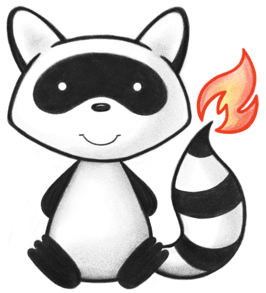
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.r5.utils.ToolingExtensions; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 052/** 053 * A collection of error, warning, or information messages that result from a system action. 054 */ 055@ResourceDef(name="OperationOutcome", profile="http://hl7.org/fhir/StructureDefinition/OperationOutcome") 056public class OperationOutcome extends DomainResource implements IBaseOperationOutcome { 057 058 public enum IssueSeverity { 059 /** 060 * The issue caused the action to fail and no further checking could be performed. 061 */ 062 FATAL, 063 /** 064 * The issue is sufficiently important to cause the action to fail. 065 */ 066 ERROR, 067 /** 068 * The issue is not important enough to cause the action to fail but may cause it to be performed suboptimally or in a way that is not as desired. 069 */ 070 WARNING, 071 /** 072 * The issue has no relation to the degree of success of the action. 073 */ 074 INFORMATION, 075 /** 076 * The operation completed successfully. 077 */ 078 SUCCESS, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 public static IssueSeverity fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("fatal".equals(codeString)) 087 return FATAL; 088 if ("error".equals(codeString)) 089 return ERROR; 090 if ("warning".equals(codeString)) 091 return WARNING; 092 if ("information".equals(codeString)) 093 return INFORMATION; 094 if ("success".equals(codeString)) 095 return SUCCESS; 096 if (Configuration.isAcceptInvalidEnums()) 097 return null; 098 else 099 throw new FHIRException("Unknown IssueSeverity code '"+codeString+"'"); 100 } 101 public String toCode() { 102 switch (this) { 103 case FATAL: return "fatal"; 104 case ERROR: return "error"; 105 case WARNING: return "warning"; 106 case INFORMATION: return "information"; 107 case SUCCESS: return "success"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getSystem() { 113 switch (this) { 114 case FATAL: return "http://hl7.org/fhir/issue-severity"; 115 case ERROR: return "http://hl7.org/fhir/issue-severity"; 116 case WARNING: return "http://hl7.org/fhir/issue-severity"; 117 case INFORMATION: return "http://hl7.org/fhir/issue-severity"; 118 case SUCCESS: return "http://hl7.org/fhir/issue-severity"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDefinition() { 124 switch (this) { 125 case FATAL: return "The issue caused the action to fail and no further checking could be performed."; 126 case ERROR: return "The issue is sufficiently important to cause the action to fail."; 127 case WARNING: return "The issue is not important enough to cause the action to fail but may cause it to be performed suboptimally or in a way that is not as desired."; 128 case INFORMATION: return "The issue has no relation to the degree of success of the action."; 129 case SUCCESS: return "The operation completed successfully."; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getDisplay() { 135 switch (this) { 136 case FATAL: return "Fatal"; 137 case ERROR: return "Error"; 138 case WARNING: return "Warning"; 139 case INFORMATION: return "Information"; 140 case SUCCESS: return "Operation Successful"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public boolean isHigherThan(IssueSeverity other) { 146 return this.ordinal() < other.ordinal(); 147 } 148 } 149 150 public static class IssueSeverityEnumFactory implements EnumFactory<IssueSeverity> { 151 public IssueSeverity fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("fatal".equals(codeString)) 156 return IssueSeverity.FATAL; 157 if ("error".equals(codeString)) 158 return IssueSeverity.ERROR; 159 if ("warning".equals(codeString)) 160 return IssueSeverity.WARNING; 161 if ("information".equals(codeString)) 162 return IssueSeverity.INFORMATION; 163 if ("success".equals(codeString)) 164 return IssueSeverity.SUCCESS; 165 throw new IllegalArgumentException("Unknown IssueSeverity code '"+codeString+"'"); 166 } 167 public Enumeration<IssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 168 if (code == null) 169 return null; 170 if (code.isEmpty()) 171 return new Enumeration<IssueSeverity>(this, IssueSeverity.NULL, code); 172 String codeString = ((PrimitiveType) code).asStringValue(); 173 if (codeString == null || "".equals(codeString)) 174 return new Enumeration<IssueSeverity>(this, IssueSeverity.NULL, code); 175 if ("fatal".equals(codeString)) 176 return new Enumeration<IssueSeverity>(this, IssueSeverity.FATAL, code); 177 if ("error".equals(codeString)) 178 return new Enumeration<IssueSeverity>(this, IssueSeverity.ERROR, code); 179 if ("warning".equals(codeString)) 180 return new Enumeration<IssueSeverity>(this, IssueSeverity.WARNING, code); 181 if ("information".equals(codeString)) 182 return new Enumeration<IssueSeverity>(this, IssueSeverity.INFORMATION, code); 183 if ("success".equals(codeString)) 184 return new Enumeration<IssueSeverity>(this, IssueSeverity.SUCCESS, code); 185 throw new FHIRException("Unknown IssueSeverity code '"+codeString+"'"); 186 } 187 public String toCode(IssueSeverity code) { 188 if (code == IssueSeverity.NULL) 189 return null; 190 if (code == IssueSeverity.FATAL) 191 return "fatal"; 192 if (code == IssueSeverity.ERROR) 193 return "error"; 194 if (code == IssueSeverity.WARNING) 195 return "warning"; 196 if (code == IssueSeverity.INFORMATION) 197 return "information"; 198 if (code == IssueSeverity.SUCCESS) 199 return "success"; 200 return "?"; 201 } 202 public String toSystem(IssueSeverity code) { 203 return code.getSystem(); 204 } 205 } 206 207 public enum IssueType { 208 /** 209 * Content invalid against the specification or a profile. 210 */ 211 INVALID, 212 /** 213 * A structural issue in the content such as wrong namespace, unable to parse the content completely, invalid syntax, etc. 214 */ 215 STRUCTURE, 216 /** 217 * A required element is missing. 218 */ 219 REQUIRED, 220 /** 221 * An element or header value is invalid. 222 */ 223 VALUE, 224 /** 225 * A content validation rule failed - e.g. a schematron rule. 226 */ 227 INVARIANT, 228 /** 229 * An authentication/authorization/permissions issue of some kind. 230 */ 231 SECURITY, 232 /** 233 * The client needs to initiate an authentication process. 234 */ 235 LOGIN, 236 /** 237 * The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable). 238 */ 239 UNKNOWN, 240 /** 241 * User session expired; a login may be required. 242 */ 243 EXPIRED, 244 /** 245 * The user does not have the rights to perform this action. 246 */ 247 FORBIDDEN, 248 /** 249 * Some information was not or might not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes. 250 */ 251 SUPPRESSED, 252 /** 253 * Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged. 254 */ 255 PROCESSING, 256 /** 257 * The interaction, operation, resource or profile is not supported. 258 */ 259 NOTSUPPORTED, 260 /** 261 * An attempt was made to create a duplicate record. 262 */ 263 DUPLICATE, 264 /** 265 * Multiple matching records were found when the operation required only one match. 266 */ 267 MULTIPLEMATCHES, 268 /** 269 * The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture. 270 */ 271 NOTFOUND, 272 /** 273 * The reference pointed to content (usually a resource) that has been deleted. 274 */ 275 DELETED, 276 /** 277 * Provided content is too long (typically, this is a denial of service protection type of error). 278 */ 279 TOOLONG, 280 /** 281 * The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code. 282 */ 283 CODEINVALID, 284 /** 285 * An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized. 286 */ 287 EXTENSION, 288 /** 289 * The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT. 290 */ 291 TOOCOSTLY, 292 /** 293 * The content/operation failed to pass some business rule and so could not proceed. 294 */ 295 BUSINESSRULE, 296 /** 297 * Content could not be accepted because of an edit conflict (i.e. version aware updates). (In a pure RESTful environment, this would be an HTTP 409 error, but this code may be used where the conflict is discovered further into the application architecture.). 298 */ 299 CONFLICT, 300 /** 301 * Some search filters might not have applied on all results. Data may have been included that does not meet all of the filters listed in the `self` `Bundle.link`. 302 */ 303 LIMITEDFILTER, 304 /** 305 * Transient processing issues. The system receiving the message may be able to resubmit the same content once an underlying issue is resolved. 306 */ 307 TRANSIENT, 308 /** 309 * A resource/record locking failure (usually in an underlying database). 310 */ 311 LOCKERROR, 312 /** 313 * The persistent store is unavailable; e.g. the database is down for maintenance or similar action, and the interaction or operation cannot be processed. 314 */ 315 NOSTORE, 316 /** 317 * An unexpected internal error has occurred. 318 */ 319 EXCEPTION, 320 /** 321 * An internal timeout has occurred. 322 */ 323 TIMEOUT, 324 /** 325 * Not all data sources typically accessed could be reached or responded in time, so the returned information might not be complete (applies to search interactions and some operations). 326 */ 327 INCOMPLETE, 328 /** 329 * The system is not prepared to handle this request due to load management. 330 */ 331 THROTTLED, 332 /** 333 * A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.). 334 */ 335 INFORMATIONAL, 336 /** 337 * The operation completed successfully. 338 */ 339 SUCCESS, 340 /** 341 * added to help the parsers with the generic types 342 */ 343 NULL; 344 public static IssueType fromCode(String codeString) throws FHIRException { 345 if (codeString == null || "".equals(codeString)) 346 return null; 347 if ("invalid".equals(codeString)) 348 return INVALID; 349 if ("structure".equals(codeString)) 350 return STRUCTURE; 351 if ("required".equals(codeString)) 352 return REQUIRED; 353 if ("value".equals(codeString)) 354 return VALUE; 355 if ("invariant".equals(codeString)) 356 return INVARIANT; 357 if ("security".equals(codeString)) 358 return SECURITY; 359 if ("login".equals(codeString)) 360 return LOGIN; 361 if ("unknown".equals(codeString)) 362 return UNKNOWN; 363 if ("expired".equals(codeString)) 364 return EXPIRED; 365 if ("forbidden".equals(codeString)) 366 return FORBIDDEN; 367 if ("suppressed".equals(codeString)) 368 return SUPPRESSED; 369 if ("processing".equals(codeString)) 370 return PROCESSING; 371 if ("not-supported".equals(codeString)) 372 return NOTSUPPORTED; 373 if ("duplicate".equals(codeString)) 374 return DUPLICATE; 375 if ("multiple-matches".equals(codeString)) 376 return MULTIPLEMATCHES; 377 if ("not-found".equals(codeString)) 378 return NOTFOUND; 379 if ("deleted".equals(codeString)) 380 return DELETED; 381 if ("too-long".equals(codeString)) 382 return TOOLONG; 383 if ("code-invalid".equals(codeString)) 384 return CODEINVALID; 385 if ("extension".equals(codeString)) 386 return EXTENSION; 387 if ("too-costly".equals(codeString)) 388 return TOOCOSTLY; 389 if ("business-rule".equals(codeString)) 390 return BUSINESSRULE; 391 if ("conflict".equals(codeString)) 392 return CONFLICT; 393 if ("limited-filter".equals(codeString)) 394 return LIMITEDFILTER; 395 if ("transient".equals(codeString)) 396 return TRANSIENT; 397 if ("lock-error".equals(codeString)) 398 return LOCKERROR; 399 if ("no-store".equals(codeString)) 400 return NOSTORE; 401 if ("exception".equals(codeString)) 402 return EXCEPTION; 403 if ("timeout".equals(codeString)) 404 return TIMEOUT; 405 if ("incomplete".equals(codeString)) 406 return INCOMPLETE; 407 if ("throttled".equals(codeString)) 408 return THROTTLED; 409 if ("informational".equals(codeString)) 410 return INFORMATIONAL; 411 if ("success".equals(codeString)) 412 return SUCCESS; 413 if (Configuration.isAcceptInvalidEnums()) 414 return null; 415 else 416 throw new FHIRException("Unknown IssueType code '"+codeString+"'"); 417 } 418 public String toCode() { 419 switch (this) { 420 case INVALID: return "invalid"; 421 case STRUCTURE: return "structure"; 422 case REQUIRED: return "required"; 423 case VALUE: return "value"; 424 case INVARIANT: return "invariant"; 425 case SECURITY: return "security"; 426 case LOGIN: return "login"; 427 case UNKNOWN: return "unknown"; 428 case EXPIRED: return "expired"; 429 case FORBIDDEN: return "forbidden"; 430 case SUPPRESSED: return "suppressed"; 431 case PROCESSING: return "processing"; 432 case NOTSUPPORTED: return "not-supported"; 433 case DUPLICATE: return "duplicate"; 434 case MULTIPLEMATCHES: return "multiple-matches"; 435 case NOTFOUND: return "not-found"; 436 case DELETED: return "deleted"; 437 case TOOLONG: return "too-long"; 438 case CODEINVALID: return "code-invalid"; 439 case EXTENSION: return "extension"; 440 case TOOCOSTLY: return "too-costly"; 441 case BUSINESSRULE: return "business-rule"; 442 case CONFLICT: return "conflict"; 443 case LIMITEDFILTER: return "limited-filter"; 444 case TRANSIENT: return "transient"; 445 case LOCKERROR: return "lock-error"; 446 case NOSTORE: return "no-store"; 447 case EXCEPTION: return "exception"; 448 case TIMEOUT: return "timeout"; 449 case INCOMPLETE: return "incomplete"; 450 case THROTTLED: return "throttled"; 451 case INFORMATIONAL: return "informational"; 452 case SUCCESS: return "success"; 453 case NULL: return null; 454 default: return "?"; 455 } 456 } 457 public String getSystem() { 458 switch (this) { 459 case INVALID: return "http://hl7.org/fhir/issue-type"; 460 case STRUCTURE: return "http://hl7.org/fhir/issue-type"; 461 case REQUIRED: return "http://hl7.org/fhir/issue-type"; 462 case VALUE: return "http://hl7.org/fhir/issue-type"; 463 case INVARIANT: return "http://hl7.org/fhir/issue-type"; 464 case SECURITY: return "http://hl7.org/fhir/issue-type"; 465 case LOGIN: return "http://hl7.org/fhir/issue-type"; 466 case UNKNOWN: return "http://hl7.org/fhir/issue-type"; 467 case EXPIRED: return "http://hl7.org/fhir/issue-type"; 468 case FORBIDDEN: return "http://hl7.org/fhir/issue-type"; 469 case SUPPRESSED: return "http://hl7.org/fhir/issue-type"; 470 case PROCESSING: return "http://hl7.org/fhir/issue-type"; 471 case NOTSUPPORTED: return "http://hl7.org/fhir/issue-type"; 472 case DUPLICATE: return "http://hl7.org/fhir/issue-type"; 473 case MULTIPLEMATCHES: return "http://hl7.org/fhir/issue-type"; 474 case NOTFOUND: return "http://hl7.org/fhir/issue-type"; 475 case DELETED: return "http://hl7.org/fhir/issue-type"; 476 case TOOLONG: return "http://hl7.org/fhir/issue-type"; 477 case CODEINVALID: return "http://hl7.org/fhir/issue-type"; 478 case EXTENSION: return "http://hl7.org/fhir/issue-type"; 479 case TOOCOSTLY: return "http://hl7.org/fhir/issue-type"; 480 case BUSINESSRULE: return "http://hl7.org/fhir/issue-type"; 481 case CONFLICT: return "http://hl7.org/fhir/issue-type"; 482 case LIMITEDFILTER: return "http://hl7.org/fhir/issue-type"; 483 case TRANSIENT: return "http://hl7.org/fhir/issue-type"; 484 case LOCKERROR: return "http://hl7.org/fhir/issue-type"; 485 case NOSTORE: return "http://hl7.org/fhir/issue-type"; 486 case EXCEPTION: return "http://hl7.org/fhir/issue-type"; 487 case TIMEOUT: return "http://hl7.org/fhir/issue-type"; 488 case INCOMPLETE: return "http://hl7.org/fhir/issue-type"; 489 case THROTTLED: return "http://hl7.org/fhir/issue-type"; 490 case INFORMATIONAL: return "http://hl7.org/fhir/issue-type"; 491 case SUCCESS: return "http://hl7.org/fhir/issue-type"; 492 case NULL: return null; 493 default: return "?"; 494 } 495 } 496 public String getDefinition() { 497 switch (this) { 498 case INVALID: return "Content invalid against the specification or a profile."; 499 case STRUCTURE: return "A structural issue in the content such as wrong namespace, unable to parse the content completely, invalid syntax, etc."; 500 case REQUIRED: return "A required element is missing."; 501 case VALUE: return "An element or header value is invalid."; 502 case INVARIANT: return "A content validation rule failed - e.g. a schematron rule."; 503 case SECURITY: return "An authentication/authorization/permissions issue of some kind."; 504 case LOGIN: return "The client needs to initiate an authentication process."; 505 case UNKNOWN: return "The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable)."; 506 case EXPIRED: return "User session expired; a login may be required."; 507 case FORBIDDEN: return "The user does not have the rights to perform this action."; 508 case SUPPRESSED: return "Some information was not or might not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes."; 509 case PROCESSING: return "Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged."; 510 case NOTSUPPORTED: return "The interaction, operation, resource or profile is not supported."; 511 case DUPLICATE: return "An attempt was made to create a duplicate record."; 512 case MULTIPLEMATCHES: return "Multiple matching records were found when the operation required only one match."; 513 case NOTFOUND: return "The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture."; 514 case DELETED: return "The reference pointed to content (usually a resource) that has been deleted."; 515 case TOOLONG: return "Provided content is too long (typically, this is a denial of service protection type of error)."; 516 case CODEINVALID: return "The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code."; 517 case EXTENSION: return "An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized."; 518 case TOOCOSTLY: return "The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT."; 519 case BUSINESSRULE: return "The content/operation failed to pass some business rule and so could not proceed."; 520 case CONFLICT: return "Content could not be accepted because of an edit conflict (i.e. version aware updates). (In a pure RESTful environment, this would be an HTTP 409 error, but this code may be used where the conflict is discovered further into the application architecture.)."; 521 case LIMITEDFILTER: return "Some search filters might not have applied on all results. Data may have been included that does not meet all of the filters listed in the `self` `Bundle.link`."; 522 case TRANSIENT: return "Transient processing issues. The system receiving the message may be able to resubmit the same content once an underlying issue is resolved."; 523 case LOCKERROR: return "A resource/record locking failure (usually in an underlying database)."; 524 case NOSTORE: return "The persistent store is unavailable; e.g. the database is down for maintenance or similar action, and the interaction or operation cannot be processed."; 525 case EXCEPTION: return "An unexpected internal error has occurred."; 526 case TIMEOUT: return "An internal timeout has occurred."; 527 case INCOMPLETE: return "Not all data sources typically accessed could be reached or responded in time, so the returned information might not be complete (applies to search interactions and some operations)."; 528 case THROTTLED: return "The system is not prepared to handle this request due to load management."; 529 case INFORMATIONAL: return "A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.)."; 530 case SUCCESS: return "The operation completed successfully."; 531 case NULL: return null; 532 default: return "?"; 533 } 534 } 535 public String getDisplay() { 536 switch (this) { 537 case INVALID: return "Invalid Content"; 538 case STRUCTURE: return "Structural Issue"; 539 case REQUIRED: return "Required element missing"; 540 case VALUE: return "Element value invalid"; 541 case INVARIANT: return "Validation rule failed"; 542 case SECURITY: return "Security Problem"; 543 case LOGIN: return "Login Required"; 544 case UNKNOWN: return "Unknown User"; 545 case EXPIRED: return "Session Expired"; 546 case FORBIDDEN: return "Forbidden"; 547 case SUPPRESSED: return "Information Suppressed"; 548 case PROCESSING: return "Processing Failure"; 549 case NOTSUPPORTED: return "Content not supported"; 550 case DUPLICATE: return "Duplicate"; 551 case MULTIPLEMATCHES: return "Multiple Matches"; 552 case NOTFOUND: return "Not Found"; 553 case DELETED: return "Deleted"; 554 case TOOLONG: return "Content Too Long"; 555 case CODEINVALID: return "Invalid Code"; 556 case EXTENSION: return "Unacceptable Extension"; 557 case TOOCOSTLY: return "Operation Too Costly"; 558 case BUSINESSRULE: return "Business Rule Violation"; 559 case CONFLICT: return "Edit Version Conflict"; 560 case LIMITEDFILTER: return "Limited Filter Application"; 561 case TRANSIENT: return "Transient Issue"; 562 case LOCKERROR: return "Lock Error"; 563 case NOSTORE: return "No Store Available"; 564 case EXCEPTION: return "Exception"; 565 case TIMEOUT: return "Timeout"; 566 case INCOMPLETE: return "Incomplete Results"; 567 case THROTTLED: return "Throttled"; 568 case INFORMATIONAL: return "Informational Note"; 569 case SUCCESS: return "Operation Successful"; 570 case NULL: return null; 571 default: return "?"; 572 } 573 } 574 } 575 576 public static class IssueTypeEnumFactory implements EnumFactory<IssueType> { 577 public IssueType fromCode(String codeString) throws IllegalArgumentException { 578 if (codeString == null || "".equals(codeString)) 579 if (codeString == null || "".equals(codeString)) 580 return null; 581 if ("invalid".equals(codeString)) 582 return IssueType.INVALID; 583 if ("structure".equals(codeString)) 584 return IssueType.STRUCTURE; 585 if ("required".equals(codeString)) 586 return IssueType.REQUIRED; 587 if ("value".equals(codeString)) 588 return IssueType.VALUE; 589 if ("invariant".equals(codeString)) 590 return IssueType.INVARIANT; 591 if ("security".equals(codeString)) 592 return IssueType.SECURITY; 593 if ("login".equals(codeString)) 594 return IssueType.LOGIN; 595 if ("unknown".equals(codeString)) 596 return IssueType.UNKNOWN; 597 if ("expired".equals(codeString)) 598 return IssueType.EXPIRED; 599 if ("forbidden".equals(codeString)) 600 return IssueType.FORBIDDEN; 601 if ("suppressed".equals(codeString)) 602 return IssueType.SUPPRESSED; 603 if ("processing".equals(codeString)) 604 return IssueType.PROCESSING; 605 if ("not-supported".equals(codeString)) 606 return IssueType.NOTSUPPORTED; 607 if ("duplicate".equals(codeString)) 608 return IssueType.DUPLICATE; 609 if ("multiple-matches".equals(codeString)) 610 return IssueType.MULTIPLEMATCHES; 611 if ("not-found".equals(codeString)) 612 return IssueType.NOTFOUND; 613 if ("deleted".equals(codeString)) 614 return IssueType.DELETED; 615 if ("too-long".equals(codeString)) 616 return IssueType.TOOLONG; 617 if ("code-invalid".equals(codeString)) 618 return IssueType.CODEINVALID; 619 if ("extension".equals(codeString)) 620 return IssueType.EXTENSION; 621 if ("too-costly".equals(codeString)) 622 return IssueType.TOOCOSTLY; 623 if ("business-rule".equals(codeString)) 624 return IssueType.BUSINESSRULE; 625 if ("conflict".equals(codeString)) 626 return IssueType.CONFLICT; 627 if ("limited-filter".equals(codeString)) 628 return IssueType.LIMITEDFILTER; 629 if ("transient".equals(codeString)) 630 return IssueType.TRANSIENT; 631 if ("lock-error".equals(codeString)) 632 return IssueType.LOCKERROR; 633 if ("no-store".equals(codeString)) 634 return IssueType.NOSTORE; 635 if ("exception".equals(codeString)) 636 return IssueType.EXCEPTION; 637 if ("timeout".equals(codeString)) 638 return IssueType.TIMEOUT; 639 if ("incomplete".equals(codeString)) 640 return IssueType.INCOMPLETE; 641 if ("throttled".equals(codeString)) 642 return IssueType.THROTTLED; 643 if ("informational".equals(codeString)) 644 return IssueType.INFORMATIONAL; 645 if ("success".equals(codeString)) 646 return IssueType.SUCCESS; 647 throw new IllegalArgumentException("Unknown IssueType code '"+codeString+"'"); 648 } 649 public Enumeration<IssueType> fromType(PrimitiveType<?> code) throws FHIRException { 650 if (code == null) 651 return null; 652 if (code.isEmpty()) 653 return new Enumeration<IssueType>(this, IssueType.NULL, code); 654 String codeString = ((PrimitiveType) code).asStringValue(); 655 if (codeString == null || "".equals(codeString)) 656 return new Enumeration<IssueType>(this, IssueType.NULL, code); 657 if ("invalid".equals(codeString)) 658 return new Enumeration<IssueType>(this, IssueType.INVALID, code); 659 if ("structure".equals(codeString)) 660 return new Enumeration<IssueType>(this, IssueType.STRUCTURE, code); 661 if ("required".equals(codeString)) 662 return new Enumeration<IssueType>(this, IssueType.REQUIRED, code); 663 if ("value".equals(codeString)) 664 return new Enumeration<IssueType>(this, IssueType.VALUE, code); 665 if ("invariant".equals(codeString)) 666 return new Enumeration<IssueType>(this, IssueType.INVARIANT, code); 667 if ("security".equals(codeString)) 668 return new Enumeration<IssueType>(this, IssueType.SECURITY, code); 669 if ("login".equals(codeString)) 670 return new Enumeration<IssueType>(this, IssueType.LOGIN, code); 671 if ("unknown".equals(codeString)) 672 return new Enumeration<IssueType>(this, IssueType.UNKNOWN, code); 673 if ("expired".equals(codeString)) 674 return new Enumeration<IssueType>(this, IssueType.EXPIRED, code); 675 if ("forbidden".equals(codeString)) 676 return new Enumeration<IssueType>(this, IssueType.FORBIDDEN, code); 677 if ("suppressed".equals(codeString)) 678 return new Enumeration<IssueType>(this, IssueType.SUPPRESSED, code); 679 if ("processing".equals(codeString)) 680 return new Enumeration<IssueType>(this, IssueType.PROCESSING, code); 681 if ("not-supported".equals(codeString)) 682 return new Enumeration<IssueType>(this, IssueType.NOTSUPPORTED, code); 683 if ("duplicate".equals(codeString)) 684 return new Enumeration<IssueType>(this, IssueType.DUPLICATE, code); 685 if ("multiple-matches".equals(codeString)) 686 return new Enumeration<IssueType>(this, IssueType.MULTIPLEMATCHES, code); 687 if ("not-found".equals(codeString)) 688 return new Enumeration<IssueType>(this, IssueType.NOTFOUND, code); 689 if ("deleted".equals(codeString)) 690 return new Enumeration<IssueType>(this, IssueType.DELETED, code); 691 if ("too-long".equals(codeString)) 692 return new Enumeration<IssueType>(this, IssueType.TOOLONG, code); 693 if ("code-invalid".equals(codeString)) 694 return new Enumeration<IssueType>(this, IssueType.CODEINVALID, code); 695 if ("extension".equals(codeString)) 696 return new Enumeration<IssueType>(this, IssueType.EXTENSION, code); 697 if ("too-costly".equals(codeString)) 698 return new Enumeration<IssueType>(this, IssueType.TOOCOSTLY, code); 699 if ("business-rule".equals(codeString)) 700 return new Enumeration<IssueType>(this, IssueType.BUSINESSRULE, code); 701 if ("conflict".equals(codeString)) 702 return new Enumeration<IssueType>(this, IssueType.CONFLICT, code); 703 if ("limited-filter".equals(codeString)) 704 return new Enumeration<IssueType>(this, IssueType.LIMITEDFILTER, code); 705 if ("transient".equals(codeString)) 706 return new Enumeration<IssueType>(this, IssueType.TRANSIENT, code); 707 if ("lock-error".equals(codeString)) 708 return new Enumeration<IssueType>(this, IssueType.LOCKERROR, code); 709 if ("no-store".equals(codeString)) 710 return new Enumeration<IssueType>(this, IssueType.NOSTORE, code); 711 if ("exception".equals(codeString)) 712 return new Enumeration<IssueType>(this, IssueType.EXCEPTION, code); 713 if ("timeout".equals(codeString)) 714 return new Enumeration<IssueType>(this, IssueType.TIMEOUT, code); 715 if ("incomplete".equals(codeString)) 716 return new Enumeration<IssueType>(this, IssueType.INCOMPLETE, code); 717 if ("throttled".equals(codeString)) 718 return new Enumeration<IssueType>(this, IssueType.THROTTLED, code); 719 if ("informational".equals(codeString)) 720 return new Enumeration<IssueType>(this, IssueType.INFORMATIONAL, code); 721 if ("success".equals(codeString)) 722 return new Enumeration<IssueType>(this, IssueType.SUCCESS, code); 723 throw new FHIRException("Unknown IssueType code '"+codeString+"'"); 724 } 725 public String toCode(IssueType code) { 726 if (code == IssueType.NULL) 727 return null; 728 if (code == IssueType.INVALID) 729 return "invalid"; 730 if (code == IssueType.STRUCTURE) 731 return "structure"; 732 if (code == IssueType.REQUIRED) 733 return "required"; 734 if (code == IssueType.VALUE) 735 return "value"; 736 if (code == IssueType.INVARIANT) 737 return "invariant"; 738 if (code == IssueType.SECURITY) 739 return "security"; 740 if (code == IssueType.LOGIN) 741 return "login"; 742 if (code == IssueType.UNKNOWN) 743 return "unknown"; 744 if (code == IssueType.EXPIRED) 745 return "expired"; 746 if (code == IssueType.FORBIDDEN) 747 return "forbidden"; 748 if (code == IssueType.SUPPRESSED) 749 return "suppressed"; 750 if (code == IssueType.PROCESSING) 751 return "processing"; 752 if (code == IssueType.NOTSUPPORTED) 753 return "not-supported"; 754 if (code == IssueType.DUPLICATE) 755 return "duplicate"; 756 if (code == IssueType.MULTIPLEMATCHES) 757 return "multiple-matches"; 758 if (code == IssueType.NOTFOUND) 759 return "not-found"; 760 if (code == IssueType.DELETED) 761 return "deleted"; 762 if (code == IssueType.TOOLONG) 763 return "too-long"; 764 if (code == IssueType.CODEINVALID) 765 return "code-invalid"; 766 if (code == IssueType.EXTENSION) 767 return "extension"; 768 if (code == IssueType.TOOCOSTLY) 769 return "too-costly"; 770 if (code == IssueType.BUSINESSRULE) 771 return "business-rule"; 772 if (code == IssueType.CONFLICT) 773 return "conflict"; 774 if (code == IssueType.LIMITEDFILTER) 775 return "limited-filter"; 776 if (code == IssueType.TRANSIENT) 777 return "transient"; 778 if (code == IssueType.LOCKERROR) 779 return "lock-error"; 780 if (code == IssueType.NOSTORE) 781 return "no-store"; 782 if (code == IssueType.EXCEPTION) 783 return "exception"; 784 if (code == IssueType.TIMEOUT) 785 return "timeout"; 786 if (code == IssueType.INCOMPLETE) 787 return "incomplete"; 788 if (code == IssueType.THROTTLED) 789 return "throttled"; 790 if (code == IssueType.INFORMATIONAL) 791 return "informational"; 792 if (code == IssueType.SUCCESS) 793 return "success"; 794 return "?"; 795 } 796 public String toSystem(IssueType code) { 797 return code.getSystem(); 798 } 799 } 800 801 @Block() 802 public static class OperationOutcomeIssueComponent extends BackboneElement implements IBaseBackboneElement { 803 /** 804 * Indicates whether the issue indicates a variation from successful processing. 805 */ 806 @Child(name = "severity", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 807 @Description(shortDefinition="fatal | error | warning | information | success", formalDefinition="Indicates whether the issue indicates a variation from successful processing." ) 808 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/issue-severity") 809 protected Enumeration<IssueSeverity> severity; 810 811 /** 812 * Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element. 813 */ 814 @Child(name = "code", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 815 @Description(shortDefinition="Error or warning code", formalDefinition="Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element." ) 816 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/issue-type") 817 protected Enumeration<IssueType> code; 818 819 /** 820 * Additional details about the error. This may be a text description of the error or a system code that identifies the error. 821 */ 822 @Child(name = "details", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 823 @Description(shortDefinition="Additional details about the error", formalDefinition="Additional details about the error. This may be a text description of the error or a system code that identifies the error." ) 824 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-outcome") 825 protected CodeableConcept details; 826 827 /** 828 * Additional diagnostic information about the issue. 829 */ 830 @Child(name = "diagnostics", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 831 @Description(shortDefinition="Additional diagnostic information about the issue", formalDefinition="Additional diagnostic information about the issue." ) 832 protected StringType diagnostics; 833 834 /** 835 * This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. 836 837For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name. 838 */ 839 @Child(name = "location", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 840 @Description(shortDefinition="Deprecated: Path of element(s) related to issue", formalDefinition="This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. \n\nFor resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name." ) 841 protected List<StringType> location; 842 843 /** 844 * A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. 845 */ 846 @Child(name = "expression", type = {StringType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 847 @Description(shortDefinition="FHIRPath of element(s) related to issue", formalDefinition="A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised." ) 848 protected List<StringType> expression; 849 850 private static final long serialVersionUID = -1681095438L; 851 852 /** 853 * Constructor 854 */ 855 public OperationOutcomeIssueComponent() { 856 super(); 857 } 858 859 /** 860 * Constructor 861 */ 862 public OperationOutcomeIssueComponent(IssueSeverity severity, IssueType code) { 863 super(); 864 this.setSeverity(severity); 865 this.setCode(code); 866 } 867 868 /** 869 * @return {@link #severity} (Indicates whether the issue indicates a variation from successful processing.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 870 */ 871 public Enumeration<IssueSeverity> getSeverityElement() { 872 if (this.severity == null) 873 if (Configuration.errorOnAutoCreate()) 874 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.severity"); 875 else if (Configuration.doAutoCreate()) 876 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); // bb 877 return this.severity; 878 } 879 880 public boolean hasSeverityElement() { 881 return this.severity != null && !this.severity.isEmpty(); 882 } 883 884 public boolean hasSeverity() { 885 return this.severity != null && !this.severity.isEmpty(); 886 } 887 888 /** 889 * @param value {@link #severity} (Indicates whether the issue indicates a variation from successful processing.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 890 */ 891 public OperationOutcomeIssueComponent setSeverityElement(Enumeration<IssueSeverity> value) { 892 this.severity = value; 893 return this; 894 } 895 896 /** 897 * @return Indicates whether the issue indicates a variation from successful processing. 898 */ 899 public IssueSeverity getSeverity() { 900 return this.severity == null ? null : this.severity.getValue(); 901 } 902 903 /** 904 * @param value Indicates whether the issue indicates a variation from successful processing. 905 */ 906 public OperationOutcomeIssueComponent setSeverity(IssueSeverity value) { 907 if (this.severity == null) 908 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); 909 this.severity.setValue(value); 910 return this; 911 } 912 913 /** 914 * @return {@link #code} (Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 915 */ 916 public Enumeration<IssueType> getCodeElement() { 917 if (this.code == null) 918 if (Configuration.errorOnAutoCreate()) 919 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.code"); 920 else if (Configuration.doAutoCreate()) 921 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); // bb 922 return this.code; 923 } 924 925 public boolean hasCodeElement() { 926 return this.code != null && !this.code.isEmpty(); 927 } 928 929 public boolean hasCode() { 930 return this.code != null && !this.code.isEmpty(); 931 } 932 933 /** 934 * @param value {@link #code} (Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 935 */ 936 public OperationOutcomeIssueComponent setCodeElement(Enumeration<IssueType> value) { 937 this.code = value; 938 return this; 939 } 940 941 /** 942 * @return Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element. 943 */ 944 public IssueType getCode() { 945 return this.code == null ? null : this.code.getValue(); 946 } 947 948 /** 949 * @param value Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element. 950 */ 951 public OperationOutcomeIssueComponent setCode(IssueType value) { 952 if (this.code == null) 953 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); 954 this.code.setValue(value); 955 return this; 956 } 957 958 /** 959 * @return {@link #details} (Additional details about the error. This may be a text description of the error or a system code that identifies the error.) 960 */ 961 public CodeableConcept getDetails() { 962 if (this.details == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.details"); 965 else if (Configuration.doAutoCreate()) 966 this.details = new CodeableConcept(); // cc 967 return this.details; 968 } 969 970 public boolean hasDetails() { 971 return this.details != null && !this.details.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #details} (Additional details about the error. This may be a text description of the error or a system code that identifies the error.) 976 */ 977 public OperationOutcomeIssueComponent setDetails(CodeableConcept value) { 978 this.details = value; 979 return this; 980 } 981 982 /** 983 * @return {@link #diagnostics} (Additional diagnostic information about the issue.). This is the underlying object with id, value and extensions. The accessor "getDiagnostics" gives direct access to the value 984 */ 985 public StringType getDiagnosticsElement() { 986 if (this.diagnostics == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.diagnostics"); 989 else if (Configuration.doAutoCreate()) 990 this.diagnostics = new StringType(); // bb 991 return this.diagnostics; 992 } 993 994 public boolean hasDiagnosticsElement() { 995 return this.diagnostics != null && !this.diagnostics.isEmpty(); 996 } 997 998 public boolean hasDiagnostics() { 999 return this.diagnostics != null && !this.diagnostics.isEmpty(); 1000 } 1001 1002 /** 1003 * @param value {@link #diagnostics} (Additional diagnostic information about the issue.). This is the underlying object with id, value and extensions. The accessor "getDiagnostics" gives direct access to the value 1004 */ 1005 public OperationOutcomeIssueComponent setDiagnosticsElement(StringType value) { 1006 this.diagnostics = value; 1007 return this; 1008 } 1009 1010 /** 1011 * @return Additional diagnostic information about the issue. 1012 */ 1013 public String getDiagnostics() { 1014 return this.diagnostics == null ? null : this.diagnostics.getValue(); 1015 } 1016 1017 /** 1018 * @param value Additional diagnostic information about the issue. 1019 */ 1020 public OperationOutcomeIssueComponent setDiagnostics(String value) { 1021 if (Utilities.noString(value)) 1022 this.diagnostics = null; 1023 else { 1024 if (this.diagnostics == null) 1025 this.diagnostics = new StringType(); 1026 this.diagnostics.setValue(value); 1027 } 1028 return this; 1029 } 1030 1031 /** 1032 * @return {@link #location} (This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. 1033 1034For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 1035 */ 1036 public List<StringType> getLocation() { 1037 if (this.location == null) 1038 this.location = new ArrayList<StringType>(); 1039 return this.location; 1040 } 1041 1042 /** 1043 * @return Returns a reference to <code>this</code> for easy method chaining 1044 */ 1045 public OperationOutcomeIssueComponent setLocation(List<StringType> theLocation) { 1046 this.location = theLocation; 1047 return this; 1048 } 1049 1050 public boolean hasLocation() { 1051 if (this.location == null) 1052 return false; 1053 for (StringType item : this.location) 1054 if (!item.isEmpty()) 1055 return true; 1056 return false; 1057 } 1058 1059 /** 1060 * @return {@link #location} (This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. 1061 1062For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 1063 */ 1064 public StringType addLocationElement() {//2 1065 StringType t = new StringType(); 1066 if (this.location == null) 1067 this.location = new ArrayList<StringType>(); 1068 this.location.add(t); 1069 return t; 1070 } 1071 1072 /** 1073 * @param value {@link #location} (This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. 1074 1075For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 1076 */ 1077 public OperationOutcomeIssueComponent addLocation(String value) { //1 1078 StringType t = new StringType(); 1079 t.setValue(value); 1080 if (this.location == null) 1081 this.location = new ArrayList<StringType>(); 1082 this.location.add(t); 1083 return this; 1084 } 1085 1086 /** 1087 * @param value {@link #location} (This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. 1088 1089For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 1090 */ 1091 public boolean hasLocation(String value) { 1092 if (this.location == null) 1093 return false; 1094 for (StringType v : this.location) 1095 if (v.getValue().equals(value)) // string 1096 return true; 1097 return false; 1098 } 1099 1100 /** 1101 * @return {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 1102 */ 1103 public List<StringType> getExpression() { 1104 if (this.expression == null) 1105 this.expression = new ArrayList<StringType>(); 1106 return this.expression; 1107 } 1108 1109 /** 1110 * @return Returns a reference to <code>this</code> for easy method chaining 1111 */ 1112 public OperationOutcomeIssueComponent setExpression(List<StringType> theExpression) { 1113 this.expression = theExpression; 1114 return this; 1115 } 1116 1117 public boolean hasExpression() { 1118 if (this.expression == null) 1119 return false; 1120 for (StringType item : this.expression) 1121 if (!item.isEmpty()) 1122 return true; 1123 return false; 1124 } 1125 1126 /** 1127 * @return {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 1128 */ 1129 public StringType addExpressionElement() {//2 1130 StringType t = new StringType(); 1131 if (this.expression == null) 1132 this.expression = new ArrayList<StringType>(); 1133 this.expression.add(t); 1134 return t; 1135 } 1136 1137 /** 1138 * @param value {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 1139 */ 1140 public OperationOutcomeIssueComponent addExpression(String value) { //1 1141 StringType t = new StringType(); 1142 t.setValue(value); 1143 if (this.expression == null) 1144 this.expression = new ArrayList<StringType>(); 1145 this.expression.add(t); 1146 return this; 1147 } 1148 1149 /** 1150 * @param value {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 1151 */ 1152 public boolean hasExpression(String value) { 1153 if (this.expression == null) 1154 return false; 1155 for (StringType v : this.expression) 1156 if (v.getValue().equals(value)) // string 1157 return true; 1158 return false; 1159 } 1160 1161 protected void listChildren(List<Property> children) { 1162 super.listChildren(children); 1163 children.add(new Property("severity", "code", "Indicates whether the issue indicates a variation from successful processing.", 0, 1, severity)); 1164 children.add(new Property("code", "code", "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.", 0, 1, code)); 1165 children.add(new Property("details", "CodeableConcept", "Additional details about the error. This may be a text description of the error or a system code that identifies the error.", 0, 1, details)); 1166 children.add(new Property("diagnostics", "string", "Additional diagnostic information about the issue.", 0, 1, diagnostics)); 1167 children.add(new Property("location", "string", "This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. \n\nFor resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name.", 0, java.lang.Integer.MAX_VALUE, location)); 1168 children.add(new Property("expression", "string", "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression)); 1169 } 1170 1171 @Override 1172 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1173 switch (_hash) { 1174 case 1478300413: /*severity*/ return new Property("severity", "code", "Indicates whether the issue indicates a variation from successful processing.", 0, 1, severity); 1175 case 3059181: /*code*/ return new Property("code", "code", "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.", 0, 1, code); 1176 case 1557721666: /*details*/ return new Property("details", "CodeableConcept", "Additional details about the error. This may be a text description of the error or a system code that identifies the error.", 0, 1, details); 1177 case -740386388: /*diagnostics*/ return new Property("diagnostics", "string", "Additional diagnostic information about the issue.", 0, 1, diagnostics); 1178 case 1901043637: /*location*/ return new Property("location", "string", "This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. \n\nFor resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name.", 0, java.lang.Integer.MAX_VALUE, location); 1179 case -1795452264: /*expression*/ return new Property("expression", "string", "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression); 1180 default: return super.getNamedProperty(_hash, _name, _checkValid); 1181 } 1182 1183 } 1184 1185 @Override 1186 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1187 switch (hash) { 1188 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<IssueSeverity> 1189 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<IssueType> 1190 case 1557721666: /*details*/ return this.details == null ? new Base[0] : new Base[] {this.details}; // CodeableConcept 1191 case -740386388: /*diagnostics*/ return this.diagnostics == null ? new Base[0] : new Base[] {this.diagnostics}; // StringType 1192 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // StringType 1193 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : this.expression.toArray(new Base[this.expression.size()]); // StringType 1194 default: return super.getProperty(hash, name, checkValid); 1195 } 1196 1197 } 1198 1199 @Override 1200 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1201 switch (hash) { 1202 case 1478300413: // severity 1203 value = new IssueSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1204 this.severity = (Enumeration) value; // Enumeration<IssueSeverity> 1205 return value; 1206 case 3059181: // code 1207 value = new IssueTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1208 this.code = (Enumeration) value; // Enumeration<IssueType> 1209 return value; 1210 case 1557721666: // details 1211 this.details = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1212 return value; 1213 case -740386388: // diagnostics 1214 this.diagnostics = TypeConvertor.castToString(value); // StringType 1215 return value; 1216 case 1901043637: // location 1217 this.getLocation().add(TypeConvertor.castToString(value)); // StringType 1218 return value; 1219 case -1795452264: // expression 1220 this.getExpression().add(TypeConvertor.castToString(value)); // StringType 1221 return value; 1222 default: return super.setProperty(hash, name, value); 1223 } 1224 1225 } 1226 1227 @Override 1228 public Base setProperty(String name, Base value) throws FHIRException { 1229 if (name.equals("severity")) { 1230 value = new IssueSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1231 this.severity = (Enumeration) value; // Enumeration<IssueSeverity> 1232 } else if (name.equals("code")) { 1233 value = new IssueTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1234 this.code = (Enumeration) value; // Enumeration<IssueType> 1235 } else if (name.equals("details")) { 1236 this.details = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1237 } else if (name.equals("diagnostics")) { 1238 this.diagnostics = TypeConvertor.castToString(value); // StringType 1239 } else if (name.equals("location")) { 1240 this.getLocation().add(TypeConvertor.castToString(value)); 1241 } else if (name.equals("expression")) { 1242 this.getExpression().add(TypeConvertor.castToString(value)); 1243 } else 1244 return super.setProperty(name, value); 1245 return value; 1246 } 1247 1248 @Override 1249 public void removeChild(String name, Base value) throws FHIRException { 1250 if (name.equals("severity")) { 1251 value = new IssueSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1252 this.severity = (Enumeration) value; // Enumeration<IssueSeverity> 1253 } else if (name.equals("code")) { 1254 value = new IssueTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1255 this.code = (Enumeration) value; // Enumeration<IssueType> 1256 } else if (name.equals("details")) { 1257 this.details = null; 1258 } else if (name.equals("diagnostics")) { 1259 this.diagnostics = null; 1260 } else if (name.equals("location")) { 1261 this.getLocation().remove(value); 1262 } else if (name.equals("expression")) { 1263 this.getExpression().remove(value); 1264 } else 1265 super.removeChild(name, value); 1266 1267 } 1268 1269 @Override 1270 public Base makeProperty(int hash, String name) throws FHIRException { 1271 switch (hash) { 1272 case 1478300413: return getSeverityElement(); 1273 case 3059181: return getCodeElement(); 1274 case 1557721666: return getDetails(); 1275 case -740386388: return getDiagnosticsElement(); 1276 case 1901043637: return addLocationElement(); 1277 case -1795452264: return addExpressionElement(); 1278 default: return super.makeProperty(hash, name); 1279 } 1280 1281 } 1282 1283 @Override 1284 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1285 switch (hash) { 1286 case 1478300413: /*severity*/ return new String[] {"code"}; 1287 case 3059181: /*code*/ return new String[] {"code"}; 1288 case 1557721666: /*details*/ return new String[] {"CodeableConcept"}; 1289 case -740386388: /*diagnostics*/ return new String[] {"string"}; 1290 case 1901043637: /*location*/ return new String[] {"string"}; 1291 case -1795452264: /*expression*/ return new String[] {"string"}; 1292 default: return super.getTypesForProperty(hash, name); 1293 } 1294 1295 } 1296 1297 @Override 1298 public Base addChild(String name) throws FHIRException { 1299 if (name.equals("severity")) { 1300 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.issue.severity"); 1301 } 1302 else if (name.equals("code")) { 1303 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.issue.code"); 1304 } 1305 else if (name.equals("details")) { 1306 this.details = new CodeableConcept(); 1307 return this.details; 1308 } 1309 else if (name.equals("diagnostics")) { 1310 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.issue.diagnostics"); 1311 } 1312 else if (name.equals("location")) { 1313 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.issue.location"); 1314 } 1315 else if (name.equals("expression")) { 1316 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.issue.expression"); 1317 } 1318 else 1319 return super.addChild(name); 1320 } 1321 1322 public OperationOutcomeIssueComponent copy() { 1323 OperationOutcomeIssueComponent dst = new OperationOutcomeIssueComponent(); 1324 copyValues(dst); 1325 return dst; 1326 } 1327 1328 public void copyValues(OperationOutcomeIssueComponent dst) { 1329 super.copyValues(dst); 1330 dst.severity = severity == null ? null : severity.copy(); 1331 dst.code = code == null ? null : code.copy(); 1332 dst.details = details == null ? null : details.copy(); 1333 dst.diagnostics = diagnostics == null ? null : diagnostics.copy(); 1334 if (location != null) { 1335 dst.location = new ArrayList<StringType>(); 1336 for (StringType i : location) 1337 dst.location.add(i.copy()); 1338 }; 1339 if (expression != null) { 1340 dst.expression = new ArrayList<StringType>(); 1341 for (StringType i : expression) 1342 dst.expression.add(i.copy()); 1343 }; 1344 } 1345 1346 @Override 1347 public boolean equalsDeep(Base other_) { 1348 if (!super.equalsDeep(other_)) 1349 return false; 1350 if (!(other_ instanceof OperationOutcomeIssueComponent)) 1351 return false; 1352 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other_; 1353 return compareDeep(severity, o.severity, true) && compareDeep(code, o.code, true) && compareDeep(details, o.details, true) 1354 && compareDeep(diagnostics, o.diagnostics, true) && compareDeep(location, o.location, true) && compareDeep(expression, o.expression, true) 1355 ; 1356 } 1357 1358 @Override 1359 public boolean equalsShallow(Base other_) { 1360 if (!super.equalsShallow(other_)) 1361 return false; 1362 if (!(other_ instanceof OperationOutcomeIssueComponent)) 1363 return false; 1364 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other_; 1365 return compareValues(severity, o.severity, true) && compareValues(code, o.code, true) && compareValues(diagnostics, o.diagnostics, true) 1366 && compareValues(location, o.location, true) && compareValues(expression, o.expression, true); 1367 } 1368 1369 public boolean isEmpty() { 1370 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(severity, code, details 1371 , diagnostics, location, expression); 1372 } 1373 1374 public String fhirType() { 1375 return "OperationOutcome.issue"; 1376 1377 } 1378 1379 // added from java-adornments.txt: 1380 @Override 1381 public String toString() { 1382 String srvr = hasExtension(ToolingExtensions.EXT_ISSUE_SERVER) ? " (from "+getExtensionString(ToolingExtensions.EXT_ISSUE_SERVER)+")" : ""; 1383 if (getExpression().size() == 1) { 1384 return getSeverity().toCode()+"/"+getCode().toCode()+" @ "+getExpression().get(0)+(hasDiagnostics() ? " "+getDiagnostics() : "")+": "+getDetails().getText()+srvr; 1385 } else { 1386 return getSeverity().toCode()+"/"+getCode().toCode()+" @ "+getExpression()+(hasDiagnostics() ? " "+getDiagnostics() : "")+": "+getDetails().getText()+srvr; 1387 } 1388 } 1389 1390 public boolean isWarningOrMore() { 1391 switch (getSeverity()) { 1392 case FATAL: return true; 1393 case ERROR: return true; 1394 case WARNING: return true; 1395 case INFORMATION: return false; 1396 case SUCCESS: return false; 1397 case NULL: return false; 1398 default: return false; 1399 } 1400 } 1401 public boolean isInformationorLess() { 1402 switch (getSeverity()) { 1403 case FATAL: return false; 1404 case ERROR: return true; 1405 case WARNING: return false; 1406 case INFORMATION: return true; 1407 case SUCCESS: return true; 1408 case NULL: return true; 1409 default: return false; 1410 } 1411 } 1412 1413 public List<StringType> getExpressionOrLocation() { 1414 return hasExpression() ? getExpression() : getLocation(); 1415 } 1416 1417 public boolean hasExpressionOrLocation() { 1418 return hasExpression() || hasLocation(); 1419 } 1420 1421 public void resetPath(String root, String newRoot) { 1422 for (StringType st : getLocation()) { 1423 if (st.hasValue() && st.getValue().startsWith(root+".")) { 1424 st.setValue(newRoot+st.getValue().substring(root.length())); 1425 } 1426 } 1427 for (StringType st : getExpression()) { 1428 if (st.hasValue() && st.getValue().startsWith(root+".")) { 1429 st.setValue(newRoot+st.getValue().substring(root.length())); 1430 } 1431 } 1432 } 1433 1434 public String getText() { 1435 if (getDetails().hasText()) { 1436 return getDetails().getText(); 1437 } 1438 if (hasDiagnostics()) { 1439 return getDiagnostics(); 1440 } 1441 return null; 1442 } 1443 // end addition 1444 } 1445 1446 /** 1447 * An error, warning, or information message that results from a system action. 1448 */ 1449 @Child(name = "issue", type = {}, order=0, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1450 @Description(shortDefinition="A single issue associated with the action", formalDefinition="An error, warning, or information message that results from a system action." ) 1451 protected List<OperationOutcomeIssueComponent> issue; 1452 1453 private static final long serialVersionUID = -152150052L; 1454 1455 /** 1456 * Constructor 1457 */ 1458 public OperationOutcome() { 1459 super(); 1460 } 1461 1462 /** 1463 * Constructor 1464 */ 1465 public OperationOutcome(OperationOutcomeIssueComponent issue) { 1466 super(); 1467 this.addIssue(issue); 1468 } 1469 1470 /** 1471 * @return {@link #issue} (An error, warning, or information message that results from a system action.) 1472 */ 1473 public List<OperationOutcomeIssueComponent> getIssue() { 1474 if (this.issue == null) 1475 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1476 return this.issue; 1477 } 1478 1479 /** 1480 * @return Returns a reference to <code>this</code> for easy method chaining 1481 */ 1482 public OperationOutcome setIssue(List<OperationOutcomeIssueComponent> theIssue) { 1483 this.issue = theIssue; 1484 return this; 1485 } 1486 1487 public boolean hasIssue() { 1488 if (this.issue == null) 1489 return false; 1490 for (OperationOutcomeIssueComponent item : this.issue) 1491 if (!item.isEmpty()) 1492 return true; 1493 return false; 1494 } 1495 1496 public OperationOutcomeIssueComponent addIssue() { //3 1497 OperationOutcomeIssueComponent t = new OperationOutcomeIssueComponent(); 1498 if (this.issue == null) 1499 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1500 this.issue.add(t); 1501 return t; 1502 } 1503 1504 public OperationOutcome addIssue(OperationOutcomeIssueComponent t) { //3 1505 if (t == null) 1506 return this; 1507 if (this.issue == null) 1508 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1509 this.issue.add(t); 1510 return this; 1511 } 1512 1513 /** 1514 * @return The first repetition of repeating field {@link #issue}, creating it if it does not already exist {3} 1515 */ 1516 public OperationOutcomeIssueComponent getIssueFirstRep() { 1517 if (getIssue().isEmpty()) { 1518 addIssue(); 1519 } 1520 return getIssue().get(0); 1521 } 1522 1523 protected void listChildren(List<Property> children) { 1524 super.listChildren(children); 1525 children.add(new Property("issue", "", "An error, warning, or information message that results from a system action.", 0, java.lang.Integer.MAX_VALUE, issue)); 1526 } 1527 1528 @Override 1529 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1530 switch (_hash) { 1531 case 100509913: /*issue*/ return new Property("issue", "", "An error, warning, or information message that results from a system action.", 0, java.lang.Integer.MAX_VALUE, issue); 1532 default: return super.getNamedProperty(_hash, _name, _checkValid); 1533 } 1534 1535 } 1536 1537 @Override 1538 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1539 switch (hash) { 1540 case 100509913: /*issue*/ return this.issue == null ? new Base[0] : this.issue.toArray(new Base[this.issue.size()]); // OperationOutcomeIssueComponent 1541 default: return super.getProperty(hash, name, checkValid); 1542 } 1543 1544 } 1545 1546 @Override 1547 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1548 switch (hash) { 1549 case 100509913: // issue 1550 this.getIssue().add((OperationOutcomeIssueComponent) value); // OperationOutcomeIssueComponent 1551 return value; 1552 default: return super.setProperty(hash, name, value); 1553 } 1554 1555 } 1556 1557 @Override 1558 public Base setProperty(String name, Base value) throws FHIRException { 1559 if (name.equals("issue")) { 1560 this.getIssue().add((OperationOutcomeIssueComponent) value); 1561 } else 1562 return super.setProperty(name, value); 1563 return value; 1564 } 1565 1566 @Override 1567 public void removeChild(String name, Base value) throws FHIRException { 1568 if (name.equals("issue")) { 1569 this.getIssue().remove((OperationOutcomeIssueComponent) value); 1570 } else 1571 super.removeChild(name, value); 1572 1573 } 1574 1575 @Override 1576 public Base makeProperty(int hash, String name) throws FHIRException { 1577 switch (hash) { 1578 case 100509913: return addIssue(); 1579 default: return super.makeProperty(hash, name); 1580 } 1581 1582 } 1583 1584 @Override 1585 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1586 switch (hash) { 1587 case 100509913: /*issue*/ return new String[] {}; 1588 default: return super.getTypesForProperty(hash, name); 1589 } 1590 1591 } 1592 1593 @Override 1594 public Base addChild(String name) throws FHIRException { 1595 if (name.equals("issue")) { 1596 return addIssue(); 1597 } 1598 else 1599 return super.addChild(name); 1600 } 1601 1602 public String fhirType() { 1603 return "OperationOutcome"; 1604 1605 } 1606 1607 public OperationOutcome copy() { 1608 OperationOutcome dst = new OperationOutcome(); 1609 copyValues(dst); 1610 return dst; 1611 } 1612 1613 public void copyValues(OperationOutcome dst) { 1614 super.copyValues(dst); 1615 if (issue != null) { 1616 dst.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1617 for (OperationOutcomeIssueComponent i : issue) 1618 dst.issue.add(i.copy()); 1619 }; 1620 } 1621 1622 protected OperationOutcome typedCopy() { 1623 return copy(); 1624 } 1625 1626 @Override 1627 public boolean equalsDeep(Base other_) { 1628 if (!super.equalsDeep(other_)) 1629 return false; 1630 if (!(other_ instanceof OperationOutcome)) 1631 return false; 1632 OperationOutcome o = (OperationOutcome) other_; 1633 return compareDeep(issue, o.issue, true); 1634 } 1635 1636 @Override 1637 public boolean equalsShallow(Base other_) { 1638 if (!super.equalsShallow(other_)) 1639 return false; 1640 if (!(other_ instanceof OperationOutcome)) 1641 return false; 1642 OperationOutcome o = (OperationOutcome) other_; 1643 return true; 1644 } 1645 1646 public boolean isEmpty() { 1647 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(issue); 1648 } 1649 1650 @Override 1651 public ResourceType getResourceType() { 1652 return ResourceType.OperationOutcome; 1653 } 1654 1655// Manual code (from Configuration.txt): 1656 public boolean supportsCopyright() { 1657 return true; 1658 } 1659 1660 1661 public boolean isSuccess() { 1662 for (OperationOutcomeIssueComponent iss : getIssue()) { 1663 if (iss.isWarningOrMore() || iss.getCode() != IssueType.INFORMATIONAL) { 1664 return false; 1665 } 1666 if (iss.isInformationorLess() || iss.getCode() != IssueType.INFORMATIONAL) { 1667 return true; 1668 } 1669 } 1670 return false; 1671 } 1672 1673// end addition 1674 1675} 1676