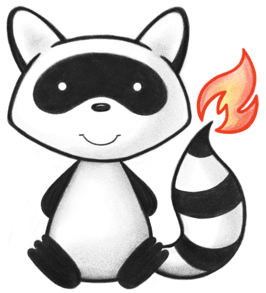
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A medically related item or items, in a container or package. 052 */ 053@ResourceDef(name="PackagedProductDefinition", profile="http://hl7.org/fhir/StructureDefinition/PackagedProductDefinition") 054public class PackagedProductDefinition extends DomainResource { 055 056 @Block() 057 public static class PackagedProductDefinitionLegalStatusOfSupplyComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The actual status of supply. Conveys in what situation this package type may be supplied for use. 060 */ 061 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="The actual status of supply. In what situation this package type may be supplied for use", formalDefinition="The actual status of supply. Conveys in what situation this package type may be supplied for use." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/legal-status-of-supply") 064 protected CodeableConcept code; 065 066 /** 067 * The place where the legal status of supply applies. When not specified, this indicates it is unknown in this context. 068 */ 069 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="The place where the legal status of supply applies", formalDefinition="The place where the legal status of supply applies. When not specified, this indicates it is unknown in this context." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 072 protected CodeableConcept jurisdiction; 073 074 private static final long serialVersionUID = 1072410156L; 075 076 /** 077 * Constructor 078 */ 079 public PackagedProductDefinitionLegalStatusOfSupplyComponent() { 080 super(); 081 } 082 083 /** 084 * @return {@link #code} (The actual status of supply. Conveys in what situation this package type may be supplied for use.) 085 */ 086 public CodeableConcept getCode() { 087 if (this.code == null) 088 if (Configuration.errorOnAutoCreate()) 089 throw new Error("Attempt to auto-create PackagedProductDefinitionLegalStatusOfSupplyComponent.code"); 090 else if (Configuration.doAutoCreate()) 091 this.code = new CodeableConcept(); // cc 092 return this.code; 093 } 094 095 public boolean hasCode() { 096 return this.code != null && !this.code.isEmpty(); 097 } 098 099 /** 100 * @param value {@link #code} (The actual status of supply. Conveys in what situation this package type may be supplied for use.) 101 */ 102 public PackagedProductDefinitionLegalStatusOfSupplyComponent setCode(CodeableConcept value) { 103 this.code = value; 104 return this; 105 } 106 107 /** 108 * @return {@link #jurisdiction} (The place where the legal status of supply applies. When not specified, this indicates it is unknown in this context.) 109 */ 110 public CodeableConcept getJurisdiction() { 111 if (this.jurisdiction == null) 112 if (Configuration.errorOnAutoCreate()) 113 throw new Error("Attempt to auto-create PackagedProductDefinitionLegalStatusOfSupplyComponent.jurisdiction"); 114 else if (Configuration.doAutoCreate()) 115 this.jurisdiction = new CodeableConcept(); // cc 116 return this.jurisdiction; 117 } 118 119 public boolean hasJurisdiction() { 120 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 121 } 122 123 /** 124 * @param value {@link #jurisdiction} (The place where the legal status of supply applies. When not specified, this indicates it is unknown in this context.) 125 */ 126 public PackagedProductDefinitionLegalStatusOfSupplyComponent setJurisdiction(CodeableConcept value) { 127 this.jurisdiction = value; 128 return this; 129 } 130 131 protected void listChildren(List<Property> children) { 132 super.listChildren(children); 133 children.add(new Property("code", "CodeableConcept", "The actual status of supply. Conveys in what situation this package type may be supplied for use.", 0, 1, code)); 134 children.add(new Property("jurisdiction", "CodeableConcept", "The place where the legal status of supply applies. When not specified, this indicates it is unknown in this context.", 0, 1, jurisdiction)); 135 } 136 137 @Override 138 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 139 switch (_hash) { 140 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The actual status of supply. Conveys in what situation this package type may be supplied for use.", 0, 1, code); 141 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "The place where the legal status of supply applies. When not specified, this indicates it is unknown in this context.", 0, 1, jurisdiction); 142 default: return super.getNamedProperty(_hash, _name, _checkValid); 143 } 144 145 } 146 147 @Override 148 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 149 switch (hash) { 150 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 151 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // CodeableConcept 152 default: return super.getProperty(hash, name, checkValid); 153 } 154 155 } 156 157 @Override 158 public Base setProperty(int hash, String name, Base value) throws FHIRException { 159 switch (hash) { 160 case 3059181: // code 161 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 162 return value; 163 case -507075711: // jurisdiction 164 this.jurisdiction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 165 return value; 166 default: return super.setProperty(hash, name, value); 167 } 168 169 } 170 171 @Override 172 public Base setProperty(String name, Base value) throws FHIRException { 173 if (name.equals("code")) { 174 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 175 } else if (name.equals("jurisdiction")) { 176 this.jurisdiction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 177 } else 178 return super.setProperty(name, value); 179 return value; 180 } 181 182 @Override 183 public void removeChild(String name, Base value) throws FHIRException { 184 if (name.equals("code")) { 185 this.code = null; 186 } else if (name.equals("jurisdiction")) { 187 this.jurisdiction = null; 188 } else 189 super.removeChild(name, value); 190 191 } 192 193 @Override 194 public Base makeProperty(int hash, String name) throws FHIRException { 195 switch (hash) { 196 case 3059181: return getCode(); 197 case -507075711: return getJurisdiction(); 198 default: return super.makeProperty(hash, name); 199 } 200 201 } 202 203 @Override 204 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 205 switch (hash) { 206 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 207 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 208 default: return super.getTypesForProperty(hash, name); 209 } 210 211 } 212 213 @Override 214 public Base addChild(String name) throws FHIRException { 215 if (name.equals("code")) { 216 this.code = new CodeableConcept(); 217 return this.code; 218 } 219 else if (name.equals("jurisdiction")) { 220 this.jurisdiction = new CodeableConcept(); 221 return this.jurisdiction; 222 } 223 else 224 return super.addChild(name); 225 } 226 227 public PackagedProductDefinitionLegalStatusOfSupplyComponent copy() { 228 PackagedProductDefinitionLegalStatusOfSupplyComponent dst = new PackagedProductDefinitionLegalStatusOfSupplyComponent(); 229 copyValues(dst); 230 return dst; 231 } 232 233 public void copyValues(PackagedProductDefinitionLegalStatusOfSupplyComponent dst) { 234 super.copyValues(dst); 235 dst.code = code == null ? null : code.copy(); 236 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 237 } 238 239 @Override 240 public boolean equalsDeep(Base other_) { 241 if (!super.equalsDeep(other_)) 242 return false; 243 if (!(other_ instanceof PackagedProductDefinitionLegalStatusOfSupplyComponent)) 244 return false; 245 PackagedProductDefinitionLegalStatusOfSupplyComponent o = (PackagedProductDefinitionLegalStatusOfSupplyComponent) other_; 246 return compareDeep(code, o.code, true) && compareDeep(jurisdiction, o.jurisdiction, true); 247 } 248 249 @Override 250 public boolean equalsShallow(Base other_) { 251 if (!super.equalsShallow(other_)) 252 return false; 253 if (!(other_ instanceof PackagedProductDefinitionLegalStatusOfSupplyComponent)) 254 return false; 255 PackagedProductDefinitionLegalStatusOfSupplyComponent o = (PackagedProductDefinitionLegalStatusOfSupplyComponent) other_; 256 return true; 257 } 258 259 public boolean isEmpty() { 260 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, jurisdiction); 261 } 262 263 public String fhirType() { 264 return "PackagedProductDefinition.legalStatusOfSupply"; 265 266 } 267 268 } 269 270 @Block() 271 public static class PackagedProductDefinitionPackagingComponent extends BackboneElement implements IBaseBackboneElement { 272 /** 273 * A business identifier that is specific to this particular part of the packaging, often assigned by the manufacturer. Including possibly Data Carrier Identifier (a GS1 barcode). 274 */ 275 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 276 @Description(shortDefinition="An identifier that is specific to this particular part of the packaging. Including possibly a Data Carrier Identifier", formalDefinition="A business identifier that is specific to this particular part of the packaging, often assigned by the manufacturer. Including possibly Data Carrier Identifier (a GS1 barcode)." ) 277 protected List<Identifier> identifier; 278 279 /** 280 * The physical type of the container of the items. 281 */ 282 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 283 @Description(shortDefinition="The physical type of the container of the items", formalDefinition="The physical type of the container of the items." ) 284 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/packaging-type") 285 protected CodeableConcept type; 286 287 /** 288 * Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not. 289 */ 290 @Child(name = "componentPart", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 291 @Description(shortDefinition="Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial)", formalDefinition="Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not." ) 292 protected BooleanType componentPart; 293 294 /** 295 * The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2. 296 */ 297 @Child(name = "quantity", type = {IntegerType.class}, order=4, min=0, max=1, modifier=false, summary=true) 298 @Description(shortDefinition="The quantity of this level of packaging in the package that contains it (with the outermost level being 1)", formalDefinition="The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2." ) 299 protected IntegerType quantity; 300 301 /** 302 * Material type of the package item. 303 */ 304 @Child(name = "material", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 305 @Description(shortDefinition="Material type of the package item", formalDefinition="Material type of the package item." ) 306 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/package-material") 307 protected List<CodeableConcept> material; 308 309 /** 310 * A possible alternate material for this part of the packaging, that is allowed to be used instead of the usual material (e.g. different types of plastic for a blister sleeve). 311 */ 312 @Child(name = "alternateMaterial", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 313 @Description(shortDefinition="A possible alternate material for this part of the packaging, that is allowed to be used instead of the usual material", formalDefinition="A possible alternate material for this part of the packaging, that is allowed to be used instead of the usual material (e.g. different types of plastic for a blister sleeve)." ) 314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/package-material") 315 protected List<CodeableConcept> alternateMaterial; 316 317 /** 318 * Shelf Life and storage information. 319 */ 320 @Child(name = "shelfLifeStorage", type = {ProductShelfLife.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 321 @Description(shortDefinition="Shelf Life and storage information", formalDefinition="Shelf Life and storage information." ) 322 protected List<ProductShelfLife> shelfLifeStorage; 323 324 /** 325 * Manufacturer of this packaging item. When there are multiple values each one is a potential manufacturer of this packaging item. 326 */ 327 @Child(name = "manufacturer", type = {Organization.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 328 @Description(shortDefinition="Manufacturer of this packaging item (multiple means these are all potential manufacturers)", formalDefinition="Manufacturer of this packaging item. When there are multiple values each one is a potential manufacturer of this packaging item." ) 329 protected List<Reference> manufacturer; 330 331 /** 332 * General characteristics of this item. 333 */ 334 @Child(name = "property", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 335 @Description(shortDefinition="General characteristics of this item", formalDefinition="General characteristics of this item." ) 336 protected List<PackagedProductDefinitionPackagingPropertyComponent> property; 337 338 /** 339 * The item(s) within the packaging. 340 */ 341 @Child(name = "containedItem", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 342 @Description(shortDefinition="The item(s) within the packaging", formalDefinition="The item(s) within the packaging." ) 343 protected List<PackagedProductDefinitionPackagingContainedItemComponent> containedItem; 344 345 /** 346 * Allows containers (and parts of containers) within containers, still as a part of a single packaged product. See also PackagedProductDefinition.packaging.containedItem.item(PackagedProductDefinition). 347 */ 348 @Child(name = "packaging", type = {PackagedProductDefinitionPackagingComponent.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 349 @Description(shortDefinition="Allows containers (and parts of containers) within containers, still as a part of single packaged product", formalDefinition="Allows containers (and parts of containers) within containers, still as a part of a single packaged product. See also PackagedProductDefinition.packaging.containedItem.item(PackagedProductDefinition)." ) 350 protected List<PackagedProductDefinitionPackagingComponent> packaging; 351 352 private static final long serialVersionUID = 2121836225L; 353 354 /** 355 * Constructor 356 */ 357 public PackagedProductDefinitionPackagingComponent() { 358 super(); 359 } 360 361 /** 362 * @return {@link #identifier} (A business identifier that is specific to this particular part of the packaging, often assigned by the manufacturer. Including possibly Data Carrier Identifier (a GS1 barcode).) 363 */ 364 public List<Identifier> getIdentifier() { 365 if (this.identifier == null) 366 this.identifier = new ArrayList<Identifier>(); 367 return this.identifier; 368 } 369 370 /** 371 * @return Returns a reference to <code>this</code> for easy method chaining 372 */ 373 public PackagedProductDefinitionPackagingComponent setIdentifier(List<Identifier> theIdentifier) { 374 this.identifier = theIdentifier; 375 return this; 376 } 377 378 public boolean hasIdentifier() { 379 if (this.identifier == null) 380 return false; 381 for (Identifier item : this.identifier) 382 if (!item.isEmpty()) 383 return true; 384 return false; 385 } 386 387 public Identifier addIdentifier() { //3 388 Identifier t = new Identifier(); 389 if (this.identifier == null) 390 this.identifier = new ArrayList<Identifier>(); 391 this.identifier.add(t); 392 return t; 393 } 394 395 public PackagedProductDefinitionPackagingComponent addIdentifier(Identifier t) { //3 396 if (t == null) 397 return this; 398 if (this.identifier == null) 399 this.identifier = new ArrayList<Identifier>(); 400 this.identifier.add(t); 401 return this; 402 } 403 404 /** 405 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 406 */ 407 public Identifier getIdentifierFirstRep() { 408 if (getIdentifier().isEmpty()) { 409 addIdentifier(); 410 } 411 return getIdentifier().get(0); 412 } 413 414 /** 415 * @return {@link #type} (The physical type of the container of the items.) 416 */ 417 public CodeableConcept getType() { 418 if (this.type == null) 419 if (Configuration.errorOnAutoCreate()) 420 throw new Error("Attempt to auto-create PackagedProductDefinitionPackagingComponent.type"); 421 else if (Configuration.doAutoCreate()) 422 this.type = new CodeableConcept(); // cc 423 return this.type; 424 } 425 426 public boolean hasType() { 427 return this.type != null && !this.type.isEmpty(); 428 } 429 430 /** 431 * @param value {@link #type} (The physical type of the container of the items.) 432 */ 433 public PackagedProductDefinitionPackagingComponent setType(CodeableConcept value) { 434 this.type = value; 435 return this; 436 } 437 438 /** 439 * @return {@link #componentPart} (Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not.). This is the underlying object with id, value and extensions. The accessor "getComponentPart" gives direct access to the value 440 */ 441 public BooleanType getComponentPartElement() { 442 if (this.componentPart == null) 443 if (Configuration.errorOnAutoCreate()) 444 throw new Error("Attempt to auto-create PackagedProductDefinitionPackagingComponent.componentPart"); 445 else if (Configuration.doAutoCreate()) 446 this.componentPart = new BooleanType(); // bb 447 return this.componentPart; 448 } 449 450 public boolean hasComponentPartElement() { 451 return this.componentPart != null && !this.componentPart.isEmpty(); 452 } 453 454 public boolean hasComponentPart() { 455 return this.componentPart != null && !this.componentPart.isEmpty(); 456 } 457 458 /** 459 * @param value {@link #componentPart} (Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not.). This is the underlying object with id, value and extensions. The accessor "getComponentPart" gives direct access to the value 460 */ 461 public PackagedProductDefinitionPackagingComponent setComponentPartElement(BooleanType value) { 462 this.componentPart = value; 463 return this; 464 } 465 466 /** 467 * @return Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not. 468 */ 469 public boolean getComponentPart() { 470 return this.componentPart == null || this.componentPart.isEmpty() ? false : this.componentPart.getValue(); 471 } 472 473 /** 474 * @param value Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not. 475 */ 476 public PackagedProductDefinitionPackagingComponent setComponentPart(boolean value) { 477 if (this.componentPart == null) 478 this.componentPart = new BooleanType(); 479 this.componentPart.setValue(value); 480 return this; 481 } 482 483 /** 484 * @return {@link #quantity} (The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 485 */ 486 public IntegerType getQuantityElement() { 487 if (this.quantity == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create PackagedProductDefinitionPackagingComponent.quantity"); 490 else if (Configuration.doAutoCreate()) 491 this.quantity = new IntegerType(); // bb 492 return this.quantity; 493 } 494 495 public boolean hasQuantityElement() { 496 return this.quantity != null && !this.quantity.isEmpty(); 497 } 498 499 public boolean hasQuantity() { 500 return this.quantity != null && !this.quantity.isEmpty(); 501 } 502 503 /** 504 * @param value {@link #quantity} (The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 505 */ 506 public PackagedProductDefinitionPackagingComponent setQuantityElement(IntegerType value) { 507 this.quantity = value; 508 return this; 509 } 510 511 /** 512 * @return The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2. 513 */ 514 public int getQuantity() { 515 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 516 } 517 518 /** 519 * @param value The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2. 520 */ 521 public PackagedProductDefinitionPackagingComponent setQuantity(int value) { 522 if (this.quantity == null) 523 this.quantity = new IntegerType(); 524 this.quantity.setValue(value); 525 return this; 526 } 527 528 /** 529 * @return {@link #material} (Material type of the package item.) 530 */ 531 public List<CodeableConcept> getMaterial() { 532 if (this.material == null) 533 this.material = new ArrayList<CodeableConcept>(); 534 return this.material; 535 } 536 537 /** 538 * @return Returns a reference to <code>this</code> for easy method chaining 539 */ 540 public PackagedProductDefinitionPackagingComponent setMaterial(List<CodeableConcept> theMaterial) { 541 this.material = theMaterial; 542 return this; 543 } 544 545 public boolean hasMaterial() { 546 if (this.material == null) 547 return false; 548 for (CodeableConcept item : this.material) 549 if (!item.isEmpty()) 550 return true; 551 return false; 552 } 553 554 public CodeableConcept addMaterial() { //3 555 CodeableConcept t = new CodeableConcept(); 556 if (this.material == null) 557 this.material = new ArrayList<CodeableConcept>(); 558 this.material.add(t); 559 return t; 560 } 561 562 public PackagedProductDefinitionPackagingComponent addMaterial(CodeableConcept t) { //3 563 if (t == null) 564 return this; 565 if (this.material == null) 566 this.material = new ArrayList<CodeableConcept>(); 567 this.material.add(t); 568 return this; 569 } 570 571 /** 572 * @return The first repetition of repeating field {@link #material}, creating it if it does not already exist {3} 573 */ 574 public CodeableConcept getMaterialFirstRep() { 575 if (getMaterial().isEmpty()) { 576 addMaterial(); 577 } 578 return getMaterial().get(0); 579 } 580 581 /** 582 * @return {@link #alternateMaterial} (A possible alternate material for this part of the packaging, that is allowed to be used instead of the usual material (e.g. different types of plastic for a blister sleeve).) 583 */ 584 public List<CodeableConcept> getAlternateMaterial() { 585 if (this.alternateMaterial == null) 586 this.alternateMaterial = new ArrayList<CodeableConcept>(); 587 return this.alternateMaterial; 588 } 589 590 /** 591 * @return Returns a reference to <code>this</code> for easy method chaining 592 */ 593 public PackagedProductDefinitionPackagingComponent setAlternateMaterial(List<CodeableConcept> theAlternateMaterial) { 594 this.alternateMaterial = theAlternateMaterial; 595 return this; 596 } 597 598 public boolean hasAlternateMaterial() { 599 if (this.alternateMaterial == null) 600 return false; 601 for (CodeableConcept item : this.alternateMaterial) 602 if (!item.isEmpty()) 603 return true; 604 return false; 605 } 606 607 public CodeableConcept addAlternateMaterial() { //3 608 CodeableConcept t = new CodeableConcept(); 609 if (this.alternateMaterial == null) 610 this.alternateMaterial = new ArrayList<CodeableConcept>(); 611 this.alternateMaterial.add(t); 612 return t; 613 } 614 615 public PackagedProductDefinitionPackagingComponent addAlternateMaterial(CodeableConcept t) { //3 616 if (t == null) 617 return this; 618 if (this.alternateMaterial == null) 619 this.alternateMaterial = new ArrayList<CodeableConcept>(); 620 this.alternateMaterial.add(t); 621 return this; 622 } 623 624 /** 625 * @return The first repetition of repeating field {@link #alternateMaterial}, creating it if it does not already exist {3} 626 */ 627 public CodeableConcept getAlternateMaterialFirstRep() { 628 if (getAlternateMaterial().isEmpty()) { 629 addAlternateMaterial(); 630 } 631 return getAlternateMaterial().get(0); 632 } 633 634 /** 635 * @return {@link #shelfLifeStorage} (Shelf Life and storage information.) 636 */ 637 public List<ProductShelfLife> getShelfLifeStorage() { 638 if (this.shelfLifeStorage == null) 639 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 640 return this.shelfLifeStorage; 641 } 642 643 /** 644 * @return Returns a reference to <code>this</code> for easy method chaining 645 */ 646 public PackagedProductDefinitionPackagingComponent setShelfLifeStorage(List<ProductShelfLife> theShelfLifeStorage) { 647 this.shelfLifeStorage = theShelfLifeStorage; 648 return this; 649 } 650 651 public boolean hasShelfLifeStorage() { 652 if (this.shelfLifeStorage == null) 653 return false; 654 for (ProductShelfLife item : this.shelfLifeStorage) 655 if (!item.isEmpty()) 656 return true; 657 return false; 658 } 659 660 public ProductShelfLife addShelfLifeStorage() { //3 661 ProductShelfLife t = new ProductShelfLife(); 662 if (this.shelfLifeStorage == null) 663 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 664 this.shelfLifeStorage.add(t); 665 return t; 666 } 667 668 public PackagedProductDefinitionPackagingComponent addShelfLifeStorage(ProductShelfLife t) { //3 669 if (t == null) 670 return this; 671 if (this.shelfLifeStorage == null) 672 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 673 this.shelfLifeStorage.add(t); 674 return this; 675 } 676 677 /** 678 * @return The first repetition of repeating field {@link #shelfLifeStorage}, creating it if it does not already exist {3} 679 */ 680 public ProductShelfLife getShelfLifeStorageFirstRep() { 681 if (getShelfLifeStorage().isEmpty()) { 682 addShelfLifeStorage(); 683 } 684 return getShelfLifeStorage().get(0); 685 } 686 687 /** 688 * @return {@link #manufacturer} (Manufacturer of this packaging item. When there are multiple values each one is a potential manufacturer of this packaging item.) 689 */ 690 public List<Reference> getManufacturer() { 691 if (this.manufacturer == null) 692 this.manufacturer = new ArrayList<Reference>(); 693 return this.manufacturer; 694 } 695 696 /** 697 * @return Returns a reference to <code>this</code> for easy method chaining 698 */ 699 public PackagedProductDefinitionPackagingComponent setManufacturer(List<Reference> theManufacturer) { 700 this.manufacturer = theManufacturer; 701 return this; 702 } 703 704 public boolean hasManufacturer() { 705 if (this.manufacturer == null) 706 return false; 707 for (Reference item : this.manufacturer) 708 if (!item.isEmpty()) 709 return true; 710 return false; 711 } 712 713 public Reference addManufacturer() { //3 714 Reference t = new Reference(); 715 if (this.manufacturer == null) 716 this.manufacturer = new ArrayList<Reference>(); 717 this.manufacturer.add(t); 718 return t; 719 } 720 721 public PackagedProductDefinitionPackagingComponent addManufacturer(Reference t) { //3 722 if (t == null) 723 return this; 724 if (this.manufacturer == null) 725 this.manufacturer = new ArrayList<Reference>(); 726 this.manufacturer.add(t); 727 return this; 728 } 729 730 /** 731 * @return The first repetition of repeating field {@link #manufacturer}, creating it if it does not already exist {3} 732 */ 733 public Reference getManufacturerFirstRep() { 734 if (getManufacturer().isEmpty()) { 735 addManufacturer(); 736 } 737 return getManufacturer().get(0); 738 } 739 740 /** 741 * @return {@link #property} (General characteristics of this item.) 742 */ 743 public List<PackagedProductDefinitionPackagingPropertyComponent> getProperty() { 744 if (this.property == null) 745 this.property = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 746 return this.property; 747 } 748 749 /** 750 * @return Returns a reference to <code>this</code> for easy method chaining 751 */ 752 public PackagedProductDefinitionPackagingComponent setProperty(List<PackagedProductDefinitionPackagingPropertyComponent> theProperty) { 753 this.property = theProperty; 754 return this; 755 } 756 757 public boolean hasProperty() { 758 if (this.property == null) 759 return false; 760 for (PackagedProductDefinitionPackagingPropertyComponent item : this.property) 761 if (!item.isEmpty()) 762 return true; 763 return false; 764 } 765 766 public PackagedProductDefinitionPackagingPropertyComponent addProperty() { //3 767 PackagedProductDefinitionPackagingPropertyComponent t = new PackagedProductDefinitionPackagingPropertyComponent(); 768 if (this.property == null) 769 this.property = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 770 this.property.add(t); 771 return t; 772 } 773 774 public PackagedProductDefinitionPackagingComponent addProperty(PackagedProductDefinitionPackagingPropertyComponent t) { //3 775 if (t == null) 776 return this; 777 if (this.property == null) 778 this.property = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 779 this.property.add(t); 780 return this; 781 } 782 783 /** 784 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 785 */ 786 public PackagedProductDefinitionPackagingPropertyComponent getPropertyFirstRep() { 787 if (getProperty().isEmpty()) { 788 addProperty(); 789 } 790 return getProperty().get(0); 791 } 792 793 /** 794 * @return {@link #containedItem} (The item(s) within the packaging.) 795 */ 796 public List<PackagedProductDefinitionPackagingContainedItemComponent> getContainedItem() { 797 if (this.containedItem == null) 798 this.containedItem = new ArrayList<PackagedProductDefinitionPackagingContainedItemComponent>(); 799 return this.containedItem; 800 } 801 802 /** 803 * @return Returns a reference to <code>this</code> for easy method chaining 804 */ 805 public PackagedProductDefinitionPackagingComponent setContainedItem(List<PackagedProductDefinitionPackagingContainedItemComponent> theContainedItem) { 806 this.containedItem = theContainedItem; 807 return this; 808 } 809 810 public boolean hasContainedItem() { 811 if (this.containedItem == null) 812 return false; 813 for (PackagedProductDefinitionPackagingContainedItemComponent item : this.containedItem) 814 if (!item.isEmpty()) 815 return true; 816 return false; 817 } 818 819 public PackagedProductDefinitionPackagingContainedItemComponent addContainedItem() { //3 820 PackagedProductDefinitionPackagingContainedItemComponent t = new PackagedProductDefinitionPackagingContainedItemComponent(); 821 if (this.containedItem == null) 822 this.containedItem = new ArrayList<PackagedProductDefinitionPackagingContainedItemComponent>(); 823 this.containedItem.add(t); 824 return t; 825 } 826 827 public PackagedProductDefinitionPackagingComponent addContainedItem(PackagedProductDefinitionPackagingContainedItemComponent t) { //3 828 if (t == null) 829 return this; 830 if (this.containedItem == null) 831 this.containedItem = new ArrayList<PackagedProductDefinitionPackagingContainedItemComponent>(); 832 this.containedItem.add(t); 833 return this; 834 } 835 836 /** 837 * @return The first repetition of repeating field {@link #containedItem}, creating it if it does not already exist {3} 838 */ 839 public PackagedProductDefinitionPackagingContainedItemComponent getContainedItemFirstRep() { 840 if (getContainedItem().isEmpty()) { 841 addContainedItem(); 842 } 843 return getContainedItem().get(0); 844 } 845 846 /** 847 * @return {@link #packaging} (Allows containers (and parts of containers) within containers, still as a part of a single packaged product. See also PackagedProductDefinition.packaging.containedItem.item(PackagedProductDefinition).) 848 */ 849 public List<PackagedProductDefinitionPackagingComponent> getPackaging() { 850 if (this.packaging == null) 851 this.packaging = new ArrayList<PackagedProductDefinitionPackagingComponent>(); 852 return this.packaging; 853 } 854 855 /** 856 * @return Returns a reference to <code>this</code> for easy method chaining 857 */ 858 public PackagedProductDefinitionPackagingComponent setPackaging(List<PackagedProductDefinitionPackagingComponent> thePackaging) { 859 this.packaging = thePackaging; 860 return this; 861 } 862 863 public boolean hasPackaging() { 864 if (this.packaging == null) 865 return false; 866 for (PackagedProductDefinitionPackagingComponent item : this.packaging) 867 if (!item.isEmpty()) 868 return true; 869 return false; 870 } 871 872 public PackagedProductDefinitionPackagingComponent addPackaging() { //3 873 PackagedProductDefinitionPackagingComponent t = new PackagedProductDefinitionPackagingComponent(); 874 if (this.packaging == null) 875 this.packaging = new ArrayList<PackagedProductDefinitionPackagingComponent>(); 876 this.packaging.add(t); 877 return t; 878 } 879 880 public PackagedProductDefinitionPackagingComponent addPackaging(PackagedProductDefinitionPackagingComponent t) { //3 881 if (t == null) 882 return this; 883 if (this.packaging == null) 884 this.packaging = new ArrayList<PackagedProductDefinitionPackagingComponent>(); 885 this.packaging.add(t); 886 return this; 887 } 888 889 /** 890 * @return The first repetition of repeating field {@link #packaging}, creating it if it does not already exist {3} 891 */ 892 public PackagedProductDefinitionPackagingComponent getPackagingFirstRep() { 893 if (getPackaging().isEmpty()) { 894 addPackaging(); 895 } 896 return getPackaging().get(0); 897 } 898 899 protected void listChildren(List<Property> children) { 900 super.listChildren(children); 901 children.add(new Property("identifier", "Identifier", "A business identifier that is specific to this particular part of the packaging, often assigned by the manufacturer. Including possibly Data Carrier Identifier (a GS1 barcode).", 0, java.lang.Integer.MAX_VALUE, identifier)); 902 children.add(new Property("type", "CodeableConcept", "The physical type of the container of the items.", 0, 1, type)); 903 children.add(new Property("componentPart", "boolean", "Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not.", 0, 1, componentPart)); 904 children.add(new Property("quantity", "integer", "The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2.", 0, 1, quantity)); 905 children.add(new Property("material", "CodeableConcept", "Material type of the package item.", 0, java.lang.Integer.MAX_VALUE, material)); 906 children.add(new Property("alternateMaterial", "CodeableConcept", "A possible alternate material for this part of the packaging, that is allowed to be used instead of the usual material (e.g. different types of plastic for a blister sleeve).", 0, java.lang.Integer.MAX_VALUE, alternateMaterial)); 907 children.add(new Property("shelfLifeStorage", "ProductShelfLife", "Shelf Life and storage information.", 0, java.lang.Integer.MAX_VALUE, shelfLifeStorage)); 908 children.add(new Property("manufacturer", "Reference(Organization)", "Manufacturer of this packaging item. When there are multiple values each one is a potential manufacturer of this packaging item.", 0, java.lang.Integer.MAX_VALUE, manufacturer)); 909 children.add(new Property("property", "", "General characteristics of this item.", 0, java.lang.Integer.MAX_VALUE, property)); 910 children.add(new Property("containedItem", "", "The item(s) within the packaging.", 0, java.lang.Integer.MAX_VALUE, containedItem)); 911 children.add(new Property("packaging", "@PackagedProductDefinition.packaging", "Allows containers (and parts of containers) within containers, still as a part of a single packaged product. See also PackagedProductDefinition.packaging.containedItem.item(PackagedProductDefinition).", 0, java.lang.Integer.MAX_VALUE, packaging)); 912 } 913 914 @Override 915 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 916 switch (_hash) { 917 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A business identifier that is specific to this particular part of the packaging, often assigned by the manufacturer. Including possibly Data Carrier Identifier (a GS1 barcode).", 0, java.lang.Integer.MAX_VALUE, identifier); 918 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The physical type of the container of the items.", 0, 1, type); 919 case 1706307216: /*componentPart*/ return new Property("componentPart", "boolean", "Is this a part of the packaging (e.g. a cap or bottle stopper), rather than the packaging itself (e.g. a bottle or vial). The latter type are designed be a container, but the former are not.", 0, 1, componentPart); 920 case -1285004149: /*quantity*/ return new Property("quantity", "integer", "The quantity of packaging items contained at this layer of the package. This does not relate to the number of contained items but relates solely to the number of packaging items. When looking at the outermost layer it is always 1. If there are two boxes within, at the next layer it would be 2.", 0, 1, quantity); 921 case 299066663: /*material*/ return new Property("material", "CodeableConcept", "Material type of the package item.", 0, java.lang.Integer.MAX_VALUE, material); 922 case -1021448255: /*alternateMaterial*/ return new Property("alternateMaterial", "CodeableConcept", "A possible alternate material for this part of the packaging, that is allowed to be used instead of the usual material (e.g. different types of plastic for a blister sleeve).", 0, java.lang.Integer.MAX_VALUE, alternateMaterial); 923 case 172049237: /*shelfLifeStorage*/ return new Property("shelfLifeStorage", "ProductShelfLife", "Shelf Life and storage information.", 0, java.lang.Integer.MAX_VALUE, shelfLifeStorage); 924 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "Manufacturer of this packaging item. When there are multiple values each one is a potential manufacturer of this packaging item.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 925 case -993141291: /*property*/ return new Property("property", "", "General characteristics of this item.", 0, java.lang.Integer.MAX_VALUE, property); 926 case 1953679910: /*containedItem*/ return new Property("containedItem", "", "The item(s) within the packaging.", 0, java.lang.Integer.MAX_VALUE, containedItem); 927 case 1802065795: /*packaging*/ return new Property("packaging", "@PackagedProductDefinition.packaging", "Allows containers (and parts of containers) within containers, still as a part of a single packaged product. See also PackagedProductDefinition.packaging.containedItem.item(PackagedProductDefinition).", 0, java.lang.Integer.MAX_VALUE, packaging); 928 default: return super.getNamedProperty(_hash, _name, _checkValid); 929 } 930 931 } 932 933 @Override 934 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 935 switch (hash) { 936 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 937 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 938 case 1706307216: /*componentPart*/ return this.componentPart == null ? new Base[0] : new Base[] {this.componentPart}; // BooleanType 939 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // IntegerType 940 case 299066663: /*material*/ return this.material == null ? new Base[0] : this.material.toArray(new Base[this.material.size()]); // CodeableConcept 941 case -1021448255: /*alternateMaterial*/ return this.alternateMaterial == null ? new Base[0] : this.alternateMaterial.toArray(new Base[this.alternateMaterial.size()]); // CodeableConcept 942 case 172049237: /*shelfLifeStorage*/ return this.shelfLifeStorage == null ? new Base[0] : this.shelfLifeStorage.toArray(new Base[this.shelfLifeStorage.size()]); // ProductShelfLife 943 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 944 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // PackagedProductDefinitionPackagingPropertyComponent 945 case 1953679910: /*containedItem*/ return this.containedItem == null ? new Base[0] : this.containedItem.toArray(new Base[this.containedItem.size()]); // PackagedProductDefinitionPackagingContainedItemComponent 946 case 1802065795: /*packaging*/ return this.packaging == null ? new Base[0] : this.packaging.toArray(new Base[this.packaging.size()]); // PackagedProductDefinitionPackagingComponent 947 default: return super.getProperty(hash, name, checkValid); 948 } 949 950 } 951 952 @Override 953 public Base setProperty(int hash, String name, Base value) throws FHIRException { 954 switch (hash) { 955 case -1618432855: // identifier 956 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 957 return value; 958 case 3575610: // type 959 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 960 return value; 961 case 1706307216: // componentPart 962 this.componentPart = TypeConvertor.castToBoolean(value); // BooleanType 963 return value; 964 case -1285004149: // quantity 965 this.quantity = TypeConvertor.castToInteger(value); // IntegerType 966 return value; 967 case 299066663: // material 968 this.getMaterial().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 969 return value; 970 case -1021448255: // alternateMaterial 971 this.getAlternateMaterial().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 972 return value; 973 case 172049237: // shelfLifeStorage 974 this.getShelfLifeStorage().add(TypeConvertor.castToProductShelfLife(value)); // ProductShelfLife 975 return value; 976 case -1969347631: // manufacturer 977 this.getManufacturer().add(TypeConvertor.castToReference(value)); // Reference 978 return value; 979 case -993141291: // property 980 this.getProperty().add((PackagedProductDefinitionPackagingPropertyComponent) value); // PackagedProductDefinitionPackagingPropertyComponent 981 return value; 982 case 1953679910: // containedItem 983 this.getContainedItem().add((PackagedProductDefinitionPackagingContainedItemComponent) value); // PackagedProductDefinitionPackagingContainedItemComponent 984 return value; 985 case 1802065795: // packaging 986 this.getPackaging().add((PackagedProductDefinitionPackagingComponent) value); // PackagedProductDefinitionPackagingComponent 987 return value; 988 default: return super.setProperty(hash, name, value); 989 } 990 991 } 992 993 @Override 994 public Base setProperty(String name, Base value) throws FHIRException { 995 if (name.equals("identifier")) { 996 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 997 } else if (name.equals("type")) { 998 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 999 } else if (name.equals("componentPart")) { 1000 this.componentPart = TypeConvertor.castToBoolean(value); // BooleanType 1001 } else if (name.equals("quantity")) { 1002 this.quantity = TypeConvertor.castToInteger(value); // IntegerType 1003 } else if (name.equals("material")) { 1004 this.getMaterial().add(TypeConvertor.castToCodeableConcept(value)); 1005 } else if (name.equals("alternateMaterial")) { 1006 this.getAlternateMaterial().add(TypeConvertor.castToCodeableConcept(value)); 1007 } else if (name.equals("shelfLifeStorage")) { 1008 this.getShelfLifeStorage().add(TypeConvertor.castToProductShelfLife(value)); 1009 } else if (name.equals("manufacturer")) { 1010 this.getManufacturer().add(TypeConvertor.castToReference(value)); 1011 } else if (name.equals("property")) { 1012 this.getProperty().add((PackagedProductDefinitionPackagingPropertyComponent) value); 1013 } else if (name.equals("containedItem")) { 1014 this.getContainedItem().add((PackagedProductDefinitionPackagingContainedItemComponent) value); 1015 } else if (name.equals("packaging")) { 1016 this.getPackaging().add((PackagedProductDefinitionPackagingComponent) value); 1017 } else 1018 return super.setProperty(name, value); 1019 return value; 1020 } 1021 1022 @Override 1023 public void removeChild(String name, Base value) throws FHIRException { 1024 if (name.equals("identifier")) { 1025 this.getIdentifier().remove(value); 1026 } else if (name.equals("type")) { 1027 this.type = null; 1028 } else if (name.equals("componentPart")) { 1029 this.componentPart = null; 1030 } else if (name.equals("quantity")) { 1031 this.quantity = null; 1032 } else if (name.equals("material")) { 1033 this.getMaterial().remove(value); 1034 } else if (name.equals("alternateMaterial")) { 1035 this.getAlternateMaterial().remove(value); 1036 } else if (name.equals("shelfLifeStorage")) { 1037 this.getShelfLifeStorage().remove(value); 1038 } else if (name.equals("manufacturer")) { 1039 this.getManufacturer().remove(value); 1040 } else if (name.equals("property")) { 1041 this.getProperty().remove((PackagedProductDefinitionPackagingPropertyComponent) value); 1042 } else if (name.equals("containedItem")) { 1043 this.getContainedItem().remove((PackagedProductDefinitionPackagingContainedItemComponent) value); 1044 } else if (name.equals("packaging")) { 1045 this.getPackaging().remove((PackagedProductDefinitionPackagingComponent) value); 1046 } else 1047 super.removeChild(name, value); 1048 1049 } 1050 1051 @Override 1052 public Base makeProperty(int hash, String name) throws FHIRException { 1053 switch (hash) { 1054 case -1618432855: return addIdentifier(); 1055 case 3575610: return getType(); 1056 case 1706307216: return getComponentPartElement(); 1057 case -1285004149: return getQuantityElement(); 1058 case 299066663: return addMaterial(); 1059 case -1021448255: return addAlternateMaterial(); 1060 case 172049237: return addShelfLifeStorage(); 1061 case -1969347631: return addManufacturer(); 1062 case -993141291: return addProperty(); 1063 case 1953679910: return addContainedItem(); 1064 case 1802065795: return addPackaging(); 1065 default: return super.makeProperty(hash, name); 1066 } 1067 1068 } 1069 1070 @Override 1071 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1072 switch (hash) { 1073 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1074 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1075 case 1706307216: /*componentPart*/ return new String[] {"boolean"}; 1076 case -1285004149: /*quantity*/ return new String[] {"integer"}; 1077 case 299066663: /*material*/ return new String[] {"CodeableConcept"}; 1078 case -1021448255: /*alternateMaterial*/ return new String[] {"CodeableConcept"}; 1079 case 172049237: /*shelfLifeStorage*/ return new String[] {"ProductShelfLife"}; 1080 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 1081 case -993141291: /*property*/ return new String[] {}; 1082 case 1953679910: /*containedItem*/ return new String[] {}; 1083 case 1802065795: /*packaging*/ return new String[] {"@PackagedProductDefinition.packaging"}; 1084 default: return super.getTypesForProperty(hash, name); 1085 } 1086 1087 } 1088 1089 @Override 1090 public Base addChild(String name) throws FHIRException { 1091 if (name.equals("identifier")) { 1092 return addIdentifier(); 1093 } 1094 else if (name.equals("type")) { 1095 this.type = new CodeableConcept(); 1096 return this.type; 1097 } 1098 else if (name.equals("componentPart")) { 1099 throw new FHIRException("Cannot call addChild on a singleton property PackagedProductDefinition.packaging.componentPart"); 1100 } 1101 else if (name.equals("quantity")) { 1102 throw new FHIRException("Cannot call addChild on a singleton property PackagedProductDefinition.packaging.quantity"); 1103 } 1104 else if (name.equals("material")) { 1105 return addMaterial(); 1106 } 1107 else if (name.equals("alternateMaterial")) { 1108 return addAlternateMaterial(); 1109 } 1110 else if (name.equals("shelfLifeStorage")) { 1111 return addShelfLifeStorage(); 1112 } 1113 else if (name.equals("manufacturer")) { 1114 return addManufacturer(); 1115 } 1116 else if (name.equals("property")) { 1117 return addProperty(); 1118 } 1119 else if (name.equals("containedItem")) { 1120 return addContainedItem(); 1121 } 1122 else if (name.equals("packaging")) { 1123 return addPackaging(); 1124 } 1125 else 1126 return super.addChild(name); 1127 } 1128 1129 public PackagedProductDefinitionPackagingComponent copy() { 1130 PackagedProductDefinitionPackagingComponent dst = new PackagedProductDefinitionPackagingComponent(); 1131 copyValues(dst); 1132 return dst; 1133 } 1134 1135 public void copyValues(PackagedProductDefinitionPackagingComponent dst) { 1136 super.copyValues(dst); 1137 if (identifier != null) { 1138 dst.identifier = new ArrayList<Identifier>(); 1139 for (Identifier i : identifier) 1140 dst.identifier.add(i.copy()); 1141 }; 1142 dst.type = type == null ? null : type.copy(); 1143 dst.componentPart = componentPart == null ? null : componentPart.copy(); 1144 dst.quantity = quantity == null ? null : quantity.copy(); 1145 if (material != null) { 1146 dst.material = new ArrayList<CodeableConcept>(); 1147 for (CodeableConcept i : material) 1148 dst.material.add(i.copy()); 1149 }; 1150 if (alternateMaterial != null) { 1151 dst.alternateMaterial = new ArrayList<CodeableConcept>(); 1152 for (CodeableConcept i : alternateMaterial) 1153 dst.alternateMaterial.add(i.copy()); 1154 }; 1155 if (shelfLifeStorage != null) { 1156 dst.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 1157 for (ProductShelfLife i : shelfLifeStorage) 1158 dst.shelfLifeStorage.add(i.copy()); 1159 }; 1160 if (manufacturer != null) { 1161 dst.manufacturer = new ArrayList<Reference>(); 1162 for (Reference i : manufacturer) 1163 dst.manufacturer.add(i.copy()); 1164 }; 1165 if (property != null) { 1166 dst.property = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 1167 for (PackagedProductDefinitionPackagingPropertyComponent i : property) 1168 dst.property.add(i.copy()); 1169 }; 1170 if (containedItem != null) { 1171 dst.containedItem = new ArrayList<PackagedProductDefinitionPackagingContainedItemComponent>(); 1172 for (PackagedProductDefinitionPackagingContainedItemComponent i : containedItem) 1173 dst.containedItem.add(i.copy()); 1174 }; 1175 if (packaging != null) { 1176 dst.packaging = new ArrayList<PackagedProductDefinitionPackagingComponent>(); 1177 for (PackagedProductDefinitionPackagingComponent i : packaging) 1178 dst.packaging.add(i.copy()); 1179 }; 1180 } 1181 1182 @Override 1183 public boolean equalsDeep(Base other_) { 1184 if (!super.equalsDeep(other_)) 1185 return false; 1186 if (!(other_ instanceof PackagedProductDefinitionPackagingComponent)) 1187 return false; 1188 PackagedProductDefinitionPackagingComponent o = (PackagedProductDefinitionPackagingComponent) other_; 1189 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(componentPart, o.componentPart, true) 1190 && compareDeep(quantity, o.quantity, true) && compareDeep(material, o.material, true) && compareDeep(alternateMaterial, o.alternateMaterial, true) 1191 && compareDeep(shelfLifeStorage, o.shelfLifeStorage, true) && compareDeep(manufacturer, o.manufacturer, true) 1192 && compareDeep(property, o.property, true) && compareDeep(containedItem, o.containedItem, true) 1193 && compareDeep(packaging, o.packaging, true); 1194 } 1195 1196 @Override 1197 public boolean equalsShallow(Base other_) { 1198 if (!super.equalsShallow(other_)) 1199 return false; 1200 if (!(other_ instanceof PackagedProductDefinitionPackagingComponent)) 1201 return false; 1202 PackagedProductDefinitionPackagingComponent o = (PackagedProductDefinitionPackagingComponent) other_; 1203 return compareValues(componentPart, o.componentPart, true) && compareValues(quantity, o.quantity, true) 1204 ; 1205 } 1206 1207 public boolean isEmpty() { 1208 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, componentPart 1209 , quantity, material, alternateMaterial, shelfLifeStorage, manufacturer, property 1210 , containedItem, packaging); 1211 } 1212 1213 public String fhirType() { 1214 return "PackagedProductDefinition.packaging"; 1215 1216 } 1217 1218 } 1219 1220 @Block() 1221 public static class PackagedProductDefinitionPackagingPropertyComponent extends BackboneElement implements IBaseBackboneElement { 1222 /** 1223 * A code expressing the type of characteristic. 1224 */ 1225 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1226 @Description(shortDefinition="A code expressing the type of characteristic", formalDefinition="A code expressing the type of characteristic." ) 1227 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-characteristic-codes") 1228 protected CodeableConcept type; 1229 1230 /** 1231 * A value for the characteristic. 1232 */ 1233 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, DateType.class, BooleanType.class, Attachment.class}, order=2, min=0, max=1, modifier=false, summary=true) 1234 @Description(shortDefinition="A value for the characteristic", formalDefinition="A value for the characteristic." ) 1235 protected DataType value; 1236 1237 private static final long serialVersionUID = -1659186716L; 1238 1239 /** 1240 * Constructor 1241 */ 1242 public PackagedProductDefinitionPackagingPropertyComponent() { 1243 super(); 1244 } 1245 1246 /** 1247 * Constructor 1248 */ 1249 public PackagedProductDefinitionPackagingPropertyComponent(CodeableConcept type) { 1250 super(); 1251 this.setType(type); 1252 } 1253 1254 /** 1255 * @return {@link #type} (A code expressing the type of characteristic.) 1256 */ 1257 public CodeableConcept getType() { 1258 if (this.type == null) 1259 if (Configuration.errorOnAutoCreate()) 1260 throw new Error("Attempt to auto-create PackagedProductDefinitionPackagingPropertyComponent.type"); 1261 else if (Configuration.doAutoCreate()) 1262 this.type = new CodeableConcept(); // cc 1263 return this.type; 1264 } 1265 1266 public boolean hasType() { 1267 return this.type != null && !this.type.isEmpty(); 1268 } 1269 1270 /** 1271 * @param value {@link #type} (A code expressing the type of characteristic.) 1272 */ 1273 public PackagedProductDefinitionPackagingPropertyComponent setType(CodeableConcept value) { 1274 this.type = value; 1275 return this; 1276 } 1277 1278 /** 1279 * @return {@link #value} (A value for the characteristic.) 1280 */ 1281 public DataType getValue() { 1282 return this.value; 1283 } 1284 1285 /** 1286 * @return {@link #value} (A value for the characteristic.) 1287 */ 1288 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1289 if (this.value == null) 1290 this.value = new CodeableConcept(); 1291 if (!(this.value instanceof CodeableConcept)) 1292 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1293 return (CodeableConcept) this.value; 1294 } 1295 1296 public boolean hasValueCodeableConcept() { 1297 return this != null && this.value instanceof CodeableConcept; 1298 } 1299 1300 /** 1301 * @return {@link #value} (A value for the characteristic.) 1302 */ 1303 public Quantity getValueQuantity() throws FHIRException { 1304 if (this.value == null) 1305 this.value = new Quantity(); 1306 if (!(this.value instanceof Quantity)) 1307 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1308 return (Quantity) this.value; 1309 } 1310 1311 public boolean hasValueQuantity() { 1312 return this != null && this.value instanceof Quantity; 1313 } 1314 1315 /** 1316 * @return {@link #value} (A value for the characteristic.) 1317 */ 1318 public DateType getValueDateType() throws FHIRException { 1319 if (this.value == null) 1320 this.value = new DateType(); 1321 if (!(this.value instanceof DateType)) 1322 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 1323 return (DateType) this.value; 1324 } 1325 1326 public boolean hasValueDateType() { 1327 return this != null && this.value instanceof DateType; 1328 } 1329 1330 /** 1331 * @return {@link #value} (A value for the characteristic.) 1332 */ 1333 public BooleanType getValueBooleanType() throws FHIRException { 1334 if (this.value == null) 1335 this.value = new BooleanType(); 1336 if (!(this.value instanceof BooleanType)) 1337 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1338 return (BooleanType) this.value; 1339 } 1340 1341 public boolean hasValueBooleanType() { 1342 return this != null && this.value instanceof BooleanType; 1343 } 1344 1345 /** 1346 * @return {@link #value} (A value for the characteristic.) 1347 */ 1348 public Attachment getValueAttachment() throws FHIRException { 1349 if (this.value == null) 1350 this.value = new Attachment(); 1351 if (!(this.value instanceof Attachment)) 1352 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1353 return (Attachment) this.value; 1354 } 1355 1356 public boolean hasValueAttachment() { 1357 return this != null && this.value instanceof Attachment; 1358 } 1359 1360 public boolean hasValue() { 1361 return this.value != null && !this.value.isEmpty(); 1362 } 1363 1364 /** 1365 * @param value {@link #value} (A value for the characteristic.) 1366 */ 1367 public PackagedProductDefinitionPackagingPropertyComponent setValue(DataType value) { 1368 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof DateType || value instanceof BooleanType || value instanceof Attachment)) 1369 throw new FHIRException("Not the right type for PackagedProductDefinition.packaging.property.value[x]: "+value.fhirType()); 1370 this.value = value; 1371 return this; 1372 } 1373 1374 protected void listChildren(List<Property> children) { 1375 super.listChildren(children); 1376 children.add(new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type)); 1377 children.add(new Property("value[x]", "CodeableConcept|Quantity|date|boolean|Attachment", "A value for the characteristic.", 0, 1, value)); 1378 } 1379 1380 @Override 1381 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1382 switch (_hash) { 1383 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type); 1384 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|Attachment", "A value for the characteristic.", 0, 1, value); 1385 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|Attachment", "A value for the characteristic.", 0, 1, value); 1386 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "A value for the characteristic.", 0, 1, value); 1387 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "A value for the characteristic.", 0, 1, value); 1388 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "A value for the characteristic.", 0, 1, value); 1389 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "A value for the characteristic.", 0, 1, value); 1390 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "A value for the characteristic.", 0, 1, value); 1391 default: return super.getNamedProperty(_hash, _name, _checkValid); 1392 } 1393 1394 } 1395 1396 @Override 1397 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1398 switch (hash) { 1399 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1400 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1401 default: return super.getProperty(hash, name, checkValid); 1402 } 1403 1404 } 1405 1406 @Override 1407 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1408 switch (hash) { 1409 case 3575610: // type 1410 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1411 return value; 1412 case 111972721: // value 1413 this.value = TypeConvertor.castToType(value); // DataType 1414 return value; 1415 default: return super.setProperty(hash, name, value); 1416 } 1417 1418 } 1419 1420 @Override 1421 public Base setProperty(String name, Base value) throws FHIRException { 1422 if (name.equals("type")) { 1423 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1424 } else if (name.equals("value[x]")) { 1425 this.value = TypeConvertor.castToType(value); // DataType 1426 } else 1427 return super.setProperty(name, value); 1428 return value; 1429 } 1430 1431 @Override 1432 public void removeChild(String name, Base value) throws FHIRException { 1433 if (name.equals("type")) { 1434 this.type = null; 1435 } else if (name.equals("value[x]")) { 1436 this.value = null; 1437 } else 1438 super.removeChild(name, value); 1439 1440 } 1441 1442 @Override 1443 public Base makeProperty(int hash, String name) throws FHIRException { 1444 switch (hash) { 1445 case 3575610: return getType(); 1446 case -1410166417: return getValue(); 1447 case 111972721: return getValue(); 1448 default: return super.makeProperty(hash, name); 1449 } 1450 1451 } 1452 1453 @Override 1454 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1455 switch (hash) { 1456 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1457 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "date", "boolean", "Attachment"}; 1458 default: return super.getTypesForProperty(hash, name); 1459 } 1460 1461 } 1462 1463 @Override 1464 public Base addChild(String name) throws FHIRException { 1465 if (name.equals("type")) { 1466 this.type = new CodeableConcept(); 1467 return this.type; 1468 } 1469 else if (name.equals("valueCodeableConcept")) { 1470 this.value = new CodeableConcept(); 1471 return this.value; 1472 } 1473 else if (name.equals("valueQuantity")) { 1474 this.value = new Quantity(); 1475 return this.value; 1476 } 1477 else if (name.equals("valueDate")) { 1478 this.value = new DateType(); 1479 return this.value; 1480 } 1481 else if (name.equals("valueBoolean")) { 1482 this.value = new BooleanType(); 1483 return this.value; 1484 } 1485 else if (name.equals("valueAttachment")) { 1486 this.value = new Attachment(); 1487 return this.value; 1488 } 1489 else 1490 return super.addChild(name); 1491 } 1492 1493 public PackagedProductDefinitionPackagingPropertyComponent copy() { 1494 PackagedProductDefinitionPackagingPropertyComponent dst = new PackagedProductDefinitionPackagingPropertyComponent(); 1495 copyValues(dst); 1496 return dst; 1497 } 1498 1499 public void copyValues(PackagedProductDefinitionPackagingPropertyComponent dst) { 1500 super.copyValues(dst); 1501 dst.type = type == null ? null : type.copy(); 1502 dst.value = value == null ? null : value.copy(); 1503 } 1504 1505 @Override 1506 public boolean equalsDeep(Base other_) { 1507 if (!super.equalsDeep(other_)) 1508 return false; 1509 if (!(other_ instanceof PackagedProductDefinitionPackagingPropertyComponent)) 1510 return false; 1511 PackagedProductDefinitionPackagingPropertyComponent o = (PackagedProductDefinitionPackagingPropertyComponent) other_; 1512 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 1513 } 1514 1515 @Override 1516 public boolean equalsShallow(Base other_) { 1517 if (!super.equalsShallow(other_)) 1518 return false; 1519 if (!(other_ instanceof PackagedProductDefinitionPackagingPropertyComponent)) 1520 return false; 1521 PackagedProductDefinitionPackagingPropertyComponent o = (PackagedProductDefinitionPackagingPropertyComponent) other_; 1522 return true; 1523 } 1524 1525 public boolean isEmpty() { 1526 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 1527 } 1528 1529 public String fhirType() { 1530 return "PackagedProductDefinition.packaging.property"; 1531 1532 } 1533 1534 } 1535 1536 @Block() 1537 public static class PackagedProductDefinitionPackagingContainedItemComponent extends BackboneElement implements IBaseBackboneElement { 1538 /** 1539 * The actual item(s) of medication, as manufactured, or a device (typically, but not necessarily, a co-packaged one), or other medically related item (such as food, biologicals, raw materials, medical fluids, gases etc.), as contained in the package. This also allows another whole packaged product to be included, which is solely for the case where a package of other entire packages is wanted - such as a wholesale or distribution pack (for layers within one package, use PackagedProductDefinition.packaging.packaging). 1540 */ 1541 @Child(name = "item", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=true) 1542 @Description(shortDefinition="The actual item(s) of medication, as manufactured, or a device, or other medically related item (food, biologicals, raw materials, medical fluids, gases etc.), as contained in the package", formalDefinition="The actual item(s) of medication, as manufactured, or a device (typically, but not necessarily, a co-packaged one), or other medically related item (such as food, biologicals, raw materials, medical fluids, gases etc.), as contained in the package. This also allows another whole packaged product to be included, which is solely for the case where a package of other entire packages is wanted - such as a wholesale or distribution pack (for layers within one package, use PackagedProductDefinition.packaging.packaging)." ) 1543 protected CodeableReference item; 1544 1545 /** 1546 * The number of this type of item within this packaging or for continuous items such as liquids it is the quantity (for example 25ml). See also PackagedProductDefinition.containedItemQuantity (especially the long definition). 1547 */ 1548 @Child(name = "amount", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=true) 1549 @Description(shortDefinition="The number of this type of item within this packaging or for continuous items such as liquids it is the quantity (for example 25ml). See also PackagedProductDefinition.containedItemQuantity (especially the long definition)", formalDefinition="The number of this type of item within this packaging or for continuous items such as liquids it is the quantity (for example 25ml). See also PackagedProductDefinition.containedItemQuantity (especially the long definition)." ) 1550 protected Quantity amount; 1551 1552 private static final long serialVersionUID = 443863028L; 1553 1554 /** 1555 * Constructor 1556 */ 1557 public PackagedProductDefinitionPackagingContainedItemComponent() { 1558 super(); 1559 } 1560 1561 /** 1562 * Constructor 1563 */ 1564 public PackagedProductDefinitionPackagingContainedItemComponent(CodeableReference item) { 1565 super(); 1566 this.setItem(item); 1567 } 1568 1569 /** 1570 * @return {@link #item} (The actual item(s) of medication, as manufactured, or a device (typically, but not necessarily, a co-packaged one), or other medically related item (such as food, biologicals, raw materials, medical fluids, gases etc.), as contained in the package. This also allows another whole packaged product to be included, which is solely for the case where a package of other entire packages is wanted - such as a wholesale or distribution pack (for layers within one package, use PackagedProductDefinition.packaging.packaging).) 1571 */ 1572 public CodeableReference getItem() { 1573 if (this.item == null) 1574 if (Configuration.errorOnAutoCreate()) 1575 throw new Error("Attempt to auto-create PackagedProductDefinitionPackagingContainedItemComponent.item"); 1576 else if (Configuration.doAutoCreate()) 1577 this.item = new CodeableReference(); // cc 1578 return this.item; 1579 } 1580 1581 public boolean hasItem() { 1582 return this.item != null && !this.item.isEmpty(); 1583 } 1584 1585 /** 1586 * @param value {@link #item} (The actual item(s) of medication, as manufactured, or a device (typically, but not necessarily, a co-packaged one), or other medically related item (such as food, biologicals, raw materials, medical fluids, gases etc.), as contained in the package. This also allows another whole packaged product to be included, which is solely for the case where a package of other entire packages is wanted - such as a wholesale or distribution pack (for layers within one package, use PackagedProductDefinition.packaging.packaging).) 1587 */ 1588 public PackagedProductDefinitionPackagingContainedItemComponent setItem(CodeableReference value) { 1589 this.item = value; 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #amount} (The number of this type of item within this packaging or for continuous items such as liquids it is the quantity (for example 25ml). See also PackagedProductDefinition.containedItemQuantity (especially the long definition).) 1595 */ 1596 public Quantity getAmount() { 1597 if (this.amount == null) 1598 if (Configuration.errorOnAutoCreate()) 1599 throw new Error("Attempt to auto-create PackagedProductDefinitionPackagingContainedItemComponent.amount"); 1600 else if (Configuration.doAutoCreate()) 1601 this.amount = new Quantity(); // cc 1602 return this.amount; 1603 } 1604 1605 public boolean hasAmount() { 1606 return this.amount != null && !this.amount.isEmpty(); 1607 } 1608 1609 /** 1610 * @param value {@link #amount} (The number of this type of item within this packaging or for continuous items such as liquids it is the quantity (for example 25ml). See also PackagedProductDefinition.containedItemQuantity (especially the long definition).) 1611 */ 1612 public PackagedProductDefinitionPackagingContainedItemComponent setAmount(Quantity value) { 1613 this.amount = value; 1614 return this; 1615 } 1616 1617 protected void listChildren(List<Property> children) { 1618 super.listChildren(children); 1619 children.add(new Property("item", "CodeableReference(ManufacturedItemDefinition|DeviceDefinition|PackagedProductDefinition|BiologicallyDerivedProduct|NutritionProduct)", "The actual item(s) of medication, as manufactured, or a device (typically, but not necessarily, a co-packaged one), or other medically related item (such as food, biologicals, raw materials, medical fluids, gases etc.), as contained in the package. This also allows another whole packaged product to be included, which is solely for the case where a package of other entire packages is wanted - such as a wholesale or distribution pack (for layers within one package, use PackagedProductDefinition.packaging.packaging).", 0, 1, item)); 1620 children.add(new Property("amount", "Quantity", "The number of this type of item within this packaging or for continuous items such as liquids it is the quantity (for example 25ml). See also PackagedProductDefinition.containedItemQuantity (especially the long definition).", 0, 1, amount)); 1621 } 1622 1623 @Override 1624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1625 switch (_hash) { 1626 case 3242771: /*item*/ return new Property("item", "CodeableReference(ManufacturedItemDefinition|DeviceDefinition|PackagedProductDefinition|BiologicallyDerivedProduct|NutritionProduct)", "The actual item(s) of medication, as manufactured, or a device (typically, but not necessarily, a co-packaged one), or other medically related item (such as food, biologicals, raw materials, medical fluids, gases etc.), as contained in the package. This also allows another whole packaged product to be included, which is solely for the case where a package of other entire packages is wanted - such as a wholesale or distribution pack (for layers within one package, use PackagedProductDefinition.packaging.packaging).", 0, 1, item); 1627 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "The number of this type of item within this packaging or for continuous items such as liquids it is the quantity (for example 25ml). See also PackagedProductDefinition.containedItemQuantity (especially the long definition).", 0, 1, amount); 1628 default: return super.getNamedProperty(_hash, _name, _checkValid); 1629 } 1630 1631 } 1632 1633 @Override 1634 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1635 switch (hash) { 1636 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 1637 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Quantity 1638 default: return super.getProperty(hash, name, checkValid); 1639 } 1640 1641 } 1642 1643 @Override 1644 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1645 switch (hash) { 1646 case 3242771: // item 1647 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 1648 return value; 1649 case -1413853096: // amount 1650 this.amount = TypeConvertor.castToQuantity(value); // Quantity 1651 return value; 1652 default: return super.setProperty(hash, name, value); 1653 } 1654 1655 } 1656 1657 @Override 1658 public Base setProperty(String name, Base value) throws FHIRException { 1659 if (name.equals("item")) { 1660 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 1661 } else if (name.equals("amount")) { 1662 this.amount = TypeConvertor.castToQuantity(value); // Quantity 1663 } else 1664 return super.setProperty(name, value); 1665 return value; 1666 } 1667 1668 @Override 1669 public void removeChild(String name, Base value) throws FHIRException { 1670 if (name.equals("item")) { 1671 this.item = null; 1672 } else if (name.equals("amount")) { 1673 this.amount = null; 1674 } else 1675 super.removeChild(name, value); 1676 1677 } 1678 1679 @Override 1680 public Base makeProperty(int hash, String name) throws FHIRException { 1681 switch (hash) { 1682 case 3242771: return getItem(); 1683 case -1413853096: return getAmount(); 1684 default: return super.makeProperty(hash, name); 1685 } 1686 1687 } 1688 1689 @Override 1690 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1691 switch (hash) { 1692 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 1693 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 1694 default: return super.getTypesForProperty(hash, name); 1695 } 1696 1697 } 1698 1699 @Override 1700 public Base addChild(String name) throws FHIRException { 1701 if (name.equals("item")) { 1702 this.item = new CodeableReference(); 1703 return this.item; 1704 } 1705 else if (name.equals("amount")) { 1706 this.amount = new Quantity(); 1707 return this.amount; 1708 } 1709 else 1710 return super.addChild(name); 1711 } 1712 1713 public PackagedProductDefinitionPackagingContainedItemComponent copy() { 1714 PackagedProductDefinitionPackagingContainedItemComponent dst = new PackagedProductDefinitionPackagingContainedItemComponent(); 1715 copyValues(dst); 1716 return dst; 1717 } 1718 1719 public void copyValues(PackagedProductDefinitionPackagingContainedItemComponent dst) { 1720 super.copyValues(dst); 1721 dst.item = item == null ? null : item.copy(); 1722 dst.amount = amount == null ? null : amount.copy(); 1723 } 1724 1725 @Override 1726 public boolean equalsDeep(Base other_) { 1727 if (!super.equalsDeep(other_)) 1728 return false; 1729 if (!(other_ instanceof PackagedProductDefinitionPackagingContainedItemComponent)) 1730 return false; 1731 PackagedProductDefinitionPackagingContainedItemComponent o = (PackagedProductDefinitionPackagingContainedItemComponent) other_; 1732 return compareDeep(item, o.item, true) && compareDeep(amount, o.amount, true); 1733 } 1734 1735 @Override 1736 public boolean equalsShallow(Base other_) { 1737 if (!super.equalsShallow(other_)) 1738 return false; 1739 if (!(other_ instanceof PackagedProductDefinitionPackagingContainedItemComponent)) 1740 return false; 1741 PackagedProductDefinitionPackagingContainedItemComponent o = (PackagedProductDefinitionPackagingContainedItemComponent) other_; 1742 return true; 1743 } 1744 1745 public boolean isEmpty() { 1746 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, amount); 1747 } 1748 1749 public String fhirType() { 1750 return "PackagedProductDefinition.packaging.containedItem"; 1751 1752 } 1753 1754 } 1755 1756 /** 1757 * A unique identifier for this package as whole - not the the content of the package. Unique instance identifiers assigned to a package by manufacturers, regulators, drug catalogue custodians or other organizations. 1758 */ 1759 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1760 @Description(shortDefinition="A unique identifier for this package as whole - not for the content of the package", formalDefinition="A unique identifier for this package as whole - not the the content of the package. Unique instance identifiers assigned to a package by manufacturers, regulators, drug catalogue custodians or other organizations." ) 1761 protected List<Identifier> identifier; 1762 1763 /** 1764 * A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc. 1765 */ 1766 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1767 @Description(shortDefinition="A name for this package. Typically as listed in a drug formulary, catalogue, inventory etc", formalDefinition="A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc." ) 1768 protected StringType name; 1769 1770 /** 1771 * A high level category e.g. medicinal product, raw material, shipping/transport container, etc. 1772 */ 1773 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1774 @Description(shortDefinition="A high level category e.g. medicinal product, raw material, shipping container etc", formalDefinition="A high level category e.g. medicinal product, raw material, shipping/transport container, etc." ) 1775 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/package-type") 1776 protected CodeableConcept type; 1777 1778 /** 1779 * The product this package model relates to, not the contents of the package (for which see package.containedItem). 1780 */ 1781 @Child(name = "packageFor", type = {MedicinalProductDefinition.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1782 @Description(shortDefinition="The product that this is a pack for", formalDefinition="The product this package model relates to, not the contents of the package (for which see package.containedItem)." ) 1783 protected List<Reference> packageFor; 1784 1785 /** 1786 * The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status. 1787 */ 1788 @Child(name = "status", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=true, summary=true) 1789 @Description(shortDefinition="The status within the lifecycle of this item. High level - not intended to duplicate details elsewhere e.g. legal status, or authorization/marketing status", formalDefinition="The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status." ) 1790 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1791 protected CodeableConcept status; 1792 1793 /** 1794 * The date at which the given status became applicable. 1795 */ 1796 @Child(name = "statusDate", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1797 @Description(shortDefinition="The date at which the given status became applicable", formalDefinition="The date at which the given status became applicable." ) 1798 protected DateTimeType statusDate; 1799 1800 /** 1801 * A total of the complete count of contained items of a particular type/form, independent of sub-packaging or organization. This can be considered as the pack size. This attribute differs from containedItem.amount in that it can give a single aggregated count of all tablet types in a pack, even when these are different manufactured items. For example a pill pack of 21 tablets plus 7 sugar tablets, can be denoted here as '28 tablets'. This attribute is repeatable so that the different item types in one pack type can be counted (e.g. a count of vials and count of syringes). Each repeat must have different units, so that it is clear what the different sets of counted items are, and it is not intended to allow different counts of similar items (e.g. not '2 tubes and 3 tubes'). Repeats are not to be used to represent different pack sizes (e.g. 20 pack vs. 50 pack) - which would be different instances of this resource. 1802 */ 1803 @Child(name = "containedItemQuantity", type = {Quantity.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1804 @Description(shortDefinition="A total of the complete count of contained items of a particular type/form, independent of sub-packaging or organization. This can be considered as the pack size. See also packaging.containedItem.amount (especially the long definition)", formalDefinition="A total of the complete count of contained items of a particular type/form, independent of sub-packaging or organization. This can be considered as the pack size. This attribute differs from containedItem.amount in that it can give a single aggregated count of all tablet types in a pack, even when these are different manufactured items. For example a pill pack of 21 tablets plus 7 sugar tablets, can be denoted here as '28 tablets'. This attribute is repeatable so that the different item types in one pack type can be counted (e.g. a count of vials and count of syringes). Each repeat must have different units, so that it is clear what the different sets of counted items are, and it is not intended to allow different counts of similar items (e.g. not '2 tubes and 3 tubes'). Repeats are not to be used to represent different pack sizes (e.g. 20 pack vs. 50 pack) - which would be different instances of this resource." ) 1805 protected List<Quantity> containedItemQuantity; 1806 1807 /** 1808 * Textual description. Note that this is not the name of the package or product. 1809 */ 1810 @Child(name = "description", type = {MarkdownType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1811 @Description(shortDefinition="Textual description. Note that this is not the name of the package or product", formalDefinition="Textual description. Note that this is not the name of the package or product." ) 1812 protected MarkdownType description; 1813 1814 /** 1815 * The legal status of supply of the packaged item as classified by the regulator. 1816 */ 1817 @Child(name = "legalStatusOfSupply", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1818 @Description(shortDefinition="The legal status of supply of the packaged item as classified by the regulator", formalDefinition="The legal status of supply of the packaged item as classified by the regulator." ) 1819 protected List<PackagedProductDefinitionLegalStatusOfSupplyComponent> legalStatusOfSupply; 1820 1821 /** 1822 * Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated. 1823 */ 1824 @Child(name = "marketingStatus", type = {MarketingStatus.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1825 @Description(shortDefinition="Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated", formalDefinition="Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated." ) 1826 protected List<MarketingStatus> marketingStatus; 1827 1828 /** 1829 * Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant. 1830 */ 1831 @Child(name = "copackagedIndicator", type = {BooleanType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1832 @Description(shortDefinition="Identifies if the drug product is supplied with another item such as a diluent or adjuvant", formalDefinition="Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant." ) 1833 protected BooleanType copackagedIndicator; 1834 1835 /** 1836 * Manufacturer of this package type. When there are multiple it means these are all possible manufacturers. 1837 */ 1838 @Child(name = "manufacturer", type = {Organization.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1839 @Description(shortDefinition="Manufacturer of this package type (multiple means these are all possible manufacturers)", formalDefinition="Manufacturer of this package type. When there are multiple it means these are all possible manufacturers." ) 1840 protected List<Reference> manufacturer; 1841 1842 /** 1843 * Additional information or supporting documentation about the packaged product. 1844 */ 1845 @Child(name = "attachedDocument", type = {DocumentReference.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1846 @Description(shortDefinition="Additional information or supporting documentation about the packaged product", formalDefinition="Additional information or supporting documentation about the packaged product." ) 1847 protected List<Reference> attachedDocument; 1848 1849 /** 1850 * A packaging item, as a container for medically related items, possibly with other packaging items within, or a packaging component, such as bottle cap (which is not a device or a medication manufactured item). 1851 */ 1852 @Child(name = "packaging", type = {}, order=13, min=0, max=1, modifier=false, summary=true) 1853 @Description(shortDefinition="A packaging item, as a container for medically related items, possibly with other packaging items within, or a packaging component, such as bottle cap", formalDefinition="A packaging item, as a container for medically related items, possibly with other packaging items within, or a packaging component, such as bottle cap (which is not a device or a medication manufactured item)." ) 1854 protected PackagedProductDefinitionPackagingComponent packaging; 1855 1856 /** 1857 * Allows the key features to be recorded, such as "hospital pack", "nurse prescribable", "calendar pack". 1858 */ 1859 @Child(name = "characteristic", type = {PackagedProductDefinitionPackagingPropertyComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1860 @Description(shortDefinition="Allows the key features to be recorded, such as \"hospital pack\", \"nurse prescribable\"", formalDefinition="Allows the key features to be recorded, such as \"hospital pack\", \"nurse prescribable\", \"calendar pack\"." ) 1861 protected List<PackagedProductDefinitionPackagingPropertyComponent> characteristic; 1862 1863 private static final long serialVersionUID = -1670129447L; 1864 1865 /** 1866 * Constructor 1867 */ 1868 public PackagedProductDefinition() { 1869 super(); 1870 } 1871 1872 /** 1873 * @return {@link #identifier} (A unique identifier for this package as whole - not the the content of the package. Unique instance identifiers assigned to a package by manufacturers, regulators, drug catalogue custodians or other organizations.) 1874 */ 1875 public List<Identifier> getIdentifier() { 1876 if (this.identifier == null) 1877 this.identifier = new ArrayList<Identifier>(); 1878 return this.identifier; 1879 } 1880 1881 /** 1882 * @return Returns a reference to <code>this</code> for easy method chaining 1883 */ 1884 public PackagedProductDefinition setIdentifier(List<Identifier> theIdentifier) { 1885 this.identifier = theIdentifier; 1886 return this; 1887 } 1888 1889 public boolean hasIdentifier() { 1890 if (this.identifier == null) 1891 return false; 1892 for (Identifier item : this.identifier) 1893 if (!item.isEmpty()) 1894 return true; 1895 return false; 1896 } 1897 1898 public Identifier addIdentifier() { //3 1899 Identifier t = new Identifier(); 1900 if (this.identifier == null) 1901 this.identifier = new ArrayList<Identifier>(); 1902 this.identifier.add(t); 1903 return t; 1904 } 1905 1906 public PackagedProductDefinition addIdentifier(Identifier t) { //3 1907 if (t == null) 1908 return this; 1909 if (this.identifier == null) 1910 this.identifier = new ArrayList<Identifier>(); 1911 this.identifier.add(t); 1912 return this; 1913 } 1914 1915 /** 1916 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1917 */ 1918 public Identifier getIdentifierFirstRep() { 1919 if (getIdentifier().isEmpty()) { 1920 addIdentifier(); 1921 } 1922 return getIdentifier().get(0); 1923 } 1924 1925 /** 1926 * @return {@link #name} (A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1927 */ 1928 public StringType getNameElement() { 1929 if (this.name == null) 1930 if (Configuration.errorOnAutoCreate()) 1931 throw new Error("Attempt to auto-create PackagedProductDefinition.name"); 1932 else if (Configuration.doAutoCreate()) 1933 this.name = new StringType(); // bb 1934 return this.name; 1935 } 1936 1937 public boolean hasNameElement() { 1938 return this.name != null && !this.name.isEmpty(); 1939 } 1940 1941 public boolean hasName() { 1942 return this.name != null && !this.name.isEmpty(); 1943 } 1944 1945 /** 1946 * @param value {@link #name} (A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1947 */ 1948 public PackagedProductDefinition setNameElement(StringType value) { 1949 this.name = value; 1950 return this; 1951 } 1952 1953 /** 1954 * @return A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc. 1955 */ 1956 public String getName() { 1957 return this.name == null ? null : this.name.getValue(); 1958 } 1959 1960 /** 1961 * @param value A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc. 1962 */ 1963 public PackagedProductDefinition setName(String value) { 1964 if (Utilities.noString(value)) 1965 this.name = null; 1966 else { 1967 if (this.name == null) 1968 this.name = new StringType(); 1969 this.name.setValue(value); 1970 } 1971 return this; 1972 } 1973 1974 /** 1975 * @return {@link #type} (A high level category e.g. medicinal product, raw material, shipping/transport container, etc.) 1976 */ 1977 public CodeableConcept getType() { 1978 if (this.type == null) 1979 if (Configuration.errorOnAutoCreate()) 1980 throw new Error("Attempt to auto-create PackagedProductDefinition.type"); 1981 else if (Configuration.doAutoCreate()) 1982 this.type = new CodeableConcept(); // cc 1983 return this.type; 1984 } 1985 1986 public boolean hasType() { 1987 return this.type != null && !this.type.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #type} (A high level category e.g. medicinal product, raw material, shipping/transport container, etc.) 1992 */ 1993 public PackagedProductDefinition setType(CodeableConcept value) { 1994 this.type = value; 1995 return this; 1996 } 1997 1998 /** 1999 * @return {@link #packageFor} (The product this package model relates to, not the contents of the package (for which see package.containedItem).) 2000 */ 2001 public List<Reference> getPackageFor() { 2002 if (this.packageFor == null) 2003 this.packageFor = new ArrayList<Reference>(); 2004 return this.packageFor; 2005 } 2006 2007 /** 2008 * @return Returns a reference to <code>this</code> for easy method chaining 2009 */ 2010 public PackagedProductDefinition setPackageFor(List<Reference> thePackageFor) { 2011 this.packageFor = thePackageFor; 2012 return this; 2013 } 2014 2015 public boolean hasPackageFor() { 2016 if (this.packageFor == null) 2017 return false; 2018 for (Reference item : this.packageFor) 2019 if (!item.isEmpty()) 2020 return true; 2021 return false; 2022 } 2023 2024 public Reference addPackageFor() { //3 2025 Reference t = new Reference(); 2026 if (this.packageFor == null) 2027 this.packageFor = new ArrayList<Reference>(); 2028 this.packageFor.add(t); 2029 return t; 2030 } 2031 2032 public PackagedProductDefinition addPackageFor(Reference t) { //3 2033 if (t == null) 2034 return this; 2035 if (this.packageFor == null) 2036 this.packageFor = new ArrayList<Reference>(); 2037 this.packageFor.add(t); 2038 return this; 2039 } 2040 2041 /** 2042 * @return The first repetition of repeating field {@link #packageFor}, creating it if it does not already exist {3} 2043 */ 2044 public Reference getPackageForFirstRep() { 2045 if (getPackageFor().isEmpty()) { 2046 addPackageFor(); 2047 } 2048 return getPackageFor().get(0); 2049 } 2050 2051 /** 2052 * @return {@link #status} (The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status.) 2053 */ 2054 public CodeableConcept getStatus() { 2055 if (this.status == null) 2056 if (Configuration.errorOnAutoCreate()) 2057 throw new Error("Attempt to auto-create PackagedProductDefinition.status"); 2058 else if (Configuration.doAutoCreate()) 2059 this.status = new CodeableConcept(); // cc 2060 return this.status; 2061 } 2062 2063 public boolean hasStatus() { 2064 return this.status != null && !this.status.isEmpty(); 2065 } 2066 2067 /** 2068 * @param value {@link #status} (The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status.) 2069 */ 2070 public PackagedProductDefinition setStatus(CodeableConcept value) { 2071 this.status = value; 2072 return this; 2073 } 2074 2075 /** 2076 * @return {@link #statusDate} (The date at which the given status became applicable.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 2077 */ 2078 public DateTimeType getStatusDateElement() { 2079 if (this.statusDate == null) 2080 if (Configuration.errorOnAutoCreate()) 2081 throw new Error("Attempt to auto-create PackagedProductDefinition.statusDate"); 2082 else if (Configuration.doAutoCreate()) 2083 this.statusDate = new DateTimeType(); // bb 2084 return this.statusDate; 2085 } 2086 2087 public boolean hasStatusDateElement() { 2088 return this.statusDate != null && !this.statusDate.isEmpty(); 2089 } 2090 2091 public boolean hasStatusDate() { 2092 return this.statusDate != null && !this.statusDate.isEmpty(); 2093 } 2094 2095 /** 2096 * @param value {@link #statusDate} (The date at which the given status became applicable.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 2097 */ 2098 public PackagedProductDefinition setStatusDateElement(DateTimeType value) { 2099 this.statusDate = value; 2100 return this; 2101 } 2102 2103 /** 2104 * @return The date at which the given status became applicable. 2105 */ 2106 public Date getStatusDate() { 2107 return this.statusDate == null ? null : this.statusDate.getValue(); 2108 } 2109 2110 /** 2111 * @param value The date at which the given status became applicable. 2112 */ 2113 public PackagedProductDefinition setStatusDate(Date value) { 2114 if (value == null) 2115 this.statusDate = null; 2116 else { 2117 if (this.statusDate == null) 2118 this.statusDate = new DateTimeType(); 2119 this.statusDate.setValue(value); 2120 } 2121 return this; 2122 } 2123 2124 /** 2125 * @return {@link #containedItemQuantity} (A total of the complete count of contained items of a particular type/form, independent of sub-packaging or organization. This can be considered as the pack size. This attribute differs from containedItem.amount in that it can give a single aggregated count of all tablet types in a pack, even when these are different manufactured items. For example a pill pack of 21 tablets plus 7 sugar tablets, can be denoted here as '28 tablets'. This attribute is repeatable so that the different item types in one pack type can be counted (e.g. a count of vials and count of syringes). Each repeat must have different units, so that it is clear what the different sets of counted items are, and it is not intended to allow different counts of similar items (e.g. not '2 tubes and 3 tubes'). Repeats are not to be used to represent different pack sizes (e.g. 20 pack vs. 50 pack) - which would be different instances of this resource.) 2126 */ 2127 public List<Quantity> getContainedItemQuantity() { 2128 if (this.containedItemQuantity == null) 2129 this.containedItemQuantity = new ArrayList<Quantity>(); 2130 return this.containedItemQuantity; 2131 } 2132 2133 /** 2134 * @return Returns a reference to <code>this</code> for easy method chaining 2135 */ 2136 public PackagedProductDefinition setContainedItemQuantity(List<Quantity> theContainedItemQuantity) { 2137 this.containedItemQuantity = theContainedItemQuantity; 2138 return this; 2139 } 2140 2141 public boolean hasContainedItemQuantity() { 2142 if (this.containedItemQuantity == null) 2143 return false; 2144 for (Quantity item : this.containedItemQuantity) 2145 if (!item.isEmpty()) 2146 return true; 2147 return false; 2148 } 2149 2150 public Quantity addContainedItemQuantity() { //3 2151 Quantity t = new Quantity(); 2152 if (this.containedItemQuantity == null) 2153 this.containedItemQuantity = new ArrayList<Quantity>(); 2154 this.containedItemQuantity.add(t); 2155 return t; 2156 } 2157 2158 public PackagedProductDefinition addContainedItemQuantity(Quantity t) { //3 2159 if (t == null) 2160 return this; 2161 if (this.containedItemQuantity == null) 2162 this.containedItemQuantity = new ArrayList<Quantity>(); 2163 this.containedItemQuantity.add(t); 2164 return this; 2165 } 2166 2167 /** 2168 * @return The first repetition of repeating field {@link #containedItemQuantity}, creating it if it does not already exist {3} 2169 */ 2170 public Quantity getContainedItemQuantityFirstRep() { 2171 if (getContainedItemQuantity().isEmpty()) { 2172 addContainedItemQuantity(); 2173 } 2174 return getContainedItemQuantity().get(0); 2175 } 2176 2177 /** 2178 * @return {@link #description} (Textual description. Note that this is not the name of the package or product.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2179 */ 2180 public MarkdownType getDescriptionElement() { 2181 if (this.description == null) 2182 if (Configuration.errorOnAutoCreate()) 2183 throw new Error("Attempt to auto-create PackagedProductDefinition.description"); 2184 else if (Configuration.doAutoCreate()) 2185 this.description = new MarkdownType(); // bb 2186 return this.description; 2187 } 2188 2189 public boolean hasDescriptionElement() { 2190 return this.description != null && !this.description.isEmpty(); 2191 } 2192 2193 public boolean hasDescription() { 2194 return this.description != null && !this.description.isEmpty(); 2195 } 2196 2197 /** 2198 * @param value {@link #description} (Textual description. Note that this is not the name of the package or product.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2199 */ 2200 public PackagedProductDefinition setDescriptionElement(MarkdownType value) { 2201 this.description = value; 2202 return this; 2203 } 2204 2205 /** 2206 * @return Textual description. Note that this is not the name of the package or product. 2207 */ 2208 public String getDescription() { 2209 return this.description == null ? null : this.description.getValue(); 2210 } 2211 2212 /** 2213 * @param value Textual description. Note that this is not the name of the package or product. 2214 */ 2215 public PackagedProductDefinition setDescription(String value) { 2216 if (Utilities.noString(value)) 2217 this.description = null; 2218 else { 2219 if (this.description == null) 2220 this.description = new MarkdownType(); 2221 this.description.setValue(value); 2222 } 2223 return this; 2224 } 2225 2226 /** 2227 * @return {@link #legalStatusOfSupply} (The legal status of supply of the packaged item as classified by the regulator.) 2228 */ 2229 public List<PackagedProductDefinitionLegalStatusOfSupplyComponent> getLegalStatusOfSupply() { 2230 if (this.legalStatusOfSupply == null) 2231 this.legalStatusOfSupply = new ArrayList<PackagedProductDefinitionLegalStatusOfSupplyComponent>(); 2232 return this.legalStatusOfSupply; 2233 } 2234 2235 /** 2236 * @return Returns a reference to <code>this</code> for easy method chaining 2237 */ 2238 public PackagedProductDefinition setLegalStatusOfSupply(List<PackagedProductDefinitionLegalStatusOfSupplyComponent> theLegalStatusOfSupply) { 2239 this.legalStatusOfSupply = theLegalStatusOfSupply; 2240 return this; 2241 } 2242 2243 public boolean hasLegalStatusOfSupply() { 2244 if (this.legalStatusOfSupply == null) 2245 return false; 2246 for (PackagedProductDefinitionLegalStatusOfSupplyComponent item : this.legalStatusOfSupply) 2247 if (!item.isEmpty()) 2248 return true; 2249 return false; 2250 } 2251 2252 public PackagedProductDefinitionLegalStatusOfSupplyComponent addLegalStatusOfSupply() { //3 2253 PackagedProductDefinitionLegalStatusOfSupplyComponent t = new PackagedProductDefinitionLegalStatusOfSupplyComponent(); 2254 if (this.legalStatusOfSupply == null) 2255 this.legalStatusOfSupply = new ArrayList<PackagedProductDefinitionLegalStatusOfSupplyComponent>(); 2256 this.legalStatusOfSupply.add(t); 2257 return t; 2258 } 2259 2260 public PackagedProductDefinition addLegalStatusOfSupply(PackagedProductDefinitionLegalStatusOfSupplyComponent t) { //3 2261 if (t == null) 2262 return this; 2263 if (this.legalStatusOfSupply == null) 2264 this.legalStatusOfSupply = new ArrayList<PackagedProductDefinitionLegalStatusOfSupplyComponent>(); 2265 this.legalStatusOfSupply.add(t); 2266 return this; 2267 } 2268 2269 /** 2270 * @return The first repetition of repeating field {@link #legalStatusOfSupply}, creating it if it does not already exist {3} 2271 */ 2272 public PackagedProductDefinitionLegalStatusOfSupplyComponent getLegalStatusOfSupplyFirstRep() { 2273 if (getLegalStatusOfSupply().isEmpty()) { 2274 addLegalStatusOfSupply(); 2275 } 2276 return getLegalStatusOfSupply().get(0); 2277 } 2278 2279 /** 2280 * @return {@link #marketingStatus} (Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated.) 2281 */ 2282 public List<MarketingStatus> getMarketingStatus() { 2283 if (this.marketingStatus == null) 2284 this.marketingStatus = new ArrayList<MarketingStatus>(); 2285 return this.marketingStatus; 2286 } 2287 2288 /** 2289 * @return Returns a reference to <code>this</code> for easy method chaining 2290 */ 2291 public PackagedProductDefinition setMarketingStatus(List<MarketingStatus> theMarketingStatus) { 2292 this.marketingStatus = theMarketingStatus; 2293 return this; 2294 } 2295 2296 public boolean hasMarketingStatus() { 2297 if (this.marketingStatus == null) 2298 return false; 2299 for (MarketingStatus item : this.marketingStatus) 2300 if (!item.isEmpty()) 2301 return true; 2302 return false; 2303 } 2304 2305 public MarketingStatus addMarketingStatus() { //3 2306 MarketingStatus t = new MarketingStatus(); 2307 if (this.marketingStatus == null) 2308 this.marketingStatus = new ArrayList<MarketingStatus>(); 2309 this.marketingStatus.add(t); 2310 return t; 2311 } 2312 2313 public PackagedProductDefinition addMarketingStatus(MarketingStatus t) { //3 2314 if (t == null) 2315 return this; 2316 if (this.marketingStatus == null) 2317 this.marketingStatus = new ArrayList<MarketingStatus>(); 2318 this.marketingStatus.add(t); 2319 return this; 2320 } 2321 2322 /** 2323 * @return The first repetition of repeating field {@link #marketingStatus}, creating it if it does not already exist {3} 2324 */ 2325 public MarketingStatus getMarketingStatusFirstRep() { 2326 if (getMarketingStatus().isEmpty()) { 2327 addMarketingStatus(); 2328 } 2329 return getMarketingStatus().get(0); 2330 } 2331 2332 /** 2333 * @return {@link #copackagedIndicator} (Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant.). This is the underlying object with id, value and extensions. The accessor "getCopackagedIndicator" gives direct access to the value 2334 */ 2335 public BooleanType getCopackagedIndicatorElement() { 2336 if (this.copackagedIndicator == null) 2337 if (Configuration.errorOnAutoCreate()) 2338 throw new Error("Attempt to auto-create PackagedProductDefinition.copackagedIndicator"); 2339 else if (Configuration.doAutoCreate()) 2340 this.copackagedIndicator = new BooleanType(); // bb 2341 return this.copackagedIndicator; 2342 } 2343 2344 public boolean hasCopackagedIndicatorElement() { 2345 return this.copackagedIndicator != null && !this.copackagedIndicator.isEmpty(); 2346 } 2347 2348 public boolean hasCopackagedIndicator() { 2349 return this.copackagedIndicator != null && !this.copackagedIndicator.isEmpty(); 2350 } 2351 2352 /** 2353 * @param value {@link #copackagedIndicator} (Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant.). This is the underlying object with id, value and extensions. The accessor "getCopackagedIndicator" gives direct access to the value 2354 */ 2355 public PackagedProductDefinition setCopackagedIndicatorElement(BooleanType value) { 2356 this.copackagedIndicator = value; 2357 return this; 2358 } 2359 2360 /** 2361 * @return Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant. 2362 */ 2363 public boolean getCopackagedIndicator() { 2364 return this.copackagedIndicator == null || this.copackagedIndicator.isEmpty() ? false : this.copackagedIndicator.getValue(); 2365 } 2366 2367 /** 2368 * @param value Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant. 2369 */ 2370 public PackagedProductDefinition setCopackagedIndicator(boolean value) { 2371 if (this.copackagedIndicator == null) 2372 this.copackagedIndicator = new BooleanType(); 2373 this.copackagedIndicator.setValue(value); 2374 return this; 2375 } 2376 2377 /** 2378 * @return {@link #manufacturer} (Manufacturer of this package type. When there are multiple it means these are all possible manufacturers.) 2379 */ 2380 public List<Reference> getManufacturer() { 2381 if (this.manufacturer == null) 2382 this.manufacturer = new ArrayList<Reference>(); 2383 return this.manufacturer; 2384 } 2385 2386 /** 2387 * @return Returns a reference to <code>this</code> for easy method chaining 2388 */ 2389 public PackagedProductDefinition setManufacturer(List<Reference> theManufacturer) { 2390 this.manufacturer = theManufacturer; 2391 return this; 2392 } 2393 2394 public boolean hasManufacturer() { 2395 if (this.manufacturer == null) 2396 return false; 2397 for (Reference item : this.manufacturer) 2398 if (!item.isEmpty()) 2399 return true; 2400 return false; 2401 } 2402 2403 public Reference addManufacturer() { //3 2404 Reference t = new Reference(); 2405 if (this.manufacturer == null) 2406 this.manufacturer = new ArrayList<Reference>(); 2407 this.manufacturer.add(t); 2408 return t; 2409 } 2410 2411 public PackagedProductDefinition addManufacturer(Reference t) { //3 2412 if (t == null) 2413 return this; 2414 if (this.manufacturer == null) 2415 this.manufacturer = new ArrayList<Reference>(); 2416 this.manufacturer.add(t); 2417 return this; 2418 } 2419 2420 /** 2421 * @return The first repetition of repeating field {@link #manufacturer}, creating it if it does not already exist {3} 2422 */ 2423 public Reference getManufacturerFirstRep() { 2424 if (getManufacturer().isEmpty()) { 2425 addManufacturer(); 2426 } 2427 return getManufacturer().get(0); 2428 } 2429 2430 /** 2431 * @return {@link #attachedDocument} (Additional information or supporting documentation about the packaged product.) 2432 */ 2433 public List<Reference> getAttachedDocument() { 2434 if (this.attachedDocument == null) 2435 this.attachedDocument = new ArrayList<Reference>(); 2436 return this.attachedDocument; 2437 } 2438 2439 /** 2440 * @return Returns a reference to <code>this</code> for easy method chaining 2441 */ 2442 public PackagedProductDefinition setAttachedDocument(List<Reference> theAttachedDocument) { 2443 this.attachedDocument = theAttachedDocument; 2444 return this; 2445 } 2446 2447 public boolean hasAttachedDocument() { 2448 if (this.attachedDocument == null) 2449 return false; 2450 for (Reference item : this.attachedDocument) 2451 if (!item.isEmpty()) 2452 return true; 2453 return false; 2454 } 2455 2456 public Reference addAttachedDocument() { //3 2457 Reference t = new Reference(); 2458 if (this.attachedDocument == null) 2459 this.attachedDocument = new ArrayList<Reference>(); 2460 this.attachedDocument.add(t); 2461 return t; 2462 } 2463 2464 public PackagedProductDefinition addAttachedDocument(Reference t) { //3 2465 if (t == null) 2466 return this; 2467 if (this.attachedDocument == null) 2468 this.attachedDocument = new ArrayList<Reference>(); 2469 this.attachedDocument.add(t); 2470 return this; 2471 } 2472 2473 /** 2474 * @return The first repetition of repeating field {@link #attachedDocument}, creating it if it does not already exist {3} 2475 */ 2476 public Reference getAttachedDocumentFirstRep() { 2477 if (getAttachedDocument().isEmpty()) { 2478 addAttachedDocument(); 2479 } 2480 return getAttachedDocument().get(0); 2481 } 2482 2483 /** 2484 * @return {@link #packaging} (A packaging item, as a container for medically related items, possibly with other packaging items within, or a packaging component, such as bottle cap (which is not a device or a medication manufactured item).) 2485 */ 2486 public PackagedProductDefinitionPackagingComponent getPackaging() { 2487 if (this.packaging == null) 2488 if (Configuration.errorOnAutoCreate()) 2489 throw new Error("Attempt to auto-create PackagedProductDefinition.packaging"); 2490 else if (Configuration.doAutoCreate()) 2491 this.packaging = new PackagedProductDefinitionPackagingComponent(); // cc 2492 return this.packaging; 2493 } 2494 2495 public boolean hasPackaging() { 2496 return this.packaging != null && !this.packaging.isEmpty(); 2497 } 2498 2499 /** 2500 * @param value {@link #packaging} (A packaging item, as a container for medically related items, possibly with other packaging items within, or a packaging component, such as bottle cap (which is not a device or a medication manufactured item).) 2501 */ 2502 public PackagedProductDefinition setPackaging(PackagedProductDefinitionPackagingComponent value) { 2503 this.packaging = value; 2504 return this; 2505 } 2506 2507 /** 2508 * @return {@link #characteristic} (Allows the key features to be recorded, such as "hospital pack", "nurse prescribable", "calendar pack".) 2509 */ 2510 public List<PackagedProductDefinitionPackagingPropertyComponent> getCharacteristic() { 2511 if (this.characteristic == null) 2512 this.characteristic = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 2513 return this.characteristic; 2514 } 2515 2516 /** 2517 * @return Returns a reference to <code>this</code> for easy method chaining 2518 */ 2519 public PackagedProductDefinition setCharacteristic(List<PackagedProductDefinitionPackagingPropertyComponent> theCharacteristic) { 2520 this.characteristic = theCharacteristic; 2521 return this; 2522 } 2523 2524 public boolean hasCharacteristic() { 2525 if (this.characteristic == null) 2526 return false; 2527 for (PackagedProductDefinitionPackagingPropertyComponent item : this.characteristic) 2528 if (!item.isEmpty()) 2529 return true; 2530 return false; 2531 } 2532 2533 public PackagedProductDefinitionPackagingPropertyComponent addCharacteristic() { //3 2534 PackagedProductDefinitionPackagingPropertyComponent t = new PackagedProductDefinitionPackagingPropertyComponent(); 2535 if (this.characteristic == null) 2536 this.characteristic = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 2537 this.characteristic.add(t); 2538 return t; 2539 } 2540 2541 public PackagedProductDefinition addCharacteristic(PackagedProductDefinitionPackagingPropertyComponent t) { //3 2542 if (t == null) 2543 return this; 2544 if (this.characteristic == null) 2545 this.characteristic = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 2546 this.characteristic.add(t); 2547 return this; 2548 } 2549 2550 /** 2551 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 2552 */ 2553 public PackagedProductDefinitionPackagingPropertyComponent getCharacteristicFirstRep() { 2554 if (getCharacteristic().isEmpty()) { 2555 addCharacteristic(); 2556 } 2557 return getCharacteristic().get(0); 2558 } 2559 2560 protected void listChildren(List<Property> children) { 2561 super.listChildren(children); 2562 children.add(new Property("identifier", "Identifier", "A unique identifier for this package as whole - not the the content of the package. Unique instance identifiers assigned to a package by manufacturers, regulators, drug catalogue custodians or other organizations.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2563 children.add(new Property("name", "string", "A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc.", 0, 1, name)); 2564 children.add(new Property("type", "CodeableConcept", "A high level category e.g. medicinal product, raw material, shipping/transport container, etc.", 0, 1, type)); 2565 children.add(new Property("packageFor", "Reference(MedicinalProductDefinition)", "The product this package model relates to, not the contents of the package (for which see package.containedItem).", 0, java.lang.Integer.MAX_VALUE, packageFor)); 2566 children.add(new Property("status", "CodeableConcept", "The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status.", 0, 1, status)); 2567 children.add(new Property("statusDate", "dateTime", "The date at which the given status became applicable.", 0, 1, statusDate)); 2568 children.add(new Property("containedItemQuantity", "Quantity", "A total of the complete count of contained items of a particular type/form, independent of sub-packaging or organization. This can be considered as the pack size. This attribute differs from containedItem.amount in that it can give a single aggregated count of all tablet types in a pack, even when these are different manufactured items. For example a pill pack of 21 tablets plus 7 sugar tablets, can be denoted here as '28 tablets'. This attribute is repeatable so that the different item types in one pack type can be counted (e.g. a count of vials and count of syringes). Each repeat must have different units, so that it is clear what the different sets of counted items are, and it is not intended to allow different counts of similar items (e.g. not '2 tubes and 3 tubes'). Repeats are not to be used to represent different pack sizes (e.g. 20 pack vs. 50 pack) - which would be different instances of this resource.", 0, java.lang.Integer.MAX_VALUE, containedItemQuantity)); 2569 children.add(new Property("description", "markdown", "Textual description. Note that this is not the name of the package or product.", 0, 1, description)); 2570 children.add(new Property("legalStatusOfSupply", "", "The legal status of supply of the packaged item as classified by the regulator.", 0, java.lang.Integer.MAX_VALUE, legalStatusOfSupply)); 2571 children.add(new Property("marketingStatus", "MarketingStatus", "Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated.", 0, java.lang.Integer.MAX_VALUE, marketingStatus)); 2572 children.add(new Property("copackagedIndicator", "boolean", "Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant.", 0, 1, copackagedIndicator)); 2573 children.add(new Property("manufacturer", "Reference(Organization)", "Manufacturer of this package type. When there are multiple it means these are all possible manufacturers.", 0, java.lang.Integer.MAX_VALUE, manufacturer)); 2574 children.add(new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the packaged product.", 0, java.lang.Integer.MAX_VALUE, attachedDocument)); 2575 children.add(new Property("packaging", "", "A packaging item, as a container for medically related items, possibly with other packaging items within, or a packaging component, such as bottle cap (which is not a device or a medication manufactured item).", 0, 1, packaging)); 2576 children.add(new Property("characteristic", "@PackagedProductDefinition.packaging.property", "Allows the key features to be recorded, such as \"hospital pack\", \"nurse prescribable\", \"calendar pack\".", 0, java.lang.Integer.MAX_VALUE, characteristic)); 2577 } 2578 2579 @Override 2580 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2581 switch (_hash) { 2582 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier for this package as whole - not the the content of the package. Unique instance identifiers assigned to a package by manufacturers, regulators, drug catalogue custodians or other organizations.", 0, java.lang.Integer.MAX_VALUE, identifier); 2583 case 3373707: /*name*/ return new Property("name", "string", "A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc.", 0, 1, name); 2584 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A high level category e.g. medicinal product, raw material, shipping/transport container, etc.", 0, 1, type); 2585 case 29307555: /*packageFor*/ return new Property("packageFor", "Reference(MedicinalProductDefinition)", "The product this package model relates to, not the contents of the package (for which see package.containedItem).", 0, java.lang.Integer.MAX_VALUE, packageFor); 2586 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status.", 0, 1, status); 2587 case 247524032: /*statusDate*/ return new Property("statusDate", "dateTime", "The date at which the given status became applicable.", 0, 1, statusDate); 2588 case -1686893359: /*containedItemQuantity*/ return new Property("containedItemQuantity", "Quantity", "A total of the complete count of contained items of a particular type/form, independent of sub-packaging or organization. This can be considered as the pack size. This attribute differs from containedItem.amount in that it can give a single aggregated count of all tablet types in a pack, even when these are different manufactured items. For example a pill pack of 21 tablets plus 7 sugar tablets, can be denoted here as '28 tablets'. This attribute is repeatable so that the different item types in one pack type can be counted (e.g. a count of vials and count of syringes). Each repeat must have different units, so that it is clear what the different sets of counted items are, and it is not intended to allow different counts of similar items (e.g. not '2 tubes and 3 tubes'). Repeats are not to be used to represent different pack sizes (e.g. 20 pack vs. 50 pack) - which would be different instances of this resource.", 0, java.lang.Integer.MAX_VALUE, containedItemQuantity); 2589 case -1724546052: /*description*/ return new Property("description", "markdown", "Textual description. Note that this is not the name of the package or product.", 0, 1, description); 2590 case -844874031: /*legalStatusOfSupply*/ return new Property("legalStatusOfSupply", "", "The legal status of supply of the packaged item as classified by the regulator.", 0, java.lang.Integer.MAX_VALUE, legalStatusOfSupply); 2591 case 70767032: /*marketingStatus*/ return new Property("marketingStatus", "MarketingStatus", "Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated.", 0, java.lang.Integer.MAX_VALUE, marketingStatus); 2592 case -1638663195: /*copackagedIndicator*/ return new Property("copackagedIndicator", "boolean", "Identifies if the package contains different items, such as when a drug product is supplied with another item e.g. a diluent or adjuvant.", 0, 1, copackagedIndicator); 2593 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "Manufacturer of this package type. When there are multiple it means these are all possible manufacturers.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 2594 case -513945889: /*attachedDocument*/ return new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the packaged product.", 0, java.lang.Integer.MAX_VALUE, attachedDocument); 2595 case 1802065795: /*packaging*/ return new Property("packaging", "", "A packaging item, as a container for medically related items, possibly with other packaging items within, or a packaging component, such as bottle cap (which is not a device or a medication manufactured item).", 0, 1, packaging); 2596 case 366313883: /*characteristic*/ return new Property("characteristic", "@PackagedProductDefinition.packaging.property", "Allows the key features to be recorded, such as \"hospital pack\", \"nurse prescribable\", \"calendar pack\".", 0, java.lang.Integer.MAX_VALUE, characteristic); 2597 default: return super.getNamedProperty(_hash, _name, _checkValid); 2598 } 2599 2600 } 2601 2602 @Override 2603 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2604 switch (hash) { 2605 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2606 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2607 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2608 case 29307555: /*packageFor*/ return this.packageFor == null ? new Base[0] : this.packageFor.toArray(new Base[this.packageFor.size()]); // Reference 2609 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 2610 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateTimeType 2611 case -1686893359: /*containedItemQuantity*/ return this.containedItemQuantity == null ? new Base[0] : this.containedItemQuantity.toArray(new Base[this.containedItemQuantity.size()]); // Quantity 2612 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2613 case -844874031: /*legalStatusOfSupply*/ return this.legalStatusOfSupply == null ? new Base[0] : this.legalStatusOfSupply.toArray(new Base[this.legalStatusOfSupply.size()]); // PackagedProductDefinitionLegalStatusOfSupplyComponent 2614 case 70767032: /*marketingStatus*/ return this.marketingStatus == null ? new Base[0] : this.marketingStatus.toArray(new Base[this.marketingStatus.size()]); // MarketingStatus 2615 case -1638663195: /*copackagedIndicator*/ return this.copackagedIndicator == null ? new Base[0] : new Base[] {this.copackagedIndicator}; // BooleanType 2616 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 2617 case -513945889: /*attachedDocument*/ return this.attachedDocument == null ? new Base[0] : this.attachedDocument.toArray(new Base[this.attachedDocument.size()]); // Reference 2618 case 1802065795: /*packaging*/ return this.packaging == null ? new Base[0] : new Base[] {this.packaging}; // PackagedProductDefinitionPackagingComponent 2619 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // PackagedProductDefinitionPackagingPropertyComponent 2620 default: return super.getProperty(hash, name, checkValid); 2621 } 2622 2623 } 2624 2625 @Override 2626 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2627 switch (hash) { 2628 case -1618432855: // identifier 2629 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2630 return value; 2631 case 3373707: // name 2632 this.name = TypeConvertor.castToString(value); // StringType 2633 return value; 2634 case 3575610: // type 2635 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2636 return value; 2637 case 29307555: // packageFor 2638 this.getPackageFor().add(TypeConvertor.castToReference(value)); // Reference 2639 return value; 2640 case -892481550: // status 2641 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2642 return value; 2643 case 247524032: // statusDate 2644 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 2645 return value; 2646 case -1686893359: // containedItemQuantity 2647 this.getContainedItemQuantity().add(TypeConvertor.castToQuantity(value)); // Quantity 2648 return value; 2649 case -1724546052: // description 2650 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2651 return value; 2652 case -844874031: // legalStatusOfSupply 2653 this.getLegalStatusOfSupply().add((PackagedProductDefinitionLegalStatusOfSupplyComponent) value); // PackagedProductDefinitionLegalStatusOfSupplyComponent 2654 return value; 2655 case 70767032: // marketingStatus 2656 this.getMarketingStatus().add(TypeConvertor.castToMarketingStatus(value)); // MarketingStatus 2657 return value; 2658 case -1638663195: // copackagedIndicator 2659 this.copackagedIndicator = TypeConvertor.castToBoolean(value); // BooleanType 2660 return value; 2661 case -1969347631: // manufacturer 2662 this.getManufacturer().add(TypeConvertor.castToReference(value)); // Reference 2663 return value; 2664 case -513945889: // attachedDocument 2665 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); // Reference 2666 return value; 2667 case 1802065795: // packaging 2668 this.packaging = (PackagedProductDefinitionPackagingComponent) value; // PackagedProductDefinitionPackagingComponent 2669 return value; 2670 case 366313883: // characteristic 2671 this.getCharacteristic().add((PackagedProductDefinitionPackagingPropertyComponent) value); // PackagedProductDefinitionPackagingPropertyComponent 2672 return value; 2673 default: return super.setProperty(hash, name, value); 2674 } 2675 2676 } 2677 2678 @Override 2679 public Base setProperty(String name, Base value) throws FHIRException { 2680 if (name.equals("identifier")) { 2681 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2682 } else if (name.equals("name")) { 2683 this.name = TypeConvertor.castToString(value); // StringType 2684 } else if (name.equals("type")) { 2685 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2686 } else if (name.equals("packageFor")) { 2687 this.getPackageFor().add(TypeConvertor.castToReference(value)); 2688 } else if (name.equals("status")) { 2689 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2690 } else if (name.equals("statusDate")) { 2691 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 2692 } else if (name.equals("containedItemQuantity")) { 2693 this.getContainedItemQuantity().add(TypeConvertor.castToQuantity(value)); 2694 } else if (name.equals("description")) { 2695 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2696 } else if (name.equals("legalStatusOfSupply")) { 2697 this.getLegalStatusOfSupply().add((PackagedProductDefinitionLegalStatusOfSupplyComponent) value); 2698 } else if (name.equals("marketingStatus")) { 2699 this.getMarketingStatus().add(TypeConvertor.castToMarketingStatus(value)); 2700 } else if (name.equals("copackagedIndicator")) { 2701 this.copackagedIndicator = TypeConvertor.castToBoolean(value); // BooleanType 2702 } else if (name.equals("manufacturer")) { 2703 this.getManufacturer().add(TypeConvertor.castToReference(value)); 2704 } else if (name.equals("attachedDocument")) { 2705 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); 2706 } else if (name.equals("packaging")) { 2707 this.packaging = (PackagedProductDefinitionPackagingComponent) value; // PackagedProductDefinitionPackagingComponent 2708 } else if (name.equals("characteristic")) { 2709 this.getCharacteristic().add((PackagedProductDefinitionPackagingPropertyComponent) value); 2710 } else 2711 return super.setProperty(name, value); 2712 return value; 2713 } 2714 2715 @Override 2716 public void removeChild(String name, Base value) throws FHIRException { 2717 if (name.equals("identifier")) { 2718 this.getIdentifier().remove(value); 2719 } else if (name.equals("name")) { 2720 this.name = null; 2721 } else if (name.equals("type")) { 2722 this.type = null; 2723 } else if (name.equals("packageFor")) { 2724 this.getPackageFor().remove(value); 2725 } else if (name.equals("status")) { 2726 this.status = null; 2727 } else if (name.equals("statusDate")) { 2728 this.statusDate = null; 2729 } else if (name.equals("containedItemQuantity")) { 2730 this.getContainedItemQuantity().remove(value); 2731 } else if (name.equals("description")) { 2732 this.description = null; 2733 } else if (name.equals("legalStatusOfSupply")) { 2734 this.getLegalStatusOfSupply().remove((PackagedProductDefinitionLegalStatusOfSupplyComponent) value); 2735 } else if (name.equals("marketingStatus")) { 2736 this.getMarketingStatus().remove(value); 2737 } else if (name.equals("copackagedIndicator")) { 2738 this.copackagedIndicator = null; 2739 } else if (name.equals("manufacturer")) { 2740 this.getManufacturer().remove(value); 2741 } else if (name.equals("attachedDocument")) { 2742 this.getAttachedDocument().remove(value); 2743 } else if (name.equals("packaging")) { 2744 this.packaging = (PackagedProductDefinitionPackagingComponent) value; // PackagedProductDefinitionPackagingComponent 2745 } else if (name.equals("characteristic")) { 2746 this.getCharacteristic().remove((PackagedProductDefinitionPackagingPropertyComponent) value); 2747 } else 2748 super.removeChild(name, value); 2749 2750 } 2751 2752 @Override 2753 public Base makeProperty(int hash, String name) throws FHIRException { 2754 switch (hash) { 2755 case -1618432855: return addIdentifier(); 2756 case 3373707: return getNameElement(); 2757 case 3575610: return getType(); 2758 case 29307555: return addPackageFor(); 2759 case -892481550: return getStatus(); 2760 case 247524032: return getStatusDateElement(); 2761 case -1686893359: return addContainedItemQuantity(); 2762 case -1724546052: return getDescriptionElement(); 2763 case -844874031: return addLegalStatusOfSupply(); 2764 case 70767032: return addMarketingStatus(); 2765 case -1638663195: return getCopackagedIndicatorElement(); 2766 case -1969347631: return addManufacturer(); 2767 case -513945889: return addAttachedDocument(); 2768 case 1802065795: return getPackaging(); 2769 case 366313883: return addCharacteristic(); 2770 default: return super.makeProperty(hash, name); 2771 } 2772 2773 } 2774 2775 @Override 2776 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2777 switch (hash) { 2778 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2779 case 3373707: /*name*/ return new String[] {"string"}; 2780 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2781 case 29307555: /*packageFor*/ return new String[] {"Reference"}; 2782 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 2783 case 247524032: /*statusDate*/ return new String[] {"dateTime"}; 2784 case -1686893359: /*containedItemQuantity*/ return new String[] {"Quantity"}; 2785 case -1724546052: /*description*/ return new String[] {"markdown"}; 2786 case -844874031: /*legalStatusOfSupply*/ return new String[] {}; 2787 case 70767032: /*marketingStatus*/ return new String[] {"MarketingStatus"}; 2788 case -1638663195: /*copackagedIndicator*/ return new String[] {"boolean"}; 2789 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 2790 case -513945889: /*attachedDocument*/ return new String[] {"Reference"}; 2791 case 1802065795: /*packaging*/ return new String[] {}; 2792 case 366313883: /*characteristic*/ return new String[] {"@PackagedProductDefinition.packaging.property"}; 2793 default: return super.getTypesForProperty(hash, name); 2794 } 2795 2796 } 2797 2798 @Override 2799 public Base addChild(String name) throws FHIRException { 2800 if (name.equals("identifier")) { 2801 return addIdentifier(); 2802 } 2803 else if (name.equals("name")) { 2804 throw new FHIRException("Cannot call addChild on a singleton property PackagedProductDefinition.name"); 2805 } 2806 else if (name.equals("type")) { 2807 this.type = new CodeableConcept(); 2808 return this.type; 2809 } 2810 else if (name.equals("packageFor")) { 2811 return addPackageFor(); 2812 } 2813 else if (name.equals("status")) { 2814 this.status = new CodeableConcept(); 2815 return this.status; 2816 } 2817 else if (name.equals("statusDate")) { 2818 throw new FHIRException("Cannot call addChild on a singleton property PackagedProductDefinition.statusDate"); 2819 } 2820 else if (name.equals("containedItemQuantity")) { 2821 return addContainedItemQuantity(); 2822 } 2823 else if (name.equals("description")) { 2824 throw new FHIRException("Cannot call addChild on a singleton property PackagedProductDefinition.description"); 2825 } 2826 else if (name.equals("legalStatusOfSupply")) { 2827 return addLegalStatusOfSupply(); 2828 } 2829 else if (name.equals("marketingStatus")) { 2830 return addMarketingStatus(); 2831 } 2832 else if (name.equals("copackagedIndicator")) { 2833 throw new FHIRException("Cannot call addChild on a singleton property PackagedProductDefinition.copackagedIndicator"); 2834 } 2835 else if (name.equals("manufacturer")) { 2836 return addManufacturer(); 2837 } 2838 else if (name.equals("attachedDocument")) { 2839 return addAttachedDocument(); 2840 } 2841 else if (name.equals("packaging")) { 2842 this.packaging = new PackagedProductDefinitionPackagingComponent(); 2843 return this.packaging; 2844 } 2845 else if (name.equals("characteristic")) { 2846 return addCharacteristic(); 2847 } 2848 else 2849 return super.addChild(name); 2850 } 2851 2852 public String fhirType() { 2853 return "PackagedProductDefinition"; 2854 2855 } 2856 2857 public PackagedProductDefinition copy() { 2858 PackagedProductDefinition dst = new PackagedProductDefinition(); 2859 copyValues(dst); 2860 return dst; 2861 } 2862 2863 public void copyValues(PackagedProductDefinition dst) { 2864 super.copyValues(dst); 2865 if (identifier != null) { 2866 dst.identifier = new ArrayList<Identifier>(); 2867 for (Identifier i : identifier) 2868 dst.identifier.add(i.copy()); 2869 }; 2870 dst.name = name == null ? null : name.copy(); 2871 dst.type = type == null ? null : type.copy(); 2872 if (packageFor != null) { 2873 dst.packageFor = new ArrayList<Reference>(); 2874 for (Reference i : packageFor) 2875 dst.packageFor.add(i.copy()); 2876 }; 2877 dst.status = status == null ? null : status.copy(); 2878 dst.statusDate = statusDate == null ? null : statusDate.copy(); 2879 if (containedItemQuantity != null) { 2880 dst.containedItemQuantity = new ArrayList<Quantity>(); 2881 for (Quantity i : containedItemQuantity) 2882 dst.containedItemQuantity.add(i.copy()); 2883 }; 2884 dst.description = description == null ? null : description.copy(); 2885 if (legalStatusOfSupply != null) { 2886 dst.legalStatusOfSupply = new ArrayList<PackagedProductDefinitionLegalStatusOfSupplyComponent>(); 2887 for (PackagedProductDefinitionLegalStatusOfSupplyComponent i : legalStatusOfSupply) 2888 dst.legalStatusOfSupply.add(i.copy()); 2889 }; 2890 if (marketingStatus != null) { 2891 dst.marketingStatus = new ArrayList<MarketingStatus>(); 2892 for (MarketingStatus i : marketingStatus) 2893 dst.marketingStatus.add(i.copy()); 2894 }; 2895 dst.copackagedIndicator = copackagedIndicator == null ? null : copackagedIndicator.copy(); 2896 if (manufacturer != null) { 2897 dst.manufacturer = new ArrayList<Reference>(); 2898 for (Reference i : manufacturer) 2899 dst.manufacturer.add(i.copy()); 2900 }; 2901 if (attachedDocument != null) { 2902 dst.attachedDocument = new ArrayList<Reference>(); 2903 for (Reference i : attachedDocument) 2904 dst.attachedDocument.add(i.copy()); 2905 }; 2906 dst.packaging = packaging == null ? null : packaging.copy(); 2907 if (characteristic != null) { 2908 dst.characteristic = new ArrayList<PackagedProductDefinitionPackagingPropertyComponent>(); 2909 for (PackagedProductDefinitionPackagingPropertyComponent i : characteristic) 2910 dst.characteristic.add(i.copy()); 2911 }; 2912 } 2913 2914 protected PackagedProductDefinition typedCopy() { 2915 return copy(); 2916 } 2917 2918 @Override 2919 public boolean equalsDeep(Base other_) { 2920 if (!super.equalsDeep(other_)) 2921 return false; 2922 if (!(other_ instanceof PackagedProductDefinition)) 2923 return false; 2924 PackagedProductDefinition o = (PackagedProductDefinition) other_; 2925 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(type, o.type, true) 2926 && compareDeep(packageFor, o.packageFor, true) && compareDeep(status, o.status, true) && compareDeep(statusDate, o.statusDate, true) 2927 && compareDeep(containedItemQuantity, o.containedItemQuantity, true) && compareDeep(description, o.description, true) 2928 && compareDeep(legalStatusOfSupply, o.legalStatusOfSupply, true) && compareDeep(marketingStatus, o.marketingStatus, true) 2929 && compareDeep(copackagedIndicator, o.copackagedIndicator, true) && compareDeep(manufacturer, o.manufacturer, true) 2930 && compareDeep(attachedDocument, o.attachedDocument, true) && compareDeep(packaging, o.packaging, true) 2931 && compareDeep(characteristic, o.characteristic, true); 2932 } 2933 2934 @Override 2935 public boolean equalsShallow(Base other_) { 2936 if (!super.equalsShallow(other_)) 2937 return false; 2938 if (!(other_ instanceof PackagedProductDefinition)) 2939 return false; 2940 PackagedProductDefinition o = (PackagedProductDefinition) other_; 2941 return compareValues(name, o.name, true) && compareValues(statusDate, o.statusDate, true) && compareValues(description, o.description, true) 2942 && compareValues(copackagedIndicator, o.copackagedIndicator, true); 2943 } 2944 2945 public boolean isEmpty() { 2946 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, type, packageFor 2947 , status, statusDate, containedItemQuantity, description, legalStatusOfSupply, marketingStatus 2948 , copackagedIndicator, manufacturer, attachedDocument, packaging, characteristic); 2949 } 2950 2951 @Override 2952 public ResourceType getResourceType() { 2953 return ResourceType.PackagedProductDefinition; 2954 } 2955 2956 /** 2957 * Search parameter: <b>biological</b> 2958 * <p> 2959 * Description: <b>A biologically derived product within this packaged product</b><br> 2960 * Type: <b>reference</b><br> 2961 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 2962 * </p> 2963 */ 2964 @SearchParamDefinition(name="biological", path="PackagedProductDefinition.packaging.containedItem.item.reference", description="A biologically derived product within this packaged product", type="reference", target={BiologicallyDerivedProduct.class, DeviceDefinition.class, ManufacturedItemDefinition.class, NutritionProduct.class, PackagedProductDefinition.class } ) 2965 public static final String SP_BIOLOGICAL = "biological"; 2966 /** 2967 * <b>Fluent Client</b> search parameter constant for <b>biological</b> 2968 * <p> 2969 * Description: <b>A biologically derived product within this packaged product</b><br> 2970 * Type: <b>reference</b><br> 2971 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 2972 * </p> 2973 */ 2974 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BIOLOGICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BIOLOGICAL); 2975 2976/** 2977 * Constant for fluent queries to be used to add include statements. Specifies 2978 * the path value of "<b>PackagedProductDefinition:biological</b>". 2979 */ 2980 public static final ca.uhn.fhir.model.api.Include INCLUDE_BIOLOGICAL = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:biological").toLocked(); 2981 2982 /** 2983 * Search parameter: <b>contained-item</b> 2984 * <p> 2985 * Description: <b>Any of the contained items within this packaged product</b><br> 2986 * Type: <b>reference</b><br> 2987 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 2988 * </p> 2989 */ 2990 @SearchParamDefinition(name="contained-item", path="PackagedProductDefinition.packaging.containedItem.item.reference", description="Any of the contained items within this packaged product", type="reference", target={BiologicallyDerivedProduct.class, DeviceDefinition.class, ManufacturedItemDefinition.class, NutritionProduct.class, PackagedProductDefinition.class } ) 2991 public static final String SP_CONTAINED_ITEM = "contained-item"; 2992 /** 2993 * <b>Fluent Client</b> search parameter constant for <b>contained-item</b> 2994 * <p> 2995 * Description: <b>Any of the contained items within this packaged product</b><br> 2996 * Type: <b>reference</b><br> 2997 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 2998 * </p> 2999 */ 3000 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTAINED_ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTAINED_ITEM); 3001 3002/** 3003 * Constant for fluent queries to be used to add include statements. Specifies 3004 * the path value of "<b>PackagedProductDefinition:contained-item</b>". 3005 */ 3006 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTAINED_ITEM = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:contained-item").toLocked(); 3007 3008 /** 3009 * Search parameter: <b>device</b> 3010 * <p> 3011 * Description: <b>A device within this packaged product</b><br> 3012 * Type: <b>reference</b><br> 3013 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3014 * </p> 3015 */ 3016 @SearchParamDefinition(name="device", path="PackagedProductDefinition.packaging.containedItem.item.reference", description="A device within this packaged product", type="reference", target={BiologicallyDerivedProduct.class, DeviceDefinition.class, ManufacturedItemDefinition.class, NutritionProduct.class, PackagedProductDefinition.class } ) 3017 public static final String SP_DEVICE = "device"; 3018 /** 3019 * <b>Fluent Client</b> search parameter constant for <b>device</b> 3020 * <p> 3021 * Description: <b>A device within this packaged product</b><br> 3022 * Type: <b>reference</b><br> 3023 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3024 * </p> 3025 */ 3026 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 3027 3028/** 3029 * Constant for fluent queries to be used to add include statements. Specifies 3030 * the path value of "<b>PackagedProductDefinition:device</b>". 3031 */ 3032 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:device").toLocked(); 3033 3034 /** 3035 * Search parameter: <b>identifier</b> 3036 * <p> 3037 * Description: <b>Unique identifier</b><br> 3038 * Type: <b>token</b><br> 3039 * Path: <b>PackagedProductDefinition.identifier</b><br> 3040 * </p> 3041 */ 3042 @SearchParamDefinition(name="identifier", path="PackagedProductDefinition.identifier", description="Unique identifier", type="token" ) 3043 public static final String SP_IDENTIFIER = "identifier"; 3044 /** 3045 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3046 * <p> 3047 * Description: <b>Unique identifier</b><br> 3048 * Type: <b>token</b><br> 3049 * Path: <b>PackagedProductDefinition.identifier</b><br> 3050 * </p> 3051 */ 3052 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3053 3054 /** 3055 * Search parameter: <b>manufactured-item</b> 3056 * <p> 3057 * Description: <b>A manufactured item of medication within this packaged product</b><br> 3058 * Type: <b>reference</b><br> 3059 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3060 * </p> 3061 */ 3062 @SearchParamDefinition(name="manufactured-item", path="PackagedProductDefinition.packaging.containedItem.item.reference", description="A manufactured item of medication within this packaged product", type="reference", target={BiologicallyDerivedProduct.class, DeviceDefinition.class, ManufacturedItemDefinition.class, NutritionProduct.class, PackagedProductDefinition.class } ) 3063 public static final String SP_MANUFACTURED_ITEM = "manufactured-item"; 3064 /** 3065 * <b>Fluent Client</b> search parameter constant for <b>manufactured-item</b> 3066 * <p> 3067 * Description: <b>A manufactured item of medication within this packaged product</b><br> 3068 * Type: <b>reference</b><br> 3069 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3070 * </p> 3071 */ 3072 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURED_ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURED_ITEM); 3073 3074/** 3075 * Constant for fluent queries to be used to add include statements. Specifies 3076 * the path value of "<b>PackagedProductDefinition:manufactured-item</b>". 3077 */ 3078 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURED_ITEM = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:manufactured-item").toLocked(); 3079 3080 /** 3081 * Search parameter: <b>medication</b> 3082 * <p> 3083 * Description: <b>A manufactured item of medication within this packaged product</b><br> 3084 * Type: <b>reference</b><br> 3085 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3086 * </p> 3087 */ 3088 @SearchParamDefinition(name="medication", path="PackagedProductDefinition.packaging.containedItem.item.reference", description="A manufactured item of medication within this packaged product", type="reference", target={BiologicallyDerivedProduct.class, DeviceDefinition.class, ManufacturedItemDefinition.class, NutritionProduct.class, PackagedProductDefinition.class } ) 3089 public static final String SP_MEDICATION = "medication"; 3090 /** 3091 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 3092 * <p> 3093 * Description: <b>A manufactured item of medication within this packaged product</b><br> 3094 * Type: <b>reference</b><br> 3095 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3096 * </p> 3097 */ 3098 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 3099 3100/** 3101 * Constant for fluent queries to be used to add include statements. Specifies 3102 * the path value of "<b>PackagedProductDefinition:medication</b>". 3103 */ 3104 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:medication").toLocked(); 3105 3106 /** 3107 * Search parameter: <b>name</b> 3108 * <p> 3109 * Description: <b>A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc.</b><br> 3110 * Type: <b>token</b><br> 3111 * Path: <b>PackagedProductDefinition.name</b><br> 3112 * </p> 3113 */ 3114 @SearchParamDefinition(name="name", path="PackagedProductDefinition.name", description="A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc.", type="token" ) 3115 public static final String SP_NAME = "name"; 3116 /** 3117 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3118 * <p> 3119 * Description: <b>A name for this package. Typically what it would be listed as in a drug formulary or catalogue, inventory etc.</b><br> 3120 * Type: <b>token</b><br> 3121 * Path: <b>PackagedProductDefinition.name</b><br> 3122 * </p> 3123 */ 3124 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NAME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NAME); 3125 3126 /** 3127 * Search parameter: <b>nutrition</b> 3128 * <p> 3129 * Description: <b>A nutrition product within this packaged product</b><br> 3130 * Type: <b>reference</b><br> 3131 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3132 * </p> 3133 */ 3134 @SearchParamDefinition(name="nutrition", path="PackagedProductDefinition.packaging.containedItem.item.reference", description="A nutrition product within this packaged product", type="reference", target={BiologicallyDerivedProduct.class, DeviceDefinition.class, ManufacturedItemDefinition.class, NutritionProduct.class, PackagedProductDefinition.class } ) 3135 public static final String SP_NUTRITION = "nutrition"; 3136 /** 3137 * <b>Fluent Client</b> search parameter constant for <b>nutrition</b> 3138 * <p> 3139 * Description: <b>A nutrition product within this packaged product</b><br> 3140 * Type: <b>reference</b><br> 3141 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3142 * </p> 3143 */ 3144 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam NUTRITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_NUTRITION); 3145 3146/** 3147 * Constant for fluent queries to be used to add include statements. Specifies 3148 * the path value of "<b>PackagedProductDefinition:nutrition</b>". 3149 */ 3150 public static final ca.uhn.fhir.model.api.Include INCLUDE_NUTRITION = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:nutrition").toLocked(); 3151 3152 /** 3153 * Search parameter: <b>package-for</b> 3154 * <p> 3155 * Description: <b>The product that this is a pack for</b><br> 3156 * Type: <b>reference</b><br> 3157 * Path: <b>PackagedProductDefinition.packageFor</b><br> 3158 * </p> 3159 */ 3160 @SearchParamDefinition(name="package-for", path="PackagedProductDefinition.packageFor", description="The product that this is a pack for", type="reference", target={MedicinalProductDefinition.class } ) 3161 public static final String SP_PACKAGE_FOR = "package-for"; 3162 /** 3163 * <b>Fluent Client</b> search parameter constant for <b>package-for</b> 3164 * <p> 3165 * Description: <b>The product that this is a pack for</b><br> 3166 * Type: <b>reference</b><br> 3167 * Path: <b>PackagedProductDefinition.packageFor</b><br> 3168 * </p> 3169 */ 3170 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PACKAGE_FOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PACKAGE_FOR); 3171 3172/** 3173 * Constant for fluent queries to be used to add include statements. Specifies 3174 * the path value of "<b>PackagedProductDefinition:package-for</b>". 3175 */ 3176 public static final ca.uhn.fhir.model.api.Include INCLUDE_PACKAGE_FOR = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:package-for").toLocked(); 3177 3178 /** 3179 * Search parameter: <b>package</b> 3180 * <p> 3181 * Description: <b>A complete packaged product within this packaged product</b><br> 3182 * Type: <b>reference</b><br> 3183 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3184 * </p> 3185 */ 3186 @SearchParamDefinition(name="package", path="PackagedProductDefinition.packaging.containedItem.item.reference", description="A complete packaged product within this packaged product", type="reference", target={BiologicallyDerivedProduct.class, DeviceDefinition.class, ManufacturedItemDefinition.class, NutritionProduct.class, PackagedProductDefinition.class } ) 3187 public static final String SP_PACKAGE = "package"; 3188 /** 3189 * <b>Fluent Client</b> search parameter constant for <b>package</b> 3190 * <p> 3191 * Description: <b>A complete packaged product within this packaged product</b><br> 3192 * Type: <b>reference</b><br> 3193 * Path: <b>PackagedProductDefinition.packaging.containedItem.item.reference</b><br> 3194 * </p> 3195 */ 3196 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PACKAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PACKAGE); 3197 3198/** 3199 * Constant for fluent queries to be used to add include statements. Specifies 3200 * the path value of "<b>PackagedProductDefinition:package</b>". 3201 */ 3202 public static final ca.uhn.fhir.model.api.Include INCLUDE_PACKAGE = new ca.uhn.fhir.model.api.Include("PackagedProductDefinition:package").toLocked(); 3203 3204 /** 3205 * Search parameter: <b>status</b> 3206 * <p> 3207 * Description: <b>The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status</b><br> 3208 * Type: <b>token</b><br> 3209 * Path: <b>PackagedProductDefinition.status</b><br> 3210 * </p> 3211 */ 3212 @SearchParamDefinition(name="status", path="PackagedProductDefinition.status", description="The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status", type="token" ) 3213 public static final String SP_STATUS = "status"; 3214 /** 3215 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3216 * <p> 3217 * Description: <b>The status within the lifecycle of this item. A high level status, this is not intended to duplicate details carried elsewhere such as legal status, or authorization or marketing status</b><br> 3218 * Type: <b>token</b><br> 3219 * Path: <b>PackagedProductDefinition.status</b><br> 3220 * </p> 3221 */ 3222 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3223 3224 3225} 3226