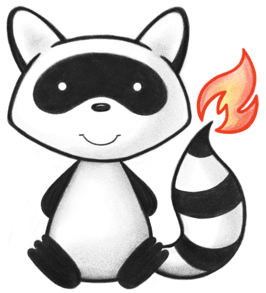
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import ca.uhn.fhir.model.api.annotation.DatatypeDef; 035 036/** 037 * Primitive type "integer" in FHIR: A signed 32-bit integer 038 */ 039@DatatypeDef(name = "positiveInt", profileOf=IntegerType.class) 040public class PositiveIntType extends IntegerType { 041 042 /** 043 * 044 */ 045 private static final long serialVersionUID = 1686497884249402429L; 046 047 /** 048 * Constructor 049 */ 050 public PositiveIntType() { 051 // nothing 052 } 053 054 /** 055 * Constructor 056 */ 057 public PositiveIntType(int theInteger) { 058 setValue(theInteger); 059 } 060 061 /** 062 * Constructor 063 * 064 * @param theIntegerAsString 065 * A string representation of an integer 066 * @throws IllegalArgumentException 067 * If the string is not a valid integer representation 068 */ 069 public PositiveIntType(String theIntegerAsString) { 070 setValueAsString(theIntegerAsString); 071 } 072 073 /** 074 * Constructor 075 * 076 * @param theValue The value 077 * @throws IllegalArgumentException If the value is too large to fit in a signed integer 078 */ 079 public PositiveIntType(Long theValue) { 080 if (theValue < 1 || theValue > java.lang.Integer.MAX_VALUE) { 081 throw new IllegalArgumentException 082 (theValue + " cannot be cast to int without changing its value."); 083 } 084 if(theValue!=null) { 085 setValue((int)theValue.longValue()); 086 } 087 } 088 089 @Override 090 public PositiveIntType copy() { 091 PositiveIntType ret = getValue() == null ? new PositiveIntType() : new PositiveIntType(getValue()); 092 copyValues(ret); 093 return ret; 094 } 095 096 public String fhirType() { 097 return "positiveInt"; 098 } 099}