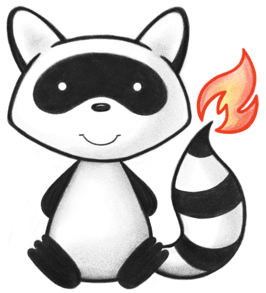
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A person who is directly or indirectly involved in the provisioning of healthcare or related services. 052 */ 053@ResourceDef(name="Practitioner", profile="http://hl7.org/fhir/StructureDefinition/Practitioner") 054public class Practitioner extends DomainResource { 055 056 @Block() 057 public static class PractitionerQualificationComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * An identifier that applies to this person's qualification. 060 */ 061 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 062 @Description(shortDefinition="An identifier for this qualification for the practitioner", formalDefinition="An identifier that applies to this person's qualification." ) 063 protected List<Identifier> identifier; 064 065 /** 066 * Coded representation of the qualification. 067 */ 068 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="Coded representation of the qualification", formalDefinition="Coded representation of the qualification." ) 070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0360") 071 protected CodeableConcept code; 072 073 /** 074 * Period during which the qualification is valid. 075 */ 076 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 077 @Description(shortDefinition="Period during which the qualification is valid", formalDefinition="Period during which the qualification is valid." ) 078 protected Period period; 079 080 /** 081 * Organization that regulates and issues the qualification. 082 */ 083 @Child(name = "issuer", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 084 @Description(shortDefinition="Organization that regulates and issues the qualification", formalDefinition="Organization that regulates and issues the qualification." ) 085 protected Reference issuer; 086 087 private static final long serialVersionUID = 1561812204L; 088 089 /** 090 * Constructor 091 */ 092 public PractitionerQualificationComponent() { 093 super(); 094 } 095 096 /** 097 * Constructor 098 */ 099 public PractitionerQualificationComponent(CodeableConcept code) { 100 super(); 101 this.setCode(code); 102 } 103 104 /** 105 * @return {@link #identifier} (An identifier that applies to this person's qualification.) 106 */ 107 public List<Identifier> getIdentifier() { 108 if (this.identifier == null) 109 this.identifier = new ArrayList<Identifier>(); 110 return this.identifier; 111 } 112 113 /** 114 * @return Returns a reference to <code>this</code> for easy method chaining 115 */ 116 public PractitionerQualificationComponent setIdentifier(List<Identifier> theIdentifier) { 117 this.identifier = theIdentifier; 118 return this; 119 } 120 121 public boolean hasIdentifier() { 122 if (this.identifier == null) 123 return false; 124 for (Identifier item : this.identifier) 125 if (!item.isEmpty()) 126 return true; 127 return false; 128 } 129 130 public Identifier addIdentifier() { //3 131 Identifier t = new Identifier(); 132 if (this.identifier == null) 133 this.identifier = new ArrayList<Identifier>(); 134 this.identifier.add(t); 135 return t; 136 } 137 138 public PractitionerQualificationComponent addIdentifier(Identifier t) { //3 139 if (t == null) 140 return this; 141 if (this.identifier == null) 142 this.identifier = new ArrayList<Identifier>(); 143 this.identifier.add(t); 144 return this; 145 } 146 147 /** 148 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 149 */ 150 public Identifier getIdentifierFirstRep() { 151 if (getIdentifier().isEmpty()) { 152 addIdentifier(); 153 } 154 return getIdentifier().get(0); 155 } 156 157 /** 158 * @return {@link #code} (Coded representation of the qualification.) 159 */ 160 public CodeableConcept getCode() { 161 if (this.code == null) 162 if (Configuration.errorOnAutoCreate()) 163 throw new Error("Attempt to auto-create PractitionerQualificationComponent.code"); 164 else if (Configuration.doAutoCreate()) 165 this.code = new CodeableConcept(); // cc 166 return this.code; 167 } 168 169 public boolean hasCode() { 170 return this.code != null && !this.code.isEmpty(); 171 } 172 173 /** 174 * @param value {@link #code} (Coded representation of the qualification.) 175 */ 176 public PractitionerQualificationComponent setCode(CodeableConcept value) { 177 this.code = value; 178 return this; 179 } 180 181 /** 182 * @return {@link #period} (Period during which the qualification is valid.) 183 */ 184 public Period getPeriod() { 185 if (this.period == null) 186 if (Configuration.errorOnAutoCreate()) 187 throw new Error("Attempt to auto-create PractitionerQualificationComponent.period"); 188 else if (Configuration.doAutoCreate()) 189 this.period = new Period(); // cc 190 return this.period; 191 } 192 193 public boolean hasPeriod() { 194 return this.period != null && !this.period.isEmpty(); 195 } 196 197 /** 198 * @param value {@link #period} (Period during which the qualification is valid.) 199 */ 200 public PractitionerQualificationComponent setPeriod(Period value) { 201 this.period = value; 202 return this; 203 } 204 205 /** 206 * @return {@link #issuer} (Organization that regulates and issues the qualification.) 207 */ 208 public Reference getIssuer() { 209 if (this.issuer == null) 210 if (Configuration.errorOnAutoCreate()) 211 throw new Error("Attempt to auto-create PractitionerQualificationComponent.issuer"); 212 else if (Configuration.doAutoCreate()) 213 this.issuer = new Reference(); // cc 214 return this.issuer; 215 } 216 217 public boolean hasIssuer() { 218 return this.issuer != null && !this.issuer.isEmpty(); 219 } 220 221 /** 222 * @param value {@link #issuer} (Organization that regulates and issues the qualification.) 223 */ 224 public PractitionerQualificationComponent setIssuer(Reference value) { 225 this.issuer = value; 226 return this; 227 } 228 229 protected void listChildren(List<Property> children) { 230 super.listChildren(children); 231 children.add(new Property("identifier", "Identifier", "An identifier that applies to this person's qualification.", 0, java.lang.Integer.MAX_VALUE, identifier)); 232 children.add(new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, code)); 233 children.add(new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, period)); 234 children.add(new Property("issuer", "Reference(Organization)", "Organization that regulates and issues the qualification.", 0, 1, issuer)); 235 } 236 237 @Override 238 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 239 switch (_hash) { 240 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier that applies to this person's qualification.", 0, java.lang.Integer.MAX_VALUE, identifier); 241 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, code); 242 case -991726143: /*period*/ return new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, period); 243 case -1179159879: /*issuer*/ return new Property("issuer", "Reference(Organization)", "Organization that regulates and issues the qualification.", 0, 1, issuer); 244 default: return super.getNamedProperty(_hash, _name, _checkValid); 245 } 246 247 } 248 249 @Override 250 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 251 switch (hash) { 252 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 253 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 254 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 255 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // Reference 256 default: return super.getProperty(hash, name, checkValid); 257 } 258 259 } 260 261 @Override 262 public Base setProperty(int hash, String name, Base value) throws FHIRException { 263 switch (hash) { 264 case -1618432855: // identifier 265 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 266 return value; 267 case 3059181: // code 268 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 269 return value; 270 case -991726143: // period 271 this.period = TypeConvertor.castToPeriod(value); // Period 272 return value; 273 case -1179159879: // issuer 274 this.issuer = TypeConvertor.castToReference(value); // Reference 275 return value; 276 default: return super.setProperty(hash, name, value); 277 } 278 279 } 280 281 @Override 282 public Base setProperty(String name, Base value) throws FHIRException { 283 if (name.equals("identifier")) { 284 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 285 } else if (name.equals("code")) { 286 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 287 } else if (name.equals("period")) { 288 this.period = TypeConvertor.castToPeriod(value); // Period 289 } else if (name.equals("issuer")) { 290 this.issuer = TypeConvertor.castToReference(value); // Reference 291 } else 292 return super.setProperty(name, value); 293 return value; 294 } 295 296 @Override 297 public void removeChild(String name, Base value) throws FHIRException { 298 if (name.equals("identifier")) { 299 this.getIdentifier().remove(value); 300 } else if (name.equals("code")) { 301 this.code = null; 302 } else if (name.equals("period")) { 303 this.period = null; 304 } else if (name.equals("issuer")) { 305 this.issuer = null; 306 } else 307 super.removeChild(name, value); 308 309 } 310 311 @Override 312 public Base makeProperty(int hash, String name) throws FHIRException { 313 switch (hash) { 314 case -1618432855: return addIdentifier(); 315 case 3059181: return getCode(); 316 case -991726143: return getPeriod(); 317 case -1179159879: return getIssuer(); 318 default: return super.makeProperty(hash, name); 319 } 320 321 } 322 323 @Override 324 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 325 switch (hash) { 326 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 327 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 328 case -991726143: /*period*/ return new String[] {"Period"}; 329 case -1179159879: /*issuer*/ return new String[] {"Reference"}; 330 default: return super.getTypesForProperty(hash, name); 331 } 332 333 } 334 335 @Override 336 public Base addChild(String name) throws FHIRException { 337 if (name.equals("identifier")) { 338 return addIdentifier(); 339 } 340 else if (name.equals("code")) { 341 this.code = new CodeableConcept(); 342 return this.code; 343 } 344 else if (name.equals("period")) { 345 this.period = new Period(); 346 return this.period; 347 } 348 else if (name.equals("issuer")) { 349 this.issuer = new Reference(); 350 return this.issuer; 351 } 352 else 353 return super.addChild(name); 354 } 355 356 public PractitionerQualificationComponent copy() { 357 PractitionerQualificationComponent dst = new PractitionerQualificationComponent(); 358 copyValues(dst); 359 return dst; 360 } 361 362 public void copyValues(PractitionerQualificationComponent dst) { 363 super.copyValues(dst); 364 if (identifier != null) { 365 dst.identifier = new ArrayList<Identifier>(); 366 for (Identifier i : identifier) 367 dst.identifier.add(i.copy()); 368 }; 369 dst.code = code == null ? null : code.copy(); 370 dst.period = period == null ? null : period.copy(); 371 dst.issuer = issuer == null ? null : issuer.copy(); 372 } 373 374 @Override 375 public boolean equalsDeep(Base other_) { 376 if (!super.equalsDeep(other_)) 377 return false; 378 if (!(other_ instanceof PractitionerQualificationComponent)) 379 return false; 380 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other_; 381 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(period, o.period, true) 382 && compareDeep(issuer, o.issuer, true); 383 } 384 385 @Override 386 public boolean equalsShallow(Base other_) { 387 if (!super.equalsShallow(other_)) 388 return false; 389 if (!(other_ instanceof PractitionerQualificationComponent)) 390 return false; 391 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other_; 392 return true; 393 } 394 395 public boolean isEmpty() { 396 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, period 397 , issuer); 398 } 399 400 public String fhirType() { 401 return "Practitioner.qualification"; 402 403 } 404 405 } 406 407 @Block() 408 public static class PractitionerCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 409 /** 410 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English. 411 */ 412 @Child(name = "language", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 413 @Description(shortDefinition="The language code used to communicate with the practitioner", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English." ) 414 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 415 protected CodeableConcept language; 416 417 /** 418 * Indicates whether or not the person prefers this language (over other languages he masters up a certain level). 419 */ 420 @Child(name = "preferred", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 421 @Description(shortDefinition="Language preference indicator", formalDefinition="Indicates whether or not the person prefers this language (over other languages he masters up a certain level)." ) 422 protected BooleanType preferred; 423 424 private static final long serialVersionUID = 633792918L; 425 426 /** 427 * Constructor 428 */ 429 public PractitionerCommunicationComponent() { 430 super(); 431 } 432 433 /** 434 * Constructor 435 */ 436 public PractitionerCommunicationComponent(CodeableConcept language) { 437 super(); 438 this.setLanguage(language); 439 } 440 441 /** 442 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 443 */ 444 public CodeableConcept getLanguage() { 445 if (this.language == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create PractitionerCommunicationComponent.language"); 448 else if (Configuration.doAutoCreate()) 449 this.language = new CodeableConcept(); // cc 450 return this.language; 451 } 452 453 public boolean hasLanguage() { 454 return this.language != null && !this.language.isEmpty(); 455 } 456 457 /** 458 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 459 */ 460 public PractitionerCommunicationComponent setLanguage(CodeableConcept value) { 461 this.language = value; 462 return this; 463 } 464 465 /** 466 * @return {@link #preferred} (Indicates whether or not the person prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 467 */ 468 public BooleanType getPreferredElement() { 469 if (this.preferred == null) 470 if (Configuration.errorOnAutoCreate()) 471 throw new Error("Attempt to auto-create PractitionerCommunicationComponent.preferred"); 472 else if (Configuration.doAutoCreate()) 473 this.preferred = new BooleanType(); // bb 474 return this.preferred; 475 } 476 477 public boolean hasPreferredElement() { 478 return this.preferred != null && !this.preferred.isEmpty(); 479 } 480 481 public boolean hasPreferred() { 482 return this.preferred != null && !this.preferred.isEmpty(); 483 } 484 485 /** 486 * @param value {@link #preferred} (Indicates whether or not the person prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 487 */ 488 public PractitionerCommunicationComponent setPreferredElement(BooleanType value) { 489 this.preferred = value; 490 return this; 491 } 492 493 /** 494 * @return Indicates whether or not the person prefers this language (over other languages he masters up a certain level). 495 */ 496 public boolean getPreferred() { 497 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 498 } 499 500 /** 501 * @param value Indicates whether or not the person prefers this language (over other languages he masters up a certain level). 502 */ 503 public PractitionerCommunicationComponent setPreferred(boolean value) { 504 if (this.preferred == null) 505 this.preferred = new BooleanType(); 506 this.preferred.setValue(value); 507 return this; 508 } 509 510 protected void listChildren(List<Property> children) { 511 super.listChildren(children); 512 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language)); 513 children.add(new Property("preferred", "boolean", "Indicates whether or not the person prefers this language (over other languages he masters up a certain level).", 0, 1, preferred)); 514 } 515 516 @Override 517 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 518 switch (_hash) { 519 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language); 520 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether or not the person prefers this language (over other languages he masters up a certain level).", 0, 1, preferred); 521 default: return super.getNamedProperty(_hash, _name, _checkValid); 522 } 523 524 } 525 526 @Override 527 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 528 switch (hash) { 529 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 530 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 531 default: return super.getProperty(hash, name, checkValid); 532 } 533 534 } 535 536 @Override 537 public Base setProperty(int hash, String name, Base value) throws FHIRException { 538 switch (hash) { 539 case -1613589672: // language 540 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 541 return value; 542 case -1294005119: // preferred 543 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 544 return value; 545 default: return super.setProperty(hash, name, value); 546 } 547 548 } 549 550 @Override 551 public Base setProperty(String name, Base value) throws FHIRException { 552 if (name.equals("language")) { 553 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 554 } else if (name.equals("preferred")) { 555 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 556 } else 557 return super.setProperty(name, value); 558 return value; 559 } 560 561 @Override 562 public void removeChild(String name, Base value) throws FHIRException { 563 if (name.equals("language")) { 564 this.language = null; 565 } else if (name.equals("preferred")) { 566 this.preferred = null; 567 } else 568 super.removeChild(name, value); 569 570 } 571 572 @Override 573 public Base makeProperty(int hash, String name) throws FHIRException { 574 switch (hash) { 575 case -1613589672: return getLanguage(); 576 case -1294005119: return getPreferredElement(); 577 default: return super.makeProperty(hash, name); 578 } 579 580 } 581 582 @Override 583 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 584 switch (hash) { 585 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 586 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 587 default: return super.getTypesForProperty(hash, name); 588 } 589 590 } 591 592 @Override 593 public Base addChild(String name) throws FHIRException { 594 if (name.equals("language")) { 595 this.language = new CodeableConcept(); 596 return this.language; 597 } 598 else if (name.equals("preferred")) { 599 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.communication.preferred"); 600 } 601 else 602 return super.addChild(name); 603 } 604 605 public PractitionerCommunicationComponent copy() { 606 PractitionerCommunicationComponent dst = new PractitionerCommunicationComponent(); 607 copyValues(dst); 608 return dst; 609 } 610 611 public void copyValues(PractitionerCommunicationComponent dst) { 612 super.copyValues(dst); 613 dst.language = language == null ? null : language.copy(); 614 dst.preferred = preferred == null ? null : preferred.copy(); 615 } 616 617 @Override 618 public boolean equalsDeep(Base other_) { 619 if (!super.equalsDeep(other_)) 620 return false; 621 if (!(other_ instanceof PractitionerCommunicationComponent)) 622 return false; 623 PractitionerCommunicationComponent o = (PractitionerCommunicationComponent) other_; 624 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 625 } 626 627 @Override 628 public boolean equalsShallow(Base other_) { 629 if (!super.equalsShallow(other_)) 630 return false; 631 if (!(other_ instanceof PractitionerCommunicationComponent)) 632 return false; 633 PractitionerCommunicationComponent o = (PractitionerCommunicationComponent) other_; 634 return compareValues(preferred, o.preferred, true); 635 } 636 637 public boolean isEmpty() { 638 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 639 } 640 641 public String fhirType() { 642 return "Practitioner.communication"; 643 644 } 645 646 } 647 648 /** 649 * An identifier that applies to this person in this role. 650 */ 651 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 652 @Description(shortDefinition="An identifier for the person as this agent", formalDefinition="An identifier that applies to this person in this role." ) 653 protected List<Identifier> identifier; 654 655 /** 656 * Whether this practitioner's record is in active use. 657 */ 658 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 659 @Description(shortDefinition="Whether this practitioner's record is in active use", formalDefinition="Whether this practitioner's record is in active use." ) 660 protected BooleanType active; 661 662 /** 663 * The name(s) associated with the practitioner. 664 */ 665 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 666 @Description(shortDefinition="The name(s) associated with the practitioner", formalDefinition="The name(s) associated with the practitioner." ) 667 protected List<HumanName> name; 668 669 /** 670 * A contact detail for the practitioner, e.g. a telephone number or an email address. 671 */ 672 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 673 @Description(shortDefinition="A contact detail for the practitioner (that apply to all roles)", formalDefinition="A contact detail for the practitioner, e.g. a telephone number or an email address." ) 674 protected List<ContactPoint> telecom; 675 676 /** 677 * Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 678 */ 679 @Child(name = "gender", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 680 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes." ) 681 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 682 protected Enumeration<AdministrativeGender> gender; 683 684 /** 685 * The date of birth for the practitioner. 686 */ 687 @Child(name = "birthDate", type = {DateType.class}, order=5, min=0, max=1, modifier=false, summary=true) 688 @Description(shortDefinition="The date on which the practitioner was born", formalDefinition="The date of birth for the practitioner." ) 689 protected DateType birthDate; 690 691 /** 692 * Indicates if the practitioner is deceased or not. 693 */ 694 @Child(name = "deceased", type = {BooleanType.class, DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 695 @Description(shortDefinition="Indicates if the practitioner is deceased or not", formalDefinition="Indicates if the practitioner is deceased or not." ) 696 protected DataType deceased; 697 698 /** 699 * Address(es) of the practitioner that are not role specific (typically home address). 700Work addresses are not typically entered in this property as they are usually role dependent. 701 */ 702 @Child(name = "address", type = {Address.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 703 @Description(shortDefinition="Address(es) of the practitioner that are not role specific (typically home address)", formalDefinition="Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent." ) 704 protected List<Address> address; 705 706 /** 707 * Image of the person. 708 */ 709 @Child(name = "photo", type = {Attachment.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 710 @Description(shortDefinition="Image of the person", formalDefinition="Image of the person." ) 711 protected List<Attachment> photo; 712 713 /** 714 * The official qualifications, certifications, accreditations, training, licenses (and other types of educations/skills/capabilities) that authorize or otherwise pertain to the provision of care by the practitioner. 715 716For example, a medical license issued by a medical board of licensure authorizing the practitioner to practice medicine within a certain locality. 717 */ 718 @Child(name = "qualification", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 719 @Description(shortDefinition="Qualifications, certifications, accreditations, licenses, training, etc. pertaining to the provision of care", formalDefinition="The official qualifications, certifications, accreditations, training, licenses (and other types of educations/skills/capabilities) that authorize or otherwise pertain to the provision of care by the practitioner.\r\rFor example, a medical license issued by a medical board of licensure authorizing the practitioner to practice medicine within a certain locality." ) 720 protected List<PractitionerQualificationComponent> qualification; 721 722 /** 723 * A language which may be used to communicate with the practitioner, often for correspondence/administrative purposes. 724 725The `PractitionerRole.communication` property should be used for publishing the languages that a practitioner is able to communicate with patients (on a per Organization/Role basis). 726 */ 727 @Child(name = "communication", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 728 @Description(shortDefinition="A language which may be used to communicate with the practitioner", formalDefinition="A language which may be used to communicate with the practitioner, often for correspondence/administrative purposes.\r\rThe `PractitionerRole.communication` property should be used for publishing the languages that a practitioner is able to communicate with patients (on a per Organization/Role basis)." ) 729 protected List<PractitionerCommunicationComponent> communication; 730 731 private static final long serialVersionUID = -54730811L; 732 733 /** 734 * Constructor 735 */ 736 public Practitioner() { 737 super(); 738 } 739 740 /** 741 * @return {@link #identifier} (An identifier that applies to this person in this role.) 742 */ 743 public List<Identifier> getIdentifier() { 744 if (this.identifier == null) 745 this.identifier = new ArrayList<Identifier>(); 746 return this.identifier; 747 } 748 749 /** 750 * @return Returns a reference to <code>this</code> for easy method chaining 751 */ 752 public Practitioner setIdentifier(List<Identifier> theIdentifier) { 753 this.identifier = theIdentifier; 754 return this; 755 } 756 757 public boolean hasIdentifier() { 758 if (this.identifier == null) 759 return false; 760 for (Identifier item : this.identifier) 761 if (!item.isEmpty()) 762 return true; 763 return false; 764 } 765 766 public Identifier addIdentifier() { //3 767 Identifier t = new Identifier(); 768 if (this.identifier == null) 769 this.identifier = new ArrayList<Identifier>(); 770 this.identifier.add(t); 771 return t; 772 } 773 774 public Practitioner addIdentifier(Identifier t) { //3 775 if (t == null) 776 return this; 777 if (this.identifier == null) 778 this.identifier = new ArrayList<Identifier>(); 779 this.identifier.add(t); 780 return this; 781 } 782 783 /** 784 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 785 */ 786 public Identifier getIdentifierFirstRep() { 787 if (getIdentifier().isEmpty()) { 788 addIdentifier(); 789 } 790 return getIdentifier().get(0); 791 } 792 793 /** 794 * @return {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 795 */ 796 public BooleanType getActiveElement() { 797 if (this.active == null) 798 if (Configuration.errorOnAutoCreate()) 799 throw new Error("Attempt to auto-create Practitioner.active"); 800 else if (Configuration.doAutoCreate()) 801 this.active = new BooleanType(); // bb 802 return this.active; 803 } 804 805 public boolean hasActiveElement() { 806 return this.active != null && !this.active.isEmpty(); 807 } 808 809 public boolean hasActive() { 810 return this.active != null && !this.active.isEmpty(); 811 } 812 813 /** 814 * @param value {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 815 */ 816 public Practitioner setActiveElement(BooleanType value) { 817 this.active = value; 818 return this; 819 } 820 821 /** 822 * @return Whether this practitioner's record is in active use. 823 */ 824 public boolean getActive() { 825 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 826 } 827 828 /** 829 * @param value Whether this practitioner's record is in active use. 830 */ 831 public Practitioner setActive(boolean value) { 832 if (this.active == null) 833 this.active = new BooleanType(); 834 this.active.setValue(value); 835 return this; 836 } 837 838 /** 839 * @return {@link #name} (The name(s) associated with the practitioner.) 840 */ 841 public List<HumanName> getName() { 842 if (this.name == null) 843 this.name = new ArrayList<HumanName>(); 844 return this.name; 845 } 846 847 /** 848 * @return Returns a reference to <code>this</code> for easy method chaining 849 */ 850 public Practitioner setName(List<HumanName> theName) { 851 this.name = theName; 852 return this; 853 } 854 855 public boolean hasName() { 856 if (this.name == null) 857 return false; 858 for (HumanName item : this.name) 859 if (!item.isEmpty()) 860 return true; 861 return false; 862 } 863 864 public HumanName addName() { //3 865 HumanName t = new HumanName(); 866 if (this.name == null) 867 this.name = new ArrayList<HumanName>(); 868 this.name.add(t); 869 return t; 870 } 871 872 public Practitioner addName(HumanName t) { //3 873 if (t == null) 874 return this; 875 if (this.name == null) 876 this.name = new ArrayList<HumanName>(); 877 this.name.add(t); 878 return this; 879 } 880 881 /** 882 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 883 */ 884 public HumanName getNameFirstRep() { 885 if (getName().isEmpty()) { 886 addName(); 887 } 888 return getName().get(0); 889 } 890 891 /** 892 * @return {@link #telecom} (A contact detail for the practitioner, e.g. a telephone number or an email address.) 893 */ 894 public List<ContactPoint> getTelecom() { 895 if (this.telecom == null) 896 this.telecom = new ArrayList<ContactPoint>(); 897 return this.telecom; 898 } 899 900 /** 901 * @return Returns a reference to <code>this</code> for easy method chaining 902 */ 903 public Practitioner setTelecom(List<ContactPoint> theTelecom) { 904 this.telecom = theTelecom; 905 return this; 906 } 907 908 public boolean hasTelecom() { 909 if (this.telecom == null) 910 return false; 911 for (ContactPoint item : this.telecom) 912 if (!item.isEmpty()) 913 return true; 914 return false; 915 } 916 917 public ContactPoint addTelecom() { //3 918 ContactPoint t = new ContactPoint(); 919 if (this.telecom == null) 920 this.telecom = new ArrayList<ContactPoint>(); 921 this.telecom.add(t); 922 return t; 923 } 924 925 public Practitioner addTelecom(ContactPoint t) { //3 926 if (t == null) 927 return this; 928 if (this.telecom == null) 929 this.telecom = new ArrayList<ContactPoint>(); 930 this.telecom.add(t); 931 return this; 932 } 933 934 /** 935 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 936 */ 937 public ContactPoint getTelecomFirstRep() { 938 if (getTelecom().isEmpty()) { 939 addTelecom(); 940 } 941 return getTelecom().get(0); 942 } 943 944 /** 945 * @return {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 946 */ 947 public Enumeration<AdministrativeGender> getGenderElement() { 948 if (this.gender == null) 949 if (Configuration.errorOnAutoCreate()) 950 throw new Error("Attempt to auto-create Practitioner.gender"); 951 else if (Configuration.doAutoCreate()) 952 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 953 return this.gender; 954 } 955 956 public boolean hasGenderElement() { 957 return this.gender != null && !this.gender.isEmpty(); 958 } 959 960 public boolean hasGender() { 961 return this.gender != null && !this.gender.isEmpty(); 962 } 963 964 /** 965 * @param value {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 966 */ 967 public Practitioner setGenderElement(Enumeration<AdministrativeGender> value) { 968 this.gender = value; 969 return this; 970 } 971 972 /** 973 * @return Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 974 */ 975 public AdministrativeGender getGender() { 976 return this.gender == null ? null : this.gender.getValue(); 977 } 978 979 /** 980 * @param value Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 981 */ 982 public Practitioner setGender(AdministrativeGender value) { 983 if (value == null) 984 this.gender = null; 985 else { 986 if (this.gender == null) 987 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 988 this.gender.setValue(value); 989 } 990 return this; 991 } 992 993 /** 994 * @return {@link #birthDate} (The date of birth for the practitioner.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 995 */ 996 public DateType getBirthDateElement() { 997 if (this.birthDate == null) 998 if (Configuration.errorOnAutoCreate()) 999 throw new Error("Attempt to auto-create Practitioner.birthDate"); 1000 else if (Configuration.doAutoCreate()) 1001 this.birthDate = new DateType(); // bb 1002 return this.birthDate; 1003 } 1004 1005 public boolean hasBirthDateElement() { 1006 return this.birthDate != null && !this.birthDate.isEmpty(); 1007 } 1008 1009 public boolean hasBirthDate() { 1010 return this.birthDate != null && !this.birthDate.isEmpty(); 1011 } 1012 1013 /** 1014 * @param value {@link #birthDate} (The date of birth for the practitioner.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 1015 */ 1016 public Practitioner setBirthDateElement(DateType value) { 1017 this.birthDate = value; 1018 return this; 1019 } 1020 1021 /** 1022 * @return The date of birth for the practitioner. 1023 */ 1024 public Date getBirthDate() { 1025 return this.birthDate == null ? null : this.birthDate.getValue(); 1026 } 1027 1028 /** 1029 * @param value The date of birth for the practitioner. 1030 */ 1031 public Practitioner setBirthDate(Date value) { 1032 if (value == null) 1033 this.birthDate = null; 1034 else { 1035 if (this.birthDate == null) 1036 this.birthDate = new DateType(); 1037 this.birthDate.setValue(value); 1038 } 1039 return this; 1040 } 1041 1042 /** 1043 * @return {@link #deceased} (Indicates if the practitioner is deceased or not.) 1044 */ 1045 public DataType getDeceased() { 1046 return this.deceased; 1047 } 1048 1049 /** 1050 * @return {@link #deceased} (Indicates if the practitioner is deceased or not.) 1051 */ 1052 public BooleanType getDeceasedBooleanType() throws FHIRException { 1053 if (this.deceased == null) 1054 this.deceased = new BooleanType(); 1055 if (!(this.deceased instanceof BooleanType)) 1056 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1057 return (BooleanType) this.deceased; 1058 } 1059 1060 public boolean hasDeceasedBooleanType() { 1061 return this.deceased instanceof BooleanType; 1062 } 1063 1064 /** 1065 * @return {@link #deceased} (Indicates if the practitioner is deceased or not.) 1066 */ 1067 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 1068 if (this.deceased == null) 1069 this.deceased = new DateTimeType(); 1070 if (!(this.deceased instanceof DateTimeType)) 1071 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1072 return (DateTimeType) this.deceased; 1073 } 1074 1075 public boolean hasDeceasedDateTimeType() { 1076 return this.deceased instanceof DateTimeType; 1077 } 1078 1079 public boolean hasDeceased() { 1080 return this.deceased != null && !this.deceased.isEmpty(); 1081 } 1082 1083 /** 1084 * @param value {@link #deceased} (Indicates if the practitioner is deceased or not.) 1085 */ 1086 public Practitioner setDeceased(DataType value) { 1087 if (value != null && !(value instanceof BooleanType || value instanceof DateTimeType)) 1088 throw new FHIRException("Not the right type for Practitioner.deceased[x]: "+value.fhirType()); 1089 this.deceased = value; 1090 return this; 1091 } 1092 1093 /** 1094 * @return {@link #address} (Address(es) of the practitioner that are not role specific (typically home address). 1095Work addresses are not typically entered in this property as they are usually role dependent.) 1096 */ 1097 public List<Address> getAddress() { 1098 if (this.address == null) 1099 this.address = new ArrayList<Address>(); 1100 return this.address; 1101 } 1102 1103 /** 1104 * @return Returns a reference to <code>this</code> for easy method chaining 1105 */ 1106 public Practitioner setAddress(List<Address> theAddress) { 1107 this.address = theAddress; 1108 return this; 1109 } 1110 1111 public boolean hasAddress() { 1112 if (this.address == null) 1113 return false; 1114 for (Address item : this.address) 1115 if (!item.isEmpty()) 1116 return true; 1117 return false; 1118 } 1119 1120 public Address addAddress() { //3 1121 Address t = new Address(); 1122 if (this.address == null) 1123 this.address = new ArrayList<Address>(); 1124 this.address.add(t); 1125 return t; 1126 } 1127 1128 public Practitioner addAddress(Address t) { //3 1129 if (t == null) 1130 return this; 1131 if (this.address == null) 1132 this.address = new ArrayList<Address>(); 1133 this.address.add(t); 1134 return this; 1135 } 1136 1137 /** 1138 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist {3} 1139 */ 1140 public Address getAddressFirstRep() { 1141 if (getAddress().isEmpty()) { 1142 addAddress(); 1143 } 1144 return getAddress().get(0); 1145 } 1146 1147 /** 1148 * @return {@link #photo} (Image of the person.) 1149 */ 1150 public List<Attachment> getPhoto() { 1151 if (this.photo == null) 1152 this.photo = new ArrayList<Attachment>(); 1153 return this.photo; 1154 } 1155 1156 /** 1157 * @return Returns a reference to <code>this</code> for easy method chaining 1158 */ 1159 public Practitioner setPhoto(List<Attachment> thePhoto) { 1160 this.photo = thePhoto; 1161 return this; 1162 } 1163 1164 public boolean hasPhoto() { 1165 if (this.photo == null) 1166 return false; 1167 for (Attachment item : this.photo) 1168 if (!item.isEmpty()) 1169 return true; 1170 return false; 1171 } 1172 1173 public Attachment addPhoto() { //3 1174 Attachment t = new Attachment(); 1175 if (this.photo == null) 1176 this.photo = new ArrayList<Attachment>(); 1177 this.photo.add(t); 1178 return t; 1179 } 1180 1181 public Practitioner addPhoto(Attachment t) { //3 1182 if (t == null) 1183 return this; 1184 if (this.photo == null) 1185 this.photo = new ArrayList<Attachment>(); 1186 this.photo.add(t); 1187 return this; 1188 } 1189 1190 /** 1191 * @return The first repetition of repeating field {@link #photo}, creating it if it does not already exist {3} 1192 */ 1193 public Attachment getPhotoFirstRep() { 1194 if (getPhoto().isEmpty()) { 1195 addPhoto(); 1196 } 1197 return getPhoto().get(0); 1198 } 1199 1200 /** 1201 * @return {@link #qualification} (The official qualifications, certifications, accreditations, training, licenses (and other types of educations/skills/capabilities) that authorize or otherwise pertain to the provision of care by the practitioner. 1202 1203For example, a medical license issued by a medical board of licensure authorizing the practitioner to practice medicine within a certain locality.) 1204 */ 1205 public List<PractitionerQualificationComponent> getQualification() { 1206 if (this.qualification == null) 1207 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1208 return this.qualification; 1209 } 1210 1211 /** 1212 * @return Returns a reference to <code>this</code> for easy method chaining 1213 */ 1214 public Practitioner setQualification(List<PractitionerQualificationComponent> theQualification) { 1215 this.qualification = theQualification; 1216 return this; 1217 } 1218 1219 public boolean hasQualification() { 1220 if (this.qualification == null) 1221 return false; 1222 for (PractitionerQualificationComponent item : this.qualification) 1223 if (!item.isEmpty()) 1224 return true; 1225 return false; 1226 } 1227 1228 public PractitionerQualificationComponent addQualification() { //3 1229 PractitionerQualificationComponent t = new PractitionerQualificationComponent(); 1230 if (this.qualification == null) 1231 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1232 this.qualification.add(t); 1233 return t; 1234 } 1235 1236 public Practitioner addQualification(PractitionerQualificationComponent t) { //3 1237 if (t == null) 1238 return this; 1239 if (this.qualification == null) 1240 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1241 this.qualification.add(t); 1242 return this; 1243 } 1244 1245 /** 1246 * @return The first repetition of repeating field {@link #qualification}, creating it if it does not already exist {3} 1247 */ 1248 public PractitionerQualificationComponent getQualificationFirstRep() { 1249 if (getQualification().isEmpty()) { 1250 addQualification(); 1251 } 1252 return getQualification().get(0); 1253 } 1254 1255 /** 1256 * @return {@link #communication} (A language which may be used to communicate with the practitioner, often for correspondence/administrative purposes. 1257 1258The `PractitionerRole.communication` property should be used for publishing the languages that a practitioner is able to communicate with patients (on a per Organization/Role basis).) 1259 */ 1260 public List<PractitionerCommunicationComponent> getCommunication() { 1261 if (this.communication == null) 1262 this.communication = new ArrayList<PractitionerCommunicationComponent>(); 1263 return this.communication; 1264 } 1265 1266 /** 1267 * @return Returns a reference to <code>this</code> for easy method chaining 1268 */ 1269 public Practitioner setCommunication(List<PractitionerCommunicationComponent> theCommunication) { 1270 this.communication = theCommunication; 1271 return this; 1272 } 1273 1274 public boolean hasCommunication() { 1275 if (this.communication == null) 1276 return false; 1277 for (PractitionerCommunicationComponent item : this.communication) 1278 if (!item.isEmpty()) 1279 return true; 1280 return false; 1281 } 1282 1283 public PractitionerCommunicationComponent addCommunication() { //3 1284 PractitionerCommunicationComponent t = new PractitionerCommunicationComponent(); 1285 if (this.communication == null) 1286 this.communication = new ArrayList<PractitionerCommunicationComponent>(); 1287 this.communication.add(t); 1288 return t; 1289 } 1290 1291 public Practitioner addCommunication(PractitionerCommunicationComponent t) { //3 1292 if (t == null) 1293 return this; 1294 if (this.communication == null) 1295 this.communication = new ArrayList<PractitionerCommunicationComponent>(); 1296 this.communication.add(t); 1297 return this; 1298 } 1299 1300 /** 1301 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist {3} 1302 */ 1303 public PractitionerCommunicationComponent getCommunicationFirstRep() { 1304 if (getCommunication().isEmpty()) { 1305 addCommunication(); 1306 } 1307 return getCommunication().get(0); 1308 } 1309 1310 protected void listChildren(List<Property> children) { 1311 super.listChildren(children); 1312 children.add(new Property("identifier", "Identifier", "An identifier that applies to this person in this role.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1313 children.add(new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active)); 1314 children.add(new Property("name", "HumanName", "The name(s) associated with the practitioner.", 0, java.lang.Integer.MAX_VALUE, name)); 1315 children.add(new Property("telecom", "ContactPoint", "A contact detail for the practitioner, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1316 children.add(new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender)); 1317 children.add(new Property("birthDate", "date", "The date of birth for the practitioner.", 0, 1, birthDate)); 1318 children.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the practitioner is deceased or not.", 0, 1, deceased)); 1319 children.add(new Property("address", "Address", "Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent.", 0, java.lang.Integer.MAX_VALUE, address)); 1320 children.add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 1321 children.add(new Property("qualification", "", "The official qualifications, certifications, accreditations, training, licenses (and other types of educations/skills/capabilities) that authorize or otherwise pertain to the provision of care by the practitioner.\r\rFor example, a medical license issued by a medical board of licensure authorizing the practitioner to practice medicine within a certain locality.", 0, java.lang.Integer.MAX_VALUE, qualification)); 1322 children.add(new Property("communication", "", "A language which may be used to communicate with the practitioner, often for correspondence/administrative purposes.\r\rThe `PractitionerRole.communication` property should be used for publishing the languages that a practitioner is able to communicate with patients (on a per Organization/Role basis).", 0, java.lang.Integer.MAX_VALUE, communication)); 1323 } 1324 1325 @Override 1326 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1327 switch (_hash) { 1328 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier that applies to this person in this role.", 0, java.lang.Integer.MAX_VALUE, identifier); 1329 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active); 1330 case 3373707: /*name*/ return new Property("name", "HumanName", "The name(s) associated with the practitioner.", 0, java.lang.Integer.MAX_VALUE, name); 1331 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the practitioner, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 1332 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender); 1333 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The date of birth for the practitioner.", 0, 1, birthDate); 1334 case -1311442804: /*deceased[x]*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the practitioner is deceased or not.", 0, 1, deceased); 1335 case 561497972: /*deceased*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the practitioner is deceased or not.", 0, 1, deceased); 1336 case 497463828: /*deceasedBoolean*/ return new Property("deceased[x]", "boolean", "Indicates if the practitioner is deceased or not.", 0, 1, deceased); 1337 case -1971804369: /*deceasedDateTime*/ return new Property("deceased[x]", "dateTime", "Indicates if the practitioner is deceased or not.", 0, 1, deceased); 1338 case -1147692044: /*address*/ return new Property("address", "Address", "Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent.", 0, java.lang.Integer.MAX_VALUE, address); 1339 case 106642994: /*photo*/ return new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo); 1340 case -631333393: /*qualification*/ return new Property("qualification", "", "The official qualifications, certifications, accreditations, training, licenses (and other types of educations/skills/capabilities) that authorize or otherwise pertain to the provision of care by the practitioner.\r\rFor example, a medical license issued by a medical board of licensure authorizing the practitioner to practice medicine within a certain locality.", 0, java.lang.Integer.MAX_VALUE, qualification); 1341 case -1035284522: /*communication*/ return new Property("communication", "", "A language which may be used to communicate with the practitioner, often for correspondence/administrative purposes.\r\rThe `PractitionerRole.communication` property should be used for publishing the languages that a practitioner is able to communicate with patients (on a per Organization/Role basis).", 0, java.lang.Integer.MAX_VALUE, communication); 1342 default: return super.getNamedProperty(_hash, _name, _checkValid); 1343 } 1344 1345 } 1346 1347 @Override 1348 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1349 switch (hash) { 1350 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1351 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1352 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1353 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1354 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1355 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 1356 case 561497972: /*deceased*/ return this.deceased == null ? new Base[0] : new Base[] {this.deceased}; // DataType 1357 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1358 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 1359 case -631333393: /*qualification*/ return this.qualification == null ? new Base[0] : this.qualification.toArray(new Base[this.qualification.size()]); // PractitionerQualificationComponent 1360 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // PractitionerCommunicationComponent 1361 default: return super.getProperty(hash, name, checkValid); 1362 } 1363 1364 } 1365 1366 @Override 1367 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1368 switch (hash) { 1369 case -1618432855: // identifier 1370 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1371 return value; 1372 case -1422950650: // active 1373 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1374 return value; 1375 case 3373707: // name 1376 this.getName().add(TypeConvertor.castToHumanName(value)); // HumanName 1377 return value; 1378 case -1429363305: // telecom 1379 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 1380 return value; 1381 case -1249512767: // gender 1382 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1383 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1384 return value; 1385 case -1210031859: // birthDate 1386 this.birthDate = TypeConvertor.castToDate(value); // DateType 1387 return value; 1388 case 561497972: // deceased 1389 this.deceased = TypeConvertor.castToType(value); // DataType 1390 return value; 1391 case -1147692044: // address 1392 this.getAddress().add(TypeConvertor.castToAddress(value)); // Address 1393 return value; 1394 case 106642994: // photo 1395 this.getPhoto().add(TypeConvertor.castToAttachment(value)); // Attachment 1396 return value; 1397 case -631333393: // qualification 1398 this.getQualification().add((PractitionerQualificationComponent) value); // PractitionerQualificationComponent 1399 return value; 1400 case -1035284522: // communication 1401 this.getCommunication().add((PractitionerCommunicationComponent) value); // PractitionerCommunicationComponent 1402 return value; 1403 default: return super.setProperty(hash, name, value); 1404 } 1405 1406 } 1407 1408 @Override 1409 public Base setProperty(String name, Base value) throws FHIRException { 1410 if (name.equals("identifier")) { 1411 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1412 } else if (name.equals("active")) { 1413 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1414 } else if (name.equals("name")) { 1415 this.getName().add(TypeConvertor.castToHumanName(value)); 1416 } else if (name.equals("telecom")) { 1417 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 1418 } else if (name.equals("gender")) { 1419 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1420 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1421 } else if (name.equals("birthDate")) { 1422 this.birthDate = TypeConvertor.castToDate(value); // DateType 1423 } else if (name.equals("deceased[x]")) { 1424 this.deceased = TypeConvertor.castToType(value); // DataType 1425 } else if (name.equals("address")) { 1426 this.getAddress().add(TypeConvertor.castToAddress(value)); 1427 } else if (name.equals("photo")) { 1428 this.getPhoto().add(TypeConvertor.castToAttachment(value)); 1429 } else if (name.equals("qualification")) { 1430 this.getQualification().add((PractitionerQualificationComponent) value); 1431 } else if (name.equals("communication")) { 1432 this.getCommunication().add((PractitionerCommunicationComponent) value); 1433 } else 1434 return super.setProperty(name, value); 1435 return value; 1436 } 1437 1438 @Override 1439 public void removeChild(String name, Base value) throws FHIRException { 1440 if (name.equals("identifier")) { 1441 this.getIdentifier().remove(value); 1442 } else if (name.equals("active")) { 1443 this.active = null; 1444 } else if (name.equals("name")) { 1445 this.getName().remove(value); 1446 } else if (name.equals("telecom")) { 1447 this.getTelecom().remove(value); 1448 } else if (name.equals("gender")) { 1449 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1450 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1451 } else if (name.equals("birthDate")) { 1452 this.birthDate = null; 1453 } else if (name.equals("deceased[x]")) { 1454 this.deceased = null; 1455 } else if (name.equals("address")) { 1456 this.getAddress().remove(value); 1457 } else if (name.equals("photo")) { 1458 this.getPhoto().remove(value); 1459 } else if (name.equals("qualification")) { 1460 this.getQualification().remove((PractitionerQualificationComponent) value); 1461 } else if (name.equals("communication")) { 1462 this.getCommunication().remove((PractitionerCommunicationComponent) value); 1463 } else 1464 super.removeChild(name, value); 1465 1466 } 1467 1468 @Override 1469 public Base makeProperty(int hash, String name) throws FHIRException { 1470 switch (hash) { 1471 case -1618432855: return addIdentifier(); 1472 case -1422950650: return getActiveElement(); 1473 case 3373707: return addName(); 1474 case -1429363305: return addTelecom(); 1475 case -1249512767: return getGenderElement(); 1476 case -1210031859: return getBirthDateElement(); 1477 case -1311442804: return getDeceased(); 1478 case 561497972: return getDeceased(); 1479 case -1147692044: return addAddress(); 1480 case 106642994: return addPhoto(); 1481 case -631333393: return addQualification(); 1482 case -1035284522: return addCommunication(); 1483 default: return super.makeProperty(hash, name); 1484 } 1485 1486 } 1487 1488 @Override 1489 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1490 switch (hash) { 1491 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1492 case -1422950650: /*active*/ return new String[] {"boolean"}; 1493 case 3373707: /*name*/ return new String[] {"HumanName"}; 1494 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1495 case -1249512767: /*gender*/ return new String[] {"code"}; 1496 case -1210031859: /*birthDate*/ return new String[] {"date"}; 1497 case 561497972: /*deceased*/ return new String[] {"boolean", "dateTime"}; 1498 case -1147692044: /*address*/ return new String[] {"Address"}; 1499 case 106642994: /*photo*/ return new String[] {"Attachment"}; 1500 case -631333393: /*qualification*/ return new String[] {}; 1501 case -1035284522: /*communication*/ return new String[] {}; 1502 default: return super.getTypesForProperty(hash, name); 1503 } 1504 1505 } 1506 1507 @Override 1508 public Base addChild(String name) throws FHIRException { 1509 if (name.equals("identifier")) { 1510 return addIdentifier(); 1511 } 1512 else if (name.equals("active")) { 1513 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.active"); 1514 } 1515 else if (name.equals("name")) { 1516 return addName(); 1517 } 1518 else if (name.equals("telecom")) { 1519 return addTelecom(); 1520 } 1521 else if (name.equals("gender")) { 1522 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.gender"); 1523 } 1524 else if (name.equals("birthDate")) { 1525 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.birthDate"); 1526 } 1527 else if (name.equals("deceasedBoolean")) { 1528 this.deceased = new BooleanType(); 1529 return this.deceased; 1530 } 1531 else if (name.equals("deceasedDateTime")) { 1532 this.deceased = new DateTimeType(); 1533 return this.deceased; 1534 } 1535 else if (name.equals("address")) { 1536 return addAddress(); 1537 } 1538 else if (name.equals("photo")) { 1539 return addPhoto(); 1540 } 1541 else if (name.equals("qualification")) { 1542 return addQualification(); 1543 } 1544 else if (name.equals("communication")) { 1545 return addCommunication(); 1546 } 1547 else 1548 return super.addChild(name); 1549 } 1550 1551 public String fhirType() { 1552 return "Practitioner"; 1553 1554 } 1555 1556 public Practitioner copy() { 1557 Practitioner dst = new Practitioner(); 1558 copyValues(dst); 1559 return dst; 1560 } 1561 1562 public void copyValues(Practitioner dst) { 1563 super.copyValues(dst); 1564 if (identifier != null) { 1565 dst.identifier = new ArrayList<Identifier>(); 1566 for (Identifier i : identifier) 1567 dst.identifier.add(i.copy()); 1568 }; 1569 dst.active = active == null ? null : active.copy(); 1570 if (name != null) { 1571 dst.name = new ArrayList<HumanName>(); 1572 for (HumanName i : name) 1573 dst.name.add(i.copy()); 1574 }; 1575 if (telecom != null) { 1576 dst.telecom = new ArrayList<ContactPoint>(); 1577 for (ContactPoint i : telecom) 1578 dst.telecom.add(i.copy()); 1579 }; 1580 dst.gender = gender == null ? null : gender.copy(); 1581 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1582 dst.deceased = deceased == null ? null : deceased.copy(); 1583 if (address != null) { 1584 dst.address = new ArrayList<Address>(); 1585 for (Address i : address) 1586 dst.address.add(i.copy()); 1587 }; 1588 if (photo != null) { 1589 dst.photo = new ArrayList<Attachment>(); 1590 for (Attachment i : photo) 1591 dst.photo.add(i.copy()); 1592 }; 1593 if (qualification != null) { 1594 dst.qualification = new ArrayList<PractitionerQualificationComponent>(); 1595 for (PractitionerQualificationComponent i : qualification) 1596 dst.qualification.add(i.copy()); 1597 }; 1598 if (communication != null) { 1599 dst.communication = new ArrayList<PractitionerCommunicationComponent>(); 1600 for (PractitionerCommunicationComponent i : communication) 1601 dst.communication.add(i.copy()); 1602 }; 1603 } 1604 1605 protected Practitioner typedCopy() { 1606 return copy(); 1607 } 1608 1609 @Override 1610 public boolean equalsDeep(Base other_) { 1611 if (!super.equalsDeep(other_)) 1612 return false; 1613 if (!(other_ instanceof Practitioner)) 1614 return false; 1615 Practitioner o = (Practitioner) other_; 1616 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(name, o.name, true) 1617 && compareDeep(telecom, o.telecom, true) && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 1618 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) && compareDeep(photo, o.photo, true) 1619 && compareDeep(qualification, o.qualification, true) && compareDeep(communication, o.communication, true) 1620 ; 1621 } 1622 1623 @Override 1624 public boolean equalsShallow(Base other_) { 1625 if (!super.equalsShallow(other_)) 1626 return false; 1627 if (!(other_ instanceof Practitioner)) 1628 return false; 1629 Practitioner o = (Practitioner) other_; 1630 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 1631 ; 1632 } 1633 1634 public boolean isEmpty() { 1635 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name 1636 , telecom, gender, birthDate, deceased, address, photo, qualification, communication 1637 ); 1638 } 1639 1640 @Override 1641 public ResourceType getResourceType() { 1642 return ResourceType.Practitioner; 1643 } 1644 1645 /** 1646 * Search parameter: <b>active</b> 1647 * <p> 1648 * Description: <b>Whether the practitioner record is active</b><br> 1649 * Type: <b>token</b><br> 1650 * Path: <b>Practitioner.active</b><br> 1651 * </p> 1652 */ 1653 @SearchParamDefinition(name="active", path="Practitioner.active", description="Whether the practitioner record is active", type="token" ) 1654 public static final String SP_ACTIVE = "active"; 1655 /** 1656 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1657 * <p> 1658 * Description: <b>Whether the practitioner record is active</b><br> 1659 * Type: <b>token</b><br> 1660 * Path: <b>Practitioner.active</b><br> 1661 * </p> 1662 */ 1663 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1664 1665 /** 1666 * Search parameter: <b>communication</b> 1667 * <p> 1668 * Description: <b>A language to communicate with the practitioner</b><br> 1669 * Type: <b>token</b><br> 1670 * Path: <b>Practitioner.communication.language</b><br> 1671 * </p> 1672 */ 1673 @SearchParamDefinition(name="communication", path="Practitioner.communication.language", description="A language to communicate with the practitioner", type="token" ) 1674 public static final String SP_COMMUNICATION = "communication"; 1675 /** 1676 * <b>Fluent Client</b> search parameter constant for <b>communication</b> 1677 * <p> 1678 * Description: <b>A language to communicate with the practitioner</b><br> 1679 * Type: <b>token</b><br> 1680 * Path: <b>Practitioner.communication.language</b><br> 1681 * </p> 1682 */ 1683 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMMUNICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMMUNICATION); 1684 1685 /** 1686 * Search parameter: <b>death-date</b> 1687 * <p> 1688 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 1689 * Type: <b>date</b><br> 1690 * Path: <b>(Practitioner.deceased.ofType(dateTime))</b><br> 1691 * </p> 1692 */ 1693 @SearchParamDefinition(name="death-date", path="(Practitioner.deceased.ofType(dateTime))", description="The date of death has been provided and satisfies this search value", type="date" ) 1694 public static final String SP_DEATH_DATE = "death-date"; 1695 /** 1696 * <b>Fluent Client</b> search parameter constant for <b>death-date</b> 1697 * <p> 1698 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 1699 * Type: <b>date</b><br> 1700 * Path: <b>(Practitioner.deceased.ofType(dateTime))</b><br> 1701 * </p> 1702 */ 1703 public static final ca.uhn.fhir.rest.gclient.DateClientParam DEATH_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DEATH_DATE); 1704 1705 /** 1706 * Search parameter: <b>deceased</b> 1707 * <p> 1708 * Description: <b>This Practitioner has been marked as deceased, or has a death date entered</b><br> 1709 * Type: <b>token</b><br> 1710 * Path: <b>Practitioner.deceased.exists() and Practitioner.deceased != false</b><br> 1711 * </p> 1712 */ 1713 @SearchParamDefinition(name="deceased", path="Practitioner.deceased.exists() and Practitioner.deceased != false", description="This Practitioner has been marked as deceased, or has a death date entered", type="token" ) 1714 public static final String SP_DECEASED = "deceased"; 1715 /** 1716 * <b>Fluent Client</b> search parameter constant for <b>deceased</b> 1717 * <p> 1718 * Description: <b>This Practitioner has been marked as deceased, or has a death date entered</b><br> 1719 * Type: <b>token</b><br> 1720 * Path: <b>Practitioner.deceased.exists() and Practitioner.deceased != false</b><br> 1721 * </p> 1722 */ 1723 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DECEASED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DECEASED); 1724 1725 /** 1726 * Search parameter: <b>identifier</b> 1727 * <p> 1728 * Description: <b>A practitioner's Identifier</b><br> 1729 * Type: <b>token</b><br> 1730 * Path: <b>Practitioner.identifier | Practitioner.qualification.identifier</b><br> 1731 * </p> 1732 */ 1733 @SearchParamDefinition(name="identifier", path="Practitioner.identifier | Practitioner.qualification.identifier", description="A practitioner's Identifier", type="token" ) 1734 public static final String SP_IDENTIFIER = "identifier"; 1735 /** 1736 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1737 * <p> 1738 * Description: <b>A practitioner's Identifier</b><br> 1739 * Type: <b>token</b><br> 1740 * Path: <b>Practitioner.identifier | Practitioner.qualification.identifier</b><br> 1741 * </p> 1742 */ 1743 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1744 1745 /** 1746 * Search parameter: <b>name</b> 1747 * <p> 1748 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1749 * Type: <b>string</b><br> 1750 * Path: <b>Practitioner.name</b><br> 1751 * </p> 1752 */ 1753 @SearchParamDefinition(name="name", path="Practitioner.name", description="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type="string" ) 1754 public static final String SP_NAME = "name"; 1755 /** 1756 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1757 * <p> 1758 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1759 * Type: <b>string</b><br> 1760 * Path: <b>Practitioner.name</b><br> 1761 * </p> 1762 */ 1763 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1764 1765 /** 1766 * Search parameter: <b>qualification-period</b> 1767 * <p> 1768 * Description: <b>The date(s) a qualification is valid for</b><br> 1769 * Type: <b>date</b><br> 1770 * Path: <b>Practitioner.qualification.period</b><br> 1771 * </p> 1772 */ 1773 @SearchParamDefinition(name="qualification-period", path="Practitioner.qualification.period", description="The date(s) a qualification is valid for", type="date" ) 1774 public static final String SP_QUALIFICATION_PERIOD = "qualification-period"; 1775 /** 1776 * <b>Fluent Client</b> search parameter constant for <b>qualification-period</b> 1777 * <p> 1778 * Description: <b>The date(s) a qualification is valid for</b><br> 1779 * Type: <b>date</b><br> 1780 * Path: <b>Practitioner.qualification.period</b><br> 1781 * </p> 1782 */ 1783 public static final ca.uhn.fhir.rest.gclient.DateClientParam QUALIFICATION_PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_QUALIFICATION_PERIOD); 1784 1785 /** 1786 * Search parameter: <b>address-city</b> 1787 * <p> 1788 * Description: <b>Multiple Resources: 1789 1790* [Patient](patient.html): A city specified in an address 1791* [Person](person.html): A city specified in an address 1792* [Practitioner](practitioner.html): A city specified in an address 1793* [RelatedPerson](relatedperson.html): A city specified in an address 1794</b><br> 1795 * Type: <b>string</b><br> 1796 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 1797 * </p> 1798 */ 1799 @SearchParamDefinition(name="address-city", path="Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A city specified in an address\r\n* [Person](person.html): A city specified in an address\r\n* [Practitioner](practitioner.html): A city specified in an address\r\n* [RelatedPerson](relatedperson.html): A city specified in an address\r\n", type="string" ) 1800 public static final String SP_ADDRESS_CITY = "address-city"; 1801 /** 1802 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1803 * <p> 1804 * Description: <b>Multiple Resources: 1805 1806* [Patient](patient.html): A city specified in an address 1807* [Person](person.html): A city specified in an address 1808* [Practitioner](practitioner.html): A city specified in an address 1809* [RelatedPerson](relatedperson.html): A city specified in an address 1810</b><br> 1811 * Type: <b>string</b><br> 1812 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 1813 * </p> 1814 */ 1815 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 1816 1817 /** 1818 * Search parameter: <b>address-country</b> 1819 * <p> 1820 * Description: <b>Multiple Resources: 1821 1822* [Patient](patient.html): A country specified in an address 1823* [Person](person.html): A country specified in an address 1824* [Practitioner](practitioner.html): A country specified in an address 1825* [RelatedPerson](relatedperson.html): A country specified in an address 1826</b><br> 1827 * Type: <b>string</b><br> 1828 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 1829 * </p> 1830 */ 1831 @SearchParamDefinition(name="address-country", path="Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A country specified in an address\r\n* [Person](person.html): A country specified in an address\r\n* [Practitioner](practitioner.html): A country specified in an address\r\n* [RelatedPerson](relatedperson.html): A country specified in an address\r\n", type="string" ) 1832 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1833 /** 1834 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1835 * <p> 1836 * Description: <b>Multiple Resources: 1837 1838* [Patient](patient.html): A country specified in an address 1839* [Person](person.html): A country specified in an address 1840* [Practitioner](practitioner.html): A country specified in an address 1841* [RelatedPerson](relatedperson.html): A country specified in an address 1842</b><br> 1843 * Type: <b>string</b><br> 1844 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 1845 * </p> 1846 */ 1847 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1848 1849 /** 1850 * Search parameter: <b>address-postalcode</b> 1851 * <p> 1852 * Description: <b>Multiple Resources: 1853 1854* [Patient](patient.html): A postalCode specified in an address 1855* [Person](person.html): A postal code specified in an address 1856* [Practitioner](practitioner.html): A postalCode specified in an address 1857* [RelatedPerson](relatedperson.html): A postal code specified in an address 1858</b><br> 1859 * Type: <b>string</b><br> 1860 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 1861 * </p> 1862 */ 1863 @SearchParamDefinition(name="address-postalcode", path="Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A postalCode specified in an address\r\n* [Person](person.html): A postal code specified in an address\r\n* [Practitioner](practitioner.html): A postalCode specified in an address\r\n* [RelatedPerson](relatedperson.html): A postal code specified in an address\r\n", type="string" ) 1864 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1865 /** 1866 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1867 * <p> 1868 * Description: <b>Multiple Resources: 1869 1870* [Patient](patient.html): A postalCode specified in an address 1871* [Person](person.html): A postal code specified in an address 1872* [Practitioner](practitioner.html): A postalCode specified in an address 1873* [RelatedPerson](relatedperson.html): A postal code specified in an address 1874</b><br> 1875 * Type: <b>string</b><br> 1876 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 1877 * </p> 1878 */ 1879 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1880 1881 /** 1882 * Search parameter: <b>address-state</b> 1883 * <p> 1884 * Description: <b>Multiple Resources: 1885 1886* [Patient](patient.html): A state specified in an address 1887* [Person](person.html): A state specified in an address 1888* [Practitioner](practitioner.html): A state specified in an address 1889* [RelatedPerson](relatedperson.html): A state specified in an address 1890</b><br> 1891 * Type: <b>string</b><br> 1892 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 1893 * </p> 1894 */ 1895 @SearchParamDefinition(name="address-state", path="Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A state specified in an address\r\n* [Person](person.html): A state specified in an address\r\n* [Practitioner](practitioner.html): A state specified in an address\r\n* [RelatedPerson](relatedperson.html): A state specified in an address\r\n", type="string" ) 1896 public static final String SP_ADDRESS_STATE = "address-state"; 1897 /** 1898 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1899 * <p> 1900 * Description: <b>Multiple Resources: 1901 1902* [Patient](patient.html): A state specified in an address 1903* [Person](person.html): A state specified in an address 1904* [Practitioner](practitioner.html): A state specified in an address 1905* [RelatedPerson](relatedperson.html): A state specified in an address 1906</b><br> 1907 * Type: <b>string</b><br> 1908 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 1909 * </p> 1910 */ 1911 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1912 1913 /** 1914 * Search parameter: <b>address-use</b> 1915 * <p> 1916 * Description: <b>Multiple Resources: 1917 1918* [Patient](patient.html): A use code specified in an address 1919* [Person](person.html): A use code specified in an address 1920* [Practitioner](practitioner.html): A use code specified in an address 1921* [RelatedPerson](relatedperson.html): A use code specified in an address 1922</b><br> 1923 * Type: <b>token</b><br> 1924 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 1925 * </p> 1926 */ 1927 @SearchParamDefinition(name="address-use", path="Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A use code specified in an address\r\n* [Person](person.html): A use code specified in an address\r\n* [Practitioner](practitioner.html): A use code specified in an address\r\n* [RelatedPerson](relatedperson.html): A use code specified in an address\r\n", type="token" ) 1928 public static final String SP_ADDRESS_USE = "address-use"; 1929 /** 1930 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1931 * <p> 1932 * Description: <b>Multiple Resources: 1933 1934* [Patient](patient.html): A use code specified in an address 1935* [Person](person.html): A use code specified in an address 1936* [Practitioner](practitioner.html): A use code specified in an address 1937* [RelatedPerson](relatedperson.html): A use code specified in an address 1938</b><br> 1939 * Type: <b>token</b><br> 1940 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 1941 * </p> 1942 */ 1943 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 1944 1945 /** 1946 * Search parameter: <b>address</b> 1947 * <p> 1948 * Description: <b>Multiple Resources: 1949 1950* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1951* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1952* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1953* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1954</b><br> 1955 * Type: <b>string</b><br> 1956 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 1957 * </p> 1958 */ 1959 @SearchParamDefinition(name="address", path="Patient.address | Person.address | Practitioner.address | RelatedPerson.address", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n", type="string" ) 1960 public static final String SP_ADDRESS = "address"; 1961 /** 1962 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1963 * <p> 1964 * Description: <b>Multiple Resources: 1965 1966* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1967* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1968* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1969* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1970</b><br> 1971 * Type: <b>string</b><br> 1972 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 1973 * </p> 1974 */ 1975 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 1976 1977 /** 1978 * Search parameter: <b>email</b> 1979 * <p> 1980 * Description: <b>Multiple Resources: 1981 1982* [Patient](patient.html): A value in an email contact 1983* [Person](person.html): A value in an email contact 1984* [Practitioner](practitioner.html): A value in an email contact 1985* [PractitionerRole](practitionerrole.html): A value in an email contact 1986* [RelatedPerson](relatedperson.html): A value in an email contact 1987</b><br> 1988 * Type: <b>token</b><br> 1989 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 1990 * </p> 1991 */ 1992 @SearchParamDefinition(name="email", path="Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in an email contact\r\n* [Person](person.html): A value in an email contact\r\n* [Practitioner](practitioner.html): A value in an email contact\r\n* [PractitionerRole](practitionerrole.html): A value in an email contact\r\n* [RelatedPerson](relatedperson.html): A value in an email contact\r\n", type="token" ) 1993 public static final String SP_EMAIL = "email"; 1994 /** 1995 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1996 * <p> 1997 * Description: <b>Multiple Resources: 1998 1999* [Patient](patient.html): A value in an email contact 2000* [Person](person.html): A value in an email contact 2001* [Practitioner](practitioner.html): A value in an email contact 2002* [PractitionerRole](practitionerrole.html): A value in an email contact 2003* [RelatedPerson](relatedperson.html): A value in an email contact 2004</b><br> 2005 * Type: <b>token</b><br> 2006 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 2007 * </p> 2008 */ 2009 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 2010 2011 /** 2012 * Search parameter: <b>family</b> 2013 * <p> 2014 * Description: <b>Multiple Resources: 2015 2016* [Patient](patient.html): A portion of the family name of the patient 2017* [Practitioner](practitioner.html): A portion of the family name 2018</b><br> 2019 * Type: <b>string</b><br> 2020 * Path: <b>Patient.name.family | Practitioner.name.family</b><br> 2021 * </p> 2022 */ 2023 @SearchParamDefinition(name="family", path="Patient.name.family | Practitioner.name.family", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of the family name of the patient\r\n* [Practitioner](practitioner.html): A portion of the family name\r\n", type="string" ) 2024 public static final String SP_FAMILY = "family"; 2025 /** 2026 * <b>Fluent Client</b> search parameter constant for <b>family</b> 2027 * <p> 2028 * Description: <b>Multiple Resources: 2029 2030* [Patient](patient.html): A portion of the family name of the patient 2031* [Practitioner](practitioner.html): A portion of the family name 2032</b><br> 2033 * Type: <b>string</b><br> 2034 * Path: <b>Patient.name.family | Practitioner.name.family</b><br> 2035 * </p> 2036 */ 2037 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_FAMILY); 2038 2039 /** 2040 * Search parameter: <b>gender</b> 2041 * <p> 2042 * Description: <b>Multiple Resources: 2043 2044* [Patient](patient.html): Gender of the patient 2045* [Person](person.html): The gender of the person 2046* [Practitioner](practitioner.html): Gender of the practitioner 2047* [RelatedPerson](relatedperson.html): Gender of the related person 2048</b><br> 2049 * Type: <b>token</b><br> 2050 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 2051 * </p> 2052 */ 2053 @SearchParamDefinition(name="gender", path="Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): Gender of the patient\r\n* [Person](person.html): The gender of the person\r\n* [Practitioner](practitioner.html): Gender of the practitioner\r\n* [RelatedPerson](relatedperson.html): Gender of the related person\r\n", type="token" ) 2054 public static final String SP_GENDER = "gender"; 2055 /** 2056 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 2057 * <p> 2058 * Description: <b>Multiple Resources: 2059 2060* [Patient](patient.html): Gender of the patient 2061* [Person](person.html): The gender of the person 2062* [Practitioner](practitioner.html): Gender of the practitioner 2063* [RelatedPerson](relatedperson.html): Gender of the related person 2064</b><br> 2065 * Type: <b>token</b><br> 2066 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 2067 * </p> 2068 */ 2069 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 2070 2071 /** 2072 * Search parameter: <b>given</b> 2073 * <p> 2074 * Description: <b>Multiple Resources: 2075 2076* [Patient](patient.html): A portion of the given name of the patient 2077* [Practitioner](practitioner.html): A portion of the given name 2078</b><br> 2079 * Type: <b>string</b><br> 2080 * Path: <b>Patient.name.given | Practitioner.name.given</b><br> 2081 * </p> 2082 */ 2083 @SearchParamDefinition(name="given", path="Patient.name.given | Practitioner.name.given", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of the given name of the patient\r\n* [Practitioner](practitioner.html): A portion of the given name\r\n", type="string" ) 2084 public static final String SP_GIVEN = "given"; 2085 /** 2086 * <b>Fluent Client</b> search parameter constant for <b>given</b> 2087 * <p> 2088 * Description: <b>Multiple Resources: 2089 2090* [Patient](patient.html): A portion of the given name of the patient 2091* [Practitioner](practitioner.html): A portion of the given name 2092</b><br> 2093 * Type: <b>string</b><br> 2094 * Path: <b>Patient.name.given | Practitioner.name.given</b><br> 2095 * </p> 2096 */ 2097 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GIVEN); 2098 2099 /** 2100 * Search parameter: <b>phone</b> 2101 * <p> 2102 * Description: <b>Multiple Resources: 2103 2104* [Patient](patient.html): A value in a phone contact 2105* [Person](person.html): A value in a phone contact 2106* [Practitioner](practitioner.html): A value in a phone contact 2107* [PractitionerRole](practitionerrole.html): A value in a phone contact 2108* [RelatedPerson](relatedperson.html): A value in a phone contact 2109</b><br> 2110 * Type: <b>token</b><br> 2111 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 2112 * </p> 2113 */ 2114 @SearchParamDefinition(name="phone", path="Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in a phone contact\r\n* [Person](person.html): A value in a phone contact\r\n* [Practitioner](practitioner.html): A value in a phone contact\r\n* [PractitionerRole](practitionerrole.html): A value in a phone contact\r\n* [RelatedPerson](relatedperson.html): A value in a phone contact\r\n", type="token" ) 2115 public static final String SP_PHONE = "phone"; 2116 /** 2117 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 2118 * <p> 2119 * Description: <b>Multiple Resources: 2120 2121* [Patient](patient.html): A value in a phone contact 2122* [Person](person.html): A value in a phone contact 2123* [Practitioner](practitioner.html): A value in a phone contact 2124* [PractitionerRole](practitionerrole.html): A value in a phone contact 2125* [RelatedPerson](relatedperson.html): A value in a phone contact 2126</b><br> 2127 * Type: <b>token</b><br> 2128 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 2129 * </p> 2130 */ 2131 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 2132 2133 /** 2134 * Search parameter: <b>phonetic</b> 2135 * <p> 2136 * Description: <b>Multiple Resources: 2137 2138* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 2139* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 2140* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 2141* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 2142</b><br> 2143 * Type: <b>string</b><br> 2144 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 2145 * </p> 2146 */ 2147 @SearchParamDefinition(name="phonetic", path="Patient.name | Person.name | Practitioner.name | RelatedPerson.name", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [Person](person.html): A portion of name using some kind of phonetic matching algorithm\r\n* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm\r\n", type="string" ) 2148 public static final String SP_PHONETIC = "phonetic"; 2149 /** 2150 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 2151 * <p> 2152 * Description: <b>Multiple Resources: 2153 2154* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 2155* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 2156* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 2157* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 2158</b><br> 2159 * Type: <b>string</b><br> 2160 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 2161 * </p> 2162 */ 2163 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 2164 2165 /** 2166 * Search parameter: <b>telecom</b> 2167 * <p> 2168 * Description: <b>Multiple Resources: 2169 2170* [Patient](patient.html): The value in any kind of telecom details of the patient 2171* [Person](person.html): The value in any kind of contact 2172* [Practitioner](practitioner.html): The value in any kind of contact 2173* [PractitionerRole](practitionerrole.html): The value in any kind of contact 2174* [RelatedPerson](relatedperson.html): The value in any kind of contact 2175</b><br> 2176 * Type: <b>token</b><br> 2177 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 2178 * </p> 2179 */ 2180 @SearchParamDefinition(name="telecom", path="Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The value in any kind of telecom details of the patient\r\n* [Person](person.html): The value in any kind of contact\r\n* [Practitioner](practitioner.html): The value in any kind of contact\r\n* [PractitionerRole](practitionerrole.html): The value in any kind of contact\r\n* [RelatedPerson](relatedperson.html): The value in any kind of contact\r\n", type="token" ) 2181 public static final String SP_TELECOM = "telecom"; 2182 /** 2183 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2184 * <p> 2185 * Description: <b>Multiple Resources: 2186 2187* [Patient](patient.html): The value in any kind of telecom details of the patient 2188* [Person](person.html): The value in any kind of contact 2189* [Practitioner](practitioner.html): The value in any kind of contact 2190* [PractitionerRole](practitionerrole.html): The value in any kind of contact 2191* [RelatedPerson](relatedperson.html): The value in any kind of contact 2192</b><br> 2193 * Type: <b>token</b><br> 2194 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 2195 * </p> 2196 */ 2197 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 2198 2199 2200} 2201