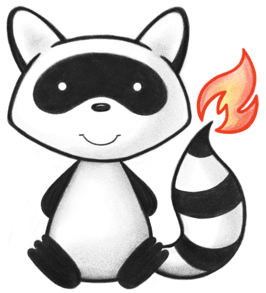
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050import org.hl7.fhir.instance.model.api.IBaseParameters; 051import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 052/** 053 * This resource is used to pass information into and back from an operation (whether invoked directly from REST or within a messaging environment). It is not persisted or allowed to be referenced by other resources except as described in the definition of the Parameters resource. 054 */ 055@ResourceDef(name="Parameters", profile="http://hl7.org/fhir/StructureDefinition/Parameters") 056public class Parameters extends Resource implements IBaseParameters { 057 058 @Block() 059 public static class ParametersParameterComponent extends BackboneElement implements IBaseBackboneElement { 060 /** 061 * The name of the parameter (reference to the operation definition). 062 */ 063 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="Name from the definition", formalDefinition="The name of the parameter (reference to the operation definition)." ) 065 protected StringType name; 066 067 /** 068 * Conveys the content if the parameter is a data type. 069 */ 070 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=2, min=0, max=1, modifier=false, summary=true) 071 @Description(shortDefinition="If parameter is a data type", formalDefinition="Conveys the content if the parameter is a data type." ) 072 protected DataType value; 073 074 /** 075 * Conveys the content if the parameter is a whole resource. 076 */ 077 @Child(name = "resource", type = {Resource.class}, order=3, min=0, max=1, modifier=false, summary=true) 078 @Description(shortDefinition="If parameter is a whole resource", formalDefinition="Conveys the content if the parameter is a whole resource." ) 079 protected Resource resource; 080 081 /** 082 * A named part of a multi-part parameter. 083 */ 084 @Child(name = "part", type = {ParametersParameterComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 085 @Description(shortDefinition="Named part of a multi-part parameter", formalDefinition="A named part of a multi-part parameter." ) 086 protected List<ParametersParameterComponent> part; 087 088 private static final long serialVersionUID = -1755858390L; 089 090 /** 091 * Constructor 092 */ 093 public ParametersParameterComponent() { 094 super(); 095 } 096 097 /** 098 * Constructor 099 */ 100 public ParametersParameterComponent(String name) { 101 super(); 102 this.setName(name); 103 } 104 105 /** 106 * @return {@link #name} (The name of the parameter (reference to the operation definition).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 107 */ 108 public StringType getNameElement() { 109 if (this.name == null) 110 if (Configuration.errorOnAutoCreate()) 111 throw new Error("Attempt to auto-create ParametersParameterComponent.name"); 112 else if (Configuration.doAutoCreate()) 113 this.name = new StringType(); // bb 114 return this.name; 115 } 116 117 public boolean hasNameElement() { 118 return this.name != null && !this.name.isEmpty(); 119 } 120 121 public boolean hasName() { 122 return this.name != null && !this.name.isEmpty(); 123 } 124 125 /** 126 * @param value {@link #name} (The name of the parameter (reference to the operation definition).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 127 */ 128 public ParametersParameterComponent setNameElement(StringType value) { 129 this.name = value; 130 return this; 131 } 132 133 /** 134 * @return The name of the parameter (reference to the operation definition). 135 */ 136 public String getName() { 137 return this.name == null ? null : this.name.getValue(); 138 } 139 140 /** 141 * @param value The name of the parameter (reference to the operation definition). 142 */ 143 public ParametersParameterComponent setName(String value) { 144 if (this.name == null) 145 this.name = new StringType(); 146 this.name.setValue(value); 147 return this; 148 } 149 150 /** 151 * @return {@link #value} (Conveys the content if the parameter is a data type.) 152 */ 153 public DataType getValue() { 154 return this.value; 155 } 156 157 /** 158 * @return {@link #value} (Conveys the content if the parameter is a data type.) 159 */ 160 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 161 if (this.value == null) 162 this.value = new Base64BinaryType(); 163 if (!(this.value instanceof Base64BinaryType)) 164 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 165 return (Base64BinaryType) this.value; 166 } 167 168 public boolean hasValueBase64BinaryType() { 169 return this != null && this.value instanceof Base64BinaryType; 170 } 171 172 /** 173 * @return {@link #value} (Conveys the content if the parameter is a data type.) 174 */ 175 public BooleanType getValueBooleanType() throws FHIRException { 176 if (this.value == null) 177 this.value = new BooleanType(); 178 if (!(this.value instanceof BooleanType)) 179 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 180 return (BooleanType) this.value; 181 } 182 183 public boolean hasValueBooleanType() { 184 return this != null && this.value instanceof BooleanType; 185 } 186 187 /** 188 * @return {@link #value} (Conveys the content if the parameter is a data type.) 189 */ 190 public CanonicalType getValueCanonicalType() throws FHIRException { 191 if (this.value == null) 192 this.value = new CanonicalType(); 193 if (!(this.value instanceof CanonicalType)) 194 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 195 return (CanonicalType) this.value; 196 } 197 198 public boolean hasValueCanonicalType() { 199 return this != null && this.value instanceof CanonicalType; 200 } 201 202 /** 203 * @return {@link #value} (Conveys the content if the parameter is a data type.) 204 */ 205 public CodeType getValueCodeType() throws FHIRException { 206 if (this.value == null) 207 this.value = new CodeType(); 208 if (!(this.value instanceof CodeType)) 209 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 210 return (CodeType) this.value; 211 } 212 213 public boolean hasValueCodeType() { 214 return this != null && this.value instanceof CodeType; 215 } 216 217 /** 218 * @return {@link #value} (Conveys the content if the parameter is a data type.) 219 */ 220 public DateType getValueDateType() throws FHIRException { 221 if (this.value == null) 222 this.value = new DateType(); 223 if (!(this.value instanceof DateType)) 224 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 225 return (DateType) this.value; 226 } 227 228 public boolean hasValueDateType() { 229 return this != null && this.value instanceof DateType; 230 } 231 232 /** 233 * @return {@link #value} (Conveys the content if the parameter is a data type.) 234 */ 235 public DateTimeType getValueDateTimeType() throws FHIRException { 236 if (this.value == null) 237 this.value = new DateTimeType(); 238 if (!(this.value instanceof DateTimeType)) 239 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 240 return (DateTimeType) this.value; 241 } 242 243 public boolean hasValueDateTimeType() { 244 return this != null && this.value instanceof DateTimeType; 245 } 246 247 /** 248 * @return {@link #value} (Conveys the content if the parameter is a data type.) 249 */ 250 public DecimalType getValueDecimalType() throws FHIRException { 251 if (this.value == null) 252 this.value = new DecimalType(); 253 if (!(this.value instanceof DecimalType)) 254 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 255 return (DecimalType) this.value; 256 } 257 258 public boolean hasValueDecimalType() { 259 return this != null && this.value instanceof DecimalType; 260 } 261 262 /** 263 * @return {@link #value} (Conveys the content if the parameter is a data type.) 264 */ 265 public IdType getValueIdType() throws FHIRException { 266 if (this.value == null) 267 this.value = new IdType(); 268 if (!(this.value instanceof IdType)) 269 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 270 return (IdType) this.value; 271 } 272 273 public boolean hasValueIdType() { 274 return this != null && this.value instanceof IdType; 275 } 276 277 /** 278 * @return {@link #value} (Conveys the content if the parameter is a data type.) 279 */ 280 public InstantType getValueInstantType() throws FHIRException { 281 if (this.value == null) 282 this.value = new InstantType(); 283 if (!(this.value instanceof InstantType)) 284 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 285 return (InstantType) this.value; 286 } 287 288 public boolean hasValueInstantType() { 289 return this != null && this.value instanceof InstantType; 290 } 291 292 /** 293 * @return {@link #value} (Conveys the content if the parameter is a data type.) 294 */ 295 public IntegerType getValueIntegerType() throws FHIRException { 296 if (this.value == null) 297 this.value = new IntegerType(); 298 if (!(this.value instanceof IntegerType)) 299 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 300 return (IntegerType) this.value; 301 } 302 303 public boolean hasValueIntegerType() { 304 return this != null && this.value instanceof IntegerType; 305 } 306 307 /** 308 * @return {@link #value} (Conveys the content if the parameter is a data type.) 309 */ 310 public Integer64Type getValueInteger64Type() throws FHIRException { 311 if (this.value == null) 312 this.value = new Integer64Type(); 313 if (!(this.value instanceof Integer64Type)) 314 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 315 return (Integer64Type) this.value; 316 } 317 318 public boolean hasValueInteger64Type() { 319 return this != null && this.value instanceof Integer64Type; 320 } 321 322 /** 323 * @return {@link #value} (Conveys the content if the parameter is a data type.) 324 */ 325 public MarkdownType getValueMarkdownType() throws FHIRException { 326 if (this.value == null) 327 this.value = new MarkdownType(); 328 if (!(this.value instanceof MarkdownType)) 329 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 330 return (MarkdownType) this.value; 331 } 332 333 public boolean hasValueMarkdownType() { 334 return this != null && this.value instanceof MarkdownType; 335 } 336 337 /** 338 * @return {@link #value} (Conveys the content if the parameter is a data type.) 339 */ 340 public OidType getValueOidType() throws FHIRException { 341 if (this.value == null) 342 this.value = new OidType(); 343 if (!(this.value instanceof OidType)) 344 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 345 return (OidType) this.value; 346 } 347 348 public boolean hasValueOidType() { 349 return this != null && this.value instanceof OidType; 350 } 351 352 /** 353 * @return {@link #value} (Conveys the content if the parameter is a data type.) 354 */ 355 public PositiveIntType getValuePositiveIntType() throws FHIRException { 356 if (this.value == null) 357 this.value = new PositiveIntType(); 358 if (!(this.value instanceof PositiveIntType)) 359 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 360 return (PositiveIntType) this.value; 361 } 362 363 public boolean hasValuePositiveIntType() { 364 return this != null && this.value instanceof PositiveIntType; 365 } 366 367 /** 368 * @return {@link #value} (Conveys the content if the parameter is a data type.) 369 */ 370 public StringType getValueStringType() throws FHIRException { 371 if (this.value == null) 372 this.value = new StringType(); 373 if (!(this.value instanceof StringType)) 374 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 375 return (StringType) this.value; 376 } 377 378 public boolean hasValueStringType() { 379 return this != null && this.value instanceof StringType; 380 } 381 382 /** 383 * @return {@link #value} (Conveys the content if the parameter is a data type.) 384 */ 385 public TimeType getValueTimeType() throws FHIRException { 386 if (this.value == null) 387 this.value = new TimeType(); 388 if (!(this.value instanceof TimeType)) 389 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 390 return (TimeType) this.value; 391 } 392 393 public boolean hasValueTimeType() { 394 return this != null && this.value instanceof TimeType; 395 } 396 397 /** 398 * @return {@link #value} (Conveys the content if the parameter is a data type.) 399 */ 400 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 401 if (this.value == null) 402 this.value = new UnsignedIntType(); 403 if (!(this.value instanceof UnsignedIntType)) 404 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 405 return (UnsignedIntType) this.value; 406 } 407 408 public boolean hasValueUnsignedIntType() { 409 return this != null && this.value instanceof UnsignedIntType; 410 } 411 412 /** 413 * @return {@link #value} (Conveys the content if the parameter is a data type.) 414 */ 415 public UriType getValueUriType() throws FHIRException { 416 if (this.value == null) 417 this.value = new UriType(); 418 if (!(this.value instanceof UriType)) 419 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 420 return (UriType) this.value; 421 } 422 423 public boolean hasValueUriType() { 424 return this != null && this.value instanceof UriType; 425 } 426 427 /** 428 * @return {@link #value} (Conveys the content if the parameter is a data type.) 429 */ 430 public UrlType getValueUrlType() throws FHIRException { 431 if (this.value == null) 432 this.value = new UrlType(); 433 if (!(this.value instanceof UrlType)) 434 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 435 return (UrlType) this.value; 436 } 437 438 public boolean hasValueUrlType() { 439 return this != null && this.value instanceof UrlType; 440 } 441 442 /** 443 * @return {@link #value} (Conveys the content if the parameter is a data type.) 444 */ 445 public UuidType getValueUuidType() throws FHIRException { 446 if (this.value == null) 447 this.value = new UuidType(); 448 if (!(this.value instanceof UuidType)) 449 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 450 return (UuidType) this.value; 451 } 452 453 public boolean hasValueUuidType() { 454 return this != null && this.value instanceof UuidType; 455 } 456 457 /** 458 * @return {@link #value} (Conveys the content if the parameter is a data type.) 459 */ 460 public Address getValueAddress() throws FHIRException { 461 if (this.value == null) 462 this.value = new Address(); 463 if (!(this.value instanceof Address)) 464 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 465 return (Address) this.value; 466 } 467 468 public boolean hasValueAddress() { 469 return this != null && this.value instanceof Address; 470 } 471 472 /** 473 * @return {@link #value} (Conveys the content if the parameter is a data type.) 474 */ 475 public Age getValueAge() throws FHIRException { 476 if (this.value == null) 477 this.value = new Age(); 478 if (!(this.value instanceof Age)) 479 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 480 return (Age) this.value; 481 } 482 483 public boolean hasValueAge() { 484 return this != null && this.value instanceof Age; 485 } 486 487 /** 488 * @return {@link #value} (Conveys the content if the parameter is a data type.) 489 */ 490 public Annotation getValueAnnotation() throws FHIRException { 491 if (this.value == null) 492 this.value = new Annotation(); 493 if (!(this.value instanceof Annotation)) 494 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 495 return (Annotation) this.value; 496 } 497 498 public boolean hasValueAnnotation() { 499 return this != null && this.value instanceof Annotation; 500 } 501 502 /** 503 * @return {@link #value} (Conveys the content if the parameter is a data type.) 504 */ 505 public Attachment getValueAttachment() throws FHIRException { 506 if (this.value == null) 507 this.value = new Attachment(); 508 if (!(this.value instanceof Attachment)) 509 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 510 return (Attachment) this.value; 511 } 512 513 public boolean hasValueAttachment() { 514 return this != null && this.value instanceof Attachment; 515 } 516 517 /** 518 * @return {@link #value} (Conveys the content if the parameter is a data type.) 519 */ 520 public CodeableConcept getValueCodeableConcept() throws FHIRException { 521 if (this.value == null) 522 this.value = new CodeableConcept(); 523 if (!(this.value instanceof CodeableConcept)) 524 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 525 return (CodeableConcept) this.value; 526 } 527 528 public boolean hasValueCodeableConcept() { 529 return this != null && this.value instanceof CodeableConcept; 530 } 531 532 /** 533 * @return {@link #value} (Conveys the content if the parameter is a data type.) 534 */ 535 public CodeableReference getValueCodeableReference() throws FHIRException { 536 if (this.value == null) 537 this.value = new CodeableReference(); 538 if (!(this.value instanceof CodeableReference)) 539 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 540 return (CodeableReference) this.value; 541 } 542 543 public boolean hasValueCodeableReference() { 544 return this != null && this.value instanceof CodeableReference; 545 } 546 547 /** 548 * @return {@link #value} (Conveys the content if the parameter is a data type.) 549 */ 550 public Coding getValueCoding() throws FHIRException { 551 if (this.value == null) 552 this.value = new Coding(); 553 if (!(this.value instanceof Coding)) 554 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 555 return (Coding) this.value; 556 } 557 558 public boolean hasValueCoding() { 559 return this != null && this.value instanceof Coding; 560 } 561 562 /** 563 * @return {@link #value} (Conveys the content if the parameter is a data type.) 564 */ 565 public ContactPoint getValueContactPoint() throws FHIRException { 566 if (this.value == null) 567 this.value = new ContactPoint(); 568 if (!(this.value instanceof ContactPoint)) 569 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 570 return (ContactPoint) this.value; 571 } 572 573 public boolean hasValueContactPoint() { 574 return this != null && this.value instanceof ContactPoint; 575 } 576 577 /** 578 * @return {@link #value} (Conveys the content if the parameter is a data type.) 579 */ 580 public Count getValueCount() throws FHIRException { 581 if (this.value == null) 582 this.value = new Count(); 583 if (!(this.value instanceof Count)) 584 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 585 return (Count) this.value; 586 } 587 588 public boolean hasValueCount() { 589 return this != null && this.value instanceof Count; 590 } 591 592 /** 593 * @return {@link #value} (Conveys the content if the parameter is a data type.) 594 */ 595 public Distance getValueDistance() throws FHIRException { 596 if (this.value == null) 597 this.value = new Distance(); 598 if (!(this.value instanceof Distance)) 599 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 600 return (Distance) this.value; 601 } 602 603 public boolean hasValueDistance() { 604 return this != null && this.value instanceof Distance; 605 } 606 607 /** 608 * @return {@link #value} (Conveys the content if the parameter is a data type.) 609 */ 610 public Duration getValueDuration() throws FHIRException { 611 if (this.value == null) 612 this.value = new Duration(); 613 if (!(this.value instanceof Duration)) 614 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 615 return (Duration) this.value; 616 } 617 618 public boolean hasValueDuration() { 619 return this != null && this.value instanceof Duration; 620 } 621 622 /** 623 * @return {@link #value} (Conveys the content if the parameter is a data type.) 624 */ 625 public HumanName getValueHumanName() throws FHIRException { 626 if (this.value == null) 627 this.value = new HumanName(); 628 if (!(this.value instanceof HumanName)) 629 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 630 return (HumanName) this.value; 631 } 632 633 public boolean hasValueHumanName() { 634 return this != null && this.value instanceof HumanName; 635 } 636 637 /** 638 * @return {@link #value} (Conveys the content if the parameter is a data type.) 639 */ 640 public Identifier getValueIdentifier() throws FHIRException { 641 if (this.value == null) 642 this.value = new Identifier(); 643 if (!(this.value instanceof Identifier)) 644 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 645 return (Identifier) this.value; 646 } 647 648 public boolean hasValueIdentifier() { 649 return this != null && this.value instanceof Identifier; 650 } 651 652 /** 653 * @return {@link #value} (Conveys the content if the parameter is a data type.) 654 */ 655 public Money getValueMoney() throws FHIRException { 656 if (this.value == null) 657 this.value = new Money(); 658 if (!(this.value instanceof Money)) 659 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 660 return (Money) this.value; 661 } 662 663 public boolean hasValueMoney() { 664 return this != null && this.value instanceof Money; 665 } 666 667 /** 668 * @return {@link #value} (Conveys the content if the parameter is a data type.) 669 */ 670 public Period getValuePeriod() throws FHIRException { 671 if (this.value == null) 672 this.value = new Period(); 673 if (!(this.value instanceof Period)) 674 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 675 return (Period) this.value; 676 } 677 678 public boolean hasValuePeriod() { 679 return this != null && this.value instanceof Period; 680 } 681 682 /** 683 * @return {@link #value} (Conveys the content if the parameter is a data type.) 684 */ 685 public Quantity getValueQuantity() throws FHIRException { 686 if (this.value == null) 687 this.value = new Quantity(); 688 if (!(this.value instanceof Quantity)) 689 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 690 return (Quantity) this.value; 691 } 692 693 public boolean hasValueQuantity() { 694 return this != null && this.value instanceof Quantity; 695 } 696 697 /** 698 * @return {@link #value} (Conveys the content if the parameter is a data type.) 699 */ 700 public Range getValueRange() throws FHIRException { 701 if (this.value == null) 702 this.value = new Range(); 703 if (!(this.value instanceof Range)) 704 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 705 return (Range) this.value; 706 } 707 708 public boolean hasValueRange() { 709 return this != null && this.value instanceof Range; 710 } 711 712 /** 713 * @return {@link #value} (Conveys the content if the parameter is a data type.) 714 */ 715 public Ratio getValueRatio() throws FHIRException { 716 if (this.value == null) 717 this.value = new Ratio(); 718 if (!(this.value instanceof Ratio)) 719 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 720 return (Ratio) this.value; 721 } 722 723 public boolean hasValueRatio() { 724 return this != null && this.value instanceof Ratio; 725 } 726 727 /** 728 * @return {@link #value} (Conveys the content if the parameter is a data type.) 729 */ 730 public RatioRange getValueRatioRange() throws FHIRException { 731 if (this.value == null) 732 this.value = new RatioRange(); 733 if (!(this.value instanceof RatioRange)) 734 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 735 return (RatioRange) this.value; 736 } 737 738 public boolean hasValueRatioRange() { 739 return this != null && this.value instanceof RatioRange; 740 } 741 742 /** 743 * @return {@link #value} (Conveys the content if the parameter is a data type.) 744 */ 745 public Reference getValueReference() throws FHIRException { 746 if (this.value == null) 747 this.value = new Reference(); 748 if (!(this.value instanceof Reference)) 749 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 750 return (Reference) this.value; 751 } 752 753 public boolean hasValueReference() { 754 return this != null && this.value instanceof Reference; 755 } 756 757 /** 758 * @return {@link #value} (Conveys the content if the parameter is a data type.) 759 */ 760 public SampledData getValueSampledData() throws FHIRException { 761 if (this.value == null) 762 this.value = new SampledData(); 763 if (!(this.value instanceof SampledData)) 764 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 765 return (SampledData) this.value; 766 } 767 768 public boolean hasValueSampledData() { 769 return this != null && this.value instanceof SampledData; 770 } 771 772 /** 773 * @return {@link #value} (Conveys the content if the parameter is a data type.) 774 */ 775 public Signature getValueSignature() throws FHIRException { 776 if (this.value == null) 777 this.value = new Signature(); 778 if (!(this.value instanceof Signature)) 779 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 780 return (Signature) this.value; 781 } 782 783 public boolean hasValueSignature() { 784 return this != null && this.value instanceof Signature; 785 } 786 787 /** 788 * @return {@link #value} (Conveys the content if the parameter is a data type.) 789 */ 790 public Timing getValueTiming() throws FHIRException { 791 if (this.value == null) 792 this.value = new Timing(); 793 if (!(this.value instanceof Timing)) 794 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 795 return (Timing) this.value; 796 } 797 798 public boolean hasValueTiming() { 799 return this != null && this.value instanceof Timing; 800 } 801 802 /** 803 * @return {@link #value} (Conveys the content if the parameter is a data type.) 804 */ 805 public ContactDetail getValueContactDetail() throws FHIRException { 806 if (this.value == null) 807 this.value = new ContactDetail(); 808 if (!(this.value instanceof ContactDetail)) 809 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 810 return (ContactDetail) this.value; 811 } 812 813 public boolean hasValueContactDetail() { 814 return this != null && this.value instanceof ContactDetail; 815 } 816 817 /** 818 * @return {@link #value} (Conveys the content if the parameter is a data type.) 819 */ 820 public DataRequirement getValueDataRequirement() throws FHIRException { 821 if (this.value == null) 822 this.value = new DataRequirement(); 823 if (!(this.value instanceof DataRequirement)) 824 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 825 return (DataRequirement) this.value; 826 } 827 828 public boolean hasValueDataRequirement() { 829 return this != null && this.value instanceof DataRequirement; 830 } 831 832 /** 833 * @return {@link #value} (Conveys the content if the parameter is a data type.) 834 */ 835 public Expression getValueExpression() throws FHIRException { 836 if (this.value == null) 837 this.value = new Expression(); 838 if (!(this.value instanceof Expression)) 839 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 840 return (Expression) this.value; 841 } 842 843 public boolean hasValueExpression() { 844 return this != null && this.value instanceof Expression; 845 } 846 847 /** 848 * @return {@link #value} (Conveys the content if the parameter is a data type.) 849 */ 850 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 851 if (this.value == null) 852 this.value = new ParameterDefinition(); 853 if (!(this.value instanceof ParameterDefinition)) 854 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 855 return (ParameterDefinition) this.value; 856 } 857 858 public boolean hasValueParameterDefinition() { 859 return this != null && this.value instanceof ParameterDefinition; 860 } 861 862 /** 863 * @return {@link #value} (Conveys the content if the parameter is a data type.) 864 */ 865 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 866 if (this.value == null) 867 this.value = new RelatedArtifact(); 868 if (!(this.value instanceof RelatedArtifact)) 869 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 870 return (RelatedArtifact) this.value; 871 } 872 873 public boolean hasValueRelatedArtifact() { 874 return this != null && this.value instanceof RelatedArtifact; 875 } 876 877 /** 878 * @return {@link #value} (Conveys the content if the parameter is a data type.) 879 */ 880 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 881 if (this.value == null) 882 this.value = new TriggerDefinition(); 883 if (!(this.value instanceof TriggerDefinition)) 884 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 885 return (TriggerDefinition) this.value; 886 } 887 888 public boolean hasValueTriggerDefinition() { 889 return this != null && this.value instanceof TriggerDefinition; 890 } 891 892 /** 893 * @return {@link #value} (Conveys the content if the parameter is a data type.) 894 */ 895 public UsageContext getValueUsageContext() throws FHIRException { 896 if (this.value == null) 897 this.value = new UsageContext(); 898 if (!(this.value instanceof UsageContext)) 899 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 900 return (UsageContext) this.value; 901 } 902 903 public boolean hasValueUsageContext() { 904 return this != null && this.value instanceof UsageContext; 905 } 906 907 /** 908 * @return {@link #value} (Conveys the content if the parameter is a data type.) 909 */ 910 public Availability getValueAvailability() throws FHIRException { 911 if (this.value == null) 912 this.value = new Availability(); 913 if (!(this.value instanceof Availability)) 914 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 915 return (Availability) this.value; 916 } 917 918 public boolean hasValueAvailability() { 919 return this != null && this.value instanceof Availability; 920 } 921 922 /** 923 * @return {@link #value} (Conveys the content if the parameter is a data type.) 924 */ 925 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 926 if (this.value == null) 927 this.value = new ExtendedContactDetail(); 928 if (!(this.value instanceof ExtendedContactDetail)) 929 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 930 return (ExtendedContactDetail) this.value; 931 } 932 933 public boolean hasValueExtendedContactDetail() { 934 return this != null && this.value instanceof ExtendedContactDetail; 935 } 936 937 /** 938 * @return {@link #value} (Conveys the content if the parameter is a data type.) 939 */ 940 public Dosage getValueDosage() throws FHIRException { 941 if (this.value == null) 942 this.value = new Dosage(); 943 if (!(this.value instanceof Dosage)) 944 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 945 return (Dosage) this.value; 946 } 947 948 public boolean hasValueDosage() { 949 return this != null && this.value instanceof Dosage; 950 } 951 952 /** 953 * @return {@link #value} (Conveys the content if the parameter is a data type.) 954 */ 955 public Meta getValueMeta() throws FHIRException { 956 if (this.value == null) 957 this.value = new Meta(); 958 if (!(this.value instanceof Meta)) 959 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 960 return (Meta) this.value; 961 } 962 963 public boolean hasValueMeta() { 964 return this != null && this.value instanceof Meta; 965 } 966 967 public boolean hasValue() { 968 return this.value != null && !this.value.isEmpty(); 969 } 970 971 /** 972 * @param value {@link #value} (Conveys the content if the parameter is a data type.) 973 */ 974 public ParametersParameterComponent setValue(DataType value) { 975 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 976 throw new FHIRException("Not the right type for Parameters.parameter.value[x]: "+value.fhirType()); 977 this.value = value; 978 return this; 979 } 980 981 /** 982 * @return {@link #resource} (Conveys the content if the parameter is a whole resource.) 983 */ 984 public Resource getResource() { 985 return this.resource; 986 } 987 988 public boolean hasResource() { 989 return this.resource != null && !this.resource.isEmpty(); 990 } 991 992 /** 993 * @param value {@link #resource} (Conveys the content if the parameter is a whole resource.) 994 */ 995 public ParametersParameterComponent setResource(Resource value) { 996 this.resource = value; 997 return this; 998 } 999 1000 /** 1001 * @return {@link #part} (A named part of a multi-part parameter.) 1002 */ 1003 public List<ParametersParameterComponent> getPart() { 1004 if (this.part == null) 1005 this.part = new ArrayList<ParametersParameterComponent>(); 1006 return this.part; 1007 } 1008 1009 /** 1010 * @return Returns a reference to <code>this</code> for easy method chaining 1011 */ 1012 public ParametersParameterComponent setPart(List<ParametersParameterComponent> thePart) { 1013 this.part = thePart; 1014 return this; 1015 } 1016 1017 public boolean hasPart() { 1018 if (this.part == null) 1019 return false; 1020 for (ParametersParameterComponent item : this.part) 1021 if (!item.isEmpty()) 1022 return true; 1023 return false; 1024 } 1025 1026 public ParametersParameterComponent addPart() { //3 1027 ParametersParameterComponent t = new ParametersParameterComponent(); 1028 if (this.part == null) 1029 this.part = new ArrayList<ParametersParameterComponent>(); 1030 this.part.add(t); 1031 return t; 1032 } 1033 1034 public ParametersParameterComponent addPart(ParametersParameterComponent t) { //3 1035 if (t == null) 1036 return this; 1037 if (this.part == null) 1038 this.part = new ArrayList<ParametersParameterComponent>(); 1039 this.part.add(t); 1040 return this; 1041 } 1042 1043 /** 1044 * @return The first repetition of repeating field {@link #part}, creating it if it does not already exist {3} 1045 */ 1046 public ParametersParameterComponent getPartFirstRep() { 1047 if (getPart().isEmpty()) { 1048 addPart(); 1049 } 1050 return getPart().get(0); 1051 } 1052 1053 protected void listChildren(List<Property> children) { 1054 super.listChildren(children); 1055 children.add(new Property("name", "string", "The name of the parameter (reference to the operation definition).", 0, 1, name)); 1056 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Conveys the content if the parameter is a data type.", 0, 1, value)); 1057 children.add(new Property("resource", "Resource", "Conveys the content if the parameter is a whole resource.", 0, 1, resource)); 1058 children.add(new Property("part", "@Parameters.parameter", "A named part of a multi-part parameter.", 0, java.lang.Integer.MAX_VALUE, part)); 1059 } 1060 1061 @Override 1062 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1063 switch (_hash) { 1064 case 3373707: /*name*/ return new Property("name", "string", "The name of the parameter (reference to the operation definition).", 0, 1, name); 1065 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Conveys the content if the parameter is a data type.", 0, 1, value); 1066 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Conveys the content if the parameter is a data type.", 0, 1, value); 1067 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "Conveys the content if the parameter is a data type.", 0, 1, value); 1068 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Conveys the content if the parameter is a data type.", 0, 1, value); 1069 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "Conveys the content if the parameter is a data type.", 0, 1, value); 1070 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "Conveys the content if the parameter is a data type.", 0, 1, value); 1071 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "Conveys the content if the parameter is a data type.", 0, 1, value); 1072 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "Conveys the content if the parameter is a data type.", 0, 1, value); 1073 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "Conveys the content if the parameter is a data type.", 0, 1, value); 1074 case 231604844: /*valueId*/ return new Property("value[x]", "id", "Conveys the content if the parameter is a data type.", 0, 1, value); 1075 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "Conveys the content if the parameter is a data type.", 0, 1, value); 1076 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "Conveys the content if the parameter is a data type.", 0, 1, value); 1077 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "Conveys the content if the parameter is a data type.", 0, 1, value); 1078 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "Conveys the content if the parameter is a data type.", 0, 1, value); 1079 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "Conveys the content if the parameter is a data type.", 0, 1, value); 1080 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "Conveys the content if the parameter is a data type.", 0, 1, value); 1081 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Conveys the content if the parameter is a data type.", 0, 1, value); 1082 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "Conveys the content if the parameter is a data type.", 0, 1, value); 1083 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "Conveys the content if the parameter is a data type.", 0, 1, value); 1084 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "Conveys the content if the parameter is a data type.", 0, 1, value); 1085 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "Conveys the content if the parameter is a data type.", 0, 1, value); 1086 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "Conveys the content if the parameter is a data type.", 0, 1, value); 1087 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "Conveys the content if the parameter is a data type.", 0, 1, value); 1088 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "Conveys the content if the parameter is a data type.", 0, 1, value); 1089 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "Conveys the content if the parameter is a data type.", 0, 1, value); 1090 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Conveys the content if the parameter is a data type.", 0, 1, value); 1091 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Conveys the content if the parameter is a data type.", 0, 1, value); 1092 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "Conveys the content if the parameter is a data type.", 0, 1, value); 1093 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "Conveys the content if the parameter is a data type.", 0, 1, value); 1094 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "Conveys the content if the parameter is a data type.", 0, 1, value); 1095 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "Conveys the content if the parameter is a data type.", 0, 1, value); 1096 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "Conveys the content if the parameter is a data type.", 0, 1, value); 1097 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "Conveys the content if the parameter is a data type.", 0, 1, value); 1098 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "Conveys the content if the parameter is a data type.", 0, 1, value); 1099 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "Conveys the content if the parameter is a data type.", 0, 1, value); 1100 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "Conveys the content if the parameter is a data type.", 0, 1, value); 1101 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "Conveys the content if the parameter is a data type.", 0, 1, value); 1102 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Conveys the content if the parameter is a data type.", 0, 1, value); 1103 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Conveys the content if the parameter is a data type.", 0, 1, value); 1104 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "Conveys the content if the parameter is a data type.", 0, 1, value); 1105 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "Conveys the content if the parameter is a data type.", 0, 1, value); 1106 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "Conveys the content if the parameter is a data type.", 0, 1, value); 1107 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "Conveys the content if the parameter is a data type.", 0, 1, value); 1108 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "Conveys the content if the parameter is a data type.", 0, 1, value); 1109 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "Conveys the content if the parameter is a data type.", 0, 1, value); 1110 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "Conveys the content if the parameter is a data type.", 0, 1, value); 1111 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "Conveys the content if the parameter is a data type.", 0, 1, value); 1112 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "Conveys the content if the parameter is a data type.", 0, 1, value); 1113 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "Conveys the content if the parameter is a data type.", 0, 1, value); 1114 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "Conveys the content if the parameter is a data type.", 0, 1, value); 1115 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "Conveys the content if the parameter is a data type.", 0, 1, value); 1116 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "Conveys the content if the parameter is a data type.", 0, 1, value); 1117 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "Conveys the content if the parameter is a data type.", 0, 1, value); 1118 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "Conveys the content if the parameter is a data type.", 0, 1, value); 1119 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "Conveys the content if the parameter is a data type.", 0, 1, value); 1120 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "Conveys the content if the parameter is a data type.", 0, 1, value); 1121 case -341064690: /*resource*/ return new Property("resource", "Resource", "Conveys the content if the parameter is a whole resource.", 0, 1, resource); 1122 case 3433459: /*part*/ return new Property("part", "@Parameters.parameter", "A named part of a multi-part parameter.", 0, java.lang.Integer.MAX_VALUE, part); 1123 default: return super.getNamedProperty(_hash, _name, _checkValid); 1124 } 1125 1126 } 1127 1128 @Override 1129 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1130 switch (hash) { 1131 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1132 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1133 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Resource 1134 case 3433459: /*part*/ return this.part == null ? new Base[0] : this.part.toArray(new Base[this.part.size()]); // ParametersParameterComponent 1135 default: return super.getProperty(hash, name, checkValid); 1136 } 1137 1138 } 1139 1140 @Override 1141 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1142 switch (hash) { 1143 case 3373707: // name 1144 this.name = TypeConvertor.castToString(value); // StringType 1145 return value; 1146 case 111972721: // value 1147 this.value = TypeConvertor.castToType(value); // DataType 1148 return value; 1149 case -341064690: // resource 1150 this.resource = TypeConvertor.castToResource(value); // Resource 1151 return value; 1152 case 3433459: // part 1153 this.getPart().add((ParametersParameterComponent) value); // ParametersParameterComponent 1154 return value; 1155 default: return super.setProperty(hash, name, value); 1156 } 1157 1158 } 1159 1160 @Override 1161 public Base setProperty(String name, Base value) throws FHIRException { 1162 if (name.equals("name")) { 1163 this.name = TypeConvertor.castToString(value); // StringType 1164 } else if (name.equals("value[x]")) { 1165 this.value = TypeConvertor.castToType(value); // DataType 1166 } else if (name.equals("resource")) { 1167 this.resource = TypeConvertor.castToResource(value); // Resource 1168 } else if (name.equals("part")) { 1169 this.getPart().add((ParametersParameterComponent) value); 1170 } else 1171 return super.setProperty(name, value); 1172 return value; 1173 } 1174 1175 @Override 1176 public void removeChild(String name, Base value) throws FHIRException { 1177 if (name.equals("name")) { 1178 this.name = null; 1179 } else if (name.equals("value[x]")) { 1180 this.value = null; 1181 } else if (name.equals("resource")) { 1182 this.resource = null; 1183 } else if (name.equals("part")) { 1184 this.getPart().remove((ParametersParameterComponent) value); 1185 } else 1186 super.removeChild(name, value); 1187 1188 } 1189 1190 @Override 1191 public Base makeProperty(int hash, String name) throws FHIRException { 1192 switch (hash) { 1193 case 3373707: return getNameElement(); 1194 case -1410166417: return getValue(); 1195 case 111972721: return getValue(); 1196 case -341064690: throw new FHIRException("Cannot make property resource as it is not a complex type"); // Resource 1197 case 3433459: return addPart(); 1198 default: return super.makeProperty(hash, name); 1199 } 1200 1201 } 1202 1203 @Override 1204 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1205 switch (hash) { 1206 case 3373707: /*name*/ return new String[] {"string"}; 1207 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 1208 case -341064690: /*resource*/ return new String[] {"Resource"}; 1209 case 3433459: /*part*/ return new String[] {"@Parameters.parameter"}; 1210 default: return super.getTypesForProperty(hash, name); 1211 } 1212 1213 } 1214 1215 @Override 1216 public Base addChild(String name) throws FHIRException { 1217 if (name.equals("name")) { 1218 throw new FHIRException("Cannot call addChild on a singleton property Parameters.parameter.name"); 1219 } 1220 else if (name.equals("valueBase64Binary")) { 1221 this.value = new Base64BinaryType(); 1222 return this.value; 1223 } 1224 else if (name.equals("valueBoolean")) { 1225 this.value = new BooleanType(); 1226 return this.value; 1227 } 1228 else if (name.equals("valueCanonical")) { 1229 this.value = new CanonicalType(); 1230 return this.value; 1231 } 1232 else if (name.equals("valueCode")) { 1233 this.value = new CodeType(); 1234 return this.value; 1235 } 1236 else if (name.equals("valueDate")) { 1237 this.value = new DateType(); 1238 return this.value; 1239 } 1240 else if (name.equals("valueDateTime")) { 1241 this.value = new DateTimeType(); 1242 return this.value; 1243 } 1244 else if (name.equals("valueDecimal")) { 1245 this.value = new DecimalType(); 1246 return this.value; 1247 } 1248 else if (name.equals("valueId")) { 1249 this.value = new IdType(); 1250 return this.value; 1251 } 1252 else if (name.equals("valueInstant")) { 1253 this.value = new InstantType(); 1254 return this.value; 1255 } 1256 else if (name.equals("valueInteger")) { 1257 this.value = new IntegerType(); 1258 return this.value; 1259 } 1260 else if (name.equals("valueInteger64")) { 1261 this.value = new Integer64Type(); 1262 return this.value; 1263 } 1264 else if (name.equals("valueMarkdown")) { 1265 this.value = new MarkdownType(); 1266 return this.value; 1267 } 1268 else if (name.equals("valueOid")) { 1269 this.value = new OidType(); 1270 return this.value; 1271 } 1272 else if (name.equals("valuePositiveInt")) { 1273 this.value = new PositiveIntType(); 1274 return this.value; 1275 } 1276 else if (name.equals("valueString")) { 1277 this.value = new StringType(); 1278 return this.value; 1279 } 1280 else if (name.equals("valueTime")) { 1281 this.value = new TimeType(); 1282 return this.value; 1283 } 1284 else if (name.equals("valueUnsignedInt")) { 1285 this.value = new UnsignedIntType(); 1286 return this.value; 1287 } 1288 else if (name.equals("valueUri")) { 1289 this.value = new UriType(); 1290 return this.value; 1291 } 1292 else if (name.equals("valueUrl")) { 1293 this.value = new UrlType(); 1294 return this.value; 1295 } 1296 else if (name.equals("valueUuid")) { 1297 this.value = new UuidType(); 1298 return this.value; 1299 } 1300 else if (name.equals("valueAddress")) { 1301 this.value = new Address(); 1302 return this.value; 1303 } 1304 else if (name.equals("valueAge")) { 1305 this.value = new Age(); 1306 return this.value; 1307 } 1308 else if (name.equals("valueAnnotation")) { 1309 this.value = new Annotation(); 1310 return this.value; 1311 } 1312 else if (name.equals("valueAttachment")) { 1313 this.value = new Attachment(); 1314 return this.value; 1315 } 1316 else if (name.equals("valueCodeableConcept")) { 1317 this.value = new CodeableConcept(); 1318 return this.value; 1319 } 1320 else if (name.equals("valueCodeableReference")) { 1321 this.value = new CodeableReference(); 1322 return this.value; 1323 } 1324 else if (name.equals("valueCoding")) { 1325 this.value = new Coding(); 1326 return this.value; 1327 } 1328 else if (name.equals("valueContactPoint")) { 1329 this.value = new ContactPoint(); 1330 return this.value; 1331 } 1332 else if (name.equals("valueCount")) { 1333 this.value = new Count(); 1334 return this.value; 1335 } 1336 else if (name.equals("valueDistance")) { 1337 this.value = new Distance(); 1338 return this.value; 1339 } 1340 else if (name.equals("valueDuration")) { 1341 this.value = new Duration(); 1342 return this.value; 1343 } 1344 else if (name.equals("valueHumanName")) { 1345 this.value = new HumanName(); 1346 return this.value; 1347 } 1348 else if (name.equals("valueIdentifier")) { 1349 this.value = new Identifier(); 1350 return this.value; 1351 } 1352 else if (name.equals("valueMoney")) { 1353 this.value = new Money(); 1354 return this.value; 1355 } 1356 else if (name.equals("valuePeriod")) { 1357 this.value = new Period(); 1358 return this.value; 1359 } 1360 else if (name.equals("valueQuantity")) { 1361 this.value = new Quantity(); 1362 return this.value; 1363 } 1364 else if (name.equals("valueRange")) { 1365 this.value = new Range(); 1366 return this.value; 1367 } 1368 else if (name.equals("valueRatio")) { 1369 this.value = new Ratio(); 1370 return this.value; 1371 } 1372 else if (name.equals("valueRatioRange")) { 1373 this.value = new RatioRange(); 1374 return this.value; 1375 } 1376 else if (name.equals("valueReference")) { 1377 this.value = new Reference(); 1378 return this.value; 1379 } 1380 else if (name.equals("valueSampledData")) { 1381 this.value = new SampledData(); 1382 return this.value; 1383 } 1384 else if (name.equals("valueSignature")) { 1385 this.value = new Signature(); 1386 return this.value; 1387 } 1388 else if (name.equals("valueTiming")) { 1389 this.value = new Timing(); 1390 return this.value; 1391 } 1392 else if (name.equals("valueContactDetail")) { 1393 this.value = new ContactDetail(); 1394 return this.value; 1395 } 1396 else if (name.equals("valueDataRequirement")) { 1397 this.value = new DataRequirement(); 1398 return this.value; 1399 } 1400 else if (name.equals("valueExpression")) { 1401 this.value = new Expression(); 1402 return this.value; 1403 } 1404 else if (name.equals("valueParameterDefinition")) { 1405 this.value = new ParameterDefinition(); 1406 return this.value; 1407 } 1408 else if (name.equals("valueRelatedArtifact")) { 1409 this.value = new RelatedArtifact(); 1410 return this.value; 1411 } 1412 else if (name.equals("valueTriggerDefinition")) { 1413 this.value = new TriggerDefinition(); 1414 return this.value; 1415 } 1416 else if (name.equals("valueUsageContext")) { 1417 this.value = new UsageContext(); 1418 return this.value; 1419 } 1420 else if (name.equals("valueAvailability")) { 1421 this.value = new Availability(); 1422 return this.value; 1423 } 1424 else if (name.equals("valueExtendedContactDetail")) { 1425 this.value = new ExtendedContactDetail(); 1426 return this.value; 1427 } 1428 else if (name.equals("valueDosage")) { 1429 this.value = new Dosage(); 1430 return this.value; 1431 } 1432 else if (name.equals("valueMeta")) { 1433 this.value = new Meta(); 1434 return this.value; 1435 } 1436 else if (name.equals("resource")) { 1437 throw new FHIRException("Cannot call addChild on an abstract type Parameters.parameter.resource"); 1438 } 1439 else if (name.equals("part")) { 1440 return addPart(); 1441 } 1442 else 1443 return super.addChild(name); 1444 } 1445 1446 public ParametersParameterComponent copy() { 1447 ParametersParameterComponent dst = new ParametersParameterComponent(); 1448 copyValues(dst); 1449 return dst; 1450 } 1451 1452 public void copyValues(ParametersParameterComponent dst) { 1453 super.copyValues(dst); 1454 dst.name = name == null ? null : name.copy(); 1455 dst.value = value == null ? null : value.copy(); 1456 dst.resource = resource == null ? null : resource.copy(); 1457 if (part != null) { 1458 dst.part = new ArrayList<ParametersParameterComponent>(); 1459 for (ParametersParameterComponent i : part) 1460 dst.part.add(i.copy()); 1461 }; 1462 } 1463 1464 @Override 1465 public boolean equalsDeep(Base other_) { 1466 if (!super.equalsDeep(other_)) 1467 return false; 1468 if (!(other_ instanceof ParametersParameterComponent)) 1469 return false; 1470 ParametersParameterComponent o = (ParametersParameterComponent) other_; 1471 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true) && compareDeep(resource, o.resource, true) 1472 && compareDeep(part, o.part, true); 1473 } 1474 1475 @Override 1476 public boolean equalsShallow(Base other_) { 1477 if (!super.equalsShallow(other_)) 1478 return false; 1479 if (!(other_ instanceof ParametersParameterComponent)) 1480 return false; 1481 ParametersParameterComponent o = (ParametersParameterComponent) other_; 1482 return compareValues(name, o.name, true); 1483 } 1484 1485 public boolean isEmpty() { 1486 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value, resource, part 1487 ); 1488 } 1489 1490 public String fhirType() { 1491 return "Parameters.parameter"; 1492 1493 } 1494 1495// added from java-adornments.txt: 1496public String toString() { 1497 String s = getName() + " = "; 1498 if (hasValue()) { 1499 if (getValue().isPrimitive()) { 1500 s = s + getValue().primitiveValue(); 1501 } else { 1502 s = s + "["+getValue().fhirType()+"]"; 1503 } 1504 } else if (hasResource()) { 1505 s = s + "["+getResource().fhirType()+"]"; 1506 } else { 1507 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder(); 1508 for (ParametersParameterComponent p : getPart()) { 1509 b.append(p.getName()); 1510 } 1511 s = s + "{"+b.toString()+"}"; 1512 } 1513 return s; 1514 } 1515 1516 1517 public boolean hasValuePrimitive() { 1518 return hasValue() && getValue() instanceof PrimitiveType<?>; 1519 } 1520 1521 public ParametersParameterComponent getPart(String name) { 1522 for (ParametersParameterComponent t : getPart()) { 1523 if (name.equals(t.getName())) { 1524 return t; 1525 } 1526 } 1527 return null; 1528 } 1529 1530 1531 public boolean hasPart(String name) { 1532 for (ParametersParameterComponent t : getPart()) { 1533 if (name.equals(t.getName())) { 1534 return true; 1535 } 1536 } 1537 return false; 1538 } 1539 1540// end addition 1541 1542 } 1543 1544 /** 1545 * A parameter passed to or received from the operation. 1546 */ 1547 @Child(name = "parameter", type = {}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1548 @Description(shortDefinition="Operation Parameter", formalDefinition="A parameter passed to or received from the operation." ) 1549 protected List<ParametersParameterComponent> parameter; 1550 1551 private static final long serialVersionUID = -1495940293L; 1552 1553 /** 1554 * Constructor 1555 */ 1556 public Parameters() { 1557 super(); 1558 } 1559 1560 /** 1561 * @return {@link #parameter} (A parameter passed to or received from the operation.) 1562 */ 1563 public List<ParametersParameterComponent> getParameter() { 1564 if (this.parameter == null) 1565 this.parameter = new ArrayList<ParametersParameterComponent>(); 1566 return this.parameter; 1567 } 1568 1569 /** 1570 * @return Returns a reference to <code>this</code> for easy method chaining 1571 */ 1572 public Parameters setParameter(List<ParametersParameterComponent> theParameter) { 1573 this.parameter = theParameter; 1574 return this; 1575 } 1576 1577 public boolean hasParameter() { 1578 if (this.parameter == null) 1579 return false; 1580 for (ParametersParameterComponent item : this.parameter) 1581 if (!item.isEmpty()) 1582 return true; 1583 return false; 1584 } 1585 1586 public ParametersParameterComponent addParameter() { //3 1587 ParametersParameterComponent t = new ParametersParameterComponent(); 1588 if (this.parameter == null) 1589 this.parameter = new ArrayList<ParametersParameterComponent>(); 1590 this.parameter.add(t); 1591 return t; 1592 } 1593 1594 public Parameters addParameter(ParametersParameterComponent t) { //3 1595 if (t == null) 1596 return this; 1597 if (this.parameter == null) 1598 this.parameter = new ArrayList<ParametersParameterComponent>(); 1599 this.parameter.add(t); 1600 return this; 1601 } 1602 1603 /** 1604 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 1605 */ 1606 public ParametersParameterComponent getParameterFirstRep() { 1607 if (getParameter().isEmpty()) { 1608 addParameter(); 1609 } 1610 return getParameter().get(0); 1611 } 1612 1613 protected void listChildren(List<Property> children) { 1614 super.listChildren(children); 1615 children.add(new Property("parameter", "", "A parameter passed to or received from the operation.", 0, java.lang.Integer.MAX_VALUE, parameter)); 1616 } 1617 1618 @Override 1619 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1620 switch (_hash) { 1621 case 1954460585: /*parameter*/ return new Property("parameter", "", "A parameter passed to or received from the operation.", 0, java.lang.Integer.MAX_VALUE, parameter); 1622 default: return super.getNamedProperty(_hash, _name, _checkValid); 1623 } 1624 1625 } 1626 1627 @Override 1628 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1629 switch (hash) { 1630 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ParametersParameterComponent 1631 default: return super.getProperty(hash, name, checkValid); 1632 } 1633 1634 } 1635 1636 @Override 1637 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1638 switch (hash) { 1639 case 1954460585: // parameter 1640 this.getParameter().add((ParametersParameterComponent) value); // ParametersParameterComponent 1641 return value; 1642 default: return super.setProperty(hash, name, value); 1643 } 1644 1645 } 1646 1647 @Override 1648 public Base setProperty(String name, Base value) throws FHIRException { 1649 if (name.equals("parameter")) { 1650 this.getParameter().add((ParametersParameterComponent) value); 1651 } else 1652 return super.setProperty(name, value); 1653 return value; 1654 } 1655 1656 @Override 1657 public void removeChild(String name, Base value) throws FHIRException { 1658 if (name.equals("parameter")) { 1659 this.getParameter().remove((ParametersParameterComponent) value); 1660 } else 1661 super.removeChild(name, value); 1662 1663 } 1664 1665 @Override 1666 public Base makeProperty(int hash, String name) throws FHIRException { 1667 switch (hash) { 1668 case 1954460585: return addParameter(); 1669 default: return super.makeProperty(hash, name); 1670 } 1671 1672 } 1673 1674 @Override 1675 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1676 switch (hash) { 1677 case 1954460585: /*parameter*/ return new String[] {}; 1678 default: return super.getTypesForProperty(hash, name); 1679 } 1680 1681 } 1682 1683 @Override 1684 public Base addChild(String name) throws FHIRException { 1685 if (name.equals("parameter")) { 1686 return addParameter(); 1687 } 1688 else 1689 return super.addChild(name); 1690 } 1691 1692 public String fhirType() { 1693 return "Parameters"; 1694 1695 } 1696 1697 public Parameters copy() { 1698 Parameters dst = new Parameters(); 1699 copyValues(dst); 1700 return dst; 1701 } 1702 1703 public void copyValues(Parameters dst) { 1704 super.copyValues(dst); 1705 if (parameter != null) { 1706 dst.parameter = new ArrayList<ParametersParameterComponent>(); 1707 for (ParametersParameterComponent i : parameter) 1708 dst.parameter.add(i.copy()); 1709 }; 1710 } 1711 1712 protected Parameters typedCopy() { 1713 return copy(); 1714 } 1715 1716 @Override 1717 public boolean equalsDeep(Base other_) { 1718 if (!super.equalsDeep(other_)) 1719 return false; 1720 if (!(other_ instanceof Parameters)) 1721 return false; 1722 Parameters o = (Parameters) other_; 1723 return compareDeep(parameter, o.parameter, true); 1724 } 1725 1726 @Override 1727 public boolean equalsShallow(Base other_) { 1728 if (!super.equalsShallow(other_)) 1729 return false; 1730 if (!(other_ instanceof Parameters)) 1731 return false; 1732 Parameters o = (Parameters) other_; 1733 return true; 1734 } 1735 1736 public boolean isEmpty() { 1737 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(parameter); 1738 } 1739 1740 @Override 1741 public ResourceType getResourceType() { 1742 return ResourceType.Parameters; 1743 } 1744 1745 // Manual code (from Configuration.txt): 1746 public Parameters addParameter(String name, boolean b) { 1747 addParameter().setName(name).setValue(new BooleanType(b)); 1748 return this; 1749 } 1750 1751 public Parameters addParameter(String name, int i) { 1752 addParameter().setName(name).setValue(new IntegerType(i)); 1753 return this; 1754 } 1755 1756 public Parameters addParameter(String name, String s) { 1757 if (s != null) 1758 addParameter().setName(name).setValue(new StringType(s)); 1759 return this; 1760 } 1761 1762 public Parameters addParameter(String name, DataType v) { 1763 if (v != null) 1764 addParameter().setName(name).setValue(v); 1765 return this; 1766 } 1767 1768 public Parameters setParameter(String name, boolean b) { 1769 for (ParametersParameterComponent p : getParameter()) { 1770 if (p.getName().equals(name)) { 1771 p.setValue(new BooleanType(b)); 1772 return this; 1773 } 1774 } 1775 addParameter().setName(name).setValue(new BooleanType(b)); 1776 return this; 1777 } 1778 1779 public Parameters setParameter(String name, String s) { 1780 if (s != null) { 1781 for (ParametersParameterComponent p : getParameter()) { 1782 if (p.getName().equals(name)) { 1783 p.setValue(new StringType(s)); 1784 return this; 1785 } 1786 } 1787 addParameter().setName(name).setValue(new StringType(s)); 1788 } 1789 return this; 1790 } 1791 1792 public Parameters setParameter(String name, DataType v) { 1793 if (v != null) { 1794 for (ParametersParameterComponent p : getParameter() ) { 1795 if (p.getName().equals(name)) { 1796 p.setValue(v); 1797 return this; 1798 } 1799 } 1800 addParameter().setName(name).setValue(v); 1801 } 1802 return this; 1803 } 1804 1805 public boolean hasParameterValue(String name) { 1806 for (ParametersParameterComponent p : getParameter()) { 1807 if (p.getName().equals(name) && p.hasValue()) 1808 return true; 1809 } 1810 return false; 1811 } 1812 1813 public boolean hasParameterValue(String name, String value) { 1814 for (ParametersParameterComponent p : getParameter()) { 1815 if (p.getName().equals(name) && p.hasValue() && value.equals(p.getValue().primitiveValue())) 1816 return true; 1817 } 1818 return false; 1819 } 1820 1821 public boolean hasParameter(String name) { 1822 for (ParametersParameterComponent p : getParameter()) { 1823 if (p.getName().equals(name)) 1824 return true; 1825 } 1826 return false; 1827 } 1828 1829 public DataType getParameterValue(String name) { 1830 for (ParametersParameterComponent p : getParameter()) { 1831 if (p.getName().equals(name)) 1832 return p.getValue(); 1833 } 1834 return null; 1835 } 1836 1837 public ParametersParameterComponent getParameter(String name) { 1838 for (ParametersParameterComponent p : getParameter()) { 1839 if (p.getName().equals(name)) 1840 return p; 1841 } 1842 return null; 1843 } 1844 1845 public List<DataType> getParameterValues(String name) { 1846 List<DataType> res = new ArrayList<>(); 1847 for (ParametersParameterComponent p : getParameter()) { 1848 if (p.getName().equals(name)) 1849 res.add(p.getValue()); 1850 } 1851 return res; 1852 } 1853 1854 public List<ParametersParameterComponent> getParameters(String name) { 1855 List<ParametersParameterComponent> res = new ArrayList<ParametersParameterComponent>(); 1856 for (ParametersParameterComponent p : getParameter()) { 1857 if (p.getName().equals(name)) 1858 res.add(p); 1859 } 1860 return res; 1861 } 1862 1863 public boolean getParameterBool(String name) { 1864 for (ParametersParameterComponent p : getParameter()) { 1865 if (p.getName().equals(name)) { 1866 if (p.getValue() instanceof BooleanType) 1867 return ((BooleanType) p.getValue()).booleanValue(); 1868 boolean ok = Boolean.getBoolean(p.getValue().primitiveValue()); 1869 return ok; 1870 } 1871 } 1872 return false; 1873 } 1874 1875 1876 public String getParameterString(String name) { 1877 for (ParametersParameterComponent p : getParameter()) { 1878 if (p.getName().equals(name)) { 1879 if (p.getValue() instanceof PrimitiveType) 1880 return ((PrimitiveType) p.getValue()).primitiveValue(); 1881 } 1882 } 1883 return null; 1884 } 1885 1886 public void clearParameters(String name) { 1887 getParameter().removeIf(p -> name.equals(p.getName())); 1888 } 1889 1890 public void addParameters(Parameters expParameters) { 1891 addParameters(expParameters.getParameter()); 1892 } 1893 1894 private void addParameters(List<ParametersParameterComponent> parameters) { 1895 for (ParametersParameterComponent p : parameters) { 1896 if (!hasParameter(p.getName())) { 1897 addParameter(p); 1898 } 1899 } 1900 } 1901 1902 // end addition 1903 1904} 1905