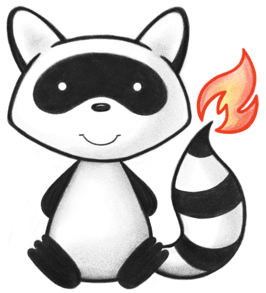
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc. 052 */ 053@ResourceDef(name="Organization", profile="http://hl7.org/fhir/StructureDefinition/Organization") 054public class Organization extends DomainResource { 055 056 @Block() 057 public static class OrganizationQualificationComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * An identifier allocated to this qualification for this organization. 060 */ 061 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 062 @Description(shortDefinition="An identifier for this qualification for the organization", formalDefinition="An identifier allocated to this qualification for this organization." ) 063 protected List<Identifier> identifier; 064 065 /** 066 * Coded representation of the qualification. 067 */ 068 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="Coded representation of the qualification", formalDefinition="Coded representation of the qualification." ) 070 protected CodeableConcept code; 071 072 /** 073 * Period during which the qualification is valid. 074 */ 075 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 076 @Description(shortDefinition="Period during which the qualification is valid", formalDefinition="Period during which the qualification is valid." ) 077 protected Period period; 078 079 /** 080 * Organization that regulates and issues the qualification. 081 */ 082 @Child(name = "issuer", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 083 @Description(shortDefinition="Organization that regulates and issues the qualification", formalDefinition="Organization that regulates and issues the qualification." ) 084 protected Reference issuer; 085 086 private static final long serialVersionUID = 1561812204L; 087 088 /** 089 * Constructor 090 */ 091 public OrganizationQualificationComponent() { 092 super(); 093 } 094 095 /** 096 * Constructor 097 */ 098 public OrganizationQualificationComponent(CodeableConcept code) { 099 super(); 100 this.setCode(code); 101 } 102 103 /** 104 * @return {@link #identifier} (An identifier allocated to this qualification for this organization.) 105 */ 106 public List<Identifier> getIdentifier() { 107 if (this.identifier == null) 108 this.identifier = new ArrayList<Identifier>(); 109 return this.identifier; 110 } 111 112 /** 113 * @return Returns a reference to <code>this</code> for easy method chaining 114 */ 115 public OrganizationQualificationComponent setIdentifier(List<Identifier> theIdentifier) { 116 this.identifier = theIdentifier; 117 return this; 118 } 119 120 public boolean hasIdentifier() { 121 if (this.identifier == null) 122 return false; 123 for (Identifier item : this.identifier) 124 if (!item.isEmpty()) 125 return true; 126 return false; 127 } 128 129 public Identifier addIdentifier() { //3 130 Identifier t = new Identifier(); 131 if (this.identifier == null) 132 this.identifier = new ArrayList<Identifier>(); 133 this.identifier.add(t); 134 return t; 135 } 136 137 public OrganizationQualificationComponent addIdentifier(Identifier t) { //3 138 if (t == null) 139 return this; 140 if (this.identifier == null) 141 this.identifier = new ArrayList<Identifier>(); 142 this.identifier.add(t); 143 return this; 144 } 145 146 /** 147 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 148 */ 149 public Identifier getIdentifierFirstRep() { 150 if (getIdentifier().isEmpty()) { 151 addIdentifier(); 152 } 153 return getIdentifier().get(0); 154 } 155 156 /** 157 * @return {@link #code} (Coded representation of the qualification.) 158 */ 159 public CodeableConcept getCode() { 160 if (this.code == null) 161 if (Configuration.errorOnAutoCreate()) 162 throw new Error("Attempt to auto-create OrganizationQualificationComponent.code"); 163 else if (Configuration.doAutoCreate()) 164 this.code = new CodeableConcept(); // cc 165 return this.code; 166 } 167 168 public boolean hasCode() { 169 return this.code != null && !this.code.isEmpty(); 170 } 171 172 /** 173 * @param value {@link #code} (Coded representation of the qualification.) 174 */ 175 public OrganizationQualificationComponent setCode(CodeableConcept value) { 176 this.code = value; 177 return this; 178 } 179 180 /** 181 * @return {@link #period} (Period during which the qualification is valid.) 182 */ 183 public Period getPeriod() { 184 if (this.period == null) 185 if (Configuration.errorOnAutoCreate()) 186 throw new Error("Attempt to auto-create OrganizationQualificationComponent.period"); 187 else if (Configuration.doAutoCreate()) 188 this.period = new Period(); // cc 189 return this.period; 190 } 191 192 public boolean hasPeriod() { 193 return this.period != null && !this.period.isEmpty(); 194 } 195 196 /** 197 * @param value {@link #period} (Period during which the qualification is valid.) 198 */ 199 public OrganizationQualificationComponent setPeriod(Period value) { 200 this.period = value; 201 return this; 202 } 203 204 /** 205 * @return {@link #issuer} (Organization that regulates and issues the qualification.) 206 */ 207 public Reference getIssuer() { 208 if (this.issuer == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create OrganizationQualificationComponent.issuer"); 211 else if (Configuration.doAutoCreate()) 212 this.issuer = new Reference(); // cc 213 return this.issuer; 214 } 215 216 public boolean hasIssuer() { 217 return this.issuer != null && !this.issuer.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #issuer} (Organization that regulates and issues the qualification.) 222 */ 223 public OrganizationQualificationComponent setIssuer(Reference value) { 224 this.issuer = value; 225 return this; 226 } 227 228 protected void listChildren(List<Property> children) { 229 super.listChildren(children); 230 children.add(new Property("identifier", "Identifier", "An identifier allocated to this qualification for this organization.", 0, java.lang.Integer.MAX_VALUE, identifier)); 231 children.add(new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, code)); 232 children.add(new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, period)); 233 children.add(new Property("issuer", "Reference(Organization)", "Organization that regulates and issues the qualification.", 0, 1, issuer)); 234 } 235 236 @Override 237 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 238 switch (_hash) { 239 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier allocated to this qualification for this organization.", 0, java.lang.Integer.MAX_VALUE, identifier); 240 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, code); 241 case -991726143: /*period*/ return new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, period); 242 case -1179159879: /*issuer*/ return new Property("issuer", "Reference(Organization)", "Organization that regulates and issues the qualification.", 0, 1, issuer); 243 default: return super.getNamedProperty(_hash, _name, _checkValid); 244 } 245 246 } 247 248 @Override 249 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 250 switch (hash) { 251 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 252 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 253 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 254 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // Reference 255 default: return super.getProperty(hash, name, checkValid); 256 } 257 258 } 259 260 @Override 261 public Base setProperty(int hash, String name, Base value) throws FHIRException { 262 switch (hash) { 263 case -1618432855: // identifier 264 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 265 return value; 266 case 3059181: // code 267 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 268 return value; 269 case -991726143: // period 270 this.period = TypeConvertor.castToPeriod(value); // Period 271 return value; 272 case -1179159879: // issuer 273 this.issuer = TypeConvertor.castToReference(value); // Reference 274 return value; 275 default: return super.setProperty(hash, name, value); 276 } 277 278 } 279 280 @Override 281 public Base setProperty(String name, Base value) throws FHIRException { 282 if (name.equals("identifier")) { 283 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 284 } else if (name.equals("code")) { 285 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 286 } else if (name.equals("period")) { 287 this.period = TypeConvertor.castToPeriod(value); // Period 288 } else if (name.equals("issuer")) { 289 this.issuer = TypeConvertor.castToReference(value); // Reference 290 } else 291 return super.setProperty(name, value); 292 return value; 293 } 294 295 @Override 296 public void removeChild(String name, Base value) throws FHIRException { 297 if (name.equals("identifier")) { 298 this.getIdentifier().remove(value); 299 } else if (name.equals("code")) { 300 this.code = null; 301 } else if (name.equals("period")) { 302 this.period = null; 303 } else if (name.equals("issuer")) { 304 this.issuer = null; 305 } else 306 super.removeChild(name, value); 307 308 } 309 310 @Override 311 public Base makeProperty(int hash, String name) throws FHIRException { 312 switch (hash) { 313 case -1618432855: return addIdentifier(); 314 case 3059181: return getCode(); 315 case -991726143: return getPeriod(); 316 case -1179159879: return getIssuer(); 317 default: return super.makeProperty(hash, name); 318 } 319 320 } 321 322 @Override 323 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 324 switch (hash) { 325 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 326 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 327 case -991726143: /*period*/ return new String[] {"Period"}; 328 case -1179159879: /*issuer*/ return new String[] {"Reference"}; 329 default: return super.getTypesForProperty(hash, name); 330 } 331 332 } 333 334 @Override 335 public Base addChild(String name) throws FHIRException { 336 if (name.equals("identifier")) { 337 return addIdentifier(); 338 } 339 else if (name.equals("code")) { 340 this.code = new CodeableConcept(); 341 return this.code; 342 } 343 else if (name.equals("period")) { 344 this.period = new Period(); 345 return this.period; 346 } 347 else if (name.equals("issuer")) { 348 this.issuer = new Reference(); 349 return this.issuer; 350 } 351 else 352 return super.addChild(name); 353 } 354 355 public OrganizationQualificationComponent copy() { 356 OrganizationQualificationComponent dst = new OrganizationQualificationComponent(); 357 copyValues(dst); 358 return dst; 359 } 360 361 public void copyValues(OrganizationQualificationComponent dst) { 362 super.copyValues(dst); 363 if (identifier != null) { 364 dst.identifier = new ArrayList<Identifier>(); 365 for (Identifier i : identifier) 366 dst.identifier.add(i.copy()); 367 }; 368 dst.code = code == null ? null : code.copy(); 369 dst.period = period == null ? null : period.copy(); 370 dst.issuer = issuer == null ? null : issuer.copy(); 371 } 372 373 @Override 374 public boolean equalsDeep(Base other_) { 375 if (!super.equalsDeep(other_)) 376 return false; 377 if (!(other_ instanceof OrganizationQualificationComponent)) 378 return false; 379 OrganizationQualificationComponent o = (OrganizationQualificationComponent) other_; 380 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(period, o.period, true) 381 && compareDeep(issuer, o.issuer, true); 382 } 383 384 @Override 385 public boolean equalsShallow(Base other_) { 386 if (!super.equalsShallow(other_)) 387 return false; 388 if (!(other_ instanceof OrganizationQualificationComponent)) 389 return false; 390 OrganizationQualificationComponent o = (OrganizationQualificationComponent) other_; 391 return true; 392 } 393 394 public boolean isEmpty() { 395 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, period 396 , issuer); 397 } 398 399 public String fhirType() { 400 return "Organization.qualification"; 401 402 } 403 404 } 405 406 /** 407 * Identifier for the organization that is used to identify the organization across multiple disparate systems. 408 */ 409 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 410 @Description(shortDefinition="Identifies this organization across multiple systems", formalDefinition="Identifier for the organization that is used to identify the organization across multiple disparate systems." ) 411 protected List<Identifier> identifier; 412 413 /** 414 * Whether the organization's record is still in active use. 415 */ 416 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 417 @Description(shortDefinition="Whether the organization's record is still in active use", formalDefinition="Whether the organization's record is still in active use." ) 418 protected BooleanType active; 419 420 /** 421 * The kind(s) of organization that this is. 422 */ 423 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 424 @Description(shortDefinition="Kind of organization", formalDefinition="The kind(s) of organization that this is." ) 425 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/organization-type") 426 protected List<CodeableConcept> type; 427 428 /** 429 * A name associated with the organization. 430 */ 431 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 432 @Description(shortDefinition="Name used for the organization", formalDefinition="A name associated with the organization." ) 433 protected StringType name; 434 435 /** 436 * A list of alternate names that the organization is known as, or was known as in the past. 437 */ 438 @Child(name = "alias", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 439 @Description(shortDefinition="A list of alternate names that the organization is known as, or was known as in the past", formalDefinition="A list of alternate names that the organization is known as, or was known as in the past." ) 440 protected List<StringType> alias; 441 442 /** 443 * Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected. 444 */ 445 @Child(name = "description", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=true) 446 @Description(shortDefinition="Additional details about the Organization that could be displayed as further information to identify the Organization beyond its name", formalDefinition="Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected." ) 447 protected MarkdownType description; 448 449 /** 450 * The contact details of communication devices available relevant to the specific Organization. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites. 451 */ 452 @Child(name = "contact", type = {ExtendedContactDetail.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 453 @Description(shortDefinition="Official contact details for the Organization", formalDefinition="The contact details of communication devices available relevant to the specific Organization. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites." ) 454 protected List<ExtendedContactDetail> contact; 455 456 /** 457 * The organization of which this organization forms a part. 458 */ 459 @Child(name = "partOf", type = {Organization.class}, order=7, min=0, max=1, modifier=false, summary=true) 460 @Description(shortDefinition="The organization of which this organization forms a part", formalDefinition="The organization of which this organization forms a part." ) 461 protected Reference partOf; 462 463 /** 464 * Technical endpoints providing access to services operated for the organization. 465 */ 466 @Child(name = "endpoint", type = {Endpoint.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 467 @Description(shortDefinition="Technical endpoints providing access to services operated for the organization", formalDefinition="Technical endpoints providing access to services operated for the organization." ) 468 protected List<Reference> endpoint; 469 470 /** 471 * The official certifications, accreditations, training, designations and licenses that authorize and/or otherwise endorse the provision of care by the organization. 472 473For example, an approval to provide a type of services issued by a certifying body (such as the US Joint Commission) to an organization. 474 */ 475 @Child(name = "qualification", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 476 @Description(shortDefinition="Qualifications, certifications, accreditations, licenses, training, etc. pertaining to the provision of care", formalDefinition="The official certifications, accreditations, training, designations and licenses that authorize and/or otherwise endorse the provision of care by the organization.\r\rFor example, an approval to provide a type of services issued by a certifying body (such as the US Joint Commission) to an organization." ) 477 protected List<OrganizationQualificationComponent> qualification; 478 479 private static final long serialVersionUID = 1270045104L; 480 481 /** 482 * Constructor 483 */ 484 public Organization() { 485 super(); 486 } 487 488 /** 489 * @return {@link #identifier} (Identifier for the organization that is used to identify the organization across multiple disparate systems.) 490 */ 491 public List<Identifier> getIdentifier() { 492 if (this.identifier == null) 493 this.identifier = new ArrayList<Identifier>(); 494 return this.identifier; 495 } 496 497 /** 498 * @return Returns a reference to <code>this</code> for easy method chaining 499 */ 500 public Organization setIdentifier(List<Identifier> theIdentifier) { 501 this.identifier = theIdentifier; 502 return this; 503 } 504 505 public boolean hasIdentifier() { 506 if (this.identifier == null) 507 return false; 508 for (Identifier item : this.identifier) 509 if (!item.isEmpty()) 510 return true; 511 return false; 512 } 513 514 public Identifier addIdentifier() { //3 515 Identifier t = new Identifier(); 516 if (this.identifier == null) 517 this.identifier = new ArrayList<Identifier>(); 518 this.identifier.add(t); 519 return t; 520 } 521 522 public Organization addIdentifier(Identifier t) { //3 523 if (t == null) 524 return this; 525 if (this.identifier == null) 526 this.identifier = new ArrayList<Identifier>(); 527 this.identifier.add(t); 528 return this; 529 } 530 531 /** 532 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 533 */ 534 public Identifier getIdentifierFirstRep() { 535 if (getIdentifier().isEmpty()) { 536 addIdentifier(); 537 } 538 return getIdentifier().get(0); 539 } 540 541 /** 542 * @return {@link #active} (Whether the organization's record is still in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 543 */ 544 public BooleanType getActiveElement() { 545 if (this.active == null) 546 if (Configuration.errorOnAutoCreate()) 547 throw new Error("Attempt to auto-create Organization.active"); 548 else if (Configuration.doAutoCreate()) 549 this.active = new BooleanType(); // bb 550 return this.active; 551 } 552 553 public boolean hasActiveElement() { 554 return this.active != null && !this.active.isEmpty(); 555 } 556 557 public boolean hasActive() { 558 return this.active != null && !this.active.isEmpty(); 559 } 560 561 /** 562 * @param value {@link #active} (Whether the organization's record is still in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 563 */ 564 public Organization setActiveElement(BooleanType value) { 565 this.active = value; 566 return this; 567 } 568 569 /** 570 * @return Whether the organization's record is still in active use. 571 */ 572 public boolean getActive() { 573 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 574 } 575 576 /** 577 * @param value Whether the organization's record is still in active use. 578 */ 579 public Organization setActive(boolean value) { 580 if (this.active == null) 581 this.active = new BooleanType(); 582 this.active.setValue(value); 583 return this; 584 } 585 586 /** 587 * @return {@link #type} (The kind(s) of organization that this is.) 588 */ 589 public List<CodeableConcept> getType() { 590 if (this.type == null) 591 this.type = new ArrayList<CodeableConcept>(); 592 return this.type; 593 } 594 595 /** 596 * @return Returns a reference to <code>this</code> for easy method chaining 597 */ 598 public Organization setType(List<CodeableConcept> theType) { 599 this.type = theType; 600 return this; 601 } 602 603 public boolean hasType() { 604 if (this.type == null) 605 return false; 606 for (CodeableConcept item : this.type) 607 if (!item.isEmpty()) 608 return true; 609 return false; 610 } 611 612 public CodeableConcept addType() { //3 613 CodeableConcept t = new CodeableConcept(); 614 if (this.type == null) 615 this.type = new ArrayList<CodeableConcept>(); 616 this.type.add(t); 617 return t; 618 } 619 620 public Organization addType(CodeableConcept t) { //3 621 if (t == null) 622 return this; 623 if (this.type == null) 624 this.type = new ArrayList<CodeableConcept>(); 625 this.type.add(t); 626 return this; 627 } 628 629 /** 630 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 631 */ 632 public CodeableConcept getTypeFirstRep() { 633 if (getType().isEmpty()) { 634 addType(); 635 } 636 return getType().get(0); 637 } 638 639 /** 640 * @return {@link #name} (A name associated with the organization.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 641 */ 642 public StringType getNameElement() { 643 if (this.name == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create Organization.name"); 646 else if (Configuration.doAutoCreate()) 647 this.name = new StringType(); // bb 648 return this.name; 649 } 650 651 public boolean hasNameElement() { 652 return this.name != null && !this.name.isEmpty(); 653 } 654 655 public boolean hasName() { 656 return this.name != null && !this.name.isEmpty(); 657 } 658 659 /** 660 * @param value {@link #name} (A name associated with the organization.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 661 */ 662 public Organization setNameElement(StringType value) { 663 this.name = value; 664 return this; 665 } 666 667 /** 668 * @return A name associated with the organization. 669 */ 670 public String getName() { 671 return this.name == null ? null : this.name.getValue(); 672 } 673 674 /** 675 * @param value A name associated with the organization. 676 */ 677 public Organization setName(String value) { 678 if (Utilities.noString(value)) 679 this.name = null; 680 else { 681 if (this.name == null) 682 this.name = new StringType(); 683 this.name.setValue(value); 684 } 685 return this; 686 } 687 688 /** 689 * @return {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 690 */ 691 public List<StringType> getAlias() { 692 if (this.alias == null) 693 this.alias = new ArrayList<StringType>(); 694 return this.alias; 695 } 696 697 /** 698 * @return Returns a reference to <code>this</code> for easy method chaining 699 */ 700 public Organization setAlias(List<StringType> theAlias) { 701 this.alias = theAlias; 702 return this; 703 } 704 705 public boolean hasAlias() { 706 if (this.alias == null) 707 return false; 708 for (StringType item : this.alias) 709 if (!item.isEmpty()) 710 return true; 711 return false; 712 } 713 714 /** 715 * @return {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 716 */ 717 public StringType addAliasElement() {//2 718 StringType t = new StringType(); 719 if (this.alias == null) 720 this.alias = new ArrayList<StringType>(); 721 this.alias.add(t); 722 return t; 723 } 724 725 /** 726 * @param value {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 727 */ 728 public Organization addAlias(String value) { //1 729 StringType t = new StringType(); 730 t.setValue(value); 731 if (this.alias == null) 732 this.alias = new ArrayList<StringType>(); 733 this.alias.add(t); 734 return this; 735 } 736 737 /** 738 * @param value {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 739 */ 740 public boolean hasAlias(String value) { 741 if (this.alias == null) 742 return false; 743 for (StringType v : this.alias) 744 if (v.getValue().equals(value)) // string 745 return true; 746 return false; 747 } 748 749 /** 750 * @return {@link #description} (Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 751 */ 752 public MarkdownType getDescriptionElement() { 753 if (this.description == null) 754 if (Configuration.errorOnAutoCreate()) 755 throw new Error("Attempt to auto-create Organization.description"); 756 else if (Configuration.doAutoCreate()) 757 this.description = new MarkdownType(); // bb 758 return this.description; 759 } 760 761 public boolean hasDescriptionElement() { 762 return this.description != null && !this.description.isEmpty(); 763 } 764 765 public boolean hasDescription() { 766 return this.description != null && !this.description.isEmpty(); 767 } 768 769 /** 770 * @param value {@link #description} (Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 771 */ 772 public Organization setDescriptionElement(MarkdownType value) { 773 this.description = value; 774 return this; 775 } 776 777 /** 778 * @return Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected. 779 */ 780 public String getDescription() { 781 return this.description == null ? null : this.description.getValue(); 782 } 783 784 /** 785 * @param value Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected. 786 */ 787 public Organization setDescription(String value) { 788 if (Utilities.noString(value)) 789 this.description = null; 790 else { 791 if (this.description == null) 792 this.description = new MarkdownType(); 793 this.description.setValue(value); 794 } 795 return this; 796 } 797 798 /** 799 * @return {@link #contact} (The contact details of communication devices available relevant to the specific Organization. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.) 800 */ 801 public List<ExtendedContactDetail> getContact() { 802 if (this.contact == null) 803 this.contact = new ArrayList<ExtendedContactDetail>(); 804 return this.contact; 805 } 806 807 /** 808 * @return Returns a reference to <code>this</code> for easy method chaining 809 */ 810 public Organization setContact(List<ExtendedContactDetail> theContact) { 811 this.contact = theContact; 812 return this; 813 } 814 815 public boolean hasContact() { 816 if (this.contact == null) 817 return false; 818 for (ExtendedContactDetail item : this.contact) 819 if (!item.isEmpty()) 820 return true; 821 return false; 822 } 823 824 public ExtendedContactDetail addContact() { //3 825 ExtendedContactDetail t = new ExtendedContactDetail(); 826 if (this.contact == null) 827 this.contact = new ArrayList<ExtendedContactDetail>(); 828 this.contact.add(t); 829 return t; 830 } 831 832 public Organization addContact(ExtendedContactDetail t) { //3 833 if (t == null) 834 return this; 835 if (this.contact == null) 836 this.contact = new ArrayList<ExtendedContactDetail>(); 837 this.contact.add(t); 838 return this; 839 } 840 841 /** 842 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 843 */ 844 public ExtendedContactDetail getContactFirstRep() { 845 if (getContact().isEmpty()) { 846 addContact(); 847 } 848 return getContact().get(0); 849 } 850 851 /** 852 * @return {@link #partOf} (The organization of which this organization forms a part.) 853 */ 854 public Reference getPartOf() { 855 if (this.partOf == null) 856 if (Configuration.errorOnAutoCreate()) 857 throw new Error("Attempt to auto-create Organization.partOf"); 858 else if (Configuration.doAutoCreate()) 859 this.partOf = new Reference(); // cc 860 return this.partOf; 861 } 862 863 public boolean hasPartOf() { 864 return this.partOf != null && !this.partOf.isEmpty(); 865 } 866 867 /** 868 * @param value {@link #partOf} (The organization of which this organization forms a part.) 869 */ 870 public Organization setPartOf(Reference value) { 871 this.partOf = value; 872 return this; 873 } 874 875 /** 876 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the organization.) 877 */ 878 public List<Reference> getEndpoint() { 879 if (this.endpoint == null) 880 this.endpoint = new ArrayList<Reference>(); 881 return this.endpoint; 882 } 883 884 /** 885 * @return Returns a reference to <code>this</code> for easy method chaining 886 */ 887 public Organization setEndpoint(List<Reference> theEndpoint) { 888 this.endpoint = theEndpoint; 889 return this; 890 } 891 892 public boolean hasEndpoint() { 893 if (this.endpoint == null) 894 return false; 895 for (Reference item : this.endpoint) 896 if (!item.isEmpty()) 897 return true; 898 return false; 899 } 900 901 public Reference addEndpoint() { //3 902 Reference t = new Reference(); 903 if (this.endpoint == null) 904 this.endpoint = new ArrayList<Reference>(); 905 this.endpoint.add(t); 906 return t; 907 } 908 909 public Organization addEndpoint(Reference t) { //3 910 if (t == null) 911 return this; 912 if (this.endpoint == null) 913 this.endpoint = new ArrayList<Reference>(); 914 this.endpoint.add(t); 915 return this; 916 } 917 918 /** 919 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 920 */ 921 public Reference getEndpointFirstRep() { 922 if (getEndpoint().isEmpty()) { 923 addEndpoint(); 924 } 925 return getEndpoint().get(0); 926 } 927 928 /** 929 * @return {@link #qualification} (The official certifications, accreditations, training, designations and licenses that authorize and/or otherwise endorse the provision of care by the organization. 930 931For example, an approval to provide a type of services issued by a certifying body (such as the US Joint Commission) to an organization.) 932 */ 933 public List<OrganizationQualificationComponent> getQualification() { 934 if (this.qualification == null) 935 this.qualification = new ArrayList<OrganizationQualificationComponent>(); 936 return this.qualification; 937 } 938 939 /** 940 * @return Returns a reference to <code>this</code> for easy method chaining 941 */ 942 public Organization setQualification(List<OrganizationQualificationComponent> theQualification) { 943 this.qualification = theQualification; 944 return this; 945 } 946 947 public boolean hasQualification() { 948 if (this.qualification == null) 949 return false; 950 for (OrganizationQualificationComponent item : this.qualification) 951 if (!item.isEmpty()) 952 return true; 953 return false; 954 } 955 956 public OrganizationQualificationComponent addQualification() { //3 957 OrganizationQualificationComponent t = new OrganizationQualificationComponent(); 958 if (this.qualification == null) 959 this.qualification = new ArrayList<OrganizationQualificationComponent>(); 960 this.qualification.add(t); 961 return t; 962 } 963 964 public Organization addQualification(OrganizationQualificationComponent t) { //3 965 if (t == null) 966 return this; 967 if (this.qualification == null) 968 this.qualification = new ArrayList<OrganizationQualificationComponent>(); 969 this.qualification.add(t); 970 return this; 971 } 972 973 /** 974 * @return The first repetition of repeating field {@link #qualification}, creating it if it does not already exist {3} 975 */ 976 public OrganizationQualificationComponent getQualificationFirstRep() { 977 if (getQualification().isEmpty()) { 978 addQualification(); 979 } 980 return getQualification().get(0); 981 } 982 983 protected void listChildren(List<Property> children) { 984 super.listChildren(children); 985 children.add(new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the organization across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 986 children.add(new Property("active", "boolean", "Whether the organization's record is still in active use.", 0, 1, active)); 987 children.add(new Property("type", "CodeableConcept", "The kind(s) of organization that this is.", 0, java.lang.Integer.MAX_VALUE, type)); 988 children.add(new Property("name", "string", "A name associated with the organization.", 0, 1, name)); 989 children.add(new Property("alias", "string", "A list of alternate names that the organization is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias)); 990 children.add(new Property("description", "markdown", "Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected.", 0, 1, description)); 991 children.add(new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific Organization. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact)); 992 children.add(new Property("partOf", "Reference(Organization)", "The organization of which this organization forms a part.", 0, 1, partOf)); 993 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the organization.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 994 children.add(new Property("qualification", "", "The official certifications, accreditations, training, designations and licenses that authorize and/or otherwise endorse the provision of care by the organization.\r\rFor example, an approval to provide a type of services issued by a certifying body (such as the US Joint Commission) to an organization.", 0, java.lang.Integer.MAX_VALUE, qualification)); 995 } 996 997 @Override 998 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 999 switch (_hash) { 1000 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the organization across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1001 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether the organization's record is still in active use.", 0, 1, active); 1002 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind(s) of organization that this is.", 0, java.lang.Integer.MAX_VALUE, type); 1003 case 3373707: /*name*/ return new Property("name", "string", "A name associated with the organization.", 0, 1, name); 1004 case 92902992: /*alias*/ return new Property("alias", "string", "A list of alternate names that the organization is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias); 1005 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of the organization, which helps provide additional general context on the organization to ensure that the correct organization is selected.", 0, 1, description); 1006 case 951526432: /*contact*/ return new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific Organization. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact); 1007 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Organization)", "The organization of which this organization forms a part.", 0, 1, partOf); 1008 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the organization.", 0, java.lang.Integer.MAX_VALUE, endpoint); 1009 case -631333393: /*qualification*/ return new Property("qualification", "", "The official certifications, accreditations, training, designations and licenses that authorize and/or otherwise endorse the provision of care by the organization.\r\rFor example, an approval to provide a type of services issued by a certifying body (such as the US Joint Commission) to an organization.", 0, java.lang.Integer.MAX_VALUE, qualification); 1010 default: return super.getNamedProperty(_hash, _name, _checkValid); 1011 } 1012 1013 } 1014 1015 @Override 1016 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1017 switch (hash) { 1018 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1019 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1020 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1021 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1022 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 1023 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1024 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ExtendedContactDetail 1025 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : new Base[] {this.partOf}; // Reference 1026 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1027 case -631333393: /*qualification*/ return this.qualification == null ? new Base[0] : this.qualification.toArray(new Base[this.qualification.size()]); // OrganizationQualificationComponent 1028 default: return super.getProperty(hash, name, checkValid); 1029 } 1030 1031 } 1032 1033 @Override 1034 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1035 switch (hash) { 1036 case -1618432855: // identifier 1037 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1038 return value; 1039 case -1422950650: // active 1040 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1041 return value; 1042 case 3575610: // type 1043 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1044 return value; 1045 case 3373707: // name 1046 this.name = TypeConvertor.castToString(value); // StringType 1047 return value; 1048 case 92902992: // alias 1049 this.getAlias().add(TypeConvertor.castToString(value)); // StringType 1050 return value; 1051 case -1724546052: // description 1052 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1053 return value; 1054 case 951526432: // contact 1055 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); // ExtendedContactDetail 1056 return value; 1057 case -995410646: // partOf 1058 this.partOf = TypeConvertor.castToReference(value); // Reference 1059 return value; 1060 case 1741102485: // endpoint 1061 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 1062 return value; 1063 case -631333393: // qualification 1064 this.getQualification().add((OrganizationQualificationComponent) value); // OrganizationQualificationComponent 1065 return value; 1066 default: return super.setProperty(hash, name, value); 1067 } 1068 1069 } 1070 1071 @Override 1072 public Base setProperty(String name, Base value) throws FHIRException { 1073 if (name.equals("identifier")) { 1074 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1075 } else if (name.equals("active")) { 1076 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1077 } else if (name.equals("type")) { 1078 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1079 } else if (name.equals("name")) { 1080 this.name = TypeConvertor.castToString(value); // StringType 1081 } else if (name.equals("alias")) { 1082 this.getAlias().add(TypeConvertor.castToString(value)); 1083 } else if (name.equals("description")) { 1084 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1085 } else if (name.equals("contact")) { 1086 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); 1087 } else if (name.equals("partOf")) { 1088 this.partOf = TypeConvertor.castToReference(value); // Reference 1089 } else if (name.equals("endpoint")) { 1090 this.getEndpoint().add(TypeConvertor.castToReference(value)); 1091 } else if (name.equals("qualification")) { 1092 this.getQualification().add((OrganizationQualificationComponent) value); 1093 } else 1094 return super.setProperty(name, value); 1095 return value; 1096 } 1097 1098 @Override 1099 public void removeChild(String name, Base value) throws FHIRException { 1100 if (name.equals("identifier")) { 1101 this.getIdentifier().remove(value); 1102 } else if (name.equals("active")) { 1103 this.active = null; 1104 } else if (name.equals("type")) { 1105 this.getType().remove(value); 1106 } else if (name.equals("name")) { 1107 this.name = null; 1108 } else if (name.equals("alias")) { 1109 this.getAlias().remove(value); 1110 } else if (name.equals("description")) { 1111 this.description = null; 1112 } else if (name.equals("contact")) { 1113 this.getContact().remove(value); 1114 } else if (name.equals("partOf")) { 1115 this.partOf = null; 1116 } else if (name.equals("endpoint")) { 1117 this.getEndpoint().remove(value); 1118 } else if (name.equals("qualification")) { 1119 this.getQualification().remove((OrganizationQualificationComponent) value); 1120 } else 1121 super.removeChild(name, value); 1122 1123 } 1124 1125 @Override 1126 public Base makeProperty(int hash, String name) throws FHIRException { 1127 switch (hash) { 1128 case -1618432855: return addIdentifier(); 1129 case -1422950650: return getActiveElement(); 1130 case 3575610: return addType(); 1131 case 3373707: return getNameElement(); 1132 case 92902992: return addAliasElement(); 1133 case -1724546052: return getDescriptionElement(); 1134 case 951526432: return addContact(); 1135 case -995410646: return getPartOf(); 1136 case 1741102485: return addEndpoint(); 1137 case -631333393: return addQualification(); 1138 default: return super.makeProperty(hash, name); 1139 } 1140 1141 } 1142 1143 @Override 1144 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1145 switch (hash) { 1146 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1147 case -1422950650: /*active*/ return new String[] {"boolean"}; 1148 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1149 case 3373707: /*name*/ return new String[] {"string"}; 1150 case 92902992: /*alias*/ return new String[] {"string"}; 1151 case -1724546052: /*description*/ return new String[] {"markdown"}; 1152 case 951526432: /*contact*/ return new String[] {"ExtendedContactDetail"}; 1153 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1154 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1155 case -631333393: /*qualification*/ return new String[] {}; 1156 default: return super.getTypesForProperty(hash, name); 1157 } 1158 1159 } 1160 1161 @Override 1162 public Base addChild(String name) throws FHIRException { 1163 if (name.equals("identifier")) { 1164 return addIdentifier(); 1165 } 1166 else if (name.equals("active")) { 1167 throw new FHIRException("Cannot call addChild on a singleton property Organization.active"); 1168 } 1169 else if (name.equals("type")) { 1170 return addType(); 1171 } 1172 else if (name.equals("name")) { 1173 throw new FHIRException("Cannot call addChild on a singleton property Organization.name"); 1174 } 1175 else if (name.equals("alias")) { 1176 throw new FHIRException("Cannot call addChild on a singleton property Organization.alias"); 1177 } 1178 else if (name.equals("description")) { 1179 throw new FHIRException("Cannot call addChild on a singleton property Organization.description"); 1180 } 1181 else if (name.equals("contact")) { 1182 return addContact(); 1183 } 1184 else if (name.equals("partOf")) { 1185 this.partOf = new Reference(); 1186 return this.partOf; 1187 } 1188 else if (name.equals("endpoint")) { 1189 return addEndpoint(); 1190 } 1191 else if (name.equals("qualification")) { 1192 return addQualification(); 1193 } 1194 else 1195 return super.addChild(name); 1196 } 1197 1198 public String fhirType() { 1199 return "Organization"; 1200 1201 } 1202 1203 public Organization copy() { 1204 Organization dst = new Organization(); 1205 copyValues(dst); 1206 return dst; 1207 } 1208 1209 public void copyValues(Organization dst) { 1210 super.copyValues(dst); 1211 if (identifier != null) { 1212 dst.identifier = new ArrayList<Identifier>(); 1213 for (Identifier i : identifier) 1214 dst.identifier.add(i.copy()); 1215 }; 1216 dst.active = active == null ? null : active.copy(); 1217 if (type != null) { 1218 dst.type = new ArrayList<CodeableConcept>(); 1219 for (CodeableConcept i : type) 1220 dst.type.add(i.copy()); 1221 }; 1222 dst.name = name == null ? null : name.copy(); 1223 if (alias != null) { 1224 dst.alias = new ArrayList<StringType>(); 1225 for (StringType i : alias) 1226 dst.alias.add(i.copy()); 1227 }; 1228 dst.description = description == null ? null : description.copy(); 1229 if (contact != null) { 1230 dst.contact = new ArrayList<ExtendedContactDetail>(); 1231 for (ExtendedContactDetail i : contact) 1232 dst.contact.add(i.copy()); 1233 }; 1234 dst.partOf = partOf == null ? null : partOf.copy(); 1235 if (endpoint != null) { 1236 dst.endpoint = new ArrayList<Reference>(); 1237 for (Reference i : endpoint) 1238 dst.endpoint.add(i.copy()); 1239 }; 1240 if (qualification != null) { 1241 dst.qualification = new ArrayList<OrganizationQualificationComponent>(); 1242 for (OrganizationQualificationComponent i : qualification) 1243 dst.qualification.add(i.copy()); 1244 }; 1245 } 1246 1247 protected Organization typedCopy() { 1248 return copy(); 1249 } 1250 1251 @Override 1252 public boolean equalsDeep(Base other_) { 1253 if (!super.equalsDeep(other_)) 1254 return false; 1255 if (!(other_ instanceof Organization)) 1256 return false; 1257 Organization o = (Organization) other_; 1258 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(type, o.type, true) 1259 && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) && compareDeep(description, o.description, true) 1260 && compareDeep(contact, o.contact, true) && compareDeep(partOf, o.partOf, true) && compareDeep(endpoint, o.endpoint, true) 1261 && compareDeep(qualification, o.qualification, true); 1262 } 1263 1264 @Override 1265 public boolean equalsShallow(Base other_) { 1266 if (!super.equalsShallow(other_)) 1267 return false; 1268 if (!(other_ instanceof Organization)) 1269 return false; 1270 Organization o = (Organization) other_; 1271 return compareValues(active, o.active, true) && compareValues(name, o.name, true) && compareValues(alias, o.alias, true) 1272 && compareValues(description, o.description, true); 1273 } 1274 1275 public boolean isEmpty() { 1276 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type 1277 , name, alias, description, contact, partOf, endpoint, qualification); 1278 } 1279 1280 @Override 1281 public ResourceType getResourceType() { 1282 return ResourceType.Organization; 1283 } 1284 1285 /** 1286 * Search parameter: <b>active</b> 1287 * <p> 1288 * Description: <b>Is the Organization record active</b><br> 1289 * Type: <b>token</b><br> 1290 * Path: <b>Organization.active</b><br> 1291 * </p> 1292 */ 1293 @SearchParamDefinition(name="active", path="Organization.active", description="Is the Organization record active", type="token" ) 1294 public static final String SP_ACTIVE = "active"; 1295 /** 1296 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1297 * <p> 1298 * Description: <b>Is the Organization record active</b><br> 1299 * Type: <b>token</b><br> 1300 * Path: <b>Organization.active</b><br> 1301 * </p> 1302 */ 1303 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1304 1305 /** 1306 * Search parameter: <b>address-city</b> 1307 * <p> 1308 * Description: <b>A city specified in an address</b><br> 1309 * Type: <b>string</b><br> 1310 * Path: <b>Organization.contact.address.city</b><br> 1311 * </p> 1312 */ 1313 @SearchParamDefinition(name="address-city", path="Organization.contact.address.city", description="A city specified in an address", type="string" ) 1314 public static final String SP_ADDRESS_CITY = "address-city"; 1315 /** 1316 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1317 * <p> 1318 * Description: <b>A city specified in an address</b><br> 1319 * Type: <b>string</b><br> 1320 * Path: <b>Organization.contact.address.city</b><br> 1321 * </p> 1322 */ 1323 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 1324 1325 /** 1326 * Search parameter: <b>address-country</b> 1327 * <p> 1328 * Description: <b>A country specified in an address</b><br> 1329 * Type: <b>string</b><br> 1330 * Path: <b>Organization.contact.address.country</b><br> 1331 * </p> 1332 */ 1333 @SearchParamDefinition(name="address-country", path="Organization.contact.address.country", description="A country specified in an address", type="string" ) 1334 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1335 /** 1336 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1337 * <p> 1338 * Description: <b>A country specified in an address</b><br> 1339 * Type: <b>string</b><br> 1340 * Path: <b>Organization.contact.address.country</b><br> 1341 * </p> 1342 */ 1343 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1344 1345 /** 1346 * Search parameter: <b>address-postalcode</b> 1347 * <p> 1348 * Description: <b>A postal code specified in an address</b><br> 1349 * Type: <b>string</b><br> 1350 * Path: <b>Organization.contact.address.postalCode</b><br> 1351 * </p> 1352 */ 1353 @SearchParamDefinition(name="address-postalcode", path="Organization.contact.address.postalCode", description="A postal code specified in an address", type="string" ) 1354 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1355 /** 1356 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1357 * <p> 1358 * Description: <b>A postal code specified in an address</b><br> 1359 * Type: <b>string</b><br> 1360 * Path: <b>Organization.contact.address.postalCode</b><br> 1361 * </p> 1362 */ 1363 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1364 1365 /** 1366 * Search parameter: <b>address-state</b> 1367 * <p> 1368 * Description: <b>A state specified in an address</b><br> 1369 * Type: <b>string</b><br> 1370 * Path: <b>Organization.contact.address.state</b><br> 1371 * </p> 1372 */ 1373 @SearchParamDefinition(name="address-state", path="Organization.contact.address.state", description="A state specified in an address", type="string" ) 1374 public static final String SP_ADDRESS_STATE = "address-state"; 1375 /** 1376 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1377 * <p> 1378 * Description: <b>A state specified in an address</b><br> 1379 * Type: <b>string</b><br> 1380 * Path: <b>Organization.contact.address.state</b><br> 1381 * </p> 1382 */ 1383 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1384 1385 /** 1386 * Search parameter: <b>address-use</b> 1387 * <p> 1388 * Description: <b>A use code specified in an address</b><br> 1389 * Type: <b>token</b><br> 1390 * Path: <b>Organization.contact.address.use</b><br> 1391 * </p> 1392 */ 1393 @SearchParamDefinition(name="address-use", path="Organization.contact.address.use", description="A use code specified in an address", type="token" ) 1394 public static final String SP_ADDRESS_USE = "address-use"; 1395 /** 1396 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1397 * <p> 1398 * Description: <b>A use code specified in an address</b><br> 1399 * Type: <b>token</b><br> 1400 * Path: <b>Organization.contact.address.use</b><br> 1401 * </p> 1402 */ 1403 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 1404 1405 /** 1406 * Search parameter: <b>address</b> 1407 * <p> 1408 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text</b><br> 1409 * Type: <b>string</b><br> 1410 * Path: <b>Organization.contact.address</b><br> 1411 * </p> 1412 */ 1413 @SearchParamDefinition(name="address", path="Organization.contact.address", description="A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type="string" ) 1414 public static final String SP_ADDRESS = "address"; 1415 /** 1416 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1417 * <p> 1418 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text</b><br> 1419 * Type: <b>string</b><br> 1420 * Path: <b>Organization.contact.address</b><br> 1421 * </p> 1422 */ 1423 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 1424 1425 /** 1426 * Search parameter: <b>endpoint</b> 1427 * <p> 1428 * Description: <b>Technical endpoints providing access to services operated for the organization</b><br> 1429 * Type: <b>reference</b><br> 1430 * Path: <b>Organization.endpoint</b><br> 1431 * </p> 1432 */ 1433 @SearchParamDefinition(name="endpoint", path="Organization.endpoint", description="Technical endpoints providing access to services operated for the organization", type="reference", target={Endpoint.class } ) 1434 public static final String SP_ENDPOINT = "endpoint"; 1435 /** 1436 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1437 * <p> 1438 * Description: <b>Technical endpoints providing access to services operated for the organization</b><br> 1439 * Type: <b>reference</b><br> 1440 * Path: <b>Organization.endpoint</b><br> 1441 * </p> 1442 */ 1443 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 1444 1445/** 1446 * Constant for fluent queries to be used to add include statements. Specifies 1447 * the path value of "<b>Organization:endpoint</b>". 1448 */ 1449 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("Organization:endpoint").toLocked(); 1450 1451 /** 1452 * Search parameter: <b>identifier</b> 1453 * <p> 1454 * Description: <b>Any identifier for the organization (not the accreditation issuer's identifier)</b><br> 1455 * Type: <b>token</b><br> 1456 * Path: <b>Organization.identifier | Organization.qualification.identifier</b><br> 1457 * </p> 1458 */ 1459 @SearchParamDefinition(name="identifier", path="Organization.identifier | Organization.qualification.identifier", description="Any identifier for the organization (not the accreditation issuer's identifier)", type="token" ) 1460 public static final String SP_IDENTIFIER = "identifier"; 1461 /** 1462 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1463 * <p> 1464 * Description: <b>Any identifier for the organization (not the accreditation issuer's identifier)</b><br> 1465 * Type: <b>token</b><br> 1466 * Path: <b>Organization.identifier | Organization.qualification.identifier</b><br> 1467 * </p> 1468 */ 1469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1470 1471 /** 1472 * Search parameter: <b>name</b> 1473 * <p> 1474 * Description: <b>A portion of the organization's name or alias</b><br> 1475 * Type: <b>string</b><br> 1476 * Path: <b>Organization.name | Organization.alias</b><br> 1477 * </p> 1478 */ 1479 @SearchParamDefinition(name="name", path="Organization.name | Organization.alias", description="A portion of the organization's name or alias", type="string" ) 1480 public static final String SP_NAME = "name"; 1481 /** 1482 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1483 * <p> 1484 * Description: <b>A portion of the organization's name or alias</b><br> 1485 * Type: <b>string</b><br> 1486 * Path: <b>Organization.name | Organization.alias</b><br> 1487 * </p> 1488 */ 1489 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1490 1491 /** 1492 * Search parameter: <b>partof</b> 1493 * <p> 1494 * Description: <b>An organization of which this organization forms a part</b><br> 1495 * Type: <b>reference</b><br> 1496 * Path: <b>Organization.partOf</b><br> 1497 * </p> 1498 */ 1499 @SearchParamDefinition(name="partof", path="Organization.partOf", description="An organization of which this organization forms a part", type="reference", target={Organization.class } ) 1500 public static final String SP_PARTOF = "partof"; 1501 /** 1502 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 1503 * <p> 1504 * Description: <b>An organization of which this organization forms a part</b><br> 1505 * Type: <b>reference</b><br> 1506 * Path: <b>Organization.partOf</b><br> 1507 * </p> 1508 */ 1509 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTOF); 1510 1511/** 1512 * Constant for fluent queries to be used to add include statements. Specifies 1513 * the path value of "<b>Organization:partof</b>". 1514 */ 1515 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include("Organization:partof").toLocked(); 1516 1517 /** 1518 * Search parameter: <b>phonetic</b> 1519 * <p> 1520 * Description: <b>A portion of the organization's name using some kind of phonetic matching algorithm</b><br> 1521 * Type: <b>string</b><br> 1522 * Path: <b>Organization.name</b><br> 1523 * </p> 1524 */ 1525 @SearchParamDefinition(name="phonetic", path="Organization.name", description="A portion of the organization's name using some kind of phonetic matching algorithm", type="string" ) 1526 public static final String SP_PHONETIC = "phonetic"; 1527 /** 1528 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1529 * <p> 1530 * Description: <b>A portion of the organization's name using some kind of phonetic matching algorithm</b><br> 1531 * Type: <b>string</b><br> 1532 * Path: <b>Organization.name</b><br> 1533 * </p> 1534 */ 1535 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 1536 1537 /** 1538 * Search parameter: <b>type</b> 1539 * <p> 1540 * Description: <b>A code for the type of organization</b><br> 1541 * Type: <b>token</b><br> 1542 * Path: <b>Organization.type</b><br> 1543 * </p> 1544 */ 1545 @SearchParamDefinition(name="type", path="Organization.type", description="A code for the type of organization", type="token" ) 1546 public static final String SP_TYPE = "type"; 1547 /** 1548 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1549 * <p> 1550 * Description: <b>A code for the type of organization</b><br> 1551 * Type: <b>token</b><br> 1552 * Path: <b>Organization.type</b><br> 1553 * </p> 1554 */ 1555 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1556 1557 1558} 1559