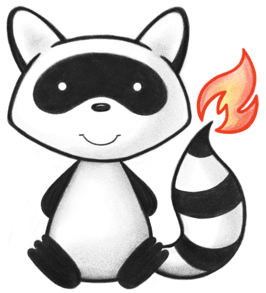
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Tue, May 4, 2021 07:17+1000 for FHIR v5.0.0-snapshot2 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Base StructureDefinition for OrderedDistribution Type: An ordered list (distribution) of statistics. 050 */ 051@DatatypeDef(name="OrderedDistribution") 052public class OrderedDistribution extends BackboneType implements ICompositeType { 053 054 @Block() 055 public static class OrderedDistributionIntervalComponent extends Element implements IBaseDatatypeElement { 056 /** 057 * Relative order of interval. 058 */ 059 @Child(name = "rankOrder", type = {IntegerType.class}, order=1, min=1, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="Relative order of interval", formalDefinition="Relative order of interval." ) 061 protected IntegerType rankOrder; 062 063 /** 064 * Values and parameters for a single statistic related to the interval. 065 */ 066 @Child(name = "intervalStatistic", type = {Statistic.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 067 @Description(shortDefinition="Values and parameters for a single statistic related to the interval", formalDefinition="Values and parameters for a single statistic related to the interval." ) 068 protected List<Statistic> intervalStatistic; 069 070 private static final long serialVersionUID = 1714817635L; 071 072 /** 073 * Constructor 074 */ 075 public OrderedDistributionIntervalComponent() { 076 super(); 077 } 078 079 /** 080 * Constructor 081 */ 082 public OrderedDistributionIntervalComponent(int rankOrder) { 083 super(); 084 this.setRankOrder(rankOrder); 085 } 086 087 /** 088 * @return {@link #rankOrder} (Relative order of interval.). This is the underlying object with id, value and extensions. The accessor "getRankOrder" gives direct access to the value 089 */ 090 public IntegerType getRankOrderElement() { 091 if (this.rankOrder == null) 092 if (Configuration.errorOnAutoCreate()) 093 throw new Error("Attempt to auto-create OrderedDistributionIntervalComponent.rankOrder"); 094 else if (Configuration.doAutoCreate()) 095 this.rankOrder = new IntegerType(); // bb 096 return this.rankOrder; 097 } 098 099 public boolean hasRankOrderElement() { 100 return this.rankOrder != null && !this.rankOrder.isEmpty(); 101 } 102 103 public boolean hasRankOrder() { 104 return this.rankOrder != null && !this.rankOrder.isEmpty(); 105 } 106 107 /** 108 * @param value {@link #rankOrder} (Relative order of interval.). This is the underlying object with id, value and extensions. The accessor "getRankOrder" gives direct access to the value 109 */ 110 public OrderedDistributionIntervalComponent setRankOrderElement(IntegerType value) { 111 this.rankOrder = value; 112 return this; 113 } 114 115 /** 116 * @return Relative order of interval. 117 */ 118 public int getRankOrder() { 119 return this.rankOrder == null || this.rankOrder.isEmpty() ? 0 : this.rankOrder.getValue(); 120 } 121 122 /** 123 * @param value Relative order of interval. 124 */ 125 public OrderedDistributionIntervalComponent setRankOrder(int value) { 126 if (this.rankOrder == null) 127 this.rankOrder = new IntegerType(); 128 this.rankOrder.setValue(value); 129 return this; 130 } 131 132 /** 133 * @return {@link #intervalStatistic} (Values and parameters for a single statistic related to the interval.) 134 */ 135 public List<Statistic> getIntervalStatistic() { 136 if (this.intervalStatistic == null) 137 this.intervalStatistic = new ArrayList<Statistic>(); 138 return this.intervalStatistic; 139 } 140 141 /** 142 * @return Returns a reference to <code>this</code> for easy method chaining 143 */ 144 public OrderedDistributionIntervalComponent setIntervalStatistic(List<Statistic> theIntervalStatistic) { 145 this.intervalStatistic = theIntervalStatistic; 146 return this; 147 } 148 149 public boolean hasIntervalStatistic() { 150 if (this.intervalStatistic == null) 151 return false; 152 for (Statistic item : this.intervalStatistic) 153 if (!item.isEmpty()) 154 return true; 155 return false; 156 } 157 158 public Statistic addIntervalStatistic() { //3 159 Statistic t = new Statistic(); 160 if (this.intervalStatistic == null) 161 this.intervalStatistic = new ArrayList<Statistic>(); 162 this.intervalStatistic.add(t); 163 return t; 164 } 165 166 public OrderedDistributionIntervalComponent addIntervalStatistic(Statistic t) { //3 167 if (t == null) 168 return this; 169 if (this.intervalStatistic == null) 170 this.intervalStatistic = new ArrayList<Statistic>(); 171 this.intervalStatistic.add(t); 172 return this; 173 } 174 175 /** 176 * @return The first repetition of repeating field {@link #intervalStatistic}, creating it if it does not already exist {3} 177 */ 178 public Statistic getIntervalStatisticFirstRep() { 179 if (getIntervalStatistic().isEmpty()) { 180 addIntervalStatistic(); 181 } 182 return getIntervalStatistic().get(0); 183 } 184 185 protected void listChildren(List<Property> children) { 186 super.listChildren(children); 187 children.add(new Property("rankOrder", "integer", "Relative order of interval.", 0, 1, rankOrder)); 188 children.add(new Property("intervalStatistic", "Statistic", "Values and parameters for a single statistic related to the interval.", 0, java.lang.Integer.MAX_VALUE, intervalStatistic)); 189 } 190 191 @Override 192 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 193 switch (_hash) { 194 case -656537982: /*rankOrder*/ return new Property("rankOrder", "integer", "Relative order of interval.", 0, 1, rankOrder); 195 case 227099147: /*intervalStatistic*/ return new Property("intervalStatistic", "Statistic", "Values and parameters for a single statistic related to the interval.", 0, java.lang.Integer.MAX_VALUE, intervalStatistic); 196 default: return super.getNamedProperty(_hash, _name, _checkValid); 197 } 198 199 } 200 201 @Override 202 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 203 switch (hash) { 204 case -656537982: /*rankOrder*/ return this.rankOrder == null ? new Base[0] : new Base[] {this.rankOrder}; // IntegerType 205 case 227099147: /*intervalStatistic*/ return this.intervalStatistic == null ? new Base[0] : this.intervalStatistic.toArray(new Base[this.intervalStatistic.size()]); // Statistic 206 default: return super.getProperty(hash, name, checkValid); 207 } 208 209 } 210 211 @Override 212 public Base setProperty(int hash, String name, Base value) throws FHIRException { 213 switch (hash) { 214 case -656537982: // rankOrder 215 this.rankOrder = TypeConvertor.castToInteger(value); // IntegerType 216 return value; 217 case 227099147: // intervalStatistic 218 this.getIntervalStatistic().add(TypeConvertor.castToStatistic(value)); // Statistic 219 return value; 220 default: return super.setProperty(hash, name, value); 221 } 222 223 } 224 225 @Override 226 public Base setProperty(String name, Base value) throws FHIRException { 227 if (name.equals("rankOrder")) { 228 this.rankOrder = TypeConvertor.castToInteger(value); // IntegerType 229 } else if (name.equals("intervalStatistic")) { 230 this.getIntervalStatistic().add(TypeConvertor.castToStatistic(value)); 231 } else 232 return super.setProperty(name, value); 233 return value; 234 } 235 236 @Override 237 public void removeChild(String name, Base value) throws FHIRException { 238 if (name.equals("rankOrder")) { 239 this.rankOrder = null; 240 } else if (name.equals("intervalStatistic")) { 241 this.getIntervalStatistic().remove(value); 242 } else 243 super.removeChild(name, value); 244 245 } 246 247 @Override 248 public Base makeProperty(int hash, String name) throws FHIRException { 249 switch (hash) { 250 case -656537982: return getRankOrderElement(); 251 case 227099147: return addIntervalStatistic(); 252 default: return super.makeProperty(hash, name); 253 } 254 255 } 256 257 @Override 258 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 259 switch (hash) { 260 case -656537982: /*rankOrder*/ return new String[] {"integer"}; 261 case 227099147: /*intervalStatistic*/ return new String[] {"Statistic"}; 262 default: return super.getTypesForProperty(hash, name); 263 } 264 265 } 266 267 @Override 268 public Base addChild(String name) throws FHIRException { 269 if (name.equals("rankOrder")) { 270 throw new FHIRException("Cannot call addChild on a singleton property OrderedDistribution.interval.rankOrder"); 271 } 272 else if (name.equals("intervalStatistic")) { 273 return addIntervalStatistic(); 274 } 275 else 276 return super.addChild(name); 277 } 278 279 public OrderedDistributionIntervalComponent copy() { 280 OrderedDistributionIntervalComponent dst = new OrderedDistributionIntervalComponent(); 281 copyValues(dst); 282 return dst; 283 } 284 285 public void copyValues(OrderedDistributionIntervalComponent dst) { 286 super.copyValues(dst); 287 dst.rankOrder = rankOrder == null ? null : rankOrder.copy(); 288 if (intervalStatistic != null) { 289 dst.intervalStatistic = new ArrayList<Statistic>(); 290 for (Statistic i : intervalStatistic) 291 dst.intervalStatistic.add(i.copy()); 292 }; 293 } 294 295 @Override 296 public boolean equalsDeep(Base other_) { 297 if (!super.equalsDeep(other_)) 298 return false; 299 if (!(other_ instanceof OrderedDistributionIntervalComponent)) 300 return false; 301 OrderedDistributionIntervalComponent o = (OrderedDistributionIntervalComponent) other_; 302 return compareDeep(rankOrder, o.rankOrder, true) && compareDeep(intervalStatistic, o.intervalStatistic, true) 303 ; 304 } 305 306 @Override 307 public boolean equalsShallow(Base other_) { 308 if (!super.equalsShallow(other_)) 309 return false; 310 if (!(other_ instanceof OrderedDistributionIntervalComponent)) 311 return false; 312 OrderedDistributionIntervalComponent o = (OrderedDistributionIntervalComponent) other_; 313 return compareValues(rankOrder, o.rankOrder, true); 314 } 315 316 public boolean isEmpty() { 317 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(rankOrder, intervalStatistic 318 ); 319 } 320 321 public String fhirType() { 322 return "OrderedDistribution.interval"; 323 324 } 325 326 } 327 328 /** 329 * A description of the content and value of the statistic. 330 */ 331 @Child(name = "description", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=false) 332 @Description(shortDefinition="A description of the content and value of the statistic", formalDefinition="A description of the content and value of the statistic." ) 333 protected StringType description; 334 335 /** 336 * Footnotes and/or explanatory notes. 337 */ 338 @Child(name = "note", type = {Annotation.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 339 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Footnotes and/or explanatory notes." ) 340 protected List<Annotation> note; 341 342 /** 343 * Number of intervals in an array, eg 4 for quartiles. 344 */ 345 @Child(name = "numberOfIntervals", type = {IntegerType.class}, order=2, min=1, max=1, modifier=false, summary=true) 346 @Description(shortDefinition="Number of intervals in an array, eg 4 for quartiles", formalDefinition="Number of intervals in an array, eg 4 for quartiles." ) 347 protected IntegerType numberOfIntervals; 348 349 /** 350 * Bottom of first interval. 351 */ 352 @Child(name = "bottomOfFirstInterval", type = {Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 353 @Description(shortDefinition="Bottom of first interval", formalDefinition="Bottom of first interval." ) 354 protected Quantity bottomOfFirstInterval; 355 356 /** 357 * Interval. 358 */ 359 @Child(name = "interval", type = {}, order=4, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 360 @Description(shortDefinition="Interval", formalDefinition="Interval." ) 361 protected List<OrderedDistributionIntervalComponent> interval; 362 363 /** 364 * Singular value of the statistic at the upper bound of the interval. 365 */ 366 @Child(name = "topOfInterval", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 367 @Description(shortDefinition="Singular value of the statistic at the upper bound of the interval", formalDefinition="Singular value of the statistic at the upper bound of the interval." ) 368 protected Quantity topOfInterval; 369 370 private static final long serialVersionUID = -1559333328L; 371 372 /** 373 * Constructor 374 */ 375 public OrderedDistribution() { 376 super(); 377 } 378 379 /** 380 * Constructor 381 */ 382 public OrderedDistribution(int numberOfIntervals, OrderedDistributionIntervalComponent interval) { 383 super(); 384 this.setNumberOfIntervals(numberOfIntervals); 385 this.addInterval(interval); 386 } 387 388 /** 389 * @return {@link #description} (A description of the content and value of the statistic.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 390 */ 391 public StringType getDescriptionElement() { 392 if (this.description == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create OrderedDistribution.description"); 395 else if (Configuration.doAutoCreate()) 396 this.description = new StringType(); // bb 397 return this.description; 398 } 399 400 public boolean hasDescriptionElement() { 401 return this.description != null && !this.description.isEmpty(); 402 } 403 404 public boolean hasDescription() { 405 return this.description != null && !this.description.isEmpty(); 406 } 407 408 /** 409 * @param value {@link #description} (A description of the content and value of the statistic.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 410 */ 411 public OrderedDistribution setDescriptionElement(StringType value) { 412 this.description = value; 413 return this; 414 } 415 416 /** 417 * @return A description of the content and value of the statistic. 418 */ 419 public String getDescription() { 420 return this.description == null ? null : this.description.getValue(); 421 } 422 423 /** 424 * @param value A description of the content and value of the statistic. 425 */ 426 public OrderedDistribution setDescription(String value) { 427 if (Utilities.noString(value)) 428 this.description = null; 429 else { 430 if (this.description == null) 431 this.description = new StringType(); 432 this.description.setValue(value); 433 } 434 return this; 435 } 436 437 /** 438 * @return {@link #note} (Footnotes and/or explanatory notes.) 439 */ 440 public List<Annotation> getNote() { 441 if (this.note == null) 442 this.note = new ArrayList<Annotation>(); 443 return this.note; 444 } 445 446 /** 447 * @return Returns a reference to <code>this</code> for easy method chaining 448 */ 449 public OrderedDistribution setNote(List<Annotation> theNote) { 450 this.note = theNote; 451 return this; 452 } 453 454 public boolean hasNote() { 455 if (this.note == null) 456 return false; 457 for (Annotation item : this.note) 458 if (!item.isEmpty()) 459 return true; 460 return false; 461 } 462 463 public Annotation addNote() { //3 464 Annotation t = new Annotation(); 465 if (this.note == null) 466 this.note = new ArrayList<Annotation>(); 467 this.note.add(t); 468 return t; 469 } 470 471 public OrderedDistribution addNote(Annotation t) { //3 472 if (t == null) 473 return this; 474 if (this.note == null) 475 this.note = new ArrayList<Annotation>(); 476 this.note.add(t); 477 return this; 478 } 479 480 /** 481 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 482 */ 483 public Annotation getNoteFirstRep() { 484 if (getNote().isEmpty()) { 485 addNote(); 486 } 487 return getNote().get(0); 488 } 489 490 /** 491 * @return {@link #numberOfIntervals} (Number of intervals in an array, eg 4 for quartiles.). This is the underlying object with id, value and extensions. The accessor "getNumberOfIntervals" gives direct access to the value 492 */ 493 public IntegerType getNumberOfIntervalsElement() { 494 if (this.numberOfIntervals == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create OrderedDistribution.numberOfIntervals"); 497 else if (Configuration.doAutoCreate()) 498 this.numberOfIntervals = new IntegerType(); // bb 499 return this.numberOfIntervals; 500 } 501 502 public boolean hasNumberOfIntervalsElement() { 503 return this.numberOfIntervals != null && !this.numberOfIntervals.isEmpty(); 504 } 505 506 public boolean hasNumberOfIntervals() { 507 return this.numberOfIntervals != null && !this.numberOfIntervals.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #numberOfIntervals} (Number of intervals in an array, eg 4 for quartiles.). This is the underlying object with id, value and extensions. The accessor "getNumberOfIntervals" gives direct access to the value 512 */ 513 public OrderedDistribution setNumberOfIntervalsElement(IntegerType value) { 514 this.numberOfIntervals = value; 515 return this; 516 } 517 518 /** 519 * @return Number of intervals in an array, eg 4 for quartiles. 520 */ 521 public int getNumberOfIntervals() { 522 return this.numberOfIntervals == null || this.numberOfIntervals.isEmpty() ? 0 : this.numberOfIntervals.getValue(); 523 } 524 525 /** 526 * @param value Number of intervals in an array, eg 4 for quartiles. 527 */ 528 public OrderedDistribution setNumberOfIntervals(int value) { 529 if (this.numberOfIntervals == null) 530 this.numberOfIntervals = new IntegerType(); 531 this.numberOfIntervals.setValue(value); 532 return this; 533 } 534 535 /** 536 * @return {@link #bottomOfFirstInterval} (Bottom of first interval.) 537 */ 538 public Quantity getBottomOfFirstInterval() { 539 if (this.bottomOfFirstInterval == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create OrderedDistribution.bottomOfFirstInterval"); 542 else if (Configuration.doAutoCreate()) 543 this.bottomOfFirstInterval = new Quantity(); // cc 544 return this.bottomOfFirstInterval; 545 } 546 547 public boolean hasBottomOfFirstInterval() { 548 return this.bottomOfFirstInterval != null && !this.bottomOfFirstInterval.isEmpty(); 549 } 550 551 /** 552 * @param value {@link #bottomOfFirstInterval} (Bottom of first interval.) 553 */ 554 public OrderedDistribution setBottomOfFirstInterval(Quantity value) { 555 this.bottomOfFirstInterval = value; 556 return this; 557 } 558 559 /** 560 * @return {@link #interval} (Interval.) 561 */ 562 public List<OrderedDistributionIntervalComponent> getInterval() { 563 if (this.interval == null) 564 this.interval = new ArrayList<OrderedDistributionIntervalComponent>(); 565 return this.interval; 566 } 567 568 /** 569 * @return Returns a reference to <code>this</code> for easy method chaining 570 */ 571 public OrderedDistribution setInterval(List<OrderedDistributionIntervalComponent> theInterval) { 572 this.interval = theInterval; 573 return this; 574 } 575 576 public boolean hasInterval() { 577 if (this.interval == null) 578 return false; 579 for (OrderedDistributionIntervalComponent item : this.interval) 580 if (!item.isEmpty()) 581 return true; 582 return false; 583 } 584 585 public OrderedDistributionIntervalComponent addInterval() { //3 586 OrderedDistributionIntervalComponent t = new OrderedDistributionIntervalComponent(); 587 if (this.interval == null) 588 this.interval = new ArrayList<OrderedDistributionIntervalComponent>(); 589 this.interval.add(t); 590 return t; 591 } 592 593 public OrderedDistribution addInterval(OrderedDistributionIntervalComponent t) { //3 594 if (t == null) 595 return this; 596 if (this.interval == null) 597 this.interval = new ArrayList<OrderedDistributionIntervalComponent>(); 598 this.interval.add(t); 599 return this; 600 } 601 602 /** 603 * @return The first repetition of repeating field {@link #interval}, creating it if it does not already exist {3} 604 */ 605 public OrderedDistributionIntervalComponent getIntervalFirstRep() { 606 if (getInterval().isEmpty()) { 607 addInterval(); 608 } 609 return getInterval().get(0); 610 } 611 612 /** 613 * @return {@link #topOfInterval} (Singular value of the statistic at the upper bound of the interval.) 614 */ 615 public Quantity getTopOfInterval() { 616 if (this.topOfInterval == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create OrderedDistribution.topOfInterval"); 619 else if (Configuration.doAutoCreate()) 620 this.topOfInterval = new Quantity(); // cc 621 return this.topOfInterval; 622 } 623 624 public boolean hasTopOfInterval() { 625 return this.topOfInterval != null && !this.topOfInterval.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #topOfInterval} (Singular value of the statistic at the upper bound of the interval.) 630 */ 631 public OrderedDistribution setTopOfInterval(Quantity value) { 632 this.topOfInterval = value; 633 return this; 634 } 635 636 protected void listChildren(List<Property> children) { 637 super.listChildren(children); 638 children.add(new Property("description", "string", "A description of the content and value of the statistic.", 0, 1, description)); 639 children.add(new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note)); 640 children.add(new Property("numberOfIntervals", "integer", "Number of intervals in an array, eg 4 for quartiles.", 0, 1, numberOfIntervals)); 641 children.add(new Property("bottomOfFirstInterval", "Quantity", "Bottom of first interval.", 0, 1, bottomOfFirstInterval)); 642 children.add(new Property("interval", "", "Interval.", 0, java.lang.Integer.MAX_VALUE, interval)); 643 children.add(new Property("topOfInterval", "Quantity", "Singular value of the statistic at the upper bound of the interval.", 0, 1, topOfInterval)); 644 } 645 646 @Override 647 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 648 switch (_hash) { 649 case -1724546052: /*description*/ return new Property("description", "string", "A description of the content and value of the statistic.", 0, 1, description); 650 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note); 651 case -569541330: /*numberOfIntervals*/ return new Property("numberOfIntervals", "integer", "Number of intervals in an array, eg 4 for quartiles.", 0, 1, numberOfIntervals); 652 case 37889363: /*bottomOfFirstInterval*/ return new Property("bottomOfFirstInterval", "Quantity", "Bottom of first interval.", 0, 1, bottomOfFirstInterval); 653 case 570418373: /*interval*/ return new Property("interval", "", "Interval.", 0, java.lang.Integer.MAX_VALUE, interval); 654 case 691816177: /*topOfInterval*/ return new Property("topOfInterval", "Quantity", "Singular value of the statistic at the upper bound of the interval.", 0, 1, topOfInterval); 655 default: return super.getNamedProperty(_hash, _name, _checkValid); 656 } 657 658 } 659 660 @Override 661 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 662 switch (hash) { 663 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 664 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 665 case -569541330: /*numberOfIntervals*/ return this.numberOfIntervals == null ? new Base[0] : new Base[] {this.numberOfIntervals}; // IntegerType 666 case 37889363: /*bottomOfFirstInterval*/ return this.bottomOfFirstInterval == null ? new Base[0] : new Base[] {this.bottomOfFirstInterval}; // Quantity 667 case 570418373: /*interval*/ return this.interval == null ? new Base[0] : this.interval.toArray(new Base[this.interval.size()]); // OrderedDistributionIntervalComponent 668 case 691816177: /*topOfInterval*/ return this.topOfInterval == null ? new Base[0] : new Base[] {this.topOfInterval}; // Quantity 669 default: return super.getProperty(hash, name, checkValid); 670 } 671 672 } 673 674 @Override 675 public Base setProperty(int hash, String name, Base value) throws FHIRException { 676 switch (hash) { 677 case -1724546052: // description 678 this.description = TypeConvertor.castToString(value); // StringType 679 return value; 680 case 3387378: // note 681 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 682 return value; 683 case -569541330: // numberOfIntervals 684 this.numberOfIntervals = TypeConvertor.castToInteger(value); // IntegerType 685 return value; 686 case 37889363: // bottomOfFirstInterval 687 this.bottomOfFirstInterval = TypeConvertor.castToQuantity(value); // Quantity 688 return value; 689 case 570418373: // interval 690 this.getInterval().add((OrderedDistributionIntervalComponent) value); // OrderedDistributionIntervalComponent 691 return value; 692 case 691816177: // topOfInterval 693 this.topOfInterval = TypeConvertor.castToQuantity(value); // Quantity 694 return value; 695 default: return super.setProperty(hash, name, value); 696 } 697 698 } 699 700 @Override 701 public Base setProperty(String name, Base value) throws FHIRException { 702 if (name.equals("description")) { 703 this.description = TypeConvertor.castToString(value); // StringType 704 } else if (name.equals("note")) { 705 this.getNote().add(TypeConvertor.castToAnnotation(value)); 706 } else if (name.equals("numberOfIntervals")) { 707 this.numberOfIntervals = TypeConvertor.castToInteger(value); // IntegerType 708 } else if (name.equals("bottomOfFirstInterval")) { 709 this.bottomOfFirstInterval = TypeConvertor.castToQuantity(value); // Quantity 710 } else if (name.equals("interval")) { 711 this.getInterval().add((OrderedDistributionIntervalComponent) value); 712 } else if (name.equals("topOfInterval")) { 713 this.topOfInterval = TypeConvertor.castToQuantity(value); // Quantity 714 } else 715 return super.setProperty(name, value); 716 return value; 717 } 718 719 @Override 720 public void removeChild(String name, Base value) throws FHIRException { 721 if (name.equals("description")) { 722 this.description = null; 723 } else if (name.equals("note")) { 724 this.getNote().remove(value); 725 } else if (name.equals("numberOfIntervals")) { 726 this.numberOfIntervals = null; 727 } else if (name.equals("bottomOfFirstInterval")) { 728 this.bottomOfFirstInterval = null; 729 } else if (name.equals("interval")) { 730 this.getInterval().remove((OrderedDistributionIntervalComponent) value); 731 } else if (name.equals("topOfInterval")) { 732 this.topOfInterval = null; 733 } else 734 super.removeChild(name, value); 735 736 } 737 738 @Override 739 public Base makeProperty(int hash, String name) throws FHIRException { 740 switch (hash) { 741 case -1724546052: return getDescriptionElement(); 742 case 3387378: return addNote(); 743 case -569541330: return getNumberOfIntervalsElement(); 744 case 37889363: return getBottomOfFirstInterval(); 745 case 570418373: return addInterval(); 746 case 691816177: return getTopOfInterval(); 747 default: return super.makeProperty(hash, name); 748 } 749 750 } 751 752 @Override 753 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 754 switch (hash) { 755 case -1724546052: /*description*/ return new String[] {"string"}; 756 case 3387378: /*note*/ return new String[] {"Annotation"}; 757 case -569541330: /*numberOfIntervals*/ return new String[] {"integer"}; 758 case 37889363: /*bottomOfFirstInterval*/ return new String[] {"Quantity"}; 759 case 570418373: /*interval*/ return new String[] {}; 760 case 691816177: /*topOfInterval*/ return new String[] {"Quantity"}; 761 default: return super.getTypesForProperty(hash, name); 762 } 763 764 } 765 766 @Override 767 public Base addChild(String name) throws FHIRException { 768 if (name.equals("description")) { 769 throw new FHIRException("Cannot call addChild on a singleton property OrderedDistribution.description"); 770 } 771 else if (name.equals("note")) { 772 return addNote(); 773 } 774 else if (name.equals("numberOfIntervals")) { 775 throw new FHIRException("Cannot call addChild on a singleton property OrderedDistribution.numberOfIntervals"); 776 } 777 else if (name.equals("bottomOfFirstInterval")) { 778 this.bottomOfFirstInterval = new Quantity(); 779 return this.bottomOfFirstInterval; 780 } 781 else if (name.equals("interval")) { 782 return addInterval(); 783 } 784 else if (name.equals("topOfInterval")) { 785 this.topOfInterval = new Quantity(); 786 return this.topOfInterval; 787 } 788 else 789 return super.addChild(name); 790 } 791 792 public String fhirType() { 793 return "OrderedDistribution"; 794 795 } 796 797 public OrderedDistribution copy() { 798 OrderedDistribution dst = new OrderedDistribution(); 799 copyValues(dst); 800 return dst; 801 } 802 803 public void copyValues(OrderedDistribution dst) { 804 super.copyValues(dst); 805 dst.description = description == null ? null : description.copy(); 806 if (note != null) { 807 dst.note = new ArrayList<Annotation>(); 808 for (Annotation i : note) 809 dst.note.add(i.copy()); 810 }; 811 dst.numberOfIntervals = numberOfIntervals == null ? null : numberOfIntervals.copy(); 812 dst.bottomOfFirstInterval = bottomOfFirstInterval == null ? null : bottomOfFirstInterval.copy(); 813 if (interval != null) { 814 dst.interval = new ArrayList<OrderedDistributionIntervalComponent>(); 815 for (OrderedDistributionIntervalComponent i : interval) 816 dst.interval.add(i.copy()); 817 }; 818 dst.topOfInterval = topOfInterval == null ? null : topOfInterval.copy(); 819 } 820 821 protected OrderedDistribution typedCopy() { 822 return copy(); 823 } 824 825 @Override 826 public boolean equalsDeep(Base other_) { 827 if (!super.equalsDeep(other_)) 828 return false; 829 if (!(other_ instanceof OrderedDistribution)) 830 return false; 831 OrderedDistribution o = (OrderedDistribution) other_; 832 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(numberOfIntervals, o.numberOfIntervals, true) 833 && compareDeep(bottomOfFirstInterval, o.bottomOfFirstInterval, true) && compareDeep(interval, o.interval, true) 834 && compareDeep(topOfInterval, o.topOfInterval, true); 835 } 836 837 @Override 838 public boolean equalsShallow(Base other_) { 839 if (!super.equalsShallow(other_)) 840 return false; 841 if (!(other_ instanceof OrderedDistribution)) 842 return false; 843 OrderedDistribution o = (OrderedDistribution) other_; 844 return compareValues(description, o.description, true) && compareValues(numberOfIntervals, o.numberOfIntervals, true) 845 ; 846 } 847 848 public boolean isEmpty() { 849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, numberOfIntervals 850 , bottomOfFirstInterval, interval, topOfInterval); 851 } 852 853 854} 855