDefinition="If for testing purposes, not real usage", formalDefinition="A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage." ) 1808 protected BooleanType experimental; 1809 1810 /** 1811 * The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes. 1812 */ 1813 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1814 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes." ) 1815 protected DateTimeType date; 1816 1817 /** 1818 * Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact. 1819 */ 1820 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1821 @Description(shortDefinition="The name of the individual or organization that published the ObservationDefinition", formalDefinition="Helps establish the \"authority/credibility\" of the ObservationDefinition. May also allow for contact." ) 1822 protected StringType publisher; 1823 1824 /** 1825 * Contact details to assist a user in finding and communicating with the publisher. 1826 */ 1827 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1828 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1829 protected List<ContactDetail> contact; 1830 1831 /** 1832 * A free text natural language description of the ObservationDefinition from the consumer's perspective. 1833 */ 1834 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1835 @Description(shortDefinition="Natural language description of the ObservationDefinition", formalDefinition="A free text natural language description of the ObservationDefinition from the consumer's perspective." ) 1836 protected MarkdownType description; 1837 1838 /** 1839 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances. 1840 */ 1841 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1842 @Description(shortDefinition="Content intends to support these contexts", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances." ) 1843 protected List<UsageContext> useContext; 1844 1845 /** 1846 * A jurisdiction in which the ObservationDefinition is intended to be used. 1847 */ 1848 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1849 @Description(shortDefinition="Intended jurisdiction for this ObservationDefinition (if applicable)", formalDefinition="A jurisdiction in which the ObservationDefinition is intended to be used." ) 1850 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1851 protected List<CodeableConcept> jurisdiction; 1852 1853 /** 1854 * Explains why this ObservationDefinition is needed and why it has been designed as it has. 1855 */ 1856 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1857 @Description(shortDefinition="Why this ObservationDefinition is defined", formalDefinition="Explains why this ObservationDefinition is needed and why it has been designed as it has." ) 1858 protected MarkdownType purpose; 1859 1860 /** 1861 * Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition. 1862 */ 1863 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1864 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition." ) 1865 protected MarkdownType copyright; 1866 1867 /** 1868 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1869 */ 1870 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1871 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1872 protected StringType copyrightLabel; 1873 1874 /** 1875 * The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1876 */ 1877 @Child(name = "approvalDate", type = {DateType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1878 @Description(shortDefinition="When ObservationDefinition was approved by publisher", formalDefinition="The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 1879 protected DateType approvalDate; 1880 1881 /** 1882 * The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 1883 */ 1884 @Child(name = "lastReviewDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 1885 @Description(shortDefinition="Date on which the asset content was last reviewed by the publisher", formalDefinition="The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date." ) 1886 protected DateType lastReviewDate; 1887 1888 /** 1889 * The period during which the ObservationDefinition content was or is planned to be effective. 1890 */ 1891 @Child(name = "effectivePeriod", type = {Period.class}, order=19, min=0, max=1, modifier=false, summary=true) 1892 @Description(shortDefinition="The effective date range for the ObservationDefinition", formalDefinition="The period during which the ObservationDefinition content was or is planned to be effective." ) 1893 protected Period effectivePeriod; 1894 1895 /** 1896 * The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition. 1897 */ 1898 @Child(name = "derivedFromCanonical", type = {CanonicalType.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1899 @Description(shortDefinition="Based on FHIR definition of another observation", formalDefinition="The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition." ) 1900 protected List<CanonicalType> derivedFromCanonical; 1901 1902 /** 1903 * The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition. 1904 */ 1905 @Child(name = "derivedFromUri", type = {UriType.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1906 @Description(shortDefinition="Based on external definition", formalDefinition="The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition." ) 1907 protected List<UriType> derivedFromUri; 1908 1909 /** 1910 * A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition. 1911 */ 1912 @Child(name = "subject", type = {CodeableConcept.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1913 @Description(shortDefinition="Type of subject for the defined observation", formalDefinition="A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition." ) 1914 protected List<CodeableConcept> subject; 1915 1916 /** 1917 * The type of individual/organization/device that is expected to act upon instances of this definition. 1918 */ 1919 @Child(name = "performerType", type = {CodeableConcept.class}, order=23, min=0, max=1, modifier=false, summary=true) 1920 @Description(shortDefinition="Desired kind of performer for such kind of observation", formalDefinition="The type of individual/organization/device that is expected to act upon instances of this definition." ) 1921 protected CodeableConcept performerType; 1922 1923 /** 1924 * A code that classifies the general type of observation. 1925 */ 1926 @Child(name = "category", type = {CodeableConcept.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1927 @Description(shortDefinition="General type of observation", formalDefinition="A code that classifies the general type of observation." ) 1928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-category") 1929 protected List<CodeableConcept> category; 1930 1931 /** 1932 * Describes what will be observed. Sometimes this is called the observation "name". 1933 */ 1934 @Child(name = "code", type = {CodeableConcept.class}, order=25, min=1, max=1, modifier=false, summary=true) 1935 @Description(shortDefinition="Type of observation", formalDefinition="Describes what will be observed. Sometimes this is called the observation \"name\"." ) 1936 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1937 protected CodeableConcept code; 1938 1939 /** 1940 * The data types allowed for the value element of the instance observations conforming to this ObservationDefinition. 1941 */ 1942 @Child(name = "permittedDataType", type = {CodeType.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1943 @Description(shortDefinition="Quantity | CodeableConcept | string | boolean | integer | Range | Ratio | SampledData | time | dateTime | Period", formalDefinition="The data types allowed for the value element of the instance observations conforming to this ObservationDefinition." ) 1944 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/permitted-data-type") 1945 protected List<Enumeration<ObservationDataType>> permittedDataType; 1946 1947 /** 1948 * Multiple results allowed for observations conforming to this ObservationDefinition. 1949 */ 1950 @Child(name = "multipleResultsAllowed", type = {BooleanType.class}, order=27, min=0, max=1, modifier=false, summary=false) 1951 @Description(shortDefinition="Multiple results allowed for conforming observations", formalDefinition="Multiple results allowed for observations conforming to this ObservationDefinition." ) 1952 protected BooleanType multipleResultsAllowed; 1953 1954 /** 1955 * The site on the subject's body where the observation is to be made. 1956 */ 1957 @Child(name = "bodySite", type = {CodeableConcept.class}, order=28, min=0, max=1, modifier=false, summary=false) 1958 @Description(shortDefinition="Body part to be observed", formalDefinition="The site on the subject's body where the observation is to be made." ) 1959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1960 protected CodeableConcept bodySite; 1961 1962 /** 1963 * The method or technique used to perform the observation. 1964 */ 1965 @Child(name = "method", type = {CodeableConcept.class}, order=29, min=0, max=1, modifier=false, summary=false) 1966 @Description(shortDefinition="Method used to produce the observation", formalDefinition="The method or technique used to perform the observation." ) 1967 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-methods") 1968 protected CodeableConcept method; 1969 1970 /** 1971 * The kind of specimen that this type of observation is produced on. 1972 */ 1973 @Child(name = "specimen", type = {SpecimenDefinition.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1974 @Description(shortDefinition="Kind of specimen used by this type of observation", formalDefinition="The kind of specimen that this type of observation is produced on." ) 1975 protected List<Reference> specimen; 1976 1977 /** 1978 * The measurement model of device or actual device used to produce observations of this type. 1979 */ 1980 @Child(name = "device", type = {DeviceDefinition.class, Device.class}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1981 @Description(shortDefinition="Measurement device or model of device", formalDefinition="The measurement model of device or actual device used to produce observations of this type." ) 1982 protected List<Reference> device; 1983 1984 /** 1985 * The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition. 1986 */ 1987 @Child(name = "preferredReportName", type = {StringType.class}, order=32, min=0, max=1, modifier=false, summary=false) 1988 @Description(shortDefinition="The preferred name to be used when reporting the observation results", formalDefinition="The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition." ) 1989 protected StringType preferredReportName; 1990 1991 /** 1992 * Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition. 1993 */ 1994 @Child(name = "permittedUnit", type = {Coding.class}, order=33, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1995 @Description(shortDefinition="Unit for quantitative results", formalDefinition="Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition." ) 1996 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ucum-units") 1997 protected List<Coding> permittedUnit; 1998 1999 /** 2000 * A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations. 2001 */ 2002 @Child(name = "qualifiedValue", type = {}, order=34, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2003 @Description(shortDefinition="Set of qualified values for observation results", formalDefinition="A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations." ) 2004 protected List<ObservationDefinitionQualifiedValueComponent> qualifiedValue; 2005 2006 /** 2007 * This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group. 2008 */ 2009 @Child(name = "hasMember", type = {ObservationDefinition.class, Questionnaire.class}, order=35, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2010 @Description(shortDefinition="Definitions of related resources belonging to this kind of observation group", formalDefinition="This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group." ) 2011 protected List<Reference> hasMember; 2012 2013 /** 2014 * Some observations have multiple component observations, expressed as separate code value pairs. 2015 */ 2016 @Child(name = "component", type = {}, order=36, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2017 @Description(shortDefinition="Component results", formalDefinition="Some observations have multiple component observations, expressed as separate code value pairs." ) 2018 protected List<ObservationDefinitionComponentComponent> component; 2019 2020 private static final long serialVersionUID = -415480292L; 2021 2022 /** 2023 * Constructor 2024 */ 2025 public ObservationDefinition() { 2026 super(); 2027 } 2028 2029 /** 2030 * Constructor 2031 */ 2032 public ObservationDefinition(PublicationStatus status, CodeableConcept code) { 2033 super(); 2034 this.setStatus(status); 2035 this.setCode(code); 2036 } 2037 2038 /** 2039 * @return {@link #url} (An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2040 */ 2041 public UriType getUrlElement() { 2042 if (this.url == null) 2043 if (Configuration.errorOnAutoCreate()) 2044 throw new Error("Attempt to auto-create ObservationDefinition.url"); 2045 else if (Configuration.doAutoCreate()) 2046 this.url = new UriType(); // bb 2047 return this.url; 2048 } 2049 2050 public boolean hasUrlElement() { 2051 return this.url != null && !this.url.isEmpty(); 2052 } 2053 2054 public boolean hasUrl() { 2055 return this.url != null && !this.url.isEmpty(); 2056 } 2057 2058 /** 2059 * @param value {@link #url} (An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2060 */ 2061 public ObservationDefinition setUrlElement(UriType value) { 2062 this.url = value; 2063 return this; 2064 } 2065 2066 /** 2067 * @return An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions. 2068 */ 2069 public String getUrl() { 2070 return this.url == null ? null : this.url.getValue(); 2071 } 2072 2073 /** 2074 * @param value An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions. 2075 */ 2076 public ObservationDefinition setUrl(String value) { 2077 if (Utilities.noString(value)) 2078 this.url = null; 2079 else { 2080 if (this.url == null) 2081 this.url = new UriType(); 2082 this.url.setValue(value); 2083 } 2084 return this; 2085 } 2086 2087 /** 2088 * @return {@link #identifier} (Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 2089 */ 2090 public Identifier getIdentifier() { 2091 if (this.identifier == null) 2092 if (Configuration.errorOnAutoCreate()) 2093 throw new Error("Attempt to auto-create ObservationDefinition.identifier"); 2094 else if (Configuration.doAutoCreate()) 2095 this.identifier = new Identifier(); // cc 2096 return this.identifier; 2097 } 2098 2099 public boolean hasIdentifier() { 2100 return this.identifier != null && !this.identifier.isEmpty(); 2101 } 2102 2103 /** 2104 * @param value {@link #identifier} (Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 2105 */ 2106 public ObservationDefinition setIdentifier(Identifier value) { 2107 this.identifier = value; 2108 return this; 2109 } 2110 2111 /** 2112 * @return {@link #version} (The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2113 */ 2114 public StringType getVersionElement() { 2115 if (this.version == null) 2116 if (Configuration.errorOnAutoCreate()) 2117 throw new Error("Attempt to auto-create ObservationDefinition.version"); 2118 else if (Configuration.doAutoCreate()) 2119 this.version = new StringType(); // bb 2120 return this.version; 2121 } 2122 2123 public boolean hasVersionElement() { 2124 return this.version != null && !this.version.isEmpty(); 2125 } 2126 2127 public boolean hasVersion() { 2128 return this.version != null && !this.version.isEmpty(); 2129 } 2130 2131 /** 2132 * @param value {@link #version} (The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2133 */ 2134 public ObservationDefinition setVersionElement(StringType value) { 2135 this.version = value; 2136 return this; 2137 } 2138 2139 /** 2140 * @return The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2141 */ 2142 public String getVersion() { 2143 return this.version == null ? null : this.version.getValue(); 2144 } 2145 2146 /** 2147 * @param value The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2148 */ 2149 public ObservationDefinition setVersion(String value) { 2150 if (Utilities.noString(value)) 2151 this.version = null; 2152 else { 2153 if (this.version == null) 2154 this.version = new StringType(); 2155 this.version.setValue(value); 2156 } 2157 return this; 2158 } 2159 2160 /** 2161 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2162 */ 2163 public DataType getVersionAlgorithm() { 2164 return this.versionAlgorithm; 2165 } 2166 2167 /** 2168 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2169 */ 2170 public StringType getVersionAlgorithmStringType() throws FHIRException { 2171 if (this.versionAlgorithm == null) 2172 this.versionAlgorithm = new StringType(); 2173 if (!(this.versionAlgorithm instanceof StringType)) 2174 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2175 return (StringType) this.versionAlgorithm; 2176 } 2177 2178 public boolean hasVersionAlgorithmStringType() { 2179 return this.versionAlgorithm instanceof StringType; 2180 } 2181 2182 /** 2183 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2184 */ 2185 public Coding getVersionAlgorithmCoding() throws FHIRException { 2186 if (this.versionAlgorithm == null) 2187 this.versionAlgorithm = new Coding(); 2188 if (!(this.versionAlgorithm instanceof Coding)) 2189 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2190 return (Coding) this.versionAlgorithm; 2191 } 2192 2193 public boolean hasVersionAlgorithmCoding() { 2194 return this.versionAlgorithm instanceof Coding; 2195 } 2196 2197 public boolean hasVersionAlgorithm() { 2198 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2199 } 2200 2201 /** 2202 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2203 */ 2204 public ObservationDefinition setVersionAlgorithm(DataType value) { 2205 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2206 throw new FHIRException("Not the right type for ObservationDefinition.versionAlgorithm[x]: "+value.fhirType()); 2207 this.versionAlgorithm = value; 2208 return this; 2209 } 2210 2211 /** 2212 * @return {@link #name} (A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2213 */ 2214 public StringType getNameElement() { 2215 if (this.name == null) 2216 if (Configuration.errorOnAutoCreate()) 2217 throw new Error("Attempt to auto-create ObservationDefinition.name"); 2218 else if (Configuration.doAutoCreate()) 2219 this.name = new StringType(); // bb 2220 return this.name; 2221 } 2222 2223 public boolean hasNameElement() { 2224 return this.name != null && !this.name.isEmpty(); 2225 } 2226 2227 public boolean hasName() { 2228 return this.name != null && !this.name.isEmpty(); 2229 } 2230 2231 /** 2232 * @param value {@link #name} (A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2233 */ 2234 public ObservationDefinition setNameElement(StringType value) { 2235 this.name = value; 2236 return this; 2237 } 2238 2239 /** 2240 * @return A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2241 */ 2242 public String getName() { 2243 return this.name == null ? null : this.name.getValue(); 2244 } 2245 2246 /** 2247 * @param value A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2248 */ 2249 public ObservationDefinition setName(String value) { 2250 if (Utilities.noString(value)) 2251 this.name = null; 2252 else { 2253 if (this.name == null) 2254 this.name = new StringType(); 2255 this.name.setValue(value); 2256 } 2257 return this; 2258 } 2259 2260 /** 2261 * @return {@link #title} (A short, descriptive, user-friendly title for the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2262 */ 2263 public StringType getTitleElement() { 2264 if (this.title == null) 2265 if (Configuration.errorOnAutoCreate()) 2266 throw new Error("Attempt to auto-create ObservationDefinition.title"); 2267 else if (Configuration.doAutoCreate()) 2268 this.title = new StringType(); // bb 2269 return this.title; 2270 } 2271 2272 public boolean hasTitleElement() { 2273 return this.title != null && !this.title.isEmpty(); 2274 } 2275 2276 public boolean hasTitle() { 2277 return this.title != null && !this.title.isEmpty(); 2278 } 2279 2280 /** 2281 * @param value {@link #title} (A short, descriptive, user-friendly title for the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2282 */ 2283 public ObservationDefinition setTitleElement(StringType value) { 2284 this.title = value; 2285 return this; 2286 } 2287 2288 /** 2289 * @return A short, descriptive, user-friendly title for the ObservationDefinition. 2290 */ 2291 public String getTitle() { 2292 return this.title == null ? null : this.title.getValue(); 2293 } 2294 2295 /** 2296 * @param value A short, descriptive, user-friendly title for the ObservationDefinition. 2297 */ 2298 public ObservationDefinition setTitle(String value) { 2299 if (Utilities.noString(value)) 2300 this.title = null; 2301 else { 2302 if (this.title == null) 2303 this.title = new StringType(); 2304 this.title.setValue(value); 2305 } 2306 return this; 2307 } 2308 2309 /** 2310 * @return {@link #status} (The current state of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2311 */ 2312 public Enumeration<PublicationStatus> getStatusElement() { 2313 if (this.status == null) 2314 if (Configuration.errorOnAutoCreate()) 2315 throw new Error("Attempt to auto-create ObservationDefinition.status"); 2316 else if (Configuration.doAutoCreate()) 2317 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2318 return this.status; 2319 } 2320 2321 public boolean hasStatusElement() { 2322 return this.status != null && !this.status.isEmpty(); 2323 } 2324 2325 public boolean hasStatus() { 2326 return this.status != null && !this.status.isEmpty(); 2327 } 2328 2329 /** 2330 * @param value {@link #status} (The current state of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2331 */ 2332 public ObservationDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2333 this.status = value; 2334 return this; 2335 } 2336 2337 /** 2338 * @return The current state of the ObservationDefinition. 2339 */ 2340 public PublicationStatus getStatus() { 2341 return this.status == null ? null : this.status.getValue(); 2342 } 2343 2344 /** 2345 * @param value The current state of the ObservationDefinition. 2346 */ 2347 public ObservationDefinition setStatus(PublicationStatus value) { 2348 if (this.status == null) 2349 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2350 this.status.setValue(value); 2351 return this; 2352 } 2353 2354 /** 2355 * @return {@link #experimental} (A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2356 */ 2357 public BooleanType getExperimentalElement() { 2358 if (this.experimental == null) 2359 if (Configuration.errorOnAutoCreate()) 2360 throw new Error("Attempt to auto-create ObservationDefinition.experimental"); 2361 else if (Configuration.doAutoCreate()) 2362 this.experimental = new BooleanType(); // bb 2363 return this.experimental; 2364 } 2365 2366 public boolean hasExperimentalElement() { 2367 return this.experimental != null && !this.experimental.isEmpty(); 2368 } 2369 2370 public boolean hasExperimental() { 2371 return this.experimental != null && !this.experimental.isEmpty(); 2372 } 2373 2374 /** 2375 * @param value {@link #experimental} (A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2376 */ 2377 public ObservationDefinition setExperimentalElement(BooleanType value) { 2378 this.experimental = value; 2379 return this; 2380 } 2381 2382 /** 2383 * @return A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2384 */ 2385 public boolean getExperimental() { 2386 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2387 } 2388 2389 /** 2390 * @param value A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2391 */ 2392 public ObservationDefinition setExperimental(boolean value) { 2393 if (this.experimental == null) 2394 this.experimental = new BooleanType(); 2395 this.experimental.setValue(value); 2396 return this; 2397 } 2398 2399 /** 2400 * @return {@link #date} (The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2401 */ 2402 public DateTimeType getDateElement() { 2403 if (this.date == null) 2404 if (Configuration.errorOnAutoCreate()) 2405 throw new Error("Attempt to auto-create ObservationDefinition.date"); 2406 else if (Configuration.doAutoCreate()) 2407 this.date = new DateTimeType(); // bb 2408 return this.date; 2409 } 2410 2411 public boolean hasDateElement() { 2412 return this.date != null && !this.date.isEmpty(); 2413 } 2414 2415 public boolean hasDate() { 2416 return this.date != null && !this.date.isEmpty(); 2417 } 2418 2419 /** 2420 * @param value {@link #date} (The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2421 */ 2422 public ObservationDefinition setDateElement(DateTimeType value) { 2423 this.date = value; 2424 return this; 2425 } 2426 2427 /** 2428 * @return The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes. 2429 */ 2430 public Date getDate() { 2431 return this.date == null ? null : this.date.getValue(); 2432 } 2433 2434 /** 2435 * @param value The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes. 2436 */ 2437 public ObservationDefinition setDate(Date value) { 2438 if (value == null) 2439 this.date = null; 2440 else { 2441 if (this.date == null) 2442 this.date = new DateTimeType(); 2443 this.date.setValue(value); 2444 } 2445 return this; 2446 } 2447 2448 /** 2449 * @return {@link #publisher} (Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2450 */ 2451 public StringType getPublisherElement() { 2452 if (this.publisher == null) 2453 if (Configuration.errorOnAutoCreate()) 2454 throw new Error("Attempt to auto-create ObservationDefinition.publisher"); 2455 else if (Configuration.doAutoCreate()) 2456 this.publisher = new StringType(); // bb 2457 return this.publisher; 2458 } 2459 2460 public boolean hasPublisherElement() { 2461 return this.publisher != null && !this.publisher.isEmpty(); 2462 } 2463 2464 public boolean hasPublisher() { 2465 return this.publisher != null && !this.publisher.isEmpty(); 2466 } 2467 2468 /** 2469 * @param value {@link #publisher} (Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2470 */ 2471 public ObservationDefinition setPublisherElement(StringType value) { 2472 this.publisher = value; 2473 return this; 2474 } 2475 2476 /** 2477 * @return Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact. 2478 */ 2479 public String getPublisher() { 2480 return this.publisher == null ? null : this.publisher.getValue(); 2481 } 2482 2483 /** 2484 * @param value Helps establish the "authority/credibility" of the ObservationDefinition. May also allow for contact. 2485 */ 2486 public ObservationDefinition setPublisher(String value) { 2487 if (Utilities.noString(value)) 2488 this.publisher = null; 2489 else { 2490 if (this.publisher == null) 2491 this.publisher = new StringType(); 2492 this.publisher.setValue(value); 2493 } 2494 return this; 2495 } 2496 2497 /** 2498 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2499 */ 2500 public List<ContactDetail> getContact() { 2501 if (this.contact == null) 2502 this.contact = new ArrayList<ContactDetail>(); 2503 return this.contact; 2504 } 2505 2506 /** 2507 * @return Returns a reference to <code>this</code> for easy method chaining 2508 */ 2509 public ObservationDefinition setContact(List<ContactDetail> theContact) { 2510 this.contact = theContact; 2511 return this; 2512 } 2513 2514 public boolean hasContact() { 2515 if (this.contact == null) 2516 return false; 2517 for (ContactDetail item : this.contact) 2518 if (!item.isEmpty()) 2519 return true; 2520 return false; 2521 } 2522 2523 public ContactDetail addContact() { //3 2524 ContactDetail t = new ContactDetail(); 2525 if (this.contact == null) 2526 this.contact = new ArrayList<ContactDetail>(); 2527 this.contact.add(t); 2528 return t; 2529 } 2530 2531 public ObservationDefinition addContact(ContactDetail t) { //3 2532 if (t == null) 2533 return this; 2534 if (this.contact == null) 2535 this.contact = new ArrayList<ContactDetail>(); 2536 this.contact.add(t); 2537 return this; 2538 } 2539 2540 /** 2541 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2542 */ 2543 public ContactDetail getContactFirstRep() { 2544 if (getContact().isEmpty()) { 2545 addContact(); 2546 } 2547 return getContact().get(0); 2548 } 2549 2550 /** 2551 * @return {@link #description} (A free text natural language description of the ObservationDefinition from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2552 */ 2553 public MarkdownType getDescriptionElement() { 2554 if (this.description == null) 2555 if (Configuration.errorOnAutoCreate()) 2556 throw new Error("Attempt to auto-create ObservationDefinition.description"); 2557 else if (Configuration.doAutoCreate()) 2558 this.description = new MarkdownType(); // bb 2559 return this.description; 2560 } 2561 2562 public boolean hasDescriptionElement() { 2563 return this.description != null && !this.description.isEmpty(); 2564 } 2565 2566 public boolean hasDescription() { 2567 return this.description != null && !this.description.isEmpty(); 2568 } 2569 2570 /** 2571 * @param value {@link #description} (A free text natural language description of the ObservationDefinition from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2572 */ 2573 public ObservationDefinition setDescriptionElement(MarkdownType value) { 2574 this.description = value; 2575 return this; 2576 } 2577 2578 /** 2579 * @return A free text natural language description of the ObservationDefinition from the consumer's perspective. 2580 */ 2581 public String getDescription() { 2582 return this.description == null ? null : this.description.getValue(); 2583 } 2584 2585 /** 2586 * @param value A free text natural language description of the ObservationDefinition from the consumer's perspective. 2587 */ 2588 public ObservationDefinition setDescription(String value) { 2589 if (Utilities.noString(value)) 2590 this.description = null; 2591 else { 2592 if (this.description == null) 2593 this.description = new MarkdownType(); 2594 this.description.setValue(value); 2595 } 2596 return this; 2597 } 2598 2599 /** 2600 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances.) 2601 */ 2602 public List<UsageContext> getUseContext() { 2603 if (this.useContext == null) 2604 this.useContext = new ArrayList<UsageContext>(); 2605 return this.useContext; 2606 } 2607 2608 /** 2609 * @return Returns a reference to <code>this</code> for easy method chaining 2610 */ 2611 public ObservationDefinition setUseContext(List<UsageContext> theUseContext) { 2612 this.useContext = theUseContext; 2613 return this; 2614 } 2615 2616 public boolean hasUseContext() { 2617 if (this.useContext == null) 2618 return false; 2619 for (UsageContext item : this.useContext) 2620 if (!item.isEmpty()) 2621 return true; 2622 return false; 2623 } 2624 2625 public UsageContext addUseContext() { //3 2626 UsageContext t = new UsageContext(); 2627 if (this.useContext == null) 2628 this.useContext = new ArrayList<UsageContext>(); 2629 this.useContext.add(t); 2630 return t; 2631 } 2632 2633 public ObservationDefinition addUseContext(UsageContext t) { //3 2634 if (t == null) 2635 return this; 2636 if (this.useContext == null) 2637 this.useContext = new ArrayList<UsageContext>(); 2638 this.useContext.add(t); 2639 return this; 2640 } 2641 2642 /** 2643 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2644 */ 2645 public UsageContext getUseContextFirstRep() { 2646 if (getUseContext().isEmpty()) { 2647 addUseContext(); 2648 } 2649 return getUseContext().get(0); 2650 } 2651 2652 /** 2653 * @return {@link #jurisdiction} (A jurisdiction in which the ObservationDefinition is intended to be used.) 2654 */ 2655 public List<CodeableConcept> getJurisdiction() { 2656 if (this.jurisdiction == null) 2657 this.jurisdiction = new ArrayList<CodeableConcept>(); 2658 return this.jurisdiction; 2659 } 2660 2661 /** 2662 * @return Returns a reference to <code>this</code> for easy method chaining 2663 */ 2664 public ObservationDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2665 this.jurisdiction = theJurisdiction; 2666 return this; 2667 } 2668 2669 public boolean hasJurisdiction() { 2670 if (this.jurisdiction == null) 2671 return false; 2672 for (CodeableConcept item : this.jurisdiction) 2673 if (!item.isEmpty()) 2674 return true; 2675 return false; 2676 } 2677 2678 public CodeableConcept addJurisdiction() { //3 2679 CodeableConcept t = new CodeableConcept(); 2680 if (this.jurisdiction == null) 2681 this.jurisdiction = new ArrayList<CodeableConcept>(); 2682 this.jurisdiction.add(t); 2683 return t; 2684 } 2685 2686 public ObservationDefinition addJurisdiction(CodeableConcept t) { //3 2687 if (t == null) 2688 return this; 2689 if (this.jurisdiction == null) 2690 this.jurisdiction = new ArrayList<CodeableConcept>(); 2691 this.jurisdiction.add(t); 2692 return this; 2693 } 2694 2695 /** 2696 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2697 */ 2698 public CodeableConcept getJurisdictionFirstRep() { 2699 if (getJurisdiction().isEmpty()) { 2700 addJurisdiction(); 2701 } 2702 return getJurisdiction().get(0); 2703 } 2704 2705 /** 2706 * @return {@link #purpose} (Explains why this ObservationDefinition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2707 */ 2708 public MarkdownType getPurposeElement() { 2709 if (this.purpose == null) 2710 if (Configuration.errorOnAutoCreate()) 2711 throw new Error("Attempt to auto-create ObservationDefinition.purpose"); 2712 else if (Configuration.doAutoCreate()) 2713 this.purpose = new MarkdownType(); // bb 2714 return this.purpose; 2715 } 2716 2717 public boolean hasPurposeElement() { 2718 return this.purpose != null && !this.purpose.isEmpty(); 2719 } 2720 2721 public boolean hasPurpose() { 2722 return this.purpose != null && !this.purpose.isEmpty(); 2723 } 2724 2725 /** 2726 * @param value {@link #purpose} (Explains why this ObservationDefinition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2727 */ 2728 public ObservationDefinition setPurposeElement(MarkdownType value) { 2729 this.purpose = value; 2730 return this; 2731 } 2732 2733 /** 2734 * @return Explains why this ObservationDefinition is needed and why it has been designed as it has. 2735 */ 2736 public String getPurpose() { 2737 return this.purpose == null ? null : this.purpose.getValue(); 2738 } 2739 2740 /** 2741 * @param value Explains why this ObservationDefinition is needed and why it has been designed as it has. 2742 */ 2743 public ObservationDefinition setPurpose(String value) { 2744 if (Utilities.noString(value)) 2745 this.purpose = null; 2746 else { 2747 if (this.purpose == null) 2748 this.purpose = new MarkdownType(); 2749 this.purpose.setValue(value); 2750 } 2751 return this; 2752 } 2753 2754 /** 2755 * @return {@link #copyright} (Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2756 */ 2757 public MarkdownType getCopyrightElement() { 2758 if (this.copyright == null) 2759 if (Configuration.errorOnAutoCreate()) 2760 throw new Error("Attempt to auto-create ObservationDefinition.copyright"); 2761 else if (Configuration.doAutoCreate()) 2762 this.copyright = new MarkdownType(); // bb 2763 return this.copyright; 2764 } 2765 2766 public boolean hasCopyrightElement() { 2767 return this.copyright != null && !this.copyright.isEmpty(); 2768 } 2769 2770 public boolean hasCopyright() { 2771 return this.copyright != null && !this.copyright.isEmpty(); 2772 } 2773 2774 /** 2775 * @param value {@link #copyright} (Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2776 */ 2777 public ObservationDefinition setCopyrightElement(MarkdownType value) { 2778 this.copyright = value; 2779 return this; 2780 } 2781 2782 /** 2783 * @return Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition. 2784 */ 2785 public String getCopyright() { 2786 return this.copyright == null ? null : this.copyright.getValue(); 2787 } 2788 2789 /** 2790 * @param value Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition. 2791 */ 2792 public ObservationDefinition setCopyright(String value) { 2793 if (Utilities.noString(value)) 2794 this.copyright = null; 2795 else { 2796 if (this.copyright == null) 2797 this.copyright = new MarkdownType(); 2798 this.copyright.setValue(value); 2799 } 2800 return this; 2801 } 2802 2803 /** 2804 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2805 */ 2806 public StringType getCopyrightLabelElement() { 2807 if (this.copyrightLabel == null) 2808 if (Configuration.errorOnAutoCreate()) 2809 throw new Error("Attempt to auto-create ObservationDefinition.copyrightLabel"); 2810 else if (Configuration.doAutoCreate()) 2811 this.copyrightLabel = new StringType(); // bb 2812 return this.copyrightLabel; 2813 } 2814 2815 public boolean hasCopyrightLabelElement() { 2816 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2817 } 2818 2819 public boolean hasCopyrightLabel() { 2820 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2821 } 2822 2823 /** 2824 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2825 */ 2826 public ObservationDefinition setCopyrightLabelElement(StringType value) { 2827 this.copyrightLabel = value; 2828 return this; 2829 } 2830 2831 /** 2832 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2833 */ 2834 public String getCopyrightLabel() { 2835 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2836 } 2837 2838 /** 2839 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2840 */ 2841 public ObservationDefinition setCopyrightLabel(String value) { 2842 if (Utilities.noString(value)) 2843 this.copyrightLabel = null; 2844 else { 2845 if (this.copyrightLabel == null) 2846 this.copyrightLabel = new StringType(); 2847 this.copyrightLabel.setValue(value); 2848 } 2849 return this; 2850 } 2851 2852 /** 2853 * @return {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2854 */ 2855 public DateType getApprovalDateElement() { 2856 if (this.approvalDate == null) 2857 if (Configuration.errorOnAutoCreate()) 2858 throw new Error("Attempt to auto-create ObservationDefinition.approvalDate"); 2859 else if (Configuration.doAutoCreate()) 2860 this.approvalDate = new DateType(); // bb 2861 return this.approvalDate; 2862 } 2863 2864 public boolean hasApprovalDateElement() { 2865 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2866 } 2867 2868 public boolean hasApprovalDate() { 2869 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2870 } 2871 2872 /** 2873 * @param value {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2874 */ 2875 public ObservationDefinition setApprovalDateElement(DateType value) { 2876 this.approvalDate = value; 2877 return this; 2878 } 2879 2880 /** 2881 * @return The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2882 */ 2883 public Date getApprovalDate() { 2884 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2885 } 2886 2887 /** 2888 * @param value The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2889 */ 2890 public ObservationDefinition setApprovalDate(Date value) { 2891 if (value == null) 2892 this.approvalDate = null; 2893 else { 2894 if (this.approvalDate == null) 2895 this.approvalDate = new DateType(); 2896 this.approvalDate.setValue(value); 2897 } 2898 return this; 2899 } 2900 2901 /** 2902 * @return {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2903 */ 2904 public DateType getLastReviewDateElement() { 2905 if (this.lastReviewDate == null) 2906 if (Configuration.errorOnAutoCreate()) 2907 throw new Error("Attempt to auto-create ObservationDefinition.lastReviewDate"); 2908 else if (Configuration.doAutoCreate()) 2909 this.lastReviewDate = new DateType(); // bb 2910 return this.lastReviewDate; 2911 } 2912 2913 public boolean hasLastReviewDateElement() { 2914 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2915 } 2916 2917 public boolean hasLastReviewDate() { 2918 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2919 } 2920 2921 /** 2922 * @param value {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2923 */ 2924 public ObservationDefinition setLastReviewDateElement(DateType value) { 2925 this.lastReviewDate = value; 2926 return this; 2927 } 2928 2929 /** 2930 * @return The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 2931 */ 2932 public Date getLastReviewDate() { 2933 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2934 } 2935 2936 /** 2937 * @param value The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 2938 */ 2939 public ObservationDefinition setLastReviewDate(Date value) { 2940 if (value == null) 2941 this.lastReviewDate = null; 2942 else { 2943 if (this.lastReviewDate == null) 2944 this.lastReviewDate = new DateType(); 2945 this.lastReviewDate.setValue(value); 2946 } 2947 return this; 2948 } 2949 2950 /** 2951 * @return {@link #effectivePeriod} (The period during which the ObservationDefinition content was or is planned to be effective.) 2952 */ 2953 public Period getEffectivePeriod() { 2954 if (this.effectivePeriod == null) 2955 if (Configuration.errorOnAutoCreate()) 2956 throw new Error("Attempt to auto-create ObservationDefinition.effectivePeriod"); 2957 else if (Configuration.doAutoCreate()) 2958 this.effectivePeriod = new Period(); // cc 2959 return this.effectivePeriod; 2960 } 2961 2962 public boolean hasEffectivePeriod() { 2963 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2964 } 2965 2966 /** 2967 * @param value {@link #effectivePeriod} (The period during which the ObservationDefinition content was or is planned to be effective.) 2968 */ 2969 public ObservationDefinition setEffectivePeriod(Period value) { 2970 this.effectivePeriod = value; 2971 return this; 2972 } 2973 2974 /** 2975 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 2976 */ 2977 public List<CanonicalType> getDerivedFromCanonical() { 2978 if (this.derivedFromCanonical == null) 2979 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 2980 return this.derivedFromCanonical; 2981 } 2982 2983 /** 2984 * @return Returns a reference to <code>this</code> for easy method chaining 2985 */ 2986 public ObservationDefinition setDerivedFromCanonical(List<CanonicalType> theDerivedFromCanonical) { 2987 this.derivedFromCanonical = theDerivedFromCanonical; 2988 return this; 2989 } 2990 2991 public boolean hasDerivedFromCanonical() { 2992 if (this.derivedFromCanonical == null) 2993 return false; 2994 for (CanonicalType item : this.derivedFromCanonical) 2995 if (!item.isEmpty()) 2996 return true; 2997 return false; 2998 } 2999 3000 /** 3001 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 3002 */ 3003 public CanonicalType addDerivedFromCanonicalElement() {//2 3004 CanonicalType t = new CanonicalType(); 3005 if (this.derivedFromCanonical == null) 3006 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 3007 this.derivedFromCanonical.add(t); 3008 return t; 3009 } 3010 3011 /** 3012 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 3013 */ 3014 public ObservationDefinition addDerivedFromCanonical(String value) { //1 3015 CanonicalType t = new CanonicalType(); 3016 t.setValue(value); 3017 if (this.derivedFromCanonical == null) 3018 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 3019 this.derivedFromCanonical.add(t); 3020 return this; 3021 } 3022 3023 /** 3024 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.) 3025 */ 3026 public boolean hasDerivedFromCanonical(String value) { 3027 if (this.derivedFromCanonical == null) 3028 return false; 3029 for (CanonicalType v : this.derivedFromCanonical) 3030 if (v.getValue().equals(value)) // canonical 3031 return true; 3032 return false; 3033 } 3034 3035 /** 3036 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 3037 */ 3038 public List<UriType> getDerivedFromUri() { 3039 if (this.derivedFromUri == null) 3040 this.derivedFromUri = new ArrayList<UriType>(); 3041 return this.derivedFromUri; 3042 } 3043 3044 /** 3045 * @return Returns a reference to <code>this</code> for easy method chaining 3046 */ 3047 public ObservationDefinition setDerivedFromUri(List<UriType> theDerivedFromUri) { 3048 this.derivedFromUri = theDerivedFromUri; 3049 return this; 3050 } 3051 3052 public boolean hasDerivedFromUri() { 3053 if (this.derivedFromUri == null) 3054 return false; 3055 for (UriType item : this.derivedFromUri) 3056 if (!item.isEmpty()) 3057 return true; 3058 return false; 3059 } 3060 3061 /** 3062 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 3063 */ 3064 public UriType addDerivedFromUriElement() {//2 3065 UriType t = new UriType(); 3066 if (this.derivedFromUri == null) 3067 this.derivedFromUri = new ArrayList<UriType>(); 3068 this.derivedFromUri.add(t); 3069 return t; 3070 } 3071 3072 /** 3073 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 3074 */ 3075 public ObservationDefinition addDerivedFromUri(String value) { //1 3076 UriType t = new UriType(); 3077 t.setValue(value); 3078 if (this.derivedFromUri == null) 3079 this.derivedFromUri = new ArrayList<UriType>(); 3080 this.derivedFromUri.add(t); 3081 return this; 3082 } 3083 3084 /** 3085 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.) 3086 */ 3087 public boolean hasDerivedFromUri(String value) { 3088 if (this.derivedFromUri == null) 3089 return false; 3090 for (UriType v : this.derivedFromUri) 3091 if (v.getValue().equals(value)) // uri 3092 return true; 3093 return false; 3094 } 3095 3096 /** 3097 * @return {@link #subject} (A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition.) 3098 */ 3099 public List<CodeableConcept> getSubject() { 3100 if (this.subject == null) 3101 this.subject = new ArrayList<CodeableConcept>(); 3102 return this.subject; 3103 } 3104 3105 /** 3106 * @return Returns a reference to <code>this</code> for easy method chaining 3107 */ 3108 public ObservationDefinition setSubject(List<CodeableConcept> theSubject) { 3109 this.subject = theSubject; 3110 return this; 3111 } 3112 3113 public boolean hasSubject() { 3114 if (this.subject == null) 3115 return false; 3116 for (CodeableConcept item : this.subject) 3117 if (!item.isEmpty()) 3118 return true; 3119 return false; 3120 } 3121 3122 public CodeableConcept addSubject() { //3 3123 CodeableConcept t = new CodeableConcept(); 3124 if (this.subject == null) 3125 this.subject = new ArrayList<CodeableConcept>(); 3126 this.subject.add(t); 3127 return t; 3128 } 3129 3130 public ObservationDefinition addSubject(CodeableConcept t) { //3 3131 if (t == null) 3132 return this; 3133 if (this.subject == null) 3134 this.subject = new ArrayList<CodeableConcept>(); 3135 this.subject.add(t); 3136 return this; 3137 } 3138 3139 /** 3140 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 3141 */ 3142 public CodeableConcept getSubjectFirstRep() { 3143 if (getSubject().isEmpty()) { 3144 addSubject(); 3145 } 3146 return getSubject().get(0); 3147 } 3148 3149 /** 3150 * @return {@link #performerType} (The type of individual/organization/device that is expected to act upon instances of this definition.) 3151 */ 3152 public CodeableConcept getPerformerType() { 3153 if (this.performerType == null) 3154 if (Configuration.errorOnAutoCreate()) 3155 throw new Error("Attempt to auto-create ObservationDefinition.performerType"); 3156 else if (Configuration.doAutoCreate()) 3157 this.performerType = new CodeableConcept(); // cc 3158 return this.performerType; 3159 } 3160 3161 public boolean hasPerformerType() { 3162 return this.performerType != null && !this.performerType.isEmpty(); 3163 } 3164 3165 /** 3166 * @param value {@link #performerType} (The type of individual/organization/device that is expected to act upon instances of this definition.) 3167 */ 3168 public ObservationDefinition setPerformerType(CodeableConcept value) { 3169 this.performerType = value; 3170 return this; 3171 } 3172 3173 /** 3174 * @return {@link #category} (A code that classifies the general type of observation.) 3175 */ 3176 public List<CodeableConcept> getCategory() { 3177 if (this.category == null) 3178 this.category = new ArrayList<CodeableConcept>(); 3179 return this.category; 3180 } 3181 3182 /** 3183 * @return Returns a reference to <code>this</code> for easy method chaining 3184 */ 3185 public ObservationDefinition setCategory(List<CodeableConcept> theCategory) { 3186 this.category = theCategory; 3187 return this; 3188 } 3189 3190 public boolean hasCategory() { 3191 if (this.category == null) 3192 return false; 3193 for (CodeableConcept item : this.category) 3194 if (!item.isEmpty()) 3195 return true; 3196 return false; 3197 } 3198 3199 public CodeableConcept addCategory() { //3 3200 CodeableConcept t = new CodeableConcept(); 3201 if (this.category == null) 3202 this.category = new ArrayList<CodeableConcept>(); 3203 this.category.add(t); 3204 return t; 3205 } 3206 3207 public ObservationDefinition addCategory(CodeableConcept t) { //3 3208 if (t == null) 3209 return this; 3210 if (this.category == null) 3211 this.category = new ArrayList<CodeableConcept>(); 3212 this.category.add(t); 3213 return this; 3214 } 3215 3216 /** 3217 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 3218 */ 3219 public CodeableConcept getCategoryFirstRep() { 3220 if (getCategory().isEmpty()) { 3221 addCategory(); 3222 } 3223 return getCategory().get(0); 3224 } 3225 3226 /** 3227 * @return {@link #code} (Describes what will be observed. Sometimes this is called the observation "name".) 3228 */ 3229 public CodeableConcept getCode() { 3230 if (this.code == null) 3231 if (Configuration.errorOnAutoCreate()) 3232 throw new Error("Attempt to auto-create ObservationDefinition.code"); 3233 else if (Configuration.doAutoCreate()) 3234 this.code = new CodeableConcept(); // cc 3235 return this.code; 3236 } 3237 3238 public boolean hasCode() { 3239 return this.code != null && !this.code.isEmpty(); 3240 } 3241 3242 /** 3243 * @param value {@link #code} (Describes what will be observed. Sometimes this is called the observation "name".) 3244 */ 3245 public ObservationDefinition setCode(CodeableConcept value) { 3246 this.code = value; 3247 return this; 3248 } 3249 3250 /** 3251 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3252 */ 3253 public List<Enumeration<ObservationDataType>> getPermittedDataType() { 3254 if (this.permittedDataType == null) 3255 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 3256 return this.permittedDataType; 3257 } 3258 3259 /** 3260 * @return Returns a reference to <code>this</code> for easy method chaining 3261 */ 3262 public ObservationDefinition setPermittedDataType(List<Enumeration<ObservationDataType>> thePermittedDataType) { 3263 this.permittedDataType = thePermittedDataType; 3264 return this; 3265 } 3266 3267 public boolean hasPermittedDataType() { 3268 if (this.permittedDataType == null) 3269 return false; 3270 for (Enumeration<ObservationDataType> item : this.permittedDataType) 3271 if (!item.isEmpty()) 3272 return true; 3273 return false; 3274 } 3275 3276 /** 3277 * @return {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3278 */ 3279 public Enumeration<ObservationDataType> addPermittedDataTypeElement() {//2 3280 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 3281 if (this.permittedDataType == null) 3282 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 3283 this.permittedDataType.add(t); 3284 return t; 3285 } 3286 3287 /** 3288 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3289 */ 3290 public ObservationDefinition addPermittedDataType(ObservationDataType value) { //1 3291 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 3292 t.setValue(value); 3293 if (this.permittedDataType == null) 3294 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 3295 this.permittedDataType.add(t); 3296 return this; 3297 } 3298 3299 /** 3300 * @param value {@link #permittedDataType} (The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.) 3301 */ 3302 public boolean hasPermittedDataType(ObservationDataType value) { 3303 if (this.permittedDataType == null) 3304 return false; 3305 for (Enumeration<ObservationDataType> v : this.permittedDataType) 3306 if (v.getValue().equals(value)) // code 3307 return true; 3308 return false; 3309 } 3310 3311 /** 3312 * @return {@link #multipleResultsAllowed} (Multiple results allowed for observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getMultipleResultsAllowed" gives direct access to the value 3313 */ 3314 public BooleanType getMultipleResultsAllowedElement() { 3315 if (this.multipleResultsAllowed == null) 3316 if (Configuration.errorOnAutoCreate()) 3317 throw new Error("Attempt to auto-create ObservationDefinition.multipleResultsAllowed"); 3318 else if (Configuration.doAutoCreate()) 3319 this.multipleResultsAllowed = new BooleanType(); // bb 3320 return this.multipleResultsAllowed; 3321 } 3322 3323 public boolean hasMultipleResultsAllowedElement() { 3324 return this.multipleResultsAllowed != null && !this.multipleResultsAllowed.isEmpty(); 3325 } 3326 3327 public boolean hasMultipleResultsAllowed() { 3328 return this.multipleResultsAllowed != null && !this.multipleResultsAllowed.isEmpty(); 3329 } 3330 3331 /** 3332 * @param value {@link #multipleResultsAllowed} (Multiple results allowed for observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getMultipleResultsAllowed" gives direct access to the value 3333 */ 3334 public ObservationDefinition setMultipleResultsAllowedElement(BooleanType value) { 3335 this.multipleResultsAllowed = value; 3336 return this; 3337 } 3338 3339 /** 3340 * @return Multiple results allowed for observations conforming to this ObservationDefinition. 3341 */ 3342 public boolean getMultipleResultsAllowed() { 3343 return this.multipleResultsAllowed == null || this.multipleResultsAllowed.isEmpty() ? false : this.multipleResultsAllowed.getValue(); 3344 } 3345 3346 /** 3347 * @param value Multiple results allowed for observations conforming to this ObservationDefinition. 3348 */ 3349 public ObservationDefinition setMultipleResultsAllowed(boolean value) { 3350 if (this.multipleResultsAllowed == null) 3351 this.multipleResultsAllowed = new BooleanType(); 3352 this.multipleResultsAllowed.setValue(value); 3353 return this; 3354 } 3355 3356 /** 3357 * @return {@link #bodySite} (The site on the subject's body where the observation is to be made.) 3358 */ 3359 public CodeableConcept getBodySite() { 3360 if (this.bodySite == null) 3361 if (Configuration.errorOnAutoCreate()) 3362 throw new Error("Attempt to auto-create ObservationDefinition.bodySite"); 3363 else if (Configuration.doAutoCreate()) 3364 this.bodySite = new CodeableConcept(); // cc 3365 return this.bodySite; 3366 } 3367 3368 public boolean hasBodySite() { 3369 return this.bodySite != null && !this.bodySite.isEmpty(); 3370 } 3371 3372 /** 3373 * @param value {@link #bodySite} (The site on the subject's body where the observation is to be made.) 3374 */ 3375 public ObservationDefinition setBodySite(CodeableConcept value) { 3376 this.bodySite = value; 3377 return this; 3378 } 3379 3380 /** 3381 * @return {@link #method} (The method or technique used to perform the observation.) 3382 */ 3383 public CodeableConcept getMethod() { 3384 if (this.method == null) 3385 if (Configuration.errorOnAutoCreate()) 3386 throw new Error("Attempt to auto-create ObservationDefinition.method"); 3387 else if (Configuration.doAutoCreate()) 3388 this.method = new CodeableConcept(); // cc 3389 return this.method; 3390 } 3391 3392 public boolean hasMethod() { 3393 return this.method != null && !this.method.isEmpty(); 3394 } 3395 3396 /** 3397 * @param value {@link #method} (The method or technique used to perform the observation.) 3398 */ 3399 public ObservationDefinition setMethod(CodeableConcept value) { 3400 this.method = value; 3401 return this; 3402 } 3403 3404 /** 3405 * @return {@link #specimen} (The kind of specimen that this type of observation is produced on.) 3406 */ 3407 public List<Reference> getSpecimen() { 3408 if (this.specimen == null) 3409 this.specimen = new ArrayList<Reference>(); 3410 return this.specimen; 3411 } 3412 3413 /** 3414 * @return Returns a reference to <code>this</code> for easy method chaining 3415 */ 3416 public ObservationDefinition setSpecimen(List<Reference> theSpecimen) { 3417 this.specimen = theSpecimen; 3418 return this; 3419 } 3420 3421 public boolean hasSpecimen() { 3422 if (this.specimen == null) 3423 return false; 3424 for (Reference item : this.specimen) 3425 if (!item.isEmpty()) 3426 return true; 3427 return false; 3428 } 3429 3430 public Reference addSpecimen() { //3 3431 Reference t = new Reference(); 3432 if (this.specimen == null) 3433 this.specimen = new ArrayList<Reference>(); 3434 this.specimen.add(t); 3435 return t; 3436 } 3437 3438 public ObservationDefinition addSpecimen(Reference t) { //3 3439 if (t == null) 3440 return this; 3441 if (this.specimen == null) 3442 this.specimen = new ArrayList<Reference>(); 3443 this.specimen.add(t); 3444 return this; 3445 } 3446 3447 /** 3448 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist {3} 3449 */ 3450 public Reference getSpecimenFirstRep() { 3451 if (getSpecimen().isEmpty()) { 3452 addSpecimen(); 3453 } 3454 return getSpecimen().get(0); 3455 } 3456 3457 /** 3458 * @return {@link #device} (The measurement model of device or actual device used to produce observations of this type.) 3459 */ 3460 public List<Reference> getDevice() { 3461 if (this.device == null) 3462 this.device = new ArrayList<Reference>(); 3463 return this.device; 3464 } 3465 3466 /** 3467 * @return Returns a reference to <code>this</code> for easy method chaining 3468 */ 3469 public ObservationDefinition setDevice(List<Reference> theDevice) { 3470 this.device = theDevice; 3471 return this; 3472 } 3473 3474 public boolean hasDevice() { 3475 if (this.device == null) 3476 return false; 3477 for (Reference item : this.device) 3478 if (!item.isEmpty()) 3479 return true; 3480 return false; 3481 } 3482 3483 public Reference addDevice() { //3 3484 Reference t = new Reference(); 3485 if (this.device == null) 3486 this.device = new ArrayList<Reference>(); 3487 this.device.add(t); 3488 return t; 3489 } 3490 3491 public ObservationDefinition addDevice(Reference t) { //3 3492 if (t == null) 3493 return this; 3494 if (this.device == null) 3495 this.device = new ArrayList<Reference>(); 3496 this.device.add(t); 3497 return this; 3498 } 3499 3500 /** 3501 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 3502 */ 3503 public Reference getDeviceFirstRep() { 3504 if (getDevice().isEmpty()) { 3505 addDevice(); 3506 } 3507 return getDevice().get(0); 3508 } 3509 3510 /** 3511 * @return {@link #preferredReportName} (The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getPreferredReportName" gives direct access to the value 3512 */ 3513 public StringType getPreferredReportNameElement() { 3514 if (this.preferredReportName == null) 3515 if (Configuration.errorOnAutoCreate()) 3516 throw new Error("Attempt to auto-create ObservationDefinition.preferredReportName"); 3517 else if (Configuration.doAutoCreate()) 3518 this.preferredReportName = new StringType(); // bb 3519 return this.preferredReportName; 3520 } 3521 3522 public boolean hasPreferredReportNameElement() { 3523 return this.preferredReportName != null && !this.preferredReportName.isEmpty(); 3524 } 3525 3526 public boolean hasPreferredReportName() { 3527 return this.preferredReportName != null && !this.preferredReportName.isEmpty(); 3528 } 3529 3530 /** 3531 * @param value {@link #preferredReportName} (The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.). This is the underlying object with id, value and extensions. The accessor "getPreferredReportName" gives direct access to the value 3532 */ 3533 public ObservationDefinition setPreferredReportNameElement(StringType value) { 3534 this.preferredReportName = value; 3535 return this; 3536 } 3537 3538 /** 3539 * @return The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition. 3540 */ 3541 public String getPreferredReportName() { 3542 return this.preferredReportName == null ? null : this.preferredReportName.getValue(); 3543 } 3544 3545 /** 3546 * @param value The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition. 3547 */ 3548 public ObservationDefinition setPreferredReportName(String value) { 3549 if (Utilities.noString(value)) 3550 this.preferredReportName = null; 3551 else { 3552 if (this.preferredReportName == null) 3553 this.preferredReportName = new StringType(); 3554 this.preferredReportName.setValue(value); 3555 } 3556 return this; 3557 } 3558 3559 /** 3560 * @return {@link #permittedUnit} (Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.) 3561 */ 3562 public List<Coding> getPermittedUnit() { 3563 if (this.permittedUnit == null) 3564 this.permittedUnit = new ArrayList<Coding>(); 3565 return this.permittedUnit; 3566 } 3567 3568 /** 3569 * @return Returns a reference to <code>this</code> for easy method chaining 3570 */ 3571 public ObservationDefinition setPermittedUnit(List<Coding> thePermittedUnit) { 3572 this.permittedUnit = thePermittedUnit; 3573 return this; 3574 } 3575 3576 public boolean hasPermittedUnit() { 3577 if (this.permittedUnit == null) 3578 return false; 3579 for (Coding item : this.permittedUnit) 3580 if (!item.isEmpty()) 3581 return true; 3582 return false; 3583 } 3584 3585 public Coding addPermittedUnit() { //3 3586 Coding t = new Coding(); 3587 if (this.permittedUnit == null) 3588 this.permittedUnit = new ArrayList<Coding>(); 3589 this.permittedUnit.add(t); 3590 return t; 3591 } 3592 3593 public ObservationDefinition addPermittedUnit(Coding t) { //3 3594 if (t == null) 3595 return this; 3596 if (this.permittedUnit == null) 3597 this.permittedUnit = new ArrayList<Coding>(); 3598 this.permittedUnit.add(t); 3599 return this; 3600 } 3601 3602 /** 3603 * @return The first repetition of repeating field {@link #permittedUnit}, creating it if it does not already exist {3} 3604 */ 3605 public Coding getPermittedUnitFirstRep() { 3606 if (getPermittedUnit().isEmpty()) { 3607 addPermittedUnit(); 3608 } 3609 return getPermittedUnit().get(0); 3610 } 3611 3612 /** 3613 * @return {@link #qualifiedValue} (A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.) 3614 */ 3615 public List<ObservationDefinitionQualifiedValueComponent> getQualifiedValue() { 3616 if (this.qualifiedValue == null) 3617 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 3618 return this.qualifiedValue; 3619 } 3620 3621 /** 3622 * @return Returns a reference to <code>this</code> for easy method chaining 3623 */ 3624 public ObservationDefinition setQualifiedValue(List<ObservationDefinitionQualifiedValueComponent> theQualifiedValue) { 3625 this.qualifiedValue = theQualifiedValue; 3626 return this; 3627 } 3628 3629 public boolean hasQualifiedValue() { 3630 if (this.qualifiedValue == null) 3631 return false; 3632 for (ObservationDefinitionQualifiedValueComponent item : this.qualifiedValue) 3633 if (!item.isEmpty()) 3634 return true; 3635 return false; 3636 } 3637 3638 public ObservationDefinitionQualifiedValueComponent addQualifiedValue() { //3 3639 ObservationDefinitionQualifiedValueComponent t = new ObservationDefinitionQualifiedValueComponent(); 3640 if (this.qualifiedValue == null) 3641 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 3642 this.qualifiedValue.add(t); 3643 return t; 3644 } 3645 3646 public ObservationDefinition addQualifiedValue(ObservationDefinitionQualifiedValueComponent t) { //3 3647 if (t == null) 3648 return this; 3649 if (this.qualifiedValue == null) 3650 this.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 3651 this.qualifiedValue.add(t); 3652 return this; 3653 } 3654 3655 /** 3656 * @return The first repetition of repeating field {@link #qualifiedValue}, creating it if it does not already exist {3} 3657 */ 3658 public ObservationDefinitionQualifiedValueComponent getQualifiedValueFirstRep() { 3659 if (getQualifiedValue().isEmpty()) { 3660 addQualifiedValue(); 3661 } 3662 return getQualifiedValue().get(0); 3663 } 3664 3665 /** 3666 * @return {@link #hasMember} (This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.) 3667 */ 3668 public List<Reference> getHasMember() { 3669 if (this.hasMember == null) 3670 this.hasMember = new ArrayList<Reference>(); 3671 return this.hasMember; 3672 } 3673 3674 /** 3675 * @return Returns a reference to <code>this</code> for easy method chaining 3676 */ 3677 public ObservationDefinition setHasMember(List<Reference> theHasMember) { 3678 this.hasMember = theHasMember; 3679 return this; 3680 } 3681 3682 public boolean hasHasMember() { 3683 if (this.hasMember == null) 3684 return false; 3685 for (Reference item : this.hasMember) 3686 if (!item.isEmpty()) 3687 return true; 3688 return false; 3689 } 3690 3691 public Reference addHasMember() { //3 3692 Reference t = new Reference(); 3693 if (this.hasMember == null) 3694 this.hasMember = new ArrayList<Reference>(); 3695 this.hasMember.add(t); 3696 return t; 3697 } 3698 3699 public ObservationDefinition addHasMember(Reference t) { //3 3700 if (t == null) 3701 return this; 3702 if (this.hasMember == null) 3703 this.hasMember = new ArrayList<Reference>(); 3704 this.hasMember.add(t); 3705 return this; 3706 } 3707 3708 /** 3709 * @return The first repetition of repeating field {@link #hasMember}, creating it if it does not already exist {3} 3710 */ 3711 public Reference getHasMemberFirstRep() { 3712 if (getHasMember().isEmpty()) { 3713 addHasMember(); 3714 } 3715 return getHasMember().get(0); 3716 } 3717 3718 /** 3719 * @return {@link #component} (Some observations have multiple component observations, expressed as separate code value pairs.) 3720 */ 3721 public List<ObservationDefinitionComponentComponent> getComponent() { 3722 if (this.component == null) 3723 this.component = new ArrayList<ObservationDefinitionComponentComponent>(); 3724 return this.component; 3725 } 3726 3727 /** 3728 * @return Returns a reference to <code>this</code> for easy method chaining 3729 */ 3730 public ObservationDefinition setComponent(List<ObservationDefinitionComponentComponent> theComponent) { 3731 this.component = theComponent; 3732 return this; 3733 } 3734 3735 public boolean hasComponent() { 3736 if (this.component == null) 3737 return false; 3738 for (ObservationDefinitionComponentComponent item : this.component) 3739 if (!item.isEmpty()) 3740 return true; 3741 return false; 3742 } 3743 3744 public ObservationDefinitionComponentComponent addComponent() { //3 3745 ObservationDefinitionComponentComponent t = new ObservationDefinitionComponentComponent(); 3746 if (this.component == null) 3747 this.component = new ArrayList<ObservationDefinitionComponentComponent>(); 3748 this.component.add(t); 3749 return t; 3750 } 3751 3752 public ObservationDefinition addComponent(ObservationDefinitionComponentComponent t) { //3 3753 if (t == null) 3754 return this; 3755 if (this.component == null) 3756 this.component = new ArrayList<ObservationDefinitionComponentComponent>(); 3757 this.component.add(t); 3758 return this; 3759 } 3760 3761 /** 3762 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 3763 */ 3764 public ObservationDefinitionComponentComponent getComponentFirstRep() { 3765 if (getComponent().isEmpty()) { 3766 addComponent(); 3767 } 3768 return getComponent().get(0); 3769 } 3770 3771 protected void listChildren(List<Property> children) { 3772 super.listChildren(children); 3773 children.add(new Property("url", "uri", "An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.", 0, 1, url)); 3774 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, 1, identifier)); 3775 children.add(new Property("version", "string", "The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version)); 3776 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3777 children.add(new Property("name", "string", "A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3778 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the ObservationDefinition.", 0, 1, title)); 3779 children.add(new Property("status", "code", "The current state of the ObservationDefinition.", 0, 1, status)); 3780 children.add(new Property("experimental", "boolean", "A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3781 children.add(new Property("date", "dateTime", "The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.", 0, 1, date)); 3782 children.add(new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the ObservationDefinition. May also allow for contact.", 0, 1, publisher)); 3783 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3784 children.add(new Property("description", "markdown", "A free text natural language description of the ObservationDefinition from the consumer's perspective.", 0, 1, description)); 3785 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3786 children.add(new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the ObservationDefinition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3787 children.add(new Property("purpose", "markdown", "Explains why this ObservationDefinition is needed and why it has been designed as it has.", 0, 1, purpose)); 3788 children.add(new Property("copyright", "markdown", "Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.", 0, 1, copyright)); 3789 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3790 children.add(new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3791 children.add(new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 3792 children.add(new Property("effectivePeriod", "Period", "The period during which the ObservationDefinition content was or is planned to be effective.", 0, 1, effectivePeriod)); 3793 children.add(new Property("derivedFromCanonical", "canonical(ObservationDefinition)", "The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromCanonical)); 3794 children.add(new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri)); 3795 children.add(new Property("subject", "CodeableConcept", "A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, subject)); 3796 children.add(new Property("performerType", "CodeableConcept", "The type of individual/organization/device that is expected to act upon instances of this definition.", 0, 1, performerType)); 3797 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of observation.", 0, java.lang.Integer.MAX_VALUE, category)); 3798 children.add(new Property("code", "CodeableConcept", "Describes what will be observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 3799 children.add(new Property("permittedDataType", "code", "The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedDataType)); 3800 children.add(new Property("multipleResultsAllowed", "boolean", "Multiple results allowed for observations conforming to this ObservationDefinition.", 0, 1, multipleResultsAllowed)); 3801 children.add(new Property("bodySite", "CodeableConcept", "The site on the subject's body where the observation is to be made.", 0, 1, bodySite)); 3802 children.add(new Property("method", "CodeableConcept", "The method or technique used to perform the observation.", 0, 1, method)); 3803 children.add(new Property("specimen", "Reference(SpecimenDefinition)", "The kind of specimen that this type of observation is produced on.", 0, java.lang.Integer.MAX_VALUE, specimen)); 3804 children.add(new Property("device", "Reference(DeviceDefinition|Device)", "The measurement model of device or actual device used to produce observations of this type.", 0, java.lang.Integer.MAX_VALUE, device)); 3805 children.add(new Property("preferredReportName", "string", "The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.", 0, 1, preferredReportName)); 3806 children.add(new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit)); 3807 children.add(new Property("qualifiedValue", "", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue)); 3808 children.add(new Property("hasMember", "Reference(ObservationDefinition|Questionnaire)", "This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember)); 3809 children.add(new Property("component", "", "Some observations have multiple component observations, expressed as separate code value pairs.", 0, java.lang.Integer.MAX_VALUE, component)); 3810 } 3811 3812 @Override 3813 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3814 switch (_hash) { 3815 case 116079: /*url*/ return new Property("url", "uri", "An absolute URL that is used to identify this ObservationDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this ObservationDefinition is (or will be) published. The URL SHOULD include the major version of the ObservationDefinition. For more information see Technical and Business Versions.", 0, 1, url); 3816 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this ObservationDefinition. by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, 1, identifier); 3817 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the ObservationDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the ObservationDefinition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version); 3818 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3819 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3820 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3821 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3822 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the ObservationDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3823 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the ObservationDefinition.", 0, 1, title); 3824 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ObservationDefinition.", 0, 1, status); 3825 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A flag to indicate that this ObservationDefinition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3826 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the ObservationDefinition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ObservationDefinition changes.", 0, 1, date); 3827 case 1447404028: /*publisher*/ return new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the ObservationDefinition. May also allow for contact.", 0, 1, publisher); 3828 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3829 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the ObservationDefinition from the consumer's perspective.", 0, 1, description); 3830 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate ObservationDefinition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3831 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the ObservationDefinition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3832 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explains why this ObservationDefinition is needed and why it has been designed as it has.", 0, 1, purpose); 3833 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "Copyright statement relating to the ObservationDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the ObservationDefinition.", 0, 1, copyright); 3834 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3835 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 3836 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate); 3837 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the ObservationDefinition content was or is planned to be effective.", 0, 1, effectivePeriod); 3838 case -978133683: /*derivedFromCanonical*/ return new Property("derivedFromCanonical", "canonical(ObservationDefinition)", "The canonical URL pointing to another FHIR-defined ObservationDefinition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromCanonical); 3839 case -1076333435: /*derivedFromUri*/ return new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined observation definition, guideline or other definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri); 3840 case -1867885268: /*subject*/ return new Property("subject", "CodeableConcept", "A code that describes the intended kind of subject of Observation instances conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, subject); 3841 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "The type of individual/organization/device that is expected to act upon instances of this definition.", 0, 1, performerType); 3842 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of observation.", 0, java.lang.Integer.MAX_VALUE, category); 3843 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what will be observed. Sometimes this is called the observation \"name\".", 0, 1, code); 3844 case -99492804: /*permittedDataType*/ return new Property("permittedDataType", "code", "The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedDataType); 3845 case -2102414590: /*multipleResultsAllowed*/ return new Property("multipleResultsAllowed", "boolean", "Multiple results allowed for observations conforming to this ObservationDefinition.", 0, 1, multipleResultsAllowed); 3846 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "The site on the subject's body where the observation is to be made.", 0, 1, bodySite); 3847 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "The method or technique used to perform the observation.", 0, 1, method); 3848 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(SpecimenDefinition)", "The kind of specimen that this type of observation is produced on.", 0, java.lang.Integer.MAX_VALUE, specimen); 3849 case -1335157162: /*device*/ return new Property("device", "Reference(DeviceDefinition|Device)", "The measurement model of device or actual device used to produce observations of this type.", 0, java.lang.Integer.MAX_VALUE, device); 3850 case -1851030208: /*preferredReportName*/ return new Property("preferredReportName", "string", "The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.", 0, 1, preferredReportName); 3851 case 1073054652: /*permittedUnit*/ return new Property("permittedUnit", "Coding", "Units allowed for the valueQuantity element in the instance observations conforming to this ObservationDefinition.", 0, java.lang.Integer.MAX_VALUE, permittedUnit); 3852 case -558517707: /*qualifiedValue*/ return new Property("qualifiedValue", "", "A set of qualified values associated with a context and a set of conditions - provides a range for quantitative and ordinal observations and a collection of value sets for qualitative observations.", 0, java.lang.Integer.MAX_VALUE, qualifiedValue); 3853 case -458019372: /*hasMember*/ return new Property("hasMember", "Reference(ObservationDefinition|Questionnaire)", "This ObservationDefinition defines a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 0, java.lang.Integer.MAX_VALUE, hasMember); 3854 case -1399907075: /*component*/ return new Property("component", "", "Some observations have multiple component observations, expressed as separate code value pairs.", 0, java.lang.Integer.MAX_VALUE, component); 3855 default: return super.getNamedProperty(_hash, _name, _checkValid); 3856 } 3857 3858 } 3859 3860 @Override 3861 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3862 switch (hash) { 3863 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3864 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3865 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3866 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3867 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3868 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3869 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3870 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3871 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3872 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3873 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3874 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3875 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3876 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3877 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3878 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3879 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3880 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 3881 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 3882 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 3883 case -978133683: /*derivedFromCanonical*/ return this.derivedFromCanonical == null ? new Base[0] : this.derivedFromCanonical.toArray(new Base[this.derivedFromCanonical.size()]); // CanonicalType 3884 case -1076333435: /*derivedFromUri*/ return this.derivedFromUri == null ? new Base[0] : this.derivedFromUri.toArray(new Base[this.derivedFromUri.size()]); // UriType 3885 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // CodeableConcept 3886 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 3887 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3888 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3889 case -99492804: /*permittedDataType*/ return this.permittedDataType == null ? new Base[0] : this.permittedDataType.toArray(new Base[this.permittedDataType.size()]); // Enumeration<ObservationDataType> 3890 case -2102414590: /*multipleResultsAllowed*/ return this.multipleResultsAllowed == null ? new Base[0] : new Base[] {this.multipleResultsAllowed}; // BooleanType 3891 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 3892 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 3893 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 3894 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 3895 case -1851030208: /*preferredReportName*/ return this.preferredReportName == null ? new Base[0] : new Base[] {this.preferredReportName}; // StringType 3896 case 1073054652: /*permittedUnit*/ return this.permittedUnit == null ? new Base[0] : this.permittedUnit.toArray(new Base[this.permittedUnit.size()]); // Coding 3897 case -558517707: /*qualifiedValue*/ return this.qualifiedValue == null ? new Base[0] : this.qualifiedValue.toArray(new Base[this.qualifiedValue.size()]); // ObservationDefinitionQualifiedValueComponent 3898 case -458019372: /*hasMember*/ return this.hasMember == null ? new Base[0] : this.hasMember.toArray(new Base[this.hasMember.size()]); // Reference 3899 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ObservationDefinitionComponentComponent 3900 default: return super.getProperty(hash, name, checkValid); 3901 } 3902 3903 } 3904 3905 @Override 3906 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3907 switch (hash) { 3908 case 116079: // url 3909 this.url = TypeConvertor.castToUri(value); // UriType 3910 return value; 3911 case -1618432855: // identifier 3912 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3913 return value; 3914 case 351608024: // version 3915 this.version = TypeConvertor.castToString(value); // StringType 3916 return value; 3917 case 1508158071: // versionAlgorithm 3918 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3919 return value; 3920 case 3373707: // name 3921 this.name = TypeConvertor.castToString(value); // StringType 3922 return value; 3923 case 110371416: // title 3924 this.title = TypeConvertor.castToString(value); // StringType 3925 return value; 3926 case -892481550: // status 3927 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3928 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3929 return value; 3930 case -404562712: // experimental 3931 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3932 return value; 3933 case 3076014: // date 3934 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3935 return value; 3936 case 1447404028: // publisher 3937 this.publisher = TypeConvertor.castToString(value); // StringType 3938 return value; 3939 case 951526432: // contact 3940 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3941 return value; 3942 case -1724546052: // description 3943 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3944 return value; 3945 case -669707736: // useContext 3946 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3947 return value; 3948 case -507075711: // jurisdiction 3949 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3950 return value; 3951 case -220463842: // purpose 3952 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3953 return value; 3954 case 1522889671: // copyright 3955 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3956 return value; 3957 case 765157229: // copyrightLabel 3958 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3959 return value; 3960 case 223539345: // approvalDate 3961 this.approvalDate = TypeConvertor.castToDate(value); // DateType 3962 return value; 3963 case -1687512484: // lastReviewDate 3964 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 3965 return value; 3966 case -403934648: // effectivePeriod 3967 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 3968 return value; 3969 case -978133683: // derivedFromCanonical 3970 this.getDerivedFromCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 3971 return value; 3972 case -1076333435: // derivedFromUri 3973 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); // UriType 3974 return value; 3975 case -1867885268: // subject 3976 this.getSubject().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3977 return value; 3978 case -901444568: // performerType 3979 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3980 return value; 3981 case 50511102: // category 3982 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3983 return value; 3984 case 3059181: // code 3985 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3986 return value; 3987 case -99492804: // permittedDataType 3988 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3989 this.getPermittedDataType().add((Enumeration) value); // Enumeration<ObservationDataType> 3990 return value; 3991 case -2102414590: // multipleResultsAllowed 3992 this.multipleResultsAllowed = TypeConvertor.castToBoolean(value); // BooleanType 3993 return value; 3994 case 1702620169: // bodySite 3995 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3996 return value; 3997 case -1077554975: // method 3998 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3999 return value; 4000 case -2132868344: // specimen 4001 this.getSpecimen().add(TypeConvertor.castToReference(value)); // Reference 4002 return value; 4003 case -1335157162: // device 4004 this.getDevice().add(TypeConvertor.castToReference(value)); // Reference 4005 return value; 4006 case -1851030208: // preferredReportName 4007 this.preferredReportName = TypeConvertor.castToString(value); // StringType 4008 return value; 4009 case 1073054652: // permittedUnit 4010 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); // Coding 4011 return value; 4012 case -558517707: // qualifiedValue 4013 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); // ObservationDefinitionQualifiedValueComponent 4014 return value; 4015 case -458019372: // hasMember 4016 this.getHasMember().add(TypeConvertor.castToReference(value)); // Reference 4017 return value; 4018 case -1399907075: // component 4019 this.getComponent().add((ObservationDefinitionComponentComponent) value); // ObservationDefinitionComponentComponent 4020 return value; 4021 default: return super.setProperty(hash, name, value); 4022 } 4023 4024 } 4025 4026 @Override 4027 public Base setProperty(String name, Base value) throws FHIRException { 4028 if (name.equals("url")) { 4029 this.url = TypeConvertor.castToUri(value); // UriType 4030 } else if (name.equals("identifier")) { 4031 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 4032 } else if (name.equals("version")) { 4033 this.version = TypeConvertor.castToString(value); // StringType 4034 } else if (name.equals("versionAlgorithm[x]")) { 4035 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4036 } else if (name.equals("name")) { 4037 this.name = TypeConvertor.castToString(value); // StringType 4038 } else if (name.equals("title")) { 4039 this.title = TypeConvertor.castToString(value); // StringType 4040 } else if (name.equals("status")) { 4041 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4042 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4043 } else if (name.equals("experimental")) { 4044 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4045 } else if (name.equals("date")) { 4046 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4047 } else if (name.equals("publisher")) { 4048 this.publisher = TypeConvertor.castToString(value); // StringType 4049 } else if (name.equals("contact")) { 4050 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4051 } else if (name.equals("description")) { 4052 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4053 } else if (name.equals("useContext")) { 4054 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4055 } else if (name.equals("jurisdiction")) { 4056 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4057 } else if (name.equals("purpose")) { 4058 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4059 } else if (name.equals("copyright")) { 4060 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4061 } else if (name.equals("copyrightLabel")) { 4062 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4063 } else if (name.equals("approvalDate")) { 4064 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4065 } else if (name.equals("lastReviewDate")) { 4066 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4067 } else if (name.equals("effectivePeriod")) { 4068 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4069 } else if (name.equals("derivedFromCanonical")) { 4070 this.getDerivedFromCanonical().add(TypeConvertor.castToCanonical(value)); 4071 } else if (name.equals("derivedFromUri")) { 4072 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); 4073 } else if (name.equals("subject")) { 4074 this.getSubject().add(TypeConvertor.castToCodeableConcept(value)); 4075 } else if (name.equals("performerType")) { 4076 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4077 } else if (name.equals("category")) { 4078 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 4079 } else if (name.equals("code")) { 4080 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4081 } else if (name.equals("permittedDataType")) { 4082 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4083 this.getPermittedDataType().add((Enumeration) value); 4084 } else if (name.equals("multipleResultsAllowed")) { 4085 this.multipleResultsAllowed = TypeConvertor.castToBoolean(value); // BooleanType 4086 } else if (name.equals("bodySite")) { 4087 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4088 } else if (name.equals("method")) { 4089 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4090 } else if (name.equals("specimen")) { 4091 this.getSpecimen().add(TypeConvertor.castToReference(value)); 4092 } else if (name.equals("device")) { 4093 this.getDevice().add(TypeConvertor.castToReference(value)); 4094 } else if (name.equals("preferredReportName")) { 4095 this.preferredReportName = TypeConvertor.castToString(value); // StringType 4096 } else if (name.equals("permittedUnit")) { 4097 this.getPermittedUnit().add(TypeConvertor.castToCoding(value)); 4098 } else if (name.equals("qualifiedValue")) { 4099 this.getQualifiedValue().add((ObservationDefinitionQualifiedValueComponent) value); 4100 } else if (name.equals("hasMember")) { 4101 this.getHasMember().add(TypeConvertor.castToReference(value)); 4102 } else if (name.equals("component")) { 4103 this.getComponent().add((ObservationDefinitionComponentComponent) value); 4104 } else 4105 return super.setProperty(name, value); 4106 return value; 4107 } 4108 4109 @Override 4110 public void removeChild(String name, Base value) throws FHIRException { 4111 if (name.equals("url")) { 4112 this.url = null; 4113 } else if (name.equals("identifier")) { 4114 this.identifier = null; 4115 } else if (name.equals("version")) { 4116 this.version = null; 4117 } else if (name.equals("versionAlgorithm[x]")) { 4118 this.versionAlgorithm = null; 4119 } else if (name.equals("name")) { 4120 this.name = null; 4121 } else if (name.equals("title")) { 4122 this.title = null; 4123 } else if (name.equals("status")) { 4124 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4125 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4126 } else if (name.equals("experimental")) { 4127 this.experimental = null; 4128 } else if (name.equals("date")) { 4129 this.date = null; 4130 } else if (name.equals("publisher")) { 4131 this.publisher = null; 4132 } else if (name.equals("contact")) { 4133 this.getContact().remove(value); 4134 } else if (name.equals("description")) { 4135 this.description = null; 4136 } else if (name.equals("useContext")) { 4137 this.getUseContext().remove(value); 4138 } else if (name.equals("jurisdiction")) { 4139 this.getJurisdiction().remove(value); 4140 } else if (name.equals("purpose")) { 4141 this.purpose = null; 4142 } else if (name.equals("copyright")) { 4143 this.copyright = null; 4144 } else if (name.equals("copyrightLabel")) { 4145 this.copyrightLabel = null; 4146 } else if (name.equals("approvalDate")) { 4147 this.approvalDate = null; 4148 } else if (name.equals("lastReviewDate")) { 4149 this.lastReviewDate = null; 4150 } else if (name.equals("effectivePeriod")) { 4151 this.effectivePeriod = null; 4152 } else if (name.equals("derivedFromCanonical")) { 4153 this.getDerivedFromCanonical().remove(value); 4154 } else if (name.equals("derivedFromUri")) { 4155 this.getDerivedFromUri().remove(value); 4156 } else if (name.equals("subject")) { 4157 this.getSubject().remove(value); 4158 } else if (name.equals("performerType")) { 4159 this.performerType = null; 4160 } else if (name.equals("category")) { 4161 this.getCategory().remove(value); 4162 } else if (name.equals("code")) { 4163 this.code = null; 4164 } else if (name.equals("permittedDataType")) { 4165 value = new ObservationDataTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4166 this.getPermittedDataType().remove((Enumeration) value); 4167 } else if (name.equals("multipleResultsAllowed")) { 4168 this.multipleResultsAllowed = null; 4169 } else if (name.equals("bodySite")) { 4170 this.bodySite = null; 4171 } else if (name.equals("method")) { 4172 this.method = null; 4173 } else if (name.equals("specimen")) { 4174 this.getSpecimen().remove(value); 4175 } else if (name.equals("device")) { 4176 this.getDevice().remove(value); 4177 } else if (name.equals("preferredReportName")) { 4178 this.preferredReportName = null; 4179 } else if (name.equals("permittedUnit")) { 4180 this.getPermittedUnit().remove(value); 4181 } else if (name.equals("qualifiedValue")) { 4182 this.getQualifiedValue().remove((ObservationDefinitionQualifiedValueComponent) value); 4183 } else if (name.equals("hasMember")) { 4184 this.getHasMember().remove(value); 4185 } else if (name.equals("component")) { 4186 this.getComponent().remove((ObservationDefinitionComponentComponent) value); 4187 } else 4188 super.removeChild(name, value); 4189 4190 } 4191 4192 @Override 4193 public Base makeProperty(int hash, String name) throws FHIRException { 4194 switch (hash) { 4195 case 116079: return getUrlElement(); 4196 case -1618432855: return getIdentifier(); 4197 case 351608024: return getVersionElement(); 4198 case -115699031: return getVersionAlgorithm(); 4199 case 1508158071: return getVersionAlgorithm(); 4200 case 3373707: return getNameElement(); 4201 case 110371416: return getTitleElement(); 4202 case -892481550: return getStatusElement(); 4203 case -404562712: return getExperimentalElement(); 4204 case 3076014: return getDateElement(); 4205 case 1447404028: return getPublisherElement(); 4206 case 951526432: return addContact(); 4207 case -1724546052: return getDescriptionElement(); 4208 case -669707736: return addUseContext(); 4209 case -507075711: return addJurisdiction(); 4210 case -220463842: return getPurposeElement(); 4211 case 1522889671: return getCopyrightElement(); 4212 case 765157229: return getCopyrightLabelElement(); 4213 case 223539345: return getApprovalDateElement(); 4214 case -1687512484: return getLastReviewDateElement(); 4215 case -403934648: return getEffectivePeriod(); 4216 case -978133683: return addDerivedFromCanonicalElement(); 4217 case -1076333435: return addDerivedFromUriElement(); 4218 case -1867885268: return addSubject(); 4219 case -901444568: return getPerformerType(); 4220 case 50511102: return addCategory(); 4221 case 3059181: return getCode(); 4222 case -99492804: return addPermittedDataTypeElement(); 4223 case -2102414590: return getMultipleResultsAllowedElement(); 4224 case 1702620169: return getBodySite(); 4225 case -1077554975: return getMethod(); 4226 case -2132868344: return addSpecimen(); 4227 case -1335157162: return addDevice(); 4228 case -1851030208: return getPreferredReportNameElement(); 4229 case 1073054652: return addPermittedUnit(); 4230 case -558517707: return addQualifiedValue(); 4231 case -458019372: return addHasMember(); 4232 case -1399907075: return addComponent(); 4233 default: return super.makeProperty(hash, name); 4234 } 4235 4236 } 4237 4238 @Override 4239 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4240 switch (hash) { 4241 case 116079: /*url*/ return new String[] {"uri"}; 4242 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4243 case 351608024: /*version*/ return new String[] {"string"}; 4244 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 4245 case 3373707: /*name*/ return new String[] {"string"}; 4246 case 110371416: /*title*/ return new String[] {"string"}; 4247 case -892481550: /*status*/ return new String[] {"code"}; 4248 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4249 case 3076014: /*date*/ return new String[] {"dateTime"}; 4250 case 1447404028: /*publisher*/ return new String[] {"string"}; 4251 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4252 case -1724546052: /*description*/ return new String[] {"markdown"}; 4253 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4254 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4255 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4256 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4257 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 4258 case 223539345: /*approvalDate*/ return new String[] {"date"}; 4259 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 4260 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 4261 case -978133683: /*derivedFromCanonical*/ return new String[] {"canonical"}; 4262 case -1076333435: /*derivedFromUri*/ return new String[] {"uri"}; 4263 case -1867885268: /*subject*/ return new String[] {"CodeableConcept"}; 4264 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 4265 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4266 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 4267 case -99492804: /*permittedDataType*/ return new String[] {"code"}; 4268 case -2102414590: /*multipleResultsAllowed*/ return new String[] {"boolean"}; 4269 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 4270 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 4271 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 4272 case -1335157162: /*device*/ return new String[] {"Reference"}; 4273 case -1851030208: /*preferredReportName*/ return new String[] {"string"}; 4274 case 1073054652: /*permittedUnit*/ return new String[] {"Coding"}; 4275 case -558517707: /*qualifiedValue*/ return new String[] {}; 4276 case -458019372: /*hasMember*/ return new String[] {"Reference"}; 4277 case -1399907075: /*component*/ return new String[] {}; 4278 default: return super.getTypesForProperty(hash, name); 4279 } 4280 4281 } 4282 4283 @Override 4284 public Base addChild(String name) throws FHIRException { 4285 if (name.equals("url")) { 4286 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.url"); 4287 } 4288 else if (name.equals("identifier")) { 4289 this.identifier = new Identifier(); 4290 return this.identifier; 4291 } 4292 else if (name.equals("version")) { 4293 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.version"); 4294 } 4295 else if (name.equals("versionAlgorithmString")) { 4296 this.versionAlgorithm = new StringType(); 4297 return this.versionAlgorithm; 4298 } 4299 else if (name.equals("versionAlgorithmCoding")) { 4300 this.versionAlgorithm = new Coding(); 4301 return this.versionAlgorithm; 4302 } 4303 else if (name.equals("name")) { 4304 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.name"); 4305 } 4306 else if (name.equals("title")) { 4307 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.title"); 4308 } 4309 else if (name.equals("status")) { 4310 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.status"); 4311 } 4312 else if (name.equals("experimental")) { 4313 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.experimental"); 4314 } 4315 else if (name.equals("date")) { 4316 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.date"); 4317 } 4318 else if (name.equals("publisher")) { 4319 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.publisher"); 4320 } 4321 else if (name.equals("contact")) { 4322 return addContact(); 4323 } 4324 else if (name.equals("description")) { 4325 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.description"); 4326 } 4327 else if (name.equals("useContext")) { 4328 return addUseContext(); 4329 } 4330 else if (name.equals("jurisdiction")) { 4331 return addJurisdiction(); 4332 } 4333 else if (name.equals("purpose")) { 4334 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.purpose"); 4335 } 4336 else if (name.equals("copyright")) { 4337 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.copyright"); 4338 } 4339 else if (name.equals("copyrightLabel")) { 4340 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.copyrightLabel"); 4341 } 4342 else if (name.equals("approvalDate")) { 4343 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.approvalDate"); 4344 } 4345 else if (name.equals("lastReviewDate")) { 4346 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.lastReviewDate"); 4347 } 4348 else if (name.equals("effectivePeriod")) { 4349 this.effectivePeriod = new Period(); 4350 return this.effectivePeriod; 4351 } 4352 else if (name.equals("derivedFromCanonical")) { 4353 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.derivedFromCanonical"); 4354 } 4355 else if (name.equals("derivedFromUri")) { 4356 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.derivedFromUri"); 4357 } 4358 else if (name.equals("subject")) { 4359 return addSubject(); 4360 } 4361 else if (name.equals("performerType")) { 4362 this.performerType = new CodeableConcept(); 4363 return this.performerType; 4364 } 4365 else if (name.equals("category")) { 4366 return addCategory(); 4367 } 4368 else if (name.equals("code")) { 4369 this.code = new CodeableConcept(); 4370 return this.code; 4371 } 4372 else if (name.equals("permittedDataType")) { 4373 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.permittedDataType"); 4374 } 4375 else if (name.equals("multipleResultsAllowed")) { 4376 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.multipleResultsAllowed"); 4377 } 4378 else if (name.equals("bodySite")) { 4379 this.bodySite = new CodeableConcept(); 4380 return this.bodySite; 4381 } 4382 else if (name.equals("method")) { 4383 this.method = new CodeableConcept(); 4384 return this.method; 4385 } 4386 else if (name.equals("specimen")) { 4387 return addSpecimen(); 4388 } 4389 else if (name.equals("device")) { 4390 return addDevice(); 4391 } 4392 else if (name.equals("preferredReportName")) { 4393 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.preferredReportName"); 4394 } 4395 else if (name.equals("permittedUnit")) { 4396 return addPermittedUnit(); 4397 } 4398 else if (name.equals("qualifiedValue")) { 4399 return addQualifiedValue(); 4400 } 4401 else if (name.equals("hasMember")) { 4402 return addHasMember(); 4403 } 4404 else if (name.equals("component")) { 4405 return addComponent(); 4406 } 4407 else 4408 return super.addChild(name); 4409 } 4410 4411 public String fhirType() { 4412 return "ObservationDefinition"; 4413 4414 } 4415 4416 public ObservationDefinition copy() { 4417 ObservationDefinition dst = new ObservationDefinition(); 4418 copyValues(dst); 4419 return dst; 4420 } 4421 4422 public void copyValues(ObservationDefinition dst) { 4423 super.copyValues(dst); 4424 dst.url = url == null ? null : url.copy(); 4425 dst.identifier = identifier == null ? null : identifier.copy(); 4426 dst.version = version == null ? null : version.copy(); 4427 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4428 dst.name = name == null ? null : name.copy(); 4429 dst.title = title == null ? null : title.copy(); 4430 dst.status = status == null ? null : status.copy(); 4431 dst.experimental = experimental == null ? null : experimental.copy(); 4432 dst.date = date == null ? null : date.copy(); 4433 dst.publisher = publisher == null ? null : publisher.copy(); 4434 if (contact != null) { 4435 dst.contact = new ArrayList<ContactDetail>(); 4436 for (ContactDetail i : contact) 4437 dst.contact.add(i.copy()); 4438 }; 4439 dst.description = description == null ? null : description.copy(); 4440 if (useContext != null) { 4441 dst.useContext = new ArrayList<UsageContext>(); 4442 for (UsageContext i : useContext) 4443 dst.useContext.add(i.copy()); 4444 }; 4445 if (jurisdiction != null) { 4446 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4447 for (CodeableConcept i : jurisdiction) 4448 dst.jurisdiction.add(i.copy()); 4449 }; 4450 dst.purpose = purpose == null ? null : purpose.copy(); 4451 dst.copyright = copyright == null ? null : copyright.copy(); 4452 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4453 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4454 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4455 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4456 if (derivedFromCanonical != null) { 4457 dst.derivedFromCanonical = new ArrayList<CanonicalType>(); 4458 for (CanonicalType i : derivedFromCanonical) 4459 dst.derivedFromCanonical.add(i.copy()); 4460 }; 4461 if (derivedFromUri != null) { 4462 dst.derivedFromUri = new ArrayList<UriType>(); 4463 for (UriType i : derivedFromUri) 4464 dst.derivedFromUri.add(i.copy()); 4465 }; 4466 if (subject != null) { 4467 dst.subject = new ArrayList<CodeableConcept>(); 4468 for (CodeableConcept i : subject) 4469 dst.subject.add(i.copy()); 4470 }; 4471 dst.performerType = performerType == null ? null : performerType.copy(); 4472 if (category != null) { 4473 dst.category = new ArrayList<CodeableConcept>(); 4474 for (CodeableConcept i : category) 4475 dst.category.add(i.copy()); 4476 }; 4477 dst.code = code == null ? null : code.copy(); 4478 if (permittedDataType != null) { 4479 dst.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 4480 for (Enumeration<ObservationDataType> i : permittedDataType) 4481 dst.permittedDataType.add(i.copy()); 4482 }; 4483 dst.multipleResultsAllowed = multipleResultsAllowed == null ? null : multipleResultsAllowed.copy(); 4484 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4485 dst.method = method == null ? null : method.copy(); 4486 if (specimen != null) { 4487 dst.specimen = new ArrayList<Reference>(); 4488 for (Reference i : specimen) 4489 dst.specimen.add(i.copy()); 4490 }; 4491 if (device != null) { 4492 dst.device = new ArrayList<Reference>(); 4493 for (Reference i : device) 4494 dst.device.add(i.copy()); 4495 }; 4496 dst.preferredReportName = preferredReportName == null ? null : preferredReportName.copy(); 4497 if (permittedUnit != null) { 4498 dst.permittedUnit = new ArrayList<Coding>(); 4499 for (Coding i : permittedUnit) 4500 dst.permittedUnit.add(i.copy()); 4501 }; 4502 if (qualifiedValue != null) { 4503 dst.qualifiedValue = new ArrayList<ObservationDefinitionQualifiedValueComponent>(); 4504 for (ObservationDefinitionQualifiedValueComponent i : qualifiedValue) 4505 dst.qualifiedValue.add(i.copy()); 4506 }; 4507 if (hasMember != null) { 4508 dst.hasMember = new ArrayList<Reference>(); 4509 for (Reference i : hasMember) 4510 dst.hasMember.add(i.copy()); 4511 }; 4512 if (component != null) { 4513 dst.component = new ArrayList<ObservationDefinitionComponentComponent>(); 4514 for (ObservationDefinitionComponentComponent i : component) 4515 dst.component.add(i.copy()); 4516 }; 4517 } 4518 4519 protected ObservationDefinition typedCopy() { 4520 return copy(); 4521 } 4522 4523 @Override 4524 public boolean equalsDeep(Base other_) { 4525 if (!super.equalsDeep(other_)) 4526 return false; 4527 if (!(other_ instanceof ObservationDefinition)) 4528 return false; 4529 ObservationDefinition o = (ObservationDefinition) other_; 4530 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4531 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4532 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 4533 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 4534 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 4535 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 4536 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 4537 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(derivedFromCanonical, o.derivedFromCanonical, true) 4538 && compareDeep(derivedFromUri, o.derivedFromUri, true) && compareDeep(subject, o.subject, true) 4539 && compareDeep(performerType, o.performerType, true) && compareDeep(category, o.category, true) 4540 && compareDeep(code, o.code, true) && compareDeep(permittedDataType, o.permittedDataType, true) 4541 && compareDeep(multipleResultsAllowed, o.multipleResultsAllowed, true) && compareDeep(bodySite, o.bodySite, true) 4542 && compareDeep(method, o.method, true) && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 4543 && compareDeep(preferredReportName, o.preferredReportName, true) && compareDeep(permittedUnit, o.permittedUnit, true) 4544 && compareDeep(qualifiedValue, o.qualifiedValue, true) && compareDeep(hasMember, o.hasMember, true) 4545 && compareDeep(component, o.component, true); 4546 } 4547 4548 @Override 4549 public boolean equalsShallow(Base other_) { 4550 if (!super.equalsShallow(other_)) 4551 return false; 4552 if (!(other_ instanceof ObservationDefinition)) 4553 return false; 4554 ObservationDefinition o = (ObservationDefinition) other_; 4555 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4556 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 4557 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 4558 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 4559 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 4560 && compareValues(derivedFromCanonical, o.derivedFromCanonical, true) && compareValues(derivedFromUri, o.derivedFromUri, true) 4561 && compareValues(permittedDataType, o.permittedDataType, true) && compareValues(multipleResultsAllowed, o.multipleResultsAllowed, true) 4562 && compareValues(preferredReportName, o.preferredReportName, true); 4563 } 4564 4565 public boolean isEmpty() { 4566 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4567 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 4568 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, approvalDate 4569 , lastReviewDate, effectivePeriod, derivedFromCanonical, derivedFromUri, subject, performerType 4570 , category, code, permittedDataType, multipleResultsAllowed, bodySite, method, specimen 4571 , device, preferredReportName, permittedUnit, qualifiedValue, hasMember, component 4572 ); 4573 } 4574 4575 @Override 4576 public ResourceType getResourceType() { 4577 return ResourceType.ObservationDefinition; 4578 } 4579 4580 /** 4581 * Search parameter: <b>identifier</b> 4582 * <p> 4583 * Description: <b>Multiple Resources: 4584 4585* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4586* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4587* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4588* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4589* [Citation](citation.html): External identifier for the citation 4590* [CodeSystem](codesystem.html): External identifier for the code system 4591* [ConceptMap](conceptmap.html): External identifier for the concept map 4592* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4593* [EventDefinition](eventdefinition.html): External identifier for the event definition 4594* [Evidence](evidence.html): External identifier for the evidence 4595* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4596* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4597* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4598* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4599* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4600* [Library](library.html): External identifier for the library 4601* [Measure](measure.html): External identifier for the measure 4602* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4603* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4604* [NamingSystem](namingsystem.html): External identifier for the naming system 4605* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4606* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4607* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4608* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4609* [Requirements](requirements.html): External identifier for the requirements 4610* [SearchParameter](searchparameter.html): External identifier for the search parameter 4611* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4612* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4613* [StructureMap](structuremap.html): External identifier for the structure map 4614* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4615* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4616* [TestPlan](testplan.html): An identifier for the test plan 4617* [TestScript](testscript.html): External identifier for the test script 4618* [ValueSet](valueset.html): External identifier for the value set 4619</b><br> 4620 * Type: <b>token</b><br> 4621 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4622 * </p> 4623 */ 4624 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4625 public static final String SP_IDENTIFIER = "identifier"; 4626 /** 4627 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4628 * <p> 4629 * Description: <b>Multiple Resources: 4630 4631* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4632* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4633* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4634* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4635* [Citation](citation.html): External identifier for the citation 4636* [CodeSystem](codesystem.html): External identifier for the code system 4637* [ConceptMap](conceptmap.html): External identifier for the concept map 4638* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4639* [EventDefinition](eventdefinition.html): External identifier for the event definition 4640* [Evidence](evidence.html): External identifier for the evidence 4641* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4642* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4643* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4644* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4645* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4646* [Library](library.html): External identifier for the library 4647* [Measure](measure.html): External identifier for the measure 4648* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4649* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4650* [NamingSystem](namingsystem.html): External identifier for the naming system 4651* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4652* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4653* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4654* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4655* [Requirements](requirements.html): External identifier for the requirements 4656* [SearchParameter](searchparameter.html): External identifier for the search parameter 4657* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4658* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4659* [StructureMap](structuremap.html): External identifier for the structure map 4660* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4661* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4662* [TestPlan](testplan.html): An identifier for the test plan 4663* [TestScript](testscript.html): External identifier for the test script 4664* [ValueSet](valueset.html): External identifier for the value set 4665</b><br> 4666 * Type: <b>token</b><br> 4667 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4668 * </p> 4669 */ 4670 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4671 4672 /** 4673 * Search parameter: <b>status</b> 4674 * <p> 4675 * Description: <b>Multiple Resources: 4676 4677* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4678* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4679* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4680* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4681* [Citation](citation.html): The current status of the citation 4682* [CodeSystem](codesystem.html): The current status of the code system 4683* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4684* [ConceptMap](conceptmap.html): The current status of the concept map 4685* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4686* [EventDefinition](eventdefinition.html): The current status of the event definition 4687* [Evidence](evidence.html): The current status of the evidence 4688* [EvidenceReport](evidencereport.html): The current status of the evidence report 4689* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4690* [ExampleScenario](examplescenario.html): The current status of the example scenario 4691* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4692* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4693* [Library](library.html): The current status of the library 4694* [Measure](measure.html): The current status of the measure 4695* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4696* [MessageDefinition](messagedefinition.html): The current status of the message definition 4697* [NamingSystem](namingsystem.html): The current status of the naming system 4698* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4699* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4700* [PlanDefinition](plandefinition.html): The current status of the plan definition 4701* [Questionnaire](questionnaire.html): The current status of the questionnaire 4702* [Requirements](requirements.html): The current status of the requirements 4703* [SearchParameter](searchparameter.html): The current status of the search parameter 4704* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4705* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4706* [StructureMap](structuremap.html): The current status of the structure map 4707* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4708* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4709* [TestPlan](testplan.html): The current status of the test plan 4710* [TestScript](testscript.html): The current status of the test script 4711* [ValueSet](valueset.html): The current status of the value set 4712</b><br> 4713 * Type: <b>token</b><br> 4714 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4715 * </p> 4716 */ 4717 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4718 public static final String SP_STATUS = "status"; 4719 /** 4720 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4721 * <p> 4722 * Description: <b>Multiple Resources: 4723 4724* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4725* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4726* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4727* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4728* [Citation](citation.html): The current status of the citation 4729* [CodeSystem](codesystem.html): The current status of the code system 4730* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4731* [ConceptMap](conceptmap.html): The current status of the concept map 4732* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4733* [EventDefinition](eventdefinition.html): The current status of the event definition 4734* [Evidence](evidence.html): The current status of the evidence 4735* [EvidenceReport](evidencereport.html): The current status of the evidence report 4736* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4737* [ExampleScenario](examplescenario.html): The current status of the example scenario 4738* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4739* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4740* [Library](library.html): The current status of the library 4741* [Measure](measure.html): The current status of the measure 4742* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4743* [MessageDefinition](messagedefinition.html): The current status of the message definition 4744* [NamingSystem](namingsystem.html): The current status of the naming system 4745* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4746* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4747* [PlanDefinition](plandefinition.html): The current status of the plan definition 4748* [Questionnaire](questionnaire.html): The current status of the questionnaire 4749* [Requirements](requirements.html): The current status of the requirements 4750* [SearchParameter](searchparameter.html): The current status of the search parameter 4751* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4752* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4753* [StructureMap](structuremap.html): The current status of the structure map 4754* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4755* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4756* [TestPlan](testplan.html): The current status of the test plan 4757* [TestScript](testscript.html): The current status of the test script 4758* [ValueSet](valueset.html): The current status of the value set 4759</b><br> 4760 * Type: <b>token</b><br> 4761 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4762 * </p> 4763 */ 4764 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4765 4766 /** 4767 * Search parameter: <b>title</b> 4768 * <p> 4769 * Description: <b>Multiple Resources: 4770 4771* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4772* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4773* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4774* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4775* [Citation](citation.html): The human-friendly name of the citation 4776* [CodeSystem](codesystem.html): The human-friendly name of the code system 4777* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4778* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4779* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4780* [Evidence](evidence.html): The human-friendly name of the evidence 4781* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4782* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4783* [Library](library.html): The human-friendly name of the library 4784* [Measure](measure.html): The human-friendly name of the measure 4785* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4786* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4787* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4788* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4789* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4790* [Requirements](requirements.html): The human-friendly name of the requirements 4791* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4792* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4793* [StructureMap](structuremap.html): The human-friendly name of the structure map 4794* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4795* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4796* [TestScript](testscript.html): The human-friendly name of the test script 4797* [ValueSet](valueset.html): The human-friendly name of the value set 4798</b><br> 4799 * Type: <b>string</b><br> 4800 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4801 * </p> 4802 */ 4803 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4804 public static final String SP_TITLE = "title"; 4805 /** 4806 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4807 * <p> 4808 * Description: <b>Multiple Resources: 4809 4810* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4811* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4812* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4813* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4814* [Citation](citation.html): The human-friendly name of the citation 4815* [CodeSystem](codesystem.html): The human-friendly name of the code system 4816* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4817* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4818* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4819* [Evidence](evidence.html): The human-friendly name of the evidence 4820* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4821* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4822* [Library](library.html): The human-friendly name of the library 4823* [Measure](measure.html): The human-friendly name of the measure 4824* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4825* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4826* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4827* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4828* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4829* [Requirements](requirements.html): The human-friendly name of the requirements 4830* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4831* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4832* [StructureMap](structuremap.html): The human-friendly name of the structure map 4833* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4834* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4835* [TestScript](testscript.html): The human-friendly name of the test script 4836* [ValueSet](valueset.html): The human-friendly name of the value set 4837</b><br> 4838 * Type: <b>string</b><br> 4839 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4840 * </p> 4841 */ 4842 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4843 4844 /** 4845 * Search parameter: <b>url</b> 4846 * <p> 4847 * Description: <b>Multiple Resources: 4848 4849* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4850* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4851* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4852* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4853* [Citation](citation.html): The uri that identifies the citation 4854* [CodeSystem](codesystem.html): The uri that identifies the code system 4855* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4856* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4857* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4858* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4859* [Evidence](evidence.html): The uri that identifies the evidence 4860* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4861* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4862* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4863* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4864* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4865* [Library](library.html): The uri that identifies the library 4866* [Measure](measure.html): The uri that identifies the measure 4867* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4868* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4869* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4870* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4871* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4872* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4873* [Requirements](requirements.html): The uri that identifies the requirements 4874* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4875* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4876* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4877* [StructureMap](structuremap.html): The uri that identifies the structure map 4878* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4879* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4880* [TestPlan](testplan.html): The uri that identifies the test plan 4881* [TestScript](testscript.html): The uri that identifies the test script 4882* [ValueSet](valueset.html): The uri that identifies the value set 4883</b><br> 4884 * Type: <b>uri</b><br> 4885 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4886 * </p> 4887 */ 4888 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4889 public static final String SP_URL = "url"; 4890 /** 4891 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4892 * <p> 4893 * Description: <b>Multiple Resources: 4894 4895* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4896* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4897* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4898* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4899* [Citation](citation.html): The uri that identifies the citation 4900* [CodeSystem](codesystem.html): The uri that identifies the code system 4901* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4902* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4903* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4904* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4905* [Evidence](evidence.html): The uri that identifies the evidence 4906* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4907* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4908* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4909* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4910* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4911* [Library](library.html): The uri that identifies the library 4912* [Measure](measure.html): The uri that identifies the measure 4913* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4914* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4915* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4916* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4917* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4918* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4919* [Requirements](requirements.html): The uri that identifies the requirements 4920* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4921* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4922* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4923* [StructureMap](structuremap.html): The uri that identifies the structure map 4924* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4925* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4926* [TestPlan](testplan.html): The uri that identifies the test plan 4927* [TestScript](testscript.html): The uri that identifies the test script 4928* [ValueSet](valueset.html): The uri that identifies the value set 4929</b><br> 4930 * Type: <b>uri</b><br> 4931 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4932 * </p> 4933 */ 4934 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4935 4936 /** 4937 * Search parameter: <b>category</b> 4938 * <p> 4939 * Description: <b>Category (class) of observation</b><br> 4940 * Type: <b>token</b><br> 4941 * Path: <b>ObservationDefinition.category</b><br> 4942 * </p> 4943 */ 4944 @SearchParamDefinition(name="category", path="ObservationDefinition.category", description="Category (class) of observation", type="token" ) 4945 public static final String SP_CATEGORY = "category"; 4946 /** 4947 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4948 * <p> 4949 * Description: <b>Category (class) of observation</b><br> 4950 * Type: <b>token</b><br> 4951 * Path: <b>ObservationDefinition.category</b><br> 4952 * </p> 4953 */ 4954 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4955 4956 /** 4957 * Search parameter: <b>code</b> 4958 * <p> 4959 * Description: <b>Observation code</b><br> 4960 * Type: <b>token</b><br> 4961 * Path: <b>ObservationDefinition.code</b><br> 4962 * </p> 4963 */ 4964 @SearchParamDefinition(name="code", path="ObservationDefinition.code", description="Observation code", type="token" ) 4965 public static final String SP_CODE = "code"; 4966 /** 4967 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4968 * <p> 4969 * Description: <b>Observation code</b><br> 4970 * Type: <b>token</b><br> 4971 * Path: <b>ObservationDefinition.code</b><br> 4972 * </p> 4973 */ 4974 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4975 4976 /** 4977 * Search parameter: <b>experimental</b> 4978 * <p> 4979 * Description: <b>Not for genuine usage (true)</b><br> 4980 * Type: <b>token</b><br> 4981 * Path: <b>ObservationDefinition.experimental</b><br> 4982 * </p> 4983 */ 4984 @SearchParamDefinition(name="experimental", path="ObservationDefinition.experimental", description="Not for genuine usage (true)", type="token" ) 4985 public static final String SP_EXPERIMENTAL = "experimental"; 4986 /** 4987 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 4988 * <p> 4989 * Description: <b>Not for genuine usage (true)</b><br> 4990 * Type: <b>token</b><br> 4991 * Path: <b>ObservationDefinition.experimental</b><br> 4992 * </p> 4993 */ 4994 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 4995 4996 /** 4997 * Search parameter: <b>method</b> 4998 * <p> 4999 * Description: <b>Method of observation</b><br> 5000 * Type: <b>token</b><br> 5001 * Path: <b>ObservationDefinition.method</b><br> 5002 * </p> 5003 */ 5004 @SearchParamDefinition(name="method", path="ObservationDefinition.method", description="Method of observation", type="token" ) 5005 public static final String SP_METHOD = "method"; 5006 /** 5007 * <b>Fluent Client</b> search parameter constant for <b>method</b> 5008 * <p> 5009 * Description: <b>Method of observation</b><br> 5010 * Type: <b>token</b><br> 5011 * Path: <b>ObservationDefinition.method</b><br> 5012 * </p> 5013 */ 5014 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_METHOD); 5015 5016 5017} 5018