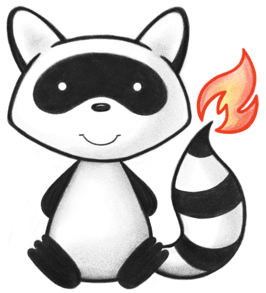
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 052 */ 053@ResourceDef(name="PaymentNotice", profile="http://hl7.org/fhir/StructureDefinition/PaymentNotice") 054public class PaymentNotice extends DomainResource { 055 056 /** 057 * A unique identifier assigned to this payment notice. 058 */ 059 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 060 @Description(shortDefinition="Business Identifier for the payment notice", formalDefinition="A unique identifier assigned to this payment notice." ) 061 protected List<Identifier> identifier; 062 063 /** 064 * The status of the resource instance. 065 */ 066 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 067 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 069 protected Enumeration<FinancialResourceStatusCodes> status; 070 071 /** 072 * Reference of resource for which payment is being made. 073 */ 074 @Child(name = "request", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 075 @Description(shortDefinition="Request reference", formalDefinition="Reference of resource for which payment is being made." ) 076 protected Reference request; 077 078 /** 079 * Reference of response to resource for which payment is being made. 080 */ 081 @Child(name = "response", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 082 @Description(shortDefinition="Response reference", formalDefinition="Reference of response to resource for which payment is being made." ) 083 protected Reference response; 084 085 /** 086 * The date when this resource was created. 087 */ 088 @Child(name = "created", type = {DateTimeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 089 @Description(shortDefinition="Creation date", formalDefinition="The date when this resource was created." ) 090 protected DateTimeType created; 091 092 /** 093 * The party who reports the payment notice. 094 */ 095 @Child(name = "reporter", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=5, min=0, max=1, modifier=false, summary=false) 096 @Description(shortDefinition="Responsible practitioner", formalDefinition="The party who reports the payment notice." ) 097 protected Reference reporter; 098 099 /** 100 * A reference to the payment which is the subject of this notice. 101 */ 102 @Child(name = "payment", type = {PaymentReconciliation.class}, order=6, min=0, max=1, modifier=false, summary=true) 103 @Description(shortDefinition="Payment reference", formalDefinition="A reference to the payment which is the subject of this notice." ) 104 protected Reference payment; 105 106 /** 107 * The date when the above payment action occurred. 108 */ 109 @Child(name = "paymentDate", type = {DateType.class}, order=7, min=0, max=1, modifier=false, summary=false) 110 @Description(shortDefinition="Payment or clearing date", formalDefinition="The date when the above payment action occurred." ) 111 protected DateType paymentDate; 112 113 /** 114 * The party who will receive or has received payment that is the subject of this notification. 115 */ 116 @Child(name = "payee", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 117 @Description(shortDefinition="Party being paid", formalDefinition="The party who will receive or has received payment that is the subject of this notification." ) 118 protected Reference payee; 119 120 /** 121 * The party who is notified of the payment status. 122 */ 123 @Child(name = "recipient", type = {Organization.class}, order=9, min=1, max=1, modifier=false, summary=true) 124 @Description(shortDefinition="Party being notified", formalDefinition="The party who is notified of the payment status." ) 125 protected Reference recipient; 126 127 /** 128 * The amount sent to the payee. 129 */ 130 @Child(name = "amount", type = {Money.class}, order=10, min=1, max=1, modifier=false, summary=true) 131 @Description(shortDefinition="Monetary amount of the payment", formalDefinition="The amount sent to the payee." ) 132 protected Money amount; 133 134 /** 135 * A code indicating whether payment has been sent or cleared. 136 */ 137 @Child(name = "paymentStatus", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=false) 138 @Description(shortDefinition="Issued or cleared Status of the payment", formalDefinition="A code indicating whether payment has been sent or cleared." ) 139 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-status") 140 protected CodeableConcept paymentStatus; 141 142 private static final long serialVersionUID = 1568561168L; 143 144 /** 145 * Constructor 146 */ 147 public PaymentNotice() { 148 super(); 149 } 150 151 /** 152 * Constructor 153 */ 154 public PaymentNotice(FinancialResourceStatusCodes status, Date created, Reference recipient, Money amount) { 155 super(); 156 this.setStatus(status); 157 this.setCreated(created); 158 this.setRecipient(recipient); 159 this.setAmount(amount); 160 } 161 162 /** 163 * @return {@link #identifier} (A unique identifier assigned to this payment notice.) 164 */ 165 public List<Identifier> getIdentifier() { 166 if (this.identifier == null) 167 this.identifier = new ArrayList<Identifier>(); 168 return this.identifier; 169 } 170 171 /** 172 * @return Returns a reference to <code>this</code> for easy method chaining 173 */ 174 public PaymentNotice setIdentifier(List<Identifier> theIdentifier) { 175 this.identifier = theIdentifier; 176 return this; 177 } 178 179 public boolean hasIdentifier() { 180 if (this.identifier == null) 181 return false; 182 for (Identifier item : this.identifier) 183 if (!item.isEmpty()) 184 return true; 185 return false; 186 } 187 188 public Identifier addIdentifier() { //3 189 Identifier t = new Identifier(); 190 if (this.identifier == null) 191 this.identifier = new ArrayList<Identifier>(); 192 this.identifier.add(t); 193 return t; 194 } 195 196 public PaymentNotice addIdentifier(Identifier t) { //3 197 if (t == null) 198 return this; 199 if (this.identifier == null) 200 this.identifier = new ArrayList<Identifier>(); 201 this.identifier.add(t); 202 return this; 203 } 204 205 /** 206 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 207 */ 208 public Identifier getIdentifierFirstRep() { 209 if (getIdentifier().isEmpty()) { 210 addIdentifier(); 211 } 212 return getIdentifier().get(0); 213 } 214 215 /** 216 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 217 */ 218 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 219 if (this.status == null) 220 if (Configuration.errorOnAutoCreate()) 221 throw new Error("Attempt to auto-create PaymentNotice.status"); 222 else if (Configuration.doAutoCreate()) 223 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 224 return this.status; 225 } 226 227 public boolean hasStatusElement() { 228 return this.status != null && !this.status.isEmpty(); 229 } 230 231 public boolean hasStatus() { 232 return this.status != null && !this.status.isEmpty(); 233 } 234 235 /** 236 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 237 */ 238 public PaymentNotice setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 239 this.status = value; 240 return this; 241 } 242 243 /** 244 * @return The status of the resource instance. 245 */ 246 public FinancialResourceStatusCodes getStatus() { 247 return this.status == null ? null : this.status.getValue(); 248 } 249 250 /** 251 * @param value The status of the resource instance. 252 */ 253 public PaymentNotice setStatus(FinancialResourceStatusCodes value) { 254 if (this.status == null) 255 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 256 this.status.setValue(value); 257 return this; 258 } 259 260 /** 261 * @return {@link #request} (Reference of resource for which payment is being made.) 262 */ 263 public Reference getRequest() { 264 if (this.request == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create PaymentNotice.request"); 267 else if (Configuration.doAutoCreate()) 268 this.request = new Reference(); // cc 269 return this.request; 270 } 271 272 public boolean hasRequest() { 273 return this.request != null && !this.request.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #request} (Reference of resource for which payment is being made.) 278 */ 279 public PaymentNotice setRequest(Reference value) { 280 this.request = value; 281 return this; 282 } 283 284 /** 285 * @return {@link #response} (Reference of response to resource for which payment is being made.) 286 */ 287 public Reference getResponse() { 288 if (this.response == null) 289 if (Configuration.errorOnAutoCreate()) 290 throw new Error("Attempt to auto-create PaymentNotice.response"); 291 else if (Configuration.doAutoCreate()) 292 this.response = new Reference(); // cc 293 return this.response; 294 } 295 296 public boolean hasResponse() { 297 return this.response != null && !this.response.isEmpty(); 298 } 299 300 /** 301 * @param value {@link #response} (Reference of response to resource for which payment is being made.) 302 */ 303 public PaymentNotice setResponse(Reference value) { 304 this.response = value; 305 return this; 306 } 307 308 /** 309 * @return {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 310 */ 311 public DateTimeType getCreatedElement() { 312 if (this.created == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create PaymentNotice.created"); 315 else if (Configuration.doAutoCreate()) 316 this.created = new DateTimeType(); // bb 317 return this.created; 318 } 319 320 public boolean hasCreatedElement() { 321 return this.created != null && !this.created.isEmpty(); 322 } 323 324 public boolean hasCreated() { 325 return this.created != null && !this.created.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 330 */ 331 public PaymentNotice setCreatedElement(DateTimeType value) { 332 this.created = value; 333 return this; 334 } 335 336 /** 337 * @return The date when this resource was created. 338 */ 339 public Date getCreated() { 340 return this.created == null ? null : this.created.getValue(); 341 } 342 343 /** 344 * @param value The date when this resource was created. 345 */ 346 public PaymentNotice setCreated(Date value) { 347 if (this.created == null) 348 this.created = new DateTimeType(); 349 this.created.setValue(value); 350 return this; 351 } 352 353 /** 354 * @return {@link #reporter} (The party who reports the payment notice.) 355 */ 356 public Reference getReporter() { 357 if (this.reporter == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create PaymentNotice.reporter"); 360 else if (Configuration.doAutoCreate()) 361 this.reporter = new Reference(); // cc 362 return this.reporter; 363 } 364 365 public boolean hasReporter() { 366 return this.reporter != null && !this.reporter.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #reporter} (The party who reports the payment notice.) 371 */ 372 public PaymentNotice setReporter(Reference value) { 373 this.reporter = value; 374 return this; 375 } 376 377 /** 378 * @return {@link #payment} (A reference to the payment which is the subject of this notice.) 379 */ 380 public Reference getPayment() { 381 if (this.payment == null) 382 if (Configuration.errorOnAutoCreate()) 383 throw new Error("Attempt to auto-create PaymentNotice.payment"); 384 else if (Configuration.doAutoCreate()) 385 this.payment = new Reference(); // cc 386 return this.payment; 387 } 388 389 public boolean hasPayment() { 390 return this.payment != null && !this.payment.isEmpty(); 391 } 392 393 /** 394 * @param value {@link #payment} (A reference to the payment which is the subject of this notice.) 395 */ 396 public PaymentNotice setPayment(Reference value) { 397 this.payment = value; 398 return this; 399 } 400 401 /** 402 * @return {@link #paymentDate} (The date when the above payment action occurred.). This is the underlying object with id, value and extensions. The accessor "getPaymentDate" gives direct access to the value 403 */ 404 public DateType getPaymentDateElement() { 405 if (this.paymentDate == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create PaymentNotice.paymentDate"); 408 else if (Configuration.doAutoCreate()) 409 this.paymentDate = new DateType(); // bb 410 return this.paymentDate; 411 } 412 413 public boolean hasPaymentDateElement() { 414 return this.paymentDate != null && !this.paymentDate.isEmpty(); 415 } 416 417 public boolean hasPaymentDate() { 418 return this.paymentDate != null && !this.paymentDate.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #paymentDate} (The date when the above payment action occurred.). This is the underlying object with id, value and extensions. The accessor "getPaymentDate" gives direct access to the value 423 */ 424 public PaymentNotice setPaymentDateElement(DateType value) { 425 this.paymentDate = value; 426 return this; 427 } 428 429 /** 430 * @return The date when the above payment action occurred. 431 */ 432 public Date getPaymentDate() { 433 return this.paymentDate == null ? null : this.paymentDate.getValue(); 434 } 435 436 /** 437 * @param value The date when the above payment action occurred. 438 */ 439 public PaymentNotice setPaymentDate(Date value) { 440 if (value == null) 441 this.paymentDate = null; 442 else { 443 if (this.paymentDate == null) 444 this.paymentDate = new DateType(); 445 this.paymentDate.setValue(value); 446 } 447 return this; 448 } 449 450 /** 451 * @return {@link #payee} (The party who will receive or has received payment that is the subject of this notification.) 452 */ 453 public Reference getPayee() { 454 if (this.payee == null) 455 if (Configuration.errorOnAutoCreate()) 456 throw new Error("Attempt to auto-create PaymentNotice.payee"); 457 else if (Configuration.doAutoCreate()) 458 this.payee = new Reference(); // cc 459 return this.payee; 460 } 461 462 public boolean hasPayee() { 463 return this.payee != null && !this.payee.isEmpty(); 464 } 465 466 /** 467 * @param value {@link #payee} (The party who will receive or has received payment that is the subject of this notification.) 468 */ 469 public PaymentNotice setPayee(Reference value) { 470 this.payee = value; 471 return this; 472 } 473 474 /** 475 * @return {@link #recipient} (The party who is notified of the payment status.) 476 */ 477 public Reference getRecipient() { 478 if (this.recipient == null) 479 if (Configuration.errorOnAutoCreate()) 480 throw new Error("Attempt to auto-create PaymentNotice.recipient"); 481 else if (Configuration.doAutoCreate()) 482 this.recipient = new Reference(); // cc 483 return this.recipient; 484 } 485 486 public boolean hasRecipient() { 487 return this.recipient != null && !this.recipient.isEmpty(); 488 } 489 490 /** 491 * @param value {@link #recipient} (The party who is notified of the payment status.) 492 */ 493 public PaymentNotice setRecipient(Reference value) { 494 this.recipient = value; 495 return this; 496 } 497 498 /** 499 * @return {@link #amount} (The amount sent to the payee.) 500 */ 501 public Money getAmount() { 502 if (this.amount == null) 503 if (Configuration.errorOnAutoCreate()) 504 throw new Error("Attempt to auto-create PaymentNotice.amount"); 505 else if (Configuration.doAutoCreate()) 506 this.amount = new Money(); // cc 507 return this.amount; 508 } 509 510 public boolean hasAmount() { 511 return this.amount != null && !this.amount.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #amount} (The amount sent to the payee.) 516 */ 517 public PaymentNotice setAmount(Money value) { 518 this.amount = value; 519 return this; 520 } 521 522 /** 523 * @return {@link #paymentStatus} (A code indicating whether payment has been sent or cleared.) 524 */ 525 public CodeableConcept getPaymentStatus() { 526 if (this.paymentStatus == null) 527 if (Configuration.errorOnAutoCreate()) 528 throw new Error("Attempt to auto-create PaymentNotice.paymentStatus"); 529 else if (Configuration.doAutoCreate()) 530 this.paymentStatus = new CodeableConcept(); // cc 531 return this.paymentStatus; 532 } 533 534 public boolean hasPaymentStatus() { 535 return this.paymentStatus != null && !this.paymentStatus.isEmpty(); 536 } 537 538 /** 539 * @param value {@link #paymentStatus} (A code indicating whether payment has been sent or cleared.) 540 */ 541 public PaymentNotice setPaymentStatus(CodeableConcept value) { 542 this.paymentStatus = value; 543 return this; 544 } 545 546 protected void listChildren(List<Property> children) { 547 super.listChildren(children); 548 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this payment notice.", 0, java.lang.Integer.MAX_VALUE, identifier)); 549 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 550 children.add(new Property("request", "Reference(Any)", "Reference of resource for which payment is being made.", 0, 1, request)); 551 children.add(new Property("response", "Reference(Any)", "Reference of response to resource for which payment is being made.", 0, 1, response)); 552 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 553 children.add(new Property("reporter", "Reference(Practitioner|PractitionerRole|Organization)", "The party who reports the payment notice.", 0, 1, reporter)); 554 children.add(new Property("payment", "Reference(PaymentReconciliation)", "A reference to the payment which is the subject of this notice.", 0, 1, payment)); 555 children.add(new Property("paymentDate", "date", "The date when the above payment action occurred.", 0, 1, paymentDate)); 556 children.add(new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", "The party who will receive or has received payment that is the subject of this notification.", 0, 1, payee)); 557 children.add(new Property("recipient", "Reference(Organization)", "The party who is notified of the payment status.", 0, 1, recipient)); 558 children.add(new Property("amount", "Money", "The amount sent to the payee.", 0, 1, amount)); 559 children.add(new Property("paymentStatus", "CodeableConcept", "A code indicating whether payment has been sent or cleared.", 0, 1, paymentStatus)); 560 } 561 562 @Override 563 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 564 switch (_hash) { 565 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this payment notice.", 0, java.lang.Integer.MAX_VALUE, identifier); 566 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 567 case 1095692943: /*request*/ return new Property("request", "Reference(Any)", "Reference of resource for which payment is being made.", 0, 1, request); 568 case -340323263: /*response*/ return new Property("response", "Reference(Any)", "Reference of response to resource for which payment is being made.", 0, 1, response); 569 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created); 570 case -427039519: /*reporter*/ return new Property("reporter", "Reference(Practitioner|PractitionerRole|Organization)", "The party who reports the payment notice.", 0, 1, reporter); 571 case -786681338: /*payment*/ return new Property("payment", "Reference(PaymentReconciliation)", "A reference to the payment which is the subject of this notice.", 0, 1, payment); 572 case -1540873516: /*paymentDate*/ return new Property("paymentDate", "date", "The date when the above payment action occurred.", 0, 1, paymentDate); 573 case 106443592: /*payee*/ return new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", "The party who will receive or has received payment that is the subject of this notification.", 0, 1, payee); 574 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Organization)", "The party who is notified of the payment status.", 0, 1, recipient); 575 case -1413853096: /*amount*/ return new Property("amount", "Money", "The amount sent to the payee.", 0, 1, amount); 576 case 1430704536: /*paymentStatus*/ return new Property("paymentStatus", "CodeableConcept", "A code indicating whether payment has been sent or cleared.", 0, 1, paymentStatus); 577 default: return super.getNamedProperty(_hash, _name, _checkValid); 578 } 579 580 } 581 582 @Override 583 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 584 switch (hash) { 585 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 586 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 587 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 588 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Reference 589 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 590 case -427039519: /*reporter*/ return this.reporter == null ? new Base[0] : new Base[] {this.reporter}; // Reference 591 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // Reference 592 case -1540873516: /*paymentDate*/ return this.paymentDate == null ? new Base[0] : new Base[] {this.paymentDate}; // DateType 593 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // Reference 594 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : new Base[] {this.recipient}; // Reference 595 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 596 case 1430704536: /*paymentStatus*/ return this.paymentStatus == null ? new Base[0] : new Base[] {this.paymentStatus}; // CodeableConcept 597 default: return super.getProperty(hash, name, checkValid); 598 } 599 600 } 601 602 @Override 603 public Base setProperty(int hash, String name, Base value) throws FHIRException { 604 switch (hash) { 605 case -1618432855: // identifier 606 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 607 return value; 608 case -892481550: // status 609 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 610 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 611 return value; 612 case 1095692943: // request 613 this.request = TypeConvertor.castToReference(value); // Reference 614 return value; 615 case -340323263: // response 616 this.response = TypeConvertor.castToReference(value); // Reference 617 return value; 618 case 1028554472: // created 619 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 620 return value; 621 case -427039519: // reporter 622 this.reporter = TypeConvertor.castToReference(value); // Reference 623 return value; 624 case -786681338: // payment 625 this.payment = TypeConvertor.castToReference(value); // Reference 626 return value; 627 case -1540873516: // paymentDate 628 this.paymentDate = TypeConvertor.castToDate(value); // DateType 629 return value; 630 case 106443592: // payee 631 this.payee = TypeConvertor.castToReference(value); // Reference 632 return value; 633 case 820081177: // recipient 634 this.recipient = TypeConvertor.castToReference(value); // Reference 635 return value; 636 case -1413853096: // amount 637 this.amount = TypeConvertor.castToMoney(value); // Money 638 return value; 639 case 1430704536: // paymentStatus 640 this.paymentStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 641 return value; 642 default: return super.setProperty(hash, name, value); 643 } 644 645 } 646 647 @Override 648 public Base setProperty(String name, Base value) throws FHIRException { 649 if (name.equals("identifier")) { 650 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 651 } else if (name.equals("status")) { 652 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 653 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 654 } else if (name.equals("request")) { 655 this.request = TypeConvertor.castToReference(value); // Reference 656 } else if (name.equals("response")) { 657 this.response = TypeConvertor.castToReference(value); // Reference 658 } else if (name.equals("created")) { 659 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 660 } else if (name.equals("reporter")) { 661 this.reporter = TypeConvertor.castToReference(value); // Reference 662 } else if (name.equals("payment")) { 663 this.payment = TypeConvertor.castToReference(value); // Reference 664 } else if (name.equals("paymentDate")) { 665 this.paymentDate = TypeConvertor.castToDate(value); // DateType 666 } else if (name.equals("payee")) { 667 this.payee = TypeConvertor.castToReference(value); // Reference 668 } else if (name.equals("recipient")) { 669 this.recipient = TypeConvertor.castToReference(value); // Reference 670 } else if (name.equals("amount")) { 671 this.amount = TypeConvertor.castToMoney(value); // Money 672 } else if (name.equals("paymentStatus")) { 673 this.paymentStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 674 } else 675 return super.setProperty(name, value); 676 return value; 677 } 678 679 @Override 680 public void removeChild(String name, Base value) throws FHIRException { 681 if (name.equals("identifier")) { 682 this.getIdentifier().remove(value); 683 } else if (name.equals("status")) { 684 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 685 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 686 } else if (name.equals("request")) { 687 this.request = null; 688 } else if (name.equals("response")) { 689 this.response = null; 690 } else if (name.equals("created")) { 691 this.created = null; 692 } else if (name.equals("reporter")) { 693 this.reporter = null; 694 } else if (name.equals("payment")) { 695 this.payment = null; 696 } else if (name.equals("paymentDate")) { 697 this.paymentDate = null; 698 } else if (name.equals("payee")) { 699 this.payee = null; 700 } else if (name.equals("recipient")) { 701 this.recipient = null; 702 } else if (name.equals("amount")) { 703 this.amount = null; 704 } else if (name.equals("paymentStatus")) { 705 this.paymentStatus = null; 706 } else 707 super.removeChild(name, value); 708 709 } 710 711 @Override 712 public Base makeProperty(int hash, String name) throws FHIRException { 713 switch (hash) { 714 case -1618432855: return addIdentifier(); 715 case -892481550: return getStatusElement(); 716 case 1095692943: return getRequest(); 717 case -340323263: return getResponse(); 718 case 1028554472: return getCreatedElement(); 719 case -427039519: return getReporter(); 720 case -786681338: return getPayment(); 721 case -1540873516: return getPaymentDateElement(); 722 case 106443592: return getPayee(); 723 case 820081177: return getRecipient(); 724 case -1413853096: return getAmount(); 725 case 1430704536: return getPaymentStatus(); 726 default: return super.makeProperty(hash, name); 727 } 728 729 } 730 731 @Override 732 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 733 switch (hash) { 734 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 735 case -892481550: /*status*/ return new String[] {"code"}; 736 case 1095692943: /*request*/ return new String[] {"Reference"}; 737 case -340323263: /*response*/ return new String[] {"Reference"}; 738 case 1028554472: /*created*/ return new String[] {"dateTime"}; 739 case -427039519: /*reporter*/ return new String[] {"Reference"}; 740 case -786681338: /*payment*/ return new String[] {"Reference"}; 741 case -1540873516: /*paymentDate*/ return new String[] {"date"}; 742 case 106443592: /*payee*/ return new String[] {"Reference"}; 743 case 820081177: /*recipient*/ return new String[] {"Reference"}; 744 case -1413853096: /*amount*/ return new String[] {"Money"}; 745 case 1430704536: /*paymentStatus*/ return new String[] {"CodeableConcept"}; 746 default: return super.getTypesForProperty(hash, name); 747 } 748 749 } 750 751 @Override 752 public Base addChild(String name) throws FHIRException { 753 if (name.equals("identifier")) { 754 return addIdentifier(); 755 } 756 else if (name.equals("status")) { 757 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.status"); 758 } 759 else if (name.equals("request")) { 760 this.request = new Reference(); 761 return this.request; 762 } 763 else if (name.equals("response")) { 764 this.response = new Reference(); 765 return this.response; 766 } 767 else if (name.equals("created")) { 768 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.created"); 769 } 770 else if (name.equals("reporter")) { 771 this.reporter = new Reference(); 772 return this.reporter; 773 } 774 else if (name.equals("payment")) { 775 this.payment = new Reference(); 776 return this.payment; 777 } 778 else if (name.equals("paymentDate")) { 779 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.paymentDate"); 780 } 781 else if (name.equals("payee")) { 782 this.payee = new Reference(); 783 return this.payee; 784 } 785 else if (name.equals("recipient")) { 786 this.recipient = new Reference(); 787 return this.recipient; 788 } 789 else if (name.equals("amount")) { 790 this.amount = new Money(); 791 return this.amount; 792 } 793 else if (name.equals("paymentStatus")) { 794 this.paymentStatus = new CodeableConcept(); 795 return this.paymentStatus; 796 } 797 else 798 return super.addChild(name); 799 } 800 801 public String fhirType() { 802 return "PaymentNotice"; 803 804 } 805 806 public PaymentNotice copy() { 807 PaymentNotice dst = new PaymentNotice(); 808 copyValues(dst); 809 return dst; 810 } 811 812 public void copyValues(PaymentNotice dst) { 813 super.copyValues(dst); 814 if (identifier != null) { 815 dst.identifier = new ArrayList<Identifier>(); 816 for (Identifier i : identifier) 817 dst.identifier.add(i.copy()); 818 }; 819 dst.status = status == null ? null : status.copy(); 820 dst.request = request == null ? null : request.copy(); 821 dst.response = response == null ? null : response.copy(); 822 dst.created = created == null ? null : created.copy(); 823 dst.reporter = reporter == null ? null : reporter.copy(); 824 dst.payment = payment == null ? null : payment.copy(); 825 dst.paymentDate = paymentDate == null ? null : paymentDate.copy(); 826 dst.payee = payee == null ? null : payee.copy(); 827 dst.recipient = recipient == null ? null : recipient.copy(); 828 dst.amount = amount == null ? null : amount.copy(); 829 dst.paymentStatus = paymentStatus == null ? null : paymentStatus.copy(); 830 } 831 832 protected PaymentNotice typedCopy() { 833 return copy(); 834 } 835 836 @Override 837 public boolean equalsDeep(Base other_) { 838 if (!super.equalsDeep(other_)) 839 return false; 840 if (!(other_ instanceof PaymentNotice)) 841 return false; 842 PaymentNotice o = (PaymentNotice) other_; 843 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(request, o.request, true) 844 && compareDeep(response, o.response, true) && compareDeep(created, o.created, true) && compareDeep(reporter, o.reporter, true) 845 && compareDeep(payment, o.payment, true) && compareDeep(paymentDate, o.paymentDate, true) && compareDeep(payee, o.payee, true) 846 && compareDeep(recipient, o.recipient, true) && compareDeep(amount, o.amount, true) && compareDeep(paymentStatus, o.paymentStatus, true) 847 ; 848 } 849 850 @Override 851 public boolean equalsShallow(Base other_) { 852 if (!super.equalsShallow(other_)) 853 return false; 854 if (!(other_ instanceof PaymentNotice)) 855 return false; 856 PaymentNotice o = (PaymentNotice) other_; 857 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(paymentDate, o.paymentDate, true) 858 ; 859 } 860 861 public boolean isEmpty() { 862 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, request 863 , response, created, reporter, payment, paymentDate, payee, recipient, amount 864 , paymentStatus); 865 } 866 867 @Override 868 public ResourceType getResourceType() { 869 return ResourceType.PaymentNotice; 870 } 871 872 /** 873 * Search parameter: <b>created</b> 874 * <p> 875 * Description: <b>Creation date for the notice</b><br> 876 * Type: <b>date</b><br> 877 * Path: <b>PaymentNotice.created</b><br> 878 * </p> 879 */ 880 @SearchParamDefinition(name="created", path="PaymentNotice.created", description="Creation date for the notice", type="date" ) 881 public static final String SP_CREATED = "created"; 882 /** 883 * <b>Fluent Client</b> search parameter constant for <b>created</b> 884 * <p> 885 * Description: <b>Creation date for the notice</b><br> 886 * Type: <b>date</b><br> 887 * Path: <b>PaymentNotice.created</b><br> 888 * </p> 889 */ 890 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 891 892 /** 893 * Search parameter: <b>identifier</b> 894 * <p> 895 * Description: <b>The business identifier of the notice</b><br> 896 * Type: <b>token</b><br> 897 * Path: <b>PaymentNotice.identifier</b><br> 898 * </p> 899 */ 900 @SearchParamDefinition(name="identifier", path="PaymentNotice.identifier", description="The business identifier of the notice", type="token" ) 901 public static final String SP_IDENTIFIER = "identifier"; 902 /** 903 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 904 * <p> 905 * Description: <b>The business identifier of the notice</b><br> 906 * Type: <b>token</b><br> 907 * Path: <b>PaymentNotice.identifier</b><br> 908 * </p> 909 */ 910 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 911 912 /** 913 * Search parameter: <b>payment-status</b> 914 * <p> 915 * Description: <b>The type of payment notice</b><br> 916 * Type: <b>token</b><br> 917 * Path: <b>PaymentNotice.paymentStatus</b><br> 918 * </p> 919 */ 920 @SearchParamDefinition(name="payment-status", path="PaymentNotice.paymentStatus", description="The type of payment notice", type="token" ) 921 public static final String SP_PAYMENT_STATUS = "payment-status"; 922 /** 923 * <b>Fluent Client</b> search parameter constant for <b>payment-status</b> 924 * <p> 925 * Description: <b>The type of payment notice</b><br> 926 * Type: <b>token</b><br> 927 * Path: <b>PaymentNotice.paymentStatus</b><br> 928 * </p> 929 */ 930 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYMENT_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PAYMENT_STATUS); 931 932 /** 933 * Search parameter: <b>reporter</b> 934 * <p> 935 * Description: <b>The reference to the reporter</b><br> 936 * Type: <b>reference</b><br> 937 * Path: <b>PaymentNotice.reporter</b><br> 938 * </p> 939 */ 940 @SearchParamDefinition(name="reporter", path="PaymentNotice.reporter", description="The reference to the reporter", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 941 public static final String SP_REPORTER = "reporter"; 942 /** 943 * <b>Fluent Client</b> search parameter constant for <b>reporter</b> 944 * <p> 945 * Description: <b>The reference to the reporter</b><br> 946 * Type: <b>reference</b><br> 947 * Path: <b>PaymentNotice.reporter</b><br> 948 * </p> 949 */ 950 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPORTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPORTER); 951 952/** 953 * Constant for fluent queries to be used to add include statements. Specifies 954 * the path value of "<b>PaymentNotice:reporter</b>". 955 */ 956 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPORTER = new ca.uhn.fhir.model.api.Include("PaymentNotice:reporter").toLocked(); 957 958 /** 959 * Search parameter: <b>request</b> 960 * <p> 961 * Description: <b>The Claim</b><br> 962 * Type: <b>reference</b><br> 963 * Path: <b>PaymentNotice.request</b><br> 964 * </p> 965 */ 966 @SearchParamDefinition(name="request", path="PaymentNotice.request", description="The Claim", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 967 public static final String SP_REQUEST = "request"; 968 /** 969 * <b>Fluent Client</b> search parameter constant for <b>request</b> 970 * <p> 971 * Description: <b>The Claim</b><br> 972 * Type: <b>reference</b><br> 973 * Path: <b>PaymentNotice.request</b><br> 974 * </p> 975 */ 976 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 977 978/** 979 * Constant for fluent queries to be used to add include statements. Specifies 980 * the path value of "<b>PaymentNotice:request</b>". 981 */ 982 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("PaymentNotice:request").toLocked(); 983 984 /** 985 * Search parameter: <b>response</b> 986 * <p> 987 * Description: <b>The ClaimResponse</b><br> 988 * Type: <b>reference</b><br> 989 * Path: <b>PaymentNotice.response</b><br> 990 * </p> 991 */ 992 @SearchParamDefinition(name="response", path="PaymentNotice.response", description="The ClaimResponse", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 993 public static final String SP_RESPONSE = "response"; 994 /** 995 * <b>Fluent Client</b> search parameter constant for <b>response</b> 996 * <p> 997 * Description: <b>The ClaimResponse</b><br> 998 * Type: <b>reference</b><br> 999 * Path: <b>PaymentNotice.response</b><br> 1000 * </p> 1001 */ 1002 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESPONSE); 1003 1004/** 1005 * Constant for fluent queries to be used to add include statements. Specifies 1006 * the path value of "<b>PaymentNotice:response</b>". 1007 */ 1008 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSE = new ca.uhn.fhir.model.api.Include("PaymentNotice:response").toLocked(); 1009 1010 /** 1011 * Search parameter: <b>status</b> 1012 * <p> 1013 * Description: <b>The status of the payment notice</b><br> 1014 * Type: <b>token</b><br> 1015 * Path: <b>PaymentNotice.status</b><br> 1016 * </p> 1017 */ 1018 @SearchParamDefinition(name="status", path="PaymentNotice.status", description="The status of the payment notice", type="token" ) 1019 public static final String SP_STATUS = "status"; 1020 /** 1021 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1022 * <p> 1023 * Description: <b>The status of the payment notice</b><br> 1024 * Type: <b>token</b><br> 1025 * Path: <b>PaymentNotice.status</b><br> 1026 * </p> 1027 */ 1028 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1029 1030 1031} 1032