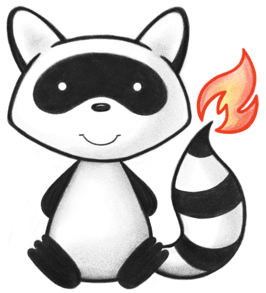
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Tue, Dec 28, 2021 07:16+1100 for FHIR v5.0.0-snapshot1 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Base StructureDefinition for ProdCharacteristic Type: The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available. 050 */ 051@DatatypeDef(name="ProdCharacteristic") 052public class ProdCharacteristic extends BackboneType implements ICompositeType { 053 054 /** 055 * Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used. 056 */ 057 @Child(name = "height", type = {Quantity.class}, order=0, min=0, max=1, modifier=false, summary=true) 058 @Description(shortDefinition="Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition="Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used." ) 059 protected Quantity height; 060 061 /** 062 * Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used. 063 */ 064 @Child(name = "width", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=true) 065 @Description(shortDefinition="Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition="Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used." ) 066 protected Quantity width; 067 068 /** 069 * Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used. 070 */ 071 @Child(name = "depth", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=true) 072 @Description(shortDefinition="Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition="Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used." ) 073 protected Quantity depth; 074 075 /** 076 * Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used. 077 */ 078 @Child(name = "weight", type = {Quantity.class}, order=3, min=0, max=1, modifier=false, summary=true) 079 @Description(shortDefinition="Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition="Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used." ) 080 protected Quantity weight; 081 082 /** 083 * Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used. 084 */ 085 @Child(name = "nominalVolume", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=true) 086 @Description(shortDefinition="Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition="Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used." ) 087 protected Quantity nominalVolume; 088 089 /** 090 * Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used. 091 */ 092 @Child(name = "externalDiameter", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=true) 093 @Description(shortDefinition="Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition="Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used." ) 094 protected Quantity externalDiameter; 095 096 /** 097 * Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used. 098 */ 099 @Child(name = "shape", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 100 @Description(shortDefinition="Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used", formalDefinition="Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used." ) 101 protected StringType shape; 102 103 /** 104 * Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used. 105 */ 106 @Child(name = "color", type = {StringType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 107 @Description(shortDefinition="Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used", formalDefinition="Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used." ) 108 protected List<StringType> color; 109 110 /** 111 * Where applicable, the imprint can be specified as text. 112 */ 113 @Child(name = "imprint", type = {StringType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 114 @Description(shortDefinition="Where applicable, the imprint can be specified as text", formalDefinition="Where applicable, the imprint can be specified as text." ) 115 protected List<StringType> imprint; 116 117 /** 118 * Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations. 119 */ 120 @Child(name = "image", type = {Attachment.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 121 @Description(shortDefinition="Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations", formalDefinition="Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations." ) 122 protected List<Attachment> image; 123 124 /** 125 * Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used. 126 */ 127 @Child(name = "scoring", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 128 @Description(shortDefinition="Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used", formalDefinition="Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used." ) 129 protected CodeableConcept scoring; 130 131 private static final long serialVersionUID = 1521671432L; 132 133 /** 134 * Constructor 135 */ 136 public ProdCharacteristic() { 137 super(); 138 } 139 140 /** 141 * @return {@link #height} (Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 142 */ 143 public Quantity getHeight() { 144 if (this.height == null) 145 if (Configuration.errorOnAutoCreate()) 146 throw new Error("Attempt to auto-create ProdCharacteristic.height"); 147 else if (Configuration.doAutoCreate()) 148 this.height = new Quantity(); // cc 149 return this.height; 150 } 151 152 public boolean hasHeight() { 153 return this.height != null && !this.height.isEmpty(); 154 } 155 156 /** 157 * @param value {@link #height} (Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 158 */ 159 public ProdCharacteristic setHeight(Quantity value) { 160 this.height = value; 161 return this; 162 } 163 164 /** 165 * @return {@link #width} (Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 166 */ 167 public Quantity getWidth() { 168 if (this.width == null) 169 if (Configuration.errorOnAutoCreate()) 170 throw new Error("Attempt to auto-create ProdCharacteristic.width"); 171 else if (Configuration.doAutoCreate()) 172 this.width = new Quantity(); // cc 173 return this.width; 174 } 175 176 public boolean hasWidth() { 177 return this.width != null && !this.width.isEmpty(); 178 } 179 180 /** 181 * @param value {@link #width} (Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 182 */ 183 public ProdCharacteristic setWidth(Quantity value) { 184 this.width = value; 185 return this; 186 } 187 188 /** 189 * @return {@link #depth} (Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 190 */ 191 public Quantity getDepth() { 192 if (this.depth == null) 193 if (Configuration.errorOnAutoCreate()) 194 throw new Error("Attempt to auto-create ProdCharacteristic.depth"); 195 else if (Configuration.doAutoCreate()) 196 this.depth = new Quantity(); // cc 197 return this.depth; 198 } 199 200 public boolean hasDepth() { 201 return this.depth != null && !this.depth.isEmpty(); 202 } 203 204 /** 205 * @param value {@link #depth} (Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 206 */ 207 public ProdCharacteristic setDepth(Quantity value) { 208 this.depth = value; 209 return this; 210 } 211 212 /** 213 * @return {@link #weight} (Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 214 */ 215 public Quantity getWeight() { 216 if (this.weight == null) 217 if (Configuration.errorOnAutoCreate()) 218 throw new Error("Attempt to auto-create ProdCharacteristic.weight"); 219 else if (Configuration.doAutoCreate()) 220 this.weight = new Quantity(); // cc 221 return this.weight; 222 } 223 224 public boolean hasWeight() { 225 return this.weight != null && !this.weight.isEmpty(); 226 } 227 228 /** 229 * @param value {@link #weight} (Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 230 */ 231 public ProdCharacteristic setWeight(Quantity value) { 232 this.weight = value; 233 return this; 234 } 235 236 /** 237 * @return {@link #nominalVolume} (Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 238 */ 239 public Quantity getNominalVolume() { 240 if (this.nominalVolume == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create ProdCharacteristic.nominalVolume"); 243 else if (Configuration.doAutoCreate()) 244 this.nominalVolume = new Quantity(); // cc 245 return this.nominalVolume; 246 } 247 248 public boolean hasNominalVolume() { 249 return this.nominalVolume != null && !this.nominalVolume.isEmpty(); 250 } 251 252 /** 253 * @param value {@link #nominalVolume} (Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 254 */ 255 public ProdCharacteristic setNominalVolume(Quantity value) { 256 this.nominalVolume = value; 257 return this; 258 } 259 260 /** 261 * @return {@link #externalDiameter} (Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 262 */ 263 public Quantity getExternalDiameter() { 264 if (this.externalDiameter == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create ProdCharacteristic.externalDiameter"); 267 else if (Configuration.doAutoCreate()) 268 this.externalDiameter = new Quantity(); // cc 269 return this.externalDiameter; 270 } 271 272 public boolean hasExternalDiameter() { 273 return this.externalDiameter != null && !this.externalDiameter.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #externalDiameter} (Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 278 */ 279 public ProdCharacteristic setExternalDiameter(Quantity value) { 280 this.externalDiameter = value; 281 return this; 282 } 283 284 /** 285 * @return {@link #shape} (Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.). This is the underlying object with id, value and extensions. The accessor "getShape" gives direct access to the value 286 */ 287 public StringType getShapeElement() { 288 if (this.shape == null) 289 if (Configuration.errorOnAutoCreate()) 290 throw new Error("Attempt to auto-create ProdCharacteristic.shape"); 291 else if (Configuration.doAutoCreate()) 292 this.shape = new StringType(); // bb 293 return this.shape; 294 } 295 296 public boolean hasShapeElement() { 297 return this.shape != null && !this.shape.isEmpty(); 298 } 299 300 public boolean hasShape() { 301 return this.shape != null && !this.shape.isEmpty(); 302 } 303 304 /** 305 * @param value {@link #shape} (Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.). This is the underlying object with id, value and extensions. The accessor "getShape" gives direct access to the value 306 */ 307 public ProdCharacteristic setShapeElement(StringType value) { 308 this.shape = value; 309 return this; 310 } 311 312 /** 313 * @return Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used. 314 */ 315 public String getShape() { 316 return this.shape == null ? null : this.shape.getValue(); 317 } 318 319 /** 320 * @param value Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used. 321 */ 322 public ProdCharacteristic setShape(String value) { 323 if (Utilities.noString(value)) 324 this.shape = null; 325 else { 326 if (this.shape == null) 327 this.shape = new StringType(); 328 this.shape.setValue(value); 329 } 330 return this; 331 } 332 333 /** 334 * @return {@link #color} (Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.) 335 */ 336 public List<StringType> getColor() { 337 if (this.color == null) 338 this.color = new ArrayList<StringType>(); 339 return this.color; 340 } 341 342 /** 343 * @return Returns a reference to <code>this</code> for easy method chaining 344 */ 345 public ProdCharacteristic setColor(List<StringType> theColor) { 346 this.color = theColor; 347 return this; 348 } 349 350 public boolean hasColor() { 351 if (this.color == null) 352 return false; 353 for (StringType item : this.color) 354 if (!item.isEmpty()) 355 return true; 356 return false; 357 } 358 359 /** 360 * @return {@link #color} (Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.) 361 */ 362 public StringType addColorElement() {//2 363 StringType t = new StringType(); 364 if (this.color == null) 365 this.color = new ArrayList<StringType>(); 366 this.color.add(t); 367 return t; 368 } 369 370 /** 371 * @param value {@link #color} (Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.) 372 */ 373 public ProdCharacteristic addColor(String value) { //1 374 StringType t = new StringType(); 375 t.setValue(value); 376 if (this.color == null) 377 this.color = new ArrayList<StringType>(); 378 this.color.add(t); 379 return this; 380 } 381 382 /** 383 * @param value {@link #color} (Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.) 384 */ 385 public boolean hasColor(String value) { 386 if (this.color == null) 387 return false; 388 for (StringType v : this.color) 389 if (v.getValue().equals(value)) // string 390 return true; 391 return false; 392 } 393 394 /** 395 * @return {@link #imprint} (Where applicable, the imprint can be specified as text.) 396 */ 397 public List<StringType> getImprint() { 398 if (this.imprint == null) 399 this.imprint = new ArrayList<StringType>(); 400 return this.imprint; 401 } 402 403 /** 404 * @return Returns a reference to <code>this</code> for easy method chaining 405 */ 406 public ProdCharacteristic setImprint(List<StringType> theImprint) { 407 this.imprint = theImprint; 408 return this; 409 } 410 411 public boolean hasImprint() { 412 if (this.imprint == null) 413 return false; 414 for (StringType item : this.imprint) 415 if (!item.isEmpty()) 416 return true; 417 return false; 418 } 419 420 /** 421 * @return {@link #imprint} (Where applicable, the imprint can be specified as text.) 422 */ 423 public StringType addImprintElement() {//2 424 StringType t = new StringType(); 425 if (this.imprint == null) 426 this.imprint = new ArrayList<StringType>(); 427 this.imprint.add(t); 428 return t; 429 } 430 431 /** 432 * @param value {@link #imprint} (Where applicable, the imprint can be specified as text.) 433 */ 434 public ProdCharacteristic addImprint(String value) { //1 435 StringType t = new StringType(); 436 t.setValue(value); 437 if (this.imprint == null) 438 this.imprint = new ArrayList<StringType>(); 439 this.imprint.add(t); 440 return this; 441 } 442 443 /** 444 * @param value {@link #imprint} (Where applicable, the imprint can be specified as text.) 445 */ 446 public boolean hasImprint(String value) { 447 if (this.imprint == null) 448 return false; 449 for (StringType v : this.imprint) 450 if (v.getValue().equals(value)) // string 451 return true; 452 return false; 453 } 454 455 /** 456 * @return {@link #image} (Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations.) 457 */ 458 public List<Attachment> getImage() { 459 if (this.image == null) 460 this.image = new ArrayList<Attachment>(); 461 return this.image; 462 } 463 464 /** 465 * @return Returns a reference to <code>this</code> for easy method chaining 466 */ 467 public ProdCharacteristic setImage(List<Attachment> theImage) { 468 this.image = theImage; 469 return this; 470 } 471 472 public boolean hasImage() { 473 if (this.image == null) 474 return false; 475 for (Attachment item : this.image) 476 if (!item.isEmpty()) 477 return true; 478 return false; 479 } 480 481 public Attachment addImage() { //3 482 Attachment t = new Attachment(); 483 if (this.image == null) 484 this.image = new ArrayList<Attachment>(); 485 this.image.add(t); 486 return t; 487 } 488 489 public ProdCharacteristic addImage(Attachment t) { //3 490 if (t == null) 491 return this; 492 if (this.image == null) 493 this.image = new ArrayList<Attachment>(); 494 this.image.add(t); 495 return this; 496 } 497 498 /** 499 * @return The first repetition of repeating field {@link #image}, creating it if it does not already exist {3} 500 */ 501 public Attachment getImageFirstRep() { 502 if (getImage().isEmpty()) { 503 addImage(); 504 } 505 return getImage().get(0); 506 } 507 508 /** 509 * @return {@link #scoring} (Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.) 510 */ 511 public CodeableConcept getScoring() { 512 if (this.scoring == null) 513 if (Configuration.errorOnAutoCreate()) 514 throw new Error("Attempt to auto-create ProdCharacteristic.scoring"); 515 else if (Configuration.doAutoCreate()) 516 this.scoring = new CodeableConcept(); // cc 517 return this.scoring; 518 } 519 520 public boolean hasScoring() { 521 return this.scoring != null && !this.scoring.isEmpty(); 522 } 523 524 /** 525 * @param value {@link #scoring} (Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.) 526 */ 527 public ProdCharacteristic setScoring(CodeableConcept value) { 528 this.scoring = value; 529 return this; 530 } 531 532 protected void listChildren(List<Property> children) { 533 super.listChildren(children); 534 children.add(new Property("height", "Quantity", "Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, height)); 535 children.add(new Property("width", "Quantity", "Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, width)); 536 children.add(new Property("depth", "Quantity", "Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, depth)); 537 children.add(new Property("weight", "Quantity", "Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, weight)); 538 children.add(new Property("nominalVolume", "Quantity", "Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, nominalVolume)); 539 children.add(new Property("externalDiameter", "Quantity", "Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, externalDiameter)); 540 children.add(new Property("shape", "string", "Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 0, 1, shape)); 541 children.add(new Property("color", "string", "Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 0, java.lang.Integer.MAX_VALUE, color)); 542 children.add(new Property("imprint", "string", "Where applicable, the imprint can be specified as text.", 0, java.lang.Integer.MAX_VALUE, imprint)); 543 children.add(new Property("image", "Attachment", "Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations.", 0, java.lang.Integer.MAX_VALUE, image)); 544 children.add(new Property("scoring", "CodeableConcept", "Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 0, 1, scoring)); 545 } 546 547 @Override 548 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 549 switch (_hash) { 550 case -1221029593: /*height*/ return new Property("height", "Quantity", "Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, height); 551 case 113126854: /*width*/ return new Property("width", "Quantity", "Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, width); 552 case 95472323: /*depth*/ return new Property("depth", "Quantity", "Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, depth); 553 case -791592328: /*weight*/ return new Property("weight", "Quantity", "Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, weight); 554 case 1706919702: /*nominalVolume*/ return new Property("nominalVolume", "Quantity", "Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, nominalVolume); 555 case 161374584: /*externalDiameter*/ return new Property("externalDiameter", "Quantity", "Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, externalDiameter); 556 case 109399969: /*shape*/ return new Property("shape", "string", "Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 0, 1, shape); 557 case 94842723: /*color*/ return new Property("color", "string", "Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 0, java.lang.Integer.MAX_VALUE, color); 558 case 1926118409: /*imprint*/ return new Property("imprint", "string", "Where applicable, the imprint can be specified as text.", 0, java.lang.Integer.MAX_VALUE, imprint); 559 case 100313435: /*image*/ return new Property("image", "Attachment", "Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations.", 0, java.lang.Integer.MAX_VALUE, image); 560 case 1924005583: /*scoring*/ return new Property("scoring", "CodeableConcept", "Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 0, 1, scoring); 561 default: return super.getNamedProperty(_hash, _name, _checkValid); 562 } 563 564 } 565 566 @Override 567 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 568 switch (hash) { 569 case -1221029593: /*height*/ return this.height == null ? new Base[0] : new Base[] {this.height}; // Quantity 570 case 113126854: /*width*/ return this.width == null ? new Base[0] : new Base[] {this.width}; // Quantity 571 case 95472323: /*depth*/ return this.depth == null ? new Base[0] : new Base[] {this.depth}; // Quantity 572 case -791592328: /*weight*/ return this.weight == null ? new Base[0] : new Base[] {this.weight}; // Quantity 573 case 1706919702: /*nominalVolume*/ return this.nominalVolume == null ? new Base[0] : new Base[] {this.nominalVolume}; // Quantity 574 case 161374584: /*externalDiameter*/ return this.externalDiameter == null ? new Base[0] : new Base[] {this.externalDiameter}; // Quantity 575 case 109399969: /*shape*/ return this.shape == null ? new Base[0] : new Base[] {this.shape}; // StringType 576 case 94842723: /*color*/ return this.color == null ? new Base[0] : this.color.toArray(new Base[this.color.size()]); // StringType 577 case 1926118409: /*imprint*/ return this.imprint == null ? new Base[0] : this.imprint.toArray(new Base[this.imprint.size()]); // StringType 578 case 100313435: /*image*/ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // Attachment 579 case 1924005583: /*scoring*/ return this.scoring == null ? new Base[0] : new Base[] {this.scoring}; // CodeableConcept 580 default: return super.getProperty(hash, name, checkValid); 581 } 582 583 } 584 585 @Override 586 public Base setProperty(int hash, String name, Base value) throws FHIRException { 587 switch (hash) { 588 case -1221029593: // height 589 this.height = TypeConvertor.castToQuantity(value); // Quantity 590 return value; 591 case 113126854: // width 592 this.width = TypeConvertor.castToQuantity(value); // Quantity 593 return value; 594 case 95472323: // depth 595 this.depth = TypeConvertor.castToQuantity(value); // Quantity 596 return value; 597 case -791592328: // weight 598 this.weight = TypeConvertor.castToQuantity(value); // Quantity 599 return value; 600 case 1706919702: // nominalVolume 601 this.nominalVolume = TypeConvertor.castToQuantity(value); // Quantity 602 return value; 603 case 161374584: // externalDiameter 604 this.externalDiameter = TypeConvertor.castToQuantity(value); // Quantity 605 return value; 606 case 109399969: // shape 607 this.shape = TypeConvertor.castToString(value); // StringType 608 return value; 609 case 94842723: // color 610 this.getColor().add(TypeConvertor.castToString(value)); // StringType 611 return value; 612 case 1926118409: // imprint 613 this.getImprint().add(TypeConvertor.castToString(value)); // StringType 614 return value; 615 case 100313435: // image 616 this.getImage().add(TypeConvertor.castToAttachment(value)); // Attachment 617 return value; 618 case 1924005583: // scoring 619 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 620 return value; 621 default: return super.setProperty(hash, name, value); 622 } 623 624 } 625 626 @Override 627 public Base setProperty(String name, Base value) throws FHIRException { 628 if (name.equals("height")) { 629 this.height = TypeConvertor.castToQuantity(value); // Quantity 630 } else if (name.equals("width")) { 631 this.width = TypeConvertor.castToQuantity(value); // Quantity 632 } else if (name.equals("depth")) { 633 this.depth = TypeConvertor.castToQuantity(value); // Quantity 634 } else if (name.equals("weight")) { 635 this.weight = TypeConvertor.castToQuantity(value); // Quantity 636 } else if (name.equals("nominalVolume")) { 637 this.nominalVolume = TypeConvertor.castToQuantity(value); // Quantity 638 } else if (name.equals("externalDiameter")) { 639 this.externalDiameter = TypeConvertor.castToQuantity(value); // Quantity 640 } else if (name.equals("shape")) { 641 this.shape = TypeConvertor.castToString(value); // StringType 642 } else if (name.equals("color")) { 643 this.getColor().add(TypeConvertor.castToString(value)); 644 } else if (name.equals("imprint")) { 645 this.getImprint().add(TypeConvertor.castToString(value)); 646 } else if (name.equals("image")) { 647 this.getImage().add(TypeConvertor.castToAttachment(value)); 648 } else if (name.equals("scoring")) { 649 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 650 } else 651 return super.setProperty(name, value); 652 return value; 653 } 654 655 @Override 656 public void removeChild(String name, Base value) throws FHIRException { 657 if (name.equals("height")) { 658 this.height = null; 659 } else if (name.equals("width")) { 660 this.width = null; 661 } else if (name.equals("depth")) { 662 this.depth = null; 663 } else if (name.equals("weight")) { 664 this.weight = null; 665 } else if (name.equals("nominalVolume")) { 666 this.nominalVolume = null; 667 } else if (name.equals("externalDiameter")) { 668 this.externalDiameter = null; 669 } else if (name.equals("shape")) { 670 this.shape = null; 671 } else if (name.equals("color")) { 672 this.getColor().remove(value); 673 } else if (name.equals("imprint")) { 674 this.getImprint().remove(value); 675 } else if (name.equals("image")) { 676 this.getImage().remove(value); 677 } else if (name.equals("scoring")) { 678 this.scoring = null; 679 } else 680 super.removeChild(name, value); 681 682 } 683 684 @Override 685 public Base makeProperty(int hash, String name) throws FHIRException { 686 switch (hash) { 687 case -1221029593: return getHeight(); 688 case 113126854: return getWidth(); 689 case 95472323: return getDepth(); 690 case -791592328: return getWeight(); 691 case 1706919702: return getNominalVolume(); 692 case 161374584: return getExternalDiameter(); 693 case 109399969: return getShapeElement(); 694 case 94842723: return addColorElement(); 695 case 1926118409: return addImprintElement(); 696 case 100313435: return addImage(); 697 case 1924005583: return getScoring(); 698 default: return super.makeProperty(hash, name); 699 } 700 701 } 702 703 @Override 704 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 705 switch (hash) { 706 case -1221029593: /*height*/ return new String[] {"Quantity"}; 707 case 113126854: /*width*/ return new String[] {"Quantity"}; 708 case 95472323: /*depth*/ return new String[] {"Quantity"}; 709 case -791592328: /*weight*/ return new String[] {"Quantity"}; 710 case 1706919702: /*nominalVolume*/ return new String[] {"Quantity"}; 711 case 161374584: /*externalDiameter*/ return new String[] {"Quantity"}; 712 case 109399969: /*shape*/ return new String[] {"string"}; 713 case 94842723: /*color*/ return new String[] {"string"}; 714 case 1926118409: /*imprint*/ return new String[] {"string"}; 715 case 100313435: /*image*/ return new String[] {"Attachment"}; 716 case 1924005583: /*scoring*/ return new String[] {"CodeableConcept"}; 717 default: return super.getTypesForProperty(hash, name); 718 } 719 720 } 721 722 @Override 723 public Base addChild(String name) throws FHIRException { 724 if (name.equals("height")) { 725 this.height = new Quantity(); 726 return this.height; 727 } 728 else if (name.equals("width")) { 729 this.width = new Quantity(); 730 return this.width; 731 } 732 else if (name.equals("depth")) { 733 this.depth = new Quantity(); 734 return this.depth; 735 } 736 else if (name.equals("weight")) { 737 this.weight = new Quantity(); 738 return this.weight; 739 } 740 else if (name.equals("nominalVolume")) { 741 this.nominalVolume = new Quantity(); 742 return this.nominalVolume; 743 } 744 else if (name.equals("externalDiameter")) { 745 this.externalDiameter = new Quantity(); 746 return this.externalDiameter; 747 } 748 else if (name.equals("shape")) { 749 throw new FHIRException("Cannot call addChild on a singleton property ProdCharacteristic.shape"); 750 } 751 else if (name.equals("color")) { 752 throw new FHIRException("Cannot call addChild on a singleton property ProdCharacteristic.color"); 753 } 754 else if (name.equals("imprint")) { 755 throw new FHIRException("Cannot call addChild on a singleton property ProdCharacteristic.imprint"); 756 } 757 else if (name.equals("image")) { 758 return addImage(); 759 } 760 else if (name.equals("scoring")) { 761 this.scoring = new CodeableConcept(); 762 return this.scoring; 763 } 764 else 765 return super.addChild(name); 766 } 767 768 public String fhirType() { 769 return "ProdCharacteristic"; 770 771 } 772 773 public ProdCharacteristic copy() { 774 ProdCharacteristic dst = new ProdCharacteristic(); 775 copyValues(dst); 776 return dst; 777 } 778 779 public void copyValues(ProdCharacteristic dst) { 780 super.copyValues(dst); 781 dst.height = height == null ? null : height.copy(); 782 dst.width = width == null ? null : width.copy(); 783 dst.depth = depth == null ? null : depth.copy(); 784 dst.weight = weight == null ? null : weight.copy(); 785 dst.nominalVolume = nominalVolume == null ? null : nominalVolume.copy(); 786 dst.externalDiameter = externalDiameter == null ? null : externalDiameter.copy(); 787 dst.shape = shape == null ? null : shape.copy(); 788 if (color != null) { 789 dst.color = new ArrayList<StringType>(); 790 for (StringType i : color) 791 dst.color.add(i.copy()); 792 }; 793 if (imprint != null) { 794 dst.imprint = new ArrayList<StringType>(); 795 for (StringType i : imprint) 796 dst.imprint.add(i.copy()); 797 }; 798 if (image != null) { 799 dst.image = new ArrayList<Attachment>(); 800 for (Attachment i : image) 801 dst.image.add(i.copy()); 802 }; 803 dst.scoring = scoring == null ? null : scoring.copy(); 804 } 805 806 protected ProdCharacteristic typedCopy() { 807 return copy(); 808 } 809 810 @Override 811 public boolean equalsDeep(Base other_) { 812 if (!super.equalsDeep(other_)) 813 return false; 814 if (!(other_ instanceof ProdCharacteristic)) 815 return false; 816 ProdCharacteristic o = (ProdCharacteristic) other_; 817 return compareDeep(height, o.height, true) && compareDeep(width, o.width, true) && compareDeep(depth, o.depth, true) 818 && compareDeep(weight, o.weight, true) && compareDeep(nominalVolume, o.nominalVolume, true) && compareDeep(externalDiameter, o.externalDiameter, true) 819 && compareDeep(shape, o.shape, true) && compareDeep(color, o.color, true) && compareDeep(imprint, o.imprint, true) 820 && compareDeep(image, o.image, true) && compareDeep(scoring, o.scoring, true); 821 } 822 823 @Override 824 public boolean equalsShallow(Base other_) { 825 if (!super.equalsShallow(other_)) 826 return false; 827 if (!(other_ instanceof ProdCharacteristic)) 828 return false; 829 ProdCharacteristic o = (ProdCharacteristic) other_; 830 return compareValues(shape, o.shape, true) && compareValues(color, o.color, true) && compareValues(imprint, o.imprint, true) 831 ; 832 } 833 834 public boolean isEmpty() { 835 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(height, width, depth, weight 836 , nominalVolume, externalDiameter, shape, color, imprint, image, scoring); 837 } 838 839 840} 841