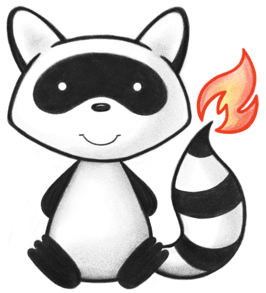
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Defines an affiliation/assotiation/relationship between 2 distinct organizations, that is not a part-of relationship/sub-division relationship. 051 */ 052@ResourceDef(name="OrganizationAffiliation", profile="http://hl7.org/fhir/StructureDefinition/OrganizationAffiliation") 053public class OrganizationAffiliation extends DomainResource { 054 055 /** 056 * Business identifiers that are specific to this role. 057 */ 058 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 059 @Description(shortDefinition="Business identifiers that are specific to this role", formalDefinition="Business identifiers that are specific to this role." ) 060 protected List<Identifier> identifier; 061 062 /** 063 * Whether this organization affiliation record is in active use. 064 */ 065 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="Whether this organization affiliation record is in active use", formalDefinition="Whether this organization affiliation record is in active use." ) 067 protected BooleanType active; 068 069 /** 070 * The period during which the participatingOrganization is affiliated with the primary organization. 071 */ 072 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 073 @Description(shortDefinition="The period during which the participatingOrganization is affiliated with the primary organization", formalDefinition="The period during which the participatingOrganization is affiliated with the primary organization." ) 074 protected Period period; 075 076 /** 077 * Organization where the role is available (primary organization/has members). 078 */ 079 @Child(name = "organization", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 080 @Description(shortDefinition="Organization where the role is available", formalDefinition="Organization where the role is available (primary organization/has members)." ) 081 protected Reference organization; 082 083 /** 084 * The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of). 085 */ 086 @Child(name = "participatingOrganization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 087 @Description(shortDefinition="Organization that provides/performs the role (e.g. providing services or is a member of)", formalDefinition="The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of)." ) 088 protected Reference participatingOrganization; 089 090 /** 091 * The network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined). 092 */ 093 @Child(name = "network", type = {Organization.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 094 @Description(shortDefinition="The network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined)", formalDefinition="The network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined)." ) 095 protected List<Reference> network; 096 097 /** 098 * Definition of the role the participatingOrganization plays in the association. 099 */ 100 @Child(name = "code", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 101 @Description(shortDefinition="Definition of the role the participatingOrganization plays", formalDefinition="Definition of the role the participatingOrganization plays in the association." ) 102 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/organization-role") 103 protected List<CodeableConcept> code; 104 105 /** 106 * Specific specialty of the participatingOrganization in the context of the role. 107 */ 108 @Child(name = "specialty", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 109 @Description(shortDefinition="Specific specialty of the participatingOrganization in the context of the role", formalDefinition="Specific specialty of the participatingOrganization in the context of the role." ) 110 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 111 protected List<CodeableConcept> specialty; 112 113 /** 114 * The location(s) at which the role occurs. 115 */ 116 @Child(name = "location", type = {Location.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 117 @Description(shortDefinition="The location(s) at which the role occurs", formalDefinition="The location(s) at which the role occurs." ) 118 protected List<Reference> location; 119 120 /** 121 * Healthcare services provided through the role. 122 */ 123 @Child(name = "healthcareService", type = {HealthcareService.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 124 @Description(shortDefinition="Healthcare services provided through the role", formalDefinition="Healthcare services provided through the role." ) 125 protected List<Reference> healthcareService; 126 127 /** 128 * The contact details of communication devices available at the participatingOrganization relevant to this Affiliation. 129 */ 130 @Child(name = "contact", type = {ExtendedContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 131 @Description(shortDefinition="Official contact details at the participatingOrganization relevant to this Affiliation", formalDefinition="The contact details of communication devices available at the participatingOrganization relevant to this Affiliation." ) 132 protected List<ExtendedContactDetail> contact; 133 134 /** 135 * Technical endpoints providing access to services operated for this role. 136 */ 137 @Child(name = "endpoint", type = {Endpoint.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 138 @Description(shortDefinition="Technical endpoints providing access to services operated for this role", formalDefinition="Technical endpoints providing access to services operated for this role." ) 139 protected List<Reference> endpoint; 140 141 private static final long serialVersionUID = -509714744L; 142 143 /** 144 * Constructor 145 */ 146 public OrganizationAffiliation() { 147 super(); 148 } 149 150 /** 151 * @return {@link #identifier} (Business identifiers that are specific to this role.) 152 */ 153 public List<Identifier> getIdentifier() { 154 if (this.identifier == null) 155 this.identifier = new ArrayList<Identifier>(); 156 return this.identifier; 157 } 158 159 /** 160 * @return Returns a reference to <code>this</code> for easy method chaining 161 */ 162 public OrganizationAffiliation setIdentifier(List<Identifier> theIdentifier) { 163 this.identifier = theIdentifier; 164 return this; 165 } 166 167 public boolean hasIdentifier() { 168 if (this.identifier == null) 169 return false; 170 for (Identifier item : this.identifier) 171 if (!item.isEmpty()) 172 return true; 173 return false; 174 } 175 176 public Identifier addIdentifier() { //3 177 Identifier t = new Identifier(); 178 if (this.identifier == null) 179 this.identifier = new ArrayList<Identifier>(); 180 this.identifier.add(t); 181 return t; 182 } 183 184 public OrganizationAffiliation addIdentifier(Identifier t) { //3 185 if (t == null) 186 return this; 187 if (this.identifier == null) 188 this.identifier = new ArrayList<Identifier>(); 189 this.identifier.add(t); 190 return this; 191 } 192 193 /** 194 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 195 */ 196 public Identifier getIdentifierFirstRep() { 197 if (getIdentifier().isEmpty()) { 198 addIdentifier(); 199 } 200 return getIdentifier().get(0); 201 } 202 203 /** 204 * @return {@link #active} (Whether this organization affiliation record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 205 */ 206 public BooleanType getActiveElement() { 207 if (this.active == null) 208 if (Configuration.errorOnAutoCreate()) 209 throw new Error("Attempt to auto-create OrganizationAffiliation.active"); 210 else if (Configuration.doAutoCreate()) 211 this.active = new BooleanType(); // bb 212 return this.active; 213 } 214 215 public boolean hasActiveElement() { 216 return this.active != null && !this.active.isEmpty(); 217 } 218 219 public boolean hasActive() { 220 return this.active != null && !this.active.isEmpty(); 221 } 222 223 /** 224 * @param value {@link #active} (Whether this organization affiliation record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 225 */ 226 public OrganizationAffiliation setActiveElement(BooleanType value) { 227 this.active = value; 228 return this; 229 } 230 231 /** 232 * @return Whether this organization affiliation record is in active use. 233 */ 234 public boolean getActive() { 235 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 236 } 237 238 /** 239 * @param value Whether this organization affiliation record is in active use. 240 */ 241 public OrganizationAffiliation setActive(boolean value) { 242 if (this.active == null) 243 this.active = new BooleanType(); 244 this.active.setValue(value); 245 return this; 246 } 247 248 /** 249 * @return {@link #period} (The period during which the participatingOrganization is affiliated with the primary organization.) 250 */ 251 public Period getPeriod() { 252 if (this.period == null) 253 if (Configuration.errorOnAutoCreate()) 254 throw new Error("Attempt to auto-create OrganizationAffiliation.period"); 255 else if (Configuration.doAutoCreate()) 256 this.period = new Period(); // cc 257 return this.period; 258 } 259 260 public boolean hasPeriod() { 261 return this.period != null && !this.period.isEmpty(); 262 } 263 264 /** 265 * @param value {@link #period} (The period during which the participatingOrganization is affiliated with the primary organization.) 266 */ 267 public OrganizationAffiliation setPeriod(Period value) { 268 this.period = value; 269 return this; 270 } 271 272 /** 273 * @return {@link #organization} (Organization where the role is available (primary organization/has members).) 274 */ 275 public Reference getOrganization() { 276 if (this.organization == null) 277 if (Configuration.errorOnAutoCreate()) 278 throw new Error("Attempt to auto-create OrganizationAffiliation.organization"); 279 else if (Configuration.doAutoCreate()) 280 this.organization = new Reference(); // cc 281 return this.organization; 282 } 283 284 public boolean hasOrganization() { 285 return this.organization != null && !this.organization.isEmpty(); 286 } 287 288 /** 289 * @param value {@link #organization} (Organization where the role is available (primary organization/has members).) 290 */ 291 public OrganizationAffiliation setOrganization(Reference value) { 292 this.organization = value; 293 return this; 294 } 295 296 /** 297 * @return {@link #participatingOrganization} (The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of).) 298 */ 299 public Reference getParticipatingOrganization() { 300 if (this.participatingOrganization == null) 301 if (Configuration.errorOnAutoCreate()) 302 throw new Error("Attempt to auto-create OrganizationAffiliation.participatingOrganization"); 303 else if (Configuration.doAutoCreate()) 304 this.participatingOrganization = new Reference(); // cc 305 return this.participatingOrganization; 306 } 307 308 public boolean hasParticipatingOrganization() { 309 return this.participatingOrganization != null && !this.participatingOrganization.isEmpty(); 310 } 311 312 /** 313 * @param value {@link #participatingOrganization} (The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of).) 314 */ 315 public OrganizationAffiliation setParticipatingOrganization(Reference value) { 316 this.participatingOrganization = value; 317 return this; 318 } 319 320 /** 321 * @return {@link #network} (The network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined).) 322 */ 323 public List<Reference> getNetwork() { 324 if (this.network == null) 325 this.network = new ArrayList<Reference>(); 326 return this.network; 327 } 328 329 /** 330 * @return Returns a reference to <code>this</code> for easy method chaining 331 */ 332 public OrganizationAffiliation setNetwork(List<Reference> theNetwork) { 333 this.network = theNetwork; 334 return this; 335 } 336 337 public boolean hasNetwork() { 338 if (this.network == null) 339 return false; 340 for (Reference item : this.network) 341 if (!item.isEmpty()) 342 return true; 343 return false; 344 } 345 346 public Reference addNetwork() { //3 347 Reference t = new Reference(); 348 if (this.network == null) 349 this.network = new ArrayList<Reference>(); 350 this.network.add(t); 351 return t; 352 } 353 354 public OrganizationAffiliation addNetwork(Reference t) { //3 355 if (t == null) 356 return this; 357 if (this.network == null) 358 this.network = new ArrayList<Reference>(); 359 this.network.add(t); 360 return this; 361 } 362 363 /** 364 * @return The first repetition of repeating field {@link #network}, creating it if it does not already exist {3} 365 */ 366 public Reference getNetworkFirstRep() { 367 if (getNetwork().isEmpty()) { 368 addNetwork(); 369 } 370 return getNetwork().get(0); 371 } 372 373 /** 374 * @return {@link #code} (Definition of the role the participatingOrganization plays in the association.) 375 */ 376 public List<CodeableConcept> getCode() { 377 if (this.code == null) 378 this.code = new ArrayList<CodeableConcept>(); 379 return this.code; 380 } 381 382 /** 383 * @return Returns a reference to <code>this</code> for easy method chaining 384 */ 385 public OrganizationAffiliation setCode(List<CodeableConcept> theCode) { 386 this.code = theCode; 387 return this; 388 } 389 390 public boolean hasCode() { 391 if (this.code == null) 392 return false; 393 for (CodeableConcept item : this.code) 394 if (!item.isEmpty()) 395 return true; 396 return false; 397 } 398 399 public CodeableConcept addCode() { //3 400 CodeableConcept t = new CodeableConcept(); 401 if (this.code == null) 402 this.code = new ArrayList<CodeableConcept>(); 403 this.code.add(t); 404 return t; 405 } 406 407 public OrganizationAffiliation addCode(CodeableConcept t) { //3 408 if (t == null) 409 return this; 410 if (this.code == null) 411 this.code = new ArrayList<CodeableConcept>(); 412 this.code.add(t); 413 return this; 414 } 415 416 /** 417 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 418 */ 419 public CodeableConcept getCodeFirstRep() { 420 if (getCode().isEmpty()) { 421 addCode(); 422 } 423 return getCode().get(0); 424 } 425 426 /** 427 * @return {@link #specialty} (Specific specialty of the participatingOrganization in the context of the role.) 428 */ 429 public List<CodeableConcept> getSpecialty() { 430 if (this.specialty == null) 431 this.specialty = new ArrayList<CodeableConcept>(); 432 return this.specialty; 433 } 434 435 /** 436 * @return Returns a reference to <code>this</code> for easy method chaining 437 */ 438 public OrganizationAffiliation setSpecialty(List<CodeableConcept> theSpecialty) { 439 this.specialty = theSpecialty; 440 return this; 441 } 442 443 public boolean hasSpecialty() { 444 if (this.specialty == null) 445 return false; 446 for (CodeableConcept item : this.specialty) 447 if (!item.isEmpty()) 448 return true; 449 return false; 450 } 451 452 public CodeableConcept addSpecialty() { //3 453 CodeableConcept t = new CodeableConcept(); 454 if (this.specialty == null) 455 this.specialty = new ArrayList<CodeableConcept>(); 456 this.specialty.add(t); 457 return t; 458 } 459 460 public OrganizationAffiliation addSpecialty(CodeableConcept t) { //3 461 if (t == null) 462 return this; 463 if (this.specialty == null) 464 this.specialty = new ArrayList<CodeableConcept>(); 465 this.specialty.add(t); 466 return this; 467 } 468 469 /** 470 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist {3} 471 */ 472 public CodeableConcept getSpecialtyFirstRep() { 473 if (getSpecialty().isEmpty()) { 474 addSpecialty(); 475 } 476 return getSpecialty().get(0); 477 } 478 479 /** 480 * @return {@link #location} (The location(s) at which the role occurs.) 481 */ 482 public List<Reference> getLocation() { 483 if (this.location == null) 484 this.location = new ArrayList<Reference>(); 485 return this.location; 486 } 487 488 /** 489 * @return Returns a reference to <code>this</code> for easy method chaining 490 */ 491 public OrganizationAffiliation setLocation(List<Reference> theLocation) { 492 this.location = theLocation; 493 return this; 494 } 495 496 public boolean hasLocation() { 497 if (this.location == null) 498 return false; 499 for (Reference item : this.location) 500 if (!item.isEmpty()) 501 return true; 502 return false; 503 } 504 505 public Reference addLocation() { //3 506 Reference t = new Reference(); 507 if (this.location == null) 508 this.location = new ArrayList<Reference>(); 509 this.location.add(t); 510 return t; 511 } 512 513 public OrganizationAffiliation addLocation(Reference t) { //3 514 if (t == null) 515 return this; 516 if (this.location == null) 517 this.location = new ArrayList<Reference>(); 518 this.location.add(t); 519 return this; 520 } 521 522 /** 523 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist {3} 524 */ 525 public Reference getLocationFirstRep() { 526 if (getLocation().isEmpty()) { 527 addLocation(); 528 } 529 return getLocation().get(0); 530 } 531 532 /** 533 * @return {@link #healthcareService} (Healthcare services provided through the role.) 534 */ 535 public List<Reference> getHealthcareService() { 536 if (this.healthcareService == null) 537 this.healthcareService = new ArrayList<Reference>(); 538 return this.healthcareService; 539 } 540 541 /** 542 * @return Returns a reference to <code>this</code> for easy method chaining 543 */ 544 public OrganizationAffiliation setHealthcareService(List<Reference> theHealthcareService) { 545 this.healthcareService = theHealthcareService; 546 return this; 547 } 548 549 public boolean hasHealthcareService() { 550 if (this.healthcareService == null) 551 return false; 552 for (Reference item : this.healthcareService) 553 if (!item.isEmpty()) 554 return true; 555 return false; 556 } 557 558 public Reference addHealthcareService() { //3 559 Reference t = new Reference(); 560 if (this.healthcareService == null) 561 this.healthcareService = new ArrayList<Reference>(); 562 this.healthcareService.add(t); 563 return t; 564 } 565 566 public OrganizationAffiliation addHealthcareService(Reference t) { //3 567 if (t == null) 568 return this; 569 if (this.healthcareService == null) 570 this.healthcareService = new ArrayList<Reference>(); 571 this.healthcareService.add(t); 572 return this; 573 } 574 575 /** 576 * @return The first repetition of repeating field {@link #healthcareService}, creating it if it does not already exist {3} 577 */ 578 public Reference getHealthcareServiceFirstRep() { 579 if (getHealthcareService().isEmpty()) { 580 addHealthcareService(); 581 } 582 return getHealthcareService().get(0); 583 } 584 585 /** 586 * @return {@link #contact} (The contact details of communication devices available at the participatingOrganization relevant to this Affiliation.) 587 */ 588 public List<ExtendedContactDetail> getContact() { 589 if (this.contact == null) 590 this.contact = new ArrayList<ExtendedContactDetail>(); 591 return this.contact; 592 } 593 594 /** 595 * @return Returns a reference to <code>this</code> for easy method chaining 596 */ 597 public OrganizationAffiliation setContact(List<ExtendedContactDetail> theContact) { 598 this.contact = theContact; 599 return this; 600 } 601 602 public boolean hasContact() { 603 if (this.contact == null) 604 return false; 605 for (ExtendedContactDetail item : this.contact) 606 if (!item.isEmpty()) 607 return true; 608 return false; 609 } 610 611 public ExtendedContactDetail addContact() { //3 612 ExtendedContactDetail t = new ExtendedContactDetail(); 613 if (this.contact == null) 614 this.contact = new ArrayList<ExtendedContactDetail>(); 615 this.contact.add(t); 616 return t; 617 } 618 619 public OrganizationAffiliation addContact(ExtendedContactDetail t) { //3 620 if (t == null) 621 return this; 622 if (this.contact == null) 623 this.contact = new ArrayList<ExtendedContactDetail>(); 624 this.contact.add(t); 625 return this; 626 } 627 628 /** 629 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 630 */ 631 public ExtendedContactDetail getContactFirstRep() { 632 if (getContact().isEmpty()) { 633 addContact(); 634 } 635 return getContact().get(0); 636 } 637 638 /** 639 * @return {@link #endpoint} (Technical endpoints providing access to services operated for this role.) 640 */ 641 public List<Reference> getEndpoint() { 642 if (this.endpoint == null) 643 this.endpoint = new ArrayList<Reference>(); 644 return this.endpoint; 645 } 646 647 /** 648 * @return Returns a reference to <code>this</code> for easy method chaining 649 */ 650 public OrganizationAffiliation setEndpoint(List<Reference> theEndpoint) { 651 this.endpoint = theEndpoint; 652 return this; 653 } 654 655 public boolean hasEndpoint() { 656 if (this.endpoint == null) 657 return false; 658 for (Reference item : this.endpoint) 659 if (!item.isEmpty()) 660 return true; 661 return false; 662 } 663 664 public Reference addEndpoint() { //3 665 Reference t = new Reference(); 666 if (this.endpoint == null) 667 this.endpoint = new ArrayList<Reference>(); 668 this.endpoint.add(t); 669 return t; 670 } 671 672 public OrganizationAffiliation addEndpoint(Reference t) { //3 673 if (t == null) 674 return this; 675 if (this.endpoint == null) 676 this.endpoint = new ArrayList<Reference>(); 677 this.endpoint.add(t); 678 return this; 679 } 680 681 /** 682 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 683 */ 684 public Reference getEndpointFirstRep() { 685 if (getEndpoint().isEmpty()) { 686 addEndpoint(); 687 } 688 return getEndpoint().get(0); 689 } 690 691 protected void listChildren(List<Property> children) { 692 super.listChildren(children); 693 children.add(new Property("identifier", "Identifier", "Business identifiers that are specific to this role.", 0, java.lang.Integer.MAX_VALUE, identifier)); 694 children.add(new Property("active", "boolean", "Whether this organization affiliation record is in active use.", 0, 1, active)); 695 children.add(new Property("period", "Period", "The period during which the participatingOrganization is affiliated with the primary organization.", 0, 1, period)); 696 children.add(new Property("organization", "Reference(Organization)", "Organization where the role is available (primary organization/has members).", 0, 1, organization)); 697 children.add(new Property("participatingOrganization", "Reference(Organization)", "The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of).", 0, 1, participatingOrganization)); 698 children.add(new Property("network", "Reference(Organization)", "The network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined).", 0, java.lang.Integer.MAX_VALUE, network)); 699 children.add(new Property("code", "CodeableConcept", "Definition of the role the participatingOrganization plays in the association.", 0, java.lang.Integer.MAX_VALUE, code)); 700 children.add(new Property("specialty", "CodeableConcept", "Specific specialty of the participatingOrganization in the context of the role.", 0, java.lang.Integer.MAX_VALUE, specialty)); 701 children.add(new Property("location", "Reference(Location)", "The location(s) at which the role occurs.", 0, java.lang.Integer.MAX_VALUE, location)); 702 children.add(new Property("healthcareService", "Reference(HealthcareService)", "Healthcare services provided through the role.", 0, java.lang.Integer.MAX_VALUE, healthcareService)); 703 children.add(new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available at the participatingOrganization relevant to this Affiliation.", 0, java.lang.Integer.MAX_VALUE, contact)); 704 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for this role.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 705 } 706 707 @Override 708 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 709 switch (_hash) { 710 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers that are specific to this role.", 0, java.lang.Integer.MAX_VALUE, identifier); 711 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this organization affiliation record is in active use.", 0, 1, active); 712 case -991726143: /*period*/ return new Property("period", "Period", "The period during which the participatingOrganization is affiliated with the primary organization.", 0, 1, period); 713 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "Organization where the role is available (primary organization/has members).", 0, 1, organization); 714 case 1593310702: /*participatingOrganization*/ return new Property("participatingOrganization", "Reference(Organization)", "The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of).", 0, 1, participatingOrganization); 715 case 1843485230: /*network*/ return new Property("network", "Reference(Organization)", "The network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined).", 0, java.lang.Integer.MAX_VALUE, network); 716 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Definition of the role the participatingOrganization plays in the association.", 0, java.lang.Integer.MAX_VALUE, code); 717 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "Specific specialty of the participatingOrganization in the context of the role.", 0, java.lang.Integer.MAX_VALUE, specialty); 718 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location(s) at which the role occurs.", 0, java.lang.Integer.MAX_VALUE, location); 719 case 1289661064: /*healthcareService*/ return new Property("healthcareService", "Reference(HealthcareService)", "Healthcare services provided through the role.", 0, java.lang.Integer.MAX_VALUE, healthcareService); 720 case 951526432: /*contact*/ return new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available at the participatingOrganization relevant to this Affiliation.", 0, java.lang.Integer.MAX_VALUE, contact); 721 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for this role.", 0, java.lang.Integer.MAX_VALUE, endpoint); 722 default: return super.getNamedProperty(_hash, _name, _checkValid); 723 } 724 725 } 726 727 @Override 728 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 729 switch (hash) { 730 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 731 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 732 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 733 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 734 case 1593310702: /*participatingOrganization*/ return this.participatingOrganization == null ? new Base[0] : new Base[] {this.participatingOrganization}; // Reference 735 case 1843485230: /*network*/ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 736 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 737 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 738 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 739 case 1289661064: /*healthcareService*/ return this.healthcareService == null ? new Base[0] : this.healthcareService.toArray(new Base[this.healthcareService.size()]); // Reference 740 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ExtendedContactDetail 741 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 742 default: return super.getProperty(hash, name, checkValid); 743 } 744 745 } 746 747 @Override 748 public Base setProperty(int hash, String name, Base value) throws FHIRException { 749 switch (hash) { 750 case -1618432855: // identifier 751 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 752 return value; 753 case -1422950650: // active 754 this.active = TypeConvertor.castToBoolean(value); // BooleanType 755 return value; 756 case -991726143: // period 757 this.period = TypeConvertor.castToPeriod(value); // Period 758 return value; 759 case 1178922291: // organization 760 this.organization = TypeConvertor.castToReference(value); // Reference 761 return value; 762 case 1593310702: // participatingOrganization 763 this.participatingOrganization = TypeConvertor.castToReference(value); // Reference 764 return value; 765 case 1843485230: // network 766 this.getNetwork().add(TypeConvertor.castToReference(value)); // Reference 767 return value; 768 case 3059181: // code 769 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 770 return value; 771 case -1694759682: // specialty 772 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 773 return value; 774 case 1901043637: // location 775 this.getLocation().add(TypeConvertor.castToReference(value)); // Reference 776 return value; 777 case 1289661064: // healthcareService 778 this.getHealthcareService().add(TypeConvertor.castToReference(value)); // Reference 779 return value; 780 case 951526432: // contact 781 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); // ExtendedContactDetail 782 return value; 783 case 1741102485: // endpoint 784 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 785 return value; 786 default: return super.setProperty(hash, name, value); 787 } 788 789 } 790 791 @Override 792 public Base setProperty(String name, Base value) throws FHIRException { 793 if (name.equals("identifier")) { 794 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 795 } else if (name.equals("active")) { 796 this.active = TypeConvertor.castToBoolean(value); // BooleanType 797 } else if (name.equals("period")) { 798 this.period = TypeConvertor.castToPeriod(value); // Period 799 } else if (name.equals("organization")) { 800 this.organization = TypeConvertor.castToReference(value); // Reference 801 } else if (name.equals("participatingOrganization")) { 802 this.participatingOrganization = TypeConvertor.castToReference(value); // Reference 803 } else if (name.equals("network")) { 804 this.getNetwork().add(TypeConvertor.castToReference(value)); 805 } else if (name.equals("code")) { 806 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 807 } else if (name.equals("specialty")) { 808 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); 809 } else if (name.equals("location")) { 810 this.getLocation().add(TypeConvertor.castToReference(value)); 811 } else if (name.equals("healthcareService")) { 812 this.getHealthcareService().add(TypeConvertor.castToReference(value)); 813 } else if (name.equals("contact")) { 814 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); 815 } else if (name.equals("endpoint")) { 816 this.getEndpoint().add(TypeConvertor.castToReference(value)); 817 } else 818 return super.setProperty(name, value); 819 return value; 820 } 821 822 @Override 823 public void removeChild(String name, Base value) throws FHIRException { 824 if (name.equals("identifier")) { 825 this.getIdentifier().remove(value); 826 } else if (name.equals("active")) { 827 this.active = null; 828 } else if (name.equals("period")) { 829 this.period = null; 830 } else if (name.equals("organization")) { 831 this.organization = null; 832 } else if (name.equals("participatingOrganization")) { 833 this.participatingOrganization = null; 834 } else if (name.equals("network")) { 835 this.getNetwork().remove(value); 836 } else if (name.equals("code")) { 837 this.getCode().remove(value); 838 } else if (name.equals("specialty")) { 839 this.getSpecialty().remove(value); 840 } else if (name.equals("location")) { 841 this.getLocation().remove(value); 842 } else if (name.equals("healthcareService")) { 843 this.getHealthcareService().remove(value); 844 } else if (name.equals("contact")) { 845 this.getContact().remove(value); 846 } else if (name.equals("endpoint")) { 847 this.getEndpoint().remove(value); 848 } else 849 super.removeChild(name, value); 850 851 } 852 853 @Override 854 public Base makeProperty(int hash, String name) throws FHIRException { 855 switch (hash) { 856 case -1618432855: return addIdentifier(); 857 case -1422950650: return getActiveElement(); 858 case -991726143: return getPeriod(); 859 case 1178922291: return getOrganization(); 860 case 1593310702: return getParticipatingOrganization(); 861 case 1843485230: return addNetwork(); 862 case 3059181: return addCode(); 863 case -1694759682: return addSpecialty(); 864 case 1901043637: return addLocation(); 865 case 1289661064: return addHealthcareService(); 866 case 951526432: return addContact(); 867 case 1741102485: return addEndpoint(); 868 default: return super.makeProperty(hash, name); 869 } 870 871 } 872 873 @Override 874 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 875 switch (hash) { 876 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 877 case -1422950650: /*active*/ return new String[] {"boolean"}; 878 case -991726143: /*period*/ return new String[] {"Period"}; 879 case 1178922291: /*organization*/ return new String[] {"Reference"}; 880 case 1593310702: /*participatingOrganization*/ return new String[] {"Reference"}; 881 case 1843485230: /*network*/ return new String[] {"Reference"}; 882 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 883 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 884 case 1901043637: /*location*/ return new String[] {"Reference"}; 885 case 1289661064: /*healthcareService*/ return new String[] {"Reference"}; 886 case 951526432: /*contact*/ return new String[] {"ExtendedContactDetail"}; 887 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 888 default: return super.getTypesForProperty(hash, name); 889 } 890 891 } 892 893 @Override 894 public Base addChild(String name) throws FHIRException { 895 if (name.equals("identifier")) { 896 return addIdentifier(); 897 } 898 else if (name.equals("active")) { 899 throw new FHIRException("Cannot call addChild on a singleton property OrganizationAffiliation.active"); 900 } 901 else if (name.equals("period")) { 902 this.period = new Period(); 903 return this.period; 904 } 905 else if (name.equals("organization")) { 906 this.organization = new Reference(); 907 return this.organization; 908 } 909 else if (name.equals("participatingOrganization")) { 910 this.participatingOrganization = new Reference(); 911 return this.participatingOrganization; 912 } 913 else if (name.equals("network")) { 914 return addNetwork(); 915 } 916 else if (name.equals("code")) { 917 return addCode(); 918 } 919 else if (name.equals("specialty")) { 920 return addSpecialty(); 921 } 922 else if (name.equals("location")) { 923 return addLocation(); 924 } 925 else if (name.equals("healthcareService")) { 926 return addHealthcareService(); 927 } 928 else if (name.equals("contact")) { 929 return addContact(); 930 } 931 else if (name.equals("endpoint")) { 932 return addEndpoint(); 933 } 934 else 935 return super.addChild(name); 936 } 937 938 public String fhirType() { 939 return "OrganizationAffiliation"; 940 941 } 942 943 public OrganizationAffiliation copy() { 944 OrganizationAffiliation dst = new OrganizationAffiliation(); 945 copyValues(dst); 946 return dst; 947 } 948 949 public void copyValues(OrganizationAffiliation dst) { 950 super.copyValues(dst); 951 if (identifier != null) { 952 dst.identifier = new ArrayList<Identifier>(); 953 for (Identifier i : identifier) 954 dst.identifier.add(i.copy()); 955 }; 956 dst.active = active == null ? null : active.copy(); 957 dst.period = period == null ? null : period.copy(); 958 dst.organization = organization == null ? null : organization.copy(); 959 dst.participatingOrganization = participatingOrganization == null ? null : participatingOrganization.copy(); 960 if (network != null) { 961 dst.network = new ArrayList<Reference>(); 962 for (Reference i : network) 963 dst.network.add(i.copy()); 964 }; 965 if (code != null) { 966 dst.code = new ArrayList<CodeableConcept>(); 967 for (CodeableConcept i : code) 968 dst.code.add(i.copy()); 969 }; 970 if (specialty != null) { 971 dst.specialty = new ArrayList<CodeableConcept>(); 972 for (CodeableConcept i : specialty) 973 dst.specialty.add(i.copy()); 974 }; 975 if (location != null) { 976 dst.location = new ArrayList<Reference>(); 977 for (Reference i : location) 978 dst.location.add(i.copy()); 979 }; 980 if (healthcareService != null) { 981 dst.healthcareService = new ArrayList<Reference>(); 982 for (Reference i : healthcareService) 983 dst.healthcareService.add(i.copy()); 984 }; 985 if (contact != null) { 986 dst.contact = new ArrayList<ExtendedContactDetail>(); 987 for (ExtendedContactDetail i : contact) 988 dst.contact.add(i.copy()); 989 }; 990 if (endpoint != null) { 991 dst.endpoint = new ArrayList<Reference>(); 992 for (Reference i : endpoint) 993 dst.endpoint.add(i.copy()); 994 }; 995 } 996 997 protected OrganizationAffiliation typedCopy() { 998 return copy(); 999 } 1000 1001 @Override 1002 public boolean equalsDeep(Base other_) { 1003 if (!super.equalsDeep(other_)) 1004 return false; 1005 if (!(other_ instanceof OrganizationAffiliation)) 1006 return false; 1007 OrganizationAffiliation o = (OrganizationAffiliation) other_; 1008 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(period, o.period, true) 1009 && compareDeep(organization, o.organization, true) && compareDeep(participatingOrganization, o.participatingOrganization, true) 1010 && compareDeep(network, o.network, true) && compareDeep(code, o.code, true) && compareDeep(specialty, o.specialty, true) 1011 && compareDeep(location, o.location, true) && compareDeep(healthcareService, o.healthcareService, true) 1012 && compareDeep(contact, o.contact, true) && compareDeep(endpoint, o.endpoint, true); 1013 } 1014 1015 @Override 1016 public boolean equalsShallow(Base other_) { 1017 if (!super.equalsShallow(other_)) 1018 return false; 1019 if (!(other_ instanceof OrganizationAffiliation)) 1020 return false; 1021 OrganizationAffiliation o = (OrganizationAffiliation) other_; 1022 return compareValues(active, o.active, true); 1023 } 1024 1025 public boolean isEmpty() { 1026 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, period 1027 , organization, participatingOrganization, network, code, specialty, location, healthcareService 1028 , contact, endpoint); 1029 } 1030 1031 @Override 1032 public ResourceType getResourceType() { 1033 return ResourceType.OrganizationAffiliation; 1034 } 1035 1036 /** 1037 * Search parameter: <b>active</b> 1038 * <p> 1039 * Description: <b>Whether this organization affiliation record is in active use</b><br> 1040 * Type: <b>token</b><br> 1041 * Path: <b>OrganizationAffiliation.active</b><br> 1042 * </p> 1043 */ 1044 @SearchParamDefinition(name="active", path="OrganizationAffiliation.active", description="Whether this organization affiliation record is in active use", type="token" ) 1045 public static final String SP_ACTIVE = "active"; 1046 /** 1047 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1048 * <p> 1049 * Description: <b>Whether this organization affiliation record is in active use</b><br> 1050 * Type: <b>token</b><br> 1051 * Path: <b>OrganizationAffiliation.active</b><br> 1052 * </p> 1053 */ 1054 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1055 1056 /** 1057 * Search parameter: <b>date</b> 1058 * <p> 1059 * Description: <b>The period during which the participatingOrganization is affiliated with the primary organization</b><br> 1060 * Type: <b>date</b><br> 1061 * Path: <b>OrganizationAffiliation.period</b><br> 1062 * </p> 1063 */ 1064 @SearchParamDefinition(name="date", path="OrganizationAffiliation.period", description="The period during which the participatingOrganization is affiliated with the primary organization", type="date" ) 1065 public static final String SP_DATE = "date"; 1066 /** 1067 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1068 * <p> 1069 * Description: <b>The period during which the participatingOrganization is affiliated with the primary organization</b><br> 1070 * Type: <b>date</b><br> 1071 * Path: <b>OrganizationAffiliation.period</b><br> 1072 * </p> 1073 */ 1074 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1075 1076 /** 1077 * Search parameter: <b>email</b> 1078 * <p> 1079 * Description: <b>A value in an email contact</b><br> 1080 * Type: <b>token</b><br> 1081 * Path: <b>OrganizationAffiliation.contact.telecom.where(system='email')</b><br> 1082 * </p> 1083 */ 1084 @SearchParamDefinition(name="email", path="OrganizationAffiliation.contact.telecom.where(system='email')", description="A value in an email contact", type="token" ) 1085 public static final String SP_EMAIL = "email"; 1086 /** 1087 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1088 * <p> 1089 * Description: <b>A value in an email contact</b><br> 1090 * Type: <b>token</b><br> 1091 * Path: <b>OrganizationAffiliation.contact.telecom.where(system='email')</b><br> 1092 * </p> 1093 */ 1094 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 1095 1096 /** 1097 * Search parameter: <b>endpoint</b> 1098 * <p> 1099 * Description: <b>Technical endpoints providing access to services operated for this role</b><br> 1100 * Type: <b>reference</b><br> 1101 * Path: <b>OrganizationAffiliation.endpoint</b><br> 1102 * </p> 1103 */ 1104 @SearchParamDefinition(name="endpoint", path="OrganizationAffiliation.endpoint", description="Technical endpoints providing access to services operated for this role", type="reference", target={Endpoint.class } ) 1105 public static final String SP_ENDPOINT = "endpoint"; 1106 /** 1107 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1108 * <p> 1109 * Description: <b>Technical endpoints providing access to services operated for this role</b><br> 1110 * Type: <b>reference</b><br> 1111 * Path: <b>OrganizationAffiliation.endpoint</b><br> 1112 * </p> 1113 */ 1114 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 1115 1116/** 1117 * Constant for fluent queries to be used to add include statements. Specifies 1118 * the path value of "<b>OrganizationAffiliation:endpoint</b>". 1119 */ 1120 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("OrganizationAffiliation:endpoint").toLocked(); 1121 1122 /** 1123 * Search parameter: <b>identifier</b> 1124 * <p> 1125 * Description: <b>An organization affiliation's Identifier</b><br> 1126 * Type: <b>token</b><br> 1127 * Path: <b>OrganizationAffiliation.identifier</b><br> 1128 * </p> 1129 */ 1130 @SearchParamDefinition(name="identifier", path="OrganizationAffiliation.identifier", description="An organization affiliation's Identifier", type="token" ) 1131 public static final String SP_IDENTIFIER = "identifier"; 1132 /** 1133 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1134 * <p> 1135 * Description: <b>An organization affiliation's Identifier</b><br> 1136 * Type: <b>token</b><br> 1137 * Path: <b>OrganizationAffiliation.identifier</b><br> 1138 * </p> 1139 */ 1140 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1141 1142 /** 1143 * Search parameter: <b>location</b> 1144 * <p> 1145 * Description: <b>The location(s) at which the role occurs</b><br> 1146 * Type: <b>reference</b><br> 1147 * Path: <b>OrganizationAffiliation.location</b><br> 1148 * </p> 1149 */ 1150 @SearchParamDefinition(name="location", path="OrganizationAffiliation.location", description="The location(s) at which the role occurs", type="reference", target={Location.class } ) 1151 public static final String SP_LOCATION = "location"; 1152 /** 1153 * <b>Fluent Client</b> search parameter constant for <b>location</b> 1154 * <p> 1155 * Description: <b>The location(s) at which the role occurs</b><br> 1156 * Type: <b>reference</b><br> 1157 * Path: <b>OrganizationAffiliation.location</b><br> 1158 * </p> 1159 */ 1160 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 1161 1162/** 1163 * Constant for fluent queries to be used to add include statements. Specifies 1164 * the path value of "<b>OrganizationAffiliation:location</b>". 1165 */ 1166 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("OrganizationAffiliation:location").toLocked(); 1167 1168 /** 1169 * Search parameter: <b>network</b> 1170 * <p> 1171 * Description: <b>Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined)</b><br> 1172 * Type: <b>reference</b><br> 1173 * Path: <b>OrganizationAffiliation.network</b><br> 1174 * </p> 1175 */ 1176 @SearchParamDefinition(name="network", path="OrganizationAffiliation.network", description="Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined)", type="reference", target={Organization.class } ) 1177 public static final String SP_NETWORK = "network"; 1178 /** 1179 * <b>Fluent Client</b> search parameter constant for <b>network</b> 1180 * <p> 1181 * Description: <b>Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined)</b><br> 1182 * Type: <b>reference</b><br> 1183 * Path: <b>OrganizationAffiliation.network</b><br> 1184 * </p> 1185 */ 1186 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam NETWORK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_NETWORK); 1187 1188/** 1189 * Constant for fluent queries to be used to add include statements. Specifies 1190 * the path value of "<b>OrganizationAffiliation:network</b>". 1191 */ 1192 public static final ca.uhn.fhir.model.api.Include INCLUDE_NETWORK = new ca.uhn.fhir.model.api.Include("OrganizationAffiliation:network").toLocked(); 1193 1194 /** 1195 * Search parameter: <b>participating-organization</b> 1196 * <p> 1197 * Description: <b>The organization that provides services to the primary organization</b><br> 1198 * Type: <b>reference</b><br> 1199 * Path: <b>OrganizationAffiliation.participatingOrganization</b><br> 1200 * </p> 1201 */ 1202 @SearchParamDefinition(name="participating-organization", path="OrganizationAffiliation.participatingOrganization", description="The organization that provides services to the primary organization", type="reference", target={Organization.class } ) 1203 public static final String SP_PARTICIPATING_ORGANIZATION = "participating-organization"; 1204 /** 1205 * <b>Fluent Client</b> search parameter constant for <b>participating-organization</b> 1206 * <p> 1207 * Description: <b>The organization that provides services to the primary organization</b><br> 1208 * Type: <b>reference</b><br> 1209 * Path: <b>OrganizationAffiliation.participatingOrganization</b><br> 1210 * </p> 1211 */ 1212 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPATING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPATING_ORGANIZATION); 1213 1214/** 1215 * Constant for fluent queries to be used to add include statements. Specifies 1216 * the path value of "<b>OrganizationAffiliation:participating-organization</b>". 1217 */ 1218 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPATING_ORGANIZATION = new ca.uhn.fhir.model.api.Include("OrganizationAffiliation:participating-organization").toLocked(); 1219 1220 /** 1221 * Search parameter: <b>phone</b> 1222 * <p> 1223 * Description: <b>A value in a phone contact</b><br> 1224 * Type: <b>token</b><br> 1225 * Path: <b>OrganizationAffiliation.contact.telecom.where(system='phone')</b><br> 1226 * </p> 1227 */ 1228 @SearchParamDefinition(name="phone", path="OrganizationAffiliation.contact.telecom.where(system='phone')", description="A value in a phone contact", type="token" ) 1229 public static final String SP_PHONE = "phone"; 1230 /** 1231 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1232 * <p> 1233 * Description: <b>A value in a phone contact</b><br> 1234 * Type: <b>token</b><br> 1235 * Path: <b>OrganizationAffiliation.contact.telecom.where(system='phone')</b><br> 1236 * </p> 1237 */ 1238 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 1239 1240 /** 1241 * Search parameter: <b>primary-organization</b> 1242 * <p> 1243 * Description: <b>The organization that receives the services from the participating organization</b><br> 1244 * Type: <b>reference</b><br> 1245 * Path: <b>OrganizationAffiliation.organization</b><br> 1246 * </p> 1247 */ 1248 @SearchParamDefinition(name="primary-organization", path="OrganizationAffiliation.organization", description="The organization that receives the services from the participating organization", type="reference", target={Organization.class } ) 1249 public static final String SP_PRIMARY_ORGANIZATION = "primary-organization"; 1250 /** 1251 * <b>Fluent Client</b> search parameter constant for <b>primary-organization</b> 1252 * <p> 1253 * Description: <b>The organization that receives the services from the participating organization</b><br> 1254 * Type: <b>reference</b><br> 1255 * Path: <b>OrganizationAffiliation.organization</b><br> 1256 * </p> 1257 */ 1258 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRIMARY_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRIMARY_ORGANIZATION); 1259 1260/** 1261 * Constant for fluent queries to be used to add include statements. Specifies 1262 * the path value of "<b>OrganizationAffiliation:primary-organization</b>". 1263 */ 1264 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRIMARY_ORGANIZATION = new ca.uhn.fhir.model.api.Include("OrganizationAffiliation:primary-organization").toLocked(); 1265 1266 /** 1267 * Search parameter: <b>role</b> 1268 * <p> 1269 * Description: <b>Definition of the role the participatingOrganization plays</b><br> 1270 * Type: <b>token</b><br> 1271 * Path: <b>OrganizationAffiliation.code</b><br> 1272 * </p> 1273 */ 1274 @SearchParamDefinition(name="role", path="OrganizationAffiliation.code", description="Definition of the role the participatingOrganization plays", type="token" ) 1275 public static final String SP_ROLE = "role"; 1276 /** 1277 * <b>Fluent Client</b> search parameter constant for <b>role</b> 1278 * <p> 1279 * Description: <b>Definition of the role the participatingOrganization plays</b><br> 1280 * Type: <b>token</b><br> 1281 * Path: <b>OrganizationAffiliation.code</b><br> 1282 * </p> 1283 */ 1284 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROLE); 1285 1286 /** 1287 * Search parameter: <b>service</b> 1288 * <p> 1289 * Description: <b>Healthcare services provided through the role</b><br> 1290 * Type: <b>reference</b><br> 1291 * Path: <b>OrganizationAffiliation.healthcareService</b><br> 1292 * </p> 1293 */ 1294 @SearchParamDefinition(name="service", path="OrganizationAffiliation.healthcareService", description="Healthcare services provided through the role", type="reference", target={HealthcareService.class } ) 1295 public static final String SP_SERVICE = "service"; 1296 /** 1297 * <b>Fluent Client</b> search parameter constant for <b>service</b> 1298 * <p> 1299 * Description: <b>Healthcare services provided through the role</b><br> 1300 * Type: <b>reference</b><br> 1301 * Path: <b>OrganizationAffiliation.healthcareService</b><br> 1302 * </p> 1303 */ 1304 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE); 1305 1306/** 1307 * Constant for fluent queries to be used to add include statements. Specifies 1308 * the path value of "<b>OrganizationAffiliation:service</b>". 1309 */ 1310 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include("OrganizationAffiliation:service").toLocked(); 1311 1312 /** 1313 * Search parameter: <b>specialty</b> 1314 * <p> 1315 * Description: <b>Specific specialty of the participatingOrganization in the context of the role</b><br> 1316 * Type: <b>token</b><br> 1317 * Path: <b>OrganizationAffiliation.specialty</b><br> 1318 * </p> 1319 */ 1320 @SearchParamDefinition(name="specialty", path="OrganizationAffiliation.specialty", description="Specific specialty of the participatingOrganization in the context of the role", type="token" ) 1321 public static final String SP_SPECIALTY = "specialty"; 1322 /** 1323 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 1324 * <p> 1325 * Description: <b>Specific specialty of the participatingOrganization in the context of the role</b><br> 1326 * Type: <b>token</b><br> 1327 * Path: <b>OrganizationAffiliation.specialty</b><br> 1328 * </p> 1329 */ 1330 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 1331 1332 /** 1333 * Search parameter: <b>telecom</b> 1334 * <p> 1335 * Description: <b>The value in any kind of contact</b><br> 1336 * Type: <b>token</b><br> 1337 * Path: <b>OrganizationAffiliation.contact.telecom</b><br> 1338 * </p> 1339 */ 1340 @SearchParamDefinition(name="telecom", path="OrganizationAffiliation.contact.telecom", description="The value in any kind of contact", type="token" ) 1341 public static final String SP_TELECOM = "telecom"; 1342 /** 1343 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1344 * <p> 1345 * Description: <b>The value in any kind of contact</b><br> 1346 * Type: <b>token</b><br> 1347 * Path: <b>OrganizationAffiliation.contact.telecom</b><br> 1348 * </p> 1349 */ 1350 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 1351 1352 1353} 1354