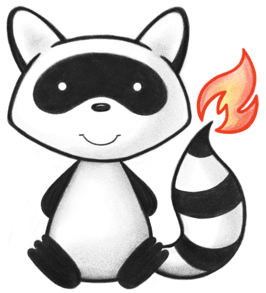
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 048/** 049 * Period Type: A time period defined by a start and end date and optionally time. 050 */ 051@DatatypeDef(name="Period") 052public class Period extends DataType implements ICompositeType { 053 054 /** 055 * The start of the period. The boundary is inclusive. 056 */ 057 @Child(name = "start", type = {DateTimeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 058 @Description(shortDefinition="Starting time with inclusive boundary", formalDefinition="The start of the period. The boundary is inclusive." ) 059 protected DateTimeType start; 060 061 /** 062 * The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time. 063 */ 064 @Child(name = "end", type = {DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 065 @Description(shortDefinition="End time with inclusive boundary, if not ongoing", formalDefinition="The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time." ) 066 protected DateTimeType end; 067 068 private static final long serialVersionUID = 649791751L; 069 070 /** 071 * Constructor 072 */ 073 public Period() { 074 super(); 075 } 076 077 /** 078 * @return {@link #start} (The start of the period. The boundary is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 079 */ 080 public DateTimeType getStartElement() { 081 if (this.start == null) 082 if (Configuration.errorOnAutoCreate()) 083 throw new Error("Attempt to auto-create Period.start"); 084 else if (Configuration.doAutoCreate()) 085 this.start = new DateTimeType(); // bb 086 return this.start; 087 } 088 089 public boolean hasStartElement() { 090 return this.start != null && !this.start.isEmpty(); 091 } 092 093 public boolean hasStart() { 094 return this.start != null && !this.start.isEmpty(); 095 } 096 097 /** 098 * @param value {@link #start} (The start of the period. The boundary is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 099 */ 100 public Period setStartElement(DateTimeType value) { 101 this.start = value; 102 return this; 103 } 104 105 /** 106 * @return The start of the period. The boundary is inclusive. 107 */ 108 public Date getStart() { 109 return this.start == null ? null : this.start.getValue(); 110 } 111 112 /** 113 * @param value The start of the period. The boundary is inclusive. 114 */ 115 public Period setStart(Date value) { 116 if (value == null) 117 this.start = null; 118 else { 119 if (this.start == null) 120 this.start = new DateTimeType(); 121 this.start.setValue(value); 122 } 123 return this; 124 } 125 126 /** 127 * @return {@link #end} (The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 128 */ 129 public DateTimeType getEndElement() { 130 if (this.end == null) 131 if (Configuration.errorOnAutoCreate()) 132 throw new Error("Attempt to auto-create Period.end"); 133 else if (Configuration.doAutoCreate()) 134 this.end = new DateTimeType(); // bb 135 return this.end; 136 } 137 138 public boolean hasEndElement() { 139 return this.end != null && !this.end.isEmpty(); 140 } 141 142 public boolean hasEnd() { 143 return this.end != null && !this.end.isEmpty(); 144 } 145 146 /** 147 * @param value {@link #end} (The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 148 */ 149 public Period setEndElement(DateTimeType value) { 150 this.end = value; 151 return this; 152 } 153 154 /** 155 * @return The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time. 156 */ 157 public Date getEnd() { 158 return this.end == null ? null : this.end.getValue(); 159 } 160 161 /** 162 * @param value The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time. 163 */ 164 public Period setEnd(Date value) { 165 if (value == null) 166 this.end = null; 167 else { 168 if (this.end == null) 169 this.end = new DateTimeType(); 170 this.end.setValue(value); 171 } 172 return this; 173 } 174 175 protected void listChildren(List<Property> children) { 176 super.listChildren(children); 177 children.add(new Property("start", "dateTime", "The start of the period. The boundary is inclusive.", 0, 1, start)); 178 children.add(new Property("end", "dateTime", "The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.", 0, 1, end)); 179 } 180 181 @Override 182 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 183 switch (_hash) { 184 case 109757538: /*start*/ return new Property("start", "dateTime", "The start of the period. The boundary is inclusive.", 0, 1, start); 185 case 100571: /*end*/ return new Property("end", "dateTime", "The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.", 0, 1, end); 186 default: return super.getNamedProperty(_hash, _name, _checkValid); 187 } 188 189 } 190 191 @Override 192 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 193 switch (hash) { 194 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // DateTimeType 195 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // DateTimeType 196 default: return super.getProperty(hash, name, checkValid); 197 } 198 199 } 200 201 @Override 202 public Base setProperty(int hash, String name, Base value) throws FHIRException { 203 switch (hash) { 204 case 109757538: // start 205 this.start = TypeConvertor.castToDateTime(value); // DateTimeType 206 return value; 207 case 100571: // end 208 this.end = TypeConvertor.castToDateTime(value); // DateTimeType 209 return value; 210 default: return super.setProperty(hash, name, value); 211 } 212 213 } 214 215 @Override 216 public Base setProperty(String name, Base value) throws FHIRException { 217 if (name.equals("start")) { 218 this.start = TypeConvertor.castToDateTime(value); // DateTimeType 219 } else if (name.equals("end")) { 220 this.end = TypeConvertor.castToDateTime(value); // DateTimeType 221 } else 222 return super.setProperty(name, value); 223 return value; 224 } 225 226 @Override 227 public void removeChild(String name, Base value) throws FHIRException { 228 if (name.equals("start")) { 229 this.start = null; 230 } else if (name.equals("end")) { 231 this.end = null; 232 } else 233 super.removeChild(name, value); 234 235 } 236 237 @Override 238 public Base makeProperty(int hash, String name) throws FHIRException { 239 switch (hash) { 240 case 109757538: return getStartElement(); 241 case 100571: return getEndElement(); 242 default: return super.makeProperty(hash, name); 243 } 244 245 } 246 247 @Override 248 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 249 switch (hash) { 250 case 109757538: /*start*/ return new String[] {"dateTime"}; 251 case 100571: /*end*/ return new String[] {"dateTime"}; 252 default: return super.getTypesForProperty(hash, name); 253 } 254 255 } 256 257 @Override 258 public Base addChild(String name) throws FHIRException { 259 if (name.equals("start")) { 260 throw new FHIRException("Cannot call addChild on a singleton property Period.start"); 261 } 262 else if (name.equals("end")) { 263 throw new FHIRException("Cannot call addChild on a singleton property Period.end"); 264 } 265 else 266 return super.addChild(name); 267 } 268 269 public String fhirType() { 270 return "Period"; 271 272 } 273 274 public Period copy() { 275 Period dst = new Period(); 276 copyValues(dst); 277 return dst; 278 } 279 280 public void copyValues(Period dst) { 281 super.copyValues(dst); 282 dst.start = start == null ? null : start.copy(); 283 dst.end = end == null ? null : end.copy(); 284 } 285 286 protected Period typedCopy() { 287 return copy(); 288 } 289 290 @Override 291 public boolean equalsDeep(Base other_) { 292 if (!super.equalsDeep(other_)) 293 return false; 294 if (!(other_ instanceof Period)) 295 return false; 296 Period o = (Period) other_; 297 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 298 } 299 300 @Override 301 public boolean equalsShallow(Base other_) { 302 if (!super.equalsShallow(other_)) 303 return false; 304 if (!(other_ instanceof Period)) 305 return false; 306 Period o = (Period) other_; 307 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 308 } 309 310 public boolean isEmpty() { 311 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end); 312 } 313 314// Manual code (from Configuration.txt): 315/** 316 * Sets the value for <b>start</b> () 317 * 318 * <p> 319 * <b>Definition:</b> 320 * The start of the period. The boundary is inclusive. 321 * </p> 322 */ 323 public Period setStart( Date theDate, TemporalPrecisionEnum thePrecision) { 324 start = new DateTimeType(theDate, thePrecision); 325 return this; 326 } 327 328 /** 329 * Sets the value for <b>end</b> () 330 * 331 * <p> 332 * <b>Definition:</b> 333 * The end of the period. The boundary is inclusive. 334 * </p> 335 */ 336 public Period setEnd( Date theDate, TemporalPrecisionEnum thePrecision) { 337 end = new DateTimeType(theDate, thePrecision); 338 return this; 339 } 340// end addition 341 342} 343