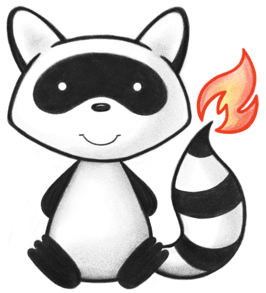
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 052 */ 053@ResourceDef(name="OperationDefinition", profile="http://hl7.org/fhir/StructureDefinition/OperationDefinition") 054public class OperationDefinition extends CanonicalResource { 055 056 public enum OperationKind { 057 /** 058 * This operation is invoked as an operation. 059 */ 060 OPERATION, 061 /** 062 * This operation is a named query, invoked using the search mechanism. 063 */ 064 QUERY, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static OperationKind fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("operation".equals(codeString)) 073 return OPERATION; 074 if ("query".equals(codeString)) 075 return QUERY; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown OperationKind code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case OPERATION: return "operation"; 084 case QUERY: return "query"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case OPERATION: return "http://hl7.org/fhir/operation-kind"; 092 case QUERY: return "http://hl7.org/fhir/operation-kind"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case OPERATION: return "This operation is invoked as an operation."; 100 case QUERY: return "This operation is a named query, invoked using the search mechanism."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case OPERATION: return "Operation"; 108 case QUERY: return "Query"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class OperationKindEnumFactory implements EnumFactory<OperationKind> { 116 public OperationKind fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("operation".equals(codeString)) 121 return OperationKind.OPERATION; 122 if ("query".equals(codeString)) 123 return OperationKind.QUERY; 124 throw new IllegalArgumentException("Unknown OperationKind code '"+codeString+"'"); 125 } 126 public Enumeration<OperationKind> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<OperationKind>(this, OperationKind.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<OperationKind>(this, OperationKind.NULL, code); 134 if ("operation".equals(codeString)) 135 return new Enumeration<OperationKind>(this, OperationKind.OPERATION, code); 136 if ("query".equals(codeString)) 137 return new Enumeration<OperationKind>(this, OperationKind.QUERY, code); 138 throw new FHIRException("Unknown OperationKind code '"+codeString+"'"); 139 } 140 public String toCode(OperationKind code) { 141 if (code == OperationKind.NULL) 142 return null; 143 if (code == OperationKind.OPERATION) 144 return "operation"; 145 if (code == OperationKind.QUERY) 146 return "query"; 147 return "?"; 148 } 149 public String toSystem(OperationKind code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum OperationParameterScope { 155 /** 156 * This is a parameter that can be used at the instance level. 157 */ 158 INSTANCE, 159 /** 160 * This is a parameter that can be used at the type level. 161 */ 162 TYPE, 163 /** 164 * This is a parameter that can be used at the system level. 165 */ 166 SYSTEM, 167 /** 168 * added to help the parsers with the generic types 169 */ 170 NULL; 171 public static OperationParameterScope fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("instance".equals(codeString)) 175 return INSTANCE; 176 if ("type".equals(codeString)) 177 return TYPE; 178 if ("system".equals(codeString)) 179 return SYSTEM; 180 if (Configuration.isAcceptInvalidEnums()) 181 return null; 182 else 183 throw new FHIRException("Unknown OperationParameterScope code '"+codeString+"'"); 184 } 185 public String toCode() { 186 switch (this) { 187 case INSTANCE: return "instance"; 188 case TYPE: return "type"; 189 case SYSTEM: return "system"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case INSTANCE: return "http://hl7.org/fhir/operation-parameter-scope"; 197 case TYPE: return "http://hl7.org/fhir/operation-parameter-scope"; 198 case SYSTEM: return "http://hl7.org/fhir/operation-parameter-scope"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getDefinition() { 204 switch (this) { 205 case INSTANCE: return "This is a parameter that can be used at the instance level."; 206 case TYPE: return "This is a parameter that can be used at the type level."; 207 case SYSTEM: return "This is a parameter that can be used at the system level."; 208 case NULL: return null; 209 default: return "?"; 210 } 211 } 212 public String getDisplay() { 213 switch (this) { 214 case INSTANCE: return "Instance"; 215 case TYPE: return "Type"; 216 case SYSTEM: return "System"; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 } 222 223 public static class OperationParameterScopeEnumFactory implements EnumFactory<OperationParameterScope> { 224 public OperationParameterScope fromCode(String codeString) throws IllegalArgumentException { 225 if (codeString == null || "".equals(codeString)) 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("instance".equals(codeString)) 229 return OperationParameterScope.INSTANCE; 230 if ("type".equals(codeString)) 231 return OperationParameterScope.TYPE; 232 if ("system".equals(codeString)) 233 return OperationParameterScope.SYSTEM; 234 throw new IllegalArgumentException("Unknown OperationParameterScope code '"+codeString+"'"); 235 } 236 public Enumeration<OperationParameterScope> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<OperationParameterScope>(this, OperationParameterScope.NULL, code); 241 String codeString = ((PrimitiveType) code).asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<OperationParameterScope>(this, OperationParameterScope.NULL, code); 244 if ("instance".equals(codeString)) 245 return new Enumeration<OperationParameterScope>(this, OperationParameterScope.INSTANCE, code); 246 if ("type".equals(codeString)) 247 return new Enumeration<OperationParameterScope>(this, OperationParameterScope.TYPE, code); 248 if ("system".equals(codeString)) 249 return new Enumeration<OperationParameterScope>(this, OperationParameterScope.SYSTEM, code); 250 throw new FHIRException("Unknown OperationParameterScope code '"+codeString+"'"); 251 } 252 public String toCode(OperationParameterScope code) { 253 if (code == OperationParameterScope.NULL) 254 return null; 255 if (code == OperationParameterScope.INSTANCE) 256 return "instance"; 257 if (code == OperationParameterScope.TYPE) 258 return "type"; 259 if (code == OperationParameterScope.SYSTEM) 260 return "system"; 261 return "?"; 262 } 263 public String toSystem(OperationParameterScope code) { 264 return code.getSystem(); 265 } 266 } 267 268 @Block() 269 public static class OperationDefinitionParameterComponent extends BackboneElement implements IBaseBackboneElement { 270 /** 271 * The name of used to identify the parameter. 272 */ 273 @Child(name = "name", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 274 @Description(shortDefinition="Name in Parameters.parameter.name or in URL", formalDefinition="The name of used to identify the parameter." ) 275 protected CodeType name; 276 277 /** 278 * Whether this is an input or an output parameter. 279 */ 280 @Child(name = "use", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 281 @Description(shortDefinition="in | out", formalDefinition="Whether this is an input or an output parameter." ) 282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-parameter-use") 283 protected Enumeration<OperationParameterUse> use; 284 285 /** 286 * If present, indicates that the parameter applies when the operation is being invoked at the specified level. 287 */ 288 @Child(name = "scope", type = {CodeType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 289 @Description(shortDefinition="instance | type | system", formalDefinition="If present, indicates that the parameter applies when the operation is being invoked at the specified level." ) 290 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-parameter-scope") 291 protected List<Enumeration<OperationParameterScope>> scope; 292 293 /** 294 * The minimum number of times this parameter SHALL appear in the request or response. 295 */ 296 @Child(name = "min", type = {IntegerType.class}, order=4, min=1, max=1, modifier=false, summary=false) 297 @Description(shortDefinition="Minimum Cardinality", formalDefinition="The minimum number of times this parameter SHALL appear in the request or response." ) 298 protected IntegerType min; 299 300 /** 301 * The maximum number of times this element is permitted to appear in the request or response. 302 */ 303 @Child(name = "max", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=false) 304 @Description(shortDefinition="Maximum Cardinality (a number or *)", formalDefinition="The maximum number of times this element is permitted to appear in the request or response." ) 305 protected StringType max; 306 307 /** 308 * Describes the meaning or use of this parameter. 309 */ 310 @Child(name = "documentation", type = {MarkdownType.class}, order=6, min=0, max=1, modifier=false, summary=false) 311 @Description(shortDefinition="Description of meaning/use", formalDefinition="Describes the meaning or use of this parameter." ) 312 protected MarkdownType documentation; 313 314 /** 315 * The type for this parameter. 316 */ 317 @Child(name = "type", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 318 @Description(shortDefinition="What type this parameter has", formalDefinition="The type for this parameter." ) 319 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 320 protected Enumeration<FHIRTypes> type; 321 322 /** 323 * Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter. 324 */ 325 @Child(name = "allowedType", type = {CodeType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 326 @Description(shortDefinition="Allowed sub-type this parameter can have (if type is abstract)", formalDefinition="Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter." ) 327 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 328 protected List<Enumeration<FHIRTypes>> allowedType; 329 330 /** 331 * Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide. 332 */ 333 @Child(name = "targetProfile", type = {CanonicalType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 334 @Description(shortDefinition="If type is Reference | canonical, allowed targets. If type is 'Resource', then this constrains the allowed resource types", formalDefinition="Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide." ) 335 protected List<CanonicalType> targetProfile; 336 337 /** 338 * How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string. 339 */ 340 @Child(name = "searchType", type = {CodeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 341 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri | special", formalDefinition="How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string." ) 342 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 343 protected Enumeration<SearchParamType> searchType; 344 345 /** 346 * Binds to a value set if this parameter is coded (code, Coding, CodeableConcept). 347 */ 348 @Child(name = "binding", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 349 @Description(shortDefinition="ValueSet details if this is coded", formalDefinition="Binds to a value set if this parameter is coded (code, Coding, CodeableConcept)." ) 350 protected OperationDefinitionParameterBindingComponent binding; 351 352 /** 353 * Identifies other resource parameters within the operation invocation that are expected to resolve to this resource. 354 */ 355 @Child(name = "referencedFrom", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 356 @Description(shortDefinition="References to this parameter", formalDefinition="Identifies other resource parameters within the operation invocation that are expected to resolve to this resource." ) 357 protected List<OperationDefinitionParameterReferencedFromComponent> referencedFrom; 358 359 /** 360 * The parts of a nested Parameter. 361 */ 362 @Child(name = "part", type = {OperationDefinitionParameterComponent.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 363 @Description(shortDefinition="Parts of a nested Parameter", formalDefinition="The parts of a nested Parameter." ) 364 protected List<OperationDefinitionParameterComponent> part; 365 366 private static final long serialVersionUID = -395202201L; 367 368 /** 369 * Constructor 370 */ 371 public OperationDefinitionParameterComponent() { 372 super(); 373 } 374 375 /** 376 * Constructor 377 */ 378 public OperationDefinitionParameterComponent(String name, OperationParameterUse use, int min, String max) { 379 super(); 380 this.setName(name); 381 this.setUse(use); 382 this.setMin(min); 383 this.setMax(max); 384 } 385 386 /** 387 * @return {@link #name} (The name of used to identify the parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 388 */ 389 public CodeType getNameElement() { 390 if (this.name == null) 391 if (Configuration.errorOnAutoCreate()) 392 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.name"); 393 else if (Configuration.doAutoCreate()) 394 this.name = new CodeType(); // bb 395 return this.name; 396 } 397 398 public boolean hasNameElement() { 399 return this.name != null && !this.name.isEmpty(); 400 } 401 402 public boolean hasName() { 403 return this.name != null && !this.name.isEmpty(); 404 } 405 406 /** 407 * @param value {@link #name} (The name of used to identify the parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 408 */ 409 public OperationDefinitionParameterComponent setNameElement(CodeType value) { 410 this.name = value; 411 return this; 412 } 413 414 /** 415 * @return The name of used to identify the parameter. 416 */ 417 public String getName() { 418 return this.name == null ? null : this.name.getValue(); 419 } 420 421 /** 422 * @param value The name of used to identify the parameter. 423 */ 424 public OperationDefinitionParameterComponent setName(String value) { 425 if (this.name == null) 426 this.name = new CodeType(); 427 this.name.setValue(value); 428 return this; 429 } 430 431 /** 432 * @return {@link #use} (Whether this is an input or an output parameter.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 433 */ 434 public Enumeration<OperationParameterUse> getUseElement() { 435 if (this.use == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.use"); 438 else if (Configuration.doAutoCreate()) 439 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); // bb 440 return this.use; 441 } 442 443 public boolean hasUseElement() { 444 return this.use != null && !this.use.isEmpty(); 445 } 446 447 public boolean hasUse() { 448 return this.use != null && !this.use.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #use} (Whether this is an input or an output parameter.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 453 */ 454 public OperationDefinitionParameterComponent setUseElement(Enumeration<OperationParameterUse> value) { 455 this.use = value; 456 return this; 457 } 458 459 /** 460 * @return Whether this is an input or an output parameter. 461 */ 462 public OperationParameterUse getUse() { 463 return this.use == null ? null : this.use.getValue(); 464 } 465 466 /** 467 * @param value Whether this is an input or an output parameter. 468 */ 469 public OperationDefinitionParameterComponent setUse(OperationParameterUse value) { 470 if (this.use == null) 471 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); 472 this.use.setValue(value); 473 return this; 474 } 475 476 /** 477 * @return {@link #scope} (If present, indicates that the parameter applies when the operation is being invoked at the specified level.) 478 */ 479 public List<Enumeration<OperationParameterScope>> getScope() { 480 if (this.scope == null) 481 this.scope = new ArrayList<Enumeration<OperationParameterScope>>(); 482 return this.scope; 483 } 484 485 /** 486 * @return Returns a reference to <code>this</code> for easy method chaining 487 */ 488 public OperationDefinitionParameterComponent setScope(List<Enumeration<OperationParameterScope>> theScope) { 489 this.scope = theScope; 490 return this; 491 } 492 493 public boolean hasScope() { 494 if (this.scope == null) 495 return false; 496 for (Enumeration<OperationParameterScope> item : this.scope) 497 if (!item.isEmpty()) 498 return true; 499 return false; 500 } 501 502 /** 503 * @return {@link #scope} (If present, indicates that the parameter applies when the operation is being invoked at the specified level.) 504 */ 505 public Enumeration<OperationParameterScope> addScopeElement() {//2 506 Enumeration<OperationParameterScope> t = new Enumeration<OperationParameterScope>(new OperationParameterScopeEnumFactory()); 507 if (this.scope == null) 508 this.scope = new ArrayList<Enumeration<OperationParameterScope>>(); 509 this.scope.add(t); 510 return t; 511 } 512 513 /** 514 * @param value {@link #scope} (If present, indicates that the parameter applies when the operation is being invoked at the specified level.) 515 */ 516 public OperationDefinitionParameterComponent addScope(OperationParameterScope value) { //1 517 Enumeration<OperationParameterScope> t = new Enumeration<OperationParameterScope>(new OperationParameterScopeEnumFactory()); 518 t.setValue(value); 519 if (this.scope == null) 520 this.scope = new ArrayList<Enumeration<OperationParameterScope>>(); 521 this.scope.add(t); 522 return this; 523 } 524 525 /** 526 * @param value {@link #scope} (If present, indicates that the parameter applies when the operation is being invoked at the specified level.) 527 */ 528 public boolean hasScope(OperationParameterScope value) { 529 if (this.scope == null) 530 return false; 531 for (Enumeration<OperationParameterScope> v : this.scope) 532 if (v.getValue().equals(value)) // code 533 return true; 534 return false; 535 } 536 537 /** 538 * @return {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 539 */ 540 public IntegerType getMinElement() { 541 if (this.min == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.min"); 544 else if (Configuration.doAutoCreate()) 545 this.min = new IntegerType(); // bb 546 return this.min; 547 } 548 549 public boolean hasMinElement() { 550 return this.min != null && !this.min.isEmpty(); 551 } 552 553 public boolean hasMin() { 554 return this.min != null && !this.min.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 559 */ 560 public OperationDefinitionParameterComponent setMinElement(IntegerType value) { 561 this.min = value; 562 return this; 563 } 564 565 /** 566 * @return The minimum number of times this parameter SHALL appear in the request or response. 567 */ 568 public int getMin() { 569 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 570 } 571 572 /** 573 * @param value The minimum number of times this parameter SHALL appear in the request or response. 574 */ 575 public OperationDefinitionParameterComponent setMin(int value) { 576 if (this.min == null) 577 this.min = new IntegerType(); 578 this.min.setValue(value); 579 return this; 580 } 581 582 /** 583 * @return {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 584 */ 585 public StringType getMaxElement() { 586 if (this.max == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.max"); 589 else if (Configuration.doAutoCreate()) 590 this.max = new StringType(); // bb 591 return this.max; 592 } 593 594 public boolean hasMaxElement() { 595 return this.max != null && !this.max.isEmpty(); 596 } 597 598 public boolean hasMax() { 599 return this.max != null && !this.max.isEmpty(); 600 } 601 602 /** 603 * @param value {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 604 */ 605 public OperationDefinitionParameterComponent setMaxElement(StringType value) { 606 this.max = value; 607 return this; 608 } 609 610 /** 611 * @return The maximum number of times this element is permitted to appear in the request or response. 612 */ 613 public String getMax() { 614 return this.max == null ? null : this.max.getValue(); 615 } 616 617 /** 618 * @param value The maximum number of times this element is permitted to appear in the request or response. 619 */ 620 public OperationDefinitionParameterComponent setMax(String value) { 621 if (this.max == null) 622 this.max = new StringType(); 623 this.max.setValue(value); 624 return this; 625 } 626 627 /** 628 * @return {@link #documentation} (Describes the meaning or use of this parameter.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 629 */ 630 public MarkdownType getDocumentationElement() { 631 if (this.documentation == null) 632 if (Configuration.errorOnAutoCreate()) 633 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.documentation"); 634 else if (Configuration.doAutoCreate()) 635 this.documentation = new MarkdownType(); // bb 636 return this.documentation; 637 } 638 639 public boolean hasDocumentationElement() { 640 return this.documentation != null && !this.documentation.isEmpty(); 641 } 642 643 public boolean hasDocumentation() { 644 return this.documentation != null && !this.documentation.isEmpty(); 645 } 646 647 /** 648 * @param value {@link #documentation} (Describes the meaning or use of this parameter.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 649 */ 650 public OperationDefinitionParameterComponent setDocumentationElement(MarkdownType value) { 651 this.documentation = value; 652 return this; 653 } 654 655 /** 656 * @return Describes the meaning or use of this parameter. 657 */ 658 public String getDocumentation() { 659 return this.documentation == null ? null : this.documentation.getValue(); 660 } 661 662 /** 663 * @param value Describes the meaning or use of this parameter. 664 */ 665 public OperationDefinitionParameterComponent setDocumentation(String value) { 666 if (Utilities.noString(value)) 667 this.documentation = null; 668 else { 669 if (this.documentation == null) 670 this.documentation = new MarkdownType(); 671 this.documentation.setValue(value); 672 } 673 return this; 674 } 675 676 /** 677 * @return {@link #type} (The type for this parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 678 */ 679 public Enumeration<FHIRTypes> getTypeElement() { 680 if (this.type == null) 681 if (Configuration.errorOnAutoCreate()) 682 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.type"); 683 else if (Configuration.doAutoCreate()) 684 this.type = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); // bb 685 return this.type; 686 } 687 688 public boolean hasTypeElement() { 689 return this.type != null && !this.type.isEmpty(); 690 } 691 692 public boolean hasType() { 693 return this.type != null && !this.type.isEmpty(); 694 } 695 696 /** 697 * @param value {@link #type} (The type for this parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 698 */ 699 public OperationDefinitionParameterComponent setTypeElement(Enumeration<FHIRTypes> value) { 700 this.type = value; 701 return this; 702 } 703 704 /** 705 * @return The type for this parameter. 706 */ 707 public FHIRTypes getType() { 708 return this.type == null ? null : this.type.getValue(); 709 } 710 711 /** 712 * @param value The type for this parameter. 713 */ 714 public OperationDefinitionParameterComponent setType(FHIRTypes value) { 715 if (value == null) 716 this.type = null; 717 else { 718 if (this.type == null) 719 this.type = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); 720 this.type.setValue(value); 721 } 722 return this; 723 } 724 725 /** 726 * @return {@link #allowedType} (Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter.) 727 */ 728 public List<Enumeration<FHIRTypes>> getAllowedType() { 729 if (this.allowedType == null) 730 this.allowedType = new ArrayList<Enumeration<FHIRTypes>>(); 731 return this.allowedType; 732 } 733 734 /** 735 * @return Returns a reference to <code>this</code> for easy method chaining 736 */ 737 public OperationDefinitionParameterComponent setAllowedType(List<Enumeration<FHIRTypes>> theAllowedType) { 738 this.allowedType = theAllowedType; 739 return this; 740 } 741 742 public boolean hasAllowedType() { 743 if (this.allowedType == null) 744 return false; 745 for (Enumeration<FHIRTypes> item : this.allowedType) 746 if (!item.isEmpty()) 747 return true; 748 return false; 749 } 750 751 /** 752 * @return {@link #allowedType} (Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter.) 753 */ 754 public Enumeration<FHIRTypes> addAllowedTypeElement() {//2 755 Enumeration<FHIRTypes> t = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); 756 if (this.allowedType == null) 757 this.allowedType = new ArrayList<Enumeration<FHIRTypes>>(); 758 this.allowedType.add(t); 759 return t; 760 } 761 762 /** 763 * @param value {@link #allowedType} (Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter.) 764 */ 765 public OperationDefinitionParameterComponent addAllowedType(FHIRTypes value) { //1 766 Enumeration<FHIRTypes> t = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); 767 t.setValue(value); 768 if (this.allowedType == null) 769 this.allowedType = new ArrayList<Enumeration<FHIRTypes>>(); 770 this.allowedType.add(t); 771 return this; 772 } 773 774 /** 775 * @param value {@link #allowedType} (Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter.) 776 */ 777 public boolean hasAllowedType(FHIRTypes value) { 778 if (this.allowedType == null) 779 return false; 780 for (Enumeration<FHIRTypes> v : this.allowedType) 781 if (v.getValue().equals(value)) // code 782 return true; 783 return false; 784 } 785 786 /** 787 * @return {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 788 */ 789 public List<CanonicalType> getTargetProfile() { 790 if (this.targetProfile == null) 791 this.targetProfile = new ArrayList<CanonicalType>(); 792 return this.targetProfile; 793 } 794 795 /** 796 * @return Returns a reference to <code>this</code> for easy method chaining 797 */ 798 public OperationDefinitionParameterComponent setTargetProfile(List<CanonicalType> theTargetProfile) { 799 this.targetProfile = theTargetProfile; 800 return this; 801 } 802 803 public boolean hasTargetProfile() { 804 if (this.targetProfile == null) 805 return false; 806 for (CanonicalType item : this.targetProfile) 807 if (!item.isEmpty()) 808 return true; 809 return false; 810 } 811 812 /** 813 * @return {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 814 */ 815 public CanonicalType addTargetProfileElement() {//2 816 CanonicalType t = new CanonicalType(); 817 if (this.targetProfile == null) 818 this.targetProfile = new ArrayList<CanonicalType>(); 819 this.targetProfile.add(t); 820 return t; 821 } 822 823 /** 824 * @param value {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 825 */ 826 public OperationDefinitionParameterComponent addTargetProfile(String value) { //1 827 CanonicalType t = new CanonicalType(); 828 t.setValue(value); 829 if (this.targetProfile == null) 830 this.targetProfile = new ArrayList<CanonicalType>(); 831 this.targetProfile.add(t); 832 return this; 833 } 834 835 /** 836 * @param value {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 837 */ 838 public boolean hasTargetProfile(String value) { 839 if (this.targetProfile == null) 840 return false; 841 for (CanonicalType v : this.targetProfile) 842 if (v.getValue().equals(value)) // canonical 843 return true; 844 return false; 845 } 846 847 /** 848 * @return {@link #searchType} (How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string.). This is the underlying object with id, value and extensions. The accessor "getSearchType" gives direct access to the value 849 */ 850 public Enumeration<SearchParamType> getSearchTypeElement() { 851 if (this.searchType == null) 852 if (Configuration.errorOnAutoCreate()) 853 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.searchType"); 854 else if (Configuration.doAutoCreate()) 855 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 856 return this.searchType; 857 } 858 859 public boolean hasSearchTypeElement() { 860 return this.searchType != null && !this.searchType.isEmpty(); 861 } 862 863 public boolean hasSearchType() { 864 return this.searchType != null && !this.searchType.isEmpty(); 865 } 866 867 /** 868 * @param value {@link #searchType} (How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string.). This is the underlying object with id, value and extensions. The accessor "getSearchType" gives direct access to the value 869 */ 870 public OperationDefinitionParameterComponent setSearchTypeElement(Enumeration<SearchParamType> value) { 871 this.searchType = value; 872 return this; 873 } 874 875 /** 876 * @return How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string. 877 */ 878 public SearchParamType getSearchType() { 879 return this.searchType == null ? null : this.searchType.getValue(); 880 } 881 882 /** 883 * @param value How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string. 884 */ 885 public OperationDefinitionParameterComponent setSearchType(SearchParamType value) { 886 if (value == null) 887 this.searchType = null; 888 else { 889 if (this.searchType == null) 890 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 891 this.searchType.setValue(value); 892 } 893 return this; 894 } 895 896 /** 897 * @return {@link #binding} (Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).) 898 */ 899 public OperationDefinitionParameterBindingComponent getBinding() { 900 if (this.binding == null) 901 if (Configuration.errorOnAutoCreate()) 902 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.binding"); 903 else if (Configuration.doAutoCreate()) 904 this.binding = new OperationDefinitionParameterBindingComponent(); // cc 905 return this.binding; 906 } 907 908 public boolean hasBinding() { 909 return this.binding != null && !this.binding.isEmpty(); 910 } 911 912 /** 913 * @param value {@link #binding} (Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).) 914 */ 915 public OperationDefinitionParameterComponent setBinding(OperationDefinitionParameterBindingComponent value) { 916 this.binding = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #referencedFrom} (Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.) 922 */ 923 public List<OperationDefinitionParameterReferencedFromComponent> getReferencedFrom() { 924 if (this.referencedFrom == null) 925 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 926 return this.referencedFrom; 927 } 928 929 /** 930 * @return Returns a reference to <code>this</code> for easy method chaining 931 */ 932 public OperationDefinitionParameterComponent setReferencedFrom(List<OperationDefinitionParameterReferencedFromComponent> theReferencedFrom) { 933 this.referencedFrom = theReferencedFrom; 934 return this; 935 } 936 937 public boolean hasReferencedFrom() { 938 if (this.referencedFrom == null) 939 return false; 940 for (OperationDefinitionParameterReferencedFromComponent item : this.referencedFrom) 941 if (!item.isEmpty()) 942 return true; 943 return false; 944 } 945 946 public OperationDefinitionParameterReferencedFromComponent addReferencedFrom() { //3 947 OperationDefinitionParameterReferencedFromComponent t = new OperationDefinitionParameterReferencedFromComponent(); 948 if (this.referencedFrom == null) 949 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 950 this.referencedFrom.add(t); 951 return t; 952 } 953 954 public OperationDefinitionParameterComponent addReferencedFrom(OperationDefinitionParameterReferencedFromComponent t) { //3 955 if (t == null) 956 return this; 957 if (this.referencedFrom == null) 958 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 959 this.referencedFrom.add(t); 960 return this; 961 } 962 963 /** 964 * @return The first repetition of repeating field {@link #referencedFrom}, creating it if it does not already exist {3} 965 */ 966 public OperationDefinitionParameterReferencedFromComponent getReferencedFromFirstRep() { 967 if (getReferencedFrom().isEmpty()) { 968 addReferencedFrom(); 969 } 970 return getReferencedFrom().get(0); 971 } 972 973 /** 974 * @return {@link #part} (The parts of a nested Parameter.) 975 */ 976 public List<OperationDefinitionParameterComponent> getPart() { 977 if (this.part == null) 978 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 979 return this.part; 980 } 981 982 /** 983 * @return Returns a reference to <code>this</code> for easy method chaining 984 */ 985 public OperationDefinitionParameterComponent setPart(List<OperationDefinitionParameterComponent> thePart) { 986 this.part = thePart; 987 return this; 988 } 989 990 public boolean hasPart() { 991 if (this.part == null) 992 return false; 993 for (OperationDefinitionParameterComponent item : this.part) 994 if (!item.isEmpty()) 995 return true; 996 return false; 997 } 998 999 public OperationDefinitionParameterComponent addPart() { //3 1000 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 1001 if (this.part == null) 1002 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 1003 this.part.add(t); 1004 return t; 1005 } 1006 1007 public OperationDefinitionParameterComponent addPart(OperationDefinitionParameterComponent t) { //3 1008 if (t == null) 1009 return this; 1010 if (this.part == null) 1011 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 1012 this.part.add(t); 1013 return this; 1014 } 1015 1016 /** 1017 * @return The first repetition of repeating field {@link #part}, creating it if it does not already exist {3} 1018 */ 1019 public OperationDefinitionParameterComponent getPartFirstRep() { 1020 if (getPart().isEmpty()) { 1021 addPart(); 1022 } 1023 return getPart().get(0); 1024 } 1025 1026 protected void listChildren(List<Property> children) { 1027 super.listChildren(children); 1028 children.add(new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name)); 1029 children.add(new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use)); 1030 children.add(new Property("scope", "code", "If present, indicates that the parameter applies when the operation is being invoked at the specified level.", 0, java.lang.Integer.MAX_VALUE, scope)); 1031 children.add(new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min)); 1032 children.add(new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max)); 1033 children.add(new Property("documentation", "markdown", "Describes the meaning or use of this parameter.", 0, 1, documentation)); 1034 children.add(new Property("type", "code", "The type for this parameter.", 0, 1, type)); 1035 children.add(new Property("allowedType", "code", "Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter.", 0, java.lang.Integer.MAX_VALUE, allowedType)); 1036 children.add(new Property("targetProfile", "canonical(StructureDefinition)", "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, targetProfile)); 1037 children.add(new Property("searchType", "code", "How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string.", 0, 1, searchType)); 1038 children.add(new Property("binding", "", "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding)); 1039 children.add(new Property("referencedFrom", "", "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.", 0, java.lang.Integer.MAX_VALUE, referencedFrom)); 1040 children.add(new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, java.lang.Integer.MAX_VALUE, part)); 1041 } 1042 1043 @Override 1044 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1045 switch (_hash) { 1046 case 3373707: /*name*/ return new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name); 1047 case 116103: /*use*/ return new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use); 1048 case 109264468: /*scope*/ return new Property("scope", "code", "If present, indicates that the parameter applies when the operation is being invoked at the specified level.", 0, java.lang.Integer.MAX_VALUE, scope); 1049 case 108114: /*min*/ return new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min); 1050 case 107876: /*max*/ return new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max); 1051 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Describes the meaning or use of this parameter.", 0, 1, documentation); 1052 case 3575610: /*type*/ return new Property("type", "code", "The type for this parameter.", 0, 1, type); 1053 case 1512894722: /*allowedType*/ return new Property("allowedType", "code", "Support for polymorphic types. If the parameter type is abstract, this element lists allowed sub-types for the parameter.", 0, java.lang.Integer.MAX_VALUE, allowedType); 1054 case 1994521304: /*targetProfile*/ return new Property("targetProfile", "canonical(StructureDefinition)", "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, targetProfile); 1055 case -710454014: /*searchType*/ return new Property("searchType", "code", "How the parameter is understood if/when it used as search parameter. This is only used if the parameter is a string.", 0, 1, searchType); 1056 case -108220795: /*binding*/ return new Property("binding", "", "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding); 1057 case -1896721981: /*referencedFrom*/ return new Property("referencedFrom", "", "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.", 0, java.lang.Integer.MAX_VALUE, referencedFrom); 1058 case 3433459: /*part*/ return new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, java.lang.Integer.MAX_VALUE, part); 1059 default: return super.getNamedProperty(_hash, _name, _checkValid); 1060 } 1061 1062 } 1063 1064 @Override 1065 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1066 switch (hash) { 1067 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // CodeType 1068 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<OperationParameterUse> 1069 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : this.scope.toArray(new Base[this.scope.size()]); // Enumeration<OperationParameterScope> 1070 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 1071 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 1072 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 1073 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<FHIRTypes> 1074 case 1512894722: /*allowedType*/ return this.allowedType == null ? new Base[0] : this.allowedType.toArray(new Base[this.allowedType.size()]); // Enumeration<FHIRTypes> 1075 case 1994521304: /*targetProfile*/ return this.targetProfile == null ? new Base[0] : this.targetProfile.toArray(new Base[this.targetProfile.size()]); // CanonicalType 1076 case -710454014: /*searchType*/ return this.searchType == null ? new Base[0] : new Base[] {this.searchType}; // Enumeration<SearchParamType> 1077 case -108220795: /*binding*/ return this.binding == null ? new Base[0] : new Base[] {this.binding}; // OperationDefinitionParameterBindingComponent 1078 case -1896721981: /*referencedFrom*/ return this.referencedFrom == null ? new Base[0] : this.referencedFrom.toArray(new Base[this.referencedFrom.size()]); // OperationDefinitionParameterReferencedFromComponent 1079 case 3433459: /*part*/ return this.part == null ? new Base[0] : this.part.toArray(new Base[this.part.size()]); // OperationDefinitionParameterComponent 1080 default: return super.getProperty(hash, name, checkValid); 1081 } 1082 1083 } 1084 1085 @Override 1086 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1087 switch (hash) { 1088 case 3373707: // name 1089 this.name = TypeConvertor.castToCode(value); // CodeType 1090 return value; 1091 case 116103: // use 1092 value = new OperationParameterUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1093 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 1094 return value; 1095 case 109264468: // scope 1096 value = new OperationParameterScopeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1097 this.getScope().add((Enumeration) value); // Enumeration<OperationParameterScope> 1098 return value; 1099 case 108114: // min 1100 this.min = TypeConvertor.castToInteger(value); // IntegerType 1101 return value; 1102 case 107876: // max 1103 this.max = TypeConvertor.castToString(value); // StringType 1104 return value; 1105 case 1587405498: // documentation 1106 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 1107 return value; 1108 case 3575610: // type 1109 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1110 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 1111 return value; 1112 case 1512894722: // allowedType 1113 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1114 this.getAllowedType().add((Enumeration) value); // Enumeration<FHIRTypes> 1115 return value; 1116 case 1994521304: // targetProfile 1117 this.getTargetProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 1118 return value; 1119 case -710454014: // searchType 1120 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1121 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 1122 return value; 1123 case -108220795: // binding 1124 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1125 return value; 1126 case -1896721981: // referencedFrom 1127 this.getReferencedFrom().add((OperationDefinitionParameterReferencedFromComponent) value); // OperationDefinitionParameterReferencedFromComponent 1128 return value; 1129 case 3433459: // part 1130 this.getPart().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 1131 return value; 1132 default: return super.setProperty(hash, name, value); 1133 } 1134 1135 } 1136 1137 @Override 1138 public Base setProperty(String name, Base value) throws FHIRException { 1139 if (name.equals("name")) { 1140 this.name = TypeConvertor.castToCode(value); // CodeType 1141 } else if (name.equals("use")) { 1142 value = new OperationParameterUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1143 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 1144 } else if (name.equals("scope")) { 1145 value = new OperationParameterScopeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1146 this.getScope().add((Enumeration) value); 1147 } else if (name.equals("min")) { 1148 this.min = TypeConvertor.castToInteger(value); // IntegerType 1149 } else if (name.equals("max")) { 1150 this.max = TypeConvertor.castToString(value); // StringType 1151 } else if (name.equals("documentation")) { 1152 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 1153 } else if (name.equals("type")) { 1154 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1155 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 1156 } else if (name.equals("allowedType")) { 1157 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1158 this.getAllowedType().add((Enumeration) value); 1159 } else if (name.equals("targetProfile")) { 1160 this.getTargetProfile().add(TypeConvertor.castToCanonical(value)); 1161 } else if (name.equals("searchType")) { 1162 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1163 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 1164 } else if (name.equals("binding")) { 1165 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1166 } else if (name.equals("referencedFrom")) { 1167 this.getReferencedFrom().add((OperationDefinitionParameterReferencedFromComponent) value); 1168 } else if (name.equals("part")) { 1169 this.getPart().add((OperationDefinitionParameterComponent) value); 1170 } else 1171 return super.setProperty(name, value); 1172 return value; 1173 } 1174 1175 @Override 1176 public void removeChild(String name, Base value) throws FHIRException { 1177 if (name.equals("name")) { 1178 this.name = null; 1179 } else if (name.equals("use")) { 1180 value = new OperationParameterUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1181 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 1182 } else if (name.equals("scope")) { 1183 value = new OperationParameterScopeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1184 this.getScope().remove((Enumeration) value); 1185 } else if (name.equals("min")) { 1186 this.min = null; 1187 } else if (name.equals("max")) { 1188 this.max = null; 1189 } else if (name.equals("documentation")) { 1190 this.documentation = null; 1191 } else if (name.equals("type")) { 1192 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1193 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 1194 } else if (name.equals("allowedType")) { 1195 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1196 this.getAllowedType().remove((Enumeration) value); 1197 } else if (name.equals("targetProfile")) { 1198 this.getTargetProfile().remove(value); 1199 } else if (name.equals("searchType")) { 1200 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1201 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 1202 } else if (name.equals("binding")) { 1203 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1204 } else if (name.equals("referencedFrom")) { 1205 this.getReferencedFrom().remove((OperationDefinitionParameterReferencedFromComponent) value); 1206 } else if (name.equals("part")) { 1207 this.getPart().remove((OperationDefinitionParameterComponent) value); 1208 } else 1209 super.removeChild(name, value); 1210 1211 } 1212 1213 @Override 1214 public Base makeProperty(int hash, String name) throws FHIRException { 1215 switch (hash) { 1216 case 3373707: return getNameElement(); 1217 case 116103: return getUseElement(); 1218 case 109264468: return addScopeElement(); 1219 case 108114: return getMinElement(); 1220 case 107876: return getMaxElement(); 1221 case 1587405498: return getDocumentationElement(); 1222 case 3575610: return getTypeElement(); 1223 case 1512894722: return addAllowedTypeElement(); 1224 case 1994521304: return addTargetProfileElement(); 1225 case -710454014: return getSearchTypeElement(); 1226 case -108220795: return getBinding(); 1227 case -1896721981: return addReferencedFrom(); 1228 case 3433459: return addPart(); 1229 default: return super.makeProperty(hash, name); 1230 } 1231 1232 } 1233 1234 @Override 1235 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1236 switch (hash) { 1237 case 3373707: /*name*/ return new String[] {"code"}; 1238 case 116103: /*use*/ return new String[] {"code"}; 1239 case 109264468: /*scope*/ return new String[] {"code"}; 1240 case 108114: /*min*/ return new String[] {"integer"}; 1241 case 107876: /*max*/ return new String[] {"string"}; 1242 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 1243 case 3575610: /*type*/ return new String[] {"code"}; 1244 case 1512894722: /*allowedType*/ return new String[] {"code"}; 1245 case 1994521304: /*targetProfile*/ return new String[] {"canonical"}; 1246 case -710454014: /*searchType*/ return new String[] {"code"}; 1247 case -108220795: /*binding*/ return new String[] {}; 1248 case -1896721981: /*referencedFrom*/ return new String[] {}; 1249 case 3433459: /*part*/ return new String[] {"@OperationDefinition.parameter"}; 1250 default: return super.getTypesForProperty(hash, name); 1251 } 1252 1253 } 1254 1255 @Override 1256 public Base addChild(String name) throws FHIRException { 1257 if (name.equals("name")) { 1258 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.name"); 1259 } 1260 else if (name.equals("use")) { 1261 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.use"); 1262 } 1263 else if (name.equals("scope")) { 1264 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.scope"); 1265 } 1266 else if (name.equals("min")) { 1267 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.min"); 1268 } 1269 else if (name.equals("max")) { 1270 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.max"); 1271 } 1272 else if (name.equals("documentation")) { 1273 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.documentation"); 1274 } 1275 else if (name.equals("type")) { 1276 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.type"); 1277 } 1278 else if (name.equals("allowedType")) { 1279 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.allowedType"); 1280 } 1281 else if (name.equals("targetProfile")) { 1282 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.targetProfile"); 1283 } 1284 else if (name.equals("searchType")) { 1285 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.searchType"); 1286 } 1287 else if (name.equals("binding")) { 1288 this.binding = new OperationDefinitionParameterBindingComponent(); 1289 return this.binding; 1290 } 1291 else if (name.equals("referencedFrom")) { 1292 return addReferencedFrom(); 1293 } 1294 else if (name.equals("part")) { 1295 return addPart(); 1296 } 1297 else 1298 return super.addChild(name); 1299 } 1300 1301 public OperationDefinitionParameterComponent copy() { 1302 OperationDefinitionParameterComponent dst = new OperationDefinitionParameterComponent(); 1303 copyValues(dst); 1304 return dst; 1305 } 1306 1307 public void copyValues(OperationDefinitionParameterComponent dst) { 1308 super.copyValues(dst); 1309 dst.name = name == null ? null : name.copy(); 1310 dst.use = use == null ? null : use.copy(); 1311 if (scope != null) { 1312 dst.scope = new ArrayList<Enumeration<OperationParameterScope>>(); 1313 for (Enumeration<OperationParameterScope> i : scope) 1314 dst.scope.add(i.copy()); 1315 }; 1316 dst.min = min == null ? null : min.copy(); 1317 dst.max = max == null ? null : max.copy(); 1318 dst.documentation = documentation == null ? null : documentation.copy(); 1319 dst.type = type == null ? null : type.copy(); 1320 if (allowedType != null) { 1321 dst.allowedType = new ArrayList<Enumeration<FHIRTypes>>(); 1322 for (Enumeration<FHIRTypes> i : allowedType) 1323 dst.allowedType.add(i.copy()); 1324 }; 1325 if (targetProfile != null) { 1326 dst.targetProfile = new ArrayList<CanonicalType>(); 1327 for (CanonicalType i : targetProfile) 1328 dst.targetProfile.add(i.copy()); 1329 }; 1330 dst.searchType = searchType == null ? null : searchType.copy(); 1331 dst.binding = binding == null ? null : binding.copy(); 1332 if (referencedFrom != null) { 1333 dst.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 1334 for (OperationDefinitionParameterReferencedFromComponent i : referencedFrom) 1335 dst.referencedFrom.add(i.copy()); 1336 }; 1337 if (part != null) { 1338 dst.part = new ArrayList<OperationDefinitionParameterComponent>(); 1339 for (OperationDefinitionParameterComponent i : part) 1340 dst.part.add(i.copy()); 1341 }; 1342 } 1343 1344 @Override 1345 public boolean equalsDeep(Base other_) { 1346 if (!super.equalsDeep(other_)) 1347 return false; 1348 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1349 return false; 1350 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1351 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(scope, o.scope, true) 1352 && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) 1353 && compareDeep(type, o.type, true) && compareDeep(allowedType, o.allowedType, true) && compareDeep(targetProfile, o.targetProfile, true) 1354 && compareDeep(searchType, o.searchType, true) && compareDeep(binding, o.binding, true) && compareDeep(referencedFrom, o.referencedFrom, true) 1355 && compareDeep(part, o.part, true); 1356 } 1357 1358 @Override 1359 public boolean equalsShallow(Base other_) { 1360 if (!super.equalsShallow(other_)) 1361 return false; 1362 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1363 return false; 1364 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1365 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(scope, o.scope, true) 1366 && compareValues(min, o.min, true) && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) 1367 && compareValues(type, o.type, true) && compareValues(allowedType, o.allowedType, true) && compareValues(targetProfile, o.targetProfile, true) 1368 && compareValues(searchType, o.searchType, true); 1369 } 1370 1371 public boolean isEmpty() { 1372 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, use, scope, min 1373 , max, documentation, type, allowedType, targetProfile, searchType, binding, referencedFrom 1374 , part); 1375 } 1376 1377 public String fhirType() { 1378 return "OperationDefinition.parameter"; 1379 1380 } 1381 1382 } 1383 1384 @Block() 1385 public static class OperationDefinitionParameterBindingComponent extends BackboneElement implements IBaseBackboneElement { 1386 /** 1387 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 1388 */ 1389 @Child(name = "strength", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1390 @Description(shortDefinition="required | extensible | preferred | example", formalDefinition="Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances." ) 1391 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/binding-strength") 1392 protected Enumeration<BindingStrength> strength; 1393 1394 /** 1395 * Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. 1396 */ 1397 @Child(name = "valueSet", type = {CanonicalType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1398 @Description(shortDefinition="Source of value set", formalDefinition="Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used." ) 1399 protected CanonicalType valueSet; 1400 1401 private static final long serialVersionUID = -2048653907L; 1402 1403 /** 1404 * Constructor 1405 */ 1406 public OperationDefinitionParameterBindingComponent() { 1407 super(); 1408 } 1409 1410 /** 1411 * Constructor 1412 */ 1413 public OperationDefinitionParameterBindingComponent(BindingStrength strength, String valueSet) { 1414 super(); 1415 this.setStrength(strength); 1416 this.setValueSet(valueSet); 1417 } 1418 1419 /** 1420 * @return {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 1421 */ 1422 public Enumeration<BindingStrength> getStrengthElement() { 1423 if (this.strength == null) 1424 if (Configuration.errorOnAutoCreate()) 1425 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.strength"); 1426 else if (Configuration.doAutoCreate()) 1427 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 1428 return this.strength; 1429 } 1430 1431 public boolean hasStrengthElement() { 1432 return this.strength != null && !this.strength.isEmpty(); 1433 } 1434 1435 public boolean hasStrength() { 1436 return this.strength != null && !this.strength.isEmpty(); 1437 } 1438 1439 /** 1440 * @param value {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 1441 */ 1442 public OperationDefinitionParameterBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 1443 this.strength = value; 1444 return this; 1445 } 1446 1447 /** 1448 * @return Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 1449 */ 1450 public BindingStrength getStrength() { 1451 return this.strength == null ? null : this.strength.getValue(); 1452 } 1453 1454 /** 1455 * @param value Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 1456 */ 1457 public OperationDefinitionParameterBindingComponent setStrength(BindingStrength value) { 1458 if (this.strength == null) 1459 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 1460 this.strength.setValue(value); 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 1466 */ 1467 public CanonicalType getValueSetElement() { 1468 if (this.valueSet == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.valueSet"); 1471 else if (Configuration.doAutoCreate()) 1472 this.valueSet = new CanonicalType(); // bb 1473 return this.valueSet; 1474 } 1475 1476 public boolean hasValueSetElement() { 1477 return this.valueSet != null && !this.valueSet.isEmpty(); 1478 } 1479 1480 public boolean hasValueSet() { 1481 return this.valueSet != null && !this.valueSet.isEmpty(); 1482 } 1483 1484 /** 1485 * @param value {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 1486 */ 1487 public OperationDefinitionParameterBindingComponent setValueSetElement(CanonicalType value) { 1488 this.valueSet = value; 1489 return this; 1490 } 1491 1492 /** 1493 * @return Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. 1494 */ 1495 public String getValueSet() { 1496 return this.valueSet == null ? null : this.valueSet.getValue(); 1497 } 1498 1499 /** 1500 * @param value Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. 1501 */ 1502 public OperationDefinitionParameterBindingComponent setValueSet(String value) { 1503 if (this.valueSet == null) 1504 this.valueSet = new CanonicalType(); 1505 this.valueSet.setValue(value); 1506 return this; 1507 } 1508 1509 protected void listChildren(List<Property> children) { 1510 super.listChildren(children); 1511 children.add(new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength)); 1512 children.add(new Property("valueSet", "canonical(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 0, 1, valueSet)); 1513 } 1514 1515 @Override 1516 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1517 switch (_hash) { 1518 case 1791316033: /*strength*/ return new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength); 1519 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 0, 1, valueSet); 1520 default: return super.getNamedProperty(_hash, _name, _checkValid); 1521 } 1522 1523 } 1524 1525 @Override 1526 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1527 switch (hash) { 1528 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // Enumeration<BindingStrength> 1529 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 1530 default: return super.getProperty(hash, name, checkValid); 1531 } 1532 1533 } 1534 1535 @Override 1536 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1537 switch (hash) { 1538 case 1791316033: // strength 1539 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 1540 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1541 return value; 1542 case -1410174671: // valueSet 1543 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1544 return value; 1545 default: return super.setProperty(hash, name, value); 1546 } 1547 1548 } 1549 1550 @Override 1551 public Base setProperty(String name, Base value) throws FHIRException { 1552 if (name.equals("strength")) { 1553 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 1554 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1555 } else if (name.equals("valueSet")) { 1556 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 1557 } else 1558 return super.setProperty(name, value); 1559 return value; 1560 } 1561 1562 @Override 1563 public void removeChild(String name, Base value) throws FHIRException { 1564 if (name.equals("strength")) { 1565 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 1566 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1567 } else if (name.equals("valueSet")) { 1568 this.valueSet = null; 1569 } else 1570 super.removeChild(name, value); 1571 1572 } 1573 1574 @Override 1575 public Base makeProperty(int hash, String name) throws FHIRException { 1576 switch (hash) { 1577 case 1791316033: return getStrengthElement(); 1578 case -1410174671: return getValueSetElement(); 1579 default: return super.makeProperty(hash, name); 1580 } 1581 1582 } 1583 1584 @Override 1585 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1586 switch (hash) { 1587 case 1791316033: /*strength*/ return new String[] {"code"}; 1588 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 1589 default: return super.getTypesForProperty(hash, name); 1590 } 1591 1592 } 1593 1594 @Override 1595 public Base addChild(String name) throws FHIRException { 1596 if (name.equals("strength")) { 1597 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.binding.strength"); 1598 } 1599 else if (name.equals("valueSet")) { 1600 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.binding.valueSet"); 1601 } 1602 else 1603 return super.addChild(name); 1604 } 1605 1606 public OperationDefinitionParameterBindingComponent copy() { 1607 OperationDefinitionParameterBindingComponent dst = new OperationDefinitionParameterBindingComponent(); 1608 copyValues(dst); 1609 return dst; 1610 } 1611 1612 public void copyValues(OperationDefinitionParameterBindingComponent dst) { 1613 super.copyValues(dst); 1614 dst.strength = strength == null ? null : strength.copy(); 1615 dst.valueSet = valueSet == null ? null : valueSet.copy(); 1616 } 1617 1618 @Override 1619 public boolean equalsDeep(Base other_) { 1620 if (!super.equalsDeep(other_)) 1621 return false; 1622 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1623 return false; 1624 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1625 return compareDeep(strength, o.strength, true) && compareDeep(valueSet, o.valueSet, true); 1626 } 1627 1628 @Override 1629 public boolean equalsShallow(Base other_) { 1630 if (!super.equalsShallow(other_)) 1631 return false; 1632 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1633 return false; 1634 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1635 return compareValues(strength, o.strength, true) && compareValues(valueSet, o.valueSet, true); 1636 } 1637 1638 public boolean isEmpty() { 1639 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, valueSet); 1640 } 1641 1642 public String fhirType() { 1643 return "OperationDefinition.parameter.binding"; 1644 1645 } 1646 1647 } 1648 1649 @Block() 1650 public static class OperationDefinitionParameterReferencedFromComponent extends BackboneElement implements IBaseBackboneElement { 1651 /** 1652 * The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource. 1653 */ 1654 @Child(name = "source", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1655 @Description(shortDefinition="Referencing parameter", formalDefinition="The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource." ) 1656 protected StringType source; 1657 1658 /** 1659 * The id of the element in the referencing resource that is expected to resolve to this resource. 1660 */ 1661 @Child(name = "sourceId", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1662 @Description(shortDefinition="Element id of reference", formalDefinition="The id of the element in the referencing resource that is expected to resolve to this resource." ) 1663 protected StringType sourceId; 1664 1665 private static final long serialVersionUID = -104239783L; 1666 1667 /** 1668 * Constructor 1669 */ 1670 public OperationDefinitionParameterReferencedFromComponent() { 1671 super(); 1672 } 1673 1674 /** 1675 * Constructor 1676 */ 1677 public OperationDefinitionParameterReferencedFromComponent(String source) { 1678 super(); 1679 this.setSource(source); 1680 } 1681 1682 /** 1683 * @return {@link #source} (The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 1684 */ 1685 public StringType getSourceElement() { 1686 if (this.source == null) 1687 if (Configuration.errorOnAutoCreate()) 1688 throw new Error("Attempt to auto-create OperationDefinitionParameterReferencedFromComponent.source"); 1689 else if (Configuration.doAutoCreate()) 1690 this.source = new StringType(); // bb 1691 return this.source; 1692 } 1693 1694 public boolean hasSourceElement() { 1695 return this.source != null && !this.source.isEmpty(); 1696 } 1697 1698 public boolean hasSource() { 1699 return this.source != null && !this.source.isEmpty(); 1700 } 1701 1702 /** 1703 * @param value {@link #source} (The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 1704 */ 1705 public OperationDefinitionParameterReferencedFromComponent setSourceElement(StringType value) { 1706 this.source = value; 1707 return this; 1708 } 1709 1710 /** 1711 * @return The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource. 1712 */ 1713 public String getSource() { 1714 return this.source == null ? null : this.source.getValue(); 1715 } 1716 1717 /** 1718 * @param value The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource. 1719 */ 1720 public OperationDefinitionParameterReferencedFromComponent setSource(String value) { 1721 if (this.source == null) 1722 this.source = new StringType(); 1723 this.source.setValue(value); 1724 return this; 1725 } 1726 1727 /** 1728 * @return {@link #sourceId} (The id of the element in the referencing resource that is expected to resolve to this resource.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 1729 */ 1730 public StringType getSourceIdElement() { 1731 if (this.sourceId == null) 1732 if (Configuration.errorOnAutoCreate()) 1733 throw new Error("Attempt to auto-create OperationDefinitionParameterReferencedFromComponent.sourceId"); 1734 else if (Configuration.doAutoCreate()) 1735 this.sourceId = new StringType(); // bb 1736 return this.sourceId; 1737 } 1738 1739 public boolean hasSourceIdElement() { 1740 return this.sourceId != null && !this.sourceId.isEmpty(); 1741 } 1742 1743 public boolean hasSourceId() { 1744 return this.sourceId != null && !this.sourceId.isEmpty(); 1745 } 1746 1747 /** 1748 * @param value {@link #sourceId} (The id of the element in the referencing resource that is expected to resolve to this resource.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 1749 */ 1750 public OperationDefinitionParameterReferencedFromComponent setSourceIdElement(StringType value) { 1751 this.sourceId = value; 1752 return this; 1753 } 1754 1755 /** 1756 * @return The id of the element in the referencing resource that is expected to resolve to this resource. 1757 */ 1758 public String getSourceId() { 1759 return this.sourceId == null ? null : this.sourceId.getValue(); 1760 } 1761 1762 /** 1763 * @param value The id of the element in the referencing resource that is expected to resolve to this resource. 1764 */ 1765 public OperationDefinitionParameterReferencedFromComponent setSourceId(String value) { 1766 if (Utilities.noString(value)) 1767 this.sourceId = null; 1768 else { 1769 if (this.sourceId == null) 1770 this.sourceId = new StringType(); 1771 this.sourceId.setValue(value); 1772 } 1773 return this; 1774 } 1775 1776 protected void listChildren(List<Property> children) { 1777 super.listChildren(children); 1778 children.add(new Property("source", "string", "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.", 0, 1, source)); 1779 children.add(new Property("sourceId", "string", "The id of the element in the referencing resource that is expected to resolve to this resource.", 0, 1, sourceId)); 1780 } 1781 1782 @Override 1783 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1784 switch (_hash) { 1785 case -896505829: /*source*/ return new Property("source", "string", "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.", 0, 1, source); 1786 case 1746327190: /*sourceId*/ return new Property("sourceId", "string", "The id of the element in the referencing resource that is expected to resolve to this resource.", 0, 1, sourceId); 1787 default: return super.getNamedProperty(_hash, _name, _checkValid); 1788 } 1789 1790 } 1791 1792 @Override 1793 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1794 switch (hash) { 1795 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // StringType 1796 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // StringType 1797 default: return super.getProperty(hash, name, checkValid); 1798 } 1799 1800 } 1801 1802 @Override 1803 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1804 switch (hash) { 1805 case -896505829: // source 1806 this.source = TypeConvertor.castToString(value); // StringType 1807 return value; 1808 case 1746327190: // sourceId 1809 this.sourceId = TypeConvertor.castToString(value); // StringType 1810 return value; 1811 default: return super.setProperty(hash, name, value); 1812 } 1813 1814 } 1815 1816 @Override 1817 public Base setProperty(String name, Base value) throws FHIRException { 1818 if (name.equals("source")) { 1819 this.source = TypeConvertor.castToString(value); // StringType 1820 } else if (name.equals("sourceId")) { 1821 this.sourceId = TypeConvertor.castToString(value); // StringType 1822 } else 1823 return super.setProperty(name, value); 1824 return value; 1825 } 1826 1827 @Override 1828 public void removeChild(String name, Base value) throws FHIRException { 1829 if (name.equals("source")) { 1830 this.source = null; 1831 } else if (name.equals("sourceId")) { 1832 this.sourceId = null; 1833 } else 1834 super.removeChild(name, value); 1835 1836 } 1837 1838 @Override 1839 public Base makeProperty(int hash, String name) throws FHIRException { 1840 switch (hash) { 1841 case -896505829: return getSourceElement(); 1842 case 1746327190: return getSourceIdElement(); 1843 default: return super.makeProperty(hash, name); 1844 } 1845 1846 } 1847 1848 @Override 1849 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1850 switch (hash) { 1851 case -896505829: /*source*/ return new String[] {"string"}; 1852 case 1746327190: /*sourceId*/ return new String[] {"string"}; 1853 default: return super.getTypesForProperty(hash, name); 1854 } 1855 1856 } 1857 1858 @Override 1859 public Base addChild(String name) throws FHIRException { 1860 if (name.equals("source")) { 1861 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.referencedFrom.source"); 1862 } 1863 else if (name.equals("sourceId")) { 1864 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameter.referencedFrom.sourceId"); 1865 } 1866 else 1867 return super.addChild(name); 1868 } 1869 1870 public OperationDefinitionParameterReferencedFromComponent copy() { 1871 OperationDefinitionParameterReferencedFromComponent dst = new OperationDefinitionParameterReferencedFromComponent(); 1872 copyValues(dst); 1873 return dst; 1874 } 1875 1876 public void copyValues(OperationDefinitionParameterReferencedFromComponent dst) { 1877 super.copyValues(dst); 1878 dst.source = source == null ? null : source.copy(); 1879 dst.sourceId = sourceId == null ? null : sourceId.copy(); 1880 } 1881 1882 @Override 1883 public boolean equalsDeep(Base other_) { 1884 if (!super.equalsDeep(other_)) 1885 return false; 1886 if (!(other_ instanceof OperationDefinitionParameterReferencedFromComponent)) 1887 return false; 1888 OperationDefinitionParameterReferencedFromComponent o = (OperationDefinitionParameterReferencedFromComponent) other_; 1889 return compareDeep(source, o.source, true) && compareDeep(sourceId, o.sourceId, true); 1890 } 1891 1892 @Override 1893 public boolean equalsShallow(Base other_) { 1894 if (!super.equalsShallow(other_)) 1895 return false; 1896 if (!(other_ instanceof OperationDefinitionParameterReferencedFromComponent)) 1897 return false; 1898 OperationDefinitionParameterReferencedFromComponent o = (OperationDefinitionParameterReferencedFromComponent) other_; 1899 return compareValues(source, o.source, true) && compareValues(sourceId, o.sourceId, true); 1900 } 1901 1902 public boolean isEmpty() { 1903 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(source, sourceId); 1904 } 1905 1906 public String fhirType() { 1907 return "OperationDefinition.parameter.referencedFrom"; 1908 1909 } 1910 1911 } 1912 1913 @Block() 1914 public static class OperationDefinitionOverloadComponent extends BackboneElement implements IBaseBackboneElement { 1915 /** 1916 * Name of parameter to include in overload. 1917 */ 1918 @Child(name = "parameterName", type = {StringType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1919 @Description(shortDefinition="Name of parameter to include in overload", formalDefinition="Name of parameter to include in overload." ) 1920 protected List<StringType> parameterName; 1921 1922 /** 1923 * Comments to go on overload. 1924 */ 1925 @Child(name = "comment", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1926 @Description(shortDefinition="Comments to go on overload", formalDefinition="Comments to go on overload." ) 1927 protected StringType comment; 1928 1929 private static final long serialVersionUID = -907948545L; 1930 1931 /** 1932 * Constructor 1933 */ 1934 public OperationDefinitionOverloadComponent() { 1935 super(); 1936 } 1937 1938 /** 1939 * @return {@link #parameterName} (Name of parameter to include in overload.) 1940 */ 1941 public List<StringType> getParameterName() { 1942 if (this.parameterName == null) 1943 this.parameterName = new ArrayList<StringType>(); 1944 return this.parameterName; 1945 } 1946 1947 /** 1948 * @return Returns a reference to <code>this</code> for easy method chaining 1949 */ 1950 public OperationDefinitionOverloadComponent setParameterName(List<StringType> theParameterName) { 1951 this.parameterName = theParameterName; 1952 return this; 1953 } 1954 1955 public boolean hasParameterName() { 1956 if (this.parameterName == null) 1957 return false; 1958 for (StringType item : this.parameterName) 1959 if (!item.isEmpty()) 1960 return true; 1961 return false; 1962 } 1963 1964 /** 1965 * @return {@link #parameterName} (Name of parameter to include in overload.) 1966 */ 1967 public StringType addParameterNameElement() {//2 1968 StringType t = new StringType(); 1969 if (this.parameterName == null) 1970 this.parameterName = new ArrayList<StringType>(); 1971 this.parameterName.add(t); 1972 return t; 1973 } 1974 1975 /** 1976 * @param value {@link #parameterName} (Name of parameter to include in overload.) 1977 */ 1978 public OperationDefinitionOverloadComponent addParameterName(String value) { //1 1979 StringType t = new StringType(); 1980 t.setValue(value); 1981 if (this.parameterName == null) 1982 this.parameterName = new ArrayList<StringType>(); 1983 this.parameterName.add(t); 1984 return this; 1985 } 1986 1987 /** 1988 * @param value {@link #parameterName} (Name of parameter to include in overload.) 1989 */ 1990 public boolean hasParameterName(String value) { 1991 if (this.parameterName == null) 1992 return false; 1993 for (StringType v : this.parameterName) 1994 if (v.getValue().equals(value)) // string 1995 return true; 1996 return false; 1997 } 1998 1999 /** 2000 * @return {@link #comment} (Comments to go on overload.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2001 */ 2002 public StringType getCommentElement() { 2003 if (this.comment == null) 2004 if (Configuration.errorOnAutoCreate()) 2005 throw new Error("Attempt to auto-create OperationDefinitionOverloadComponent.comment"); 2006 else if (Configuration.doAutoCreate()) 2007 this.comment = new StringType(); // bb 2008 return this.comment; 2009 } 2010 2011 public boolean hasCommentElement() { 2012 return this.comment != null && !this.comment.isEmpty(); 2013 } 2014 2015 public boolean hasComment() { 2016 return this.comment != null && !this.comment.isEmpty(); 2017 } 2018 2019 /** 2020 * @param value {@link #comment} (Comments to go on overload.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2021 */ 2022 public OperationDefinitionOverloadComponent setCommentElement(StringType value) { 2023 this.comment = value; 2024 return this; 2025 } 2026 2027 /** 2028 * @return Comments to go on overload. 2029 */ 2030 public String getComment() { 2031 return this.comment == null ? null : this.comment.getValue(); 2032 } 2033 2034 /** 2035 * @param value Comments to go on overload. 2036 */ 2037 public OperationDefinitionOverloadComponent setComment(String value) { 2038 if (Utilities.noString(value)) 2039 this.comment = null; 2040 else { 2041 if (this.comment == null) 2042 this.comment = new StringType(); 2043 this.comment.setValue(value); 2044 } 2045 return this; 2046 } 2047 2048 protected void listChildren(List<Property> children) { 2049 super.listChildren(children); 2050 children.add(new Property("parameterName", "string", "Name of parameter to include in overload.", 0, java.lang.Integer.MAX_VALUE, parameterName)); 2051 children.add(new Property("comment", "string", "Comments to go on overload.", 0, 1, comment)); 2052 } 2053 2054 @Override 2055 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2056 switch (_hash) { 2057 case -379607596: /*parameterName*/ return new Property("parameterName", "string", "Name of parameter to include in overload.", 0, java.lang.Integer.MAX_VALUE, parameterName); 2058 case 950398559: /*comment*/ return new Property("comment", "string", "Comments to go on overload.", 0, 1, comment); 2059 default: return super.getNamedProperty(_hash, _name, _checkValid); 2060 } 2061 2062 } 2063 2064 @Override 2065 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2066 switch (hash) { 2067 case -379607596: /*parameterName*/ return this.parameterName == null ? new Base[0] : this.parameterName.toArray(new Base[this.parameterName.size()]); // StringType 2068 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 2069 default: return super.getProperty(hash, name, checkValid); 2070 } 2071 2072 } 2073 2074 @Override 2075 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2076 switch (hash) { 2077 case -379607596: // parameterName 2078 this.getParameterName().add(TypeConvertor.castToString(value)); // StringType 2079 return value; 2080 case 950398559: // comment 2081 this.comment = TypeConvertor.castToString(value); // StringType 2082 return value; 2083 default: return super.setProperty(hash, name, value); 2084 } 2085 2086 } 2087 2088 @Override 2089 public Base setProperty(String name, Base value) throws FHIRException { 2090 if (name.equals("parameterName")) { 2091 this.getParameterName().add(TypeConvertor.castToString(value)); 2092 } else if (name.equals("comment")) { 2093 this.comment = TypeConvertor.castToString(value); // StringType 2094 } else 2095 return super.setProperty(name, value); 2096 return value; 2097 } 2098 2099 @Override 2100 public void removeChild(String name, Base value) throws FHIRException { 2101 if (name.equals("parameterName")) { 2102 this.getParameterName().remove(value); 2103 } else if (name.equals("comment")) { 2104 this.comment = null; 2105 } else 2106 super.removeChild(name, value); 2107 2108 } 2109 2110 @Override 2111 public Base makeProperty(int hash, String name) throws FHIRException { 2112 switch (hash) { 2113 case -379607596: return addParameterNameElement(); 2114 case 950398559: return getCommentElement(); 2115 default: return super.makeProperty(hash, name); 2116 } 2117 2118 } 2119 2120 @Override 2121 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2122 switch (hash) { 2123 case -379607596: /*parameterName*/ return new String[] {"string"}; 2124 case 950398559: /*comment*/ return new String[] {"string"}; 2125 default: return super.getTypesForProperty(hash, name); 2126 } 2127 2128 } 2129 2130 @Override 2131 public Base addChild(String name) throws FHIRException { 2132 if (name.equals("parameterName")) { 2133 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.overload.parameterName"); 2134 } 2135 else if (name.equals("comment")) { 2136 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.overload.comment"); 2137 } 2138 else 2139 return super.addChild(name); 2140 } 2141 2142 public OperationDefinitionOverloadComponent copy() { 2143 OperationDefinitionOverloadComponent dst = new OperationDefinitionOverloadComponent(); 2144 copyValues(dst); 2145 return dst; 2146 } 2147 2148 public void copyValues(OperationDefinitionOverloadComponent dst) { 2149 super.copyValues(dst); 2150 if (parameterName != null) { 2151 dst.parameterName = new ArrayList<StringType>(); 2152 for (StringType i : parameterName) 2153 dst.parameterName.add(i.copy()); 2154 }; 2155 dst.comment = comment == null ? null : comment.copy(); 2156 } 2157 2158 @Override 2159 public boolean equalsDeep(Base other_) { 2160 if (!super.equalsDeep(other_)) 2161 return false; 2162 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 2163 return false; 2164 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 2165 return compareDeep(parameterName, o.parameterName, true) && compareDeep(comment, o.comment, true) 2166 ; 2167 } 2168 2169 @Override 2170 public boolean equalsShallow(Base other_) { 2171 if (!super.equalsShallow(other_)) 2172 return false; 2173 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 2174 return false; 2175 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 2176 return compareValues(parameterName, o.parameterName, true) && compareValues(comment, o.comment, true) 2177 ; 2178 } 2179 2180 public boolean isEmpty() { 2181 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(parameterName, comment); 2182 } 2183 2184 public String fhirType() { 2185 return "OperationDefinition.overload"; 2186 2187 } 2188 2189 } 2190 2191 /** 2192 * An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers. 2193 */ 2194 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2195 @Description(shortDefinition="Canonical identifier for this operation definition, represented as an absolute URI (globally unique)", formalDefinition="An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers." ) 2196 protected UriType url; 2197 2198 /** 2199 * A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance. 2200 */ 2201 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2202 @Description(shortDefinition="Additional identifier for the implementation guide (business identifier)", formalDefinition="A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2203 protected List<Identifier> identifier; 2204 2205 /** 2206 * The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2207 */ 2208 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2209 @Description(shortDefinition="Business version of the operation definition", formalDefinition="The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 2210 protected StringType version; 2211 2212 /** 2213 * Indicates the mechanism used to compare versions to determine which is more current. 2214 */ 2215 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2216 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 2217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2218 protected DataType versionAlgorithm; 2219 2220 /** 2221 * A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2222 */ 2223 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 2224 @Description(shortDefinition="Name for this operation definition (computer friendly)", formalDefinition="A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2225 protected StringType name; 2226 2227 /** 2228 * A short, descriptive, user-friendly title for the operation definition. 2229 */ 2230 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2231 @Description(shortDefinition="Name for this operation definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the operation definition." ) 2232 protected StringType title; 2233 2234 /** 2235 * The current state of this operation definition. 2236 */ 2237 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 2238 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of this operation definition." ) 2239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2240 protected Enumeration<PublicationStatus> status; 2241 2242 /** 2243 * Whether this is an operation or a named query. 2244 */ 2245 @Child(name = "kind", type = {CodeType.class}, order=7, min=1, max=1, modifier=false, summary=true) 2246 @Description(shortDefinition="operation | query", formalDefinition="Whether this is an operation or a named query." ) 2247 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-kind") 2248 protected Enumeration<OperationKind> kind; 2249 2250 /** 2251 * A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 2252 */ 2253 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 2254 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage." ) 2255 protected BooleanType experimental; 2256 2257 /** 2258 * The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes. 2259 */ 2260 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2261 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes." ) 2262 protected DateTimeType date; 2263 2264 /** 2265 * The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition. 2266 */ 2267 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 2268 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition." ) 2269 protected StringType publisher; 2270 2271 /** 2272 * Contact details to assist a user in finding and communicating with the publisher. 2273 */ 2274 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2275 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2276 protected List<ContactDetail> contact; 2277 2278 /** 2279 * A free text natural language description of the operation definition from a consumer's perspective. 2280 */ 2281 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=false) 2282 @Description(shortDefinition="Natural language description of the operation definition", formalDefinition="A free text natural language description of the operation definition from a consumer's perspective." ) 2283 protected MarkdownType description; 2284 2285 /** 2286 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition. 2287 */ 2288 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2289 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition." ) 2290 protected List<UsageContext> useContext; 2291 2292 /** 2293 * A legal or geographic region in which the operation definition is intended to be used. 2294 */ 2295 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2296 @Description(shortDefinition="Intended jurisdiction for operation definition (if applicable)", formalDefinition="A legal or geographic region in which the operation definition is intended to be used." ) 2297 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2298 protected List<CodeableConcept> jurisdiction; 2299 2300 /** 2301 * Explanation of why this operation definition is needed and why it has been designed as it has. 2302 */ 2303 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 2304 @Description(shortDefinition="Why this operation definition is defined", formalDefinition="Explanation of why this operation definition is needed and why it has been designed as it has." ) 2305 protected MarkdownType purpose; 2306 2307 /** 2308 * A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition. 2309 */ 2310 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2311 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition." ) 2312 protected MarkdownType copyright; 2313 2314 /** 2315 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2316 */ 2317 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 2318 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 2319 protected StringType copyrightLabel; 2320 2321 /** 2322 * Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'. 2323 */ 2324 @Child(name = "affectsState", type = {BooleanType.class}, order=18, min=0, max=1, modifier=false, summary=true) 2325 @Description(shortDefinition="Whether content is changed by the operation", formalDefinition="Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'." ) 2326 protected BooleanType affectsState; 2327 2328 /** 2329 * The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code. 2330 */ 2331 @Child(name = "code", type = {CodeType.class}, order=19, min=1, max=1, modifier=false, summary=true) 2332 @Description(shortDefinition="Recommended name for operation in search url", formalDefinition="The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code." ) 2333 protected CodeType code; 2334 2335 /** 2336 * Additional information about how to use this operation or named query. 2337 */ 2338 @Child(name = "comment", type = {MarkdownType.class}, order=20, min=0, max=1, modifier=false, summary=false) 2339 @Description(shortDefinition="Additional information about use", formalDefinition="Additional information about how to use this operation or named query." ) 2340 protected MarkdownType comment; 2341 2342 /** 2343 * Indicates that this operation definition is a constraining profile on the base. 2344 */ 2345 @Child(name = "base", type = {CanonicalType.class}, order=21, min=0, max=1, modifier=false, summary=true) 2346 @Description(shortDefinition="Marks this as a profile of the base", formalDefinition="Indicates that this operation definition is a constraining profile on the base." ) 2347 protected CanonicalType base; 2348 2349 /** 2350 * The types on which this operation can be executed. 2351 */ 2352 @Child(name = "resource", type = {CodeType.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2353 @Description(shortDefinition="Types this operation applies to", formalDefinition="The types on which this operation can be executed." ) 2354 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-independent-all-resource-types") 2355 protected List<Enumeration<VersionIndependentResourceTypesAll>> resource; 2356 2357 /** 2358 * Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context). 2359 */ 2360 @Child(name = "system", type = {BooleanType.class}, order=23, min=1, max=1, modifier=false, summary=true) 2361 @Description(shortDefinition="Invoke at the system level?", formalDefinition="Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context)." ) 2362 protected BooleanType system; 2363 2364 /** 2365 * Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context). 2366 */ 2367 @Child(name = "type", type = {BooleanType.class}, order=24, min=1, max=1, modifier=false, summary=true) 2368 @Description(shortDefinition="Invoke at the type level?", formalDefinition="Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context)." ) 2369 protected BooleanType type; 2370 2371 /** 2372 * Indicates whether this operation can be invoked on a particular instance of one of the given types. 2373 */ 2374 @Child(name = "instance", type = {BooleanType.class}, order=25, min=1, max=1, modifier=false, summary=true) 2375 @Description(shortDefinition="Invoke on an instance?", formalDefinition="Indicates whether this operation can be invoked on a particular instance of one of the given types." ) 2376 protected BooleanType instance; 2377 2378 /** 2379 * Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole. 2380 */ 2381 @Child(name = "inputProfile", type = {CanonicalType.class}, order=26, min=0, max=1, modifier=false, summary=false) 2382 @Description(shortDefinition="Validation information for in parameters", formalDefinition="Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole." ) 2383 protected CanonicalType inputProfile; 2384 2385 /** 2386 * Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource. 2387 */ 2388 @Child(name = "outputProfile", type = {CanonicalType.class}, order=27, min=0, max=1, modifier=false, summary=false) 2389 @Description(shortDefinition="Validation information for out parameters", formalDefinition="Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource." ) 2390 protected CanonicalType outputProfile; 2391 2392 /** 2393 * The parameters for the operation/query. 2394 */ 2395 @Child(name = "parameter", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2396 @Description(shortDefinition="Parameters for the operation/query", formalDefinition="The parameters for the operation/query." ) 2397 protected List<OperationDefinitionParameterComponent> parameter; 2398 2399 /** 2400 * Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation. 2401 */ 2402 @Child(name = "overload", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2403 @Description(shortDefinition="Define overloaded variants for when generating code", formalDefinition="Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation." ) 2404 protected List<OperationDefinitionOverloadComponent> overload; 2405 2406 private static final long serialVersionUID = -1811668120L; 2407 2408 /** 2409 * Constructor 2410 */ 2411 public OperationDefinition() { 2412 super(); 2413 } 2414 2415 /** 2416 * Constructor 2417 */ 2418 public OperationDefinition(String name, PublicationStatus status, OperationKind kind, String code, boolean system, boolean type, boolean instance) { 2419 super(); 2420 this.setName(name); 2421 this.setStatus(status); 2422 this.setKind(kind); 2423 this.setCode(code); 2424 this.setSystem(system); 2425 this.setType(type); 2426 this.setInstance(instance); 2427 } 2428 2429 /** 2430 * @return {@link #url} (An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2431 */ 2432 public UriType getUrlElement() { 2433 if (this.url == null) 2434 if (Configuration.errorOnAutoCreate()) 2435 throw new Error("Attempt to auto-create OperationDefinition.url"); 2436 else if (Configuration.doAutoCreate()) 2437 this.url = new UriType(); // bb 2438 return this.url; 2439 } 2440 2441 public boolean hasUrlElement() { 2442 return this.url != null && !this.url.isEmpty(); 2443 } 2444 2445 public boolean hasUrl() { 2446 return this.url != null && !this.url.isEmpty(); 2447 } 2448 2449 /** 2450 * @param value {@link #url} (An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2451 */ 2452 public OperationDefinition setUrlElement(UriType value) { 2453 this.url = value; 2454 return this; 2455 } 2456 2457 /** 2458 * @return An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers. 2459 */ 2460 public String getUrl() { 2461 return this.url == null ? null : this.url.getValue(); 2462 } 2463 2464 /** 2465 * @param value An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers. 2466 */ 2467 public OperationDefinition setUrl(String value) { 2468 if (Utilities.noString(value)) 2469 this.url = null; 2470 else { 2471 if (this.url == null) 2472 this.url = new UriType(); 2473 this.url.setValue(value); 2474 } 2475 return this; 2476 } 2477 2478 /** 2479 * @return {@link #identifier} (A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2480 */ 2481 public List<Identifier> getIdentifier() { 2482 if (this.identifier == null) 2483 this.identifier = new ArrayList<Identifier>(); 2484 return this.identifier; 2485 } 2486 2487 /** 2488 * @return Returns a reference to <code>this</code> for easy method chaining 2489 */ 2490 public OperationDefinition setIdentifier(List<Identifier> theIdentifier) { 2491 this.identifier = theIdentifier; 2492 return this; 2493 } 2494 2495 public boolean hasIdentifier() { 2496 if (this.identifier == null) 2497 return false; 2498 for (Identifier item : this.identifier) 2499 if (!item.isEmpty()) 2500 return true; 2501 return false; 2502 } 2503 2504 public Identifier addIdentifier() { //3 2505 Identifier t = new Identifier(); 2506 if (this.identifier == null) 2507 this.identifier = new ArrayList<Identifier>(); 2508 this.identifier.add(t); 2509 return t; 2510 } 2511 2512 public OperationDefinition addIdentifier(Identifier t) { //3 2513 if (t == null) 2514 return this; 2515 if (this.identifier == null) 2516 this.identifier = new ArrayList<Identifier>(); 2517 this.identifier.add(t); 2518 return this; 2519 } 2520 2521 /** 2522 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2523 */ 2524 public Identifier getIdentifierFirstRep() { 2525 if (getIdentifier().isEmpty()) { 2526 addIdentifier(); 2527 } 2528 return getIdentifier().get(0); 2529 } 2530 2531 /** 2532 * @return {@link #version} (The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2533 */ 2534 public StringType getVersionElement() { 2535 if (this.version == null) 2536 if (Configuration.errorOnAutoCreate()) 2537 throw new Error("Attempt to auto-create OperationDefinition.version"); 2538 else if (Configuration.doAutoCreate()) 2539 this.version = new StringType(); // bb 2540 return this.version; 2541 } 2542 2543 public boolean hasVersionElement() { 2544 return this.version != null && !this.version.isEmpty(); 2545 } 2546 2547 public boolean hasVersion() { 2548 return this.version != null && !this.version.isEmpty(); 2549 } 2550 2551 /** 2552 * @param value {@link #version} (The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2553 */ 2554 public OperationDefinition setVersionElement(StringType value) { 2555 this.version = value; 2556 return this; 2557 } 2558 2559 /** 2560 * @return The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2561 */ 2562 public String getVersion() { 2563 return this.version == null ? null : this.version.getValue(); 2564 } 2565 2566 /** 2567 * @param value The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2568 */ 2569 public OperationDefinition setVersion(String value) { 2570 if (Utilities.noString(value)) 2571 this.version = null; 2572 else { 2573 if (this.version == null) 2574 this.version = new StringType(); 2575 this.version.setValue(value); 2576 } 2577 return this; 2578 } 2579 2580 /** 2581 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2582 */ 2583 public DataType getVersionAlgorithm() { 2584 return this.versionAlgorithm; 2585 } 2586 2587 /** 2588 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2589 */ 2590 public StringType getVersionAlgorithmStringType() throws FHIRException { 2591 if (this.versionAlgorithm == null) 2592 this.versionAlgorithm = new StringType(); 2593 if (!(this.versionAlgorithm instanceof StringType)) 2594 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2595 return (StringType) this.versionAlgorithm; 2596 } 2597 2598 public boolean hasVersionAlgorithmStringType() { 2599 return this.versionAlgorithm instanceof StringType; 2600 } 2601 2602 /** 2603 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2604 */ 2605 public Coding getVersionAlgorithmCoding() throws FHIRException { 2606 if (this.versionAlgorithm == null) 2607 this.versionAlgorithm = new Coding(); 2608 if (!(this.versionAlgorithm instanceof Coding)) 2609 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2610 return (Coding) this.versionAlgorithm; 2611 } 2612 2613 public boolean hasVersionAlgorithmCoding() { 2614 return this.versionAlgorithm instanceof Coding; 2615 } 2616 2617 public boolean hasVersionAlgorithm() { 2618 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2619 } 2620 2621 /** 2622 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2623 */ 2624 public OperationDefinition setVersionAlgorithm(DataType value) { 2625 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2626 throw new FHIRException("Not the right type for OperationDefinition.versionAlgorithm[x]: "+value.fhirType()); 2627 this.versionAlgorithm = value; 2628 return this; 2629 } 2630 2631 /** 2632 * @return {@link #name} (A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2633 */ 2634 public StringType getNameElement() { 2635 if (this.name == null) 2636 if (Configuration.errorOnAutoCreate()) 2637 throw new Error("Attempt to auto-create OperationDefinition.name"); 2638 else if (Configuration.doAutoCreate()) 2639 this.name = new StringType(); // bb 2640 return this.name; 2641 } 2642 2643 public boolean hasNameElement() { 2644 return this.name != null && !this.name.isEmpty(); 2645 } 2646 2647 public boolean hasName() { 2648 return this.name != null && !this.name.isEmpty(); 2649 } 2650 2651 /** 2652 * @param value {@link #name} (A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2653 */ 2654 public OperationDefinition setNameElement(StringType value) { 2655 this.name = value; 2656 return this; 2657 } 2658 2659 /** 2660 * @return A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2661 */ 2662 public String getName() { 2663 return this.name == null ? null : this.name.getValue(); 2664 } 2665 2666 /** 2667 * @param value A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2668 */ 2669 public OperationDefinition setName(String value) { 2670 if (this.name == null) 2671 this.name = new StringType(); 2672 this.name.setValue(value); 2673 return this; 2674 } 2675 2676 /** 2677 * @return {@link #title} (A short, descriptive, user-friendly title for the operation definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2678 */ 2679 public StringType getTitleElement() { 2680 if (this.title == null) 2681 if (Configuration.errorOnAutoCreate()) 2682 throw new Error("Attempt to auto-create OperationDefinition.title"); 2683 else if (Configuration.doAutoCreate()) 2684 this.title = new StringType(); // bb 2685 return this.title; 2686 } 2687 2688 public boolean hasTitleElement() { 2689 return this.title != null && !this.title.isEmpty(); 2690 } 2691 2692 public boolean hasTitle() { 2693 return this.title != null && !this.title.isEmpty(); 2694 } 2695 2696 /** 2697 * @param value {@link #title} (A short, descriptive, user-friendly title for the operation definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2698 */ 2699 public OperationDefinition setTitleElement(StringType value) { 2700 this.title = value; 2701 return this; 2702 } 2703 2704 /** 2705 * @return A short, descriptive, user-friendly title for the operation definition. 2706 */ 2707 public String getTitle() { 2708 return this.title == null ? null : this.title.getValue(); 2709 } 2710 2711 /** 2712 * @param value A short, descriptive, user-friendly title for the operation definition. 2713 */ 2714 public OperationDefinition setTitle(String value) { 2715 if (Utilities.noString(value)) 2716 this.title = null; 2717 else { 2718 if (this.title == null) 2719 this.title = new StringType(); 2720 this.title.setValue(value); 2721 } 2722 return this; 2723 } 2724 2725 /** 2726 * @return {@link #status} (The current state of this operation definition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2727 */ 2728 public Enumeration<PublicationStatus> getStatusElement() { 2729 if (this.status == null) 2730 if (Configuration.errorOnAutoCreate()) 2731 throw new Error("Attempt to auto-create OperationDefinition.status"); 2732 else if (Configuration.doAutoCreate()) 2733 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2734 return this.status; 2735 } 2736 2737 public boolean hasStatusElement() { 2738 return this.status != null && !this.status.isEmpty(); 2739 } 2740 2741 public boolean hasStatus() { 2742 return this.status != null && !this.status.isEmpty(); 2743 } 2744 2745 /** 2746 * @param value {@link #status} (The current state of this operation definition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2747 */ 2748 public OperationDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2749 this.status = value; 2750 return this; 2751 } 2752 2753 /** 2754 * @return The current state of this operation definition. 2755 */ 2756 public PublicationStatus getStatus() { 2757 return this.status == null ? null : this.status.getValue(); 2758 } 2759 2760 /** 2761 * @param value The current state of this operation definition. 2762 */ 2763 public OperationDefinition setStatus(PublicationStatus value) { 2764 if (this.status == null) 2765 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2766 this.status.setValue(value); 2767 return this; 2768 } 2769 2770 /** 2771 * @return {@link #kind} (Whether this is an operation or a named query.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2772 */ 2773 public Enumeration<OperationKind> getKindElement() { 2774 if (this.kind == null) 2775 if (Configuration.errorOnAutoCreate()) 2776 throw new Error("Attempt to auto-create OperationDefinition.kind"); 2777 else if (Configuration.doAutoCreate()) 2778 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); // bb 2779 return this.kind; 2780 } 2781 2782 public boolean hasKindElement() { 2783 return this.kind != null && !this.kind.isEmpty(); 2784 } 2785 2786 public boolean hasKind() { 2787 return this.kind != null && !this.kind.isEmpty(); 2788 } 2789 2790 /** 2791 * @param value {@link #kind} (Whether this is an operation or a named query.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2792 */ 2793 public OperationDefinition setKindElement(Enumeration<OperationKind> value) { 2794 this.kind = value; 2795 return this; 2796 } 2797 2798 /** 2799 * @return Whether this is an operation or a named query. 2800 */ 2801 public OperationKind getKind() { 2802 return this.kind == null ? null : this.kind.getValue(); 2803 } 2804 2805 /** 2806 * @param value Whether this is an operation or a named query. 2807 */ 2808 public OperationDefinition setKind(OperationKind value) { 2809 if (this.kind == null) 2810 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); 2811 this.kind.setValue(value); 2812 return this; 2813 } 2814 2815 /** 2816 * @return {@link #experimental} (A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2817 */ 2818 public BooleanType getExperimentalElement() { 2819 if (this.experimental == null) 2820 if (Configuration.errorOnAutoCreate()) 2821 throw new Error("Attempt to auto-create OperationDefinition.experimental"); 2822 else if (Configuration.doAutoCreate()) 2823 this.experimental = new BooleanType(); // bb 2824 return this.experimental; 2825 } 2826 2827 public boolean hasExperimentalElement() { 2828 return this.experimental != null && !this.experimental.isEmpty(); 2829 } 2830 2831 public boolean hasExperimental() { 2832 return this.experimental != null && !this.experimental.isEmpty(); 2833 } 2834 2835 /** 2836 * @param value {@link #experimental} (A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2837 */ 2838 public OperationDefinition setExperimentalElement(BooleanType value) { 2839 this.experimental = value; 2840 return this; 2841 } 2842 2843 /** 2844 * @return A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 2845 */ 2846 public boolean getExperimental() { 2847 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2848 } 2849 2850 /** 2851 * @param value A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 2852 */ 2853 public OperationDefinition setExperimental(boolean value) { 2854 if (this.experimental == null) 2855 this.experimental = new BooleanType(); 2856 this.experimental.setValue(value); 2857 return this; 2858 } 2859 2860 /** 2861 * @return {@link #date} (The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2862 */ 2863 public DateTimeType getDateElement() { 2864 if (this.date == null) 2865 if (Configuration.errorOnAutoCreate()) 2866 throw new Error("Attempt to auto-create OperationDefinition.date"); 2867 else if (Configuration.doAutoCreate()) 2868 this.date = new DateTimeType(); // bb 2869 return this.date; 2870 } 2871 2872 public boolean hasDateElement() { 2873 return this.date != null && !this.date.isEmpty(); 2874 } 2875 2876 public boolean hasDate() { 2877 return this.date != null && !this.date.isEmpty(); 2878 } 2879 2880 /** 2881 * @param value {@link #date} (The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2882 */ 2883 public OperationDefinition setDateElement(DateTimeType value) { 2884 this.date = value; 2885 return this; 2886 } 2887 2888 /** 2889 * @return The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes. 2890 */ 2891 public Date getDate() { 2892 return this.date == null ? null : this.date.getValue(); 2893 } 2894 2895 /** 2896 * @param value The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes. 2897 */ 2898 public OperationDefinition setDate(Date value) { 2899 if (value == null) 2900 this.date = null; 2901 else { 2902 if (this.date == null) 2903 this.date = new DateTimeType(); 2904 this.date.setValue(value); 2905 } 2906 return this; 2907 } 2908 2909 /** 2910 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2911 */ 2912 public StringType getPublisherElement() { 2913 if (this.publisher == null) 2914 if (Configuration.errorOnAutoCreate()) 2915 throw new Error("Attempt to auto-create OperationDefinition.publisher"); 2916 else if (Configuration.doAutoCreate()) 2917 this.publisher = new StringType(); // bb 2918 return this.publisher; 2919 } 2920 2921 public boolean hasPublisherElement() { 2922 return this.publisher != null && !this.publisher.isEmpty(); 2923 } 2924 2925 public boolean hasPublisher() { 2926 return this.publisher != null && !this.publisher.isEmpty(); 2927 } 2928 2929 /** 2930 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2931 */ 2932 public OperationDefinition setPublisherElement(StringType value) { 2933 this.publisher = value; 2934 return this; 2935 } 2936 2937 /** 2938 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition. 2939 */ 2940 public String getPublisher() { 2941 return this.publisher == null ? null : this.publisher.getValue(); 2942 } 2943 2944 /** 2945 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition. 2946 */ 2947 public OperationDefinition setPublisher(String value) { 2948 if (Utilities.noString(value)) 2949 this.publisher = null; 2950 else { 2951 if (this.publisher == null) 2952 this.publisher = new StringType(); 2953 this.publisher.setValue(value); 2954 } 2955 return this; 2956 } 2957 2958 /** 2959 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2960 */ 2961 public List<ContactDetail> getContact() { 2962 if (this.contact == null) 2963 this.contact = new ArrayList<ContactDetail>(); 2964 return this.contact; 2965 } 2966 2967 /** 2968 * @return Returns a reference to <code>this</code> for easy method chaining 2969 */ 2970 public OperationDefinition setContact(List<ContactDetail> theContact) { 2971 this.contact = theContact; 2972 return this; 2973 } 2974 2975 public boolean hasContact() { 2976 if (this.contact == null) 2977 return false; 2978 for (ContactDetail item : this.contact) 2979 if (!item.isEmpty()) 2980 return true; 2981 return false; 2982 } 2983 2984 public ContactDetail addContact() { //3 2985 ContactDetail t = new ContactDetail(); 2986 if (this.contact == null) 2987 this.contact = new ArrayList<ContactDetail>(); 2988 this.contact.add(t); 2989 return t; 2990 } 2991 2992 public OperationDefinition addContact(ContactDetail t) { //3 2993 if (t == null) 2994 return this; 2995 if (this.contact == null) 2996 this.contact = new ArrayList<ContactDetail>(); 2997 this.contact.add(t); 2998 return this; 2999 } 3000 3001 /** 3002 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3003 */ 3004 public ContactDetail getContactFirstRep() { 3005 if (getContact().isEmpty()) { 3006 addContact(); 3007 } 3008 return getContact().get(0); 3009 } 3010 3011 /** 3012 * @return {@link #description} (A free text natural language description of the operation definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3013 */ 3014 public MarkdownType getDescriptionElement() { 3015 if (this.description == null) 3016 if (Configuration.errorOnAutoCreate()) 3017 throw new Error("Attempt to auto-create OperationDefinition.description"); 3018 else if (Configuration.doAutoCreate()) 3019 this.description = new MarkdownType(); // bb 3020 return this.description; 3021 } 3022 3023 public boolean hasDescriptionElement() { 3024 return this.description != null && !this.description.isEmpty(); 3025 } 3026 3027 public boolean hasDescription() { 3028 return this.description != null && !this.description.isEmpty(); 3029 } 3030 3031 /** 3032 * @param value {@link #description} (A free text natural language description of the operation definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3033 */ 3034 public OperationDefinition setDescriptionElement(MarkdownType value) { 3035 this.description = value; 3036 return this; 3037 } 3038 3039 /** 3040 * @return A free text natural language description of the operation definition from a consumer's perspective. 3041 */ 3042 public String getDescription() { 3043 return this.description == null ? null : this.description.getValue(); 3044 } 3045 3046 /** 3047 * @param value A free text natural language description of the operation definition from a consumer's perspective. 3048 */ 3049 public OperationDefinition setDescription(String value) { 3050 if (Utilities.noString(value)) 3051 this.description = null; 3052 else { 3053 if (this.description == null) 3054 this.description = new MarkdownType(); 3055 this.description.setValue(value); 3056 } 3057 return this; 3058 } 3059 3060 /** 3061 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition.) 3062 */ 3063 public List<UsageContext> getUseContext() { 3064 if (this.useContext == null) 3065 this.useContext = new ArrayList<UsageContext>(); 3066 return this.useContext; 3067 } 3068 3069 /** 3070 * @return Returns a reference to <code>this</code> for easy method chaining 3071 */ 3072 public OperationDefinition setUseContext(List<UsageContext> theUseContext) { 3073 this.useContext = theUseContext; 3074 return this; 3075 } 3076 3077 public boolean hasUseContext() { 3078 if (this.useContext == null) 3079 return false; 3080 for (UsageContext item : this.useContext) 3081 if (!item.isEmpty()) 3082 return true; 3083 return false; 3084 } 3085 3086 public UsageContext addUseContext() { //3 3087 UsageContext t = new UsageContext(); 3088 if (this.useContext == null) 3089 this.useContext = new ArrayList<UsageContext>(); 3090 this.useContext.add(t); 3091 return t; 3092 } 3093 3094 public OperationDefinition addUseContext(UsageContext t) { //3 3095 if (t == null) 3096 return this; 3097 if (this.useContext == null) 3098 this.useContext = new ArrayList<UsageContext>(); 3099 this.useContext.add(t); 3100 return this; 3101 } 3102 3103 /** 3104 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3105 */ 3106 public UsageContext getUseContextFirstRep() { 3107 if (getUseContext().isEmpty()) { 3108 addUseContext(); 3109 } 3110 return getUseContext().get(0); 3111 } 3112 3113 /** 3114 * @return {@link #jurisdiction} (A legal or geographic region in which the operation definition is intended to be used.) 3115 */ 3116 public List<CodeableConcept> getJurisdiction() { 3117 if (this.jurisdiction == null) 3118 this.jurisdiction = new ArrayList<CodeableConcept>(); 3119 return this.jurisdiction; 3120 } 3121 3122 /** 3123 * @return Returns a reference to <code>this</code> for easy method chaining 3124 */ 3125 public OperationDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 3126 this.jurisdiction = theJurisdiction; 3127 return this; 3128 } 3129 3130 public boolean hasJurisdiction() { 3131 if (this.jurisdiction == null) 3132 return false; 3133 for (CodeableConcept item : this.jurisdiction) 3134 if (!item.isEmpty()) 3135 return true; 3136 return false; 3137 } 3138 3139 public CodeableConcept addJurisdiction() { //3 3140 CodeableConcept t = new CodeableConcept(); 3141 if (this.jurisdiction == null) 3142 this.jurisdiction = new ArrayList<CodeableConcept>(); 3143 this.jurisdiction.add(t); 3144 return t; 3145 } 3146 3147 public OperationDefinition addJurisdiction(CodeableConcept t) { //3 3148 if (t == null) 3149 return this; 3150 if (this.jurisdiction == null) 3151 this.jurisdiction = new ArrayList<CodeableConcept>(); 3152 this.jurisdiction.add(t); 3153 return this; 3154 } 3155 3156 /** 3157 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 3158 */ 3159 public CodeableConcept getJurisdictionFirstRep() { 3160 if (getJurisdiction().isEmpty()) { 3161 addJurisdiction(); 3162 } 3163 return getJurisdiction().get(0); 3164 } 3165 3166 /** 3167 * @return {@link #purpose} (Explanation of why this operation definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3168 */ 3169 public MarkdownType getPurposeElement() { 3170 if (this.purpose == null) 3171 if (Configuration.errorOnAutoCreate()) 3172 throw new Error("Attempt to auto-create OperationDefinition.purpose"); 3173 else if (Configuration.doAutoCreate()) 3174 this.purpose = new MarkdownType(); // bb 3175 return this.purpose; 3176 } 3177 3178 public boolean hasPurposeElement() { 3179 return this.purpose != null && !this.purpose.isEmpty(); 3180 } 3181 3182 public boolean hasPurpose() { 3183 return this.purpose != null && !this.purpose.isEmpty(); 3184 } 3185 3186 /** 3187 * @param value {@link #purpose} (Explanation of why this operation definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3188 */ 3189 public OperationDefinition setPurposeElement(MarkdownType value) { 3190 this.purpose = value; 3191 return this; 3192 } 3193 3194 /** 3195 * @return Explanation of why this operation definition is needed and why it has been designed as it has. 3196 */ 3197 public String getPurpose() { 3198 return this.purpose == null ? null : this.purpose.getValue(); 3199 } 3200 3201 /** 3202 * @param value Explanation of why this operation definition is needed and why it has been designed as it has. 3203 */ 3204 public OperationDefinition setPurpose(String value) { 3205 if (Utilities.noString(value)) 3206 this.purpose = null; 3207 else { 3208 if (this.purpose == null) 3209 this.purpose = new MarkdownType(); 3210 this.purpose.setValue(value); 3211 } 3212 return this; 3213 } 3214 3215 /** 3216 * @return {@link #copyright} (A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3217 */ 3218 public MarkdownType getCopyrightElement() { 3219 if (this.copyright == null) 3220 if (Configuration.errorOnAutoCreate()) 3221 throw new Error("Attempt to auto-create OperationDefinition.copyright"); 3222 else if (Configuration.doAutoCreate()) 3223 this.copyright = new MarkdownType(); // bb 3224 return this.copyright; 3225 } 3226 3227 public boolean hasCopyrightElement() { 3228 return this.copyright != null && !this.copyright.isEmpty(); 3229 } 3230 3231 public boolean hasCopyright() { 3232 return this.copyright != null && !this.copyright.isEmpty(); 3233 } 3234 3235 /** 3236 * @param value {@link #copyright} (A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3237 */ 3238 public OperationDefinition setCopyrightElement(MarkdownType value) { 3239 this.copyright = value; 3240 return this; 3241 } 3242 3243 /** 3244 * @return A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition. 3245 */ 3246 public String getCopyright() { 3247 return this.copyright == null ? null : this.copyright.getValue(); 3248 } 3249 3250 /** 3251 * @param value A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition. 3252 */ 3253 public OperationDefinition setCopyright(String value) { 3254 if (Utilities.noString(value)) 3255 this.copyright = null; 3256 else { 3257 if (this.copyright == null) 3258 this.copyright = new MarkdownType(); 3259 this.copyright.setValue(value); 3260 } 3261 return this; 3262 } 3263 3264 /** 3265 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3266 */ 3267 public StringType getCopyrightLabelElement() { 3268 if (this.copyrightLabel == null) 3269 if (Configuration.errorOnAutoCreate()) 3270 throw new Error("Attempt to auto-create OperationDefinition.copyrightLabel"); 3271 else if (Configuration.doAutoCreate()) 3272 this.copyrightLabel = new StringType(); // bb 3273 return this.copyrightLabel; 3274 } 3275 3276 public boolean hasCopyrightLabelElement() { 3277 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3278 } 3279 3280 public boolean hasCopyrightLabel() { 3281 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3282 } 3283 3284 /** 3285 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3286 */ 3287 public OperationDefinition setCopyrightLabelElement(StringType value) { 3288 this.copyrightLabel = value; 3289 return this; 3290 } 3291 3292 /** 3293 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3294 */ 3295 public String getCopyrightLabel() { 3296 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 3297 } 3298 3299 /** 3300 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3301 */ 3302 public OperationDefinition setCopyrightLabel(String value) { 3303 if (Utilities.noString(value)) 3304 this.copyrightLabel = null; 3305 else { 3306 if (this.copyrightLabel == null) 3307 this.copyrightLabel = new StringType(); 3308 this.copyrightLabel.setValue(value); 3309 } 3310 return this; 3311 } 3312 3313 /** 3314 * @return {@link #affectsState} (Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.). This is the underlying object with id, value and extensions. The accessor "getAffectsState" gives direct access to the value 3315 */ 3316 public BooleanType getAffectsStateElement() { 3317 if (this.affectsState == null) 3318 if (Configuration.errorOnAutoCreate()) 3319 throw new Error("Attempt to auto-create OperationDefinition.affectsState"); 3320 else if (Configuration.doAutoCreate()) 3321 this.affectsState = new BooleanType(); // bb 3322 return this.affectsState; 3323 } 3324 3325 public boolean hasAffectsStateElement() { 3326 return this.affectsState != null && !this.affectsState.isEmpty(); 3327 } 3328 3329 public boolean hasAffectsState() { 3330 return this.affectsState != null && !this.affectsState.isEmpty(); 3331 } 3332 3333 /** 3334 * @param value {@link #affectsState} (Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.). This is the underlying object with id, value and extensions. The accessor "getAffectsState" gives direct access to the value 3335 */ 3336 public OperationDefinition setAffectsStateElement(BooleanType value) { 3337 this.affectsState = value; 3338 return this; 3339 } 3340 3341 /** 3342 * @return Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'. 3343 */ 3344 public boolean getAffectsState() { 3345 return this.affectsState == null || this.affectsState.isEmpty() ? false : this.affectsState.getValue(); 3346 } 3347 3348 /** 3349 * @param value Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'. 3350 */ 3351 public OperationDefinition setAffectsState(boolean value) { 3352 if (this.affectsState == null) 3353 this.affectsState = new BooleanType(); 3354 this.affectsState.setValue(value); 3355 return this; 3356 } 3357 3358 /** 3359 * @return {@link #code} (The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3360 */ 3361 public CodeType getCodeElement() { 3362 if (this.code == null) 3363 if (Configuration.errorOnAutoCreate()) 3364 throw new Error("Attempt to auto-create OperationDefinition.code"); 3365 else if (Configuration.doAutoCreate()) 3366 this.code = new CodeType(); // bb 3367 return this.code; 3368 } 3369 3370 public boolean hasCodeElement() { 3371 return this.code != null && !this.code.isEmpty(); 3372 } 3373 3374 public boolean hasCode() { 3375 return this.code != null && !this.code.isEmpty(); 3376 } 3377 3378 /** 3379 * @param value {@link #code} (The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3380 */ 3381 public OperationDefinition setCodeElement(CodeType value) { 3382 this.code = value; 3383 return this; 3384 } 3385 3386 /** 3387 * @return The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code. 3388 */ 3389 public String getCode() { 3390 return this.code == null ? null : this.code.getValue(); 3391 } 3392 3393 /** 3394 * @param value The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code. 3395 */ 3396 public OperationDefinition setCode(String value) { 3397 if (this.code == null) 3398 this.code = new CodeType(); 3399 this.code.setValue(value); 3400 return this; 3401 } 3402 3403 /** 3404 * @return {@link #comment} (Additional information about how to use this operation or named query.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 3405 */ 3406 public MarkdownType getCommentElement() { 3407 if (this.comment == null) 3408 if (Configuration.errorOnAutoCreate()) 3409 throw new Error("Attempt to auto-create OperationDefinition.comment"); 3410 else if (Configuration.doAutoCreate()) 3411 this.comment = new MarkdownType(); // bb 3412 return this.comment; 3413 } 3414 3415 public boolean hasCommentElement() { 3416 return this.comment != null && !this.comment.isEmpty(); 3417 } 3418 3419 public boolean hasComment() { 3420 return this.comment != null && !this.comment.isEmpty(); 3421 } 3422 3423 /** 3424 * @param value {@link #comment} (Additional information about how to use this operation or named query.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 3425 */ 3426 public OperationDefinition setCommentElement(MarkdownType value) { 3427 this.comment = value; 3428 return this; 3429 } 3430 3431 /** 3432 * @return Additional information about how to use this operation or named query. 3433 */ 3434 public String getComment() { 3435 return this.comment == null ? null : this.comment.getValue(); 3436 } 3437 3438 /** 3439 * @param value Additional information about how to use this operation or named query. 3440 */ 3441 public OperationDefinition setComment(String value) { 3442 if (Utilities.noString(value)) 3443 this.comment = null; 3444 else { 3445 if (this.comment == null) 3446 this.comment = new MarkdownType(); 3447 this.comment.setValue(value); 3448 } 3449 return this; 3450 } 3451 3452 /** 3453 * @return {@link #base} (Indicates that this operation definition is a constraining profile on the base.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 3454 */ 3455 public CanonicalType getBaseElement() { 3456 if (this.base == null) 3457 if (Configuration.errorOnAutoCreate()) 3458 throw new Error("Attempt to auto-create OperationDefinition.base"); 3459 else if (Configuration.doAutoCreate()) 3460 this.base = new CanonicalType(); // bb 3461 return this.base; 3462 } 3463 3464 public boolean hasBaseElement() { 3465 return this.base != null && !this.base.isEmpty(); 3466 } 3467 3468 public boolean hasBase() { 3469 return this.base != null && !this.base.isEmpty(); 3470 } 3471 3472 /** 3473 * @param value {@link #base} (Indicates that this operation definition is a constraining profile on the base.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 3474 */ 3475 public OperationDefinition setBaseElement(CanonicalType value) { 3476 this.base = value; 3477 return this; 3478 } 3479 3480 /** 3481 * @return Indicates that this operation definition is a constraining profile on the base. 3482 */ 3483 public String getBase() { 3484 return this.base == null ? null : this.base.getValue(); 3485 } 3486 3487 /** 3488 * @param value Indicates that this operation definition is a constraining profile on the base. 3489 */ 3490 public OperationDefinition setBase(String value) { 3491 if (Utilities.noString(value)) 3492 this.base = null; 3493 else { 3494 if (this.base == null) 3495 this.base = new CanonicalType(); 3496 this.base.setValue(value); 3497 } 3498 return this; 3499 } 3500 3501 /** 3502 * @return {@link #resource} (The types on which this operation can be executed.) 3503 */ 3504 public List<Enumeration<VersionIndependentResourceTypesAll>> getResource() { 3505 if (this.resource == null) 3506 this.resource = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 3507 return this.resource; 3508 } 3509 3510 /** 3511 * @return Returns a reference to <code>this</code> for easy method chaining 3512 */ 3513 public OperationDefinition setResource(List<Enumeration<VersionIndependentResourceTypesAll>> theResource) { 3514 this.resource = theResource; 3515 return this; 3516 } 3517 3518 public boolean hasResource() { 3519 if (this.resource == null) 3520 return false; 3521 for (Enumeration<VersionIndependentResourceTypesAll> item : this.resource) 3522 if (!item.isEmpty()) 3523 return true; 3524 return false; 3525 } 3526 3527 /** 3528 * @return {@link #resource} (The types on which this operation can be executed.) 3529 */ 3530 public Enumeration<VersionIndependentResourceTypesAll> addResourceElement() {//2 3531 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 3532 if (this.resource == null) 3533 this.resource = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 3534 this.resource.add(t); 3535 return t; 3536 } 3537 3538 /** 3539 * @param value {@link #resource} (The types on which this operation can be executed.) 3540 */ 3541 public OperationDefinition addResource(VersionIndependentResourceTypesAll value) { //1 3542 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 3543 t.setValue(value); 3544 if (this.resource == null) 3545 this.resource = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 3546 this.resource.add(t); 3547 return this; 3548 } 3549 3550 /** 3551 * @param value {@link #resource} (The types on which this operation can be executed.) 3552 */ 3553 public boolean hasResource(VersionIndependentResourceTypesAll value) { 3554 if (this.resource == null) 3555 return false; 3556 for (Enumeration<VersionIndependentResourceTypesAll> v : this.resource) 3557 if (v.getValue().equals(value)) // code 3558 return true; 3559 return false; 3560 } 3561 3562 /** 3563 * @return {@link #system} (Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 3564 */ 3565 public BooleanType getSystemElement() { 3566 if (this.system == null) 3567 if (Configuration.errorOnAutoCreate()) 3568 throw new Error("Attempt to auto-create OperationDefinition.system"); 3569 else if (Configuration.doAutoCreate()) 3570 this.system = new BooleanType(); // bb 3571 return this.system; 3572 } 3573 3574 public boolean hasSystemElement() { 3575 return this.system != null && !this.system.isEmpty(); 3576 } 3577 3578 public boolean hasSystem() { 3579 return this.system != null && !this.system.isEmpty(); 3580 } 3581 3582 /** 3583 * @param value {@link #system} (Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 3584 */ 3585 public OperationDefinition setSystemElement(BooleanType value) { 3586 this.system = value; 3587 return this; 3588 } 3589 3590 /** 3591 * @return Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context). 3592 */ 3593 public boolean getSystem() { 3594 return this.system == null || this.system.isEmpty() ? false : this.system.getValue(); 3595 } 3596 3597 /** 3598 * @param value Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context). 3599 */ 3600 public OperationDefinition setSystem(boolean value) { 3601 if (this.system == null) 3602 this.system = new BooleanType(); 3603 this.system.setValue(value); 3604 return this; 3605 } 3606 3607 /** 3608 * @return {@link #type} (Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3609 */ 3610 public BooleanType getTypeElement() { 3611 if (this.type == null) 3612 if (Configuration.errorOnAutoCreate()) 3613 throw new Error("Attempt to auto-create OperationDefinition.type"); 3614 else if (Configuration.doAutoCreate()) 3615 this.type = new BooleanType(); // bb 3616 return this.type; 3617 } 3618 3619 public boolean hasTypeElement() { 3620 return this.type != null && !this.type.isEmpty(); 3621 } 3622 3623 public boolean hasType() { 3624 return this.type != null && !this.type.isEmpty(); 3625 } 3626 3627 /** 3628 * @param value {@link #type} (Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3629 */ 3630 public OperationDefinition setTypeElement(BooleanType value) { 3631 this.type = value; 3632 return this; 3633 } 3634 3635 /** 3636 * @return Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context). 3637 */ 3638 public boolean getType() { 3639 return this.type == null || this.type.isEmpty() ? false : this.type.getValue(); 3640 } 3641 3642 /** 3643 * @param value Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context). 3644 */ 3645 public OperationDefinition setType(boolean value) { 3646 if (this.type == null) 3647 this.type = new BooleanType(); 3648 this.type.setValue(value); 3649 return this; 3650 } 3651 3652 /** 3653 * @return {@link #instance} (Indicates whether this operation can be invoked on a particular instance of one of the given types.). This is the underlying object with id, value and extensions. The accessor "getInstance" gives direct access to the value 3654 */ 3655 public BooleanType getInstanceElement() { 3656 if (this.instance == null) 3657 if (Configuration.errorOnAutoCreate()) 3658 throw new Error("Attempt to auto-create OperationDefinition.instance"); 3659 else if (Configuration.doAutoCreate()) 3660 this.instance = new BooleanType(); // bb 3661 return this.instance; 3662 } 3663 3664 public boolean hasInstanceElement() { 3665 return this.instance != null && !this.instance.isEmpty(); 3666 } 3667 3668 public boolean hasInstance() { 3669 return this.instance != null && !this.instance.isEmpty(); 3670 } 3671 3672 /** 3673 * @param value {@link #instance} (Indicates whether this operation can be invoked on a particular instance of one of the given types.). This is the underlying object with id, value and extensions. The accessor "getInstance" gives direct access to the value 3674 */ 3675 public OperationDefinition setInstanceElement(BooleanType value) { 3676 this.instance = value; 3677 return this; 3678 } 3679 3680 /** 3681 * @return Indicates whether this operation can be invoked on a particular instance of one of the given types. 3682 */ 3683 public boolean getInstance() { 3684 return this.instance == null || this.instance.isEmpty() ? false : this.instance.getValue(); 3685 } 3686 3687 /** 3688 * @param value Indicates whether this operation can be invoked on a particular instance of one of the given types. 3689 */ 3690 public OperationDefinition setInstance(boolean value) { 3691 if (this.instance == null) 3692 this.instance = new BooleanType(); 3693 this.instance.setValue(value); 3694 return this; 3695 } 3696 3697 /** 3698 * @return {@link #inputProfile} (Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.). This is the underlying object with id, value and extensions. The accessor "getInputProfile" gives direct access to the value 3699 */ 3700 public CanonicalType getInputProfileElement() { 3701 if (this.inputProfile == null) 3702 if (Configuration.errorOnAutoCreate()) 3703 throw new Error("Attempt to auto-create OperationDefinition.inputProfile"); 3704 else if (Configuration.doAutoCreate()) 3705 this.inputProfile = new CanonicalType(); // bb 3706 return this.inputProfile; 3707 } 3708 3709 public boolean hasInputProfileElement() { 3710 return this.inputProfile != null && !this.inputProfile.isEmpty(); 3711 } 3712 3713 public boolean hasInputProfile() { 3714 return this.inputProfile != null && !this.inputProfile.isEmpty(); 3715 } 3716 3717 /** 3718 * @param value {@link #inputProfile} (Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.). This is the underlying object with id, value and extensions. The accessor "getInputProfile" gives direct access to the value 3719 */ 3720 public OperationDefinition setInputProfileElement(CanonicalType value) { 3721 this.inputProfile = value; 3722 return this; 3723 } 3724 3725 /** 3726 * @return Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole. 3727 */ 3728 public String getInputProfile() { 3729 return this.inputProfile == null ? null : this.inputProfile.getValue(); 3730 } 3731 3732 /** 3733 * @param value Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole. 3734 */ 3735 public OperationDefinition setInputProfile(String value) { 3736 if (Utilities.noString(value)) 3737 this.inputProfile = null; 3738 else { 3739 if (this.inputProfile == null) 3740 this.inputProfile = new CanonicalType(); 3741 this.inputProfile.setValue(value); 3742 } 3743 return this; 3744 } 3745 3746 /** 3747 * @return {@link #outputProfile} (Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.). This is the underlying object with id, value and extensions. The accessor "getOutputProfile" gives direct access to the value 3748 */ 3749 public CanonicalType getOutputProfileElement() { 3750 if (this.outputProfile == null) 3751 if (Configuration.errorOnAutoCreate()) 3752 throw new Error("Attempt to auto-create OperationDefinition.outputProfile"); 3753 else if (Configuration.doAutoCreate()) 3754 this.outputProfile = new CanonicalType(); // bb 3755 return this.outputProfile; 3756 } 3757 3758 public boolean hasOutputProfileElement() { 3759 return this.outputProfile != null && !this.outputProfile.isEmpty(); 3760 } 3761 3762 public boolean hasOutputProfile() { 3763 return this.outputProfile != null && !this.outputProfile.isEmpty(); 3764 } 3765 3766 /** 3767 * @param value {@link #outputProfile} (Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.). This is the underlying object with id, value and extensions. The accessor "getOutputProfile" gives direct access to the value 3768 */ 3769 public OperationDefinition setOutputProfileElement(CanonicalType value) { 3770 this.outputProfile = value; 3771 return this; 3772 } 3773 3774 /** 3775 * @return Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource. 3776 */ 3777 public String getOutputProfile() { 3778 return this.outputProfile == null ? null : this.outputProfile.getValue(); 3779 } 3780 3781 /** 3782 * @param value Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource. 3783 */ 3784 public OperationDefinition setOutputProfile(String value) { 3785 if (Utilities.noString(value)) 3786 this.outputProfile = null; 3787 else { 3788 if (this.outputProfile == null) 3789 this.outputProfile = new CanonicalType(); 3790 this.outputProfile.setValue(value); 3791 } 3792 return this; 3793 } 3794 3795 /** 3796 * @return {@link #parameter} (The parameters for the operation/query.) 3797 */ 3798 public List<OperationDefinitionParameterComponent> getParameter() { 3799 if (this.parameter == null) 3800 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3801 return this.parameter; 3802 } 3803 3804 /** 3805 * @return Returns a reference to <code>this</code> for easy method chaining 3806 */ 3807 public OperationDefinition setParameter(List<OperationDefinitionParameterComponent> theParameter) { 3808 this.parameter = theParameter; 3809 return this; 3810 } 3811 3812 public boolean hasParameter() { 3813 if (this.parameter == null) 3814 return false; 3815 for (OperationDefinitionParameterComponent item : this.parameter) 3816 if (!item.isEmpty()) 3817 return true; 3818 return false; 3819 } 3820 3821 public OperationDefinitionParameterComponent addParameter() { //3 3822 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 3823 if (this.parameter == null) 3824 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3825 this.parameter.add(t); 3826 return t; 3827 } 3828 3829 public OperationDefinition addParameter(OperationDefinitionParameterComponent t) { //3 3830 if (t == null) 3831 return this; 3832 if (this.parameter == null) 3833 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3834 this.parameter.add(t); 3835 return this; 3836 } 3837 3838 /** 3839 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 3840 */ 3841 public OperationDefinitionParameterComponent getParameterFirstRep() { 3842 if (getParameter().isEmpty()) { 3843 addParameter(); 3844 } 3845 return getParameter().get(0); 3846 } 3847 3848 /** 3849 * @return {@link #overload} (Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.) 3850 */ 3851 public List<OperationDefinitionOverloadComponent> getOverload() { 3852 if (this.overload == null) 3853 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3854 return this.overload; 3855 } 3856 3857 /** 3858 * @return Returns a reference to <code>this</code> for easy method chaining 3859 */ 3860 public OperationDefinition setOverload(List<OperationDefinitionOverloadComponent> theOverload) { 3861 this.overload = theOverload; 3862 return this; 3863 } 3864 3865 public boolean hasOverload() { 3866 if (this.overload == null) 3867 return false; 3868 for (OperationDefinitionOverloadComponent item : this.overload) 3869 if (!item.isEmpty()) 3870 return true; 3871 return false; 3872 } 3873 3874 public OperationDefinitionOverloadComponent addOverload() { //3 3875 OperationDefinitionOverloadComponent t = new OperationDefinitionOverloadComponent(); 3876 if (this.overload == null) 3877 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3878 this.overload.add(t); 3879 return t; 3880 } 3881 3882 public OperationDefinition addOverload(OperationDefinitionOverloadComponent t) { //3 3883 if (t == null) 3884 return this; 3885 if (this.overload == null) 3886 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3887 this.overload.add(t); 3888 return this; 3889 } 3890 3891 /** 3892 * @return The first repetition of repeating field {@link #overload}, creating it if it does not already exist {3} 3893 */ 3894 public OperationDefinitionOverloadComponent getOverloadFirstRep() { 3895 if (getOverload().isEmpty()) { 3896 addOverload(); 3897 } 3898 return getOverload().get(0); 3899 } 3900 3901 protected void listChildren(List<Property> children) { 3902 super.listChildren(children); 3903 children.add(new Property("url", "uri", "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.", 0, 1, url)); 3904 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3905 children.add(new Property("version", "string", "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3906 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3907 children.add(new Property("name", "string", "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3908 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the operation definition.", 0, 1, title)); 3909 children.add(new Property("status", "code", "The current state of this operation definition.", 0, 1, status)); 3910 children.add(new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind)); 3911 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.", 0, 1, experimental)); 3912 children.add(new Property("date", "dateTime", "The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 0, 1, date)); 3913 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition.", 0, 1, publisher)); 3914 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3915 children.add(new Property("description", "markdown", "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, description)); 3916 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3917 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the operation definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3918 children.add(new Property("purpose", "markdown", "Explanation of why this operation definition is needed and why it has been designed as it has.", 0, 1, purpose)); 3919 children.add(new Property("copyright", "markdown", "A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition.", 0, 1, copyright)); 3920 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3921 children.add(new Property("affectsState", "boolean", "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.", 0, 1, affectsState)); 3922 children.add(new Property("code", "code", "The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code.", 0, 1, code)); 3923 children.add(new Property("comment", "markdown", "Additional information about how to use this operation or named query.", 0, 1, comment)); 3924 children.add(new Property("base", "canonical(OperationDefinition)", "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base)); 3925 children.add(new Property("resource", "code", "The types on which this operation can be executed.", 0, java.lang.Integer.MAX_VALUE, resource)); 3926 children.add(new Property("system", "boolean", "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 0, 1, system)); 3927 children.add(new Property("type", "boolean", "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 0, 1, type)); 3928 children.add(new Property("instance", "boolean", "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, instance)); 3929 children.add(new Property("inputProfile", "canonical(StructureDefinition)", "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.", 0, 1, inputProfile)); 3930 children.add(new Property("outputProfile", "canonical(StructureDefinition)", "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.", 0, 1, outputProfile)); 3931 children.add(new Property("parameter", "", "The parameters for the operation/query.", 0, java.lang.Integer.MAX_VALUE, parameter)); 3932 children.add(new Property("overload", "", "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 0, java.lang.Integer.MAX_VALUE, overload)); 3933 } 3934 3935 @Override 3936 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3937 switch (_hash) { 3938 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.", 0, 1, url); 3939 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3940 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3941 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3942 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3943 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3944 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3945 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3946 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the operation definition.", 0, 1, title); 3947 case -892481550: /*status*/ return new Property("status", "code", "The current state of this operation definition.", 0, 1, status); 3948 case 3292052: /*kind*/ return new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind); 3949 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.", 0, 1, experimental); 3950 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the operation definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 0, 1, date); 3951 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the operation definition.", 0, 1, publisher); 3952 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3953 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, description); 3954 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition.", 0, java.lang.Integer.MAX_VALUE, useContext); 3955 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the operation definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3956 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this operation definition is needed and why it has been designed as it has.", 0, 1, purpose); 3957 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the operation definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the operation definition.", 0, 1, copyright); 3958 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3959 case -14805197: /*affectsState*/ return new Property("affectsState", "boolean", "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.", 0, 1, affectsState); 3960 case 3059181: /*code*/ return new Property("code", "code", "The label that is recommended to be used in the URL for this operation. In some cases, servers may need to use a different CapabilityStatement operation.name to differentiate between multiple SearchParameters that happen to have the same code.", 0, 1, code); 3961 case 950398559: /*comment*/ return new Property("comment", "markdown", "Additional information about how to use this operation or named query.", 0, 1, comment); 3962 case 3016401: /*base*/ return new Property("base", "canonical(OperationDefinition)", "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base); 3963 case -341064690: /*resource*/ return new Property("resource", "code", "The types on which this operation can be executed.", 0, java.lang.Integer.MAX_VALUE, resource); 3964 case -887328209: /*system*/ return new Property("system", "boolean", "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 0, 1, system); 3965 case 3575610: /*type*/ return new Property("type", "boolean", "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 0, 1, type); 3966 case 555127957: /*instance*/ return new Property("instance", "boolean", "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, instance); 3967 case 676942463: /*inputProfile*/ return new Property("inputProfile", "canonical(StructureDefinition)", "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.", 0, 1, inputProfile); 3968 case 1826166120: /*outputProfile*/ return new Property("outputProfile", "canonical(StructureDefinition)", "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.", 0, 1, outputProfile); 3969 case 1954460585: /*parameter*/ return new Property("parameter", "", "The parameters for the operation/query.", 0, java.lang.Integer.MAX_VALUE, parameter); 3970 case 529823674: /*overload*/ return new Property("overload", "", "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 0, java.lang.Integer.MAX_VALUE, overload); 3971 default: return super.getNamedProperty(_hash, _name, _checkValid); 3972 } 3973 3974 } 3975 3976 @Override 3977 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3978 switch (hash) { 3979 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3980 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3981 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3982 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3983 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3984 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3985 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3986 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<OperationKind> 3987 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3988 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3989 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3990 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3991 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3992 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3993 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3994 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3995 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3996 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3997 case -14805197: /*affectsState*/ return this.affectsState == null ? new Base[0] : new Base[] {this.affectsState}; // BooleanType 3998 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 3999 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 4000 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // CanonicalType 4001 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // Enumeration<VersionIndependentResourceTypesAll> 4002 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // BooleanType 4003 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // BooleanType 4004 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // BooleanType 4005 case 676942463: /*inputProfile*/ return this.inputProfile == null ? new Base[0] : new Base[] {this.inputProfile}; // CanonicalType 4006 case 1826166120: /*outputProfile*/ return this.outputProfile == null ? new Base[0] : new Base[] {this.outputProfile}; // CanonicalType 4007 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // OperationDefinitionParameterComponent 4008 case 529823674: /*overload*/ return this.overload == null ? new Base[0] : this.overload.toArray(new Base[this.overload.size()]); // OperationDefinitionOverloadComponent 4009 default: return super.getProperty(hash, name, checkValid); 4010 } 4011 4012 } 4013 4014 @Override 4015 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4016 switch (hash) { 4017 case 116079: // url 4018 this.url = TypeConvertor.castToUri(value); // UriType 4019 return value; 4020 case -1618432855: // identifier 4021 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4022 return value; 4023 case 351608024: // version 4024 this.version = TypeConvertor.castToString(value); // StringType 4025 return value; 4026 case 1508158071: // versionAlgorithm 4027 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4028 return value; 4029 case 3373707: // name 4030 this.name = TypeConvertor.castToString(value); // StringType 4031 return value; 4032 case 110371416: // title 4033 this.title = TypeConvertor.castToString(value); // StringType 4034 return value; 4035 case -892481550: // status 4036 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4037 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4038 return value; 4039 case 3292052: // kind 4040 value = new OperationKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4041 this.kind = (Enumeration) value; // Enumeration<OperationKind> 4042 return value; 4043 case -404562712: // experimental 4044 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4045 return value; 4046 case 3076014: // date 4047 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4048 return value; 4049 case 1447404028: // publisher 4050 this.publisher = TypeConvertor.castToString(value); // StringType 4051 return value; 4052 case 951526432: // contact 4053 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4054 return value; 4055 case -1724546052: // description 4056 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4057 return value; 4058 case -669707736: // useContext 4059 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4060 return value; 4061 case -507075711: // jurisdiction 4062 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4063 return value; 4064 case -220463842: // purpose 4065 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4066 return value; 4067 case 1522889671: // copyright 4068 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4069 return value; 4070 case 765157229: // copyrightLabel 4071 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4072 return value; 4073 case -14805197: // affectsState 4074 this.affectsState = TypeConvertor.castToBoolean(value); // BooleanType 4075 return value; 4076 case 3059181: // code 4077 this.code = TypeConvertor.castToCode(value); // CodeType 4078 return value; 4079 case 950398559: // comment 4080 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 4081 return value; 4082 case 3016401: // base 4083 this.base = TypeConvertor.castToCanonical(value); // CanonicalType 4084 return value; 4085 case -341064690: // resource 4086 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 4087 this.getResource().add((Enumeration) value); // Enumeration<VersionIndependentResourceTypesAll> 4088 return value; 4089 case -887328209: // system 4090 this.system = TypeConvertor.castToBoolean(value); // BooleanType 4091 return value; 4092 case 3575610: // type 4093 this.type = TypeConvertor.castToBoolean(value); // BooleanType 4094 return value; 4095 case 555127957: // instance 4096 this.instance = TypeConvertor.castToBoolean(value); // BooleanType 4097 return value; 4098 case 676942463: // inputProfile 4099 this.inputProfile = TypeConvertor.castToCanonical(value); // CanonicalType 4100 return value; 4101 case 1826166120: // outputProfile 4102 this.outputProfile = TypeConvertor.castToCanonical(value); // CanonicalType 4103 return value; 4104 case 1954460585: // parameter 4105 this.getParameter().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 4106 return value; 4107 case 529823674: // overload 4108 this.getOverload().add((OperationDefinitionOverloadComponent) value); // OperationDefinitionOverloadComponent 4109 return value; 4110 default: return super.setProperty(hash, name, value); 4111 } 4112 4113 } 4114 4115 @Override 4116 public Base setProperty(String name, Base value) throws FHIRException { 4117 if (name.equals("url")) { 4118 this.url = TypeConvertor.castToUri(value); // UriType 4119 } else if (name.equals("identifier")) { 4120 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4121 } else if (name.equals("version")) { 4122 this.version = TypeConvertor.castToString(value); // StringType 4123 } else if (name.equals("versionAlgorithm[x]")) { 4124 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4125 } else if (name.equals("name")) { 4126 this.name = TypeConvertor.castToString(value); // StringType 4127 } else if (name.equals("title")) { 4128 this.title = TypeConvertor.castToString(value); // StringType 4129 } else if (name.equals("status")) { 4130 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4131 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4132 } else if (name.equals("kind")) { 4133 value = new OperationKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4134 this.kind = (Enumeration) value; // Enumeration<OperationKind> 4135 } else if (name.equals("experimental")) { 4136 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4137 } else if (name.equals("date")) { 4138 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4139 } else if (name.equals("publisher")) { 4140 this.publisher = TypeConvertor.castToString(value); // StringType 4141 } else if (name.equals("contact")) { 4142 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4143 } else if (name.equals("description")) { 4144 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4145 } else if (name.equals("useContext")) { 4146 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4147 } else if (name.equals("jurisdiction")) { 4148 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4149 } else if (name.equals("purpose")) { 4150 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4151 } else if (name.equals("copyright")) { 4152 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4153 } else if (name.equals("copyrightLabel")) { 4154 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4155 } else if (name.equals("affectsState")) { 4156 this.affectsState = TypeConvertor.castToBoolean(value); // BooleanType 4157 } else if (name.equals("code")) { 4158 this.code = TypeConvertor.castToCode(value); // CodeType 4159 } else if (name.equals("comment")) { 4160 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 4161 } else if (name.equals("base")) { 4162 this.base = TypeConvertor.castToCanonical(value); // CanonicalType 4163 } else if (name.equals("resource")) { 4164 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 4165 this.getResource().add((Enumeration) value); 4166 } else if (name.equals("system")) { 4167 this.system = TypeConvertor.castToBoolean(value); // BooleanType 4168 } else if (name.equals("type")) { 4169 this.type = TypeConvertor.castToBoolean(value); // BooleanType 4170 } else if (name.equals("instance")) { 4171 this.instance = TypeConvertor.castToBoolean(value); // BooleanType 4172 } else if (name.equals("inputProfile")) { 4173 this.inputProfile = TypeConvertor.castToCanonical(value); // CanonicalType 4174 } else if (name.equals("outputProfile")) { 4175 this.outputProfile = TypeConvertor.castToCanonical(value); // CanonicalType 4176 } else if (name.equals("parameter")) { 4177 this.getParameter().add((OperationDefinitionParameterComponent) value); 4178 } else if (name.equals("overload")) { 4179 this.getOverload().add((OperationDefinitionOverloadComponent) value); 4180 } else 4181 return super.setProperty(name, value); 4182 return value; 4183 } 4184 4185 @Override 4186 public void removeChild(String name, Base value) throws FHIRException { 4187 if (name.equals("url")) { 4188 this.url = null; 4189 } else if (name.equals("identifier")) { 4190 this.getIdentifier().remove(value); 4191 } else if (name.equals("version")) { 4192 this.version = null; 4193 } else if (name.equals("versionAlgorithm[x]")) { 4194 this.versionAlgorithm = null; 4195 } else if (name.equals("name")) { 4196 this.name = null; 4197 } else if (name.equals("title")) { 4198 this.title = null; 4199 } else if (name.equals("status")) { 4200 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4201 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4202 } else if (name.equals("kind")) { 4203 value = new OperationKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4204 this.kind = (Enumeration) value; // Enumeration<OperationKind> 4205 } else if (name.equals("experimental")) { 4206 this.experimental = null; 4207 } else if (name.equals("date")) { 4208 this.date = null; 4209 } else if (name.equals("publisher")) { 4210 this.publisher = null; 4211 } else if (name.equals("contact")) { 4212 this.getContact().remove(value); 4213 } else if (name.equals("description")) { 4214 this.description = null; 4215 } else if (name.equals("useContext")) { 4216 this.getUseContext().remove(value); 4217 } else if (name.equals("jurisdiction")) { 4218 this.getJurisdiction().remove(value); 4219 } else if (name.equals("purpose")) { 4220 this.purpose = null; 4221 } else if (name.equals("copyright")) { 4222 this.copyright = null; 4223 } else if (name.equals("copyrightLabel")) { 4224 this.copyrightLabel = null; 4225 } else if (name.equals("affectsState")) { 4226 this.affectsState = null; 4227 } else if (name.equals("code")) { 4228 this.code = null; 4229 } else if (name.equals("comment")) { 4230 this.comment = null; 4231 } else if (name.equals("base")) { 4232 this.base = null; 4233 } else if (name.equals("resource")) { 4234 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 4235 this.getResource().remove((Enumeration) value); 4236 } else if (name.equals("system")) { 4237 this.system = null; 4238 } else if (name.equals("type")) { 4239 this.type = null; 4240 } else if (name.equals("instance")) { 4241 this.instance = null; 4242 } else if (name.equals("inputProfile")) { 4243 this.inputProfile = null; 4244 } else if (name.equals("outputProfile")) { 4245 this.outputProfile = null; 4246 } else if (name.equals("parameter")) { 4247 this.getParameter().remove((OperationDefinitionParameterComponent) value); 4248 } else if (name.equals("overload")) { 4249 this.getOverload().remove((OperationDefinitionOverloadComponent) value); 4250 } else 4251 super.removeChild(name, value); 4252 4253 } 4254 4255 @Override 4256 public Base makeProperty(int hash, String name) throws FHIRException { 4257 switch (hash) { 4258 case 116079: return getUrlElement(); 4259 case -1618432855: return addIdentifier(); 4260 case 351608024: return getVersionElement(); 4261 case -115699031: return getVersionAlgorithm(); 4262 case 1508158071: return getVersionAlgorithm(); 4263 case 3373707: return getNameElement(); 4264 case 110371416: return getTitleElement(); 4265 case -892481550: return getStatusElement(); 4266 case 3292052: return getKindElement(); 4267 case -404562712: return getExperimentalElement(); 4268 case 3076014: return getDateElement(); 4269 case 1447404028: return getPublisherElement(); 4270 case 951526432: return addContact(); 4271 case -1724546052: return getDescriptionElement(); 4272 case -669707736: return addUseContext(); 4273 case -507075711: return addJurisdiction(); 4274 case -220463842: return getPurposeElement(); 4275 case 1522889671: return getCopyrightElement(); 4276 case 765157229: return getCopyrightLabelElement(); 4277 case -14805197: return getAffectsStateElement(); 4278 case 3059181: return getCodeElement(); 4279 case 950398559: return getCommentElement(); 4280 case 3016401: return getBaseElement(); 4281 case -341064690: return addResourceElement(); 4282 case -887328209: return getSystemElement(); 4283 case 3575610: return getTypeElement(); 4284 case 555127957: return getInstanceElement(); 4285 case 676942463: return getInputProfileElement(); 4286 case 1826166120: return getOutputProfileElement(); 4287 case 1954460585: return addParameter(); 4288 case 529823674: return addOverload(); 4289 default: return super.makeProperty(hash, name); 4290 } 4291 4292 } 4293 4294 @Override 4295 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4296 switch (hash) { 4297 case 116079: /*url*/ return new String[] {"uri"}; 4298 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4299 case 351608024: /*version*/ return new String[] {"string"}; 4300 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 4301 case 3373707: /*name*/ return new String[] {"string"}; 4302 case 110371416: /*title*/ return new String[] {"string"}; 4303 case -892481550: /*status*/ return new String[] {"code"}; 4304 case 3292052: /*kind*/ return new String[] {"code"}; 4305 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4306 case 3076014: /*date*/ return new String[] {"dateTime"}; 4307 case 1447404028: /*publisher*/ return new String[] {"string"}; 4308 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4309 case -1724546052: /*description*/ return new String[] {"markdown"}; 4310 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4311 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4312 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4313 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4314 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 4315 case -14805197: /*affectsState*/ return new String[] {"boolean"}; 4316 case 3059181: /*code*/ return new String[] {"code"}; 4317 case 950398559: /*comment*/ return new String[] {"markdown"}; 4318 case 3016401: /*base*/ return new String[] {"canonical"}; 4319 case -341064690: /*resource*/ return new String[] {"code"}; 4320 case -887328209: /*system*/ return new String[] {"boolean"}; 4321 case 3575610: /*type*/ return new String[] {"boolean"}; 4322 case 555127957: /*instance*/ return new String[] {"boolean"}; 4323 case 676942463: /*inputProfile*/ return new String[] {"canonical"}; 4324 case 1826166120: /*outputProfile*/ return new String[] {"canonical"}; 4325 case 1954460585: /*parameter*/ return new String[] {}; 4326 case 529823674: /*overload*/ return new String[] {}; 4327 default: return super.getTypesForProperty(hash, name); 4328 } 4329 4330 } 4331 4332 @Override 4333 public Base addChild(String name) throws FHIRException { 4334 if (name.equals("url")) { 4335 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.url"); 4336 } 4337 else if (name.equals("identifier")) { 4338 return addIdentifier(); 4339 } 4340 else if (name.equals("version")) { 4341 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.version"); 4342 } 4343 else if (name.equals("versionAlgorithmString")) { 4344 this.versionAlgorithm = new StringType(); 4345 return this.versionAlgorithm; 4346 } 4347 else if (name.equals("versionAlgorithmCoding")) { 4348 this.versionAlgorithm = new Coding(); 4349 return this.versionAlgorithm; 4350 } 4351 else if (name.equals("name")) { 4352 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 4353 } 4354 else if (name.equals("title")) { 4355 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.title"); 4356 } 4357 else if (name.equals("status")) { 4358 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.status"); 4359 } 4360 else if (name.equals("kind")) { 4361 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.kind"); 4362 } 4363 else if (name.equals("experimental")) { 4364 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.experimental"); 4365 } 4366 else if (name.equals("date")) { 4367 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.date"); 4368 } 4369 else if (name.equals("publisher")) { 4370 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.publisher"); 4371 } 4372 else if (name.equals("contact")) { 4373 return addContact(); 4374 } 4375 else if (name.equals("description")) { 4376 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.description"); 4377 } 4378 else if (name.equals("useContext")) { 4379 return addUseContext(); 4380 } 4381 else if (name.equals("jurisdiction")) { 4382 return addJurisdiction(); 4383 } 4384 else if (name.equals("purpose")) { 4385 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.purpose"); 4386 } 4387 else if (name.equals("copyright")) { 4388 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.copyright"); 4389 } 4390 else if (name.equals("copyrightLabel")) { 4391 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.copyrightLabel"); 4392 } 4393 else if (name.equals("affectsState")) { 4394 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.affectsState"); 4395 } 4396 else if (name.equals("code")) { 4397 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.code"); 4398 } 4399 else if (name.equals("comment")) { 4400 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.comment"); 4401 } 4402 else if (name.equals("base")) { 4403 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.base"); 4404 } 4405 else if (name.equals("resource")) { 4406 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.resource"); 4407 } 4408 else if (name.equals("system")) { 4409 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.system"); 4410 } 4411 else if (name.equals("type")) { 4412 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 4413 } 4414 else if (name.equals("instance")) { 4415 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.instance"); 4416 } 4417 else if (name.equals("inputProfile")) { 4418 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.inputProfile"); 4419 } 4420 else if (name.equals("outputProfile")) { 4421 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.outputProfile"); 4422 } 4423 else if (name.equals("parameter")) { 4424 return addParameter(); 4425 } 4426 else if (name.equals("overload")) { 4427 return addOverload(); 4428 } 4429 else 4430 return super.addChild(name); 4431 } 4432 4433 public String fhirType() { 4434 return "OperationDefinition"; 4435 4436 } 4437 4438 public OperationDefinition copy() { 4439 OperationDefinition dst = new OperationDefinition(); 4440 copyValues(dst); 4441 return dst; 4442 } 4443 4444 public void copyValues(OperationDefinition dst) { 4445 super.copyValues(dst); 4446 dst.url = url == null ? null : url.copy(); 4447 if (identifier != null) { 4448 dst.identifier = new ArrayList<Identifier>(); 4449 for (Identifier i : identifier) 4450 dst.identifier.add(i.copy()); 4451 }; 4452 dst.version = version == null ? null : version.copy(); 4453 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4454 dst.name = name == null ? null : name.copy(); 4455 dst.title = title == null ? null : title.copy(); 4456 dst.status = status == null ? null : status.copy(); 4457 dst.kind = kind == null ? null : kind.copy(); 4458 dst.experimental = experimental == null ? null : experimental.copy(); 4459 dst.date = date == null ? null : date.copy(); 4460 dst.publisher = publisher == null ? null : publisher.copy(); 4461 if (contact != null) { 4462 dst.contact = new ArrayList<ContactDetail>(); 4463 for (ContactDetail i : contact) 4464 dst.contact.add(i.copy()); 4465 }; 4466 dst.description = description == null ? null : description.copy(); 4467 if (useContext != null) { 4468 dst.useContext = new ArrayList<UsageContext>(); 4469 for (UsageContext i : useContext) 4470 dst.useContext.add(i.copy()); 4471 }; 4472 if (jurisdiction != null) { 4473 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4474 for (CodeableConcept i : jurisdiction) 4475 dst.jurisdiction.add(i.copy()); 4476 }; 4477 dst.purpose = purpose == null ? null : purpose.copy(); 4478 dst.copyright = copyright == null ? null : copyright.copy(); 4479 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4480 dst.affectsState = affectsState == null ? null : affectsState.copy(); 4481 dst.code = code == null ? null : code.copy(); 4482 dst.comment = comment == null ? null : comment.copy(); 4483 dst.base = base == null ? null : base.copy(); 4484 if (resource != null) { 4485 dst.resource = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 4486 for (Enumeration<VersionIndependentResourceTypesAll> i : resource) 4487 dst.resource.add(i.copy()); 4488 }; 4489 dst.system = system == null ? null : system.copy(); 4490 dst.type = type == null ? null : type.copy(); 4491 dst.instance = instance == null ? null : instance.copy(); 4492 dst.inputProfile = inputProfile == null ? null : inputProfile.copy(); 4493 dst.outputProfile = outputProfile == null ? null : outputProfile.copy(); 4494 if (parameter != null) { 4495 dst.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 4496 for (OperationDefinitionParameterComponent i : parameter) 4497 dst.parameter.add(i.copy()); 4498 }; 4499 if (overload != null) { 4500 dst.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 4501 for (OperationDefinitionOverloadComponent i : overload) 4502 dst.overload.add(i.copy()); 4503 }; 4504 } 4505 4506 protected OperationDefinition typedCopy() { 4507 return copy(); 4508 } 4509 4510 @Override 4511 public boolean equalsDeep(Base other_) { 4512 if (!super.equalsDeep(other_)) 4513 return false; 4514 if (!(other_ instanceof OperationDefinition)) 4515 return false; 4516 OperationDefinition o = (OperationDefinition) other_; 4517 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4518 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4519 && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) && compareDeep(experimental, o.experimental, true) 4520 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 4521 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 4522 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 4523 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(affectsState, o.affectsState, true) 4524 && compareDeep(code, o.code, true) && compareDeep(comment, o.comment, true) && compareDeep(base, o.base, true) 4525 && compareDeep(resource, o.resource, true) && compareDeep(system, o.system, true) && compareDeep(type, o.type, true) 4526 && compareDeep(instance, o.instance, true) && compareDeep(inputProfile, o.inputProfile, true) && compareDeep(outputProfile, o.outputProfile, true) 4527 && compareDeep(parameter, o.parameter, true) && compareDeep(overload, o.overload, true); 4528 } 4529 4530 @Override 4531 public boolean equalsShallow(Base other_) { 4532 if (!super.equalsShallow(other_)) 4533 return false; 4534 if (!(other_ instanceof OperationDefinition)) 4535 return false; 4536 OperationDefinition o = (OperationDefinition) other_; 4537 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4538 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(kind, o.kind, true) 4539 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 4540 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 4541 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(affectsState, o.affectsState, true) 4542 && compareValues(code, o.code, true) && compareValues(comment, o.comment, true) && compareValues(base, o.base, true) 4543 && compareValues(resource, o.resource, true) && compareValues(system, o.system, true) && compareValues(type, o.type, true) 4544 && compareValues(instance, o.instance, true) && compareValues(inputProfile, o.inputProfile, true) && compareValues(outputProfile, o.outputProfile, true) 4545 ; 4546 } 4547 4548 public boolean isEmpty() { 4549 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4550 , versionAlgorithm, name, title, status, kind, experimental, date, publisher 4551 , contact, description, useContext, jurisdiction, purpose, copyright, copyrightLabel 4552 , affectsState, code, comment, base, resource, system, type, instance, inputProfile 4553 , outputProfile, parameter, overload); 4554 } 4555 4556 @Override 4557 public ResourceType getResourceType() { 4558 return ResourceType.OperationDefinition; 4559 } 4560 4561 /** 4562 * Search parameter: <b>context-quantity</b> 4563 * <p> 4564 * Description: <b>Multiple Resources: 4565 4566* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4567* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4568* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4569* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4570* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4571* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4572* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4573* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4574* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4575* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4576* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4577* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4578* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4579* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4580* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4581* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4582* [Library](library.html): A quantity- or range-valued use context assigned to the library 4583* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4584* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4585* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4586* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4587* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4588* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4589* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4590* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4591* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4592* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4593* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4594* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4595* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4596</b><br> 4597 * Type: <b>quantity</b><br> 4598 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4599 * </p> 4600 */ 4601 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 4602 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4603 /** 4604 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4605 * <p> 4606 * Description: <b>Multiple Resources: 4607 4608* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4609* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4610* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4611* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4612* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4613* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4614* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4615* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4616* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4617* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4618* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4619* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4620* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4621* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4622* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4623* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4624* [Library](library.html): A quantity- or range-valued use context assigned to the library 4625* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4626* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4627* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4628* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4629* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4630* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4631* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4632* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4633* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4634* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4635* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4636* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4637* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4638</b><br> 4639 * Type: <b>quantity</b><br> 4640 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4641 * </p> 4642 */ 4643 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 4644 4645 /** 4646 * Search parameter: <b>context-type-quantity</b> 4647 * <p> 4648 * Description: <b>Multiple Resources: 4649 4650* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4651* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4652* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4653* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4654* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4655* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4656* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4657* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4658* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4659* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4660* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4661* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4662* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4663* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4664* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4665* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4666* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4667* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4668* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4669* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4670* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4671* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4672* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4673* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4674* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4675* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4676* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4677* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4678* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4679* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4680</b><br> 4681 * Type: <b>composite</b><br> 4682 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4683 * </p> 4684 */ 4685 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 4686 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4687 /** 4688 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 4689 * <p> 4690 * Description: <b>Multiple Resources: 4691 4692* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4693* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4694* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4695* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4696* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4697* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4698* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4699* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4700* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4701* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4702* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4703* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4704* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4705* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4706* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4707* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4708* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4709* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4710* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4711* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4712* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4713* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4714* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4715* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4716* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4717* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4718* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4719* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4720* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4721* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4722</b><br> 4723 * Type: <b>composite</b><br> 4724 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4725 * </p> 4726 */ 4727 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 4728 4729 /** 4730 * Search parameter: <b>context-type-value</b> 4731 * <p> 4732 * Description: <b>Multiple Resources: 4733 4734* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4735* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4736* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4737* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4738* [Citation](citation.html): A use context type and value assigned to the citation 4739* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4740* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4741* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4742* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4743* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4744* [Evidence](evidence.html): A use context type and value assigned to the evidence 4745* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4746* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4747* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4748* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4749* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4750* [Library](library.html): A use context type and value assigned to the library 4751* [Measure](measure.html): A use context type and value assigned to the measure 4752* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4753* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4754* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4755* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4756* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4757* [Requirements](requirements.html): A use context type and value assigned to the requirements 4758* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4759* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4760* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4761* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4762* [TestScript](testscript.html): A use context type and value assigned to the test script 4763* [ValueSet](valueset.html): A use context type and value assigned to the value set 4764</b><br> 4765 * Type: <b>composite</b><br> 4766 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4767 * </p> 4768 */ 4769 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 4770 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4771 /** 4772 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4773 * <p> 4774 * Description: <b>Multiple Resources: 4775 4776* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4777* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4778* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4779* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4780* [Citation](citation.html): A use context type and value assigned to the citation 4781* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4782* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4783* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4784* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4785* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4786* [Evidence](evidence.html): A use context type and value assigned to the evidence 4787* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4788* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4789* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4790* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4791* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4792* [Library](library.html): A use context type and value assigned to the library 4793* [Measure](measure.html): A use context type and value assigned to the measure 4794* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4795* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4796* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4797* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4798* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4799* [Requirements](requirements.html): A use context type and value assigned to the requirements 4800* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4801* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4802* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4803* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4804* [TestScript](testscript.html): A use context type and value assigned to the test script 4805* [ValueSet](valueset.html): A use context type and value assigned to the value set 4806</b><br> 4807 * Type: <b>composite</b><br> 4808 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4809 * </p> 4810 */ 4811 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 4812 4813 /** 4814 * Search parameter: <b>context-type</b> 4815 * <p> 4816 * Description: <b>Multiple Resources: 4817 4818* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4819* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4820* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4821* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4822* [Citation](citation.html): A type of use context assigned to the citation 4823* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4824* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4825* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4826* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4827* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4828* [Evidence](evidence.html): A type of use context assigned to the evidence 4829* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4830* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4831* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4832* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4833* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4834* [Library](library.html): A type of use context assigned to the library 4835* [Measure](measure.html): A type of use context assigned to the measure 4836* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4837* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4838* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4839* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4840* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4841* [Requirements](requirements.html): A type of use context assigned to the requirements 4842* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4843* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4844* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4845* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4846* [TestScript](testscript.html): A type of use context assigned to the test script 4847* [ValueSet](valueset.html): A type of use context assigned to the value set 4848</b><br> 4849 * Type: <b>token</b><br> 4850 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4851 * </p> 4852 */ 4853 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 4854 public static final String SP_CONTEXT_TYPE = "context-type"; 4855 /** 4856 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4857 * <p> 4858 * Description: <b>Multiple Resources: 4859 4860* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4861* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4862* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4863* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4864* [Citation](citation.html): A type of use context assigned to the citation 4865* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4866* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4867* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4868* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4869* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4870* [Evidence](evidence.html): A type of use context assigned to the evidence 4871* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4872* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4873* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4874* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4875* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4876* [Library](library.html): A type of use context assigned to the library 4877* [Measure](measure.html): A type of use context assigned to the measure 4878* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4879* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4880* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4881* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4882* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4883* [Requirements](requirements.html): A type of use context assigned to the requirements 4884* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4885* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4886* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4887* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4888* [TestScript](testscript.html): A type of use context assigned to the test script 4889* [ValueSet](valueset.html): A type of use context assigned to the value set 4890</b><br> 4891 * Type: <b>token</b><br> 4892 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4893 * </p> 4894 */ 4895 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 4896 4897 /** 4898 * Search parameter: <b>context</b> 4899 * <p> 4900 * Description: <b>Multiple Resources: 4901 4902* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4903* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4904* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4905* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4906* [Citation](citation.html): A use context assigned to the citation 4907* [CodeSystem](codesystem.html): A use context assigned to the code system 4908* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4909* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4910* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4911* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4912* [Evidence](evidence.html): A use context assigned to the evidence 4913* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4914* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4915* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4916* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4917* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4918* [Library](library.html): A use context assigned to the library 4919* [Measure](measure.html): A use context assigned to the measure 4920* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4921* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4922* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4923* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4924* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4925* [Requirements](requirements.html): A use context assigned to the requirements 4926* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4927* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4928* [StructureMap](structuremap.html): A use context assigned to the structure map 4929* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4930* [TestScript](testscript.html): A use context assigned to the test script 4931* [ValueSet](valueset.html): A use context assigned to the value set 4932</b><br> 4933 * Type: <b>token</b><br> 4934 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4935 * </p> 4936 */ 4937 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 4938 public static final String SP_CONTEXT = "context"; 4939 /** 4940 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4941 * <p> 4942 * Description: <b>Multiple Resources: 4943 4944* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4945* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4946* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4947* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4948* [Citation](citation.html): A use context assigned to the citation 4949* [CodeSystem](codesystem.html): A use context assigned to the code system 4950* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4951* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4952* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4953* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4954* [Evidence](evidence.html): A use context assigned to the evidence 4955* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4956* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4957* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4958* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4959* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4960* [Library](library.html): A use context assigned to the library 4961* [Measure](measure.html): A use context assigned to the measure 4962* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4963* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4964* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4965* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4966* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4967* [Requirements](requirements.html): A use context assigned to the requirements 4968* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4969* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4970* [StructureMap](structuremap.html): A use context assigned to the structure map 4971* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4972* [TestScript](testscript.html): A use context assigned to the test script 4973* [ValueSet](valueset.html): A use context assigned to the value set 4974</b><br> 4975 * Type: <b>token</b><br> 4976 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4977 * </p> 4978 */ 4979 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4980 4981 /** 4982 * Search parameter: <b>date</b> 4983 * <p> 4984 * Description: <b>Multiple Resources: 4985 4986* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4987* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4988* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4989* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4990* [Citation](citation.html): The citation publication date 4991* [CodeSystem](codesystem.html): The code system publication date 4992* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4993* [ConceptMap](conceptmap.html): The concept map publication date 4994* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4995* [EventDefinition](eventdefinition.html): The event definition publication date 4996* [Evidence](evidence.html): The evidence publication date 4997* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4998* [ExampleScenario](examplescenario.html): The example scenario publication date 4999* [GraphDefinition](graphdefinition.html): The graph definition publication date 5000* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5001* [Library](library.html): The library publication date 5002* [Measure](measure.html): The measure publication date 5003* [MessageDefinition](messagedefinition.html): The message definition publication date 5004* [NamingSystem](namingsystem.html): The naming system publication date 5005* [OperationDefinition](operationdefinition.html): The operation definition publication date 5006* [PlanDefinition](plandefinition.html): The plan definition publication date 5007* [Questionnaire](questionnaire.html): The questionnaire publication date 5008* [Requirements](requirements.html): The requirements publication date 5009* [SearchParameter](searchparameter.html): The search parameter publication date 5010* [StructureDefinition](structuredefinition.html): The structure definition publication date 5011* [StructureMap](structuremap.html): The structure map publication date 5012* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5013* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5014* [TestScript](testscript.html): The test script publication date 5015* [ValueSet](valueset.html): The value set publication date 5016</b><br> 5017 * Type: <b>date</b><br> 5018 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5019 * </p> 5020 */ 5021 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 5022 public static final String SP_DATE = "date"; 5023 /** 5024 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5025 * <p> 5026 * Description: <b>Multiple Resources: 5027 5028* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5029* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5030* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5031* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5032* [Citation](citation.html): The citation publication date 5033* [CodeSystem](codesystem.html): The code system publication date 5034* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5035* [ConceptMap](conceptmap.html): The concept map publication date 5036* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5037* [EventDefinition](eventdefinition.html): The event definition publication date 5038* [Evidence](evidence.html): The evidence publication date 5039* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5040* [ExampleScenario](examplescenario.html): The example scenario publication date 5041* [GraphDefinition](graphdefinition.html): The graph definition publication date 5042* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5043* [Library](library.html): The library publication date 5044* [Measure](measure.html): The measure publication date 5045* [MessageDefinition](messagedefinition.html): The message definition publication date 5046* [NamingSystem](namingsystem.html): The naming system publication date 5047* [OperationDefinition](operationdefinition.html): The operation definition publication date 5048* [PlanDefinition](plandefinition.html): The plan definition publication date 5049* [Questionnaire](questionnaire.html): The questionnaire publication date 5050* [Requirements](requirements.html): The requirements publication date 5051* [SearchParameter](searchparameter.html): The search parameter publication date 5052* [StructureDefinition](structuredefinition.html): The structure definition publication date 5053* [StructureMap](structuremap.html): The structure map publication date 5054* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5055* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5056* [TestScript](testscript.html): The test script publication date 5057* [ValueSet](valueset.html): The value set publication date 5058</b><br> 5059 * Type: <b>date</b><br> 5060 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5061 * </p> 5062 */ 5063 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5064 5065 /** 5066 * Search parameter: <b>description</b> 5067 * <p> 5068 * Description: <b>Multiple Resources: 5069 5070* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5071* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5072* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5073* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5074* [Citation](citation.html): The description of the citation 5075* [CodeSystem](codesystem.html): The description of the code system 5076* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5077* [ConceptMap](conceptmap.html): The description of the concept map 5078* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5079* [EventDefinition](eventdefinition.html): The description of the event definition 5080* [Evidence](evidence.html): The description of the evidence 5081* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5082* [GraphDefinition](graphdefinition.html): The description of the graph definition 5083* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5084* [Library](library.html): The description of the library 5085* [Measure](measure.html): The description of the measure 5086* [MessageDefinition](messagedefinition.html): The description of the message definition 5087* [NamingSystem](namingsystem.html): The description of the naming system 5088* [OperationDefinition](operationdefinition.html): The description of the operation definition 5089* [PlanDefinition](plandefinition.html): The description of the plan definition 5090* [Questionnaire](questionnaire.html): The description of the questionnaire 5091* [Requirements](requirements.html): The description of the requirements 5092* [SearchParameter](searchparameter.html): The description of the search parameter 5093* [StructureDefinition](structuredefinition.html): The description of the structure definition 5094* [StructureMap](structuremap.html): The description of the structure map 5095* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5096* [TestScript](testscript.html): The description of the test script 5097* [ValueSet](valueset.html): The description of the value set 5098</b><br> 5099 * Type: <b>string</b><br> 5100 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5101 * </p> 5102 */ 5103 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 5104 public static final String SP_DESCRIPTION = "description"; 5105 /** 5106 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5107 * <p> 5108 * Description: <b>Multiple Resources: 5109 5110* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5111* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5112* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5113* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5114* [Citation](citation.html): The description of the citation 5115* [CodeSystem](codesystem.html): The description of the code system 5116* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5117* [ConceptMap](conceptmap.html): The description of the concept map 5118* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5119* [EventDefinition](eventdefinition.html): The description of the event definition 5120* [Evidence](evidence.html): The description of the evidence 5121* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5122* [GraphDefinition](graphdefinition.html): The description of the graph definition 5123* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5124* [Library](library.html): The description of the library 5125* [Measure](measure.html): The description of the measure 5126* [MessageDefinition](messagedefinition.html): The description of the message definition 5127* [NamingSystem](namingsystem.html): The description of the naming system 5128* [OperationDefinition](operationdefinition.html): The description of the operation definition 5129* [PlanDefinition](plandefinition.html): The description of the plan definition 5130* [Questionnaire](questionnaire.html): The description of the questionnaire 5131* [Requirements](requirements.html): The description of the requirements 5132* [SearchParameter](searchparameter.html): The description of the search parameter 5133* [StructureDefinition](structuredefinition.html): The description of the structure definition 5134* [StructureMap](structuremap.html): The description of the structure map 5135* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5136* [TestScript](testscript.html): The description of the test script 5137* [ValueSet](valueset.html): The description of the value set 5138</b><br> 5139 * Type: <b>string</b><br> 5140 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5141 * </p> 5142 */ 5143 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5144 5145 /** 5146 * Search parameter: <b>identifier</b> 5147 * <p> 5148 * Description: <b>Multiple Resources: 5149 5150* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5151* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5152* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5153* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5154* [Citation](citation.html): External identifier for the citation 5155* [CodeSystem](codesystem.html): External identifier for the code system 5156* [ConceptMap](conceptmap.html): External identifier for the concept map 5157* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5158* [EventDefinition](eventdefinition.html): External identifier for the event definition 5159* [Evidence](evidence.html): External identifier for the evidence 5160* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5161* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5162* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5163* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5164* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5165* [Library](library.html): External identifier for the library 5166* [Measure](measure.html): External identifier for the measure 5167* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5168* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5169* [NamingSystem](namingsystem.html): External identifier for the naming system 5170* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 5171* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 5172* [PlanDefinition](plandefinition.html): External identifier for the plan definition 5173* [Questionnaire](questionnaire.html): External identifier for the questionnaire 5174* [Requirements](requirements.html): External identifier for the requirements 5175* [SearchParameter](searchparameter.html): External identifier for the search parameter 5176* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5177* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5178* [StructureMap](structuremap.html): External identifier for the structure map 5179* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5180* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5181* [TestPlan](testplan.html): An identifier for the test plan 5182* [TestScript](testscript.html): External identifier for the test script 5183* [ValueSet](valueset.html): External identifier for the value set 5184</b><br> 5185 * Type: <b>token</b><br> 5186 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5187 * </p> 5188 */ 5189 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 5190 public static final String SP_IDENTIFIER = "identifier"; 5191 /** 5192 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5193 * <p> 5194 * Description: <b>Multiple Resources: 5195 5196* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5197* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5198* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5199* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5200* [Citation](citation.html): External identifier for the citation 5201* [CodeSystem](codesystem.html): External identifier for the code system 5202* [ConceptMap](conceptmap.html): External identifier for the concept map 5203* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5204* [EventDefinition](eventdefinition.html): External identifier for the event definition 5205* [Evidence](evidence.html): External identifier for the evidence 5206* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5207* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5208* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5209* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5210* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5211* [Library](library.html): External identifier for the library 5212* [Measure](measure.html): External identifier for the measure 5213* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5214* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5215* [NamingSystem](namingsystem.html): External identifier for the naming system 5216* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 5217* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 5218* [PlanDefinition](plandefinition.html): External identifier for the plan definition 5219* [Questionnaire](questionnaire.html): External identifier for the questionnaire 5220* [Requirements](requirements.html): External identifier for the requirements 5221* [SearchParameter](searchparameter.html): External identifier for the search parameter 5222* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5223* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5224* [StructureMap](structuremap.html): External identifier for the structure map 5225* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5226* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5227* [TestPlan](testplan.html): An identifier for the test plan 5228* [TestScript](testscript.html): External identifier for the test script 5229* [ValueSet](valueset.html): External identifier for the value set 5230</b><br> 5231 * Type: <b>token</b><br> 5232 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5233 * </p> 5234 */ 5235 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5236 5237 /** 5238 * Search parameter: <b>jurisdiction</b> 5239 * <p> 5240 * Description: <b>Multiple Resources: 5241 5242* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 5243* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 5244* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 5245* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 5246* [Citation](citation.html): Intended jurisdiction for the citation 5247* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 5248* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 5249* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 5250* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 5251* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 5252* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 5253* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 5254* [Library](library.html): Intended jurisdiction for the library 5255* [Measure](measure.html): Intended jurisdiction for the measure 5256* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 5257* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 5258* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 5259* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 5260* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 5261* [Requirements](requirements.html): Intended jurisdiction for the requirements 5262* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 5263* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 5264* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 5265* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 5266* [TestScript](testscript.html): Intended jurisdiction for the test script 5267* [ValueSet](valueset.html): Intended jurisdiction for the value set 5268</b><br> 5269 * Type: <b>token</b><br> 5270 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 5271 * </p> 5272 */ 5273 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 5274 public static final String SP_JURISDICTION = "jurisdiction"; 5275 /** 5276 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5277 * <p> 5278 * Description: <b>Multiple Resources: 5279 5280* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 5281* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 5282* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 5283* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 5284* [Citation](citation.html): Intended jurisdiction for the citation 5285* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 5286* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 5287* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 5288* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 5289* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 5290* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 5291* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 5292* [Library](library.html): Intended jurisdiction for the library 5293* [Measure](measure.html): Intended jurisdiction for the measure 5294* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 5295* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 5296* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 5297* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 5298* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 5299* [Requirements](requirements.html): Intended jurisdiction for the requirements 5300* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 5301* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 5302* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 5303* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 5304* [TestScript](testscript.html): Intended jurisdiction for the test script 5305* [ValueSet](valueset.html): Intended jurisdiction for the value set 5306</b><br> 5307 * Type: <b>token</b><br> 5308 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 5309 * </p> 5310 */ 5311 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 5312 5313 /** 5314 * Search parameter: <b>name</b> 5315 * <p> 5316 * Description: <b>Multiple Resources: 5317 5318* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 5319* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 5320* [Citation](citation.html): Computationally friendly name of the citation 5321* [CodeSystem](codesystem.html): Computationally friendly name of the code system 5322* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 5323* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 5324* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 5325* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 5326* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 5327* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 5328* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 5329* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 5330* [Library](library.html): Computationally friendly name of the library 5331* [Measure](measure.html): Computationally friendly name of the measure 5332* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 5333* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 5334* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 5335* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 5336* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 5337* [Requirements](requirements.html): Computationally friendly name of the requirements 5338* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 5339* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 5340* [StructureMap](structuremap.html): Computationally friendly name of the structure map 5341* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 5342* [TestScript](testscript.html): Computationally friendly name of the test script 5343* [ValueSet](valueset.html): Computationally friendly name of the value set 5344</b><br> 5345 * Type: <b>string</b><br> 5346 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 5347 * </p> 5348 */ 5349 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 5350 public static final String SP_NAME = "name"; 5351 /** 5352 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5353 * <p> 5354 * Description: <b>Multiple Resources: 5355 5356* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 5357* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 5358* [Citation](citation.html): Computationally friendly name of the citation 5359* [CodeSystem](codesystem.html): Computationally friendly name of the code system 5360* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 5361* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 5362* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 5363* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 5364* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 5365* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 5366* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 5367* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 5368* [Library](library.html): Computationally friendly name of the library 5369* [Measure](measure.html): Computationally friendly name of the measure 5370* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 5371* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 5372* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 5373* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 5374* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 5375* [Requirements](requirements.html): Computationally friendly name of the requirements 5376* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 5377* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 5378* [StructureMap](structuremap.html): Computationally friendly name of the structure map 5379* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 5380* [TestScript](testscript.html): Computationally friendly name of the test script 5381* [ValueSet](valueset.html): Computationally friendly name of the value set 5382</b><br> 5383 * Type: <b>string</b><br> 5384 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 5385 * </p> 5386 */ 5387 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 5388 5389 /** 5390 * Search parameter: <b>publisher</b> 5391 * <p> 5392 * Description: <b>Multiple Resources: 5393 5394* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5395* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5396* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5397* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5398* [Citation](citation.html): Name of the publisher of the citation 5399* [CodeSystem](codesystem.html): Name of the publisher of the code system 5400* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5401* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5402* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5403* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5404* [Evidence](evidence.html): Name of the publisher of the evidence 5405* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5406* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5407* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5408* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5409* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5410* [Library](library.html): Name of the publisher of the library 5411* [Measure](measure.html): Name of the publisher of the measure 5412* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5413* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5414* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5415* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5416* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5417* [Requirements](requirements.html): Name of the publisher of the requirements 5418* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5419* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5420* [StructureMap](structuremap.html): Name of the publisher of the structure map 5421* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5422* [TestScript](testscript.html): Name of the publisher of the test script 5423* [ValueSet](valueset.html): Name of the publisher of the value set 5424</b><br> 5425 * Type: <b>string</b><br> 5426 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5427 * </p> 5428 */ 5429 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 5430 public static final String SP_PUBLISHER = "publisher"; 5431 /** 5432 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5433 * <p> 5434 * Description: <b>Multiple Resources: 5435 5436* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5437* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5438* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5439* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5440* [Citation](citation.html): Name of the publisher of the citation 5441* [CodeSystem](codesystem.html): Name of the publisher of the code system 5442* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5443* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5444* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5445* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5446* [Evidence](evidence.html): Name of the publisher of the evidence 5447* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5448* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5449* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5450* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5451* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5452* [Library](library.html): Name of the publisher of the library 5453* [Measure](measure.html): Name of the publisher of the measure 5454* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5455* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5456* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5457* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5458* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5459* [Requirements](requirements.html): Name of the publisher of the requirements 5460* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5461* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5462* [StructureMap](structuremap.html): Name of the publisher of the structure map 5463* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5464* [TestScript](testscript.html): Name of the publisher of the test script 5465* [ValueSet](valueset.html): Name of the publisher of the value set 5466</b><br> 5467 * Type: <b>string</b><br> 5468 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5469 * </p> 5470 */ 5471 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5472 5473 /** 5474 * Search parameter: <b>status</b> 5475 * <p> 5476 * Description: <b>Multiple Resources: 5477 5478* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5479* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5480* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5481* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5482* [Citation](citation.html): The current status of the citation 5483* [CodeSystem](codesystem.html): The current status of the code system 5484* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5485* [ConceptMap](conceptmap.html): The current status of the concept map 5486* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5487* [EventDefinition](eventdefinition.html): The current status of the event definition 5488* [Evidence](evidence.html): The current status of the evidence 5489* [EvidenceReport](evidencereport.html): The current status of the evidence report 5490* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5491* [ExampleScenario](examplescenario.html): The current status of the example scenario 5492* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5493* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5494* [Library](library.html): The current status of the library 5495* [Measure](measure.html): The current status of the measure 5496* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5497* [MessageDefinition](messagedefinition.html): The current status of the message definition 5498* [NamingSystem](namingsystem.html): The current status of the naming system 5499* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5500* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5501* [PlanDefinition](plandefinition.html): The current status of the plan definition 5502* [Questionnaire](questionnaire.html): The current status of the questionnaire 5503* [Requirements](requirements.html): The current status of the requirements 5504* [SearchParameter](searchparameter.html): The current status of the search parameter 5505* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5506* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5507* [StructureMap](structuremap.html): The current status of the structure map 5508* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5509* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5510* [TestPlan](testplan.html): The current status of the test plan 5511* [TestScript](testscript.html): The current status of the test script 5512* [ValueSet](valueset.html): The current status of the value set 5513</b><br> 5514 * Type: <b>token</b><br> 5515 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5516 * </p> 5517 */ 5518 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 5519 public static final String SP_STATUS = "status"; 5520 /** 5521 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5522 * <p> 5523 * Description: <b>Multiple Resources: 5524 5525* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5526* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5527* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5528* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5529* [Citation](citation.html): The current status of the citation 5530* [CodeSystem](codesystem.html): The current status of the code system 5531* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5532* [ConceptMap](conceptmap.html): The current status of the concept map 5533* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5534* [EventDefinition](eventdefinition.html): The current status of the event definition 5535* [Evidence](evidence.html): The current status of the evidence 5536* [EvidenceReport](evidencereport.html): The current status of the evidence report 5537* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5538* [ExampleScenario](examplescenario.html): The current status of the example scenario 5539* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5540* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5541* [Library](library.html): The current status of the library 5542* [Measure](measure.html): The current status of the measure 5543* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5544* [MessageDefinition](messagedefinition.html): The current status of the message definition 5545* [NamingSystem](namingsystem.html): The current status of the naming system 5546* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5547* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5548* [PlanDefinition](plandefinition.html): The current status of the plan definition 5549* [Questionnaire](questionnaire.html): The current status of the questionnaire 5550* [Requirements](requirements.html): The current status of the requirements 5551* [SearchParameter](searchparameter.html): The current status of the search parameter 5552* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5553* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5554* [StructureMap](structuremap.html): The current status of the structure map 5555* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5556* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5557* [TestPlan](testplan.html): The current status of the test plan 5558* [TestScript](testscript.html): The current status of the test script 5559* [ValueSet](valueset.html): The current status of the value set 5560</b><br> 5561 * Type: <b>token</b><br> 5562 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5563 * </p> 5564 */ 5565 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5566 5567 /** 5568 * Search parameter: <b>title</b> 5569 * <p> 5570 * Description: <b>Multiple Resources: 5571 5572* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 5573* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 5574* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 5575* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 5576* [Citation](citation.html): The human-friendly name of the citation 5577* [CodeSystem](codesystem.html): The human-friendly name of the code system 5578* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 5579* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 5580* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 5581* [Evidence](evidence.html): The human-friendly name of the evidence 5582* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 5583* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 5584* [Library](library.html): The human-friendly name of the library 5585* [Measure](measure.html): The human-friendly name of the measure 5586* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 5587* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 5588* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 5589* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 5590* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 5591* [Requirements](requirements.html): The human-friendly name of the requirements 5592* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 5593* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 5594* [StructureMap](structuremap.html): The human-friendly name of the structure map 5595* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 5596* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 5597* [TestScript](testscript.html): The human-friendly name of the test script 5598* [ValueSet](valueset.html): The human-friendly name of the value set 5599</b><br> 5600 * Type: <b>string</b><br> 5601 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 5602 * </p> 5603 */ 5604 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 5605 public static final String SP_TITLE = "title"; 5606 /** 5607 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5608 * <p> 5609 * Description: <b>Multiple Resources: 5610 5611* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 5612* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 5613* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 5614* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 5615* [Citation](citation.html): The human-friendly name of the citation 5616* [CodeSystem](codesystem.html): The human-friendly name of the code system 5617* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 5618* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 5619* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 5620* [Evidence](evidence.html): The human-friendly name of the evidence 5621* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 5622* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 5623* [Library](library.html): The human-friendly name of the library 5624* [Measure](measure.html): The human-friendly name of the measure 5625* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 5626* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 5627* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 5628* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 5629* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 5630* [Requirements](requirements.html): The human-friendly name of the requirements 5631* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 5632* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 5633* [StructureMap](structuremap.html): The human-friendly name of the structure map 5634* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 5635* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 5636* [TestScript](testscript.html): The human-friendly name of the test script 5637* [ValueSet](valueset.html): The human-friendly name of the value set 5638</b><br> 5639 * Type: <b>string</b><br> 5640 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 5641 * </p> 5642 */ 5643 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 5644 5645 /** 5646 * Search parameter: <b>url</b> 5647 * <p> 5648 * Description: <b>Multiple Resources: 5649 5650* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5651* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5652* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5653* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5654* [Citation](citation.html): The uri that identifies the citation 5655* [CodeSystem](codesystem.html): The uri that identifies the code system 5656* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5657* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5658* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5659* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5660* [Evidence](evidence.html): The uri that identifies the evidence 5661* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5662* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5663* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5664* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5665* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5666* [Library](library.html): The uri that identifies the library 5667* [Measure](measure.html): The uri that identifies the measure 5668* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5669* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5670* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5671* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5672* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5673* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5674* [Requirements](requirements.html): The uri that identifies the requirements 5675* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5676* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5677* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5678* [StructureMap](structuremap.html): The uri that identifies the structure map 5679* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5680* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5681* [TestPlan](testplan.html): The uri that identifies the test plan 5682* [TestScript](testscript.html): The uri that identifies the test script 5683* [ValueSet](valueset.html): The uri that identifies the value set 5684</b><br> 5685 * Type: <b>uri</b><br> 5686 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5687 * </p> 5688 */ 5689 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 5690 public static final String SP_URL = "url"; 5691 /** 5692 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5693 * <p> 5694 * Description: <b>Multiple Resources: 5695 5696* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5697* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5698* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5699* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5700* [Citation](citation.html): The uri that identifies the citation 5701* [CodeSystem](codesystem.html): The uri that identifies the code system 5702* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5703* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5704* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5705* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5706* [Evidence](evidence.html): The uri that identifies the evidence 5707* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5708* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5709* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5710* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5711* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5712* [Library](library.html): The uri that identifies the library 5713* [Measure](measure.html): The uri that identifies the measure 5714* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5715* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5716* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5717* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5718* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5719* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5720* [Requirements](requirements.html): The uri that identifies the requirements 5721* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5722* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5723* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5724* [StructureMap](structuremap.html): The uri that identifies the structure map 5725* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5726* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5727* [TestPlan](testplan.html): The uri that identifies the test plan 5728* [TestScript](testscript.html): The uri that identifies the test script 5729* [ValueSet](valueset.html): The uri that identifies the value set 5730</b><br> 5731 * Type: <b>uri</b><br> 5732 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5733 * </p> 5734 */ 5735 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5736 5737 /** 5738 * Search parameter: <b>version</b> 5739 * <p> 5740 * Description: <b>Multiple Resources: 5741 5742* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5743* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5744* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5745* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5746* [Citation](citation.html): The business version of the citation 5747* [CodeSystem](codesystem.html): The business version of the code system 5748* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5749* [ConceptMap](conceptmap.html): The business version of the concept map 5750* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5751* [EventDefinition](eventdefinition.html): The business version of the event definition 5752* [Evidence](evidence.html): The business version of the evidence 5753* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5754* [ExampleScenario](examplescenario.html): The business version of the example scenario 5755* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5756* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5757* [Library](library.html): The business version of the library 5758* [Measure](measure.html): The business version of the measure 5759* [MessageDefinition](messagedefinition.html): The business version of the message definition 5760* [NamingSystem](namingsystem.html): The business version of the naming system 5761* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5762* [PlanDefinition](plandefinition.html): The business version of the plan definition 5763* [Questionnaire](questionnaire.html): The business version of the questionnaire 5764* [Requirements](requirements.html): The business version of the requirements 5765* [SearchParameter](searchparameter.html): The business version of the search parameter 5766* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5767* [StructureMap](structuremap.html): The business version of the structure map 5768* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5769* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5770* [TestScript](testscript.html): The business version of the test script 5771* [ValueSet](valueset.html): The business version of the value set 5772</b><br> 5773 * Type: <b>token</b><br> 5774 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5775 * </p> 5776 */ 5777 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 5778 public static final String SP_VERSION = "version"; 5779 /** 5780 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5781 * <p> 5782 * Description: <b>Multiple Resources: 5783 5784* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5785* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5786* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5787* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5788* [Citation](citation.html): The business version of the citation 5789* [CodeSystem](codesystem.html): The business version of the code system 5790* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5791* [ConceptMap](conceptmap.html): The business version of the concept map 5792* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5793* [EventDefinition](eventdefinition.html): The business version of the event definition 5794* [Evidence](evidence.html): The business version of the evidence 5795* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5796* [ExampleScenario](examplescenario.html): The business version of the example scenario 5797* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5798* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5799* [Library](library.html): The business version of the library 5800* [Measure](measure.html): The business version of the measure 5801* [MessageDefinition](messagedefinition.html): The business version of the message definition 5802* [NamingSystem](namingsystem.html): The business version of the naming system 5803* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5804* [PlanDefinition](plandefinition.html): The business version of the plan definition 5805* [Questionnaire](questionnaire.html): The business version of the questionnaire 5806* [Requirements](requirements.html): The business version of the requirements 5807* [SearchParameter](searchparameter.html): The business version of the search parameter 5808* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5809* [StructureMap](structuremap.html): The business version of the structure map 5810* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5811* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5812* [TestScript](testscript.html): The business version of the test script 5813* [ValueSet](valueset.html): The business version of the value set 5814</b><br> 5815 * Type: <b>token</b><br> 5816 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5817 * </p> 5818 */ 5819 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5820 5821 /** 5822 * Search parameter: <b>base</b> 5823 * <p> 5824 * Description: <b>Marks this as a profile of the base</b><br> 5825 * Type: <b>reference</b><br> 5826 * Path: <b>OperationDefinition.base</b><br> 5827 * </p> 5828 */ 5829 @SearchParamDefinition(name="base", path="OperationDefinition.base", description="Marks this as a profile of the base", type="reference", target={OperationDefinition.class } ) 5830 public static final String SP_BASE = "base"; 5831 /** 5832 * <b>Fluent Client</b> search parameter constant for <b>base</b> 5833 * <p> 5834 * Description: <b>Marks this as a profile of the base</b><br> 5835 * Type: <b>reference</b><br> 5836 * Path: <b>OperationDefinition.base</b><br> 5837 * </p> 5838 */ 5839 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASE); 5840 5841/** 5842 * Constant for fluent queries to be used to add include statements. Specifies 5843 * the path value of "<b>OperationDefinition:base</b>". 5844 */ 5845 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASE = new ca.uhn.fhir.model.api.Include("OperationDefinition:base").toLocked(); 5846 5847 /** 5848 * Search parameter: <b>code</b> 5849 * <p> 5850 * Description: <b>Name used to invoke the operation</b><br> 5851 * Type: <b>token</b><br> 5852 * Path: <b>OperationDefinition.code</b><br> 5853 * </p> 5854 */ 5855 @SearchParamDefinition(name="code", path="OperationDefinition.code", description="Name used to invoke the operation", type="token" ) 5856 public static final String SP_CODE = "code"; 5857 /** 5858 * <b>Fluent Client</b> search parameter constant for <b>code</b> 5859 * <p> 5860 * Description: <b>Name used to invoke the operation</b><br> 5861 * Type: <b>token</b><br> 5862 * Path: <b>OperationDefinition.code</b><br> 5863 * </p> 5864 */ 5865 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 5866 5867 /** 5868 * Search parameter: <b>input-profile</b> 5869 * <p> 5870 * Description: <b>Validation information for in parameters</b><br> 5871 * Type: <b>reference</b><br> 5872 * Path: <b>OperationDefinition.inputProfile</b><br> 5873 * </p> 5874 */ 5875 @SearchParamDefinition(name="input-profile", path="OperationDefinition.inputProfile", description="Validation information for in parameters", type="reference", target={StructureDefinition.class } ) 5876 public static final String SP_INPUT_PROFILE = "input-profile"; 5877 /** 5878 * <b>Fluent Client</b> search parameter constant for <b>input-profile</b> 5879 * <p> 5880 * Description: <b>Validation information for in parameters</b><br> 5881 * Type: <b>reference</b><br> 5882 * Path: <b>OperationDefinition.inputProfile</b><br> 5883 * </p> 5884 */ 5885 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INPUT_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INPUT_PROFILE); 5886 5887/** 5888 * Constant for fluent queries to be used to add include statements. Specifies 5889 * the path value of "<b>OperationDefinition:input-profile</b>". 5890 */ 5891 public static final ca.uhn.fhir.model.api.Include INCLUDE_INPUT_PROFILE = new ca.uhn.fhir.model.api.Include("OperationDefinition:input-profile").toLocked(); 5892 5893 /** 5894 * Search parameter: <b>instance</b> 5895 * <p> 5896 * Description: <b>Invoke on an instance?</b><br> 5897 * Type: <b>token</b><br> 5898 * Path: <b>OperationDefinition.instance</b><br> 5899 * </p> 5900 */ 5901 @SearchParamDefinition(name="instance", path="OperationDefinition.instance", description="Invoke on an instance?", type="token" ) 5902 public static final String SP_INSTANCE = "instance"; 5903 /** 5904 * <b>Fluent Client</b> search parameter constant for <b>instance</b> 5905 * <p> 5906 * Description: <b>Invoke on an instance?</b><br> 5907 * Type: <b>token</b><br> 5908 * Path: <b>OperationDefinition.instance</b><br> 5909 * </p> 5910 */ 5911 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INSTANCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INSTANCE); 5912 5913 /** 5914 * Search parameter: <b>kind</b> 5915 * <p> 5916 * Description: <b>operation | query</b><br> 5917 * Type: <b>token</b><br> 5918 * Path: <b>OperationDefinition.kind</b><br> 5919 * </p> 5920 */ 5921 @SearchParamDefinition(name="kind", path="OperationDefinition.kind", description="operation | query", type="token" ) 5922 public static final String SP_KIND = "kind"; 5923 /** 5924 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 5925 * <p> 5926 * Description: <b>operation | query</b><br> 5927 * Type: <b>token</b><br> 5928 * Path: <b>OperationDefinition.kind</b><br> 5929 * </p> 5930 */ 5931 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 5932 5933 /** 5934 * Search parameter: <b>output-profile</b> 5935 * <p> 5936 * Description: <b>Validation information for out parameters</b><br> 5937 * Type: <b>reference</b><br> 5938 * Path: <b>OperationDefinition.outputProfile</b><br> 5939 * </p> 5940 */ 5941 @SearchParamDefinition(name="output-profile", path="OperationDefinition.outputProfile", description="Validation information for out parameters", type="reference", target={StructureDefinition.class } ) 5942 public static final String SP_OUTPUT_PROFILE = "output-profile"; 5943 /** 5944 * <b>Fluent Client</b> search parameter constant for <b>output-profile</b> 5945 * <p> 5946 * Description: <b>Validation information for out parameters</b><br> 5947 * Type: <b>reference</b><br> 5948 * Path: <b>OperationDefinition.outputProfile</b><br> 5949 * </p> 5950 */ 5951 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OUTPUT_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OUTPUT_PROFILE); 5952 5953/** 5954 * Constant for fluent queries to be used to add include statements. Specifies 5955 * the path value of "<b>OperationDefinition:output-profile</b>". 5956 */ 5957 public static final ca.uhn.fhir.model.api.Include INCLUDE_OUTPUT_PROFILE = new ca.uhn.fhir.model.api.Include("OperationDefinition:output-profile").toLocked(); 5958 5959 /** 5960 * Search parameter: <b>system</b> 5961 * <p> 5962 * Description: <b>Invoke at the system level?</b><br> 5963 * Type: <b>token</b><br> 5964 * Path: <b>OperationDefinition.system</b><br> 5965 * </p> 5966 */ 5967 @SearchParamDefinition(name="system", path="OperationDefinition.system", description="Invoke at the system level?", type="token" ) 5968 public static final String SP_SYSTEM = "system"; 5969 /** 5970 * <b>Fluent Client</b> search parameter constant for <b>system</b> 5971 * <p> 5972 * Description: <b>Invoke at the system level?</b><br> 5973 * Type: <b>token</b><br> 5974 * Path: <b>OperationDefinition.system</b><br> 5975 * </p> 5976 */ 5977 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SYSTEM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SYSTEM); 5978 5979 /** 5980 * Search parameter: <b>type</b> 5981 * <p> 5982 * Description: <b>Invoke at the type level?</b><br> 5983 * Type: <b>token</b><br> 5984 * Path: <b>OperationDefinition.type</b><br> 5985 * </p> 5986 */ 5987 @SearchParamDefinition(name="type", path="OperationDefinition.type", description="Invoke at the type level?", type="token" ) 5988 public static final String SP_TYPE = "type"; 5989 /** 5990 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5991 * <p> 5992 * Description: <b>Invoke at the type level?</b><br> 5993 * Type: <b>token</b><br> 5994 * Path: <b>OperationDefinition.type</b><br> 5995 * </p> 5996 */ 5997 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 5998 5999// Manual code (from Configuration.txt): 6000 public boolean supportsCopyright() { 6001 return true; 6002 } 6003 6004// end addition 6005 6006} 6007