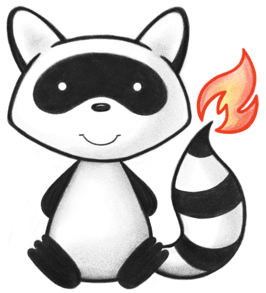
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Permission resource holds access rules for a given data and context. 052 */ 053@ResourceDef(name="Permission", profile="http://hl7.org/fhir/StructureDefinition/Permission") 054public class Permission extends DomainResource { 055 056 public enum PermissionRuleCombining { 057 /** 058 * The deny overrides combining algorithm is intended for those cases where a deny decision should have priority over a permit decision. 059 */ 060 DENYOVERRIDES, 061 /** 062 * The permit overrides combining algorithm is intended for those cases where a permit decision should have priority over a deny decision. 063 */ 064 PERMITOVERRIDES, 065 /** 066 * The behavior of this algorithm is identical to that of the ?Deny-overrides? rule-combining algorithm with one exception. The order in which the collection of rules is evaluated SHALL match the order as listed in the permission. 067 */ 068 ORDEREDDENYOVERRIDES, 069 /** 070 * The behavior of this algorithm is identical to that of the ?Permit-overrides? rule-combining algorithm with one exception. The order in which the collection of rules is evaluated SHALL match the order as listed in the permission. 071 */ 072 ORDEREDPERMITOVERRIDES, 073 /** 074 * The ?Deny-unless-permit? combining algorithm is intended for those cases where a permit decision should have priority over a deny decision, and an ?Indeterminate? or ?NotApplicable? must never be the result. It is particularly useful at the top level in a policy structure to ensure that a PDP will always return a definite ?Permit? or ?Deny? result. 075 */ 076 DENYUNLESSPERMIT, 077 /** 078 * The ?Permit-unless-deny? combining algorithm is intended for those cases where a deny decision should have priority over a permit decision, and an ?Indeterminate? or ?NotApplicable? must never be the result. It is particularly useful at the top level in a policy structure to ensure that a PDP will always return a definite ?Permit? or ?Deny? result. This algorithm has the following behavior. 079 */ 080 PERMITUNLESSDENY, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static PermissionRuleCombining fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("deny-overrides".equals(codeString)) 089 return DENYOVERRIDES; 090 if ("permit-overrides".equals(codeString)) 091 return PERMITOVERRIDES; 092 if ("ordered-deny-overrides".equals(codeString)) 093 return ORDEREDDENYOVERRIDES; 094 if ("ordered-permit-overrides".equals(codeString)) 095 return ORDEREDPERMITOVERRIDES; 096 if ("deny-unless-permit".equals(codeString)) 097 return DENYUNLESSPERMIT; 098 if ("permit-unless-deny".equals(codeString)) 099 return PERMITUNLESSDENY; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown PermissionRuleCombining code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case DENYOVERRIDES: return "deny-overrides"; 108 case PERMITOVERRIDES: return "permit-overrides"; 109 case ORDEREDDENYOVERRIDES: return "ordered-deny-overrides"; 110 case ORDEREDPERMITOVERRIDES: return "ordered-permit-overrides"; 111 case DENYUNLESSPERMIT: return "deny-unless-permit"; 112 case PERMITUNLESSDENY: return "permit-unless-deny"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getSystem() { 118 switch (this) { 119 case DENYOVERRIDES: return "http://hl7.org/fhir/permission-rule-combining"; 120 case PERMITOVERRIDES: return "http://hl7.org/fhir/permission-rule-combining"; 121 case ORDEREDDENYOVERRIDES: return "http://hl7.org/fhir/permission-rule-combining"; 122 case ORDEREDPERMITOVERRIDES: return "http://hl7.org/fhir/permission-rule-combining"; 123 case DENYUNLESSPERMIT: return "http://hl7.org/fhir/permission-rule-combining"; 124 case PERMITUNLESSDENY: return "http://hl7.org/fhir/permission-rule-combining"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDefinition() { 130 switch (this) { 131 case DENYOVERRIDES: return "The deny overrides combining algorithm is intended for those cases where a deny decision should have priority over a permit decision."; 132 case PERMITOVERRIDES: return "The permit overrides combining algorithm is intended for those cases where a permit decision should have priority over a deny decision."; 133 case ORDEREDDENYOVERRIDES: return "The behavior of this algorithm is identical to that of the ?Deny-overrides? rule-combining algorithm with one exception. The order in which the collection of rules is evaluated SHALL match the order as listed in the permission."; 134 case ORDEREDPERMITOVERRIDES: return "The behavior of this algorithm is identical to that of the ?Permit-overrides? rule-combining algorithm with one exception. The order in which the collection of rules is evaluated SHALL match the order as listed in the permission."; 135 case DENYUNLESSPERMIT: return "The ?Deny-unless-permit? combining algorithm is intended for those cases where a permit decision should have priority over a deny decision, and an ?Indeterminate? or ?NotApplicable? must never be the result. It is particularly useful at the top level in a policy structure to ensure that a PDP will always return a definite ?Permit? or ?Deny? result."; 136 case PERMITUNLESSDENY: return "The ?Permit-unless-deny? combining algorithm is intended for those cases where a deny decision should have priority over a permit decision, and an ?Indeterminate? or ?NotApplicable? must never be the result. It is particularly useful at the top level in a policy structure to ensure that a PDP will always return a definite ?Permit? or ?Deny? result. This algorithm has the following behavior."; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getDisplay() { 142 switch (this) { 143 case DENYOVERRIDES: return "Deny-overrides"; 144 case PERMITOVERRIDES: return "Permit-overrides"; 145 case ORDEREDDENYOVERRIDES: return "Ordered-deny-overrides"; 146 case ORDEREDPERMITOVERRIDES: return "Ordered-permit-overrides"; 147 case DENYUNLESSPERMIT: return "Deny-unless-permit"; 148 case PERMITUNLESSDENY: return "Permit-unless-deny"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 } 154 155 public static class PermissionRuleCombiningEnumFactory implements EnumFactory<PermissionRuleCombining> { 156 public PermissionRuleCombining fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("deny-overrides".equals(codeString)) 161 return PermissionRuleCombining.DENYOVERRIDES; 162 if ("permit-overrides".equals(codeString)) 163 return PermissionRuleCombining.PERMITOVERRIDES; 164 if ("ordered-deny-overrides".equals(codeString)) 165 return PermissionRuleCombining.ORDEREDDENYOVERRIDES; 166 if ("ordered-permit-overrides".equals(codeString)) 167 return PermissionRuleCombining.ORDEREDPERMITOVERRIDES; 168 if ("deny-unless-permit".equals(codeString)) 169 return PermissionRuleCombining.DENYUNLESSPERMIT; 170 if ("permit-unless-deny".equals(codeString)) 171 return PermissionRuleCombining.PERMITUNLESSDENY; 172 throw new IllegalArgumentException("Unknown PermissionRuleCombining code '"+codeString+"'"); 173 } 174 public Enumeration<PermissionRuleCombining> fromType(PrimitiveType<?> code) throws FHIRException { 175 if (code == null) 176 return null; 177 if (code.isEmpty()) 178 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.NULL, code); 179 String codeString = ((PrimitiveType) code).asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.NULL, code); 182 if ("deny-overrides".equals(codeString)) 183 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.DENYOVERRIDES, code); 184 if ("permit-overrides".equals(codeString)) 185 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.PERMITOVERRIDES, code); 186 if ("ordered-deny-overrides".equals(codeString)) 187 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.ORDEREDDENYOVERRIDES, code); 188 if ("ordered-permit-overrides".equals(codeString)) 189 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.ORDEREDPERMITOVERRIDES, code); 190 if ("deny-unless-permit".equals(codeString)) 191 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.DENYUNLESSPERMIT, code); 192 if ("permit-unless-deny".equals(codeString)) 193 return new Enumeration<PermissionRuleCombining>(this, PermissionRuleCombining.PERMITUNLESSDENY, code); 194 throw new FHIRException("Unknown PermissionRuleCombining code '"+codeString+"'"); 195 } 196 public String toCode(PermissionRuleCombining code) { 197 if (code == PermissionRuleCombining.NULL) 198 return null; 199 if (code == PermissionRuleCombining.DENYOVERRIDES) 200 return "deny-overrides"; 201 if (code == PermissionRuleCombining.PERMITOVERRIDES) 202 return "permit-overrides"; 203 if (code == PermissionRuleCombining.ORDEREDDENYOVERRIDES) 204 return "ordered-deny-overrides"; 205 if (code == PermissionRuleCombining.ORDEREDPERMITOVERRIDES) 206 return "ordered-permit-overrides"; 207 if (code == PermissionRuleCombining.DENYUNLESSPERMIT) 208 return "deny-unless-permit"; 209 if (code == PermissionRuleCombining.PERMITUNLESSDENY) 210 return "permit-unless-deny"; 211 return "?"; 212 } 213 public String toSystem(PermissionRuleCombining code) { 214 return code.getSystem(); 215 } 216 } 217 218 public enum PermissionStatus { 219 /** 220 * Permission is given. 221 */ 222 ACTIVE, 223 /** 224 * Permission was entered in error and is not active. 225 */ 226 ENTEREDINERROR, 227 /** 228 * Permission is being defined. 229 */ 230 DRAFT, 231 /** 232 * Permission not granted. 233 */ 234 REJECTED, 235 /** 236 * added to help the parsers with the generic types 237 */ 238 NULL; 239 public static PermissionStatus fromCode(String codeString) throws FHIRException { 240 if (codeString == null || "".equals(codeString)) 241 return null; 242 if ("active".equals(codeString)) 243 return ACTIVE; 244 if ("entered-in-error".equals(codeString)) 245 return ENTEREDINERROR; 246 if ("draft".equals(codeString)) 247 return DRAFT; 248 if ("rejected".equals(codeString)) 249 return REJECTED; 250 if (Configuration.isAcceptInvalidEnums()) 251 return null; 252 else 253 throw new FHIRException("Unknown PermissionStatus code '"+codeString+"'"); 254 } 255 public String toCode() { 256 switch (this) { 257 case ACTIVE: return "active"; 258 case ENTEREDINERROR: return "entered-in-error"; 259 case DRAFT: return "draft"; 260 case REJECTED: return "rejected"; 261 case NULL: return null; 262 default: return "?"; 263 } 264 } 265 public String getSystem() { 266 switch (this) { 267 case ACTIVE: return "http://hl7.org/fhir/permission-status"; 268 case ENTEREDINERROR: return "http://hl7.org/fhir/permission-status"; 269 case DRAFT: return "http://hl7.org/fhir/permission-status"; 270 case REJECTED: return "http://hl7.org/fhir/permission-status"; 271 case NULL: return null; 272 default: return "?"; 273 } 274 } 275 public String getDefinition() { 276 switch (this) { 277 case ACTIVE: return "Permission is given."; 278 case ENTEREDINERROR: return "Permission was entered in error and is not active."; 279 case DRAFT: return "Permission is being defined."; 280 case REJECTED: return "Permission not granted."; 281 case NULL: return null; 282 default: return "?"; 283 } 284 } 285 public String getDisplay() { 286 switch (this) { 287 case ACTIVE: return "Active"; 288 case ENTEREDINERROR: return "Entered in Error"; 289 case DRAFT: return "Draft"; 290 case REJECTED: return "Rejected"; 291 case NULL: return null; 292 default: return "?"; 293 } 294 } 295 } 296 297 public static class PermissionStatusEnumFactory implements EnumFactory<PermissionStatus> { 298 public PermissionStatus fromCode(String codeString) throws IllegalArgumentException { 299 if (codeString == null || "".equals(codeString)) 300 if (codeString == null || "".equals(codeString)) 301 return null; 302 if ("active".equals(codeString)) 303 return PermissionStatus.ACTIVE; 304 if ("entered-in-error".equals(codeString)) 305 return PermissionStatus.ENTEREDINERROR; 306 if ("draft".equals(codeString)) 307 return PermissionStatus.DRAFT; 308 if ("rejected".equals(codeString)) 309 return PermissionStatus.REJECTED; 310 throw new IllegalArgumentException("Unknown PermissionStatus code '"+codeString+"'"); 311 } 312 public Enumeration<PermissionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 313 if (code == null) 314 return null; 315 if (code.isEmpty()) 316 return new Enumeration<PermissionStatus>(this, PermissionStatus.NULL, code); 317 String codeString = ((PrimitiveType) code).asStringValue(); 318 if (codeString == null || "".equals(codeString)) 319 return new Enumeration<PermissionStatus>(this, PermissionStatus.NULL, code); 320 if ("active".equals(codeString)) 321 return new Enumeration<PermissionStatus>(this, PermissionStatus.ACTIVE, code); 322 if ("entered-in-error".equals(codeString)) 323 return new Enumeration<PermissionStatus>(this, PermissionStatus.ENTEREDINERROR, code); 324 if ("draft".equals(codeString)) 325 return new Enumeration<PermissionStatus>(this, PermissionStatus.DRAFT, code); 326 if ("rejected".equals(codeString)) 327 return new Enumeration<PermissionStatus>(this, PermissionStatus.REJECTED, code); 328 throw new FHIRException("Unknown PermissionStatus code '"+codeString+"'"); 329 } 330 public String toCode(PermissionStatus code) { 331 if (code == PermissionStatus.NULL) 332 return null; 333 if (code == PermissionStatus.ACTIVE) 334 return "active"; 335 if (code == PermissionStatus.ENTEREDINERROR) 336 return "entered-in-error"; 337 if (code == PermissionStatus.DRAFT) 338 return "draft"; 339 if (code == PermissionStatus.REJECTED) 340 return "rejected"; 341 return "?"; 342 } 343 public String toSystem(PermissionStatus code) { 344 return code.getSystem(); 345 } 346 } 347 348 @Block() 349 public static class PermissionJustificationComponent extends BackboneElement implements IBaseBackboneElement { 350 /** 351 * This would be a codeableconcept, or a coding, which can be constrained to , for example, the 6 grounds for processing in GDPR. 352 */ 353 @Child(name = "basis", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 354 @Description(shortDefinition="The regulatory grounds upon which this Permission builds", formalDefinition="This would be a codeableconcept, or a coding, which can be constrained to , for example, the 6 grounds for processing in GDPR." ) 355 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-policy") 356 protected List<CodeableConcept> basis; 357 358 /** 359 * Justifing rational. 360 */ 361 @Child(name = "evidence", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 362 @Description(shortDefinition="Justifing rational", formalDefinition="Justifing rational." ) 363 protected List<Reference> evidence; 364 365 private static final long serialVersionUID = -2023272721L; 366 367 /** 368 * Constructor 369 */ 370 public PermissionJustificationComponent() { 371 super(); 372 } 373 374 /** 375 * @return {@link #basis} (This would be a codeableconcept, or a coding, which can be constrained to , for example, the 6 grounds for processing in GDPR.) 376 */ 377 public List<CodeableConcept> getBasis() { 378 if (this.basis == null) 379 this.basis = new ArrayList<CodeableConcept>(); 380 return this.basis; 381 } 382 383 /** 384 * @return Returns a reference to <code>this</code> for easy method chaining 385 */ 386 public PermissionJustificationComponent setBasis(List<CodeableConcept> theBasis) { 387 this.basis = theBasis; 388 return this; 389 } 390 391 public boolean hasBasis() { 392 if (this.basis == null) 393 return false; 394 for (CodeableConcept item : this.basis) 395 if (!item.isEmpty()) 396 return true; 397 return false; 398 } 399 400 public CodeableConcept addBasis() { //3 401 CodeableConcept t = new CodeableConcept(); 402 if (this.basis == null) 403 this.basis = new ArrayList<CodeableConcept>(); 404 this.basis.add(t); 405 return t; 406 } 407 408 public PermissionJustificationComponent addBasis(CodeableConcept t) { //3 409 if (t == null) 410 return this; 411 if (this.basis == null) 412 this.basis = new ArrayList<CodeableConcept>(); 413 this.basis.add(t); 414 return this; 415 } 416 417 /** 418 * @return The first repetition of repeating field {@link #basis}, creating it if it does not already exist {3} 419 */ 420 public CodeableConcept getBasisFirstRep() { 421 if (getBasis().isEmpty()) { 422 addBasis(); 423 } 424 return getBasis().get(0); 425 } 426 427 /** 428 * @return {@link #evidence} (Justifing rational.) 429 */ 430 public List<Reference> getEvidence() { 431 if (this.evidence == null) 432 this.evidence = new ArrayList<Reference>(); 433 return this.evidence; 434 } 435 436 /** 437 * @return Returns a reference to <code>this</code> for easy method chaining 438 */ 439 public PermissionJustificationComponent setEvidence(List<Reference> theEvidence) { 440 this.evidence = theEvidence; 441 return this; 442 } 443 444 public boolean hasEvidence() { 445 if (this.evidence == null) 446 return false; 447 for (Reference item : this.evidence) 448 if (!item.isEmpty()) 449 return true; 450 return false; 451 } 452 453 public Reference addEvidence() { //3 454 Reference t = new Reference(); 455 if (this.evidence == null) 456 this.evidence = new ArrayList<Reference>(); 457 this.evidence.add(t); 458 return t; 459 } 460 461 public PermissionJustificationComponent addEvidence(Reference t) { //3 462 if (t == null) 463 return this; 464 if (this.evidence == null) 465 this.evidence = new ArrayList<Reference>(); 466 this.evidence.add(t); 467 return this; 468 } 469 470 /** 471 * @return The first repetition of repeating field {@link #evidence}, creating it if it does not already exist {3} 472 */ 473 public Reference getEvidenceFirstRep() { 474 if (getEvidence().isEmpty()) { 475 addEvidence(); 476 } 477 return getEvidence().get(0); 478 } 479 480 protected void listChildren(List<Property> children) { 481 super.listChildren(children); 482 children.add(new Property("basis", "CodeableConcept", "This would be a codeableconcept, or a coding, which can be constrained to , for example, the 6 grounds for processing in GDPR.", 0, java.lang.Integer.MAX_VALUE, basis)); 483 children.add(new Property("evidence", "Reference(Any)", "Justifing rational.", 0, java.lang.Integer.MAX_VALUE, evidence)); 484 } 485 486 @Override 487 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 488 switch (_hash) { 489 case 93508670: /*basis*/ return new Property("basis", "CodeableConcept", "This would be a codeableconcept, or a coding, which can be constrained to , for example, the 6 grounds for processing in GDPR.", 0, java.lang.Integer.MAX_VALUE, basis); 490 case 382967383: /*evidence*/ return new Property("evidence", "Reference(Any)", "Justifing rational.", 0, java.lang.Integer.MAX_VALUE, evidence); 491 default: return super.getNamedProperty(_hash, _name, _checkValid); 492 } 493 494 } 495 496 @Override 497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 498 switch (hash) { 499 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : this.basis.toArray(new Base[this.basis.size()]); // CodeableConcept 500 case 382967383: /*evidence*/ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // Reference 501 default: return super.getProperty(hash, name, checkValid); 502 } 503 504 } 505 506 @Override 507 public Base setProperty(int hash, String name, Base value) throws FHIRException { 508 switch (hash) { 509 case 93508670: // basis 510 this.getBasis().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 511 return value; 512 case 382967383: // evidence 513 this.getEvidence().add(TypeConvertor.castToReference(value)); // Reference 514 return value; 515 default: return super.setProperty(hash, name, value); 516 } 517 518 } 519 520 @Override 521 public Base setProperty(String name, Base value) throws FHIRException { 522 if (name.equals("basis")) { 523 this.getBasis().add(TypeConvertor.castToCodeableConcept(value)); 524 } else if (name.equals("evidence")) { 525 this.getEvidence().add(TypeConvertor.castToReference(value)); 526 } else 527 return super.setProperty(name, value); 528 return value; 529 } 530 531 @Override 532 public void removeChild(String name, Base value) throws FHIRException { 533 if (name.equals("basis")) { 534 this.getBasis().remove(value); 535 } else if (name.equals("evidence")) { 536 this.getEvidence().remove(value); 537 } else 538 super.removeChild(name, value); 539 540 } 541 542 @Override 543 public Base makeProperty(int hash, String name) throws FHIRException { 544 switch (hash) { 545 case 93508670: return addBasis(); 546 case 382967383: return addEvidence(); 547 default: return super.makeProperty(hash, name); 548 } 549 550 } 551 552 @Override 553 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 554 switch (hash) { 555 case 93508670: /*basis*/ return new String[] {"CodeableConcept"}; 556 case 382967383: /*evidence*/ return new String[] {"Reference"}; 557 default: return super.getTypesForProperty(hash, name); 558 } 559 560 } 561 562 @Override 563 public Base addChild(String name) throws FHIRException { 564 if (name.equals("basis")) { 565 return addBasis(); 566 } 567 else if (name.equals("evidence")) { 568 return addEvidence(); 569 } 570 else 571 return super.addChild(name); 572 } 573 574 public PermissionJustificationComponent copy() { 575 PermissionJustificationComponent dst = new PermissionJustificationComponent(); 576 copyValues(dst); 577 return dst; 578 } 579 580 public void copyValues(PermissionJustificationComponent dst) { 581 super.copyValues(dst); 582 if (basis != null) { 583 dst.basis = new ArrayList<CodeableConcept>(); 584 for (CodeableConcept i : basis) 585 dst.basis.add(i.copy()); 586 }; 587 if (evidence != null) { 588 dst.evidence = new ArrayList<Reference>(); 589 for (Reference i : evidence) 590 dst.evidence.add(i.copy()); 591 }; 592 } 593 594 @Override 595 public boolean equalsDeep(Base other_) { 596 if (!super.equalsDeep(other_)) 597 return false; 598 if (!(other_ instanceof PermissionJustificationComponent)) 599 return false; 600 PermissionJustificationComponent o = (PermissionJustificationComponent) other_; 601 return compareDeep(basis, o.basis, true) && compareDeep(evidence, o.evidence, true); 602 } 603 604 @Override 605 public boolean equalsShallow(Base other_) { 606 if (!super.equalsShallow(other_)) 607 return false; 608 if (!(other_ instanceof PermissionJustificationComponent)) 609 return false; 610 PermissionJustificationComponent o = (PermissionJustificationComponent) other_; 611 return true; 612 } 613 614 public boolean isEmpty() { 615 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(basis, evidence); 616 } 617 618 public String fhirType() { 619 return "Permission.justification"; 620 621 } 622 623 } 624 625 @Block() 626 public static class RuleComponent extends BackboneElement implements IBaseBackboneElement { 627 /** 628 * deny | permit. 629 */ 630 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 631 @Description(shortDefinition="deny | permit", formalDefinition="deny | permit." ) 632 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-provision-type") 633 protected Enumeration<ConsentProvisionType> type; 634 635 /** 636 * A description or definition of which activities are allowed to be done on the data. 637 */ 638 @Child(name = "data", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 639 @Description(shortDefinition="The selection criteria to identify data that is within scope of this provision", formalDefinition="A description or definition of which activities are allowed to be done on the data." ) 640 protected List<RuleDataComponent> data; 641 642 /** 643 * A description or definition of which activities are allowed to be done on the data. 644 */ 645 @Child(name = "activity", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 646 @Description(shortDefinition="A description or definition of which activities are allowed to be done on the data", formalDefinition="A description or definition of which activities are allowed to be done on the data." ) 647 protected List<RuleActivityComponent> activity; 648 649 /** 650 * What limits apply to the use of the data. 651 */ 652 @Child(name = "limit", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 653 @Description(shortDefinition="What limits apply to the use of the data", formalDefinition="What limits apply to the use of the data." ) 654 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-label-event-examples") 655 protected List<CodeableConcept> limit; 656 657 private static final long serialVersionUID = 1376717588L; 658 659 /** 660 * Constructor 661 */ 662 public RuleComponent() { 663 super(); 664 } 665 666 /** 667 * @return {@link #type} (deny | permit.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 668 */ 669 public Enumeration<ConsentProvisionType> getTypeElement() { 670 if (this.type == null) 671 if (Configuration.errorOnAutoCreate()) 672 throw new Error("Attempt to auto-create RuleComponent.type"); 673 else if (Configuration.doAutoCreate()) 674 this.type = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); // bb 675 return this.type; 676 } 677 678 public boolean hasTypeElement() { 679 return this.type != null && !this.type.isEmpty(); 680 } 681 682 public boolean hasType() { 683 return this.type != null && !this.type.isEmpty(); 684 } 685 686 /** 687 * @param value {@link #type} (deny | permit.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 688 */ 689 public RuleComponent setTypeElement(Enumeration<ConsentProvisionType> value) { 690 this.type = value; 691 return this; 692 } 693 694 /** 695 * @return deny | permit. 696 */ 697 public ConsentProvisionType getType() { 698 return this.type == null ? null : this.type.getValue(); 699 } 700 701 /** 702 * @param value deny | permit. 703 */ 704 public RuleComponent setType(ConsentProvisionType value) { 705 if (value == null) 706 this.type = null; 707 else { 708 if (this.type == null) 709 this.type = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); 710 this.type.setValue(value); 711 } 712 return this; 713 } 714 715 /** 716 * @return {@link #data} (A description or definition of which activities are allowed to be done on the data.) 717 */ 718 public List<RuleDataComponent> getData() { 719 if (this.data == null) 720 this.data = new ArrayList<RuleDataComponent>(); 721 return this.data; 722 } 723 724 /** 725 * @return Returns a reference to <code>this</code> for easy method chaining 726 */ 727 public RuleComponent setData(List<RuleDataComponent> theData) { 728 this.data = theData; 729 return this; 730 } 731 732 public boolean hasData() { 733 if (this.data == null) 734 return false; 735 for (RuleDataComponent item : this.data) 736 if (!item.isEmpty()) 737 return true; 738 return false; 739 } 740 741 public RuleDataComponent addData() { //3 742 RuleDataComponent t = new RuleDataComponent(); 743 if (this.data == null) 744 this.data = new ArrayList<RuleDataComponent>(); 745 this.data.add(t); 746 return t; 747 } 748 749 public RuleComponent addData(RuleDataComponent t) { //3 750 if (t == null) 751 return this; 752 if (this.data == null) 753 this.data = new ArrayList<RuleDataComponent>(); 754 this.data.add(t); 755 return this; 756 } 757 758 /** 759 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist {3} 760 */ 761 public RuleDataComponent getDataFirstRep() { 762 if (getData().isEmpty()) { 763 addData(); 764 } 765 return getData().get(0); 766 } 767 768 /** 769 * @return {@link #activity} (A description or definition of which activities are allowed to be done on the data.) 770 */ 771 public List<RuleActivityComponent> getActivity() { 772 if (this.activity == null) 773 this.activity = new ArrayList<RuleActivityComponent>(); 774 return this.activity; 775 } 776 777 /** 778 * @return Returns a reference to <code>this</code> for easy method chaining 779 */ 780 public RuleComponent setActivity(List<RuleActivityComponent> theActivity) { 781 this.activity = theActivity; 782 return this; 783 } 784 785 public boolean hasActivity() { 786 if (this.activity == null) 787 return false; 788 for (RuleActivityComponent item : this.activity) 789 if (!item.isEmpty()) 790 return true; 791 return false; 792 } 793 794 public RuleActivityComponent addActivity() { //3 795 RuleActivityComponent t = new RuleActivityComponent(); 796 if (this.activity == null) 797 this.activity = new ArrayList<RuleActivityComponent>(); 798 this.activity.add(t); 799 return t; 800 } 801 802 public RuleComponent addActivity(RuleActivityComponent t) { //3 803 if (t == null) 804 return this; 805 if (this.activity == null) 806 this.activity = new ArrayList<RuleActivityComponent>(); 807 this.activity.add(t); 808 return this; 809 } 810 811 /** 812 * @return The first repetition of repeating field {@link #activity}, creating it if it does not already exist {3} 813 */ 814 public RuleActivityComponent getActivityFirstRep() { 815 if (getActivity().isEmpty()) { 816 addActivity(); 817 } 818 return getActivity().get(0); 819 } 820 821 /** 822 * @return {@link #limit} (What limits apply to the use of the data.) 823 */ 824 public List<CodeableConcept> getLimit() { 825 if (this.limit == null) 826 this.limit = new ArrayList<CodeableConcept>(); 827 return this.limit; 828 } 829 830 /** 831 * @return Returns a reference to <code>this</code> for easy method chaining 832 */ 833 public RuleComponent setLimit(List<CodeableConcept> theLimit) { 834 this.limit = theLimit; 835 return this; 836 } 837 838 public boolean hasLimit() { 839 if (this.limit == null) 840 return false; 841 for (CodeableConcept item : this.limit) 842 if (!item.isEmpty()) 843 return true; 844 return false; 845 } 846 847 public CodeableConcept addLimit() { //3 848 CodeableConcept t = new CodeableConcept(); 849 if (this.limit == null) 850 this.limit = new ArrayList<CodeableConcept>(); 851 this.limit.add(t); 852 return t; 853 } 854 855 public RuleComponent addLimit(CodeableConcept t) { //3 856 if (t == null) 857 return this; 858 if (this.limit == null) 859 this.limit = new ArrayList<CodeableConcept>(); 860 this.limit.add(t); 861 return this; 862 } 863 864 /** 865 * @return The first repetition of repeating field {@link #limit}, creating it if it does not already exist {3} 866 */ 867 public CodeableConcept getLimitFirstRep() { 868 if (getLimit().isEmpty()) { 869 addLimit(); 870 } 871 return getLimit().get(0); 872 } 873 874 protected void listChildren(List<Property> children) { 875 super.listChildren(children); 876 children.add(new Property("type", "code", "deny | permit.", 0, 1, type)); 877 children.add(new Property("data", "", "A description or definition of which activities are allowed to be done on the data.", 0, java.lang.Integer.MAX_VALUE, data)); 878 children.add(new Property("activity", "", "A description or definition of which activities are allowed to be done on the data.", 0, java.lang.Integer.MAX_VALUE, activity)); 879 children.add(new Property("limit", "CodeableConcept", "What limits apply to the use of the data.", 0, java.lang.Integer.MAX_VALUE, limit)); 880 } 881 882 @Override 883 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 884 switch (_hash) { 885 case 3575610: /*type*/ return new Property("type", "code", "deny | permit.", 0, 1, type); 886 case 3076010: /*data*/ return new Property("data", "", "A description or definition of which activities are allowed to be done on the data.", 0, java.lang.Integer.MAX_VALUE, data); 887 case -1655966961: /*activity*/ return new Property("activity", "", "A description or definition of which activities are allowed to be done on the data.", 0, java.lang.Integer.MAX_VALUE, activity); 888 case 102976443: /*limit*/ return new Property("limit", "CodeableConcept", "What limits apply to the use of the data.", 0, java.lang.Integer.MAX_VALUE, limit); 889 default: return super.getNamedProperty(_hash, _name, _checkValid); 890 } 891 892 } 893 894 @Override 895 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 896 switch (hash) { 897 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ConsentProvisionType> 898 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // RuleDataComponent 899 case -1655966961: /*activity*/ return this.activity == null ? new Base[0] : this.activity.toArray(new Base[this.activity.size()]); // RuleActivityComponent 900 case 102976443: /*limit*/ return this.limit == null ? new Base[0] : this.limit.toArray(new Base[this.limit.size()]); // CodeableConcept 901 default: return super.getProperty(hash, name, checkValid); 902 } 903 904 } 905 906 @Override 907 public Base setProperty(int hash, String name, Base value) throws FHIRException { 908 switch (hash) { 909 case 3575610: // type 910 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 911 this.type = (Enumeration) value; // Enumeration<ConsentProvisionType> 912 return value; 913 case 3076010: // data 914 this.getData().add((RuleDataComponent) value); // RuleDataComponent 915 return value; 916 case -1655966961: // activity 917 this.getActivity().add((RuleActivityComponent) value); // RuleActivityComponent 918 return value; 919 case 102976443: // limit 920 this.getLimit().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 921 return value; 922 default: return super.setProperty(hash, name, value); 923 } 924 925 } 926 927 @Override 928 public Base setProperty(String name, Base value) throws FHIRException { 929 if (name.equals("type")) { 930 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 931 this.type = (Enumeration) value; // Enumeration<ConsentProvisionType> 932 } else if (name.equals("data")) { 933 this.getData().add((RuleDataComponent) value); 934 } else if (name.equals("activity")) { 935 this.getActivity().add((RuleActivityComponent) value); 936 } else if (name.equals("limit")) { 937 this.getLimit().add(TypeConvertor.castToCodeableConcept(value)); 938 } else 939 return super.setProperty(name, value); 940 return value; 941 } 942 943 @Override 944 public void removeChild(String name, Base value) throws FHIRException { 945 if (name.equals("type")) { 946 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 947 this.type = (Enumeration) value; // Enumeration<ConsentProvisionType> 948 } else if (name.equals("data")) { 949 this.getData().remove((RuleDataComponent) value); 950 } else if (name.equals("activity")) { 951 this.getActivity().remove((RuleActivityComponent) value); 952 } else if (name.equals("limit")) { 953 this.getLimit().remove(value); 954 } else 955 super.removeChild(name, value); 956 957 } 958 959 @Override 960 public Base makeProperty(int hash, String name) throws FHIRException { 961 switch (hash) { 962 case 3575610: return getTypeElement(); 963 case 3076010: return addData(); 964 case -1655966961: return addActivity(); 965 case 102976443: return addLimit(); 966 default: return super.makeProperty(hash, name); 967 } 968 969 } 970 971 @Override 972 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 973 switch (hash) { 974 case 3575610: /*type*/ return new String[] {"code"}; 975 case 3076010: /*data*/ return new String[] {}; 976 case -1655966961: /*activity*/ return new String[] {}; 977 case 102976443: /*limit*/ return new String[] {"CodeableConcept"}; 978 default: return super.getTypesForProperty(hash, name); 979 } 980 981 } 982 983 @Override 984 public Base addChild(String name) throws FHIRException { 985 if (name.equals("type")) { 986 throw new FHIRException("Cannot call addChild on a singleton property Permission.rule.type"); 987 } 988 else if (name.equals("data")) { 989 return addData(); 990 } 991 else if (name.equals("activity")) { 992 return addActivity(); 993 } 994 else if (name.equals("limit")) { 995 return addLimit(); 996 } 997 else 998 return super.addChild(name); 999 } 1000 1001 public RuleComponent copy() { 1002 RuleComponent dst = new RuleComponent(); 1003 copyValues(dst); 1004 return dst; 1005 } 1006 1007 public void copyValues(RuleComponent dst) { 1008 super.copyValues(dst); 1009 dst.type = type == null ? null : type.copy(); 1010 if (data != null) { 1011 dst.data = new ArrayList<RuleDataComponent>(); 1012 for (RuleDataComponent i : data) 1013 dst.data.add(i.copy()); 1014 }; 1015 if (activity != null) { 1016 dst.activity = new ArrayList<RuleActivityComponent>(); 1017 for (RuleActivityComponent i : activity) 1018 dst.activity.add(i.copy()); 1019 }; 1020 if (limit != null) { 1021 dst.limit = new ArrayList<CodeableConcept>(); 1022 for (CodeableConcept i : limit) 1023 dst.limit.add(i.copy()); 1024 }; 1025 } 1026 1027 @Override 1028 public boolean equalsDeep(Base other_) { 1029 if (!super.equalsDeep(other_)) 1030 return false; 1031 if (!(other_ instanceof RuleComponent)) 1032 return false; 1033 RuleComponent o = (RuleComponent) other_; 1034 return compareDeep(type, o.type, true) && compareDeep(data, o.data, true) && compareDeep(activity, o.activity, true) 1035 && compareDeep(limit, o.limit, true); 1036 } 1037 1038 @Override 1039 public boolean equalsShallow(Base other_) { 1040 if (!super.equalsShallow(other_)) 1041 return false; 1042 if (!(other_ instanceof RuleComponent)) 1043 return false; 1044 RuleComponent o = (RuleComponent) other_; 1045 return compareValues(type, o.type, true); 1046 } 1047 1048 public boolean isEmpty() { 1049 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, data, activity, limit 1050 ); 1051 } 1052 1053 public String fhirType() { 1054 return "Permission.rule"; 1055 1056 } 1057 1058 } 1059 1060 @Block() 1061 public static class RuleDataComponent extends BackboneElement implements IBaseBackboneElement { 1062 /** 1063 * Explicit FHIR Resource references. 1064 */ 1065 @Child(name = "resource", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1066 @Description(shortDefinition="Explicit FHIR Resource references", formalDefinition="Explicit FHIR Resource references." ) 1067 protected List<RuleDataResourceComponent> resource; 1068 1069 /** 1070 * The data in scope are those with the given codes present in that data .meta.security element. 1071 */ 1072 @Child(name = "security", type = {Coding.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1073 @Description(shortDefinition="Security tag code on .meta.security", formalDefinition="The data in scope are those with the given codes present in that data .meta.security element." ) 1074 protected List<Coding> security; 1075 1076 /** 1077 * Clinical or Operational Relevant period of time that bounds the data controlled by this rule. 1078 */ 1079 @Child(name = "period", type = {Period.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1080 @Description(shortDefinition="Timeframe encompasing data create/update", formalDefinition="Clinical or Operational Relevant period of time that bounds the data controlled by this rule." ) 1081 protected List<Period> period; 1082 1083 /** 1084 * Used when other data selection elements are insufficient. 1085 */ 1086 @Child(name = "expression", type = {Expression.class}, order=4, min=0, max=1, modifier=false, summary=true) 1087 @Description(shortDefinition="Expression identifying the data", formalDefinition="Used when other data selection elements are insufficient." ) 1088 protected Expression expression; 1089 1090 private static final long serialVersionUID = -774403139L; 1091 1092 /** 1093 * Constructor 1094 */ 1095 public RuleDataComponent() { 1096 super(); 1097 } 1098 1099 /** 1100 * @return {@link #resource} (Explicit FHIR Resource references.) 1101 */ 1102 public List<RuleDataResourceComponent> getResource() { 1103 if (this.resource == null) 1104 this.resource = new ArrayList<RuleDataResourceComponent>(); 1105 return this.resource; 1106 } 1107 1108 /** 1109 * @return Returns a reference to <code>this</code> for easy method chaining 1110 */ 1111 public RuleDataComponent setResource(List<RuleDataResourceComponent> theResource) { 1112 this.resource = theResource; 1113 return this; 1114 } 1115 1116 public boolean hasResource() { 1117 if (this.resource == null) 1118 return false; 1119 for (RuleDataResourceComponent item : this.resource) 1120 if (!item.isEmpty()) 1121 return true; 1122 return false; 1123 } 1124 1125 public RuleDataResourceComponent addResource() { //3 1126 RuleDataResourceComponent t = new RuleDataResourceComponent(); 1127 if (this.resource == null) 1128 this.resource = new ArrayList<RuleDataResourceComponent>(); 1129 this.resource.add(t); 1130 return t; 1131 } 1132 1133 public RuleDataComponent addResource(RuleDataResourceComponent t) { //3 1134 if (t == null) 1135 return this; 1136 if (this.resource == null) 1137 this.resource = new ArrayList<RuleDataResourceComponent>(); 1138 this.resource.add(t); 1139 return this; 1140 } 1141 1142 /** 1143 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist {3} 1144 */ 1145 public RuleDataResourceComponent getResourceFirstRep() { 1146 if (getResource().isEmpty()) { 1147 addResource(); 1148 } 1149 return getResource().get(0); 1150 } 1151 1152 /** 1153 * @return {@link #security} (The data in scope are those with the given codes present in that data .meta.security element.) 1154 */ 1155 public List<Coding> getSecurity() { 1156 if (this.security == null) 1157 this.security = new ArrayList<Coding>(); 1158 return this.security; 1159 } 1160 1161 /** 1162 * @return Returns a reference to <code>this</code> for easy method chaining 1163 */ 1164 public RuleDataComponent setSecurity(List<Coding> theSecurity) { 1165 this.security = theSecurity; 1166 return this; 1167 } 1168 1169 public boolean hasSecurity() { 1170 if (this.security == null) 1171 return false; 1172 for (Coding item : this.security) 1173 if (!item.isEmpty()) 1174 return true; 1175 return false; 1176 } 1177 1178 public Coding addSecurity() { //3 1179 Coding t = new Coding(); 1180 if (this.security == null) 1181 this.security = new ArrayList<Coding>(); 1182 this.security.add(t); 1183 return t; 1184 } 1185 1186 public RuleDataComponent addSecurity(Coding t) { //3 1187 if (t == null) 1188 return this; 1189 if (this.security == null) 1190 this.security = new ArrayList<Coding>(); 1191 this.security.add(t); 1192 return this; 1193 } 1194 1195 /** 1196 * @return The first repetition of repeating field {@link #security}, creating it if it does not already exist {3} 1197 */ 1198 public Coding getSecurityFirstRep() { 1199 if (getSecurity().isEmpty()) { 1200 addSecurity(); 1201 } 1202 return getSecurity().get(0); 1203 } 1204 1205 /** 1206 * @return {@link #period} (Clinical or Operational Relevant period of time that bounds the data controlled by this rule.) 1207 */ 1208 public List<Period> getPeriod() { 1209 if (this.period == null) 1210 this.period = new ArrayList<Period>(); 1211 return this.period; 1212 } 1213 1214 /** 1215 * @return Returns a reference to <code>this</code> for easy method chaining 1216 */ 1217 public RuleDataComponent setPeriod(List<Period> thePeriod) { 1218 this.period = thePeriod; 1219 return this; 1220 } 1221 1222 public boolean hasPeriod() { 1223 if (this.period == null) 1224 return false; 1225 for (Period item : this.period) 1226 if (!item.isEmpty()) 1227 return true; 1228 return false; 1229 } 1230 1231 public Period addPeriod() { //3 1232 Period t = new Period(); 1233 if (this.period == null) 1234 this.period = new ArrayList<Period>(); 1235 this.period.add(t); 1236 return t; 1237 } 1238 1239 public RuleDataComponent addPeriod(Period t) { //3 1240 if (t == null) 1241 return this; 1242 if (this.period == null) 1243 this.period = new ArrayList<Period>(); 1244 this.period.add(t); 1245 return this; 1246 } 1247 1248 /** 1249 * @return The first repetition of repeating field {@link #period}, creating it if it does not already exist {3} 1250 */ 1251 public Period getPeriodFirstRep() { 1252 if (getPeriod().isEmpty()) { 1253 addPeriod(); 1254 } 1255 return getPeriod().get(0); 1256 } 1257 1258 /** 1259 * @return {@link #expression} (Used when other data selection elements are insufficient.) 1260 */ 1261 public Expression getExpression() { 1262 if (this.expression == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create RuleDataComponent.expression"); 1265 else if (Configuration.doAutoCreate()) 1266 this.expression = new Expression(); // cc 1267 return this.expression; 1268 } 1269 1270 public boolean hasExpression() { 1271 return this.expression != null && !this.expression.isEmpty(); 1272 } 1273 1274 /** 1275 * @param value {@link #expression} (Used when other data selection elements are insufficient.) 1276 */ 1277 public RuleDataComponent setExpression(Expression value) { 1278 this.expression = value; 1279 return this; 1280 } 1281 1282 protected void listChildren(List<Property> children) { 1283 super.listChildren(children); 1284 children.add(new Property("resource", "", "Explicit FHIR Resource references.", 0, java.lang.Integer.MAX_VALUE, resource)); 1285 children.add(new Property("security", "Coding", "The data in scope are those with the given codes present in that data .meta.security element.", 0, java.lang.Integer.MAX_VALUE, security)); 1286 children.add(new Property("period", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this rule.", 0, java.lang.Integer.MAX_VALUE, period)); 1287 children.add(new Property("expression", "Expression", "Used when other data selection elements are insufficient.", 0, 1, expression)); 1288 } 1289 1290 @Override 1291 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1292 switch (_hash) { 1293 case -341064690: /*resource*/ return new Property("resource", "", "Explicit FHIR Resource references.", 0, java.lang.Integer.MAX_VALUE, resource); 1294 case 949122880: /*security*/ return new Property("security", "Coding", "The data in scope are those with the given codes present in that data .meta.security element.", 0, java.lang.Integer.MAX_VALUE, security); 1295 case -991726143: /*period*/ return new Property("period", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this rule.", 0, java.lang.Integer.MAX_VALUE, period); 1296 case -1795452264: /*expression*/ return new Property("expression", "Expression", "Used when other data selection elements are insufficient.", 0, 1, expression); 1297 default: return super.getNamedProperty(_hash, _name, _checkValid); 1298 } 1299 1300 } 1301 1302 @Override 1303 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1304 switch (hash) { 1305 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // RuleDataResourceComponent 1306 case 949122880: /*security*/ return this.security == null ? new Base[0] : this.security.toArray(new Base[this.security.size()]); // Coding 1307 case -991726143: /*period*/ return this.period == null ? new Base[0] : this.period.toArray(new Base[this.period.size()]); // Period 1308 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 1309 default: return super.getProperty(hash, name, checkValid); 1310 } 1311 1312 } 1313 1314 @Override 1315 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1316 switch (hash) { 1317 case -341064690: // resource 1318 this.getResource().add((RuleDataResourceComponent) value); // RuleDataResourceComponent 1319 return value; 1320 case 949122880: // security 1321 this.getSecurity().add(TypeConvertor.castToCoding(value)); // Coding 1322 return value; 1323 case -991726143: // period 1324 this.getPeriod().add(TypeConvertor.castToPeriod(value)); // Period 1325 return value; 1326 case -1795452264: // expression 1327 this.expression = TypeConvertor.castToExpression(value); // Expression 1328 return value; 1329 default: return super.setProperty(hash, name, value); 1330 } 1331 1332 } 1333 1334 @Override 1335 public Base setProperty(String name, Base value) throws FHIRException { 1336 if (name.equals("resource")) { 1337 this.getResource().add((RuleDataResourceComponent) value); 1338 } else if (name.equals("security")) { 1339 this.getSecurity().add(TypeConvertor.castToCoding(value)); 1340 } else if (name.equals("period")) { 1341 this.getPeriod().add(TypeConvertor.castToPeriod(value)); 1342 } else if (name.equals("expression")) { 1343 this.expression = TypeConvertor.castToExpression(value); // Expression 1344 } else 1345 return super.setProperty(name, value); 1346 return value; 1347 } 1348 1349 @Override 1350 public void removeChild(String name, Base value) throws FHIRException { 1351 if (name.equals("resource")) { 1352 this.getResource().remove((RuleDataResourceComponent) value); 1353 } else if (name.equals("security")) { 1354 this.getSecurity().remove(value); 1355 } else if (name.equals("period")) { 1356 this.getPeriod().remove(value); 1357 } else if (name.equals("expression")) { 1358 this.expression = null; 1359 } else 1360 super.removeChild(name, value); 1361 1362 } 1363 1364 @Override 1365 public Base makeProperty(int hash, String name) throws FHIRException { 1366 switch (hash) { 1367 case -341064690: return addResource(); 1368 case 949122880: return addSecurity(); 1369 case -991726143: return addPeriod(); 1370 case -1795452264: return getExpression(); 1371 default: return super.makeProperty(hash, name); 1372 } 1373 1374 } 1375 1376 @Override 1377 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1378 switch (hash) { 1379 case -341064690: /*resource*/ return new String[] {}; 1380 case 949122880: /*security*/ return new String[] {"Coding"}; 1381 case -991726143: /*period*/ return new String[] {"Period"}; 1382 case -1795452264: /*expression*/ return new String[] {"Expression"}; 1383 default: return super.getTypesForProperty(hash, name); 1384 } 1385 1386 } 1387 1388 @Override 1389 public Base addChild(String name) throws FHIRException { 1390 if (name.equals("resource")) { 1391 return addResource(); 1392 } 1393 else if (name.equals("security")) { 1394 return addSecurity(); 1395 } 1396 else if (name.equals("period")) { 1397 return addPeriod(); 1398 } 1399 else if (name.equals("expression")) { 1400 this.expression = new Expression(); 1401 return this.expression; 1402 } 1403 else 1404 return super.addChild(name); 1405 } 1406 1407 public RuleDataComponent copy() { 1408 RuleDataComponent dst = new RuleDataComponent(); 1409 copyValues(dst); 1410 return dst; 1411 } 1412 1413 public void copyValues(RuleDataComponent dst) { 1414 super.copyValues(dst); 1415 if (resource != null) { 1416 dst.resource = new ArrayList<RuleDataResourceComponent>(); 1417 for (RuleDataResourceComponent i : resource) 1418 dst.resource.add(i.copy()); 1419 }; 1420 if (security != null) { 1421 dst.security = new ArrayList<Coding>(); 1422 for (Coding i : security) 1423 dst.security.add(i.copy()); 1424 }; 1425 if (period != null) { 1426 dst.period = new ArrayList<Period>(); 1427 for (Period i : period) 1428 dst.period.add(i.copy()); 1429 }; 1430 dst.expression = expression == null ? null : expression.copy(); 1431 } 1432 1433 @Override 1434 public boolean equalsDeep(Base other_) { 1435 if (!super.equalsDeep(other_)) 1436 return false; 1437 if (!(other_ instanceof RuleDataComponent)) 1438 return false; 1439 RuleDataComponent o = (RuleDataComponent) other_; 1440 return compareDeep(resource, o.resource, true) && compareDeep(security, o.security, true) && compareDeep(period, o.period, true) 1441 && compareDeep(expression, o.expression, true); 1442 } 1443 1444 @Override 1445 public boolean equalsShallow(Base other_) { 1446 if (!super.equalsShallow(other_)) 1447 return false; 1448 if (!(other_ instanceof RuleDataComponent)) 1449 return false; 1450 RuleDataComponent o = (RuleDataComponent) other_; 1451 return true; 1452 } 1453 1454 public boolean isEmpty() { 1455 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resource, security, period 1456 , expression); 1457 } 1458 1459 public String fhirType() { 1460 return "Permission.rule.data"; 1461 1462 } 1463 1464 } 1465 1466 @Block() 1467 public static class RuleDataResourceComponent extends BackboneElement implements IBaseBackboneElement { 1468 /** 1469 * How the resource reference is interpreted when testing consent restrictions. 1470 */ 1471 @Child(name = "meaning", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1472 @Description(shortDefinition="instance | related | dependents | authoredby", formalDefinition="How the resource reference is interpreted when testing consent restrictions." ) 1473 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-data-meaning") 1474 protected Enumeration<ConsentDataMeaning> meaning; 1475 1476 /** 1477 * A reference to a specific resource that defines which resources are covered by this consent. 1478 */ 1479 @Child(name = "reference", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 1480 @Description(shortDefinition="The actual data reference", formalDefinition="A reference to a specific resource that defines which resources are covered by this consent." ) 1481 protected Reference reference; 1482 1483 private static final long serialVersionUID = 1735979153L; 1484 1485 /** 1486 * Constructor 1487 */ 1488 public RuleDataResourceComponent() { 1489 super(); 1490 } 1491 1492 /** 1493 * Constructor 1494 */ 1495 public RuleDataResourceComponent(ConsentDataMeaning meaning, Reference reference) { 1496 super(); 1497 this.setMeaning(meaning); 1498 this.setReference(reference); 1499 } 1500 1501 /** 1502 * @return {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 1503 */ 1504 public Enumeration<ConsentDataMeaning> getMeaningElement() { 1505 if (this.meaning == null) 1506 if (Configuration.errorOnAutoCreate()) 1507 throw new Error("Attempt to auto-create RuleDataResourceComponent.meaning"); 1508 else if (Configuration.doAutoCreate()) 1509 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 1510 return this.meaning; 1511 } 1512 1513 public boolean hasMeaningElement() { 1514 return this.meaning != null && !this.meaning.isEmpty(); 1515 } 1516 1517 public boolean hasMeaning() { 1518 return this.meaning != null && !this.meaning.isEmpty(); 1519 } 1520 1521 /** 1522 * @param value {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 1523 */ 1524 public RuleDataResourceComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 1525 this.meaning = value; 1526 return this; 1527 } 1528 1529 /** 1530 * @return How the resource reference is interpreted when testing consent restrictions. 1531 */ 1532 public ConsentDataMeaning getMeaning() { 1533 return this.meaning == null ? null : this.meaning.getValue(); 1534 } 1535 1536 /** 1537 * @param value How the resource reference is interpreted when testing consent restrictions. 1538 */ 1539 public RuleDataResourceComponent setMeaning(ConsentDataMeaning value) { 1540 if (this.meaning == null) 1541 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 1542 this.meaning.setValue(value); 1543 return this; 1544 } 1545 1546 /** 1547 * @return {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 1548 */ 1549 public Reference getReference() { 1550 if (this.reference == null) 1551 if (Configuration.errorOnAutoCreate()) 1552 throw new Error("Attempt to auto-create RuleDataResourceComponent.reference"); 1553 else if (Configuration.doAutoCreate()) 1554 this.reference = new Reference(); // cc 1555 return this.reference; 1556 } 1557 1558 public boolean hasReference() { 1559 return this.reference != null && !this.reference.isEmpty(); 1560 } 1561 1562 /** 1563 * @param value {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 1564 */ 1565 public RuleDataResourceComponent setReference(Reference value) { 1566 this.reference = value; 1567 return this; 1568 } 1569 1570 protected void listChildren(List<Property> children) { 1571 super.listChildren(children); 1572 children.add(new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 1573 children.add(new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference)); 1574 } 1575 1576 @Override 1577 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1578 switch (_hash) { 1579 case 938160637: /*meaning*/ return new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 1580 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference); 1581 default: return super.getNamedProperty(_hash, _name, _checkValid); 1582 } 1583 1584 } 1585 1586 @Override 1587 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1588 switch (hash) { 1589 case 938160637: /*meaning*/ return this.meaning == null ? new Base[0] : new Base[] {this.meaning}; // Enumeration<ConsentDataMeaning> 1590 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1591 default: return super.getProperty(hash, name, checkValid); 1592 } 1593 1594 } 1595 1596 @Override 1597 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1598 switch (hash) { 1599 case 938160637: // meaning 1600 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 1601 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 1602 return value; 1603 case -925155509: // reference 1604 this.reference = TypeConvertor.castToReference(value); // Reference 1605 return value; 1606 default: return super.setProperty(hash, name, value); 1607 } 1608 1609 } 1610 1611 @Override 1612 public Base setProperty(String name, Base value) throws FHIRException { 1613 if (name.equals("meaning")) { 1614 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 1615 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 1616 } else if (name.equals("reference")) { 1617 this.reference = TypeConvertor.castToReference(value); // Reference 1618 } else 1619 return super.setProperty(name, value); 1620 return value; 1621 } 1622 1623 @Override 1624 public void removeChild(String name, Base value) throws FHIRException { 1625 if (name.equals("meaning")) { 1626 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 1627 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 1628 } else if (name.equals("reference")) { 1629 this.reference = null; 1630 } else 1631 super.removeChild(name, value); 1632 1633 } 1634 1635 @Override 1636 public Base makeProperty(int hash, String name) throws FHIRException { 1637 switch (hash) { 1638 case 938160637: return getMeaningElement(); 1639 case -925155509: return getReference(); 1640 default: return super.makeProperty(hash, name); 1641 } 1642 1643 } 1644 1645 @Override 1646 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1647 switch (hash) { 1648 case 938160637: /*meaning*/ return new String[] {"code"}; 1649 case -925155509: /*reference*/ return new String[] {"Reference"}; 1650 default: return super.getTypesForProperty(hash, name); 1651 } 1652 1653 } 1654 1655 @Override 1656 public Base addChild(String name) throws FHIRException { 1657 if (name.equals("meaning")) { 1658 throw new FHIRException("Cannot call addChild on a singleton property Permission.rule.data.resource.meaning"); 1659 } 1660 else if (name.equals("reference")) { 1661 this.reference = new Reference(); 1662 return this.reference; 1663 } 1664 else 1665 return super.addChild(name); 1666 } 1667 1668 public RuleDataResourceComponent copy() { 1669 RuleDataResourceComponent dst = new RuleDataResourceComponent(); 1670 copyValues(dst); 1671 return dst; 1672 } 1673 1674 public void copyValues(RuleDataResourceComponent dst) { 1675 super.copyValues(dst); 1676 dst.meaning = meaning == null ? null : meaning.copy(); 1677 dst.reference = reference == null ? null : reference.copy(); 1678 } 1679 1680 @Override 1681 public boolean equalsDeep(Base other_) { 1682 if (!super.equalsDeep(other_)) 1683 return false; 1684 if (!(other_ instanceof RuleDataResourceComponent)) 1685 return false; 1686 RuleDataResourceComponent o = (RuleDataResourceComponent) other_; 1687 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 1688 } 1689 1690 @Override 1691 public boolean equalsShallow(Base other_) { 1692 if (!super.equalsShallow(other_)) 1693 return false; 1694 if (!(other_ instanceof RuleDataResourceComponent)) 1695 return false; 1696 RuleDataResourceComponent o = (RuleDataResourceComponent) other_; 1697 return compareValues(meaning, o.meaning, true); 1698 } 1699 1700 public boolean isEmpty() { 1701 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 1702 } 1703 1704 public String fhirType() { 1705 return "Permission.rule.data.resource"; 1706 1707 } 1708 1709 } 1710 1711 @Block() 1712 public static class RuleActivityComponent extends BackboneElement implements IBaseBackboneElement { 1713 /** 1714 * The actor(s) authorized for the defined activity. 1715 */ 1716 @Child(name = "actor", type = {Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, PractitionerRole.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1717 @Description(shortDefinition="Authorized actor(s)", formalDefinition="The actor(s) authorized for the defined activity." ) 1718 protected List<Reference> actor; 1719 1720 /** 1721 * Actions controlled by this Rule. 1722 */ 1723 @Child(name = "action", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1724 @Description(shortDefinition="Actions controlled by this rule", formalDefinition="Actions controlled by this Rule." ) 1725 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-action") 1726 protected List<CodeableConcept> action; 1727 1728 /** 1729 * The purpose for which the permission is given. 1730 */ 1731 @Child(name = "purpose", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1732 @Description(shortDefinition="The purpose for which the permission is given", formalDefinition="The purpose for which the permission is given." ) 1733 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 1734 protected List<CodeableConcept> purpose; 1735 1736 private static final long serialVersionUID = 1403721720L; 1737 1738 /** 1739 * Constructor 1740 */ 1741 public RuleActivityComponent() { 1742 super(); 1743 } 1744 1745 /** 1746 * @return {@link #actor} (The actor(s) authorized for the defined activity.) 1747 */ 1748 public List<Reference> getActor() { 1749 if (this.actor == null) 1750 this.actor = new ArrayList<Reference>(); 1751 return this.actor; 1752 } 1753 1754 /** 1755 * @return Returns a reference to <code>this</code> for easy method chaining 1756 */ 1757 public RuleActivityComponent setActor(List<Reference> theActor) { 1758 this.actor = theActor; 1759 return this; 1760 } 1761 1762 public boolean hasActor() { 1763 if (this.actor == null) 1764 return false; 1765 for (Reference item : this.actor) 1766 if (!item.isEmpty()) 1767 return true; 1768 return false; 1769 } 1770 1771 public Reference addActor() { //3 1772 Reference t = new Reference(); 1773 if (this.actor == null) 1774 this.actor = new ArrayList<Reference>(); 1775 this.actor.add(t); 1776 return t; 1777 } 1778 1779 public RuleActivityComponent addActor(Reference t) { //3 1780 if (t == null) 1781 return this; 1782 if (this.actor == null) 1783 this.actor = new ArrayList<Reference>(); 1784 this.actor.add(t); 1785 return this; 1786 } 1787 1788 /** 1789 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist {3} 1790 */ 1791 public Reference getActorFirstRep() { 1792 if (getActor().isEmpty()) { 1793 addActor(); 1794 } 1795 return getActor().get(0); 1796 } 1797 1798 /** 1799 * @return {@link #action} (Actions controlled by this Rule.) 1800 */ 1801 public List<CodeableConcept> getAction() { 1802 if (this.action == null) 1803 this.action = new ArrayList<CodeableConcept>(); 1804 return this.action; 1805 } 1806 1807 /** 1808 * @return Returns a reference to <code>this</code> for easy method chaining 1809 */ 1810 public RuleActivityComponent setAction(List<CodeableConcept> theAction) { 1811 this.action = theAction; 1812 return this; 1813 } 1814 1815 public boolean hasAction() { 1816 if (this.action == null) 1817 return false; 1818 for (CodeableConcept item : this.action) 1819 if (!item.isEmpty()) 1820 return true; 1821 return false; 1822 } 1823 1824 public CodeableConcept addAction() { //3 1825 CodeableConcept t = new CodeableConcept(); 1826 if (this.action == null) 1827 this.action = new ArrayList<CodeableConcept>(); 1828 this.action.add(t); 1829 return t; 1830 } 1831 1832 public RuleActivityComponent addAction(CodeableConcept t) { //3 1833 if (t == null) 1834 return this; 1835 if (this.action == null) 1836 this.action = new ArrayList<CodeableConcept>(); 1837 this.action.add(t); 1838 return this; 1839 } 1840 1841 /** 1842 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 1843 */ 1844 public CodeableConcept getActionFirstRep() { 1845 if (getAction().isEmpty()) { 1846 addAction(); 1847 } 1848 return getAction().get(0); 1849 } 1850 1851 /** 1852 * @return {@link #purpose} (The purpose for which the permission is given.) 1853 */ 1854 public List<CodeableConcept> getPurpose() { 1855 if (this.purpose == null) 1856 this.purpose = new ArrayList<CodeableConcept>(); 1857 return this.purpose; 1858 } 1859 1860 /** 1861 * @return Returns a reference to <code>this</code> for easy method chaining 1862 */ 1863 public RuleActivityComponent setPurpose(List<CodeableConcept> thePurpose) { 1864 this.purpose = thePurpose; 1865 return this; 1866 } 1867 1868 public boolean hasPurpose() { 1869 if (this.purpose == null) 1870 return false; 1871 for (CodeableConcept item : this.purpose) 1872 if (!item.isEmpty()) 1873 return true; 1874 return false; 1875 } 1876 1877 public CodeableConcept addPurpose() { //3 1878 CodeableConcept t = new CodeableConcept(); 1879 if (this.purpose == null) 1880 this.purpose = new ArrayList<CodeableConcept>(); 1881 this.purpose.add(t); 1882 return t; 1883 } 1884 1885 public RuleActivityComponent addPurpose(CodeableConcept t) { //3 1886 if (t == null) 1887 return this; 1888 if (this.purpose == null) 1889 this.purpose = new ArrayList<CodeableConcept>(); 1890 this.purpose.add(t); 1891 return this; 1892 } 1893 1894 /** 1895 * @return The first repetition of repeating field {@link #purpose}, creating it if it does not already exist {3} 1896 */ 1897 public CodeableConcept getPurposeFirstRep() { 1898 if (getPurpose().isEmpty()) { 1899 addPurpose(); 1900 } 1901 return getPurpose().get(0); 1902 } 1903 1904 protected void listChildren(List<Property> children) { 1905 super.listChildren(children); 1906 children.add(new Property("actor", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The actor(s) authorized for the defined activity.", 0, java.lang.Integer.MAX_VALUE, actor)); 1907 children.add(new Property("action", "CodeableConcept", "Actions controlled by this Rule.", 0, java.lang.Integer.MAX_VALUE, action)); 1908 children.add(new Property("purpose", "CodeableConcept", "The purpose for which the permission is given.", 0, java.lang.Integer.MAX_VALUE, purpose)); 1909 } 1910 1911 @Override 1912 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1913 switch (_hash) { 1914 case 92645877: /*actor*/ return new Property("actor", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The actor(s) authorized for the defined activity.", 0, java.lang.Integer.MAX_VALUE, actor); 1915 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Actions controlled by this Rule.", 0, java.lang.Integer.MAX_VALUE, action); 1916 case -220463842: /*purpose*/ return new Property("purpose", "CodeableConcept", "The purpose for which the permission is given.", 0, java.lang.Integer.MAX_VALUE, purpose); 1917 default: return super.getNamedProperty(_hash, _name, _checkValid); 1918 } 1919 1920 } 1921 1922 @Override 1923 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1924 switch (hash) { 1925 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // Reference 1926 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 1927 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // CodeableConcept 1928 default: return super.getProperty(hash, name, checkValid); 1929 } 1930 1931 } 1932 1933 @Override 1934 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1935 switch (hash) { 1936 case 92645877: // actor 1937 this.getActor().add(TypeConvertor.castToReference(value)); // Reference 1938 return value; 1939 case -1422950858: // action 1940 this.getAction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1941 return value; 1942 case -220463842: // purpose 1943 this.getPurpose().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1944 return value; 1945 default: return super.setProperty(hash, name, value); 1946 } 1947 1948 } 1949 1950 @Override 1951 public Base setProperty(String name, Base value) throws FHIRException { 1952 if (name.equals("actor")) { 1953 this.getActor().add(TypeConvertor.castToReference(value)); 1954 } else if (name.equals("action")) { 1955 this.getAction().add(TypeConvertor.castToCodeableConcept(value)); 1956 } else if (name.equals("purpose")) { 1957 this.getPurpose().add(TypeConvertor.castToCodeableConcept(value)); 1958 } else 1959 return super.setProperty(name, value); 1960 return value; 1961 } 1962 1963 @Override 1964 public void removeChild(String name, Base value) throws FHIRException { 1965 if (name.equals("actor")) { 1966 this.getActor().remove(value); 1967 } else if (name.equals("action")) { 1968 this.getAction().remove(value); 1969 } else if (name.equals("purpose")) { 1970 this.getPurpose().remove(value); 1971 } else 1972 super.removeChild(name, value); 1973 1974 } 1975 1976 @Override 1977 public Base makeProperty(int hash, String name) throws FHIRException { 1978 switch (hash) { 1979 case 92645877: return addActor(); 1980 case -1422950858: return addAction(); 1981 case -220463842: return addPurpose(); 1982 default: return super.makeProperty(hash, name); 1983 } 1984 1985 } 1986 1987 @Override 1988 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1989 switch (hash) { 1990 case 92645877: /*actor*/ return new String[] {"Reference"}; 1991 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 1992 case -220463842: /*purpose*/ return new String[] {"CodeableConcept"}; 1993 default: return super.getTypesForProperty(hash, name); 1994 } 1995 1996 } 1997 1998 @Override 1999 public Base addChild(String name) throws FHIRException { 2000 if (name.equals("actor")) { 2001 return addActor(); 2002 } 2003 else if (name.equals("action")) { 2004 return addAction(); 2005 } 2006 else if (name.equals("purpose")) { 2007 return addPurpose(); 2008 } 2009 else 2010 return super.addChild(name); 2011 } 2012 2013 public RuleActivityComponent copy() { 2014 RuleActivityComponent dst = new RuleActivityComponent(); 2015 copyValues(dst); 2016 return dst; 2017 } 2018 2019 public void copyValues(RuleActivityComponent dst) { 2020 super.copyValues(dst); 2021 if (actor != null) { 2022 dst.actor = new ArrayList<Reference>(); 2023 for (Reference i : actor) 2024 dst.actor.add(i.copy()); 2025 }; 2026 if (action != null) { 2027 dst.action = new ArrayList<CodeableConcept>(); 2028 for (CodeableConcept i : action) 2029 dst.action.add(i.copy()); 2030 }; 2031 if (purpose != null) { 2032 dst.purpose = new ArrayList<CodeableConcept>(); 2033 for (CodeableConcept i : purpose) 2034 dst.purpose.add(i.copy()); 2035 }; 2036 } 2037 2038 @Override 2039 public boolean equalsDeep(Base other_) { 2040 if (!super.equalsDeep(other_)) 2041 return false; 2042 if (!(other_ instanceof RuleActivityComponent)) 2043 return false; 2044 RuleActivityComponent o = (RuleActivityComponent) other_; 2045 return compareDeep(actor, o.actor, true) && compareDeep(action, o.action, true) && compareDeep(purpose, o.purpose, true) 2046 ; 2047 } 2048 2049 @Override 2050 public boolean equalsShallow(Base other_) { 2051 if (!super.equalsShallow(other_)) 2052 return false; 2053 if (!(other_ instanceof RuleActivityComponent)) 2054 return false; 2055 RuleActivityComponent o = (RuleActivityComponent) other_; 2056 return true; 2057 } 2058 2059 public boolean isEmpty() { 2060 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actor, action, purpose); 2061 } 2062 2063 public String fhirType() { 2064 return "Permission.rule.activity"; 2065 2066 } 2067 2068 } 2069 2070 /** 2071 * Status. 2072 */ 2073 @Child(name = "status", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 2074 @Description(shortDefinition="active | entered-in-error | draft | rejected", formalDefinition="Status." ) 2075 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/permission-status") 2076 protected Enumeration<PermissionStatus> status; 2077 2078 /** 2079 * The person or entity that asserts the permission. 2080 */ 2081 @Child(name = "asserter", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, RelatedPerson.class, HealthcareService.class}, order=1, min=0, max=1, modifier=false, summary=true) 2082 @Description(shortDefinition="The person or entity that asserts the permission", formalDefinition="The person or entity that asserts the permission." ) 2083 protected Reference asserter; 2084 2085 /** 2086 * The date that permission was asserted. 2087 */ 2088 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2089 @Description(shortDefinition="The date that permission was asserted", formalDefinition="The date that permission was asserted." ) 2090 protected List<DateTimeType> date; 2091 2092 /** 2093 * The period in which the permission is active. 2094 */ 2095 @Child(name = "validity", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 2096 @Description(shortDefinition="The period in which the permission is active", formalDefinition="The period in which the permission is active." ) 2097 protected Period validity; 2098 2099 /** 2100 * The asserted justification for using the data. 2101 */ 2102 @Child(name = "justification", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 2103 @Description(shortDefinition="The asserted justification for using the data", formalDefinition="The asserted justification for using the data." ) 2104 protected PermissionJustificationComponent justification; 2105 2106 /** 2107 * Defines a procedure for arriving at an access decision given the set of rules. 2108 */ 2109 @Child(name = "combining", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 2110 @Description(shortDefinition="deny-overrides | permit-overrides | ordered-deny-overrides | ordered-permit-overrides | deny-unless-permit | permit-unless-deny", formalDefinition="Defines a procedure for arriving at an access decision given the set of rules." ) 2111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/permission-rule-combining") 2112 protected Enumeration<PermissionRuleCombining> combining; 2113 2114 /** 2115 * A set of rules. 2116 */ 2117 @Child(name = "rule", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2118 @Description(shortDefinition="Constraints to the Permission", formalDefinition="A set of rules." ) 2119 protected List<RuleComponent> rule; 2120 2121 private static final long serialVersionUID = 1252321973L; 2122 2123 /** 2124 * Constructor 2125 */ 2126 public Permission() { 2127 super(); 2128 } 2129 2130 /** 2131 * Constructor 2132 */ 2133 public Permission(PermissionStatus status, PermissionRuleCombining combining) { 2134 super(); 2135 this.setStatus(status); 2136 this.setCombining(combining); 2137 } 2138 2139 /** 2140 * @return {@link #status} (Status.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2141 */ 2142 public Enumeration<PermissionStatus> getStatusElement() { 2143 if (this.status == null) 2144 if (Configuration.errorOnAutoCreate()) 2145 throw new Error("Attempt to auto-create Permission.status"); 2146 else if (Configuration.doAutoCreate()) 2147 this.status = new Enumeration<PermissionStatus>(new PermissionStatusEnumFactory()); // bb 2148 return this.status; 2149 } 2150 2151 public boolean hasStatusElement() { 2152 return this.status != null && !this.status.isEmpty(); 2153 } 2154 2155 public boolean hasStatus() { 2156 return this.status != null && !this.status.isEmpty(); 2157 } 2158 2159 /** 2160 * @param value {@link #status} (Status.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2161 */ 2162 public Permission setStatusElement(Enumeration<PermissionStatus> value) { 2163 this.status = value; 2164 return this; 2165 } 2166 2167 /** 2168 * @return Status. 2169 */ 2170 public PermissionStatus getStatus() { 2171 return this.status == null ? null : this.status.getValue(); 2172 } 2173 2174 /** 2175 * @param value Status. 2176 */ 2177 public Permission setStatus(PermissionStatus value) { 2178 if (this.status == null) 2179 this.status = new Enumeration<PermissionStatus>(new PermissionStatusEnumFactory()); 2180 this.status.setValue(value); 2181 return this; 2182 } 2183 2184 /** 2185 * @return {@link #asserter} (The person or entity that asserts the permission.) 2186 */ 2187 public Reference getAsserter() { 2188 if (this.asserter == null) 2189 if (Configuration.errorOnAutoCreate()) 2190 throw new Error("Attempt to auto-create Permission.asserter"); 2191 else if (Configuration.doAutoCreate()) 2192 this.asserter = new Reference(); // cc 2193 return this.asserter; 2194 } 2195 2196 public boolean hasAsserter() { 2197 return this.asserter != null && !this.asserter.isEmpty(); 2198 } 2199 2200 /** 2201 * @param value {@link #asserter} (The person or entity that asserts the permission.) 2202 */ 2203 public Permission setAsserter(Reference value) { 2204 this.asserter = value; 2205 return this; 2206 } 2207 2208 /** 2209 * @return {@link #date} (The date that permission was asserted.) 2210 */ 2211 public List<DateTimeType> getDate() { 2212 if (this.date == null) 2213 this.date = new ArrayList<DateTimeType>(); 2214 return this.date; 2215 } 2216 2217 /** 2218 * @return Returns a reference to <code>this</code> for easy method chaining 2219 */ 2220 public Permission setDate(List<DateTimeType> theDate) { 2221 this.date = theDate; 2222 return this; 2223 } 2224 2225 public boolean hasDate() { 2226 if (this.date == null) 2227 return false; 2228 for (DateTimeType item : this.date) 2229 if (!item.isEmpty()) 2230 return true; 2231 return false; 2232 } 2233 2234 /** 2235 * @return {@link #date} (The date that permission was asserted.) 2236 */ 2237 public DateTimeType addDateElement() {//2 2238 DateTimeType t = new DateTimeType(); 2239 if (this.date == null) 2240 this.date = new ArrayList<DateTimeType>(); 2241 this.date.add(t); 2242 return t; 2243 } 2244 2245 /** 2246 * @param value {@link #date} (The date that permission was asserted.) 2247 */ 2248 public Permission addDate(Date value) { //1 2249 DateTimeType t = new DateTimeType(); 2250 t.setValue(value); 2251 if (this.date == null) 2252 this.date = new ArrayList<DateTimeType>(); 2253 this.date.add(t); 2254 return this; 2255 } 2256 2257 /** 2258 * @param value {@link #date} (The date that permission was asserted.) 2259 */ 2260 public boolean hasDate(Date value) { 2261 if (this.date == null) 2262 return false; 2263 for (DateTimeType v : this.date) 2264 if (v.getValue().equals(value)) // dateTime 2265 return true; 2266 return false; 2267 } 2268 2269 /** 2270 * @return {@link #validity} (The period in which the permission is active.) 2271 */ 2272 public Period getValidity() { 2273 if (this.validity == null) 2274 if (Configuration.errorOnAutoCreate()) 2275 throw new Error("Attempt to auto-create Permission.validity"); 2276 else if (Configuration.doAutoCreate()) 2277 this.validity = new Period(); // cc 2278 return this.validity; 2279 } 2280 2281 public boolean hasValidity() { 2282 return this.validity != null && !this.validity.isEmpty(); 2283 } 2284 2285 /** 2286 * @param value {@link #validity} (The period in which the permission is active.) 2287 */ 2288 public Permission setValidity(Period value) { 2289 this.validity = value; 2290 return this; 2291 } 2292 2293 /** 2294 * @return {@link #justification} (The asserted justification for using the data.) 2295 */ 2296 public PermissionJustificationComponent getJustification() { 2297 if (this.justification == null) 2298 if (Configuration.errorOnAutoCreate()) 2299 throw new Error("Attempt to auto-create Permission.justification"); 2300 else if (Configuration.doAutoCreate()) 2301 this.justification = new PermissionJustificationComponent(); // cc 2302 return this.justification; 2303 } 2304 2305 public boolean hasJustification() { 2306 return this.justification != null && !this.justification.isEmpty(); 2307 } 2308 2309 /** 2310 * @param value {@link #justification} (The asserted justification for using the data.) 2311 */ 2312 public Permission setJustification(PermissionJustificationComponent value) { 2313 this.justification = value; 2314 return this; 2315 } 2316 2317 /** 2318 * @return {@link #combining} (Defines a procedure for arriving at an access decision given the set of rules.). This is the underlying object with id, value and extensions. The accessor "getCombining" gives direct access to the value 2319 */ 2320 public Enumeration<PermissionRuleCombining> getCombiningElement() { 2321 if (this.combining == null) 2322 if (Configuration.errorOnAutoCreate()) 2323 throw new Error("Attempt to auto-create Permission.combining"); 2324 else if (Configuration.doAutoCreate()) 2325 this.combining = new Enumeration<PermissionRuleCombining>(new PermissionRuleCombiningEnumFactory()); // bb 2326 return this.combining; 2327 } 2328 2329 public boolean hasCombiningElement() { 2330 return this.combining != null && !this.combining.isEmpty(); 2331 } 2332 2333 public boolean hasCombining() { 2334 return this.combining != null && !this.combining.isEmpty(); 2335 } 2336 2337 /** 2338 * @param value {@link #combining} (Defines a procedure for arriving at an access decision given the set of rules.). This is the underlying object with id, value and extensions. The accessor "getCombining" gives direct access to the value 2339 */ 2340 public Permission setCombiningElement(Enumeration<PermissionRuleCombining> value) { 2341 this.combining = value; 2342 return this; 2343 } 2344 2345 /** 2346 * @return Defines a procedure for arriving at an access decision given the set of rules. 2347 */ 2348 public PermissionRuleCombining getCombining() { 2349 return this.combining == null ? null : this.combining.getValue(); 2350 } 2351 2352 /** 2353 * @param value Defines a procedure for arriving at an access decision given the set of rules. 2354 */ 2355 public Permission setCombining(PermissionRuleCombining value) { 2356 if (this.combining == null) 2357 this.combining = new Enumeration<PermissionRuleCombining>(new PermissionRuleCombiningEnumFactory()); 2358 this.combining.setValue(value); 2359 return this; 2360 } 2361 2362 /** 2363 * @return {@link #rule} (A set of rules.) 2364 */ 2365 public List<RuleComponent> getRule() { 2366 if (this.rule == null) 2367 this.rule = new ArrayList<RuleComponent>(); 2368 return this.rule; 2369 } 2370 2371 /** 2372 * @return Returns a reference to <code>this</code> for easy method chaining 2373 */ 2374 public Permission setRule(List<RuleComponent> theRule) { 2375 this.rule = theRule; 2376 return this; 2377 } 2378 2379 public boolean hasRule() { 2380 if (this.rule == null) 2381 return false; 2382 for (RuleComponent item : this.rule) 2383 if (!item.isEmpty()) 2384 return true; 2385 return false; 2386 } 2387 2388 public RuleComponent addRule() { //3 2389 RuleComponent t = new RuleComponent(); 2390 if (this.rule == null) 2391 this.rule = new ArrayList<RuleComponent>(); 2392 this.rule.add(t); 2393 return t; 2394 } 2395 2396 public Permission addRule(RuleComponent t) { //3 2397 if (t == null) 2398 return this; 2399 if (this.rule == null) 2400 this.rule = new ArrayList<RuleComponent>(); 2401 this.rule.add(t); 2402 return this; 2403 } 2404 2405 /** 2406 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist {3} 2407 */ 2408 public RuleComponent getRuleFirstRep() { 2409 if (getRule().isEmpty()) { 2410 addRule(); 2411 } 2412 return getRule().get(0); 2413 } 2414 2415 protected void listChildren(List<Property> children) { 2416 super.listChildren(children); 2417 children.add(new Property("status", "code", "Status.", 0, 1, status)); 2418 children.add(new Property("asserter", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson|HealthcareService)", "The person or entity that asserts the permission.", 0, 1, asserter)); 2419 children.add(new Property("date", "dateTime", "The date that permission was asserted.", 0, java.lang.Integer.MAX_VALUE, date)); 2420 children.add(new Property("validity", "Period", "The period in which the permission is active.", 0, 1, validity)); 2421 children.add(new Property("justification", "", "The asserted justification for using the data.", 0, 1, justification)); 2422 children.add(new Property("combining", "code", "Defines a procedure for arriving at an access decision given the set of rules.", 0, 1, combining)); 2423 children.add(new Property("rule", "", "A set of rules.", 0, java.lang.Integer.MAX_VALUE, rule)); 2424 } 2425 2426 @Override 2427 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2428 switch (_hash) { 2429 case -892481550: /*status*/ return new Property("status", "code", "Status.", 0, 1, status); 2430 case -373242253: /*asserter*/ return new Property("asserter", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson|HealthcareService)", "The person or entity that asserts the permission.", 0, 1, asserter); 2431 case 3076014: /*date*/ return new Property("date", "dateTime", "The date that permission was asserted.", 0, java.lang.Integer.MAX_VALUE, date); 2432 case -1421265102: /*validity*/ return new Property("validity", "Period", "The period in which the permission is active.", 0, 1, validity); 2433 case 1864993522: /*justification*/ return new Property("justification", "", "The asserted justification for using the data.", 0, 1, justification); 2434 case -1806252484: /*combining*/ return new Property("combining", "code", "Defines a procedure for arriving at an access decision given the set of rules.", 0, 1, combining); 2435 case 3512060: /*rule*/ return new Property("rule", "", "A set of rules.", 0, java.lang.Integer.MAX_VALUE, rule); 2436 default: return super.getNamedProperty(_hash, _name, _checkValid); 2437 } 2438 2439 } 2440 2441 @Override 2442 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2443 switch (hash) { 2444 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PermissionStatus> 2445 case -373242253: /*asserter*/ return this.asserter == null ? new Base[0] : new Base[] {this.asserter}; // Reference 2446 case 3076014: /*date*/ return this.date == null ? new Base[0] : this.date.toArray(new Base[this.date.size()]); // DateTimeType 2447 case -1421265102: /*validity*/ return this.validity == null ? new Base[0] : new Base[] {this.validity}; // Period 2448 case 1864993522: /*justification*/ return this.justification == null ? new Base[0] : new Base[] {this.justification}; // PermissionJustificationComponent 2449 case -1806252484: /*combining*/ return this.combining == null ? new Base[0] : new Base[] {this.combining}; // Enumeration<PermissionRuleCombining> 2450 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // RuleComponent 2451 default: return super.getProperty(hash, name, checkValid); 2452 } 2453 2454 } 2455 2456 @Override 2457 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2458 switch (hash) { 2459 case -892481550: // status 2460 value = new PermissionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2461 this.status = (Enumeration) value; // Enumeration<PermissionStatus> 2462 return value; 2463 case -373242253: // asserter 2464 this.asserter = TypeConvertor.castToReference(value); // Reference 2465 return value; 2466 case 3076014: // date 2467 this.getDate().add(TypeConvertor.castToDateTime(value)); // DateTimeType 2468 return value; 2469 case -1421265102: // validity 2470 this.validity = TypeConvertor.castToPeriod(value); // Period 2471 return value; 2472 case 1864993522: // justification 2473 this.justification = (PermissionJustificationComponent) value; // PermissionJustificationComponent 2474 return value; 2475 case -1806252484: // combining 2476 value = new PermissionRuleCombiningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2477 this.combining = (Enumeration) value; // Enumeration<PermissionRuleCombining> 2478 return value; 2479 case 3512060: // rule 2480 this.getRule().add((RuleComponent) value); // RuleComponent 2481 return value; 2482 default: return super.setProperty(hash, name, value); 2483 } 2484 2485 } 2486 2487 @Override 2488 public Base setProperty(String name, Base value) throws FHIRException { 2489 if (name.equals("status")) { 2490 value = new PermissionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2491 this.status = (Enumeration) value; // Enumeration<PermissionStatus> 2492 } else if (name.equals("asserter")) { 2493 this.asserter = TypeConvertor.castToReference(value); // Reference 2494 } else if (name.equals("date")) { 2495 this.getDate().add(TypeConvertor.castToDateTime(value)); 2496 } else if (name.equals("validity")) { 2497 this.validity = TypeConvertor.castToPeriod(value); // Period 2498 } else if (name.equals("justification")) { 2499 this.justification = (PermissionJustificationComponent) value; // PermissionJustificationComponent 2500 } else if (name.equals("combining")) { 2501 value = new PermissionRuleCombiningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2502 this.combining = (Enumeration) value; // Enumeration<PermissionRuleCombining> 2503 } else if (name.equals("rule")) { 2504 this.getRule().add((RuleComponent) value); 2505 } else 2506 return super.setProperty(name, value); 2507 return value; 2508 } 2509 2510 @Override 2511 public void removeChild(String name, Base value) throws FHIRException { 2512 if (name.equals("status")) { 2513 value = new PermissionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2514 this.status = (Enumeration) value; // Enumeration<PermissionStatus> 2515 } else if (name.equals("asserter")) { 2516 this.asserter = null; 2517 } else if (name.equals("date")) { 2518 this.getDate().remove(value); 2519 } else if (name.equals("validity")) { 2520 this.validity = null; 2521 } else if (name.equals("justification")) { 2522 this.justification = (PermissionJustificationComponent) value; // PermissionJustificationComponent 2523 } else if (name.equals("combining")) { 2524 value = new PermissionRuleCombiningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2525 this.combining = (Enumeration) value; // Enumeration<PermissionRuleCombining> 2526 } else if (name.equals("rule")) { 2527 this.getRule().remove((RuleComponent) value); 2528 } else 2529 super.removeChild(name, value); 2530 2531 } 2532 2533 @Override 2534 public Base makeProperty(int hash, String name) throws FHIRException { 2535 switch (hash) { 2536 case -892481550: return getStatusElement(); 2537 case -373242253: return getAsserter(); 2538 case 3076014: return addDateElement(); 2539 case -1421265102: return getValidity(); 2540 case 1864993522: return getJustification(); 2541 case -1806252484: return getCombiningElement(); 2542 case 3512060: return addRule(); 2543 default: return super.makeProperty(hash, name); 2544 } 2545 2546 } 2547 2548 @Override 2549 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2550 switch (hash) { 2551 case -892481550: /*status*/ return new String[] {"code"}; 2552 case -373242253: /*asserter*/ return new String[] {"Reference"}; 2553 case 3076014: /*date*/ return new String[] {"dateTime"}; 2554 case -1421265102: /*validity*/ return new String[] {"Period"}; 2555 case 1864993522: /*justification*/ return new String[] {}; 2556 case -1806252484: /*combining*/ return new String[] {"code"}; 2557 case 3512060: /*rule*/ return new String[] {}; 2558 default: return super.getTypesForProperty(hash, name); 2559 } 2560 2561 } 2562 2563 @Override 2564 public Base addChild(String name) throws FHIRException { 2565 if (name.equals("status")) { 2566 throw new FHIRException("Cannot call addChild on a singleton property Permission.status"); 2567 } 2568 else if (name.equals("asserter")) { 2569 this.asserter = new Reference(); 2570 return this.asserter; 2571 } 2572 else if (name.equals("date")) { 2573 throw new FHIRException("Cannot call addChild on a singleton property Permission.date"); 2574 } 2575 else if (name.equals("validity")) { 2576 this.validity = new Period(); 2577 return this.validity; 2578 } 2579 else if (name.equals("justification")) { 2580 this.justification = new PermissionJustificationComponent(); 2581 return this.justification; 2582 } 2583 else if (name.equals("combining")) { 2584 throw new FHIRException("Cannot call addChild on a singleton property Permission.combining"); 2585 } 2586 else if (name.equals("rule")) { 2587 return addRule(); 2588 } 2589 else 2590 return super.addChild(name); 2591 } 2592 2593 public String fhirType() { 2594 return "Permission"; 2595 2596 } 2597 2598 public Permission copy() { 2599 Permission dst = new Permission(); 2600 copyValues(dst); 2601 return dst; 2602 } 2603 2604 public void copyValues(Permission dst) { 2605 super.copyValues(dst); 2606 dst.status = status == null ? null : status.copy(); 2607 dst.asserter = asserter == null ? null : asserter.copy(); 2608 if (date != null) { 2609 dst.date = new ArrayList<DateTimeType>(); 2610 for (DateTimeType i : date) 2611 dst.date.add(i.copy()); 2612 }; 2613 dst.validity = validity == null ? null : validity.copy(); 2614 dst.justification = justification == null ? null : justification.copy(); 2615 dst.combining = combining == null ? null : combining.copy(); 2616 if (rule != null) { 2617 dst.rule = new ArrayList<RuleComponent>(); 2618 for (RuleComponent i : rule) 2619 dst.rule.add(i.copy()); 2620 }; 2621 } 2622 2623 protected Permission typedCopy() { 2624 return copy(); 2625 } 2626 2627 @Override 2628 public boolean equalsDeep(Base other_) { 2629 if (!super.equalsDeep(other_)) 2630 return false; 2631 if (!(other_ instanceof Permission)) 2632 return false; 2633 Permission o = (Permission) other_; 2634 return compareDeep(status, o.status, true) && compareDeep(asserter, o.asserter, true) && compareDeep(date, o.date, true) 2635 && compareDeep(validity, o.validity, true) && compareDeep(justification, o.justification, true) 2636 && compareDeep(combining, o.combining, true) && compareDeep(rule, o.rule, true); 2637 } 2638 2639 @Override 2640 public boolean equalsShallow(Base other_) { 2641 if (!super.equalsShallow(other_)) 2642 return false; 2643 if (!(other_ instanceof Permission)) 2644 return false; 2645 Permission o = (Permission) other_; 2646 return compareValues(status, o.status, true) && compareValues(date, o.date, true) && compareValues(combining, o.combining, true) 2647 ; 2648 } 2649 2650 public boolean isEmpty() { 2651 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, asserter, date, validity 2652 , justification, combining, rule); 2653 } 2654 2655 @Override 2656 public ResourceType getResourceType() { 2657 return ResourceType.Permission; 2658 } 2659 2660 /** 2661 * Search parameter: <b>status</b> 2662 * <p> 2663 * Description: <b>active | entered-in-error | draft | rejected</b><br> 2664 * Type: <b>token</b><br> 2665 * Path: <b>Permission.status</b><br> 2666 * </p> 2667 */ 2668 @SearchParamDefinition(name="status", path="Permission.status", description="active | entered-in-error | draft | rejected", type="token" ) 2669 public static final String SP_STATUS = "status"; 2670 /** 2671 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2672 * <p> 2673 * Description: <b>active | entered-in-error | draft | rejected</b><br> 2674 * Type: <b>token</b><br> 2675 * Path: <b>Permission.status</b><br> 2676 * </p> 2677 */ 2678 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2679 2680 2681} 2682