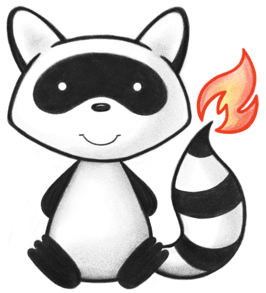
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 052 */ 053@ResourceDef(name="Patient", profile="http://hl7.org/fhir/StructureDefinition/Patient") 054public class Patient extends DomainResource { 055 056 public enum LinkType { 057 /** 058 * The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link. 059 */ 060 REPLACEDBY, 061 /** 062 * The patient resource containing this link is the current active patient record. The link points back to an inactive patient resource that has been merged into this resource, and should be consulted to retrieve additional referenced information. 063 */ 064 REPLACES, 065 /** 066 * The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information. 067 */ 068 REFER, 069 /** 070 * The patient resource containing this link is in use and valid, but points to another Patient or RelatedPerson resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other Patient/RelatedPerson resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid. 071 */ 072 SEEALSO, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static LinkType fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("replaced-by".equals(codeString)) 081 return REPLACEDBY; 082 if ("replaces".equals(codeString)) 083 return REPLACES; 084 if ("refer".equals(codeString)) 085 return REFER; 086 if ("seealso".equals(codeString)) 087 return SEEALSO; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown LinkType code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case REPLACEDBY: return "replaced-by"; 096 case REPLACES: return "replaces"; 097 case REFER: return "refer"; 098 case SEEALSO: return "seealso"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case REPLACEDBY: return "http://hl7.org/fhir/link-type"; 106 case REPLACES: return "http://hl7.org/fhir/link-type"; 107 case REFER: return "http://hl7.org/fhir/link-type"; 108 case SEEALSO: return "http://hl7.org/fhir/link-type"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case REPLACEDBY: return "The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link."; 116 case REPLACES: return "The patient resource containing this link is the current active patient record. The link points back to an inactive patient resource that has been merged into this resource, and should be consulted to retrieve additional referenced information."; 117 case REFER: return "The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information."; 118 case SEEALSO: return "The patient resource containing this link is in use and valid, but points to another Patient or RelatedPerson resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other Patient/RelatedPerson resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case REPLACEDBY: return "Replaced-by"; 126 case REPLACES: return "Replaces"; 127 case REFER: return "Refer"; 128 case SEEALSO: return "See also"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class LinkTypeEnumFactory implements EnumFactory<LinkType> { 136 public LinkType fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("replaced-by".equals(codeString)) 141 return LinkType.REPLACEDBY; 142 if ("replaces".equals(codeString)) 143 return LinkType.REPLACES; 144 if ("refer".equals(codeString)) 145 return LinkType.REFER; 146 if ("seealso".equals(codeString)) 147 return LinkType.SEEALSO; 148 throw new IllegalArgumentException("Unknown LinkType code '"+codeString+"'"); 149 } 150 public Enumeration<LinkType> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<LinkType>(this, LinkType.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<LinkType>(this, LinkType.NULL, code); 158 if ("replaced-by".equals(codeString)) 159 return new Enumeration<LinkType>(this, LinkType.REPLACEDBY, code); 160 if ("replaces".equals(codeString)) 161 return new Enumeration<LinkType>(this, LinkType.REPLACES, code); 162 if ("refer".equals(codeString)) 163 return new Enumeration<LinkType>(this, LinkType.REFER, code); 164 if ("seealso".equals(codeString)) 165 return new Enumeration<LinkType>(this, LinkType.SEEALSO, code); 166 throw new FHIRException("Unknown LinkType code '"+codeString+"'"); 167 } 168 public String toCode(LinkType code) { 169 if (code == LinkType.NULL) 170 return null; 171 if (code == LinkType.REPLACEDBY) 172 return "replaced-by"; 173 if (code == LinkType.REPLACES) 174 return "replaces"; 175 if (code == LinkType.REFER) 176 return "refer"; 177 if (code == LinkType.SEEALSO) 178 return "seealso"; 179 return "?"; 180 } 181 public String toSystem(LinkType code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class ContactComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * The nature of the relationship between the patient and the contact person. 190 */ 191 @Child(name = "relationship", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 192 @Description(shortDefinition="The kind of relationship", formalDefinition="The nature of the relationship between the patient and the contact person." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/patient-contactrelationship") 194 protected List<CodeableConcept> relationship; 195 196 /** 197 * A name associated with the contact person. 198 */ 199 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="A name associated with the contact person", formalDefinition="A name associated with the contact person." ) 201 protected HumanName name; 202 203 /** 204 * A contact detail for the person, e.g. a telephone number or an email address. 205 */ 206 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 207 @Description(shortDefinition="A contact detail for the person", formalDefinition="A contact detail for the person, e.g. a telephone number or an email address." ) 208 protected List<ContactPoint> telecom; 209 210 /** 211 * Address for the contact person. 212 */ 213 @Child(name = "address", type = {Address.class}, order=4, min=0, max=1, modifier=false, summary=false) 214 @Description(shortDefinition="Address for the contact person", formalDefinition="Address for the contact person." ) 215 protected Address address; 216 217 /** 218 * Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes. 219 */ 220 @Child(name = "gender", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 221 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes." ) 222 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 223 protected Enumeration<AdministrativeGender> gender; 224 225 /** 226 * Organization on behalf of which the contact is acting or for which the contact is working. 227 */ 228 @Child(name = "organization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 229 @Description(shortDefinition="Organization that is associated with the contact", formalDefinition="Organization on behalf of which the contact is acting or for which the contact is working." ) 230 protected Reference organization; 231 232 /** 233 * The period during which this contact person or organization is valid to be contacted relating to this patient. 234 */ 235 @Child(name = "period", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=false) 236 @Description(shortDefinition="The period during which this contact person or organization is valid to be contacted relating to this patient", formalDefinition="The period during which this contact person or organization is valid to be contacted relating to this patient." ) 237 protected Period period; 238 239 private static final long serialVersionUID = 117984384L; 240 241 /** 242 * Constructor 243 */ 244 public ContactComponent() { 245 super(); 246 } 247 248 /** 249 * @return {@link #relationship} (The nature of the relationship between the patient and the contact person.) 250 */ 251 public List<CodeableConcept> getRelationship() { 252 if (this.relationship == null) 253 this.relationship = new ArrayList<CodeableConcept>(); 254 return this.relationship; 255 } 256 257 /** 258 * @return Returns a reference to <code>this</code> for easy method chaining 259 */ 260 public ContactComponent setRelationship(List<CodeableConcept> theRelationship) { 261 this.relationship = theRelationship; 262 return this; 263 } 264 265 public boolean hasRelationship() { 266 if (this.relationship == null) 267 return false; 268 for (CodeableConcept item : this.relationship) 269 if (!item.isEmpty()) 270 return true; 271 return false; 272 } 273 274 public CodeableConcept addRelationship() { //3 275 CodeableConcept t = new CodeableConcept(); 276 if (this.relationship == null) 277 this.relationship = new ArrayList<CodeableConcept>(); 278 this.relationship.add(t); 279 return t; 280 } 281 282 public ContactComponent addRelationship(CodeableConcept t) { //3 283 if (t == null) 284 return this; 285 if (this.relationship == null) 286 this.relationship = new ArrayList<CodeableConcept>(); 287 this.relationship.add(t); 288 return this; 289 } 290 291 /** 292 * @return The first repetition of repeating field {@link #relationship}, creating it if it does not already exist {3} 293 */ 294 public CodeableConcept getRelationshipFirstRep() { 295 if (getRelationship().isEmpty()) { 296 addRelationship(); 297 } 298 return getRelationship().get(0); 299 } 300 301 /** 302 * @return {@link #name} (A name associated with the contact person.) 303 */ 304 public HumanName getName() { 305 if (this.name == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create ContactComponent.name"); 308 else if (Configuration.doAutoCreate()) 309 this.name = new HumanName(); // cc 310 return this.name; 311 } 312 313 public boolean hasName() { 314 return this.name != null && !this.name.isEmpty(); 315 } 316 317 /** 318 * @param value {@link #name} (A name associated with the contact person.) 319 */ 320 public ContactComponent setName(HumanName value) { 321 this.name = value; 322 return this; 323 } 324 325 /** 326 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone number or an email address.) 327 */ 328 public List<ContactPoint> getTelecom() { 329 if (this.telecom == null) 330 this.telecom = new ArrayList<ContactPoint>(); 331 return this.telecom; 332 } 333 334 /** 335 * @return Returns a reference to <code>this</code> for easy method chaining 336 */ 337 public ContactComponent setTelecom(List<ContactPoint> theTelecom) { 338 this.telecom = theTelecom; 339 return this; 340 } 341 342 public boolean hasTelecom() { 343 if (this.telecom == null) 344 return false; 345 for (ContactPoint item : this.telecom) 346 if (!item.isEmpty()) 347 return true; 348 return false; 349 } 350 351 public ContactPoint addTelecom() { //3 352 ContactPoint t = new ContactPoint(); 353 if (this.telecom == null) 354 this.telecom = new ArrayList<ContactPoint>(); 355 this.telecom.add(t); 356 return t; 357 } 358 359 public ContactComponent addTelecom(ContactPoint t) { //3 360 if (t == null) 361 return this; 362 if (this.telecom == null) 363 this.telecom = new ArrayList<ContactPoint>(); 364 this.telecom.add(t); 365 return this; 366 } 367 368 /** 369 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 370 */ 371 public ContactPoint getTelecomFirstRep() { 372 if (getTelecom().isEmpty()) { 373 addTelecom(); 374 } 375 return getTelecom().get(0); 376 } 377 378 /** 379 * @return {@link #address} (Address for the contact person.) 380 */ 381 public Address getAddress() { 382 if (this.address == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create ContactComponent.address"); 385 else if (Configuration.doAutoCreate()) 386 this.address = new Address(); // cc 387 return this.address; 388 } 389 390 public boolean hasAddress() { 391 return this.address != null && !this.address.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #address} (Address for the contact person.) 396 */ 397 public ContactComponent setAddress(Address value) { 398 this.address = value; 399 return this; 400 } 401 402 /** 403 * @return {@link #gender} (Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 404 */ 405 public Enumeration<AdministrativeGender> getGenderElement() { 406 if (this.gender == null) 407 if (Configuration.errorOnAutoCreate()) 408 throw new Error("Attempt to auto-create ContactComponent.gender"); 409 else if (Configuration.doAutoCreate()) 410 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 411 return this.gender; 412 } 413 414 public boolean hasGenderElement() { 415 return this.gender != null && !this.gender.isEmpty(); 416 } 417 418 public boolean hasGender() { 419 return this.gender != null && !this.gender.isEmpty(); 420 } 421 422 /** 423 * @param value {@link #gender} (Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 424 */ 425 public ContactComponent setGenderElement(Enumeration<AdministrativeGender> value) { 426 this.gender = value; 427 return this; 428 } 429 430 /** 431 * @return Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes. 432 */ 433 public AdministrativeGender getGender() { 434 return this.gender == null ? null : this.gender.getValue(); 435 } 436 437 /** 438 * @param value Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes. 439 */ 440 public ContactComponent setGender(AdministrativeGender value) { 441 if (value == null) 442 this.gender = null; 443 else { 444 if (this.gender == null) 445 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 446 this.gender.setValue(value); 447 } 448 return this; 449 } 450 451 /** 452 * @return {@link #organization} (Organization on behalf of which the contact is acting or for which the contact is working.) 453 */ 454 public Reference getOrganization() { 455 if (this.organization == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create ContactComponent.organization"); 458 else if (Configuration.doAutoCreate()) 459 this.organization = new Reference(); // cc 460 return this.organization; 461 } 462 463 public boolean hasOrganization() { 464 return this.organization != null && !this.organization.isEmpty(); 465 } 466 467 /** 468 * @param value {@link #organization} (Organization on behalf of which the contact is acting or for which the contact is working.) 469 */ 470 public ContactComponent setOrganization(Reference value) { 471 this.organization = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #period} (The period during which this contact person or organization is valid to be contacted relating to this patient.) 477 */ 478 public Period getPeriod() { 479 if (this.period == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create ContactComponent.period"); 482 else if (Configuration.doAutoCreate()) 483 this.period = new Period(); // cc 484 return this.period; 485 } 486 487 public boolean hasPeriod() { 488 return this.period != null && !this.period.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #period} (The period during which this contact person or organization is valid to be contacted relating to this patient.) 493 */ 494 public ContactComponent setPeriod(Period value) { 495 this.period = value; 496 return this; 497 } 498 499 protected void listChildren(List<Property> children) { 500 super.listChildren(children); 501 children.add(new Property("relationship", "CodeableConcept", "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, relationship)); 502 children.add(new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name)); 503 children.add(new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 504 children.add(new Property("address", "Address", "Address for the contact person.", 0, 1, address)); 505 children.add(new Property("gender", "code", "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 0, 1, gender)); 506 children.add(new Property("organization", "Reference(Organization)", "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, organization)); 507 children.add(new Property("period", "Period", "The period during which this contact person or organization is valid to be contacted relating to this patient.", 0, 1, period)); 508 } 509 510 @Override 511 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 512 switch (_hash) { 513 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, relationship); 514 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name); 515 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 516 case -1147692044: /*address*/ return new Property("address", "Address", "Address for the contact person.", 0, 1, address); 517 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 0, 1, gender); 518 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, organization); 519 case -991726143: /*period*/ return new Property("period", "Period", "The period during which this contact person or organization is valid to be contacted relating to this patient.", 0, 1, period); 520 default: return super.getNamedProperty(_hash, _name, _checkValid); 521 } 522 523 } 524 525 @Override 526 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 527 switch (hash) { 528 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : this.relationship.toArray(new Base[this.relationship.size()]); // CodeableConcept 529 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // HumanName 530 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 531 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // Address 532 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 533 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 534 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 535 default: return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case -261851592: // relationship 544 this.getRelationship().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 545 return value; 546 case 3373707: // name 547 this.name = TypeConvertor.castToHumanName(value); // HumanName 548 return value; 549 case -1429363305: // telecom 550 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 551 return value; 552 case -1147692044: // address 553 this.address = TypeConvertor.castToAddress(value); // Address 554 return value; 555 case -1249512767: // gender 556 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 557 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 558 return value; 559 case 1178922291: // organization 560 this.organization = TypeConvertor.castToReference(value); // Reference 561 return value; 562 case -991726143: // period 563 this.period = TypeConvertor.castToPeriod(value); // Period 564 return value; 565 default: return super.setProperty(hash, name, value); 566 } 567 568 } 569 570 @Override 571 public Base setProperty(String name, Base value) throws FHIRException { 572 if (name.equals("relationship")) { 573 this.getRelationship().add(TypeConvertor.castToCodeableConcept(value)); 574 } else if (name.equals("name")) { 575 this.name = TypeConvertor.castToHumanName(value); // HumanName 576 } else if (name.equals("telecom")) { 577 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 578 } else if (name.equals("address")) { 579 this.address = TypeConvertor.castToAddress(value); // Address 580 } else if (name.equals("gender")) { 581 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 582 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 583 } else if (name.equals("organization")) { 584 this.organization = TypeConvertor.castToReference(value); // Reference 585 } else if (name.equals("period")) { 586 this.period = TypeConvertor.castToPeriod(value); // Period 587 } else 588 return super.setProperty(name, value); 589 return value; 590 } 591 592 @Override 593 public void removeChild(String name, Base value) throws FHIRException { 594 if (name.equals("relationship")) { 595 this.getRelationship().remove(value); 596 } else if (name.equals("name")) { 597 this.name = null; 598 } else if (name.equals("telecom")) { 599 this.getTelecom().remove(value); 600 } else if (name.equals("address")) { 601 this.address = null; 602 } else if (name.equals("gender")) { 603 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 604 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 605 } else if (name.equals("organization")) { 606 this.organization = null; 607 } else if (name.equals("period")) { 608 this.period = null; 609 } else 610 super.removeChild(name, value); 611 612 } 613 614 @Override 615 public Base makeProperty(int hash, String name) throws FHIRException { 616 switch (hash) { 617 case -261851592: return addRelationship(); 618 case 3373707: return getName(); 619 case -1429363305: return addTelecom(); 620 case -1147692044: return getAddress(); 621 case -1249512767: return getGenderElement(); 622 case 1178922291: return getOrganization(); 623 case -991726143: return getPeriod(); 624 default: return super.makeProperty(hash, name); 625 } 626 627 } 628 629 @Override 630 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 631 switch (hash) { 632 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 633 case 3373707: /*name*/ return new String[] {"HumanName"}; 634 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 635 case -1147692044: /*address*/ return new String[] {"Address"}; 636 case -1249512767: /*gender*/ return new String[] {"code"}; 637 case 1178922291: /*organization*/ return new String[] {"Reference"}; 638 case -991726143: /*period*/ return new String[] {"Period"}; 639 default: return super.getTypesForProperty(hash, name); 640 } 641 642 } 643 644 @Override 645 public Base addChild(String name) throws FHIRException { 646 if (name.equals("relationship")) { 647 return addRelationship(); 648 } 649 else if (name.equals("name")) { 650 this.name = new HumanName(); 651 return this.name; 652 } 653 else if (name.equals("telecom")) { 654 return addTelecom(); 655 } 656 else if (name.equals("address")) { 657 this.address = new Address(); 658 return this.address; 659 } 660 else if (name.equals("gender")) { 661 throw new FHIRException("Cannot call addChild on a singleton property Patient.contact.gender"); 662 } 663 else if (name.equals("organization")) { 664 this.organization = new Reference(); 665 return this.organization; 666 } 667 else if (name.equals("period")) { 668 this.period = new Period(); 669 return this.period; 670 } 671 else 672 return super.addChild(name); 673 } 674 675 public ContactComponent copy() { 676 ContactComponent dst = new ContactComponent(); 677 copyValues(dst); 678 return dst; 679 } 680 681 public void copyValues(ContactComponent dst) { 682 super.copyValues(dst); 683 if (relationship != null) { 684 dst.relationship = new ArrayList<CodeableConcept>(); 685 for (CodeableConcept i : relationship) 686 dst.relationship.add(i.copy()); 687 }; 688 dst.name = name == null ? null : name.copy(); 689 if (telecom != null) { 690 dst.telecom = new ArrayList<ContactPoint>(); 691 for (ContactPoint i : telecom) 692 dst.telecom.add(i.copy()); 693 }; 694 dst.address = address == null ? null : address.copy(); 695 dst.gender = gender == null ? null : gender.copy(); 696 dst.organization = organization == null ? null : organization.copy(); 697 dst.period = period == null ? null : period.copy(); 698 } 699 700 @Override 701 public boolean equalsDeep(Base other_) { 702 if (!super.equalsDeep(other_)) 703 return false; 704 if (!(other_ instanceof ContactComponent)) 705 return false; 706 ContactComponent o = (ContactComponent) other_; 707 return compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 708 && compareDeep(address, o.address, true) && compareDeep(gender, o.gender, true) && compareDeep(organization, o.organization, true) 709 && compareDeep(period, o.period, true); 710 } 711 712 @Override 713 public boolean equalsShallow(Base other_) { 714 if (!super.equalsShallow(other_)) 715 return false; 716 if (!(other_ instanceof ContactComponent)) 717 return false; 718 ContactComponent o = (ContactComponent) other_; 719 return compareValues(gender, o.gender, true); 720 } 721 722 public boolean isEmpty() { 723 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relationship, name, telecom 724 , address, gender, organization, period); 725 } 726 727 public String fhirType() { 728 return "Patient.contact"; 729 730 } 731 732 } 733 734 @Block() 735 public static class PatientCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 736 /** 737 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English. 738 */ 739 @Child(name = "language", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 740 @Description(shortDefinition="The language which can be used to communicate with the patient about his or her health", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English." ) 741 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 742 protected CodeableConcept language; 743 744 /** 745 * Indicates whether or not the patient prefers this language (over other languages he masters up a certain level). 746 */ 747 @Child(name = "preferred", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 748 @Description(shortDefinition="Language preference indicator", formalDefinition="Indicates whether or not the patient prefers this language (over other languages he masters up a certain level)." ) 749 protected BooleanType preferred; 750 751 private static final long serialVersionUID = 633792918L; 752 753 /** 754 * Constructor 755 */ 756 public PatientCommunicationComponent() { 757 super(); 758 } 759 760 /** 761 * Constructor 762 */ 763 public PatientCommunicationComponent(CodeableConcept language) { 764 super(); 765 this.setLanguage(language); 766 } 767 768 /** 769 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 770 */ 771 public CodeableConcept getLanguage() { 772 if (this.language == null) 773 if (Configuration.errorOnAutoCreate()) 774 throw new Error("Attempt to auto-create PatientCommunicationComponent.language"); 775 else if (Configuration.doAutoCreate()) 776 this.language = new CodeableConcept(); // cc 777 return this.language; 778 } 779 780 public boolean hasLanguage() { 781 return this.language != null && !this.language.isEmpty(); 782 } 783 784 /** 785 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 786 */ 787 public PatientCommunicationComponent setLanguage(CodeableConcept value) { 788 this.language = value; 789 return this; 790 } 791 792 /** 793 * @return {@link #preferred} (Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 794 */ 795 public BooleanType getPreferredElement() { 796 if (this.preferred == null) 797 if (Configuration.errorOnAutoCreate()) 798 throw new Error("Attempt to auto-create PatientCommunicationComponent.preferred"); 799 else if (Configuration.doAutoCreate()) 800 this.preferred = new BooleanType(); // bb 801 return this.preferred; 802 } 803 804 public boolean hasPreferredElement() { 805 return this.preferred != null && !this.preferred.isEmpty(); 806 } 807 808 public boolean hasPreferred() { 809 return this.preferred != null && !this.preferred.isEmpty(); 810 } 811 812 /** 813 * @param value {@link #preferred} (Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 814 */ 815 public PatientCommunicationComponent setPreferredElement(BooleanType value) { 816 this.preferred = value; 817 return this; 818 } 819 820 /** 821 * @return Indicates whether or not the patient prefers this language (over other languages he masters up a certain level). 822 */ 823 public boolean getPreferred() { 824 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 825 } 826 827 /** 828 * @param value Indicates whether or not the patient prefers this language (over other languages he masters up a certain level). 829 */ 830 public PatientCommunicationComponent setPreferred(boolean value) { 831 if (this.preferred == null) 832 this.preferred = new BooleanType(); 833 this.preferred.setValue(value); 834 return this; 835 } 836 837 protected void listChildren(List<Property> children) { 838 super.listChildren(children); 839 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language)); 840 children.add(new Property("preferred", "boolean", "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 0, 1, preferred)); 841 } 842 843 @Override 844 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 845 switch (_hash) { 846 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language); 847 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 0, 1, preferred); 848 default: return super.getNamedProperty(_hash, _name, _checkValid); 849 } 850 851 } 852 853 @Override 854 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 855 switch (hash) { 856 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 857 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 858 default: return super.getProperty(hash, name, checkValid); 859 } 860 861 } 862 863 @Override 864 public Base setProperty(int hash, String name, Base value) throws FHIRException { 865 switch (hash) { 866 case -1613589672: // language 867 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 868 return value; 869 case -1294005119: // preferred 870 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 871 return value; 872 default: return super.setProperty(hash, name, value); 873 } 874 875 } 876 877 @Override 878 public Base setProperty(String name, Base value) throws FHIRException { 879 if (name.equals("language")) { 880 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 881 } else if (name.equals("preferred")) { 882 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 883 } else 884 return super.setProperty(name, value); 885 return value; 886 } 887 888 @Override 889 public void removeChild(String name, Base value) throws FHIRException { 890 if (name.equals("language")) { 891 this.language = null; 892 } else if (name.equals("preferred")) { 893 this.preferred = null; 894 } else 895 super.removeChild(name, value); 896 897 } 898 899 @Override 900 public Base makeProperty(int hash, String name) throws FHIRException { 901 switch (hash) { 902 case -1613589672: return getLanguage(); 903 case -1294005119: return getPreferredElement(); 904 default: return super.makeProperty(hash, name); 905 } 906 907 } 908 909 @Override 910 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 911 switch (hash) { 912 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 913 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 914 default: return super.getTypesForProperty(hash, name); 915 } 916 917 } 918 919 @Override 920 public Base addChild(String name) throws FHIRException { 921 if (name.equals("language")) { 922 this.language = new CodeableConcept(); 923 return this.language; 924 } 925 else if (name.equals("preferred")) { 926 throw new FHIRException("Cannot call addChild on a singleton property Patient.communication.preferred"); 927 } 928 else 929 return super.addChild(name); 930 } 931 932 public PatientCommunicationComponent copy() { 933 PatientCommunicationComponent dst = new PatientCommunicationComponent(); 934 copyValues(dst); 935 return dst; 936 } 937 938 public void copyValues(PatientCommunicationComponent dst) { 939 super.copyValues(dst); 940 dst.language = language == null ? null : language.copy(); 941 dst.preferred = preferred == null ? null : preferred.copy(); 942 } 943 944 @Override 945 public boolean equalsDeep(Base other_) { 946 if (!super.equalsDeep(other_)) 947 return false; 948 if (!(other_ instanceof PatientCommunicationComponent)) 949 return false; 950 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 951 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 952 } 953 954 @Override 955 public boolean equalsShallow(Base other_) { 956 if (!super.equalsShallow(other_)) 957 return false; 958 if (!(other_ instanceof PatientCommunicationComponent)) 959 return false; 960 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 961 return compareValues(preferred, o.preferred, true); 962 } 963 964 public boolean isEmpty() { 965 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 966 } 967 968 public String fhirType() { 969 return "Patient.communication"; 970 971 } 972 973 } 974 975 @Block() 976 public static class PatientLinkComponent extends BackboneElement implements IBaseBackboneElement { 977 /** 978 * Link to a Patient or RelatedPerson resource that concerns the same actual individual. 979 */ 980 @Child(name = "other", type = {Patient.class, RelatedPerson.class}, order=1, min=1, max=1, modifier=false, summary=true) 981 @Description(shortDefinition="The other patient or related person resource that the link refers to", formalDefinition="Link to a Patient or RelatedPerson resource that concerns the same actual individual." ) 982 protected Reference other; 983 984 /** 985 * The type of link between this patient resource and another patient resource. 986 */ 987 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 988 @Description(shortDefinition="replaced-by | replaces | refer | seealso", formalDefinition="The type of link between this patient resource and another patient resource." ) 989 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/link-type") 990 protected Enumeration<LinkType> type; 991 992 private static final long serialVersionUID = 182421394L; 993 994 /** 995 * Constructor 996 */ 997 public PatientLinkComponent() { 998 super(); 999 } 1000 1001 /** 1002 * Constructor 1003 */ 1004 public PatientLinkComponent(Reference other, LinkType type) { 1005 super(); 1006 this.setOther(other); 1007 this.setType(type); 1008 } 1009 1010 /** 1011 * @return {@link #other} (Link to a Patient or RelatedPerson resource that concerns the same actual individual.) 1012 */ 1013 public Reference getOther() { 1014 if (this.other == null) 1015 if (Configuration.errorOnAutoCreate()) 1016 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1017 else if (Configuration.doAutoCreate()) 1018 this.other = new Reference(); // cc 1019 return this.other; 1020 } 1021 1022 public boolean hasOther() { 1023 return this.other != null && !this.other.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #other} (Link to a Patient or RelatedPerson resource that concerns the same actual individual.) 1028 */ 1029 public PatientLinkComponent setOther(Reference value) { 1030 this.other = value; 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #type} (The type of link between this patient resource and another patient resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1036 */ 1037 public Enumeration<LinkType> getTypeElement() { 1038 if (this.type == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create PatientLinkComponent.type"); 1041 else if (Configuration.doAutoCreate()) 1042 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); // bb 1043 return this.type; 1044 } 1045 1046 public boolean hasTypeElement() { 1047 return this.type != null && !this.type.isEmpty(); 1048 } 1049 1050 public boolean hasType() { 1051 return this.type != null && !this.type.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #type} (The type of link between this patient resource and another patient resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1056 */ 1057 public PatientLinkComponent setTypeElement(Enumeration<LinkType> value) { 1058 this.type = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return The type of link between this patient resource and another patient resource. 1064 */ 1065 public LinkType getType() { 1066 return this.type == null ? null : this.type.getValue(); 1067 } 1068 1069 /** 1070 * @param value The type of link between this patient resource and another patient resource. 1071 */ 1072 public PatientLinkComponent setType(LinkType value) { 1073 if (this.type == null) 1074 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); 1075 this.type.setValue(value); 1076 return this; 1077 } 1078 1079 protected void listChildren(List<Property> children) { 1080 super.listChildren(children); 1081 children.add(new Property("other", "Reference(Patient|RelatedPerson)", "Link to a Patient or RelatedPerson resource that concerns the same actual individual.", 0, 1, other)); 1082 children.add(new Property("type", "code", "The type of link between this patient resource and another patient resource.", 0, 1, type)); 1083 } 1084 1085 @Override 1086 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1087 switch (_hash) { 1088 case 106069776: /*other*/ return new Property("other", "Reference(Patient|RelatedPerson)", "Link to a Patient or RelatedPerson resource that concerns the same actual individual.", 0, 1, other); 1089 case 3575610: /*type*/ return new Property("type", "code", "The type of link between this patient resource and another patient resource.", 0, 1, type); 1090 default: return super.getNamedProperty(_hash, _name, _checkValid); 1091 } 1092 1093 } 1094 1095 @Override 1096 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1097 switch (hash) { 1098 case 106069776: /*other*/ return this.other == null ? new Base[0] : new Base[] {this.other}; // Reference 1099 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<LinkType> 1100 default: return super.getProperty(hash, name, checkValid); 1101 } 1102 1103 } 1104 1105 @Override 1106 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1107 switch (hash) { 1108 case 106069776: // other 1109 this.other = TypeConvertor.castToReference(value); // Reference 1110 return value; 1111 case 3575610: // type 1112 value = new LinkTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1113 this.type = (Enumeration) value; // Enumeration<LinkType> 1114 return value; 1115 default: return super.setProperty(hash, name, value); 1116 } 1117 1118 } 1119 1120 @Override 1121 public Base setProperty(String name, Base value) throws FHIRException { 1122 if (name.equals("other")) { 1123 this.other = TypeConvertor.castToReference(value); // Reference 1124 } else if (name.equals("type")) { 1125 value = new LinkTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1126 this.type = (Enumeration) value; // Enumeration<LinkType> 1127 } else 1128 return super.setProperty(name, value); 1129 return value; 1130 } 1131 1132 @Override 1133 public void removeChild(String name, Base value) throws FHIRException { 1134 if (name.equals("other")) { 1135 this.other = null; 1136 } else if (name.equals("type")) { 1137 value = new LinkTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1138 this.type = (Enumeration) value; // Enumeration<LinkType> 1139 } else 1140 super.removeChild(name, value); 1141 1142 } 1143 1144 @Override 1145 public Base makeProperty(int hash, String name) throws FHIRException { 1146 switch (hash) { 1147 case 106069776: return getOther(); 1148 case 3575610: return getTypeElement(); 1149 default: return super.makeProperty(hash, name); 1150 } 1151 1152 } 1153 1154 @Override 1155 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1156 switch (hash) { 1157 case 106069776: /*other*/ return new String[] {"Reference"}; 1158 case 3575610: /*type*/ return new String[] {"code"}; 1159 default: return super.getTypesForProperty(hash, name); 1160 } 1161 1162 } 1163 1164 @Override 1165 public Base addChild(String name) throws FHIRException { 1166 if (name.equals("other")) { 1167 this.other = new Reference(); 1168 return this.other; 1169 } 1170 else if (name.equals("type")) { 1171 throw new FHIRException("Cannot call addChild on a singleton property Patient.link.type"); 1172 } 1173 else 1174 return super.addChild(name); 1175 } 1176 1177 public PatientLinkComponent copy() { 1178 PatientLinkComponent dst = new PatientLinkComponent(); 1179 copyValues(dst); 1180 return dst; 1181 } 1182 1183 public void copyValues(PatientLinkComponent dst) { 1184 super.copyValues(dst); 1185 dst.other = other == null ? null : other.copy(); 1186 dst.type = type == null ? null : type.copy(); 1187 } 1188 1189 @Override 1190 public boolean equalsDeep(Base other_) { 1191 if (!super.equalsDeep(other_)) 1192 return false; 1193 if (!(other_ instanceof PatientLinkComponent)) 1194 return false; 1195 PatientLinkComponent o = (PatientLinkComponent) other_; 1196 return compareDeep(other, o.other, true) && compareDeep(type, o.type, true); 1197 } 1198 1199 @Override 1200 public boolean equalsShallow(Base other_) { 1201 if (!super.equalsShallow(other_)) 1202 return false; 1203 if (!(other_ instanceof PatientLinkComponent)) 1204 return false; 1205 PatientLinkComponent o = (PatientLinkComponent) other_; 1206 return compareValues(type, o.type, true); 1207 } 1208 1209 public boolean isEmpty() { 1210 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(other, type); 1211 } 1212 1213 public String fhirType() { 1214 return "Patient.link"; 1215 1216 } 1217 1218 } 1219 1220 /** 1221 * An identifier for this patient. 1222 */ 1223 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1224 @Description(shortDefinition="An identifier for this patient", formalDefinition="An identifier for this patient." ) 1225 protected List<Identifier> identifier; 1226 1227 /** 1228 * Whether this patient record is in active use. 1229Many systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules. 1230 1231It is often used to filter patient lists to exclude inactive patients 1232 1233Deceased patients may also be marked as inactive for the same reasons, but may be active for some time after death. 1234 */ 1235 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1236 @Description(shortDefinition="Whether this patient's record is in active use", formalDefinition="Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death." ) 1237 protected BooleanType active; 1238 1239 /** 1240 * A name associated with the individual. 1241 */ 1242 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1243 @Description(shortDefinition="A name associated with the patient", formalDefinition="A name associated with the individual." ) 1244 protected List<HumanName> name; 1245 1246 /** 1247 * A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted. 1248 */ 1249 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1250 @Description(shortDefinition="A contact detail for the individual", formalDefinition="A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted." ) 1251 protected List<ContactPoint> telecom; 1252 1253 /** 1254 * Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes. 1255 */ 1256 @Child(name = "gender", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1257 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes." ) 1258 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 1259 protected Enumeration<AdministrativeGender> gender; 1260 1261 /** 1262 * The date of birth for the individual. 1263 */ 1264 @Child(name = "birthDate", type = {DateType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1265 @Description(shortDefinition="The date of birth for the individual", formalDefinition="The date of birth for the individual." ) 1266 protected DateType birthDate; 1267 1268 /** 1269 * Indicates if the individual is deceased or not. 1270 */ 1271 @Child(name = "deceased", type = {BooleanType.class, DateTimeType.class}, order=6, min=0, max=1, modifier=true, summary=true) 1272 @Description(shortDefinition="Indicates if the individual is deceased or not", formalDefinition="Indicates if the individual is deceased or not." ) 1273 protected DataType deceased; 1274 1275 /** 1276 * An address for the individual. 1277 */ 1278 @Child(name = "address", type = {Address.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1279 @Description(shortDefinition="An address for the individual", formalDefinition="An address for the individual." ) 1280 protected List<Address> address; 1281 1282 /** 1283 * This field contains a patient's most recent marital (civil) status. 1284 */ 1285 @Child(name = "maritalStatus", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 1286 @Description(shortDefinition="Marital (civil) status of a patient", formalDefinition="This field contains a patient's most recent marital (civil) status." ) 1287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/marital-status") 1288 protected CodeableConcept maritalStatus; 1289 1290 /** 1291 * Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer). 1292 */ 1293 @Child(name = "multipleBirth", type = {BooleanType.class, IntegerType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1294 @Description(shortDefinition="Whether patient is part of a multiple birth", formalDefinition="Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer)." ) 1295 protected DataType multipleBirth; 1296 1297 /** 1298 * Image of the patient. 1299 */ 1300 @Child(name = "photo", type = {Attachment.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1301 @Description(shortDefinition="Image of the patient", formalDefinition="Image of the patient." ) 1302 protected List<Attachment> photo; 1303 1304 /** 1305 * A contact party (e.g. guardian, partner, friend) for the patient. 1306 */ 1307 @Child(name = "contact", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1308 @Description(shortDefinition="A contact party (e.g. guardian, partner, friend) for the patient", formalDefinition="A contact party (e.g. guardian, partner, friend) for the patient." ) 1309 protected List<ContactComponent> contact; 1310 1311 /** 1312 * A language which may be used to communicate with the patient about his or her health. 1313 */ 1314 @Child(name = "communication", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1315 @Description(shortDefinition="A language which may be used to communicate with the patient about his or her health", formalDefinition="A language which may be used to communicate with the patient about his or her health." ) 1316 protected List<PatientCommunicationComponent> communication; 1317 1318 /** 1319 * Patient's nominated care provider. 1320 */ 1321 @Child(name = "generalPractitioner", type = {Organization.class, Practitioner.class, PractitionerRole.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1322 @Description(shortDefinition="Patient's nominated primary care provider", formalDefinition="Patient's nominated care provider." ) 1323 protected List<Reference> generalPractitioner; 1324 1325 /** 1326 * Organization that is the custodian of the patient record. 1327 */ 1328 @Child(name = "managingOrganization", type = {Organization.class}, order=14, min=0, max=1, modifier=false, summary=true) 1329 @Description(shortDefinition="Organization that is the custodian of the patient record", formalDefinition="Organization that is the custodian of the patient record." ) 1330 protected Reference managingOrganization; 1331 1332 /** 1333 * Link to a Patient or RelatedPerson resource that concerns the same actual individual. 1334 */ 1335 @Child(name = "link", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 1336 @Description(shortDefinition="Link to a Patient or RelatedPerson resource that concerns the same actual individual", formalDefinition="Link to a Patient or RelatedPerson resource that concerns the same actual individual." ) 1337 protected List<PatientLinkComponent> link; 1338 1339 private static final long serialVersionUID = 1376657499L; 1340 1341 /** 1342 * Constructor 1343 */ 1344 public Patient() { 1345 super(); 1346 } 1347 1348 /** 1349 * @return {@link #identifier} (An identifier for this patient.) 1350 */ 1351 public List<Identifier> getIdentifier() { 1352 if (this.identifier == null) 1353 this.identifier = new ArrayList<Identifier>(); 1354 return this.identifier; 1355 } 1356 1357 /** 1358 * @return Returns a reference to <code>this</code> for easy method chaining 1359 */ 1360 public Patient setIdentifier(List<Identifier> theIdentifier) { 1361 this.identifier = theIdentifier; 1362 return this; 1363 } 1364 1365 public boolean hasIdentifier() { 1366 if (this.identifier == null) 1367 return false; 1368 for (Identifier item : this.identifier) 1369 if (!item.isEmpty()) 1370 return true; 1371 return false; 1372 } 1373 1374 public Identifier addIdentifier() { //3 1375 Identifier t = new Identifier(); 1376 if (this.identifier == null) 1377 this.identifier = new ArrayList<Identifier>(); 1378 this.identifier.add(t); 1379 return t; 1380 } 1381 1382 public Patient addIdentifier(Identifier t) { //3 1383 if (t == null) 1384 return this; 1385 if (this.identifier == null) 1386 this.identifier = new ArrayList<Identifier>(); 1387 this.identifier.add(t); 1388 return this; 1389 } 1390 1391 /** 1392 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1393 */ 1394 public Identifier getIdentifierFirstRep() { 1395 if (getIdentifier().isEmpty()) { 1396 addIdentifier(); 1397 } 1398 return getIdentifier().get(0); 1399 } 1400 1401 /** 1402 * @return {@link #active} (Whether this patient record is in active use. 1403Many systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules. 1404 1405It is often used to filter patient lists to exclude inactive patients 1406 1407Deceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1408 */ 1409 public BooleanType getActiveElement() { 1410 if (this.active == null) 1411 if (Configuration.errorOnAutoCreate()) 1412 throw new Error("Attempt to auto-create Patient.active"); 1413 else if (Configuration.doAutoCreate()) 1414 this.active = new BooleanType(); // bb 1415 return this.active; 1416 } 1417 1418 public boolean hasActiveElement() { 1419 return this.active != null && !this.active.isEmpty(); 1420 } 1421 1422 public boolean hasActive() { 1423 return this.active != null && !this.active.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #active} (Whether this patient record is in active use. 1428Many systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules. 1429 1430It is often used to filter patient lists to exclude inactive patients 1431 1432Deceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1433 */ 1434 public Patient setActiveElement(BooleanType value) { 1435 this.active = value; 1436 return this; 1437 } 1438 1439 /** 1440 * @return Whether this patient record is in active use. 1441Many systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules. 1442 1443It is often used to filter patient lists to exclude inactive patients 1444 1445Deceased patients may also be marked as inactive for the same reasons, but may be active for some time after death. 1446 */ 1447 public boolean getActive() { 1448 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1449 } 1450 1451 /** 1452 * @param value Whether this patient record is in active use. 1453Many systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules. 1454 1455It is often used to filter patient lists to exclude inactive patients 1456 1457Deceased patients may also be marked as inactive for the same reasons, but may be active for some time after death. 1458 */ 1459 public Patient setActive(boolean value) { 1460 if (this.active == null) 1461 this.active = new BooleanType(); 1462 this.active.setValue(value); 1463 return this; 1464 } 1465 1466 /** 1467 * @return {@link #name} (A name associated with the individual.) 1468 */ 1469 public List<HumanName> getName() { 1470 if (this.name == null) 1471 this.name = new ArrayList<HumanName>(); 1472 return this.name; 1473 } 1474 1475 /** 1476 * @return Returns a reference to <code>this</code> for easy method chaining 1477 */ 1478 public Patient setName(List<HumanName> theName) { 1479 this.name = theName; 1480 return this; 1481 } 1482 1483 public boolean hasName() { 1484 if (this.name == null) 1485 return false; 1486 for (HumanName item : this.name) 1487 if (!item.isEmpty()) 1488 return true; 1489 return false; 1490 } 1491 1492 public HumanName addName() { //3 1493 HumanName t = new HumanName(); 1494 if (this.name == null) 1495 this.name = new ArrayList<HumanName>(); 1496 this.name.add(t); 1497 return t; 1498 } 1499 1500 public Patient addName(HumanName t) { //3 1501 if (t == null) 1502 return this; 1503 if (this.name == null) 1504 this.name = new ArrayList<HumanName>(); 1505 this.name.add(t); 1506 return this; 1507 } 1508 1509 /** 1510 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 1511 */ 1512 public HumanName getNameFirstRep() { 1513 if (getName().isEmpty()) { 1514 addName(); 1515 } 1516 return getName().get(0); 1517 } 1518 1519 /** 1520 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.) 1521 */ 1522 public List<ContactPoint> getTelecom() { 1523 if (this.telecom == null) 1524 this.telecom = new ArrayList<ContactPoint>(); 1525 return this.telecom; 1526 } 1527 1528 /** 1529 * @return Returns a reference to <code>this</code> for easy method chaining 1530 */ 1531 public Patient setTelecom(List<ContactPoint> theTelecom) { 1532 this.telecom = theTelecom; 1533 return this; 1534 } 1535 1536 public boolean hasTelecom() { 1537 if (this.telecom == null) 1538 return false; 1539 for (ContactPoint item : this.telecom) 1540 if (!item.isEmpty()) 1541 return true; 1542 return false; 1543 } 1544 1545 public ContactPoint addTelecom() { //3 1546 ContactPoint t = new ContactPoint(); 1547 if (this.telecom == null) 1548 this.telecom = new ArrayList<ContactPoint>(); 1549 this.telecom.add(t); 1550 return t; 1551 } 1552 1553 public Patient addTelecom(ContactPoint t) { //3 1554 if (t == null) 1555 return this; 1556 if (this.telecom == null) 1557 this.telecom = new ArrayList<ContactPoint>(); 1558 this.telecom.add(t); 1559 return this; 1560 } 1561 1562 /** 1563 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 1564 */ 1565 public ContactPoint getTelecomFirstRep() { 1566 if (getTelecom().isEmpty()) { 1567 addTelecom(); 1568 } 1569 return getTelecom().get(0); 1570 } 1571 1572 /** 1573 * @return {@link #gender} (Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 1574 */ 1575 public Enumeration<AdministrativeGender> getGenderElement() { 1576 if (this.gender == null) 1577 if (Configuration.errorOnAutoCreate()) 1578 throw new Error("Attempt to auto-create Patient.gender"); 1579 else if (Configuration.doAutoCreate()) 1580 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1581 return this.gender; 1582 } 1583 1584 public boolean hasGenderElement() { 1585 return this.gender != null && !this.gender.isEmpty(); 1586 } 1587 1588 public boolean hasGender() { 1589 return this.gender != null && !this.gender.isEmpty(); 1590 } 1591 1592 /** 1593 * @param value {@link #gender} (Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 1594 */ 1595 public Patient setGenderElement(Enumeration<AdministrativeGender> value) { 1596 this.gender = value; 1597 return this; 1598 } 1599 1600 /** 1601 * @return Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes. 1602 */ 1603 public AdministrativeGender getGender() { 1604 return this.gender == null ? null : this.gender.getValue(); 1605 } 1606 1607 /** 1608 * @param value Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes. 1609 */ 1610 public Patient setGender(AdministrativeGender value) { 1611 if (value == null) 1612 this.gender = null; 1613 else { 1614 if (this.gender == null) 1615 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1616 this.gender.setValue(value); 1617 } 1618 return this; 1619 } 1620 1621 /** 1622 * @return {@link #birthDate} (The date of birth for the individual.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 1623 */ 1624 public DateType getBirthDateElement() { 1625 if (this.birthDate == null) 1626 if (Configuration.errorOnAutoCreate()) 1627 throw new Error("Attempt to auto-create Patient.birthDate"); 1628 else if (Configuration.doAutoCreate()) 1629 this.birthDate = new DateType(); // bb 1630 return this.birthDate; 1631 } 1632 1633 public boolean hasBirthDateElement() { 1634 return this.birthDate != null && !this.birthDate.isEmpty(); 1635 } 1636 1637 public boolean hasBirthDate() { 1638 return this.birthDate != null && !this.birthDate.isEmpty(); 1639 } 1640 1641 /** 1642 * @param value {@link #birthDate} (The date of birth for the individual.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 1643 */ 1644 public Patient setBirthDateElement(DateType value) { 1645 this.birthDate = value; 1646 return this; 1647 } 1648 1649 /** 1650 * @return The date of birth for the individual. 1651 */ 1652 public Date getBirthDate() { 1653 return this.birthDate == null ? null : this.birthDate.getValue(); 1654 } 1655 1656 /** 1657 * @param value The date of birth for the individual. 1658 */ 1659 public Patient setBirthDate(Date value) { 1660 if (value == null) 1661 this.birthDate = null; 1662 else { 1663 if (this.birthDate == null) 1664 this.birthDate = new DateType(); 1665 this.birthDate.setValue(value); 1666 } 1667 return this; 1668 } 1669 1670 /** 1671 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1672 */ 1673 public DataType getDeceased() { 1674 return this.deceased; 1675 } 1676 1677 /** 1678 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1679 */ 1680 public BooleanType getDeceasedBooleanType() throws FHIRException { 1681 if (this.deceased == null) 1682 this.deceased = new BooleanType(); 1683 if (!(this.deceased instanceof BooleanType)) 1684 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1685 return (BooleanType) this.deceased; 1686 } 1687 1688 public boolean hasDeceasedBooleanType() { 1689 return this != null && this.deceased instanceof BooleanType; 1690 } 1691 1692 /** 1693 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1694 */ 1695 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 1696 if (this.deceased == null) 1697 this.deceased = new DateTimeType(); 1698 if (!(this.deceased instanceof DateTimeType)) 1699 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1700 return (DateTimeType) this.deceased; 1701 } 1702 1703 public boolean hasDeceasedDateTimeType() { 1704 return this != null && this.deceased instanceof DateTimeType; 1705 } 1706 1707 public boolean hasDeceased() { 1708 return this.deceased != null && !this.deceased.isEmpty(); 1709 } 1710 1711 /** 1712 * @param value {@link #deceased} (Indicates if the individual is deceased or not.) 1713 */ 1714 public Patient setDeceased(DataType value) { 1715 if (value != null && !(value instanceof BooleanType || value instanceof DateTimeType)) 1716 throw new FHIRException("Not the right type for Patient.deceased[x]: "+value.fhirType()); 1717 this.deceased = value; 1718 return this; 1719 } 1720 1721 /** 1722 * @return {@link #address} (An address for the individual.) 1723 */ 1724 public List<Address> getAddress() { 1725 if (this.address == null) 1726 this.address = new ArrayList<Address>(); 1727 return this.address; 1728 } 1729 1730 /** 1731 * @return Returns a reference to <code>this</code> for easy method chaining 1732 */ 1733 public Patient setAddress(List<Address> theAddress) { 1734 this.address = theAddress; 1735 return this; 1736 } 1737 1738 public boolean hasAddress() { 1739 if (this.address == null) 1740 return false; 1741 for (Address item : this.address) 1742 if (!item.isEmpty()) 1743 return true; 1744 return false; 1745 } 1746 1747 public Address addAddress() { //3 1748 Address t = new Address(); 1749 if (this.address == null) 1750 this.address = new ArrayList<Address>(); 1751 this.address.add(t); 1752 return t; 1753 } 1754 1755 public Patient addAddress(Address t) { //3 1756 if (t == null) 1757 return this; 1758 if (this.address == null) 1759 this.address = new ArrayList<Address>(); 1760 this.address.add(t); 1761 return this; 1762 } 1763 1764 /** 1765 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist {3} 1766 */ 1767 public Address getAddressFirstRep() { 1768 if (getAddress().isEmpty()) { 1769 addAddress(); 1770 } 1771 return getAddress().get(0); 1772 } 1773 1774 /** 1775 * @return {@link #maritalStatus} (This field contains a patient's most recent marital (civil) status.) 1776 */ 1777 public CodeableConcept getMaritalStatus() { 1778 if (this.maritalStatus == null) 1779 if (Configuration.errorOnAutoCreate()) 1780 throw new Error("Attempt to auto-create Patient.maritalStatus"); 1781 else if (Configuration.doAutoCreate()) 1782 this.maritalStatus = new CodeableConcept(); // cc 1783 return this.maritalStatus; 1784 } 1785 1786 public boolean hasMaritalStatus() { 1787 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 1788 } 1789 1790 /** 1791 * @param value {@link #maritalStatus} (This field contains a patient's most recent marital (civil) status.) 1792 */ 1793 public Patient setMaritalStatus(CodeableConcept value) { 1794 this.maritalStatus = value; 1795 return this; 1796 } 1797 1798 /** 1799 * @return {@link #multipleBirth} (Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).) 1800 */ 1801 public DataType getMultipleBirth() { 1802 return this.multipleBirth; 1803 } 1804 1805 /** 1806 * @return {@link #multipleBirth} (Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).) 1807 */ 1808 public BooleanType getMultipleBirthBooleanType() throws FHIRException { 1809 if (this.multipleBirth == null) 1810 this.multipleBirth = new BooleanType(); 1811 if (!(this.multipleBirth instanceof BooleanType)) 1812 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.multipleBirth.getClass().getName()+" was encountered"); 1813 return (BooleanType) this.multipleBirth; 1814 } 1815 1816 public boolean hasMultipleBirthBooleanType() { 1817 return this != null && this.multipleBirth instanceof BooleanType; 1818 } 1819 1820 /** 1821 * @return {@link #multipleBirth} (Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).) 1822 */ 1823 public IntegerType getMultipleBirthIntegerType() throws FHIRException { 1824 if (this.multipleBirth == null) 1825 this.multipleBirth = new IntegerType(); 1826 if (!(this.multipleBirth instanceof IntegerType)) 1827 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.multipleBirth.getClass().getName()+" was encountered"); 1828 return (IntegerType) this.multipleBirth; 1829 } 1830 1831 public boolean hasMultipleBirthIntegerType() { 1832 return this != null && this.multipleBirth instanceof IntegerType; 1833 } 1834 1835 public boolean hasMultipleBirth() { 1836 return this.multipleBirth != null && !this.multipleBirth.isEmpty(); 1837 } 1838 1839 /** 1840 * @param value {@link #multipleBirth} (Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).) 1841 */ 1842 public Patient setMultipleBirth(DataType value) { 1843 if (value != null && !(value instanceof BooleanType || value instanceof IntegerType)) 1844 throw new FHIRException("Not the right type for Patient.multipleBirth[x]: "+value.fhirType()); 1845 this.multipleBirth = value; 1846 return this; 1847 } 1848 1849 /** 1850 * @return {@link #photo} (Image of the patient.) 1851 */ 1852 public List<Attachment> getPhoto() { 1853 if (this.photo == null) 1854 this.photo = new ArrayList<Attachment>(); 1855 return this.photo; 1856 } 1857 1858 /** 1859 * @return Returns a reference to <code>this</code> for easy method chaining 1860 */ 1861 public Patient setPhoto(List<Attachment> thePhoto) { 1862 this.photo = thePhoto; 1863 return this; 1864 } 1865 1866 public boolean hasPhoto() { 1867 if (this.photo == null) 1868 return false; 1869 for (Attachment item : this.photo) 1870 if (!item.isEmpty()) 1871 return true; 1872 return false; 1873 } 1874 1875 public Attachment addPhoto() { //3 1876 Attachment t = new Attachment(); 1877 if (this.photo == null) 1878 this.photo = new ArrayList<Attachment>(); 1879 this.photo.add(t); 1880 return t; 1881 } 1882 1883 public Patient addPhoto(Attachment t) { //3 1884 if (t == null) 1885 return this; 1886 if (this.photo == null) 1887 this.photo = new ArrayList<Attachment>(); 1888 this.photo.add(t); 1889 return this; 1890 } 1891 1892 /** 1893 * @return The first repetition of repeating field {@link #photo}, creating it if it does not already exist {3} 1894 */ 1895 public Attachment getPhotoFirstRep() { 1896 if (getPhoto().isEmpty()) { 1897 addPhoto(); 1898 } 1899 return getPhoto().get(0); 1900 } 1901 1902 /** 1903 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) for the patient.) 1904 */ 1905 public List<ContactComponent> getContact() { 1906 if (this.contact == null) 1907 this.contact = new ArrayList<ContactComponent>(); 1908 return this.contact; 1909 } 1910 1911 /** 1912 * @return Returns a reference to <code>this</code> for easy method chaining 1913 */ 1914 public Patient setContact(List<ContactComponent> theContact) { 1915 this.contact = theContact; 1916 return this; 1917 } 1918 1919 public boolean hasContact() { 1920 if (this.contact == null) 1921 return false; 1922 for (ContactComponent item : this.contact) 1923 if (!item.isEmpty()) 1924 return true; 1925 return false; 1926 } 1927 1928 public ContactComponent addContact() { //3 1929 ContactComponent t = new ContactComponent(); 1930 if (this.contact == null) 1931 this.contact = new ArrayList<ContactComponent>(); 1932 this.contact.add(t); 1933 return t; 1934 } 1935 1936 public Patient addContact(ContactComponent t) { //3 1937 if (t == null) 1938 return this; 1939 if (this.contact == null) 1940 this.contact = new ArrayList<ContactComponent>(); 1941 this.contact.add(t); 1942 return this; 1943 } 1944 1945 /** 1946 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1947 */ 1948 public ContactComponent getContactFirstRep() { 1949 if (getContact().isEmpty()) { 1950 addContact(); 1951 } 1952 return getContact().get(0); 1953 } 1954 1955 /** 1956 * @return {@link #communication} (A language which may be used to communicate with the patient about his or her health.) 1957 */ 1958 public List<PatientCommunicationComponent> getCommunication() { 1959 if (this.communication == null) 1960 this.communication = new ArrayList<PatientCommunicationComponent>(); 1961 return this.communication; 1962 } 1963 1964 /** 1965 * @return Returns a reference to <code>this</code> for easy method chaining 1966 */ 1967 public Patient setCommunication(List<PatientCommunicationComponent> theCommunication) { 1968 this.communication = theCommunication; 1969 return this; 1970 } 1971 1972 public boolean hasCommunication() { 1973 if (this.communication == null) 1974 return false; 1975 for (PatientCommunicationComponent item : this.communication) 1976 if (!item.isEmpty()) 1977 return true; 1978 return false; 1979 } 1980 1981 public PatientCommunicationComponent addCommunication() { //3 1982 PatientCommunicationComponent t = new PatientCommunicationComponent(); 1983 if (this.communication == null) 1984 this.communication = new ArrayList<PatientCommunicationComponent>(); 1985 this.communication.add(t); 1986 return t; 1987 } 1988 1989 public Patient addCommunication(PatientCommunicationComponent t) { //3 1990 if (t == null) 1991 return this; 1992 if (this.communication == null) 1993 this.communication = new ArrayList<PatientCommunicationComponent>(); 1994 this.communication.add(t); 1995 return this; 1996 } 1997 1998 /** 1999 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist {3} 2000 */ 2001 public PatientCommunicationComponent getCommunicationFirstRep() { 2002 if (getCommunication().isEmpty()) { 2003 addCommunication(); 2004 } 2005 return getCommunication().get(0); 2006 } 2007 2008 /** 2009 * @return {@link #generalPractitioner} (Patient's nominated care provider.) 2010 */ 2011 public List<Reference> getGeneralPractitioner() { 2012 if (this.generalPractitioner == null) 2013 this.generalPractitioner = new ArrayList<Reference>(); 2014 return this.generalPractitioner; 2015 } 2016 2017 /** 2018 * @return Returns a reference to <code>this</code> for easy method chaining 2019 */ 2020 public Patient setGeneralPractitioner(List<Reference> theGeneralPractitioner) { 2021 this.generalPractitioner = theGeneralPractitioner; 2022 return this; 2023 } 2024 2025 public boolean hasGeneralPractitioner() { 2026 if (this.generalPractitioner == null) 2027 return false; 2028 for (Reference item : this.generalPractitioner) 2029 if (!item.isEmpty()) 2030 return true; 2031 return false; 2032 } 2033 2034 public Reference addGeneralPractitioner() { //3 2035 Reference t = new Reference(); 2036 if (this.generalPractitioner == null) 2037 this.generalPractitioner = new ArrayList<Reference>(); 2038 this.generalPractitioner.add(t); 2039 return t; 2040 } 2041 2042 public Patient addGeneralPractitioner(Reference t) { //3 2043 if (t == null) 2044 return this; 2045 if (this.generalPractitioner == null) 2046 this.generalPractitioner = new ArrayList<Reference>(); 2047 this.generalPractitioner.add(t); 2048 return this; 2049 } 2050 2051 /** 2052 * @return The first repetition of repeating field {@link #generalPractitioner}, creating it if it does not already exist {3} 2053 */ 2054 public Reference getGeneralPractitionerFirstRep() { 2055 if (getGeneralPractitioner().isEmpty()) { 2056 addGeneralPractitioner(); 2057 } 2058 return getGeneralPractitioner().get(0); 2059 } 2060 2061 /** 2062 * @return {@link #managingOrganization} (Organization that is the custodian of the patient record.) 2063 */ 2064 public Reference getManagingOrganization() { 2065 if (this.managingOrganization == null) 2066 if (Configuration.errorOnAutoCreate()) 2067 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2068 else if (Configuration.doAutoCreate()) 2069 this.managingOrganization = new Reference(); // cc 2070 return this.managingOrganization; 2071 } 2072 2073 public boolean hasManagingOrganization() { 2074 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2075 } 2076 2077 /** 2078 * @param value {@link #managingOrganization} (Organization that is the custodian of the patient record.) 2079 */ 2080 public Patient setManagingOrganization(Reference value) { 2081 this.managingOrganization = value; 2082 return this; 2083 } 2084 2085 /** 2086 * @return {@link #link} (Link to a Patient or RelatedPerson resource that concerns the same actual individual.) 2087 */ 2088 public List<PatientLinkComponent> getLink() { 2089 if (this.link == null) 2090 this.link = new ArrayList<PatientLinkComponent>(); 2091 return this.link; 2092 } 2093 2094 /** 2095 * @return Returns a reference to <code>this</code> for easy method chaining 2096 */ 2097 public Patient setLink(List<PatientLinkComponent> theLink) { 2098 this.link = theLink; 2099 return this; 2100 } 2101 2102 public boolean hasLink() { 2103 if (this.link == null) 2104 return false; 2105 for (PatientLinkComponent item : this.link) 2106 if (!item.isEmpty()) 2107 return true; 2108 return false; 2109 } 2110 2111 public PatientLinkComponent addLink() { //3 2112 PatientLinkComponent t = new PatientLinkComponent(); 2113 if (this.link == null) 2114 this.link = new ArrayList<PatientLinkComponent>(); 2115 this.link.add(t); 2116 return t; 2117 } 2118 2119 public Patient addLink(PatientLinkComponent t) { //3 2120 if (t == null) 2121 return this; 2122 if (this.link == null) 2123 this.link = new ArrayList<PatientLinkComponent>(); 2124 this.link.add(t); 2125 return this; 2126 } 2127 2128 /** 2129 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist {3} 2130 */ 2131 public PatientLinkComponent getLinkFirstRep() { 2132 if (getLink().isEmpty()) { 2133 addLink(); 2134 } 2135 return getLink().get(0); 2136 } 2137 2138 protected void listChildren(List<Property> children) { 2139 super.listChildren(children); 2140 children.add(new Property("identifier", "Identifier", "An identifier for this patient.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2141 children.add(new Property("active", "boolean", "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 0, 1, active)); 2142 children.add(new Property("name", "HumanName", "A name associated with the individual.", 0, java.lang.Integer.MAX_VALUE, name)); 2143 children.add(new Property("telecom", "ContactPoint", "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, java.lang.Integer.MAX_VALUE, telecom)); 2144 children.add(new Property("gender", "code", "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 0, 1, gender)); 2145 children.add(new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, birthDate)); 2146 children.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased)); 2147 children.add(new Property("address", "Address", "An address for the individual.", 0, java.lang.Integer.MAX_VALUE, address)); 2148 children.add(new Property("maritalStatus", "CodeableConcept", "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus)); 2149 children.add(new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 0, 1, multipleBirth)); 2150 children.add(new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo)); 2151 children.add(new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, java.lang.Integer.MAX_VALUE, contact)); 2152 children.add(new Property("communication", "", "A language which may be used to communicate with the patient about his or her health.", 0, java.lang.Integer.MAX_VALUE, communication)); 2153 children.add(new Property("generalPractitioner", "Reference(Organization|Practitioner|PractitionerRole)", "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, generalPractitioner)); 2154 children.add(new Property("managingOrganization", "Reference(Organization)", "Organization that is the custodian of the patient record.", 0, 1, managingOrganization)); 2155 children.add(new Property("link", "", "Link to a Patient or RelatedPerson resource that concerns the same actual individual.", 0, java.lang.Integer.MAX_VALUE, link)); 2156 } 2157 2158 @Override 2159 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2160 switch (_hash) { 2161 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier for this patient.", 0, java.lang.Integer.MAX_VALUE, identifier); 2162 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 0, 1, active); 2163 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the individual.", 0, java.lang.Integer.MAX_VALUE, name); 2164 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, java.lang.Integer.MAX_VALUE, telecom); 2165 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 0, 1, gender); 2166 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, birthDate); 2167 case -1311442804: /*deceased[x]*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2168 case 561497972: /*deceased*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2169 case 497463828: /*deceasedBoolean*/ return new Property("deceased[x]", "boolean", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2170 case -1971804369: /*deceasedDateTime*/ return new Property("deceased[x]", "dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2171 case -1147692044: /*address*/ return new Property("address", "Address", "An address for the individual.", 0, java.lang.Integer.MAX_VALUE, address); 2172 case 1756919302: /*maritalStatus*/ return new Property("maritalStatus", "CodeableConcept", "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus); 2173 case -1764672111: /*multipleBirth[x]*/ return new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2174 case -677369713: /*multipleBirth*/ return new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2175 case -247534439: /*multipleBirthBoolean*/ return new Property("multipleBirth[x]", "boolean", "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2176 case 1645805999: /*multipleBirthInteger*/ return new Property("multipleBirth[x]", "integer", "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2177 case 106642994: /*photo*/ return new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo); 2178 case 951526432: /*contact*/ return new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, java.lang.Integer.MAX_VALUE, contact); 2179 case -1035284522: /*communication*/ return new Property("communication", "", "A language which may be used to communicate with the patient about his or her health.", 0, java.lang.Integer.MAX_VALUE, communication); 2180 case 1488292898: /*generalPractitioner*/ return new Property("generalPractitioner", "Reference(Organization|Practitioner|PractitionerRole)", "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, generalPractitioner); 2181 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "Organization that is the custodian of the patient record.", 0, 1, managingOrganization); 2182 case 3321850: /*link*/ return new Property("link", "", "Link to a Patient or RelatedPerson resource that concerns the same actual individual.", 0, java.lang.Integer.MAX_VALUE, link); 2183 default: return super.getNamedProperty(_hash, _name, _checkValid); 2184 } 2185 2186 } 2187 2188 @Override 2189 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2190 switch (hash) { 2191 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2192 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 2193 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 2194 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2195 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 2196 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 2197 case 561497972: /*deceased*/ return this.deceased == null ? new Base[0] : new Base[] {this.deceased}; // DataType 2198 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 2199 case 1756919302: /*maritalStatus*/ return this.maritalStatus == null ? new Base[0] : new Base[] {this.maritalStatus}; // CodeableConcept 2200 case -677369713: /*multipleBirth*/ return this.multipleBirth == null ? new Base[0] : new Base[] {this.multipleBirth}; // DataType 2201 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 2202 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactComponent 2203 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // PatientCommunicationComponent 2204 case 1488292898: /*generalPractitioner*/ return this.generalPractitioner == null ? new Base[0] : this.generalPractitioner.toArray(new Base[this.generalPractitioner.size()]); // Reference 2205 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 2206 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PatientLinkComponent 2207 default: return super.getProperty(hash, name, checkValid); 2208 } 2209 2210 } 2211 2212 @Override 2213 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2214 switch (hash) { 2215 case -1618432855: // identifier 2216 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2217 return value; 2218 case -1422950650: // active 2219 this.active = TypeConvertor.castToBoolean(value); // BooleanType 2220 return value; 2221 case 3373707: // name 2222 this.getName().add(TypeConvertor.castToHumanName(value)); // HumanName 2223 return value; 2224 case -1429363305: // telecom 2225 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 2226 return value; 2227 case -1249512767: // gender 2228 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 2229 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2230 return value; 2231 case -1210031859: // birthDate 2232 this.birthDate = TypeConvertor.castToDate(value); // DateType 2233 return value; 2234 case 561497972: // deceased 2235 this.deceased = TypeConvertor.castToType(value); // DataType 2236 return value; 2237 case -1147692044: // address 2238 this.getAddress().add(TypeConvertor.castToAddress(value)); // Address 2239 return value; 2240 case 1756919302: // maritalStatus 2241 this.maritalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2242 return value; 2243 case -677369713: // multipleBirth 2244 this.multipleBirth = TypeConvertor.castToType(value); // DataType 2245 return value; 2246 case 106642994: // photo 2247 this.getPhoto().add(TypeConvertor.castToAttachment(value)); // Attachment 2248 return value; 2249 case 951526432: // contact 2250 this.getContact().add((ContactComponent) value); // ContactComponent 2251 return value; 2252 case -1035284522: // communication 2253 this.getCommunication().add((PatientCommunicationComponent) value); // PatientCommunicationComponent 2254 return value; 2255 case 1488292898: // generalPractitioner 2256 this.getGeneralPractitioner().add(TypeConvertor.castToReference(value)); // Reference 2257 return value; 2258 case -2058947787: // managingOrganization 2259 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 2260 return value; 2261 case 3321850: // link 2262 this.getLink().add((PatientLinkComponent) value); // PatientLinkComponent 2263 return value; 2264 default: return super.setProperty(hash, name, value); 2265 } 2266 2267 } 2268 2269 @Override 2270 public Base setProperty(String name, Base value) throws FHIRException { 2271 if (name.equals("identifier")) { 2272 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2273 } else if (name.equals("active")) { 2274 this.active = TypeConvertor.castToBoolean(value); // BooleanType 2275 } else if (name.equals("name")) { 2276 this.getName().add(TypeConvertor.castToHumanName(value)); 2277 } else if (name.equals("telecom")) { 2278 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 2279 } else if (name.equals("gender")) { 2280 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 2281 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2282 } else if (name.equals("birthDate")) { 2283 this.birthDate = TypeConvertor.castToDate(value); // DateType 2284 } else if (name.equals("deceased[x]")) { 2285 this.deceased = TypeConvertor.castToType(value); // DataType 2286 } else if (name.equals("address")) { 2287 this.getAddress().add(TypeConvertor.castToAddress(value)); 2288 } else if (name.equals("maritalStatus")) { 2289 this.maritalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2290 } else if (name.equals("multipleBirth[x]")) { 2291 this.multipleBirth = TypeConvertor.castToType(value); // DataType 2292 } else if (name.equals("photo")) { 2293 this.getPhoto().add(TypeConvertor.castToAttachment(value)); 2294 } else if (name.equals("contact")) { 2295 this.getContact().add((ContactComponent) value); 2296 } else if (name.equals("communication")) { 2297 this.getCommunication().add((PatientCommunicationComponent) value); 2298 } else if (name.equals("generalPractitioner")) { 2299 this.getGeneralPractitioner().add(TypeConvertor.castToReference(value)); 2300 } else if (name.equals("managingOrganization")) { 2301 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 2302 } else if (name.equals("link")) { 2303 this.getLink().add((PatientLinkComponent) value); 2304 } else 2305 return super.setProperty(name, value); 2306 return value; 2307 } 2308 2309 @Override 2310 public void removeChild(String name, Base value) throws FHIRException { 2311 if (name.equals("identifier")) { 2312 this.getIdentifier().remove(value); 2313 } else if (name.equals("active")) { 2314 this.active = null; 2315 } else if (name.equals("name")) { 2316 this.getName().remove(value); 2317 } else if (name.equals("telecom")) { 2318 this.getTelecom().remove(value); 2319 } else if (name.equals("gender")) { 2320 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 2321 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2322 } else if (name.equals("birthDate")) { 2323 this.birthDate = null; 2324 } else if (name.equals("deceased[x]")) { 2325 this.deceased = null; 2326 } else if (name.equals("address")) { 2327 this.getAddress().remove(value); 2328 } else if (name.equals("maritalStatus")) { 2329 this.maritalStatus = null; 2330 } else if (name.equals("multipleBirth[x]")) { 2331 this.multipleBirth = null; 2332 } else if (name.equals("photo")) { 2333 this.getPhoto().remove(value); 2334 } else if (name.equals("contact")) { 2335 this.getContact().remove((ContactComponent) value); 2336 } else if (name.equals("communication")) { 2337 this.getCommunication().remove((PatientCommunicationComponent) value); 2338 } else if (name.equals("generalPractitioner")) { 2339 this.getGeneralPractitioner().remove(value); 2340 } else if (name.equals("managingOrganization")) { 2341 this.managingOrganization = null; 2342 } else if (name.equals("link")) { 2343 this.getLink().remove((PatientLinkComponent) value); 2344 } else 2345 super.removeChild(name, value); 2346 2347 } 2348 2349 @Override 2350 public Base makeProperty(int hash, String name) throws FHIRException { 2351 switch (hash) { 2352 case -1618432855: return addIdentifier(); 2353 case -1422950650: return getActiveElement(); 2354 case 3373707: return addName(); 2355 case -1429363305: return addTelecom(); 2356 case -1249512767: return getGenderElement(); 2357 case -1210031859: return getBirthDateElement(); 2358 case -1311442804: return getDeceased(); 2359 case 561497972: return getDeceased(); 2360 case -1147692044: return addAddress(); 2361 case 1756919302: return getMaritalStatus(); 2362 case -1764672111: return getMultipleBirth(); 2363 case -677369713: return getMultipleBirth(); 2364 case 106642994: return addPhoto(); 2365 case 951526432: return addContact(); 2366 case -1035284522: return addCommunication(); 2367 case 1488292898: return addGeneralPractitioner(); 2368 case -2058947787: return getManagingOrganization(); 2369 case 3321850: return addLink(); 2370 default: return super.makeProperty(hash, name); 2371 } 2372 2373 } 2374 2375 @Override 2376 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2377 switch (hash) { 2378 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2379 case -1422950650: /*active*/ return new String[] {"boolean"}; 2380 case 3373707: /*name*/ return new String[] {"HumanName"}; 2381 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 2382 case -1249512767: /*gender*/ return new String[] {"code"}; 2383 case -1210031859: /*birthDate*/ return new String[] {"date"}; 2384 case 561497972: /*deceased*/ return new String[] {"boolean", "dateTime"}; 2385 case -1147692044: /*address*/ return new String[] {"Address"}; 2386 case 1756919302: /*maritalStatus*/ return new String[] {"CodeableConcept"}; 2387 case -677369713: /*multipleBirth*/ return new String[] {"boolean", "integer"}; 2388 case 106642994: /*photo*/ return new String[] {"Attachment"}; 2389 case 951526432: /*contact*/ return new String[] {}; 2390 case -1035284522: /*communication*/ return new String[] {}; 2391 case 1488292898: /*generalPractitioner*/ return new String[] {"Reference"}; 2392 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 2393 case 3321850: /*link*/ return new String[] {}; 2394 default: return super.getTypesForProperty(hash, name); 2395 } 2396 2397 } 2398 2399 @Override 2400 public Base addChild(String name) throws FHIRException { 2401 if (name.equals("identifier")) { 2402 return addIdentifier(); 2403 } 2404 else if (name.equals("active")) { 2405 throw new FHIRException("Cannot call addChild on a singleton property Patient.active"); 2406 } 2407 else if (name.equals("name")) { 2408 return addName(); 2409 } 2410 else if (name.equals("telecom")) { 2411 return addTelecom(); 2412 } 2413 else if (name.equals("gender")) { 2414 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 2415 } 2416 else if (name.equals("birthDate")) { 2417 throw new FHIRException("Cannot call addChild on a singleton property Patient.birthDate"); 2418 } 2419 else if (name.equals("deceasedBoolean")) { 2420 this.deceased = new BooleanType(); 2421 return this.deceased; 2422 } 2423 else if (name.equals("deceasedDateTime")) { 2424 this.deceased = new DateTimeType(); 2425 return this.deceased; 2426 } 2427 else if (name.equals("address")) { 2428 return addAddress(); 2429 } 2430 else if (name.equals("maritalStatus")) { 2431 this.maritalStatus = new CodeableConcept(); 2432 return this.maritalStatus; 2433 } 2434 else if (name.equals("multipleBirthBoolean")) { 2435 this.multipleBirth = new BooleanType(); 2436 return this.multipleBirth; 2437 } 2438 else if (name.equals("multipleBirthInteger")) { 2439 this.multipleBirth = new IntegerType(); 2440 return this.multipleBirth; 2441 } 2442 else if (name.equals("photo")) { 2443 return addPhoto(); 2444 } 2445 else if (name.equals("contact")) { 2446 return addContact(); 2447 } 2448 else if (name.equals("communication")) { 2449 return addCommunication(); 2450 } 2451 else if (name.equals("generalPractitioner")) { 2452 return addGeneralPractitioner(); 2453 } 2454 else if (name.equals("managingOrganization")) { 2455 this.managingOrganization = new Reference(); 2456 return this.managingOrganization; 2457 } 2458 else if (name.equals("link")) { 2459 return addLink(); 2460 } 2461 else 2462 return super.addChild(name); 2463 } 2464 2465 public String fhirType() { 2466 return "Patient"; 2467 2468 } 2469 2470 public Patient copy() { 2471 Patient dst = new Patient(); 2472 copyValues(dst); 2473 return dst; 2474 } 2475 2476 public void copyValues(Patient dst) { 2477 super.copyValues(dst); 2478 if (identifier != null) { 2479 dst.identifier = new ArrayList<Identifier>(); 2480 for (Identifier i : identifier) 2481 dst.identifier.add(i.copy()); 2482 }; 2483 dst.active = active == null ? null : active.copy(); 2484 if (name != null) { 2485 dst.name = new ArrayList<HumanName>(); 2486 for (HumanName i : name) 2487 dst.name.add(i.copy()); 2488 }; 2489 if (telecom != null) { 2490 dst.telecom = new ArrayList<ContactPoint>(); 2491 for (ContactPoint i : telecom) 2492 dst.telecom.add(i.copy()); 2493 }; 2494 dst.gender = gender == null ? null : gender.copy(); 2495 dst.birthDate = birthDate == null ? null : birthDate.copy(); 2496 dst.deceased = deceased == null ? null : deceased.copy(); 2497 if (address != null) { 2498 dst.address = new ArrayList<Address>(); 2499 for (Address i : address) 2500 dst.address.add(i.copy()); 2501 }; 2502 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 2503 dst.multipleBirth = multipleBirth == null ? null : multipleBirth.copy(); 2504 if (photo != null) { 2505 dst.photo = new ArrayList<Attachment>(); 2506 for (Attachment i : photo) 2507 dst.photo.add(i.copy()); 2508 }; 2509 if (contact != null) { 2510 dst.contact = new ArrayList<ContactComponent>(); 2511 for (ContactComponent i : contact) 2512 dst.contact.add(i.copy()); 2513 }; 2514 if (communication != null) { 2515 dst.communication = new ArrayList<PatientCommunicationComponent>(); 2516 for (PatientCommunicationComponent i : communication) 2517 dst.communication.add(i.copy()); 2518 }; 2519 if (generalPractitioner != null) { 2520 dst.generalPractitioner = new ArrayList<Reference>(); 2521 for (Reference i : generalPractitioner) 2522 dst.generalPractitioner.add(i.copy()); 2523 }; 2524 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 2525 if (link != null) { 2526 dst.link = new ArrayList<PatientLinkComponent>(); 2527 for (PatientLinkComponent i : link) 2528 dst.link.add(i.copy()); 2529 }; 2530 } 2531 2532 protected Patient typedCopy() { 2533 return copy(); 2534 } 2535 2536 @Override 2537 public boolean equalsDeep(Base other_) { 2538 if (!super.equalsDeep(other_)) 2539 return false; 2540 if (!(other_ instanceof Patient)) 2541 return false; 2542 Patient o = (Patient) other_; 2543 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(name, o.name, true) 2544 && compareDeep(telecom, o.telecom, true) && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 2545 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) && compareDeep(maritalStatus, o.maritalStatus, true) 2546 && compareDeep(multipleBirth, o.multipleBirth, true) && compareDeep(photo, o.photo, true) && compareDeep(contact, o.contact, true) 2547 && compareDeep(communication, o.communication, true) && compareDeep(generalPractitioner, o.generalPractitioner, true) 2548 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(link, o.link, true) 2549 ; 2550 } 2551 2552 @Override 2553 public boolean equalsShallow(Base other_) { 2554 if (!super.equalsShallow(other_)) 2555 return false; 2556 if (!(other_ instanceof Patient)) 2557 return false; 2558 Patient o = (Patient) other_; 2559 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 2560 ; 2561 } 2562 2563 public boolean isEmpty() { 2564 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name 2565 , telecom, gender, birthDate, deceased, address, maritalStatus, multipleBirth 2566 , photo, contact, communication, generalPractitioner, managingOrganization, link 2567 ); 2568 } 2569 2570 @Override 2571 public ResourceType getResourceType() { 2572 return ResourceType.Patient; 2573 } 2574 2575 /** 2576 * Search parameter: <b>active</b> 2577 * <p> 2578 * Description: <b>Whether the patient record is active</b><br> 2579 * Type: <b>token</b><br> 2580 * Path: <b>Patient.active</b><br> 2581 * </p> 2582 */ 2583 @SearchParamDefinition(name="active", path="Patient.active", description="Whether the patient record is active", type="token" ) 2584 public static final String SP_ACTIVE = "active"; 2585 /** 2586 * <b>Fluent Client</b> search parameter constant for <b>active</b> 2587 * <p> 2588 * Description: <b>Whether the patient record is active</b><br> 2589 * Type: <b>token</b><br> 2590 * Path: <b>Patient.active</b><br> 2591 * </p> 2592 */ 2593 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 2594 2595 /** 2596 * Search parameter: <b>death-date</b> 2597 * <p> 2598 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 2599 * Type: <b>date</b><br> 2600 * Path: <b>(Patient.deceased.ofType(dateTime))</b><br> 2601 * </p> 2602 */ 2603 @SearchParamDefinition(name="death-date", path="(Patient.deceased.ofType(dateTime))", description="The date of death has been provided and satisfies this search value", type="date" ) 2604 public static final String SP_DEATH_DATE = "death-date"; 2605 /** 2606 * <b>Fluent Client</b> search parameter constant for <b>death-date</b> 2607 * <p> 2608 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 2609 * Type: <b>date</b><br> 2610 * Path: <b>(Patient.deceased.ofType(dateTime))</b><br> 2611 * </p> 2612 */ 2613 public static final ca.uhn.fhir.rest.gclient.DateClientParam DEATH_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DEATH_DATE); 2614 2615 /** 2616 * Search parameter: <b>deceased</b> 2617 * <p> 2618 * Description: <b>This patient has been marked as deceased, or has a death date entered</b><br> 2619 * Type: <b>token</b><br> 2620 * Path: <b>Patient.deceased.exists() and Patient.deceased != false</b><br> 2621 * </p> 2622 */ 2623 @SearchParamDefinition(name="deceased", path="Patient.deceased.exists() and Patient.deceased != false", description="This patient has been marked as deceased, or has a death date entered", type="token" ) 2624 public static final String SP_DECEASED = "deceased"; 2625 /** 2626 * <b>Fluent Client</b> search parameter constant for <b>deceased</b> 2627 * <p> 2628 * Description: <b>This patient has been marked as deceased, or has a death date entered</b><br> 2629 * Type: <b>token</b><br> 2630 * Path: <b>Patient.deceased.exists() and Patient.deceased != false</b><br> 2631 * </p> 2632 */ 2633 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DECEASED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DECEASED); 2634 2635 /** 2636 * Search parameter: <b>general-practitioner</b> 2637 * <p> 2638 * Description: <b>Patient's nominated general practitioner, not the organization that manages the record</b><br> 2639 * Type: <b>reference</b><br> 2640 * Path: <b>Patient.generalPractitioner</b><br> 2641 * </p> 2642 */ 2643 @SearchParamDefinition(name="general-practitioner", path="Patient.generalPractitioner", description="Patient's nominated general practitioner, not the organization that manages the record", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 2644 public static final String SP_GENERAL_PRACTITIONER = "general-practitioner"; 2645 /** 2646 * <b>Fluent Client</b> search parameter constant for <b>general-practitioner</b> 2647 * <p> 2648 * Description: <b>Patient's nominated general practitioner, not the organization that manages the record</b><br> 2649 * Type: <b>reference</b><br> 2650 * Path: <b>Patient.generalPractitioner</b><br> 2651 * </p> 2652 */ 2653 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GENERAL_PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GENERAL_PRACTITIONER); 2654 2655/** 2656 * Constant for fluent queries to be used to add include statements. Specifies 2657 * the path value of "<b>Patient:general-practitioner</b>". 2658 */ 2659 public static final ca.uhn.fhir.model.api.Include INCLUDE_GENERAL_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Patient:general-practitioner").toLocked(); 2660 2661 /** 2662 * Search parameter: <b>identifier</b> 2663 * <p> 2664 * Description: <b>A patient identifier</b><br> 2665 * Type: <b>token</b><br> 2666 * Path: <b>Patient.identifier</b><br> 2667 * </p> 2668 */ 2669 @SearchParamDefinition(name="identifier", path="Patient.identifier", description="A patient identifier", type="token" ) 2670 public static final String SP_IDENTIFIER = "identifier"; 2671 /** 2672 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2673 * <p> 2674 * Description: <b>A patient identifier</b><br> 2675 * Type: <b>token</b><br> 2676 * Path: <b>Patient.identifier</b><br> 2677 * </p> 2678 */ 2679 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2680 2681 /** 2682 * Search parameter: <b>language</b> 2683 * <p> 2684 * Description: <b>Language code (irrespective of use value)</b><br> 2685 * Type: <b>token</b><br> 2686 * Path: <b>Patient.communication.language</b><br> 2687 * </p> 2688 */ 2689 @SearchParamDefinition(name="language", path="Patient.communication.language", description="Language code (irrespective of use value)", type="token" ) 2690 public static final String SP_LANGUAGE = "language"; 2691 /** 2692 * <b>Fluent Client</b> search parameter constant for <b>language</b> 2693 * <p> 2694 * Description: <b>Language code (irrespective of use value)</b><br> 2695 * Type: <b>token</b><br> 2696 * Path: <b>Patient.communication.language</b><br> 2697 * </p> 2698 */ 2699 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LANGUAGE); 2700 2701 /** 2702 * Search parameter: <b>link</b> 2703 * <p> 2704 * Description: <b>All patients/related persons linked to the given patient</b><br> 2705 * Type: <b>reference</b><br> 2706 * Path: <b>Patient.link.other</b><br> 2707 * </p> 2708 */ 2709 @SearchParamDefinition(name="link", path="Patient.link.other", description="All patients/related persons linked to the given patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Patient.class, RelatedPerson.class } ) 2710 public static final String SP_LINK = "link"; 2711 /** 2712 * <b>Fluent Client</b> search parameter constant for <b>link</b> 2713 * <p> 2714 * Description: <b>All patients/related persons linked to the given patient</b><br> 2715 * Type: <b>reference</b><br> 2716 * Path: <b>Patient.link.other</b><br> 2717 * </p> 2718 */ 2719 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LINK); 2720 2721/** 2722 * Constant for fluent queries to be used to add include statements. Specifies 2723 * the path value of "<b>Patient:link</b>". 2724 */ 2725 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Patient:link").toLocked(); 2726 2727 /** 2728 * Search parameter: <b>name</b> 2729 * <p> 2730 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, given, prefix, suffix, and/or text</b><br> 2731 * Type: <b>string</b><br> 2732 * Path: <b>Patient.name</b><br> 2733 * </p> 2734 */ 2735 @SearchParamDefinition(name="name", path="Patient.name", description="A server defined search that may match any of the string fields in the HumanName, including family, given, prefix, suffix, and/or text", type="string" ) 2736 public static final String SP_NAME = "name"; 2737 /** 2738 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2739 * <p> 2740 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, given, prefix, suffix, and/or text</b><br> 2741 * Type: <b>string</b><br> 2742 * Path: <b>Patient.name</b><br> 2743 * </p> 2744 */ 2745 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2746 2747 /** 2748 * Search parameter: <b>organization</b> 2749 * <p> 2750 * Description: <b>The organization that is the custodian of the patient record</b><br> 2751 * Type: <b>reference</b><br> 2752 * Path: <b>Patient.managingOrganization</b><br> 2753 * </p> 2754 */ 2755 @SearchParamDefinition(name="organization", path="Patient.managingOrganization", description="The organization that is the custodian of the patient record", type="reference", target={Organization.class } ) 2756 public static final String SP_ORGANIZATION = "organization"; 2757 /** 2758 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2759 * <p> 2760 * Description: <b>The organization that is the custodian of the patient record</b><br> 2761 * Type: <b>reference</b><br> 2762 * Path: <b>Patient.managingOrganization</b><br> 2763 * </p> 2764 */ 2765 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2766 2767/** 2768 * Constant for fluent queries to be used to add include statements. Specifies 2769 * the path value of "<b>Patient:organization</b>". 2770 */ 2771 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Patient:organization").toLocked(); 2772 2773 /** 2774 * Search parameter: <b>part-agree</b> 2775 * <p> 2776 * Description: <b>Search by url for a participation agreement, which is stored as an extension referencing a DocumentReference</b><br> 2777 * Type: <b>reference</b><br> 2778 * Path: <b>Patient.extension('http://example.org/fhir/StructureDefinition/participation-agreement').value</b><br> 2779 * </p> 2780 */ 2781 @SearchParamDefinition(name="part-agree", path="Patient.extension('http://example.org/fhir/StructureDefinition/participation-agreement').value", description="Search by url for a participation agreement, which is stored as an extension referencing a DocumentReference", type="reference", target={DocumentReference.class } ) 2782 public static final String SP_PART_AGREE = "part-agree"; 2783 /** 2784 * <b>Fluent Client</b> search parameter constant for <b>part-agree</b> 2785 * <p> 2786 * Description: <b>Search by url for a participation agreement, which is stored as an extension referencing a DocumentReference</b><br> 2787 * Type: <b>reference</b><br> 2788 * Path: <b>Patient.extension('http://example.org/fhir/StructureDefinition/participation-agreement').value</b><br> 2789 * </p> 2790 */ 2791 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_AGREE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_AGREE); 2792 2793/** 2794 * Constant for fluent queries to be used to add include statements. Specifies 2795 * the path value of "<b>Patient:part-agree</b>". 2796 */ 2797 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_AGREE = new ca.uhn.fhir.model.api.Include("Patient:part-agree").toLocked(); 2798 2799 /** 2800 * Search parameter: <b>address-city</b> 2801 * <p> 2802 * Description: <b>Multiple Resources: 2803 2804* [Patient](patient.html): A city specified in an address 2805* [Person](person.html): A city specified in an address 2806* [Practitioner](practitioner.html): A city specified in an address 2807* [RelatedPerson](relatedperson.html): A city specified in an address 2808</b><br> 2809 * Type: <b>string</b><br> 2810 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 2811 * </p> 2812 */ 2813 @SearchParamDefinition(name="address-city", path="Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A city specified in an address\r\n* [Person](person.html): A city specified in an address\r\n* [Practitioner](practitioner.html): A city specified in an address\r\n* [RelatedPerson](relatedperson.html): A city specified in an address\r\n", type="string" ) 2814 public static final String SP_ADDRESS_CITY = "address-city"; 2815 /** 2816 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 2817 * <p> 2818 * Description: <b>Multiple Resources: 2819 2820* [Patient](patient.html): A city specified in an address 2821* [Person](person.html): A city specified in an address 2822* [Practitioner](practitioner.html): A city specified in an address 2823* [RelatedPerson](relatedperson.html): A city specified in an address 2824</b><br> 2825 * Type: <b>string</b><br> 2826 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 2827 * </p> 2828 */ 2829 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 2830 2831 /** 2832 * Search parameter: <b>address-country</b> 2833 * <p> 2834 * Description: <b>Multiple Resources: 2835 2836* [Patient](patient.html): A country specified in an address 2837* [Person](person.html): A country specified in an address 2838* [Practitioner](practitioner.html): A country specified in an address 2839* [RelatedPerson](relatedperson.html): A country specified in an address 2840</b><br> 2841 * Type: <b>string</b><br> 2842 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 2843 * </p> 2844 */ 2845 @SearchParamDefinition(name="address-country", path="Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A country specified in an address\r\n* [Person](person.html): A country specified in an address\r\n* [Practitioner](practitioner.html): A country specified in an address\r\n* [RelatedPerson](relatedperson.html): A country specified in an address\r\n", type="string" ) 2846 public static final String SP_ADDRESS_COUNTRY = "address-country"; 2847 /** 2848 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 2849 * <p> 2850 * Description: <b>Multiple Resources: 2851 2852* [Patient](patient.html): A country specified in an address 2853* [Person](person.html): A country specified in an address 2854* [Practitioner](practitioner.html): A country specified in an address 2855* [RelatedPerson](relatedperson.html): A country specified in an address 2856</b><br> 2857 * Type: <b>string</b><br> 2858 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 2859 * </p> 2860 */ 2861 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 2862 2863 /** 2864 * Search parameter: <b>address-postalcode</b> 2865 * <p> 2866 * Description: <b>Multiple Resources: 2867 2868* [Patient](patient.html): A postalCode specified in an address 2869* [Person](person.html): A postal code specified in an address 2870* [Practitioner](practitioner.html): A postalCode specified in an address 2871* [RelatedPerson](relatedperson.html): A postal code specified in an address 2872</b><br> 2873 * Type: <b>string</b><br> 2874 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 2875 * </p> 2876 */ 2877 @SearchParamDefinition(name="address-postalcode", path="Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A postalCode specified in an address\r\n* [Person](person.html): A postal code specified in an address\r\n* [Practitioner](practitioner.html): A postalCode specified in an address\r\n* [RelatedPerson](relatedperson.html): A postal code specified in an address\r\n", type="string" ) 2878 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 2879 /** 2880 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 2881 * <p> 2882 * Description: <b>Multiple Resources: 2883 2884* [Patient](patient.html): A postalCode specified in an address 2885* [Person](person.html): A postal code specified in an address 2886* [Practitioner](practitioner.html): A postalCode specified in an address 2887* [RelatedPerson](relatedperson.html): A postal code specified in an address 2888</b><br> 2889 * Type: <b>string</b><br> 2890 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 2891 * </p> 2892 */ 2893 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 2894 2895 /** 2896 * Search parameter: <b>address-state</b> 2897 * <p> 2898 * Description: <b>Multiple Resources: 2899 2900* [Patient](patient.html): A state specified in an address 2901* [Person](person.html): A state specified in an address 2902* [Practitioner](practitioner.html): A state specified in an address 2903* [RelatedPerson](relatedperson.html): A state specified in an address 2904</b><br> 2905 * Type: <b>string</b><br> 2906 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 2907 * </p> 2908 */ 2909 @SearchParamDefinition(name="address-state", path="Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A state specified in an address\r\n* [Person](person.html): A state specified in an address\r\n* [Practitioner](practitioner.html): A state specified in an address\r\n* [RelatedPerson](relatedperson.html): A state specified in an address\r\n", type="string" ) 2910 public static final String SP_ADDRESS_STATE = "address-state"; 2911 /** 2912 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 2913 * <p> 2914 * Description: <b>Multiple Resources: 2915 2916* [Patient](patient.html): A state specified in an address 2917* [Person](person.html): A state specified in an address 2918* [Practitioner](practitioner.html): A state specified in an address 2919* [RelatedPerson](relatedperson.html): A state specified in an address 2920</b><br> 2921 * Type: <b>string</b><br> 2922 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 2923 * </p> 2924 */ 2925 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 2926 2927 /** 2928 * Search parameter: <b>address-use</b> 2929 * <p> 2930 * Description: <b>Multiple Resources: 2931 2932* [Patient](patient.html): A use code specified in an address 2933* [Person](person.html): A use code specified in an address 2934* [Practitioner](practitioner.html): A use code specified in an address 2935* [RelatedPerson](relatedperson.html): A use code specified in an address 2936</b><br> 2937 * Type: <b>token</b><br> 2938 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 2939 * </p> 2940 */ 2941 @SearchParamDefinition(name="address-use", path="Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A use code specified in an address\r\n* [Person](person.html): A use code specified in an address\r\n* [Practitioner](practitioner.html): A use code specified in an address\r\n* [RelatedPerson](relatedperson.html): A use code specified in an address\r\n", type="token" ) 2942 public static final String SP_ADDRESS_USE = "address-use"; 2943 /** 2944 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 2945 * <p> 2946 * Description: <b>Multiple Resources: 2947 2948* [Patient](patient.html): A use code specified in an address 2949* [Person](person.html): A use code specified in an address 2950* [Practitioner](practitioner.html): A use code specified in an address 2951* [RelatedPerson](relatedperson.html): A use code specified in an address 2952</b><br> 2953 * Type: <b>token</b><br> 2954 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 2955 * </p> 2956 */ 2957 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 2958 2959 /** 2960 * Search parameter: <b>address</b> 2961 * <p> 2962 * Description: <b>Multiple Resources: 2963 2964* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2965* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2966* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2967* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2968</b><br> 2969 * Type: <b>string</b><br> 2970 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 2971 * </p> 2972 */ 2973 @SearchParamDefinition(name="address", path="Patient.address | Person.address | Practitioner.address | RelatedPerson.address", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n", type="string" ) 2974 public static final String SP_ADDRESS = "address"; 2975 /** 2976 * <b>Fluent Client</b> search parameter constant for <b>address</b> 2977 * <p> 2978 * Description: <b>Multiple Resources: 2979 2980* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2981* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2982* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2983* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2984</b><br> 2985 * Type: <b>string</b><br> 2986 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 2987 * </p> 2988 */ 2989 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 2990 2991 /** 2992 * Search parameter: <b>birthdate</b> 2993 * <p> 2994 * Description: <b>Multiple Resources: 2995 2996* [Patient](patient.html): The patient's date of birth 2997* [Person](person.html): The person's date of birth 2998* [RelatedPerson](relatedperson.html): The Related Person's date of birth 2999</b><br> 3000 * Type: <b>date</b><br> 3001 * Path: <b>Patient.birthDate | Person.birthDate | RelatedPerson.birthDate</b><br> 3002 * </p> 3003 */ 3004 @SearchParamDefinition(name="birthdate", path="Patient.birthDate | Person.birthDate | RelatedPerson.birthDate", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The patient's date of birth\r\n* [Person](person.html): The person's date of birth\r\n* [RelatedPerson](relatedperson.html): The Related Person's date of birth\r\n", type="date" ) 3005 public static final String SP_BIRTHDATE = "birthdate"; 3006 /** 3007 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 3008 * <p> 3009 * Description: <b>Multiple Resources: 3010 3011* [Patient](patient.html): The patient's date of birth 3012* [Person](person.html): The person's date of birth 3013* [RelatedPerson](relatedperson.html): The Related Person's date of birth 3014</b><br> 3015 * Type: <b>date</b><br> 3016 * Path: <b>Patient.birthDate | Person.birthDate | RelatedPerson.birthDate</b><br> 3017 * </p> 3018 */ 3019 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_BIRTHDATE); 3020 3021 /** 3022 * Search parameter: <b>email</b> 3023 * <p> 3024 * Description: <b>Multiple Resources: 3025 3026* [Patient](patient.html): A value in an email contact 3027* [Person](person.html): A value in an email contact 3028* [Practitioner](practitioner.html): A value in an email contact 3029* [PractitionerRole](practitionerrole.html): A value in an email contact 3030* [RelatedPerson](relatedperson.html): A value in an email contact 3031</b><br> 3032 * Type: <b>token</b><br> 3033 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 3034 * </p> 3035 */ 3036 @SearchParamDefinition(name="email", path="Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in an email contact\r\n* [Person](person.html): A value in an email contact\r\n* [Practitioner](practitioner.html): A value in an email contact\r\n* [PractitionerRole](practitionerrole.html): A value in an email contact\r\n* [RelatedPerson](relatedperson.html): A value in an email contact\r\n", type="token" ) 3037 public static final String SP_EMAIL = "email"; 3038 /** 3039 * <b>Fluent Client</b> search parameter constant for <b>email</b> 3040 * <p> 3041 * Description: <b>Multiple Resources: 3042 3043* [Patient](patient.html): A value in an email contact 3044* [Person](person.html): A value in an email contact 3045* [Practitioner](practitioner.html): A value in an email contact 3046* [PractitionerRole](practitionerrole.html): A value in an email contact 3047* [RelatedPerson](relatedperson.html): A value in an email contact 3048</b><br> 3049 * Type: <b>token</b><br> 3050 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 3051 * </p> 3052 */ 3053 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 3054 3055 /** 3056 * Search parameter: <b>family</b> 3057 * <p> 3058 * Description: <b>Multiple Resources: 3059 3060* [Patient](patient.html): A portion of the family name of the patient 3061* [Practitioner](practitioner.html): A portion of the family name 3062</b><br> 3063 * Type: <b>string</b><br> 3064 * Path: <b>Patient.name.family | Practitioner.name.family</b><br> 3065 * </p> 3066 */ 3067 @SearchParamDefinition(name="family", path="Patient.name.family | Practitioner.name.family", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of the family name of the patient\r\n* [Practitioner](practitioner.html): A portion of the family name\r\n", type="string" ) 3068 public static final String SP_FAMILY = "family"; 3069 /** 3070 * <b>Fluent Client</b> search parameter constant for <b>family</b> 3071 * <p> 3072 * Description: <b>Multiple Resources: 3073 3074* [Patient](patient.html): A portion of the family name of the patient 3075* [Practitioner](practitioner.html): A portion of the family name 3076</b><br> 3077 * Type: <b>string</b><br> 3078 * Path: <b>Patient.name.family | Practitioner.name.family</b><br> 3079 * </p> 3080 */ 3081 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_FAMILY); 3082 3083 /** 3084 * Search parameter: <b>gender</b> 3085 * <p> 3086 * Description: <b>Multiple Resources: 3087 3088* [Patient](patient.html): Gender of the patient 3089* [Person](person.html): The gender of the person 3090* [Practitioner](practitioner.html): Gender of the practitioner 3091* [RelatedPerson](relatedperson.html): Gender of the related person 3092</b><br> 3093 * Type: <b>token</b><br> 3094 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 3095 * </p> 3096 */ 3097 @SearchParamDefinition(name="gender", path="Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): Gender of the patient\r\n* [Person](person.html): The gender of the person\r\n* [Practitioner](practitioner.html): Gender of the practitioner\r\n* [RelatedPerson](relatedperson.html): Gender of the related person\r\n", type="token" ) 3098 public static final String SP_GENDER = "gender"; 3099 /** 3100 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 3101 * <p> 3102 * Description: <b>Multiple Resources: 3103 3104* [Patient](patient.html): Gender of the patient 3105* [Person](person.html): The gender of the person 3106* [Practitioner](practitioner.html): Gender of the practitioner 3107* [RelatedPerson](relatedperson.html): Gender of the related person 3108</b><br> 3109 * Type: <b>token</b><br> 3110 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 3111 * </p> 3112 */ 3113 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 3114 3115 /** 3116 * Search parameter: <b>given</b> 3117 * <p> 3118 * Description: <b>Multiple Resources: 3119 3120* [Patient](patient.html): A portion of the given name of the patient 3121* [Practitioner](practitioner.html): A portion of the given name 3122</b><br> 3123 * Type: <b>string</b><br> 3124 * Path: <b>Patient.name.given | Practitioner.name.given</b><br> 3125 * </p> 3126 */ 3127 @SearchParamDefinition(name="given", path="Patient.name.given | Practitioner.name.given", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of the given name of the patient\r\n* [Practitioner](practitioner.html): A portion of the given name\r\n", type="string" ) 3128 public static final String SP_GIVEN = "given"; 3129 /** 3130 * <b>Fluent Client</b> search parameter constant for <b>given</b> 3131 * <p> 3132 * Description: <b>Multiple Resources: 3133 3134* [Patient](patient.html): A portion of the given name of the patient 3135* [Practitioner](practitioner.html): A portion of the given name 3136</b><br> 3137 * Type: <b>string</b><br> 3138 * Path: <b>Patient.name.given | Practitioner.name.given</b><br> 3139 * </p> 3140 */ 3141 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GIVEN); 3142 3143 /** 3144 * Search parameter: <b>phone</b> 3145 * <p> 3146 * Description: <b>Multiple Resources: 3147 3148* [Patient](patient.html): A value in a phone contact 3149* [Person](person.html): A value in a phone contact 3150* [Practitioner](practitioner.html): A value in a phone contact 3151* [PractitionerRole](practitionerrole.html): A value in a phone contact 3152* [RelatedPerson](relatedperson.html): A value in a phone contact 3153</b><br> 3154 * Type: <b>token</b><br> 3155 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 3156 * </p> 3157 */ 3158 @SearchParamDefinition(name="phone", path="Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in a phone contact\r\n* [Person](person.html): A value in a phone contact\r\n* [Practitioner](practitioner.html): A value in a phone contact\r\n* [PractitionerRole](practitionerrole.html): A value in a phone contact\r\n* [RelatedPerson](relatedperson.html): A value in a phone contact\r\n", type="token" ) 3159 public static final String SP_PHONE = "phone"; 3160 /** 3161 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 3162 * <p> 3163 * Description: <b>Multiple Resources: 3164 3165* [Patient](patient.html): A value in a phone contact 3166* [Person](person.html): A value in a phone contact 3167* [Practitioner](practitioner.html): A value in a phone contact 3168* [PractitionerRole](practitionerrole.html): A value in a phone contact 3169* [RelatedPerson](relatedperson.html): A value in a phone contact 3170</b><br> 3171 * Type: <b>token</b><br> 3172 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 3173 * </p> 3174 */ 3175 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 3176 3177 /** 3178 * Search parameter: <b>phonetic</b> 3179 * <p> 3180 * Description: <b>Multiple Resources: 3181 3182* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 3183* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 3184* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 3185* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 3186</b><br> 3187 * Type: <b>string</b><br> 3188 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 3189 * </p> 3190 */ 3191 @SearchParamDefinition(name="phonetic", path="Patient.name | Person.name | Practitioner.name | RelatedPerson.name", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [Person](person.html): A portion of name using some kind of phonetic matching algorithm\r\n* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm\r\n", type="string" ) 3192 public static final String SP_PHONETIC = "phonetic"; 3193 /** 3194 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 3195 * <p> 3196 * Description: <b>Multiple Resources: 3197 3198* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 3199* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 3200* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 3201* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 3202</b><br> 3203 * Type: <b>string</b><br> 3204 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 3205 * </p> 3206 */ 3207 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 3208 3209 /** 3210 * Search parameter: <b>telecom</b> 3211 * <p> 3212 * Description: <b>Multiple Resources: 3213 3214* [Patient](patient.html): The value in any kind of telecom details of the patient 3215* [Person](person.html): The value in any kind of contact 3216* [Practitioner](practitioner.html): The value in any kind of contact 3217* [PractitionerRole](practitionerrole.html): The value in any kind of contact 3218* [RelatedPerson](relatedperson.html): The value in any kind of contact 3219</b><br> 3220 * Type: <b>token</b><br> 3221 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 3222 * </p> 3223 */ 3224 @SearchParamDefinition(name="telecom", path="Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The value in any kind of telecom details of the patient\r\n* [Person](person.html): The value in any kind of contact\r\n* [Practitioner](practitioner.html): The value in any kind of contact\r\n* [PractitionerRole](practitionerrole.html): The value in any kind of contact\r\n* [RelatedPerson](relatedperson.html): The value in any kind of contact\r\n", type="token" ) 3225 public static final String SP_TELECOM = "telecom"; 3226 /** 3227 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 3228 * <p> 3229 * Description: <b>Multiple Resources: 3230 3231* [Patient](patient.html): The value in any kind of telecom details of the patient 3232* [Person](person.html): The value in any kind of contact 3233* [Practitioner](practitioner.html): The value in any kind of contact 3234* [PractitionerRole](practitionerrole.html): The value in any kind of contact 3235* [RelatedPerson](relatedperson.html): The value in any kind of contact 3236</b><br> 3237 * Type: <b>token</b><br> 3238 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 3239 * </p> 3240 */ 3241 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 3242 3243 3244} 3245