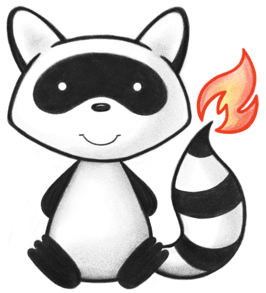
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical and non-clinical artifacts such as clinical decision support rules, order sets, protocols, and drug quality specifications. 052 */ 053@ResourceDef(name="PlanDefinition", profile="http://hl7.org/fhir/StructureDefinition/PlanDefinition") 054public class PlanDefinition extends MetadataResource { 055 056 @Block() 057 public static class PlanDefinitionGoalComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Indicates a category the goal falls within. 060 */ 061 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="E.g. Treatment, dietary, behavioral", formalDefinition="Indicates a category the goal falls within." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-category") 064 protected CodeableConcept category; 065 066 /** 067 * Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding". 068 */ 069 @Child(name = "description", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Code or text describing the goal", formalDefinition="Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\"." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 072 protected CodeableConcept description; 073 074 /** 075 * Identifies the expected level of importance associated with reaching/sustaining the defined goal. 076 */ 077 @Child(name = "priority", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 078 @Description(shortDefinition="high-priority | medium-priority | low-priority", formalDefinition="Identifies the expected level of importance associated with reaching/sustaining the defined goal." ) 079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-priority") 080 protected CodeableConcept priority; 081 082 /** 083 * The event after which the goal should begin being pursued. 084 */ 085 @Child(name = "start", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 086 @Description(shortDefinition="When goal pursuit begins", formalDefinition="The event after which the goal should begin being pursued." ) 087 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-start-event") 088 protected CodeableConcept start; 089 090 /** 091 * Identifies problems, conditions, issues, or concerns the goal is intended to address. 092 */ 093 @Child(name = "addresses", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 094 @Description(shortDefinition="What does the goal address", formalDefinition="Identifies problems, conditions, issues, or concerns the goal is intended to address." ) 095 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 096 protected List<CodeableConcept> addresses; 097 098 /** 099 * Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources. 100 */ 101 @Child(name = "documentation", type = {RelatedArtifact.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 102 @Description(shortDefinition="Supporting documentation for the goal", formalDefinition="Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources." ) 103 protected List<RelatedArtifact> documentation; 104 105 /** 106 * Indicates what should be done and within what timeframe. 107 */ 108 @Child(name = "target", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 109 @Description(shortDefinition="Target outcome for the goal", formalDefinition="Indicates what should be done and within what timeframe." ) 110 protected List<PlanDefinitionGoalTargetComponent> target; 111 112 private static final long serialVersionUID = -795308926L; 113 114 /** 115 * Constructor 116 */ 117 public PlanDefinitionGoalComponent() { 118 super(); 119 } 120 121 /** 122 * Constructor 123 */ 124 public PlanDefinitionGoalComponent(CodeableConcept description) { 125 super(); 126 this.setDescription(description); 127 } 128 129 /** 130 * @return {@link #category} (Indicates a category the goal falls within.) 131 */ 132 public CodeableConcept getCategory() { 133 if (this.category == null) 134 if (Configuration.errorOnAutoCreate()) 135 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.category"); 136 else if (Configuration.doAutoCreate()) 137 this.category = new CodeableConcept(); // cc 138 return this.category; 139 } 140 141 public boolean hasCategory() { 142 return this.category != null && !this.category.isEmpty(); 143 } 144 145 /** 146 * @param value {@link #category} (Indicates a category the goal falls within.) 147 */ 148 public PlanDefinitionGoalComponent setCategory(CodeableConcept value) { 149 this.category = value; 150 return this; 151 } 152 153 /** 154 * @return {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 155 */ 156 public CodeableConcept getDescription() { 157 if (this.description == null) 158 if (Configuration.errorOnAutoCreate()) 159 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.description"); 160 else if (Configuration.doAutoCreate()) 161 this.description = new CodeableConcept(); // cc 162 return this.description; 163 } 164 165 public boolean hasDescription() { 166 return this.description != null && !this.description.isEmpty(); 167 } 168 169 /** 170 * @param value {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 171 */ 172 public PlanDefinitionGoalComponent setDescription(CodeableConcept value) { 173 this.description = value; 174 return this; 175 } 176 177 /** 178 * @return {@link #priority} (Identifies the expected level of importance associated with reaching/sustaining the defined goal.) 179 */ 180 public CodeableConcept getPriority() { 181 if (this.priority == null) 182 if (Configuration.errorOnAutoCreate()) 183 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.priority"); 184 else if (Configuration.doAutoCreate()) 185 this.priority = new CodeableConcept(); // cc 186 return this.priority; 187 } 188 189 public boolean hasPriority() { 190 return this.priority != null && !this.priority.isEmpty(); 191 } 192 193 /** 194 * @param value {@link #priority} (Identifies the expected level of importance associated with reaching/sustaining the defined goal.) 195 */ 196 public PlanDefinitionGoalComponent setPriority(CodeableConcept value) { 197 this.priority = value; 198 return this; 199 } 200 201 /** 202 * @return {@link #start} (The event after which the goal should begin being pursued.) 203 */ 204 public CodeableConcept getStart() { 205 if (this.start == null) 206 if (Configuration.errorOnAutoCreate()) 207 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.start"); 208 else if (Configuration.doAutoCreate()) 209 this.start = new CodeableConcept(); // cc 210 return this.start; 211 } 212 213 public boolean hasStart() { 214 return this.start != null && !this.start.isEmpty(); 215 } 216 217 /** 218 * @param value {@link #start} (The event after which the goal should begin being pursued.) 219 */ 220 public PlanDefinitionGoalComponent setStart(CodeableConcept value) { 221 this.start = value; 222 return this; 223 } 224 225 /** 226 * @return {@link #addresses} (Identifies problems, conditions, issues, or concerns the goal is intended to address.) 227 */ 228 public List<CodeableConcept> getAddresses() { 229 if (this.addresses == null) 230 this.addresses = new ArrayList<CodeableConcept>(); 231 return this.addresses; 232 } 233 234 /** 235 * @return Returns a reference to <code>this</code> for easy method chaining 236 */ 237 public PlanDefinitionGoalComponent setAddresses(List<CodeableConcept> theAddresses) { 238 this.addresses = theAddresses; 239 return this; 240 } 241 242 public boolean hasAddresses() { 243 if (this.addresses == null) 244 return false; 245 for (CodeableConcept item : this.addresses) 246 if (!item.isEmpty()) 247 return true; 248 return false; 249 } 250 251 public CodeableConcept addAddresses() { //3 252 CodeableConcept t = new CodeableConcept(); 253 if (this.addresses == null) 254 this.addresses = new ArrayList<CodeableConcept>(); 255 this.addresses.add(t); 256 return t; 257 } 258 259 public PlanDefinitionGoalComponent addAddresses(CodeableConcept t) { //3 260 if (t == null) 261 return this; 262 if (this.addresses == null) 263 this.addresses = new ArrayList<CodeableConcept>(); 264 this.addresses.add(t); 265 return this; 266 } 267 268 /** 269 * @return The first repetition of repeating field {@link #addresses}, creating it if it does not already exist {3} 270 */ 271 public CodeableConcept getAddressesFirstRep() { 272 if (getAddresses().isEmpty()) { 273 addAddresses(); 274 } 275 return getAddresses().get(0); 276 } 277 278 /** 279 * @return {@link #documentation} (Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.) 280 */ 281 public List<RelatedArtifact> getDocumentation() { 282 if (this.documentation == null) 283 this.documentation = new ArrayList<RelatedArtifact>(); 284 return this.documentation; 285 } 286 287 /** 288 * @return Returns a reference to <code>this</code> for easy method chaining 289 */ 290 public PlanDefinitionGoalComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 291 this.documentation = theDocumentation; 292 return this; 293 } 294 295 public boolean hasDocumentation() { 296 if (this.documentation == null) 297 return false; 298 for (RelatedArtifact item : this.documentation) 299 if (!item.isEmpty()) 300 return true; 301 return false; 302 } 303 304 public RelatedArtifact addDocumentation() { //3 305 RelatedArtifact t = new RelatedArtifact(); 306 if (this.documentation == null) 307 this.documentation = new ArrayList<RelatedArtifact>(); 308 this.documentation.add(t); 309 return t; 310 } 311 312 public PlanDefinitionGoalComponent addDocumentation(RelatedArtifact t) { //3 313 if (t == null) 314 return this; 315 if (this.documentation == null) 316 this.documentation = new ArrayList<RelatedArtifact>(); 317 this.documentation.add(t); 318 return this; 319 } 320 321 /** 322 * @return The first repetition of repeating field {@link #documentation}, creating it if it does not already exist {3} 323 */ 324 public RelatedArtifact getDocumentationFirstRep() { 325 if (getDocumentation().isEmpty()) { 326 addDocumentation(); 327 } 328 return getDocumentation().get(0); 329 } 330 331 /** 332 * @return {@link #target} (Indicates what should be done and within what timeframe.) 333 */ 334 public List<PlanDefinitionGoalTargetComponent> getTarget() { 335 if (this.target == null) 336 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 337 return this.target; 338 } 339 340 /** 341 * @return Returns a reference to <code>this</code> for easy method chaining 342 */ 343 public PlanDefinitionGoalComponent setTarget(List<PlanDefinitionGoalTargetComponent> theTarget) { 344 this.target = theTarget; 345 return this; 346 } 347 348 public boolean hasTarget() { 349 if (this.target == null) 350 return false; 351 for (PlanDefinitionGoalTargetComponent item : this.target) 352 if (!item.isEmpty()) 353 return true; 354 return false; 355 } 356 357 public PlanDefinitionGoalTargetComponent addTarget() { //3 358 PlanDefinitionGoalTargetComponent t = new PlanDefinitionGoalTargetComponent(); 359 if (this.target == null) 360 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 361 this.target.add(t); 362 return t; 363 } 364 365 public PlanDefinitionGoalComponent addTarget(PlanDefinitionGoalTargetComponent t) { //3 366 if (t == null) 367 return this; 368 if (this.target == null) 369 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 370 this.target.add(t); 371 return this; 372 } 373 374 /** 375 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 376 */ 377 public PlanDefinitionGoalTargetComponent getTargetFirstRep() { 378 if (getTarget().isEmpty()) { 379 addTarget(); 380 } 381 return getTarget().get(0); 382 } 383 384 protected void listChildren(List<Property> children) { 385 super.listChildren(children); 386 children.add(new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1, category)); 387 children.add(new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description)); 388 children.add(new Property("priority", "CodeableConcept", "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, priority)); 389 children.add(new Property("start", "CodeableConcept", "The event after which the goal should begin being pursued.", 0, 1, start)); 390 children.add(new Property("addresses", "CodeableConcept", "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, java.lang.Integer.MAX_VALUE, addresses)); 391 children.add(new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation)); 392 children.add(new Property("target", "", "Indicates what should be done and within what timeframe.", 0, java.lang.Integer.MAX_VALUE, target)); 393 } 394 395 @Override 396 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 397 switch (_hash) { 398 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1, category); 399 case -1724546052: /*description*/ return new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description); 400 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, priority); 401 case 109757538: /*start*/ return new Property("start", "CodeableConcept", "The event after which the goal should begin being pursued.", 0, 1, start); 402 case 874544034: /*addresses*/ return new Property("addresses", "CodeableConcept", "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, java.lang.Integer.MAX_VALUE, addresses); 403 case 1587405498: /*documentation*/ return new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation); 404 case -880905839: /*target*/ return new Property("target", "", "Indicates what should be done and within what timeframe.", 0, java.lang.Integer.MAX_VALUE, target); 405 default: return super.getNamedProperty(_hash, _name, _checkValid); 406 } 407 408 } 409 410 @Override 411 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 412 switch (hash) { 413 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 414 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // CodeableConcept 415 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 416 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // CodeableConcept 417 case 874544034: /*addresses*/ return this.addresses == null ? new Base[0] : this.addresses.toArray(new Base[this.addresses.size()]); // CodeableConcept 418 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 419 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // PlanDefinitionGoalTargetComponent 420 default: return super.getProperty(hash, name, checkValid); 421 } 422 423 } 424 425 @Override 426 public Base setProperty(int hash, String name, Base value) throws FHIRException { 427 switch (hash) { 428 case 50511102: // category 429 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 430 return value; 431 case -1724546052: // description 432 this.description = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 433 return value; 434 case -1165461084: // priority 435 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 436 return value; 437 case 109757538: // start 438 this.start = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 439 return value; 440 case 874544034: // addresses 441 this.getAddresses().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 442 return value; 443 case 1587405498: // documentation 444 this.getDocumentation().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 445 return value; 446 case -880905839: // target 447 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); // PlanDefinitionGoalTargetComponent 448 return value; 449 default: return super.setProperty(hash, name, value); 450 } 451 452 } 453 454 @Override 455 public Base setProperty(String name, Base value) throws FHIRException { 456 if (name.equals("category")) { 457 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 458 } else if (name.equals("description")) { 459 this.description = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 460 } else if (name.equals("priority")) { 461 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 462 } else if (name.equals("start")) { 463 this.start = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 464 } else if (name.equals("addresses")) { 465 this.getAddresses().add(TypeConvertor.castToCodeableConcept(value)); 466 } else if (name.equals("documentation")) { 467 this.getDocumentation().add(TypeConvertor.castToRelatedArtifact(value)); 468 } else if (name.equals("target")) { 469 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); 470 } else 471 return super.setProperty(name, value); 472 return value; 473 } 474 475 @Override 476 public void removeChild(String name, Base value) throws FHIRException { 477 if (name.equals("category")) { 478 this.category = null; 479 } else if (name.equals("description")) { 480 this.description = null; 481 } else if (name.equals("priority")) { 482 this.priority = null; 483 } else if (name.equals("start")) { 484 this.start = null; 485 } else if (name.equals("addresses")) { 486 this.getAddresses().remove(value); 487 } else if (name.equals("documentation")) { 488 this.getDocumentation().remove(value); 489 } else if (name.equals("target")) { 490 this.getTarget().remove((PlanDefinitionGoalTargetComponent) value); 491 } else 492 super.removeChild(name, value); 493 494 } 495 496 @Override 497 public Base makeProperty(int hash, String name) throws FHIRException { 498 switch (hash) { 499 case 50511102: return getCategory(); 500 case -1724546052: return getDescription(); 501 case -1165461084: return getPriority(); 502 case 109757538: return getStart(); 503 case 874544034: return addAddresses(); 504 case 1587405498: return addDocumentation(); 505 case -880905839: return addTarget(); 506 default: return super.makeProperty(hash, name); 507 } 508 509 } 510 511 @Override 512 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 513 switch (hash) { 514 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 515 case -1724546052: /*description*/ return new String[] {"CodeableConcept"}; 516 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 517 case 109757538: /*start*/ return new String[] {"CodeableConcept"}; 518 case 874544034: /*addresses*/ return new String[] {"CodeableConcept"}; 519 case 1587405498: /*documentation*/ return new String[] {"RelatedArtifact"}; 520 case -880905839: /*target*/ return new String[] {}; 521 default: return super.getTypesForProperty(hash, name); 522 } 523 524 } 525 526 @Override 527 public Base addChild(String name) throws FHIRException { 528 if (name.equals("category")) { 529 this.category = new CodeableConcept(); 530 return this.category; 531 } 532 else if (name.equals("description")) { 533 this.description = new CodeableConcept(); 534 return this.description; 535 } 536 else if (name.equals("priority")) { 537 this.priority = new CodeableConcept(); 538 return this.priority; 539 } 540 else if (name.equals("start")) { 541 this.start = new CodeableConcept(); 542 return this.start; 543 } 544 else if (name.equals("addresses")) { 545 return addAddresses(); 546 } 547 else if (name.equals("documentation")) { 548 return addDocumentation(); 549 } 550 else if (name.equals("target")) { 551 return addTarget(); 552 } 553 else 554 return super.addChild(name); 555 } 556 557 public PlanDefinitionGoalComponent copy() { 558 PlanDefinitionGoalComponent dst = new PlanDefinitionGoalComponent(); 559 copyValues(dst); 560 return dst; 561 } 562 563 public void copyValues(PlanDefinitionGoalComponent dst) { 564 super.copyValues(dst); 565 dst.category = category == null ? null : category.copy(); 566 dst.description = description == null ? null : description.copy(); 567 dst.priority = priority == null ? null : priority.copy(); 568 dst.start = start == null ? null : start.copy(); 569 if (addresses != null) { 570 dst.addresses = new ArrayList<CodeableConcept>(); 571 for (CodeableConcept i : addresses) 572 dst.addresses.add(i.copy()); 573 }; 574 if (documentation != null) { 575 dst.documentation = new ArrayList<RelatedArtifact>(); 576 for (RelatedArtifact i : documentation) 577 dst.documentation.add(i.copy()); 578 }; 579 if (target != null) { 580 dst.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 581 for (PlanDefinitionGoalTargetComponent i : target) 582 dst.target.add(i.copy()); 583 }; 584 } 585 586 @Override 587 public boolean equalsDeep(Base other_) { 588 if (!super.equalsDeep(other_)) 589 return false; 590 if (!(other_ instanceof PlanDefinitionGoalComponent)) 591 return false; 592 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 593 return compareDeep(category, o.category, true) && compareDeep(description, o.description, true) 594 && compareDeep(priority, o.priority, true) && compareDeep(start, o.start, true) && compareDeep(addresses, o.addresses, true) 595 && compareDeep(documentation, o.documentation, true) && compareDeep(target, o.target, true); 596 } 597 598 @Override 599 public boolean equalsShallow(Base other_) { 600 if (!super.equalsShallow(other_)) 601 return false; 602 if (!(other_ instanceof PlanDefinitionGoalComponent)) 603 return false; 604 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 605 return true; 606 } 607 608 public boolean isEmpty() { 609 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, description, priority 610 , start, addresses, documentation, target); 611 } 612 613 public String fhirType() { 614 return "PlanDefinition.goal"; 615 616 } 617 618 } 619 620 @Block() 621 public static class PlanDefinitionGoalTargetComponent extends BackboneElement implements IBaseBackboneElement { 622 /** 623 * The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level. 624 */ 625 @Child(name = "measure", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 626 @Description(shortDefinition="The parameter whose value is to be tracked", formalDefinition="The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level." ) 627 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 628 protected CodeableConcept measure; 629 630 /** 631 * The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value. 632 */ 633 @Child(name = "detail", type = {Quantity.class, Range.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Ratio.class}, order=2, min=0, max=1, modifier=false, summary=false) 634 @Description(shortDefinition="The target value to be achieved", formalDefinition="The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value." ) 635 protected DataType detail; 636 637 /** 638 * Indicates the timeframe after the start of the goal in which the goal should be met. 639 */ 640 @Child(name = "due", type = {Duration.class}, order=3, min=0, max=1, modifier=false, summary=false) 641 @Description(shortDefinition="Reach goal within", formalDefinition="Indicates the timeframe after the start of the goal in which the goal should be met." ) 642 protected Duration due; 643 644 private static final long serialVersionUID = -1464475626L; 645 646 /** 647 * Constructor 648 */ 649 public PlanDefinitionGoalTargetComponent() { 650 super(); 651 } 652 653 /** 654 * @return {@link #measure} (The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.) 655 */ 656 public CodeableConcept getMeasure() { 657 if (this.measure == null) 658 if (Configuration.errorOnAutoCreate()) 659 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.measure"); 660 else if (Configuration.doAutoCreate()) 661 this.measure = new CodeableConcept(); // cc 662 return this.measure; 663 } 664 665 public boolean hasMeasure() { 666 return this.measure != null && !this.measure.isEmpty(); 667 } 668 669 /** 670 * @param value {@link #measure} (The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.) 671 */ 672 public PlanDefinitionGoalTargetComponent setMeasure(CodeableConcept value) { 673 this.measure = value; 674 return this; 675 } 676 677 /** 678 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 679 */ 680 public DataType getDetail() { 681 return this.detail; 682 } 683 684 /** 685 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 686 */ 687 public Quantity getDetailQuantity() throws FHIRException { 688 if (this.detail == null) 689 this.detail = new Quantity(); 690 if (!(this.detail instanceof Quantity)) 691 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.detail.getClass().getName()+" was encountered"); 692 return (Quantity) this.detail; 693 } 694 695 public boolean hasDetailQuantity() { 696 return this.detail instanceof Quantity; 697 } 698 699 /** 700 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 701 */ 702 public Range getDetailRange() throws FHIRException { 703 if (this.detail == null) 704 this.detail = new Range(); 705 if (!(this.detail instanceof Range)) 706 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.detail.getClass().getName()+" was encountered"); 707 return (Range) this.detail; 708 } 709 710 public boolean hasDetailRange() { 711 return this.detail instanceof Range; 712 } 713 714 /** 715 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 716 */ 717 public CodeableConcept getDetailCodeableConcept() throws FHIRException { 718 if (this.detail == null) 719 this.detail = new CodeableConcept(); 720 if (!(this.detail instanceof CodeableConcept)) 721 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.detail.getClass().getName()+" was encountered"); 722 return (CodeableConcept) this.detail; 723 } 724 725 public boolean hasDetailCodeableConcept() { 726 return this.detail instanceof CodeableConcept; 727 } 728 729 /** 730 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 731 */ 732 public StringType getDetailStringType() throws FHIRException { 733 if (this.detail == null) 734 this.detail = new StringType(); 735 if (!(this.detail instanceof StringType)) 736 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.detail.getClass().getName()+" was encountered"); 737 return (StringType) this.detail; 738 } 739 740 public boolean hasDetailStringType() { 741 return this.detail instanceof StringType; 742 } 743 744 /** 745 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 746 */ 747 public BooleanType getDetailBooleanType() throws FHIRException { 748 if (this.detail == null) 749 this.detail = new BooleanType(); 750 if (!(this.detail instanceof BooleanType)) 751 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.detail.getClass().getName()+" was encountered"); 752 return (BooleanType) this.detail; 753 } 754 755 public boolean hasDetailBooleanType() { 756 return this.detail instanceof BooleanType; 757 } 758 759 /** 760 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 761 */ 762 public IntegerType getDetailIntegerType() throws FHIRException { 763 if (this.detail == null) 764 this.detail = new IntegerType(); 765 if (!(this.detail instanceof IntegerType)) 766 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.detail.getClass().getName()+" was encountered"); 767 return (IntegerType) this.detail; 768 } 769 770 public boolean hasDetailIntegerType() { 771 return this.detail instanceof IntegerType; 772 } 773 774 /** 775 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 776 */ 777 public Ratio getDetailRatio() throws FHIRException { 778 if (this.detail == null) 779 this.detail = new Ratio(); 780 if (!(this.detail instanceof Ratio)) 781 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.detail.getClass().getName()+" was encountered"); 782 return (Ratio) this.detail; 783 } 784 785 public boolean hasDetailRatio() { 786 return this.detail instanceof Ratio; 787 } 788 789 public boolean hasDetail() { 790 return this.detail != null && !this.detail.isEmpty(); 791 } 792 793 /** 794 * @param value {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 795 */ 796 public PlanDefinitionGoalTargetComponent setDetail(DataType value) { 797 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Ratio)) 798 throw new FHIRException("Not the right type for PlanDefinition.goal.target.detail[x]: "+value.fhirType()); 799 this.detail = value; 800 return this; 801 } 802 803 /** 804 * @return {@link #due} (Indicates the timeframe after the start of the goal in which the goal should be met.) 805 */ 806 public Duration getDue() { 807 if (this.due == null) 808 if (Configuration.errorOnAutoCreate()) 809 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.due"); 810 else if (Configuration.doAutoCreate()) 811 this.due = new Duration(); // cc 812 return this.due; 813 } 814 815 public boolean hasDue() { 816 return this.due != null && !this.due.isEmpty(); 817 } 818 819 /** 820 * @param value {@link #due} (Indicates the timeframe after the start of the goal in which the goal should be met.) 821 */ 822 public PlanDefinitionGoalTargetComponent setDue(Duration value) { 823 this.due = value; 824 return this; 825 } 826 827 protected void listChildren(List<Property> children) { 828 super.listChildren(children); 829 children.add(new Property("measure", "CodeableConcept", "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 1, measure)); 830 children.add(new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail)); 831 children.add(new Property("due", "Duration", "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due)); 832 } 833 834 @Override 835 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 836 switch (_hash) { 837 case 938321246: /*measure*/ return new Property("measure", "CodeableConcept", "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 1, measure); 838 case -1973084529: /*detail[x]*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 839 case -1335224239: /*detail*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 840 case -1313079300: /*detailQuantity*/ return new Property("detail[x]", "Quantity", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 841 case -2062632084: /*detailRange*/ return new Property("detail[x]", "Range", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 842 case -175586544: /*detailCodeableConcept*/ return new Property("detail[x]", "CodeableConcept", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 843 case 529212354: /*detailString*/ return new Property("detail[x]", "string", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 844 case 1172184727: /*detailBoolean*/ return new Property("detail[x]", "boolean", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 845 case -1229442131: /*detailInteger*/ return new Property("detail[x]", "integer", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 846 case -2062626246: /*detailRatio*/ return new Property("detail[x]", "Ratio", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%, or in the case of pharmaceutical quality - NMT 0.6%, Clear solution, etc. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 847 case 99828: /*due*/ return new Property("due", "Duration", "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due); 848 default: return super.getNamedProperty(_hash, _name, _checkValid); 849 } 850 851 } 852 853 @Override 854 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 855 switch (hash) { 856 case 938321246: /*measure*/ return this.measure == null ? new Base[0] : new Base[] {this.measure}; // CodeableConcept 857 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // DataType 858 case 99828: /*due*/ return this.due == null ? new Base[0] : new Base[] {this.due}; // Duration 859 default: return super.getProperty(hash, name, checkValid); 860 } 861 862 } 863 864 @Override 865 public Base setProperty(int hash, String name, Base value) throws FHIRException { 866 switch (hash) { 867 case 938321246: // measure 868 this.measure = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 869 return value; 870 case -1335224239: // detail 871 this.detail = TypeConvertor.castToType(value); // DataType 872 return value; 873 case 99828: // due 874 this.due = TypeConvertor.castToDuration(value); // Duration 875 return value; 876 default: return super.setProperty(hash, name, value); 877 } 878 879 } 880 881 @Override 882 public Base setProperty(String name, Base value) throws FHIRException { 883 if (name.equals("measure")) { 884 this.measure = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 885 } else if (name.equals("detail[x]")) { 886 this.detail = TypeConvertor.castToType(value); // DataType 887 } else if (name.equals("due")) { 888 this.due = TypeConvertor.castToDuration(value); // Duration 889 } else 890 return super.setProperty(name, value); 891 return value; 892 } 893 894 @Override 895 public void removeChild(String name, Base value) throws FHIRException { 896 if (name.equals("measure")) { 897 this.measure = null; 898 } else if (name.equals("detail[x]")) { 899 this.detail = null; 900 } else if (name.equals("due")) { 901 this.due = null; 902 } else 903 super.removeChild(name, value); 904 905 } 906 907 @Override 908 public Base makeProperty(int hash, String name) throws FHIRException { 909 switch (hash) { 910 case 938321246: return getMeasure(); 911 case -1973084529: return getDetail(); 912 case -1335224239: return getDetail(); 913 case 99828: return getDue(); 914 default: return super.makeProperty(hash, name); 915 } 916 917 } 918 919 @Override 920 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 921 switch (hash) { 922 case 938321246: /*measure*/ return new String[] {"CodeableConcept"}; 923 case -1335224239: /*detail*/ return new String[] {"Quantity", "Range", "CodeableConcept", "string", "boolean", "integer", "Ratio"}; 924 case 99828: /*due*/ return new String[] {"Duration"}; 925 default: return super.getTypesForProperty(hash, name); 926 } 927 928 } 929 930 @Override 931 public Base addChild(String name) throws FHIRException { 932 if (name.equals("measure")) { 933 this.measure = new CodeableConcept(); 934 return this.measure; 935 } 936 else if (name.equals("detailQuantity")) { 937 this.detail = new Quantity(); 938 return this.detail; 939 } 940 else if (name.equals("detailRange")) { 941 this.detail = new Range(); 942 return this.detail; 943 } 944 else if (name.equals("detailCodeableConcept")) { 945 this.detail = new CodeableConcept(); 946 return this.detail; 947 } 948 else if (name.equals("detailString")) { 949 this.detail = new StringType(); 950 return this.detail; 951 } 952 else if (name.equals("detailBoolean")) { 953 this.detail = new BooleanType(); 954 return this.detail; 955 } 956 else if (name.equals("detailInteger")) { 957 this.detail = new IntegerType(); 958 return this.detail; 959 } 960 else if (name.equals("detailRatio")) { 961 this.detail = new Ratio(); 962 return this.detail; 963 } 964 else if (name.equals("due")) { 965 this.due = new Duration(); 966 return this.due; 967 } 968 else 969 return super.addChild(name); 970 } 971 972 public PlanDefinitionGoalTargetComponent copy() { 973 PlanDefinitionGoalTargetComponent dst = new PlanDefinitionGoalTargetComponent(); 974 copyValues(dst); 975 return dst; 976 } 977 978 public void copyValues(PlanDefinitionGoalTargetComponent dst) { 979 super.copyValues(dst); 980 dst.measure = measure == null ? null : measure.copy(); 981 dst.detail = detail == null ? null : detail.copy(); 982 dst.due = due == null ? null : due.copy(); 983 } 984 985 @Override 986 public boolean equalsDeep(Base other_) { 987 if (!super.equalsDeep(other_)) 988 return false; 989 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 990 return false; 991 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 992 return compareDeep(measure, o.measure, true) && compareDeep(detail, o.detail, true) && compareDeep(due, o.due, true) 993 ; 994 } 995 996 @Override 997 public boolean equalsShallow(Base other_) { 998 if (!super.equalsShallow(other_)) 999 return false; 1000 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 1001 return false; 1002 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 1003 return true; 1004 } 1005 1006 public boolean isEmpty() { 1007 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(measure, detail, due); 1008 } 1009 1010 public String fhirType() { 1011 return "PlanDefinition.goal.target"; 1012 1013 } 1014 1015 } 1016 1017 @Block() 1018 public static class PlanDefinitionActorComponent extends BackboneElement implements IBaseBackboneElement { 1019 /** 1020 * A descriptive label for the actor. 1021 */ 1022 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1023 @Description(shortDefinition="User-visible title", formalDefinition="A descriptive label for the actor." ) 1024 protected StringType title; 1025 1026 /** 1027 * A description of how the actor fits into the overall actions of the plan definition. 1028 */ 1029 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1030 @Description(shortDefinition="Describes the actor", formalDefinition="A description of how the actor fits into the overall actions of the plan definition." ) 1031 protected MarkdownType description; 1032 1033 /** 1034 * The characteristics of the candidates that could serve as the actor. 1035 */ 1036 @Child(name = "option", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1037 @Description(shortDefinition="Who or what can be this actor", formalDefinition="The characteristics of the candidates that could serve as the actor." ) 1038 protected List<PlanDefinitionActorOptionComponent> option; 1039 1040 private static final long serialVersionUID = -571302300L; 1041 1042 /** 1043 * Constructor 1044 */ 1045 public PlanDefinitionActorComponent() { 1046 super(); 1047 } 1048 1049 /** 1050 * Constructor 1051 */ 1052 public PlanDefinitionActorComponent(PlanDefinitionActorOptionComponent option) { 1053 super(); 1054 this.addOption(option); 1055 } 1056 1057 /** 1058 * @return {@link #title} (A descriptive label for the actor.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1059 */ 1060 public StringType getTitleElement() { 1061 if (this.title == null) 1062 if (Configuration.errorOnAutoCreate()) 1063 throw new Error("Attempt to auto-create PlanDefinitionActorComponent.title"); 1064 else if (Configuration.doAutoCreate()) 1065 this.title = new StringType(); // bb 1066 return this.title; 1067 } 1068 1069 public boolean hasTitleElement() { 1070 return this.title != null && !this.title.isEmpty(); 1071 } 1072 1073 public boolean hasTitle() { 1074 return this.title != null && !this.title.isEmpty(); 1075 } 1076 1077 /** 1078 * @param value {@link #title} (A descriptive label for the actor.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1079 */ 1080 public PlanDefinitionActorComponent setTitleElement(StringType value) { 1081 this.title = value; 1082 return this; 1083 } 1084 1085 /** 1086 * @return A descriptive label for the actor. 1087 */ 1088 public String getTitle() { 1089 return this.title == null ? null : this.title.getValue(); 1090 } 1091 1092 /** 1093 * @param value A descriptive label for the actor. 1094 */ 1095 public PlanDefinitionActorComponent setTitle(String value) { 1096 if (Utilities.noString(value)) 1097 this.title = null; 1098 else { 1099 if (this.title == null) 1100 this.title = new StringType(); 1101 this.title.setValue(value); 1102 } 1103 return this; 1104 } 1105 1106 /** 1107 * @return {@link #description} (A description of how the actor fits into the overall actions of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1108 */ 1109 public MarkdownType getDescriptionElement() { 1110 if (this.description == null) 1111 if (Configuration.errorOnAutoCreate()) 1112 throw new Error("Attempt to auto-create PlanDefinitionActorComponent.description"); 1113 else if (Configuration.doAutoCreate()) 1114 this.description = new MarkdownType(); // bb 1115 return this.description; 1116 } 1117 1118 public boolean hasDescriptionElement() { 1119 return this.description != null && !this.description.isEmpty(); 1120 } 1121 1122 public boolean hasDescription() { 1123 return this.description != null && !this.description.isEmpty(); 1124 } 1125 1126 /** 1127 * @param value {@link #description} (A description of how the actor fits into the overall actions of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1128 */ 1129 public PlanDefinitionActorComponent setDescriptionElement(MarkdownType value) { 1130 this.description = value; 1131 return this; 1132 } 1133 1134 /** 1135 * @return A description of how the actor fits into the overall actions of the plan definition. 1136 */ 1137 public String getDescription() { 1138 return this.description == null ? null : this.description.getValue(); 1139 } 1140 1141 /** 1142 * @param value A description of how the actor fits into the overall actions of the plan definition. 1143 */ 1144 public PlanDefinitionActorComponent setDescription(String value) { 1145 if (Utilities.noString(value)) 1146 this.description = null; 1147 else { 1148 if (this.description == null) 1149 this.description = new MarkdownType(); 1150 this.description.setValue(value); 1151 } 1152 return this; 1153 } 1154 1155 /** 1156 * @return {@link #option} (The characteristics of the candidates that could serve as the actor.) 1157 */ 1158 public List<PlanDefinitionActorOptionComponent> getOption() { 1159 if (this.option == null) 1160 this.option = new ArrayList<PlanDefinitionActorOptionComponent>(); 1161 return this.option; 1162 } 1163 1164 /** 1165 * @return Returns a reference to <code>this</code> for easy method chaining 1166 */ 1167 public PlanDefinitionActorComponent setOption(List<PlanDefinitionActorOptionComponent> theOption) { 1168 this.option = theOption; 1169 return this; 1170 } 1171 1172 public boolean hasOption() { 1173 if (this.option == null) 1174 return false; 1175 for (PlanDefinitionActorOptionComponent item : this.option) 1176 if (!item.isEmpty()) 1177 return true; 1178 return false; 1179 } 1180 1181 public PlanDefinitionActorOptionComponent addOption() { //3 1182 PlanDefinitionActorOptionComponent t = new PlanDefinitionActorOptionComponent(); 1183 if (this.option == null) 1184 this.option = new ArrayList<PlanDefinitionActorOptionComponent>(); 1185 this.option.add(t); 1186 return t; 1187 } 1188 1189 public PlanDefinitionActorComponent addOption(PlanDefinitionActorOptionComponent t) { //3 1190 if (t == null) 1191 return this; 1192 if (this.option == null) 1193 this.option = new ArrayList<PlanDefinitionActorOptionComponent>(); 1194 this.option.add(t); 1195 return this; 1196 } 1197 1198 /** 1199 * @return The first repetition of repeating field {@link #option}, creating it if it does not already exist {3} 1200 */ 1201 public PlanDefinitionActorOptionComponent getOptionFirstRep() { 1202 if (getOption().isEmpty()) { 1203 addOption(); 1204 } 1205 return getOption().get(0); 1206 } 1207 1208 protected void listChildren(List<Property> children) { 1209 super.listChildren(children); 1210 children.add(new Property("title", "string", "A descriptive label for the actor.", 0, 1, title)); 1211 children.add(new Property("description", "markdown", "A description of how the actor fits into the overall actions of the plan definition.", 0, 1, description)); 1212 children.add(new Property("option", "", "The characteristics of the candidates that could serve as the actor.", 0, java.lang.Integer.MAX_VALUE, option)); 1213 } 1214 1215 @Override 1216 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1217 switch (_hash) { 1218 case 110371416: /*title*/ return new Property("title", "string", "A descriptive label for the actor.", 0, 1, title); 1219 case -1724546052: /*description*/ return new Property("description", "markdown", "A description of how the actor fits into the overall actions of the plan definition.", 0, 1, description); 1220 case -1010136971: /*option*/ return new Property("option", "", "The characteristics of the candidates that could serve as the actor.", 0, java.lang.Integer.MAX_VALUE, option); 1221 default: return super.getNamedProperty(_hash, _name, _checkValid); 1222 } 1223 1224 } 1225 1226 @Override 1227 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1228 switch (hash) { 1229 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1230 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1231 case -1010136971: /*option*/ return this.option == null ? new Base[0] : this.option.toArray(new Base[this.option.size()]); // PlanDefinitionActorOptionComponent 1232 default: return super.getProperty(hash, name, checkValid); 1233 } 1234 1235 } 1236 1237 @Override 1238 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1239 switch (hash) { 1240 case 110371416: // title 1241 this.title = TypeConvertor.castToString(value); // StringType 1242 return value; 1243 case -1724546052: // description 1244 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1245 return value; 1246 case -1010136971: // option 1247 this.getOption().add((PlanDefinitionActorOptionComponent) value); // PlanDefinitionActorOptionComponent 1248 return value; 1249 default: return super.setProperty(hash, name, value); 1250 } 1251 1252 } 1253 1254 @Override 1255 public Base setProperty(String name, Base value) throws FHIRException { 1256 if (name.equals("title")) { 1257 this.title = TypeConvertor.castToString(value); // StringType 1258 } else if (name.equals("description")) { 1259 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1260 } else if (name.equals("option")) { 1261 this.getOption().add((PlanDefinitionActorOptionComponent) value); 1262 } else 1263 return super.setProperty(name, value); 1264 return value; 1265 } 1266 1267 @Override 1268 public void removeChild(String name, Base value) throws FHIRException { 1269 if (name.equals("title")) { 1270 this.title = null; 1271 } else if (name.equals("description")) { 1272 this.description = null; 1273 } else if (name.equals("option")) { 1274 this.getOption().remove((PlanDefinitionActorOptionComponent) value); 1275 } else 1276 super.removeChild(name, value); 1277 1278 } 1279 1280 @Override 1281 public Base makeProperty(int hash, String name) throws FHIRException { 1282 switch (hash) { 1283 case 110371416: return getTitleElement(); 1284 case -1724546052: return getDescriptionElement(); 1285 case -1010136971: return addOption(); 1286 default: return super.makeProperty(hash, name); 1287 } 1288 1289 } 1290 1291 @Override 1292 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1293 switch (hash) { 1294 case 110371416: /*title*/ return new String[] {"string"}; 1295 case -1724546052: /*description*/ return new String[] {"markdown"}; 1296 case -1010136971: /*option*/ return new String[] {}; 1297 default: return super.getTypesForProperty(hash, name); 1298 } 1299 1300 } 1301 1302 @Override 1303 public Base addChild(String name) throws FHIRException { 1304 if (name.equals("title")) { 1305 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.actor.title"); 1306 } 1307 else if (name.equals("description")) { 1308 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.actor.description"); 1309 } 1310 else if (name.equals("option")) { 1311 return addOption(); 1312 } 1313 else 1314 return super.addChild(name); 1315 } 1316 1317 public PlanDefinitionActorComponent copy() { 1318 PlanDefinitionActorComponent dst = new PlanDefinitionActorComponent(); 1319 copyValues(dst); 1320 return dst; 1321 } 1322 1323 public void copyValues(PlanDefinitionActorComponent dst) { 1324 super.copyValues(dst); 1325 dst.title = title == null ? null : title.copy(); 1326 dst.description = description == null ? null : description.copy(); 1327 if (option != null) { 1328 dst.option = new ArrayList<PlanDefinitionActorOptionComponent>(); 1329 for (PlanDefinitionActorOptionComponent i : option) 1330 dst.option.add(i.copy()); 1331 }; 1332 } 1333 1334 @Override 1335 public boolean equalsDeep(Base other_) { 1336 if (!super.equalsDeep(other_)) 1337 return false; 1338 if (!(other_ instanceof PlanDefinitionActorComponent)) 1339 return false; 1340 PlanDefinitionActorComponent o = (PlanDefinitionActorComponent) other_; 1341 return compareDeep(title, o.title, true) && compareDeep(description, o.description, true) && compareDeep(option, o.option, true) 1342 ; 1343 } 1344 1345 @Override 1346 public boolean equalsShallow(Base other_) { 1347 if (!super.equalsShallow(other_)) 1348 return false; 1349 if (!(other_ instanceof PlanDefinitionActorComponent)) 1350 return false; 1351 PlanDefinitionActorComponent o = (PlanDefinitionActorComponent) other_; 1352 return compareValues(title, o.title, true) && compareValues(description, o.description, true); 1353 } 1354 1355 public boolean isEmpty() { 1356 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, description, option 1357 ); 1358 } 1359 1360 public String fhirType() { 1361 return "PlanDefinition.actor"; 1362 1363 } 1364 1365 } 1366 1367 @Block() 1368 public static class PlanDefinitionActorOptionComponent extends BackboneElement implements IBaseBackboneElement { 1369 /** 1370 * The type of participant in the action. 1371 */ 1372 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1373 @Description(shortDefinition="careteam | device | group | healthcareservice | location | organization | patient | practitioner | practitionerrole | relatedperson", formalDefinition="The type of participant in the action." ) 1374 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-type") 1375 protected Enumeration<ActionParticipantType> type; 1376 1377 /** 1378 * The type of participant in the action. 1379 */ 1380 @Child(name = "typeCanonical", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1381 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 1382 protected CanonicalType typeCanonical; 1383 1384 /** 1385 * The type of participant in the action. 1386 */ 1387 @Child(name = "typeReference", type = {CareTeam.class, Device.class, DeviceDefinition.class, Endpoint.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=false) 1388 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 1389 protected Reference typeReference; 1390 1391 /** 1392 * The role the participant should play in performing the described action. 1393 */ 1394 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1395 @Description(shortDefinition="E.g. Nurse, Surgeon, Parent", formalDefinition="The role the participant should play in performing the described action." ) 1396 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/action-participant-role") 1397 protected CodeableConcept role; 1398 1399 private static final long serialVersionUID = 1881639157L; 1400 1401 /** 1402 * Constructor 1403 */ 1404 public PlanDefinitionActorOptionComponent() { 1405 super(); 1406 } 1407 1408 /** 1409 * @return {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1410 */ 1411 public Enumeration<ActionParticipantType> getTypeElement() { 1412 if (this.type == null) 1413 if (Configuration.errorOnAutoCreate()) 1414 throw new Error("Attempt to auto-create PlanDefinitionActorOptionComponent.type"); 1415 else if (Configuration.doAutoCreate()) 1416 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); // bb 1417 return this.type; 1418 } 1419 1420 public boolean hasTypeElement() { 1421 return this.type != null && !this.type.isEmpty(); 1422 } 1423 1424 public boolean hasType() { 1425 return this.type != null && !this.type.isEmpty(); 1426 } 1427 1428 /** 1429 * @param value {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1430 */ 1431 public PlanDefinitionActorOptionComponent setTypeElement(Enumeration<ActionParticipantType> value) { 1432 this.type = value; 1433 return this; 1434 } 1435 1436 /** 1437 * @return The type of participant in the action. 1438 */ 1439 public ActionParticipantType getType() { 1440 return this.type == null ? null : this.type.getValue(); 1441 } 1442 1443 /** 1444 * @param value The type of participant in the action. 1445 */ 1446 public PlanDefinitionActorOptionComponent setType(ActionParticipantType value) { 1447 if (value == null) 1448 this.type = null; 1449 else { 1450 if (this.type == null) 1451 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); 1452 this.type.setValue(value); 1453 } 1454 return this; 1455 } 1456 1457 /** 1458 * @return {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 1459 */ 1460 public CanonicalType getTypeCanonicalElement() { 1461 if (this.typeCanonical == null) 1462 if (Configuration.errorOnAutoCreate()) 1463 throw new Error("Attempt to auto-create PlanDefinitionActorOptionComponent.typeCanonical"); 1464 else if (Configuration.doAutoCreate()) 1465 this.typeCanonical = new CanonicalType(); // bb 1466 return this.typeCanonical; 1467 } 1468 1469 public boolean hasTypeCanonicalElement() { 1470 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 1471 } 1472 1473 public boolean hasTypeCanonical() { 1474 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 1475 } 1476 1477 /** 1478 * @param value {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 1479 */ 1480 public PlanDefinitionActorOptionComponent setTypeCanonicalElement(CanonicalType value) { 1481 this.typeCanonical = value; 1482 return this; 1483 } 1484 1485 /** 1486 * @return The type of participant in the action. 1487 */ 1488 public String getTypeCanonical() { 1489 return this.typeCanonical == null ? null : this.typeCanonical.getValue(); 1490 } 1491 1492 /** 1493 * @param value The type of participant in the action. 1494 */ 1495 public PlanDefinitionActorOptionComponent setTypeCanonical(String value) { 1496 if (Utilities.noString(value)) 1497 this.typeCanonical = null; 1498 else { 1499 if (this.typeCanonical == null) 1500 this.typeCanonical = new CanonicalType(); 1501 this.typeCanonical.setValue(value); 1502 } 1503 return this; 1504 } 1505 1506 /** 1507 * @return {@link #typeReference} (The type of participant in the action.) 1508 */ 1509 public Reference getTypeReference() { 1510 if (this.typeReference == null) 1511 if (Configuration.errorOnAutoCreate()) 1512 throw new Error("Attempt to auto-create PlanDefinitionActorOptionComponent.typeReference"); 1513 else if (Configuration.doAutoCreate()) 1514 this.typeReference = new Reference(); // cc 1515 return this.typeReference; 1516 } 1517 1518 public boolean hasTypeReference() { 1519 return this.typeReference != null && !this.typeReference.isEmpty(); 1520 } 1521 1522 /** 1523 * @param value {@link #typeReference} (The type of participant in the action.) 1524 */ 1525 public PlanDefinitionActorOptionComponent setTypeReference(Reference value) { 1526 this.typeReference = value; 1527 return this; 1528 } 1529 1530 /** 1531 * @return {@link #role} (The role the participant should play in performing the described action.) 1532 */ 1533 public CodeableConcept getRole() { 1534 if (this.role == null) 1535 if (Configuration.errorOnAutoCreate()) 1536 throw new Error("Attempt to auto-create PlanDefinitionActorOptionComponent.role"); 1537 else if (Configuration.doAutoCreate()) 1538 this.role = new CodeableConcept(); // cc 1539 return this.role; 1540 } 1541 1542 public boolean hasRole() { 1543 return this.role != null && !this.role.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #role} (The role the participant should play in performing the described action.) 1548 */ 1549 public PlanDefinitionActorOptionComponent setRole(CodeableConcept value) { 1550 this.role = value; 1551 return this; 1552 } 1553 1554 protected void listChildren(List<Property> children) { 1555 super.listChildren(children); 1556 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 1557 children.add(new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical)); 1558 children.add(new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference)); 1559 children.add(new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role)); 1560 } 1561 1562 @Override 1563 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1564 switch (_hash) { 1565 case 3575610: /*type*/ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 1566 case -466635046: /*typeCanonical*/ return new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical); 1567 case 2074825009: /*typeReference*/ return new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference); 1568 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role); 1569 default: return super.getNamedProperty(_hash, _name, _checkValid); 1570 } 1571 1572 } 1573 1574 @Override 1575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1576 switch (hash) { 1577 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ActionParticipantType> 1578 case -466635046: /*typeCanonical*/ return this.typeCanonical == null ? new Base[0] : new Base[] {this.typeCanonical}; // CanonicalType 1579 case 2074825009: /*typeReference*/ return this.typeReference == null ? new Base[0] : new Base[] {this.typeReference}; // Reference 1580 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1581 default: return super.getProperty(hash, name, checkValid); 1582 } 1583 1584 } 1585 1586 @Override 1587 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1588 switch (hash) { 1589 case 3575610: // type 1590 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1591 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 1592 return value; 1593 case -466635046: // typeCanonical 1594 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 1595 return value; 1596 case 2074825009: // typeReference 1597 this.typeReference = TypeConvertor.castToReference(value); // Reference 1598 return value; 1599 case 3506294: // role 1600 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1601 return value; 1602 default: return super.setProperty(hash, name, value); 1603 } 1604 1605 } 1606 1607 @Override 1608 public Base setProperty(String name, Base value) throws FHIRException { 1609 if (name.equals("type")) { 1610 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1611 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 1612 } else if (name.equals("typeCanonical")) { 1613 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 1614 } else if (name.equals("typeReference")) { 1615 this.typeReference = TypeConvertor.castToReference(value); // Reference 1616 } else if (name.equals("role")) { 1617 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1618 } else 1619 return super.setProperty(name, value); 1620 return value; 1621 } 1622 1623 @Override 1624 public void removeChild(String name, Base value) throws FHIRException { 1625 if (name.equals("type")) { 1626 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1627 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 1628 } else if (name.equals("typeCanonical")) { 1629 this.typeCanonical = null; 1630 } else if (name.equals("typeReference")) { 1631 this.typeReference = null; 1632 } else if (name.equals("role")) { 1633 this.role = null; 1634 } else 1635 super.removeChild(name, value); 1636 1637 } 1638 1639 @Override 1640 public Base makeProperty(int hash, String name) throws FHIRException { 1641 switch (hash) { 1642 case 3575610: return getTypeElement(); 1643 case -466635046: return getTypeCanonicalElement(); 1644 case 2074825009: return getTypeReference(); 1645 case 3506294: return getRole(); 1646 default: return super.makeProperty(hash, name); 1647 } 1648 1649 } 1650 1651 @Override 1652 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1653 switch (hash) { 1654 case 3575610: /*type*/ return new String[] {"code"}; 1655 case -466635046: /*typeCanonical*/ return new String[] {"canonical"}; 1656 case 2074825009: /*typeReference*/ return new String[] {"Reference"}; 1657 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1658 default: return super.getTypesForProperty(hash, name); 1659 } 1660 1661 } 1662 1663 @Override 1664 public Base addChild(String name) throws FHIRException { 1665 if (name.equals("type")) { 1666 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.actor.option.type"); 1667 } 1668 else if (name.equals("typeCanonical")) { 1669 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.actor.option.typeCanonical"); 1670 } 1671 else if (name.equals("typeReference")) { 1672 this.typeReference = new Reference(); 1673 return this.typeReference; 1674 } 1675 else if (name.equals("role")) { 1676 this.role = new CodeableConcept(); 1677 return this.role; 1678 } 1679 else 1680 return super.addChild(name); 1681 } 1682 1683 public PlanDefinitionActorOptionComponent copy() { 1684 PlanDefinitionActorOptionComponent dst = new PlanDefinitionActorOptionComponent(); 1685 copyValues(dst); 1686 return dst; 1687 } 1688 1689 public void copyValues(PlanDefinitionActorOptionComponent dst) { 1690 super.copyValues(dst); 1691 dst.type = type == null ? null : type.copy(); 1692 dst.typeCanonical = typeCanonical == null ? null : typeCanonical.copy(); 1693 dst.typeReference = typeReference == null ? null : typeReference.copy(); 1694 dst.role = role == null ? null : role.copy(); 1695 } 1696 1697 @Override 1698 public boolean equalsDeep(Base other_) { 1699 if (!super.equalsDeep(other_)) 1700 return false; 1701 if (!(other_ instanceof PlanDefinitionActorOptionComponent)) 1702 return false; 1703 PlanDefinitionActorOptionComponent o = (PlanDefinitionActorOptionComponent) other_; 1704 return compareDeep(type, o.type, true) && compareDeep(typeCanonical, o.typeCanonical, true) && compareDeep(typeReference, o.typeReference, true) 1705 && compareDeep(role, o.role, true); 1706 } 1707 1708 @Override 1709 public boolean equalsShallow(Base other_) { 1710 if (!super.equalsShallow(other_)) 1711 return false; 1712 if (!(other_ instanceof PlanDefinitionActorOptionComponent)) 1713 return false; 1714 PlanDefinitionActorOptionComponent o = (PlanDefinitionActorOptionComponent) other_; 1715 return compareValues(type, o.type, true) && compareValues(typeCanonical, o.typeCanonical, true); 1716 } 1717 1718 public boolean isEmpty() { 1719 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, typeCanonical, typeReference 1720 , role); 1721 } 1722 1723 public String fhirType() { 1724 return "PlanDefinition.actor.option"; 1725 1726 } 1727 1728 } 1729 1730 @Block() 1731 public static class PlanDefinitionActionComponent extends BackboneElement implements IBaseBackboneElement { 1732 /** 1733 * An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration. 1734 */ 1735 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1736 @Description(shortDefinition="Unique id for the action in the PlanDefinition", formalDefinition="An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration." ) 1737 protected StringType linkId; 1738 1739 /** 1740 * A user-visible prefix for the action. For example a section or item numbering such as 1. or A. 1741 */ 1742 @Child(name = "prefix", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1743 @Description(shortDefinition="User-visible prefix for the action (e.g. 1. or A.)", formalDefinition="A user-visible prefix for the action. For example a section or item numbering such as 1. or A." ) 1744 protected StringType prefix; 1745 1746 /** 1747 * The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC. 1748 */ 1749 @Child(name = "title", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1750 @Description(shortDefinition="User-visible title", formalDefinition="The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC." ) 1751 protected StringType title; 1752 1753 /** 1754 * A brief description of the action used to provide a summary to display to the user. 1755 */ 1756 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1757 @Description(shortDefinition="Brief description of the action", formalDefinition="A brief description of the action used to provide a summary to display to the user." ) 1758 protected MarkdownType description; 1759 1760 /** 1761 * A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically. 1762 */ 1763 @Child(name = "textEquivalent", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1764 @Description(shortDefinition="Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition="A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically." ) 1765 protected MarkdownType textEquivalent; 1766 1767 /** 1768 * Indicates how quickly the action should be addressed with respect to other actions. 1769 */ 1770 @Child(name = "priority", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1771 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the action should be addressed with respect to other actions." ) 1772 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 1773 protected Enumeration<RequestPriority> priority; 1774 1775 /** 1776 * A code that provides a meaning, grouping, or classification for the action or action group. For example, a section may have a LOINC code for the section of a documentation template. In pharmaceutical quality, an action (Test) such as pH could be classified as a physical property. 1777 */ 1778 @Child(name = "code", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1779 @Description(shortDefinition="Code representing the meaning of the action or sub-actions", formalDefinition="A code that provides a meaning, grouping, or classification for the action or action group. For example, a section may have a LOINC code for the section of a documentation template. In pharmaceutical quality, an action (Test) such as pH could be classified as a physical property." ) 1780 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-code") 1781 protected CodeableConcept code; 1782 1783 /** 1784 * A description of why this action is necessary or appropriate. 1785 */ 1786 @Child(name = "reason", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1787 @Description(shortDefinition="Why the action should be performed", formalDefinition="A description of why this action is necessary or appropriate." ) 1788 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-reason-code") 1789 protected List<CodeableConcept> reason; 1790 1791 /** 1792 * Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources. 1793 */ 1794 @Child(name = "documentation", type = {RelatedArtifact.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1795 @Description(shortDefinition="Supporting documentation for the intended performer of the action", formalDefinition="Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources." ) 1796 protected List<RelatedArtifact> documentation; 1797 1798 /** 1799 * Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action. 1800 */ 1801 @Child(name = "goalId", type = {IdType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1802 @Description(shortDefinition="What goals this action supports", formalDefinition="Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action." ) 1803 protected List<IdType> goalId; 1804 1805 /** 1806 * A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource. 1807 */ 1808 @Child(name = "subject", type = {CodeableConcept.class, Group.class, CanonicalType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1809 @Description(shortDefinition="Type of individual the action is focused on", formalDefinition="A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource." ) 1810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 1811 protected DataType subject; 1812 1813 /** 1814 * A description of when the action should be triggered. When multiple triggers are specified on an action, any triggering event invokes the action. 1815 */ 1816 @Child(name = "trigger", type = {TriggerDefinition.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1817 @Description(shortDefinition="When the action should be triggered", formalDefinition="A description of when the action should be triggered. When multiple triggers are specified on an action, any triggering event invokes the action." ) 1818 protected List<TriggerDefinition> trigger; 1819 1820 /** 1821 * An expression that describes applicability criteria or start/stop conditions for the action. 1822 */ 1823 @Child(name = "condition", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1824 @Description(shortDefinition="Whether or not the action is applicable", formalDefinition="An expression that describes applicability criteria or start/stop conditions for the action." ) 1825 protected List<PlanDefinitionActionConditionComponent> condition; 1826 1827 /** 1828 * Defines input data requirements for the action. 1829 */ 1830 @Child(name = "input", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1831 @Description(shortDefinition="Input data requirements", formalDefinition="Defines input data requirements for the action." ) 1832 protected List<PlanDefinitionActionInputComponent> input; 1833 1834 /** 1835 * Defines the outputs of the action, if any. 1836 */ 1837 @Child(name = "output", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1838 @Description(shortDefinition="Output data definition", formalDefinition="Defines the outputs of the action, if any." ) 1839 protected List<PlanDefinitionActionOutputComponent> output; 1840 1841 /** 1842 * A relationship to another action such as "before" or "30-60 minutes after start of". 1843 */ 1844 @Child(name = "relatedAction", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1845 @Description(shortDefinition="Relationship to another action", formalDefinition="A relationship to another action such as \"before\" or \"30-60 minutes after start of\"." ) 1846 protected List<PlanDefinitionActionRelatedActionComponent> relatedAction; 1847 1848 /** 1849 * An optional value describing when the action should be performed. 1850 */ 1851 @Child(name = "timing", type = {Age.class, Duration.class, Range.class, Timing.class}, order=17, min=0, max=1, modifier=false, summary=false) 1852 @Description(shortDefinition="When the action should take place", formalDefinition="An optional value describing when the action should be performed." ) 1853 protected DataType timing; 1854 1855 /** 1856 * Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc. 1857 */ 1858 @Child(name = "location", type = {CodeableReference.class}, order=18, min=0, max=1, modifier=false, summary=false) 1859 @Description(shortDefinition="Where it should happen", formalDefinition="Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc." ) 1860 protected CodeableReference location; 1861 1862 /** 1863 * Indicates who should participate in performing the action described. 1864 */ 1865 @Child(name = "participant", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1866 @Description(shortDefinition="Who should participate in the action", formalDefinition="Indicates who should participate in performing the action described." ) 1867 protected List<PlanDefinitionActionParticipantComponent> participant; 1868 1869 /** 1870 * The type of action to perform (create, update, remove). 1871 */ 1872 @Child(name = "type", type = {CodeableConcept.class}, order=20, min=0, max=1, modifier=false, summary=false) 1873 @Description(shortDefinition="create | update | remove | fire-event", formalDefinition="The type of action to perform (create, update, remove)." ) 1874 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-type") 1875 protected CodeableConcept type; 1876 1877 /** 1878 * Defines the grouping behavior for the action and its children. 1879 */ 1880 @Child(name = "groupingBehavior", type = {CodeType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1881 @Description(shortDefinition="visual-group | logical-group | sentence-group", formalDefinition="Defines the grouping behavior for the action and its children." ) 1882 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-grouping-behavior") 1883 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 1884 1885 /** 1886 * Defines the selection behavior for the action and its children. 1887 */ 1888 @Child(name = "selectionBehavior", type = {CodeType.class}, order=22, min=0, max=1, modifier=false, summary=false) 1889 @Description(shortDefinition="any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition="Defines the selection behavior for the action and its children." ) 1890 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-selection-behavior") 1891 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 1892 1893 /** 1894 * Defines the required behavior for the action. 1895 */ 1896 @Child(name = "requiredBehavior", type = {CodeType.class}, order=23, min=0, max=1, modifier=false, summary=false) 1897 @Description(shortDefinition="must | could | must-unless-documented", formalDefinition="Defines the required behavior for the action." ) 1898 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-required-behavior") 1899 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 1900 1901 /** 1902 * Defines whether the action should usually be preselected. 1903 */ 1904 @Child(name = "precheckBehavior", type = {CodeType.class}, order=24, min=0, max=1, modifier=false, summary=false) 1905 @Description(shortDefinition="yes | no", formalDefinition="Defines whether the action should usually be preselected." ) 1906 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-precheck-behavior") 1907 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 1908 1909 /** 1910 * Defines whether the action can be selected multiple times. 1911 */ 1912 @Child(name = "cardinalityBehavior", type = {CodeType.class}, order=25, min=0, max=1, modifier=false, summary=false) 1913 @Description(shortDefinition="single | multiple", formalDefinition="Defines whether the action can be selected multiple times." ) 1914 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 1915 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 1916 1917 /** 1918 * A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured. 1919 */ 1920 @Child(name = "definition", type = {CanonicalType.class, UriType.class}, order=26, min=0, max=1, modifier=false, summary=false) 1921 @Description(shortDefinition="Description of the activity to be performed", formalDefinition="A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured." ) 1922 protected DataType definition; 1923 1924 /** 1925 * A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 1926 */ 1927 @Child(name = "transform", type = {CanonicalType.class}, order=27, min=0, max=1, modifier=false, summary=false) 1928 @Description(shortDefinition="Transform to apply the template", formalDefinition="A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input." ) 1929 protected CanonicalType transform; 1930 1931 /** 1932 * Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result. 1933 */ 1934 @Child(name = "dynamicValue", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1935 @Description(shortDefinition="Dynamic aspects of the definition", formalDefinition="Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result." ) 1936 protected List<PlanDefinitionActionDynamicValueComponent> dynamicValue; 1937 1938 /** 1939 * Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition. 1940 */ 1941 @Child(name = "action", type = {PlanDefinitionActionComponent.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1942 @Description(shortDefinition="A sub-action", formalDefinition="Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition." ) 1943 protected List<PlanDefinitionActionComponent> action; 1944 1945 private static final long serialVersionUID = 1296604536L; 1946 1947 /** 1948 * Constructor 1949 */ 1950 public PlanDefinitionActionComponent() { 1951 super(); 1952 } 1953 1954 /** 1955 * @return {@link #linkId} (An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1956 */ 1957 public StringType getLinkIdElement() { 1958 if (this.linkId == null) 1959 if (Configuration.errorOnAutoCreate()) 1960 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.linkId"); 1961 else if (Configuration.doAutoCreate()) 1962 this.linkId = new StringType(); // bb 1963 return this.linkId; 1964 } 1965 1966 public boolean hasLinkIdElement() { 1967 return this.linkId != null && !this.linkId.isEmpty(); 1968 } 1969 1970 public boolean hasLinkId() { 1971 return this.linkId != null && !this.linkId.isEmpty(); 1972 } 1973 1974 /** 1975 * @param value {@link #linkId} (An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1976 */ 1977 public PlanDefinitionActionComponent setLinkIdElement(StringType value) { 1978 this.linkId = value; 1979 return this; 1980 } 1981 1982 /** 1983 * @return An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration. 1984 */ 1985 public String getLinkId() { 1986 return this.linkId == null ? null : this.linkId.getValue(); 1987 } 1988 1989 /** 1990 * @param value An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration. 1991 */ 1992 public PlanDefinitionActionComponent setLinkId(String value) { 1993 if (Utilities.noString(value)) 1994 this.linkId = null; 1995 else { 1996 if (this.linkId == null) 1997 this.linkId = new StringType(); 1998 this.linkId.setValue(value); 1999 } 2000 return this; 2001 } 2002 2003 /** 2004 * @return {@link #prefix} (A user-visible prefix for the action. For example a section or item numbering such as 1. or A.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 2005 */ 2006 public StringType getPrefixElement() { 2007 if (this.prefix == null) 2008 if (Configuration.errorOnAutoCreate()) 2009 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.prefix"); 2010 else if (Configuration.doAutoCreate()) 2011 this.prefix = new StringType(); // bb 2012 return this.prefix; 2013 } 2014 2015 public boolean hasPrefixElement() { 2016 return this.prefix != null && !this.prefix.isEmpty(); 2017 } 2018 2019 public boolean hasPrefix() { 2020 return this.prefix != null && !this.prefix.isEmpty(); 2021 } 2022 2023 /** 2024 * @param value {@link #prefix} (A user-visible prefix for the action. For example a section or item numbering such as 1. or A.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 2025 */ 2026 public PlanDefinitionActionComponent setPrefixElement(StringType value) { 2027 this.prefix = value; 2028 return this; 2029 } 2030 2031 /** 2032 * @return A user-visible prefix for the action. For example a section or item numbering such as 1. or A. 2033 */ 2034 public String getPrefix() { 2035 return this.prefix == null ? null : this.prefix.getValue(); 2036 } 2037 2038 /** 2039 * @param value A user-visible prefix for the action. For example a section or item numbering such as 1. or A. 2040 */ 2041 public PlanDefinitionActionComponent setPrefix(String value) { 2042 if (Utilities.noString(value)) 2043 this.prefix = null; 2044 else { 2045 if (this.prefix == null) 2046 this.prefix = new StringType(); 2047 this.prefix.setValue(value); 2048 } 2049 return this; 2050 } 2051 2052 /** 2053 * @return {@link #title} (The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2054 */ 2055 public StringType getTitleElement() { 2056 if (this.title == null) 2057 if (Configuration.errorOnAutoCreate()) 2058 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.title"); 2059 else if (Configuration.doAutoCreate()) 2060 this.title = new StringType(); // bb 2061 return this.title; 2062 } 2063 2064 public boolean hasTitleElement() { 2065 return this.title != null && !this.title.isEmpty(); 2066 } 2067 2068 public boolean hasTitle() { 2069 return this.title != null && !this.title.isEmpty(); 2070 } 2071 2072 /** 2073 * @param value {@link #title} (The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2074 */ 2075 public PlanDefinitionActionComponent setTitleElement(StringType value) { 2076 this.title = value; 2077 return this; 2078 } 2079 2080 /** 2081 * @return The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC. 2082 */ 2083 public String getTitle() { 2084 return this.title == null ? null : this.title.getValue(); 2085 } 2086 2087 /** 2088 * @param value The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC. 2089 */ 2090 public PlanDefinitionActionComponent setTitle(String value) { 2091 if (Utilities.noString(value)) 2092 this.title = null; 2093 else { 2094 if (this.title == null) 2095 this.title = new StringType(); 2096 this.title.setValue(value); 2097 } 2098 return this; 2099 } 2100 2101 /** 2102 * @return {@link #description} (A brief description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2103 */ 2104 public MarkdownType getDescriptionElement() { 2105 if (this.description == null) 2106 if (Configuration.errorOnAutoCreate()) 2107 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.description"); 2108 else if (Configuration.doAutoCreate()) 2109 this.description = new MarkdownType(); // bb 2110 return this.description; 2111 } 2112 2113 public boolean hasDescriptionElement() { 2114 return this.description != null && !this.description.isEmpty(); 2115 } 2116 2117 public boolean hasDescription() { 2118 return this.description != null && !this.description.isEmpty(); 2119 } 2120 2121 /** 2122 * @param value {@link #description} (A brief description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2123 */ 2124 public PlanDefinitionActionComponent setDescriptionElement(MarkdownType value) { 2125 this.description = value; 2126 return this; 2127 } 2128 2129 /** 2130 * @return A brief description of the action used to provide a summary to display to the user. 2131 */ 2132 public String getDescription() { 2133 return this.description == null ? null : this.description.getValue(); 2134 } 2135 2136 /** 2137 * @param value A brief description of the action used to provide a summary to display to the user. 2138 */ 2139 public PlanDefinitionActionComponent setDescription(String value) { 2140 if (Utilities.noString(value)) 2141 this.description = null; 2142 else { 2143 if (this.description == null) 2144 this.description = new MarkdownType(); 2145 this.description.setValue(value); 2146 } 2147 return this; 2148 } 2149 2150 /** 2151 * @return {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 2152 */ 2153 public MarkdownType getTextEquivalentElement() { 2154 if (this.textEquivalent == null) 2155 if (Configuration.errorOnAutoCreate()) 2156 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.textEquivalent"); 2157 else if (Configuration.doAutoCreate()) 2158 this.textEquivalent = new MarkdownType(); // bb 2159 return this.textEquivalent; 2160 } 2161 2162 public boolean hasTextEquivalentElement() { 2163 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2164 } 2165 2166 public boolean hasTextEquivalent() { 2167 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2168 } 2169 2170 /** 2171 * @param value {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 2172 */ 2173 public PlanDefinitionActionComponent setTextEquivalentElement(MarkdownType value) { 2174 this.textEquivalent = value; 2175 return this; 2176 } 2177 2178 /** 2179 * @return A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically. 2180 */ 2181 public String getTextEquivalent() { 2182 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 2183 } 2184 2185 /** 2186 * @param value A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically. 2187 */ 2188 public PlanDefinitionActionComponent setTextEquivalent(String value) { 2189 if (Utilities.noString(value)) 2190 this.textEquivalent = null; 2191 else { 2192 if (this.textEquivalent == null) 2193 this.textEquivalent = new MarkdownType(); 2194 this.textEquivalent.setValue(value); 2195 } 2196 return this; 2197 } 2198 2199 /** 2200 * @return {@link #priority} (Indicates how quickly the action should be addressed with respect to other actions.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2201 */ 2202 public Enumeration<RequestPriority> getPriorityElement() { 2203 if (this.priority == null) 2204 if (Configuration.errorOnAutoCreate()) 2205 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.priority"); 2206 else if (Configuration.doAutoCreate()) 2207 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 2208 return this.priority; 2209 } 2210 2211 public boolean hasPriorityElement() { 2212 return this.priority != null && !this.priority.isEmpty(); 2213 } 2214 2215 public boolean hasPriority() { 2216 return this.priority != null && !this.priority.isEmpty(); 2217 } 2218 2219 /** 2220 * @param value {@link #priority} (Indicates how quickly the action should be addressed with respect to other actions.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2221 */ 2222 public PlanDefinitionActionComponent setPriorityElement(Enumeration<RequestPriority> value) { 2223 this.priority = value; 2224 return this; 2225 } 2226 2227 /** 2228 * @return Indicates how quickly the action should be addressed with respect to other actions. 2229 */ 2230 public RequestPriority getPriority() { 2231 return this.priority == null ? null : this.priority.getValue(); 2232 } 2233 2234 /** 2235 * @param value Indicates how quickly the action should be addressed with respect to other actions. 2236 */ 2237 public PlanDefinitionActionComponent setPriority(RequestPriority value) { 2238 if (value == null) 2239 this.priority = null; 2240 else { 2241 if (this.priority == null) 2242 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 2243 this.priority.setValue(value); 2244 } 2245 return this; 2246 } 2247 2248 /** 2249 * @return {@link #code} (A code that provides a meaning, grouping, or classification for the action or action group. For example, a section may have a LOINC code for the section of a documentation template. In pharmaceutical quality, an action (Test) such as pH could be classified as a physical property.) 2250 */ 2251 public CodeableConcept getCode() { 2252 if (this.code == null) 2253 if (Configuration.errorOnAutoCreate()) 2254 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.code"); 2255 else if (Configuration.doAutoCreate()) 2256 this.code = new CodeableConcept(); // cc 2257 return this.code; 2258 } 2259 2260 public boolean hasCode() { 2261 return this.code != null && !this.code.isEmpty(); 2262 } 2263 2264 /** 2265 * @param value {@link #code} (A code that provides a meaning, grouping, or classification for the action or action group. For example, a section may have a LOINC code for the section of a documentation template. In pharmaceutical quality, an action (Test) such as pH could be classified as a physical property.) 2266 */ 2267 public PlanDefinitionActionComponent setCode(CodeableConcept value) { 2268 this.code = value; 2269 return this; 2270 } 2271 2272 /** 2273 * @return {@link #reason} (A description of why this action is necessary or appropriate.) 2274 */ 2275 public List<CodeableConcept> getReason() { 2276 if (this.reason == null) 2277 this.reason = new ArrayList<CodeableConcept>(); 2278 return this.reason; 2279 } 2280 2281 /** 2282 * @return Returns a reference to <code>this</code> for easy method chaining 2283 */ 2284 public PlanDefinitionActionComponent setReason(List<CodeableConcept> theReason) { 2285 this.reason = theReason; 2286 return this; 2287 } 2288 2289 public boolean hasReason() { 2290 if (this.reason == null) 2291 return false; 2292 for (CodeableConcept item : this.reason) 2293 if (!item.isEmpty()) 2294 return true; 2295 return false; 2296 } 2297 2298 public CodeableConcept addReason() { //3 2299 CodeableConcept t = new CodeableConcept(); 2300 if (this.reason == null) 2301 this.reason = new ArrayList<CodeableConcept>(); 2302 this.reason.add(t); 2303 return t; 2304 } 2305 2306 public PlanDefinitionActionComponent addReason(CodeableConcept t) { //3 2307 if (t == null) 2308 return this; 2309 if (this.reason == null) 2310 this.reason = new ArrayList<CodeableConcept>(); 2311 this.reason.add(t); 2312 return this; 2313 } 2314 2315 /** 2316 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2317 */ 2318 public CodeableConcept getReasonFirstRep() { 2319 if (getReason().isEmpty()) { 2320 addReason(); 2321 } 2322 return getReason().get(0); 2323 } 2324 2325 /** 2326 * @return {@link #documentation} (Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.) 2327 */ 2328 public List<RelatedArtifact> getDocumentation() { 2329 if (this.documentation == null) 2330 this.documentation = new ArrayList<RelatedArtifact>(); 2331 return this.documentation; 2332 } 2333 2334 /** 2335 * @return Returns a reference to <code>this</code> for easy method chaining 2336 */ 2337 public PlanDefinitionActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 2338 this.documentation = theDocumentation; 2339 return this; 2340 } 2341 2342 public boolean hasDocumentation() { 2343 if (this.documentation == null) 2344 return false; 2345 for (RelatedArtifact item : this.documentation) 2346 if (!item.isEmpty()) 2347 return true; 2348 return false; 2349 } 2350 2351 public RelatedArtifact addDocumentation() { //3 2352 RelatedArtifact t = new RelatedArtifact(); 2353 if (this.documentation == null) 2354 this.documentation = new ArrayList<RelatedArtifact>(); 2355 this.documentation.add(t); 2356 return t; 2357 } 2358 2359 public PlanDefinitionActionComponent addDocumentation(RelatedArtifact t) { //3 2360 if (t == null) 2361 return this; 2362 if (this.documentation == null) 2363 this.documentation = new ArrayList<RelatedArtifact>(); 2364 this.documentation.add(t); 2365 return this; 2366 } 2367 2368 /** 2369 * @return The first repetition of repeating field {@link #documentation}, creating it if it does not already exist {3} 2370 */ 2371 public RelatedArtifact getDocumentationFirstRep() { 2372 if (getDocumentation().isEmpty()) { 2373 addDocumentation(); 2374 } 2375 return getDocumentation().get(0); 2376 } 2377 2378 /** 2379 * @return {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action.) 2380 */ 2381 public List<IdType> getGoalId() { 2382 if (this.goalId == null) 2383 this.goalId = new ArrayList<IdType>(); 2384 return this.goalId; 2385 } 2386 2387 /** 2388 * @return Returns a reference to <code>this</code> for easy method chaining 2389 */ 2390 public PlanDefinitionActionComponent setGoalId(List<IdType> theGoalId) { 2391 this.goalId = theGoalId; 2392 return this; 2393 } 2394 2395 public boolean hasGoalId() { 2396 if (this.goalId == null) 2397 return false; 2398 for (IdType item : this.goalId) 2399 if (!item.isEmpty()) 2400 return true; 2401 return false; 2402 } 2403 2404 /** 2405 * @return {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action.) 2406 */ 2407 public IdType addGoalIdElement() {//2 2408 IdType t = new IdType(); 2409 if (this.goalId == null) 2410 this.goalId = new ArrayList<IdType>(); 2411 this.goalId.add(t); 2412 return t; 2413 } 2414 2415 /** 2416 * @param value {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action.) 2417 */ 2418 public PlanDefinitionActionComponent addGoalId(String value) { //1 2419 IdType t = new IdType(); 2420 t.setValue(value); 2421 if (this.goalId == null) 2422 this.goalId = new ArrayList<IdType>(); 2423 this.goalId.add(t); 2424 return this; 2425 } 2426 2427 /** 2428 * @param value {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action.) 2429 */ 2430 public boolean hasGoalId(String value) { 2431 if (this.goalId == null) 2432 return false; 2433 for (IdType v : this.goalId) 2434 if (v.getValue().equals(value)) // id 2435 return true; 2436 return false; 2437 } 2438 2439 /** 2440 * @return {@link #subject} (A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 2441 */ 2442 public DataType getSubject() { 2443 return this.subject; 2444 } 2445 2446 /** 2447 * @return {@link #subject} (A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 2448 */ 2449 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2450 if (this.subject == null) 2451 this.subject = new CodeableConcept(); 2452 if (!(this.subject instanceof CodeableConcept)) 2453 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 2454 return (CodeableConcept) this.subject; 2455 } 2456 2457 public boolean hasSubjectCodeableConcept() { 2458 return this.subject instanceof CodeableConcept; 2459 } 2460 2461 /** 2462 * @return {@link #subject} (A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 2463 */ 2464 public Reference getSubjectReference() throws FHIRException { 2465 if (this.subject == null) 2466 this.subject = new Reference(); 2467 if (!(this.subject instanceof Reference)) 2468 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 2469 return (Reference) this.subject; 2470 } 2471 2472 public boolean hasSubjectReference() { 2473 return this.subject instanceof Reference; 2474 } 2475 2476 /** 2477 * @return {@link #subject} (A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 2478 */ 2479 public CanonicalType getSubjectCanonicalType() throws FHIRException { 2480 if (this.subject == null) 2481 this.subject = new CanonicalType(); 2482 if (!(this.subject instanceof CanonicalType)) 2483 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.subject.getClass().getName()+" was encountered"); 2484 return (CanonicalType) this.subject; 2485 } 2486 2487 public boolean hasSubjectCanonicalType() { 2488 return this.subject instanceof CanonicalType; 2489 } 2490 2491 public boolean hasSubject() { 2492 return this.subject != null && !this.subject.isEmpty(); 2493 } 2494 2495 /** 2496 * @param value {@link #subject} (A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 2497 */ 2498 public PlanDefinitionActionComponent setSubject(DataType value) { 2499 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference || value instanceof CanonicalType)) 2500 throw new FHIRException("Not the right type for PlanDefinition.action.subject[x]: "+value.fhirType()); 2501 this.subject = value; 2502 return this; 2503 } 2504 2505 /** 2506 * @return {@link #trigger} (A description of when the action should be triggered. When multiple triggers are specified on an action, any triggering event invokes the action.) 2507 */ 2508 public List<TriggerDefinition> getTrigger() { 2509 if (this.trigger == null) 2510 this.trigger = new ArrayList<TriggerDefinition>(); 2511 return this.trigger; 2512 } 2513 2514 /** 2515 * @return Returns a reference to <code>this</code> for easy method chaining 2516 */ 2517 public PlanDefinitionActionComponent setTrigger(List<TriggerDefinition> theTrigger) { 2518 this.trigger = theTrigger; 2519 return this; 2520 } 2521 2522 public boolean hasTrigger() { 2523 if (this.trigger == null) 2524 return false; 2525 for (TriggerDefinition item : this.trigger) 2526 if (!item.isEmpty()) 2527 return true; 2528 return false; 2529 } 2530 2531 public TriggerDefinition addTrigger() { //3 2532 TriggerDefinition t = new TriggerDefinition(); 2533 if (this.trigger == null) 2534 this.trigger = new ArrayList<TriggerDefinition>(); 2535 this.trigger.add(t); 2536 return t; 2537 } 2538 2539 public PlanDefinitionActionComponent addTrigger(TriggerDefinition t) { //3 2540 if (t == null) 2541 return this; 2542 if (this.trigger == null) 2543 this.trigger = new ArrayList<TriggerDefinition>(); 2544 this.trigger.add(t); 2545 return this; 2546 } 2547 2548 /** 2549 * @return The first repetition of repeating field {@link #trigger}, creating it if it does not already exist {3} 2550 */ 2551 public TriggerDefinition getTriggerFirstRep() { 2552 if (getTrigger().isEmpty()) { 2553 addTrigger(); 2554 } 2555 return getTrigger().get(0); 2556 } 2557 2558 /** 2559 * @return {@link #condition} (An expression that describes applicability criteria or start/stop conditions for the action.) 2560 */ 2561 public List<PlanDefinitionActionConditionComponent> getCondition() { 2562 if (this.condition == null) 2563 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 2564 return this.condition; 2565 } 2566 2567 /** 2568 * @return Returns a reference to <code>this</code> for easy method chaining 2569 */ 2570 public PlanDefinitionActionComponent setCondition(List<PlanDefinitionActionConditionComponent> theCondition) { 2571 this.condition = theCondition; 2572 return this; 2573 } 2574 2575 public boolean hasCondition() { 2576 if (this.condition == null) 2577 return false; 2578 for (PlanDefinitionActionConditionComponent item : this.condition) 2579 if (!item.isEmpty()) 2580 return true; 2581 return false; 2582 } 2583 2584 public PlanDefinitionActionConditionComponent addCondition() { //3 2585 PlanDefinitionActionConditionComponent t = new PlanDefinitionActionConditionComponent(); 2586 if (this.condition == null) 2587 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 2588 this.condition.add(t); 2589 return t; 2590 } 2591 2592 public PlanDefinitionActionComponent addCondition(PlanDefinitionActionConditionComponent t) { //3 2593 if (t == null) 2594 return this; 2595 if (this.condition == null) 2596 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 2597 this.condition.add(t); 2598 return this; 2599 } 2600 2601 /** 2602 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 2603 */ 2604 public PlanDefinitionActionConditionComponent getConditionFirstRep() { 2605 if (getCondition().isEmpty()) { 2606 addCondition(); 2607 } 2608 return getCondition().get(0); 2609 } 2610 2611 /** 2612 * @return {@link #input} (Defines input data requirements for the action.) 2613 */ 2614 public List<PlanDefinitionActionInputComponent> getInput() { 2615 if (this.input == null) 2616 this.input = new ArrayList<PlanDefinitionActionInputComponent>(); 2617 return this.input; 2618 } 2619 2620 /** 2621 * @return Returns a reference to <code>this</code> for easy method chaining 2622 */ 2623 public PlanDefinitionActionComponent setInput(List<PlanDefinitionActionInputComponent> theInput) { 2624 this.input = theInput; 2625 return this; 2626 } 2627 2628 public boolean hasInput() { 2629 if (this.input == null) 2630 return false; 2631 for (PlanDefinitionActionInputComponent item : this.input) 2632 if (!item.isEmpty()) 2633 return true; 2634 return false; 2635 } 2636 2637 public PlanDefinitionActionInputComponent addInput() { //3 2638 PlanDefinitionActionInputComponent t = new PlanDefinitionActionInputComponent(); 2639 if (this.input == null) 2640 this.input = new ArrayList<PlanDefinitionActionInputComponent>(); 2641 this.input.add(t); 2642 return t; 2643 } 2644 2645 public PlanDefinitionActionComponent addInput(PlanDefinitionActionInputComponent t) { //3 2646 if (t == null) 2647 return this; 2648 if (this.input == null) 2649 this.input = new ArrayList<PlanDefinitionActionInputComponent>(); 2650 this.input.add(t); 2651 return this; 2652 } 2653 2654 /** 2655 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 2656 */ 2657 public PlanDefinitionActionInputComponent getInputFirstRep() { 2658 if (getInput().isEmpty()) { 2659 addInput(); 2660 } 2661 return getInput().get(0); 2662 } 2663 2664 /** 2665 * @return {@link #output} (Defines the outputs of the action, if any.) 2666 */ 2667 public List<PlanDefinitionActionOutputComponent> getOutput() { 2668 if (this.output == null) 2669 this.output = new ArrayList<PlanDefinitionActionOutputComponent>(); 2670 return this.output; 2671 } 2672 2673 /** 2674 * @return Returns a reference to <code>this</code> for easy method chaining 2675 */ 2676 public PlanDefinitionActionComponent setOutput(List<PlanDefinitionActionOutputComponent> theOutput) { 2677 this.output = theOutput; 2678 return this; 2679 } 2680 2681 public boolean hasOutput() { 2682 if (this.output == null) 2683 return false; 2684 for (PlanDefinitionActionOutputComponent item : this.output) 2685 if (!item.isEmpty()) 2686 return true; 2687 return false; 2688 } 2689 2690 public PlanDefinitionActionOutputComponent addOutput() { //3 2691 PlanDefinitionActionOutputComponent t = new PlanDefinitionActionOutputComponent(); 2692 if (this.output == null) 2693 this.output = new ArrayList<PlanDefinitionActionOutputComponent>(); 2694 this.output.add(t); 2695 return t; 2696 } 2697 2698 public PlanDefinitionActionComponent addOutput(PlanDefinitionActionOutputComponent t) { //3 2699 if (t == null) 2700 return this; 2701 if (this.output == null) 2702 this.output = new ArrayList<PlanDefinitionActionOutputComponent>(); 2703 this.output.add(t); 2704 return this; 2705 } 2706 2707 /** 2708 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist {3} 2709 */ 2710 public PlanDefinitionActionOutputComponent getOutputFirstRep() { 2711 if (getOutput().isEmpty()) { 2712 addOutput(); 2713 } 2714 return getOutput().get(0); 2715 } 2716 2717 /** 2718 * @return {@link #relatedAction} (A relationship to another action such as "before" or "30-60 minutes after start of".) 2719 */ 2720 public List<PlanDefinitionActionRelatedActionComponent> getRelatedAction() { 2721 if (this.relatedAction == null) 2722 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 2723 return this.relatedAction; 2724 } 2725 2726 /** 2727 * @return Returns a reference to <code>this</code> for easy method chaining 2728 */ 2729 public PlanDefinitionActionComponent setRelatedAction(List<PlanDefinitionActionRelatedActionComponent> theRelatedAction) { 2730 this.relatedAction = theRelatedAction; 2731 return this; 2732 } 2733 2734 public boolean hasRelatedAction() { 2735 if (this.relatedAction == null) 2736 return false; 2737 for (PlanDefinitionActionRelatedActionComponent item : this.relatedAction) 2738 if (!item.isEmpty()) 2739 return true; 2740 return false; 2741 } 2742 2743 public PlanDefinitionActionRelatedActionComponent addRelatedAction() { //3 2744 PlanDefinitionActionRelatedActionComponent t = new PlanDefinitionActionRelatedActionComponent(); 2745 if (this.relatedAction == null) 2746 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 2747 this.relatedAction.add(t); 2748 return t; 2749 } 2750 2751 public PlanDefinitionActionComponent addRelatedAction(PlanDefinitionActionRelatedActionComponent t) { //3 2752 if (t == null) 2753 return this; 2754 if (this.relatedAction == null) 2755 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 2756 this.relatedAction.add(t); 2757 return this; 2758 } 2759 2760 /** 2761 * @return The first repetition of repeating field {@link #relatedAction}, creating it if it does not already exist {3} 2762 */ 2763 public PlanDefinitionActionRelatedActionComponent getRelatedActionFirstRep() { 2764 if (getRelatedAction().isEmpty()) { 2765 addRelatedAction(); 2766 } 2767 return getRelatedAction().get(0); 2768 } 2769 2770 /** 2771 * @return {@link #timing} (An optional value describing when the action should be performed.) 2772 */ 2773 public DataType getTiming() { 2774 return this.timing; 2775 } 2776 2777 /** 2778 * @return {@link #timing} (An optional value describing when the action should be performed.) 2779 */ 2780 public Age getTimingAge() throws FHIRException { 2781 if (this.timing == null) 2782 this.timing = new Age(); 2783 if (!(this.timing instanceof Age)) 2784 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.timing.getClass().getName()+" was encountered"); 2785 return (Age) this.timing; 2786 } 2787 2788 public boolean hasTimingAge() { 2789 return this.timing instanceof Age; 2790 } 2791 2792 /** 2793 * @return {@link #timing} (An optional value describing when the action should be performed.) 2794 */ 2795 public Duration getTimingDuration() throws FHIRException { 2796 if (this.timing == null) 2797 this.timing = new Duration(); 2798 if (!(this.timing instanceof Duration)) 2799 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.timing.getClass().getName()+" was encountered"); 2800 return (Duration) this.timing; 2801 } 2802 2803 public boolean hasTimingDuration() { 2804 return this.timing instanceof Duration; 2805 } 2806 2807 /** 2808 * @return {@link #timing} (An optional value describing when the action should be performed.) 2809 */ 2810 public Range getTimingRange() throws FHIRException { 2811 if (this.timing == null) 2812 this.timing = new Range(); 2813 if (!(this.timing instanceof Range)) 2814 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.timing.getClass().getName()+" was encountered"); 2815 return (Range) this.timing; 2816 } 2817 2818 public boolean hasTimingRange() { 2819 return this.timing instanceof Range; 2820 } 2821 2822 /** 2823 * @return {@link #timing} (An optional value describing when the action should be performed.) 2824 */ 2825 public Timing getTimingTiming() throws FHIRException { 2826 if (this.timing == null) 2827 this.timing = new Timing(); 2828 if (!(this.timing instanceof Timing)) 2829 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 2830 return (Timing) this.timing; 2831 } 2832 2833 public boolean hasTimingTiming() { 2834 return this.timing instanceof Timing; 2835 } 2836 2837 public boolean hasTiming() { 2838 return this.timing != null && !this.timing.isEmpty(); 2839 } 2840 2841 /** 2842 * @param value {@link #timing} (An optional value describing when the action should be performed.) 2843 */ 2844 public PlanDefinitionActionComponent setTiming(DataType value) { 2845 if (value != null && !(value instanceof Age || value instanceof Duration || value instanceof Range || value instanceof Timing)) 2846 throw new FHIRException("Not the right type for PlanDefinition.action.timing[x]: "+value.fhirType()); 2847 this.timing = value; 2848 return this; 2849 } 2850 2851 /** 2852 * @return {@link #location} (Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.) 2853 */ 2854 public CodeableReference getLocation() { 2855 if (this.location == null) 2856 if (Configuration.errorOnAutoCreate()) 2857 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.location"); 2858 else if (Configuration.doAutoCreate()) 2859 this.location = new CodeableReference(); // cc 2860 return this.location; 2861 } 2862 2863 public boolean hasLocation() { 2864 return this.location != null && !this.location.isEmpty(); 2865 } 2866 2867 /** 2868 * @param value {@link #location} (Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.) 2869 */ 2870 public PlanDefinitionActionComponent setLocation(CodeableReference value) { 2871 this.location = value; 2872 return this; 2873 } 2874 2875 /** 2876 * @return {@link #participant} (Indicates who should participate in performing the action described.) 2877 */ 2878 public List<PlanDefinitionActionParticipantComponent> getParticipant() { 2879 if (this.participant == null) 2880 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 2881 return this.participant; 2882 } 2883 2884 /** 2885 * @return Returns a reference to <code>this</code> for easy method chaining 2886 */ 2887 public PlanDefinitionActionComponent setParticipant(List<PlanDefinitionActionParticipantComponent> theParticipant) { 2888 this.participant = theParticipant; 2889 return this; 2890 } 2891 2892 public boolean hasParticipant() { 2893 if (this.participant == null) 2894 return false; 2895 for (PlanDefinitionActionParticipantComponent item : this.participant) 2896 if (!item.isEmpty()) 2897 return true; 2898 return false; 2899 } 2900 2901 public PlanDefinitionActionParticipantComponent addParticipant() { //3 2902 PlanDefinitionActionParticipantComponent t = new PlanDefinitionActionParticipantComponent(); 2903 if (this.participant == null) 2904 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 2905 this.participant.add(t); 2906 return t; 2907 } 2908 2909 public PlanDefinitionActionComponent addParticipant(PlanDefinitionActionParticipantComponent t) { //3 2910 if (t == null) 2911 return this; 2912 if (this.participant == null) 2913 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 2914 this.participant.add(t); 2915 return this; 2916 } 2917 2918 /** 2919 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 2920 */ 2921 public PlanDefinitionActionParticipantComponent getParticipantFirstRep() { 2922 if (getParticipant().isEmpty()) { 2923 addParticipant(); 2924 } 2925 return getParticipant().get(0); 2926 } 2927 2928 /** 2929 * @return {@link #type} (The type of action to perform (create, update, remove).) 2930 */ 2931 public CodeableConcept getType() { 2932 if (this.type == null) 2933 if (Configuration.errorOnAutoCreate()) 2934 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.type"); 2935 else if (Configuration.doAutoCreate()) 2936 this.type = new CodeableConcept(); // cc 2937 return this.type; 2938 } 2939 2940 public boolean hasType() { 2941 return this.type != null && !this.type.isEmpty(); 2942 } 2943 2944 /** 2945 * @param value {@link #type} (The type of action to perform (create, update, remove).) 2946 */ 2947 public PlanDefinitionActionComponent setType(CodeableConcept value) { 2948 this.type = value; 2949 return this; 2950 } 2951 2952 /** 2953 * @return {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 2954 */ 2955 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 2956 if (this.groupingBehavior == null) 2957 if (Configuration.errorOnAutoCreate()) 2958 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.groupingBehavior"); 2959 else if (Configuration.doAutoCreate()) 2960 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 2961 return this.groupingBehavior; 2962 } 2963 2964 public boolean hasGroupingBehaviorElement() { 2965 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2966 } 2967 2968 public boolean hasGroupingBehavior() { 2969 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2970 } 2971 2972 /** 2973 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 2974 */ 2975 public PlanDefinitionActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 2976 this.groupingBehavior = value; 2977 return this; 2978 } 2979 2980 /** 2981 * @return Defines the grouping behavior for the action and its children. 2982 */ 2983 public ActionGroupingBehavior getGroupingBehavior() { 2984 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 2985 } 2986 2987 /** 2988 * @param value Defines the grouping behavior for the action and its children. 2989 */ 2990 public PlanDefinitionActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 2991 if (value == null) 2992 this.groupingBehavior = null; 2993 else { 2994 if (this.groupingBehavior == null) 2995 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 2996 this.groupingBehavior.setValue(value); 2997 } 2998 return this; 2999 } 3000 3001 /** 3002 * @return {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 3003 */ 3004 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 3005 if (this.selectionBehavior == null) 3006 if (Configuration.errorOnAutoCreate()) 3007 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.selectionBehavior"); 3008 else if (Configuration.doAutoCreate()) 3009 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 3010 return this.selectionBehavior; 3011 } 3012 3013 public boolean hasSelectionBehaviorElement() { 3014 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3015 } 3016 3017 public boolean hasSelectionBehavior() { 3018 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3019 } 3020 3021 /** 3022 * @param value {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 3023 */ 3024 public PlanDefinitionActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 3025 this.selectionBehavior = value; 3026 return this; 3027 } 3028 3029 /** 3030 * @return Defines the selection behavior for the action and its children. 3031 */ 3032 public ActionSelectionBehavior getSelectionBehavior() { 3033 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 3034 } 3035 3036 /** 3037 * @param value Defines the selection behavior for the action and its children. 3038 */ 3039 public PlanDefinitionActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 3040 if (value == null) 3041 this.selectionBehavior = null; 3042 else { 3043 if (this.selectionBehavior == null) 3044 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 3045 this.selectionBehavior.setValue(value); 3046 } 3047 return this; 3048 } 3049 3050 /** 3051 * @return {@link #requiredBehavior} (Defines the required behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 3052 */ 3053 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 3054 if (this.requiredBehavior == null) 3055 if (Configuration.errorOnAutoCreate()) 3056 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.requiredBehavior"); 3057 else if (Configuration.doAutoCreate()) 3058 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 3059 return this.requiredBehavior; 3060 } 3061 3062 public boolean hasRequiredBehaviorElement() { 3063 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 3064 } 3065 3066 public boolean hasRequiredBehavior() { 3067 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 3068 } 3069 3070 /** 3071 * @param value {@link #requiredBehavior} (Defines the required behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 3072 */ 3073 public PlanDefinitionActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 3074 this.requiredBehavior = value; 3075 return this; 3076 } 3077 3078 /** 3079 * @return Defines the required behavior for the action. 3080 */ 3081 public ActionRequiredBehavior getRequiredBehavior() { 3082 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 3083 } 3084 3085 /** 3086 * @param value Defines the required behavior for the action. 3087 */ 3088 public PlanDefinitionActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 3089 if (value == null) 3090 this.requiredBehavior = null; 3091 else { 3092 if (this.requiredBehavior == null) 3093 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 3094 this.requiredBehavior.setValue(value); 3095 } 3096 return this; 3097 } 3098 3099 /** 3100 * @return {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 3101 */ 3102 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 3103 if (this.precheckBehavior == null) 3104 if (Configuration.errorOnAutoCreate()) 3105 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.precheckBehavior"); 3106 else if (Configuration.doAutoCreate()) 3107 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 3108 return this.precheckBehavior; 3109 } 3110 3111 public boolean hasPrecheckBehaviorElement() { 3112 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 3113 } 3114 3115 public boolean hasPrecheckBehavior() { 3116 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 3117 } 3118 3119 /** 3120 * @param value {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 3121 */ 3122 public PlanDefinitionActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 3123 this.precheckBehavior = value; 3124 return this; 3125 } 3126 3127 /** 3128 * @return Defines whether the action should usually be preselected. 3129 */ 3130 public ActionPrecheckBehavior getPrecheckBehavior() { 3131 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 3132 } 3133 3134 /** 3135 * @param value Defines whether the action should usually be preselected. 3136 */ 3137 public PlanDefinitionActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 3138 if (value == null) 3139 this.precheckBehavior = null; 3140 else { 3141 if (this.precheckBehavior == null) 3142 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 3143 this.precheckBehavior.setValue(value); 3144 } 3145 return this; 3146 } 3147 3148 /** 3149 * @return {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 3150 */ 3151 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 3152 if (this.cardinalityBehavior == null) 3153 if (Configuration.errorOnAutoCreate()) 3154 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.cardinalityBehavior"); 3155 else if (Configuration.doAutoCreate()) 3156 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); // bb 3157 return this.cardinalityBehavior; 3158 } 3159 3160 public boolean hasCardinalityBehaviorElement() { 3161 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3162 } 3163 3164 public boolean hasCardinalityBehavior() { 3165 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3166 } 3167 3168 /** 3169 * @param value {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 3170 */ 3171 public PlanDefinitionActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 3172 this.cardinalityBehavior = value; 3173 return this; 3174 } 3175 3176 /** 3177 * @return Defines whether the action can be selected multiple times. 3178 */ 3179 public ActionCardinalityBehavior getCardinalityBehavior() { 3180 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 3181 } 3182 3183 /** 3184 * @param value Defines whether the action can be selected multiple times. 3185 */ 3186 public PlanDefinitionActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 3187 if (value == null) 3188 this.cardinalityBehavior = null; 3189 else { 3190 if (this.cardinalityBehavior == null) 3191 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); 3192 this.cardinalityBehavior.setValue(value); 3193 } 3194 return this; 3195 } 3196 3197 /** 3198 * @return {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 3199 */ 3200 public DataType getDefinition() { 3201 return this.definition; 3202 } 3203 3204 /** 3205 * @return {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 3206 */ 3207 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 3208 if (this.definition == null) 3209 this.definition = new CanonicalType(); 3210 if (!(this.definition instanceof CanonicalType)) 3211 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.definition.getClass().getName()+" was encountered"); 3212 return (CanonicalType) this.definition; 3213 } 3214 3215 public boolean hasDefinitionCanonicalType() { 3216 return this.definition instanceof CanonicalType; 3217 } 3218 3219 /** 3220 * @return {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 3221 */ 3222 public UriType getDefinitionUriType() throws FHIRException { 3223 if (this.definition == null) 3224 this.definition = new UriType(); 3225 if (!(this.definition instanceof UriType)) 3226 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.definition.getClass().getName()+" was encountered"); 3227 return (UriType) this.definition; 3228 } 3229 3230 public boolean hasDefinitionUriType() { 3231 return this.definition instanceof UriType; 3232 } 3233 3234 public boolean hasDefinition() { 3235 return this.definition != null && !this.definition.isEmpty(); 3236 } 3237 3238 /** 3239 * @param value {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 3240 */ 3241 public PlanDefinitionActionComponent setDefinition(DataType value) { 3242 if (value != null && !(value instanceof CanonicalType || value instanceof UriType)) 3243 throw new FHIRException("Not the right type for PlanDefinition.action.definition[x]: "+value.fhirType()); 3244 this.definition = value; 3245 return this; 3246 } 3247 3248 /** 3249 * @return {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 3250 */ 3251 public CanonicalType getTransformElement() { 3252 if (this.transform == null) 3253 if (Configuration.errorOnAutoCreate()) 3254 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.transform"); 3255 else if (Configuration.doAutoCreate()) 3256 this.transform = new CanonicalType(); // bb 3257 return this.transform; 3258 } 3259 3260 public boolean hasTransformElement() { 3261 return this.transform != null && !this.transform.isEmpty(); 3262 } 3263 3264 public boolean hasTransform() { 3265 return this.transform != null && !this.transform.isEmpty(); 3266 } 3267 3268 /** 3269 * @param value {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 3270 */ 3271 public PlanDefinitionActionComponent setTransformElement(CanonicalType value) { 3272 this.transform = value; 3273 return this; 3274 } 3275 3276 /** 3277 * @return A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 3278 */ 3279 public String getTransform() { 3280 return this.transform == null ? null : this.transform.getValue(); 3281 } 3282 3283 /** 3284 * @param value A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 3285 */ 3286 public PlanDefinitionActionComponent setTransform(String value) { 3287 if (Utilities.noString(value)) 3288 this.transform = null; 3289 else { 3290 if (this.transform == null) 3291 this.transform = new CanonicalType(); 3292 this.transform.setValue(value); 3293 } 3294 return this; 3295 } 3296 3297 /** 3298 * @return {@link #dynamicValue} (Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.) 3299 */ 3300 public List<PlanDefinitionActionDynamicValueComponent> getDynamicValue() { 3301 if (this.dynamicValue == null) 3302 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 3303 return this.dynamicValue; 3304 } 3305 3306 /** 3307 * @return Returns a reference to <code>this</code> for easy method chaining 3308 */ 3309 public PlanDefinitionActionComponent setDynamicValue(List<PlanDefinitionActionDynamicValueComponent> theDynamicValue) { 3310 this.dynamicValue = theDynamicValue; 3311 return this; 3312 } 3313 3314 public boolean hasDynamicValue() { 3315 if (this.dynamicValue == null) 3316 return false; 3317 for (PlanDefinitionActionDynamicValueComponent item : this.dynamicValue) 3318 if (!item.isEmpty()) 3319 return true; 3320 return false; 3321 } 3322 3323 public PlanDefinitionActionDynamicValueComponent addDynamicValue() { //3 3324 PlanDefinitionActionDynamicValueComponent t = new PlanDefinitionActionDynamicValueComponent(); 3325 if (this.dynamicValue == null) 3326 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 3327 this.dynamicValue.add(t); 3328 return t; 3329 } 3330 3331 public PlanDefinitionActionComponent addDynamicValue(PlanDefinitionActionDynamicValueComponent t) { //3 3332 if (t == null) 3333 return this; 3334 if (this.dynamicValue == null) 3335 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 3336 this.dynamicValue.add(t); 3337 return this; 3338 } 3339 3340 /** 3341 * @return The first repetition of repeating field {@link #dynamicValue}, creating it if it does not already exist {3} 3342 */ 3343 public PlanDefinitionActionDynamicValueComponent getDynamicValueFirstRep() { 3344 if (getDynamicValue().isEmpty()) { 3345 addDynamicValue(); 3346 } 3347 return getDynamicValue().get(0); 3348 } 3349 3350 /** 3351 * @return {@link #action} (Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.) 3352 */ 3353 public List<PlanDefinitionActionComponent> getAction() { 3354 if (this.action == null) 3355 this.action = new ArrayList<PlanDefinitionActionComponent>(); 3356 return this.action; 3357 } 3358 3359 /** 3360 * @return Returns a reference to <code>this</code> for easy method chaining 3361 */ 3362 public PlanDefinitionActionComponent setAction(List<PlanDefinitionActionComponent> theAction) { 3363 this.action = theAction; 3364 return this; 3365 } 3366 3367 public boolean hasAction() { 3368 if (this.action == null) 3369 return false; 3370 for (PlanDefinitionActionComponent item : this.action) 3371 if (!item.isEmpty()) 3372 return true; 3373 return false; 3374 } 3375 3376 public PlanDefinitionActionComponent addAction() { //3 3377 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 3378 if (this.action == null) 3379 this.action = new ArrayList<PlanDefinitionActionComponent>(); 3380 this.action.add(t); 3381 return t; 3382 } 3383 3384 public PlanDefinitionActionComponent addAction(PlanDefinitionActionComponent t) { //3 3385 if (t == null) 3386 return this; 3387 if (this.action == null) 3388 this.action = new ArrayList<PlanDefinitionActionComponent>(); 3389 this.action.add(t); 3390 return this; 3391 } 3392 3393 /** 3394 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 3395 */ 3396 public PlanDefinitionActionComponent getActionFirstRep() { 3397 if (getAction().isEmpty()) { 3398 addAction(); 3399 } 3400 return getAction().get(0); 3401 } 3402 3403 protected void listChildren(List<Property> children) { 3404 super.listChildren(children); 3405 children.add(new Property("linkId", "string", "An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration.", 0, 1, linkId)); 3406 children.add(new Property("prefix", "string", "A user-visible prefix for the action. For example a section or item numbering such as 1. or A.", 0, 1, prefix)); 3407 children.add(new Property("title", "string", "The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC.", 0, 1, title)); 3408 children.add(new Property("description", "markdown", "A brief description of the action used to provide a summary to display to the user.", 0, 1, description)); 3409 children.add(new Property("textEquivalent", "markdown", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 0, 1, textEquivalent)); 3410 children.add(new Property("priority", "code", "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority)); 3411 children.add(new Property("code", "CodeableConcept", "A code that provides a meaning, grouping, or classification for the action or action group. For example, a section may have a LOINC code for the section of a documentation template. In pharmaceutical quality, an action (Test) such as pH could be classified as a physical property.", 0, 1, code)); 3412 children.add(new Property("reason", "CodeableConcept", "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason)); 3413 children.add(new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation)); 3414 children.add(new Property("goalId", "id", "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action.", 0, java.lang.Integer.MAX_VALUE, goalId)); 3415 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)|canonical", "A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject)); 3416 children.add(new Property("trigger", "TriggerDefinition", "A description of when the action should be triggered. When multiple triggers are specified on an action, any triggering event invokes the action.", 0, java.lang.Integer.MAX_VALUE, trigger)); 3417 children.add(new Property("condition", "", "An expression that describes applicability criteria or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition)); 3418 children.add(new Property("input", "", "Defines input data requirements for the action.", 0, java.lang.Integer.MAX_VALUE, input)); 3419 children.add(new Property("output", "", "Defines the outputs of the action, if any.", 0, java.lang.Integer.MAX_VALUE, output)); 3420 children.add(new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction)); 3421 children.add(new Property("timing[x]", "Age|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing)); 3422 children.add(new Property("location", "CodeableReference(Location)", "Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location)); 3423 children.add(new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant)); 3424 children.add(new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 1, type)); 3425 children.add(new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 3426 children.add(new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 3427 children.add(new Property("requiredBehavior", "code", "Defines the required behavior for the action.", 0, 1, requiredBehavior)); 3428 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior)); 3429 children.add(new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 3430 children.add(new Property("definition[x]", "canonical(ActivityDefinition|MessageDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)|uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition)); 3431 children.add(new Property("transform", "canonical(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform)); 3432 children.add(new Property("dynamicValue", "", "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 3433 children.add(new Property("action", "@PlanDefinition.action", "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 0, java.lang.Integer.MAX_VALUE, action)); 3434 } 3435 3436 @Override 3437 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3438 switch (_hash) { 3439 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the PlanDefinition to allow linkage within the realized CarePlan and/or RequestOrchestration.", 0, 1, linkId); 3440 case -980110702: /*prefix*/ return new Property("prefix", "string", "A user-visible prefix for the action. For example a section or item numbering such as 1. or A.", 0, 1, prefix); 3441 case 110371416: /*title*/ return new Property("title", "string", "The textual description of the action displayed to a user. For example, when the action is a test to be performed, the title would be the title of the test such as Assay by HPLC.", 0, 1, title); 3442 case -1724546052: /*description*/ return new Property("description", "markdown", "A brief description of the action used to provide a summary to display to the user.", 0, 1, description); 3443 case -900391049: /*textEquivalent*/ return new Property("textEquivalent", "markdown", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 0, 1, textEquivalent); 3444 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority); 3445 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that provides a meaning, grouping, or classification for the action or action group. For example, a section may have a LOINC code for the section of a documentation template. In pharmaceutical quality, an action (Test) such as pH could be classified as a physical property.", 0, 1, code); 3446 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason); 3447 case 1587405498: /*documentation*/ return new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation); 3448 case -1240658034: /*goalId*/ return new Property("goalId", "id", "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. In pharmaceutical quality, a goal represents acceptance criteria (Goal) for a given action (Test), so the goalId would be the unique id of a defined goal element establishing the acceptance criteria for the action.", 0, java.lang.Integer.MAX_VALUE, goalId); 3449 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)|canonical", "A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3450 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)|canonical", "A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3451 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3452 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group)", "A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3453 case -1768521432: /*subjectCanonical*/ return new Property("subject[x]", "canonical", "A code, group definition, or canonical reference that describes the intended subject of the action and its children, if any. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3454 case -1059891784: /*trigger*/ return new Property("trigger", "TriggerDefinition", "A description of when the action should be triggered. When multiple triggers are specified on an action, any triggering event invokes the action.", 0, java.lang.Integer.MAX_VALUE, trigger); 3455 case -861311717: /*condition*/ return new Property("condition", "", "An expression that describes applicability criteria or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition); 3456 case 100358090: /*input*/ return new Property("input", "", "Defines input data requirements for the action.", 0, java.lang.Integer.MAX_VALUE, input); 3457 case -1005512447: /*output*/ return new Property("output", "", "Defines the outputs of the action, if any.", 0, java.lang.Integer.MAX_VALUE, output); 3458 case -384107967: /*relatedAction*/ return new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction); 3459 case 164632566: /*timing[x]*/ return new Property("timing[x]", "Age|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3460 case -873664438: /*timing*/ return new Property("timing[x]", "Age|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3461 case 164607061: /*timingAge*/ return new Property("timing[x]", "Age", "An optional value describing when the action should be performed.", 0, 1, timing); 3462 case -1327253506: /*timingDuration*/ return new Property("timing[x]", "Duration", "An optional value describing when the action should be performed.", 0, 1, timing); 3463 case -710871277: /*timingRange*/ return new Property("timing[x]", "Range", "An optional value describing when the action should be performed.", 0, 1, timing); 3464 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3465 case 1901043637: /*location*/ return new Property("location", "CodeableReference(Location)", "Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location); 3466 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant); 3467 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 1, type); 3468 case 586678389: /*groupingBehavior*/ return new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 3469 case 168639486: /*selectionBehavior*/ return new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 3470 case -1163906287: /*requiredBehavior*/ return new Property("requiredBehavior", "code", "Defines the required behavior for the action.", 0, 1, requiredBehavior); 3471 case -1174249033: /*precheckBehavior*/ return new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 3472 case -922577408: /*cardinalityBehavior*/ return new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 3473 case -1139422643: /*definition[x]*/ return new Property("definition[x]", "canonical(ActivityDefinition|MessageDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)|uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 3474 case -1014418093: /*definition*/ return new Property("definition[x]", "canonical(ActivityDefinition|MessageDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)|uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 3475 case 933485793: /*definitionCanonical*/ return new Property("definition[x]", "canonical(ActivityDefinition|MessageDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)", "A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 3476 case -1139428583: /*definitionUri*/ return new Property("definition[x]", "uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a MessageDefinition describing a message to be snet, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 3477 case 1052666732: /*transform*/ return new Property("transform", "canonical(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform); 3478 case 572625010: /*dynamicValue*/ return new Property("dynamicValue", "", "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue); 3479 case -1422950858: /*action*/ return new Property("action", "@PlanDefinition.action", "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 0, java.lang.Integer.MAX_VALUE, action); 3480 default: return super.getNamedProperty(_hash, _name, _checkValid); 3481 } 3482 3483 } 3484 3485 @Override 3486 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3487 switch (hash) { 3488 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 3489 case -980110702: /*prefix*/ return this.prefix == null ? new Base[0] : new Base[] {this.prefix}; // StringType 3490 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3491 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3492 case -900391049: /*textEquivalent*/ return this.textEquivalent == null ? new Base[0] : new Base[] {this.textEquivalent}; // MarkdownType 3493 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 3494 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3495 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 3496 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 3497 case -1240658034: /*goalId*/ return this.goalId == null ? new Base[0] : this.goalId.toArray(new Base[this.goalId.size()]); // IdType 3498 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 3499 case -1059891784: /*trigger*/ return this.trigger == null ? new Base[0] : this.trigger.toArray(new Base[this.trigger.size()]); // TriggerDefinition 3500 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // PlanDefinitionActionConditionComponent 3501 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // PlanDefinitionActionInputComponent 3502 case -1005512447: /*output*/ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // PlanDefinitionActionOutputComponent 3503 case -384107967: /*relatedAction*/ return this.relatedAction == null ? new Base[0] : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // PlanDefinitionActionRelatedActionComponent 3504 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 3505 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // CodeableReference 3506 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // PlanDefinitionActionParticipantComponent 3507 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3508 case 586678389: /*groupingBehavior*/ return this.groupingBehavior == null ? new Base[0] : new Base[] {this.groupingBehavior}; // Enumeration<ActionGroupingBehavior> 3509 case 168639486: /*selectionBehavior*/ return this.selectionBehavior == null ? new Base[0] : new Base[] {this.selectionBehavior}; // Enumeration<ActionSelectionBehavior> 3510 case -1163906287: /*requiredBehavior*/ return this.requiredBehavior == null ? new Base[0] : new Base[] {this.requiredBehavior}; // Enumeration<ActionRequiredBehavior> 3511 case -1174249033: /*precheckBehavior*/ return this.precheckBehavior == null ? new Base[0] : new Base[] {this.precheckBehavior}; // Enumeration<ActionPrecheckBehavior> 3512 case -922577408: /*cardinalityBehavior*/ return this.cardinalityBehavior == null ? new Base[0] : new Base[] {this.cardinalityBehavior}; // Enumeration<ActionCardinalityBehavior> 3513 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // DataType 3514 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // CanonicalType 3515 case 572625010: /*dynamicValue*/ return this.dynamicValue == null ? new Base[0] : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // PlanDefinitionActionDynamicValueComponent 3516 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 3517 default: return super.getProperty(hash, name, checkValid); 3518 } 3519 3520 } 3521 3522 @Override 3523 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3524 switch (hash) { 3525 case -1102667083: // linkId 3526 this.linkId = TypeConvertor.castToString(value); // StringType 3527 return value; 3528 case -980110702: // prefix 3529 this.prefix = TypeConvertor.castToString(value); // StringType 3530 return value; 3531 case 110371416: // title 3532 this.title = TypeConvertor.castToString(value); // StringType 3533 return value; 3534 case -1724546052: // description 3535 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3536 return value; 3537 case -900391049: // textEquivalent 3538 this.textEquivalent = TypeConvertor.castToMarkdown(value); // MarkdownType 3539 return value; 3540 case -1165461084: // priority 3541 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3542 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3543 return value; 3544 case 3059181: // code 3545 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3546 return value; 3547 case -934964668: // reason 3548 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3549 return value; 3550 case 1587405498: // documentation 3551 this.getDocumentation().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 3552 return value; 3553 case -1240658034: // goalId 3554 this.getGoalId().add(TypeConvertor.castToId(value)); // IdType 3555 return value; 3556 case -1867885268: // subject 3557 this.subject = TypeConvertor.castToType(value); // DataType 3558 return value; 3559 case -1059891784: // trigger 3560 this.getTrigger().add(TypeConvertor.castToTriggerDefinition(value)); // TriggerDefinition 3561 return value; 3562 case -861311717: // condition 3563 this.getCondition().add((PlanDefinitionActionConditionComponent) value); // PlanDefinitionActionConditionComponent 3564 return value; 3565 case 100358090: // input 3566 this.getInput().add((PlanDefinitionActionInputComponent) value); // PlanDefinitionActionInputComponent 3567 return value; 3568 case -1005512447: // output 3569 this.getOutput().add((PlanDefinitionActionOutputComponent) value); // PlanDefinitionActionOutputComponent 3570 return value; 3571 case -384107967: // relatedAction 3572 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); // PlanDefinitionActionRelatedActionComponent 3573 return value; 3574 case -873664438: // timing 3575 this.timing = TypeConvertor.castToType(value); // DataType 3576 return value; 3577 case 1901043637: // location 3578 this.location = TypeConvertor.castToCodeableReference(value); // CodeableReference 3579 return value; 3580 case 767422259: // participant 3581 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); // PlanDefinitionActionParticipantComponent 3582 return value; 3583 case 3575610: // type 3584 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3585 return value; 3586 case 586678389: // groupingBehavior 3587 value = new ActionGroupingBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3588 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3589 return value; 3590 case 168639486: // selectionBehavior 3591 value = new ActionSelectionBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3592 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3593 return value; 3594 case -1163906287: // requiredBehavior 3595 value = new ActionRequiredBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3596 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3597 return value; 3598 case -1174249033: // precheckBehavior 3599 value = new ActionPrecheckBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3600 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3601 return value; 3602 case -922577408: // cardinalityBehavior 3603 value = new ActionCardinalityBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3604 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3605 return value; 3606 case -1014418093: // definition 3607 this.definition = TypeConvertor.castToType(value); // DataType 3608 return value; 3609 case 1052666732: // transform 3610 this.transform = TypeConvertor.castToCanonical(value); // CanonicalType 3611 return value; 3612 case 572625010: // dynamicValue 3613 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); // PlanDefinitionActionDynamicValueComponent 3614 return value; 3615 case -1422950858: // action 3616 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 3617 return value; 3618 default: return super.setProperty(hash, name, value); 3619 } 3620 3621 } 3622 3623 @Override 3624 public Base setProperty(String name, Base value) throws FHIRException { 3625 if (name.equals("linkId")) { 3626 this.linkId = TypeConvertor.castToString(value); // StringType 3627 } else if (name.equals("prefix")) { 3628 this.prefix = TypeConvertor.castToString(value); // StringType 3629 } else if (name.equals("title")) { 3630 this.title = TypeConvertor.castToString(value); // StringType 3631 } else if (name.equals("description")) { 3632 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3633 } else if (name.equals("textEquivalent")) { 3634 this.textEquivalent = TypeConvertor.castToMarkdown(value); // MarkdownType 3635 } else if (name.equals("priority")) { 3636 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3637 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3638 } else if (name.equals("code")) { 3639 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3640 } else if (name.equals("reason")) { 3641 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); 3642 } else if (name.equals("documentation")) { 3643 this.getDocumentation().add(TypeConvertor.castToRelatedArtifact(value)); 3644 } else if (name.equals("goalId")) { 3645 this.getGoalId().add(TypeConvertor.castToId(value)); 3646 } else if (name.equals("subject[x]")) { 3647 this.subject = TypeConvertor.castToType(value); // DataType 3648 } else if (name.equals("trigger")) { 3649 this.getTrigger().add(TypeConvertor.castToTriggerDefinition(value)); 3650 } else if (name.equals("condition")) { 3651 this.getCondition().add((PlanDefinitionActionConditionComponent) value); 3652 } else if (name.equals("input")) { 3653 this.getInput().add((PlanDefinitionActionInputComponent) value); 3654 } else if (name.equals("output")) { 3655 this.getOutput().add((PlanDefinitionActionOutputComponent) value); 3656 } else if (name.equals("relatedAction")) { 3657 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); 3658 } else if (name.equals("timing[x]")) { 3659 this.timing = TypeConvertor.castToType(value); // DataType 3660 } else if (name.equals("location")) { 3661 this.location = TypeConvertor.castToCodeableReference(value); // CodeableReference 3662 } else if (name.equals("participant")) { 3663 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); 3664 } else if (name.equals("type")) { 3665 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3666 } else if (name.equals("groupingBehavior")) { 3667 value = new ActionGroupingBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3668 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3669 } else if (name.equals("selectionBehavior")) { 3670 value = new ActionSelectionBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3671 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3672 } else if (name.equals("requiredBehavior")) { 3673 value = new ActionRequiredBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3674 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3675 } else if (name.equals("precheckBehavior")) { 3676 value = new ActionPrecheckBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3677 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3678 } else if (name.equals("cardinalityBehavior")) { 3679 value = new ActionCardinalityBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3680 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3681 } else if (name.equals("definition[x]")) { 3682 this.definition = TypeConvertor.castToType(value); // DataType 3683 } else if (name.equals("transform")) { 3684 this.transform = TypeConvertor.castToCanonical(value); // CanonicalType 3685 } else if (name.equals("dynamicValue")) { 3686 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); 3687 } else if (name.equals("action")) { 3688 this.getAction().add((PlanDefinitionActionComponent) value); 3689 } else 3690 return super.setProperty(name, value); 3691 return value; 3692 } 3693 3694 @Override 3695 public void removeChild(String name, Base value) throws FHIRException { 3696 if (name.equals("linkId")) { 3697 this.linkId = null; 3698 } else if (name.equals("prefix")) { 3699 this.prefix = null; 3700 } else if (name.equals("title")) { 3701 this.title = null; 3702 } else if (name.equals("description")) { 3703 this.description = null; 3704 } else if (name.equals("textEquivalent")) { 3705 this.textEquivalent = null; 3706 } else if (name.equals("priority")) { 3707 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3708 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3709 } else if (name.equals("code")) { 3710 this.code = null; 3711 } else if (name.equals("reason")) { 3712 this.getReason().remove(value); 3713 } else if (name.equals("documentation")) { 3714 this.getDocumentation().remove(value); 3715 } else if (name.equals("goalId")) { 3716 this.getGoalId().remove(value); 3717 } else if (name.equals("subject[x]")) { 3718 this.subject = null; 3719 } else if (name.equals("trigger")) { 3720 this.getTrigger().remove(value); 3721 } else if (name.equals("condition")) { 3722 this.getCondition().remove((PlanDefinitionActionConditionComponent) value); 3723 } else if (name.equals("input")) { 3724 this.getInput().remove((PlanDefinitionActionInputComponent) value); 3725 } else if (name.equals("output")) { 3726 this.getOutput().remove((PlanDefinitionActionOutputComponent) value); 3727 } else if (name.equals("relatedAction")) { 3728 this.getRelatedAction().remove((PlanDefinitionActionRelatedActionComponent) value); 3729 } else if (name.equals("timing[x]")) { 3730 this.timing = null; 3731 } else if (name.equals("location")) { 3732 this.location = null; 3733 } else if (name.equals("participant")) { 3734 this.getParticipant().remove((PlanDefinitionActionParticipantComponent) value); 3735 } else if (name.equals("type")) { 3736 this.type = null; 3737 } else if (name.equals("groupingBehavior")) { 3738 value = new ActionGroupingBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3739 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3740 } else if (name.equals("selectionBehavior")) { 3741 value = new ActionSelectionBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3742 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3743 } else if (name.equals("requiredBehavior")) { 3744 value = new ActionRequiredBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3745 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3746 } else if (name.equals("precheckBehavior")) { 3747 value = new ActionPrecheckBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3748 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3749 } else if (name.equals("cardinalityBehavior")) { 3750 value = new ActionCardinalityBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 3751 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3752 } else if (name.equals("definition[x]")) { 3753 this.definition = null; 3754 } else if (name.equals("transform")) { 3755 this.transform = null; 3756 } else if (name.equals("dynamicValue")) { 3757 this.getDynamicValue().remove((PlanDefinitionActionDynamicValueComponent) value); 3758 } else if (name.equals("action")) { 3759 this.getAction().remove((PlanDefinitionActionComponent) value); 3760 } else 3761 super.removeChild(name, value); 3762 3763 } 3764 3765 @Override 3766 public Base makeProperty(int hash, String name) throws FHIRException { 3767 switch (hash) { 3768 case -1102667083: return getLinkIdElement(); 3769 case -980110702: return getPrefixElement(); 3770 case 110371416: return getTitleElement(); 3771 case -1724546052: return getDescriptionElement(); 3772 case -900391049: return getTextEquivalentElement(); 3773 case -1165461084: return getPriorityElement(); 3774 case 3059181: return getCode(); 3775 case -934964668: return addReason(); 3776 case 1587405498: return addDocumentation(); 3777 case -1240658034: return addGoalIdElement(); 3778 case -573640748: return getSubject(); 3779 case -1867885268: return getSubject(); 3780 case -1059891784: return addTrigger(); 3781 case -861311717: return addCondition(); 3782 case 100358090: return addInput(); 3783 case -1005512447: return addOutput(); 3784 case -384107967: return addRelatedAction(); 3785 case 164632566: return getTiming(); 3786 case -873664438: return getTiming(); 3787 case 1901043637: return getLocation(); 3788 case 767422259: return addParticipant(); 3789 case 3575610: return getType(); 3790 case 586678389: return getGroupingBehaviorElement(); 3791 case 168639486: return getSelectionBehaviorElement(); 3792 case -1163906287: return getRequiredBehaviorElement(); 3793 case -1174249033: return getPrecheckBehaviorElement(); 3794 case -922577408: return getCardinalityBehaviorElement(); 3795 case -1139422643: return getDefinition(); 3796 case -1014418093: return getDefinition(); 3797 case 1052666732: return getTransformElement(); 3798 case 572625010: return addDynamicValue(); 3799 case -1422950858: return addAction(); 3800 default: return super.makeProperty(hash, name); 3801 } 3802 3803 } 3804 3805 @Override 3806 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3807 switch (hash) { 3808 case -1102667083: /*linkId*/ return new String[] {"string"}; 3809 case -980110702: /*prefix*/ return new String[] {"string"}; 3810 case 110371416: /*title*/ return new String[] {"string"}; 3811 case -1724546052: /*description*/ return new String[] {"markdown"}; 3812 case -900391049: /*textEquivalent*/ return new String[] {"markdown"}; 3813 case -1165461084: /*priority*/ return new String[] {"code"}; 3814 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3815 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 3816 case 1587405498: /*documentation*/ return new String[] {"RelatedArtifact"}; 3817 case -1240658034: /*goalId*/ return new String[] {"id"}; 3818 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference", "canonical"}; 3819 case -1059891784: /*trigger*/ return new String[] {"TriggerDefinition"}; 3820 case -861311717: /*condition*/ return new String[] {}; 3821 case 100358090: /*input*/ return new String[] {}; 3822 case -1005512447: /*output*/ return new String[] {}; 3823 case -384107967: /*relatedAction*/ return new String[] {}; 3824 case -873664438: /*timing*/ return new String[] {"Age", "Duration", "Range", "Timing"}; 3825 case 1901043637: /*location*/ return new String[] {"CodeableReference"}; 3826 case 767422259: /*participant*/ return new String[] {}; 3827 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3828 case 586678389: /*groupingBehavior*/ return new String[] {"code"}; 3829 case 168639486: /*selectionBehavior*/ return new String[] {"code"}; 3830 case -1163906287: /*requiredBehavior*/ return new String[] {"code"}; 3831 case -1174249033: /*precheckBehavior*/ return new String[] {"code"}; 3832 case -922577408: /*cardinalityBehavior*/ return new String[] {"code"}; 3833 case -1014418093: /*definition*/ return new String[] {"canonical", "uri"}; 3834 case 1052666732: /*transform*/ return new String[] {"canonical"}; 3835 case 572625010: /*dynamicValue*/ return new String[] {}; 3836 case -1422950858: /*action*/ return new String[] {"@PlanDefinition.action"}; 3837 default: return super.getTypesForProperty(hash, name); 3838 } 3839 3840 } 3841 3842 @Override 3843 public Base addChild(String name) throws FHIRException { 3844 if (name.equals("linkId")) { 3845 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.linkId"); 3846 } 3847 else if (name.equals("prefix")) { 3848 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.prefix"); 3849 } 3850 else if (name.equals("title")) { 3851 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.title"); 3852 } 3853 else if (name.equals("description")) { 3854 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.description"); 3855 } 3856 else if (name.equals("textEquivalent")) { 3857 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.textEquivalent"); 3858 } 3859 else if (name.equals("priority")) { 3860 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.priority"); 3861 } 3862 else if (name.equals("code")) { 3863 this.code = new CodeableConcept(); 3864 return this.code; 3865 } 3866 else if (name.equals("reason")) { 3867 return addReason(); 3868 } 3869 else if (name.equals("documentation")) { 3870 return addDocumentation(); 3871 } 3872 else if (name.equals("goalId")) { 3873 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.goalId"); 3874 } 3875 else if (name.equals("subjectCodeableConcept")) { 3876 this.subject = new CodeableConcept(); 3877 return this.subject; 3878 } 3879 else if (name.equals("subjectReference")) { 3880 this.subject = new Reference(); 3881 return this.subject; 3882 } 3883 else if (name.equals("subjectCanonical")) { 3884 this.subject = new CanonicalType(); 3885 return this.subject; 3886 } 3887 else if (name.equals("trigger")) { 3888 return addTrigger(); 3889 } 3890 else if (name.equals("condition")) { 3891 return addCondition(); 3892 } 3893 else if (name.equals("input")) { 3894 return addInput(); 3895 } 3896 else if (name.equals("output")) { 3897 return addOutput(); 3898 } 3899 else if (name.equals("relatedAction")) { 3900 return addRelatedAction(); 3901 } 3902 else if (name.equals("timingAge")) { 3903 this.timing = new Age(); 3904 return this.timing; 3905 } 3906 else if (name.equals("timingDuration")) { 3907 this.timing = new Duration(); 3908 return this.timing; 3909 } 3910 else if (name.equals("timingRange")) { 3911 this.timing = new Range(); 3912 return this.timing; 3913 } 3914 else if (name.equals("timingTiming")) { 3915 this.timing = new Timing(); 3916 return this.timing; 3917 } 3918 else if (name.equals("location")) { 3919 this.location = new CodeableReference(); 3920 return this.location; 3921 } 3922 else if (name.equals("participant")) { 3923 return addParticipant(); 3924 } 3925 else if (name.equals("type")) { 3926 this.type = new CodeableConcept(); 3927 return this.type; 3928 } 3929 else if (name.equals("groupingBehavior")) { 3930 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.groupingBehavior"); 3931 } 3932 else if (name.equals("selectionBehavior")) { 3933 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.selectionBehavior"); 3934 } 3935 else if (name.equals("requiredBehavior")) { 3936 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.requiredBehavior"); 3937 } 3938 else if (name.equals("precheckBehavior")) { 3939 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.precheckBehavior"); 3940 } 3941 else if (name.equals("cardinalityBehavior")) { 3942 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.cardinalityBehavior"); 3943 } 3944 else if (name.equals("definitionCanonical")) { 3945 this.definition = new CanonicalType(); 3946 return this.definition; 3947 } 3948 else if (name.equals("definitionUri")) { 3949 this.definition = new UriType(); 3950 return this.definition; 3951 } 3952 else if (name.equals("transform")) { 3953 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.transform"); 3954 } 3955 else if (name.equals("dynamicValue")) { 3956 return addDynamicValue(); 3957 } 3958 else if (name.equals("action")) { 3959 return addAction(); 3960 } 3961 else 3962 return super.addChild(name); 3963 } 3964 3965 public PlanDefinitionActionComponent copy() { 3966 PlanDefinitionActionComponent dst = new PlanDefinitionActionComponent(); 3967 copyValues(dst); 3968 return dst; 3969 } 3970 3971 public void copyValues(PlanDefinitionActionComponent dst) { 3972 super.copyValues(dst); 3973 dst.linkId = linkId == null ? null : linkId.copy(); 3974 dst.prefix = prefix == null ? null : prefix.copy(); 3975 dst.title = title == null ? null : title.copy(); 3976 dst.description = description == null ? null : description.copy(); 3977 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 3978 dst.priority = priority == null ? null : priority.copy(); 3979 dst.code = code == null ? null : code.copy(); 3980 if (reason != null) { 3981 dst.reason = new ArrayList<CodeableConcept>(); 3982 for (CodeableConcept i : reason) 3983 dst.reason.add(i.copy()); 3984 }; 3985 if (documentation != null) { 3986 dst.documentation = new ArrayList<RelatedArtifact>(); 3987 for (RelatedArtifact i : documentation) 3988 dst.documentation.add(i.copy()); 3989 }; 3990 if (goalId != null) { 3991 dst.goalId = new ArrayList<IdType>(); 3992 for (IdType i : goalId) 3993 dst.goalId.add(i.copy()); 3994 }; 3995 dst.subject = subject == null ? null : subject.copy(); 3996 if (trigger != null) { 3997 dst.trigger = new ArrayList<TriggerDefinition>(); 3998 for (TriggerDefinition i : trigger) 3999 dst.trigger.add(i.copy()); 4000 }; 4001 if (condition != null) { 4002 dst.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 4003 for (PlanDefinitionActionConditionComponent i : condition) 4004 dst.condition.add(i.copy()); 4005 }; 4006 if (input != null) { 4007 dst.input = new ArrayList<PlanDefinitionActionInputComponent>(); 4008 for (PlanDefinitionActionInputComponent i : input) 4009 dst.input.add(i.copy()); 4010 }; 4011 if (output != null) { 4012 dst.output = new ArrayList<PlanDefinitionActionOutputComponent>(); 4013 for (PlanDefinitionActionOutputComponent i : output) 4014 dst.output.add(i.copy()); 4015 }; 4016 if (relatedAction != null) { 4017 dst.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 4018 for (PlanDefinitionActionRelatedActionComponent i : relatedAction) 4019 dst.relatedAction.add(i.copy()); 4020 }; 4021 dst.timing = timing == null ? null : timing.copy(); 4022 dst.location = location == null ? null : location.copy(); 4023 if (participant != null) { 4024 dst.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 4025 for (PlanDefinitionActionParticipantComponent i : participant) 4026 dst.participant.add(i.copy()); 4027 }; 4028 dst.type = type == null ? null : type.copy(); 4029 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 4030 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 4031 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 4032 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 4033 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 4034 dst.definition = definition == null ? null : definition.copy(); 4035 dst.transform = transform == null ? null : transform.copy(); 4036 if (dynamicValue != null) { 4037 dst.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 4038 for (PlanDefinitionActionDynamicValueComponent i : dynamicValue) 4039 dst.dynamicValue.add(i.copy()); 4040 }; 4041 if (action != null) { 4042 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 4043 for (PlanDefinitionActionComponent i : action) 4044 dst.action.add(i.copy()); 4045 }; 4046 } 4047 4048 @Override 4049 public boolean equalsDeep(Base other_) { 4050 if (!super.equalsDeep(other_)) 4051 return false; 4052 if (!(other_ instanceof PlanDefinitionActionComponent)) 4053 return false; 4054 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 4055 return compareDeep(linkId, o.linkId, true) && compareDeep(prefix, o.prefix, true) && compareDeep(title, o.title, true) 4056 && compareDeep(description, o.description, true) && compareDeep(textEquivalent, o.textEquivalent, true) 4057 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) && compareDeep(reason, o.reason, true) 4058 && compareDeep(documentation, o.documentation, true) && compareDeep(goalId, o.goalId, true) && compareDeep(subject, o.subject, true) 4059 && compareDeep(trigger, o.trigger, true) && compareDeep(condition, o.condition, true) && compareDeep(input, o.input, true) 4060 && compareDeep(output, o.output, true) && compareDeep(relatedAction, o.relatedAction, true) && compareDeep(timing, o.timing, true) 4061 && compareDeep(location, o.location, true) && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 4062 && compareDeep(groupingBehavior, o.groupingBehavior, true) && compareDeep(selectionBehavior, o.selectionBehavior, true) 4063 && compareDeep(requiredBehavior, o.requiredBehavior, true) && compareDeep(precheckBehavior, o.precheckBehavior, true) 4064 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) && compareDeep(definition, o.definition, true) 4065 && compareDeep(transform, o.transform, true) && compareDeep(dynamicValue, o.dynamicValue, true) 4066 && compareDeep(action, o.action, true); 4067 } 4068 4069 @Override 4070 public boolean equalsShallow(Base other_) { 4071 if (!super.equalsShallow(other_)) 4072 return false; 4073 if (!(other_ instanceof PlanDefinitionActionComponent)) 4074 return false; 4075 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 4076 return compareValues(linkId, o.linkId, true) && compareValues(prefix, o.prefix, true) && compareValues(title, o.title, true) 4077 && compareValues(description, o.description, true) && compareValues(textEquivalent, o.textEquivalent, true) 4078 && compareValues(priority, o.priority, true) && compareValues(goalId, o.goalId, true) && compareValues(groupingBehavior, o.groupingBehavior, true) 4079 && compareValues(selectionBehavior, o.selectionBehavior, true) && compareValues(requiredBehavior, o.requiredBehavior, true) 4080 && compareValues(precheckBehavior, o.precheckBehavior, true) && compareValues(cardinalityBehavior, o.cardinalityBehavior, true) 4081 && compareValues(transform, o.transform, true); 4082 } 4083 4084 public boolean isEmpty() { 4085 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, prefix, title, description 4086 , textEquivalent, priority, code, reason, documentation, goalId, subject, trigger 4087 , condition, input, output, relatedAction, timing, location, participant, type 4088 , groupingBehavior, selectionBehavior, requiredBehavior, precheckBehavior, cardinalityBehavior 4089 , definition, transform, dynamicValue, action); 4090 } 4091 4092 public String fhirType() { 4093 return "PlanDefinition.action"; 4094 4095 } 4096 4097 } 4098 4099 @Block() 4100 public static class PlanDefinitionActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 4101 /** 4102 * The kind of condition. 4103 */ 4104 @Child(name = "kind", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4105 @Description(shortDefinition="applicability | start | stop", formalDefinition="The kind of condition." ) 4106 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-condition-kind") 4107 protected Enumeration<ActionConditionKind> kind; 4108 4109 /** 4110 * An expression that returns true or false, indicating whether the condition is satisfied. 4111 */ 4112 @Child(name = "expression", type = {Expression.class}, order=2, min=0, max=1, modifier=false, summary=false) 4113 @Description(shortDefinition="Boolean-valued expression", formalDefinition="An expression that returns true or false, indicating whether the condition is satisfied." ) 4114 protected Expression expression; 4115 4116 private static final long serialVersionUID = -455150438L; 4117 4118 /** 4119 * Constructor 4120 */ 4121 public PlanDefinitionActionConditionComponent() { 4122 super(); 4123 } 4124 4125 /** 4126 * Constructor 4127 */ 4128 public PlanDefinitionActionConditionComponent(ActionConditionKind kind) { 4129 super(); 4130 this.setKind(kind); 4131 } 4132 4133 /** 4134 * @return {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4135 */ 4136 public Enumeration<ActionConditionKind> getKindElement() { 4137 if (this.kind == null) 4138 if (Configuration.errorOnAutoCreate()) 4139 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.kind"); 4140 else if (Configuration.doAutoCreate()) 4141 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 4142 return this.kind; 4143 } 4144 4145 public boolean hasKindElement() { 4146 return this.kind != null && !this.kind.isEmpty(); 4147 } 4148 4149 public boolean hasKind() { 4150 return this.kind != null && !this.kind.isEmpty(); 4151 } 4152 4153 /** 4154 * @param value {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4155 */ 4156 public PlanDefinitionActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 4157 this.kind = value; 4158 return this; 4159 } 4160 4161 /** 4162 * @return The kind of condition. 4163 */ 4164 public ActionConditionKind getKind() { 4165 return this.kind == null ? null : this.kind.getValue(); 4166 } 4167 4168 /** 4169 * @param value The kind of condition. 4170 */ 4171 public PlanDefinitionActionConditionComponent setKind(ActionConditionKind value) { 4172 if (this.kind == null) 4173 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 4174 this.kind.setValue(value); 4175 return this; 4176 } 4177 4178 /** 4179 * @return {@link #expression} (An expression that returns true or false, indicating whether the condition is satisfied.) 4180 */ 4181 public Expression getExpression() { 4182 if (this.expression == null) 4183 if (Configuration.errorOnAutoCreate()) 4184 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.expression"); 4185 else if (Configuration.doAutoCreate()) 4186 this.expression = new Expression(); // cc 4187 return this.expression; 4188 } 4189 4190 public boolean hasExpression() { 4191 return this.expression != null && !this.expression.isEmpty(); 4192 } 4193 4194 /** 4195 * @param value {@link #expression} (An expression that returns true or false, indicating whether the condition is satisfied.) 4196 */ 4197 public PlanDefinitionActionConditionComponent setExpression(Expression value) { 4198 this.expression = value; 4199 return this; 4200 } 4201 4202 protected void listChildren(List<Property> children) { 4203 super.listChildren(children); 4204 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 4205 children.add(new Property("expression", "Expression", "An expression that returns true or false, indicating whether the condition is satisfied.", 0, 1, expression)); 4206 } 4207 4208 @Override 4209 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4210 switch (_hash) { 4211 case 3292052: /*kind*/ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 4212 case -1795452264: /*expression*/ return new Property("expression", "Expression", "An expression that returns true or false, indicating whether the condition is satisfied.", 0, 1, expression); 4213 default: return super.getNamedProperty(_hash, _name, _checkValid); 4214 } 4215 4216 } 4217 4218 @Override 4219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4220 switch (hash) { 4221 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<ActionConditionKind> 4222 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 4223 default: return super.getProperty(hash, name, checkValid); 4224 } 4225 4226 } 4227 4228 @Override 4229 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4230 switch (hash) { 4231 case 3292052: // kind 4232 value = new ActionConditionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4233 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 4234 return value; 4235 case -1795452264: // expression 4236 this.expression = TypeConvertor.castToExpression(value); // Expression 4237 return value; 4238 default: return super.setProperty(hash, name, value); 4239 } 4240 4241 } 4242 4243 @Override 4244 public Base setProperty(String name, Base value) throws FHIRException { 4245 if (name.equals("kind")) { 4246 value = new ActionConditionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4247 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 4248 } else if (name.equals("expression")) { 4249 this.expression = TypeConvertor.castToExpression(value); // Expression 4250 } else 4251 return super.setProperty(name, value); 4252 return value; 4253 } 4254 4255 @Override 4256 public void removeChild(String name, Base value) throws FHIRException { 4257 if (name.equals("kind")) { 4258 value = new ActionConditionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4259 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 4260 } else if (name.equals("expression")) { 4261 this.expression = null; 4262 } else 4263 super.removeChild(name, value); 4264 4265 } 4266 4267 @Override 4268 public Base makeProperty(int hash, String name) throws FHIRException { 4269 switch (hash) { 4270 case 3292052: return getKindElement(); 4271 case -1795452264: return getExpression(); 4272 default: return super.makeProperty(hash, name); 4273 } 4274 4275 } 4276 4277 @Override 4278 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4279 switch (hash) { 4280 case 3292052: /*kind*/ return new String[] {"code"}; 4281 case -1795452264: /*expression*/ return new String[] {"Expression"}; 4282 default: return super.getTypesForProperty(hash, name); 4283 } 4284 4285 } 4286 4287 @Override 4288 public Base addChild(String name) throws FHIRException { 4289 if (name.equals("kind")) { 4290 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.condition.kind"); 4291 } 4292 else if (name.equals("expression")) { 4293 this.expression = new Expression(); 4294 return this.expression; 4295 } 4296 else 4297 return super.addChild(name); 4298 } 4299 4300 public PlanDefinitionActionConditionComponent copy() { 4301 PlanDefinitionActionConditionComponent dst = new PlanDefinitionActionConditionComponent(); 4302 copyValues(dst); 4303 return dst; 4304 } 4305 4306 public void copyValues(PlanDefinitionActionConditionComponent dst) { 4307 super.copyValues(dst); 4308 dst.kind = kind == null ? null : kind.copy(); 4309 dst.expression = expression == null ? null : expression.copy(); 4310 } 4311 4312 @Override 4313 public boolean equalsDeep(Base other_) { 4314 if (!super.equalsDeep(other_)) 4315 return false; 4316 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 4317 return false; 4318 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 4319 return compareDeep(kind, o.kind, true) && compareDeep(expression, o.expression, true); 4320 } 4321 4322 @Override 4323 public boolean equalsShallow(Base other_) { 4324 if (!super.equalsShallow(other_)) 4325 return false; 4326 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 4327 return false; 4328 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 4329 return compareValues(kind, o.kind, true); 4330 } 4331 4332 public boolean isEmpty() { 4333 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, expression); 4334 } 4335 4336 public String fhirType() { 4337 return "PlanDefinition.action.condition"; 4338 4339 } 4340 4341 } 4342 4343 @Block() 4344 public static class PlanDefinitionActionInputComponent extends BackboneElement implements IBaseBackboneElement { 4345 /** 4346 * A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 4347 */ 4348 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 4349 @Description(shortDefinition="User-visible title", formalDefinition="A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers." ) 4350 protected StringType title; 4351 4352 /** 4353 * Defines the data that is to be provided as input to the action. 4354 */ 4355 @Child(name = "requirement", type = {DataRequirement.class}, order=2, min=0, max=1, modifier=false, summary=false) 4356 @Description(shortDefinition="What data is provided", formalDefinition="Defines the data that is to be provided as input to the action." ) 4357 protected DataRequirement requirement; 4358 4359 /** 4360 * Points to an existing input or output element that provides data to this input. 4361 */ 4362 @Child(name = "relatedData", type = {IdType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4363 @Description(shortDefinition="What data is provided", formalDefinition="Points to an existing input or output element that provides data to this input." ) 4364 protected IdType relatedData; 4365 4366 private static final long serialVersionUID = -1064046709L; 4367 4368 /** 4369 * Constructor 4370 */ 4371 public PlanDefinitionActionInputComponent() { 4372 super(); 4373 } 4374 4375 /** 4376 * @return {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4377 */ 4378 public StringType getTitleElement() { 4379 if (this.title == null) 4380 if (Configuration.errorOnAutoCreate()) 4381 throw new Error("Attempt to auto-create PlanDefinitionActionInputComponent.title"); 4382 else if (Configuration.doAutoCreate()) 4383 this.title = new StringType(); // bb 4384 return this.title; 4385 } 4386 4387 public boolean hasTitleElement() { 4388 return this.title != null && !this.title.isEmpty(); 4389 } 4390 4391 public boolean hasTitle() { 4392 return this.title != null && !this.title.isEmpty(); 4393 } 4394 4395 /** 4396 * @param value {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4397 */ 4398 public PlanDefinitionActionInputComponent setTitleElement(StringType value) { 4399 this.title = value; 4400 return this; 4401 } 4402 4403 /** 4404 * @return A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 4405 */ 4406 public String getTitle() { 4407 return this.title == null ? null : this.title.getValue(); 4408 } 4409 4410 /** 4411 * @param value A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 4412 */ 4413 public PlanDefinitionActionInputComponent setTitle(String value) { 4414 if (Utilities.noString(value)) 4415 this.title = null; 4416 else { 4417 if (this.title == null) 4418 this.title = new StringType(); 4419 this.title.setValue(value); 4420 } 4421 return this; 4422 } 4423 4424 /** 4425 * @return {@link #requirement} (Defines the data that is to be provided as input to the action.) 4426 */ 4427 public DataRequirement getRequirement() { 4428 if (this.requirement == null) 4429 if (Configuration.errorOnAutoCreate()) 4430 throw new Error("Attempt to auto-create PlanDefinitionActionInputComponent.requirement"); 4431 else if (Configuration.doAutoCreate()) 4432 this.requirement = new DataRequirement(); // cc 4433 return this.requirement; 4434 } 4435 4436 public boolean hasRequirement() { 4437 return this.requirement != null && !this.requirement.isEmpty(); 4438 } 4439 4440 /** 4441 * @param value {@link #requirement} (Defines the data that is to be provided as input to the action.) 4442 */ 4443 public PlanDefinitionActionInputComponent setRequirement(DataRequirement value) { 4444 this.requirement = value; 4445 return this; 4446 } 4447 4448 /** 4449 * @return {@link #relatedData} (Points to an existing input or output element that provides data to this input.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 4450 */ 4451 public IdType getRelatedDataElement() { 4452 if (this.relatedData == null) 4453 if (Configuration.errorOnAutoCreate()) 4454 throw new Error("Attempt to auto-create PlanDefinitionActionInputComponent.relatedData"); 4455 else if (Configuration.doAutoCreate()) 4456 this.relatedData = new IdType(); // bb 4457 return this.relatedData; 4458 } 4459 4460 public boolean hasRelatedDataElement() { 4461 return this.relatedData != null && !this.relatedData.isEmpty(); 4462 } 4463 4464 public boolean hasRelatedData() { 4465 return this.relatedData != null && !this.relatedData.isEmpty(); 4466 } 4467 4468 /** 4469 * @param value {@link #relatedData} (Points to an existing input or output element that provides data to this input.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 4470 */ 4471 public PlanDefinitionActionInputComponent setRelatedDataElement(IdType value) { 4472 this.relatedData = value; 4473 return this; 4474 } 4475 4476 /** 4477 * @return Points to an existing input or output element that provides data to this input. 4478 */ 4479 public String getRelatedData() { 4480 return this.relatedData == null ? null : this.relatedData.getValue(); 4481 } 4482 4483 /** 4484 * @param value Points to an existing input or output element that provides data to this input. 4485 */ 4486 public PlanDefinitionActionInputComponent setRelatedData(String value) { 4487 if (Utilities.noString(value)) 4488 this.relatedData = null; 4489 else { 4490 if (this.relatedData == null) 4491 this.relatedData = new IdType(); 4492 this.relatedData.setValue(value); 4493 } 4494 return this; 4495 } 4496 4497 protected void listChildren(List<Property> children) { 4498 super.listChildren(children); 4499 children.add(new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title)); 4500 children.add(new Property("requirement", "DataRequirement", "Defines the data that is to be provided as input to the action.", 0, 1, requirement)); 4501 children.add(new Property("relatedData", "id", "Points to an existing input or output element that provides data to this input.", 0, 1, relatedData)); 4502 } 4503 4504 @Override 4505 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4506 switch (_hash) { 4507 case 110371416: /*title*/ return new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title); 4508 case 363387971: /*requirement*/ return new Property("requirement", "DataRequirement", "Defines the data that is to be provided as input to the action.", 0, 1, requirement); 4509 case 1112535669: /*relatedData*/ return new Property("relatedData", "id", "Points to an existing input or output element that provides data to this input.", 0, 1, relatedData); 4510 default: return super.getNamedProperty(_hash, _name, _checkValid); 4511 } 4512 4513 } 4514 4515 @Override 4516 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4517 switch (hash) { 4518 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4519 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // DataRequirement 4520 case 1112535669: /*relatedData*/ return this.relatedData == null ? new Base[0] : new Base[] {this.relatedData}; // IdType 4521 default: return super.getProperty(hash, name, checkValid); 4522 } 4523 4524 } 4525 4526 @Override 4527 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4528 switch (hash) { 4529 case 110371416: // title 4530 this.title = TypeConvertor.castToString(value); // StringType 4531 return value; 4532 case 363387971: // requirement 4533 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 4534 return value; 4535 case 1112535669: // relatedData 4536 this.relatedData = TypeConvertor.castToId(value); // IdType 4537 return value; 4538 default: return super.setProperty(hash, name, value); 4539 } 4540 4541 } 4542 4543 @Override 4544 public Base setProperty(String name, Base value) throws FHIRException { 4545 if (name.equals("title")) { 4546 this.title = TypeConvertor.castToString(value); // StringType 4547 } else if (name.equals("requirement")) { 4548 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 4549 } else if (name.equals("relatedData")) { 4550 this.relatedData = TypeConvertor.castToId(value); // IdType 4551 } else 4552 return super.setProperty(name, value); 4553 return value; 4554 } 4555 4556 @Override 4557 public void removeChild(String name, Base value) throws FHIRException { 4558 if (name.equals("title")) { 4559 this.title = null; 4560 } else if (name.equals("requirement")) { 4561 this.requirement = null; 4562 } else if (name.equals("relatedData")) { 4563 this.relatedData = null; 4564 } else 4565 super.removeChild(name, value); 4566 4567 } 4568 4569 @Override 4570 public Base makeProperty(int hash, String name) throws FHIRException { 4571 switch (hash) { 4572 case 110371416: return getTitleElement(); 4573 case 363387971: return getRequirement(); 4574 case 1112535669: return getRelatedDataElement(); 4575 default: return super.makeProperty(hash, name); 4576 } 4577 4578 } 4579 4580 @Override 4581 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4582 switch (hash) { 4583 case 110371416: /*title*/ return new String[] {"string"}; 4584 case 363387971: /*requirement*/ return new String[] {"DataRequirement"}; 4585 case 1112535669: /*relatedData*/ return new String[] {"id"}; 4586 default: return super.getTypesForProperty(hash, name); 4587 } 4588 4589 } 4590 4591 @Override 4592 public Base addChild(String name) throws FHIRException { 4593 if (name.equals("title")) { 4594 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.input.title"); 4595 } 4596 else if (name.equals("requirement")) { 4597 this.requirement = new DataRequirement(); 4598 return this.requirement; 4599 } 4600 else if (name.equals("relatedData")) { 4601 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.input.relatedData"); 4602 } 4603 else 4604 return super.addChild(name); 4605 } 4606 4607 public PlanDefinitionActionInputComponent copy() { 4608 PlanDefinitionActionInputComponent dst = new PlanDefinitionActionInputComponent(); 4609 copyValues(dst); 4610 return dst; 4611 } 4612 4613 public void copyValues(PlanDefinitionActionInputComponent dst) { 4614 super.copyValues(dst); 4615 dst.title = title == null ? null : title.copy(); 4616 dst.requirement = requirement == null ? null : requirement.copy(); 4617 dst.relatedData = relatedData == null ? null : relatedData.copy(); 4618 } 4619 4620 @Override 4621 public boolean equalsDeep(Base other_) { 4622 if (!super.equalsDeep(other_)) 4623 return false; 4624 if (!(other_ instanceof PlanDefinitionActionInputComponent)) 4625 return false; 4626 PlanDefinitionActionInputComponent o = (PlanDefinitionActionInputComponent) other_; 4627 return compareDeep(title, o.title, true) && compareDeep(requirement, o.requirement, true) && compareDeep(relatedData, o.relatedData, true) 4628 ; 4629 } 4630 4631 @Override 4632 public boolean equalsShallow(Base other_) { 4633 if (!super.equalsShallow(other_)) 4634 return false; 4635 if (!(other_ instanceof PlanDefinitionActionInputComponent)) 4636 return false; 4637 PlanDefinitionActionInputComponent o = (PlanDefinitionActionInputComponent) other_; 4638 return compareValues(title, o.title, true) && compareValues(relatedData, o.relatedData, true); 4639 } 4640 4641 public boolean isEmpty() { 4642 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, requirement, relatedData 4643 ); 4644 } 4645 4646 public String fhirType() { 4647 return "PlanDefinition.action.input"; 4648 4649 } 4650 4651 } 4652 4653 @Block() 4654 public static class PlanDefinitionActionOutputComponent extends BackboneElement implements IBaseBackboneElement { 4655 /** 4656 * A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 4657 */ 4658 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 4659 @Description(shortDefinition="User-visible title", formalDefinition="A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers." ) 4660 protected StringType title; 4661 4662 /** 4663 * Defines the data that results as output from the action. 4664 */ 4665 @Child(name = "requirement", type = {DataRequirement.class}, order=2, min=0, max=1, modifier=false, summary=false) 4666 @Description(shortDefinition="What data is provided", formalDefinition="Defines the data that results as output from the action." ) 4667 protected DataRequirement requirement; 4668 4669 /** 4670 * Points to an existing input or output element that is results as output from the action. 4671 */ 4672 @Child(name = "relatedData", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4673 @Description(shortDefinition="What data is provided", formalDefinition="Points to an existing input or output element that is results as output from the action." ) 4674 protected StringType relatedData; 4675 4676 private static final long serialVersionUID = 1822414421L; 4677 4678 /** 4679 * Constructor 4680 */ 4681 public PlanDefinitionActionOutputComponent() { 4682 super(); 4683 } 4684 4685 /** 4686 * @return {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4687 */ 4688 public StringType getTitleElement() { 4689 if (this.title == null) 4690 if (Configuration.errorOnAutoCreate()) 4691 throw new Error("Attempt to auto-create PlanDefinitionActionOutputComponent.title"); 4692 else if (Configuration.doAutoCreate()) 4693 this.title = new StringType(); // bb 4694 return this.title; 4695 } 4696 4697 public boolean hasTitleElement() { 4698 return this.title != null && !this.title.isEmpty(); 4699 } 4700 4701 public boolean hasTitle() { 4702 return this.title != null && !this.title.isEmpty(); 4703 } 4704 4705 /** 4706 * @param value {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4707 */ 4708 public PlanDefinitionActionOutputComponent setTitleElement(StringType value) { 4709 this.title = value; 4710 return this; 4711 } 4712 4713 /** 4714 * @return A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 4715 */ 4716 public String getTitle() { 4717 return this.title == null ? null : this.title.getValue(); 4718 } 4719 4720 /** 4721 * @param value A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 4722 */ 4723 public PlanDefinitionActionOutputComponent setTitle(String value) { 4724 if (Utilities.noString(value)) 4725 this.title = null; 4726 else { 4727 if (this.title == null) 4728 this.title = new StringType(); 4729 this.title.setValue(value); 4730 } 4731 return this; 4732 } 4733 4734 /** 4735 * @return {@link #requirement} (Defines the data that results as output from the action.) 4736 */ 4737 public DataRequirement getRequirement() { 4738 if (this.requirement == null) 4739 if (Configuration.errorOnAutoCreate()) 4740 throw new Error("Attempt to auto-create PlanDefinitionActionOutputComponent.requirement"); 4741 else if (Configuration.doAutoCreate()) 4742 this.requirement = new DataRequirement(); // cc 4743 return this.requirement; 4744 } 4745 4746 public boolean hasRequirement() { 4747 return this.requirement != null && !this.requirement.isEmpty(); 4748 } 4749 4750 /** 4751 * @param value {@link #requirement} (Defines the data that results as output from the action.) 4752 */ 4753 public PlanDefinitionActionOutputComponent setRequirement(DataRequirement value) { 4754 this.requirement = value; 4755 return this; 4756 } 4757 4758 /** 4759 * @return {@link #relatedData} (Points to an existing input or output element that is results as output from the action.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 4760 */ 4761 public StringType getRelatedDataElement() { 4762 if (this.relatedData == null) 4763 if (Configuration.errorOnAutoCreate()) 4764 throw new Error("Attempt to auto-create PlanDefinitionActionOutputComponent.relatedData"); 4765 else if (Configuration.doAutoCreate()) 4766 this.relatedData = new StringType(); // bb 4767 return this.relatedData; 4768 } 4769 4770 public boolean hasRelatedDataElement() { 4771 return this.relatedData != null && !this.relatedData.isEmpty(); 4772 } 4773 4774 public boolean hasRelatedData() { 4775 return this.relatedData != null && !this.relatedData.isEmpty(); 4776 } 4777 4778 /** 4779 * @param value {@link #relatedData} (Points to an existing input or output element that is results as output from the action.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 4780 */ 4781 public PlanDefinitionActionOutputComponent setRelatedDataElement(StringType value) { 4782 this.relatedData = value; 4783 return this; 4784 } 4785 4786 /** 4787 * @return Points to an existing input or output element that is results as output from the action. 4788 */ 4789 public String getRelatedData() { 4790 return this.relatedData == null ? null : this.relatedData.getValue(); 4791 } 4792 4793 /** 4794 * @param value Points to an existing input or output element that is results as output from the action. 4795 */ 4796 public PlanDefinitionActionOutputComponent setRelatedData(String value) { 4797 if (Utilities.noString(value)) 4798 this.relatedData = null; 4799 else { 4800 if (this.relatedData == null) 4801 this.relatedData = new StringType(); 4802 this.relatedData.setValue(value); 4803 } 4804 return this; 4805 } 4806 4807 protected void listChildren(List<Property> children) { 4808 super.listChildren(children); 4809 children.add(new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title)); 4810 children.add(new Property("requirement", "DataRequirement", "Defines the data that results as output from the action.", 0, 1, requirement)); 4811 children.add(new Property("relatedData", "string", "Points to an existing input or output element that is results as output from the action.", 0, 1, relatedData)); 4812 } 4813 4814 @Override 4815 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4816 switch (_hash) { 4817 case 110371416: /*title*/ return new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title); 4818 case 363387971: /*requirement*/ return new Property("requirement", "DataRequirement", "Defines the data that results as output from the action.", 0, 1, requirement); 4819 case 1112535669: /*relatedData*/ return new Property("relatedData", "string", "Points to an existing input or output element that is results as output from the action.", 0, 1, relatedData); 4820 default: return super.getNamedProperty(_hash, _name, _checkValid); 4821 } 4822 4823 } 4824 4825 @Override 4826 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4827 switch (hash) { 4828 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4829 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // DataRequirement 4830 case 1112535669: /*relatedData*/ return this.relatedData == null ? new Base[0] : new Base[] {this.relatedData}; // StringType 4831 default: return super.getProperty(hash, name, checkValid); 4832 } 4833 4834 } 4835 4836 @Override 4837 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4838 switch (hash) { 4839 case 110371416: // title 4840 this.title = TypeConvertor.castToString(value); // StringType 4841 return value; 4842 case 363387971: // requirement 4843 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 4844 return value; 4845 case 1112535669: // relatedData 4846 this.relatedData = TypeConvertor.castToString(value); // StringType 4847 return value; 4848 default: return super.setProperty(hash, name, value); 4849 } 4850 4851 } 4852 4853 @Override 4854 public Base setProperty(String name, Base value) throws FHIRException { 4855 if (name.equals("title")) { 4856 this.title = TypeConvertor.castToString(value); // StringType 4857 } else if (name.equals("requirement")) { 4858 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 4859 } else if (name.equals("relatedData")) { 4860 this.relatedData = TypeConvertor.castToString(value); // StringType 4861 } else 4862 return super.setProperty(name, value); 4863 return value; 4864 } 4865 4866 @Override 4867 public void removeChild(String name, Base value) throws FHIRException { 4868 if (name.equals("title")) { 4869 this.title = null; 4870 } else if (name.equals("requirement")) { 4871 this.requirement = null; 4872 } else if (name.equals("relatedData")) { 4873 this.relatedData = null; 4874 } else 4875 super.removeChild(name, value); 4876 4877 } 4878 4879 @Override 4880 public Base makeProperty(int hash, String name) throws FHIRException { 4881 switch (hash) { 4882 case 110371416: return getTitleElement(); 4883 case 363387971: return getRequirement(); 4884 case 1112535669: return getRelatedDataElement(); 4885 default: return super.makeProperty(hash, name); 4886 } 4887 4888 } 4889 4890 @Override 4891 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4892 switch (hash) { 4893 case 110371416: /*title*/ return new String[] {"string"}; 4894 case 363387971: /*requirement*/ return new String[] {"DataRequirement"}; 4895 case 1112535669: /*relatedData*/ return new String[] {"string"}; 4896 default: return super.getTypesForProperty(hash, name); 4897 } 4898 4899 } 4900 4901 @Override 4902 public Base addChild(String name) throws FHIRException { 4903 if (name.equals("title")) { 4904 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.output.title"); 4905 } 4906 else if (name.equals("requirement")) { 4907 this.requirement = new DataRequirement(); 4908 return this.requirement; 4909 } 4910 else if (name.equals("relatedData")) { 4911 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.output.relatedData"); 4912 } 4913 else 4914 return super.addChild(name); 4915 } 4916 4917 public PlanDefinitionActionOutputComponent copy() { 4918 PlanDefinitionActionOutputComponent dst = new PlanDefinitionActionOutputComponent(); 4919 copyValues(dst); 4920 return dst; 4921 } 4922 4923 public void copyValues(PlanDefinitionActionOutputComponent dst) { 4924 super.copyValues(dst); 4925 dst.title = title == null ? null : title.copy(); 4926 dst.requirement = requirement == null ? null : requirement.copy(); 4927 dst.relatedData = relatedData == null ? null : relatedData.copy(); 4928 } 4929 4930 @Override 4931 public boolean equalsDeep(Base other_) { 4932 if (!super.equalsDeep(other_)) 4933 return false; 4934 if (!(other_ instanceof PlanDefinitionActionOutputComponent)) 4935 return false; 4936 PlanDefinitionActionOutputComponent o = (PlanDefinitionActionOutputComponent) other_; 4937 return compareDeep(title, o.title, true) && compareDeep(requirement, o.requirement, true) && compareDeep(relatedData, o.relatedData, true) 4938 ; 4939 } 4940 4941 @Override 4942 public boolean equalsShallow(Base other_) { 4943 if (!super.equalsShallow(other_)) 4944 return false; 4945 if (!(other_ instanceof PlanDefinitionActionOutputComponent)) 4946 return false; 4947 PlanDefinitionActionOutputComponent o = (PlanDefinitionActionOutputComponent) other_; 4948 return compareValues(title, o.title, true) && compareValues(relatedData, o.relatedData, true); 4949 } 4950 4951 public boolean isEmpty() { 4952 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, requirement, relatedData 4953 ); 4954 } 4955 4956 public String fhirType() { 4957 return "PlanDefinition.action.output"; 4958 4959 } 4960 4961 } 4962 4963 @Block() 4964 public static class PlanDefinitionActionRelatedActionComponent extends BackboneElement implements IBaseBackboneElement { 4965 /** 4966 * The element id of the target related action. 4967 */ 4968 @Child(name = "targetId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4969 @Description(shortDefinition="What action is this related to", formalDefinition="The element id of the target related action." ) 4970 protected IdType targetId; 4971 4972 /** 4973 * The relationship of the start of this action to the related action. 4974 */ 4975 @Child(name = "relationship", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 4976 @Description(shortDefinition="before | before-start | before-end | concurrent | concurrent-with-start | concurrent-with-end | after | after-start | after-end", formalDefinition="The relationship of the start of this action to the related action." ) 4977 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-relationship-type") 4978 protected Enumeration<ActionRelationshipType> relationship; 4979 4980 /** 4981 * The relationship of the end of this action to the related action. 4982 */ 4983 @Child(name = "endRelationship", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4984 @Description(shortDefinition="before | before-start | before-end | concurrent | concurrent-with-start | concurrent-with-end | after | after-start | after-end", formalDefinition="The relationship of the end of this action to the related action." ) 4985 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-relationship-type") 4986 protected Enumeration<ActionRelationshipType> endRelationship; 4987 4988 /** 4989 * A duration or range of durations to apply to the relationship. For example, 30-60 minutes before. 4990 */ 4991 @Child(name = "offset", type = {Duration.class, Range.class}, order=4, min=0, max=1, modifier=false, summary=false) 4992 @Description(shortDefinition="Time offset for the relationship", formalDefinition="A duration or range of durations to apply to the relationship. For example, 30-60 minutes before." ) 4993 protected DataType offset; 4994 4995 private static final long serialVersionUID = 1997058061L; 4996 4997 /** 4998 * Constructor 4999 */ 5000 public PlanDefinitionActionRelatedActionComponent() { 5001 super(); 5002 } 5003 5004 /** 5005 * Constructor 5006 */ 5007 public PlanDefinitionActionRelatedActionComponent(String targetId, ActionRelationshipType relationship) { 5008 super(); 5009 this.setTargetId(targetId); 5010 this.setRelationship(relationship); 5011 } 5012 5013 /** 5014 * @return {@link #targetId} (The element id of the target related action.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 5015 */ 5016 public IdType getTargetIdElement() { 5017 if (this.targetId == null) 5018 if (Configuration.errorOnAutoCreate()) 5019 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.targetId"); 5020 else if (Configuration.doAutoCreate()) 5021 this.targetId = new IdType(); // bb 5022 return this.targetId; 5023 } 5024 5025 public boolean hasTargetIdElement() { 5026 return this.targetId != null && !this.targetId.isEmpty(); 5027 } 5028 5029 public boolean hasTargetId() { 5030 return this.targetId != null && !this.targetId.isEmpty(); 5031 } 5032 5033 /** 5034 * @param value {@link #targetId} (The element id of the target related action.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 5035 */ 5036 public PlanDefinitionActionRelatedActionComponent setTargetIdElement(IdType value) { 5037 this.targetId = value; 5038 return this; 5039 } 5040 5041 /** 5042 * @return The element id of the target related action. 5043 */ 5044 public String getTargetId() { 5045 return this.targetId == null ? null : this.targetId.getValue(); 5046 } 5047 5048 /** 5049 * @param value The element id of the target related action. 5050 */ 5051 public PlanDefinitionActionRelatedActionComponent setTargetId(String value) { 5052 if (this.targetId == null) 5053 this.targetId = new IdType(); 5054 this.targetId.setValue(value); 5055 return this; 5056 } 5057 5058 /** 5059 * @return {@link #relationship} (The relationship of the start of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 5060 */ 5061 public Enumeration<ActionRelationshipType> getRelationshipElement() { 5062 if (this.relationship == null) 5063 if (Configuration.errorOnAutoCreate()) 5064 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.relationship"); 5065 else if (Configuration.doAutoCreate()) 5066 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 5067 return this.relationship; 5068 } 5069 5070 public boolean hasRelationshipElement() { 5071 return this.relationship != null && !this.relationship.isEmpty(); 5072 } 5073 5074 public boolean hasRelationship() { 5075 return this.relationship != null && !this.relationship.isEmpty(); 5076 } 5077 5078 /** 5079 * @param value {@link #relationship} (The relationship of the start of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 5080 */ 5081 public PlanDefinitionActionRelatedActionComponent setRelationshipElement(Enumeration<ActionRelationshipType> value) { 5082 this.relationship = value; 5083 return this; 5084 } 5085 5086 /** 5087 * @return The relationship of the start of this action to the related action. 5088 */ 5089 public ActionRelationshipType getRelationship() { 5090 return this.relationship == null ? null : this.relationship.getValue(); 5091 } 5092 5093 /** 5094 * @param value The relationship of the start of this action to the related action. 5095 */ 5096 public PlanDefinitionActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 5097 if (this.relationship == null) 5098 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 5099 this.relationship.setValue(value); 5100 return this; 5101 } 5102 5103 /** 5104 * @return {@link #endRelationship} (The relationship of the end of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getEndRelationship" gives direct access to the value 5105 */ 5106 public Enumeration<ActionRelationshipType> getEndRelationshipElement() { 5107 if (this.endRelationship == null) 5108 if (Configuration.errorOnAutoCreate()) 5109 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.endRelationship"); 5110 else if (Configuration.doAutoCreate()) 5111 this.endRelationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 5112 return this.endRelationship; 5113 } 5114 5115 public boolean hasEndRelationshipElement() { 5116 return this.endRelationship != null && !this.endRelationship.isEmpty(); 5117 } 5118 5119 public boolean hasEndRelationship() { 5120 return this.endRelationship != null && !this.endRelationship.isEmpty(); 5121 } 5122 5123 /** 5124 * @param value {@link #endRelationship} (The relationship of the end of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getEndRelationship" gives direct access to the value 5125 */ 5126 public PlanDefinitionActionRelatedActionComponent setEndRelationshipElement(Enumeration<ActionRelationshipType> value) { 5127 this.endRelationship = value; 5128 return this; 5129 } 5130 5131 /** 5132 * @return The relationship of the end of this action to the related action. 5133 */ 5134 public ActionRelationshipType getEndRelationship() { 5135 return this.endRelationship == null ? null : this.endRelationship.getValue(); 5136 } 5137 5138 /** 5139 * @param value The relationship of the end of this action to the related action. 5140 */ 5141 public PlanDefinitionActionRelatedActionComponent setEndRelationship(ActionRelationshipType value) { 5142 if (value == null) 5143 this.endRelationship = null; 5144 else { 5145 if (this.endRelationship == null) 5146 this.endRelationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 5147 this.endRelationship.setValue(value); 5148 } 5149 return this; 5150 } 5151 5152 /** 5153 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 5154 */ 5155 public DataType getOffset() { 5156 return this.offset; 5157 } 5158 5159 /** 5160 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 5161 */ 5162 public Duration getOffsetDuration() throws FHIRException { 5163 if (this.offset == null) 5164 this.offset = new Duration(); 5165 if (!(this.offset instanceof Duration)) 5166 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.offset.getClass().getName()+" was encountered"); 5167 return (Duration) this.offset; 5168 } 5169 5170 public boolean hasOffsetDuration() { 5171 return this.offset instanceof Duration; 5172 } 5173 5174 /** 5175 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 5176 */ 5177 public Range getOffsetRange() throws FHIRException { 5178 if (this.offset == null) 5179 this.offset = new Range(); 5180 if (!(this.offset instanceof Range)) 5181 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.offset.getClass().getName()+" was encountered"); 5182 return (Range) this.offset; 5183 } 5184 5185 public boolean hasOffsetRange() { 5186 return this.offset instanceof Range; 5187 } 5188 5189 public boolean hasOffset() { 5190 return this.offset != null && !this.offset.isEmpty(); 5191 } 5192 5193 /** 5194 * @param value {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 5195 */ 5196 public PlanDefinitionActionRelatedActionComponent setOffset(DataType value) { 5197 if (value != null && !(value instanceof Duration || value instanceof Range)) 5198 throw new FHIRException("Not the right type for PlanDefinition.action.relatedAction.offset[x]: "+value.fhirType()); 5199 this.offset = value; 5200 return this; 5201 } 5202 5203 protected void listChildren(List<Property> children) { 5204 super.listChildren(children); 5205 children.add(new Property("targetId", "id", "The element id of the target related action.", 0, 1, targetId)); 5206 children.add(new Property("relationship", "code", "The relationship of the start of this action to the related action.", 0, 1, relationship)); 5207 children.add(new Property("endRelationship", "code", "The relationship of the end of this action to the related action.", 0, 1, endRelationship)); 5208 children.add(new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset)); 5209 } 5210 5211 @Override 5212 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5213 switch (_hash) { 5214 case -441951604: /*targetId*/ return new Property("targetId", "id", "The element id of the target related action.", 0, 1, targetId); 5215 case -261851592: /*relationship*/ return new Property("relationship", "code", "The relationship of the start of this action to the related action.", 0, 1, relationship); 5216 case -1506024781: /*endRelationship*/ return new Property("endRelationship", "code", "The relationship of the end of this action to the related action.", 0, 1, endRelationship); 5217 case -1960684787: /*offset[x]*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 5218 case -1019779949: /*offset*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 5219 case 134075207: /*offsetDuration*/ return new Property("offset[x]", "Duration", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 5220 case 1263585386: /*offsetRange*/ return new Property("offset[x]", "Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 5221 default: return super.getNamedProperty(_hash, _name, _checkValid); 5222 } 5223 5224 } 5225 5226 @Override 5227 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5228 switch (hash) { 5229 case -441951604: /*targetId*/ return this.targetId == null ? new Base[0] : new Base[] {this.targetId}; // IdType 5230 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Enumeration<ActionRelationshipType> 5231 case -1506024781: /*endRelationship*/ return this.endRelationship == null ? new Base[0] : new Base[] {this.endRelationship}; // Enumeration<ActionRelationshipType> 5232 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // DataType 5233 default: return super.getProperty(hash, name, checkValid); 5234 } 5235 5236 } 5237 5238 @Override 5239 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5240 switch (hash) { 5241 case -441951604: // targetId 5242 this.targetId = TypeConvertor.castToId(value); // IdType 5243 return value; 5244 case -261851592: // relationship 5245 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5246 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5247 return value; 5248 case -1506024781: // endRelationship 5249 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5250 this.endRelationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5251 return value; 5252 case -1019779949: // offset 5253 this.offset = TypeConvertor.castToType(value); // DataType 5254 return value; 5255 default: return super.setProperty(hash, name, value); 5256 } 5257 5258 } 5259 5260 @Override 5261 public Base setProperty(String name, Base value) throws FHIRException { 5262 if (name.equals("targetId")) { 5263 this.targetId = TypeConvertor.castToId(value); // IdType 5264 } else if (name.equals("relationship")) { 5265 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5266 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5267 } else if (name.equals("endRelationship")) { 5268 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5269 this.endRelationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5270 } else if (name.equals("offset[x]")) { 5271 this.offset = TypeConvertor.castToType(value); // DataType 5272 } else 5273 return super.setProperty(name, value); 5274 return value; 5275 } 5276 5277 @Override 5278 public void removeChild(String name, Base value) throws FHIRException { 5279 if (name.equals("targetId")) { 5280 this.targetId = null; 5281 } else if (name.equals("relationship")) { 5282 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5283 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5284 } else if (name.equals("endRelationship")) { 5285 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5286 this.endRelationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5287 } else if (name.equals("offset[x]")) { 5288 this.offset = null; 5289 } else 5290 super.removeChild(name, value); 5291 5292 } 5293 5294 @Override 5295 public Base makeProperty(int hash, String name) throws FHIRException { 5296 switch (hash) { 5297 case -441951604: return getTargetIdElement(); 5298 case -261851592: return getRelationshipElement(); 5299 case -1506024781: return getEndRelationshipElement(); 5300 case -1960684787: return getOffset(); 5301 case -1019779949: return getOffset(); 5302 default: return super.makeProperty(hash, name); 5303 } 5304 5305 } 5306 5307 @Override 5308 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5309 switch (hash) { 5310 case -441951604: /*targetId*/ return new String[] {"id"}; 5311 case -261851592: /*relationship*/ return new String[] {"code"}; 5312 case -1506024781: /*endRelationship*/ return new String[] {"code"}; 5313 case -1019779949: /*offset*/ return new String[] {"Duration", "Range"}; 5314 default: return super.getTypesForProperty(hash, name); 5315 } 5316 5317 } 5318 5319 @Override 5320 public Base addChild(String name) throws FHIRException { 5321 if (name.equals("targetId")) { 5322 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.relatedAction.targetId"); 5323 } 5324 else if (name.equals("relationship")) { 5325 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.relatedAction.relationship"); 5326 } 5327 else if (name.equals("endRelationship")) { 5328 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.relatedAction.endRelationship"); 5329 } 5330 else if (name.equals("offsetDuration")) { 5331 this.offset = new Duration(); 5332 return this.offset; 5333 } 5334 else if (name.equals("offsetRange")) { 5335 this.offset = new Range(); 5336 return this.offset; 5337 } 5338 else 5339 return super.addChild(name); 5340 } 5341 5342 public PlanDefinitionActionRelatedActionComponent copy() { 5343 PlanDefinitionActionRelatedActionComponent dst = new PlanDefinitionActionRelatedActionComponent(); 5344 copyValues(dst); 5345 return dst; 5346 } 5347 5348 public void copyValues(PlanDefinitionActionRelatedActionComponent dst) { 5349 super.copyValues(dst); 5350 dst.targetId = targetId == null ? null : targetId.copy(); 5351 dst.relationship = relationship == null ? null : relationship.copy(); 5352 dst.endRelationship = endRelationship == null ? null : endRelationship.copy(); 5353 dst.offset = offset == null ? null : offset.copy(); 5354 } 5355 5356 @Override 5357 public boolean equalsDeep(Base other_) { 5358 if (!super.equalsDeep(other_)) 5359 return false; 5360 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 5361 return false; 5362 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 5363 return compareDeep(targetId, o.targetId, true) && compareDeep(relationship, o.relationship, true) 5364 && compareDeep(endRelationship, o.endRelationship, true) && compareDeep(offset, o.offset, true) 5365 ; 5366 } 5367 5368 @Override 5369 public boolean equalsShallow(Base other_) { 5370 if (!super.equalsShallow(other_)) 5371 return false; 5372 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 5373 return false; 5374 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 5375 return compareValues(targetId, o.targetId, true) && compareValues(relationship, o.relationship, true) 5376 && compareValues(endRelationship, o.endRelationship, true); 5377 } 5378 5379 public boolean isEmpty() { 5380 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(targetId, relationship, endRelationship 5381 , offset); 5382 } 5383 5384 public String fhirType() { 5385 return "PlanDefinition.action.relatedAction"; 5386 5387 } 5388 5389 } 5390 5391 @Block() 5392 public static class PlanDefinitionActionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 5393 /** 5394 * A reference to the id element of the actor who will participate in this action. 5395 */ 5396 @Child(name = "actorId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 5397 @Description(shortDefinition="What actor", formalDefinition="A reference to the id element of the actor who will participate in this action." ) 5398 protected StringType actorId; 5399 5400 /** 5401 * The type of participant in the action. 5402 */ 5403 @Child(name = "type", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5404 @Description(shortDefinition="careteam | device | group | healthcareservice | location | organization | patient | practitioner | practitionerrole | relatedperson", formalDefinition="The type of participant in the action." ) 5405 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-type") 5406 protected Enumeration<ActionParticipantType> type; 5407 5408 /** 5409 * The type of participant in the action. 5410 */ 5411 @Child(name = "typeCanonical", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5412 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 5413 protected CanonicalType typeCanonical; 5414 5415 /** 5416 * The type of participant in the action. 5417 */ 5418 @Child(name = "typeReference", type = {CareTeam.class, Device.class, DeviceDefinition.class, Endpoint.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=4, min=0, max=1, modifier=false, summary=false) 5419 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 5420 protected Reference typeReference; 5421 5422 /** 5423 * The role the participant should play in performing the described action. 5424 */ 5425 @Child(name = "role", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 5426 @Description(shortDefinition="E.g. Nurse, Surgeon, Parent", formalDefinition="The role the participant should play in performing the described action." ) 5427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/action-participant-role") 5428 protected CodeableConcept role; 5429 5430 /** 5431 * Indicates how the actor will be involved in the action - author, reviewer, witness, etc. 5432 */ 5433 @Child(name = "function", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 5434 @Description(shortDefinition="E.g. Author, Reviewer, Witness, etc", formalDefinition="Indicates how the actor will be involved in the action - author, reviewer, witness, etc." ) 5435 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-function") 5436 protected CodeableConcept function; 5437 5438 private static final long serialVersionUID = -1467052283L; 5439 5440 /** 5441 * Constructor 5442 */ 5443 public PlanDefinitionActionParticipantComponent() { 5444 super(); 5445 } 5446 5447 /** 5448 * @return {@link #actorId} (A reference to the id element of the actor who will participate in this action.). This is the underlying object with id, value and extensions. The accessor "getActorId" gives direct access to the value 5449 */ 5450 public StringType getActorIdElement() { 5451 if (this.actorId == null) 5452 if (Configuration.errorOnAutoCreate()) 5453 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.actorId"); 5454 else if (Configuration.doAutoCreate()) 5455 this.actorId = new StringType(); // bb 5456 return this.actorId; 5457 } 5458 5459 public boolean hasActorIdElement() { 5460 return this.actorId != null && !this.actorId.isEmpty(); 5461 } 5462 5463 public boolean hasActorId() { 5464 return this.actorId != null && !this.actorId.isEmpty(); 5465 } 5466 5467 /** 5468 * @param value {@link #actorId} (A reference to the id element of the actor who will participate in this action.). This is the underlying object with id, value and extensions. The accessor "getActorId" gives direct access to the value 5469 */ 5470 public PlanDefinitionActionParticipantComponent setActorIdElement(StringType value) { 5471 this.actorId = value; 5472 return this; 5473 } 5474 5475 /** 5476 * @return A reference to the id element of the actor who will participate in this action. 5477 */ 5478 public String getActorId() { 5479 return this.actorId == null ? null : this.actorId.getValue(); 5480 } 5481 5482 /** 5483 * @param value A reference to the id element of the actor who will participate in this action. 5484 */ 5485 public PlanDefinitionActionParticipantComponent setActorId(String value) { 5486 if (Utilities.noString(value)) 5487 this.actorId = null; 5488 else { 5489 if (this.actorId == null) 5490 this.actorId = new StringType(); 5491 this.actorId.setValue(value); 5492 } 5493 return this; 5494 } 5495 5496 /** 5497 * @return {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 5498 */ 5499 public Enumeration<ActionParticipantType> getTypeElement() { 5500 if (this.type == null) 5501 if (Configuration.errorOnAutoCreate()) 5502 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.type"); 5503 else if (Configuration.doAutoCreate()) 5504 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); // bb 5505 return this.type; 5506 } 5507 5508 public boolean hasTypeElement() { 5509 return this.type != null && !this.type.isEmpty(); 5510 } 5511 5512 public boolean hasType() { 5513 return this.type != null && !this.type.isEmpty(); 5514 } 5515 5516 /** 5517 * @param value {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 5518 */ 5519 public PlanDefinitionActionParticipantComponent setTypeElement(Enumeration<ActionParticipantType> value) { 5520 this.type = value; 5521 return this; 5522 } 5523 5524 /** 5525 * @return The type of participant in the action. 5526 */ 5527 public ActionParticipantType getType() { 5528 return this.type == null ? null : this.type.getValue(); 5529 } 5530 5531 /** 5532 * @param value The type of participant in the action. 5533 */ 5534 public PlanDefinitionActionParticipantComponent setType(ActionParticipantType value) { 5535 if (value == null) 5536 this.type = null; 5537 else { 5538 if (this.type == null) 5539 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); 5540 this.type.setValue(value); 5541 } 5542 return this; 5543 } 5544 5545 /** 5546 * @return {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 5547 */ 5548 public CanonicalType getTypeCanonicalElement() { 5549 if (this.typeCanonical == null) 5550 if (Configuration.errorOnAutoCreate()) 5551 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.typeCanonical"); 5552 else if (Configuration.doAutoCreate()) 5553 this.typeCanonical = new CanonicalType(); // bb 5554 return this.typeCanonical; 5555 } 5556 5557 public boolean hasTypeCanonicalElement() { 5558 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 5559 } 5560 5561 public boolean hasTypeCanonical() { 5562 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 5563 } 5564 5565 /** 5566 * @param value {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 5567 */ 5568 public PlanDefinitionActionParticipantComponent setTypeCanonicalElement(CanonicalType value) { 5569 this.typeCanonical = value; 5570 return this; 5571 } 5572 5573 /** 5574 * @return The type of participant in the action. 5575 */ 5576 public String getTypeCanonical() { 5577 return this.typeCanonical == null ? null : this.typeCanonical.getValue(); 5578 } 5579 5580 /** 5581 * @param value The type of participant in the action. 5582 */ 5583 public PlanDefinitionActionParticipantComponent setTypeCanonical(String value) { 5584 if (Utilities.noString(value)) 5585 this.typeCanonical = null; 5586 else { 5587 if (this.typeCanonical == null) 5588 this.typeCanonical = new CanonicalType(); 5589 this.typeCanonical.setValue(value); 5590 } 5591 return this; 5592 } 5593 5594 /** 5595 * @return {@link #typeReference} (The type of participant in the action.) 5596 */ 5597 public Reference getTypeReference() { 5598 if (this.typeReference == null) 5599 if (Configuration.errorOnAutoCreate()) 5600 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.typeReference"); 5601 else if (Configuration.doAutoCreate()) 5602 this.typeReference = new Reference(); // cc 5603 return this.typeReference; 5604 } 5605 5606 public boolean hasTypeReference() { 5607 return this.typeReference != null && !this.typeReference.isEmpty(); 5608 } 5609 5610 /** 5611 * @param value {@link #typeReference} (The type of participant in the action.) 5612 */ 5613 public PlanDefinitionActionParticipantComponent setTypeReference(Reference value) { 5614 this.typeReference = value; 5615 return this; 5616 } 5617 5618 /** 5619 * @return {@link #role} (The role the participant should play in performing the described action.) 5620 */ 5621 public CodeableConcept getRole() { 5622 if (this.role == null) 5623 if (Configuration.errorOnAutoCreate()) 5624 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.role"); 5625 else if (Configuration.doAutoCreate()) 5626 this.role = new CodeableConcept(); // cc 5627 return this.role; 5628 } 5629 5630 public boolean hasRole() { 5631 return this.role != null && !this.role.isEmpty(); 5632 } 5633 5634 /** 5635 * @param value {@link #role} (The role the participant should play in performing the described action.) 5636 */ 5637 public PlanDefinitionActionParticipantComponent setRole(CodeableConcept value) { 5638 this.role = value; 5639 return this; 5640 } 5641 5642 /** 5643 * @return {@link #function} (Indicates how the actor will be involved in the action - author, reviewer, witness, etc.) 5644 */ 5645 public CodeableConcept getFunction() { 5646 if (this.function == null) 5647 if (Configuration.errorOnAutoCreate()) 5648 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.function"); 5649 else if (Configuration.doAutoCreate()) 5650 this.function = new CodeableConcept(); // cc 5651 return this.function; 5652 } 5653 5654 public boolean hasFunction() { 5655 return this.function != null && !this.function.isEmpty(); 5656 } 5657 5658 /** 5659 * @param value {@link #function} (Indicates how the actor will be involved in the action - author, reviewer, witness, etc.) 5660 */ 5661 public PlanDefinitionActionParticipantComponent setFunction(CodeableConcept value) { 5662 this.function = value; 5663 return this; 5664 } 5665 5666 protected void listChildren(List<Property> children) { 5667 super.listChildren(children); 5668 children.add(new Property("actorId", "string", "A reference to the id element of the actor who will participate in this action.", 0, 1, actorId)); 5669 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 5670 children.add(new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical)); 5671 children.add(new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference)); 5672 children.add(new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role)); 5673 children.add(new Property("function", "CodeableConcept", "Indicates how the actor will be involved in the action - author, reviewer, witness, etc.", 0, 1, function)); 5674 } 5675 5676 @Override 5677 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5678 switch (_hash) { 5679 case -1161623056: /*actorId*/ return new Property("actorId", "string", "A reference to the id element of the actor who will participate in this action.", 0, 1, actorId); 5680 case 3575610: /*type*/ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 5681 case -466635046: /*typeCanonical*/ return new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical); 5682 case 2074825009: /*typeReference*/ return new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference); 5683 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role); 5684 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Indicates how the actor will be involved in the action - author, reviewer, witness, etc.", 0, 1, function); 5685 default: return super.getNamedProperty(_hash, _name, _checkValid); 5686 } 5687 5688 } 5689 5690 @Override 5691 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5692 switch (hash) { 5693 case -1161623056: /*actorId*/ return this.actorId == null ? new Base[0] : new Base[] {this.actorId}; // StringType 5694 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ActionParticipantType> 5695 case -466635046: /*typeCanonical*/ return this.typeCanonical == null ? new Base[0] : new Base[] {this.typeCanonical}; // CanonicalType 5696 case 2074825009: /*typeReference*/ return this.typeReference == null ? new Base[0] : new Base[] {this.typeReference}; // Reference 5697 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 5698 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 5699 default: return super.getProperty(hash, name, checkValid); 5700 } 5701 5702 } 5703 5704 @Override 5705 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5706 switch (hash) { 5707 case -1161623056: // actorId 5708 this.actorId = TypeConvertor.castToString(value); // StringType 5709 return value; 5710 case 3575610: // type 5711 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5712 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 5713 return value; 5714 case -466635046: // typeCanonical 5715 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 5716 return value; 5717 case 2074825009: // typeReference 5718 this.typeReference = TypeConvertor.castToReference(value); // Reference 5719 return value; 5720 case 3506294: // role 5721 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5722 return value; 5723 case 1380938712: // function 5724 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5725 return value; 5726 default: return super.setProperty(hash, name, value); 5727 } 5728 5729 } 5730 5731 @Override 5732 public Base setProperty(String name, Base value) throws FHIRException { 5733 if (name.equals("actorId")) { 5734 this.actorId = TypeConvertor.castToString(value); // StringType 5735 } else if (name.equals("type")) { 5736 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5737 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 5738 } else if (name.equals("typeCanonical")) { 5739 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 5740 } else if (name.equals("typeReference")) { 5741 this.typeReference = TypeConvertor.castToReference(value); // Reference 5742 } else if (name.equals("role")) { 5743 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5744 } else if (name.equals("function")) { 5745 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5746 } else 5747 return super.setProperty(name, value); 5748 return value; 5749 } 5750 5751 @Override 5752 public void removeChild(String name, Base value) throws FHIRException { 5753 if (name.equals("actorId")) { 5754 this.actorId = null; 5755 } else if (name.equals("type")) { 5756 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5757 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 5758 } else if (name.equals("typeCanonical")) { 5759 this.typeCanonical = null; 5760 } else if (name.equals("typeReference")) { 5761 this.typeReference = null; 5762 } else if (name.equals("role")) { 5763 this.role = null; 5764 } else if (name.equals("function")) { 5765 this.function = null; 5766 } else 5767 super.removeChild(name, value); 5768 5769 } 5770 5771 @Override 5772 public Base makeProperty(int hash, String name) throws FHIRException { 5773 switch (hash) { 5774 case -1161623056: return getActorIdElement(); 5775 case 3575610: return getTypeElement(); 5776 case -466635046: return getTypeCanonicalElement(); 5777 case 2074825009: return getTypeReference(); 5778 case 3506294: return getRole(); 5779 case 1380938712: return getFunction(); 5780 default: return super.makeProperty(hash, name); 5781 } 5782 5783 } 5784 5785 @Override 5786 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5787 switch (hash) { 5788 case -1161623056: /*actorId*/ return new String[] {"string"}; 5789 case 3575610: /*type*/ return new String[] {"code"}; 5790 case -466635046: /*typeCanonical*/ return new String[] {"canonical"}; 5791 case 2074825009: /*typeReference*/ return new String[] {"Reference"}; 5792 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 5793 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 5794 default: return super.getTypesForProperty(hash, name); 5795 } 5796 5797 } 5798 5799 @Override 5800 public Base addChild(String name) throws FHIRException { 5801 if (name.equals("actorId")) { 5802 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.participant.actorId"); 5803 } 5804 else if (name.equals("type")) { 5805 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.participant.type"); 5806 } 5807 else if (name.equals("typeCanonical")) { 5808 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.participant.typeCanonical"); 5809 } 5810 else if (name.equals("typeReference")) { 5811 this.typeReference = new Reference(); 5812 return this.typeReference; 5813 } 5814 else if (name.equals("role")) { 5815 this.role = new CodeableConcept(); 5816 return this.role; 5817 } 5818 else if (name.equals("function")) { 5819 this.function = new CodeableConcept(); 5820 return this.function; 5821 } 5822 else 5823 return super.addChild(name); 5824 } 5825 5826 public PlanDefinitionActionParticipantComponent copy() { 5827 PlanDefinitionActionParticipantComponent dst = new PlanDefinitionActionParticipantComponent(); 5828 copyValues(dst); 5829 return dst; 5830 } 5831 5832 public void copyValues(PlanDefinitionActionParticipantComponent dst) { 5833 super.copyValues(dst); 5834 dst.actorId = actorId == null ? null : actorId.copy(); 5835 dst.type = type == null ? null : type.copy(); 5836 dst.typeCanonical = typeCanonical == null ? null : typeCanonical.copy(); 5837 dst.typeReference = typeReference == null ? null : typeReference.copy(); 5838 dst.role = role == null ? null : role.copy(); 5839 dst.function = function == null ? null : function.copy(); 5840 } 5841 5842 @Override 5843 public boolean equalsDeep(Base other_) { 5844 if (!super.equalsDeep(other_)) 5845 return false; 5846 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 5847 return false; 5848 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 5849 return compareDeep(actorId, o.actorId, true) && compareDeep(type, o.type, true) && compareDeep(typeCanonical, o.typeCanonical, true) 5850 && compareDeep(typeReference, o.typeReference, true) && compareDeep(role, o.role, true) && compareDeep(function, o.function, true) 5851 ; 5852 } 5853 5854 @Override 5855 public boolean equalsShallow(Base other_) { 5856 if (!super.equalsShallow(other_)) 5857 return false; 5858 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 5859 return false; 5860 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 5861 return compareValues(actorId, o.actorId, true) && compareValues(type, o.type, true) && compareValues(typeCanonical, o.typeCanonical, true) 5862 ; 5863 } 5864 5865 public boolean isEmpty() { 5866 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actorId, type, typeCanonical 5867 , typeReference, role, function); 5868 } 5869 5870 public String fhirType() { 5871 return "PlanDefinition.action.participant"; 5872 5873 } 5874 5875 } 5876 5877 @Block() 5878 public static class PlanDefinitionActionDynamicValueComponent extends BackboneElement implements IBaseBackboneElement { 5879 /** 5880 * The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 5881 */ 5882 @Child(name = "path", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 5883 @Description(shortDefinition="The path to the element to be set dynamically", formalDefinition="The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details)." ) 5884 protected StringType path; 5885 5886 /** 5887 * An expression specifying the value of the customized element. 5888 */ 5889 @Child(name = "expression", type = {Expression.class}, order=2, min=0, max=1, modifier=false, summary=false) 5890 @Description(shortDefinition="An expression that provides the dynamic value for the customization", formalDefinition="An expression specifying the value of the customized element." ) 5891 protected Expression expression; 5892 5893 private static final long serialVersionUID = 1064529082L; 5894 5895 /** 5896 * Constructor 5897 */ 5898 public PlanDefinitionActionDynamicValueComponent() { 5899 super(); 5900 } 5901 5902 /** 5903 * @return {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 5904 */ 5905 public StringType getPathElement() { 5906 if (this.path == null) 5907 if (Configuration.errorOnAutoCreate()) 5908 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.path"); 5909 else if (Configuration.doAutoCreate()) 5910 this.path = new StringType(); // bb 5911 return this.path; 5912 } 5913 5914 public boolean hasPathElement() { 5915 return this.path != null && !this.path.isEmpty(); 5916 } 5917 5918 public boolean hasPath() { 5919 return this.path != null && !this.path.isEmpty(); 5920 } 5921 5922 /** 5923 * @param value {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 5924 */ 5925 public PlanDefinitionActionDynamicValueComponent setPathElement(StringType value) { 5926 this.path = value; 5927 return this; 5928 } 5929 5930 /** 5931 * @return The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 5932 */ 5933 public String getPath() { 5934 return this.path == null ? null : this.path.getValue(); 5935 } 5936 5937 /** 5938 * @param value The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 5939 */ 5940 public PlanDefinitionActionDynamicValueComponent setPath(String value) { 5941 if (Utilities.noString(value)) 5942 this.path = null; 5943 else { 5944 if (this.path == null) 5945 this.path = new StringType(); 5946 this.path.setValue(value); 5947 } 5948 return this; 5949 } 5950 5951 /** 5952 * @return {@link #expression} (An expression specifying the value of the customized element.) 5953 */ 5954 public Expression getExpression() { 5955 if (this.expression == null) 5956 if (Configuration.errorOnAutoCreate()) 5957 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.expression"); 5958 else if (Configuration.doAutoCreate()) 5959 this.expression = new Expression(); // cc 5960 return this.expression; 5961 } 5962 5963 public boolean hasExpression() { 5964 return this.expression != null && !this.expression.isEmpty(); 5965 } 5966 5967 /** 5968 * @param value {@link #expression} (An expression specifying the value of the customized element.) 5969 */ 5970 public PlanDefinitionActionDynamicValueComponent setExpression(Expression value) { 5971 this.expression = value; 5972 return this; 5973 } 5974 5975 protected void listChildren(List<Property> children) { 5976 super.listChildren(children); 5977 children.add(new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, 1, path)); 5978 children.add(new Property("expression", "Expression", "An expression specifying the value of the customized element.", 0, 1, expression)); 5979 } 5980 5981 @Override 5982 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5983 switch (_hash) { 5984 case 3433509: /*path*/ return new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, 1, path); 5985 case -1795452264: /*expression*/ return new Property("expression", "Expression", "An expression specifying the value of the customized element.", 0, 1, expression); 5986 default: return super.getNamedProperty(_hash, _name, _checkValid); 5987 } 5988 5989 } 5990 5991 @Override 5992 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5993 switch (hash) { 5994 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 5995 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 5996 default: return super.getProperty(hash, name, checkValid); 5997 } 5998 5999 } 6000 6001 @Override 6002 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6003 switch (hash) { 6004 case 3433509: // path 6005 this.path = TypeConvertor.castToString(value); // StringType 6006 return value; 6007 case -1795452264: // expression 6008 this.expression = TypeConvertor.castToExpression(value); // Expression 6009 return value; 6010 default: return super.setProperty(hash, name, value); 6011 } 6012 6013 } 6014 6015 @Override 6016 public Base setProperty(String name, Base value) throws FHIRException { 6017 if (name.equals("path")) { 6018 this.path = TypeConvertor.castToString(value); // StringType 6019 } else if (name.equals("expression")) { 6020 this.expression = TypeConvertor.castToExpression(value); // Expression 6021 } else 6022 return super.setProperty(name, value); 6023 return value; 6024 } 6025 6026 @Override 6027 public void removeChild(String name, Base value) throws FHIRException { 6028 if (name.equals("path")) { 6029 this.path = null; 6030 } else if (name.equals("expression")) { 6031 this.expression = null; 6032 } else 6033 super.removeChild(name, value); 6034 6035 } 6036 6037 @Override 6038 public Base makeProperty(int hash, String name) throws FHIRException { 6039 switch (hash) { 6040 case 3433509: return getPathElement(); 6041 case -1795452264: return getExpression(); 6042 default: return super.makeProperty(hash, name); 6043 } 6044 6045 } 6046 6047 @Override 6048 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6049 switch (hash) { 6050 case 3433509: /*path*/ return new String[] {"string"}; 6051 case -1795452264: /*expression*/ return new String[] {"Expression"}; 6052 default: return super.getTypesForProperty(hash, name); 6053 } 6054 6055 } 6056 6057 @Override 6058 public Base addChild(String name) throws FHIRException { 6059 if (name.equals("path")) { 6060 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.action.dynamicValue.path"); 6061 } 6062 else if (name.equals("expression")) { 6063 this.expression = new Expression(); 6064 return this.expression; 6065 } 6066 else 6067 return super.addChild(name); 6068 } 6069 6070 public PlanDefinitionActionDynamicValueComponent copy() { 6071 PlanDefinitionActionDynamicValueComponent dst = new PlanDefinitionActionDynamicValueComponent(); 6072 copyValues(dst); 6073 return dst; 6074 } 6075 6076 public void copyValues(PlanDefinitionActionDynamicValueComponent dst) { 6077 super.copyValues(dst); 6078 dst.path = path == null ? null : path.copy(); 6079 dst.expression = expression == null ? null : expression.copy(); 6080 } 6081 6082 @Override 6083 public boolean equalsDeep(Base other_) { 6084 if (!super.equalsDeep(other_)) 6085 return false; 6086 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 6087 return false; 6088 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 6089 return compareDeep(path, o.path, true) && compareDeep(expression, o.expression, true); 6090 } 6091 6092 @Override 6093 public boolean equalsShallow(Base other_) { 6094 if (!super.equalsShallow(other_)) 6095 return false; 6096 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 6097 return false; 6098 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 6099 return compareValues(path, o.path, true); 6100 } 6101 6102 public boolean isEmpty() { 6103 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, expression); 6104 } 6105 6106 public String fhirType() { 6107 return "PlanDefinition.action.dynamicValue"; 6108 6109 } 6110 6111 } 6112 6113 /** 6114 * An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers. 6115 */ 6116 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 6117 @Description(shortDefinition="Canonical identifier for this plan definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers." ) 6118 protected UriType url; 6119 6120 /** 6121 * A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 6122 */ 6123 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6124 @Description(shortDefinition="Additional identifier for the plan definition", formalDefinition="A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 6125 protected List<Identifier> identifier; 6126 6127 /** 6128 * The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 6129 */ 6130 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 6131 @Description(shortDefinition="Business version of the plan definition", formalDefinition="The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts." ) 6132 protected StringType version; 6133 6134 /** 6135 * Indicates the mechanism used to compare versions to determine which is more current. 6136 */ 6137 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 6138 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 6139 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 6140 protected DataType versionAlgorithm; 6141 6142 /** 6143 * A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 6144 */ 6145 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 6146 @Description(shortDefinition="Name for this plan definition (computer friendly)", formalDefinition="A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 6147 protected StringType name; 6148 6149 /** 6150 * A short, descriptive, user-friendly title for the plan definition. 6151 */ 6152 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 6153 @Description(shortDefinition="Name for this plan definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the plan definition." ) 6154 protected StringType title; 6155 6156 /** 6157 * An explanatory or alternate title for the plan definition giving additional information about its content. 6158 */ 6159 @Child(name = "subtitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 6160 @Description(shortDefinition="Subordinate title of the plan definition", formalDefinition="An explanatory or alternate title for the plan definition giving additional information about its content." ) 6161 protected StringType subtitle; 6162 6163 /** 6164 * A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition. 6165 */ 6166 @Child(name = "type", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 6167 @Description(shortDefinition="order-set | clinical-protocol | eca-rule | workflow-definition", formalDefinition="A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition." ) 6168 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/plan-definition-type") 6169 protected CodeableConcept type; 6170 6171 /** 6172 * The status of this plan definition. Enables tracking the life-cycle of the content. 6173 */ 6174 @Child(name = "status", type = {CodeType.class}, order=8, min=1, max=1, modifier=true, summary=true) 6175 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this plan definition. Enables tracking the life-cycle of the content." ) 6176 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 6177 protected Enumeration<PublicationStatus> status; 6178 6179 /** 6180 * A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 6181 */ 6182 @Child(name = "experimental", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=true) 6183 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 6184 protected BooleanType experimental; 6185 6186 /** 6187 * A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource. 6188 */ 6189 @Child(name = "subject", type = {CodeableConcept.class, Group.class, MedicinalProductDefinition.class, SubstanceDefinition.class, AdministrableProductDefinition.class, ManufacturedItemDefinition.class, PackagedProductDefinition.class, CanonicalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 6190 @Description(shortDefinition="Type of individual the plan definition is focused on", formalDefinition="A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource." ) 6191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 6192 protected DataType subject; 6193 6194 /** 6195 * The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes. 6196 */ 6197 @Child(name = "date", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 6198 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes." ) 6199 protected DateTimeType date; 6200 6201 /** 6202 * The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition. 6203 */ 6204 @Child(name = "publisher", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 6205 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition." ) 6206 protected StringType publisher; 6207 6208 /** 6209 * Contact details to assist a user in finding and communicating with the publisher. 6210 */ 6211 @Child(name = "contact", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6212 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 6213 protected List<ContactDetail> contact; 6214 6215 /** 6216 * A free text natural language description of the plan definition from a consumer's perspective. 6217 */ 6218 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=true) 6219 @Description(shortDefinition="Natural language description of the plan definition", formalDefinition="A free text natural language description of the plan definition from a consumer's perspective." ) 6220 protected MarkdownType description; 6221 6222 /** 6223 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances. 6224 */ 6225 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6226 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances." ) 6227 protected List<UsageContext> useContext; 6228 6229 /** 6230 * A legal or geographic region in which the plan definition is intended to be used. 6231 */ 6232 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6233 @Description(shortDefinition="Intended jurisdiction for plan definition (if applicable)", formalDefinition="A legal or geographic region in which the plan definition is intended to be used." ) 6234 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 6235 protected List<CodeableConcept> jurisdiction; 6236 6237 /** 6238 * Explanation of why this plan definition is needed and why it has been designed as it has. 6239 */ 6240 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 6241 @Description(shortDefinition="Why this plan definition is defined", formalDefinition="Explanation of why this plan definition is needed and why it has been designed as it has." ) 6242 protected MarkdownType purpose; 6243 6244 /** 6245 * A detailed description of how the plan definition is used from a clinical perspective. 6246 */ 6247 @Child(name = "usage", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 6248 @Description(shortDefinition="Describes the clinical usage of the plan", formalDefinition="A detailed description of how the plan definition is used from a clinical perspective." ) 6249 protected MarkdownType usage; 6250 6251 /** 6252 * A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition. 6253 */ 6254 @Child(name = "copyright", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 6255 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition." ) 6256 protected MarkdownType copyright; 6257 6258 /** 6259 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 6260 */ 6261 @Child(name = "copyrightLabel", type = {StringType.class}, order=20, min=0, max=1, modifier=false, summary=false) 6262 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 6263 protected StringType copyrightLabel; 6264 6265 /** 6266 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 6267 */ 6268 @Child(name = "approvalDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 6269 @Description(shortDefinition="When the plan definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 6270 protected DateType approvalDate; 6271 6272 /** 6273 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 6274 */ 6275 @Child(name = "lastReviewDate", type = {DateType.class}, order=22, min=0, max=1, modifier=false, summary=false) 6276 @Description(shortDefinition="When the plan definition was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 6277 protected DateType lastReviewDate; 6278 6279 /** 6280 * The period during which the plan definition content was or is planned to be in active use. 6281 */ 6282 @Child(name = "effectivePeriod", type = {Period.class}, order=23, min=0, max=1, modifier=false, summary=true) 6283 @Description(shortDefinition="When the plan definition is expected to be used", formalDefinition="The period during which the plan definition content was or is planned to be in active use." ) 6284 protected Period effectivePeriod; 6285 6286 /** 6287 * Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching. 6288 */ 6289 @Child(name = "topic", type = {CodeableConcept.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6290 @Description(shortDefinition="E.g. Education, Treatment, Assessment", formalDefinition="Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching." ) 6291 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 6292 protected List<CodeableConcept> topic; 6293 6294 /** 6295 * An individiual or organization primarily involved in the creation and maintenance of the content. 6296 */ 6297 @Child(name = "author", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6298 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the content." ) 6299 protected List<ContactDetail> author; 6300 6301 /** 6302 * An individual or organization primarily responsible for internal coherence of the content. 6303 */ 6304 @Child(name = "editor", type = {ContactDetail.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6305 @Description(shortDefinition="Who edited the content", formalDefinition="An individual or organization primarily responsible for internal coherence of the content." ) 6306 protected List<ContactDetail> editor; 6307 6308 /** 6309 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content. 6310 */ 6311 @Child(name = "reviewer", type = {ContactDetail.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6312 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content." ) 6313 protected List<ContactDetail> reviewer; 6314 6315 /** 6316 * An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting. 6317 */ 6318 @Child(name = "endorser", type = {ContactDetail.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6319 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting." ) 6320 protected List<ContactDetail> endorser; 6321 6322 /** 6323 * Related artifacts such as additional documentation, justification, or bibliographic references. 6324 */ 6325 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6326 @Description(shortDefinition="Additional documentation, citations", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 6327 protected List<RelatedArtifact> relatedArtifact; 6328 6329 /** 6330 * A reference to a Library resource containing any formal logic used by the plan definition. 6331 */ 6332 @Child(name = "library", type = {CanonicalType.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6333 @Description(shortDefinition="Logic used by the plan definition", formalDefinition="A reference to a Library resource containing any formal logic used by the plan definition." ) 6334 protected List<CanonicalType> library; 6335 6336 /** 6337 * A goal describes an expected outcome that activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, meeting the acceptance criteria for a test as specified by a quality specification, etc. 6338 */ 6339 @Child(name = "goal", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6340 @Description(shortDefinition="What the plan is trying to accomplish", formalDefinition="A goal describes an expected outcome that activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, meeting the acceptance criteria for a test as specified by a quality specification, etc." ) 6341 protected List<PlanDefinitionGoalComponent> goal; 6342 6343 /** 6344 * Actors represent the individuals or groups involved in the execution of the defined set of activities. 6345 */ 6346 @Child(name = "actor", type = {}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6347 @Description(shortDefinition="Actors within the plan", formalDefinition="Actors represent the individuals or groups involved in the execution of the defined set of activities." ) 6348 protected List<PlanDefinitionActorComponent> actor; 6349 6350 /** 6351 * An action or group of actions to be taken as part of the plan. For example, in clinical care, an action would be to prescribe a particular indicated medication, or perform a particular test as appropriate. In pharmaceutical quality, an action would be the test that needs to be performed on a drug product as defined in the quality specification. 6352 */ 6353 @Child(name = "action", type = {}, order=33, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6354 @Description(shortDefinition="Action defined by the plan", formalDefinition="An action or group of actions to be taken as part of the plan. For example, in clinical care, an action would be to prescribe a particular indicated medication, or perform a particular test as appropriate. In pharmaceutical quality, an action would be the test that needs to be performed on a drug product as defined in the quality specification." ) 6355 protected List<PlanDefinitionActionComponent> action; 6356 6357 /** 6358 * If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc. 6359 */ 6360 @Child(name = "asNeeded", type = {BooleanType.class, CodeableConcept.class}, order=34, min=0, max=1, modifier=false, summary=true) 6361 @Description(shortDefinition="Preconditions for service", formalDefinition="If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc." ) 6362 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 6363 protected DataType asNeeded; 6364 6365 private static final long serialVersionUID = 324754414L; 6366 6367 /** 6368 * Constructor 6369 */ 6370 public PlanDefinition() { 6371 super(); 6372 } 6373 6374 /** 6375 * Constructor 6376 */ 6377 public PlanDefinition(PublicationStatus status) { 6378 super(); 6379 this.setStatus(status); 6380 } 6381 6382 /** 6383 * @return {@link #url} (An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 6384 */ 6385 public UriType getUrlElement() { 6386 if (this.url == null) 6387 if (Configuration.errorOnAutoCreate()) 6388 throw new Error("Attempt to auto-create PlanDefinition.url"); 6389 else if (Configuration.doAutoCreate()) 6390 this.url = new UriType(); // bb 6391 return this.url; 6392 } 6393 6394 public boolean hasUrlElement() { 6395 return this.url != null && !this.url.isEmpty(); 6396 } 6397 6398 public boolean hasUrl() { 6399 return this.url != null && !this.url.isEmpty(); 6400 } 6401 6402 /** 6403 * @param value {@link #url} (An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 6404 */ 6405 public PlanDefinition setUrlElement(UriType value) { 6406 this.url = value; 6407 return this; 6408 } 6409 6410 /** 6411 * @return An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers. 6412 */ 6413 public String getUrl() { 6414 return this.url == null ? null : this.url.getValue(); 6415 } 6416 6417 /** 6418 * @param value An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers. 6419 */ 6420 public PlanDefinition setUrl(String value) { 6421 if (Utilities.noString(value)) 6422 this.url = null; 6423 else { 6424 if (this.url == null) 6425 this.url = new UriType(); 6426 this.url.setValue(value); 6427 } 6428 return this; 6429 } 6430 6431 /** 6432 * @return {@link #identifier} (A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 6433 */ 6434 public List<Identifier> getIdentifier() { 6435 if (this.identifier == null) 6436 this.identifier = new ArrayList<Identifier>(); 6437 return this.identifier; 6438 } 6439 6440 /** 6441 * @return Returns a reference to <code>this</code> for easy method chaining 6442 */ 6443 public PlanDefinition setIdentifier(List<Identifier> theIdentifier) { 6444 this.identifier = theIdentifier; 6445 return this; 6446 } 6447 6448 public boolean hasIdentifier() { 6449 if (this.identifier == null) 6450 return false; 6451 for (Identifier item : this.identifier) 6452 if (!item.isEmpty()) 6453 return true; 6454 return false; 6455 } 6456 6457 public Identifier addIdentifier() { //3 6458 Identifier t = new Identifier(); 6459 if (this.identifier == null) 6460 this.identifier = new ArrayList<Identifier>(); 6461 this.identifier.add(t); 6462 return t; 6463 } 6464 6465 public PlanDefinition addIdentifier(Identifier t) { //3 6466 if (t == null) 6467 return this; 6468 if (this.identifier == null) 6469 this.identifier = new ArrayList<Identifier>(); 6470 this.identifier.add(t); 6471 return this; 6472 } 6473 6474 /** 6475 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 6476 */ 6477 public Identifier getIdentifierFirstRep() { 6478 if (getIdentifier().isEmpty()) { 6479 addIdentifier(); 6480 } 6481 return getIdentifier().get(0); 6482 } 6483 6484 /** 6485 * @return {@link #version} (The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 6486 */ 6487 public StringType getVersionElement() { 6488 if (this.version == null) 6489 if (Configuration.errorOnAutoCreate()) 6490 throw new Error("Attempt to auto-create PlanDefinition.version"); 6491 else if (Configuration.doAutoCreate()) 6492 this.version = new StringType(); // bb 6493 return this.version; 6494 } 6495 6496 public boolean hasVersionElement() { 6497 return this.version != null && !this.version.isEmpty(); 6498 } 6499 6500 public boolean hasVersion() { 6501 return this.version != null && !this.version.isEmpty(); 6502 } 6503 6504 /** 6505 * @param value {@link #version} (The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 6506 */ 6507 public PlanDefinition setVersionElement(StringType value) { 6508 this.version = value; 6509 return this; 6510 } 6511 6512 /** 6513 * @return The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 6514 */ 6515 public String getVersion() { 6516 return this.version == null ? null : this.version.getValue(); 6517 } 6518 6519 /** 6520 * @param value The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 6521 */ 6522 public PlanDefinition setVersion(String value) { 6523 if (Utilities.noString(value)) 6524 this.version = null; 6525 else { 6526 if (this.version == null) 6527 this.version = new StringType(); 6528 this.version.setValue(value); 6529 } 6530 return this; 6531 } 6532 6533 /** 6534 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 6535 */ 6536 public DataType getVersionAlgorithm() { 6537 return this.versionAlgorithm; 6538 } 6539 6540 /** 6541 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 6542 */ 6543 public StringType getVersionAlgorithmStringType() throws FHIRException { 6544 if (this.versionAlgorithm == null) 6545 this.versionAlgorithm = new StringType(); 6546 if (!(this.versionAlgorithm instanceof StringType)) 6547 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 6548 return (StringType) this.versionAlgorithm; 6549 } 6550 6551 public boolean hasVersionAlgorithmStringType() { 6552 return this.versionAlgorithm instanceof StringType; 6553 } 6554 6555 /** 6556 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 6557 */ 6558 public Coding getVersionAlgorithmCoding() throws FHIRException { 6559 if (this.versionAlgorithm == null) 6560 this.versionAlgorithm = new Coding(); 6561 if (!(this.versionAlgorithm instanceof Coding)) 6562 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 6563 return (Coding) this.versionAlgorithm; 6564 } 6565 6566 public boolean hasVersionAlgorithmCoding() { 6567 return this.versionAlgorithm instanceof Coding; 6568 } 6569 6570 public boolean hasVersionAlgorithm() { 6571 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 6572 } 6573 6574 /** 6575 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 6576 */ 6577 public PlanDefinition setVersionAlgorithm(DataType value) { 6578 if (value != null && !(value instanceof StringType || value instanceof Coding)) 6579 throw new FHIRException("Not the right type for PlanDefinition.versionAlgorithm[x]: "+value.fhirType()); 6580 this.versionAlgorithm = value; 6581 return this; 6582 } 6583 6584 /** 6585 * @return {@link #name} (A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 6586 */ 6587 public StringType getNameElement() { 6588 if (this.name == null) 6589 if (Configuration.errorOnAutoCreate()) 6590 throw new Error("Attempt to auto-create PlanDefinition.name"); 6591 else if (Configuration.doAutoCreate()) 6592 this.name = new StringType(); // bb 6593 return this.name; 6594 } 6595 6596 public boolean hasNameElement() { 6597 return this.name != null && !this.name.isEmpty(); 6598 } 6599 6600 public boolean hasName() { 6601 return this.name != null && !this.name.isEmpty(); 6602 } 6603 6604 /** 6605 * @param value {@link #name} (A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 6606 */ 6607 public PlanDefinition setNameElement(StringType value) { 6608 this.name = value; 6609 return this; 6610 } 6611 6612 /** 6613 * @return A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 6614 */ 6615 public String getName() { 6616 return this.name == null ? null : this.name.getValue(); 6617 } 6618 6619 /** 6620 * @param value A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 6621 */ 6622 public PlanDefinition setName(String value) { 6623 if (Utilities.noString(value)) 6624 this.name = null; 6625 else { 6626 if (this.name == null) 6627 this.name = new StringType(); 6628 this.name.setValue(value); 6629 } 6630 return this; 6631 } 6632 6633 /** 6634 * @return {@link #title} (A short, descriptive, user-friendly title for the plan definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 6635 */ 6636 public StringType getTitleElement() { 6637 if (this.title == null) 6638 if (Configuration.errorOnAutoCreate()) 6639 throw new Error("Attempt to auto-create PlanDefinition.title"); 6640 else if (Configuration.doAutoCreate()) 6641 this.title = new StringType(); // bb 6642 return this.title; 6643 } 6644 6645 public boolean hasTitleElement() { 6646 return this.title != null && !this.title.isEmpty(); 6647 } 6648 6649 public boolean hasTitle() { 6650 return this.title != null && !this.title.isEmpty(); 6651 } 6652 6653 /** 6654 * @param value {@link #title} (A short, descriptive, user-friendly title for the plan definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 6655 */ 6656 public PlanDefinition setTitleElement(StringType value) { 6657 this.title = value; 6658 return this; 6659 } 6660 6661 /** 6662 * @return A short, descriptive, user-friendly title for the plan definition. 6663 */ 6664 public String getTitle() { 6665 return this.title == null ? null : this.title.getValue(); 6666 } 6667 6668 /** 6669 * @param value A short, descriptive, user-friendly title for the plan definition. 6670 */ 6671 public PlanDefinition setTitle(String value) { 6672 if (Utilities.noString(value)) 6673 this.title = null; 6674 else { 6675 if (this.title == null) 6676 this.title = new StringType(); 6677 this.title.setValue(value); 6678 } 6679 return this; 6680 } 6681 6682 /** 6683 * @return {@link #subtitle} (An explanatory or alternate title for the plan definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 6684 */ 6685 public StringType getSubtitleElement() { 6686 if (this.subtitle == null) 6687 if (Configuration.errorOnAutoCreate()) 6688 throw new Error("Attempt to auto-create PlanDefinition.subtitle"); 6689 else if (Configuration.doAutoCreate()) 6690 this.subtitle = new StringType(); // bb 6691 return this.subtitle; 6692 } 6693 6694 public boolean hasSubtitleElement() { 6695 return this.subtitle != null && !this.subtitle.isEmpty(); 6696 } 6697 6698 public boolean hasSubtitle() { 6699 return this.subtitle != null && !this.subtitle.isEmpty(); 6700 } 6701 6702 /** 6703 * @param value {@link #subtitle} (An explanatory or alternate title for the plan definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 6704 */ 6705 public PlanDefinition setSubtitleElement(StringType value) { 6706 this.subtitle = value; 6707 return this; 6708 } 6709 6710 /** 6711 * @return An explanatory or alternate title for the plan definition giving additional information about its content. 6712 */ 6713 public String getSubtitle() { 6714 return this.subtitle == null ? null : this.subtitle.getValue(); 6715 } 6716 6717 /** 6718 * @param value An explanatory or alternate title for the plan definition giving additional information about its content. 6719 */ 6720 public PlanDefinition setSubtitle(String value) { 6721 if (Utilities.noString(value)) 6722 this.subtitle = null; 6723 else { 6724 if (this.subtitle == null) 6725 this.subtitle = new StringType(); 6726 this.subtitle.setValue(value); 6727 } 6728 return this; 6729 } 6730 6731 /** 6732 * @return {@link #type} (A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.) 6733 */ 6734 public CodeableConcept getType() { 6735 if (this.type == null) 6736 if (Configuration.errorOnAutoCreate()) 6737 throw new Error("Attempt to auto-create PlanDefinition.type"); 6738 else if (Configuration.doAutoCreate()) 6739 this.type = new CodeableConcept(); // cc 6740 return this.type; 6741 } 6742 6743 public boolean hasType() { 6744 return this.type != null && !this.type.isEmpty(); 6745 } 6746 6747 /** 6748 * @param value {@link #type} (A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.) 6749 */ 6750 public PlanDefinition setType(CodeableConcept value) { 6751 this.type = value; 6752 return this; 6753 } 6754 6755 /** 6756 * @return {@link #status} (The status of this plan definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 6757 */ 6758 public Enumeration<PublicationStatus> getStatusElement() { 6759 if (this.status == null) 6760 if (Configuration.errorOnAutoCreate()) 6761 throw new Error("Attempt to auto-create PlanDefinition.status"); 6762 else if (Configuration.doAutoCreate()) 6763 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 6764 return this.status; 6765 } 6766 6767 public boolean hasStatusElement() { 6768 return this.status != null && !this.status.isEmpty(); 6769 } 6770 6771 public boolean hasStatus() { 6772 return this.status != null && !this.status.isEmpty(); 6773 } 6774 6775 /** 6776 * @param value {@link #status} (The status of this plan definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 6777 */ 6778 public PlanDefinition setStatusElement(Enumeration<PublicationStatus> value) { 6779 this.status = value; 6780 return this; 6781 } 6782 6783 /** 6784 * @return The status of this plan definition. Enables tracking the life-cycle of the content. 6785 */ 6786 public PublicationStatus getStatus() { 6787 return this.status == null ? null : this.status.getValue(); 6788 } 6789 6790 /** 6791 * @param value The status of this plan definition. Enables tracking the life-cycle of the content. 6792 */ 6793 public PlanDefinition setStatus(PublicationStatus value) { 6794 if (this.status == null) 6795 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 6796 this.status.setValue(value); 6797 return this; 6798 } 6799 6800 /** 6801 * @return {@link #experimental} (A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 6802 */ 6803 public BooleanType getExperimentalElement() { 6804 if (this.experimental == null) 6805 if (Configuration.errorOnAutoCreate()) 6806 throw new Error("Attempt to auto-create PlanDefinition.experimental"); 6807 else if (Configuration.doAutoCreate()) 6808 this.experimental = new BooleanType(); // bb 6809 return this.experimental; 6810 } 6811 6812 public boolean hasExperimentalElement() { 6813 return this.experimental != null && !this.experimental.isEmpty(); 6814 } 6815 6816 public boolean hasExperimental() { 6817 return this.experimental != null && !this.experimental.isEmpty(); 6818 } 6819 6820 /** 6821 * @param value {@link #experimental} (A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 6822 */ 6823 public PlanDefinition setExperimentalElement(BooleanType value) { 6824 this.experimental = value; 6825 return this; 6826 } 6827 6828 /** 6829 * @return A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 6830 */ 6831 public boolean getExperimental() { 6832 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 6833 } 6834 6835 /** 6836 * @param value A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 6837 */ 6838 public PlanDefinition setExperimental(boolean value) { 6839 if (this.experimental == null) 6840 this.experimental = new BooleanType(); 6841 this.experimental.setValue(value); 6842 return this; 6843 } 6844 6845 /** 6846 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 6847 */ 6848 public DataType getSubject() { 6849 return this.subject; 6850 } 6851 6852 /** 6853 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 6854 */ 6855 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 6856 if (this.subject == null) 6857 this.subject = new CodeableConcept(); 6858 if (!(this.subject instanceof CodeableConcept)) 6859 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 6860 return (CodeableConcept) this.subject; 6861 } 6862 6863 public boolean hasSubjectCodeableConcept() { 6864 return this.subject instanceof CodeableConcept; 6865 } 6866 6867 /** 6868 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 6869 */ 6870 public Reference getSubjectReference() throws FHIRException { 6871 if (this.subject == null) 6872 this.subject = new Reference(); 6873 if (!(this.subject instanceof Reference)) 6874 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 6875 return (Reference) this.subject; 6876 } 6877 6878 public boolean hasSubjectReference() { 6879 return this.subject instanceof Reference; 6880 } 6881 6882 /** 6883 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 6884 */ 6885 public CanonicalType getSubjectCanonicalType() throws FHIRException { 6886 if (this.subject == null) 6887 this.subject = new CanonicalType(); 6888 if (!(this.subject instanceof CanonicalType)) 6889 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.subject.getClass().getName()+" was encountered"); 6890 return (CanonicalType) this.subject; 6891 } 6892 6893 public boolean hasSubjectCanonicalType() { 6894 return this.subject instanceof CanonicalType; 6895 } 6896 6897 public boolean hasSubject() { 6898 return this.subject != null && !this.subject.isEmpty(); 6899 } 6900 6901 /** 6902 * @param value {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 6903 */ 6904 public PlanDefinition setSubject(DataType value) { 6905 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference || value instanceof CanonicalType)) 6906 throw new FHIRException("Not the right type for PlanDefinition.subject[x]: "+value.fhirType()); 6907 this.subject = value; 6908 return this; 6909 } 6910 6911 /** 6912 * @return {@link #date} (The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 6913 */ 6914 public DateTimeType getDateElement() { 6915 if (this.date == null) 6916 if (Configuration.errorOnAutoCreate()) 6917 throw new Error("Attempt to auto-create PlanDefinition.date"); 6918 else if (Configuration.doAutoCreate()) 6919 this.date = new DateTimeType(); // bb 6920 return this.date; 6921 } 6922 6923 public boolean hasDateElement() { 6924 return this.date != null && !this.date.isEmpty(); 6925 } 6926 6927 public boolean hasDate() { 6928 return this.date != null && !this.date.isEmpty(); 6929 } 6930 6931 /** 6932 * @param value {@link #date} (The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 6933 */ 6934 public PlanDefinition setDateElement(DateTimeType value) { 6935 this.date = value; 6936 return this; 6937 } 6938 6939 /** 6940 * @return The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes. 6941 */ 6942 public Date getDate() { 6943 return this.date == null ? null : this.date.getValue(); 6944 } 6945 6946 /** 6947 * @param value The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes. 6948 */ 6949 public PlanDefinition setDate(Date value) { 6950 if (value == null) 6951 this.date = null; 6952 else { 6953 if (this.date == null) 6954 this.date = new DateTimeType(); 6955 this.date.setValue(value); 6956 } 6957 return this; 6958 } 6959 6960 /** 6961 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6962 */ 6963 public StringType getPublisherElement() { 6964 if (this.publisher == null) 6965 if (Configuration.errorOnAutoCreate()) 6966 throw new Error("Attempt to auto-create PlanDefinition.publisher"); 6967 else if (Configuration.doAutoCreate()) 6968 this.publisher = new StringType(); // bb 6969 return this.publisher; 6970 } 6971 6972 public boolean hasPublisherElement() { 6973 return this.publisher != null && !this.publisher.isEmpty(); 6974 } 6975 6976 public boolean hasPublisher() { 6977 return this.publisher != null && !this.publisher.isEmpty(); 6978 } 6979 6980 /** 6981 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6982 */ 6983 public PlanDefinition setPublisherElement(StringType value) { 6984 this.publisher = value; 6985 return this; 6986 } 6987 6988 /** 6989 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition. 6990 */ 6991 public String getPublisher() { 6992 return this.publisher == null ? null : this.publisher.getValue(); 6993 } 6994 6995 /** 6996 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition. 6997 */ 6998 public PlanDefinition setPublisher(String value) { 6999 if (Utilities.noString(value)) 7000 this.publisher = null; 7001 else { 7002 if (this.publisher == null) 7003 this.publisher = new StringType(); 7004 this.publisher.setValue(value); 7005 } 7006 return this; 7007 } 7008 7009 /** 7010 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 7011 */ 7012 public List<ContactDetail> getContact() { 7013 if (this.contact == null) 7014 this.contact = new ArrayList<ContactDetail>(); 7015 return this.contact; 7016 } 7017 7018 /** 7019 * @return Returns a reference to <code>this</code> for easy method chaining 7020 */ 7021 public PlanDefinition setContact(List<ContactDetail> theContact) { 7022 this.contact = theContact; 7023 return this; 7024 } 7025 7026 public boolean hasContact() { 7027 if (this.contact == null) 7028 return false; 7029 for (ContactDetail item : this.contact) 7030 if (!item.isEmpty()) 7031 return true; 7032 return false; 7033 } 7034 7035 public ContactDetail addContact() { //3 7036 ContactDetail t = new ContactDetail(); 7037 if (this.contact == null) 7038 this.contact = new ArrayList<ContactDetail>(); 7039 this.contact.add(t); 7040 return t; 7041 } 7042 7043 public PlanDefinition addContact(ContactDetail t) { //3 7044 if (t == null) 7045 return this; 7046 if (this.contact == null) 7047 this.contact = new ArrayList<ContactDetail>(); 7048 this.contact.add(t); 7049 return this; 7050 } 7051 7052 /** 7053 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 7054 */ 7055 public ContactDetail getContactFirstRep() { 7056 if (getContact().isEmpty()) { 7057 addContact(); 7058 } 7059 return getContact().get(0); 7060 } 7061 7062 /** 7063 * @return {@link #description} (A free text natural language description of the plan definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 7064 */ 7065 public MarkdownType getDescriptionElement() { 7066 if (this.description == null) 7067 if (Configuration.errorOnAutoCreate()) 7068 throw new Error("Attempt to auto-create PlanDefinition.description"); 7069 else if (Configuration.doAutoCreate()) 7070 this.description = new MarkdownType(); // bb 7071 return this.description; 7072 } 7073 7074 public boolean hasDescriptionElement() { 7075 return this.description != null && !this.description.isEmpty(); 7076 } 7077 7078 public boolean hasDescription() { 7079 return this.description != null && !this.description.isEmpty(); 7080 } 7081 7082 /** 7083 * @param value {@link #description} (A free text natural language description of the plan definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 7084 */ 7085 public PlanDefinition setDescriptionElement(MarkdownType value) { 7086 this.description = value; 7087 return this; 7088 } 7089 7090 /** 7091 * @return A free text natural language description of the plan definition from a consumer's perspective. 7092 */ 7093 public String getDescription() { 7094 return this.description == null ? null : this.description.getValue(); 7095 } 7096 7097 /** 7098 * @param value A free text natural language description of the plan definition from a consumer's perspective. 7099 */ 7100 public PlanDefinition setDescription(String value) { 7101 if (Utilities.noString(value)) 7102 this.description = null; 7103 else { 7104 if (this.description == null) 7105 this.description = new MarkdownType(); 7106 this.description.setValue(value); 7107 } 7108 return this; 7109 } 7110 7111 /** 7112 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances.) 7113 */ 7114 public List<UsageContext> getUseContext() { 7115 if (this.useContext == null) 7116 this.useContext = new ArrayList<UsageContext>(); 7117 return this.useContext; 7118 } 7119 7120 /** 7121 * @return Returns a reference to <code>this</code> for easy method chaining 7122 */ 7123 public PlanDefinition setUseContext(List<UsageContext> theUseContext) { 7124 this.useContext = theUseContext; 7125 return this; 7126 } 7127 7128 public boolean hasUseContext() { 7129 if (this.useContext == null) 7130 return false; 7131 for (UsageContext item : this.useContext) 7132 if (!item.isEmpty()) 7133 return true; 7134 return false; 7135 } 7136 7137 public UsageContext addUseContext() { //3 7138 UsageContext t = new UsageContext(); 7139 if (this.useContext == null) 7140 this.useContext = new ArrayList<UsageContext>(); 7141 this.useContext.add(t); 7142 return t; 7143 } 7144 7145 public PlanDefinition addUseContext(UsageContext t) { //3 7146 if (t == null) 7147 return this; 7148 if (this.useContext == null) 7149 this.useContext = new ArrayList<UsageContext>(); 7150 this.useContext.add(t); 7151 return this; 7152 } 7153 7154 /** 7155 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 7156 */ 7157 public UsageContext getUseContextFirstRep() { 7158 if (getUseContext().isEmpty()) { 7159 addUseContext(); 7160 } 7161 return getUseContext().get(0); 7162 } 7163 7164 /** 7165 * @return {@link #jurisdiction} (A legal or geographic region in which the plan definition is intended to be used.) 7166 */ 7167 public List<CodeableConcept> getJurisdiction() { 7168 if (this.jurisdiction == null) 7169 this.jurisdiction = new ArrayList<CodeableConcept>(); 7170 return this.jurisdiction; 7171 } 7172 7173 /** 7174 * @return Returns a reference to <code>this</code> for easy method chaining 7175 */ 7176 public PlanDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 7177 this.jurisdiction = theJurisdiction; 7178 return this; 7179 } 7180 7181 public boolean hasJurisdiction() { 7182 if (this.jurisdiction == null) 7183 return false; 7184 for (CodeableConcept item : this.jurisdiction) 7185 if (!item.isEmpty()) 7186 return true; 7187 return false; 7188 } 7189 7190 public CodeableConcept addJurisdiction() { //3 7191 CodeableConcept t = new CodeableConcept(); 7192 if (this.jurisdiction == null) 7193 this.jurisdiction = new ArrayList<CodeableConcept>(); 7194 this.jurisdiction.add(t); 7195 return t; 7196 } 7197 7198 public PlanDefinition addJurisdiction(CodeableConcept t) { //3 7199 if (t == null) 7200 return this; 7201 if (this.jurisdiction == null) 7202 this.jurisdiction = new ArrayList<CodeableConcept>(); 7203 this.jurisdiction.add(t); 7204 return this; 7205 } 7206 7207 /** 7208 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 7209 */ 7210 public CodeableConcept getJurisdictionFirstRep() { 7211 if (getJurisdiction().isEmpty()) { 7212 addJurisdiction(); 7213 } 7214 return getJurisdiction().get(0); 7215 } 7216 7217 /** 7218 * @return {@link #purpose} (Explanation of why this plan definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 7219 */ 7220 public MarkdownType getPurposeElement() { 7221 if (this.purpose == null) 7222 if (Configuration.errorOnAutoCreate()) 7223 throw new Error("Attempt to auto-create PlanDefinition.purpose"); 7224 else if (Configuration.doAutoCreate()) 7225 this.purpose = new MarkdownType(); // bb 7226 return this.purpose; 7227 } 7228 7229 public boolean hasPurposeElement() { 7230 return this.purpose != null && !this.purpose.isEmpty(); 7231 } 7232 7233 public boolean hasPurpose() { 7234 return this.purpose != null && !this.purpose.isEmpty(); 7235 } 7236 7237 /** 7238 * @param value {@link #purpose} (Explanation of why this plan definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 7239 */ 7240 public PlanDefinition setPurposeElement(MarkdownType value) { 7241 this.purpose = value; 7242 return this; 7243 } 7244 7245 /** 7246 * @return Explanation of why this plan definition is needed and why it has been designed as it has. 7247 */ 7248 public String getPurpose() { 7249 return this.purpose == null ? null : this.purpose.getValue(); 7250 } 7251 7252 /** 7253 * @param value Explanation of why this plan definition is needed and why it has been designed as it has. 7254 */ 7255 public PlanDefinition setPurpose(String value) { 7256 if (Utilities.noString(value)) 7257 this.purpose = null; 7258 else { 7259 if (this.purpose == null) 7260 this.purpose = new MarkdownType(); 7261 this.purpose.setValue(value); 7262 } 7263 return this; 7264 } 7265 7266 /** 7267 * @return {@link #usage} (A detailed description of how the plan definition is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 7268 */ 7269 public MarkdownType getUsageElement() { 7270 if (this.usage == null) 7271 if (Configuration.errorOnAutoCreate()) 7272 throw new Error("Attempt to auto-create PlanDefinition.usage"); 7273 else if (Configuration.doAutoCreate()) 7274 this.usage = new MarkdownType(); // bb 7275 return this.usage; 7276 } 7277 7278 public boolean hasUsageElement() { 7279 return this.usage != null && !this.usage.isEmpty(); 7280 } 7281 7282 public boolean hasUsage() { 7283 return this.usage != null && !this.usage.isEmpty(); 7284 } 7285 7286 /** 7287 * @param value {@link #usage} (A detailed description of how the plan definition is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 7288 */ 7289 public PlanDefinition setUsageElement(MarkdownType value) { 7290 this.usage = value; 7291 return this; 7292 } 7293 7294 /** 7295 * @return A detailed description of how the plan definition is used from a clinical perspective. 7296 */ 7297 public String getUsage() { 7298 return this.usage == null ? null : this.usage.getValue(); 7299 } 7300 7301 /** 7302 * @param value A detailed description of how the plan definition is used from a clinical perspective. 7303 */ 7304 public PlanDefinition setUsage(String value) { 7305 if (Utilities.noString(value)) 7306 this.usage = null; 7307 else { 7308 if (this.usage == null) 7309 this.usage = new MarkdownType(); 7310 this.usage.setValue(value); 7311 } 7312 return this; 7313 } 7314 7315 /** 7316 * @return {@link #copyright} (A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 7317 */ 7318 public MarkdownType getCopyrightElement() { 7319 if (this.copyright == null) 7320 if (Configuration.errorOnAutoCreate()) 7321 throw new Error("Attempt to auto-create PlanDefinition.copyright"); 7322 else if (Configuration.doAutoCreate()) 7323 this.copyright = new MarkdownType(); // bb 7324 return this.copyright; 7325 } 7326 7327 public boolean hasCopyrightElement() { 7328 return this.copyright != null && !this.copyright.isEmpty(); 7329 } 7330 7331 public boolean hasCopyright() { 7332 return this.copyright != null && !this.copyright.isEmpty(); 7333 } 7334 7335 /** 7336 * @param value {@link #copyright} (A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 7337 */ 7338 public PlanDefinition setCopyrightElement(MarkdownType value) { 7339 this.copyright = value; 7340 return this; 7341 } 7342 7343 /** 7344 * @return A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition. 7345 */ 7346 public String getCopyright() { 7347 return this.copyright == null ? null : this.copyright.getValue(); 7348 } 7349 7350 /** 7351 * @param value A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition. 7352 */ 7353 public PlanDefinition setCopyright(String value) { 7354 if (Utilities.noString(value)) 7355 this.copyright = null; 7356 else { 7357 if (this.copyright == null) 7358 this.copyright = new MarkdownType(); 7359 this.copyright.setValue(value); 7360 } 7361 return this; 7362 } 7363 7364 /** 7365 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 7366 */ 7367 public StringType getCopyrightLabelElement() { 7368 if (this.copyrightLabel == null) 7369 if (Configuration.errorOnAutoCreate()) 7370 throw new Error("Attempt to auto-create PlanDefinition.copyrightLabel"); 7371 else if (Configuration.doAutoCreate()) 7372 this.copyrightLabel = new StringType(); // bb 7373 return this.copyrightLabel; 7374 } 7375 7376 public boolean hasCopyrightLabelElement() { 7377 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 7378 } 7379 7380 public boolean hasCopyrightLabel() { 7381 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 7382 } 7383 7384 /** 7385 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 7386 */ 7387 public PlanDefinition setCopyrightLabelElement(StringType value) { 7388 this.copyrightLabel = value; 7389 return this; 7390 } 7391 7392 /** 7393 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 7394 */ 7395 public String getCopyrightLabel() { 7396 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 7397 } 7398 7399 /** 7400 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 7401 */ 7402 public PlanDefinition setCopyrightLabel(String value) { 7403 if (Utilities.noString(value)) 7404 this.copyrightLabel = null; 7405 else { 7406 if (this.copyrightLabel == null) 7407 this.copyrightLabel = new StringType(); 7408 this.copyrightLabel.setValue(value); 7409 } 7410 return this; 7411 } 7412 7413 /** 7414 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 7415 */ 7416 public DateType getApprovalDateElement() { 7417 if (this.approvalDate == null) 7418 if (Configuration.errorOnAutoCreate()) 7419 throw new Error("Attempt to auto-create PlanDefinition.approvalDate"); 7420 else if (Configuration.doAutoCreate()) 7421 this.approvalDate = new DateType(); // bb 7422 return this.approvalDate; 7423 } 7424 7425 public boolean hasApprovalDateElement() { 7426 return this.approvalDate != null && !this.approvalDate.isEmpty(); 7427 } 7428 7429 public boolean hasApprovalDate() { 7430 return this.approvalDate != null && !this.approvalDate.isEmpty(); 7431 } 7432 7433 /** 7434 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 7435 */ 7436 public PlanDefinition setApprovalDateElement(DateType value) { 7437 this.approvalDate = value; 7438 return this; 7439 } 7440 7441 /** 7442 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 7443 */ 7444 public Date getApprovalDate() { 7445 return this.approvalDate == null ? null : this.approvalDate.getValue(); 7446 } 7447 7448 /** 7449 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 7450 */ 7451 public PlanDefinition setApprovalDate(Date value) { 7452 if (value == null) 7453 this.approvalDate = null; 7454 else { 7455 if (this.approvalDate == null) 7456 this.approvalDate = new DateType(); 7457 this.approvalDate.setValue(value); 7458 } 7459 return this; 7460 } 7461 7462 /** 7463 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 7464 */ 7465 public DateType getLastReviewDateElement() { 7466 if (this.lastReviewDate == null) 7467 if (Configuration.errorOnAutoCreate()) 7468 throw new Error("Attempt to auto-create PlanDefinition.lastReviewDate"); 7469 else if (Configuration.doAutoCreate()) 7470 this.lastReviewDate = new DateType(); // bb 7471 return this.lastReviewDate; 7472 } 7473 7474 public boolean hasLastReviewDateElement() { 7475 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 7476 } 7477 7478 public boolean hasLastReviewDate() { 7479 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 7480 } 7481 7482 /** 7483 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 7484 */ 7485 public PlanDefinition setLastReviewDateElement(DateType value) { 7486 this.lastReviewDate = value; 7487 return this; 7488 } 7489 7490 /** 7491 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 7492 */ 7493 public Date getLastReviewDate() { 7494 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 7495 } 7496 7497 /** 7498 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 7499 */ 7500 public PlanDefinition setLastReviewDate(Date value) { 7501 if (value == null) 7502 this.lastReviewDate = null; 7503 else { 7504 if (this.lastReviewDate == null) 7505 this.lastReviewDate = new DateType(); 7506 this.lastReviewDate.setValue(value); 7507 } 7508 return this; 7509 } 7510 7511 /** 7512 * @return {@link #effectivePeriod} (The period during which the plan definition content was or is planned to be in active use.) 7513 */ 7514 public Period getEffectivePeriod() { 7515 if (this.effectivePeriod == null) 7516 if (Configuration.errorOnAutoCreate()) 7517 throw new Error("Attempt to auto-create PlanDefinition.effectivePeriod"); 7518 else if (Configuration.doAutoCreate()) 7519 this.effectivePeriod = new Period(); // cc 7520 return this.effectivePeriod; 7521 } 7522 7523 public boolean hasEffectivePeriod() { 7524 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 7525 } 7526 7527 /** 7528 * @param value {@link #effectivePeriod} (The period during which the plan definition content was or is planned to be in active use.) 7529 */ 7530 public PlanDefinition setEffectivePeriod(Period value) { 7531 this.effectivePeriod = value; 7532 return this; 7533 } 7534 7535 /** 7536 * @return {@link #topic} (Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.) 7537 */ 7538 public List<CodeableConcept> getTopic() { 7539 if (this.topic == null) 7540 this.topic = new ArrayList<CodeableConcept>(); 7541 return this.topic; 7542 } 7543 7544 /** 7545 * @return Returns a reference to <code>this</code> for easy method chaining 7546 */ 7547 public PlanDefinition setTopic(List<CodeableConcept> theTopic) { 7548 this.topic = theTopic; 7549 return this; 7550 } 7551 7552 public boolean hasTopic() { 7553 if (this.topic == null) 7554 return false; 7555 for (CodeableConcept item : this.topic) 7556 if (!item.isEmpty()) 7557 return true; 7558 return false; 7559 } 7560 7561 public CodeableConcept addTopic() { //3 7562 CodeableConcept t = new CodeableConcept(); 7563 if (this.topic == null) 7564 this.topic = new ArrayList<CodeableConcept>(); 7565 this.topic.add(t); 7566 return t; 7567 } 7568 7569 public PlanDefinition addTopic(CodeableConcept t) { //3 7570 if (t == null) 7571 return this; 7572 if (this.topic == null) 7573 this.topic = new ArrayList<CodeableConcept>(); 7574 this.topic.add(t); 7575 return this; 7576 } 7577 7578 /** 7579 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 7580 */ 7581 public CodeableConcept getTopicFirstRep() { 7582 if (getTopic().isEmpty()) { 7583 addTopic(); 7584 } 7585 return getTopic().get(0); 7586 } 7587 7588 /** 7589 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the content.) 7590 */ 7591 public List<ContactDetail> getAuthor() { 7592 if (this.author == null) 7593 this.author = new ArrayList<ContactDetail>(); 7594 return this.author; 7595 } 7596 7597 /** 7598 * @return Returns a reference to <code>this</code> for easy method chaining 7599 */ 7600 public PlanDefinition setAuthor(List<ContactDetail> theAuthor) { 7601 this.author = theAuthor; 7602 return this; 7603 } 7604 7605 public boolean hasAuthor() { 7606 if (this.author == null) 7607 return false; 7608 for (ContactDetail item : this.author) 7609 if (!item.isEmpty()) 7610 return true; 7611 return false; 7612 } 7613 7614 public ContactDetail addAuthor() { //3 7615 ContactDetail t = new ContactDetail(); 7616 if (this.author == null) 7617 this.author = new ArrayList<ContactDetail>(); 7618 this.author.add(t); 7619 return t; 7620 } 7621 7622 public PlanDefinition addAuthor(ContactDetail t) { //3 7623 if (t == null) 7624 return this; 7625 if (this.author == null) 7626 this.author = new ArrayList<ContactDetail>(); 7627 this.author.add(t); 7628 return this; 7629 } 7630 7631 /** 7632 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 7633 */ 7634 public ContactDetail getAuthorFirstRep() { 7635 if (getAuthor().isEmpty()) { 7636 addAuthor(); 7637 } 7638 return getAuthor().get(0); 7639 } 7640 7641 /** 7642 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the content.) 7643 */ 7644 public List<ContactDetail> getEditor() { 7645 if (this.editor == null) 7646 this.editor = new ArrayList<ContactDetail>(); 7647 return this.editor; 7648 } 7649 7650 /** 7651 * @return Returns a reference to <code>this</code> for easy method chaining 7652 */ 7653 public PlanDefinition setEditor(List<ContactDetail> theEditor) { 7654 this.editor = theEditor; 7655 return this; 7656 } 7657 7658 public boolean hasEditor() { 7659 if (this.editor == null) 7660 return false; 7661 for (ContactDetail item : this.editor) 7662 if (!item.isEmpty()) 7663 return true; 7664 return false; 7665 } 7666 7667 public ContactDetail addEditor() { //3 7668 ContactDetail t = new ContactDetail(); 7669 if (this.editor == null) 7670 this.editor = new ArrayList<ContactDetail>(); 7671 this.editor.add(t); 7672 return t; 7673 } 7674 7675 public PlanDefinition addEditor(ContactDetail t) { //3 7676 if (t == null) 7677 return this; 7678 if (this.editor == null) 7679 this.editor = new ArrayList<ContactDetail>(); 7680 this.editor.add(t); 7681 return this; 7682 } 7683 7684 /** 7685 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 7686 */ 7687 public ContactDetail getEditorFirstRep() { 7688 if (getEditor().isEmpty()) { 7689 addEditor(); 7690 } 7691 return getEditor().get(0); 7692 } 7693 7694 /** 7695 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.) 7696 */ 7697 public List<ContactDetail> getReviewer() { 7698 if (this.reviewer == null) 7699 this.reviewer = new ArrayList<ContactDetail>(); 7700 return this.reviewer; 7701 } 7702 7703 /** 7704 * @return Returns a reference to <code>this</code> for easy method chaining 7705 */ 7706 public PlanDefinition setReviewer(List<ContactDetail> theReviewer) { 7707 this.reviewer = theReviewer; 7708 return this; 7709 } 7710 7711 public boolean hasReviewer() { 7712 if (this.reviewer == null) 7713 return false; 7714 for (ContactDetail item : this.reviewer) 7715 if (!item.isEmpty()) 7716 return true; 7717 return false; 7718 } 7719 7720 public ContactDetail addReviewer() { //3 7721 ContactDetail t = new ContactDetail(); 7722 if (this.reviewer == null) 7723 this.reviewer = new ArrayList<ContactDetail>(); 7724 this.reviewer.add(t); 7725 return t; 7726 } 7727 7728 public PlanDefinition addReviewer(ContactDetail t) { //3 7729 if (t == null) 7730 return this; 7731 if (this.reviewer == null) 7732 this.reviewer = new ArrayList<ContactDetail>(); 7733 this.reviewer.add(t); 7734 return this; 7735 } 7736 7737 /** 7738 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 7739 */ 7740 public ContactDetail getReviewerFirstRep() { 7741 if (getReviewer().isEmpty()) { 7742 addReviewer(); 7743 } 7744 return getReviewer().get(0); 7745 } 7746 7747 /** 7748 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.) 7749 */ 7750 public List<ContactDetail> getEndorser() { 7751 if (this.endorser == null) 7752 this.endorser = new ArrayList<ContactDetail>(); 7753 return this.endorser; 7754 } 7755 7756 /** 7757 * @return Returns a reference to <code>this</code> for easy method chaining 7758 */ 7759 public PlanDefinition setEndorser(List<ContactDetail> theEndorser) { 7760 this.endorser = theEndorser; 7761 return this; 7762 } 7763 7764 public boolean hasEndorser() { 7765 if (this.endorser == null) 7766 return false; 7767 for (ContactDetail item : this.endorser) 7768 if (!item.isEmpty()) 7769 return true; 7770 return false; 7771 } 7772 7773 public ContactDetail addEndorser() { //3 7774 ContactDetail t = new ContactDetail(); 7775 if (this.endorser == null) 7776 this.endorser = new ArrayList<ContactDetail>(); 7777 this.endorser.add(t); 7778 return t; 7779 } 7780 7781 public PlanDefinition addEndorser(ContactDetail t) { //3 7782 if (t == null) 7783 return this; 7784 if (this.endorser == null) 7785 this.endorser = new ArrayList<ContactDetail>(); 7786 this.endorser.add(t); 7787 return this; 7788 } 7789 7790 /** 7791 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 7792 */ 7793 public ContactDetail getEndorserFirstRep() { 7794 if (getEndorser().isEmpty()) { 7795 addEndorser(); 7796 } 7797 return getEndorser().get(0); 7798 } 7799 7800 /** 7801 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 7802 */ 7803 public List<RelatedArtifact> getRelatedArtifact() { 7804 if (this.relatedArtifact == null) 7805 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 7806 return this.relatedArtifact; 7807 } 7808 7809 /** 7810 * @return Returns a reference to <code>this</code> for easy method chaining 7811 */ 7812 public PlanDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 7813 this.relatedArtifact = theRelatedArtifact; 7814 return this; 7815 } 7816 7817 public boolean hasRelatedArtifact() { 7818 if (this.relatedArtifact == null) 7819 return false; 7820 for (RelatedArtifact item : this.relatedArtifact) 7821 if (!item.isEmpty()) 7822 return true; 7823 return false; 7824 } 7825 7826 public RelatedArtifact addRelatedArtifact() { //3 7827 RelatedArtifact t = new RelatedArtifact(); 7828 if (this.relatedArtifact == null) 7829 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 7830 this.relatedArtifact.add(t); 7831 return t; 7832 } 7833 7834 public PlanDefinition addRelatedArtifact(RelatedArtifact t) { //3 7835 if (t == null) 7836 return this; 7837 if (this.relatedArtifact == null) 7838 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 7839 this.relatedArtifact.add(t); 7840 return this; 7841 } 7842 7843 /** 7844 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 7845 */ 7846 public RelatedArtifact getRelatedArtifactFirstRep() { 7847 if (getRelatedArtifact().isEmpty()) { 7848 addRelatedArtifact(); 7849 } 7850 return getRelatedArtifact().get(0); 7851 } 7852 7853 /** 7854 * @return {@link #library} (A reference to a Library resource containing any formal logic used by the plan definition.) 7855 */ 7856 public List<CanonicalType> getLibrary() { 7857 if (this.library == null) 7858 this.library = new ArrayList<CanonicalType>(); 7859 return this.library; 7860 } 7861 7862 /** 7863 * @return Returns a reference to <code>this</code> for easy method chaining 7864 */ 7865 public PlanDefinition setLibrary(List<CanonicalType> theLibrary) { 7866 this.library = theLibrary; 7867 return this; 7868 } 7869 7870 public boolean hasLibrary() { 7871 if (this.library == null) 7872 return false; 7873 for (CanonicalType item : this.library) 7874 if (!item.isEmpty()) 7875 return true; 7876 return false; 7877 } 7878 7879 /** 7880 * @return {@link #library} (A reference to a Library resource containing any formal logic used by the plan definition.) 7881 */ 7882 public CanonicalType addLibraryElement() {//2 7883 CanonicalType t = new CanonicalType(); 7884 if (this.library == null) 7885 this.library = new ArrayList<CanonicalType>(); 7886 this.library.add(t); 7887 return t; 7888 } 7889 7890 /** 7891 * @param value {@link #library} (A reference to a Library resource containing any formal logic used by the plan definition.) 7892 */ 7893 public PlanDefinition addLibrary(String value) { //1 7894 CanonicalType t = new CanonicalType(); 7895 t.setValue(value); 7896 if (this.library == null) 7897 this.library = new ArrayList<CanonicalType>(); 7898 this.library.add(t); 7899 return this; 7900 } 7901 7902 /** 7903 * @param value {@link #library} (A reference to a Library resource containing any formal logic used by the plan definition.) 7904 */ 7905 public boolean hasLibrary(String value) { 7906 if (this.library == null) 7907 return false; 7908 for (CanonicalType v : this.library) 7909 if (v.getValue().equals(value)) // canonical 7910 return true; 7911 return false; 7912 } 7913 7914 /** 7915 * @return {@link #goal} (A goal describes an expected outcome that activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, meeting the acceptance criteria for a test as specified by a quality specification, etc.) 7916 */ 7917 public List<PlanDefinitionGoalComponent> getGoal() { 7918 if (this.goal == null) 7919 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 7920 return this.goal; 7921 } 7922 7923 /** 7924 * @return Returns a reference to <code>this</code> for easy method chaining 7925 */ 7926 public PlanDefinition setGoal(List<PlanDefinitionGoalComponent> theGoal) { 7927 this.goal = theGoal; 7928 return this; 7929 } 7930 7931 public boolean hasGoal() { 7932 if (this.goal == null) 7933 return false; 7934 for (PlanDefinitionGoalComponent item : this.goal) 7935 if (!item.isEmpty()) 7936 return true; 7937 return false; 7938 } 7939 7940 public PlanDefinitionGoalComponent addGoal() { //3 7941 PlanDefinitionGoalComponent t = new PlanDefinitionGoalComponent(); 7942 if (this.goal == null) 7943 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 7944 this.goal.add(t); 7945 return t; 7946 } 7947 7948 public PlanDefinition addGoal(PlanDefinitionGoalComponent t) { //3 7949 if (t == null) 7950 return this; 7951 if (this.goal == null) 7952 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 7953 this.goal.add(t); 7954 return this; 7955 } 7956 7957 /** 7958 * @return The first repetition of repeating field {@link #goal}, creating it if it does not already exist {3} 7959 */ 7960 public PlanDefinitionGoalComponent getGoalFirstRep() { 7961 if (getGoal().isEmpty()) { 7962 addGoal(); 7963 } 7964 return getGoal().get(0); 7965 } 7966 7967 /** 7968 * @return {@link #actor} (Actors represent the individuals or groups involved in the execution of the defined set of activities.) 7969 */ 7970 public List<PlanDefinitionActorComponent> getActor() { 7971 if (this.actor == null) 7972 this.actor = new ArrayList<PlanDefinitionActorComponent>(); 7973 return this.actor; 7974 } 7975 7976 /** 7977 * @return Returns a reference to <code>this</code> for easy method chaining 7978 */ 7979 public PlanDefinition setActor(List<PlanDefinitionActorComponent> theActor) { 7980 this.actor = theActor; 7981 return this; 7982 } 7983 7984 public boolean hasActor() { 7985 if (this.actor == null) 7986 return false; 7987 for (PlanDefinitionActorComponent item : this.actor) 7988 if (!item.isEmpty()) 7989 return true; 7990 return false; 7991 } 7992 7993 public PlanDefinitionActorComponent addActor() { //3 7994 PlanDefinitionActorComponent t = new PlanDefinitionActorComponent(); 7995 if (this.actor == null) 7996 this.actor = new ArrayList<PlanDefinitionActorComponent>(); 7997 this.actor.add(t); 7998 return t; 7999 } 8000 8001 public PlanDefinition addActor(PlanDefinitionActorComponent t) { //3 8002 if (t == null) 8003 return this; 8004 if (this.actor == null) 8005 this.actor = new ArrayList<PlanDefinitionActorComponent>(); 8006 this.actor.add(t); 8007 return this; 8008 } 8009 8010 /** 8011 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist {3} 8012 */ 8013 public PlanDefinitionActorComponent getActorFirstRep() { 8014 if (getActor().isEmpty()) { 8015 addActor(); 8016 } 8017 return getActor().get(0); 8018 } 8019 8020 /** 8021 * @return {@link #action} (An action or group of actions to be taken as part of the plan. For example, in clinical care, an action would be to prescribe a particular indicated medication, or perform a particular test as appropriate. In pharmaceutical quality, an action would be the test that needs to be performed on a drug product as defined in the quality specification.) 8022 */ 8023 public List<PlanDefinitionActionComponent> getAction() { 8024 if (this.action == null) 8025 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8026 return this.action; 8027 } 8028 8029 /** 8030 * @return Returns a reference to <code>this</code> for easy method chaining 8031 */ 8032 public PlanDefinition setAction(List<PlanDefinitionActionComponent> theAction) { 8033 this.action = theAction; 8034 return this; 8035 } 8036 8037 public boolean hasAction() { 8038 if (this.action == null) 8039 return false; 8040 for (PlanDefinitionActionComponent item : this.action) 8041 if (!item.isEmpty()) 8042 return true; 8043 return false; 8044 } 8045 8046 public PlanDefinitionActionComponent addAction() { //3 8047 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 8048 if (this.action == null) 8049 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8050 this.action.add(t); 8051 return t; 8052 } 8053 8054 public PlanDefinition addAction(PlanDefinitionActionComponent t) { //3 8055 if (t == null) 8056 return this; 8057 if (this.action == null) 8058 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8059 this.action.add(t); 8060 return this; 8061 } 8062 8063 /** 8064 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 8065 */ 8066 public PlanDefinitionActionComponent getActionFirstRep() { 8067 if (getAction().isEmpty()) { 8068 addAction(); 8069 } 8070 return getAction().get(0); 8071 } 8072 8073 /** 8074 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 8075 */ 8076 public DataType getAsNeeded() { 8077 return this.asNeeded; 8078 } 8079 8080 /** 8081 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 8082 */ 8083 public BooleanType getAsNeededBooleanType() throws FHIRException { 8084 if (this.asNeeded == null) 8085 this.asNeeded = new BooleanType(); 8086 if (!(this.asNeeded instanceof BooleanType)) 8087 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 8088 return (BooleanType) this.asNeeded; 8089 } 8090 8091 public boolean hasAsNeededBooleanType() { 8092 return this.asNeeded instanceof BooleanType; 8093 } 8094 8095 /** 8096 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 8097 */ 8098 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 8099 if (this.asNeeded == null) 8100 this.asNeeded = new CodeableConcept(); 8101 if (!(this.asNeeded instanceof CodeableConcept)) 8102 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 8103 return (CodeableConcept) this.asNeeded; 8104 } 8105 8106 public boolean hasAsNeededCodeableConcept() { 8107 return this.asNeeded instanceof CodeableConcept; 8108 } 8109 8110 public boolean hasAsNeeded() { 8111 return this.asNeeded != null && !this.asNeeded.isEmpty(); 8112 } 8113 8114 /** 8115 * @param value {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 8116 */ 8117 public PlanDefinition setAsNeeded(DataType value) { 8118 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 8119 throw new FHIRException("Not the right type for PlanDefinition.asNeeded[x]: "+value.fhirType()); 8120 this.asNeeded = value; 8121 return this; 8122 } 8123 8124 protected void listChildren(List<Property> children) { 8125 super.listChildren(children); 8126 children.add(new Property("url", "uri", "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.", 0, 1, url)); 8127 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 8128 children.add(new Property("version", "string", "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 8129 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 8130 children.add(new Property("name", "string", "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 8131 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the plan definition.", 0, 1, title)); 8132 children.add(new Property("subtitle", "string", "An explanatory or alternate title for the plan definition giving additional information about its content.", 0, 1, subtitle)); 8133 children.add(new Property("type", "CodeableConcept", "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.", 0, 1, type)); 8134 children.add(new Property("status", "code", "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 8135 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 8136 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)|canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject)); 8137 children.add(new Property("date", "dateTime", "The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 0, 1, date)); 8138 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition.", 0, 1, publisher)); 8139 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 8140 children.add(new Property("description", "markdown", "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, description)); 8141 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 8142 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the plan definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 8143 children.add(new Property("purpose", "markdown", "Explanation of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose)); 8144 children.add(new Property("usage", "markdown", "A detailed description of how the plan definition is used from a clinical perspective.", 0, 1, usage)); 8145 children.add(new Property("copyright", "markdown", "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 0, 1, copyright)); 8146 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 8147 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 8148 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 8149 children.add(new Property("effectivePeriod", "Period", "The period during which the plan definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 8150 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 8151 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 8152 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 8153 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 8154 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 8155 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 8156 children.add(new Property("library", "canonical(Library)", "A reference to a Library resource containing any formal logic used by the plan definition.", 0, java.lang.Integer.MAX_VALUE, library)); 8157 children.add(new Property("goal", "", "A goal describes an expected outcome that activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, meeting the acceptance criteria for a test as specified by a quality specification, etc.", 0, java.lang.Integer.MAX_VALUE, goal)); 8158 children.add(new Property("actor", "", "Actors represent the individuals or groups involved in the execution of the defined set of activities.", 0, java.lang.Integer.MAX_VALUE, actor)); 8159 children.add(new Property("action", "", "An action or group of actions to be taken as part of the plan. For example, in clinical care, an action would be to prescribe a particular indicated medication, or perform a particular test as appropriate. In pharmaceutical quality, an action would be the test that needs to be performed on a drug product as defined in the quality specification.", 0, java.lang.Integer.MAX_VALUE, action)); 8160 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded)); 8161 } 8162 8163 @Override 8164 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8165 switch (_hash) { 8166 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.", 0, 1, url); 8167 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 8168 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 8169 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8170 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8171 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8172 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8173 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 8174 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the plan definition.", 0, 1, title); 8175 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "An explanatory or alternate title for the plan definition giving additional information about its content.", 0, 1, subtitle); 8176 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.", 0, 1, type); 8177 case -892481550: /*status*/ return new Property("status", "code", "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status); 8178 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 8179 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)|canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 8180 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)|canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 8181 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 8182 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 8183 case -1768521432: /*subjectCanonical*/ return new Property("subject[x]", "canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the plan definition. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 8184 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the plan definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 0, 1, date); 8185 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the plan definition.", 0, 1, publisher); 8186 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 8187 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, description); 8188 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 8189 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the plan definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 8190 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose); 8191 case 111574433: /*usage*/ return new Property("usage", "markdown", "A detailed description of how the plan definition is used from a clinical perspective.", 0, 1, usage); 8192 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 0, 1, copyright); 8193 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 8194 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 8195 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 8196 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the plan definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 8197 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 8198 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 8199 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 8200 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 8201 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 8202 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 8203 case 166208699: /*library*/ return new Property("library", "canonical(Library)", "A reference to a Library resource containing any formal logic used by the plan definition.", 0, java.lang.Integer.MAX_VALUE, library); 8204 case 3178259: /*goal*/ return new Property("goal", "", "A goal describes an expected outcome that activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, meeting the acceptance criteria for a test as specified by a quality specification, etc.", 0, java.lang.Integer.MAX_VALUE, goal); 8205 case 92645877: /*actor*/ return new Property("actor", "", "Actors represent the individuals or groups involved in the execution of the defined set of activities.", 0, java.lang.Integer.MAX_VALUE, actor); 8206 case -1422950858: /*action*/ return new Property("action", "", "An action or group of actions to be taken as part of the plan. For example, in clinical care, an action would be to prescribe a particular indicated medication, or perform a particular test as appropriate. In pharmaceutical quality, an action would be the test that needs to be performed on a drug product as defined in the quality specification.", 0, java.lang.Integer.MAX_VALUE, action); 8207 case -544329575: /*asNeeded[x]*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 8208 case -1432923513: /*asNeeded*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 8209 case -591717471: /*asNeededBoolean*/ return new Property("asNeeded[x]", "boolean", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 8210 case 1556420122: /*asNeededCodeableConcept*/ return new Property("asNeeded[x]", "CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 8211 default: return super.getNamedProperty(_hash, _name, _checkValid); 8212 } 8213 8214 } 8215 8216 @Override 8217 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8218 switch (hash) { 8219 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 8220 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8221 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 8222 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 8223 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 8224 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 8225 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 8226 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 8227 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 8228 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 8229 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 8230 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 8231 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 8232 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 8233 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 8234 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 8235 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 8236 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 8237 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // MarkdownType 8238 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 8239 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 8240 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 8241 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 8242 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 8243 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 8244 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 8245 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 8246 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 8247 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 8248 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 8249 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 8250 case 3178259: /*goal*/ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // PlanDefinitionGoalComponent 8251 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // PlanDefinitionActorComponent 8252 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 8253 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // DataType 8254 default: return super.getProperty(hash, name, checkValid); 8255 } 8256 8257 } 8258 8259 @Override 8260 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8261 switch (hash) { 8262 case 116079: // url 8263 this.url = TypeConvertor.castToUri(value); // UriType 8264 return value; 8265 case -1618432855: // identifier 8266 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 8267 return value; 8268 case 351608024: // version 8269 this.version = TypeConvertor.castToString(value); // StringType 8270 return value; 8271 case 1508158071: // versionAlgorithm 8272 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 8273 return value; 8274 case 3373707: // name 8275 this.name = TypeConvertor.castToString(value); // StringType 8276 return value; 8277 case 110371416: // title 8278 this.title = TypeConvertor.castToString(value); // StringType 8279 return value; 8280 case -2060497896: // subtitle 8281 this.subtitle = TypeConvertor.castToString(value); // StringType 8282 return value; 8283 case 3575610: // type 8284 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8285 return value; 8286 case -892481550: // status 8287 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 8288 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8289 return value; 8290 case -404562712: // experimental 8291 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 8292 return value; 8293 case -1867885268: // subject 8294 this.subject = TypeConvertor.castToType(value); // DataType 8295 return value; 8296 case 3076014: // date 8297 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 8298 return value; 8299 case 1447404028: // publisher 8300 this.publisher = TypeConvertor.castToString(value); // StringType 8301 return value; 8302 case 951526432: // contact 8303 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 8304 return value; 8305 case -1724546052: // description 8306 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 8307 return value; 8308 case -669707736: // useContext 8309 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 8310 return value; 8311 case -507075711: // jurisdiction 8312 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 8313 return value; 8314 case -220463842: // purpose 8315 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 8316 return value; 8317 case 111574433: // usage 8318 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 8319 return value; 8320 case 1522889671: // copyright 8321 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 8322 return value; 8323 case 765157229: // copyrightLabel 8324 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 8325 return value; 8326 case 223539345: // approvalDate 8327 this.approvalDate = TypeConvertor.castToDate(value); // DateType 8328 return value; 8329 case -1687512484: // lastReviewDate 8330 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 8331 return value; 8332 case -403934648: // effectivePeriod 8333 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 8334 return value; 8335 case 110546223: // topic 8336 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 8337 return value; 8338 case -1406328437: // author 8339 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 8340 return value; 8341 case -1307827859: // editor 8342 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 8343 return value; 8344 case -261190139: // reviewer 8345 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 8346 return value; 8347 case 1740277666: // endorser 8348 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 8349 return value; 8350 case 666807069: // relatedArtifact 8351 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 8352 return value; 8353 case 166208699: // library 8354 this.getLibrary().add(TypeConvertor.castToCanonical(value)); // CanonicalType 8355 return value; 8356 case 3178259: // goal 8357 this.getGoal().add((PlanDefinitionGoalComponent) value); // PlanDefinitionGoalComponent 8358 return value; 8359 case 92645877: // actor 8360 this.getActor().add((PlanDefinitionActorComponent) value); // PlanDefinitionActorComponent 8361 return value; 8362 case -1422950858: // action 8363 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 8364 return value; 8365 case -1432923513: // asNeeded 8366 this.asNeeded = TypeConvertor.castToType(value); // DataType 8367 return value; 8368 default: return super.setProperty(hash, name, value); 8369 } 8370 8371 } 8372 8373 @Override 8374 public Base setProperty(String name, Base value) throws FHIRException { 8375 if (name.equals("url")) { 8376 this.url = TypeConvertor.castToUri(value); // UriType 8377 } else if (name.equals("identifier")) { 8378 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 8379 } else if (name.equals("version")) { 8380 this.version = TypeConvertor.castToString(value); // StringType 8381 } else if (name.equals("versionAlgorithm[x]")) { 8382 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 8383 } else if (name.equals("name")) { 8384 this.name = TypeConvertor.castToString(value); // StringType 8385 } else if (name.equals("title")) { 8386 this.title = TypeConvertor.castToString(value); // StringType 8387 } else if (name.equals("subtitle")) { 8388 this.subtitle = TypeConvertor.castToString(value); // StringType 8389 } else if (name.equals("type")) { 8390 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8391 } else if (name.equals("status")) { 8392 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 8393 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8394 } else if (name.equals("experimental")) { 8395 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 8396 } else if (name.equals("subject[x]")) { 8397 this.subject = TypeConvertor.castToType(value); // DataType 8398 } else if (name.equals("date")) { 8399 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 8400 } else if (name.equals("publisher")) { 8401 this.publisher = TypeConvertor.castToString(value); // StringType 8402 } else if (name.equals("contact")) { 8403 this.getContact().add(TypeConvertor.castToContactDetail(value)); 8404 } else if (name.equals("description")) { 8405 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 8406 } else if (name.equals("useContext")) { 8407 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 8408 } else if (name.equals("jurisdiction")) { 8409 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 8410 } else if (name.equals("purpose")) { 8411 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 8412 } else if (name.equals("usage")) { 8413 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 8414 } else if (name.equals("copyright")) { 8415 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 8416 } else if (name.equals("copyrightLabel")) { 8417 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 8418 } else if (name.equals("approvalDate")) { 8419 this.approvalDate = TypeConvertor.castToDate(value); // DateType 8420 } else if (name.equals("lastReviewDate")) { 8421 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 8422 } else if (name.equals("effectivePeriod")) { 8423 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 8424 } else if (name.equals("topic")) { 8425 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 8426 } else if (name.equals("author")) { 8427 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 8428 } else if (name.equals("editor")) { 8429 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 8430 } else if (name.equals("reviewer")) { 8431 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 8432 } else if (name.equals("endorser")) { 8433 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 8434 } else if (name.equals("relatedArtifact")) { 8435 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 8436 } else if (name.equals("library")) { 8437 this.getLibrary().add(TypeConvertor.castToCanonical(value)); 8438 } else if (name.equals("goal")) { 8439 this.getGoal().add((PlanDefinitionGoalComponent) value); 8440 } else if (name.equals("actor")) { 8441 this.getActor().add((PlanDefinitionActorComponent) value); 8442 } else if (name.equals("action")) { 8443 this.getAction().add((PlanDefinitionActionComponent) value); 8444 } else if (name.equals("asNeeded[x]")) { 8445 this.asNeeded = TypeConvertor.castToType(value); // DataType 8446 } else 8447 return super.setProperty(name, value); 8448 return value; 8449 } 8450 8451 @Override 8452 public void removeChild(String name, Base value) throws FHIRException { 8453 if (name.equals("url")) { 8454 this.url = null; 8455 } else if (name.equals("identifier")) { 8456 this.getIdentifier().remove(value); 8457 } else if (name.equals("version")) { 8458 this.version = null; 8459 } else if (name.equals("versionAlgorithm[x]")) { 8460 this.versionAlgorithm = null; 8461 } else if (name.equals("name")) { 8462 this.name = null; 8463 } else if (name.equals("title")) { 8464 this.title = null; 8465 } else if (name.equals("subtitle")) { 8466 this.subtitle = null; 8467 } else if (name.equals("type")) { 8468 this.type = null; 8469 } else if (name.equals("status")) { 8470 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 8471 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8472 } else if (name.equals("experimental")) { 8473 this.experimental = null; 8474 } else if (name.equals("subject[x]")) { 8475 this.subject = null; 8476 } else if (name.equals("date")) { 8477 this.date = null; 8478 } else if (name.equals("publisher")) { 8479 this.publisher = null; 8480 } else if (name.equals("contact")) { 8481 this.getContact().remove(value); 8482 } else if (name.equals("description")) { 8483 this.description = null; 8484 } else if (name.equals("useContext")) { 8485 this.getUseContext().remove(value); 8486 } else if (name.equals("jurisdiction")) { 8487 this.getJurisdiction().remove(value); 8488 } else if (name.equals("purpose")) { 8489 this.purpose = null; 8490 } else if (name.equals("usage")) { 8491 this.usage = null; 8492 } else if (name.equals("copyright")) { 8493 this.copyright = null; 8494 } else if (name.equals("copyrightLabel")) { 8495 this.copyrightLabel = null; 8496 } else if (name.equals("approvalDate")) { 8497 this.approvalDate = null; 8498 } else if (name.equals("lastReviewDate")) { 8499 this.lastReviewDate = null; 8500 } else if (name.equals("effectivePeriod")) { 8501 this.effectivePeriod = null; 8502 } else if (name.equals("topic")) { 8503 this.getTopic().remove(value); 8504 } else if (name.equals("author")) { 8505 this.getAuthor().remove(value); 8506 } else if (name.equals("editor")) { 8507 this.getEditor().remove(value); 8508 } else if (name.equals("reviewer")) { 8509 this.getReviewer().remove(value); 8510 } else if (name.equals("endorser")) { 8511 this.getEndorser().remove(value); 8512 } else if (name.equals("relatedArtifact")) { 8513 this.getRelatedArtifact().remove(value); 8514 } else if (name.equals("library")) { 8515 this.getLibrary().remove(value); 8516 } else if (name.equals("goal")) { 8517 this.getGoal().remove((PlanDefinitionGoalComponent) value); 8518 } else if (name.equals("actor")) { 8519 this.getActor().remove((PlanDefinitionActorComponent) value); 8520 } else if (name.equals("action")) { 8521 this.getAction().remove((PlanDefinitionActionComponent) value); 8522 } else if (name.equals("asNeeded[x]")) { 8523 this.asNeeded = null; 8524 } else 8525 super.removeChild(name, value); 8526 8527 } 8528 8529 @Override 8530 public Base makeProperty(int hash, String name) throws FHIRException { 8531 switch (hash) { 8532 case 116079: return getUrlElement(); 8533 case -1618432855: return addIdentifier(); 8534 case 351608024: return getVersionElement(); 8535 case -115699031: return getVersionAlgorithm(); 8536 case 1508158071: return getVersionAlgorithm(); 8537 case 3373707: return getNameElement(); 8538 case 110371416: return getTitleElement(); 8539 case -2060497896: return getSubtitleElement(); 8540 case 3575610: return getType(); 8541 case -892481550: return getStatusElement(); 8542 case -404562712: return getExperimentalElement(); 8543 case -573640748: return getSubject(); 8544 case -1867885268: return getSubject(); 8545 case 3076014: return getDateElement(); 8546 case 1447404028: return getPublisherElement(); 8547 case 951526432: return addContact(); 8548 case -1724546052: return getDescriptionElement(); 8549 case -669707736: return addUseContext(); 8550 case -507075711: return addJurisdiction(); 8551 case -220463842: return getPurposeElement(); 8552 case 111574433: return getUsageElement(); 8553 case 1522889671: return getCopyrightElement(); 8554 case 765157229: return getCopyrightLabelElement(); 8555 case 223539345: return getApprovalDateElement(); 8556 case -1687512484: return getLastReviewDateElement(); 8557 case -403934648: return getEffectivePeriod(); 8558 case 110546223: return addTopic(); 8559 case -1406328437: return addAuthor(); 8560 case -1307827859: return addEditor(); 8561 case -261190139: return addReviewer(); 8562 case 1740277666: return addEndorser(); 8563 case 666807069: return addRelatedArtifact(); 8564 case 166208699: return addLibraryElement(); 8565 case 3178259: return addGoal(); 8566 case 92645877: return addActor(); 8567 case -1422950858: return addAction(); 8568 case -544329575: return getAsNeeded(); 8569 case -1432923513: return getAsNeeded(); 8570 default: return super.makeProperty(hash, name); 8571 } 8572 8573 } 8574 8575 @Override 8576 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8577 switch (hash) { 8578 case 116079: /*url*/ return new String[] {"uri"}; 8579 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 8580 case 351608024: /*version*/ return new String[] {"string"}; 8581 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 8582 case 3373707: /*name*/ return new String[] {"string"}; 8583 case 110371416: /*title*/ return new String[] {"string"}; 8584 case -2060497896: /*subtitle*/ return new String[] {"string"}; 8585 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 8586 case -892481550: /*status*/ return new String[] {"code"}; 8587 case -404562712: /*experimental*/ return new String[] {"boolean"}; 8588 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference", "canonical"}; 8589 case 3076014: /*date*/ return new String[] {"dateTime"}; 8590 case 1447404028: /*publisher*/ return new String[] {"string"}; 8591 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 8592 case -1724546052: /*description*/ return new String[] {"markdown"}; 8593 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 8594 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 8595 case -220463842: /*purpose*/ return new String[] {"markdown"}; 8596 case 111574433: /*usage*/ return new String[] {"markdown"}; 8597 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 8598 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 8599 case 223539345: /*approvalDate*/ return new String[] {"date"}; 8600 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 8601 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 8602 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 8603 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 8604 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 8605 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 8606 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 8607 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 8608 case 166208699: /*library*/ return new String[] {"canonical"}; 8609 case 3178259: /*goal*/ return new String[] {}; 8610 case 92645877: /*actor*/ return new String[] {}; 8611 case -1422950858: /*action*/ return new String[] {}; 8612 case -1432923513: /*asNeeded*/ return new String[] {"boolean", "CodeableConcept"}; 8613 default: return super.getTypesForProperty(hash, name); 8614 } 8615 8616 } 8617 8618 @Override 8619 public Base addChild(String name) throws FHIRException { 8620 if (name.equals("url")) { 8621 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.url"); 8622 } 8623 else if (name.equals("identifier")) { 8624 return addIdentifier(); 8625 } 8626 else if (name.equals("version")) { 8627 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.version"); 8628 } 8629 else if (name.equals("versionAlgorithmString")) { 8630 this.versionAlgorithm = new StringType(); 8631 return this.versionAlgorithm; 8632 } 8633 else if (name.equals("versionAlgorithmCoding")) { 8634 this.versionAlgorithm = new Coding(); 8635 return this.versionAlgorithm; 8636 } 8637 else if (name.equals("name")) { 8638 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.name"); 8639 } 8640 else if (name.equals("title")) { 8641 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.title"); 8642 } 8643 else if (name.equals("subtitle")) { 8644 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.subtitle"); 8645 } 8646 else if (name.equals("type")) { 8647 this.type = new CodeableConcept(); 8648 return this.type; 8649 } 8650 else if (name.equals("status")) { 8651 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.status"); 8652 } 8653 else if (name.equals("experimental")) { 8654 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.experimental"); 8655 } 8656 else if (name.equals("subjectCodeableConcept")) { 8657 this.subject = new CodeableConcept(); 8658 return this.subject; 8659 } 8660 else if (name.equals("subjectReference")) { 8661 this.subject = new Reference(); 8662 return this.subject; 8663 } 8664 else if (name.equals("subjectCanonical")) { 8665 this.subject = new CanonicalType(); 8666 return this.subject; 8667 } 8668 else if (name.equals("date")) { 8669 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.date"); 8670 } 8671 else if (name.equals("publisher")) { 8672 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.publisher"); 8673 } 8674 else if (name.equals("contact")) { 8675 return addContact(); 8676 } 8677 else if (name.equals("description")) { 8678 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 8679 } 8680 else if (name.equals("useContext")) { 8681 return addUseContext(); 8682 } 8683 else if (name.equals("jurisdiction")) { 8684 return addJurisdiction(); 8685 } 8686 else if (name.equals("purpose")) { 8687 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.purpose"); 8688 } 8689 else if (name.equals("usage")) { 8690 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.usage"); 8691 } 8692 else if (name.equals("copyright")) { 8693 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.copyright"); 8694 } 8695 else if (name.equals("copyrightLabel")) { 8696 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.copyrightLabel"); 8697 } 8698 else if (name.equals("approvalDate")) { 8699 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.approvalDate"); 8700 } 8701 else if (name.equals("lastReviewDate")) { 8702 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.lastReviewDate"); 8703 } 8704 else if (name.equals("effectivePeriod")) { 8705 this.effectivePeriod = new Period(); 8706 return this.effectivePeriod; 8707 } 8708 else if (name.equals("topic")) { 8709 return addTopic(); 8710 } 8711 else if (name.equals("author")) { 8712 return addAuthor(); 8713 } 8714 else if (name.equals("editor")) { 8715 return addEditor(); 8716 } 8717 else if (name.equals("reviewer")) { 8718 return addReviewer(); 8719 } 8720 else if (name.equals("endorser")) { 8721 return addEndorser(); 8722 } 8723 else if (name.equals("relatedArtifact")) { 8724 return addRelatedArtifact(); 8725 } 8726 else if (name.equals("library")) { 8727 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.library"); 8728 } 8729 else if (name.equals("goal")) { 8730 return addGoal(); 8731 } 8732 else if (name.equals("actor")) { 8733 return addActor(); 8734 } 8735 else if (name.equals("action")) { 8736 return addAction(); 8737 } 8738 else if (name.equals("asNeededBoolean")) { 8739 this.asNeeded = new BooleanType(); 8740 return this.asNeeded; 8741 } 8742 else if (name.equals("asNeededCodeableConcept")) { 8743 this.asNeeded = new CodeableConcept(); 8744 return this.asNeeded; 8745 } 8746 else 8747 return super.addChild(name); 8748 } 8749 8750 public String fhirType() { 8751 return "PlanDefinition"; 8752 8753 } 8754 8755 public PlanDefinition copy() { 8756 PlanDefinition dst = new PlanDefinition(); 8757 copyValues(dst); 8758 return dst; 8759 } 8760 8761 public void copyValues(PlanDefinition dst) { 8762 super.copyValues(dst); 8763 dst.url = url == null ? null : url.copy(); 8764 if (identifier != null) { 8765 dst.identifier = new ArrayList<Identifier>(); 8766 for (Identifier i : identifier) 8767 dst.identifier.add(i.copy()); 8768 }; 8769 dst.version = version == null ? null : version.copy(); 8770 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 8771 dst.name = name == null ? null : name.copy(); 8772 dst.title = title == null ? null : title.copy(); 8773 dst.subtitle = subtitle == null ? null : subtitle.copy(); 8774 dst.type = type == null ? null : type.copy(); 8775 dst.status = status == null ? null : status.copy(); 8776 dst.experimental = experimental == null ? null : experimental.copy(); 8777 dst.subject = subject == null ? null : subject.copy(); 8778 dst.date = date == null ? null : date.copy(); 8779 dst.publisher = publisher == null ? null : publisher.copy(); 8780 if (contact != null) { 8781 dst.contact = new ArrayList<ContactDetail>(); 8782 for (ContactDetail i : contact) 8783 dst.contact.add(i.copy()); 8784 }; 8785 dst.description = description == null ? null : description.copy(); 8786 if (useContext != null) { 8787 dst.useContext = new ArrayList<UsageContext>(); 8788 for (UsageContext i : useContext) 8789 dst.useContext.add(i.copy()); 8790 }; 8791 if (jurisdiction != null) { 8792 dst.jurisdiction = new ArrayList<CodeableConcept>(); 8793 for (CodeableConcept i : jurisdiction) 8794 dst.jurisdiction.add(i.copy()); 8795 }; 8796 dst.purpose = purpose == null ? null : purpose.copy(); 8797 dst.usage = usage == null ? null : usage.copy(); 8798 dst.copyright = copyright == null ? null : copyright.copy(); 8799 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 8800 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 8801 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 8802 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 8803 if (topic != null) { 8804 dst.topic = new ArrayList<CodeableConcept>(); 8805 for (CodeableConcept i : topic) 8806 dst.topic.add(i.copy()); 8807 }; 8808 if (author != null) { 8809 dst.author = new ArrayList<ContactDetail>(); 8810 for (ContactDetail i : author) 8811 dst.author.add(i.copy()); 8812 }; 8813 if (editor != null) { 8814 dst.editor = new ArrayList<ContactDetail>(); 8815 for (ContactDetail i : editor) 8816 dst.editor.add(i.copy()); 8817 }; 8818 if (reviewer != null) { 8819 dst.reviewer = new ArrayList<ContactDetail>(); 8820 for (ContactDetail i : reviewer) 8821 dst.reviewer.add(i.copy()); 8822 }; 8823 if (endorser != null) { 8824 dst.endorser = new ArrayList<ContactDetail>(); 8825 for (ContactDetail i : endorser) 8826 dst.endorser.add(i.copy()); 8827 }; 8828 if (relatedArtifact != null) { 8829 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 8830 for (RelatedArtifact i : relatedArtifact) 8831 dst.relatedArtifact.add(i.copy()); 8832 }; 8833 if (library != null) { 8834 dst.library = new ArrayList<CanonicalType>(); 8835 for (CanonicalType i : library) 8836 dst.library.add(i.copy()); 8837 }; 8838 if (goal != null) { 8839 dst.goal = new ArrayList<PlanDefinitionGoalComponent>(); 8840 for (PlanDefinitionGoalComponent i : goal) 8841 dst.goal.add(i.copy()); 8842 }; 8843 if (actor != null) { 8844 dst.actor = new ArrayList<PlanDefinitionActorComponent>(); 8845 for (PlanDefinitionActorComponent i : actor) 8846 dst.actor.add(i.copy()); 8847 }; 8848 if (action != null) { 8849 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 8850 for (PlanDefinitionActionComponent i : action) 8851 dst.action.add(i.copy()); 8852 }; 8853 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 8854 } 8855 8856 protected PlanDefinition typedCopy() { 8857 return copy(); 8858 } 8859 8860 @Override 8861 public boolean equalsDeep(Base other_) { 8862 if (!super.equalsDeep(other_)) 8863 return false; 8864 if (!(other_ instanceof PlanDefinition)) 8865 return false; 8866 PlanDefinition o = (PlanDefinition) other_; 8867 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 8868 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 8869 && compareDeep(subtitle, o.subtitle, true) && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 8870 && compareDeep(experimental, o.experimental, true) && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) 8871 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 8872 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 8873 && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 8874 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 8875 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 8876 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 8877 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 8878 && compareDeep(library, o.library, true) && compareDeep(goal, o.goal, true) && compareDeep(actor, o.actor, true) 8879 && compareDeep(action, o.action, true) && compareDeep(asNeeded, o.asNeeded, true); 8880 } 8881 8882 @Override 8883 public boolean equalsShallow(Base other_) { 8884 if (!super.equalsShallow(other_)) 8885 return false; 8886 if (!(other_ instanceof PlanDefinition)) 8887 return false; 8888 PlanDefinition o = (PlanDefinition) other_; 8889 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 8890 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) && compareValues(status, o.status, true) 8891 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 8892 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) 8893 && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 8894 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 8895 && compareValues(library, o.library, true); 8896 } 8897 8898 public boolean isEmpty() { 8899 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 8900 , versionAlgorithm, name, title, subtitle, type, status, experimental, subject 8901 , date, publisher, contact, description, useContext, jurisdiction, purpose, usage 8902 , copyright, copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, topic 8903 , author, editor, reviewer, endorser, relatedArtifact, library, goal, actor 8904 , action, asNeeded); 8905 } 8906 8907 @Override 8908 public ResourceType getResourceType() { 8909 return ResourceType.PlanDefinition; 8910 } 8911 8912 /** 8913 * Search parameter: <b>context-quantity</b> 8914 * <p> 8915 * Description: <b>Multiple Resources: 8916 8917* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 8918* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 8919* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 8920* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 8921* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 8922* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 8923* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 8924* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 8925* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 8926* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 8927* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 8928* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 8929* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 8930* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 8931* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 8932* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 8933* [Library](library.html): A quantity- or range-valued use context assigned to the library 8934* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 8935* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 8936* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 8937* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 8938* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 8939* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 8940* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 8941* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 8942* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 8943* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 8944* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 8945* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 8946* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 8947</b><br> 8948 * Type: <b>quantity</b><br> 8949 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 8950 * </p> 8951 */ 8952 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 8953 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 8954 /** 8955 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 8956 * <p> 8957 * Description: <b>Multiple Resources: 8958 8959* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 8960* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 8961* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 8962* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 8963* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 8964* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 8965* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 8966* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 8967* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 8968* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 8969* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 8970* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 8971* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 8972* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 8973* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 8974* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 8975* [Library](library.html): A quantity- or range-valued use context assigned to the library 8976* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 8977* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 8978* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 8979* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 8980* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 8981* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 8982* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 8983* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 8984* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 8985* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 8986* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 8987* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 8988* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 8989</b><br> 8990 * Type: <b>quantity</b><br> 8991 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 8992 * </p> 8993 */ 8994 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 8995 8996 /** 8997 * Search parameter: <b>context-type-quantity</b> 8998 * <p> 8999 * Description: <b>Multiple Resources: 9000 9001* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 9002* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 9003* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 9004* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 9005* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 9006* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 9007* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 9008* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 9009* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 9010* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 9011* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 9012* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 9013* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 9014* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 9015* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 9016* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 9017* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 9018* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 9019* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 9020* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 9021* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 9022* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 9023* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 9024* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 9025* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 9026* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 9027* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 9028* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 9029* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 9030* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 9031</b><br> 9032 * Type: <b>composite</b><br> 9033 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9034 * </p> 9035 */ 9036 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 9037 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 9038 /** 9039 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 9040 * <p> 9041 * Description: <b>Multiple Resources: 9042 9043* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 9044* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 9045* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 9046* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 9047* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 9048* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 9049* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 9050* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 9051* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 9052* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 9053* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 9054* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 9055* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 9056* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 9057* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 9058* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 9059* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 9060* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 9061* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 9062* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 9063* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 9064* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 9065* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 9066* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 9067* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 9068* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 9069* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 9070* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 9071* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 9072* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 9073</b><br> 9074 * Type: <b>composite</b><br> 9075 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9076 * </p> 9077 */ 9078 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 9079 9080 /** 9081 * Search parameter: <b>context-type-value</b> 9082 * <p> 9083 * Description: <b>Multiple Resources: 9084 9085* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 9086* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 9087* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 9088* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 9089* [Citation](citation.html): A use context type and value assigned to the citation 9090* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 9091* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 9092* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 9093* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 9094* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 9095* [Evidence](evidence.html): A use context type and value assigned to the evidence 9096* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 9097* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 9098* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 9099* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 9100* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 9101* [Library](library.html): A use context type and value assigned to the library 9102* [Measure](measure.html): A use context type and value assigned to the measure 9103* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 9104* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 9105* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 9106* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 9107* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 9108* [Requirements](requirements.html): A use context type and value assigned to the requirements 9109* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 9110* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 9111* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 9112* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 9113* [TestScript](testscript.html): A use context type and value assigned to the test script 9114* [ValueSet](valueset.html): A use context type and value assigned to the value set 9115</b><br> 9116 * Type: <b>composite</b><br> 9117 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9118 * </p> 9119 */ 9120 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 9121 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 9122 /** 9123 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 9124 * <p> 9125 * Description: <b>Multiple Resources: 9126 9127* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 9128* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 9129* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 9130* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 9131* [Citation](citation.html): A use context type and value assigned to the citation 9132* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 9133* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 9134* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 9135* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 9136* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 9137* [Evidence](evidence.html): A use context type and value assigned to the evidence 9138* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 9139* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 9140* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 9141* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 9142* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 9143* [Library](library.html): A use context type and value assigned to the library 9144* [Measure](measure.html): A use context type and value assigned to the measure 9145* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 9146* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 9147* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 9148* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 9149* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 9150* [Requirements](requirements.html): A use context type and value assigned to the requirements 9151* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 9152* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 9153* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 9154* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 9155* [TestScript](testscript.html): A use context type and value assigned to the test script 9156* [ValueSet](valueset.html): A use context type and value assigned to the value set 9157</b><br> 9158 * Type: <b>composite</b><br> 9159 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9160 * </p> 9161 */ 9162 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 9163 9164 /** 9165 * Search parameter: <b>context-type</b> 9166 * <p> 9167 * Description: <b>Multiple Resources: 9168 9169* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 9170* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 9171* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 9172* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 9173* [Citation](citation.html): A type of use context assigned to the citation 9174* [CodeSystem](codesystem.html): A type of use context assigned to the code system 9175* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 9176* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 9177* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 9178* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 9179* [Evidence](evidence.html): A type of use context assigned to the evidence 9180* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 9181* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 9182* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 9183* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 9184* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 9185* [Library](library.html): A type of use context assigned to the library 9186* [Measure](measure.html): A type of use context assigned to the measure 9187* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 9188* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 9189* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 9190* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 9191* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 9192* [Requirements](requirements.html): A type of use context assigned to the requirements 9193* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 9194* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 9195* [StructureMap](structuremap.html): A type of use context assigned to the structure map 9196* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 9197* [TestScript](testscript.html): A type of use context assigned to the test script 9198* [ValueSet](valueset.html): A type of use context assigned to the value set 9199</b><br> 9200 * Type: <b>token</b><br> 9201 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 9202 * </p> 9203 */ 9204 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 9205 public static final String SP_CONTEXT_TYPE = "context-type"; 9206 /** 9207 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 9208 * <p> 9209 * Description: <b>Multiple Resources: 9210 9211* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 9212* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 9213* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 9214* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 9215* [Citation](citation.html): A type of use context assigned to the citation 9216* [CodeSystem](codesystem.html): A type of use context assigned to the code system 9217* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 9218* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 9219* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 9220* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 9221* [Evidence](evidence.html): A type of use context assigned to the evidence 9222* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 9223* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 9224* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 9225* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 9226* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 9227* [Library](library.html): A type of use context assigned to the library 9228* [Measure](measure.html): A type of use context assigned to the measure 9229* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 9230* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 9231* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 9232* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 9233* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 9234* [Requirements](requirements.html): A type of use context assigned to the requirements 9235* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 9236* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 9237* [StructureMap](structuremap.html): A type of use context assigned to the structure map 9238* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 9239* [TestScript](testscript.html): A type of use context assigned to the test script 9240* [ValueSet](valueset.html): A type of use context assigned to the value set 9241</b><br> 9242 * Type: <b>token</b><br> 9243 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 9244 * </p> 9245 */ 9246 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 9247 9248 /** 9249 * Search parameter: <b>context</b> 9250 * <p> 9251 * Description: <b>Multiple Resources: 9252 9253* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 9254* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 9255* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 9256* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 9257* [Citation](citation.html): A use context assigned to the citation 9258* [CodeSystem](codesystem.html): A use context assigned to the code system 9259* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 9260* [ConceptMap](conceptmap.html): A use context assigned to the concept map 9261* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 9262* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 9263* [Evidence](evidence.html): A use context assigned to the evidence 9264* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 9265* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 9266* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 9267* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 9268* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 9269* [Library](library.html): A use context assigned to the library 9270* [Measure](measure.html): A use context assigned to the measure 9271* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 9272* [NamingSystem](namingsystem.html): A use context assigned to the naming system 9273* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 9274* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 9275* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 9276* [Requirements](requirements.html): A use context assigned to the requirements 9277* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 9278* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 9279* [StructureMap](structuremap.html): A use context assigned to the structure map 9280* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 9281* [TestScript](testscript.html): A use context assigned to the test script 9282* [ValueSet](valueset.html): A use context assigned to the value set 9283</b><br> 9284 * Type: <b>token</b><br> 9285 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 9286 * </p> 9287 */ 9288 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 9289 public static final String SP_CONTEXT = "context"; 9290 /** 9291 * <b>Fluent Client</b> search parameter constant for <b>context</b> 9292 * <p> 9293 * Description: <b>Multiple Resources: 9294 9295* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 9296* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 9297* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 9298* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 9299* [Citation](citation.html): A use context assigned to the citation 9300* [CodeSystem](codesystem.html): A use context assigned to the code system 9301* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 9302* [ConceptMap](conceptmap.html): A use context assigned to the concept map 9303* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 9304* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 9305* [Evidence](evidence.html): A use context assigned to the evidence 9306* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 9307* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 9308* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 9309* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 9310* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 9311* [Library](library.html): A use context assigned to the library 9312* [Measure](measure.html): A use context assigned to the measure 9313* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 9314* [NamingSystem](namingsystem.html): A use context assigned to the naming system 9315* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 9316* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 9317* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 9318* [Requirements](requirements.html): A use context assigned to the requirements 9319* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 9320* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 9321* [StructureMap](structuremap.html): A use context assigned to the structure map 9322* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 9323* [TestScript](testscript.html): A use context assigned to the test script 9324* [ValueSet](valueset.html): A use context assigned to the value set 9325</b><br> 9326 * Type: <b>token</b><br> 9327 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 9328 * </p> 9329 */ 9330 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 9331 9332 /** 9333 * Search parameter: <b>date</b> 9334 * <p> 9335 * Description: <b>Multiple Resources: 9336 9337* [ActivityDefinition](activitydefinition.html): The activity definition publication date 9338* [ActorDefinition](actordefinition.html): The Actor Definition publication date 9339* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 9340* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 9341* [Citation](citation.html): The citation publication date 9342* [CodeSystem](codesystem.html): The code system publication date 9343* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 9344* [ConceptMap](conceptmap.html): The concept map publication date 9345* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 9346* [EventDefinition](eventdefinition.html): The event definition publication date 9347* [Evidence](evidence.html): The evidence publication date 9348* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 9349* [ExampleScenario](examplescenario.html): The example scenario publication date 9350* [GraphDefinition](graphdefinition.html): The graph definition publication date 9351* [ImplementationGuide](implementationguide.html): The implementation guide publication date 9352* [Library](library.html): The library publication date 9353* [Measure](measure.html): The measure publication date 9354* [MessageDefinition](messagedefinition.html): The message definition publication date 9355* [NamingSystem](namingsystem.html): The naming system publication date 9356* [OperationDefinition](operationdefinition.html): The operation definition publication date 9357* [PlanDefinition](plandefinition.html): The plan definition publication date 9358* [Questionnaire](questionnaire.html): The questionnaire publication date 9359* [Requirements](requirements.html): The requirements publication date 9360* [SearchParameter](searchparameter.html): The search parameter publication date 9361* [StructureDefinition](structuredefinition.html): The structure definition publication date 9362* [StructureMap](structuremap.html): The structure map publication date 9363* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 9364* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 9365* [TestScript](testscript.html): The test script publication date 9366* [ValueSet](valueset.html): The value set publication date 9367</b><br> 9368 * Type: <b>date</b><br> 9369 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 9370 * </p> 9371 */ 9372 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 9373 public static final String SP_DATE = "date"; 9374 /** 9375 * <b>Fluent Client</b> search parameter constant for <b>date</b> 9376 * <p> 9377 * Description: <b>Multiple Resources: 9378 9379* [ActivityDefinition](activitydefinition.html): The activity definition publication date 9380* [ActorDefinition](actordefinition.html): The Actor Definition publication date 9381* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 9382* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 9383* [Citation](citation.html): The citation publication date 9384* [CodeSystem](codesystem.html): The code system publication date 9385* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 9386* [ConceptMap](conceptmap.html): The concept map publication date 9387* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 9388* [EventDefinition](eventdefinition.html): The event definition publication date 9389* [Evidence](evidence.html): The evidence publication date 9390* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 9391* [ExampleScenario](examplescenario.html): The example scenario publication date 9392* [GraphDefinition](graphdefinition.html): The graph definition publication date 9393* [ImplementationGuide](implementationguide.html): The implementation guide publication date 9394* [Library](library.html): The library publication date 9395* [Measure](measure.html): The measure publication date 9396* [MessageDefinition](messagedefinition.html): The message definition publication date 9397* [NamingSystem](namingsystem.html): The naming system publication date 9398* [OperationDefinition](operationdefinition.html): The operation definition publication date 9399* [PlanDefinition](plandefinition.html): The plan definition publication date 9400* [Questionnaire](questionnaire.html): The questionnaire publication date 9401* [Requirements](requirements.html): The requirements publication date 9402* [SearchParameter](searchparameter.html): The search parameter publication date 9403* [StructureDefinition](structuredefinition.html): The structure definition publication date 9404* [StructureMap](structuremap.html): The structure map publication date 9405* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 9406* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 9407* [TestScript](testscript.html): The test script publication date 9408* [ValueSet](valueset.html): The value set publication date 9409</b><br> 9410 * Type: <b>date</b><br> 9411 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 9412 * </p> 9413 */ 9414 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 9415 9416 /** 9417 * Search parameter: <b>description</b> 9418 * <p> 9419 * Description: <b>Multiple Resources: 9420 9421* [ActivityDefinition](activitydefinition.html): The description of the activity definition 9422* [ActorDefinition](actordefinition.html): The description of the Actor Definition 9423* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 9424* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 9425* [Citation](citation.html): The description of the citation 9426* [CodeSystem](codesystem.html): The description of the code system 9427* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 9428* [ConceptMap](conceptmap.html): The description of the concept map 9429* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 9430* [EventDefinition](eventdefinition.html): The description of the event definition 9431* [Evidence](evidence.html): The description of the evidence 9432* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 9433* [GraphDefinition](graphdefinition.html): The description of the graph definition 9434* [ImplementationGuide](implementationguide.html): The description of the implementation guide 9435* [Library](library.html): The description of the library 9436* [Measure](measure.html): The description of the measure 9437* [MessageDefinition](messagedefinition.html): The description of the message definition 9438* [NamingSystem](namingsystem.html): The description of the naming system 9439* [OperationDefinition](operationdefinition.html): The description of the operation definition 9440* [PlanDefinition](plandefinition.html): The description of the plan definition 9441* [Questionnaire](questionnaire.html): The description of the questionnaire 9442* [Requirements](requirements.html): The description of the requirements 9443* [SearchParameter](searchparameter.html): The description of the search parameter 9444* [StructureDefinition](structuredefinition.html): The description of the structure definition 9445* [StructureMap](structuremap.html): The description of the structure map 9446* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 9447* [TestScript](testscript.html): The description of the test script 9448* [ValueSet](valueset.html): The description of the value set 9449</b><br> 9450 * Type: <b>string</b><br> 9451 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 9452 * </p> 9453 */ 9454 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 9455 public static final String SP_DESCRIPTION = "description"; 9456 /** 9457 * <b>Fluent Client</b> search parameter constant for <b>description</b> 9458 * <p> 9459 * Description: <b>Multiple Resources: 9460 9461* [ActivityDefinition](activitydefinition.html): The description of the activity definition 9462* [ActorDefinition](actordefinition.html): The description of the Actor Definition 9463* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 9464* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 9465* [Citation](citation.html): The description of the citation 9466* [CodeSystem](codesystem.html): The description of the code system 9467* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 9468* [ConceptMap](conceptmap.html): The description of the concept map 9469* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 9470* [EventDefinition](eventdefinition.html): The description of the event definition 9471* [Evidence](evidence.html): The description of the evidence 9472* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 9473* [GraphDefinition](graphdefinition.html): The description of the graph definition 9474* [ImplementationGuide](implementationguide.html): The description of the implementation guide 9475* [Library](library.html): The description of the library 9476* [Measure](measure.html): The description of the measure 9477* [MessageDefinition](messagedefinition.html): The description of the message definition 9478* [NamingSystem](namingsystem.html): The description of the naming system 9479* [OperationDefinition](operationdefinition.html): The description of the operation definition 9480* [PlanDefinition](plandefinition.html): The description of the plan definition 9481* [Questionnaire](questionnaire.html): The description of the questionnaire 9482* [Requirements](requirements.html): The description of the requirements 9483* [SearchParameter](searchparameter.html): The description of the search parameter 9484* [StructureDefinition](structuredefinition.html): The description of the structure definition 9485* [StructureMap](structuremap.html): The description of the structure map 9486* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 9487* [TestScript](testscript.html): The description of the test script 9488* [ValueSet](valueset.html): The description of the value set 9489</b><br> 9490 * Type: <b>string</b><br> 9491 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 9492 * </p> 9493 */ 9494 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 9495 9496 /** 9497 * Search parameter: <b>identifier</b> 9498 * <p> 9499 * Description: <b>Multiple Resources: 9500 9501* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 9502* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 9503* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 9504* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 9505* [Citation](citation.html): External identifier for the citation 9506* [CodeSystem](codesystem.html): External identifier for the code system 9507* [ConceptMap](conceptmap.html): External identifier for the concept map 9508* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 9509* [EventDefinition](eventdefinition.html): External identifier for the event definition 9510* [Evidence](evidence.html): External identifier for the evidence 9511* [EvidenceReport](evidencereport.html): External identifier for the evidence report 9512* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 9513* [ExampleScenario](examplescenario.html): External identifier for the example scenario 9514* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 9515* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 9516* [Library](library.html): External identifier for the library 9517* [Measure](measure.html): External identifier for the measure 9518* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 9519* [MessageDefinition](messagedefinition.html): External identifier for the message definition 9520* [NamingSystem](namingsystem.html): External identifier for the naming system 9521* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 9522* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 9523* [PlanDefinition](plandefinition.html): External identifier for the plan definition 9524* [Questionnaire](questionnaire.html): External identifier for the questionnaire 9525* [Requirements](requirements.html): External identifier for the requirements 9526* [SearchParameter](searchparameter.html): External identifier for the search parameter 9527* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 9528* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 9529* [StructureMap](structuremap.html): External identifier for the structure map 9530* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 9531* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 9532* [TestPlan](testplan.html): An identifier for the test plan 9533* [TestScript](testscript.html): External identifier for the test script 9534* [ValueSet](valueset.html): External identifier for the value set 9535</b><br> 9536 * Type: <b>token</b><br> 9537 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 9538 * </p> 9539 */ 9540 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 9541 public static final String SP_IDENTIFIER = "identifier"; 9542 /** 9543 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 9544 * <p> 9545 * Description: <b>Multiple Resources: 9546 9547* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 9548* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 9549* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 9550* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 9551* [Citation](citation.html): External identifier for the citation 9552* [CodeSystem](codesystem.html): External identifier for the code system 9553* [ConceptMap](conceptmap.html): External identifier for the concept map 9554* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 9555* [EventDefinition](eventdefinition.html): External identifier for the event definition 9556* [Evidence](evidence.html): External identifier for the evidence 9557* [EvidenceReport](evidencereport.html): External identifier for the evidence report 9558* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 9559* [ExampleScenario](examplescenario.html): External identifier for the example scenario 9560* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 9561* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 9562* [Library](library.html): External identifier for the library 9563* [Measure](measure.html): External identifier for the measure 9564* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 9565* [MessageDefinition](messagedefinition.html): External identifier for the message definition 9566* [NamingSystem](namingsystem.html): External identifier for the naming system 9567* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 9568* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 9569* [PlanDefinition](plandefinition.html): External identifier for the plan definition 9570* [Questionnaire](questionnaire.html): External identifier for the questionnaire 9571* [Requirements](requirements.html): External identifier for the requirements 9572* [SearchParameter](searchparameter.html): External identifier for the search parameter 9573* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 9574* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 9575* [StructureMap](structuremap.html): External identifier for the structure map 9576* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 9577* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 9578* [TestPlan](testplan.html): An identifier for the test plan 9579* [TestScript](testscript.html): External identifier for the test script 9580* [ValueSet](valueset.html): External identifier for the value set 9581</b><br> 9582 * Type: <b>token</b><br> 9583 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 9584 * </p> 9585 */ 9586 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 9587 9588 /** 9589 * Search parameter: <b>jurisdiction</b> 9590 * <p> 9591 * Description: <b>Multiple Resources: 9592 9593* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 9594* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 9595* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 9596* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 9597* [Citation](citation.html): Intended jurisdiction for the citation 9598* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 9599* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 9600* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 9601* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 9602* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 9603* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 9604* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 9605* [Library](library.html): Intended jurisdiction for the library 9606* [Measure](measure.html): Intended jurisdiction for the measure 9607* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 9608* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 9609* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 9610* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 9611* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 9612* [Requirements](requirements.html): Intended jurisdiction for the requirements 9613* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 9614* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 9615* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 9616* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 9617* [TestScript](testscript.html): Intended jurisdiction for the test script 9618* [ValueSet](valueset.html): Intended jurisdiction for the value set 9619</b><br> 9620 * Type: <b>token</b><br> 9621 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 9622 * </p> 9623 */ 9624 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 9625 public static final String SP_JURISDICTION = "jurisdiction"; 9626 /** 9627 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 9628 * <p> 9629 * Description: <b>Multiple Resources: 9630 9631* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 9632* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 9633* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 9634* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 9635* [Citation](citation.html): Intended jurisdiction for the citation 9636* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 9637* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 9638* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 9639* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 9640* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 9641* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 9642* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 9643* [Library](library.html): Intended jurisdiction for the library 9644* [Measure](measure.html): Intended jurisdiction for the measure 9645* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 9646* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 9647* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 9648* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 9649* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 9650* [Requirements](requirements.html): Intended jurisdiction for the requirements 9651* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 9652* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 9653* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 9654* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 9655* [TestScript](testscript.html): Intended jurisdiction for the test script 9656* [ValueSet](valueset.html): Intended jurisdiction for the value set 9657</b><br> 9658 * Type: <b>token</b><br> 9659 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 9660 * </p> 9661 */ 9662 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 9663 9664 /** 9665 * Search parameter: <b>name</b> 9666 * <p> 9667 * Description: <b>Multiple Resources: 9668 9669* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 9670* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 9671* [Citation](citation.html): Computationally friendly name of the citation 9672* [CodeSystem](codesystem.html): Computationally friendly name of the code system 9673* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 9674* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 9675* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 9676* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 9677* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 9678* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 9679* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 9680* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 9681* [Library](library.html): Computationally friendly name of the library 9682* [Measure](measure.html): Computationally friendly name of the measure 9683* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 9684* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 9685* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 9686* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 9687* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 9688* [Requirements](requirements.html): Computationally friendly name of the requirements 9689* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 9690* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 9691* [StructureMap](structuremap.html): Computationally friendly name of the structure map 9692* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 9693* [TestScript](testscript.html): Computationally friendly name of the test script 9694* [ValueSet](valueset.html): Computationally friendly name of the value set 9695</b><br> 9696 * Type: <b>string</b><br> 9697 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 9698 * </p> 9699 */ 9700 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 9701 public static final String SP_NAME = "name"; 9702 /** 9703 * <b>Fluent Client</b> search parameter constant for <b>name</b> 9704 * <p> 9705 * Description: <b>Multiple Resources: 9706 9707* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 9708* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 9709* [Citation](citation.html): Computationally friendly name of the citation 9710* [CodeSystem](codesystem.html): Computationally friendly name of the code system 9711* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 9712* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 9713* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 9714* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 9715* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 9716* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 9717* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 9718* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 9719* [Library](library.html): Computationally friendly name of the library 9720* [Measure](measure.html): Computationally friendly name of the measure 9721* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 9722* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 9723* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 9724* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 9725* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 9726* [Requirements](requirements.html): Computationally friendly name of the requirements 9727* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 9728* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 9729* [StructureMap](structuremap.html): Computationally friendly name of the structure map 9730* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 9731* [TestScript](testscript.html): Computationally friendly name of the test script 9732* [ValueSet](valueset.html): Computationally friendly name of the value set 9733</b><br> 9734 * Type: <b>string</b><br> 9735 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 9736 * </p> 9737 */ 9738 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 9739 9740 /** 9741 * Search parameter: <b>publisher</b> 9742 * <p> 9743 * Description: <b>Multiple Resources: 9744 9745* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 9746* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 9747* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 9748* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 9749* [Citation](citation.html): Name of the publisher of the citation 9750* [CodeSystem](codesystem.html): Name of the publisher of the code system 9751* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 9752* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 9753* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 9754* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 9755* [Evidence](evidence.html): Name of the publisher of the evidence 9756* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 9757* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 9758* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 9759* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 9760* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 9761* [Library](library.html): Name of the publisher of the library 9762* [Measure](measure.html): Name of the publisher of the measure 9763* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 9764* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 9765* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 9766* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 9767* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 9768* [Requirements](requirements.html): Name of the publisher of the requirements 9769* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 9770* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 9771* [StructureMap](structuremap.html): Name of the publisher of the structure map 9772* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 9773* [TestScript](testscript.html): Name of the publisher of the test script 9774* [ValueSet](valueset.html): Name of the publisher of the value set 9775</b><br> 9776 * Type: <b>string</b><br> 9777 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 9778 * </p> 9779 */ 9780 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 9781 public static final String SP_PUBLISHER = "publisher"; 9782 /** 9783 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 9784 * <p> 9785 * Description: <b>Multiple Resources: 9786 9787* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 9788* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 9789* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 9790* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 9791* [Citation](citation.html): Name of the publisher of the citation 9792* [CodeSystem](codesystem.html): Name of the publisher of the code system 9793* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 9794* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 9795* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 9796* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 9797* [Evidence](evidence.html): Name of the publisher of the evidence 9798* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 9799* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 9800* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 9801* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 9802* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 9803* [Library](library.html): Name of the publisher of the library 9804* [Measure](measure.html): Name of the publisher of the measure 9805* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 9806* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 9807* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 9808* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 9809* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 9810* [Requirements](requirements.html): Name of the publisher of the requirements 9811* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 9812* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 9813* [StructureMap](structuremap.html): Name of the publisher of the structure map 9814* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 9815* [TestScript](testscript.html): Name of the publisher of the test script 9816* [ValueSet](valueset.html): Name of the publisher of the value set 9817</b><br> 9818 * Type: <b>string</b><br> 9819 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 9820 * </p> 9821 */ 9822 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 9823 9824 /** 9825 * Search parameter: <b>status</b> 9826 * <p> 9827 * Description: <b>Multiple Resources: 9828 9829* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 9830* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 9831* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 9832* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 9833* [Citation](citation.html): The current status of the citation 9834* [CodeSystem](codesystem.html): The current status of the code system 9835* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 9836* [ConceptMap](conceptmap.html): The current status of the concept map 9837* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 9838* [EventDefinition](eventdefinition.html): The current status of the event definition 9839* [Evidence](evidence.html): The current status of the evidence 9840* [EvidenceReport](evidencereport.html): The current status of the evidence report 9841* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 9842* [ExampleScenario](examplescenario.html): The current status of the example scenario 9843* [GraphDefinition](graphdefinition.html): The current status of the graph definition 9844* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 9845* [Library](library.html): The current status of the library 9846* [Measure](measure.html): The current status of the measure 9847* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 9848* [MessageDefinition](messagedefinition.html): The current status of the message definition 9849* [NamingSystem](namingsystem.html): The current status of the naming system 9850* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 9851* [OperationDefinition](operationdefinition.html): The current status of the operation definition 9852* [PlanDefinition](plandefinition.html): The current status of the plan definition 9853* [Questionnaire](questionnaire.html): The current status of the questionnaire 9854* [Requirements](requirements.html): The current status of the requirements 9855* [SearchParameter](searchparameter.html): The current status of the search parameter 9856* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 9857* [StructureDefinition](structuredefinition.html): The current status of the structure definition 9858* [StructureMap](structuremap.html): The current status of the structure map 9859* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 9860* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 9861* [TestPlan](testplan.html): The current status of the test plan 9862* [TestScript](testscript.html): The current status of the test script 9863* [ValueSet](valueset.html): The current status of the value set 9864</b><br> 9865 * Type: <b>token</b><br> 9866 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 9867 * </p> 9868 */ 9869 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 9870 public static final String SP_STATUS = "status"; 9871 /** 9872 * <b>Fluent Client</b> search parameter constant for <b>status</b> 9873 * <p> 9874 * Description: <b>Multiple Resources: 9875 9876* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 9877* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 9878* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 9879* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 9880* [Citation](citation.html): The current status of the citation 9881* [CodeSystem](codesystem.html): The current status of the code system 9882* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 9883* [ConceptMap](conceptmap.html): The current status of the concept map 9884* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 9885* [EventDefinition](eventdefinition.html): The current status of the event definition 9886* [Evidence](evidence.html): The current status of the evidence 9887* [EvidenceReport](evidencereport.html): The current status of the evidence report 9888* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 9889* [ExampleScenario](examplescenario.html): The current status of the example scenario 9890* [GraphDefinition](graphdefinition.html): The current status of the graph definition 9891* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 9892* [Library](library.html): The current status of the library 9893* [Measure](measure.html): The current status of the measure 9894* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 9895* [MessageDefinition](messagedefinition.html): The current status of the message definition 9896* [NamingSystem](namingsystem.html): The current status of the naming system 9897* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 9898* [OperationDefinition](operationdefinition.html): The current status of the operation definition 9899* [PlanDefinition](plandefinition.html): The current status of the plan definition 9900* [Questionnaire](questionnaire.html): The current status of the questionnaire 9901* [Requirements](requirements.html): The current status of the requirements 9902* [SearchParameter](searchparameter.html): The current status of the search parameter 9903* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 9904* [StructureDefinition](structuredefinition.html): The current status of the structure definition 9905* [StructureMap](structuremap.html): The current status of the structure map 9906* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 9907* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 9908* [TestPlan](testplan.html): The current status of the test plan 9909* [TestScript](testscript.html): The current status of the test script 9910* [ValueSet](valueset.html): The current status of the value set 9911</b><br> 9912 * Type: <b>token</b><br> 9913 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 9914 * </p> 9915 */ 9916 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 9917 9918 /** 9919 * Search parameter: <b>title</b> 9920 * <p> 9921 * Description: <b>Multiple Resources: 9922 9923* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 9924* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 9925* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 9926* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 9927* [Citation](citation.html): The human-friendly name of the citation 9928* [CodeSystem](codesystem.html): The human-friendly name of the code system 9929* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 9930* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 9931* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 9932* [Evidence](evidence.html): The human-friendly name of the evidence 9933* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 9934* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 9935* [Library](library.html): The human-friendly name of the library 9936* [Measure](measure.html): The human-friendly name of the measure 9937* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 9938* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 9939* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 9940* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 9941* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 9942* [Requirements](requirements.html): The human-friendly name of the requirements 9943* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 9944* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 9945* [StructureMap](structuremap.html): The human-friendly name of the structure map 9946* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 9947* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 9948* [TestScript](testscript.html): The human-friendly name of the test script 9949* [ValueSet](valueset.html): The human-friendly name of the value set 9950</b><br> 9951 * Type: <b>string</b><br> 9952 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 9953 * </p> 9954 */ 9955 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 9956 public static final String SP_TITLE = "title"; 9957 /** 9958 * <b>Fluent Client</b> search parameter constant for <b>title</b> 9959 * <p> 9960 * Description: <b>Multiple Resources: 9961 9962* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 9963* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 9964* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 9965* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 9966* [Citation](citation.html): The human-friendly name of the citation 9967* [CodeSystem](codesystem.html): The human-friendly name of the code system 9968* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 9969* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 9970* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 9971* [Evidence](evidence.html): The human-friendly name of the evidence 9972* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 9973* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 9974* [Library](library.html): The human-friendly name of the library 9975* [Measure](measure.html): The human-friendly name of the measure 9976* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 9977* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 9978* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 9979* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 9980* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 9981* [Requirements](requirements.html): The human-friendly name of the requirements 9982* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 9983* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 9984* [StructureMap](structuremap.html): The human-friendly name of the structure map 9985* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 9986* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 9987* [TestScript](testscript.html): The human-friendly name of the test script 9988* [ValueSet](valueset.html): The human-friendly name of the value set 9989</b><br> 9990 * Type: <b>string</b><br> 9991 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 9992 * </p> 9993 */ 9994 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 9995 9996 /** 9997 * Search parameter: <b>url</b> 9998 * <p> 9999 * Description: <b>Multiple Resources: 10000 10001* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 10002* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 10003* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 10004* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 10005* [Citation](citation.html): The uri that identifies the citation 10006* [CodeSystem](codesystem.html): The uri that identifies the code system 10007* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 10008* [ConceptMap](conceptmap.html): The URI that identifies the concept map 10009* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 10010* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 10011* [Evidence](evidence.html): The uri that identifies the evidence 10012* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 10013* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 10014* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 10015* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 10016* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 10017* [Library](library.html): The uri that identifies the library 10018* [Measure](measure.html): The uri that identifies the measure 10019* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 10020* [NamingSystem](namingsystem.html): The uri that identifies the naming system 10021* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 10022* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 10023* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 10024* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 10025* [Requirements](requirements.html): The uri that identifies the requirements 10026* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 10027* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 10028* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 10029* [StructureMap](structuremap.html): The uri that identifies the structure map 10030* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 10031* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 10032* [TestPlan](testplan.html): The uri that identifies the test plan 10033* [TestScript](testscript.html): The uri that identifies the test script 10034* [ValueSet](valueset.html): The uri that identifies the value set 10035</b><br> 10036 * Type: <b>uri</b><br> 10037 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 10038 * </p> 10039 */ 10040 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 10041 public static final String SP_URL = "url"; 10042 /** 10043 * <b>Fluent Client</b> search parameter constant for <b>url</b> 10044 * <p> 10045 * Description: <b>Multiple Resources: 10046 10047* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 10048* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 10049* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 10050* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 10051* [Citation](citation.html): The uri that identifies the citation 10052* [CodeSystem](codesystem.html): The uri that identifies the code system 10053* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 10054* [ConceptMap](conceptmap.html): The URI that identifies the concept map 10055* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 10056* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 10057* [Evidence](evidence.html): The uri that identifies the evidence 10058* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 10059* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 10060* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 10061* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 10062* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 10063* [Library](library.html): The uri that identifies the library 10064* [Measure](measure.html): The uri that identifies the measure 10065* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 10066* [NamingSystem](namingsystem.html): The uri that identifies the naming system 10067* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 10068* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 10069* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 10070* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 10071* [Requirements](requirements.html): The uri that identifies the requirements 10072* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 10073* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 10074* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 10075* [StructureMap](structuremap.html): The uri that identifies the structure map 10076* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 10077* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 10078* [TestPlan](testplan.html): The uri that identifies the test plan 10079* [TestScript](testscript.html): The uri that identifies the test script 10080* [ValueSet](valueset.html): The uri that identifies the value set 10081</b><br> 10082 * Type: <b>uri</b><br> 10083 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 10084 * </p> 10085 */ 10086 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 10087 10088 /** 10089 * Search parameter: <b>version</b> 10090 * <p> 10091 * Description: <b>Multiple Resources: 10092 10093* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 10094* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 10095* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 10096* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 10097* [Citation](citation.html): The business version of the citation 10098* [CodeSystem](codesystem.html): The business version of the code system 10099* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 10100* [ConceptMap](conceptmap.html): The business version of the concept map 10101* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 10102* [EventDefinition](eventdefinition.html): The business version of the event definition 10103* [Evidence](evidence.html): The business version of the evidence 10104* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 10105* [ExampleScenario](examplescenario.html): The business version of the example scenario 10106* [GraphDefinition](graphdefinition.html): The business version of the graph definition 10107* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 10108* [Library](library.html): The business version of the library 10109* [Measure](measure.html): The business version of the measure 10110* [MessageDefinition](messagedefinition.html): The business version of the message definition 10111* [NamingSystem](namingsystem.html): The business version of the naming system 10112* [OperationDefinition](operationdefinition.html): The business version of the operation definition 10113* [PlanDefinition](plandefinition.html): The business version of the plan definition 10114* [Questionnaire](questionnaire.html): The business version of the questionnaire 10115* [Requirements](requirements.html): The business version of the requirements 10116* [SearchParameter](searchparameter.html): The business version of the search parameter 10117* [StructureDefinition](structuredefinition.html): The business version of the structure definition 10118* [StructureMap](structuremap.html): The business version of the structure map 10119* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 10120* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 10121* [TestScript](testscript.html): The business version of the test script 10122* [ValueSet](valueset.html): The business version of the value set 10123</b><br> 10124 * Type: <b>token</b><br> 10125 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 10126 * </p> 10127 */ 10128 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 10129 public static final String SP_VERSION = "version"; 10130 /** 10131 * <b>Fluent Client</b> search parameter constant for <b>version</b> 10132 * <p> 10133 * Description: <b>Multiple Resources: 10134 10135* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 10136* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 10137* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 10138* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 10139* [Citation](citation.html): The business version of the citation 10140* [CodeSystem](codesystem.html): The business version of the code system 10141* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 10142* [ConceptMap](conceptmap.html): The business version of the concept map 10143* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 10144* [EventDefinition](eventdefinition.html): The business version of the event definition 10145* [Evidence](evidence.html): The business version of the evidence 10146* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 10147* [ExampleScenario](examplescenario.html): The business version of the example scenario 10148* [GraphDefinition](graphdefinition.html): The business version of the graph definition 10149* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 10150* [Library](library.html): The business version of the library 10151* [Measure](measure.html): The business version of the measure 10152* [MessageDefinition](messagedefinition.html): The business version of the message definition 10153* [NamingSystem](namingsystem.html): The business version of the naming system 10154* [OperationDefinition](operationdefinition.html): The business version of the operation definition 10155* [PlanDefinition](plandefinition.html): The business version of the plan definition 10156* [Questionnaire](questionnaire.html): The business version of the questionnaire 10157* [Requirements](requirements.html): The business version of the requirements 10158* [SearchParameter](searchparameter.html): The business version of the search parameter 10159* [StructureDefinition](structuredefinition.html): The business version of the structure definition 10160* [StructureMap](structuremap.html): The business version of the structure map 10161* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 10162* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 10163* [TestScript](testscript.html): The business version of the test script 10164* [ValueSet](valueset.html): The business version of the value set 10165</b><br> 10166 * Type: <b>token</b><br> 10167 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 10168 * </p> 10169 */ 10170 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 10171 10172 /** 10173 * Search parameter: <b>composed-of</b> 10174 * <p> 10175 * Description: <b>Multiple Resources: 10176 10177* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10178* [EventDefinition](eventdefinition.html): What resource is being referenced 10179* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10180* [Library](library.html): What resource is being referenced 10181* [Measure](measure.html): What resource is being referenced 10182* [PlanDefinition](plandefinition.html): What resource is being referenced 10183</b><br> 10184 * Type: <b>reference</b><br> 10185 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 10186 * </p> 10187 */ 10188 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 10189 public static final String SP_COMPOSED_OF = "composed-of"; 10190 /** 10191 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 10192 * <p> 10193 * Description: <b>Multiple Resources: 10194 10195* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10196* [EventDefinition](eventdefinition.html): What resource is being referenced 10197* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10198* [Library](library.html): What resource is being referenced 10199* [Measure](measure.html): What resource is being referenced 10200* [PlanDefinition](plandefinition.html): What resource is being referenced 10201</b><br> 10202 * Type: <b>reference</b><br> 10203 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 10204 * </p> 10205 */ 10206 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 10207 10208/** 10209 * Constant for fluent queries to be used to add include statements. Specifies 10210 * the path value of "<b>PlanDefinition:composed-of</b>". 10211 */ 10212 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("PlanDefinition:composed-of").toLocked(); 10213 10214 /** 10215 * Search parameter: <b>depends-on</b> 10216 * <p> 10217 * Description: <b>Multiple Resources: 10218 10219* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10220* [EventDefinition](eventdefinition.html): What resource is being referenced 10221* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10222* [Library](library.html): What resource is being referenced 10223* [Measure](measure.html): What resource is being referenced 10224* [PlanDefinition](plandefinition.html): What resource is being referenced 10225</b><br> 10226 * Type: <b>reference</b><br> 10227 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 10228 * </p> 10229 */ 10230 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 10231 public static final String SP_DEPENDS_ON = "depends-on"; 10232 /** 10233 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 10234 * <p> 10235 * Description: <b>Multiple Resources: 10236 10237* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10238* [EventDefinition](eventdefinition.html): What resource is being referenced 10239* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10240* [Library](library.html): What resource is being referenced 10241* [Measure](measure.html): What resource is being referenced 10242* [PlanDefinition](plandefinition.html): What resource is being referenced 10243</b><br> 10244 * Type: <b>reference</b><br> 10245 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 10246 * </p> 10247 */ 10248 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 10249 10250/** 10251 * Constant for fluent queries to be used to add include statements. Specifies 10252 * the path value of "<b>PlanDefinition:depends-on</b>". 10253 */ 10254 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("PlanDefinition:depends-on").toLocked(); 10255 10256 /** 10257 * Search parameter: <b>derived-from</b> 10258 * <p> 10259 * Description: <b>Multiple Resources: 10260 10261* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10262* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 10263* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 10264* [EventDefinition](eventdefinition.html): What resource is being referenced 10265* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10266* [Library](library.html): What resource is being referenced 10267* [Measure](measure.html): What resource is being referenced 10268* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 10269* [PlanDefinition](plandefinition.html): What resource is being referenced 10270* [ValueSet](valueset.html): A resource that the ValueSet is derived from 10271</b><br> 10272 * Type: <b>reference</b><br> 10273 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 10274 * </p> 10275 */ 10276 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 10277 public static final String SP_DERIVED_FROM = "derived-from"; 10278 /** 10279 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 10280 * <p> 10281 * Description: <b>Multiple Resources: 10282 10283* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10284* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 10285* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 10286* [EventDefinition](eventdefinition.html): What resource is being referenced 10287* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10288* [Library](library.html): What resource is being referenced 10289* [Measure](measure.html): What resource is being referenced 10290* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 10291* [PlanDefinition](plandefinition.html): What resource is being referenced 10292* [ValueSet](valueset.html): A resource that the ValueSet is derived from 10293</b><br> 10294 * Type: <b>reference</b><br> 10295 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 10296 * </p> 10297 */ 10298 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 10299 10300/** 10301 * Constant for fluent queries to be used to add include statements. Specifies 10302 * the path value of "<b>PlanDefinition:derived-from</b>". 10303 */ 10304 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("PlanDefinition:derived-from").toLocked(); 10305 10306 /** 10307 * Search parameter: <b>effective</b> 10308 * <p> 10309 * Description: <b>Multiple Resources: 10310 10311* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 10312* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 10313* [Citation](citation.html): The time during which the citation is intended to be in use 10314* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 10315* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 10316* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 10317* [Library](library.html): The time during which the library is intended to be in use 10318* [Measure](measure.html): The time during which the measure is intended to be in use 10319* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 10320* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 10321* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 10322* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 10323</b><br> 10324 * Type: <b>date</b><br> 10325 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 10326 * </p> 10327 */ 10328 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 10329 public static final String SP_EFFECTIVE = "effective"; 10330 /** 10331 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 10332 * <p> 10333 * Description: <b>Multiple Resources: 10334 10335* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 10336* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 10337* [Citation](citation.html): The time during which the citation is intended to be in use 10338* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 10339* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 10340* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 10341* [Library](library.html): The time during which the library is intended to be in use 10342* [Measure](measure.html): The time during which the measure is intended to be in use 10343* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 10344* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 10345* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 10346* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 10347</b><br> 10348 * Type: <b>date</b><br> 10349 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 10350 * </p> 10351 */ 10352 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 10353 10354 /** 10355 * Search parameter: <b>predecessor</b> 10356 * <p> 10357 * Description: <b>Multiple Resources: 10358 10359* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10360* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 10361* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 10362* [EventDefinition](eventdefinition.html): What resource is being referenced 10363* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10364* [Library](library.html): What resource is being referenced 10365* [Measure](measure.html): What resource is being referenced 10366* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 10367* [PlanDefinition](plandefinition.html): What resource is being referenced 10368* [ValueSet](valueset.html): The predecessor of the ValueSet 10369</b><br> 10370 * Type: <b>reference</b><br> 10371 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 10372 * </p> 10373 */ 10374 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 10375 public static final String SP_PREDECESSOR = "predecessor"; 10376 /** 10377 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 10378 * <p> 10379 * Description: <b>Multiple Resources: 10380 10381* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10382* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 10383* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 10384* [EventDefinition](eventdefinition.html): What resource is being referenced 10385* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10386* [Library](library.html): What resource is being referenced 10387* [Measure](measure.html): What resource is being referenced 10388* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 10389* [PlanDefinition](plandefinition.html): What resource is being referenced 10390* [ValueSet](valueset.html): The predecessor of the ValueSet 10391</b><br> 10392 * Type: <b>reference</b><br> 10393 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 10394 * </p> 10395 */ 10396 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 10397 10398/** 10399 * Constant for fluent queries to be used to add include statements. Specifies 10400 * the path value of "<b>PlanDefinition:predecessor</b>". 10401 */ 10402 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("PlanDefinition:predecessor").toLocked(); 10403 10404 /** 10405 * Search parameter: <b>successor</b> 10406 * <p> 10407 * Description: <b>Multiple Resources: 10408 10409* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10410* [EventDefinition](eventdefinition.html): What resource is being referenced 10411* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10412* [Library](library.html): What resource is being referenced 10413* [Measure](measure.html): What resource is being referenced 10414* [PlanDefinition](plandefinition.html): What resource is being referenced 10415</b><br> 10416 * Type: <b>reference</b><br> 10417 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 10418 * </p> 10419 */ 10420 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 10421 public static final String SP_SUCCESSOR = "successor"; 10422 /** 10423 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 10424 * <p> 10425 * Description: <b>Multiple Resources: 10426 10427* [ActivityDefinition](activitydefinition.html): What resource is being referenced 10428* [EventDefinition](eventdefinition.html): What resource is being referenced 10429* [EvidenceVariable](evidencevariable.html): What resource is being referenced 10430* [Library](library.html): What resource is being referenced 10431* [Measure](measure.html): What resource is being referenced 10432* [PlanDefinition](plandefinition.html): What resource is being referenced 10433</b><br> 10434 * Type: <b>reference</b><br> 10435 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 10436 * </p> 10437 */ 10438 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 10439 10440/** 10441 * Constant for fluent queries to be used to add include statements. Specifies 10442 * the path value of "<b>PlanDefinition:successor</b>". 10443 */ 10444 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("PlanDefinition:successor").toLocked(); 10445 10446 /** 10447 * Search parameter: <b>topic</b> 10448 * <p> 10449 * Description: <b>Multiple Resources: 10450 10451* [ActivityDefinition](activitydefinition.html): Topics associated with the module 10452* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 10453* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 10454* [EventDefinition](eventdefinition.html): Topics associated with the module 10455* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 10456* [Library](library.html): Topics associated with the module 10457* [Measure](measure.html): Topics associated with the measure 10458* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 10459* [PlanDefinition](plandefinition.html): Topics associated with the module 10460* [ValueSet](valueset.html): Topics associated with the ValueSet 10461</b><br> 10462 * Type: <b>token</b><br> 10463 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 10464 * </p> 10465 */ 10466 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 10467 public static final String SP_TOPIC = "topic"; 10468 /** 10469 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 10470 * <p> 10471 * Description: <b>Multiple Resources: 10472 10473* [ActivityDefinition](activitydefinition.html): Topics associated with the module 10474* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 10475* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 10476* [EventDefinition](eventdefinition.html): Topics associated with the module 10477* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 10478* [Library](library.html): Topics associated with the module 10479* [Measure](measure.html): Topics associated with the measure 10480* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 10481* [PlanDefinition](plandefinition.html): Topics associated with the module 10482* [ValueSet](valueset.html): Topics associated with the ValueSet 10483</b><br> 10484 * Type: <b>token</b><br> 10485 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 10486 * </p> 10487 */ 10488 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 10489 10490 /** 10491 * Search parameter: <b>definition</b> 10492 * <p> 10493 * Description: <b>Activity or plan definitions used by plan definition</b><br> 10494 * Type: <b>reference</b><br> 10495 * Path: <b>PlanDefinition.action.definition.ofType(canonical) | PlanDefinition.action.definition.ofType(uri)</b><br> 10496 * </p> 10497 */ 10498 @SearchParamDefinition(name="definition", path="PlanDefinition.action.definition.ofType(canonical) | PlanDefinition.action.definition.ofType(uri)", description="Activity or plan definitions used by plan definition", type="reference", target={ActivityDefinition.class, MessageDefinition.class, ObservationDefinition.class, PlanDefinition.class, Questionnaire.class, SpecimenDefinition.class } ) 10499 public static final String SP_DEFINITION = "definition"; 10500 /** 10501 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 10502 * <p> 10503 * Description: <b>Activity or plan definitions used by plan definition</b><br> 10504 * Type: <b>reference</b><br> 10505 * Path: <b>PlanDefinition.action.definition.ofType(canonical) | PlanDefinition.action.definition.ofType(uri)</b><br> 10506 * </p> 10507 */ 10508 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 10509 10510/** 10511 * Constant for fluent queries to be used to add include statements. Specifies 10512 * the path value of "<b>PlanDefinition:definition</b>". 10513 */ 10514 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("PlanDefinition:definition").toLocked(); 10515 10516 /** 10517 * Search parameter: <b>type</b> 10518 * <p> 10519 * Description: <b>The type of artifact the plan (e.g. order-set, eca-rule, protocol)</b><br> 10520 * Type: <b>token</b><br> 10521 * Path: <b>PlanDefinition.type</b><br> 10522 * </p> 10523 */ 10524 @SearchParamDefinition(name="type", path="PlanDefinition.type", description="The type of artifact the plan (e.g. order-set, eca-rule, protocol)", type="token" ) 10525 public static final String SP_TYPE = "type"; 10526 /** 10527 * <b>Fluent Client</b> search parameter constant for <b>type</b> 10528 * <p> 10529 * Description: <b>The type of artifact the plan (e.g. order-set, eca-rule, protocol)</b><br> 10530 * Type: <b>token</b><br> 10531 * Path: <b>PlanDefinition.type</b><br> 10532 * </p> 10533 */ 10534 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 10535 10536 10537} 10538