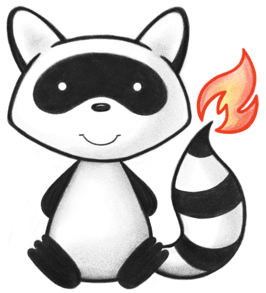
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource provides the details including amount of a payment and allocates the payment items being paid. 052 */ 053@ResourceDef(name="PaymentReconciliation", profile="http://hl7.org/fhir/StructureDefinition/PaymentReconciliation") 054public class PaymentReconciliation extends DomainResource { 055 056 public enum NoteType { 057 /** 058 * Display the note. 059 */ 060 DISPLAY, 061 /** 062 * Print the note on the form. 063 */ 064 PRINT, 065 /** 066 * Print the note for the operator. 067 */ 068 PRINTOPER, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static NoteType fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("display".equals(codeString)) 077 return DISPLAY; 078 if ("print".equals(codeString)) 079 return PRINT; 080 if ("printoper".equals(codeString)) 081 return PRINTOPER; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown NoteType code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case DISPLAY: return "display"; 090 case PRINT: return "print"; 091 case PRINTOPER: return "printoper"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case DISPLAY: return "http://hl7.org/fhir/note-type"; 099 case PRINT: return "http://hl7.org/fhir/note-type"; 100 case PRINTOPER: return "http://hl7.org/fhir/note-type"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case DISPLAY: return "Display the note."; 108 case PRINT: return "Print the note on the form."; 109 case PRINTOPER: return "Print the note for the operator."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case DISPLAY: return "Display"; 117 case PRINT: return "Print (Form)"; 118 case PRINTOPER: return "Print (Operator)"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class NoteTypeEnumFactory implements EnumFactory<NoteType> { 126 public NoteType fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("display".equals(codeString)) 131 return NoteType.DISPLAY; 132 if ("print".equals(codeString)) 133 return NoteType.PRINT; 134 if ("printoper".equals(codeString)) 135 return NoteType.PRINTOPER; 136 throw new IllegalArgumentException("Unknown NoteType code '"+codeString+"'"); 137 } 138 public Enumeration<NoteType> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<NoteType>(this, NoteType.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<NoteType>(this, NoteType.NULL, code); 146 if ("display".equals(codeString)) 147 return new Enumeration<NoteType>(this, NoteType.DISPLAY, code); 148 if ("print".equals(codeString)) 149 return new Enumeration<NoteType>(this, NoteType.PRINT, code); 150 if ("printoper".equals(codeString)) 151 return new Enumeration<NoteType>(this, NoteType.PRINTOPER, code); 152 throw new FHIRException("Unknown NoteType code '"+codeString+"'"); 153 } 154 public String toCode(NoteType code) { 155 if (code == NoteType.NULL) 156 return null; 157 if (code == NoteType.DISPLAY) 158 return "display"; 159 if (code == NoteType.PRINT) 160 return "print"; 161 if (code == NoteType.PRINTOPER) 162 return "printoper"; 163 return "?"; 164 } 165 public String toSystem(NoteType code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum PaymentOutcome { 171 /** 172 * The Claim/Pre-authorization/Pre-determination has been received but processing has not begun. 173 */ 174 QUEUED, 175 /** 176 * The processing has completed without errors 177 */ 178 COMPLETE, 179 /** 180 * One or more errors have been detected in the Claim 181 */ 182 ERROR, 183 /** 184 * No errors have been detected in the Claim and some of the adjudication has been performed. 185 */ 186 PARTIAL, 187 /** 188 * added to help the parsers with the generic types 189 */ 190 NULL; 191 public static PaymentOutcome fromCode(String codeString) throws FHIRException { 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("queued".equals(codeString)) 195 return QUEUED; 196 if ("complete".equals(codeString)) 197 return COMPLETE; 198 if ("error".equals(codeString)) 199 return ERROR; 200 if ("partial".equals(codeString)) 201 return PARTIAL; 202 if (Configuration.isAcceptInvalidEnums()) 203 return null; 204 else 205 throw new FHIRException("Unknown PaymentOutcome code '"+codeString+"'"); 206 } 207 public String toCode() { 208 switch (this) { 209 case QUEUED: return "queued"; 210 case COMPLETE: return "complete"; 211 case ERROR: return "error"; 212 case PARTIAL: return "partial"; 213 case NULL: return null; 214 default: return "?"; 215 } 216 } 217 public String getSystem() { 218 switch (this) { 219 case QUEUED: return "http://hl7.org/fhir/payment-outcome"; 220 case COMPLETE: return "http://hl7.org/fhir/payment-outcome"; 221 case ERROR: return "http://hl7.org/fhir/payment-outcome"; 222 case PARTIAL: return "http://hl7.org/fhir/payment-outcome"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 public String getDefinition() { 228 switch (this) { 229 case QUEUED: return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 230 case COMPLETE: return "The processing has completed without errors"; 231 case ERROR: return "One or more errors have been detected in the Claim"; 232 case PARTIAL: return "No errors have been detected in the Claim and some of the adjudication has been performed."; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 public String getDisplay() { 238 switch (this) { 239 case QUEUED: return "Queued"; 240 case COMPLETE: return "Processing Complete"; 241 case ERROR: return "Error"; 242 case PARTIAL: return "Partial Processing"; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 } 248 249 public static class PaymentOutcomeEnumFactory implements EnumFactory<PaymentOutcome> { 250 public PaymentOutcome fromCode(String codeString) throws IllegalArgumentException { 251 if (codeString == null || "".equals(codeString)) 252 if (codeString == null || "".equals(codeString)) 253 return null; 254 if ("queued".equals(codeString)) 255 return PaymentOutcome.QUEUED; 256 if ("complete".equals(codeString)) 257 return PaymentOutcome.COMPLETE; 258 if ("error".equals(codeString)) 259 return PaymentOutcome.ERROR; 260 if ("partial".equals(codeString)) 261 return PaymentOutcome.PARTIAL; 262 throw new IllegalArgumentException("Unknown PaymentOutcome code '"+codeString+"'"); 263 } 264 public Enumeration<PaymentOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 265 if (code == null) 266 return null; 267 if (code.isEmpty()) 268 return new Enumeration<PaymentOutcome>(this, PaymentOutcome.NULL, code); 269 String codeString = ((PrimitiveType) code).asStringValue(); 270 if (codeString == null || "".equals(codeString)) 271 return new Enumeration<PaymentOutcome>(this, PaymentOutcome.NULL, code); 272 if ("queued".equals(codeString)) 273 return new Enumeration<PaymentOutcome>(this, PaymentOutcome.QUEUED, code); 274 if ("complete".equals(codeString)) 275 return new Enumeration<PaymentOutcome>(this, PaymentOutcome.COMPLETE, code); 276 if ("error".equals(codeString)) 277 return new Enumeration<PaymentOutcome>(this, PaymentOutcome.ERROR, code); 278 if ("partial".equals(codeString)) 279 return new Enumeration<PaymentOutcome>(this, PaymentOutcome.PARTIAL, code); 280 throw new FHIRException("Unknown PaymentOutcome code '"+codeString+"'"); 281 } 282 public String toCode(PaymentOutcome code) { 283 if (code == PaymentOutcome.NULL) 284 return null; 285 if (code == PaymentOutcome.QUEUED) 286 return "queued"; 287 if (code == PaymentOutcome.COMPLETE) 288 return "complete"; 289 if (code == PaymentOutcome.ERROR) 290 return "error"; 291 if (code == PaymentOutcome.PARTIAL) 292 return "partial"; 293 return "?"; 294 } 295 public String toSystem(PaymentOutcome code) { 296 return code.getSystem(); 297 } 298 } 299 300 @Block() 301 public static class PaymentReconciliationAllocationComponent extends BackboneElement implements IBaseBackboneElement { 302 /** 303 * Unique identifier for the current payment item for the referenced payable. 304 */ 305 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 306 @Description(shortDefinition="Business identifier of the payment detail", formalDefinition="Unique identifier for the current payment item for the referenced payable." ) 307 protected Identifier identifier; 308 309 /** 310 * Unique identifier for the prior payment item for the referenced payable. 311 */ 312 @Child(name = "predecessor", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 313 @Description(shortDefinition="Business identifier of the prior payment detail", formalDefinition="Unique identifier for the prior payment item for the referenced payable." ) 314 protected Identifier predecessor; 315 316 /** 317 * Specific resource to which the payment/adjustment/advance applies. 318 */ 319 @Child(name = "target", type = {Claim.class, Account.class, Invoice.class, ChargeItem.class, Encounter.class, Contract.class}, order=3, min=0, max=1, modifier=false, summary=false) 320 @Description(shortDefinition="Subject of the payment", formalDefinition="Specific resource to which the payment/adjustment/advance applies." ) 321 protected Reference target; 322 323 /** 324 * Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred. 325 */ 326 @Child(name = "targetItem", type = {StringType.class, Identifier.class, PositiveIntType.class}, order=4, min=0, max=1, modifier=false, summary=false) 327 @Description(shortDefinition="Sub-element of the subject", formalDefinition=" Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred." ) 328 protected DataType targetItem; 329 330 /** 331 * The Encounter to which this payment applies, may be completed by the receiver, used for search. 332 */ 333 @Child(name = "encounter", type = {Encounter.class}, order=5, min=0, max=1, modifier=false, summary=false) 334 @Description(shortDefinition="Applied-to encounter", formalDefinition="The Encounter to which this payment applies, may be completed by the receiver, used for search." ) 335 protected Reference encounter; 336 337 /** 338 * The Account to which this payment applies, may be completed by the receiver, used for search. 339 */ 340 @Child(name = "account", type = {Account.class}, order=6, min=0, max=1, modifier=false, summary=false) 341 @Description(shortDefinition="Applied-to account", formalDefinition="The Account to which this payment applies, may be completed by the receiver, used for search." ) 342 protected Reference account; 343 344 /** 345 * Code to indicate the nature of the payment. 346 */ 347 @Child(name = "type", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 348 @Description(shortDefinition="Category of payment", formalDefinition="Code to indicate the nature of the payment." ) 349 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-type") 350 protected CodeableConcept type; 351 352 /** 353 * The party which submitted the claim or financial transaction. 354 */ 355 @Child(name = "submitter", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 356 @Description(shortDefinition="Submitter of the request", formalDefinition="The party which submitted the claim or financial transaction." ) 357 protected Reference submitter; 358 359 /** 360 * A resource, such as a ClaimResponse, which contains a commitment to payment. 361 */ 362 @Child(name = "response", type = {ClaimResponse.class}, order=9, min=0, max=1, modifier=false, summary=false) 363 @Description(shortDefinition="Response committing to a payment", formalDefinition="A resource, such as a ClaimResponse, which contains a commitment to payment." ) 364 protected Reference response; 365 366 /** 367 * The date from the response resource containing a commitment to pay. 368 */ 369 @Child(name = "date", type = {DateType.class}, order=10, min=0, max=1, modifier=false, summary=false) 370 @Description(shortDefinition="Date of commitment to pay", formalDefinition="The date from the response resource containing a commitment to pay." ) 371 protected DateType date; 372 373 /** 374 * A reference to the individual who is responsible for inquiries regarding the response and its payment. 375 */ 376 @Child(name = "responsible", type = {PractitionerRole.class}, order=11, min=0, max=1, modifier=false, summary=false) 377 @Description(shortDefinition="Contact for the response", formalDefinition="A reference to the individual who is responsible for inquiries regarding the response and its payment." ) 378 protected Reference responsible; 379 380 /** 381 * The party which is receiving the payment. 382 */ 383 @Child(name = "payee", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=12, min=0, max=1, modifier=false, summary=false) 384 @Description(shortDefinition="Recipient of the payment", formalDefinition="The party which is receiving the payment." ) 385 protected Reference payee; 386 387 /** 388 * The monetary amount allocated from the total payment to the payable. 389 */ 390 @Child(name = "amount", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 391 @Description(shortDefinition="Amount allocated to this payable", formalDefinition="The monetary amount allocated from the total payment to the payable." ) 392 protected Money amount; 393 394 private static final long serialVersionUID = -1153705409L; 395 396 /** 397 * Constructor 398 */ 399 public PaymentReconciliationAllocationComponent() { 400 super(); 401 } 402 403 /** 404 * @return {@link #identifier} (Unique identifier for the current payment item for the referenced payable.) 405 */ 406 public Identifier getIdentifier() { 407 if (this.identifier == null) 408 if (Configuration.errorOnAutoCreate()) 409 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.identifier"); 410 else if (Configuration.doAutoCreate()) 411 this.identifier = new Identifier(); // cc 412 return this.identifier; 413 } 414 415 public boolean hasIdentifier() { 416 return this.identifier != null && !this.identifier.isEmpty(); 417 } 418 419 /** 420 * @param value {@link #identifier} (Unique identifier for the current payment item for the referenced payable.) 421 */ 422 public PaymentReconciliationAllocationComponent setIdentifier(Identifier value) { 423 this.identifier = value; 424 return this; 425 } 426 427 /** 428 * @return {@link #predecessor} (Unique identifier for the prior payment item for the referenced payable.) 429 */ 430 public Identifier getPredecessor() { 431 if (this.predecessor == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.predecessor"); 434 else if (Configuration.doAutoCreate()) 435 this.predecessor = new Identifier(); // cc 436 return this.predecessor; 437 } 438 439 public boolean hasPredecessor() { 440 return this.predecessor != null && !this.predecessor.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #predecessor} (Unique identifier for the prior payment item for the referenced payable.) 445 */ 446 public PaymentReconciliationAllocationComponent setPredecessor(Identifier value) { 447 this.predecessor = value; 448 return this; 449 } 450 451 /** 452 * @return {@link #target} (Specific resource to which the payment/adjustment/advance applies.) 453 */ 454 public Reference getTarget() { 455 if (this.target == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.target"); 458 else if (Configuration.doAutoCreate()) 459 this.target = new Reference(); // cc 460 return this.target; 461 } 462 463 public boolean hasTarget() { 464 return this.target != null && !this.target.isEmpty(); 465 } 466 467 /** 468 * @param value {@link #target} (Specific resource to which the payment/adjustment/advance applies.) 469 */ 470 public PaymentReconciliationAllocationComponent setTarget(Reference value) { 471 this.target = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #targetItem} ( Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.) 477 */ 478 public DataType getTargetItem() { 479 return this.targetItem; 480 } 481 482 /** 483 * @return {@link #targetItem} ( Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.) 484 */ 485 public StringType getTargetItemStringType() throws FHIRException { 486 if (this.targetItem == null) 487 this.targetItem = new StringType(); 488 if (!(this.targetItem instanceof StringType)) 489 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.targetItem.getClass().getName()+" was encountered"); 490 return (StringType) this.targetItem; 491 } 492 493 public boolean hasTargetItemStringType() { 494 return this.targetItem instanceof StringType; 495 } 496 497 /** 498 * @return {@link #targetItem} ( Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.) 499 */ 500 public Identifier getTargetItemIdentifier() throws FHIRException { 501 if (this.targetItem == null) 502 this.targetItem = new Identifier(); 503 if (!(this.targetItem instanceof Identifier)) 504 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.targetItem.getClass().getName()+" was encountered"); 505 return (Identifier) this.targetItem; 506 } 507 508 public boolean hasTargetItemIdentifier() { 509 return this.targetItem instanceof Identifier; 510 } 511 512 /** 513 * @return {@link #targetItem} ( Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.) 514 */ 515 public PositiveIntType getTargetItemPositiveIntType() throws FHIRException { 516 if (this.targetItem == null) 517 this.targetItem = new PositiveIntType(); 518 if (!(this.targetItem instanceof PositiveIntType)) 519 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.targetItem.getClass().getName()+" was encountered"); 520 return (PositiveIntType) this.targetItem; 521 } 522 523 public boolean hasTargetItemPositiveIntType() { 524 return this.targetItem instanceof PositiveIntType; 525 } 526 527 public boolean hasTargetItem() { 528 return this.targetItem != null && !this.targetItem.isEmpty(); 529 } 530 531 /** 532 * @param value {@link #targetItem} ( Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.) 533 */ 534 public PaymentReconciliationAllocationComponent setTargetItem(DataType value) { 535 if (value != null && !(value instanceof StringType || value instanceof Identifier || value instanceof PositiveIntType)) 536 throw new FHIRException("Not the right type for PaymentReconciliation.allocation.targetItem[x]: "+value.fhirType()); 537 this.targetItem = value; 538 return this; 539 } 540 541 /** 542 * @return {@link #encounter} (The Encounter to which this payment applies, may be completed by the receiver, used for search.) 543 */ 544 public Reference getEncounter() { 545 if (this.encounter == null) 546 if (Configuration.errorOnAutoCreate()) 547 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.encounter"); 548 else if (Configuration.doAutoCreate()) 549 this.encounter = new Reference(); // cc 550 return this.encounter; 551 } 552 553 public boolean hasEncounter() { 554 return this.encounter != null && !this.encounter.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #encounter} (The Encounter to which this payment applies, may be completed by the receiver, used for search.) 559 */ 560 public PaymentReconciliationAllocationComponent setEncounter(Reference value) { 561 this.encounter = value; 562 return this; 563 } 564 565 /** 566 * @return {@link #account} (The Account to which this payment applies, may be completed by the receiver, used for search.) 567 */ 568 public Reference getAccount() { 569 if (this.account == null) 570 if (Configuration.errorOnAutoCreate()) 571 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.account"); 572 else if (Configuration.doAutoCreate()) 573 this.account = new Reference(); // cc 574 return this.account; 575 } 576 577 public boolean hasAccount() { 578 return this.account != null && !this.account.isEmpty(); 579 } 580 581 /** 582 * @param value {@link #account} (The Account to which this payment applies, may be completed by the receiver, used for search.) 583 */ 584 public PaymentReconciliationAllocationComponent setAccount(Reference value) { 585 this.account = value; 586 return this; 587 } 588 589 /** 590 * @return {@link #type} (Code to indicate the nature of the payment.) 591 */ 592 public CodeableConcept getType() { 593 if (this.type == null) 594 if (Configuration.errorOnAutoCreate()) 595 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.type"); 596 else if (Configuration.doAutoCreate()) 597 this.type = new CodeableConcept(); // cc 598 return this.type; 599 } 600 601 public boolean hasType() { 602 return this.type != null && !this.type.isEmpty(); 603 } 604 605 /** 606 * @param value {@link #type} (Code to indicate the nature of the payment.) 607 */ 608 public PaymentReconciliationAllocationComponent setType(CodeableConcept value) { 609 this.type = value; 610 return this; 611 } 612 613 /** 614 * @return {@link #submitter} (The party which submitted the claim or financial transaction.) 615 */ 616 public Reference getSubmitter() { 617 if (this.submitter == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.submitter"); 620 else if (Configuration.doAutoCreate()) 621 this.submitter = new Reference(); // cc 622 return this.submitter; 623 } 624 625 public boolean hasSubmitter() { 626 return this.submitter != null && !this.submitter.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #submitter} (The party which submitted the claim or financial transaction.) 631 */ 632 public PaymentReconciliationAllocationComponent setSubmitter(Reference value) { 633 this.submitter = value; 634 return this; 635 } 636 637 /** 638 * @return {@link #response} (A resource, such as a ClaimResponse, which contains a commitment to payment.) 639 */ 640 public Reference getResponse() { 641 if (this.response == null) 642 if (Configuration.errorOnAutoCreate()) 643 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.response"); 644 else if (Configuration.doAutoCreate()) 645 this.response = new Reference(); // cc 646 return this.response; 647 } 648 649 public boolean hasResponse() { 650 return this.response != null && !this.response.isEmpty(); 651 } 652 653 /** 654 * @param value {@link #response} (A resource, such as a ClaimResponse, which contains a commitment to payment.) 655 */ 656 public PaymentReconciliationAllocationComponent setResponse(Reference value) { 657 this.response = value; 658 return this; 659 } 660 661 /** 662 * @return {@link #date} (The date from the response resource containing a commitment to pay.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 663 */ 664 public DateType getDateElement() { 665 if (this.date == null) 666 if (Configuration.errorOnAutoCreate()) 667 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.date"); 668 else if (Configuration.doAutoCreate()) 669 this.date = new DateType(); // bb 670 return this.date; 671 } 672 673 public boolean hasDateElement() { 674 return this.date != null && !this.date.isEmpty(); 675 } 676 677 public boolean hasDate() { 678 return this.date != null && !this.date.isEmpty(); 679 } 680 681 /** 682 * @param value {@link #date} (The date from the response resource containing a commitment to pay.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 683 */ 684 public PaymentReconciliationAllocationComponent setDateElement(DateType value) { 685 this.date = value; 686 return this; 687 } 688 689 /** 690 * @return The date from the response resource containing a commitment to pay. 691 */ 692 public Date getDate() { 693 return this.date == null ? null : this.date.getValue(); 694 } 695 696 /** 697 * @param value The date from the response resource containing a commitment to pay. 698 */ 699 public PaymentReconciliationAllocationComponent setDate(Date value) { 700 if (value == null) 701 this.date = null; 702 else { 703 if (this.date == null) 704 this.date = new DateType(); 705 this.date.setValue(value); 706 } 707 return this; 708 } 709 710 /** 711 * @return {@link #responsible} (A reference to the individual who is responsible for inquiries regarding the response and its payment.) 712 */ 713 public Reference getResponsible() { 714 if (this.responsible == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.responsible"); 717 else if (Configuration.doAutoCreate()) 718 this.responsible = new Reference(); // cc 719 return this.responsible; 720 } 721 722 public boolean hasResponsible() { 723 return this.responsible != null && !this.responsible.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #responsible} (A reference to the individual who is responsible for inquiries regarding the response and its payment.) 728 */ 729 public PaymentReconciliationAllocationComponent setResponsible(Reference value) { 730 this.responsible = value; 731 return this; 732 } 733 734 /** 735 * @return {@link #payee} (The party which is receiving the payment.) 736 */ 737 public Reference getPayee() { 738 if (this.payee == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.payee"); 741 else if (Configuration.doAutoCreate()) 742 this.payee = new Reference(); // cc 743 return this.payee; 744 } 745 746 public boolean hasPayee() { 747 return this.payee != null && !this.payee.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #payee} (The party which is receiving the payment.) 752 */ 753 public PaymentReconciliationAllocationComponent setPayee(Reference value) { 754 this.payee = value; 755 return this; 756 } 757 758 /** 759 * @return {@link #amount} (The monetary amount allocated from the total payment to the payable.) 760 */ 761 public Money getAmount() { 762 if (this.amount == null) 763 if (Configuration.errorOnAutoCreate()) 764 throw new Error("Attempt to auto-create PaymentReconciliationAllocationComponent.amount"); 765 else if (Configuration.doAutoCreate()) 766 this.amount = new Money(); // cc 767 return this.amount; 768 } 769 770 public boolean hasAmount() { 771 return this.amount != null && !this.amount.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #amount} (The monetary amount allocated from the total payment to the payable.) 776 */ 777 public PaymentReconciliationAllocationComponent setAmount(Money value) { 778 this.amount = value; 779 return this; 780 } 781 782 protected void listChildren(List<Property> children) { 783 super.listChildren(children); 784 children.add(new Property("identifier", "Identifier", "Unique identifier for the current payment item for the referenced payable.", 0, 1, identifier)); 785 children.add(new Property("predecessor", "Identifier", "Unique identifier for the prior payment item for the referenced payable.", 0, 1, predecessor)); 786 children.add(new Property("target", "Reference(Claim|Account|Invoice|ChargeItem|Encounter|Contract)", "Specific resource to which the payment/adjustment/advance applies.", 0, 1, target)); 787 children.add(new Property("targetItem[x]", "string|Identifier|positiveInt", " Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.", 0, 1, targetItem)); 788 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter to which this payment applies, may be completed by the receiver, used for search.", 0, 1, encounter)); 789 children.add(new Property("account", "Reference(Account)", "The Account to which this payment applies, may be completed by the receiver, used for search.", 0, 1, account)); 790 children.add(new Property("type", "CodeableConcept", "Code to indicate the nature of the payment.", 0, 1, type)); 791 children.add(new Property("submitter", "Reference(Practitioner|PractitionerRole|Organization)", "The party which submitted the claim or financial transaction.", 0, 1, submitter)); 792 children.add(new Property("response", "Reference(ClaimResponse)", "A resource, such as a ClaimResponse, which contains a commitment to payment.", 0, 1, response)); 793 children.add(new Property("date", "date", "The date from the response resource containing a commitment to pay.", 0, 1, date)); 794 children.add(new Property("responsible", "Reference(PractitionerRole)", "A reference to the individual who is responsible for inquiries regarding the response and its payment.", 0, 1, responsible)); 795 children.add(new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", "The party which is receiving the payment.", 0, 1, payee)); 796 children.add(new Property("amount", "Money", "The monetary amount allocated from the total payment to the payable.", 0, 1, amount)); 797 } 798 799 @Override 800 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 801 switch (_hash) { 802 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for the current payment item for the referenced payable.", 0, 1, identifier); 803 case -1925032183: /*predecessor*/ return new Property("predecessor", "Identifier", "Unique identifier for the prior payment item for the referenced payable.", 0, 1, predecessor); 804 case -880905839: /*target*/ return new Property("target", "Reference(Claim|Account|Invoice|ChargeItem|Encounter|Contract)", "Specific resource to which the payment/adjustment/advance applies.", 0, 1, target); 805 case 125181372: /*targetItem[x]*/ return new Property("targetItem[x]", "string|Identifier|positiveInt", " Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.", 0, 1, targetItem); 806 case 486289476: /*targetItem*/ return new Property("targetItem[x]", "string|Identifier|positiveInt", " Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.", 0, 1, targetItem); 807 case 1014643061: /*targetItemString*/ return new Property("targetItem[x]", "string", " Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.", 0, 1, targetItem); 808 case -1768279027: /*targetItemIdentifier*/ return new Property("targetItem[x]", "Identifier", " Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.", 0, 1, targetItem); 809 case -481526702: /*targetItemPositiveInt*/ return new Property("targetItem[x]", "positiveInt", " Identifies the claim line item, encounter or other sub-element being paid. Note payment may be partial, that is not match the then outstanding balance or amount incurred.", 0, 1, targetItem); 810 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter to which this payment applies, may be completed by the receiver, used for search.", 0, 1, encounter); 811 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "The Account to which this payment applies, may be completed by the receiver, used for search.", 0, 1, account); 812 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code to indicate the nature of the payment.", 0, 1, type); 813 case 348678409: /*submitter*/ return new Property("submitter", "Reference(Practitioner|PractitionerRole|Organization)", "The party which submitted the claim or financial transaction.", 0, 1, submitter); 814 case -340323263: /*response*/ return new Property("response", "Reference(ClaimResponse)", "A resource, such as a ClaimResponse, which contains a commitment to payment.", 0, 1, response); 815 case 3076014: /*date*/ return new Property("date", "date", "The date from the response resource containing a commitment to pay.", 0, 1, date); 816 case 1847674614: /*responsible*/ return new Property("responsible", "Reference(PractitionerRole)", "A reference to the individual who is responsible for inquiries regarding the response and its payment.", 0, 1, responsible); 817 case 106443592: /*payee*/ return new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", "The party which is receiving the payment.", 0, 1, payee); 818 case -1413853096: /*amount*/ return new Property("amount", "Money", "The monetary amount allocated from the total payment to the payable.", 0, 1, amount); 819 default: return super.getNamedProperty(_hash, _name, _checkValid); 820 } 821 822 } 823 824 @Override 825 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 826 switch (hash) { 827 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 828 case -1925032183: /*predecessor*/ return this.predecessor == null ? new Base[0] : new Base[] {this.predecessor}; // Identifier 829 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 830 case 486289476: /*targetItem*/ return this.targetItem == null ? new Base[0] : new Base[] {this.targetItem}; // DataType 831 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 832 case -1177318867: /*account*/ return this.account == null ? new Base[0] : new Base[] {this.account}; // Reference 833 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 834 case 348678409: /*submitter*/ return this.submitter == null ? new Base[0] : new Base[] {this.submitter}; // Reference 835 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Reference 836 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 837 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // Reference 838 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // Reference 839 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 840 default: return super.getProperty(hash, name, checkValid); 841 } 842 843 } 844 845 @Override 846 public Base setProperty(int hash, String name, Base value) throws FHIRException { 847 switch (hash) { 848 case -1618432855: // identifier 849 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 850 return value; 851 case -1925032183: // predecessor 852 this.predecessor = TypeConvertor.castToIdentifier(value); // Identifier 853 return value; 854 case -880905839: // target 855 this.target = TypeConvertor.castToReference(value); // Reference 856 return value; 857 case 486289476: // targetItem 858 this.targetItem = TypeConvertor.castToType(value); // DataType 859 return value; 860 case 1524132147: // encounter 861 this.encounter = TypeConvertor.castToReference(value); // Reference 862 return value; 863 case -1177318867: // account 864 this.account = TypeConvertor.castToReference(value); // Reference 865 return value; 866 case 3575610: // type 867 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 868 return value; 869 case 348678409: // submitter 870 this.submitter = TypeConvertor.castToReference(value); // Reference 871 return value; 872 case -340323263: // response 873 this.response = TypeConvertor.castToReference(value); // Reference 874 return value; 875 case 3076014: // date 876 this.date = TypeConvertor.castToDate(value); // DateType 877 return value; 878 case 1847674614: // responsible 879 this.responsible = TypeConvertor.castToReference(value); // Reference 880 return value; 881 case 106443592: // payee 882 this.payee = TypeConvertor.castToReference(value); // Reference 883 return value; 884 case -1413853096: // amount 885 this.amount = TypeConvertor.castToMoney(value); // Money 886 return value; 887 default: return super.setProperty(hash, name, value); 888 } 889 890 } 891 892 @Override 893 public Base setProperty(String name, Base value) throws FHIRException { 894 if (name.equals("identifier")) { 895 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 896 } else if (name.equals("predecessor")) { 897 this.predecessor = TypeConvertor.castToIdentifier(value); // Identifier 898 } else if (name.equals("target")) { 899 this.target = TypeConvertor.castToReference(value); // Reference 900 } else if (name.equals("targetItem[x]")) { 901 this.targetItem = TypeConvertor.castToType(value); // DataType 902 } else if (name.equals("encounter")) { 903 this.encounter = TypeConvertor.castToReference(value); // Reference 904 } else if (name.equals("account")) { 905 this.account = TypeConvertor.castToReference(value); // Reference 906 } else if (name.equals("type")) { 907 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 908 } else if (name.equals("submitter")) { 909 this.submitter = TypeConvertor.castToReference(value); // Reference 910 } else if (name.equals("response")) { 911 this.response = TypeConvertor.castToReference(value); // Reference 912 } else if (name.equals("date")) { 913 this.date = TypeConvertor.castToDate(value); // DateType 914 } else if (name.equals("responsible")) { 915 this.responsible = TypeConvertor.castToReference(value); // Reference 916 } else if (name.equals("payee")) { 917 this.payee = TypeConvertor.castToReference(value); // Reference 918 } else if (name.equals("amount")) { 919 this.amount = TypeConvertor.castToMoney(value); // Money 920 } else 921 return super.setProperty(name, value); 922 return value; 923 } 924 925 @Override 926 public void removeChild(String name, Base value) throws FHIRException { 927 if (name.equals("identifier")) { 928 this.identifier = null; 929 } else if (name.equals("predecessor")) { 930 this.predecessor = null; 931 } else if (name.equals("target")) { 932 this.target = null; 933 } else if (name.equals("targetItem[x]")) { 934 this.targetItem = null; 935 } else if (name.equals("encounter")) { 936 this.encounter = null; 937 } else if (name.equals("account")) { 938 this.account = null; 939 } else if (name.equals("type")) { 940 this.type = null; 941 } else if (name.equals("submitter")) { 942 this.submitter = null; 943 } else if (name.equals("response")) { 944 this.response = null; 945 } else if (name.equals("date")) { 946 this.date = null; 947 } else if (name.equals("responsible")) { 948 this.responsible = null; 949 } else if (name.equals("payee")) { 950 this.payee = null; 951 } else if (name.equals("amount")) { 952 this.amount = null; 953 } else 954 super.removeChild(name, value); 955 956 } 957 958 @Override 959 public Base makeProperty(int hash, String name) throws FHIRException { 960 switch (hash) { 961 case -1618432855: return getIdentifier(); 962 case -1925032183: return getPredecessor(); 963 case -880905839: return getTarget(); 964 case 125181372: return getTargetItem(); 965 case 486289476: return getTargetItem(); 966 case 1524132147: return getEncounter(); 967 case -1177318867: return getAccount(); 968 case 3575610: return getType(); 969 case 348678409: return getSubmitter(); 970 case -340323263: return getResponse(); 971 case 3076014: return getDateElement(); 972 case 1847674614: return getResponsible(); 973 case 106443592: return getPayee(); 974 case -1413853096: return getAmount(); 975 default: return super.makeProperty(hash, name); 976 } 977 978 } 979 980 @Override 981 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 982 switch (hash) { 983 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 984 case -1925032183: /*predecessor*/ return new String[] {"Identifier"}; 985 case -880905839: /*target*/ return new String[] {"Reference"}; 986 case 486289476: /*targetItem*/ return new String[] {"string", "Identifier", "positiveInt"}; 987 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 988 case -1177318867: /*account*/ return new String[] {"Reference"}; 989 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 990 case 348678409: /*submitter*/ return new String[] {"Reference"}; 991 case -340323263: /*response*/ return new String[] {"Reference"}; 992 case 3076014: /*date*/ return new String[] {"date"}; 993 case 1847674614: /*responsible*/ return new String[] {"Reference"}; 994 case 106443592: /*payee*/ return new String[] {"Reference"}; 995 case -1413853096: /*amount*/ return new String[] {"Money"}; 996 default: return super.getTypesForProperty(hash, name); 997 } 998 999 } 1000 1001 @Override 1002 public Base addChild(String name) throws FHIRException { 1003 if (name.equals("identifier")) { 1004 this.identifier = new Identifier(); 1005 return this.identifier; 1006 } 1007 else if (name.equals("predecessor")) { 1008 this.predecessor = new Identifier(); 1009 return this.predecessor; 1010 } 1011 else if (name.equals("target")) { 1012 this.target = new Reference(); 1013 return this.target; 1014 } 1015 else if (name.equals("targetItemString")) { 1016 this.targetItem = new StringType(); 1017 return this.targetItem; 1018 } 1019 else if (name.equals("targetItemIdentifier")) { 1020 this.targetItem = new Identifier(); 1021 return this.targetItem; 1022 } 1023 else if (name.equals("targetItemPositiveInt")) { 1024 this.targetItem = new PositiveIntType(); 1025 return this.targetItem; 1026 } 1027 else if (name.equals("encounter")) { 1028 this.encounter = new Reference(); 1029 return this.encounter; 1030 } 1031 else if (name.equals("account")) { 1032 this.account = new Reference(); 1033 return this.account; 1034 } 1035 else if (name.equals("type")) { 1036 this.type = new CodeableConcept(); 1037 return this.type; 1038 } 1039 else if (name.equals("submitter")) { 1040 this.submitter = new Reference(); 1041 return this.submitter; 1042 } 1043 else if (name.equals("response")) { 1044 this.response = new Reference(); 1045 return this.response; 1046 } 1047 else if (name.equals("date")) { 1048 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.allocation.date"); 1049 } 1050 else if (name.equals("responsible")) { 1051 this.responsible = new Reference(); 1052 return this.responsible; 1053 } 1054 else if (name.equals("payee")) { 1055 this.payee = new Reference(); 1056 return this.payee; 1057 } 1058 else if (name.equals("amount")) { 1059 this.amount = new Money(); 1060 return this.amount; 1061 } 1062 else 1063 return super.addChild(name); 1064 } 1065 1066 public PaymentReconciliationAllocationComponent copy() { 1067 PaymentReconciliationAllocationComponent dst = new PaymentReconciliationAllocationComponent(); 1068 copyValues(dst); 1069 return dst; 1070 } 1071 1072 public void copyValues(PaymentReconciliationAllocationComponent dst) { 1073 super.copyValues(dst); 1074 dst.identifier = identifier == null ? null : identifier.copy(); 1075 dst.predecessor = predecessor == null ? null : predecessor.copy(); 1076 dst.target = target == null ? null : target.copy(); 1077 dst.targetItem = targetItem == null ? null : targetItem.copy(); 1078 dst.encounter = encounter == null ? null : encounter.copy(); 1079 dst.account = account == null ? null : account.copy(); 1080 dst.type = type == null ? null : type.copy(); 1081 dst.submitter = submitter == null ? null : submitter.copy(); 1082 dst.response = response == null ? null : response.copy(); 1083 dst.date = date == null ? null : date.copy(); 1084 dst.responsible = responsible == null ? null : responsible.copy(); 1085 dst.payee = payee == null ? null : payee.copy(); 1086 dst.amount = amount == null ? null : amount.copy(); 1087 } 1088 1089 @Override 1090 public boolean equalsDeep(Base other_) { 1091 if (!super.equalsDeep(other_)) 1092 return false; 1093 if (!(other_ instanceof PaymentReconciliationAllocationComponent)) 1094 return false; 1095 PaymentReconciliationAllocationComponent o = (PaymentReconciliationAllocationComponent) other_; 1096 return compareDeep(identifier, o.identifier, true) && compareDeep(predecessor, o.predecessor, true) 1097 && compareDeep(target, o.target, true) && compareDeep(targetItem, o.targetItem, true) && compareDeep(encounter, o.encounter, true) 1098 && compareDeep(account, o.account, true) && compareDeep(type, o.type, true) && compareDeep(submitter, o.submitter, true) 1099 && compareDeep(response, o.response, true) && compareDeep(date, o.date, true) && compareDeep(responsible, o.responsible, true) 1100 && compareDeep(payee, o.payee, true) && compareDeep(amount, o.amount, true); 1101 } 1102 1103 @Override 1104 public boolean equalsShallow(Base other_) { 1105 if (!super.equalsShallow(other_)) 1106 return false; 1107 if (!(other_ instanceof PaymentReconciliationAllocationComponent)) 1108 return false; 1109 PaymentReconciliationAllocationComponent o = (PaymentReconciliationAllocationComponent) other_; 1110 return compareValues(date, o.date, true); 1111 } 1112 1113 public boolean isEmpty() { 1114 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, predecessor, target 1115 , targetItem, encounter, account, type, submitter, response, date, responsible 1116 , payee, amount); 1117 } 1118 1119 public String fhirType() { 1120 return "PaymentReconciliation.allocation"; 1121 1122 } 1123 1124 } 1125 1126 @Block() 1127 public static class NotesComponent extends BackboneElement implements IBaseBackboneElement { 1128 /** 1129 * The business purpose of the note text. 1130 */ 1131 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1132 @Description(shortDefinition="display | print | printoper", formalDefinition="The business purpose of the note text." ) 1133 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 1134 protected Enumeration<NoteType> type; 1135 1136 /** 1137 * The explanation or description associated with the processing. 1138 */ 1139 @Child(name = "text", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1140 @Description(shortDefinition="Note explanatory text", formalDefinition="The explanation or description associated with the processing." ) 1141 protected StringType text; 1142 1143 private static final long serialVersionUID = 529250161L; 1144 1145 /** 1146 * Constructor 1147 */ 1148 public NotesComponent() { 1149 super(); 1150 } 1151 1152 /** 1153 * @return {@link #type} (The business purpose of the note text.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1154 */ 1155 public Enumeration<NoteType> getTypeElement() { 1156 if (this.type == null) 1157 if (Configuration.errorOnAutoCreate()) 1158 throw new Error("Attempt to auto-create NotesComponent.type"); 1159 else if (Configuration.doAutoCreate()) 1160 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); // bb 1161 return this.type; 1162 } 1163 1164 public boolean hasTypeElement() { 1165 return this.type != null && !this.type.isEmpty(); 1166 } 1167 1168 public boolean hasType() { 1169 return this.type != null && !this.type.isEmpty(); 1170 } 1171 1172 /** 1173 * @param value {@link #type} (The business purpose of the note text.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1174 */ 1175 public NotesComponent setTypeElement(Enumeration<NoteType> value) { 1176 this.type = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return The business purpose of the note text. 1182 */ 1183 public NoteType getType() { 1184 return this.type == null ? null : this.type.getValue(); 1185 } 1186 1187 /** 1188 * @param value The business purpose of the note text. 1189 */ 1190 public NotesComponent setType(NoteType value) { 1191 if (value == null) 1192 this.type = null; 1193 else { 1194 if (this.type == null) 1195 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); 1196 this.type.setValue(value); 1197 } 1198 return this; 1199 } 1200 1201 /** 1202 * @return {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 1203 */ 1204 public StringType getTextElement() { 1205 if (this.text == null) 1206 if (Configuration.errorOnAutoCreate()) 1207 throw new Error("Attempt to auto-create NotesComponent.text"); 1208 else if (Configuration.doAutoCreate()) 1209 this.text = new StringType(); // bb 1210 return this.text; 1211 } 1212 1213 public boolean hasTextElement() { 1214 return this.text != null && !this.text.isEmpty(); 1215 } 1216 1217 public boolean hasText() { 1218 return this.text != null && !this.text.isEmpty(); 1219 } 1220 1221 /** 1222 * @param value {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 1223 */ 1224 public NotesComponent setTextElement(StringType value) { 1225 this.text = value; 1226 return this; 1227 } 1228 1229 /** 1230 * @return The explanation or description associated with the processing. 1231 */ 1232 public String getText() { 1233 return this.text == null ? null : this.text.getValue(); 1234 } 1235 1236 /** 1237 * @param value The explanation or description associated with the processing. 1238 */ 1239 public NotesComponent setText(String value) { 1240 if (Utilities.noString(value)) 1241 this.text = null; 1242 else { 1243 if (this.text == null) 1244 this.text = new StringType(); 1245 this.text.setValue(value); 1246 } 1247 return this; 1248 } 1249 1250 protected void listChildren(List<Property> children) { 1251 super.listChildren(children); 1252 children.add(new Property("type", "code", "The business purpose of the note text.", 0, 1, type)); 1253 children.add(new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 1254 } 1255 1256 @Override 1257 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1258 switch (_hash) { 1259 case 3575610: /*type*/ return new Property("type", "code", "The business purpose of the note text.", 0, 1, type); 1260 case 3556653: /*text*/ return new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text); 1261 default: return super.getNamedProperty(_hash, _name, _checkValid); 1262 } 1263 1264 } 1265 1266 @Override 1267 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1268 switch (hash) { 1269 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<NoteType> 1270 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 1271 default: return super.getProperty(hash, name, checkValid); 1272 } 1273 1274 } 1275 1276 @Override 1277 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1278 switch (hash) { 1279 case 3575610: // type 1280 value = new NoteTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1281 this.type = (Enumeration) value; // Enumeration<NoteType> 1282 return value; 1283 case 3556653: // text 1284 this.text = TypeConvertor.castToString(value); // StringType 1285 return value; 1286 default: return super.setProperty(hash, name, value); 1287 } 1288 1289 } 1290 1291 @Override 1292 public Base setProperty(String name, Base value) throws FHIRException { 1293 if (name.equals("type")) { 1294 value = new NoteTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1295 this.type = (Enumeration) value; // Enumeration<NoteType> 1296 } else if (name.equals("text")) { 1297 this.text = TypeConvertor.castToString(value); // StringType 1298 } else 1299 return super.setProperty(name, value); 1300 return value; 1301 } 1302 1303 @Override 1304 public void removeChild(String name, Base value) throws FHIRException { 1305 if (name.equals("type")) { 1306 value = new NoteTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1307 this.type = (Enumeration) value; // Enumeration<NoteType> 1308 } else if (name.equals("text")) { 1309 this.text = null; 1310 } else 1311 super.removeChild(name, value); 1312 1313 } 1314 1315 @Override 1316 public Base makeProperty(int hash, String name) throws FHIRException { 1317 switch (hash) { 1318 case 3575610: return getTypeElement(); 1319 case 3556653: return getTextElement(); 1320 default: return super.makeProperty(hash, name); 1321 } 1322 1323 } 1324 1325 @Override 1326 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1327 switch (hash) { 1328 case 3575610: /*type*/ return new String[] {"code"}; 1329 case 3556653: /*text*/ return new String[] {"string"}; 1330 default: return super.getTypesForProperty(hash, name); 1331 } 1332 1333 } 1334 1335 @Override 1336 public Base addChild(String name) throws FHIRException { 1337 if (name.equals("type")) { 1338 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.processNote.type"); 1339 } 1340 else if (name.equals("text")) { 1341 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.processNote.text"); 1342 } 1343 else 1344 return super.addChild(name); 1345 } 1346 1347 public NotesComponent copy() { 1348 NotesComponent dst = new NotesComponent(); 1349 copyValues(dst); 1350 return dst; 1351 } 1352 1353 public void copyValues(NotesComponent dst) { 1354 super.copyValues(dst); 1355 dst.type = type == null ? null : type.copy(); 1356 dst.text = text == null ? null : text.copy(); 1357 } 1358 1359 @Override 1360 public boolean equalsDeep(Base other_) { 1361 if (!super.equalsDeep(other_)) 1362 return false; 1363 if (!(other_ instanceof NotesComponent)) 1364 return false; 1365 NotesComponent o = (NotesComponent) other_; 1366 return compareDeep(type, o.type, true) && compareDeep(text, o.text, true); 1367 } 1368 1369 @Override 1370 public boolean equalsShallow(Base other_) { 1371 if (!super.equalsShallow(other_)) 1372 return false; 1373 if (!(other_ instanceof NotesComponent)) 1374 return false; 1375 NotesComponent o = (NotesComponent) other_; 1376 return compareValues(type, o.type, true) && compareValues(text, o.text, true); 1377 } 1378 1379 public boolean isEmpty() { 1380 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, text); 1381 } 1382 1383 public String fhirType() { 1384 return "PaymentReconciliation.processNote"; 1385 1386 } 1387 1388 } 1389 1390 /** 1391 * A unique identifier assigned to this payment reconciliation. 1392 */ 1393 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1394 @Description(shortDefinition="Business Identifier for a payment reconciliation", formalDefinition="A unique identifier assigned to this payment reconciliation." ) 1395 protected List<Identifier> identifier; 1396 1397 /** 1398 * Code to indicate the nature of the payment such as payment, adjustment. 1399 */ 1400 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1401 @Description(shortDefinition="Category of payment", formalDefinition="Code to indicate the nature of the payment such as payment, adjustment." ) 1402 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-type") 1403 protected CodeableConcept type; 1404 1405 /** 1406 * The status of the resource instance. 1407 */ 1408 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1409 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 1410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 1411 protected Enumeration<FinancialResourceStatusCodes> status; 1412 1413 /** 1414 * The workflow or activity which gave rise to or during which the payment ocurred such as a kiosk, deposit on account, periodic payment etc. 1415 */ 1416 @Child(name = "kind", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1417 @Description(shortDefinition="Workflow originating payment", formalDefinition="The workflow or activity which gave rise to or during which the payment ocurred such as a kiosk, deposit on account, periodic payment etc." ) 1418 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-kind") 1419 protected CodeableConcept kind; 1420 1421 /** 1422 * The period of time for which payments have been gathered into this bulk payment for settlement. 1423 */ 1424 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 1425 @Description(shortDefinition="Period covered", formalDefinition="The period of time for which payments have been gathered into this bulk payment for settlement." ) 1426 protected Period period; 1427 1428 /** 1429 * The date when the resource was created. 1430 */ 1431 @Child(name = "created", type = {DateTimeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 1432 @Description(shortDefinition="Creation date", formalDefinition="The date when the resource was created." ) 1433 protected DateTimeType created; 1434 1435 /** 1436 * Payment enterer if not the actual payment issuer. 1437 */ 1438 @Child(name = "enterer", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 1439 @Description(shortDefinition="Who entered the payment", formalDefinition="Payment enterer if not the actual payment issuer." ) 1440 protected Reference enterer; 1441 1442 /** 1443 * The type of the source such as patient or insurance. 1444 */ 1445 @Child(name = "issuerType", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1446 @Description(shortDefinition="Nature of the source", formalDefinition="The type of the source such as patient or insurance." ) 1447 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-issuertype") 1448 protected CodeableConcept issuerType; 1449 1450 /** 1451 * The party who generated the payment. 1452 */ 1453 @Child(name = "paymentIssuer", type = {Organization.class, Patient.class, RelatedPerson.class}, order=8, min=0, max=1, modifier=false, summary=true) 1454 @Description(shortDefinition="Party generating payment", formalDefinition="The party who generated the payment." ) 1455 protected Reference paymentIssuer; 1456 1457 /** 1458 * Original request resource reference. 1459 */ 1460 @Child(name = "request", type = {Task.class}, order=9, min=0, max=1, modifier=false, summary=false) 1461 @Description(shortDefinition="Reference to requesting resource", formalDefinition="Original request resource reference." ) 1462 protected Reference request; 1463 1464 /** 1465 * The practitioner who is responsible for the services rendered to the patient. 1466 */ 1467 @Child(name = "requestor", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 1468 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 1469 protected Reference requestor; 1470 1471 /** 1472 * The outcome of a request for a reconciliation. 1473 */ 1474 @Child(name = "outcome", type = {CodeType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1475 @Description(shortDefinition="queued | complete | error | partial", formalDefinition="The outcome of a request for a reconciliation." ) 1476 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-outcome") 1477 protected Enumeration<PaymentOutcome> outcome; 1478 1479 /** 1480 * A human readable description of the status of the request for the reconciliation. 1481 */ 1482 @Child(name = "disposition", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1483 @Description(shortDefinition="Disposition message", formalDefinition="A human readable description of the status of the request for the reconciliation." ) 1484 protected StringType disposition; 1485 1486 /** 1487 * The date of payment as indicated on the financial instrument. 1488 */ 1489 @Child(name = "date", type = {DateType.class}, order=13, min=1, max=1, modifier=false, summary=true) 1490 @Description(shortDefinition="When payment issued", formalDefinition="The date of payment as indicated on the financial instrument." ) 1491 protected DateType date; 1492 1493 /** 1494 * The location of the site or device for electronic transfers or physical location for cash payments. 1495 */ 1496 @Child(name = "location", type = {Location.class}, order=14, min=0, max=1, modifier=false, summary=false) 1497 @Description(shortDefinition="Where payment collected", formalDefinition="The location of the site or device for electronic transfers or physical location for cash payments." ) 1498 protected Reference location; 1499 1500 /** 1501 * The means of payment such as check, card cash, or electronic funds transfer. 1502 */ 1503 @Child(name = "method", type = {CodeableConcept.class}, order=15, min=0, max=1, modifier=false, summary=false) 1504 @Description(shortDefinition="Payment instrument", formalDefinition="The means of payment such as check, card cash, or electronic funds transfer." ) 1505 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0570") 1506 protected CodeableConcept method; 1507 1508 /** 1509 * The card brand such as debit, Visa, Amex etc. used if a card is the method of payment. 1510 */ 1511 @Child(name = "cardBrand", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1512 @Description(shortDefinition="Type of card", formalDefinition="The card brand such as debit, Visa, Amex etc. used if a card is the method of payment." ) 1513 protected StringType cardBrand; 1514 1515 /** 1516 * A portion of the account number, often the last 4 digits, used for verification not charging purposes. 1517 */ 1518 @Child(name = "accountNumber", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1519 @Description(shortDefinition="Digits for verification", formalDefinition="A portion of the account number, often the last 4 digits, used for verification not charging purposes." ) 1520 protected StringType accountNumber; 1521 1522 /** 1523 * The year and month (YYYY-MM) when the instrument, typically card, expires. 1524 */ 1525 @Child(name = "expirationDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 1526 @Description(shortDefinition="Expiration year-month", formalDefinition="The year and month (YYYY-MM) when the instrument, typically card, expires." ) 1527 protected DateType expirationDate; 1528 1529 /** 1530 * The name of the card processor, etf processor, bank for checks. 1531 */ 1532 @Child(name = "processor", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 1533 @Description(shortDefinition="Processor name", formalDefinition="The name of the card processor, etf processor, bank for checks." ) 1534 protected StringType processor; 1535 1536 /** 1537 * The check number, eft reference, car processor reference. 1538 */ 1539 @Child(name = "referenceNumber", type = {StringType.class}, order=20, min=0, max=1, modifier=false, summary=false) 1540 @Description(shortDefinition="Check number or payment reference", formalDefinition="The check number, eft reference, car processor reference." ) 1541 protected StringType referenceNumber; 1542 1543 /** 1544 * An alphanumeric issued by the processor to confirm the successful issuance of payment. 1545 */ 1546 @Child(name = "authorization", type = {StringType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1547 @Description(shortDefinition="Authorization number", formalDefinition="An alphanumeric issued by the processor to confirm the successful issuance of payment." ) 1548 protected StringType authorization; 1549 1550 /** 1551 * The amount offered by the issuer, typically applies to cash when the issuer provides an amount in bank note denominations equal to or excess of the amount actually being paid. 1552 */ 1553 @Child(name = "tenderedAmount", type = {Money.class}, order=22, min=0, max=1, modifier=false, summary=false) 1554 @Description(shortDefinition="Amount offered by the issuer", formalDefinition="The amount offered by the issuer, typically applies to cash when the issuer provides an amount in bank note denominations equal to or excess of the amount actually being paid." ) 1555 protected Money tenderedAmount; 1556 1557 /** 1558 * The amount returned by the receiver which is excess to the amount payable, often referred to as 'change'. 1559 */ 1560 @Child(name = "returnedAmount", type = {Money.class}, order=23, min=0, max=1, modifier=false, summary=false) 1561 @Description(shortDefinition="Amount returned by the receiver", formalDefinition="The amount returned by the receiver which is excess to the amount payable, often referred to as 'change'." ) 1562 protected Money returnedAmount; 1563 1564 /** 1565 * Total payment amount as indicated on the financial instrument. 1566 */ 1567 @Child(name = "amount", type = {Money.class}, order=24, min=1, max=1, modifier=false, summary=true) 1568 @Description(shortDefinition="Total amount of Payment", formalDefinition="Total payment amount as indicated on the financial instrument." ) 1569 protected Money amount; 1570 1571 /** 1572 * Issuer's unique identifier for the payment instrument. 1573 */ 1574 @Child(name = "paymentIdentifier", type = {Identifier.class}, order=25, min=0, max=1, modifier=false, summary=false) 1575 @Description(shortDefinition="Business identifier for the payment", formalDefinition="Issuer's unique identifier for the payment instrument." ) 1576 protected Identifier paymentIdentifier; 1577 1578 /** 1579 * Distribution of the payment amount for a previously acknowledged payable. 1580 */ 1581 @Child(name = "allocation", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1582 @Description(shortDefinition="Settlement particulars", formalDefinition="Distribution of the payment amount for a previously acknowledged payable." ) 1583 protected List<PaymentReconciliationAllocationComponent> allocation; 1584 1585 /** 1586 * A code for the form to be used for printing the content. 1587 */ 1588 @Child(name = "formCode", type = {CodeableConcept.class}, order=27, min=0, max=1, modifier=false, summary=false) 1589 @Description(shortDefinition="Printed form identifier", formalDefinition="A code for the form to be used for printing the content." ) 1590 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 1591 protected CodeableConcept formCode; 1592 1593 /** 1594 * A note that describes or explains the processing in a human readable form. 1595 */ 1596 @Child(name = "processNote", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1597 @Description(shortDefinition="Note concerning processing", formalDefinition="A note that describes or explains the processing in a human readable form." ) 1598 protected List<NotesComponent> processNote; 1599 1600 private static final long serialVersionUID = 705873820L; 1601 1602 /** 1603 * Constructor 1604 */ 1605 public PaymentReconciliation() { 1606 super(); 1607 } 1608 1609 /** 1610 * Constructor 1611 */ 1612 public PaymentReconciliation(CodeableConcept type, FinancialResourceStatusCodes status, Date created, Date date, Money amount) { 1613 super(); 1614 this.setType(type); 1615 this.setStatus(status); 1616 this.setCreated(created); 1617 this.setDate(date); 1618 this.setAmount(amount); 1619 } 1620 1621 /** 1622 * @return {@link #identifier} (A unique identifier assigned to this payment reconciliation.) 1623 */ 1624 public List<Identifier> getIdentifier() { 1625 if (this.identifier == null) 1626 this.identifier = new ArrayList<Identifier>(); 1627 return this.identifier; 1628 } 1629 1630 /** 1631 * @return Returns a reference to <code>this</code> for easy method chaining 1632 */ 1633 public PaymentReconciliation setIdentifier(List<Identifier> theIdentifier) { 1634 this.identifier = theIdentifier; 1635 return this; 1636 } 1637 1638 public boolean hasIdentifier() { 1639 if (this.identifier == null) 1640 return false; 1641 for (Identifier item : this.identifier) 1642 if (!item.isEmpty()) 1643 return true; 1644 return false; 1645 } 1646 1647 public Identifier addIdentifier() { //3 1648 Identifier t = new Identifier(); 1649 if (this.identifier == null) 1650 this.identifier = new ArrayList<Identifier>(); 1651 this.identifier.add(t); 1652 return t; 1653 } 1654 1655 public PaymentReconciliation addIdentifier(Identifier t) { //3 1656 if (t == null) 1657 return this; 1658 if (this.identifier == null) 1659 this.identifier = new ArrayList<Identifier>(); 1660 this.identifier.add(t); 1661 return this; 1662 } 1663 1664 /** 1665 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1666 */ 1667 public Identifier getIdentifierFirstRep() { 1668 if (getIdentifier().isEmpty()) { 1669 addIdentifier(); 1670 } 1671 return getIdentifier().get(0); 1672 } 1673 1674 /** 1675 * @return {@link #type} (Code to indicate the nature of the payment such as payment, adjustment.) 1676 */ 1677 public CodeableConcept getType() { 1678 if (this.type == null) 1679 if (Configuration.errorOnAutoCreate()) 1680 throw new Error("Attempt to auto-create PaymentReconciliation.type"); 1681 else if (Configuration.doAutoCreate()) 1682 this.type = new CodeableConcept(); // cc 1683 return this.type; 1684 } 1685 1686 public boolean hasType() { 1687 return this.type != null && !this.type.isEmpty(); 1688 } 1689 1690 /** 1691 * @param value {@link #type} (Code to indicate the nature of the payment such as payment, adjustment.) 1692 */ 1693 public PaymentReconciliation setType(CodeableConcept value) { 1694 this.type = value; 1695 return this; 1696 } 1697 1698 /** 1699 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1700 */ 1701 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 1702 if (this.status == null) 1703 if (Configuration.errorOnAutoCreate()) 1704 throw new Error("Attempt to auto-create PaymentReconciliation.status"); 1705 else if (Configuration.doAutoCreate()) 1706 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 1707 return this.status; 1708 } 1709 1710 public boolean hasStatusElement() { 1711 return this.status != null && !this.status.isEmpty(); 1712 } 1713 1714 public boolean hasStatus() { 1715 return this.status != null && !this.status.isEmpty(); 1716 } 1717 1718 /** 1719 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1720 */ 1721 public PaymentReconciliation setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 1722 this.status = value; 1723 return this; 1724 } 1725 1726 /** 1727 * @return The status of the resource instance. 1728 */ 1729 public FinancialResourceStatusCodes getStatus() { 1730 return this.status == null ? null : this.status.getValue(); 1731 } 1732 1733 /** 1734 * @param value The status of the resource instance. 1735 */ 1736 public PaymentReconciliation setStatus(FinancialResourceStatusCodes value) { 1737 if (this.status == null) 1738 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 1739 this.status.setValue(value); 1740 return this; 1741 } 1742 1743 /** 1744 * @return {@link #kind} (The workflow or activity which gave rise to or during which the payment ocurred such as a kiosk, deposit on account, periodic payment etc.) 1745 */ 1746 public CodeableConcept getKind() { 1747 if (this.kind == null) 1748 if (Configuration.errorOnAutoCreate()) 1749 throw new Error("Attempt to auto-create PaymentReconciliation.kind"); 1750 else if (Configuration.doAutoCreate()) 1751 this.kind = new CodeableConcept(); // cc 1752 return this.kind; 1753 } 1754 1755 public boolean hasKind() { 1756 return this.kind != null && !this.kind.isEmpty(); 1757 } 1758 1759 /** 1760 * @param value {@link #kind} (The workflow or activity which gave rise to or during which the payment ocurred such as a kiosk, deposit on account, periodic payment etc.) 1761 */ 1762 public PaymentReconciliation setKind(CodeableConcept value) { 1763 this.kind = value; 1764 return this; 1765 } 1766 1767 /** 1768 * @return {@link #period} (The period of time for which payments have been gathered into this bulk payment for settlement.) 1769 */ 1770 public Period getPeriod() { 1771 if (this.period == null) 1772 if (Configuration.errorOnAutoCreate()) 1773 throw new Error("Attempt to auto-create PaymentReconciliation.period"); 1774 else if (Configuration.doAutoCreate()) 1775 this.period = new Period(); // cc 1776 return this.period; 1777 } 1778 1779 public boolean hasPeriod() { 1780 return this.period != null && !this.period.isEmpty(); 1781 } 1782 1783 /** 1784 * @param value {@link #period} (The period of time for which payments have been gathered into this bulk payment for settlement.) 1785 */ 1786 public PaymentReconciliation setPeriod(Period value) { 1787 this.period = value; 1788 return this; 1789 } 1790 1791 /** 1792 * @return {@link #created} (The date when the resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1793 */ 1794 public DateTimeType getCreatedElement() { 1795 if (this.created == null) 1796 if (Configuration.errorOnAutoCreate()) 1797 throw new Error("Attempt to auto-create PaymentReconciliation.created"); 1798 else if (Configuration.doAutoCreate()) 1799 this.created = new DateTimeType(); // bb 1800 return this.created; 1801 } 1802 1803 public boolean hasCreatedElement() { 1804 return this.created != null && !this.created.isEmpty(); 1805 } 1806 1807 public boolean hasCreated() { 1808 return this.created != null && !this.created.isEmpty(); 1809 } 1810 1811 /** 1812 * @param value {@link #created} (The date when the resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1813 */ 1814 public PaymentReconciliation setCreatedElement(DateTimeType value) { 1815 this.created = value; 1816 return this; 1817 } 1818 1819 /** 1820 * @return The date when the resource was created. 1821 */ 1822 public Date getCreated() { 1823 return this.created == null ? null : this.created.getValue(); 1824 } 1825 1826 /** 1827 * @param value The date when the resource was created. 1828 */ 1829 public PaymentReconciliation setCreated(Date value) { 1830 if (this.created == null) 1831 this.created = new DateTimeType(); 1832 this.created.setValue(value); 1833 return this; 1834 } 1835 1836 /** 1837 * @return {@link #enterer} (Payment enterer if not the actual payment issuer.) 1838 */ 1839 public Reference getEnterer() { 1840 if (this.enterer == null) 1841 if (Configuration.errorOnAutoCreate()) 1842 throw new Error("Attempt to auto-create PaymentReconciliation.enterer"); 1843 else if (Configuration.doAutoCreate()) 1844 this.enterer = new Reference(); // cc 1845 return this.enterer; 1846 } 1847 1848 public boolean hasEnterer() { 1849 return this.enterer != null && !this.enterer.isEmpty(); 1850 } 1851 1852 /** 1853 * @param value {@link #enterer} (Payment enterer if not the actual payment issuer.) 1854 */ 1855 public PaymentReconciliation setEnterer(Reference value) { 1856 this.enterer = value; 1857 return this; 1858 } 1859 1860 /** 1861 * @return {@link #issuerType} (The type of the source such as patient or insurance.) 1862 */ 1863 public CodeableConcept getIssuerType() { 1864 if (this.issuerType == null) 1865 if (Configuration.errorOnAutoCreate()) 1866 throw new Error("Attempt to auto-create PaymentReconciliation.issuerType"); 1867 else if (Configuration.doAutoCreate()) 1868 this.issuerType = new CodeableConcept(); // cc 1869 return this.issuerType; 1870 } 1871 1872 public boolean hasIssuerType() { 1873 return this.issuerType != null && !this.issuerType.isEmpty(); 1874 } 1875 1876 /** 1877 * @param value {@link #issuerType} (The type of the source such as patient or insurance.) 1878 */ 1879 public PaymentReconciliation setIssuerType(CodeableConcept value) { 1880 this.issuerType = value; 1881 return this; 1882 } 1883 1884 /** 1885 * @return {@link #paymentIssuer} (The party who generated the payment.) 1886 */ 1887 public Reference getPaymentIssuer() { 1888 if (this.paymentIssuer == null) 1889 if (Configuration.errorOnAutoCreate()) 1890 throw new Error("Attempt to auto-create PaymentReconciliation.paymentIssuer"); 1891 else if (Configuration.doAutoCreate()) 1892 this.paymentIssuer = new Reference(); // cc 1893 return this.paymentIssuer; 1894 } 1895 1896 public boolean hasPaymentIssuer() { 1897 return this.paymentIssuer != null && !this.paymentIssuer.isEmpty(); 1898 } 1899 1900 /** 1901 * @param value {@link #paymentIssuer} (The party who generated the payment.) 1902 */ 1903 public PaymentReconciliation setPaymentIssuer(Reference value) { 1904 this.paymentIssuer = value; 1905 return this; 1906 } 1907 1908 /** 1909 * @return {@link #request} (Original request resource reference.) 1910 */ 1911 public Reference getRequest() { 1912 if (this.request == null) 1913 if (Configuration.errorOnAutoCreate()) 1914 throw new Error("Attempt to auto-create PaymentReconciliation.request"); 1915 else if (Configuration.doAutoCreate()) 1916 this.request = new Reference(); // cc 1917 return this.request; 1918 } 1919 1920 public boolean hasRequest() { 1921 return this.request != null && !this.request.isEmpty(); 1922 } 1923 1924 /** 1925 * @param value {@link #request} (Original request resource reference.) 1926 */ 1927 public PaymentReconciliation setRequest(Reference value) { 1928 this.request = value; 1929 return this; 1930 } 1931 1932 /** 1933 * @return {@link #requestor} (The practitioner who is responsible for the services rendered to the patient.) 1934 */ 1935 public Reference getRequestor() { 1936 if (this.requestor == null) 1937 if (Configuration.errorOnAutoCreate()) 1938 throw new Error("Attempt to auto-create PaymentReconciliation.requestor"); 1939 else if (Configuration.doAutoCreate()) 1940 this.requestor = new Reference(); // cc 1941 return this.requestor; 1942 } 1943 1944 public boolean hasRequestor() { 1945 return this.requestor != null && !this.requestor.isEmpty(); 1946 } 1947 1948 /** 1949 * @param value {@link #requestor} (The practitioner who is responsible for the services rendered to the patient.) 1950 */ 1951 public PaymentReconciliation setRequestor(Reference value) { 1952 this.requestor = value; 1953 return this; 1954 } 1955 1956 /** 1957 * @return {@link #outcome} (The outcome of a request for a reconciliation.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 1958 */ 1959 public Enumeration<PaymentOutcome> getOutcomeElement() { 1960 if (this.outcome == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create PaymentReconciliation.outcome"); 1963 else if (Configuration.doAutoCreate()) 1964 this.outcome = new Enumeration<PaymentOutcome>(new PaymentOutcomeEnumFactory()); // bb 1965 return this.outcome; 1966 } 1967 1968 public boolean hasOutcomeElement() { 1969 return this.outcome != null && !this.outcome.isEmpty(); 1970 } 1971 1972 public boolean hasOutcome() { 1973 return this.outcome != null && !this.outcome.isEmpty(); 1974 } 1975 1976 /** 1977 * @param value {@link #outcome} (The outcome of a request for a reconciliation.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 1978 */ 1979 public PaymentReconciliation setOutcomeElement(Enumeration<PaymentOutcome> value) { 1980 this.outcome = value; 1981 return this; 1982 } 1983 1984 /** 1985 * @return The outcome of a request for a reconciliation. 1986 */ 1987 public PaymentOutcome getOutcome() { 1988 return this.outcome == null ? null : this.outcome.getValue(); 1989 } 1990 1991 /** 1992 * @param value The outcome of a request for a reconciliation. 1993 */ 1994 public PaymentReconciliation setOutcome(PaymentOutcome value) { 1995 if (value == null) 1996 this.outcome = null; 1997 else { 1998 if (this.outcome == null) 1999 this.outcome = new Enumeration<PaymentOutcome>(new PaymentOutcomeEnumFactory()); 2000 this.outcome.setValue(value); 2001 } 2002 return this; 2003 } 2004 2005 /** 2006 * @return {@link #disposition} (A human readable description of the status of the request for the reconciliation.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 2007 */ 2008 public StringType getDispositionElement() { 2009 if (this.disposition == null) 2010 if (Configuration.errorOnAutoCreate()) 2011 throw new Error("Attempt to auto-create PaymentReconciliation.disposition"); 2012 else if (Configuration.doAutoCreate()) 2013 this.disposition = new StringType(); // bb 2014 return this.disposition; 2015 } 2016 2017 public boolean hasDispositionElement() { 2018 return this.disposition != null && !this.disposition.isEmpty(); 2019 } 2020 2021 public boolean hasDisposition() { 2022 return this.disposition != null && !this.disposition.isEmpty(); 2023 } 2024 2025 /** 2026 * @param value {@link #disposition} (A human readable description of the status of the request for the reconciliation.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 2027 */ 2028 public PaymentReconciliation setDispositionElement(StringType value) { 2029 this.disposition = value; 2030 return this; 2031 } 2032 2033 /** 2034 * @return A human readable description of the status of the request for the reconciliation. 2035 */ 2036 public String getDisposition() { 2037 return this.disposition == null ? null : this.disposition.getValue(); 2038 } 2039 2040 /** 2041 * @param value A human readable description of the status of the request for the reconciliation. 2042 */ 2043 public PaymentReconciliation setDisposition(String value) { 2044 if (Utilities.noString(value)) 2045 this.disposition = null; 2046 else { 2047 if (this.disposition == null) 2048 this.disposition = new StringType(); 2049 this.disposition.setValue(value); 2050 } 2051 return this; 2052 } 2053 2054 /** 2055 * @return {@link #date} (The date of payment as indicated on the financial instrument.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2056 */ 2057 public DateType getDateElement() { 2058 if (this.date == null) 2059 if (Configuration.errorOnAutoCreate()) 2060 throw new Error("Attempt to auto-create PaymentReconciliation.date"); 2061 else if (Configuration.doAutoCreate()) 2062 this.date = new DateType(); // bb 2063 return this.date; 2064 } 2065 2066 public boolean hasDateElement() { 2067 return this.date != null && !this.date.isEmpty(); 2068 } 2069 2070 public boolean hasDate() { 2071 return this.date != null && !this.date.isEmpty(); 2072 } 2073 2074 /** 2075 * @param value {@link #date} (The date of payment as indicated on the financial instrument.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2076 */ 2077 public PaymentReconciliation setDateElement(DateType value) { 2078 this.date = value; 2079 return this; 2080 } 2081 2082 /** 2083 * @return The date of payment as indicated on the financial instrument. 2084 */ 2085 public Date getDate() { 2086 return this.date == null ? null : this.date.getValue(); 2087 } 2088 2089 /** 2090 * @param value The date of payment as indicated on the financial instrument. 2091 */ 2092 public PaymentReconciliation setDate(Date value) { 2093 if (this.date == null) 2094 this.date = new DateType(); 2095 this.date.setValue(value); 2096 return this; 2097 } 2098 2099 /** 2100 * @return {@link #location} (The location of the site or device for electronic transfers or physical location for cash payments.) 2101 */ 2102 public Reference getLocation() { 2103 if (this.location == null) 2104 if (Configuration.errorOnAutoCreate()) 2105 throw new Error("Attempt to auto-create PaymentReconciliation.location"); 2106 else if (Configuration.doAutoCreate()) 2107 this.location = new Reference(); // cc 2108 return this.location; 2109 } 2110 2111 public boolean hasLocation() { 2112 return this.location != null && !this.location.isEmpty(); 2113 } 2114 2115 /** 2116 * @param value {@link #location} (The location of the site or device for electronic transfers or physical location for cash payments.) 2117 */ 2118 public PaymentReconciliation setLocation(Reference value) { 2119 this.location = value; 2120 return this; 2121 } 2122 2123 /** 2124 * @return {@link #method} (The means of payment such as check, card cash, or electronic funds transfer.) 2125 */ 2126 public CodeableConcept getMethod() { 2127 if (this.method == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create PaymentReconciliation.method"); 2130 else if (Configuration.doAutoCreate()) 2131 this.method = new CodeableConcept(); // cc 2132 return this.method; 2133 } 2134 2135 public boolean hasMethod() { 2136 return this.method != null && !this.method.isEmpty(); 2137 } 2138 2139 /** 2140 * @param value {@link #method} (The means of payment such as check, card cash, or electronic funds transfer.) 2141 */ 2142 public PaymentReconciliation setMethod(CodeableConcept value) { 2143 this.method = value; 2144 return this; 2145 } 2146 2147 /** 2148 * @return {@link #cardBrand} (The card brand such as debit, Visa, Amex etc. used if a card is the method of payment.). This is the underlying object with id, value and extensions. The accessor "getCardBrand" gives direct access to the value 2149 */ 2150 public StringType getCardBrandElement() { 2151 if (this.cardBrand == null) 2152 if (Configuration.errorOnAutoCreate()) 2153 throw new Error("Attempt to auto-create PaymentReconciliation.cardBrand"); 2154 else if (Configuration.doAutoCreate()) 2155 this.cardBrand = new StringType(); // bb 2156 return this.cardBrand; 2157 } 2158 2159 public boolean hasCardBrandElement() { 2160 return this.cardBrand != null && !this.cardBrand.isEmpty(); 2161 } 2162 2163 public boolean hasCardBrand() { 2164 return this.cardBrand != null && !this.cardBrand.isEmpty(); 2165 } 2166 2167 /** 2168 * @param value {@link #cardBrand} (The card brand such as debit, Visa, Amex etc. used if a card is the method of payment.). This is the underlying object with id, value and extensions. The accessor "getCardBrand" gives direct access to the value 2169 */ 2170 public PaymentReconciliation setCardBrandElement(StringType value) { 2171 this.cardBrand = value; 2172 return this; 2173 } 2174 2175 /** 2176 * @return The card brand such as debit, Visa, Amex etc. used if a card is the method of payment. 2177 */ 2178 public String getCardBrand() { 2179 return this.cardBrand == null ? null : this.cardBrand.getValue(); 2180 } 2181 2182 /** 2183 * @param value The card brand such as debit, Visa, Amex etc. used if a card is the method of payment. 2184 */ 2185 public PaymentReconciliation setCardBrand(String value) { 2186 if (Utilities.noString(value)) 2187 this.cardBrand = null; 2188 else { 2189 if (this.cardBrand == null) 2190 this.cardBrand = new StringType(); 2191 this.cardBrand.setValue(value); 2192 } 2193 return this; 2194 } 2195 2196 /** 2197 * @return {@link #accountNumber} (A portion of the account number, often the last 4 digits, used for verification not charging purposes.). This is the underlying object with id, value and extensions. The accessor "getAccountNumber" gives direct access to the value 2198 */ 2199 public StringType getAccountNumberElement() { 2200 if (this.accountNumber == null) 2201 if (Configuration.errorOnAutoCreate()) 2202 throw new Error("Attempt to auto-create PaymentReconciliation.accountNumber"); 2203 else if (Configuration.doAutoCreate()) 2204 this.accountNumber = new StringType(); // bb 2205 return this.accountNumber; 2206 } 2207 2208 public boolean hasAccountNumberElement() { 2209 return this.accountNumber != null && !this.accountNumber.isEmpty(); 2210 } 2211 2212 public boolean hasAccountNumber() { 2213 return this.accountNumber != null && !this.accountNumber.isEmpty(); 2214 } 2215 2216 /** 2217 * @param value {@link #accountNumber} (A portion of the account number, often the last 4 digits, used for verification not charging purposes.). This is the underlying object with id, value and extensions. The accessor "getAccountNumber" gives direct access to the value 2218 */ 2219 public PaymentReconciliation setAccountNumberElement(StringType value) { 2220 this.accountNumber = value; 2221 return this; 2222 } 2223 2224 /** 2225 * @return A portion of the account number, often the last 4 digits, used for verification not charging purposes. 2226 */ 2227 public String getAccountNumber() { 2228 return this.accountNumber == null ? null : this.accountNumber.getValue(); 2229 } 2230 2231 /** 2232 * @param value A portion of the account number, often the last 4 digits, used for verification not charging purposes. 2233 */ 2234 public PaymentReconciliation setAccountNumber(String value) { 2235 if (Utilities.noString(value)) 2236 this.accountNumber = null; 2237 else { 2238 if (this.accountNumber == null) 2239 this.accountNumber = new StringType(); 2240 this.accountNumber.setValue(value); 2241 } 2242 return this; 2243 } 2244 2245 /** 2246 * @return {@link #expirationDate} (The year and month (YYYY-MM) when the instrument, typically card, expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2247 */ 2248 public DateType getExpirationDateElement() { 2249 if (this.expirationDate == null) 2250 if (Configuration.errorOnAutoCreate()) 2251 throw new Error("Attempt to auto-create PaymentReconciliation.expirationDate"); 2252 else if (Configuration.doAutoCreate()) 2253 this.expirationDate = new DateType(); // bb 2254 return this.expirationDate; 2255 } 2256 2257 public boolean hasExpirationDateElement() { 2258 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2259 } 2260 2261 public boolean hasExpirationDate() { 2262 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2263 } 2264 2265 /** 2266 * @param value {@link #expirationDate} (The year and month (YYYY-MM) when the instrument, typically card, expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2267 */ 2268 public PaymentReconciliation setExpirationDateElement(DateType value) { 2269 this.expirationDate = value; 2270 return this; 2271 } 2272 2273 /** 2274 * @return The year and month (YYYY-MM) when the instrument, typically card, expires. 2275 */ 2276 public Date getExpirationDate() { 2277 return this.expirationDate == null ? null : this.expirationDate.getValue(); 2278 } 2279 2280 /** 2281 * @param value The year and month (YYYY-MM) when the instrument, typically card, expires. 2282 */ 2283 public PaymentReconciliation setExpirationDate(Date value) { 2284 if (value == null) 2285 this.expirationDate = null; 2286 else { 2287 if (this.expirationDate == null) 2288 this.expirationDate = new DateType(); 2289 this.expirationDate.setValue(value); 2290 } 2291 return this; 2292 } 2293 2294 /** 2295 * @return {@link #processor} (The name of the card processor, etf processor, bank for checks.). This is the underlying object with id, value and extensions. The accessor "getProcessor" gives direct access to the value 2296 */ 2297 public StringType getProcessorElement() { 2298 if (this.processor == null) 2299 if (Configuration.errorOnAutoCreate()) 2300 throw new Error("Attempt to auto-create PaymentReconciliation.processor"); 2301 else if (Configuration.doAutoCreate()) 2302 this.processor = new StringType(); // bb 2303 return this.processor; 2304 } 2305 2306 public boolean hasProcessorElement() { 2307 return this.processor != null && !this.processor.isEmpty(); 2308 } 2309 2310 public boolean hasProcessor() { 2311 return this.processor != null && !this.processor.isEmpty(); 2312 } 2313 2314 /** 2315 * @param value {@link #processor} (The name of the card processor, etf processor, bank for checks.). This is the underlying object with id, value and extensions. The accessor "getProcessor" gives direct access to the value 2316 */ 2317 public PaymentReconciliation setProcessorElement(StringType value) { 2318 this.processor = value; 2319 return this; 2320 } 2321 2322 /** 2323 * @return The name of the card processor, etf processor, bank for checks. 2324 */ 2325 public String getProcessor() { 2326 return this.processor == null ? null : this.processor.getValue(); 2327 } 2328 2329 /** 2330 * @param value The name of the card processor, etf processor, bank for checks. 2331 */ 2332 public PaymentReconciliation setProcessor(String value) { 2333 if (Utilities.noString(value)) 2334 this.processor = null; 2335 else { 2336 if (this.processor == null) 2337 this.processor = new StringType(); 2338 this.processor.setValue(value); 2339 } 2340 return this; 2341 } 2342 2343 /** 2344 * @return {@link #referenceNumber} (The check number, eft reference, car processor reference.). This is the underlying object with id, value and extensions. The accessor "getReferenceNumber" gives direct access to the value 2345 */ 2346 public StringType getReferenceNumberElement() { 2347 if (this.referenceNumber == null) 2348 if (Configuration.errorOnAutoCreate()) 2349 throw new Error("Attempt to auto-create PaymentReconciliation.referenceNumber"); 2350 else if (Configuration.doAutoCreate()) 2351 this.referenceNumber = new StringType(); // bb 2352 return this.referenceNumber; 2353 } 2354 2355 public boolean hasReferenceNumberElement() { 2356 return this.referenceNumber != null && !this.referenceNumber.isEmpty(); 2357 } 2358 2359 public boolean hasReferenceNumber() { 2360 return this.referenceNumber != null && !this.referenceNumber.isEmpty(); 2361 } 2362 2363 /** 2364 * @param value {@link #referenceNumber} (The check number, eft reference, car processor reference.). This is the underlying object with id, value and extensions. The accessor "getReferenceNumber" gives direct access to the value 2365 */ 2366 public PaymentReconciliation setReferenceNumberElement(StringType value) { 2367 this.referenceNumber = value; 2368 return this; 2369 } 2370 2371 /** 2372 * @return The check number, eft reference, car processor reference. 2373 */ 2374 public String getReferenceNumber() { 2375 return this.referenceNumber == null ? null : this.referenceNumber.getValue(); 2376 } 2377 2378 /** 2379 * @param value The check number, eft reference, car processor reference. 2380 */ 2381 public PaymentReconciliation setReferenceNumber(String value) { 2382 if (Utilities.noString(value)) 2383 this.referenceNumber = null; 2384 else { 2385 if (this.referenceNumber == null) 2386 this.referenceNumber = new StringType(); 2387 this.referenceNumber.setValue(value); 2388 } 2389 return this; 2390 } 2391 2392 /** 2393 * @return {@link #authorization} (An alphanumeric issued by the processor to confirm the successful issuance of payment.). This is the underlying object with id, value and extensions. The accessor "getAuthorization" gives direct access to the value 2394 */ 2395 public StringType getAuthorizationElement() { 2396 if (this.authorization == null) 2397 if (Configuration.errorOnAutoCreate()) 2398 throw new Error("Attempt to auto-create PaymentReconciliation.authorization"); 2399 else if (Configuration.doAutoCreate()) 2400 this.authorization = new StringType(); // bb 2401 return this.authorization; 2402 } 2403 2404 public boolean hasAuthorizationElement() { 2405 return this.authorization != null && !this.authorization.isEmpty(); 2406 } 2407 2408 public boolean hasAuthorization() { 2409 return this.authorization != null && !this.authorization.isEmpty(); 2410 } 2411 2412 /** 2413 * @param value {@link #authorization} (An alphanumeric issued by the processor to confirm the successful issuance of payment.). This is the underlying object with id, value and extensions. The accessor "getAuthorization" gives direct access to the value 2414 */ 2415 public PaymentReconciliation setAuthorizationElement(StringType value) { 2416 this.authorization = value; 2417 return this; 2418 } 2419 2420 /** 2421 * @return An alphanumeric issued by the processor to confirm the successful issuance of payment. 2422 */ 2423 public String getAuthorization() { 2424 return this.authorization == null ? null : this.authorization.getValue(); 2425 } 2426 2427 /** 2428 * @param value An alphanumeric issued by the processor to confirm the successful issuance of payment. 2429 */ 2430 public PaymentReconciliation setAuthorization(String value) { 2431 if (Utilities.noString(value)) 2432 this.authorization = null; 2433 else { 2434 if (this.authorization == null) 2435 this.authorization = new StringType(); 2436 this.authorization.setValue(value); 2437 } 2438 return this; 2439 } 2440 2441 /** 2442 * @return {@link #tenderedAmount} (The amount offered by the issuer, typically applies to cash when the issuer provides an amount in bank note denominations equal to or excess of the amount actually being paid.) 2443 */ 2444 public Money getTenderedAmount() { 2445 if (this.tenderedAmount == null) 2446 if (Configuration.errorOnAutoCreate()) 2447 throw new Error("Attempt to auto-create PaymentReconciliation.tenderedAmount"); 2448 else if (Configuration.doAutoCreate()) 2449 this.tenderedAmount = new Money(); // cc 2450 return this.tenderedAmount; 2451 } 2452 2453 public boolean hasTenderedAmount() { 2454 return this.tenderedAmount != null && !this.tenderedAmount.isEmpty(); 2455 } 2456 2457 /** 2458 * @param value {@link #tenderedAmount} (The amount offered by the issuer, typically applies to cash when the issuer provides an amount in bank note denominations equal to or excess of the amount actually being paid.) 2459 */ 2460 public PaymentReconciliation setTenderedAmount(Money value) { 2461 this.tenderedAmount = value; 2462 return this; 2463 } 2464 2465 /** 2466 * @return {@link #returnedAmount} (The amount returned by the receiver which is excess to the amount payable, often referred to as 'change'.) 2467 */ 2468 public Money getReturnedAmount() { 2469 if (this.returnedAmount == null) 2470 if (Configuration.errorOnAutoCreate()) 2471 throw new Error("Attempt to auto-create PaymentReconciliation.returnedAmount"); 2472 else if (Configuration.doAutoCreate()) 2473 this.returnedAmount = new Money(); // cc 2474 return this.returnedAmount; 2475 } 2476 2477 public boolean hasReturnedAmount() { 2478 return this.returnedAmount != null && !this.returnedAmount.isEmpty(); 2479 } 2480 2481 /** 2482 * @param value {@link #returnedAmount} (The amount returned by the receiver which is excess to the amount payable, often referred to as 'change'.) 2483 */ 2484 public PaymentReconciliation setReturnedAmount(Money value) { 2485 this.returnedAmount = value; 2486 return this; 2487 } 2488 2489 /** 2490 * @return {@link #amount} (Total payment amount as indicated on the financial instrument.) 2491 */ 2492 public Money getAmount() { 2493 if (this.amount == null) 2494 if (Configuration.errorOnAutoCreate()) 2495 throw new Error("Attempt to auto-create PaymentReconciliation.amount"); 2496 else if (Configuration.doAutoCreate()) 2497 this.amount = new Money(); // cc 2498 return this.amount; 2499 } 2500 2501 public boolean hasAmount() { 2502 return this.amount != null && !this.amount.isEmpty(); 2503 } 2504 2505 /** 2506 * @param value {@link #amount} (Total payment amount as indicated on the financial instrument.) 2507 */ 2508 public PaymentReconciliation setAmount(Money value) { 2509 this.amount = value; 2510 return this; 2511 } 2512 2513 /** 2514 * @return {@link #paymentIdentifier} (Issuer's unique identifier for the payment instrument.) 2515 */ 2516 public Identifier getPaymentIdentifier() { 2517 if (this.paymentIdentifier == null) 2518 if (Configuration.errorOnAutoCreate()) 2519 throw new Error("Attempt to auto-create PaymentReconciliation.paymentIdentifier"); 2520 else if (Configuration.doAutoCreate()) 2521 this.paymentIdentifier = new Identifier(); // cc 2522 return this.paymentIdentifier; 2523 } 2524 2525 public boolean hasPaymentIdentifier() { 2526 return this.paymentIdentifier != null && !this.paymentIdentifier.isEmpty(); 2527 } 2528 2529 /** 2530 * @param value {@link #paymentIdentifier} (Issuer's unique identifier for the payment instrument.) 2531 */ 2532 public PaymentReconciliation setPaymentIdentifier(Identifier value) { 2533 this.paymentIdentifier = value; 2534 return this; 2535 } 2536 2537 /** 2538 * @return {@link #allocation} (Distribution of the payment amount for a previously acknowledged payable.) 2539 */ 2540 public List<PaymentReconciliationAllocationComponent> getAllocation() { 2541 if (this.allocation == null) 2542 this.allocation = new ArrayList<PaymentReconciliationAllocationComponent>(); 2543 return this.allocation; 2544 } 2545 2546 /** 2547 * @return Returns a reference to <code>this</code> for easy method chaining 2548 */ 2549 public PaymentReconciliation setAllocation(List<PaymentReconciliationAllocationComponent> theAllocation) { 2550 this.allocation = theAllocation; 2551 return this; 2552 } 2553 2554 public boolean hasAllocation() { 2555 if (this.allocation == null) 2556 return false; 2557 for (PaymentReconciliationAllocationComponent item : this.allocation) 2558 if (!item.isEmpty()) 2559 return true; 2560 return false; 2561 } 2562 2563 public PaymentReconciliationAllocationComponent addAllocation() { //3 2564 PaymentReconciliationAllocationComponent t = new PaymentReconciliationAllocationComponent(); 2565 if (this.allocation == null) 2566 this.allocation = new ArrayList<PaymentReconciliationAllocationComponent>(); 2567 this.allocation.add(t); 2568 return t; 2569 } 2570 2571 public PaymentReconciliation addAllocation(PaymentReconciliationAllocationComponent t) { //3 2572 if (t == null) 2573 return this; 2574 if (this.allocation == null) 2575 this.allocation = new ArrayList<PaymentReconciliationAllocationComponent>(); 2576 this.allocation.add(t); 2577 return this; 2578 } 2579 2580 /** 2581 * @return The first repetition of repeating field {@link #allocation}, creating it if it does not already exist {3} 2582 */ 2583 public PaymentReconciliationAllocationComponent getAllocationFirstRep() { 2584 if (getAllocation().isEmpty()) { 2585 addAllocation(); 2586 } 2587 return getAllocation().get(0); 2588 } 2589 2590 /** 2591 * @return {@link #formCode} (A code for the form to be used for printing the content.) 2592 */ 2593 public CodeableConcept getFormCode() { 2594 if (this.formCode == null) 2595 if (Configuration.errorOnAutoCreate()) 2596 throw new Error("Attempt to auto-create PaymentReconciliation.formCode"); 2597 else if (Configuration.doAutoCreate()) 2598 this.formCode = new CodeableConcept(); // cc 2599 return this.formCode; 2600 } 2601 2602 public boolean hasFormCode() { 2603 return this.formCode != null && !this.formCode.isEmpty(); 2604 } 2605 2606 /** 2607 * @param value {@link #formCode} (A code for the form to be used for printing the content.) 2608 */ 2609 public PaymentReconciliation setFormCode(CodeableConcept value) { 2610 this.formCode = value; 2611 return this; 2612 } 2613 2614 /** 2615 * @return {@link #processNote} (A note that describes or explains the processing in a human readable form.) 2616 */ 2617 public List<NotesComponent> getProcessNote() { 2618 if (this.processNote == null) 2619 this.processNote = new ArrayList<NotesComponent>(); 2620 return this.processNote; 2621 } 2622 2623 /** 2624 * @return Returns a reference to <code>this</code> for easy method chaining 2625 */ 2626 public PaymentReconciliation setProcessNote(List<NotesComponent> theProcessNote) { 2627 this.processNote = theProcessNote; 2628 return this; 2629 } 2630 2631 public boolean hasProcessNote() { 2632 if (this.processNote == null) 2633 return false; 2634 for (NotesComponent item : this.processNote) 2635 if (!item.isEmpty()) 2636 return true; 2637 return false; 2638 } 2639 2640 public NotesComponent addProcessNote() { //3 2641 NotesComponent t = new NotesComponent(); 2642 if (this.processNote == null) 2643 this.processNote = new ArrayList<NotesComponent>(); 2644 this.processNote.add(t); 2645 return t; 2646 } 2647 2648 public PaymentReconciliation addProcessNote(NotesComponent t) { //3 2649 if (t == null) 2650 return this; 2651 if (this.processNote == null) 2652 this.processNote = new ArrayList<NotesComponent>(); 2653 this.processNote.add(t); 2654 return this; 2655 } 2656 2657 /** 2658 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist {3} 2659 */ 2660 public NotesComponent getProcessNoteFirstRep() { 2661 if (getProcessNote().isEmpty()) { 2662 addProcessNote(); 2663 } 2664 return getProcessNote().get(0); 2665 } 2666 2667 protected void listChildren(List<Property> children) { 2668 super.listChildren(children); 2669 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this payment reconciliation.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2670 children.add(new Property("type", "CodeableConcept", "Code to indicate the nature of the payment such as payment, adjustment.", 0, 1, type)); 2671 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2672 children.add(new Property("kind", "CodeableConcept", "The workflow or activity which gave rise to or during which the payment ocurred such as a kiosk, deposit on account, periodic payment etc.", 0, 1, kind)); 2673 children.add(new Property("period", "Period", "The period of time for which payments have been gathered into this bulk payment for settlement.", 0, 1, period)); 2674 children.add(new Property("created", "dateTime", "The date when the resource was created.", 0, 1, created)); 2675 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole|Organization)", "Payment enterer if not the actual payment issuer.", 0, 1, enterer)); 2676 children.add(new Property("issuerType", "CodeableConcept", "The type of the source such as patient or insurance.", 0, 1, issuerType)); 2677 children.add(new Property("paymentIssuer", "Reference(Organization|Patient|RelatedPerson)", "The party who generated the payment.", 0, 1, paymentIssuer)); 2678 children.add(new Property("request", "Reference(Task)", "Original request resource reference.", 0, 1, request)); 2679 children.add(new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestor)); 2680 children.add(new Property("outcome", "code", "The outcome of a request for a reconciliation.", 0, 1, outcome)); 2681 children.add(new Property("disposition", "string", "A human readable description of the status of the request for the reconciliation.", 0, 1, disposition)); 2682 children.add(new Property("date", "date", "The date of payment as indicated on the financial instrument.", 0, 1, date)); 2683 children.add(new Property("location", "Reference(Location)", "The location of the site or device for electronic transfers or physical location for cash payments.", 0, 1, location)); 2684 children.add(new Property("method", "CodeableConcept", "The means of payment such as check, card cash, or electronic funds transfer.", 0, 1, method)); 2685 children.add(new Property("cardBrand", "string", "The card brand such as debit, Visa, Amex etc. used if a card is the method of payment.", 0, 1, cardBrand)); 2686 children.add(new Property("accountNumber", "string", "A portion of the account number, often the last 4 digits, used for verification not charging purposes.", 0, 1, accountNumber)); 2687 children.add(new Property("expirationDate", "date", "The year and month (YYYY-MM) when the instrument, typically card, expires.", 0, 1, expirationDate)); 2688 children.add(new Property("processor", "string", "The name of the card processor, etf processor, bank for checks.", 0, 1, processor)); 2689 children.add(new Property("referenceNumber", "string", "The check number, eft reference, car processor reference.", 0, 1, referenceNumber)); 2690 children.add(new Property("authorization", "string", "An alphanumeric issued by the processor to confirm the successful issuance of payment.", 0, 1, authorization)); 2691 children.add(new Property("tenderedAmount", "Money", "The amount offered by the issuer, typically applies to cash when the issuer provides an amount in bank note denominations equal to or excess of the amount actually being paid.", 0, 1, tenderedAmount)); 2692 children.add(new Property("returnedAmount", "Money", "The amount returned by the receiver which is excess to the amount payable, often referred to as 'change'.", 0, 1, returnedAmount)); 2693 children.add(new Property("amount", "Money", "Total payment amount as indicated on the financial instrument.", 0, 1, amount)); 2694 children.add(new Property("paymentIdentifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, paymentIdentifier)); 2695 children.add(new Property("allocation", "", "Distribution of the payment amount for a previously acknowledged payable.", 0, java.lang.Integer.MAX_VALUE, allocation)); 2696 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode)); 2697 children.add(new Property("processNote", "", "A note that describes or explains the processing in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote)); 2698 } 2699 2700 @Override 2701 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2702 switch (_hash) { 2703 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this payment reconciliation.", 0, java.lang.Integer.MAX_VALUE, identifier); 2704 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code to indicate the nature of the payment such as payment, adjustment.", 0, 1, type); 2705 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2706 case 3292052: /*kind*/ return new Property("kind", "CodeableConcept", "The workflow or activity which gave rise to or during which the payment ocurred such as a kiosk, deposit on account, periodic payment etc.", 0, 1, kind); 2707 case -991726143: /*period*/ return new Property("period", "Period", "The period of time for which payments have been gathered into this bulk payment for settlement.", 0, 1, period); 2708 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the resource was created.", 0, 1, created); 2709 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|PractitionerRole|Organization)", "Payment enterer if not the actual payment issuer.", 0, 1, enterer); 2710 case 1459974547: /*issuerType*/ return new Property("issuerType", "CodeableConcept", "The type of the source such as patient or insurance.", 0, 1, issuerType); 2711 case 1144026207: /*paymentIssuer*/ return new Property("paymentIssuer", "Reference(Organization|Patient|RelatedPerson)", "The party who generated the payment.", 0, 1, paymentIssuer); 2712 case 1095692943: /*request*/ return new Property("request", "Reference(Task)", "Original request resource reference.", 0, 1, request); 2713 case 693934258: /*requestor*/ return new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestor); 2714 case -1106507950: /*outcome*/ return new Property("outcome", "code", "The outcome of a request for a reconciliation.", 0, 1, outcome); 2715 case 583380919: /*disposition*/ return new Property("disposition", "string", "A human readable description of the status of the request for the reconciliation.", 0, 1, disposition); 2716 case 3076014: /*date*/ return new Property("date", "date", "The date of payment as indicated on the financial instrument.", 0, 1, date); 2717 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location of the site or device for electronic transfers or physical location for cash payments.", 0, 1, location); 2718 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "The means of payment such as check, card cash, or electronic funds transfer.", 0, 1, method); 2719 case -271889833: /*cardBrand*/ return new Property("cardBrand", "string", "The card brand such as debit, Visa, Amex etc. used if a card is the method of payment.", 0, 1, cardBrand); 2720 case -1011205162: /*accountNumber*/ return new Property("accountNumber", "string", "A portion of the account number, often the last 4 digits, used for verification not charging purposes.", 0, 1, accountNumber); 2721 case -668811523: /*expirationDate*/ return new Property("expirationDate", "date", "The year and month (YYYY-MM) when the instrument, typically card, expires.", 0, 1, expirationDate); 2722 case -1094759278: /*processor*/ return new Property("processor", "string", "The name of the card processor, etf processor, bank for checks.", 0, 1, processor); 2723 case 744563316: /*referenceNumber*/ return new Property("referenceNumber", "string", "The check number, eft reference, car processor reference.", 0, 1, referenceNumber); 2724 case -1385570183: /*authorization*/ return new Property("authorization", "string", "An alphanumeric issued by the processor to confirm the successful issuance of payment.", 0, 1, authorization); 2725 case 1815344299: /*tenderedAmount*/ return new Property("tenderedAmount", "Money", "The amount offered by the issuer, typically applies to cash when the issuer provides an amount in bank note denominations equal to or excess of the amount actually being paid.", 0, 1, tenderedAmount); 2726 case -797236473: /*returnedAmount*/ return new Property("returnedAmount", "Money", "The amount returned by the receiver which is excess to the amount payable, often referred to as 'change'.", 0, 1, returnedAmount); 2727 case -1413853096: /*amount*/ return new Property("amount", "Money", "Total payment amount as indicated on the financial instrument.", 0, 1, amount); 2728 case 1555852111: /*paymentIdentifier*/ return new Property("paymentIdentifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, paymentIdentifier); 2729 case -1912450848: /*allocation*/ return new Property("allocation", "", "Distribution of the payment amount for a previously acknowledged payable.", 0, java.lang.Integer.MAX_VALUE, allocation); 2730 case 473181393: /*formCode*/ return new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode); 2731 case 202339073: /*processNote*/ return new Property("processNote", "", "A note that describes or explains the processing in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote); 2732 default: return super.getNamedProperty(_hash, _name, _checkValid); 2733 } 2734 2735 } 2736 2737 @Override 2738 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2739 switch (hash) { 2740 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2741 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2742 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 2743 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // CodeableConcept 2744 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 2745 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 2746 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 2747 case 1459974547: /*issuerType*/ return this.issuerType == null ? new Base[0] : new Base[] {this.issuerType}; // CodeableConcept 2748 case 1144026207: /*paymentIssuer*/ return this.paymentIssuer == null ? new Base[0] : new Base[] {this.paymentIssuer}; // Reference 2749 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 2750 case 693934258: /*requestor*/ return this.requestor == null ? new Base[0] : new Base[] {this.requestor}; // Reference 2751 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Enumeration<PaymentOutcome> 2752 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 2753 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 2754 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2755 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 2756 case -271889833: /*cardBrand*/ return this.cardBrand == null ? new Base[0] : new Base[] {this.cardBrand}; // StringType 2757 case -1011205162: /*accountNumber*/ return this.accountNumber == null ? new Base[0] : new Base[] {this.accountNumber}; // StringType 2758 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateType 2759 case -1094759278: /*processor*/ return this.processor == null ? new Base[0] : new Base[] {this.processor}; // StringType 2760 case 744563316: /*referenceNumber*/ return this.referenceNumber == null ? new Base[0] : new Base[] {this.referenceNumber}; // StringType 2761 case -1385570183: /*authorization*/ return this.authorization == null ? new Base[0] : new Base[] {this.authorization}; // StringType 2762 case 1815344299: /*tenderedAmount*/ return this.tenderedAmount == null ? new Base[0] : new Base[] {this.tenderedAmount}; // Money 2763 case -797236473: /*returnedAmount*/ return this.returnedAmount == null ? new Base[0] : new Base[] {this.returnedAmount}; // Money 2764 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 2765 case 1555852111: /*paymentIdentifier*/ return this.paymentIdentifier == null ? new Base[0] : new Base[] {this.paymentIdentifier}; // Identifier 2766 case -1912450848: /*allocation*/ return this.allocation == null ? new Base[0] : this.allocation.toArray(new Base[this.allocation.size()]); // PaymentReconciliationAllocationComponent 2767 case 473181393: /*formCode*/ return this.formCode == null ? new Base[0] : new Base[] {this.formCode}; // CodeableConcept 2768 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NotesComponent 2769 default: return super.getProperty(hash, name, checkValid); 2770 } 2771 2772 } 2773 2774 @Override 2775 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2776 switch (hash) { 2777 case -1618432855: // identifier 2778 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2779 return value; 2780 case 3575610: // type 2781 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2782 return value; 2783 case -892481550: // status 2784 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2785 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2786 return value; 2787 case 3292052: // kind 2788 this.kind = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2789 return value; 2790 case -991726143: // period 2791 this.period = TypeConvertor.castToPeriod(value); // Period 2792 return value; 2793 case 1028554472: // created 2794 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 2795 return value; 2796 case -1591951995: // enterer 2797 this.enterer = TypeConvertor.castToReference(value); // Reference 2798 return value; 2799 case 1459974547: // issuerType 2800 this.issuerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2801 return value; 2802 case 1144026207: // paymentIssuer 2803 this.paymentIssuer = TypeConvertor.castToReference(value); // Reference 2804 return value; 2805 case 1095692943: // request 2806 this.request = TypeConvertor.castToReference(value); // Reference 2807 return value; 2808 case 693934258: // requestor 2809 this.requestor = TypeConvertor.castToReference(value); // Reference 2810 return value; 2811 case -1106507950: // outcome 2812 value = new PaymentOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2813 this.outcome = (Enumeration) value; // Enumeration<PaymentOutcome> 2814 return value; 2815 case 583380919: // disposition 2816 this.disposition = TypeConvertor.castToString(value); // StringType 2817 return value; 2818 case 3076014: // date 2819 this.date = TypeConvertor.castToDate(value); // DateType 2820 return value; 2821 case 1901043637: // location 2822 this.location = TypeConvertor.castToReference(value); // Reference 2823 return value; 2824 case -1077554975: // method 2825 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2826 return value; 2827 case -271889833: // cardBrand 2828 this.cardBrand = TypeConvertor.castToString(value); // StringType 2829 return value; 2830 case -1011205162: // accountNumber 2831 this.accountNumber = TypeConvertor.castToString(value); // StringType 2832 return value; 2833 case -668811523: // expirationDate 2834 this.expirationDate = TypeConvertor.castToDate(value); // DateType 2835 return value; 2836 case -1094759278: // processor 2837 this.processor = TypeConvertor.castToString(value); // StringType 2838 return value; 2839 case 744563316: // referenceNumber 2840 this.referenceNumber = TypeConvertor.castToString(value); // StringType 2841 return value; 2842 case -1385570183: // authorization 2843 this.authorization = TypeConvertor.castToString(value); // StringType 2844 return value; 2845 case 1815344299: // tenderedAmount 2846 this.tenderedAmount = TypeConvertor.castToMoney(value); // Money 2847 return value; 2848 case -797236473: // returnedAmount 2849 this.returnedAmount = TypeConvertor.castToMoney(value); // Money 2850 return value; 2851 case -1413853096: // amount 2852 this.amount = TypeConvertor.castToMoney(value); // Money 2853 return value; 2854 case 1555852111: // paymentIdentifier 2855 this.paymentIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 2856 return value; 2857 case -1912450848: // allocation 2858 this.getAllocation().add((PaymentReconciliationAllocationComponent) value); // PaymentReconciliationAllocationComponent 2859 return value; 2860 case 473181393: // formCode 2861 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2862 return value; 2863 case 202339073: // processNote 2864 this.getProcessNote().add((NotesComponent) value); // NotesComponent 2865 return value; 2866 default: return super.setProperty(hash, name, value); 2867 } 2868 2869 } 2870 2871 @Override 2872 public Base setProperty(String name, Base value) throws FHIRException { 2873 if (name.equals("identifier")) { 2874 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2875 } else if (name.equals("type")) { 2876 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2877 } else if (name.equals("status")) { 2878 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2879 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2880 } else if (name.equals("kind")) { 2881 this.kind = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2882 } else if (name.equals("period")) { 2883 this.period = TypeConvertor.castToPeriod(value); // Period 2884 } else if (name.equals("created")) { 2885 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 2886 } else if (name.equals("enterer")) { 2887 this.enterer = TypeConvertor.castToReference(value); // Reference 2888 } else if (name.equals("issuerType")) { 2889 this.issuerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2890 } else if (name.equals("paymentIssuer")) { 2891 this.paymentIssuer = TypeConvertor.castToReference(value); // Reference 2892 } else if (name.equals("request")) { 2893 this.request = TypeConvertor.castToReference(value); // Reference 2894 } else if (name.equals("requestor")) { 2895 this.requestor = TypeConvertor.castToReference(value); // Reference 2896 } else if (name.equals("outcome")) { 2897 value = new PaymentOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2898 this.outcome = (Enumeration) value; // Enumeration<PaymentOutcome> 2899 } else if (name.equals("disposition")) { 2900 this.disposition = TypeConvertor.castToString(value); // StringType 2901 } else if (name.equals("date")) { 2902 this.date = TypeConvertor.castToDate(value); // DateType 2903 } else if (name.equals("location")) { 2904 this.location = TypeConvertor.castToReference(value); // Reference 2905 } else if (name.equals("method")) { 2906 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2907 } else if (name.equals("cardBrand")) { 2908 this.cardBrand = TypeConvertor.castToString(value); // StringType 2909 } else if (name.equals("accountNumber")) { 2910 this.accountNumber = TypeConvertor.castToString(value); // StringType 2911 } else if (name.equals("expirationDate")) { 2912 this.expirationDate = TypeConvertor.castToDate(value); // DateType 2913 } else if (name.equals("processor")) { 2914 this.processor = TypeConvertor.castToString(value); // StringType 2915 } else if (name.equals("referenceNumber")) { 2916 this.referenceNumber = TypeConvertor.castToString(value); // StringType 2917 } else if (name.equals("authorization")) { 2918 this.authorization = TypeConvertor.castToString(value); // StringType 2919 } else if (name.equals("tenderedAmount")) { 2920 this.tenderedAmount = TypeConvertor.castToMoney(value); // Money 2921 } else if (name.equals("returnedAmount")) { 2922 this.returnedAmount = TypeConvertor.castToMoney(value); // Money 2923 } else if (name.equals("amount")) { 2924 this.amount = TypeConvertor.castToMoney(value); // Money 2925 } else if (name.equals("paymentIdentifier")) { 2926 this.paymentIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 2927 } else if (name.equals("allocation")) { 2928 this.getAllocation().add((PaymentReconciliationAllocationComponent) value); 2929 } else if (name.equals("formCode")) { 2930 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2931 } else if (name.equals("processNote")) { 2932 this.getProcessNote().add((NotesComponent) value); 2933 } else 2934 return super.setProperty(name, value); 2935 return value; 2936 } 2937 2938 @Override 2939 public void removeChild(String name, Base value) throws FHIRException { 2940 if (name.equals("identifier")) { 2941 this.getIdentifier().remove(value); 2942 } else if (name.equals("type")) { 2943 this.type = null; 2944 } else if (name.equals("status")) { 2945 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2946 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2947 } else if (name.equals("kind")) { 2948 this.kind = null; 2949 } else if (name.equals("period")) { 2950 this.period = null; 2951 } else if (name.equals("created")) { 2952 this.created = null; 2953 } else if (name.equals("enterer")) { 2954 this.enterer = null; 2955 } else if (name.equals("issuerType")) { 2956 this.issuerType = null; 2957 } else if (name.equals("paymentIssuer")) { 2958 this.paymentIssuer = null; 2959 } else if (name.equals("request")) { 2960 this.request = null; 2961 } else if (name.equals("requestor")) { 2962 this.requestor = null; 2963 } else if (name.equals("outcome")) { 2964 value = new PaymentOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2965 this.outcome = (Enumeration) value; // Enumeration<PaymentOutcome> 2966 } else if (name.equals("disposition")) { 2967 this.disposition = null; 2968 } else if (name.equals("date")) { 2969 this.date = null; 2970 } else if (name.equals("location")) { 2971 this.location = null; 2972 } else if (name.equals("method")) { 2973 this.method = null; 2974 } else if (name.equals("cardBrand")) { 2975 this.cardBrand = null; 2976 } else if (name.equals("accountNumber")) { 2977 this.accountNumber = null; 2978 } else if (name.equals("expirationDate")) { 2979 this.expirationDate = null; 2980 } else if (name.equals("processor")) { 2981 this.processor = null; 2982 } else if (name.equals("referenceNumber")) { 2983 this.referenceNumber = null; 2984 } else if (name.equals("authorization")) { 2985 this.authorization = null; 2986 } else if (name.equals("tenderedAmount")) { 2987 this.tenderedAmount = null; 2988 } else if (name.equals("returnedAmount")) { 2989 this.returnedAmount = null; 2990 } else if (name.equals("amount")) { 2991 this.amount = null; 2992 } else if (name.equals("paymentIdentifier")) { 2993 this.paymentIdentifier = null; 2994 } else if (name.equals("allocation")) { 2995 this.getAllocation().remove((PaymentReconciliationAllocationComponent) value); 2996 } else if (name.equals("formCode")) { 2997 this.formCode = null; 2998 } else if (name.equals("processNote")) { 2999 this.getProcessNote().remove((NotesComponent) value); 3000 } else 3001 super.removeChild(name, value); 3002 3003 } 3004 3005 @Override 3006 public Base makeProperty(int hash, String name) throws FHIRException { 3007 switch (hash) { 3008 case -1618432855: return addIdentifier(); 3009 case 3575610: return getType(); 3010 case -892481550: return getStatusElement(); 3011 case 3292052: return getKind(); 3012 case -991726143: return getPeriod(); 3013 case 1028554472: return getCreatedElement(); 3014 case -1591951995: return getEnterer(); 3015 case 1459974547: return getIssuerType(); 3016 case 1144026207: return getPaymentIssuer(); 3017 case 1095692943: return getRequest(); 3018 case 693934258: return getRequestor(); 3019 case -1106507950: return getOutcomeElement(); 3020 case 583380919: return getDispositionElement(); 3021 case 3076014: return getDateElement(); 3022 case 1901043637: return getLocation(); 3023 case -1077554975: return getMethod(); 3024 case -271889833: return getCardBrandElement(); 3025 case -1011205162: return getAccountNumberElement(); 3026 case -668811523: return getExpirationDateElement(); 3027 case -1094759278: return getProcessorElement(); 3028 case 744563316: return getReferenceNumberElement(); 3029 case -1385570183: return getAuthorizationElement(); 3030 case 1815344299: return getTenderedAmount(); 3031 case -797236473: return getReturnedAmount(); 3032 case -1413853096: return getAmount(); 3033 case 1555852111: return getPaymentIdentifier(); 3034 case -1912450848: return addAllocation(); 3035 case 473181393: return getFormCode(); 3036 case 202339073: return addProcessNote(); 3037 default: return super.makeProperty(hash, name); 3038 } 3039 3040 } 3041 3042 @Override 3043 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3044 switch (hash) { 3045 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3046 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3047 case -892481550: /*status*/ return new String[] {"code"}; 3048 case 3292052: /*kind*/ return new String[] {"CodeableConcept"}; 3049 case -991726143: /*period*/ return new String[] {"Period"}; 3050 case 1028554472: /*created*/ return new String[] {"dateTime"}; 3051 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 3052 case 1459974547: /*issuerType*/ return new String[] {"CodeableConcept"}; 3053 case 1144026207: /*paymentIssuer*/ return new String[] {"Reference"}; 3054 case 1095692943: /*request*/ return new String[] {"Reference"}; 3055 case 693934258: /*requestor*/ return new String[] {"Reference"}; 3056 case -1106507950: /*outcome*/ return new String[] {"code"}; 3057 case 583380919: /*disposition*/ return new String[] {"string"}; 3058 case 3076014: /*date*/ return new String[] {"date"}; 3059 case 1901043637: /*location*/ return new String[] {"Reference"}; 3060 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 3061 case -271889833: /*cardBrand*/ return new String[] {"string"}; 3062 case -1011205162: /*accountNumber*/ return new String[] {"string"}; 3063 case -668811523: /*expirationDate*/ return new String[] {"date"}; 3064 case -1094759278: /*processor*/ return new String[] {"string"}; 3065 case 744563316: /*referenceNumber*/ return new String[] {"string"}; 3066 case -1385570183: /*authorization*/ return new String[] {"string"}; 3067 case 1815344299: /*tenderedAmount*/ return new String[] {"Money"}; 3068 case -797236473: /*returnedAmount*/ return new String[] {"Money"}; 3069 case -1413853096: /*amount*/ return new String[] {"Money"}; 3070 case 1555852111: /*paymentIdentifier*/ return new String[] {"Identifier"}; 3071 case -1912450848: /*allocation*/ return new String[] {}; 3072 case 473181393: /*formCode*/ return new String[] {"CodeableConcept"}; 3073 case 202339073: /*processNote*/ return new String[] {}; 3074 default: return super.getTypesForProperty(hash, name); 3075 } 3076 3077 } 3078 3079 @Override 3080 public Base addChild(String name) throws FHIRException { 3081 if (name.equals("identifier")) { 3082 return addIdentifier(); 3083 } 3084 else if (name.equals("type")) { 3085 this.type = new CodeableConcept(); 3086 return this.type; 3087 } 3088 else if (name.equals("status")) { 3089 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.status"); 3090 } 3091 else if (name.equals("kind")) { 3092 this.kind = new CodeableConcept(); 3093 return this.kind; 3094 } 3095 else if (name.equals("period")) { 3096 this.period = new Period(); 3097 return this.period; 3098 } 3099 else if (name.equals("created")) { 3100 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.created"); 3101 } 3102 else if (name.equals("enterer")) { 3103 this.enterer = new Reference(); 3104 return this.enterer; 3105 } 3106 else if (name.equals("issuerType")) { 3107 this.issuerType = new CodeableConcept(); 3108 return this.issuerType; 3109 } 3110 else if (name.equals("paymentIssuer")) { 3111 this.paymentIssuer = new Reference(); 3112 return this.paymentIssuer; 3113 } 3114 else if (name.equals("request")) { 3115 this.request = new Reference(); 3116 return this.request; 3117 } 3118 else if (name.equals("requestor")) { 3119 this.requestor = new Reference(); 3120 return this.requestor; 3121 } 3122 else if (name.equals("outcome")) { 3123 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.outcome"); 3124 } 3125 else if (name.equals("disposition")) { 3126 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.disposition"); 3127 } 3128 else if (name.equals("date")) { 3129 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.date"); 3130 } 3131 else if (name.equals("location")) { 3132 this.location = new Reference(); 3133 return this.location; 3134 } 3135 else if (name.equals("method")) { 3136 this.method = new CodeableConcept(); 3137 return this.method; 3138 } 3139 else if (name.equals("cardBrand")) { 3140 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.cardBrand"); 3141 } 3142 else if (name.equals("accountNumber")) { 3143 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.accountNumber"); 3144 } 3145 else if (name.equals("expirationDate")) { 3146 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.expirationDate"); 3147 } 3148 else if (name.equals("processor")) { 3149 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.processor"); 3150 } 3151 else if (name.equals("referenceNumber")) { 3152 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.referenceNumber"); 3153 } 3154 else if (name.equals("authorization")) { 3155 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.authorization"); 3156 } 3157 else if (name.equals("tenderedAmount")) { 3158 this.tenderedAmount = new Money(); 3159 return this.tenderedAmount; 3160 } 3161 else if (name.equals("returnedAmount")) { 3162 this.returnedAmount = new Money(); 3163 return this.returnedAmount; 3164 } 3165 else if (name.equals("amount")) { 3166 this.amount = new Money(); 3167 return this.amount; 3168 } 3169 else if (name.equals("paymentIdentifier")) { 3170 this.paymentIdentifier = new Identifier(); 3171 return this.paymentIdentifier; 3172 } 3173 else if (name.equals("allocation")) { 3174 return addAllocation(); 3175 } 3176 else if (name.equals("formCode")) { 3177 this.formCode = new CodeableConcept(); 3178 return this.formCode; 3179 } 3180 else if (name.equals("processNote")) { 3181 return addProcessNote(); 3182 } 3183 else 3184 return super.addChild(name); 3185 } 3186 3187 public String fhirType() { 3188 return "PaymentReconciliation"; 3189 3190 } 3191 3192 public PaymentReconciliation copy() { 3193 PaymentReconciliation dst = new PaymentReconciliation(); 3194 copyValues(dst); 3195 return dst; 3196 } 3197 3198 public void copyValues(PaymentReconciliation dst) { 3199 super.copyValues(dst); 3200 if (identifier != null) { 3201 dst.identifier = new ArrayList<Identifier>(); 3202 for (Identifier i : identifier) 3203 dst.identifier.add(i.copy()); 3204 }; 3205 dst.type = type == null ? null : type.copy(); 3206 dst.status = status == null ? null : status.copy(); 3207 dst.kind = kind == null ? null : kind.copy(); 3208 dst.period = period == null ? null : period.copy(); 3209 dst.created = created == null ? null : created.copy(); 3210 dst.enterer = enterer == null ? null : enterer.copy(); 3211 dst.issuerType = issuerType == null ? null : issuerType.copy(); 3212 dst.paymentIssuer = paymentIssuer == null ? null : paymentIssuer.copy(); 3213 dst.request = request == null ? null : request.copy(); 3214 dst.requestor = requestor == null ? null : requestor.copy(); 3215 dst.outcome = outcome == null ? null : outcome.copy(); 3216 dst.disposition = disposition == null ? null : disposition.copy(); 3217 dst.date = date == null ? null : date.copy(); 3218 dst.location = location == null ? null : location.copy(); 3219 dst.method = method == null ? null : method.copy(); 3220 dst.cardBrand = cardBrand == null ? null : cardBrand.copy(); 3221 dst.accountNumber = accountNumber == null ? null : accountNumber.copy(); 3222 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 3223 dst.processor = processor == null ? null : processor.copy(); 3224 dst.referenceNumber = referenceNumber == null ? null : referenceNumber.copy(); 3225 dst.authorization = authorization == null ? null : authorization.copy(); 3226 dst.tenderedAmount = tenderedAmount == null ? null : tenderedAmount.copy(); 3227 dst.returnedAmount = returnedAmount == null ? null : returnedAmount.copy(); 3228 dst.amount = amount == null ? null : amount.copy(); 3229 dst.paymentIdentifier = paymentIdentifier == null ? null : paymentIdentifier.copy(); 3230 if (allocation != null) { 3231 dst.allocation = new ArrayList<PaymentReconciliationAllocationComponent>(); 3232 for (PaymentReconciliationAllocationComponent i : allocation) 3233 dst.allocation.add(i.copy()); 3234 }; 3235 dst.formCode = formCode == null ? null : formCode.copy(); 3236 if (processNote != null) { 3237 dst.processNote = new ArrayList<NotesComponent>(); 3238 for (NotesComponent i : processNote) 3239 dst.processNote.add(i.copy()); 3240 }; 3241 } 3242 3243 protected PaymentReconciliation typedCopy() { 3244 return copy(); 3245 } 3246 3247 @Override 3248 public boolean equalsDeep(Base other_) { 3249 if (!super.equalsDeep(other_)) 3250 return false; 3251 if (!(other_ instanceof PaymentReconciliation)) 3252 return false; 3253 PaymentReconciliation o = (PaymentReconciliation) other_; 3254 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 3255 && compareDeep(kind, o.kind, true) && compareDeep(period, o.period, true) && compareDeep(created, o.created, true) 3256 && compareDeep(enterer, o.enterer, true) && compareDeep(issuerType, o.issuerType, true) && compareDeep(paymentIssuer, o.paymentIssuer, true) 3257 && compareDeep(request, o.request, true) && compareDeep(requestor, o.requestor, true) && compareDeep(outcome, o.outcome, true) 3258 && compareDeep(disposition, o.disposition, true) && compareDeep(date, o.date, true) && compareDeep(location, o.location, true) 3259 && compareDeep(method, o.method, true) && compareDeep(cardBrand, o.cardBrand, true) && compareDeep(accountNumber, o.accountNumber, true) 3260 && compareDeep(expirationDate, o.expirationDate, true) && compareDeep(processor, o.processor, true) 3261 && compareDeep(referenceNumber, o.referenceNumber, true) && compareDeep(authorization, o.authorization, true) 3262 && compareDeep(tenderedAmount, o.tenderedAmount, true) && compareDeep(returnedAmount, o.returnedAmount, true) 3263 && compareDeep(amount, o.amount, true) && compareDeep(paymentIdentifier, o.paymentIdentifier, true) 3264 && compareDeep(allocation, o.allocation, true) && compareDeep(formCode, o.formCode, true) && compareDeep(processNote, o.processNote, true) 3265 ; 3266 } 3267 3268 @Override 3269 public boolean equalsShallow(Base other_) { 3270 if (!super.equalsShallow(other_)) 3271 return false; 3272 if (!(other_ instanceof PaymentReconciliation)) 3273 return false; 3274 PaymentReconciliation o = (PaymentReconciliation) other_; 3275 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(outcome, o.outcome, true) 3276 && compareValues(disposition, o.disposition, true) && compareValues(date, o.date, true) && compareValues(cardBrand, o.cardBrand, true) 3277 && compareValues(accountNumber, o.accountNumber, true) && compareValues(expirationDate, o.expirationDate, true) 3278 && compareValues(processor, o.processor, true) && compareValues(referenceNumber, o.referenceNumber, true) 3279 && compareValues(authorization, o.authorization, true); 3280 } 3281 3282 public boolean isEmpty() { 3283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, status 3284 , kind, period, created, enterer, issuerType, paymentIssuer, request, requestor 3285 , outcome, disposition, date, location, method, cardBrand, accountNumber, expirationDate 3286 , processor, referenceNumber, authorization, tenderedAmount, returnedAmount, amount 3287 , paymentIdentifier, allocation, formCode, processNote); 3288 } 3289 3290 @Override 3291 public ResourceType getResourceType() { 3292 return ResourceType.PaymentReconciliation; 3293 } 3294 3295 /** 3296 * Search parameter: <b>allocation-account</b> 3297 * <p> 3298 * Description: <b>The account to which payment or adjustment was applied.</b><br> 3299 * Type: <b>reference</b><br> 3300 * Path: <b>PaymentReconciliation.allocation.account</b><br> 3301 * </p> 3302 */ 3303 @SearchParamDefinition(name="allocation-account", path="PaymentReconciliation.allocation.account", description="The account to which payment or adjustment was applied.", type="reference", target={Account.class } ) 3304 public static final String SP_ALLOCATION_ACCOUNT = "allocation-account"; 3305 /** 3306 * <b>Fluent Client</b> search parameter constant for <b>allocation-account</b> 3307 * <p> 3308 * Description: <b>The account to which payment or adjustment was applied.</b><br> 3309 * Type: <b>reference</b><br> 3310 * Path: <b>PaymentReconciliation.allocation.account</b><br> 3311 * </p> 3312 */ 3313 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ALLOCATION_ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ALLOCATION_ACCOUNT); 3314 3315/** 3316 * Constant for fluent queries to be used to add include statements. Specifies 3317 * the path value of "<b>PaymentReconciliation:allocation-account</b>". 3318 */ 3319 public static final ca.uhn.fhir.model.api.Include INCLUDE_ALLOCATION_ACCOUNT = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:allocation-account").toLocked(); 3320 3321 /** 3322 * Search parameter: <b>allocation-encounter</b> 3323 * <p> 3324 * Description: <b>The encounter to which payment or adjustment was applied.</b><br> 3325 * Type: <b>reference</b><br> 3326 * Path: <b>PaymentReconciliation.allocation.encounter</b><br> 3327 * </p> 3328 */ 3329 @SearchParamDefinition(name="allocation-encounter", path="PaymentReconciliation.allocation.encounter", description="The encounter to which payment or adjustment was applied.", type="reference", target={Encounter.class } ) 3330 public static final String SP_ALLOCATION_ENCOUNTER = "allocation-encounter"; 3331 /** 3332 * <b>Fluent Client</b> search parameter constant for <b>allocation-encounter</b> 3333 * <p> 3334 * Description: <b>The encounter to which payment or adjustment was applied.</b><br> 3335 * Type: <b>reference</b><br> 3336 * Path: <b>PaymentReconciliation.allocation.encounter</b><br> 3337 * </p> 3338 */ 3339 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ALLOCATION_ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ALLOCATION_ENCOUNTER); 3340 3341/** 3342 * Constant for fluent queries to be used to add include statements. Specifies 3343 * the path value of "<b>PaymentReconciliation:allocation-encounter</b>". 3344 */ 3345 public static final ca.uhn.fhir.model.api.Include INCLUDE_ALLOCATION_ENCOUNTER = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:allocation-encounter").toLocked(); 3346 3347 /** 3348 * Search parameter: <b>created</b> 3349 * <p> 3350 * Description: <b>The creation date</b><br> 3351 * Type: <b>date</b><br> 3352 * Path: <b>PaymentReconciliation.created</b><br> 3353 * </p> 3354 */ 3355 @SearchParamDefinition(name="created", path="PaymentReconciliation.created", description="The creation date", type="date" ) 3356 public static final String SP_CREATED = "created"; 3357 /** 3358 * <b>Fluent Client</b> search parameter constant for <b>created</b> 3359 * <p> 3360 * Description: <b>The creation date</b><br> 3361 * Type: <b>date</b><br> 3362 * Path: <b>PaymentReconciliation.created</b><br> 3363 * </p> 3364 */ 3365 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 3366 3367 /** 3368 * Search parameter: <b>disposition</b> 3369 * <p> 3370 * Description: <b>The contents of the disposition message</b><br> 3371 * Type: <b>string</b><br> 3372 * Path: <b>PaymentReconciliation.disposition</b><br> 3373 * </p> 3374 */ 3375 @SearchParamDefinition(name="disposition", path="PaymentReconciliation.disposition", description="The contents of the disposition message", type="string" ) 3376 public static final String SP_DISPOSITION = "disposition"; 3377 /** 3378 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 3379 * <p> 3380 * Description: <b>The contents of the disposition message</b><br> 3381 * Type: <b>string</b><br> 3382 * Path: <b>PaymentReconciliation.disposition</b><br> 3383 * </p> 3384 */ 3385 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 3386 3387 /** 3388 * Search parameter: <b>identifier</b> 3389 * <p> 3390 * Description: <b>The business identifier of the ExplanationOfBenefit</b><br> 3391 * Type: <b>token</b><br> 3392 * Path: <b>PaymentReconciliation.identifier</b><br> 3393 * </p> 3394 */ 3395 @SearchParamDefinition(name="identifier", path="PaymentReconciliation.identifier", description="The business identifier of the ExplanationOfBenefit", type="token" ) 3396 public static final String SP_IDENTIFIER = "identifier"; 3397 /** 3398 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3399 * <p> 3400 * Description: <b>The business identifier of the ExplanationOfBenefit</b><br> 3401 * Type: <b>token</b><br> 3402 * Path: <b>PaymentReconciliation.identifier</b><br> 3403 * </p> 3404 */ 3405 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3406 3407 /** 3408 * Search parameter: <b>outcome</b> 3409 * <p> 3410 * Description: <b>The processing outcome</b><br> 3411 * Type: <b>token</b><br> 3412 * Path: <b>PaymentReconciliation.outcome</b><br> 3413 * </p> 3414 */ 3415 @SearchParamDefinition(name="outcome", path="PaymentReconciliation.outcome", description="The processing outcome", type="token" ) 3416 public static final String SP_OUTCOME = "outcome"; 3417 /** 3418 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 3419 * <p> 3420 * Description: <b>The processing outcome</b><br> 3421 * Type: <b>token</b><br> 3422 * Path: <b>PaymentReconciliation.outcome</b><br> 3423 * </p> 3424 */ 3425 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 3426 3427 /** 3428 * Search parameter: <b>payment-issuer</b> 3429 * <p> 3430 * Description: <b>The organization which generated this resource</b><br> 3431 * Type: <b>reference</b><br> 3432 * Path: <b>PaymentReconciliation.paymentIssuer</b><br> 3433 * </p> 3434 */ 3435 @SearchParamDefinition(name="payment-issuer", path="PaymentReconciliation.paymentIssuer", description="The organization which generated this resource", type="reference", target={Organization.class, Patient.class, RelatedPerson.class } ) 3436 public static final String SP_PAYMENT_ISSUER = "payment-issuer"; 3437 /** 3438 * <b>Fluent Client</b> search parameter constant for <b>payment-issuer</b> 3439 * <p> 3440 * Description: <b>The organization which generated this resource</b><br> 3441 * Type: <b>reference</b><br> 3442 * Path: <b>PaymentReconciliation.paymentIssuer</b><br> 3443 * </p> 3444 */ 3445 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYMENT_ISSUER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYMENT_ISSUER); 3446 3447/** 3448 * Constant for fluent queries to be used to add include statements. Specifies 3449 * the path value of "<b>PaymentReconciliation:payment-issuer</b>". 3450 */ 3451 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYMENT_ISSUER = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:payment-issuer").toLocked(); 3452 3453 /** 3454 * Search parameter: <b>request</b> 3455 * <p> 3456 * Description: <b>The reference to the claim</b><br> 3457 * Type: <b>reference</b><br> 3458 * Path: <b>PaymentReconciliation.request</b><br> 3459 * </p> 3460 */ 3461 @SearchParamDefinition(name="request", path="PaymentReconciliation.request", description="The reference to the claim", type="reference", target={Task.class } ) 3462 public static final String SP_REQUEST = "request"; 3463 /** 3464 * <b>Fluent Client</b> search parameter constant for <b>request</b> 3465 * <p> 3466 * Description: <b>The reference to the claim</b><br> 3467 * Type: <b>reference</b><br> 3468 * Path: <b>PaymentReconciliation.request</b><br> 3469 * </p> 3470 */ 3471 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 3472 3473/** 3474 * Constant for fluent queries to be used to add include statements. Specifies 3475 * the path value of "<b>PaymentReconciliation:request</b>". 3476 */ 3477 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:request").toLocked(); 3478 3479 /** 3480 * Search parameter: <b>requestor</b> 3481 * <p> 3482 * Description: <b>The reference to the provider who submitted the claim</b><br> 3483 * Type: <b>reference</b><br> 3484 * Path: <b>PaymentReconciliation.requestor</b><br> 3485 * </p> 3486 */ 3487 @SearchParamDefinition(name="requestor", path="PaymentReconciliation.requestor", description="The reference to the provider who submitted the claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 3488 public static final String SP_REQUESTOR = "requestor"; 3489 /** 3490 * <b>Fluent Client</b> search parameter constant for <b>requestor</b> 3491 * <p> 3492 * Description: <b>The reference to the provider who submitted the claim</b><br> 3493 * Type: <b>reference</b><br> 3494 * Path: <b>PaymentReconciliation.requestor</b><br> 3495 * </p> 3496 */ 3497 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTOR); 3498 3499/** 3500 * Constant for fluent queries to be used to add include statements. Specifies 3501 * the path value of "<b>PaymentReconciliation:requestor</b>". 3502 */ 3503 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTOR = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:requestor").toLocked(); 3504 3505 /** 3506 * Search parameter: <b>status</b> 3507 * <p> 3508 * Description: <b>The status of the payment reconciliation</b><br> 3509 * Type: <b>token</b><br> 3510 * Path: <b>PaymentReconciliation.status</b><br> 3511 * </p> 3512 */ 3513 @SearchParamDefinition(name="status", path="PaymentReconciliation.status", description="The status of the payment reconciliation", type="token" ) 3514 public static final String SP_STATUS = "status"; 3515 /** 3516 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3517 * <p> 3518 * Description: <b>The status of the payment reconciliation</b><br> 3519 * Type: <b>token</b><br> 3520 * Path: <b>PaymentReconciliation.status</b><br> 3521 * </p> 3522 */ 3523 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3524 3525 3526} 3527