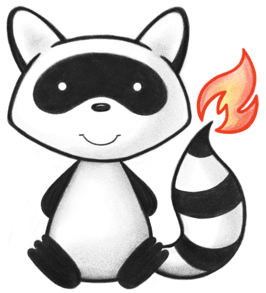
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An action that is or was performed on or for a patient, practitioner, device, organization, or location. For example, this can be a physical intervention on a patient like an operation, or less invasive like long term services, counseling, or hypnotherapy. This can be a quality or safety inspection for a location, organization, or device. This can be an accreditation procedure on a practitioner for licensing. 052 */ 053@ResourceDef(name="Procedure", profile="http://hl7.org/fhir/StructureDefinition/Procedure") 054public class Procedure extends DomainResource { 055 056 @Block() 057 public static class ProcedurePerformerComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist. 060 */ 061 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Type of performance", formalDefinition="Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 064 protected CodeableConcept function; 065 066 /** 067 * Indicates who or what performed the procedure. 068 */ 069 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class, Device.class, CareTeam.class, HealthcareService.class}, order=2, min=1, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="Who performed the procedure", formalDefinition="Indicates who or what performed the procedure." ) 071 protected Reference actor; 072 073 /** 074 * The Organization the Patient, RelatedPerson, Device, CareTeam, and HealthcareService was acting on behalf of. 075 */ 076 @Child(name = "onBehalfOf", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 077 @Description(shortDefinition="Organization the device or practitioner was acting for", formalDefinition="The Organization the Patient, RelatedPerson, Device, CareTeam, and HealthcareService was acting on behalf of." ) 078 protected Reference onBehalfOf; 079 080 /** 081 * Time period during which the performer performed the procedure. 082 */ 083 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 084 @Description(shortDefinition="When the performer performed the procedure", formalDefinition="Time period during which the performer performed the procedure." ) 085 protected Period period; 086 087 private static final long serialVersionUID = -1329650986L; 088 089 /** 090 * Constructor 091 */ 092 public ProcedurePerformerComponent() { 093 super(); 094 } 095 096 /** 097 * Constructor 098 */ 099 public ProcedurePerformerComponent(Reference actor) { 100 super(); 101 this.setActor(actor); 102 } 103 104 /** 105 * @return {@link #function} (Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist.) 106 */ 107 public CodeableConcept getFunction() { 108 if (this.function == null) 109 if (Configuration.errorOnAutoCreate()) 110 throw new Error("Attempt to auto-create ProcedurePerformerComponent.function"); 111 else if (Configuration.doAutoCreate()) 112 this.function = new CodeableConcept(); // cc 113 return this.function; 114 } 115 116 public boolean hasFunction() { 117 return this.function != null && !this.function.isEmpty(); 118 } 119 120 /** 121 * @param value {@link #function} (Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist.) 122 */ 123 public ProcedurePerformerComponent setFunction(CodeableConcept value) { 124 this.function = value; 125 return this; 126 } 127 128 /** 129 * @return {@link #actor} (Indicates who or what performed the procedure.) 130 */ 131 public Reference getActor() { 132 if (this.actor == null) 133 if (Configuration.errorOnAutoCreate()) 134 throw new Error("Attempt to auto-create ProcedurePerformerComponent.actor"); 135 else if (Configuration.doAutoCreate()) 136 this.actor = new Reference(); // cc 137 return this.actor; 138 } 139 140 public boolean hasActor() { 141 return this.actor != null && !this.actor.isEmpty(); 142 } 143 144 /** 145 * @param value {@link #actor} (Indicates who or what performed the procedure.) 146 */ 147 public ProcedurePerformerComponent setActor(Reference value) { 148 this.actor = value; 149 return this; 150 } 151 152 /** 153 * @return {@link #onBehalfOf} (The Organization the Patient, RelatedPerson, Device, CareTeam, and HealthcareService was acting on behalf of.) 154 */ 155 public Reference getOnBehalfOf() { 156 if (this.onBehalfOf == null) 157 if (Configuration.errorOnAutoCreate()) 158 throw new Error("Attempt to auto-create ProcedurePerformerComponent.onBehalfOf"); 159 else if (Configuration.doAutoCreate()) 160 this.onBehalfOf = new Reference(); // cc 161 return this.onBehalfOf; 162 } 163 164 public boolean hasOnBehalfOf() { 165 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #onBehalfOf} (The Organization the Patient, RelatedPerson, Device, CareTeam, and HealthcareService was acting on behalf of.) 170 */ 171 public ProcedurePerformerComponent setOnBehalfOf(Reference value) { 172 this.onBehalfOf = value; 173 return this; 174 } 175 176 /** 177 * @return {@link #period} (Time period during which the performer performed the procedure.) 178 */ 179 public Period getPeriod() { 180 if (this.period == null) 181 if (Configuration.errorOnAutoCreate()) 182 throw new Error("Attempt to auto-create ProcedurePerformerComponent.period"); 183 else if (Configuration.doAutoCreate()) 184 this.period = new Period(); // cc 185 return this.period; 186 } 187 188 public boolean hasPeriod() { 189 return this.period != null && !this.period.isEmpty(); 190 } 191 192 /** 193 * @param value {@link #period} (Time period during which the performer performed the procedure.) 194 */ 195 public ProcedurePerformerComponent setPeriod(Period value) { 196 this.period = value; 197 return this; 198 } 199 200 protected void listChildren(List<Property> children) { 201 super.listChildren(children); 202 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist.", 0, 1, function)); 203 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device|CareTeam|HealthcareService)", "Indicates who or what performed the procedure.", 0, 1, actor)); 204 children.add(new Property("onBehalfOf", "Reference(Organization)", "The Organization the Patient, RelatedPerson, Device, CareTeam, and HealthcareService was acting on behalf of.", 0, 1, onBehalfOf)); 205 children.add(new Property("period", "Period", "Time period during which the performer performed the procedure.", 0, 1, period)); 206 } 207 208 @Override 209 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 210 switch (_hash) { 211 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist.", 0, 1, function); 212 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device|CareTeam|HealthcareService)", "Indicates who or what performed the procedure.", 0, 1, actor); 213 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The Organization the Patient, RelatedPerson, Device, CareTeam, and HealthcareService was acting on behalf of.", 0, 1, onBehalfOf); 214 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which the performer performed the procedure.", 0, 1, period); 215 default: return super.getNamedProperty(_hash, _name, _checkValid); 216 } 217 218 } 219 220 @Override 221 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 222 switch (hash) { 223 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 224 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 225 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 226 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 227 default: return super.getProperty(hash, name, checkValid); 228 } 229 230 } 231 232 @Override 233 public Base setProperty(int hash, String name, Base value) throws FHIRException { 234 switch (hash) { 235 case 1380938712: // function 236 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 237 return value; 238 case 92645877: // actor 239 this.actor = TypeConvertor.castToReference(value); // Reference 240 return value; 241 case -14402964: // onBehalfOf 242 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 243 return value; 244 case -991726143: // period 245 this.period = TypeConvertor.castToPeriod(value); // Period 246 return value; 247 default: return super.setProperty(hash, name, value); 248 } 249 250 } 251 252 @Override 253 public Base setProperty(String name, Base value) throws FHIRException { 254 if (name.equals("function")) { 255 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 256 } else if (name.equals("actor")) { 257 this.actor = TypeConvertor.castToReference(value); // Reference 258 } else if (name.equals("onBehalfOf")) { 259 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 260 } else if (name.equals("period")) { 261 this.period = TypeConvertor.castToPeriod(value); // Period 262 } else 263 return super.setProperty(name, value); 264 return value; 265 } 266 267 @Override 268 public void removeChild(String name, Base value) throws FHIRException { 269 if (name.equals("function")) { 270 this.function = null; 271 } else if (name.equals("actor")) { 272 this.actor = null; 273 } else if (name.equals("onBehalfOf")) { 274 this.onBehalfOf = null; 275 } else if (name.equals("period")) { 276 this.period = null; 277 } else 278 super.removeChild(name, value); 279 280 } 281 282 @Override 283 public Base makeProperty(int hash, String name) throws FHIRException { 284 switch (hash) { 285 case 1380938712: return getFunction(); 286 case 92645877: return getActor(); 287 case -14402964: return getOnBehalfOf(); 288 case -991726143: return getPeriod(); 289 default: return super.makeProperty(hash, name); 290 } 291 292 } 293 294 @Override 295 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 296 switch (hash) { 297 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 298 case 92645877: /*actor*/ return new String[] {"Reference"}; 299 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 300 case -991726143: /*period*/ return new String[] {"Period"}; 301 default: return super.getTypesForProperty(hash, name); 302 } 303 304 } 305 306 @Override 307 public Base addChild(String name) throws FHIRException { 308 if (name.equals("function")) { 309 this.function = new CodeableConcept(); 310 return this.function; 311 } 312 else if (name.equals("actor")) { 313 this.actor = new Reference(); 314 return this.actor; 315 } 316 else if (name.equals("onBehalfOf")) { 317 this.onBehalfOf = new Reference(); 318 return this.onBehalfOf; 319 } 320 else if (name.equals("period")) { 321 this.period = new Period(); 322 return this.period; 323 } 324 else 325 return super.addChild(name); 326 } 327 328 public ProcedurePerformerComponent copy() { 329 ProcedurePerformerComponent dst = new ProcedurePerformerComponent(); 330 copyValues(dst); 331 return dst; 332 } 333 334 public void copyValues(ProcedurePerformerComponent dst) { 335 super.copyValues(dst); 336 dst.function = function == null ? null : function.copy(); 337 dst.actor = actor == null ? null : actor.copy(); 338 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 339 dst.period = period == null ? null : period.copy(); 340 } 341 342 @Override 343 public boolean equalsDeep(Base other_) { 344 if (!super.equalsDeep(other_)) 345 return false; 346 if (!(other_ instanceof ProcedurePerformerComponent)) 347 return false; 348 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other_; 349 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 350 && compareDeep(period, o.period, true); 351 } 352 353 @Override 354 public boolean equalsShallow(Base other_) { 355 if (!super.equalsShallow(other_)) 356 return false; 357 if (!(other_ instanceof ProcedurePerformerComponent)) 358 return false; 359 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other_; 360 return true; 361 } 362 363 public boolean isEmpty() { 364 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor, onBehalfOf 365 , period); 366 } 367 368 public String fhirType() { 369 return "Procedure.performer"; 370 371 } 372 373 } 374 375 @Block() 376 public static class ProcedureFocalDeviceComponent extends BackboneElement implements IBaseBackboneElement { 377 /** 378 * The kind of change that happened to the device during the procedure. 379 */ 380 @Child(name = "action", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 381 @Description(shortDefinition="Kind of change to device", formalDefinition="The kind of change that happened to the device during the procedure." ) 382 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-action") 383 protected CodeableConcept action; 384 385 /** 386 * The device that was manipulated (changed) during the procedure. 387 */ 388 @Child(name = "manipulated", type = {Device.class}, order=2, min=1, max=1, modifier=false, summary=false) 389 @Description(shortDefinition="Device that was changed", formalDefinition="The device that was manipulated (changed) during the procedure." ) 390 protected Reference manipulated; 391 392 private static final long serialVersionUID = -217582010L; 393 394 /** 395 * Constructor 396 */ 397 public ProcedureFocalDeviceComponent() { 398 super(); 399 } 400 401 /** 402 * Constructor 403 */ 404 public ProcedureFocalDeviceComponent(Reference manipulated) { 405 super(); 406 this.setManipulated(manipulated); 407 } 408 409 /** 410 * @return {@link #action} (The kind of change that happened to the device during the procedure.) 411 */ 412 public CodeableConcept getAction() { 413 if (this.action == null) 414 if (Configuration.errorOnAutoCreate()) 415 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.action"); 416 else if (Configuration.doAutoCreate()) 417 this.action = new CodeableConcept(); // cc 418 return this.action; 419 } 420 421 public boolean hasAction() { 422 return this.action != null && !this.action.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #action} (The kind of change that happened to the device during the procedure.) 427 */ 428 public ProcedureFocalDeviceComponent setAction(CodeableConcept value) { 429 this.action = value; 430 return this; 431 } 432 433 /** 434 * @return {@link #manipulated} (The device that was manipulated (changed) during the procedure.) 435 */ 436 public Reference getManipulated() { 437 if (this.manipulated == null) 438 if (Configuration.errorOnAutoCreate()) 439 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.manipulated"); 440 else if (Configuration.doAutoCreate()) 441 this.manipulated = new Reference(); // cc 442 return this.manipulated; 443 } 444 445 public boolean hasManipulated() { 446 return this.manipulated != null && !this.manipulated.isEmpty(); 447 } 448 449 /** 450 * @param value {@link #manipulated} (The device that was manipulated (changed) during the procedure.) 451 */ 452 public ProcedureFocalDeviceComponent setManipulated(Reference value) { 453 this.manipulated = value; 454 return this; 455 } 456 457 protected void listChildren(List<Property> children) { 458 super.listChildren(children); 459 children.add(new Property("action", "CodeableConcept", "The kind of change that happened to the device during the procedure.", 0, 1, action)); 460 children.add(new Property("manipulated", "Reference(Device)", "The device that was manipulated (changed) during the procedure.", 0, 1, manipulated)); 461 } 462 463 @Override 464 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 465 switch (_hash) { 466 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "The kind of change that happened to the device during the procedure.", 0, 1, action); 467 case 947372650: /*manipulated*/ return new Property("manipulated", "Reference(Device)", "The device that was manipulated (changed) during the procedure.", 0, 1, manipulated); 468 default: return super.getNamedProperty(_hash, _name, _checkValid); 469 } 470 471 } 472 473 @Override 474 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 475 switch (hash) { 476 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // CodeableConcept 477 case 947372650: /*manipulated*/ return this.manipulated == null ? new Base[0] : new Base[] {this.manipulated}; // Reference 478 default: return super.getProperty(hash, name, checkValid); 479 } 480 481 } 482 483 @Override 484 public Base setProperty(int hash, String name, Base value) throws FHIRException { 485 switch (hash) { 486 case -1422950858: // action 487 this.action = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 488 return value; 489 case 947372650: // manipulated 490 this.manipulated = TypeConvertor.castToReference(value); // Reference 491 return value; 492 default: return super.setProperty(hash, name, value); 493 } 494 495 } 496 497 @Override 498 public Base setProperty(String name, Base value) throws FHIRException { 499 if (name.equals("action")) { 500 this.action = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 501 } else if (name.equals("manipulated")) { 502 this.manipulated = TypeConvertor.castToReference(value); // Reference 503 } else 504 return super.setProperty(name, value); 505 return value; 506 } 507 508 @Override 509 public void removeChild(String name, Base value) throws FHIRException { 510 if (name.equals("action")) { 511 this.action = null; 512 } else if (name.equals("manipulated")) { 513 this.manipulated = null; 514 } else 515 super.removeChild(name, value); 516 517 } 518 519 @Override 520 public Base makeProperty(int hash, String name) throws FHIRException { 521 switch (hash) { 522 case -1422950858: return getAction(); 523 case 947372650: return getManipulated(); 524 default: return super.makeProperty(hash, name); 525 } 526 527 } 528 529 @Override 530 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 531 switch (hash) { 532 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 533 case 947372650: /*manipulated*/ return new String[] {"Reference"}; 534 default: return super.getTypesForProperty(hash, name); 535 } 536 537 } 538 539 @Override 540 public Base addChild(String name) throws FHIRException { 541 if (name.equals("action")) { 542 this.action = new CodeableConcept(); 543 return this.action; 544 } 545 else if (name.equals("manipulated")) { 546 this.manipulated = new Reference(); 547 return this.manipulated; 548 } 549 else 550 return super.addChild(name); 551 } 552 553 public ProcedureFocalDeviceComponent copy() { 554 ProcedureFocalDeviceComponent dst = new ProcedureFocalDeviceComponent(); 555 copyValues(dst); 556 return dst; 557 } 558 559 public void copyValues(ProcedureFocalDeviceComponent dst) { 560 super.copyValues(dst); 561 dst.action = action == null ? null : action.copy(); 562 dst.manipulated = manipulated == null ? null : manipulated.copy(); 563 } 564 565 @Override 566 public boolean equalsDeep(Base other_) { 567 if (!super.equalsDeep(other_)) 568 return false; 569 if (!(other_ instanceof ProcedureFocalDeviceComponent)) 570 return false; 571 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other_; 572 return compareDeep(action, o.action, true) && compareDeep(manipulated, o.manipulated, true); 573 } 574 575 @Override 576 public boolean equalsShallow(Base other_) { 577 if (!super.equalsShallow(other_)) 578 return false; 579 if (!(other_ instanceof ProcedureFocalDeviceComponent)) 580 return false; 581 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other_; 582 return true; 583 } 584 585 public boolean isEmpty() { 586 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, manipulated); 587 } 588 589 public String fhirType() { 590 return "Procedure.focalDevice"; 591 592 } 593 594 } 595 596 /** 597 * Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server. 598 */ 599 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 600 @Description(shortDefinition="External Identifiers for this procedure", formalDefinition="Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server." ) 601 protected List<Identifier> identifier; 602 603 /** 604 * The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure. 605 */ 606 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 607 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure." ) 608 protected List<CanonicalType> instantiatesCanonical; 609 610 /** 611 * The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure. 612 */ 613 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 614 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure." ) 615 protected List<UriType> instantiatesUri; 616 617 /** 618 * A reference to a resource that contains details of the request for this procedure. 619 */ 620 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 621 @Description(shortDefinition="A request for this procedure", formalDefinition="A reference to a resource that contains details of the request for this procedure." ) 622 protected List<Reference> basedOn; 623 624 /** 625 * A larger event of which this particular procedure is a component or step. 626 */ 627 @Child(name = "partOf", type = {Procedure.class, Observation.class, MedicationAdministration.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 628 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular procedure is a component or step." ) 629 protected List<Reference> partOf; 630 631 /** 632 * A code specifying the state of the procedure. Generally, this will be the in-progress or completed state. 633 */ 634 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 635 @Description(shortDefinition="preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition="A code specifying the state of the procedure. Generally, this will be the in-progress or completed state." ) 636 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-status") 637 protected Enumeration<EventStatus> status; 638 639 /** 640 * Captures the reason for the current state of the procedure. 641 */ 642 @Child(name = "statusReason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 643 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current state of the procedure." ) 644 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-not-performed-reason") 645 protected CodeableConcept statusReason; 646 647 /** 648 * A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure"). 649 */ 650 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 651 @Description(shortDefinition="Classification of the procedure", formalDefinition="A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\")." ) 652 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-category") 653 protected List<CodeableConcept> category; 654 655 /** 656 * The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. "Laparoscopic Appendectomy"). 657 */ 658 @Child(name = "code", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 659 @Description(shortDefinition="Identification of the procedure", formalDefinition="The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\")." ) 660 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 661 protected CodeableConcept code; 662 663 /** 664 * On whom or on what the procedure was performed. This is usually an individual human, but can also be performed on animals, groups of humans or animals, organizations or practitioners (for licensing), locations or devices (for safety inspections or regulatory authorizations). If the actual focus of the procedure is different from the subject, the focus element specifies the actual focus of the procedure. 665 */ 666 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Practitioner.class, Organization.class, Location.class}, order=9, min=1, max=1, modifier=false, summary=true) 667 @Description(shortDefinition="Individual or entity the procedure was performed on", formalDefinition="On whom or on what the procedure was performed. This is usually an individual human, but can also be performed on animals, groups of humans or animals, organizations or practitioners (for licensing), locations or devices (for safety inspections or regulatory authorizations). If the actual focus of the procedure is different from the subject, the focus element specifies the actual focus of the procedure." ) 668 protected Reference subject; 669 670 /** 671 * Who is the target of the procedure when it is not the subject of record only. If focus is not present, then subject is the focus. If focus is present and the subject is one of the targets of the procedure, include subject as a focus as well. If focus is present and the subject is not included in focus, it implies that the procedure was only targeted on the focus. For example, when a caregiver is given education for a patient, the caregiver would be the focus and the procedure record is associated with the subject (e.g. patient). For example, use focus when recording the target of the education, training, or counseling is the parent or relative of a patient. 672 */ 673 @Child(name = "focus", type = {Patient.class, Group.class, RelatedPerson.class, Practitioner.class, Organization.class, CareTeam.class, PractitionerRole.class, Specimen.class}, order=10, min=0, max=1, modifier=false, summary=true) 674 @Description(shortDefinition="Who is the target of the procedure when it is not the subject of record only", formalDefinition="Who is the target of the procedure when it is not the subject of record only. If focus is not present, then subject is the focus. If focus is present and the subject is one of the targets of the procedure, include subject as a focus as well. If focus is present and the subject is not included in focus, it implies that the procedure was only targeted on the focus. For example, when a caregiver is given education for a patient, the caregiver would be the focus and the procedure record is associated with the subject (e.g. patient). For example, use focus when recording the target of the education, training, or counseling is the parent or relative of a patient." ) 675 protected Reference focus; 676 677 /** 678 * The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated. 679 */ 680 @Child(name = "encounter", type = {Encounter.class}, order=11, min=0, max=1, modifier=false, summary=true) 681 @Description(shortDefinition="The Encounter during which this Procedure was created", formalDefinition="The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated." ) 682 protected Reference encounter; 683 684 /** 685 * Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured. 686 */ 687 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, StringType.class, Age.class, Range.class, Timing.class}, order=12, min=0, max=1, modifier=false, summary=true) 688 @Description(shortDefinition="When the procedure occurred or is occurring", formalDefinition="Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured." ) 689 protected DataType occurrence; 690 691 /** 692 * The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event). 693 */ 694 @Child(name = "recorded", type = {DateTimeType.class}, order=13, min=0, max=1, modifier=false, summary=true) 695 @Description(shortDefinition="When the procedure was first captured in the subject's record", formalDefinition="The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event)." ) 696 protected DateTimeType recorded; 697 698 /** 699 * Individual who recorded the record and takes responsibility for its content. 700 */ 701 @Child(name = "recorder", type = {Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class}, order=14, min=0, max=1, modifier=false, summary=true) 702 @Description(shortDefinition="Who recorded the procedure", formalDefinition="Individual who recorded the record and takes responsibility for its content." ) 703 protected Reference recorder; 704 705 /** 706 * Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report. 707 */ 708 @Child(name = "reported", type = {BooleanType.class, Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Organization.class}, order=15, min=0, max=1, modifier=false, summary=true) 709 @Description(shortDefinition="Reported rather than primary record", formalDefinition="Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report." ) 710 protected DataType reported; 711 712 /** 713 * Indicates who or what performed the procedure and how they were involved. 714 */ 715 @Child(name = "performer", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 716 @Description(shortDefinition="Who performed the procedure and what they did", formalDefinition="Indicates who or what performed the procedure and how they were involved." ) 717 protected List<ProcedurePerformerComponent> performer; 718 719 /** 720 * The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant. 721 */ 722 @Child(name = "location", type = {Location.class}, order=17, min=0, max=1, modifier=false, summary=true) 723 @Description(shortDefinition="Where the procedure happened", formalDefinition="The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant." ) 724 protected Reference location; 725 726 /** 727 * The coded reason or reference why the procedure was performed. This may be a coded entity of some type, be present as text, or be a reference to one of several resources that justify the procedure. 728 */ 729 @Child(name = "reason", type = {CodeableReference.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 730 @Description(shortDefinition="The justification that the procedure was performed", formalDefinition="The coded reason or reference why the procedure was performed. This may be a coded entity of some type, be present as text, or be a reference to one of several resources that justify the procedure." ) 731 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 732 protected List<CodeableReference> reason; 733 734 /** 735 * Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion. 736 */ 737 @Child(name = "bodySite", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 738 @Description(shortDefinition="Target body sites", formalDefinition="Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion." ) 739 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 740 protected List<CodeableConcept> bodySite; 741 742 /** 743 * The outcome of the procedure - did it resolve the reasons for the procedure being performed? 744 */ 745 @Child(name = "outcome", type = {CodeableConcept.class}, order=20, min=0, max=1, modifier=false, summary=true) 746 @Description(shortDefinition="The result of procedure", formalDefinition="The outcome of the procedure - did it resolve the reasons for the procedure being performed?" ) 747 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-outcome") 748 protected CodeableConcept outcome; 749 750 /** 751 * This could be a histology result, pathology report, surgical report, etc. 752 */ 753 @Child(name = "report", type = {DiagnosticReport.class, DocumentReference.class, Composition.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 754 @Description(shortDefinition="Any report resulting from the procedure", formalDefinition="This could be a histology result, pathology report, surgical report, etc." ) 755 protected List<Reference> report; 756 757 /** 758 * Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues. 759 */ 760 @Child(name = "complication", type = {CodeableReference.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 761 @Description(shortDefinition="Complication following the procedure", formalDefinition="Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues." ) 762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 763 protected List<CodeableReference> complication; 764 765 /** 766 * If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used. 767 */ 768 @Child(name = "followUp", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 769 @Description(shortDefinition="Instructions for follow up", formalDefinition="If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used." ) 770 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-followup") 771 protected List<CodeableConcept> followUp; 772 773 /** 774 * Any other notes and comments about the procedure. 775 */ 776 @Child(name = "note", type = {Annotation.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 777 @Description(shortDefinition="Additional information about the procedure", formalDefinition="Any other notes and comments about the procedure." ) 778 protected List<Annotation> note; 779 780 /** 781 * A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure. 782 */ 783 @Child(name = "focalDevice", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 784 @Description(shortDefinition="Manipulated, implanted, or removed device", formalDefinition="A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure." ) 785 protected List<ProcedureFocalDeviceComponent> focalDevice; 786 787 /** 788 * Identifies medications, devices and any other substance used as part of the procedure. 789 */ 790 @Child(name = "used", type = {CodeableReference.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 791 @Description(shortDefinition="Items used during procedure", formalDefinition="Identifies medications, devices and any other substance used as part of the procedure." ) 792 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-type") 793 protected List<CodeableReference> used; 794 795 /** 796 * Other resources from the patient record that may be relevant to the procedure. The information from these resources was either used to create the instance or is provided to help with its interpretation. This extension should not be used if more specific inline elements or extensions are available. 797 */ 798 @Child(name = "supportingInfo", type = {Reference.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 799 @Description(shortDefinition="Extra information relevant to the procedure", formalDefinition="Other resources from the patient record that may be relevant to the procedure. The information from these resources was either used to create the instance or is provided to help with its interpretation. This extension should not be used if more specific inline elements or extensions are available." ) 800 protected List<Reference> supportingInfo; 801 802 private static final long serialVersionUID = 372127918L; 803 804 /** 805 * Constructor 806 */ 807 public Procedure() { 808 super(); 809 } 810 811 /** 812 * Constructor 813 */ 814 public Procedure(EventStatus status, Reference subject) { 815 super(); 816 this.setStatus(status); 817 this.setSubject(subject); 818 } 819 820 /** 821 * @return {@link #identifier} (Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server.) 822 */ 823 public List<Identifier> getIdentifier() { 824 if (this.identifier == null) 825 this.identifier = new ArrayList<Identifier>(); 826 return this.identifier; 827 } 828 829 /** 830 * @return Returns a reference to <code>this</code> for easy method chaining 831 */ 832 public Procedure setIdentifier(List<Identifier> theIdentifier) { 833 this.identifier = theIdentifier; 834 return this; 835 } 836 837 public boolean hasIdentifier() { 838 if (this.identifier == null) 839 return false; 840 for (Identifier item : this.identifier) 841 if (!item.isEmpty()) 842 return true; 843 return false; 844 } 845 846 public Identifier addIdentifier() { //3 847 Identifier t = new Identifier(); 848 if (this.identifier == null) 849 this.identifier = new ArrayList<Identifier>(); 850 this.identifier.add(t); 851 return t; 852 } 853 854 public Procedure addIdentifier(Identifier t) { //3 855 if (t == null) 856 return this; 857 if (this.identifier == null) 858 this.identifier = new ArrayList<Identifier>(); 859 this.identifier.add(t); 860 return this; 861 } 862 863 /** 864 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 865 */ 866 public Identifier getIdentifierFirstRep() { 867 if (getIdentifier().isEmpty()) { 868 addIdentifier(); 869 } 870 return getIdentifier().get(0); 871 } 872 873 /** 874 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 875 */ 876 public List<CanonicalType> getInstantiatesCanonical() { 877 if (this.instantiatesCanonical == null) 878 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 879 return this.instantiatesCanonical; 880 } 881 882 /** 883 * @return Returns a reference to <code>this</code> for easy method chaining 884 */ 885 public Procedure setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 886 this.instantiatesCanonical = theInstantiatesCanonical; 887 return this; 888 } 889 890 public boolean hasInstantiatesCanonical() { 891 if (this.instantiatesCanonical == null) 892 return false; 893 for (CanonicalType item : this.instantiatesCanonical) 894 if (!item.isEmpty()) 895 return true; 896 return false; 897 } 898 899 /** 900 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 901 */ 902 public CanonicalType addInstantiatesCanonicalElement() {//2 903 CanonicalType t = new CanonicalType(); 904 if (this.instantiatesCanonical == null) 905 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 906 this.instantiatesCanonical.add(t); 907 return t; 908 } 909 910 /** 911 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 912 */ 913 public Procedure addInstantiatesCanonical(String value) { //1 914 CanonicalType t = new CanonicalType(); 915 t.setValue(value); 916 if (this.instantiatesCanonical == null) 917 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 918 this.instantiatesCanonical.add(t); 919 return this; 920 } 921 922 /** 923 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 924 */ 925 public boolean hasInstantiatesCanonical(String value) { 926 if (this.instantiatesCanonical == null) 927 return false; 928 for (CanonicalType v : this.instantiatesCanonical) 929 if (v.getValue().equals(value)) // canonical 930 return true; 931 return false; 932 } 933 934 /** 935 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 936 */ 937 public List<UriType> getInstantiatesUri() { 938 if (this.instantiatesUri == null) 939 this.instantiatesUri = new ArrayList<UriType>(); 940 return this.instantiatesUri; 941 } 942 943 /** 944 * @return Returns a reference to <code>this</code> for easy method chaining 945 */ 946 public Procedure setInstantiatesUri(List<UriType> theInstantiatesUri) { 947 this.instantiatesUri = theInstantiatesUri; 948 return this; 949 } 950 951 public boolean hasInstantiatesUri() { 952 if (this.instantiatesUri == null) 953 return false; 954 for (UriType item : this.instantiatesUri) 955 if (!item.isEmpty()) 956 return true; 957 return false; 958 } 959 960 /** 961 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 962 */ 963 public UriType addInstantiatesUriElement() {//2 964 UriType t = new UriType(); 965 if (this.instantiatesUri == null) 966 this.instantiatesUri = new ArrayList<UriType>(); 967 this.instantiatesUri.add(t); 968 return t; 969 } 970 971 /** 972 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 973 */ 974 public Procedure addInstantiatesUri(String value) { //1 975 UriType t = new UriType(); 976 t.setValue(value); 977 if (this.instantiatesUri == null) 978 this.instantiatesUri = new ArrayList<UriType>(); 979 this.instantiatesUri.add(t); 980 return this; 981 } 982 983 /** 984 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.) 985 */ 986 public boolean hasInstantiatesUri(String value) { 987 if (this.instantiatesUri == null) 988 return false; 989 for (UriType v : this.instantiatesUri) 990 if (v.getValue().equals(value)) // uri 991 return true; 992 return false; 993 } 994 995 /** 996 * @return {@link #basedOn} (A reference to a resource that contains details of the request for this procedure.) 997 */ 998 public List<Reference> getBasedOn() { 999 if (this.basedOn == null) 1000 this.basedOn = new ArrayList<Reference>(); 1001 return this.basedOn; 1002 } 1003 1004 /** 1005 * @return Returns a reference to <code>this</code> for easy method chaining 1006 */ 1007 public Procedure setBasedOn(List<Reference> theBasedOn) { 1008 this.basedOn = theBasedOn; 1009 return this; 1010 } 1011 1012 public boolean hasBasedOn() { 1013 if (this.basedOn == null) 1014 return false; 1015 for (Reference item : this.basedOn) 1016 if (!item.isEmpty()) 1017 return true; 1018 return false; 1019 } 1020 1021 public Reference addBasedOn() { //3 1022 Reference t = new Reference(); 1023 if (this.basedOn == null) 1024 this.basedOn = new ArrayList<Reference>(); 1025 this.basedOn.add(t); 1026 return t; 1027 } 1028 1029 public Procedure addBasedOn(Reference t) { //3 1030 if (t == null) 1031 return this; 1032 if (this.basedOn == null) 1033 this.basedOn = new ArrayList<Reference>(); 1034 this.basedOn.add(t); 1035 return this; 1036 } 1037 1038 /** 1039 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1040 */ 1041 public Reference getBasedOnFirstRep() { 1042 if (getBasedOn().isEmpty()) { 1043 addBasedOn(); 1044 } 1045 return getBasedOn().get(0); 1046 } 1047 1048 /** 1049 * @return {@link #partOf} (A larger event of which this particular procedure is a component or step.) 1050 */ 1051 public List<Reference> getPartOf() { 1052 if (this.partOf == null) 1053 this.partOf = new ArrayList<Reference>(); 1054 return this.partOf; 1055 } 1056 1057 /** 1058 * @return Returns a reference to <code>this</code> for easy method chaining 1059 */ 1060 public Procedure setPartOf(List<Reference> thePartOf) { 1061 this.partOf = thePartOf; 1062 return this; 1063 } 1064 1065 public boolean hasPartOf() { 1066 if (this.partOf == null) 1067 return false; 1068 for (Reference item : this.partOf) 1069 if (!item.isEmpty()) 1070 return true; 1071 return false; 1072 } 1073 1074 public Reference addPartOf() { //3 1075 Reference t = new Reference(); 1076 if (this.partOf == null) 1077 this.partOf = new ArrayList<Reference>(); 1078 this.partOf.add(t); 1079 return t; 1080 } 1081 1082 public Procedure addPartOf(Reference t) { //3 1083 if (t == null) 1084 return this; 1085 if (this.partOf == null) 1086 this.partOf = new ArrayList<Reference>(); 1087 this.partOf.add(t); 1088 return this; 1089 } 1090 1091 /** 1092 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 1093 */ 1094 public Reference getPartOfFirstRep() { 1095 if (getPartOf().isEmpty()) { 1096 addPartOf(); 1097 } 1098 return getPartOf().get(0); 1099 } 1100 1101 /** 1102 * @return {@link #status} (A code specifying the state of the procedure. Generally, this will be the in-progress or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1103 */ 1104 public Enumeration<EventStatus> getStatusElement() { 1105 if (this.status == null) 1106 if (Configuration.errorOnAutoCreate()) 1107 throw new Error("Attempt to auto-create Procedure.status"); 1108 else if (Configuration.doAutoCreate()) 1109 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); // bb 1110 return this.status; 1111 } 1112 1113 public boolean hasStatusElement() { 1114 return this.status != null && !this.status.isEmpty(); 1115 } 1116 1117 public boolean hasStatus() { 1118 return this.status != null && !this.status.isEmpty(); 1119 } 1120 1121 /** 1122 * @param value {@link #status} (A code specifying the state of the procedure. Generally, this will be the in-progress or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1123 */ 1124 public Procedure setStatusElement(Enumeration<EventStatus> value) { 1125 this.status = value; 1126 return this; 1127 } 1128 1129 /** 1130 * @return A code specifying the state of the procedure. Generally, this will be the in-progress or completed state. 1131 */ 1132 public EventStatus getStatus() { 1133 return this.status == null ? null : this.status.getValue(); 1134 } 1135 1136 /** 1137 * @param value A code specifying the state of the procedure. Generally, this will be the in-progress or completed state. 1138 */ 1139 public Procedure setStatus(EventStatus value) { 1140 if (this.status == null) 1141 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); 1142 this.status.setValue(value); 1143 return this; 1144 } 1145 1146 /** 1147 * @return {@link #statusReason} (Captures the reason for the current state of the procedure.) 1148 */ 1149 public CodeableConcept getStatusReason() { 1150 if (this.statusReason == null) 1151 if (Configuration.errorOnAutoCreate()) 1152 throw new Error("Attempt to auto-create Procedure.statusReason"); 1153 else if (Configuration.doAutoCreate()) 1154 this.statusReason = new CodeableConcept(); // cc 1155 return this.statusReason; 1156 } 1157 1158 public boolean hasStatusReason() { 1159 return this.statusReason != null && !this.statusReason.isEmpty(); 1160 } 1161 1162 /** 1163 * @param value {@link #statusReason} (Captures the reason for the current state of the procedure.) 1164 */ 1165 public Procedure setStatusReason(CodeableConcept value) { 1166 this.statusReason = value; 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #category} (A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure").) 1172 */ 1173 public List<CodeableConcept> getCategory() { 1174 if (this.category == null) 1175 this.category = new ArrayList<CodeableConcept>(); 1176 return this.category; 1177 } 1178 1179 /** 1180 * @return Returns a reference to <code>this</code> for easy method chaining 1181 */ 1182 public Procedure setCategory(List<CodeableConcept> theCategory) { 1183 this.category = theCategory; 1184 return this; 1185 } 1186 1187 public boolean hasCategory() { 1188 if (this.category == null) 1189 return false; 1190 for (CodeableConcept item : this.category) 1191 if (!item.isEmpty()) 1192 return true; 1193 return false; 1194 } 1195 1196 public CodeableConcept addCategory() { //3 1197 CodeableConcept t = new CodeableConcept(); 1198 if (this.category == null) 1199 this.category = new ArrayList<CodeableConcept>(); 1200 this.category.add(t); 1201 return t; 1202 } 1203 1204 public Procedure addCategory(CodeableConcept t) { //3 1205 if (t == null) 1206 return this; 1207 if (this.category == null) 1208 this.category = new ArrayList<CodeableConcept>(); 1209 this.category.add(t); 1210 return this; 1211 } 1212 1213 /** 1214 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1215 */ 1216 public CodeableConcept getCategoryFirstRep() { 1217 if (getCategory().isEmpty()) { 1218 addCategory(); 1219 } 1220 return getCategory().get(0); 1221 } 1222 1223 /** 1224 * @return {@link #code} (The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. "Laparoscopic Appendectomy").) 1225 */ 1226 public CodeableConcept getCode() { 1227 if (this.code == null) 1228 if (Configuration.errorOnAutoCreate()) 1229 throw new Error("Attempt to auto-create Procedure.code"); 1230 else if (Configuration.doAutoCreate()) 1231 this.code = new CodeableConcept(); // cc 1232 return this.code; 1233 } 1234 1235 public boolean hasCode() { 1236 return this.code != null && !this.code.isEmpty(); 1237 } 1238 1239 /** 1240 * @param value {@link #code} (The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. "Laparoscopic Appendectomy").) 1241 */ 1242 public Procedure setCode(CodeableConcept value) { 1243 this.code = value; 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #subject} (On whom or on what the procedure was performed. This is usually an individual human, but can also be performed on animals, groups of humans or animals, organizations or practitioners (for licensing), locations or devices (for safety inspections or regulatory authorizations). If the actual focus of the procedure is different from the subject, the focus element specifies the actual focus of the procedure.) 1249 */ 1250 public Reference getSubject() { 1251 if (this.subject == null) 1252 if (Configuration.errorOnAutoCreate()) 1253 throw new Error("Attempt to auto-create Procedure.subject"); 1254 else if (Configuration.doAutoCreate()) 1255 this.subject = new Reference(); // cc 1256 return this.subject; 1257 } 1258 1259 public boolean hasSubject() { 1260 return this.subject != null && !this.subject.isEmpty(); 1261 } 1262 1263 /** 1264 * @param value {@link #subject} (On whom or on what the procedure was performed. This is usually an individual human, but can also be performed on animals, groups of humans or animals, organizations or practitioners (for licensing), locations or devices (for safety inspections or regulatory authorizations). If the actual focus of the procedure is different from the subject, the focus element specifies the actual focus of the procedure.) 1265 */ 1266 public Procedure setSubject(Reference value) { 1267 this.subject = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #focus} (Who is the target of the procedure when it is not the subject of record only. If focus is not present, then subject is the focus. If focus is present and the subject is one of the targets of the procedure, include subject as a focus as well. If focus is present and the subject is not included in focus, it implies that the procedure was only targeted on the focus. For example, when a caregiver is given education for a patient, the caregiver would be the focus and the procedure record is associated with the subject (e.g. patient). For example, use focus when recording the target of the education, training, or counseling is the parent or relative of a patient.) 1273 */ 1274 public Reference getFocus() { 1275 if (this.focus == null) 1276 if (Configuration.errorOnAutoCreate()) 1277 throw new Error("Attempt to auto-create Procedure.focus"); 1278 else if (Configuration.doAutoCreate()) 1279 this.focus = new Reference(); // cc 1280 return this.focus; 1281 } 1282 1283 public boolean hasFocus() { 1284 return this.focus != null && !this.focus.isEmpty(); 1285 } 1286 1287 /** 1288 * @param value {@link #focus} (Who is the target of the procedure when it is not the subject of record only. If focus is not present, then subject is the focus. If focus is present and the subject is one of the targets of the procedure, include subject as a focus as well. If focus is present and the subject is not included in focus, it implies that the procedure was only targeted on the focus. For example, when a caregiver is given education for a patient, the caregiver would be the focus and the procedure record is associated with the subject (e.g. patient). For example, use focus when recording the target of the education, training, or counseling is the parent or relative of a patient.) 1289 */ 1290 public Procedure setFocus(Reference value) { 1291 this.focus = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return {@link #encounter} (The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated.) 1297 */ 1298 public Reference getEncounter() { 1299 if (this.encounter == null) 1300 if (Configuration.errorOnAutoCreate()) 1301 throw new Error("Attempt to auto-create Procedure.encounter"); 1302 else if (Configuration.doAutoCreate()) 1303 this.encounter = new Reference(); // cc 1304 return this.encounter; 1305 } 1306 1307 public boolean hasEncounter() { 1308 return this.encounter != null && !this.encounter.isEmpty(); 1309 } 1310 1311 /** 1312 * @param value {@link #encounter} (The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated.) 1313 */ 1314 public Procedure setEncounter(Reference value) { 1315 this.encounter = value; 1316 return this; 1317 } 1318 1319 /** 1320 * @return {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1321 */ 1322 public DataType getOccurrence() { 1323 return this.occurrence; 1324 } 1325 1326 /** 1327 * @return {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1328 */ 1329 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1330 if (this.occurrence == null) 1331 this.occurrence = new DateTimeType(); 1332 if (!(this.occurrence instanceof DateTimeType)) 1333 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1334 return (DateTimeType) this.occurrence; 1335 } 1336 1337 public boolean hasOccurrenceDateTimeType() { 1338 return this != null && this.occurrence instanceof DateTimeType; 1339 } 1340 1341 /** 1342 * @return {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1343 */ 1344 public Period getOccurrencePeriod() throws FHIRException { 1345 if (this.occurrence == null) 1346 this.occurrence = new Period(); 1347 if (!(this.occurrence instanceof Period)) 1348 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1349 return (Period) this.occurrence; 1350 } 1351 1352 public boolean hasOccurrencePeriod() { 1353 return this != null && this.occurrence instanceof Period; 1354 } 1355 1356 /** 1357 * @return {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1358 */ 1359 public StringType getOccurrenceStringType() throws FHIRException { 1360 if (this.occurrence == null) 1361 this.occurrence = new StringType(); 1362 if (!(this.occurrence instanceof StringType)) 1363 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1364 return (StringType) this.occurrence; 1365 } 1366 1367 public boolean hasOccurrenceStringType() { 1368 return this != null && this.occurrence instanceof StringType; 1369 } 1370 1371 /** 1372 * @return {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1373 */ 1374 public Age getOccurrenceAge() throws FHIRException { 1375 if (this.occurrence == null) 1376 this.occurrence = new Age(); 1377 if (!(this.occurrence instanceof Age)) 1378 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1379 return (Age) this.occurrence; 1380 } 1381 1382 public boolean hasOccurrenceAge() { 1383 return this != null && this.occurrence instanceof Age; 1384 } 1385 1386 /** 1387 * @return {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1388 */ 1389 public Range getOccurrenceRange() throws FHIRException { 1390 if (this.occurrence == null) 1391 this.occurrence = new Range(); 1392 if (!(this.occurrence instanceof Range)) 1393 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1394 return (Range) this.occurrence; 1395 } 1396 1397 public boolean hasOccurrenceRange() { 1398 return this != null && this.occurrence instanceof Range; 1399 } 1400 1401 /** 1402 * @return {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1403 */ 1404 public Timing getOccurrenceTiming() throws FHIRException { 1405 if (this.occurrence == null) 1406 this.occurrence = new Timing(); 1407 if (!(this.occurrence instanceof Timing)) 1408 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1409 return (Timing) this.occurrence; 1410 } 1411 1412 public boolean hasOccurrenceTiming() { 1413 return this != null && this.occurrence instanceof Timing; 1414 } 1415 1416 public boolean hasOccurrence() { 1417 return this.occurrence != null && !this.occurrence.isEmpty(); 1418 } 1419 1420 /** 1421 * @param value {@link #occurrence} (Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1422 */ 1423 public Procedure setOccurrence(DataType value) { 1424 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof StringType || value instanceof Age || value instanceof Range || value instanceof Timing)) 1425 throw new FHIRException("Not the right type for Procedure.occurrence[x]: "+value.fhirType()); 1426 this.occurrence = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return {@link #recorded} (The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event).). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1432 */ 1433 public DateTimeType getRecordedElement() { 1434 if (this.recorded == null) 1435 if (Configuration.errorOnAutoCreate()) 1436 throw new Error("Attempt to auto-create Procedure.recorded"); 1437 else if (Configuration.doAutoCreate()) 1438 this.recorded = new DateTimeType(); // bb 1439 return this.recorded; 1440 } 1441 1442 public boolean hasRecordedElement() { 1443 return this.recorded != null && !this.recorded.isEmpty(); 1444 } 1445 1446 public boolean hasRecorded() { 1447 return this.recorded != null && !this.recorded.isEmpty(); 1448 } 1449 1450 /** 1451 * @param value {@link #recorded} (The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event).). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1452 */ 1453 public Procedure setRecordedElement(DateTimeType value) { 1454 this.recorded = value; 1455 return this; 1456 } 1457 1458 /** 1459 * @return The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event). 1460 */ 1461 public Date getRecorded() { 1462 return this.recorded == null ? null : this.recorded.getValue(); 1463 } 1464 1465 /** 1466 * @param value The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event). 1467 */ 1468 public Procedure setRecorded(Date value) { 1469 if (value == null) 1470 this.recorded = null; 1471 else { 1472 if (this.recorded == null) 1473 this.recorded = new DateTimeType(); 1474 this.recorded.setValue(value); 1475 } 1476 return this; 1477 } 1478 1479 /** 1480 * @return {@link #recorder} (Individual who recorded the record and takes responsibility for its content.) 1481 */ 1482 public Reference getRecorder() { 1483 if (this.recorder == null) 1484 if (Configuration.errorOnAutoCreate()) 1485 throw new Error("Attempt to auto-create Procedure.recorder"); 1486 else if (Configuration.doAutoCreate()) 1487 this.recorder = new Reference(); // cc 1488 return this.recorder; 1489 } 1490 1491 public boolean hasRecorder() { 1492 return this.recorder != null && !this.recorder.isEmpty(); 1493 } 1494 1495 /** 1496 * @param value {@link #recorder} (Individual who recorded the record and takes responsibility for its content.) 1497 */ 1498 public Procedure setRecorder(Reference value) { 1499 this.recorder = value; 1500 return this; 1501 } 1502 1503 /** 1504 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1505 */ 1506 public DataType getReported() { 1507 return this.reported; 1508 } 1509 1510 /** 1511 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1512 */ 1513 public BooleanType getReportedBooleanType() throws FHIRException { 1514 if (this.reported == null) 1515 this.reported = new BooleanType(); 1516 if (!(this.reported instanceof BooleanType)) 1517 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.reported.getClass().getName()+" was encountered"); 1518 return (BooleanType) this.reported; 1519 } 1520 1521 public boolean hasReportedBooleanType() { 1522 return this != null && this.reported instanceof BooleanType; 1523 } 1524 1525 /** 1526 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1527 */ 1528 public Reference getReportedReference() throws FHIRException { 1529 if (this.reported == null) 1530 this.reported = new Reference(); 1531 if (!(this.reported instanceof Reference)) 1532 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reported.getClass().getName()+" was encountered"); 1533 return (Reference) this.reported; 1534 } 1535 1536 public boolean hasReportedReference() { 1537 return this != null && this.reported instanceof Reference; 1538 } 1539 1540 public boolean hasReported() { 1541 return this.reported != null && !this.reported.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1546 */ 1547 public Procedure setReported(DataType value) { 1548 if (value != null && !(value instanceof BooleanType || value instanceof Reference)) 1549 throw new FHIRException("Not the right type for Procedure.reported[x]: "+value.fhirType()); 1550 this.reported = value; 1551 return this; 1552 } 1553 1554 /** 1555 * @return {@link #performer} (Indicates who or what performed the procedure and how they were involved.) 1556 */ 1557 public List<ProcedurePerformerComponent> getPerformer() { 1558 if (this.performer == null) 1559 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1560 return this.performer; 1561 } 1562 1563 /** 1564 * @return Returns a reference to <code>this</code> for easy method chaining 1565 */ 1566 public Procedure setPerformer(List<ProcedurePerformerComponent> thePerformer) { 1567 this.performer = thePerformer; 1568 return this; 1569 } 1570 1571 public boolean hasPerformer() { 1572 if (this.performer == null) 1573 return false; 1574 for (ProcedurePerformerComponent item : this.performer) 1575 if (!item.isEmpty()) 1576 return true; 1577 return false; 1578 } 1579 1580 public ProcedurePerformerComponent addPerformer() { //3 1581 ProcedurePerformerComponent t = new ProcedurePerformerComponent(); 1582 if (this.performer == null) 1583 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1584 this.performer.add(t); 1585 return t; 1586 } 1587 1588 public Procedure addPerformer(ProcedurePerformerComponent t) { //3 1589 if (t == null) 1590 return this; 1591 if (this.performer == null) 1592 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1593 this.performer.add(t); 1594 return this; 1595 } 1596 1597 /** 1598 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1599 */ 1600 public ProcedurePerformerComponent getPerformerFirstRep() { 1601 if (getPerformer().isEmpty()) { 1602 addPerformer(); 1603 } 1604 return getPerformer().get(0); 1605 } 1606 1607 /** 1608 * @return {@link #location} (The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 1609 */ 1610 public Reference getLocation() { 1611 if (this.location == null) 1612 if (Configuration.errorOnAutoCreate()) 1613 throw new Error("Attempt to auto-create Procedure.location"); 1614 else if (Configuration.doAutoCreate()) 1615 this.location = new Reference(); // cc 1616 return this.location; 1617 } 1618 1619 public boolean hasLocation() { 1620 return this.location != null && !this.location.isEmpty(); 1621 } 1622 1623 /** 1624 * @param value {@link #location} (The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 1625 */ 1626 public Procedure setLocation(Reference value) { 1627 this.location = value; 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #reason} (The coded reason or reference why the procedure was performed. This may be a coded entity of some type, be present as text, or be a reference to one of several resources that justify the procedure.) 1633 */ 1634 public List<CodeableReference> getReason() { 1635 if (this.reason == null) 1636 this.reason = new ArrayList<CodeableReference>(); 1637 return this.reason; 1638 } 1639 1640 /** 1641 * @return Returns a reference to <code>this</code> for easy method chaining 1642 */ 1643 public Procedure setReason(List<CodeableReference> theReason) { 1644 this.reason = theReason; 1645 return this; 1646 } 1647 1648 public boolean hasReason() { 1649 if (this.reason == null) 1650 return false; 1651 for (CodeableReference item : this.reason) 1652 if (!item.isEmpty()) 1653 return true; 1654 return false; 1655 } 1656 1657 public CodeableReference addReason() { //3 1658 CodeableReference t = new CodeableReference(); 1659 if (this.reason == null) 1660 this.reason = new ArrayList<CodeableReference>(); 1661 this.reason.add(t); 1662 return t; 1663 } 1664 1665 public Procedure addReason(CodeableReference t) { //3 1666 if (t == null) 1667 return this; 1668 if (this.reason == null) 1669 this.reason = new ArrayList<CodeableReference>(); 1670 this.reason.add(t); 1671 return this; 1672 } 1673 1674 /** 1675 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1676 */ 1677 public CodeableReference getReasonFirstRep() { 1678 if (getReason().isEmpty()) { 1679 addReason(); 1680 } 1681 return getReason().get(0); 1682 } 1683 1684 /** 1685 * @return {@link #bodySite} (Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.) 1686 */ 1687 public List<CodeableConcept> getBodySite() { 1688 if (this.bodySite == null) 1689 this.bodySite = new ArrayList<CodeableConcept>(); 1690 return this.bodySite; 1691 } 1692 1693 /** 1694 * @return Returns a reference to <code>this</code> for easy method chaining 1695 */ 1696 public Procedure setBodySite(List<CodeableConcept> theBodySite) { 1697 this.bodySite = theBodySite; 1698 return this; 1699 } 1700 1701 public boolean hasBodySite() { 1702 if (this.bodySite == null) 1703 return false; 1704 for (CodeableConcept item : this.bodySite) 1705 if (!item.isEmpty()) 1706 return true; 1707 return false; 1708 } 1709 1710 public CodeableConcept addBodySite() { //3 1711 CodeableConcept t = new CodeableConcept(); 1712 if (this.bodySite == null) 1713 this.bodySite = new ArrayList<CodeableConcept>(); 1714 this.bodySite.add(t); 1715 return t; 1716 } 1717 1718 public Procedure addBodySite(CodeableConcept t) { //3 1719 if (t == null) 1720 return this; 1721 if (this.bodySite == null) 1722 this.bodySite = new ArrayList<CodeableConcept>(); 1723 this.bodySite.add(t); 1724 return this; 1725 } 1726 1727 /** 1728 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 1729 */ 1730 public CodeableConcept getBodySiteFirstRep() { 1731 if (getBodySite().isEmpty()) { 1732 addBodySite(); 1733 } 1734 return getBodySite().get(0); 1735 } 1736 1737 /** 1738 * @return {@link #outcome} (The outcome of the procedure - did it resolve the reasons for the procedure being performed?) 1739 */ 1740 public CodeableConcept getOutcome() { 1741 if (this.outcome == null) 1742 if (Configuration.errorOnAutoCreate()) 1743 throw new Error("Attempt to auto-create Procedure.outcome"); 1744 else if (Configuration.doAutoCreate()) 1745 this.outcome = new CodeableConcept(); // cc 1746 return this.outcome; 1747 } 1748 1749 public boolean hasOutcome() { 1750 return this.outcome != null && !this.outcome.isEmpty(); 1751 } 1752 1753 /** 1754 * @param value {@link #outcome} (The outcome of the procedure - did it resolve the reasons for the procedure being performed?) 1755 */ 1756 public Procedure setOutcome(CodeableConcept value) { 1757 this.outcome = value; 1758 return this; 1759 } 1760 1761 /** 1762 * @return {@link #report} (This could be a histology result, pathology report, surgical report, etc.) 1763 */ 1764 public List<Reference> getReport() { 1765 if (this.report == null) 1766 this.report = new ArrayList<Reference>(); 1767 return this.report; 1768 } 1769 1770 /** 1771 * @return Returns a reference to <code>this</code> for easy method chaining 1772 */ 1773 public Procedure setReport(List<Reference> theReport) { 1774 this.report = theReport; 1775 return this; 1776 } 1777 1778 public boolean hasReport() { 1779 if (this.report == null) 1780 return false; 1781 for (Reference item : this.report) 1782 if (!item.isEmpty()) 1783 return true; 1784 return false; 1785 } 1786 1787 public Reference addReport() { //3 1788 Reference t = new Reference(); 1789 if (this.report == null) 1790 this.report = new ArrayList<Reference>(); 1791 this.report.add(t); 1792 return t; 1793 } 1794 1795 public Procedure addReport(Reference t) { //3 1796 if (t == null) 1797 return this; 1798 if (this.report == null) 1799 this.report = new ArrayList<Reference>(); 1800 this.report.add(t); 1801 return this; 1802 } 1803 1804 /** 1805 * @return The first repetition of repeating field {@link #report}, creating it if it does not already exist {3} 1806 */ 1807 public Reference getReportFirstRep() { 1808 if (getReport().isEmpty()) { 1809 addReport(); 1810 } 1811 return getReport().get(0); 1812 } 1813 1814 /** 1815 * @return {@link #complication} (Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.) 1816 */ 1817 public List<CodeableReference> getComplication() { 1818 if (this.complication == null) 1819 this.complication = new ArrayList<CodeableReference>(); 1820 return this.complication; 1821 } 1822 1823 /** 1824 * @return Returns a reference to <code>this</code> for easy method chaining 1825 */ 1826 public Procedure setComplication(List<CodeableReference> theComplication) { 1827 this.complication = theComplication; 1828 return this; 1829 } 1830 1831 public boolean hasComplication() { 1832 if (this.complication == null) 1833 return false; 1834 for (CodeableReference item : this.complication) 1835 if (!item.isEmpty()) 1836 return true; 1837 return false; 1838 } 1839 1840 public CodeableReference addComplication() { //3 1841 CodeableReference t = new CodeableReference(); 1842 if (this.complication == null) 1843 this.complication = new ArrayList<CodeableReference>(); 1844 this.complication.add(t); 1845 return t; 1846 } 1847 1848 public Procedure addComplication(CodeableReference t) { //3 1849 if (t == null) 1850 return this; 1851 if (this.complication == null) 1852 this.complication = new ArrayList<CodeableReference>(); 1853 this.complication.add(t); 1854 return this; 1855 } 1856 1857 /** 1858 * @return The first repetition of repeating field {@link #complication}, creating it if it does not already exist {3} 1859 */ 1860 public CodeableReference getComplicationFirstRep() { 1861 if (getComplication().isEmpty()) { 1862 addComplication(); 1863 } 1864 return getComplication().get(0); 1865 } 1866 1867 /** 1868 * @return {@link #followUp} (If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used.) 1869 */ 1870 public List<CodeableConcept> getFollowUp() { 1871 if (this.followUp == null) 1872 this.followUp = new ArrayList<CodeableConcept>(); 1873 return this.followUp; 1874 } 1875 1876 /** 1877 * @return Returns a reference to <code>this</code> for easy method chaining 1878 */ 1879 public Procedure setFollowUp(List<CodeableConcept> theFollowUp) { 1880 this.followUp = theFollowUp; 1881 return this; 1882 } 1883 1884 public boolean hasFollowUp() { 1885 if (this.followUp == null) 1886 return false; 1887 for (CodeableConcept item : this.followUp) 1888 if (!item.isEmpty()) 1889 return true; 1890 return false; 1891 } 1892 1893 public CodeableConcept addFollowUp() { //3 1894 CodeableConcept t = new CodeableConcept(); 1895 if (this.followUp == null) 1896 this.followUp = new ArrayList<CodeableConcept>(); 1897 this.followUp.add(t); 1898 return t; 1899 } 1900 1901 public Procedure addFollowUp(CodeableConcept t) { //3 1902 if (t == null) 1903 return this; 1904 if (this.followUp == null) 1905 this.followUp = new ArrayList<CodeableConcept>(); 1906 this.followUp.add(t); 1907 return this; 1908 } 1909 1910 /** 1911 * @return The first repetition of repeating field {@link #followUp}, creating it if it does not already exist {3} 1912 */ 1913 public CodeableConcept getFollowUpFirstRep() { 1914 if (getFollowUp().isEmpty()) { 1915 addFollowUp(); 1916 } 1917 return getFollowUp().get(0); 1918 } 1919 1920 /** 1921 * @return {@link #note} (Any other notes and comments about the procedure.) 1922 */ 1923 public List<Annotation> getNote() { 1924 if (this.note == null) 1925 this.note = new ArrayList<Annotation>(); 1926 return this.note; 1927 } 1928 1929 /** 1930 * @return Returns a reference to <code>this</code> for easy method chaining 1931 */ 1932 public Procedure setNote(List<Annotation> theNote) { 1933 this.note = theNote; 1934 return this; 1935 } 1936 1937 public boolean hasNote() { 1938 if (this.note == null) 1939 return false; 1940 for (Annotation item : this.note) 1941 if (!item.isEmpty()) 1942 return true; 1943 return false; 1944 } 1945 1946 public Annotation addNote() { //3 1947 Annotation t = new Annotation(); 1948 if (this.note == null) 1949 this.note = new ArrayList<Annotation>(); 1950 this.note.add(t); 1951 return t; 1952 } 1953 1954 public Procedure addNote(Annotation t) { //3 1955 if (t == null) 1956 return this; 1957 if (this.note == null) 1958 this.note = new ArrayList<Annotation>(); 1959 this.note.add(t); 1960 return this; 1961 } 1962 1963 /** 1964 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1965 */ 1966 public Annotation getNoteFirstRep() { 1967 if (getNote().isEmpty()) { 1968 addNote(); 1969 } 1970 return getNote().get(0); 1971 } 1972 1973 /** 1974 * @return {@link #focalDevice} (A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.) 1975 */ 1976 public List<ProcedureFocalDeviceComponent> getFocalDevice() { 1977 if (this.focalDevice == null) 1978 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 1979 return this.focalDevice; 1980 } 1981 1982 /** 1983 * @return Returns a reference to <code>this</code> for easy method chaining 1984 */ 1985 public Procedure setFocalDevice(List<ProcedureFocalDeviceComponent> theFocalDevice) { 1986 this.focalDevice = theFocalDevice; 1987 return this; 1988 } 1989 1990 public boolean hasFocalDevice() { 1991 if (this.focalDevice == null) 1992 return false; 1993 for (ProcedureFocalDeviceComponent item : this.focalDevice) 1994 if (!item.isEmpty()) 1995 return true; 1996 return false; 1997 } 1998 1999 public ProcedureFocalDeviceComponent addFocalDevice() { //3 2000 ProcedureFocalDeviceComponent t = new ProcedureFocalDeviceComponent(); 2001 if (this.focalDevice == null) 2002 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2003 this.focalDevice.add(t); 2004 return t; 2005 } 2006 2007 public Procedure addFocalDevice(ProcedureFocalDeviceComponent t) { //3 2008 if (t == null) 2009 return this; 2010 if (this.focalDevice == null) 2011 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2012 this.focalDevice.add(t); 2013 return this; 2014 } 2015 2016 /** 2017 * @return The first repetition of repeating field {@link #focalDevice}, creating it if it does not already exist {3} 2018 */ 2019 public ProcedureFocalDeviceComponent getFocalDeviceFirstRep() { 2020 if (getFocalDevice().isEmpty()) { 2021 addFocalDevice(); 2022 } 2023 return getFocalDevice().get(0); 2024 } 2025 2026 /** 2027 * @return {@link #used} (Identifies medications, devices and any other substance used as part of the procedure.) 2028 */ 2029 public List<CodeableReference> getUsed() { 2030 if (this.used == null) 2031 this.used = new ArrayList<CodeableReference>(); 2032 return this.used; 2033 } 2034 2035 /** 2036 * @return Returns a reference to <code>this</code> for easy method chaining 2037 */ 2038 public Procedure setUsed(List<CodeableReference> theUsed) { 2039 this.used = theUsed; 2040 return this; 2041 } 2042 2043 public boolean hasUsed() { 2044 if (this.used == null) 2045 return false; 2046 for (CodeableReference item : this.used) 2047 if (!item.isEmpty()) 2048 return true; 2049 return false; 2050 } 2051 2052 public CodeableReference addUsed() { //3 2053 CodeableReference t = new CodeableReference(); 2054 if (this.used == null) 2055 this.used = new ArrayList<CodeableReference>(); 2056 this.used.add(t); 2057 return t; 2058 } 2059 2060 public Procedure addUsed(CodeableReference t) { //3 2061 if (t == null) 2062 return this; 2063 if (this.used == null) 2064 this.used = new ArrayList<CodeableReference>(); 2065 this.used.add(t); 2066 return this; 2067 } 2068 2069 /** 2070 * @return The first repetition of repeating field {@link #used}, creating it if it does not already exist {3} 2071 */ 2072 public CodeableReference getUsedFirstRep() { 2073 if (getUsed().isEmpty()) { 2074 addUsed(); 2075 } 2076 return getUsed().get(0); 2077 } 2078 2079 /** 2080 * @return {@link #supportingInfo} (Other resources from the patient record that may be relevant to the procedure. The information from these resources was either used to create the instance or is provided to help with its interpretation. This extension should not be used if more specific inline elements or extensions are available.) 2081 */ 2082 public List<Reference> getSupportingInfo() { 2083 if (this.supportingInfo == null) 2084 this.supportingInfo = new ArrayList<Reference>(); 2085 return this.supportingInfo; 2086 } 2087 2088 /** 2089 * @return Returns a reference to <code>this</code> for easy method chaining 2090 */ 2091 public Procedure setSupportingInfo(List<Reference> theSupportingInfo) { 2092 this.supportingInfo = theSupportingInfo; 2093 return this; 2094 } 2095 2096 public boolean hasSupportingInfo() { 2097 if (this.supportingInfo == null) 2098 return false; 2099 for (Reference item : this.supportingInfo) 2100 if (!item.isEmpty()) 2101 return true; 2102 return false; 2103 } 2104 2105 public Reference addSupportingInfo() { //3 2106 Reference t = new Reference(); 2107 if (this.supportingInfo == null) 2108 this.supportingInfo = new ArrayList<Reference>(); 2109 this.supportingInfo.add(t); 2110 return t; 2111 } 2112 2113 public Procedure addSupportingInfo(Reference t) { //3 2114 if (t == null) 2115 return this; 2116 if (this.supportingInfo == null) 2117 this.supportingInfo = new ArrayList<Reference>(); 2118 this.supportingInfo.add(t); 2119 return this; 2120 } 2121 2122 /** 2123 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 2124 */ 2125 public Reference getSupportingInfoFirstRep() { 2126 if (getSupportingInfo().isEmpty()) { 2127 addSupportingInfo(); 2128 } 2129 return getSupportingInfo().get(0); 2130 } 2131 2132 protected void listChildren(List<Property> children) { 2133 super.listChildren(children); 2134 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2135 children.add(new Property("instantiatesCanonical", "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", "The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2136 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2137 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest)", "A reference to a resource that contains details of the request for this procedure.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2138 children.add(new Property("partOf", "Reference(Procedure|Observation|MedicationAdministration)", "A larger event of which this particular procedure is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2139 children.add(new Property("status", "code", "A code specifying the state of the procedure. Generally, this will be the in-progress or completed state.", 0, 1, status)); 2140 children.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the procedure.", 0, 1, statusReason)); 2141 children.add(new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category)); 2142 children.add(new Property("code", "CodeableConcept", "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").", 0, 1, code)); 2143 children.add(new Property("subject", "Reference(Patient|Group|Device|Practitioner|Organization|Location)", "On whom or on what the procedure was performed. This is usually an individual human, but can also be performed on animals, groups of humans or animals, organizations or practitioners (for licensing), locations or devices (for safety inspections or regulatory authorizations). If the actual focus of the procedure is different from the subject, the focus element specifies the actual focus of the procedure.", 0, 1, subject)); 2144 children.add(new Property("focus", "Reference(Patient|Group|RelatedPerson|Practitioner|Organization|CareTeam|PractitionerRole|Specimen)", "Who is the target of the procedure when it is not the subject of record only. If focus is not present, then subject is the focus. If focus is present and the subject is one of the targets of the procedure, include subject as a focus as well. If focus is present and the subject is not included in focus, it implies that the procedure was only targeted on the focus. For example, when a caregiver is given education for a patient, the caregiver would be the focus and the procedure record is associated with the subject (e.g. patient). For example, use focus when recording the target of the education, training, or counseling is the parent or relative of a patient.", 0, 1, focus)); 2145 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated.", 0, 1, encounter)); 2146 children.add(new Property("occurrence[x]", "dateTime|Period|string|Age|Range|Timing", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence)); 2147 children.add(new Property("recorded", "dateTime", "The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event).", 0, 1, recorded)); 2148 children.add(new Property("recorder", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder)); 2149 children.add(new Property("reported[x]", "boolean|Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported)); 2150 children.add(new Property("performer", "", "Indicates who or what performed the procedure and how they were involved.", 0, java.lang.Integer.MAX_VALUE, performer)); 2151 children.add(new Property("location", "Reference(Location)", "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.", 0, 1, location)); 2152 children.add(new Property("reason", "CodeableReference(Condition|Observation|Procedure|DiagnosticReport|DocumentReference)", "The coded reason or reference why the procedure was performed. This may be a coded entity of some type, be present as text, or be a reference to one of several resources that justify the procedure.", 0, java.lang.Integer.MAX_VALUE, reason)); 2153 children.add(new Property("bodySite", "CodeableConcept", "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 2154 children.add(new Property("outcome", "CodeableConcept", "The outcome of the procedure - did it resolve the reasons for the procedure being performed?", 0, 1, outcome)); 2155 children.add(new Property("report", "Reference(DiagnosticReport|DocumentReference|Composition)", "This could be a histology result, pathology report, surgical report, etc.", 0, java.lang.Integer.MAX_VALUE, report)); 2156 children.add(new Property("complication", "CodeableReference(Condition)", "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.", 0, java.lang.Integer.MAX_VALUE, complication)); 2157 children.add(new Property("followUp", "CodeableConcept", "If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used.", 0, java.lang.Integer.MAX_VALUE, followUp)); 2158 children.add(new Property("note", "Annotation", "Any other notes and comments about the procedure.", 0, java.lang.Integer.MAX_VALUE, note)); 2159 children.add(new Property("focalDevice", "", "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.", 0, java.lang.Integer.MAX_VALUE, focalDevice)); 2160 children.add(new Property("used", "CodeableReference(Device|Medication|Substance|BiologicallyDerivedProduct)", "Identifies medications, devices and any other substance used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, used)); 2161 children.add(new Property("supportingInfo", "Reference(Any)", "Other resources from the patient record that may be relevant to the procedure. The information from these resources was either used to create the instance or is provided to help with its interpretation. This extension should not be used if more specific inline elements or extensions are available.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 2162 } 2163 2164 @Override 2165 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2166 switch (_hash) { 2167 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 2168 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", "The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2169 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2170 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest)", "A reference to a resource that contains details of the request for this procedure.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2171 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure|Observation|MedicationAdministration)", "A larger event of which this particular procedure is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 2172 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the procedure. Generally, this will be the in-progress or completed state.", 0, 1, status); 2173 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the procedure.", 0, 1, statusReason); 2174 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category); 2175 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").", 0, 1, code); 2176 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Practitioner|Organization|Location)", "On whom or on what the procedure was performed. This is usually an individual human, but can also be performed on animals, groups of humans or animals, organizations or practitioners (for licensing), locations or devices (for safety inspections or regulatory authorizations). If the actual focus of the procedure is different from the subject, the focus element specifies the actual focus of the procedure.", 0, 1, subject); 2177 case 97604824: /*focus*/ return new Property("focus", "Reference(Patient|Group|RelatedPerson|Practitioner|Organization|CareTeam|PractitionerRole|Specimen)", "Who is the target of the procedure when it is not the subject of record only. If focus is not present, then subject is the focus. If focus is present and the subject is one of the targets of the procedure, include subject as a focus as well. If focus is present and the subject is not included in focus, it implies that the procedure was only targeted on the focus. For example, when a caregiver is given education for a patient, the caregiver would be the focus and the procedure record is associated with the subject (e.g. patient). For example, use focus when recording the target of the education, training, or counseling is the parent or relative of a patient.", 0, 1, focus); 2178 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated.", 0, 1, encounter); 2179 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|string|Age|Range|Timing", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2180 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|string|Age|Range|Timing", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2181 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2182 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2183 case 1496896834: /*occurrenceString*/ return new Property("occurrence[x]", "string", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2184 case -2022672018: /*occurrenceAge*/ return new Property("occurrence[x]", "Age", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2185 case 1847908844: /*occurrenceRange*/ return new Property("occurrence[x]", "Range", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2186 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "Estimated or actual date, date-time, period, or age when the procedure did occur or is occurring. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, occurrence); 2187 case -799233872: /*recorded*/ return new Property("recorded", "dateTime", "The date the occurrence of the procedure was first captured in the record regardless of Procedure.status (potentially after the occurrence of the event).", 0, 1, recorded); 2188 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder); 2189 case -241505587: /*reported[x]*/ return new Property("reported[x]", "boolean|Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported); 2190 case -427039533: /*reported*/ return new Property("reported[x]", "boolean|Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported); 2191 case 1219992533: /*reportedBoolean*/ return new Property("reported[x]", "boolean", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported); 2192 case 1198143416: /*reportedReference*/ return new Property("reported[x]", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 0, 1, reported); 2193 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what performed the procedure and how they were involved.", 0, java.lang.Integer.MAX_VALUE, performer); 2194 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.", 0, 1, location); 2195 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|Procedure|DiagnosticReport|DocumentReference)", "The coded reason or reference why the procedure was performed. This may be a coded entity of some type, be present as text, or be a reference to one of several resources that justify the procedure.", 0, java.lang.Integer.MAX_VALUE, reason); 2196 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.", 0, java.lang.Integer.MAX_VALUE, bodySite); 2197 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "The outcome of the procedure - did it resolve the reasons for the procedure being performed?", 0, 1, outcome); 2198 case -934521548: /*report*/ return new Property("report", "Reference(DiagnosticReport|DocumentReference|Composition)", "This could be a histology result, pathology report, surgical report, etc.", 0, java.lang.Integer.MAX_VALUE, report); 2199 case -1644401602: /*complication*/ return new Property("complication", "CodeableReference(Condition)", "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.", 0, java.lang.Integer.MAX_VALUE, complication); 2200 case 301801004: /*followUp*/ return new Property("followUp", "CodeableConcept", "If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used.", 0, java.lang.Integer.MAX_VALUE, followUp); 2201 case 3387378: /*note*/ return new Property("note", "Annotation", "Any other notes and comments about the procedure.", 0, java.lang.Integer.MAX_VALUE, note); 2202 case -1129235173: /*focalDevice*/ return new Property("focalDevice", "", "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.", 0, java.lang.Integer.MAX_VALUE, focalDevice); 2203 case 3599293: /*used*/ return new Property("used", "CodeableReference(Device|Medication|Substance|BiologicallyDerivedProduct)", "Identifies medications, devices and any other substance used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, used); 2204 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Other resources from the patient record that may be relevant to the procedure. The information from these resources was either used to create the instance or is provided to help with its interpretation. This extension should not be used if more specific inline elements or extensions are available.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2205 default: return super.getNamedProperty(_hash, _name, _checkValid); 2206 } 2207 2208 } 2209 2210 @Override 2211 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2212 switch (hash) { 2213 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2214 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2215 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2216 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2217 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2218 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EventStatus> 2219 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 2220 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2221 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2222 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2223 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // Reference 2224 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2225 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 2226 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // DateTimeType 2227 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 2228 case -427039533: /*reported*/ return this.reported == null ? new Base[0] : new Base[] {this.reported}; // DataType 2229 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // ProcedurePerformerComponent 2230 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2231 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 2232 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 2233 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 2234 case -934521548: /*report*/ return this.report == null ? new Base[0] : this.report.toArray(new Base[this.report.size()]); // Reference 2235 case -1644401602: /*complication*/ return this.complication == null ? new Base[0] : this.complication.toArray(new Base[this.complication.size()]); // CodeableReference 2236 case 301801004: /*followUp*/ return this.followUp == null ? new Base[0] : this.followUp.toArray(new Base[this.followUp.size()]); // CodeableConcept 2237 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2238 case -1129235173: /*focalDevice*/ return this.focalDevice == null ? new Base[0] : this.focalDevice.toArray(new Base[this.focalDevice.size()]); // ProcedureFocalDeviceComponent 2239 case 3599293: /*used*/ return this.used == null ? new Base[0] : this.used.toArray(new Base[this.used.size()]); // CodeableReference 2240 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 2241 default: return super.getProperty(hash, name, checkValid); 2242 } 2243 2244 } 2245 2246 @Override 2247 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2248 switch (hash) { 2249 case -1618432855: // identifier 2250 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2251 return value; 2252 case 8911915: // instantiatesCanonical 2253 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2254 return value; 2255 case -1926393373: // instantiatesUri 2256 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 2257 return value; 2258 case -332612366: // basedOn 2259 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2260 return value; 2261 case -995410646: // partOf 2262 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 2263 return value; 2264 case -892481550: // status 2265 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2266 this.status = (Enumeration) value; // Enumeration<EventStatus> 2267 return value; 2268 case 2051346646: // statusReason 2269 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2270 return value; 2271 case 50511102: // category 2272 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2273 return value; 2274 case 3059181: // code 2275 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2276 return value; 2277 case -1867885268: // subject 2278 this.subject = TypeConvertor.castToReference(value); // Reference 2279 return value; 2280 case 97604824: // focus 2281 this.focus = TypeConvertor.castToReference(value); // Reference 2282 return value; 2283 case 1524132147: // encounter 2284 this.encounter = TypeConvertor.castToReference(value); // Reference 2285 return value; 2286 case 1687874001: // occurrence 2287 this.occurrence = TypeConvertor.castToType(value); // DataType 2288 return value; 2289 case -799233872: // recorded 2290 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2291 return value; 2292 case -799233858: // recorder 2293 this.recorder = TypeConvertor.castToReference(value); // Reference 2294 return value; 2295 case -427039533: // reported 2296 this.reported = TypeConvertor.castToType(value); // DataType 2297 return value; 2298 case 481140686: // performer 2299 this.getPerformer().add((ProcedurePerformerComponent) value); // ProcedurePerformerComponent 2300 return value; 2301 case 1901043637: // location 2302 this.location = TypeConvertor.castToReference(value); // Reference 2303 return value; 2304 case -934964668: // reason 2305 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2306 return value; 2307 case 1702620169: // bodySite 2308 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2309 return value; 2310 case -1106507950: // outcome 2311 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2312 return value; 2313 case -934521548: // report 2314 this.getReport().add(TypeConvertor.castToReference(value)); // Reference 2315 return value; 2316 case -1644401602: // complication 2317 this.getComplication().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2318 return value; 2319 case 301801004: // followUp 2320 this.getFollowUp().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2321 return value; 2322 case 3387378: // note 2323 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2324 return value; 2325 case -1129235173: // focalDevice 2326 this.getFocalDevice().add((ProcedureFocalDeviceComponent) value); // ProcedureFocalDeviceComponent 2327 return value; 2328 case 3599293: // used 2329 this.getUsed().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2330 return value; 2331 case 1922406657: // supportingInfo 2332 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); // Reference 2333 return value; 2334 default: return super.setProperty(hash, name, value); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base setProperty(String name, Base value) throws FHIRException { 2341 if (name.equals("identifier")) { 2342 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2343 } else if (name.equals("instantiatesCanonical")) { 2344 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 2345 } else if (name.equals("instantiatesUri")) { 2346 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 2347 } else if (name.equals("basedOn")) { 2348 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2349 } else if (name.equals("partOf")) { 2350 this.getPartOf().add(TypeConvertor.castToReference(value)); 2351 } else if (name.equals("status")) { 2352 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2353 this.status = (Enumeration) value; // Enumeration<EventStatus> 2354 } else if (name.equals("statusReason")) { 2355 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2356 } else if (name.equals("category")) { 2357 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2358 } else if (name.equals("code")) { 2359 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2360 } else if (name.equals("subject")) { 2361 this.subject = TypeConvertor.castToReference(value); // Reference 2362 } else if (name.equals("focus")) { 2363 this.focus = TypeConvertor.castToReference(value); // Reference 2364 } else if (name.equals("encounter")) { 2365 this.encounter = TypeConvertor.castToReference(value); // Reference 2366 } else if (name.equals("occurrence[x]")) { 2367 this.occurrence = TypeConvertor.castToType(value); // DataType 2368 } else if (name.equals("recorded")) { 2369 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2370 } else if (name.equals("recorder")) { 2371 this.recorder = TypeConvertor.castToReference(value); // Reference 2372 } else if (name.equals("reported[x]")) { 2373 this.reported = TypeConvertor.castToType(value); // DataType 2374 } else if (name.equals("performer")) { 2375 this.getPerformer().add((ProcedurePerformerComponent) value); 2376 } else if (name.equals("location")) { 2377 this.location = TypeConvertor.castToReference(value); // Reference 2378 } else if (name.equals("reason")) { 2379 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 2380 } else if (name.equals("bodySite")) { 2381 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); 2382 } else if (name.equals("outcome")) { 2383 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2384 } else if (name.equals("report")) { 2385 this.getReport().add(TypeConvertor.castToReference(value)); 2386 } else if (name.equals("complication")) { 2387 this.getComplication().add(TypeConvertor.castToCodeableReference(value)); 2388 } else if (name.equals("followUp")) { 2389 this.getFollowUp().add(TypeConvertor.castToCodeableConcept(value)); 2390 } else if (name.equals("note")) { 2391 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2392 } else if (name.equals("focalDevice")) { 2393 this.getFocalDevice().add((ProcedureFocalDeviceComponent) value); 2394 } else if (name.equals("used")) { 2395 this.getUsed().add(TypeConvertor.castToCodeableReference(value)); 2396 } else if (name.equals("supportingInfo")) { 2397 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); 2398 } else 2399 return super.setProperty(name, value); 2400 return value; 2401 } 2402 2403 @Override 2404 public void removeChild(String name, Base value) throws FHIRException { 2405 if (name.equals("identifier")) { 2406 this.getIdentifier().remove(value); 2407 } else if (name.equals("instantiatesCanonical")) { 2408 this.getInstantiatesCanonical().remove(value); 2409 } else if (name.equals("instantiatesUri")) { 2410 this.getInstantiatesUri().remove(value); 2411 } else if (name.equals("basedOn")) { 2412 this.getBasedOn().remove(value); 2413 } else if (name.equals("partOf")) { 2414 this.getPartOf().remove(value); 2415 } else if (name.equals("status")) { 2416 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2417 this.status = (Enumeration) value; // Enumeration<EventStatus> 2418 } else if (name.equals("statusReason")) { 2419 this.statusReason = null; 2420 } else if (name.equals("category")) { 2421 this.getCategory().remove(value); 2422 } else if (name.equals("code")) { 2423 this.code = null; 2424 } else if (name.equals("subject")) { 2425 this.subject = null; 2426 } else if (name.equals("focus")) { 2427 this.focus = null; 2428 } else if (name.equals("encounter")) { 2429 this.encounter = null; 2430 } else if (name.equals("occurrence[x]")) { 2431 this.occurrence = null; 2432 } else if (name.equals("recorded")) { 2433 this.recorded = null; 2434 } else if (name.equals("recorder")) { 2435 this.recorder = null; 2436 } else if (name.equals("reported[x]")) { 2437 this.reported = null; 2438 } else if (name.equals("performer")) { 2439 this.getPerformer().remove((ProcedurePerformerComponent) value); 2440 } else if (name.equals("location")) { 2441 this.location = null; 2442 } else if (name.equals("reason")) { 2443 this.getReason().remove(value); 2444 } else if (name.equals("bodySite")) { 2445 this.getBodySite().remove(value); 2446 } else if (name.equals("outcome")) { 2447 this.outcome = null; 2448 } else if (name.equals("report")) { 2449 this.getReport().remove(value); 2450 } else if (name.equals("complication")) { 2451 this.getComplication().remove(value); 2452 } else if (name.equals("followUp")) { 2453 this.getFollowUp().remove(value); 2454 } else if (name.equals("note")) { 2455 this.getNote().remove(value); 2456 } else if (name.equals("focalDevice")) { 2457 this.getFocalDevice().remove((ProcedureFocalDeviceComponent) value); 2458 } else if (name.equals("used")) { 2459 this.getUsed().remove(value); 2460 } else if (name.equals("supportingInfo")) { 2461 this.getSupportingInfo().remove(value); 2462 } else 2463 super.removeChild(name, value); 2464 2465 } 2466 2467 @Override 2468 public Base makeProperty(int hash, String name) throws FHIRException { 2469 switch (hash) { 2470 case -1618432855: return addIdentifier(); 2471 case 8911915: return addInstantiatesCanonicalElement(); 2472 case -1926393373: return addInstantiatesUriElement(); 2473 case -332612366: return addBasedOn(); 2474 case -995410646: return addPartOf(); 2475 case -892481550: return getStatusElement(); 2476 case 2051346646: return getStatusReason(); 2477 case 50511102: return addCategory(); 2478 case 3059181: return getCode(); 2479 case -1867885268: return getSubject(); 2480 case 97604824: return getFocus(); 2481 case 1524132147: return getEncounter(); 2482 case -2022646513: return getOccurrence(); 2483 case 1687874001: return getOccurrence(); 2484 case -799233872: return getRecordedElement(); 2485 case -799233858: return getRecorder(); 2486 case -241505587: return getReported(); 2487 case -427039533: return getReported(); 2488 case 481140686: return addPerformer(); 2489 case 1901043637: return getLocation(); 2490 case -934964668: return addReason(); 2491 case 1702620169: return addBodySite(); 2492 case -1106507950: return getOutcome(); 2493 case -934521548: return addReport(); 2494 case -1644401602: return addComplication(); 2495 case 301801004: return addFollowUp(); 2496 case 3387378: return addNote(); 2497 case -1129235173: return addFocalDevice(); 2498 case 3599293: return addUsed(); 2499 case 1922406657: return addSupportingInfo(); 2500 default: return super.makeProperty(hash, name); 2501 } 2502 2503 } 2504 2505 @Override 2506 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2507 switch (hash) { 2508 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2509 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 2510 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 2511 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2512 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2513 case -892481550: /*status*/ return new String[] {"code"}; 2514 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 2515 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2516 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2517 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2518 case 97604824: /*focus*/ return new String[] {"Reference"}; 2519 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2520 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "string", "Age", "Range", "Timing"}; 2521 case -799233872: /*recorded*/ return new String[] {"dateTime"}; 2522 case -799233858: /*recorder*/ return new String[] {"Reference"}; 2523 case -427039533: /*reported*/ return new String[] {"boolean", "Reference"}; 2524 case 481140686: /*performer*/ return new String[] {}; 2525 case 1901043637: /*location*/ return new String[] {"Reference"}; 2526 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 2527 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 2528 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 2529 case -934521548: /*report*/ return new String[] {"Reference"}; 2530 case -1644401602: /*complication*/ return new String[] {"CodeableReference"}; 2531 case 301801004: /*followUp*/ return new String[] {"CodeableConcept"}; 2532 case 3387378: /*note*/ return new String[] {"Annotation"}; 2533 case -1129235173: /*focalDevice*/ return new String[] {}; 2534 case 3599293: /*used*/ return new String[] {"CodeableReference"}; 2535 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2536 default: return super.getTypesForProperty(hash, name); 2537 } 2538 2539 } 2540 2541 @Override 2542 public Base addChild(String name) throws FHIRException { 2543 if (name.equals("identifier")) { 2544 return addIdentifier(); 2545 } 2546 else if (name.equals("instantiatesCanonical")) { 2547 throw new FHIRException("Cannot call addChild on a singleton property Procedure.instantiatesCanonical"); 2548 } 2549 else if (name.equals("instantiatesUri")) { 2550 throw new FHIRException("Cannot call addChild on a singleton property Procedure.instantiatesUri"); 2551 } 2552 else if (name.equals("basedOn")) { 2553 return addBasedOn(); 2554 } 2555 else if (name.equals("partOf")) { 2556 return addPartOf(); 2557 } 2558 else if (name.equals("status")) { 2559 throw new FHIRException("Cannot call addChild on a singleton property Procedure.status"); 2560 } 2561 else if (name.equals("statusReason")) { 2562 this.statusReason = new CodeableConcept(); 2563 return this.statusReason; 2564 } 2565 else if (name.equals("category")) { 2566 return addCategory(); 2567 } 2568 else if (name.equals("code")) { 2569 this.code = new CodeableConcept(); 2570 return this.code; 2571 } 2572 else if (name.equals("subject")) { 2573 this.subject = new Reference(); 2574 return this.subject; 2575 } 2576 else if (name.equals("focus")) { 2577 this.focus = new Reference(); 2578 return this.focus; 2579 } 2580 else if (name.equals("encounter")) { 2581 this.encounter = new Reference(); 2582 return this.encounter; 2583 } 2584 else if (name.equals("occurrenceDateTime")) { 2585 this.occurrence = new DateTimeType(); 2586 return this.occurrence; 2587 } 2588 else if (name.equals("occurrencePeriod")) { 2589 this.occurrence = new Period(); 2590 return this.occurrence; 2591 } 2592 else if (name.equals("occurrenceString")) { 2593 this.occurrence = new StringType(); 2594 return this.occurrence; 2595 } 2596 else if (name.equals("occurrenceAge")) { 2597 this.occurrence = new Age(); 2598 return this.occurrence; 2599 } 2600 else if (name.equals("occurrenceRange")) { 2601 this.occurrence = new Range(); 2602 return this.occurrence; 2603 } 2604 else if (name.equals("occurrenceTiming")) { 2605 this.occurrence = new Timing(); 2606 return this.occurrence; 2607 } 2608 else if (name.equals("recorded")) { 2609 throw new FHIRException("Cannot call addChild on a singleton property Procedure.recorded"); 2610 } 2611 else if (name.equals("recorder")) { 2612 this.recorder = new Reference(); 2613 return this.recorder; 2614 } 2615 else if (name.equals("reportedBoolean")) { 2616 this.reported = new BooleanType(); 2617 return this.reported; 2618 } 2619 else if (name.equals("reportedReference")) { 2620 this.reported = new Reference(); 2621 return this.reported; 2622 } 2623 else if (name.equals("performer")) { 2624 return addPerformer(); 2625 } 2626 else if (name.equals("location")) { 2627 this.location = new Reference(); 2628 return this.location; 2629 } 2630 else if (name.equals("reason")) { 2631 return addReason(); 2632 } 2633 else if (name.equals("bodySite")) { 2634 return addBodySite(); 2635 } 2636 else if (name.equals("outcome")) { 2637 this.outcome = new CodeableConcept(); 2638 return this.outcome; 2639 } 2640 else if (name.equals("report")) { 2641 return addReport(); 2642 } 2643 else if (name.equals("complication")) { 2644 return addComplication(); 2645 } 2646 else if (name.equals("followUp")) { 2647 return addFollowUp(); 2648 } 2649 else if (name.equals("note")) { 2650 return addNote(); 2651 } 2652 else if (name.equals("focalDevice")) { 2653 return addFocalDevice(); 2654 } 2655 else if (name.equals("used")) { 2656 return addUsed(); 2657 } 2658 else if (name.equals("supportingInfo")) { 2659 return addSupportingInfo(); 2660 } 2661 else 2662 return super.addChild(name); 2663 } 2664 2665 public String fhirType() { 2666 return "Procedure"; 2667 2668 } 2669 2670 public Procedure copy() { 2671 Procedure dst = new Procedure(); 2672 copyValues(dst); 2673 return dst; 2674 } 2675 2676 public void copyValues(Procedure dst) { 2677 super.copyValues(dst); 2678 if (identifier != null) { 2679 dst.identifier = new ArrayList<Identifier>(); 2680 for (Identifier i : identifier) 2681 dst.identifier.add(i.copy()); 2682 }; 2683 if (instantiatesCanonical != null) { 2684 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 2685 for (CanonicalType i : instantiatesCanonical) 2686 dst.instantiatesCanonical.add(i.copy()); 2687 }; 2688 if (instantiatesUri != null) { 2689 dst.instantiatesUri = new ArrayList<UriType>(); 2690 for (UriType i : instantiatesUri) 2691 dst.instantiatesUri.add(i.copy()); 2692 }; 2693 if (basedOn != null) { 2694 dst.basedOn = new ArrayList<Reference>(); 2695 for (Reference i : basedOn) 2696 dst.basedOn.add(i.copy()); 2697 }; 2698 if (partOf != null) { 2699 dst.partOf = new ArrayList<Reference>(); 2700 for (Reference i : partOf) 2701 dst.partOf.add(i.copy()); 2702 }; 2703 dst.status = status == null ? null : status.copy(); 2704 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2705 if (category != null) { 2706 dst.category = new ArrayList<CodeableConcept>(); 2707 for (CodeableConcept i : category) 2708 dst.category.add(i.copy()); 2709 }; 2710 dst.code = code == null ? null : code.copy(); 2711 dst.subject = subject == null ? null : subject.copy(); 2712 dst.focus = focus == null ? null : focus.copy(); 2713 dst.encounter = encounter == null ? null : encounter.copy(); 2714 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2715 dst.recorded = recorded == null ? null : recorded.copy(); 2716 dst.recorder = recorder == null ? null : recorder.copy(); 2717 dst.reported = reported == null ? null : reported.copy(); 2718 if (performer != null) { 2719 dst.performer = new ArrayList<ProcedurePerformerComponent>(); 2720 for (ProcedurePerformerComponent i : performer) 2721 dst.performer.add(i.copy()); 2722 }; 2723 dst.location = location == null ? null : location.copy(); 2724 if (reason != null) { 2725 dst.reason = new ArrayList<CodeableReference>(); 2726 for (CodeableReference i : reason) 2727 dst.reason.add(i.copy()); 2728 }; 2729 if (bodySite != null) { 2730 dst.bodySite = new ArrayList<CodeableConcept>(); 2731 for (CodeableConcept i : bodySite) 2732 dst.bodySite.add(i.copy()); 2733 }; 2734 dst.outcome = outcome == null ? null : outcome.copy(); 2735 if (report != null) { 2736 dst.report = new ArrayList<Reference>(); 2737 for (Reference i : report) 2738 dst.report.add(i.copy()); 2739 }; 2740 if (complication != null) { 2741 dst.complication = new ArrayList<CodeableReference>(); 2742 for (CodeableReference i : complication) 2743 dst.complication.add(i.copy()); 2744 }; 2745 if (followUp != null) { 2746 dst.followUp = new ArrayList<CodeableConcept>(); 2747 for (CodeableConcept i : followUp) 2748 dst.followUp.add(i.copy()); 2749 }; 2750 if (note != null) { 2751 dst.note = new ArrayList<Annotation>(); 2752 for (Annotation i : note) 2753 dst.note.add(i.copy()); 2754 }; 2755 if (focalDevice != null) { 2756 dst.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2757 for (ProcedureFocalDeviceComponent i : focalDevice) 2758 dst.focalDevice.add(i.copy()); 2759 }; 2760 if (used != null) { 2761 dst.used = new ArrayList<CodeableReference>(); 2762 for (CodeableReference i : used) 2763 dst.used.add(i.copy()); 2764 }; 2765 if (supportingInfo != null) { 2766 dst.supportingInfo = new ArrayList<Reference>(); 2767 for (Reference i : supportingInfo) 2768 dst.supportingInfo.add(i.copy()); 2769 }; 2770 } 2771 2772 protected Procedure typedCopy() { 2773 return copy(); 2774 } 2775 2776 @Override 2777 public boolean equalsDeep(Base other_) { 2778 if (!super.equalsDeep(other_)) 2779 return false; 2780 if (!(other_ instanceof Procedure)) 2781 return false; 2782 Procedure o = (Procedure) other_; 2783 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 2784 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 2785 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 2786 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 2787 && compareDeep(focus, o.focus, true) && compareDeep(encounter, o.encounter, true) && compareDeep(occurrence, o.occurrence, true) 2788 && compareDeep(recorded, o.recorded, true) && compareDeep(recorder, o.recorder, true) && compareDeep(reported, o.reported, true) 2789 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) && compareDeep(reason, o.reason, true) 2790 && compareDeep(bodySite, o.bodySite, true) && compareDeep(outcome, o.outcome, true) && compareDeep(report, o.report, true) 2791 && compareDeep(complication, o.complication, true) && compareDeep(followUp, o.followUp, true) && compareDeep(note, o.note, true) 2792 && compareDeep(focalDevice, o.focalDevice, true) && compareDeep(used, o.used, true) && compareDeep(supportingInfo, o.supportingInfo, true) 2793 ; 2794 } 2795 2796 @Override 2797 public boolean equalsShallow(Base other_) { 2798 if (!super.equalsShallow(other_)) 2799 return false; 2800 if (!(other_ instanceof Procedure)) 2801 return false; 2802 Procedure o = (Procedure) other_; 2803 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 2804 && compareValues(status, o.status, true) && compareValues(recorded, o.recorded, true); 2805 } 2806 2807 public boolean isEmpty() { 2808 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 2809 , instantiatesUri, basedOn, partOf, status, statusReason, category, code, subject 2810 , focus, encounter, occurrence, recorded, recorder, reported, performer, location 2811 , reason, bodySite, outcome, report, complication, followUp, note, focalDevice 2812 , used, supportingInfo); 2813 } 2814 2815 @Override 2816 public ResourceType getResourceType() { 2817 return ResourceType.Procedure; 2818 } 2819 2820 /** 2821 * Search parameter: <b>based-on</b> 2822 * <p> 2823 * Description: <b>A request for this procedure</b><br> 2824 * Type: <b>reference</b><br> 2825 * Path: <b>Procedure.basedOn</b><br> 2826 * </p> 2827 */ 2828 @SearchParamDefinition(name="based-on", path="Procedure.basedOn", description="A request for this procedure", type="reference", target={CarePlan.class, ServiceRequest.class } ) 2829 public static final String SP_BASED_ON = "based-on"; 2830 /** 2831 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2832 * <p> 2833 * Description: <b>A request for this procedure</b><br> 2834 * Type: <b>reference</b><br> 2835 * Path: <b>Procedure.basedOn</b><br> 2836 * </p> 2837 */ 2838 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2839 2840/** 2841 * Constant for fluent queries to be used to add include statements. Specifies 2842 * the path value of "<b>Procedure:based-on</b>". 2843 */ 2844 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Procedure:based-on").toLocked(); 2845 2846 /** 2847 * Search parameter: <b>category</b> 2848 * <p> 2849 * Description: <b>Classification of the procedure</b><br> 2850 * Type: <b>token</b><br> 2851 * Path: <b>Procedure.category</b><br> 2852 * </p> 2853 */ 2854 @SearchParamDefinition(name="category", path="Procedure.category", description="Classification of the procedure", type="token" ) 2855 public static final String SP_CATEGORY = "category"; 2856 /** 2857 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2858 * <p> 2859 * Description: <b>Classification of the procedure</b><br> 2860 * Type: <b>token</b><br> 2861 * Path: <b>Procedure.category</b><br> 2862 * </p> 2863 */ 2864 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2865 2866 /** 2867 * Search parameter: <b>instantiates-canonical</b> 2868 * <p> 2869 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2870 * Type: <b>reference</b><br> 2871 * Path: <b>Procedure.instantiatesCanonical</b><br> 2872 * </p> 2873 */ 2874 @SearchParamDefinition(name="instantiates-canonical", path="Procedure.instantiatesCanonical", description="Instantiates FHIR protocol or definition", type="reference", target={ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class } ) 2875 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 2876 /** 2877 * <b>Fluent Client</b> search parameter constant for <b>instantiates-canonical</b> 2878 * <p> 2879 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2880 * Type: <b>reference</b><br> 2881 * Path: <b>Procedure.instantiatesCanonical</b><br> 2882 * </p> 2883 */ 2884 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSTANTIATES_CANONICAL); 2885 2886/** 2887 * Constant for fluent queries to be used to add include statements. Specifies 2888 * the path value of "<b>Procedure:instantiates-canonical</b>". 2889 */ 2890 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include("Procedure:instantiates-canonical").toLocked(); 2891 2892 /** 2893 * Search parameter: <b>instantiates-uri</b> 2894 * <p> 2895 * Description: <b>Instantiates external protocol or definition</b><br> 2896 * Type: <b>uri</b><br> 2897 * Path: <b>Procedure.instantiatesUri</b><br> 2898 * </p> 2899 */ 2900 @SearchParamDefinition(name="instantiates-uri", path="Procedure.instantiatesUri", description="Instantiates external protocol or definition", type="uri" ) 2901 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 2902 /** 2903 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 2904 * <p> 2905 * Description: <b>Instantiates external protocol or definition</b><br> 2906 * Type: <b>uri</b><br> 2907 * Path: <b>Procedure.instantiatesUri</b><br> 2908 * </p> 2909 */ 2910 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES_URI); 2911 2912 /** 2913 * Search parameter: <b>location</b> 2914 * <p> 2915 * Description: <b>Where the procedure happened</b><br> 2916 * Type: <b>reference</b><br> 2917 * Path: <b>Procedure.location</b><br> 2918 * </p> 2919 */ 2920 @SearchParamDefinition(name="location", path="Procedure.location", description="Where the procedure happened", type="reference", target={Location.class } ) 2921 public static final String SP_LOCATION = "location"; 2922 /** 2923 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2924 * <p> 2925 * Description: <b>Where the procedure happened</b><br> 2926 * Type: <b>reference</b><br> 2927 * Path: <b>Procedure.location</b><br> 2928 * </p> 2929 */ 2930 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2931 2932/** 2933 * Constant for fluent queries to be used to add include statements. Specifies 2934 * the path value of "<b>Procedure:location</b>". 2935 */ 2936 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Procedure:location").toLocked(); 2937 2938 /** 2939 * Search parameter: <b>part-of</b> 2940 * <p> 2941 * Description: <b>Part of referenced event</b><br> 2942 * Type: <b>reference</b><br> 2943 * Path: <b>Procedure.partOf</b><br> 2944 * </p> 2945 */ 2946 @SearchParamDefinition(name="part-of", path="Procedure.partOf", description="Part of referenced event", type="reference", target={MedicationAdministration.class, Observation.class, Procedure.class } ) 2947 public static final String SP_PART_OF = "part-of"; 2948 /** 2949 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2950 * <p> 2951 * Description: <b>Part of referenced event</b><br> 2952 * Type: <b>reference</b><br> 2953 * Path: <b>Procedure.partOf</b><br> 2954 * </p> 2955 */ 2956 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2957 2958/** 2959 * Constant for fluent queries to be used to add include statements. Specifies 2960 * the path value of "<b>Procedure:part-of</b>". 2961 */ 2962 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Procedure:part-of").toLocked(); 2963 2964 /** 2965 * Search parameter: <b>performer</b> 2966 * <p> 2967 * Description: <b>Who performed the procedure</b><br> 2968 * Type: <b>reference</b><br> 2969 * Path: <b>Procedure.performer.actor</b><br> 2970 * </p> 2971 */ 2972 @SearchParamDefinition(name="performer", path="Procedure.performer.actor", description="Who performed the procedure", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2973 public static final String SP_PERFORMER = "performer"; 2974 /** 2975 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2976 * <p> 2977 * Description: <b>Who performed the procedure</b><br> 2978 * Type: <b>reference</b><br> 2979 * Path: <b>Procedure.performer.actor</b><br> 2980 * </p> 2981 */ 2982 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2983 2984/** 2985 * Constant for fluent queries to be used to add include statements. Specifies 2986 * the path value of "<b>Procedure:performer</b>". 2987 */ 2988 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("Procedure:performer").toLocked(); 2989 2990 /** 2991 * Search parameter: <b>reason-code</b> 2992 * <p> 2993 * Description: <b>Reference to a concept (by class)</b><br> 2994 * Type: <b>token</b><br> 2995 * Path: <b>Procedure.reason.concept</b><br> 2996 * </p> 2997 */ 2998 @SearchParamDefinition(name="reason-code", path="Procedure.reason.concept", description="Reference to a concept (by class)", type="token" ) 2999 public static final String SP_REASON_CODE = "reason-code"; 3000 /** 3001 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 3002 * <p> 3003 * Description: <b>Reference to a concept (by class)</b><br> 3004 * Type: <b>token</b><br> 3005 * Path: <b>Procedure.reason.concept</b><br> 3006 * </p> 3007 */ 3008 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_CODE); 3009 3010 /** 3011 * Search parameter: <b>reason-reference</b> 3012 * <p> 3013 * Description: <b>Reference to a resource (by instance)</b><br> 3014 * Type: <b>reference</b><br> 3015 * Path: <b>Procedure.reason.reference</b><br> 3016 * </p> 3017 */ 3018 @SearchParamDefinition(name="reason-reference", path="Procedure.reason.reference", description="Reference to a resource (by instance)", type="reference", target={Condition.class, DiagnosticReport.class, DocumentReference.class, Observation.class, Procedure.class } ) 3019 public static final String SP_REASON_REFERENCE = "reason-reference"; 3020 /** 3021 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 3022 * <p> 3023 * Description: <b>Reference to a resource (by instance)</b><br> 3024 * Type: <b>reference</b><br> 3025 * Path: <b>Procedure.reason.reference</b><br> 3026 * </p> 3027 */ 3028 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REASON_REFERENCE); 3029 3030/** 3031 * Constant for fluent queries to be used to add include statements. Specifies 3032 * the path value of "<b>Procedure:reason-reference</b>". 3033 */ 3034 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include("Procedure:reason-reference").toLocked(); 3035 3036 /** 3037 * Search parameter: <b>report</b> 3038 * <p> 3039 * Description: <b>Any report resulting from the procedure</b><br> 3040 * Type: <b>reference</b><br> 3041 * Path: <b>Procedure.report</b><br> 3042 * </p> 3043 */ 3044 @SearchParamDefinition(name="report", path="Procedure.report", description="Any report resulting from the procedure", type="reference", target={Composition.class, DiagnosticReport.class, DocumentReference.class } ) 3045 public static final String SP_REPORT = "report"; 3046 /** 3047 * <b>Fluent Client</b> search parameter constant for <b>report</b> 3048 * <p> 3049 * Description: <b>Any report resulting from the procedure</b><br> 3050 * Type: <b>reference</b><br> 3051 * Path: <b>Procedure.report</b><br> 3052 * </p> 3053 */ 3054 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPORT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPORT); 3055 3056/** 3057 * Constant for fluent queries to be used to add include statements. Specifies 3058 * the path value of "<b>Procedure:report</b>". 3059 */ 3060 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPORT = new ca.uhn.fhir.model.api.Include("Procedure:report").toLocked(); 3061 3062 /** 3063 * Search parameter: <b>status</b> 3064 * <p> 3065 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown</b><br> 3066 * Type: <b>token</b><br> 3067 * Path: <b>Procedure.status</b><br> 3068 * </p> 3069 */ 3070 @SearchParamDefinition(name="status", path="Procedure.status", description="preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", type="token" ) 3071 public static final String SP_STATUS = "status"; 3072 /** 3073 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3074 * <p> 3075 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown</b><br> 3076 * Type: <b>token</b><br> 3077 * Path: <b>Procedure.status</b><br> 3078 * </p> 3079 */ 3080 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3081 3082 /** 3083 * Search parameter: <b>subject</b> 3084 * <p> 3085 * Description: <b>Search by subject</b><br> 3086 * Type: <b>reference</b><br> 3087 * Path: <b>Procedure.subject</b><br> 3088 * </p> 3089 */ 3090 @SearchParamDefinition(name="subject", path="Procedure.subject", description="Search by subject", type="reference", target={Device.class, Group.class, Location.class, Organization.class, Patient.class, Practitioner.class } ) 3091 public static final String SP_SUBJECT = "subject"; 3092 /** 3093 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3094 * <p> 3095 * Description: <b>Search by subject</b><br> 3096 * Type: <b>reference</b><br> 3097 * Path: <b>Procedure.subject</b><br> 3098 * </p> 3099 */ 3100 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3101 3102/** 3103 * Constant for fluent queries to be used to add include statements. Specifies 3104 * the path value of "<b>Procedure:subject</b>". 3105 */ 3106 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Procedure:subject").toLocked(); 3107 3108 /** 3109 * Search parameter: <b>code</b> 3110 * <p> 3111 * Description: <b>Multiple Resources: 3112 3113* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3114* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3115* [AuditEvent](auditevent.html): More specific code for the event 3116* [Basic](basic.html): Kind of Resource 3117* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3118* [Condition](condition.html): Code for the condition 3119* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3120* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3121* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3122* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3123* [ImagingSelection](imagingselection.html): The imaging selection status 3124* [List](list.html): What the purpose of this list is 3125* [Medication](medication.html): Returns medications for a specific code 3126* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3127* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3128* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3129* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3130* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3131* [Observation](observation.html): The code of the observation type 3132* [Procedure](procedure.html): A code to identify a procedure 3133* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3134* [Task](task.html): Search by task code 3135</b><br> 3136 * Type: <b>token</b><br> 3137 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3138 * </p> 3139 */ 3140 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3141 public static final String SP_CODE = "code"; 3142 /** 3143 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3144 * <p> 3145 * Description: <b>Multiple Resources: 3146 3147* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3148* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3149* [AuditEvent](auditevent.html): More specific code for the event 3150* [Basic](basic.html): Kind of Resource 3151* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3152* [Condition](condition.html): Code for the condition 3153* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3154* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3155* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3156* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3157* [ImagingSelection](imagingselection.html): The imaging selection status 3158* [List](list.html): What the purpose of this list is 3159* [Medication](medication.html): Returns medications for a specific code 3160* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3161* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3162* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3163* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3164* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3165* [Observation](observation.html): The code of the observation type 3166* [Procedure](procedure.html): A code to identify a procedure 3167* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3168* [Task](task.html): Search by task code 3169</b><br> 3170 * Type: <b>token</b><br> 3171 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3172 * </p> 3173 */ 3174 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3175 3176 /** 3177 * Search parameter: <b>date</b> 3178 * <p> 3179 * Description: <b>Multiple Resources: 3180 3181* [AdverseEvent](adverseevent.html): When the event occurred 3182* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3183* [Appointment](appointment.html): Appointment date/time. 3184* [AuditEvent](auditevent.html): Time when the event was recorded 3185* [CarePlan](careplan.html): Time period plan covers 3186* [CareTeam](careteam.html): A date within the coverage time period. 3187* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3188* [Composition](composition.html): Composition editing time 3189* [Consent](consent.html): When consent was agreed to 3190* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3191* [DocumentReference](documentreference.html): When this document reference was created 3192* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3193* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3194* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3195* [Flag](flag.html): Time period when flag is active 3196* [Immunization](immunization.html): Vaccination (non)-Administration Date 3197* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3198* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3199* [Invoice](invoice.html): Invoice date / posting date 3200* [List](list.html): When the list was prepared 3201* [MeasureReport](measurereport.html): The date of the measure report 3202* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3203* [Observation](observation.html): Clinically relevant time/time-period for observation 3204* [Procedure](procedure.html): When the procedure occurred or is occurring 3205* [ResearchSubject](researchsubject.html): Start and end of participation 3206* [RiskAssessment](riskassessment.html): When was assessment made? 3207* [SupplyRequest](supplyrequest.html): When the request was made 3208</b><br> 3209 * Type: <b>date</b><br> 3210 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3211 * </p> 3212 */ 3213 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 3214 public static final String SP_DATE = "date"; 3215 /** 3216 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3217 * <p> 3218 * Description: <b>Multiple Resources: 3219 3220* [AdverseEvent](adverseevent.html): When the event occurred 3221* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3222* [Appointment](appointment.html): Appointment date/time. 3223* [AuditEvent](auditevent.html): Time when the event was recorded 3224* [CarePlan](careplan.html): Time period plan covers 3225* [CareTeam](careteam.html): A date within the coverage time period. 3226* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3227* [Composition](composition.html): Composition editing time 3228* [Consent](consent.html): When consent was agreed to 3229* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3230* [DocumentReference](documentreference.html): When this document reference was created 3231* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3232* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3233* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3234* [Flag](flag.html): Time period when flag is active 3235* [Immunization](immunization.html): Vaccination (non)-Administration Date 3236* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3237* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3238* [Invoice](invoice.html): Invoice date / posting date 3239* [List](list.html): When the list was prepared 3240* [MeasureReport](measurereport.html): The date of the measure report 3241* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3242* [Observation](observation.html): Clinically relevant time/time-period for observation 3243* [Procedure](procedure.html): When the procedure occurred or is occurring 3244* [ResearchSubject](researchsubject.html): Start and end of participation 3245* [RiskAssessment](riskassessment.html): When was assessment made? 3246* [SupplyRequest](supplyrequest.html): When the request was made 3247</b><br> 3248 * Type: <b>date</b><br> 3249 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3250 * </p> 3251 */ 3252 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3253 3254 /** 3255 * Search parameter: <b>encounter</b> 3256 * <p> 3257 * Description: <b>Multiple Resources: 3258 3259* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3260* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3261* [ChargeItem](chargeitem.html): Encounter associated with event 3262* [Claim](claim.html): Encounters associated with a billed line item 3263* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3264* [Communication](communication.html): The Encounter during which this Communication was created 3265* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3266* [Composition](composition.html): Context of the Composition 3267* [Condition](condition.html): The Encounter during which this Condition was created 3268* [DeviceRequest](devicerequest.html): Encounter during which request was created 3269* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3270* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3271* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3272* [Flag](flag.html): Alert relevant during encounter 3273* [ImagingStudy](imagingstudy.html): The context of the study 3274* [List](list.html): Context in which list created 3275* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3276* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3277* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3278* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3279* [Observation](observation.html): Encounter related to the observation 3280* [Procedure](procedure.html): The Encounter during which this Procedure was created 3281* [Provenance](provenance.html): Encounter related to the Provenance 3282* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3283* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3284* [RiskAssessment](riskassessment.html): Where was assessment performed? 3285* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3286* [Task](task.html): Search by encounter 3287* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3288</b><br> 3289 * Type: <b>reference</b><br> 3290 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3291 * </p> 3292 */ 3293 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 3294 public static final String SP_ENCOUNTER = "encounter"; 3295 /** 3296 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3297 * <p> 3298 * Description: <b>Multiple Resources: 3299 3300* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3301* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3302* [ChargeItem](chargeitem.html): Encounter associated with event 3303* [Claim](claim.html): Encounters associated with a billed line item 3304* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3305* [Communication](communication.html): The Encounter during which this Communication was created 3306* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3307* [Composition](composition.html): Context of the Composition 3308* [Condition](condition.html): The Encounter during which this Condition was created 3309* [DeviceRequest](devicerequest.html): Encounter during which request was created 3310* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3311* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3312* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3313* [Flag](flag.html): Alert relevant during encounter 3314* [ImagingStudy](imagingstudy.html): The context of the study 3315* [List](list.html): Context in which list created 3316* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3317* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3318* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3319* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3320* [Observation](observation.html): Encounter related to the observation 3321* [Procedure](procedure.html): The Encounter during which this Procedure was created 3322* [Provenance](provenance.html): Encounter related to the Provenance 3323* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3324* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3325* [RiskAssessment](riskassessment.html): Where was assessment performed? 3326* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3327* [Task](task.html): Search by encounter 3328* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3329</b><br> 3330 * Type: <b>reference</b><br> 3331 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3332 * </p> 3333 */ 3334 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3335 3336/** 3337 * Constant for fluent queries to be used to add include statements. Specifies 3338 * the path value of "<b>Procedure:encounter</b>". 3339 */ 3340 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Procedure:encounter").toLocked(); 3341 3342 /** 3343 * Search parameter: <b>identifier</b> 3344 * <p> 3345 * Description: <b>Multiple Resources: 3346 3347* [Account](account.html): Account number 3348* [AdverseEvent](adverseevent.html): Business identifier for the event 3349* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3350* [Appointment](appointment.html): An Identifier of the Appointment 3351* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3352* [Basic](basic.html): Business identifier 3353* [BodyStructure](bodystructure.html): Bodystructure identifier 3354* [CarePlan](careplan.html): External Ids for this plan 3355* [CareTeam](careteam.html): External Ids for this team 3356* [ChargeItem](chargeitem.html): Business Identifier for item 3357* [Claim](claim.html): The primary identifier of the financial resource 3358* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3359* [ClinicalImpression](clinicalimpression.html): Business identifier 3360* [Communication](communication.html): Unique identifier 3361* [CommunicationRequest](communicationrequest.html): Unique identifier 3362* [Composition](composition.html): Version-independent identifier for the Composition 3363* [Condition](condition.html): A unique identifier of the condition record 3364* [Consent](consent.html): Identifier for this record (external references) 3365* [Contract](contract.html): The identity of the contract 3366* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3367* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3368* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3369* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3370* [DeviceRequest](devicerequest.html): Business identifier for request/order 3371* [DeviceUsage](deviceusage.html): Search by identifier 3372* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3373* [DocumentReference](documentreference.html): Identifier of the attachment binary 3374* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3375* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3376* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3377* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3378* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3379* [Flag](flag.html): Business identifier 3380* [Goal](goal.html): External Ids for this goal 3381* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3382* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3383* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3384* [Immunization](immunization.html): Business identifier 3385* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3386* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3387* [Invoice](invoice.html): Business Identifier for item 3388* [List](list.html): Business identifier 3389* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3390* [Medication](medication.html): Returns medications with this external identifier 3391* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3392* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3393* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3394* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3395* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3396* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3397* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3398* [Observation](observation.html): The unique id for a particular observation 3399* [Person](person.html): A person Identifier 3400* [Procedure](procedure.html): A unique identifier for a procedure 3401* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3402* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3403* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3404* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3405* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3406* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3407* [Specimen](specimen.html): The unique identifier associated with the specimen 3408* [SupplyDelivery](supplydelivery.html): External identifier 3409* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3410* [Task](task.html): Search for a task instance by its business identifier 3411* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3412</b><br> 3413 * Type: <b>token</b><br> 3414 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3415 * </p> 3416 */ 3417 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3418 public static final String SP_IDENTIFIER = "identifier"; 3419 /** 3420 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3421 * <p> 3422 * Description: <b>Multiple Resources: 3423 3424* [Account](account.html): Account number 3425* [AdverseEvent](adverseevent.html): Business identifier for the event 3426* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3427* [Appointment](appointment.html): An Identifier of the Appointment 3428* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3429* [Basic](basic.html): Business identifier 3430* [BodyStructure](bodystructure.html): Bodystructure identifier 3431* [CarePlan](careplan.html): External Ids for this plan 3432* [CareTeam](careteam.html): External Ids for this team 3433* [ChargeItem](chargeitem.html): Business Identifier for item 3434* [Claim](claim.html): The primary identifier of the financial resource 3435* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3436* [ClinicalImpression](clinicalimpression.html): Business identifier 3437* [Communication](communication.html): Unique identifier 3438* [CommunicationRequest](communicationrequest.html): Unique identifier 3439* [Composition](composition.html): Version-independent identifier for the Composition 3440* [Condition](condition.html): A unique identifier of the condition record 3441* [Consent](consent.html): Identifier for this record (external references) 3442* [Contract](contract.html): The identity of the contract 3443* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3444* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3445* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3446* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3447* [DeviceRequest](devicerequest.html): Business identifier for request/order 3448* [DeviceUsage](deviceusage.html): Search by identifier 3449* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3450* [DocumentReference](documentreference.html): Identifier of the attachment binary 3451* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3452* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3453* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3454* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3455* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3456* [Flag](flag.html): Business identifier 3457* [Goal](goal.html): External Ids for this goal 3458* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3459* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3460* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3461* [Immunization](immunization.html): Business identifier 3462* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3463* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3464* [Invoice](invoice.html): Business Identifier for item 3465* [List](list.html): Business identifier 3466* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3467* [Medication](medication.html): Returns medications with this external identifier 3468* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3469* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3470* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3471* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3472* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3473* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3474* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3475* [Observation](observation.html): The unique id for a particular observation 3476* [Person](person.html): A person Identifier 3477* [Procedure](procedure.html): A unique identifier for a procedure 3478* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3479* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3480* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3481* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3482* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3483* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3484* [Specimen](specimen.html): The unique identifier associated with the specimen 3485* [SupplyDelivery](supplydelivery.html): External identifier 3486* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3487* [Task](task.html): Search for a task instance by its business identifier 3488* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3489</b><br> 3490 * Type: <b>token</b><br> 3491 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3492 * </p> 3493 */ 3494 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3495 3496 /** 3497 * Search parameter: <b>patient</b> 3498 * <p> 3499 * Description: <b>Multiple Resources: 3500 3501* [Account](account.html): The entity that caused the expenses 3502* [AdverseEvent](adverseevent.html): Subject impacted by event 3503* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3504* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3505* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3506* [AuditEvent](auditevent.html): Where the activity involved patient data 3507* [Basic](basic.html): Identifies the focus of this resource 3508* [BodyStructure](bodystructure.html): Who this is about 3509* [CarePlan](careplan.html): Who the care plan is for 3510* [CareTeam](careteam.html): Who care team is for 3511* [ChargeItem](chargeitem.html): Individual service was done for/to 3512* [Claim](claim.html): Patient receiving the products or services 3513* [ClaimResponse](claimresponse.html): The subject of care 3514* [ClinicalImpression](clinicalimpression.html): Patient assessed 3515* [Communication](communication.html): Focus of message 3516* [CommunicationRequest](communicationrequest.html): Focus of message 3517* [Composition](composition.html): Who and/or what the composition is about 3518* [Condition](condition.html): Who has the condition? 3519* [Consent](consent.html): Who the consent applies to 3520* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3521* [Coverage](coverage.html): Retrieve coverages for a patient 3522* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3523* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3524* [DetectedIssue](detectedissue.html): Associated patient 3525* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3526* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3527* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3528* [DocumentReference](documentreference.html): Who/what is the subject of the document 3529* [Encounter](encounter.html): The patient present at the encounter 3530* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3531* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3532* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3533* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3534* [Flag](flag.html): The identity of a subject to list flags for 3535* [Goal](goal.html): Who this goal is intended for 3536* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3537* [ImagingSelection](imagingselection.html): Who the study is about 3538* [ImagingStudy](imagingstudy.html): Who the study is about 3539* [Immunization](immunization.html): The patient for the vaccination record 3540* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3541* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3542* [Invoice](invoice.html): Recipient(s) of goods and services 3543* [List](list.html): If all resources have the same subject 3544* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3545* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3546* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3547* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3548* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3549* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3550* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3551* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3552* [Observation](observation.html): The subject that the observation is about (if patient) 3553* [Person](person.html): The Person links to this Patient 3554* [Procedure](procedure.html): Search by subject - a patient 3555* [Provenance](provenance.html): Where the activity involved patient data 3556* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3557* [RelatedPerson](relatedperson.html): The patient this related person is related to 3558* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3559* [ResearchSubject](researchsubject.html): Who or what is part of study 3560* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3561* [ServiceRequest](servicerequest.html): Search by subject - a patient 3562* [Specimen](specimen.html): The patient the specimen comes from 3563* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3564* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3565* [Task](task.html): Search by patient 3566* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3567</b><br> 3568 * Type: <b>reference</b><br> 3569 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3570 * </p> 3571 */ 3572 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3573 public static final String SP_PATIENT = "patient"; 3574 /** 3575 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3576 * <p> 3577 * Description: <b>Multiple Resources: 3578 3579* [Account](account.html): The entity that caused the expenses 3580* [AdverseEvent](adverseevent.html): Subject impacted by event 3581* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3582* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3583* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3584* [AuditEvent](auditevent.html): Where the activity involved patient data 3585* [Basic](basic.html): Identifies the focus of this resource 3586* [BodyStructure](bodystructure.html): Who this is about 3587* [CarePlan](careplan.html): Who the care plan is for 3588* [CareTeam](careteam.html): Who care team is for 3589* [ChargeItem](chargeitem.html): Individual service was done for/to 3590* [Claim](claim.html): Patient receiving the products or services 3591* [ClaimResponse](claimresponse.html): The subject of care 3592* [ClinicalImpression](clinicalimpression.html): Patient assessed 3593* [Communication](communication.html): Focus of message 3594* [CommunicationRequest](communicationrequest.html): Focus of message 3595* [Composition](composition.html): Who and/or what the composition is about 3596* [Condition](condition.html): Who has the condition? 3597* [Consent](consent.html): Who the consent applies to 3598* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3599* [Coverage](coverage.html): Retrieve coverages for a patient 3600* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3601* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3602* [DetectedIssue](detectedissue.html): Associated patient 3603* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3604* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3605* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3606* [DocumentReference](documentreference.html): Who/what is the subject of the document 3607* [Encounter](encounter.html): The patient present at the encounter 3608* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3609* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3610* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3611* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3612* [Flag](flag.html): The identity of a subject to list flags for 3613* [Goal](goal.html): Who this goal is intended for 3614* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3615* [ImagingSelection](imagingselection.html): Who the study is about 3616* [ImagingStudy](imagingstudy.html): Who the study is about 3617* [Immunization](immunization.html): The patient for the vaccination record 3618* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3619* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3620* [Invoice](invoice.html): Recipient(s) of goods and services 3621* [List](list.html): If all resources have the same subject 3622* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3623* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3624* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3625* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3626* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3627* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3628* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3629* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3630* [Observation](observation.html): The subject that the observation is about (if patient) 3631* [Person](person.html): The Person links to this Patient 3632* [Procedure](procedure.html): Search by subject - a patient 3633* [Provenance](provenance.html): Where the activity involved patient data 3634* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3635* [RelatedPerson](relatedperson.html): The patient this related person is related to 3636* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3637* [ResearchSubject](researchsubject.html): Who or what is part of study 3638* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3639* [ServiceRequest](servicerequest.html): Search by subject - a patient 3640* [Specimen](specimen.html): The patient the specimen comes from 3641* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3642* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3643* [Task](task.html): Search by patient 3644* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3645</b><br> 3646 * Type: <b>reference</b><br> 3647 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3648 * </p> 3649 */ 3650 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3651 3652/** 3653 * Constant for fluent queries to be used to add include statements. Specifies 3654 * the path value of "<b>Procedure:patient</b>". 3655 */ 3656 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Procedure:patient").toLocked(); 3657 3658 3659} 3660