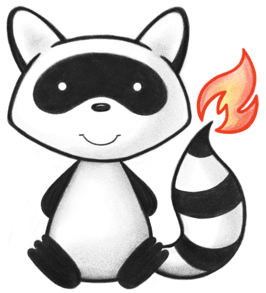
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * ParameterDefinition Type: The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 050 */ 051@DatatypeDef(name="ParameterDefinition") 052public class ParameterDefinition extends DataType implements ICompositeType { 053 054 /** 055 * The name of the parameter used to allow access to the value of the parameter in evaluation contexts. 056 */ 057 @Child(name = "name", type = {CodeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 058 @Description(shortDefinition="Name used to access the parameter value", formalDefinition="The name of the parameter used to allow access to the value of the parameter in evaluation contexts." ) 059 protected CodeType name; 060 061 /** 062 * Whether the parameter is input or output for the module. 063 */ 064 @Child(name = "use", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 065 @Description(shortDefinition="in | out", formalDefinition="Whether the parameter is input or output for the module." ) 066 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-parameter-use") 067 protected Enumeration<OperationParameterUse> use; 068 069 /** 070 * The minimum number of times this parameter SHALL appear in the request or response. 071 */ 072 @Child(name = "min", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 073 @Description(shortDefinition="Minimum cardinality", formalDefinition="The minimum number of times this parameter SHALL appear in the request or response." ) 074 protected IntegerType min; 075 076 /** 077 * The maximum number of times this element is permitted to appear in the request or response. 078 */ 079 @Child(name = "max", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 080 @Description(shortDefinition="Maximum cardinality (a number of *)", formalDefinition="The maximum number of times this element is permitted to appear in the request or response." ) 081 protected StringType max; 082 083 /** 084 * A brief discussion of what the parameter is for and how it is used by the module. 085 */ 086 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 087 @Description(shortDefinition="A brief description of the parameter", formalDefinition="A brief discussion of what the parameter is for and how it is used by the module." ) 088 protected StringType documentation; 089 090 /** 091 * The type of the parameter. 092 */ 093 @Child(name = "type", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 094 @Description(shortDefinition="What type of value", formalDefinition="The type of the parameter." ) 095 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 096 protected Enumeration<FHIRTypes> type; 097 098 /** 099 * If specified, this indicates a profile that the input data must conform to, or that the output data will conform to. 100 */ 101 @Child(name = "profile", type = {CanonicalType.class}, order=6, min=0, max=1, modifier=false, summary=true) 102 @Description(shortDefinition="What profile the value is expected to be", formalDefinition="If specified, this indicates a profile that the input data must conform to, or that the output data will conform to." ) 103 protected CanonicalType profile; 104 105 private static final long serialVersionUID = 2027429213L; 106 107 /** 108 * Constructor 109 */ 110 public ParameterDefinition() { 111 super(); 112 } 113 114 /** 115 * Constructor 116 */ 117 public ParameterDefinition(OperationParameterUse use, FHIRTypes type) { 118 super(); 119 this.setUse(use); 120 this.setType(type); 121 } 122 123 /** 124 * @return {@link #name} (The name of the parameter used to allow access to the value of the parameter in evaluation contexts.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 125 */ 126 public CodeType getNameElement() { 127 if (this.name == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create ParameterDefinition.name"); 130 else if (Configuration.doAutoCreate()) 131 this.name = new CodeType(); // bb 132 return this.name; 133 } 134 135 public boolean hasNameElement() { 136 return this.name != null && !this.name.isEmpty(); 137 } 138 139 public boolean hasName() { 140 return this.name != null && !this.name.isEmpty(); 141 } 142 143 /** 144 * @param value {@link #name} (The name of the parameter used to allow access to the value of the parameter in evaluation contexts.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 145 */ 146 public ParameterDefinition setNameElement(CodeType value) { 147 this.name = value; 148 return this; 149 } 150 151 /** 152 * @return The name of the parameter used to allow access to the value of the parameter in evaluation contexts. 153 */ 154 public String getName() { 155 return this.name == null ? null : this.name.getValue(); 156 } 157 158 /** 159 * @param value The name of the parameter used to allow access to the value of the parameter in evaluation contexts. 160 */ 161 public ParameterDefinition setName(String value) { 162 if (Utilities.noString(value)) 163 this.name = null; 164 else { 165 if (this.name == null) 166 this.name = new CodeType(); 167 this.name.setValue(value); 168 } 169 return this; 170 } 171 172 /** 173 * @return {@link #use} (Whether the parameter is input or output for the module.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 174 */ 175 public Enumeration<OperationParameterUse> getUseElement() { 176 if (this.use == null) 177 if (Configuration.errorOnAutoCreate()) 178 throw new Error("Attempt to auto-create ParameterDefinition.use"); 179 else if (Configuration.doAutoCreate()) 180 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); // bb 181 return this.use; 182 } 183 184 public boolean hasUseElement() { 185 return this.use != null && !this.use.isEmpty(); 186 } 187 188 public boolean hasUse() { 189 return this.use != null && !this.use.isEmpty(); 190 } 191 192 /** 193 * @param value {@link #use} (Whether the parameter is input or output for the module.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 194 */ 195 public ParameterDefinition setUseElement(Enumeration<OperationParameterUse> value) { 196 this.use = value; 197 return this; 198 } 199 200 /** 201 * @return Whether the parameter is input or output for the module. 202 */ 203 public OperationParameterUse getUse() { 204 return this.use == null ? null : this.use.getValue(); 205 } 206 207 /** 208 * @param value Whether the parameter is input or output for the module. 209 */ 210 public ParameterDefinition setUse(OperationParameterUse value) { 211 if (this.use == null) 212 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); 213 this.use.setValue(value); 214 return this; 215 } 216 217 /** 218 * @return {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 219 */ 220 public IntegerType getMinElement() { 221 if (this.min == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create ParameterDefinition.min"); 224 else if (Configuration.doAutoCreate()) 225 this.min = new IntegerType(); // bb 226 return this.min; 227 } 228 229 public boolean hasMinElement() { 230 return this.min != null && !this.min.isEmpty(); 231 } 232 233 public boolean hasMin() { 234 return this.min != null && !this.min.isEmpty(); 235 } 236 237 /** 238 * @param value {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 239 */ 240 public ParameterDefinition setMinElement(IntegerType value) { 241 this.min = value; 242 return this; 243 } 244 245 /** 246 * @return The minimum number of times this parameter SHALL appear in the request or response. 247 */ 248 public int getMin() { 249 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 250 } 251 252 /** 253 * @param value The minimum number of times this parameter SHALL appear in the request or response. 254 */ 255 public ParameterDefinition setMin(int value) { 256 if (this.min == null) 257 this.min = new IntegerType(); 258 this.min.setValue(value); 259 return this; 260 } 261 262 /** 263 * @return {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 264 */ 265 public StringType getMaxElement() { 266 if (this.max == null) 267 if (Configuration.errorOnAutoCreate()) 268 throw new Error("Attempt to auto-create ParameterDefinition.max"); 269 else if (Configuration.doAutoCreate()) 270 this.max = new StringType(); // bb 271 return this.max; 272 } 273 274 public boolean hasMaxElement() { 275 return this.max != null && !this.max.isEmpty(); 276 } 277 278 public boolean hasMax() { 279 return this.max != null && !this.max.isEmpty(); 280 } 281 282 /** 283 * @param value {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 284 */ 285 public ParameterDefinition setMaxElement(StringType value) { 286 this.max = value; 287 return this; 288 } 289 290 /** 291 * @return The maximum number of times this element is permitted to appear in the request or response. 292 */ 293 public String getMax() { 294 return this.max == null ? null : this.max.getValue(); 295 } 296 297 /** 298 * @param value The maximum number of times this element is permitted to appear in the request or response. 299 */ 300 public ParameterDefinition setMax(String value) { 301 if (Utilities.noString(value)) 302 this.max = null; 303 else { 304 if (this.max == null) 305 this.max = new StringType(); 306 this.max.setValue(value); 307 } 308 return this; 309 } 310 311 /** 312 * @return {@link #documentation} (A brief discussion of what the parameter is for and how it is used by the module.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 313 */ 314 public StringType getDocumentationElement() { 315 if (this.documentation == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create ParameterDefinition.documentation"); 318 else if (Configuration.doAutoCreate()) 319 this.documentation = new StringType(); // bb 320 return this.documentation; 321 } 322 323 public boolean hasDocumentationElement() { 324 return this.documentation != null && !this.documentation.isEmpty(); 325 } 326 327 public boolean hasDocumentation() { 328 return this.documentation != null && !this.documentation.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #documentation} (A brief discussion of what the parameter is for and how it is used by the module.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 333 */ 334 public ParameterDefinition setDocumentationElement(StringType value) { 335 this.documentation = value; 336 return this; 337 } 338 339 /** 340 * @return A brief discussion of what the parameter is for and how it is used by the module. 341 */ 342 public String getDocumentation() { 343 return this.documentation == null ? null : this.documentation.getValue(); 344 } 345 346 /** 347 * @param value A brief discussion of what the parameter is for and how it is used by the module. 348 */ 349 public ParameterDefinition setDocumentation(String value) { 350 if (Utilities.noString(value)) 351 this.documentation = null; 352 else { 353 if (this.documentation == null) 354 this.documentation = new StringType(); 355 this.documentation.setValue(value); 356 } 357 return this; 358 } 359 360 /** 361 * @return {@link #type} (The type of the parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 362 */ 363 public Enumeration<FHIRTypes> getTypeElement() { 364 if (this.type == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create ParameterDefinition.type"); 367 else if (Configuration.doAutoCreate()) 368 this.type = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); // bb 369 return this.type; 370 } 371 372 public boolean hasTypeElement() { 373 return this.type != null && !this.type.isEmpty(); 374 } 375 376 public boolean hasType() { 377 return this.type != null && !this.type.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #type} (The type of the parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 382 */ 383 public ParameterDefinition setTypeElement(Enumeration<FHIRTypes> value) { 384 this.type = value; 385 return this; 386 } 387 388 /** 389 * @return The type of the parameter. 390 */ 391 public FHIRTypes getType() { 392 return this.type == null ? null : this.type.getValue(); 393 } 394 395 /** 396 * @param value The type of the parameter. 397 */ 398 public ParameterDefinition setType(FHIRTypes value) { 399 if (this.type == null) 400 this.type = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); 401 this.type.setValue(value); 402 return this; 403 } 404 405 /** 406 * @return {@link #profile} (If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 407 */ 408 public CanonicalType getProfileElement() { 409 if (this.profile == null) 410 if (Configuration.errorOnAutoCreate()) 411 throw new Error("Attempt to auto-create ParameterDefinition.profile"); 412 else if (Configuration.doAutoCreate()) 413 this.profile = new CanonicalType(); // bb 414 return this.profile; 415 } 416 417 public boolean hasProfileElement() { 418 return this.profile != null && !this.profile.isEmpty(); 419 } 420 421 public boolean hasProfile() { 422 return this.profile != null && !this.profile.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #profile} (If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 427 */ 428 public ParameterDefinition setProfileElement(CanonicalType value) { 429 this.profile = value; 430 return this; 431 } 432 433 /** 434 * @return If specified, this indicates a profile that the input data must conform to, or that the output data will conform to. 435 */ 436 public String getProfile() { 437 return this.profile == null ? null : this.profile.getValue(); 438 } 439 440 /** 441 * @param value If specified, this indicates a profile that the input data must conform to, or that the output data will conform to. 442 */ 443 public ParameterDefinition setProfile(String value) { 444 if (Utilities.noString(value)) 445 this.profile = null; 446 else { 447 if (this.profile == null) 448 this.profile = new CanonicalType(); 449 this.profile.setValue(value); 450 } 451 return this; 452 } 453 454 protected void listChildren(List<Property> children) { 455 super.listChildren(children); 456 children.add(new Property("name", "code", "The name of the parameter used to allow access to the value of the parameter in evaluation contexts.", 0, 1, name)); 457 children.add(new Property("use", "code", "Whether the parameter is input or output for the module.", 0, 1, use)); 458 children.add(new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min)); 459 children.add(new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max)); 460 children.add(new Property("documentation", "string", "A brief discussion of what the parameter is for and how it is used by the module.", 0, 1, documentation)); 461 children.add(new Property("type", "code", "The type of the parameter.", 0, 1, type)); 462 children.add(new Property("profile", "canonical(StructureDefinition)", "If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.", 0, 1, profile)); 463 } 464 465 @Override 466 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 467 switch (_hash) { 468 case 3373707: /*name*/ return new Property("name", "code", "The name of the parameter used to allow access to the value of the parameter in evaluation contexts.", 0, 1, name); 469 case 116103: /*use*/ return new Property("use", "code", "Whether the parameter is input or output for the module.", 0, 1, use); 470 case 108114: /*min*/ return new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min); 471 case 107876: /*max*/ return new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max); 472 case 1587405498: /*documentation*/ return new Property("documentation", "string", "A brief discussion of what the parameter is for and how it is used by the module.", 0, 1, documentation); 473 case 3575610: /*type*/ return new Property("type", "code", "The type of the parameter.", 0, 1, type); 474 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.", 0, 1, profile); 475 default: return super.getNamedProperty(_hash, _name, _checkValid); 476 } 477 478 } 479 480 @Override 481 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 482 switch (hash) { 483 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // CodeType 484 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<OperationParameterUse> 485 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 486 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 487 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 488 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<FHIRTypes> 489 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 490 default: return super.getProperty(hash, name, checkValid); 491 } 492 493 } 494 495 @Override 496 public Base setProperty(int hash, String name, Base value) throws FHIRException { 497 switch (hash) { 498 case 3373707: // name 499 this.name = TypeConvertor.castToCode(value); // CodeType 500 return value; 501 case 116103: // use 502 value = new OperationParameterUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 503 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 504 return value; 505 case 108114: // min 506 this.min = TypeConvertor.castToInteger(value); // IntegerType 507 return value; 508 case 107876: // max 509 this.max = TypeConvertor.castToString(value); // StringType 510 return value; 511 case 1587405498: // documentation 512 this.documentation = TypeConvertor.castToString(value); // StringType 513 return value; 514 case 3575610: // type 515 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 516 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 517 return value; 518 case -309425751: // profile 519 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 520 return value; 521 default: return super.setProperty(hash, name, value); 522 } 523 524 } 525 526 @Override 527 public Base setProperty(String name, Base value) throws FHIRException { 528 if (name.equals("name")) { 529 this.name = TypeConvertor.castToCode(value); // CodeType 530 } else if (name.equals("use")) { 531 value = new OperationParameterUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 532 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 533 } else if (name.equals("min")) { 534 this.min = TypeConvertor.castToInteger(value); // IntegerType 535 } else if (name.equals("max")) { 536 this.max = TypeConvertor.castToString(value); // StringType 537 } else if (name.equals("documentation")) { 538 this.documentation = TypeConvertor.castToString(value); // StringType 539 } else if (name.equals("type")) { 540 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 541 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 542 } else if (name.equals("profile")) { 543 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 544 } else 545 return super.setProperty(name, value); 546 return value; 547 } 548 549 @Override 550 public void removeChild(String name, Base value) throws FHIRException { 551 if (name.equals("name")) { 552 this.name = null; 553 } else if (name.equals("use")) { 554 value = new OperationParameterUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 555 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 556 } else if (name.equals("min")) { 557 this.min = null; 558 } else if (name.equals("max")) { 559 this.max = null; 560 } else if (name.equals("documentation")) { 561 this.documentation = null; 562 } else if (name.equals("type")) { 563 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 564 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 565 } else if (name.equals("profile")) { 566 this.profile = null; 567 } else 568 super.removeChild(name, value); 569 570 } 571 572 @Override 573 public Base makeProperty(int hash, String name) throws FHIRException { 574 switch (hash) { 575 case 3373707: return getNameElement(); 576 case 116103: return getUseElement(); 577 case 108114: return getMinElement(); 578 case 107876: return getMaxElement(); 579 case 1587405498: return getDocumentationElement(); 580 case 3575610: return getTypeElement(); 581 case -309425751: return getProfileElement(); 582 default: return super.makeProperty(hash, name); 583 } 584 585 } 586 587 @Override 588 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 589 switch (hash) { 590 case 3373707: /*name*/ return new String[] {"code"}; 591 case 116103: /*use*/ return new String[] {"code"}; 592 case 108114: /*min*/ return new String[] {"integer"}; 593 case 107876: /*max*/ return new String[] {"string"}; 594 case 1587405498: /*documentation*/ return new String[] {"string"}; 595 case 3575610: /*type*/ return new String[] {"code"}; 596 case -309425751: /*profile*/ return new String[] {"canonical"}; 597 default: return super.getTypesForProperty(hash, name); 598 } 599 600 } 601 602 @Override 603 public Base addChild(String name) throws FHIRException { 604 if (name.equals("name")) { 605 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.name"); 606 } 607 else if (name.equals("use")) { 608 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.use"); 609 } 610 else if (name.equals("min")) { 611 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.min"); 612 } 613 else if (name.equals("max")) { 614 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.max"); 615 } 616 else if (name.equals("documentation")) { 617 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.documentation"); 618 } 619 else if (name.equals("type")) { 620 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.type"); 621 } 622 else if (name.equals("profile")) { 623 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.profile"); 624 } 625 else 626 return super.addChild(name); 627 } 628 629 public String fhirType() { 630 return "ParameterDefinition"; 631 632 } 633 634 public ParameterDefinition copy() { 635 ParameterDefinition dst = new ParameterDefinition(); 636 copyValues(dst); 637 return dst; 638 } 639 640 public void copyValues(ParameterDefinition dst) { 641 super.copyValues(dst); 642 dst.name = name == null ? null : name.copy(); 643 dst.use = use == null ? null : use.copy(); 644 dst.min = min == null ? null : min.copy(); 645 dst.max = max == null ? null : max.copy(); 646 dst.documentation = documentation == null ? null : documentation.copy(); 647 dst.type = type == null ? null : type.copy(); 648 dst.profile = profile == null ? null : profile.copy(); 649 } 650 651 protected ParameterDefinition typedCopy() { 652 return copy(); 653 } 654 655 @Override 656 public boolean equalsDeep(Base other_) { 657 if (!super.equalsDeep(other_)) 658 return false; 659 if (!(other_ instanceof ParameterDefinition)) 660 return false; 661 ParameterDefinition o = (ParameterDefinition) other_; 662 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(min, o.min, true) 663 && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) && compareDeep(type, o.type, true) 664 && compareDeep(profile, o.profile, true); 665 } 666 667 @Override 668 public boolean equalsShallow(Base other_) { 669 if (!super.equalsShallow(other_)) 670 return false; 671 if (!(other_ instanceof ParameterDefinition)) 672 return false; 673 ParameterDefinition o = (ParameterDefinition) other_; 674 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(min, o.min, true) 675 && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) && compareValues(type, o.type, true) 676 && compareValues(profile, o.profile, true); 677 } 678 679 public boolean isEmpty() { 680 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, use, min, max, documentation 681 , type, profile); 682 } 683 684 685} 686