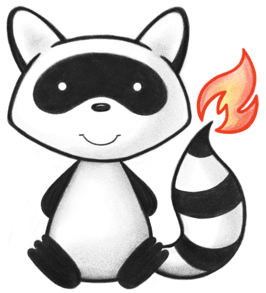
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * A specific set of Roles/Locations/specialties/services that a practitioner may perform, or has performed at an organization during a period of time. 051 */ 052@ResourceDef(name="PractitionerRole", profile="http://hl7.org/fhir/StructureDefinition/PractitionerRole") 053public class PractitionerRole extends DomainResource { 054 055 /** 056 * Business Identifiers that are specific to a role/location. 057 */ 058 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 059 @Description(shortDefinition="Identifiers for a role/location", formalDefinition="Business Identifiers that are specific to a role/location." ) 060 protected List<Identifier> identifier; 061 062 /** 063 * Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed. 064 */ 065 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="Whether this practitioner role record is in active use", formalDefinition=" Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed." ) 067 protected BooleanType active; 068 069 /** 070 * The period during which the person is authorized to act as a practitioner in these role(s) for the organization. 071 */ 072 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 073 @Description(shortDefinition="The period during which the practitioner is authorized to perform in these role(s)", formalDefinition="The period during which the person is authorized to act as a practitioner in these role(s) for the organization." ) 074 protected Period period; 075 076 /** 077 * Practitioner that is able to provide the defined services for the organization. 078 */ 079 @Child(name = "practitioner", type = {Practitioner.class}, order=3, min=0, max=1, modifier=false, summary=true) 080 @Description(shortDefinition="Practitioner that provides services for the organization", formalDefinition="Practitioner that is able to provide the defined services for the organization." ) 081 protected Reference practitioner; 082 083 /** 084 * The organization where the Practitioner performs the roles associated. 085 */ 086 @Child(name = "organization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 087 @Description(shortDefinition="Organization where the roles are available", formalDefinition="The organization where the Practitioner performs the roles associated." ) 088 protected Reference organization; 089 090 /** 091 * Roles which this practitioner is authorized to perform for the organization. 092 */ 093 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 094 @Description(shortDefinition="Roles which this practitioner may perform", formalDefinition="Roles which this practitioner is authorized to perform for the organization." ) 095 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/practitioner-role") 096 protected List<CodeableConcept> code; 097 098 /** 099 * The specialty of a practitioner that describes the functional role they are practicing at a given organization or location. 100 */ 101 @Child(name = "specialty", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 102 @Description(shortDefinition="Specific specialty of the practitioner", formalDefinition="The specialty of a practitioner that describes the functional role they are practicing at a given organization or location." ) 103 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 104 protected List<CodeableConcept> specialty; 105 106 /** 107 * The location(s) at which this practitioner provides care. 108 */ 109 @Child(name = "location", type = {Location.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 110 @Description(shortDefinition="Location(s) where the practitioner provides care", formalDefinition="The location(s) at which this practitioner provides care." ) 111 protected List<Reference> location; 112 113 /** 114 * The list of healthcare services that this worker provides for this role's Organization/Location(s). 115 */ 116 @Child(name = "healthcareService", type = {HealthcareService.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 117 @Description(shortDefinition="Healthcare services provided for this role's Organization/Location(s)", formalDefinition="The list of healthcare services that this worker provides for this role's Organization/Location(s)." ) 118 protected List<Reference> healthcareService; 119 120 /** 121 * The contact details of communication devices available relevant to the specific PractitionerRole. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites. 122 */ 123 @Child(name = "contact", type = {ExtendedContactDetail.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 124 @Description(shortDefinition="Official contact details relating to this PractitionerRole", formalDefinition="The contact details of communication devices available relevant to the specific PractitionerRole. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites." ) 125 protected List<ExtendedContactDetail> contact; 126 127 /** 128 * Collection of characteristics (attributes). 129 */ 130 @Child(name = "characteristic", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 131 @Description(shortDefinition="Collection of characteristics (attributes)", formalDefinition="Collection of characteristics (attributes)." ) 132 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-mode") 133 protected List<CodeableConcept> characteristic; 134 135 /** 136 * A language the practitioner can use in patient communication. The practitioner may know several languages (listed in practitioner.communication), however these are the languages that could be advertised in a directory for a patient to search. 137 */ 138 @Child(name = "communication", type = {CodeableConcept.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 139 @Description(shortDefinition="A language the practitioner (in this role) can use in patient communication", formalDefinition="A language the practitioner can use in patient communication. The practitioner may know several languages (listed in practitioner.communication), however these are the languages that could be advertised in a directory for a patient to search." ) 140 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 141 protected List<CodeableConcept> communication; 142 143 /** 144 * A collection of times the practitioner is available or performing this role at the location and/or healthcareservice. 145 */ 146 @Child(name = "availability", type = {Availability.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 147 @Description(shortDefinition="Times the Practitioner is available at this location and/or healthcare service (including exceptions)", formalDefinition="A collection of times the practitioner is available or performing this role at the location and/or healthcareservice." ) 148 protected List<Availability> availability; 149 150 /** 151 * Technical endpoints providing access to services operated for the practitioner with this role. Commonly used for locating scheduling services, or identifying where to send referrals electronically. 152 */ 153 @Child(name = "endpoint", type = {Endpoint.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 154 @Description(shortDefinition="Endpoints for interacting with the practitioner in this role", formalDefinition=" Technical endpoints providing access to services operated for the practitioner with this role. Commonly used for locating scheduling services, or identifying where to send referrals electronically." ) 155 protected List<Reference> endpoint; 156 157 private static final long serialVersionUID = 1286634270L; 158 159 /** 160 * Constructor 161 */ 162 public PractitionerRole() { 163 super(); 164 } 165 166 /** 167 * @return {@link #identifier} (Business Identifiers that are specific to a role/location.) 168 */ 169 public List<Identifier> getIdentifier() { 170 if (this.identifier == null) 171 this.identifier = new ArrayList<Identifier>(); 172 return this.identifier; 173 } 174 175 /** 176 * @return Returns a reference to <code>this</code> for easy method chaining 177 */ 178 public PractitionerRole setIdentifier(List<Identifier> theIdentifier) { 179 this.identifier = theIdentifier; 180 return this; 181 } 182 183 public boolean hasIdentifier() { 184 if (this.identifier == null) 185 return false; 186 for (Identifier item : this.identifier) 187 if (!item.isEmpty()) 188 return true; 189 return false; 190 } 191 192 public Identifier addIdentifier() { //3 193 Identifier t = new Identifier(); 194 if (this.identifier == null) 195 this.identifier = new ArrayList<Identifier>(); 196 this.identifier.add(t); 197 return t; 198 } 199 200 public PractitionerRole addIdentifier(Identifier t) { //3 201 if (t == null) 202 return this; 203 if (this.identifier == null) 204 this.identifier = new ArrayList<Identifier>(); 205 this.identifier.add(t); 206 return this; 207 } 208 209 /** 210 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 211 */ 212 public Identifier getIdentifierFirstRep() { 213 if (getIdentifier().isEmpty()) { 214 addIdentifier(); 215 } 216 return getIdentifier().get(0); 217 } 218 219 /** 220 * @return {@link #active} ( Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 221 */ 222 public BooleanType getActiveElement() { 223 if (this.active == null) 224 if (Configuration.errorOnAutoCreate()) 225 throw new Error("Attempt to auto-create PractitionerRole.active"); 226 else if (Configuration.doAutoCreate()) 227 this.active = new BooleanType(); // bb 228 return this.active; 229 } 230 231 public boolean hasActiveElement() { 232 return this.active != null && !this.active.isEmpty(); 233 } 234 235 public boolean hasActive() { 236 return this.active != null && !this.active.isEmpty(); 237 } 238 239 /** 240 * @param value {@link #active} ( Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 241 */ 242 public PractitionerRole setActiveElement(BooleanType value) { 243 this.active = value; 244 return this; 245 } 246 247 /** 248 * @return Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed. 249 */ 250 public boolean getActive() { 251 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 252 } 253 254 /** 255 * @param value Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed. 256 */ 257 public PractitionerRole setActive(boolean value) { 258 if (this.active == null) 259 this.active = new BooleanType(); 260 this.active.setValue(value); 261 return this; 262 } 263 264 /** 265 * @return {@link #period} (The period during which the person is authorized to act as a practitioner in these role(s) for the organization.) 266 */ 267 public Period getPeriod() { 268 if (this.period == null) 269 if (Configuration.errorOnAutoCreate()) 270 throw new Error("Attempt to auto-create PractitionerRole.period"); 271 else if (Configuration.doAutoCreate()) 272 this.period = new Period(); // cc 273 return this.period; 274 } 275 276 public boolean hasPeriod() { 277 return this.period != null && !this.period.isEmpty(); 278 } 279 280 /** 281 * @param value {@link #period} (The period during which the person is authorized to act as a practitioner in these role(s) for the organization.) 282 */ 283 public PractitionerRole setPeriod(Period value) { 284 this.period = value; 285 return this; 286 } 287 288 /** 289 * @return {@link #practitioner} (Practitioner that is able to provide the defined services for the organization.) 290 */ 291 public Reference getPractitioner() { 292 if (this.practitioner == null) 293 if (Configuration.errorOnAutoCreate()) 294 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 295 else if (Configuration.doAutoCreate()) 296 this.practitioner = new Reference(); // cc 297 return this.practitioner; 298 } 299 300 public boolean hasPractitioner() { 301 return this.practitioner != null && !this.practitioner.isEmpty(); 302 } 303 304 /** 305 * @param value {@link #practitioner} (Practitioner that is able to provide the defined services for the organization.) 306 */ 307 public PractitionerRole setPractitioner(Reference value) { 308 this.practitioner = value; 309 return this; 310 } 311 312 /** 313 * @return {@link #organization} (The organization where the Practitioner performs the roles associated.) 314 */ 315 public Reference getOrganization() { 316 if (this.organization == null) 317 if (Configuration.errorOnAutoCreate()) 318 throw new Error("Attempt to auto-create PractitionerRole.organization"); 319 else if (Configuration.doAutoCreate()) 320 this.organization = new Reference(); // cc 321 return this.organization; 322 } 323 324 public boolean hasOrganization() { 325 return this.organization != null && !this.organization.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #organization} (The organization where the Practitioner performs the roles associated.) 330 */ 331 public PractitionerRole setOrganization(Reference value) { 332 this.organization = value; 333 return this; 334 } 335 336 /** 337 * @return {@link #code} (Roles which this practitioner is authorized to perform for the organization.) 338 */ 339 public List<CodeableConcept> getCode() { 340 if (this.code == null) 341 this.code = new ArrayList<CodeableConcept>(); 342 return this.code; 343 } 344 345 /** 346 * @return Returns a reference to <code>this</code> for easy method chaining 347 */ 348 public PractitionerRole setCode(List<CodeableConcept> theCode) { 349 this.code = theCode; 350 return this; 351 } 352 353 public boolean hasCode() { 354 if (this.code == null) 355 return false; 356 for (CodeableConcept item : this.code) 357 if (!item.isEmpty()) 358 return true; 359 return false; 360 } 361 362 public CodeableConcept addCode() { //3 363 CodeableConcept t = new CodeableConcept(); 364 if (this.code == null) 365 this.code = new ArrayList<CodeableConcept>(); 366 this.code.add(t); 367 return t; 368 } 369 370 public PractitionerRole addCode(CodeableConcept t) { //3 371 if (t == null) 372 return this; 373 if (this.code == null) 374 this.code = new ArrayList<CodeableConcept>(); 375 this.code.add(t); 376 return this; 377 } 378 379 /** 380 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 381 */ 382 public CodeableConcept getCodeFirstRep() { 383 if (getCode().isEmpty()) { 384 addCode(); 385 } 386 return getCode().get(0); 387 } 388 389 /** 390 * @return {@link #specialty} (The specialty of a practitioner that describes the functional role they are practicing at a given organization or location.) 391 */ 392 public List<CodeableConcept> getSpecialty() { 393 if (this.specialty == null) 394 this.specialty = new ArrayList<CodeableConcept>(); 395 return this.specialty; 396 } 397 398 /** 399 * @return Returns a reference to <code>this</code> for easy method chaining 400 */ 401 public PractitionerRole setSpecialty(List<CodeableConcept> theSpecialty) { 402 this.specialty = theSpecialty; 403 return this; 404 } 405 406 public boolean hasSpecialty() { 407 if (this.specialty == null) 408 return false; 409 for (CodeableConcept item : this.specialty) 410 if (!item.isEmpty()) 411 return true; 412 return false; 413 } 414 415 public CodeableConcept addSpecialty() { //3 416 CodeableConcept t = new CodeableConcept(); 417 if (this.specialty == null) 418 this.specialty = new ArrayList<CodeableConcept>(); 419 this.specialty.add(t); 420 return t; 421 } 422 423 public PractitionerRole addSpecialty(CodeableConcept t) { //3 424 if (t == null) 425 return this; 426 if (this.specialty == null) 427 this.specialty = new ArrayList<CodeableConcept>(); 428 this.specialty.add(t); 429 return this; 430 } 431 432 /** 433 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist {3} 434 */ 435 public CodeableConcept getSpecialtyFirstRep() { 436 if (getSpecialty().isEmpty()) { 437 addSpecialty(); 438 } 439 return getSpecialty().get(0); 440 } 441 442 /** 443 * @return {@link #location} (The location(s) at which this practitioner provides care.) 444 */ 445 public List<Reference> getLocation() { 446 if (this.location == null) 447 this.location = new ArrayList<Reference>(); 448 return this.location; 449 } 450 451 /** 452 * @return Returns a reference to <code>this</code> for easy method chaining 453 */ 454 public PractitionerRole setLocation(List<Reference> theLocation) { 455 this.location = theLocation; 456 return this; 457 } 458 459 public boolean hasLocation() { 460 if (this.location == null) 461 return false; 462 for (Reference item : this.location) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 public Reference addLocation() { //3 469 Reference t = new Reference(); 470 if (this.location == null) 471 this.location = new ArrayList<Reference>(); 472 this.location.add(t); 473 return t; 474 } 475 476 public PractitionerRole addLocation(Reference t) { //3 477 if (t == null) 478 return this; 479 if (this.location == null) 480 this.location = new ArrayList<Reference>(); 481 this.location.add(t); 482 return this; 483 } 484 485 /** 486 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist {3} 487 */ 488 public Reference getLocationFirstRep() { 489 if (getLocation().isEmpty()) { 490 addLocation(); 491 } 492 return getLocation().get(0); 493 } 494 495 /** 496 * @return {@link #healthcareService} (The list of healthcare services that this worker provides for this role's Organization/Location(s).) 497 */ 498 public List<Reference> getHealthcareService() { 499 if (this.healthcareService == null) 500 this.healthcareService = new ArrayList<Reference>(); 501 return this.healthcareService; 502 } 503 504 /** 505 * @return Returns a reference to <code>this</code> for easy method chaining 506 */ 507 public PractitionerRole setHealthcareService(List<Reference> theHealthcareService) { 508 this.healthcareService = theHealthcareService; 509 return this; 510 } 511 512 public boolean hasHealthcareService() { 513 if (this.healthcareService == null) 514 return false; 515 for (Reference item : this.healthcareService) 516 if (!item.isEmpty()) 517 return true; 518 return false; 519 } 520 521 public Reference addHealthcareService() { //3 522 Reference t = new Reference(); 523 if (this.healthcareService == null) 524 this.healthcareService = new ArrayList<Reference>(); 525 this.healthcareService.add(t); 526 return t; 527 } 528 529 public PractitionerRole addHealthcareService(Reference t) { //3 530 if (t == null) 531 return this; 532 if (this.healthcareService == null) 533 this.healthcareService = new ArrayList<Reference>(); 534 this.healthcareService.add(t); 535 return this; 536 } 537 538 /** 539 * @return The first repetition of repeating field {@link #healthcareService}, creating it if it does not already exist {3} 540 */ 541 public Reference getHealthcareServiceFirstRep() { 542 if (getHealthcareService().isEmpty()) { 543 addHealthcareService(); 544 } 545 return getHealthcareService().get(0); 546 } 547 548 /** 549 * @return {@link #contact} (The contact details of communication devices available relevant to the specific PractitionerRole. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.) 550 */ 551 public List<ExtendedContactDetail> getContact() { 552 if (this.contact == null) 553 this.contact = new ArrayList<ExtendedContactDetail>(); 554 return this.contact; 555 } 556 557 /** 558 * @return Returns a reference to <code>this</code> for easy method chaining 559 */ 560 public PractitionerRole setContact(List<ExtendedContactDetail> theContact) { 561 this.contact = theContact; 562 return this; 563 } 564 565 public boolean hasContact() { 566 if (this.contact == null) 567 return false; 568 for (ExtendedContactDetail item : this.contact) 569 if (!item.isEmpty()) 570 return true; 571 return false; 572 } 573 574 public ExtendedContactDetail addContact() { //3 575 ExtendedContactDetail t = new ExtendedContactDetail(); 576 if (this.contact == null) 577 this.contact = new ArrayList<ExtendedContactDetail>(); 578 this.contact.add(t); 579 return t; 580 } 581 582 public PractitionerRole addContact(ExtendedContactDetail t) { //3 583 if (t == null) 584 return this; 585 if (this.contact == null) 586 this.contact = new ArrayList<ExtendedContactDetail>(); 587 this.contact.add(t); 588 return this; 589 } 590 591 /** 592 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 593 */ 594 public ExtendedContactDetail getContactFirstRep() { 595 if (getContact().isEmpty()) { 596 addContact(); 597 } 598 return getContact().get(0); 599 } 600 601 /** 602 * @return {@link #characteristic} (Collection of characteristics (attributes).) 603 */ 604 public List<CodeableConcept> getCharacteristic() { 605 if (this.characteristic == null) 606 this.characteristic = new ArrayList<CodeableConcept>(); 607 return this.characteristic; 608 } 609 610 /** 611 * @return Returns a reference to <code>this</code> for easy method chaining 612 */ 613 public PractitionerRole setCharacteristic(List<CodeableConcept> theCharacteristic) { 614 this.characteristic = theCharacteristic; 615 return this; 616 } 617 618 public boolean hasCharacteristic() { 619 if (this.characteristic == null) 620 return false; 621 for (CodeableConcept item : this.characteristic) 622 if (!item.isEmpty()) 623 return true; 624 return false; 625 } 626 627 public CodeableConcept addCharacteristic() { //3 628 CodeableConcept t = new CodeableConcept(); 629 if (this.characteristic == null) 630 this.characteristic = new ArrayList<CodeableConcept>(); 631 this.characteristic.add(t); 632 return t; 633 } 634 635 public PractitionerRole addCharacteristic(CodeableConcept t) { //3 636 if (t == null) 637 return this; 638 if (this.characteristic == null) 639 this.characteristic = new ArrayList<CodeableConcept>(); 640 this.characteristic.add(t); 641 return this; 642 } 643 644 /** 645 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 646 */ 647 public CodeableConcept getCharacteristicFirstRep() { 648 if (getCharacteristic().isEmpty()) { 649 addCharacteristic(); 650 } 651 return getCharacteristic().get(0); 652 } 653 654 /** 655 * @return {@link #communication} (A language the practitioner can use in patient communication. The practitioner may know several languages (listed in practitioner.communication), however these are the languages that could be advertised in a directory for a patient to search.) 656 */ 657 public List<CodeableConcept> getCommunication() { 658 if (this.communication == null) 659 this.communication = new ArrayList<CodeableConcept>(); 660 return this.communication; 661 } 662 663 /** 664 * @return Returns a reference to <code>this</code> for easy method chaining 665 */ 666 public PractitionerRole setCommunication(List<CodeableConcept> theCommunication) { 667 this.communication = theCommunication; 668 return this; 669 } 670 671 public boolean hasCommunication() { 672 if (this.communication == null) 673 return false; 674 for (CodeableConcept item : this.communication) 675 if (!item.isEmpty()) 676 return true; 677 return false; 678 } 679 680 public CodeableConcept addCommunication() { //3 681 CodeableConcept t = new CodeableConcept(); 682 if (this.communication == null) 683 this.communication = new ArrayList<CodeableConcept>(); 684 this.communication.add(t); 685 return t; 686 } 687 688 public PractitionerRole addCommunication(CodeableConcept t) { //3 689 if (t == null) 690 return this; 691 if (this.communication == null) 692 this.communication = new ArrayList<CodeableConcept>(); 693 this.communication.add(t); 694 return this; 695 } 696 697 /** 698 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist {3} 699 */ 700 public CodeableConcept getCommunicationFirstRep() { 701 if (getCommunication().isEmpty()) { 702 addCommunication(); 703 } 704 return getCommunication().get(0); 705 } 706 707 /** 708 * @return {@link #availability} (A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.) 709 */ 710 public List<Availability> getAvailability() { 711 if (this.availability == null) 712 this.availability = new ArrayList<Availability>(); 713 return this.availability; 714 } 715 716 /** 717 * @return Returns a reference to <code>this</code> for easy method chaining 718 */ 719 public PractitionerRole setAvailability(List<Availability> theAvailability) { 720 this.availability = theAvailability; 721 return this; 722 } 723 724 public boolean hasAvailability() { 725 if (this.availability == null) 726 return false; 727 for (Availability item : this.availability) 728 if (!item.isEmpty()) 729 return true; 730 return false; 731 } 732 733 public Availability addAvailability() { //3 734 Availability t = new Availability(); 735 if (this.availability == null) 736 this.availability = new ArrayList<Availability>(); 737 this.availability.add(t); 738 return t; 739 } 740 741 public PractitionerRole addAvailability(Availability t) { //3 742 if (t == null) 743 return this; 744 if (this.availability == null) 745 this.availability = new ArrayList<Availability>(); 746 this.availability.add(t); 747 return this; 748 } 749 750 /** 751 * @return The first repetition of repeating field {@link #availability}, creating it if it does not already exist {3} 752 */ 753 public Availability getAvailabilityFirstRep() { 754 if (getAvailability().isEmpty()) { 755 addAvailability(); 756 } 757 return getAvailability().get(0); 758 } 759 760 /** 761 * @return {@link #endpoint} ( Technical endpoints providing access to services operated for the practitioner with this role. Commonly used for locating scheduling services, or identifying where to send referrals electronically.) 762 */ 763 public List<Reference> getEndpoint() { 764 if (this.endpoint == null) 765 this.endpoint = new ArrayList<Reference>(); 766 return this.endpoint; 767 } 768 769 /** 770 * @return Returns a reference to <code>this</code> for easy method chaining 771 */ 772 public PractitionerRole setEndpoint(List<Reference> theEndpoint) { 773 this.endpoint = theEndpoint; 774 return this; 775 } 776 777 public boolean hasEndpoint() { 778 if (this.endpoint == null) 779 return false; 780 for (Reference item : this.endpoint) 781 if (!item.isEmpty()) 782 return true; 783 return false; 784 } 785 786 public Reference addEndpoint() { //3 787 Reference t = new Reference(); 788 if (this.endpoint == null) 789 this.endpoint = new ArrayList<Reference>(); 790 this.endpoint.add(t); 791 return t; 792 } 793 794 public PractitionerRole addEndpoint(Reference t) { //3 795 if (t == null) 796 return this; 797 if (this.endpoint == null) 798 this.endpoint = new ArrayList<Reference>(); 799 this.endpoint.add(t); 800 return this; 801 } 802 803 /** 804 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 805 */ 806 public Reference getEndpointFirstRep() { 807 if (getEndpoint().isEmpty()) { 808 addEndpoint(); 809 } 810 return getEndpoint().get(0); 811 } 812 813 protected void listChildren(List<Property> children) { 814 super.listChildren(children); 815 children.add(new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier)); 816 children.add(new Property("active", "boolean", " Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed.", 0, 1, active)); 817 children.add(new Property("period", "Period", "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 0, 1, period)); 818 children.add(new Property("practitioner", "Reference(Practitioner)", "Practitioner that is able to provide the defined services for the organization.", 0, 1, practitioner)); 819 children.add(new Property("organization", "Reference(Organization)", "The organization where the Practitioner performs the roles associated.", 0, 1, organization)); 820 children.add(new Property("code", "CodeableConcept", "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, code)); 821 children.add(new Property("specialty", "CodeableConcept", "The specialty of a practitioner that describes the functional role they are practicing at a given organization or location.", 0, java.lang.Integer.MAX_VALUE, specialty)); 822 children.add(new Property("location", "Reference(Location)", "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location)); 823 children.add(new Property("healthcareService", "Reference(HealthcareService)", "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, java.lang.Integer.MAX_VALUE, healthcareService)); 824 children.add(new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific PractitionerRole. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact)); 825 children.add(new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic)); 826 children.add(new Property("communication", "CodeableConcept", "A language the practitioner can use in patient communication. The practitioner may know several languages (listed in practitioner.communication), however these are the languages that could be advertised in a directory for a patient to search.", 0, java.lang.Integer.MAX_VALUE, communication)); 827 children.add(new Property("availability", "Availability", "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.", 0, java.lang.Integer.MAX_VALUE, availability)); 828 children.add(new Property("endpoint", "Reference(Endpoint)", " Technical endpoints providing access to services operated for the practitioner with this role. Commonly used for locating scheduling services, or identifying where to send referrals electronically.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 829 } 830 831 @Override 832 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 833 switch (_hash) { 834 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier); 835 case -1422950650: /*active*/ return new Property("active", "boolean", " Whether this practitioner role record is in active use. Some systems may use this property to mark non-active practitioners, such as those that are not currently employed.", 0, 1, active); 836 case -991726143: /*period*/ return new Property("period", "Period", "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 0, 1, period); 837 case 574573338: /*practitioner*/ return new Property("practitioner", "Reference(Practitioner)", "Practitioner that is able to provide the defined services for the organization.", 0, 1, practitioner); 838 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization where the Practitioner performs the roles associated.", 0, 1, organization); 839 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, code); 840 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialty of a practitioner that describes the functional role they are practicing at a given organization or location.", 0, java.lang.Integer.MAX_VALUE, specialty); 841 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location); 842 case 1289661064: /*healthcareService*/ return new Property("healthcareService", "Reference(HealthcareService)", "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, java.lang.Integer.MAX_VALUE, healthcareService); 843 case 951526432: /*contact*/ return new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific PractitionerRole. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact); 844 case 366313883: /*characteristic*/ return new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic); 845 case -1035284522: /*communication*/ return new Property("communication", "CodeableConcept", "A language the practitioner can use in patient communication. The practitioner may know several languages (listed in practitioner.communication), however these are the languages that could be advertised in a directory for a patient to search.", 0, java.lang.Integer.MAX_VALUE, communication); 846 case 1997542747: /*availability*/ return new Property("availability", "Availability", "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.", 0, java.lang.Integer.MAX_VALUE, availability); 847 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", " Technical endpoints providing access to services operated for the practitioner with this role. Commonly used for locating scheduling services, or identifying where to send referrals electronically.", 0, java.lang.Integer.MAX_VALUE, endpoint); 848 default: return super.getNamedProperty(_hash, _name, _checkValid); 849 } 850 851 } 852 853 @Override 854 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 855 switch (hash) { 856 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 857 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 858 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 859 case 574573338: /*practitioner*/ return this.practitioner == null ? new Base[0] : new Base[] {this.practitioner}; // Reference 860 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 861 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 862 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 863 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 864 case 1289661064: /*healthcareService*/ return this.healthcareService == null ? new Base[0] : this.healthcareService.toArray(new Base[this.healthcareService.size()]); // Reference 865 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ExtendedContactDetail 866 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // CodeableConcept 867 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // CodeableConcept 868 case 1997542747: /*availability*/ return this.availability == null ? new Base[0] : this.availability.toArray(new Base[this.availability.size()]); // Availability 869 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 870 default: return super.getProperty(hash, name, checkValid); 871 } 872 873 } 874 875 @Override 876 public Base setProperty(int hash, String name, Base value) throws FHIRException { 877 switch (hash) { 878 case -1618432855: // identifier 879 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 880 return value; 881 case -1422950650: // active 882 this.active = TypeConvertor.castToBoolean(value); // BooleanType 883 return value; 884 case -991726143: // period 885 this.period = TypeConvertor.castToPeriod(value); // Period 886 return value; 887 case 574573338: // practitioner 888 this.practitioner = TypeConvertor.castToReference(value); // Reference 889 return value; 890 case 1178922291: // organization 891 this.organization = TypeConvertor.castToReference(value); // Reference 892 return value; 893 case 3059181: // code 894 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 895 return value; 896 case -1694759682: // specialty 897 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 898 return value; 899 case 1901043637: // location 900 this.getLocation().add(TypeConvertor.castToReference(value)); // Reference 901 return value; 902 case 1289661064: // healthcareService 903 this.getHealthcareService().add(TypeConvertor.castToReference(value)); // Reference 904 return value; 905 case 951526432: // contact 906 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); // ExtendedContactDetail 907 return value; 908 case 366313883: // characteristic 909 this.getCharacteristic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 910 return value; 911 case -1035284522: // communication 912 this.getCommunication().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 913 return value; 914 case 1997542747: // availability 915 this.getAvailability().add(TypeConvertor.castToAvailability(value)); // Availability 916 return value; 917 case 1741102485: // endpoint 918 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 919 return value; 920 default: return super.setProperty(hash, name, value); 921 } 922 923 } 924 925 @Override 926 public Base setProperty(String name, Base value) throws FHIRException { 927 if (name.equals("identifier")) { 928 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 929 } else if (name.equals("active")) { 930 this.active = TypeConvertor.castToBoolean(value); // BooleanType 931 } else if (name.equals("period")) { 932 this.period = TypeConvertor.castToPeriod(value); // Period 933 } else if (name.equals("practitioner")) { 934 this.practitioner = TypeConvertor.castToReference(value); // Reference 935 } else if (name.equals("organization")) { 936 this.organization = TypeConvertor.castToReference(value); // Reference 937 } else if (name.equals("code")) { 938 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 939 } else if (name.equals("specialty")) { 940 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); 941 } else if (name.equals("location")) { 942 this.getLocation().add(TypeConvertor.castToReference(value)); 943 } else if (name.equals("healthcareService")) { 944 this.getHealthcareService().add(TypeConvertor.castToReference(value)); 945 } else if (name.equals("contact")) { 946 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); 947 } else if (name.equals("characteristic")) { 948 this.getCharacteristic().add(TypeConvertor.castToCodeableConcept(value)); 949 } else if (name.equals("communication")) { 950 this.getCommunication().add(TypeConvertor.castToCodeableConcept(value)); 951 } else if (name.equals("availability")) { 952 this.getAvailability().add(TypeConvertor.castToAvailability(value)); 953 } else if (name.equals("endpoint")) { 954 this.getEndpoint().add(TypeConvertor.castToReference(value)); 955 } else 956 return super.setProperty(name, value); 957 return value; 958 } 959 960 @Override 961 public void removeChild(String name, Base value) throws FHIRException { 962 if (name.equals("identifier")) { 963 this.getIdentifier().remove(value); 964 } else if (name.equals("active")) { 965 this.active = null; 966 } else if (name.equals("period")) { 967 this.period = null; 968 } else if (name.equals("practitioner")) { 969 this.practitioner = null; 970 } else if (name.equals("organization")) { 971 this.organization = null; 972 } else if (name.equals("code")) { 973 this.getCode().remove(value); 974 } else if (name.equals("specialty")) { 975 this.getSpecialty().remove(value); 976 } else if (name.equals("location")) { 977 this.getLocation().remove(value); 978 } else if (name.equals("healthcareService")) { 979 this.getHealthcareService().remove(value); 980 } else if (name.equals("contact")) { 981 this.getContact().remove(value); 982 } else if (name.equals("characteristic")) { 983 this.getCharacteristic().remove(value); 984 } else if (name.equals("communication")) { 985 this.getCommunication().remove(value); 986 } else if (name.equals("availability")) { 987 this.getAvailability().remove(value); 988 } else if (name.equals("endpoint")) { 989 this.getEndpoint().remove(value); 990 } else 991 super.removeChild(name, value); 992 993 } 994 995 @Override 996 public Base makeProperty(int hash, String name) throws FHIRException { 997 switch (hash) { 998 case -1618432855: return addIdentifier(); 999 case -1422950650: return getActiveElement(); 1000 case -991726143: return getPeriod(); 1001 case 574573338: return getPractitioner(); 1002 case 1178922291: return getOrganization(); 1003 case 3059181: return addCode(); 1004 case -1694759682: return addSpecialty(); 1005 case 1901043637: return addLocation(); 1006 case 1289661064: return addHealthcareService(); 1007 case 951526432: return addContact(); 1008 case 366313883: return addCharacteristic(); 1009 case -1035284522: return addCommunication(); 1010 case 1997542747: return addAvailability(); 1011 case 1741102485: return addEndpoint(); 1012 default: return super.makeProperty(hash, name); 1013 } 1014 1015 } 1016 1017 @Override 1018 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1019 switch (hash) { 1020 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1021 case -1422950650: /*active*/ return new String[] {"boolean"}; 1022 case -991726143: /*period*/ return new String[] {"Period"}; 1023 case 574573338: /*practitioner*/ return new String[] {"Reference"}; 1024 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1025 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1026 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1027 case 1901043637: /*location*/ return new String[] {"Reference"}; 1028 case 1289661064: /*healthcareService*/ return new String[] {"Reference"}; 1029 case 951526432: /*contact*/ return new String[] {"ExtendedContactDetail"}; 1030 case 366313883: /*characteristic*/ return new String[] {"CodeableConcept"}; 1031 case -1035284522: /*communication*/ return new String[] {"CodeableConcept"}; 1032 case 1997542747: /*availability*/ return new String[] {"Availability"}; 1033 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1034 default: return super.getTypesForProperty(hash, name); 1035 } 1036 1037 } 1038 1039 @Override 1040 public Base addChild(String name) throws FHIRException { 1041 if (name.equals("identifier")) { 1042 return addIdentifier(); 1043 } 1044 else if (name.equals("active")) { 1045 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.active"); 1046 } 1047 else if (name.equals("period")) { 1048 this.period = new Period(); 1049 return this.period; 1050 } 1051 else if (name.equals("practitioner")) { 1052 this.practitioner = new Reference(); 1053 return this.practitioner; 1054 } 1055 else if (name.equals("organization")) { 1056 this.organization = new Reference(); 1057 return this.organization; 1058 } 1059 else if (name.equals("code")) { 1060 return addCode(); 1061 } 1062 else if (name.equals("specialty")) { 1063 return addSpecialty(); 1064 } 1065 else if (name.equals("location")) { 1066 return addLocation(); 1067 } 1068 else if (name.equals("healthcareService")) { 1069 return addHealthcareService(); 1070 } 1071 else if (name.equals("contact")) { 1072 return addContact(); 1073 } 1074 else if (name.equals("characteristic")) { 1075 return addCharacteristic(); 1076 } 1077 else if (name.equals("communication")) { 1078 return addCommunication(); 1079 } 1080 else if (name.equals("availability")) { 1081 return addAvailability(); 1082 } 1083 else if (name.equals("endpoint")) { 1084 return addEndpoint(); 1085 } 1086 else 1087 return super.addChild(name); 1088 } 1089 1090 public String fhirType() { 1091 return "PractitionerRole"; 1092 1093 } 1094 1095 public PractitionerRole copy() { 1096 PractitionerRole dst = new PractitionerRole(); 1097 copyValues(dst); 1098 return dst; 1099 } 1100 1101 public void copyValues(PractitionerRole dst) { 1102 super.copyValues(dst); 1103 if (identifier != null) { 1104 dst.identifier = new ArrayList<Identifier>(); 1105 for (Identifier i : identifier) 1106 dst.identifier.add(i.copy()); 1107 }; 1108 dst.active = active == null ? null : active.copy(); 1109 dst.period = period == null ? null : period.copy(); 1110 dst.practitioner = practitioner == null ? null : practitioner.copy(); 1111 dst.organization = organization == null ? null : organization.copy(); 1112 if (code != null) { 1113 dst.code = new ArrayList<CodeableConcept>(); 1114 for (CodeableConcept i : code) 1115 dst.code.add(i.copy()); 1116 }; 1117 if (specialty != null) { 1118 dst.specialty = new ArrayList<CodeableConcept>(); 1119 for (CodeableConcept i : specialty) 1120 dst.specialty.add(i.copy()); 1121 }; 1122 if (location != null) { 1123 dst.location = new ArrayList<Reference>(); 1124 for (Reference i : location) 1125 dst.location.add(i.copy()); 1126 }; 1127 if (healthcareService != null) { 1128 dst.healthcareService = new ArrayList<Reference>(); 1129 for (Reference i : healthcareService) 1130 dst.healthcareService.add(i.copy()); 1131 }; 1132 if (contact != null) { 1133 dst.contact = new ArrayList<ExtendedContactDetail>(); 1134 for (ExtendedContactDetail i : contact) 1135 dst.contact.add(i.copy()); 1136 }; 1137 if (characteristic != null) { 1138 dst.characteristic = new ArrayList<CodeableConcept>(); 1139 for (CodeableConcept i : characteristic) 1140 dst.characteristic.add(i.copy()); 1141 }; 1142 if (communication != null) { 1143 dst.communication = new ArrayList<CodeableConcept>(); 1144 for (CodeableConcept i : communication) 1145 dst.communication.add(i.copy()); 1146 }; 1147 if (availability != null) { 1148 dst.availability = new ArrayList<Availability>(); 1149 for (Availability i : availability) 1150 dst.availability.add(i.copy()); 1151 }; 1152 if (endpoint != null) { 1153 dst.endpoint = new ArrayList<Reference>(); 1154 for (Reference i : endpoint) 1155 dst.endpoint.add(i.copy()); 1156 }; 1157 } 1158 1159 protected PractitionerRole typedCopy() { 1160 return copy(); 1161 } 1162 1163 @Override 1164 public boolean equalsDeep(Base other_) { 1165 if (!super.equalsDeep(other_)) 1166 return false; 1167 if (!(other_ instanceof PractitionerRole)) 1168 return false; 1169 PractitionerRole o = (PractitionerRole) other_; 1170 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(period, o.period, true) 1171 && compareDeep(practitioner, o.practitioner, true) && compareDeep(organization, o.organization, true) 1172 && compareDeep(code, o.code, true) && compareDeep(specialty, o.specialty, true) && compareDeep(location, o.location, true) 1173 && compareDeep(healthcareService, o.healthcareService, true) && compareDeep(contact, o.contact, true) 1174 && compareDeep(characteristic, o.characteristic, true) && compareDeep(communication, o.communication, true) 1175 && compareDeep(availability, o.availability, true) && compareDeep(endpoint, o.endpoint, true); 1176 } 1177 1178 @Override 1179 public boolean equalsShallow(Base other_) { 1180 if (!super.equalsShallow(other_)) 1181 return false; 1182 if (!(other_ instanceof PractitionerRole)) 1183 return false; 1184 PractitionerRole o = (PractitionerRole) other_; 1185 return compareValues(active, o.active, true); 1186 } 1187 1188 public boolean isEmpty() { 1189 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, period 1190 , practitioner, organization, code, specialty, location, healthcareService, contact 1191 , characteristic, communication, availability, endpoint); 1192 } 1193 1194 @Override 1195 public ResourceType getResourceType() { 1196 return ResourceType.PractitionerRole; 1197 } 1198 1199 /** 1200 * Search parameter: <b>active</b> 1201 * <p> 1202 * Description: <b>Whether this practitioner role record is in active use</b><br> 1203 * Type: <b>token</b><br> 1204 * Path: <b>PractitionerRole.active</b><br> 1205 * </p> 1206 */ 1207 @SearchParamDefinition(name="active", path="PractitionerRole.active", description="Whether this practitioner role record is in active use", type="token" ) 1208 public static final String SP_ACTIVE = "active"; 1209 /** 1210 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1211 * <p> 1212 * Description: <b>Whether this practitioner role record is in active use</b><br> 1213 * Type: <b>token</b><br> 1214 * Path: <b>PractitionerRole.active</b><br> 1215 * </p> 1216 */ 1217 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1218 1219 /** 1220 * Search parameter: <b>characteristic</b> 1221 * <p> 1222 * Description: <b>One of the PractitionerRole's characteristics</b><br> 1223 * Type: <b>token</b><br> 1224 * Path: <b>PractitionerRole.characteristic</b><br> 1225 * </p> 1226 */ 1227 @SearchParamDefinition(name="characteristic", path="PractitionerRole.characteristic", description="One of the PractitionerRole's characteristics", type="token" ) 1228 public static final String SP_CHARACTERISTIC = "characteristic"; 1229 /** 1230 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 1231 * <p> 1232 * Description: <b>One of the PractitionerRole's characteristics</b><br> 1233 * Type: <b>token</b><br> 1234 * Path: <b>PractitionerRole.characteristic</b><br> 1235 * </p> 1236 */ 1237 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC); 1238 1239 /** 1240 * Search parameter: <b>communication</b> 1241 * <p> 1242 * Description: <b>One of the languages that the practitioner can communicate with</b><br> 1243 * Type: <b>token</b><br> 1244 * Path: <b>PractitionerRole.communication</b><br> 1245 * </p> 1246 */ 1247 @SearchParamDefinition(name="communication", path="PractitionerRole.communication", description="One of the languages that the practitioner can communicate with", type="token" ) 1248 public static final String SP_COMMUNICATION = "communication"; 1249 /** 1250 * <b>Fluent Client</b> search parameter constant for <b>communication</b> 1251 * <p> 1252 * Description: <b>One of the languages that the practitioner can communicate with</b><br> 1253 * Type: <b>token</b><br> 1254 * Path: <b>PractitionerRole.communication</b><br> 1255 * </p> 1256 */ 1257 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMMUNICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMMUNICATION); 1258 1259 /** 1260 * Search parameter: <b>date</b> 1261 * <p> 1262 * Description: <b>The period during which the practitioner is authorized to perform in these role(s)</b><br> 1263 * Type: <b>date</b><br> 1264 * Path: <b>PractitionerRole.period</b><br> 1265 * </p> 1266 */ 1267 @SearchParamDefinition(name="date", path="PractitionerRole.period", description="The period during which the practitioner is authorized to perform in these role(s)", type="date" ) 1268 public static final String SP_DATE = "date"; 1269 /** 1270 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1271 * <p> 1272 * Description: <b>The period during which the practitioner is authorized to perform in these role(s)</b><br> 1273 * Type: <b>date</b><br> 1274 * Path: <b>PractitionerRole.period</b><br> 1275 * </p> 1276 */ 1277 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1278 1279 /** 1280 * Search parameter: <b>endpoint</b> 1281 * <p> 1282 * Description: <b>Technical endpoints providing access to services operated for the practitioner with this role</b><br> 1283 * Type: <b>reference</b><br> 1284 * Path: <b>PractitionerRole.endpoint</b><br> 1285 * </p> 1286 */ 1287 @SearchParamDefinition(name="endpoint", path="PractitionerRole.endpoint", description="Technical endpoints providing access to services operated for the practitioner with this role", type="reference", target={Endpoint.class } ) 1288 public static final String SP_ENDPOINT = "endpoint"; 1289 /** 1290 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1291 * <p> 1292 * Description: <b>Technical endpoints providing access to services operated for the practitioner with this role</b><br> 1293 * Type: <b>reference</b><br> 1294 * Path: <b>PractitionerRole.endpoint</b><br> 1295 * </p> 1296 */ 1297 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 1298 1299/** 1300 * Constant for fluent queries to be used to add include statements. Specifies 1301 * the path value of "<b>PractitionerRole:endpoint</b>". 1302 */ 1303 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("PractitionerRole:endpoint").toLocked(); 1304 1305 /** 1306 * Search parameter: <b>identifier</b> 1307 * <p> 1308 * Description: <b>A practitioner's Identifier</b><br> 1309 * Type: <b>token</b><br> 1310 * Path: <b>PractitionerRole.identifier</b><br> 1311 * </p> 1312 */ 1313 @SearchParamDefinition(name="identifier", path="PractitionerRole.identifier", description="A practitioner's Identifier", type="token" ) 1314 public static final String SP_IDENTIFIER = "identifier"; 1315 /** 1316 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1317 * <p> 1318 * Description: <b>A practitioner's Identifier</b><br> 1319 * Type: <b>token</b><br> 1320 * Path: <b>PractitionerRole.identifier</b><br> 1321 * </p> 1322 */ 1323 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1324 1325 /** 1326 * Search parameter: <b>location</b> 1327 * <p> 1328 * Description: <b>One of the locations at which this practitioner provides care</b><br> 1329 * Type: <b>reference</b><br> 1330 * Path: <b>PractitionerRole.location</b><br> 1331 * </p> 1332 */ 1333 @SearchParamDefinition(name="location", path="PractitionerRole.location", description="One of the locations at which this practitioner provides care", type="reference", target={Location.class } ) 1334 public static final String SP_LOCATION = "location"; 1335 /** 1336 * <b>Fluent Client</b> search parameter constant for <b>location</b> 1337 * <p> 1338 * Description: <b>One of the locations at which this practitioner provides care</b><br> 1339 * Type: <b>reference</b><br> 1340 * Path: <b>PractitionerRole.location</b><br> 1341 * </p> 1342 */ 1343 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 1344 1345/** 1346 * Constant for fluent queries to be used to add include statements. Specifies 1347 * the path value of "<b>PractitionerRole:location</b>". 1348 */ 1349 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("PractitionerRole:location").toLocked(); 1350 1351 /** 1352 * Search parameter: <b>organization</b> 1353 * <p> 1354 * Description: <b>The identity of the organization the practitioner represents / acts on behalf of</b><br> 1355 * Type: <b>reference</b><br> 1356 * Path: <b>PractitionerRole.organization</b><br> 1357 * </p> 1358 */ 1359 @SearchParamDefinition(name="organization", path="PractitionerRole.organization", description="The identity of the organization the practitioner represents / acts on behalf of", type="reference", target={Organization.class } ) 1360 public static final String SP_ORGANIZATION = "organization"; 1361 /** 1362 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1363 * <p> 1364 * Description: <b>The identity of the organization the practitioner represents / acts on behalf of</b><br> 1365 * Type: <b>reference</b><br> 1366 * Path: <b>PractitionerRole.organization</b><br> 1367 * </p> 1368 */ 1369 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1370 1371/** 1372 * Constant for fluent queries to be used to add include statements. Specifies 1373 * the path value of "<b>PractitionerRole:organization</b>". 1374 */ 1375 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("PractitionerRole:organization").toLocked(); 1376 1377 /** 1378 * Search parameter: <b>practitioner</b> 1379 * <p> 1380 * Description: <b>Practitioner that is able to provide the defined services for the organization</b><br> 1381 * Type: <b>reference</b><br> 1382 * Path: <b>PractitionerRole.practitioner</b><br> 1383 * </p> 1384 */ 1385 @SearchParamDefinition(name="practitioner", path="PractitionerRole.practitioner", description="Practitioner that is able to provide the defined services for the organization", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class } ) 1386 public static final String SP_PRACTITIONER = "practitioner"; 1387 /** 1388 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 1389 * <p> 1390 * Description: <b>Practitioner that is able to provide the defined services for the organization</b><br> 1391 * Type: <b>reference</b><br> 1392 * Path: <b>PractitionerRole.practitioner</b><br> 1393 * </p> 1394 */ 1395 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 1396 1397/** 1398 * Constant for fluent queries to be used to add include statements. Specifies 1399 * the path value of "<b>PractitionerRole:practitioner</b>". 1400 */ 1401 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("PractitionerRole:practitioner").toLocked(); 1402 1403 /** 1404 * Search parameter: <b>role</b> 1405 * <p> 1406 * Description: <b>The practitioner can perform this role at for the organization</b><br> 1407 * Type: <b>token</b><br> 1408 * Path: <b>PractitionerRole.code</b><br> 1409 * </p> 1410 */ 1411 @SearchParamDefinition(name="role", path="PractitionerRole.code", description="The practitioner can perform this role at for the organization", type="token" ) 1412 public static final String SP_ROLE = "role"; 1413 /** 1414 * <b>Fluent Client</b> search parameter constant for <b>role</b> 1415 * <p> 1416 * Description: <b>The practitioner can perform this role at for the organization</b><br> 1417 * Type: <b>token</b><br> 1418 * Path: <b>PractitionerRole.code</b><br> 1419 * </p> 1420 */ 1421 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROLE); 1422 1423 /** 1424 * Search parameter: <b>service</b> 1425 * <p> 1426 * Description: <b>The list of healthcare services that this worker provides for this role's Organization/Location(s)</b><br> 1427 * Type: <b>reference</b><br> 1428 * Path: <b>PractitionerRole.healthcareService</b><br> 1429 * </p> 1430 */ 1431 @SearchParamDefinition(name="service", path="PractitionerRole.healthcareService", description="The list of healthcare services that this worker provides for this role's Organization/Location(s)", type="reference", target={HealthcareService.class } ) 1432 public static final String SP_SERVICE = "service"; 1433 /** 1434 * <b>Fluent Client</b> search parameter constant for <b>service</b> 1435 * <p> 1436 * Description: <b>The list of healthcare services that this worker provides for this role's Organization/Location(s)</b><br> 1437 * Type: <b>reference</b><br> 1438 * Path: <b>PractitionerRole.healthcareService</b><br> 1439 * </p> 1440 */ 1441 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE); 1442 1443/** 1444 * Constant for fluent queries to be used to add include statements. Specifies 1445 * the path value of "<b>PractitionerRole:service</b>". 1446 */ 1447 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include("PractitionerRole:service").toLocked(); 1448 1449 /** 1450 * Search parameter: <b>specialty</b> 1451 * <p> 1452 * Description: <b>The practitioner has this specialty at an organization</b><br> 1453 * Type: <b>token</b><br> 1454 * Path: <b>PractitionerRole.specialty</b><br> 1455 * </p> 1456 */ 1457 @SearchParamDefinition(name="specialty", path="PractitionerRole.specialty", description="The practitioner has this specialty at an organization", type="token" ) 1458 public static final String SP_SPECIALTY = "specialty"; 1459 /** 1460 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 1461 * <p> 1462 * Description: <b>The practitioner has this specialty at an organization</b><br> 1463 * Type: <b>token</b><br> 1464 * Path: <b>PractitionerRole.specialty</b><br> 1465 * </p> 1466 */ 1467 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 1468 1469 /** 1470 * Search parameter: <b>email</b> 1471 * <p> 1472 * Description: <b>Multiple Resources: 1473 1474* [Patient](patient.html): A value in an email contact 1475* [Person](person.html): A value in an email contact 1476* [Practitioner](practitioner.html): A value in an email contact 1477* [PractitionerRole](practitionerrole.html): A value in an email contact 1478* [RelatedPerson](relatedperson.html): A value in an email contact 1479</b><br> 1480 * Type: <b>token</b><br> 1481 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 1482 * </p> 1483 */ 1484 @SearchParamDefinition(name="email", path="Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in an email contact\r\n* [Person](person.html): A value in an email contact\r\n* [Practitioner](practitioner.html): A value in an email contact\r\n* [PractitionerRole](practitionerrole.html): A value in an email contact\r\n* [RelatedPerson](relatedperson.html): A value in an email contact\r\n", type="token" ) 1485 public static final String SP_EMAIL = "email"; 1486 /** 1487 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1488 * <p> 1489 * Description: <b>Multiple Resources: 1490 1491* [Patient](patient.html): A value in an email contact 1492* [Person](person.html): A value in an email contact 1493* [Practitioner](practitioner.html): A value in an email contact 1494* [PractitionerRole](practitionerrole.html): A value in an email contact 1495* [RelatedPerson](relatedperson.html): A value in an email contact 1496</b><br> 1497 * Type: <b>token</b><br> 1498 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 1499 * </p> 1500 */ 1501 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 1502 1503 /** 1504 * Search parameter: <b>phone</b> 1505 * <p> 1506 * Description: <b>Multiple Resources: 1507 1508* [Patient](patient.html): A value in a phone contact 1509* [Person](person.html): A value in a phone contact 1510* [Practitioner](practitioner.html): A value in a phone contact 1511* [PractitionerRole](practitionerrole.html): A value in a phone contact 1512* [RelatedPerson](relatedperson.html): A value in a phone contact 1513</b><br> 1514 * Type: <b>token</b><br> 1515 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 1516 * </p> 1517 */ 1518 @SearchParamDefinition(name="phone", path="Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in a phone contact\r\n* [Person](person.html): A value in a phone contact\r\n* [Practitioner](practitioner.html): A value in a phone contact\r\n* [PractitionerRole](practitionerrole.html): A value in a phone contact\r\n* [RelatedPerson](relatedperson.html): A value in a phone contact\r\n", type="token" ) 1519 public static final String SP_PHONE = "phone"; 1520 /** 1521 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1522 * <p> 1523 * Description: <b>Multiple Resources: 1524 1525* [Patient](patient.html): A value in a phone contact 1526* [Person](person.html): A value in a phone contact 1527* [Practitioner](practitioner.html): A value in a phone contact 1528* [PractitionerRole](practitionerrole.html): A value in a phone contact 1529* [RelatedPerson](relatedperson.html): A value in a phone contact 1530</b><br> 1531 * Type: <b>token</b><br> 1532 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 1533 * </p> 1534 */ 1535 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 1536 1537 /** 1538 * Search parameter: <b>telecom</b> 1539 * <p> 1540 * Description: <b>Multiple Resources: 1541 1542* [Patient](patient.html): The value in any kind of telecom details of the patient 1543* [Person](person.html): The value in any kind of contact 1544* [Practitioner](practitioner.html): The value in any kind of contact 1545* [PractitionerRole](practitionerrole.html): The value in any kind of contact 1546* [RelatedPerson](relatedperson.html): The value in any kind of contact 1547</b><br> 1548 * Type: <b>token</b><br> 1549 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 1550 * </p> 1551 */ 1552 @SearchParamDefinition(name="telecom", path="Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The value in any kind of telecom details of the patient\r\n* [Person](person.html): The value in any kind of contact\r\n* [Practitioner](practitioner.html): The value in any kind of contact\r\n* [PractitionerRole](practitionerrole.html): The value in any kind of contact\r\n* [RelatedPerson](relatedperson.html): The value in any kind of contact\r\n", type="token" ) 1553 public static final String SP_TELECOM = "telecom"; 1554 /** 1555 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1556 * <p> 1557 * Description: <b>Multiple Resources: 1558 1559* [Patient](patient.html): The value in any kind of telecom details of the patient 1560* [Person](person.html): The value in any kind of contact 1561* [Practitioner](practitioner.html): The value in any kind of contact 1562* [PractitionerRole](practitionerrole.html): The value in any kind of contact 1563* [RelatedPerson](relatedperson.html): The value in any kind of contact 1564</b><br> 1565 * Type: <b>token</b><br> 1566 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 1567 * </p> 1568 */ 1569 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 1570 1571 1572} 1573