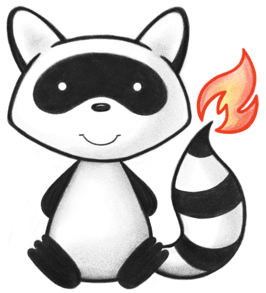
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Demographics and administrative information about a person independent of a specific health-related context. 052 */ 053@ResourceDef(name="Person", profile="http://hl7.org/fhir/StructureDefinition/Person") 054public class Person extends DomainResource { 055 056 public enum IdentityAssuranceLevel { 057 /** 058 * Little or no confidence in the asserted identity's accuracy. 059 */ 060 LEVEL1, 061 /** 062 * Some confidence in the asserted identity's accuracy. 063 */ 064 LEVEL2, 065 /** 066 * High confidence in the asserted identity's accuracy. 067 */ 068 LEVEL3, 069 /** 070 * Very high confidence in the asserted identity's accuracy. 071 */ 072 LEVEL4, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static IdentityAssuranceLevel fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("level1".equals(codeString)) 081 return LEVEL1; 082 if ("level2".equals(codeString)) 083 return LEVEL2; 084 if ("level3".equals(codeString)) 085 return LEVEL3; 086 if ("level4".equals(codeString)) 087 return LEVEL4; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown IdentityAssuranceLevel code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case LEVEL1: return "level1"; 096 case LEVEL2: return "level2"; 097 case LEVEL3: return "level3"; 098 case LEVEL4: return "level4"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case LEVEL1: return "http://hl7.org/fhir/identity-assuranceLevel"; 106 case LEVEL2: return "http://hl7.org/fhir/identity-assuranceLevel"; 107 case LEVEL3: return "http://hl7.org/fhir/identity-assuranceLevel"; 108 case LEVEL4: return "http://hl7.org/fhir/identity-assuranceLevel"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case LEVEL1: return "Little or no confidence in the asserted identity's accuracy."; 116 case LEVEL2: return "Some confidence in the asserted identity's accuracy."; 117 case LEVEL3: return "High confidence in the asserted identity's accuracy."; 118 case LEVEL4: return "Very high confidence in the asserted identity's accuracy."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case LEVEL1: return "Level 1"; 126 case LEVEL2: return "Level 2"; 127 case LEVEL3: return "Level 3"; 128 case LEVEL4: return "Level 4"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class IdentityAssuranceLevelEnumFactory implements EnumFactory<IdentityAssuranceLevel> { 136 public IdentityAssuranceLevel fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("level1".equals(codeString)) 141 return IdentityAssuranceLevel.LEVEL1; 142 if ("level2".equals(codeString)) 143 return IdentityAssuranceLevel.LEVEL2; 144 if ("level3".equals(codeString)) 145 return IdentityAssuranceLevel.LEVEL3; 146 if ("level4".equals(codeString)) 147 return IdentityAssuranceLevel.LEVEL4; 148 throw new IllegalArgumentException("Unknown IdentityAssuranceLevel code '"+codeString+"'"); 149 } 150 public Enumeration<IdentityAssuranceLevel> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.NULL, code); 158 if ("level1".equals(codeString)) 159 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL1, code); 160 if ("level2".equals(codeString)) 161 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL2, code); 162 if ("level3".equals(codeString)) 163 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL3, code); 164 if ("level4".equals(codeString)) 165 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL4, code); 166 throw new FHIRException("Unknown IdentityAssuranceLevel code '"+codeString+"'"); 167 } 168 public String toCode(IdentityAssuranceLevel code) { 169 if (code == IdentityAssuranceLevel.NULL) 170 return null; 171 if (code == IdentityAssuranceLevel.LEVEL1) 172 return "level1"; 173 if (code == IdentityAssuranceLevel.LEVEL2) 174 return "level2"; 175 if (code == IdentityAssuranceLevel.LEVEL3) 176 return "level3"; 177 if (code == IdentityAssuranceLevel.LEVEL4) 178 return "level4"; 179 return "?"; 180 } 181 public String toSystem(IdentityAssuranceLevel code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class PersonCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English. 190 */ 191 @Child(name = "language", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 192 @Description(shortDefinition="The language which can be used to communicate with the person about his or her health", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 194 protected CodeableConcept language; 195 196 /** 197 * Indicates whether or not the person prefers this language (over other languages he masters up a certain level). 198 */ 199 @Child(name = "preferred", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="Language preference indicator", formalDefinition="Indicates whether or not the person prefers this language (over other languages he masters up a certain level)." ) 201 protected BooleanType preferred; 202 203 private static final long serialVersionUID = 633792918L; 204 205 /** 206 * Constructor 207 */ 208 public PersonCommunicationComponent() { 209 super(); 210 } 211 212 /** 213 * Constructor 214 */ 215 public PersonCommunicationComponent(CodeableConcept language) { 216 super(); 217 this.setLanguage(language); 218 } 219 220 /** 221 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 222 */ 223 public CodeableConcept getLanguage() { 224 if (this.language == null) 225 if (Configuration.errorOnAutoCreate()) 226 throw new Error("Attempt to auto-create PersonCommunicationComponent.language"); 227 else if (Configuration.doAutoCreate()) 228 this.language = new CodeableConcept(); // cc 229 return this.language; 230 } 231 232 public boolean hasLanguage() { 233 return this.language != null && !this.language.isEmpty(); 234 } 235 236 /** 237 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 238 */ 239 public PersonCommunicationComponent setLanguage(CodeableConcept value) { 240 this.language = value; 241 return this; 242 } 243 244 /** 245 * @return {@link #preferred} (Indicates whether or not the person prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 246 */ 247 public BooleanType getPreferredElement() { 248 if (this.preferred == null) 249 if (Configuration.errorOnAutoCreate()) 250 throw new Error("Attempt to auto-create PersonCommunicationComponent.preferred"); 251 else if (Configuration.doAutoCreate()) 252 this.preferred = new BooleanType(); // bb 253 return this.preferred; 254 } 255 256 public boolean hasPreferredElement() { 257 return this.preferred != null && !this.preferred.isEmpty(); 258 } 259 260 public boolean hasPreferred() { 261 return this.preferred != null && !this.preferred.isEmpty(); 262 } 263 264 /** 265 * @param value {@link #preferred} (Indicates whether or not the person prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 266 */ 267 public PersonCommunicationComponent setPreferredElement(BooleanType value) { 268 this.preferred = value; 269 return this; 270 } 271 272 /** 273 * @return Indicates whether or not the person prefers this language (over other languages he masters up a certain level). 274 */ 275 public boolean getPreferred() { 276 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 277 } 278 279 /** 280 * @param value Indicates whether or not the person prefers this language (over other languages he masters up a certain level). 281 */ 282 public PersonCommunicationComponent setPreferred(boolean value) { 283 if (this.preferred == null) 284 this.preferred = new BooleanType(); 285 this.preferred.setValue(value); 286 return this; 287 } 288 289 protected void listChildren(List<Property> children) { 290 super.listChildren(children); 291 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language)); 292 children.add(new Property("preferred", "boolean", "Indicates whether or not the person prefers this language (over other languages he masters up a certain level).", 0, 1, preferred)); 293 } 294 295 @Override 296 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 297 switch (_hash) { 298 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language); 299 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether or not the person prefers this language (over other languages he masters up a certain level).", 0, 1, preferred); 300 default: return super.getNamedProperty(_hash, _name, _checkValid); 301 } 302 303 } 304 305 @Override 306 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 307 switch (hash) { 308 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 309 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 310 default: return super.getProperty(hash, name, checkValid); 311 } 312 313 } 314 315 @Override 316 public Base setProperty(int hash, String name, Base value) throws FHIRException { 317 switch (hash) { 318 case -1613589672: // language 319 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 320 return value; 321 case -1294005119: // preferred 322 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 323 return value; 324 default: return super.setProperty(hash, name, value); 325 } 326 327 } 328 329 @Override 330 public Base setProperty(String name, Base value) throws FHIRException { 331 if (name.equals("language")) { 332 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 333 } else if (name.equals("preferred")) { 334 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 335 } else 336 return super.setProperty(name, value); 337 return value; 338 } 339 340 @Override 341 public void removeChild(String name, Base value) throws FHIRException { 342 if (name.equals("language")) { 343 this.language = null; 344 } else if (name.equals("preferred")) { 345 this.preferred = null; 346 } else 347 super.removeChild(name, value); 348 349 } 350 351 @Override 352 public Base makeProperty(int hash, String name) throws FHIRException { 353 switch (hash) { 354 case -1613589672: return getLanguage(); 355 case -1294005119: return getPreferredElement(); 356 default: return super.makeProperty(hash, name); 357 } 358 359 } 360 361 @Override 362 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 363 switch (hash) { 364 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 365 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 366 default: return super.getTypesForProperty(hash, name); 367 } 368 369 } 370 371 @Override 372 public Base addChild(String name) throws FHIRException { 373 if (name.equals("language")) { 374 this.language = new CodeableConcept(); 375 return this.language; 376 } 377 else if (name.equals("preferred")) { 378 throw new FHIRException("Cannot call addChild on a singleton property Person.communication.preferred"); 379 } 380 else 381 return super.addChild(name); 382 } 383 384 public PersonCommunicationComponent copy() { 385 PersonCommunicationComponent dst = new PersonCommunicationComponent(); 386 copyValues(dst); 387 return dst; 388 } 389 390 public void copyValues(PersonCommunicationComponent dst) { 391 super.copyValues(dst); 392 dst.language = language == null ? null : language.copy(); 393 dst.preferred = preferred == null ? null : preferred.copy(); 394 } 395 396 @Override 397 public boolean equalsDeep(Base other_) { 398 if (!super.equalsDeep(other_)) 399 return false; 400 if (!(other_ instanceof PersonCommunicationComponent)) 401 return false; 402 PersonCommunicationComponent o = (PersonCommunicationComponent) other_; 403 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 404 } 405 406 @Override 407 public boolean equalsShallow(Base other_) { 408 if (!super.equalsShallow(other_)) 409 return false; 410 if (!(other_ instanceof PersonCommunicationComponent)) 411 return false; 412 PersonCommunicationComponent o = (PersonCommunicationComponent) other_; 413 return compareValues(preferred, o.preferred, true); 414 } 415 416 public boolean isEmpty() { 417 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 418 } 419 420 public String fhirType() { 421 return "Person.communication"; 422 423 } 424 425 } 426 427 @Block() 428 public static class PersonLinkComponent extends BackboneElement implements IBaseBackboneElement { 429 /** 430 * The resource to which this actual person is associated. 431 */ 432 @Child(name = "target", type = {Patient.class, Practitioner.class, RelatedPerson.class, Person.class}, order=1, min=1, max=1, modifier=false, summary=false) 433 @Description(shortDefinition="The resource to which this actual person is associated", formalDefinition="The resource to which this actual person is associated." ) 434 protected Reference target; 435 436 /** 437 * Level of assurance that this link is associated with the target resource. 438 */ 439 @Child(name = "assurance", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 440 @Description(shortDefinition="level1 | level2 | level3 | level4", formalDefinition="Level of assurance that this link is associated with the target resource." ) 441 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/identity-assuranceLevel") 442 protected Enumeration<IdentityAssuranceLevel> assurance; 443 444 private static final long serialVersionUID = -1393523223L; 445 446 /** 447 * Constructor 448 */ 449 public PersonLinkComponent() { 450 super(); 451 } 452 453 /** 454 * Constructor 455 */ 456 public PersonLinkComponent(Reference target) { 457 super(); 458 this.setTarget(target); 459 } 460 461 /** 462 * @return {@link #target} (The resource to which this actual person is associated.) 463 */ 464 public Reference getTarget() { 465 if (this.target == null) 466 if (Configuration.errorOnAutoCreate()) 467 throw new Error("Attempt to auto-create PersonLinkComponent.target"); 468 else if (Configuration.doAutoCreate()) 469 this.target = new Reference(); // cc 470 return this.target; 471 } 472 473 public boolean hasTarget() { 474 return this.target != null && !this.target.isEmpty(); 475 } 476 477 /** 478 * @param value {@link #target} (The resource to which this actual person is associated.) 479 */ 480 public PersonLinkComponent setTarget(Reference value) { 481 this.target = value; 482 return this; 483 } 484 485 /** 486 * @return {@link #assurance} (Level of assurance that this link is associated with the target resource.). This is the underlying object with id, value and extensions. The accessor "getAssurance" gives direct access to the value 487 */ 488 public Enumeration<IdentityAssuranceLevel> getAssuranceElement() { 489 if (this.assurance == null) 490 if (Configuration.errorOnAutoCreate()) 491 throw new Error("Attempt to auto-create PersonLinkComponent.assurance"); 492 else if (Configuration.doAutoCreate()) 493 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); // bb 494 return this.assurance; 495 } 496 497 public boolean hasAssuranceElement() { 498 return this.assurance != null && !this.assurance.isEmpty(); 499 } 500 501 public boolean hasAssurance() { 502 return this.assurance != null && !this.assurance.isEmpty(); 503 } 504 505 /** 506 * @param value {@link #assurance} (Level of assurance that this link is associated with the target resource.). This is the underlying object with id, value and extensions. The accessor "getAssurance" gives direct access to the value 507 */ 508 public PersonLinkComponent setAssuranceElement(Enumeration<IdentityAssuranceLevel> value) { 509 this.assurance = value; 510 return this; 511 } 512 513 /** 514 * @return Level of assurance that this link is associated with the target resource. 515 */ 516 public IdentityAssuranceLevel getAssurance() { 517 return this.assurance == null ? null : this.assurance.getValue(); 518 } 519 520 /** 521 * @param value Level of assurance that this link is associated with the target resource. 522 */ 523 public PersonLinkComponent setAssurance(IdentityAssuranceLevel value) { 524 if (value == null) 525 this.assurance = null; 526 else { 527 if (this.assurance == null) 528 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); 529 this.assurance.setValue(value); 530 } 531 return this; 532 } 533 534 protected void listChildren(List<Property> children) { 535 super.listChildren(children); 536 children.add(new Property("target", "Reference(Patient|Practitioner|RelatedPerson|Person)", "The resource to which this actual person is associated.", 0, 1, target)); 537 children.add(new Property("assurance", "code", "Level of assurance that this link is associated with the target resource.", 0, 1, assurance)); 538 } 539 540 @Override 541 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 542 switch (_hash) { 543 case -880905839: /*target*/ return new Property("target", "Reference(Patient|Practitioner|RelatedPerson|Person)", "The resource to which this actual person is associated.", 0, 1, target); 544 case 1771900717: /*assurance*/ return new Property("assurance", "code", "Level of assurance that this link is associated with the target resource.", 0, 1, assurance); 545 default: return super.getNamedProperty(_hash, _name, _checkValid); 546 } 547 548 } 549 550 @Override 551 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 552 switch (hash) { 553 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 554 case 1771900717: /*assurance*/ return this.assurance == null ? new Base[0] : new Base[] {this.assurance}; // Enumeration<IdentityAssuranceLevel> 555 default: return super.getProperty(hash, name, checkValid); 556 } 557 558 } 559 560 @Override 561 public Base setProperty(int hash, String name, Base value) throws FHIRException { 562 switch (hash) { 563 case -880905839: // target 564 this.target = TypeConvertor.castToReference(value); // Reference 565 return value; 566 case 1771900717: // assurance 567 value = new IdentityAssuranceLevelEnumFactory().fromType(TypeConvertor.castToCode(value)); 568 this.assurance = (Enumeration) value; // Enumeration<IdentityAssuranceLevel> 569 return value; 570 default: return super.setProperty(hash, name, value); 571 } 572 573 } 574 575 @Override 576 public Base setProperty(String name, Base value) throws FHIRException { 577 if (name.equals("target")) { 578 this.target = TypeConvertor.castToReference(value); // Reference 579 } else if (name.equals("assurance")) { 580 value = new IdentityAssuranceLevelEnumFactory().fromType(TypeConvertor.castToCode(value)); 581 this.assurance = (Enumeration) value; // Enumeration<IdentityAssuranceLevel> 582 } else 583 return super.setProperty(name, value); 584 return value; 585 } 586 587 @Override 588 public void removeChild(String name, Base value) throws FHIRException { 589 if (name.equals("target")) { 590 this.target = null; 591 } else if (name.equals("assurance")) { 592 value = new IdentityAssuranceLevelEnumFactory().fromType(TypeConvertor.castToCode(value)); 593 this.assurance = (Enumeration) value; // Enumeration<IdentityAssuranceLevel> 594 } else 595 super.removeChild(name, value); 596 597 } 598 599 @Override 600 public Base makeProperty(int hash, String name) throws FHIRException { 601 switch (hash) { 602 case -880905839: return getTarget(); 603 case 1771900717: return getAssuranceElement(); 604 default: return super.makeProperty(hash, name); 605 } 606 607 } 608 609 @Override 610 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 611 switch (hash) { 612 case -880905839: /*target*/ return new String[] {"Reference"}; 613 case 1771900717: /*assurance*/ return new String[] {"code"}; 614 default: return super.getTypesForProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public Base addChild(String name) throws FHIRException { 621 if (name.equals("target")) { 622 this.target = new Reference(); 623 return this.target; 624 } 625 else if (name.equals("assurance")) { 626 throw new FHIRException("Cannot call addChild on a singleton property Person.link.assurance"); 627 } 628 else 629 return super.addChild(name); 630 } 631 632 public PersonLinkComponent copy() { 633 PersonLinkComponent dst = new PersonLinkComponent(); 634 copyValues(dst); 635 return dst; 636 } 637 638 public void copyValues(PersonLinkComponent dst) { 639 super.copyValues(dst); 640 dst.target = target == null ? null : target.copy(); 641 dst.assurance = assurance == null ? null : assurance.copy(); 642 } 643 644 @Override 645 public boolean equalsDeep(Base other_) { 646 if (!super.equalsDeep(other_)) 647 return false; 648 if (!(other_ instanceof PersonLinkComponent)) 649 return false; 650 PersonLinkComponent o = (PersonLinkComponent) other_; 651 return compareDeep(target, o.target, true) && compareDeep(assurance, o.assurance, true); 652 } 653 654 @Override 655 public boolean equalsShallow(Base other_) { 656 if (!super.equalsShallow(other_)) 657 return false; 658 if (!(other_ instanceof PersonLinkComponent)) 659 return false; 660 PersonLinkComponent o = (PersonLinkComponent) other_; 661 return compareValues(assurance, o.assurance, true); 662 } 663 664 public boolean isEmpty() { 665 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, assurance); 666 } 667 668 public String fhirType() { 669 return "Person.link"; 670 671 } 672 673 } 674 675 /** 676 * Identifier for a person within a particular scope. 677 */ 678 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 679 @Description(shortDefinition="A human identifier for this person", formalDefinition="Identifier for a person within a particular scope." ) 680 protected List<Identifier> identifier; 681 682 /** 683 * Whether this person's record is in active use. 684 */ 685 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 686 @Description(shortDefinition="This person's record is in active use", formalDefinition="Whether this person's record is in active use." ) 687 protected BooleanType active; 688 689 /** 690 * A name associated with the person. 691 */ 692 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 693 @Description(shortDefinition="A name associated with the person", formalDefinition="A name associated with the person." ) 694 protected List<HumanName> name; 695 696 /** 697 * A contact detail for the person, e.g. a telephone number or an email address. 698 */ 699 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 700 @Description(shortDefinition="A contact detail for the person", formalDefinition="A contact detail for the person, e.g. a telephone number or an email address." ) 701 protected List<ContactPoint> telecom; 702 703 /** 704 * Administrative Gender. 705 */ 706 @Child(name = "gender", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 707 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender." ) 708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 709 protected Enumeration<AdministrativeGender> gender; 710 711 /** 712 * The birth date for the person. 713 */ 714 @Child(name = "birthDate", type = {DateType.class}, order=5, min=0, max=1, modifier=false, summary=true) 715 @Description(shortDefinition="The date on which the person was born", formalDefinition="The birth date for the person." ) 716 protected DateType birthDate; 717 718 /** 719 * Indicates if the individual is deceased or not. 720 */ 721 @Child(name = "deceased", type = {BooleanType.class, DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 722 @Description(shortDefinition="Indicates if the individual is deceased or not", formalDefinition="Indicates if the individual is deceased or not." ) 723 protected DataType deceased; 724 725 /** 726 * One or more addresses for the person. 727 */ 728 @Child(name = "address", type = {Address.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 729 @Description(shortDefinition="One or more addresses for the person", formalDefinition="One or more addresses for the person." ) 730 protected List<Address> address; 731 732 /** 733 * This field contains a person's most recent marital (civil) status. 734 */ 735 @Child(name = "maritalStatus", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 736 @Description(shortDefinition="Marital (civil) status of a person", formalDefinition="This field contains a person's most recent marital (civil) status." ) 737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/marital-status") 738 protected CodeableConcept maritalStatus; 739 740 /** 741 * An image that can be displayed as a thumbnail of the person to enhance the identification of the individual. 742 */ 743 @Child(name = "photo", type = {Attachment.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 744 @Description(shortDefinition="Image of the person", formalDefinition="An image that can be displayed as a thumbnail of the person to enhance the identification of the individual." ) 745 protected List<Attachment> photo; 746 747 /** 748 * A language which may be used to communicate with the person about his or her health. 749 */ 750 @Child(name = "communication", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 751 @Description(shortDefinition="A language which may be used to communicate with the person about his or her health", formalDefinition="A language which may be used to communicate with the person about his or her health." ) 752 protected List<PersonCommunicationComponent> communication; 753 754 /** 755 * The organization that is the custodian of the person record. 756 */ 757 @Child(name = "managingOrganization", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 758 @Description(shortDefinition="The organization that is the custodian of the person record", formalDefinition="The organization that is the custodian of the person record." ) 759 protected Reference managingOrganization; 760 761 /** 762 * Link to a resource that concerns the same actual person. 763 */ 764 @Child(name = "link", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 765 @Description(shortDefinition="Link to a resource that concerns the same actual person", formalDefinition="Link to a resource that concerns the same actual person." ) 766 protected List<PersonLinkComponent> link; 767 768 private static final long serialVersionUID = -1871612358L; 769 770 /** 771 * Constructor 772 */ 773 public Person() { 774 super(); 775 } 776 777 /** 778 * @return {@link #identifier} (Identifier for a person within a particular scope.) 779 */ 780 public List<Identifier> getIdentifier() { 781 if (this.identifier == null) 782 this.identifier = new ArrayList<Identifier>(); 783 return this.identifier; 784 } 785 786 /** 787 * @return Returns a reference to <code>this</code> for easy method chaining 788 */ 789 public Person setIdentifier(List<Identifier> theIdentifier) { 790 this.identifier = theIdentifier; 791 return this; 792 } 793 794 public boolean hasIdentifier() { 795 if (this.identifier == null) 796 return false; 797 for (Identifier item : this.identifier) 798 if (!item.isEmpty()) 799 return true; 800 return false; 801 } 802 803 public Identifier addIdentifier() { //3 804 Identifier t = new Identifier(); 805 if (this.identifier == null) 806 this.identifier = new ArrayList<Identifier>(); 807 this.identifier.add(t); 808 return t; 809 } 810 811 public Person addIdentifier(Identifier t) { //3 812 if (t == null) 813 return this; 814 if (this.identifier == null) 815 this.identifier = new ArrayList<Identifier>(); 816 this.identifier.add(t); 817 return this; 818 } 819 820 /** 821 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 822 */ 823 public Identifier getIdentifierFirstRep() { 824 if (getIdentifier().isEmpty()) { 825 addIdentifier(); 826 } 827 return getIdentifier().get(0); 828 } 829 830 /** 831 * @return {@link #active} (Whether this person's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 832 */ 833 public BooleanType getActiveElement() { 834 if (this.active == null) 835 if (Configuration.errorOnAutoCreate()) 836 throw new Error("Attempt to auto-create Person.active"); 837 else if (Configuration.doAutoCreate()) 838 this.active = new BooleanType(); // bb 839 return this.active; 840 } 841 842 public boolean hasActiveElement() { 843 return this.active != null && !this.active.isEmpty(); 844 } 845 846 public boolean hasActive() { 847 return this.active != null && !this.active.isEmpty(); 848 } 849 850 /** 851 * @param value {@link #active} (Whether this person's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 852 */ 853 public Person setActiveElement(BooleanType value) { 854 this.active = value; 855 return this; 856 } 857 858 /** 859 * @return Whether this person's record is in active use. 860 */ 861 public boolean getActive() { 862 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 863 } 864 865 /** 866 * @param value Whether this person's record is in active use. 867 */ 868 public Person setActive(boolean value) { 869 if (this.active == null) 870 this.active = new BooleanType(); 871 this.active.setValue(value); 872 return this; 873 } 874 875 /** 876 * @return {@link #name} (A name associated with the person.) 877 */ 878 public List<HumanName> getName() { 879 if (this.name == null) 880 this.name = new ArrayList<HumanName>(); 881 return this.name; 882 } 883 884 /** 885 * @return Returns a reference to <code>this</code> for easy method chaining 886 */ 887 public Person setName(List<HumanName> theName) { 888 this.name = theName; 889 return this; 890 } 891 892 public boolean hasName() { 893 if (this.name == null) 894 return false; 895 for (HumanName item : this.name) 896 if (!item.isEmpty()) 897 return true; 898 return false; 899 } 900 901 public HumanName addName() { //3 902 HumanName t = new HumanName(); 903 if (this.name == null) 904 this.name = new ArrayList<HumanName>(); 905 this.name.add(t); 906 return t; 907 } 908 909 public Person addName(HumanName t) { //3 910 if (t == null) 911 return this; 912 if (this.name == null) 913 this.name = new ArrayList<HumanName>(); 914 this.name.add(t); 915 return this; 916 } 917 918 /** 919 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 920 */ 921 public HumanName getNameFirstRep() { 922 if (getName().isEmpty()) { 923 addName(); 924 } 925 return getName().get(0); 926 } 927 928 /** 929 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone number or an email address.) 930 */ 931 public List<ContactPoint> getTelecom() { 932 if (this.telecom == null) 933 this.telecom = new ArrayList<ContactPoint>(); 934 return this.telecom; 935 } 936 937 /** 938 * @return Returns a reference to <code>this</code> for easy method chaining 939 */ 940 public Person setTelecom(List<ContactPoint> theTelecom) { 941 this.telecom = theTelecom; 942 return this; 943 } 944 945 public boolean hasTelecom() { 946 if (this.telecom == null) 947 return false; 948 for (ContactPoint item : this.telecom) 949 if (!item.isEmpty()) 950 return true; 951 return false; 952 } 953 954 public ContactPoint addTelecom() { //3 955 ContactPoint t = new ContactPoint(); 956 if (this.telecom == null) 957 this.telecom = new ArrayList<ContactPoint>(); 958 this.telecom.add(t); 959 return t; 960 } 961 962 public Person addTelecom(ContactPoint t) { //3 963 if (t == null) 964 return this; 965 if (this.telecom == null) 966 this.telecom = new ArrayList<ContactPoint>(); 967 this.telecom.add(t); 968 return this; 969 } 970 971 /** 972 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 973 */ 974 public ContactPoint getTelecomFirstRep() { 975 if (getTelecom().isEmpty()) { 976 addTelecom(); 977 } 978 return getTelecom().get(0); 979 } 980 981 /** 982 * @return {@link #gender} (Administrative Gender.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 983 */ 984 public Enumeration<AdministrativeGender> getGenderElement() { 985 if (this.gender == null) 986 if (Configuration.errorOnAutoCreate()) 987 throw new Error("Attempt to auto-create Person.gender"); 988 else if (Configuration.doAutoCreate()) 989 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 990 return this.gender; 991 } 992 993 public boolean hasGenderElement() { 994 return this.gender != null && !this.gender.isEmpty(); 995 } 996 997 public boolean hasGender() { 998 return this.gender != null && !this.gender.isEmpty(); 999 } 1000 1001 /** 1002 * @param value {@link #gender} (Administrative Gender.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 1003 */ 1004 public Person setGenderElement(Enumeration<AdministrativeGender> value) { 1005 this.gender = value; 1006 return this; 1007 } 1008 1009 /** 1010 * @return Administrative Gender. 1011 */ 1012 public AdministrativeGender getGender() { 1013 return this.gender == null ? null : this.gender.getValue(); 1014 } 1015 1016 /** 1017 * @param value Administrative Gender. 1018 */ 1019 public Person setGender(AdministrativeGender value) { 1020 if (value == null) 1021 this.gender = null; 1022 else { 1023 if (this.gender == null) 1024 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1025 this.gender.setValue(value); 1026 } 1027 return this; 1028 } 1029 1030 /** 1031 * @return {@link #birthDate} (The birth date for the person.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 1032 */ 1033 public DateType getBirthDateElement() { 1034 if (this.birthDate == null) 1035 if (Configuration.errorOnAutoCreate()) 1036 throw new Error("Attempt to auto-create Person.birthDate"); 1037 else if (Configuration.doAutoCreate()) 1038 this.birthDate = new DateType(); // bb 1039 return this.birthDate; 1040 } 1041 1042 public boolean hasBirthDateElement() { 1043 return this.birthDate != null && !this.birthDate.isEmpty(); 1044 } 1045 1046 public boolean hasBirthDate() { 1047 return this.birthDate != null && !this.birthDate.isEmpty(); 1048 } 1049 1050 /** 1051 * @param value {@link #birthDate} (The birth date for the person.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 1052 */ 1053 public Person setBirthDateElement(DateType value) { 1054 this.birthDate = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return The birth date for the person. 1060 */ 1061 public Date getBirthDate() { 1062 return this.birthDate == null ? null : this.birthDate.getValue(); 1063 } 1064 1065 /** 1066 * @param value The birth date for the person. 1067 */ 1068 public Person setBirthDate(Date value) { 1069 if (value == null) 1070 this.birthDate = null; 1071 else { 1072 if (this.birthDate == null) 1073 this.birthDate = new DateType(); 1074 this.birthDate.setValue(value); 1075 } 1076 return this; 1077 } 1078 1079 /** 1080 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1081 */ 1082 public DataType getDeceased() { 1083 return this.deceased; 1084 } 1085 1086 /** 1087 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1088 */ 1089 public BooleanType getDeceasedBooleanType() throws FHIRException { 1090 if (this.deceased == null) 1091 this.deceased = new BooleanType(); 1092 if (!(this.deceased instanceof BooleanType)) 1093 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1094 return (BooleanType) this.deceased; 1095 } 1096 1097 public boolean hasDeceasedBooleanType() { 1098 return this.deceased instanceof BooleanType; 1099 } 1100 1101 /** 1102 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1103 */ 1104 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 1105 if (this.deceased == null) 1106 this.deceased = new DateTimeType(); 1107 if (!(this.deceased instanceof DateTimeType)) 1108 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1109 return (DateTimeType) this.deceased; 1110 } 1111 1112 public boolean hasDeceasedDateTimeType() { 1113 return this.deceased instanceof DateTimeType; 1114 } 1115 1116 public boolean hasDeceased() { 1117 return this.deceased != null && !this.deceased.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #deceased} (Indicates if the individual is deceased or not.) 1122 */ 1123 public Person setDeceased(DataType value) { 1124 if (value != null && !(value instanceof BooleanType || value instanceof DateTimeType)) 1125 throw new FHIRException("Not the right type for Person.deceased[x]: "+value.fhirType()); 1126 this.deceased = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #address} (One or more addresses for the person.) 1132 */ 1133 public List<Address> getAddress() { 1134 if (this.address == null) 1135 this.address = new ArrayList<Address>(); 1136 return this.address; 1137 } 1138 1139 /** 1140 * @return Returns a reference to <code>this</code> for easy method chaining 1141 */ 1142 public Person setAddress(List<Address> theAddress) { 1143 this.address = theAddress; 1144 return this; 1145 } 1146 1147 public boolean hasAddress() { 1148 if (this.address == null) 1149 return false; 1150 for (Address item : this.address) 1151 if (!item.isEmpty()) 1152 return true; 1153 return false; 1154 } 1155 1156 public Address addAddress() { //3 1157 Address t = new Address(); 1158 if (this.address == null) 1159 this.address = new ArrayList<Address>(); 1160 this.address.add(t); 1161 return t; 1162 } 1163 1164 public Person addAddress(Address t) { //3 1165 if (t == null) 1166 return this; 1167 if (this.address == null) 1168 this.address = new ArrayList<Address>(); 1169 this.address.add(t); 1170 return this; 1171 } 1172 1173 /** 1174 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist {3} 1175 */ 1176 public Address getAddressFirstRep() { 1177 if (getAddress().isEmpty()) { 1178 addAddress(); 1179 } 1180 return getAddress().get(0); 1181 } 1182 1183 /** 1184 * @return {@link #maritalStatus} (This field contains a person's most recent marital (civil) status.) 1185 */ 1186 public CodeableConcept getMaritalStatus() { 1187 if (this.maritalStatus == null) 1188 if (Configuration.errorOnAutoCreate()) 1189 throw new Error("Attempt to auto-create Person.maritalStatus"); 1190 else if (Configuration.doAutoCreate()) 1191 this.maritalStatus = new CodeableConcept(); // cc 1192 return this.maritalStatus; 1193 } 1194 1195 public boolean hasMaritalStatus() { 1196 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #maritalStatus} (This field contains a person's most recent marital (civil) status.) 1201 */ 1202 public Person setMaritalStatus(CodeableConcept value) { 1203 this.maritalStatus = value; 1204 return this; 1205 } 1206 1207 /** 1208 * @return {@link #photo} (An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.) 1209 */ 1210 public List<Attachment> getPhoto() { 1211 if (this.photo == null) 1212 this.photo = new ArrayList<Attachment>(); 1213 return this.photo; 1214 } 1215 1216 /** 1217 * @return Returns a reference to <code>this</code> for easy method chaining 1218 */ 1219 public Person setPhoto(List<Attachment> thePhoto) { 1220 this.photo = thePhoto; 1221 return this; 1222 } 1223 1224 public boolean hasPhoto() { 1225 if (this.photo == null) 1226 return false; 1227 for (Attachment item : this.photo) 1228 if (!item.isEmpty()) 1229 return true; 1230 return false; 1231 } 1232 1233 public Attachment addPhoto() { //3 1234 Attachment t = new Attachment(); 1235 if (this.photo == null) 1236 this.photo = new ArrayList<Attachment>(); 1237 this.photo.add(t); 1238 return t; 1239 } 1240 1241 public Person addPhoto(Attachment t) { //3 1242 if (t == null) 1243 return this; 1244 if (this.photo == null) 1245 this.photo = new ArrayList<Attachment>(); 1246 this.photo.add(t); 1247 return this; 1248 } 1249 1250 /** 1251 * @return The first repetition of repeating field {@link #photo}, creating it if it does not already exist {3} 1252 */ 1253 public Attachment getPhotoFirstRep() { 1254 if (getPhoto().isEmpty()) { 1255 addPhoto(); 1256 } 1257 return getPhoto().get(0); 1258 } 1259 1260 /** 1261 * @return {@link #communication} (A language which may be used to communicate with the person about his or her health.) 1262 */ 1263 public List<PersonCommunicationComponent> getCommunication() { 1264 if (this.communication == null) 1265 this.communication = new ArrayList<PersonCommunicationComponent>(); 1266 return this.communication; 1267 } 1268 1269 /** 1270 * @return Returns a reference to <code>this</code> for easy method chaining 1271 */ 1272 public Person setCommunication(List<PersonCommunicationComponent> theCommunication) { 1273 this.communication = theCommunication; 1274 return this; 1275 } 1276 1277 public boolean hasCommunication() { 1278 if (this.communication == null) 1279 return false; 1280 for (PersonCommunicationComponent item : this.communication) 1281 if (!item.isEmpty()) 1282 return true; 1283 return false; 1284 } 1285 1286 public PersonCommunicationComponent addCommunication() { //3 1287 PersonCommunicationComponent t = new PersonCommunicationComponent(); 1288 if (this.communication == null) 1289 this.communication = new ArrayList<PersonCommunicationComponent>(); 1290 this.communication.add(t); 1291 return t; 1292 } 1293 1294 public Person addCommunication(PersonCommunicationComponent t) { //3 1295 if (t == null) 1296 return this; 1297 if (this.communication == null) 1298 this.communication = new ArrayList<PersonCommunicationComponent>(); 1299 this.communication.add(t); 1300 return this; 1301 } 1302 1303 /** 1304 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist {3} 1305 */ 1306 public PersonCommunicationComponent getCommunicationFirstRep() { 1307 if (getCommunication().isEmpty()) { 1308 addCommunication(); 1309 } 1310 return getCommunication().get(0); 1311 } 1312 1313 /** 1314 * @return {@link #managingOrganization} (The organization that is the custodian of the person record.) 1315 */ 1316 public Reference getManagingOrganization() { 1317 if (this.managingOrganization == null) 1318 if (Configuration.errorOnAutoCreate()) 1319 throw new Error("Attempt to auto-create Person.managingOrganization"); 1320 else if (Configuration.doAutoCreate()) 1321 this.managingOrganization = new Reference(); // cc 1322 return this.managingOrganization; 1323 } 1324 1325 public boolean hasManagingOrganization() { 1326 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1327 } 1328 1329 /** 1330 * @param value {@link #managingOrganization} (The organization that is the custodian of the person record.) 1331 */ 1332 public Person setManagingOrganization(Reference value) { 1333 this.managingOrganization = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return {@link #link} (Link to a resource that concerns the same actual person.) 1339 */ 1340 public List<PersonLinkComponent> getLink() { 1341 if (this.link == null) 1342 this.link = new ArrayList<PersonLinkComponent>(); 1343 return this.link; 1344 } 1345 1346 /** 1347 * @return Returns a reference to <code>this</code> for easy method chaining 1348 */ 1349 public Person setLink(List<PersonLinkComponent> theLink) { 1350 this.link = theLink; 1351 return this; 1352 } 1353 1354 public boolean hasLink() { 1355 if (this.link == null) 1356 return false; 1357 for (PersonLinkComponent item : this.link) 1358 if (!item.isEmpty()) 1359 return true; 1360 return false; 1361 } 1362 1363 public PersonLinkComponent addLink() { //3 1364 PersonLinkComponent t = new PersonLinkComponent(); 1365 if (this.link == null) 1366 this.link = new ArrayList<PersonLinkComponent>(); 1367 this.link.add(t); 1368 return t; 1369 } 1370 1371 public Person addLink(PersonLinkComponent t) { //3 1372 if (t == null) 1373 return this; 1374 if (this.link == null) 1375 this.link = new ArrayList<PersonLinkComponent>(); 1376 this.link.add(t); 1377 return this; 1378 } 1379 1380 /** 1381 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist {3} 1382 */ 1383 public PersonLinkComponent getLinkFirstRep() { 1384 if (getLink().isEmpty()) { 1385 addLink(); 1386 } 1387 return getLink().get(0); 1388 } 1389 1390 protected void listChildren(List<Property> children) { 1391 super.listChildren(children); 1392 children.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1393 children.add(new Property("active", "boolean", "Whether this person's record is in active use.", 0, 1, active)); 1394 children.add(new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 1395 children.add(new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1396 children.add(new Property("gender", "code", "Administrative Gender.", 0, 1, gender)); 1397 children.add(new Property("birthDate", "date", "The birth date for the person.", 0, 1, birthDate)); 1398 children.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased)); 1399 children.add(new Property("address", "Address", "One or more addresses for the person.", 0, java.lang.Integer.MAX_VALUE, address)); 1400 children.add(new Property("maritalStatus", "CodeableConcept", "This field contains a person's most recent marital (civil) status.", 0, 1, maritalStatus)); 1401 children.add(new Property("photo", "Attachment", "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.", 0, java.lang.Integer.MAX_VALUE, photo)); 1402 children.add(new Property("communication", "", "A language which may be used to communicate with the person about his or her health.", 0, java.lang.Integer.MAX_VALUE, communication)); 1403 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization that is the custodian of the person record.", 0, 1, managingOrganization)); 1404 children.add(new Property("link", "", "Link to a resource that concerns the same actual person.", 0, java.lang.Integer.MAX_VALUE, link)); 1405 } 1406 1407 @Override 1408 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1409 switch (_hash) { 1410 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier); 1411 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this person's record is in active use.", 0, 1, active); 1412 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name); 1413 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 1414 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender.", 0, 1, gender); 1415 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The birth date for the person.", 0, 1, birthDate); 1416 case -1311442804: /*deceased[x]*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 1417 case 561497972: /*deceased*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 1418 case 497463828: /*deceasedBoolean*/ return new Property("deceased[x]", "boolean", "Indicates if the individual is deceased or not.", 0, 1, deceased); 1419 case -1971804369: /*deceasedDateTime*/ return new Property("deceased[x]", "dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 1420 case -1147692044: /*address*/ return new Property("address", "Address", "One or more addresses for the person.", 0, java.lang.Integer.MAX_VALUE, address); 1421 case 1756919302: /*maritalStatus*/ return new Property("maritalStatus", "CodeableConcept", "This field contains a person's most recent marital (civil) status.", 0, 1, maritalStatus); 1422 case 106642994: /*photo*/ return new Property("photo", "Attachment", "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.", 0, java.lang.Integer.MAX_VALUE, photo); 1423 case -1035284522: /*communication*/ return new Property("communication", "", "A language which may be used to communicate with the person about his or her health.", 0, java.lang.Integer.MAX_VALUE, communication); 1424 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization that is the custodian of the person record.", 0, 1, managingOrganization); 1425 case 3321850: /*link*/ return new Property("link", "", "Link to a resource that concerns the same actual person.", 0, java.lang.Integer.MAX_VALUE, link); 1426 default: return super.getNamedProperty(_hash, _name, _checkValid); 1427 } 1428 1429 } 1430 1431 @Override 1432 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1433 switch (hash) { 1434 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1435 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1436 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1437 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1438 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1439 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 1440 case 561497972: /*deceased*/ return this.deceased == null ? new Base[0] : new Base[] {this.deceased}; // DataType 1441 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1442 case 1756919302: /*maritalStatus*/ return this.maritalStatus == null ? new Base[0] : new Base[] {this.maritalStatus}; // CodeableConcept 1443 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 1444 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // PersonCommunicationComponent 1445 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 1446 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PersonLinkComponent 1447 default: return super.getProperty(hash, name, checkValid); 1448 } 1449 1450 } 1451 1452 @Override 1453 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1454 switch (hash) { 1455 case -1618432855: // identifier 1456 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1457 return value; 1458 case -1422950650: // active 1459 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1460 return value; 1461 case 3373707: // name 1462 this.getName().add(TypeConvertor.castToHumanName(value)); // HumanName 1463 return value; 1464 case -1429363305: // telecom 1465 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 1466 return value; 1467 case -1249512767: // gender 1468 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1469 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1470 return value; 1471 case -1210031859: // birthDate 1472 this.birthDate = TypeConvertor.castToDate(value); // DateType 1473 return value; 1474 case 561497972: // deceased 1475 this.deceased = TypeConvertor.castToType(value); // DataType 1476 return value; 1477 case -1147692044: // address 1478 this.getAddress().add(TypeConvertor.castToAddress(value)); // Address 1479 return value; 1480 case 1756919302: // maritalStatus 1481 this.maritalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1482 return value; 1483 case 106642994: // photo 1484 this.getPhoto().add(TypeConvertor.castToAttachment(value)); // Attachment 1485 return value; 1486 case -1035284522: // communication 1487 this.getCommunication().add((PersonCommunicationComponent) value); // PersonCommunicationComponent 1488 return value; 1489 case -2058947787: // managingOrganization 1490 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1491 return value; 1492 case 3321850: // link 1493 this.getLink().add((PersonLinkComponent) value); // PersonLinkComponent 1494 return value; 1495 default: return super.setProperty(hash, name, value); 1496 } 1497 1498 } 1499 1500 @Override 1501 public Base setProperty(String name, Base value) throws FHIRException { 1502 if (name.equals("identifier")) { 1503 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1504 } else if (name.equals("active")) { 1505 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1506 } else if (name.equals("name")) { 1507 this.getName().add(TypeConvertor.castToHumanName(value)); 1508 } else if (name.equals("telecom")) { 1509 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 1510 } else if (name.equals("gender")) { 1511 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1512 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1513 } else if (name.equals("birthDate")) { 1514 this.birthDate = TypeConvertor.castToDate(value); // DateType 1515 } else if (name.equals("deceased[x]")) { 1516 this.deceased = TypeConvertor.castToType(value); // DataType 1517 } else if (name.equals("address")) { 1518 this.getAddress().add(TypeConvertor.castToAddress(value)); 1519 } else if (name.equals("maritalStatus")) { 1520 this.maritalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1521 } else if (name.equals("photo")) { 1522 this.getPhoto().add(TypeConvertor.castToAttachment(value)); 1523 } else if (name.equals("communication")) { 1524 this.getCommunication().add((PersonCommunicationComponent) value); 1525 } else if (name.equals("managingOrganization")) { 1526 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1527 } else if (name.equals("link")) { 1528 this.getLink().add((PersonLinkComponent) value); 1529 } else 1530 return super.setProperty(name, value); 1531 return value; 1532 } 1533 1534 @Override 1535 public void removeChild(String name, Base value) throws FHIRException { 1536 if (name.equals("identifier")) { 1537 this.getIdentifier().remove(value); 1538 } else if (name.equals("active")) { 1539 this.active = null; 1540 } else if (name.equals("name")) { 1541 this.getName().remove(value); 1542 } else if (name.equals("telecom")) { 1543 this.getTelecom().remove(value); 1544 } else if (name.equals("gender")) { 1545 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1546 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1547 } else if (name.equals("birthDate")) { 1548 this.birthDate = null; 1549 } else if (name.equals("deceased[x]")) { 1550 this.deceased = null; 1551 } else if (name.equals("address")) { 1552 this.getAddress().remove(value); 1553 } else if (name.equals("maritalStatus")) { 1554 this.maritalStatus = null; 1555 } else if (name.equals("photo")) { 1556 this.getPhoto().remove(value); 1557 } else if (name.equals("communication")) { 1558 this.getCommunication().remove((PersonCommunicationComponent) value); 1559 } else if (name.equals("managingOrganization")) { 1560 this.managingOrganization = null; 1561 } else if (name.equals("link")) { 1562 this.getLink().remove((PersonLinkComponent) value); 1563 } else 1564 super.removeChild(name, value); 1565 1566 } 1567 1568 @Override 1569 public Base makeProperty(int hash, String name) throws FHIRException { 1570 switch (hash) { 1571 case -1618432855: return addIdentifier(); 1572 case -1422950650: return getActiveElement(); 1573 case 3373707: return addName(); 1574 case -1429363305: return addTelecom(); 1575 case -1249512767: return getGenderElement(); 1576 case -1210031859: return getBirthDateElement(); 1577 case -1311442804: return getDeceased(); 1578 case 561497972: return getDeceased(); 1579 case -1147692044: return addAddress(); 1580 case 1756919302: return getMaritalStatus(); 1581 case 106642994: return addPhoto(); 1582 case -1035284522: return addCommunication(); 1583 case -2058947787: return getManagingOrganization(); 1584 case 3321850: return addLink(); 1585 default: return super.makeProperty(hash, name); 1586 } 1587 1588 } 1589 1590 @Override 1591 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1592 switch (hash) { 1593 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1594 case -1422950650: /*active*/ return new String[] {"boolean"}; 1595 case 3373707: /*name*/ return new String[] {"HumanName"}; 1596 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1597 case -1249512767: /*gender*/ return new String[] {"code"}; 1598 case -1210031859: /*birthDate*/ return new String[] {"date"}; 1599 case 561497972: /*deceased*/ return new String[] {"boolean", "dateTime"}; 1600 case -1147692044: /*address*/ return new String[] {"Address"}; 1601 case 1756919302: /*maritalStatus*/ return new String[] {"CodeableConcept"}; 1602 case 106642994: /*photo*/ return new String[] {"Attachment"}; 1603 case -1035284522: /*communication*/ return new String[] {}; 1604 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1605 case 3321850: /*link*/ return new String[] {}; 1606 default: return super.getTypesForProperty(hash, name); 1607 } 1608 1609 } 1610 1611 @Override 1612 public Base addChild(String name) throws FHIRException { 1613 if (name.equals("identifier")) { 1614 return addIdentifier(); 1615 } 1616 else if (name.equals("active")) { 1617 throw new FHIRException("Cannot call addChild on a singleton property Person.active"); 1618 } 1619 else if (name.equals("name")) { 1620 return addName(); 1621 } 1622 else if (name.equals("telecom")) { 1623 return addTelecom(); 1624 } 1625 else if (name.equals("gender")) { 1626 throw new FHIRException("Cannot call addChild on a singleton property Person.gender"); 1627 } 1628 else if (name.equals("birthDate")) { 1629 throw new FHIRException("Cannot call addChild on a singleton property Person.birthDate"); 1630 } 1631 else if (name.equals("deceasedBoolean")) { 1632 this.deceased = new BooleanType(); 1633 return this.deceased; 1634 } 1635 else if (name.equals("deceasedDateTime")) { 1636 this.deceased = new DateTimeType(); 1637 return this.deceased; 1638 } 1639 else if (name.equals("address")) { 1640 return addAddress(); 1641 } 1642 else if (name.equals("maritalStatus")) { 1643 this.maritalStatus = new CodeableConcept(); 1644 return this.maritalStatus; 1645 } 1646 else if (name.equals("photo")) { 1647 return addPhoto(); 1648 } 1649 else if (name.equals("communication")) { 1650 return addCommunication(); 1651 } 1652 else if (name.equals("managingOrganization")) { 1653 this.managingOrganization = new Reference(); 1654 return this.managingOrganization; 1655 } 1656 else if (name.equals("link")) { 1657 return addLink(); 1658 } 1659 else 1660 return super.addChild(name); 1661 } 1662 1663 public String fhirType() { 1664 return "Person"; 1665 1666 } 1667 1668 public Person copy() { 1669 Person dst = new Person(); 1670 copyValues(dst); 1671 return dst; 1672 } 1673 1674 public void copyValues(Person dst) { 1675 super.copyValues(dst); 1676 if (identifier != null) { 1677 dst.identifier = new ArrayList<Identifier>(); 1678 for (Identifier i : identifier) 1679 dst.identifier.add(i.copy()); 1680 }; 1681 dst.active = active == null ? null : active.copy(); 1682 if (name != null) { 1683 dst.name = new ArrayList<HumanName>(); 1684 for (HumanName i : name) 1685 dst.name.add(i.copy()); 1686 }; 1687 if (telecom != null) { 1688 dst.telecom = new ArrayList<ContactPoint>(); 1689 for (ContactPoint i : telecom) 1690 dst.telecom.add(i.copy()); 1691 }; 1692 dst.gender = gender == null ? null : gender.copy(); 1693 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1694 dst.deceased = deceased == null ? null : deceased.copy(); 1695 if (address != null) { 1696 dst.address = new ArrayList<Address>(); 1697 for (Address i : address) 1698 dst.address.add(i.copy()); 1699 }; 1700 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 1701 if (photo != null) { 1702 dst.photo = new ArrayList<Attachment>(); 1703 for (Attachment i : photo) 1704 dst.photo.add(i.copy()); 1705 }; 1706 if (communication != null) { 1707 dst.communication = new ArrayList<PersonCommunicationComponent>(); 1708 for (PersonCommunicationComponent i : communication) 1709 dst.communication.add(i.copy()); 1710 }; 1711 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1712 if (link != null) { 1713 dst.link = new ArrayList<PersonLinkComponent>(); 1714 for (PersonLinkComponent i : link) 1715 dst.link.add(i.copy()); 1716 }; 1717 } 1718 1719 protected Person typedCopy() { 1720 return copy(); 1721 } 1722 1723 @Override 1724 public boolean equalsDeep(Base other_) { 1725 if (!super.equalsDeep(other_)) 1726 return false; 1727 if (!(other_ instanceof Person)) 1728 return false; 1729 Person o = (Person) other_; 1730 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(name, o.name, true) 1731 && compareDeep(telecom, o.telecom, true) && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 1732 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) && compareDeep(maritalStatus, o.maritalStatus, true) 1733 && compareDeep(photo, o.photo, true) && compareDeep(communication, o.communication, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1734 && compareDeep(link, o.link, true); 1735 } 1736 1737 @Override 1738 public boolean equalsShallow(Base other_) { 1739 if (!super.equalsShallow(other_)) 1740 return false; 1741 if (!(other_ instanceof Person)) 1742 return false; 1743 Person o = (Person) other_; 1744 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 1745 ; 1746 } 1747 1748 public boolean isEmpty() { 1749 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name 1750 , telecom, gender, birthDate, deceased, address, maritalStatus, photo, communication 1751 , managingOrganization, link); 1752 } 1753 1754 @Override 1755 public ResourceType getResourceType() { 1756 return ResourceType.Person; 1757 } 1758 1759 /** 1760 * Search parameter: <b>death-date</b> 1761 * <p> 1762 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 1763 * Type: <b>date</b><br> 1764 * Path: <b>(Person.deceased.ofType(dateTime))</b><br> 1765 * </p> 1766 */ 1767 @SearchParamDefinition(name="death-date", path="(Person.deceased.ofType(dateTime))", description="The date of death has been provided and satisfies this search value", type="date" ) 1768 public static final String SP_DEATH_DATE = "death-date"; 1769 /** 1770 * <b>Fluent Client</b> search parameter constant for <b>death-date</b> 1771 * <p> 1772 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 1773 * Type: <b>date</b><br> 1774 * Path: <b>(Person.deceased.ofType(dateTime))</b><br> 1775 * </p> 1776 */ 1777 public static final ca.uhn.fhir.rest.gclient.DateClientParam DEATH_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DEATH_DATE); 1778 1779 /** 1780 * Search parameter: <b>deceased</b> 1781 * <p> 1782 * Description: <b>This person has been marked as deceased, or has a death date entered</b><br> 1783 * Type: <b>token</b><br> 1784 * Path: <b>Person.deceased.exists() and Person.deceased != false</b><br> 1785 * </p> 1786 */ 1787 @SearchParamDefinition(name="deceased", path="Person.deceased.exists() and Person.deceased != false", description="This person has been marked as deceased, or has a death date entered", type="token" ) 1788 public static final String SP_DECEASED = "deceased"; 1789 /** 1790 * <b>Fluent Client</b> search parameter constant for <b>deceased</b> 1791 * <p> 1792 * Description: <b>This person has been marked as deceased, or has a death date entered</b><br> 1793 * Type: <b>token</b><br> 1794 * Path: <b>Person.deceased.exists() and Person.deceased != false</b><br> 1795 * </p> 1796 */ 1797 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DECEASED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DECEASED); 1798 1799 /** 1800 * Search parameter: <b>family</b> 1801 * <p> 1802 * Description: <b>A portion of the family name of the person</b><br> 1803 * Type: <b>string</b><br> 1804 * Path: <b>Person.name.family</b><br> 1805 * </p> 1806 */ 1807 @SearchParamDefinition(name="family", path="Person.name.family", description="A portion of the family name of the person", type="string" ) 1808 public static final String SP_FAMILY = "family"; 1809 /** 1810 * <b>Fluent Client</b> search parameter constant for <b>family</b> 1811 * <p> 1812 * Description: <b>A portion of the family name of the person</b><br> 1813 * Type: <b>string</b><br> 1814 * Path: <b>Person.name.family</b><br> 1815 * </p> 1816 */ 1817 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_FAMILY); 1818 1819 /** 1820 * Search parameter: <b>given</b> 1821 * <p> 1822 * Description: <b>A portion of the given name of the person</b><br> 1823 * Type: <b>string</b><br> 1824 * Path: <b>Person.name.given</b><br> 1825 * </p> 1826 */ 1827 @SearchParamDefinition(name="given", path="Person.name.given", description="A portion of the given name of the person", type="string" ) 1828 public static final String SP_GIVEN = "given"; 1829 /** 1830 * <b>Fluent Client</b> search parameter constant for <b>given</b> 1831 * <p> 1832 * Description: <b>A portion of the given name of the person</b><br> 1833 * Type: <b>string</b><br> 1834 * Path: <b>Person.name.given</b><br> 1835 * </p> 1836 */ 1837 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GIVEN); 1838 1839 /** 1840 * Search parameter: <b>link</b> 1841 * <p> 1842 * Description: <b>Any link has this Patient, Person, RelatedPerson or Practitioner reference</b><br> 1843 * Type: <b>reference</b><br> 1844 * Path: <b>Person.link.target</b><br> 1845 * </p> 1846 */ 1847 @SearchParamDefinition(name="link", path="Person.link.target", description="Any link has this Patient, Person, RelatedPerson or Practitioner reference", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Patient.class, Person.class, Practitioner.class, RelatedPerson.class } ) 1848 public static final String SP_LINK = "link"; 1849 /** 1850 * <b>Fluent Client</b> search parameter constant for <b>link</b> 1851 * <p> 1852 * Description: <b>Any link has this Patient, Person, RelatedPerson or Practitioner reference</b><br> 1853 * Type: <b>reference</b><br> 1854 * Path: <b>Person.link.target</b><br> 1855 * </p> 1856 */ 1857 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LINK); 1858 1859/** 1860 * Constant for fluent queries to be used to add include statements. Specifies 1861 * the path value of "<b>Person:link</b>". 1862 */ 1863 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Person:link").toLocked(); 1864 1865 /** 1866 * Search parameter: <b>name</b> 1867 * <p> 1868 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1869 * Type: <b>string</b><br> 1870 * Path: <b>Person.name</b><br> 1871 * </p> 1872 */ 1873 @SearchParamDefinition(name="name", path="Person.name", description="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type="string" ) 1874 public static final String SP_NAME = "name"; 1875 /** 1876 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1877 * <p> 1878 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1879 * Type: <b>string</b><br> 1880 * Path: <b>Person.name</b><br> 1881 * </p> 1882 */ 1883 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1884 1885 /** 1886 * Search parameter: <b>organization</b> 1887 * <p> 1888 * Description: <b>The organization at which this person record is being managed</b><br> 1889 * Type: <b>reference</b><br> 1890 * Path: <b>Person.managingOrganization</b><br> 1891 * </p> 1892 */ 1893 @SearchParamDefinition(name="organization", path="Person.managingOrganization", description="The organization at which this person record is being managed", type="reference", target={Organization.class } ) 1894 public static final String SP_ORGANIZATION = "organization"; 1895 /** 1896 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1897 * <p> 1898 * Description: <b>The organization at which this person record is being managed</b><br> 1899 * Type: <b>reference</b><br> 1900 * Path: <b>Person.managingOrganization</b><br> 1901 * </p> 1902 */ 1903 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1904 1905/** 1906 * Constant for fluent queries to be used to add include statements. Specifies 1907 * the path value of "<b>Person:organization</b>". 1908 */ 1909 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Person:organization").toLocked(); 1910 1911 /** 1912 * Search parameter: <b>practitioner</b> 1913 * <p> 1914 * Description: <b>The Person links to this Practitioner</b><br> 1915 * Type: <b>reference</b><br> 1916 * Path: <b>Person.link.target.where(resolve() is Practitioner)</b><br> 1917 * </p> 1918 */ 1919 @SearchParamDefinition(name="practitioner", path="Person.link.target.where(resolve() is Practitioner)", description="The Person links to this Practitioner", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class } ) 1920 public static final String SP_PRACTITIONER = "practitioner"; 1921 /** 1922 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 1923 * <p> 1924 * Description: <b>The Person links to this Practitioner</b><br> 1925 * Type: <b>reference</b><br> 1926 * Path: <b>Person.link.target.where(resolve() is Practitioner)</b><br> 1927 * </p> 1928 */ 1929 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 1930 1931/** 1932 * Constant for fluent queries to be used to add include statements. Specifies 1933 * the path value of "<b>Person:practitioner</b>". 1934 */ 1935 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Person:practitioner").toLocked(); 1936 1937 /** 1938 * Search parameter: <b>relatedperson</b> 1939 * <p> 1940 * Description: <b>The Person links to this RelatedPerson</b><br> 1941 * Type: <b>reference</b><br> 1942 * Path: <b>Person.link.target.where(resolve() is RelatedPerson)</b><br> 1943 * </p> 1944 */ 1945 @SearchParamDefinition(name="relatedperson", path="Person.link.target.where(resolve() is RelatedPerson)", description="The Person links to this RelatedPerson", type="reference", target={RelatedPerson.class } ) 1946 public static final String SP_RELATEDPERSON = "relatedperson"; 1947 /** 1948 * <b>Fluent Client</b> search parameter constant for <b>relatedperson</b> 1949 * <p> 1950 * Description: <b>The Person links to this RelatedPerson</b><br> 1951 * Type: <b>reference</b><br> 1952 * Path: <b>Person.link.target.where(resolve() is RelatedPerson)</b><br> 1953 * </p> 1954 */ 1955 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATEDPERSON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATEDPERSON); 1956 1957/** 1958 * Constant for fluent queries to be used to add include statements. Specifies 1959 * the path value of "<b>Person:relatedperson</b>". 1960 */ 1961 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATEDPERSON = new ca.uhn.fhir.model.api.Include("Person:relatedperson").toLocked(); 1962 1963 /** 1964 * Search parameter: <b>identifier</b> 1965 * <p> 1966 * Description: <b>Multiple Resources: 1967 1968* [Account](account.html): Account number 1969* [AdverseEvent](adverseevent.html): Business identifier for the event 1970* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1971* [Appointment](appointment.html): An Identifier of the Appointment 1972* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1973* [Basic](basic.html): Business identifier 1974* [BodyStructure](bodystructure.html): Bodystructure identifier 1975* [CarePlan](careplan.html): External Ids for this plan 1976* [CareTeam](careteam.html): External Ids for this team 1977* [ChargeItem](chargeitem.html): Business Identifier for item 1978* [Claim](claim.html): The primary identifier of the financial resource 1979* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1980* [ClinicalImpression](clinicalimpression.html): Business identifier 1981* [Communication](communication.html): Unique identifier 1982* [CommunicationRequest](communicationrequest.html): Unique identifier 1983* [Composition](composition.html): Version-independent identifier for the Composition 1984* [Condition](condition.html): A unique identifier of the condition record 1985* [Consent](consent.html): Identifier for this record (external references) 1986* [Contract](contract.html): The identity of the contract 1987* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1988* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1989* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1990* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1991* [DeviceRequest](devicerequest.html): Business identifier for request/order 1992* [DeviceUsage](deviceusage.html): Search by identifier 1993* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1994* [DocumentReference](documentreference.html): Identifier of the attachment binary 1995* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1996* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1997* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1998* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1999* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2000* [Flag](flag.html): Business identifier 2001* [Goal](goal.html): External Ids for this goal 2002* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2003* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2004* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2005* [Immunization](immunization.html): Business identifier 2006* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2007* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2008* [Invoice](invoice.html): Business Identifier for item 2009* [List](list.html): Business identifier 2010* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2011* [Medication](medication.html): Returns medications with this external identifier 2012* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2013* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2014* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2015* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2016* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2017* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2018* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2019* [Observation](observation.html): The unique id for a particular observation 2020* [Person](person.html): A person Identifier 2021* [Procedure](procedure.html): A unique identifier for a procedure 2022* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2023* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2024* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2025* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2026* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2027* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2028* [Specimen](specimen.html): The unique identifier associated with the specimen 2029* [SupplyDelivery](supplydelivery.html): External identifier 2030* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2031* [Task](task.html): Search for a task instance by its business identifier 2032* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2033</b><br> 2034 * Type: <b>token</b><br> 2035 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2036 * </p> 2037 */ 2038 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2039 public static final String SP_IDENTIFIER = "identifier"; 2040 /** 2041 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2042 * <p> 2043 * Description: <b>Multiple Resources: 2044 2045* [Account](account.html): Account number 2046* [AdverseEvent](adverseevent.html): Business identifier for the event 2047* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2048* [Appointment](appointment.html): An Identifier of the Appointment 2049* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2050* [Basic](basic.html): Business identifier 2051* [BodyStructure](bodystructure.html): Bodystructure identifier 2052* [CarePlan](careplan.html): External Ids for this plan 2053* [CareTeam](careteam.html): External Ids for this team 2054* [ChargeItem](chargeitem.html): Business Identifier for item 2055* [Claim](claim.html): The primary identifier of the financial resource 2056* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2057* [ClinicalImpression](clinicalimpression.html): Business identifier 2058* [Communication](communication.html): Unique identifier 2059* [CommunicationRequest](communicationrequest.html): Unique identifier 2060* [Composition](composition.html): Version-independent identifier for the Composition 2061* [Condition](condition.html): A unique identifier of the condition record 2062* [Consent](consent.html): Identifier for this record (external references) 2063* [Contract](contract.html): The identity of the contract 2064* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2065* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2066* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2067* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2068* [DeviceRequest](devicerequest.html): Business identifier for request/order 2069* [DeviceUsage](deviceusage.html): Search by identifier 2070* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2071* [DocumentReference](documentreference.html): Identifier of the attachment binary 2072* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2073* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2074* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2075* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2076* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2077* [Flag](flag.html): Business identifier 2078* [Goal](goal.html): External Ids for this goal 2079* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2080* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2081* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2082* [Immunization](immunization.html): Business identifier 2083* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2084* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2085* [Invoice](invoice.html): Business Identifier for item 2086* [List](list.html): Business identifier 2087* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2088* [Medication](medication.html): Returns medications with this external identifier 2089* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2090* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2091* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2092* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2093* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2094* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2095* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2096* [Observation](observation.html): The unique id for a particular observation 2097* [Person](person.html): A person Identifier 2098* [Procedure](procedure.html): A unique identifier for a procedure 2099* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2100* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2101* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2102* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2103* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2104* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2105* [Specimen](specimen.html): The unique identifier associated with the specimen 2106* [SupplyDelivery](supplydelivery.html): External identifier 2107* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2108* [Task](task.html): Search for a task instance by its business identifier 2109* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2110</b><br> 2111 * Type: <b>token</b><br> 2112 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2113 * </p> 2114 */ 2115 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2116 2117 /** 2118 * Search parameter: <b>patient</b> 2119 * <p> 2120 * Description: <b>Multiple Resources: 2121 2122* [Account](account.html): The entity that caused the expenses 2123* [AdverseEvent](adverseevent.html): Subject impacted by event 2124* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2125* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2126* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2127* [AuditEvent](auditevent.html): Where the activity involved patient data 2128* [Basic](basic.html): Identifies the focus of this resource 2129* [BodyStructure](bodystructure.html): Who this is about 2130* [CarePlan](careplan.html): Who the care plan is for 2131* [CareTeam](careteam.html): Who care team is for 2132* [ChargeItem](chargeitem.html): Individual service was done for/to 2133* [Claim](claim.html): Patient receiving the products or services 2134* [ClaimResponse](claimresponse.html): The subject of care 2135* [ClinicalImpression](clinicalimpression.html): Patient assessed 2136* [Communication](communication.html): Focus of message 2137* [CommunicationRequest](communicationrequest.html): Focus of message 2138* [Composition](composition.html): Who and/or what the composition is about 2139* [Condition](condition.html): Who has the condition? 2140* [Consent](consent.html): Who the consent applies to 2141* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2142* [Coverage](coverage.html): Retrieve coverages for a patient 2143* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2144* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2145* [DetectedIssue](detectedissue.html): Associated patient 2146* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2147* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2148* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2149* [DocumentReference](documentreference.html): Who/what is the subject of the document 2150* [Encounter](encounter.html): The patient present at the encounter 2151* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2152* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2153* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2154* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2155* [Flag](flag.html): The identity of a subject to list flags for 2156* [Goal](goal.html): Who this goal is intended for 2157* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2158* [ImagingSelection](imagingselection.html): Who the study is about 2159* [ImagingStudy](imagingstudy.html): Who the study is about 2160* [Immunization](immunization.html): The patient for the vaccination record 2161* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2162* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2163* [Invoice](invoice.html): Recipient(s) of goods and services 2164* [List](list.html): If all resources have the same subject 2165* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2166* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2167* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2168* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2169* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2170* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2171* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2172* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2173* [Observation](observation.html): The subject that the observation is about (if patient) 2174* [Person](person.html): The Person links to this Patient 2175* [Procedure](procedure.html): Search by subject - a patient 2176* [Provenance](provenance.html): Where the activity involved patient data 2177* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2178* [RelatedPerson](relatedperson.html): The patient this related person is related to 2179* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2180* [ResearchSubject](researchsubject.html): Who or what is part of study 2181* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2182* [ServiceRequest](servicerequest.html): Search by subject - a patient 2183* [Specimen](specimen.html): The patient the specimen comes from 2184* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2185* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2186* [Task](task.html): Search by patient 2187* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2188</b><br> 2189 * Type: <b>reference</b><br> 2190 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2191 * </p> 2192 */ 2193 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2194 public static final String SP_PATIENT = "patient"; 2195 /** 2196 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2197 * <p> 2198 * Description: <b>Multiple Resources: 2199 2200* [Account](account.html): The entity that caused the expenses 2201* [AdverseEvent](adverseevent.html): Subject impacted by event 2202* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2203* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2204* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2205* [AuditEvent](auditevent.html): Where the activity involved patient data 2206* [Basic](basic.html): Identifies the focus of this resource 2207* [BodyStructure](bodystructure.html): Who this is about 2208* [CarePlan](careplan.html): Who the care plan is for 2209* [CareTeam](careteam.html): Who care team is for 2210* [ChargeItem](chargeitem.html): Individual service was done for/to 2211* [Claim](claim.html): Patient receiving the products or services 2212* [ClaimResponse](claimresponse.html): The subject of care 2213* [ClinicalImpression](clinicalimpression.html): Patient assessed 2214* [Communication](communication.html): Focus of message 2215* [CommunicationRequest](communicationrequest.html): Focus of message 2216* [Composition](composition.html): Who and/or what the composition is about 2217* [Condition](condition.html): Who has the condition? 2218* [Consent](consent.html): Who the consent applies to 2219* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2220* [Coverage](coverage.html): Retrieve coverages for a patient 2221* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2222* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2223* [DetectedIssue](detectedissue.html): Associated patient 2224* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2225* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2226* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2227* [DocumentReference](documentreference.html): Who/what is the subject of the document 2228* [Encounter](encounter.html): The patient present at the encounter 2229* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2230* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2231* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2232* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2233* [Flag](flag.html): The identity of a subject to list flags for 2234* [Goal](goal.html): Who this goal is intended for 2235* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2236* [ImagingSelection](imagingselection.html): Who the study is about 2237* [ImagingStudy](imagingstudy.html): Who the study is about 2238* [Immunization](immunization.html): The patient for the vaccination record 2239* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2240* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2241* [Invoice](invoice.html): Recipient(s) of goods and services 2242* [List](list.html): If all resources have the same subject 2243* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2244* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2245* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2246* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2247* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2248* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2249* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2250* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2251* [Observation](observation.html): The subject that the observation is about (if patient) 2252* [Person](person.html): The Person links to this Patient 2253* [Procedure](procedure.html): Search by subject - a patient 2254* [Provenance](provenance.html): Where the activity involved patient data 2255* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2256* [RelatedPerson](relatedperson.html): The patient this related person is related to 2257* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2258* [ResearchSubject](researchsubject.html): Who or what is part of study 2259* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2260* [ServiceRequest](servicerequest.html): Search by subject - a patient 2261* [Specimen](specimen.html): The patient the specimen comes from 2262* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2263* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2264* [Task](task.html): Search by patient 2265* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2266</b><br> 2267 * Type: <b>reference</b><br> 2268 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2269 * </p> 2270 */ 2271 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2272 2273/** 2274 * Constant for fluent queries to be used to add include statements. Specifies 2275 * the path value of "<b>Person:patient</b>". 2276 */ 2277 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Person:patient").toLocked(); 2278 2279 /** 2280 * Search parameter: <b>address-city</b> 2281 * <p> 2282 * Description: <b>Multiple Resources: 2283 2284* [Patient](patient.html): A city specified in an address 2285* [Person](person.html): A city specified in an address 2286* [Practitioner](practitioner.html): A city specified in an address 2287* [RelatedPerson](relatedperson.html): A city specified in an address 2288</b><br> 2289 * Type: <b>string</b><br> 2290 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 2291 * </p> 2292 */ 2293 @SearchParamDefinition(name="address-city", path="Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A city specified in an address\r\n* [Person](person.html): A city specified in an address\r\n* [Practitioner](practitioner.html): A city specified in an address\r\n* [RelatedPerson](relatedperson.html): A city specified in an address\r\n", type="string" ) 2294 public static final String SP_ADDRESS_CITY = "address-city"; 2295 /** 2296 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 2297 * <p> 2298 * Description: <b>Multiple Resources: 2299 2300* [Patient](patient.html): A city specified in an address 2301* [Person](person.html): A city specified in an address 2302* [Practitioner](practitioner.html): A city specified in an address 2303* [RelatedPerson](relatedperson.html): A city specified in an address 2304</b><br> 2305 * Type: <b>string</b><br> 2306 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 2307 * </p> 2308 */ 2309 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 2310 2311 /** 2312 * Search parameter: <b>address-country</b> 2313 * <p> 2314 * Description: <b>Multiple Resources: 2315 2316* [Patient](patient.html): A country specified in an address 2317* [Person](person.html): A country specified in an address 2318* [Practitioner](practitioner.html): A country specified in an address 2319* [RelatedPerson](relatedperson.html): A country specified in an address 2320</b><br> 2321 * Type: <b>string</b><br> 2322 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 2323 * </p> 2324 */ 2325 @SearchParamDefinition(name="address-country", path="Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A country specified in an address\r\n* [Person](person.html): A country specified in an address\r\n* [Practitioner](practitioner.html): A country specified in an address\r\n* [RelatedPerson](relatedperson.html): A country specified in an address\r\n", type="string" ) 2326 public static final String SP_ADDRESS_COUNTRY = "address-country"; 2327 /** 2328 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 2329 * <p> 2330 * Description: <b>Multiple Resources: 2331 2332* [Patient](patient.html): A country specified in an address 2333* [Person](person.html): A country specified in an address 2334* [Practitioner](practitioner.html): A country specified in an address 2335* [RelatedPerson](relatedperson.html): A country specified in an address 2336</b><br> 2337 * Type: <b>string</b><br> 2338 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 2339 * </p> 2340 */ 2341 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 2342 2343 /** 2344 * Search parameter: <b>address-postalcode</b> 2345 * <p> 2346 * Description: <b>Multiple Resources: 2347 2348* [Patient](patient.html): A postalCode specified in an address 2349* [Person](person.html): A postal code specified in an address 2350* [Practitioner](practitioner.html): A postalCode specified in an address 2351* [RelatedPerson](relatedperson.html): A postal code specified in an address 2352</b><br> 2353 * Type: <b>string</b><br> 2354 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 2355 * </p> 2356 */ 2357 @SearchParamDefinition(name="address-postalcode", path="Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A postalCode specified in an address\r\n* [Person](person.html): A postal code specified in an address\r\n* [Practitioner](practitioner.html): A postalCode specified in an address\r\n* [RelatedPerson](relatedperson.html): A postal code specified in an address\r\n", type="string" ) 2358 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 2359 /** 2360 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 2361 * <p> 2362 * Description: <b>Multiple Resources: 2363 2364* [Patient](patient.html): A postalCode specified in an address 2365* [Person](person.html): A postal code specified in an address 2366* [Practitioner](practitioner.html): A postalCode specified in an address 2367* [RelatedPerson](relatedperson.html): A postal code specified in an address 2368</b><br> 2369 * Type: <b>string</b><br> 2370 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 2371 * </p> 2372 */ 2373 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 2374 2375 /** 2376 * Search parameter: <b>address-state</b> 2377 * <p> 2378 * Description: <b>Multiple Resources: 2379 2380* [Patient](patient.html): A state specified in an address 2381* [Person](person.html): A state specified in an address 2382* [Practitioner](practitioner.html): A state specified in an address 2383* [RelatedPerson](relatedperson.html): A state specified in an address 2384</b><br> 2385 * Type: <b>string</b><br> 2386 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 2387 * </p> 2388 */ 2389 @SearchParamDefinition(name="address-state", path="Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A state specified in an address\r\n* [Person](person.html): A state specified in an address\r\n* [Practitioner](practitioner.html): A state specified in an address\r\n* [RelatedPerson](relatedperson.html): A state specified in an address\r\n", type="string" ) 2390 public static final String SP_ADDRESS_STATE = "address-state"; 2391 /** 2392 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 2393 * <p> 2394 * Description: <b>Multiple Resources: 2395 2396* [Patient](patient.html): A state specified in an address 2397* [Person](person.html): A state specified in an address 2398* [Practitioner](practitioner.html): A state specified in an address 2399* [RelatedPerson](relatedperson.html): A state specified in an address 2400</b><br> 2401 * Type: <b>string</b><br> 2402 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 2403 * </p> 2404 */ 2405 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 2406 2407 /** 2408 * Search parameter: <b>address-use</b> 2409 * <p> 2410 * Description: <b>Multiple Resources: 2411 2412* [Patient](patient.html): A use code specified in an address 2413* [Person](person.html): A use code specified in an address 2414* [Practitioner](practitioner.html): A use code specified in an address 2415* [RelatedPerson](relatedperson.html): A use code specified in an address 2416</b><br> 2417 * Type: <b>token</b><br> 2418 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 2419 * </p> 2420 */ 2421 @SearchParamDefinition(name="address-use", path="Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A use code specified in an address\r\n* [Person](person.html): A use code specified in an address\r\n* [Practitioner](practitioner.html): A use code specified in an address\r\n* [RelatedPerson](relatedperson.html): A use code specified in an address\r\n", type="token" ) 2422 public static final String SP_ADDRESS_USE = "address-use"; 2423 /** 2424 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 2425 * <p> 2426 * Description: <b>Multiple Resources: 2427 2428* [Patient](patient.html): A use code specified in an address 2429* [Person](person.html): A use code specified in an address 2430* [Practitioner](practitioner.html): A use code specified in an address 2431* [RelatedPerson](relatedperson.html): A use code specified in an address 2432</b><br> 2433 * Type: <b>token</b><br> 2434 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 2435 * </p> 2436 */ 2437 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 2438 2439 /** 2440 * Search parameter: <b>address</b> 2441 * <p> 2442 * Description: <b>Multiple Resources: 2443 2444* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2445* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2446* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2447* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2448</b><br> 2449 * Type: <b>string</b><br> 2450 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 2451 * </p> 2452 */ 2453 @SearchParamDefinition(name="address", path="Patient.address | Person.address | Practitioner.address | RelatedPerson.address", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n", type="string" ) 2454 public static final String SP_ADDRESS = "address"; 2455 /** 2456 * <b>Fluent Client</b> search parameter constant for <b>address</b> 2457 * <p> 2458 * Description: <b>Multiple Resources: 2459 2460* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2461* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2462* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2463* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 2464</b><br> 2465 * Type: <b>string</b><br> 2466 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 2467 * </p> 2468 */ 2469 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 2470 2471 /** 2472 * Search parameter: <b>birthdate</b> 2473 * <p> 2474 * Description: <b>Multiple Resources: 2475 2476* [Patient](patient.html): The patient's date of birth 2477* [Person](person.html): The person's date of birth 2478* [RelatedPerson](relatedperson.html): The Related Person's date of birth 2479</b><br> 2480 * Type: <b>date</b><br> 2481 * Path: <b>Patient.birthDate | Person.birthDate | RelatedPerson.birthDate</b><br> 2482 * </p> 2483 */ 2484 @SearchParamDefinition(name="birthdate", path="Patient.birthDate | Person.birthDate | RelatedPerson.birthDate", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The patient's date of birth\r\n* [Person](person.html): The person's date of birth\r\n* [RelatedPerson](relatedperson.html): The Related Person's date of birth\r\n", type="date" ) 2485 public static final String SP_BIRTHDATE = "birthdate"; 2486 /** 2487 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 2488 * <p> 2489 * Description: <b>Multiple Resources: 2490 2491* [Patient](patient.html): The patient's date of birth 2492* [Person](person.html): The person's date of birth 2493* [RelatedPerson](relatedperson.html): The Related Person's date of birth 2494</b><br> 2495 * Type: <b>date</b><br> 2496 * Path: <b>Patient.birthDate | Person.birthDate | RelatedPerson.birthDate</b><br> 2497 * </p> 2498 */ 2499 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_BIRTHDATE); 2500 2501 /** 2502 * Search parameter: <b>email</b> 2503 * <p> 2504 * Description: <b>Multiple Resources: 2505 2506* [Patient](patient.html): A value in an email contact 2507* [Person](person.html): A value in an email contact 2508* [Practitioner](practitioner.html): A value in an email contact 2509* [PractitionerRole](practitionerrole.html): A value in an email contact 2510* [RelatedPerson](relatedperson.html): A value in an email contact 2511</b><br> 2512 * Type: <b>token</b><br> 2513 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 2514 * </p> 2515 */ 2516 @SearchParamDefinition(name="email", path="Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in an email contact\r\n* [Person](person.html): A value in an email contact\r\n* [Practitioner](practitioner.html): A value in an email contact\r\n* [PractitionerRole](practitionerrole.html): A value in an email contact\r\n* [RelatedPerson](relatedperson.html): A value in an email contact\r\n", type="token" ) 2517 public static final String SP_EMAIL = "email"; 2518 /** 2519 * <b>Fluent Client</b> search parameter constant for <b>email</b> 2520 * <p> 2521 * Description: <b>Multiple Resources: 2522 2523* [Patient](patient.html): A value in an email contact 2524* [Person](person.html): A value in an email contact 2525* [Practitioner](practitioner.html): A value in an email contact 2526* [PractitionerRole](practitionerrole.html): A value in an email contact 2527* [RelatedPerson](relatedperson.html): A value in an email contact 2528</b><br> 2529 * Type: <b>token</b><br> 2530 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 2531 * </p> 2532 */ 2533 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 2534 2535 /** 2536 * Search parameter: <b>gender</b> 2537 * <p> 2538 * Description: <b>Multiple Resources: 2539 2540* [Patient](patient.html): Gender of the patient 2541* [Person](person.html): The gender of the person 2542* [Practitioner](practitioner.html): Gender of the practitioner 2543* [RelatedPerson](relatedperson.html): Gender of the related person 2544</b><br> 2545 * Type: <b>token</b><br> 2546 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 2547 * </p> 2548 */ 2549 @SearchParamDefinition(name="gender", path="Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): Gender of the patient\r\n* [Person](person.html): The gender of the person\r\n* [Practitioner](practitioner.html): Gender of the practitioner\r\n* [RelatedPerson](relatedperson.html): Gender of the related person\r\n", type="token" ) 2550 public static final String SP_GENDER = "gender"; 2551 /** 2552 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 2553 * <p> 2554 * Description: <b>Multiple Resources: 2555 2556* [Patient](patient.html): Gender of the patient 2557* [Person](person.html): The gender of the person 2558* [Practitioner](practitioner.html): Gender of the practitioner 2559* [RelatedPerson](relatedperson.html): Gender of the related person 2560</b><br> 2561 * Type: <b>token</b><br> 2562 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 2563 * </p> 2564 */ 2565 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 2566 2567 /** 2568 * Search parameter: <b>phone</b> 2569 * <p> 2570 * Description: <b>Multiple Resources: 2571 2572* [Patient](patient.html): A value in a phone contact 2573* [Person](person.html): A value in a phone contact 2574* [Practitioner](practitioner.html): A value in a phone contact 2575* [PractitionerRole](practitionerrole.html): A value in a phone contact 2576* [RelatedPerson](relatedperson.html): A value in a phone contact 2577</b><br> 2578 * Type: <b>token</b><br> 2579 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 2580 * </p> 2581 */ 2582 @SearchParamDefinition(name="phone", path="Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in a phone contact\r\n* [Person](person.html): A value in a phone contact\r\n* [Practitioner](practitioner.html): A value in a phone contact\r\n* [PractitionerRole](practitionerrole.html): A value in a phone contact\r\n* [RelatedPerson](relatedperson.html): A value in a phone contact\r\n", type="token" ) 2583 public static final String SP_PHONE = "phone"; 2584 /** 2585 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 2586 * <p> 2587 * Description: <b>Multiple Resources: 2588 2589* [Patient](patient.html): A value in a phone contact 2590* [Person](person.html): A value in a phone contact 2591* [Practitioner](practitioner.html): A value in a phone contact 2592* [PractitionerRole](practitionerrole.html): A value in a phone contact 2593* [RelatedPerson](relatedperson.html): A value in a phone contact 2594</b><br> 2595 * Type: <b>token</b><br> 2596 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 2597 * </p> 2598 */ 2599 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 2600 2601 /** 2602 * Search parameter: <b>phonetic</b> 2603 * <p> 2604 * Description: <b>Multiple Resources: 2605 2606* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 2607* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 2608* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 2609* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 2610</b><br> 2611 * Type: <b>string</b><br> 2612 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 2613 * </p> 2614 */ 2615 @SearchParamDefinition(name="phonetic", path="Patient.name | Person.name | Practitioner.name | RelatedPerson.name", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [Person](person.html): A portion of name using some kind of phonetic matching algorithm\r\n* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm\r\n", type="string" ) 2616 public static final String SP_PHONETIC = "phonetic"; 2617 /** 2618 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 2619 * <p> 2620 * Description: <b>Multiple Resources: 2621 2622* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 2623* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 2624* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 2625* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 2626</b><br> 2627 * Type: <b>string</b><br> 2628 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 2629 * </p> 2630 */ 2631 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 2632 2633 /** 2634 * Search parameter: <b>telecom</b> 2635 * <p> 2636 * Description: <b>Multiple Resources: 2637 2638* [Patient](patient.html): The value in any kind of telecom details of the patient 2639* [Person](person.html): The value in any kind of contact 2640* [Practitioner](practitioner.html): The value in any kind of contact 2641* [PractitionerRole](practitionerrole.html): The value in any kind of contact 2642* [RelatedPerson](relatedperson.html): The value in any kind of contact 2643</b><br> 2644 * Type: <b>token</b><br> 2645 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 2646 * </p> 2647 */ 2648 @SearchParamDefinition(name="telecom", path="Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The value in any kind of telecom details of the patient\r\n* [Person](person.html): The value in any kind of contact\r\n* [Practitioner](practitioner.html): The value in any kind of contact\r\n* [PractitionerRole](practitionerrole.html): The value in any kind of contact\r\n* [RelatedPerson](relatedperson.html): The value in any kind of contact\r\n", type="token" ) 2649 public static final String SP_TELECOM = "telecom"; 2650 /** 2651 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2652 * <p> 2653 * Description: <b>Multiple Resources: 2654 2655* [Patient](patient.html): The value in any kind of telecom details of the patient 2656* [Person](person.html): The value in any kind of contact 2657* [Practitioner](practitioner.html): The value in any kind of contact 2658* [PractitionerRole](practitionerrole.html): The value in any kind of contact 2659* [RelatedPerson](relatedperson.html): The value in any kind of contact 2660</b><br> 2661 * Type: <b>token</b><br> 2662 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 2663 * </p> 2664 */ 2665 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 2666 2667 2668} 2669