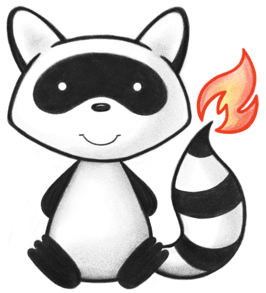
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047/** 048 * ProductShelfLife Type: The shelf-life and storage information for a medicinal product item or container can be described using this class. 049 */ 050@DatatypeDef(name="ProductShelfLife") 051public class ProductShelfLife extends BackboneType implements ICompositeType { 052 053 /** 054 * This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified. 055 */ 056 @Child(name = "type", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified", formalDefinition="This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified." ) 058 protected CodeableConcept type; 059 060 /** 061 * The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used. 062 */ 063 @Child(name = "period", type = {Duration.class, StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition="The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used." ) 065 protected DataType period; 066 067 /** 068 * Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified. 069 */ 070 @Child(name = "specialPrecautionsForStorage", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 071 @Description(shortDefinition="Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified", formalDefinition="Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified." ) 072 protected List<CodeableConcept> specialPrecautionsForStorage; 073 074 private static final long serialVersionUID = 675017411L; 075 076 /** 077 * Constructor 078 */ 079 public ProductShelfLife() { 080 super(); 081 } 082 083 /** 084 * @return {@link #type} (This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.) 085 */ 086 public CodeableConcept getType() { 087 if (this.type == null) 088 if (Configuration.errorOnAutoCreate()) 089 throw new Error("Attempt to auto-create ProductShelfLife.type"); 090 else if (Configuration.doAutoCreate()) 091 this.type = new CodeableConcept(); // cc 092 return this.type; 093 } 094 095 public boolean hasType() { 096 return this.type != null && !this.type.isEmpty(); 097 } 098 099 /** 100 * @param value {@link #type} (This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.) 101 */ 102 public ProductShelfLife setType(CodeableConcept value) { 103 this.type = value; 104 return this; 105 } 106 107 /** 108 * @return {@link #period} (The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 109 */ 110 public DataType getPeriod() { 111 return this.period; 112 } 113 114 /** 115 * @return {@link #period} (The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 116 */ 117 public Duration getPeriodDuration() throws FHIRException { 118 if (this.period == null) 119 this.period = new Duration(); 120 if (!(this.period instanceof Duration)) 121 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.period.getClass().getName()+" was encountered"); 122 return (Duration) this.period; 123 } 124 125 public boolean hasPeriodDuration() { 126 return this != null && this.period instanceof Duration; 127 } 128 129 /** 130 * @return {@link #period} (The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 131 */ 132 public StringType getPeriodStringType() throws FHIRException { 133 if (this.period == null) 134 this.period = new StringType(); 135 if (!(this.period instanceof StringType)) 136 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.period.getClass().getName()+" was encountered"); 137 return (StringType) this.period; 138 } 139 140 public boolean hasPeriodStringType() { 141 return this != null && this.period instanceof StringType; 142 } 143 144 public boolean hasPeriod() { 145 return this.period != null && !this.period.isEmpty(); 146 } 147 148 /** 149 * @param value {@link #period} (The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.) 150 */ 151 public ProductShelfLife setPeriod(DataType value) { 152 if (value != null && !(value instanceof Duration || value instanceof StringType)) 153 throw new FHIRException("Not the right type for ProductShelfLife.period[x]: "+value.fhirType()); 154 this.period = value; 155 return this; 156 } 157 158 /** 159 * @return {@link #specialPrecautionsForStorage} (Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.) 160 */ 161 public List<CodeableConcept> getSpecialPrecautionsForStorage() { 162 if (this.specialPrecautionsForStorage == null) 163 this.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 164 return this.specialPrecautionsForStorage; 165 } 166 167 /** 168 * @return Returns a reference to <code>this</code> for easy method chaining 169 */ 170 public ProductShelfLife setSpecialPrecautionsForStorage(List<CodeableConcept> theSpecialPrecautionsForStorage) { 171 this.specialPrecautionsForStorage = theSpecialPrecautionsForStorage; 172 return this; 173 } 174 175 public boolean hasSpecialPrecautionsForStorage() { 176 if (this.specialPrecautionsForStorage == null) 177 return false; 178 for (CodeableConcept item : this.specialPrecautionsForStorage) 179 if (!item.isEmpty()) 180 return true; 181 return false; 182 } 183 184 public CodeableConcept addSpecialPrecautionsForStorage() { //3 185 CodeableConcept t = new CodeableConcept(); 186 if (this.specialPrecautionsForStorage == null) 187 this.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 188 this.specialPrecautionsForStorage.add(t); 189 return t; 190 } 191 192 public ProductShelfLife addSpecialPrecautionsForStorage(CodeableConcept t) { //3 193 if (t == null) 194 return this; 195 if (this.specialPrecautionsForStorage == null) 196 this.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 197 this.specialPrecautionsForStorage.add(t); 198 return this; 199 } 200 201 /** 202 * @return The first repetition of repeating field {@link #specialPrecautionsForStorage}, creating it if it does not already exist {3} 203 */ 204 public CodeableConcept getSpecialPrecautionsForStorageFirstRep() { 205 if (getSpecialPrecautionsForStorage().isEmpty()) { 206 addSpecialPrecautionsForStorage(); 207 } 208 return getSpecialPrecautionsForStorage().get(0); 209 } 210 211 protected void listChildren(List<Property> children) { 212 super.listChildren(children); 213 children.add(new Property("type", "CodeableConcept", "This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 0, 1, type)); 214 children.add(new Property("period[x]", "Duration|string", "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, period)); 215 children.add(new Property("specialPrecautionsForStorage", "CodeableConcept", "Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 0, java.lang.Integer.MAX_VALUE, specialPrecautionsForStorage)); 216 } 217 218 @Override 219 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 220 switch (_hash) { 221 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 0, 1, type); 222 case 566594335: /*period[x]*/ return new Property("period[x]", "Duration|string", "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, period); 223 case -991726143: /*period*/ return new Property("period[x]", "Duration|string", "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, period); 224 case -850078091: /*periodDuration*/ return new Property("period[x]", "Duration", "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, period); 225 case -41636558: /*periodString*/ return new Property("period[x]", "string", "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 0, 1, period); 226 case 2103459492: /*specialPrecautionsForStorage*/ return new Property("specialPrecautionsForStorage", "CodeableConcept", "Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 0, java.lang.Integer.MAX_VALUE, specialPrecautionsForStorage); 227 default: return super.getNamedProperty(_hash, _name, _checkValid); 228 } 229 230 } 231 232 @Override 233 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 234 switch (hash) { 235 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 236 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // DataType 237 case 2103459492: /*specialPrecautionsForStorage*/ return this.specialPrecautionsForStorage == null ? new Base[0] : this.specialPrecautionsForStorage.toArray(new Base[this.specialPrecautionsForStorage.size()]); // CodeableConcept 238 default: return super.getProperty(hash, name, checkValid); 239 } 240 241 } 242 243 @Override 244 public Base setProperty(int hash, String name, Base value) throws FHIRException { 245 switch (hash) { 246 case 3575610: // type 247 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 248 return value; 249 case -991726143: // period 250 this.period = TypeConvertor.castToType(value); // DataType 251 return value; 252 case 2103459492: // specialPrecautionsForStorage 253 this.getSpecialPrecautionsForStorage().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 254 return value; 255 default: return super.setProperty(hash, name, value); 256 } 257 258 } 259 260 @Override 261 public Base setProperty(String name, Base value) throws FHIRException { 262 if (name.equals("type")) { 263 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 264 } else if (name.equals("period[x]")) { 265 this.period = TypeConvertor.castToType(value); // DataType 266 } else if (name.equals("specialPrecautionsForStorage")) { 267 this.getSpecialPrecautionsForStorage().add(TypeConvertor.castToCodeableConcept(value)); 268 } else 269 return super.setProperty(name, value); 270 return value; 271 } 272 273 @Override 274 public void removeChild(String name, Base value) throws FHIRException { 275 if (name.equals("type")) { 276 this.type = null; 277 } else if (name.equals("period[x]")) { 278 this.period = null; 279 } else if (name.equals("specialPrecautionsForStorage")) { 280 this.getSpecialPrecautionsForStorage().remove(value); 281 } else 282 super.removeChild(name, value); 283 284 } 285 286 @Override 287 public Base makeProperty(int hash, String name) throws FHIRException { 288 switch (hash) { 289 case 3575610: return getType(); 290 case 566594335: return getPeriod(); 291 case -991726143: return getPeriod(); 292 case 2103459492: return addSpecialPrecautionsForStorage(); 293 default: return super.makeProperty(hash, name); 294 } 295 296 } 297 298 @Override 299 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 300 switch (hash) { 301 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 302 case -991726143: /*period*/ return new String[] {"Duration", "string"}; 303 case 2103459492: /*specialPrecautionsForStorage*/ return new String[] {"CodeableConcept"}; 304 default: return super.getTypesForProperty(hash, name); 305 } 306 307 } 308 309 @Override 310 public Base addChild(String name) throws FHIRException { 311 if (name.equals("type")) { 312 this.type = new CodeableConcept(); 313 return this.type; 314 } 315 else if (name.equals("periodDuration")) { 316 this.period = new Duration(); 317 return this.period; 318 } 319 else if (name.equals("periodString")) { 320 this.period = new StringType(); 321 return this.period; 322 } 323 else if (name.equals("specialPrecautionsForStorage")) { 324 return addSpecialPrecautionsForStorage(); 325 } 326 else 327 return super.addChild(name); 328 } 329 330 public String fhirType() { 331 return "ProductShelfLife"; 332 333 } 334 335 public ProductShelfLife copy() { 336 ProductShelfLife dst = new ProductShelfLife(); 337 copyValues(dst); 338 return dst; 339 } 340 341 public void copyValues(ProductShelfLife dst) { 342 super.copyValues(dst); 343 dst.type = type == null ? null : type.copy(); 344 dst.period = period == null ? null : period.copy(); 345 if (specialPrecautionsForStorage != null) { 346 dst.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 347 for (CodeableConcept i : specialPrecautionsForStorage) 348 dst.specialPrecautionsForStorage.add(i.copy()); 349 }; 350 } 351 352 protected ProductShelfLife typedCopy() { 353 return copy(); 354 } 355 356 @Override 357 public boolean equalsDeep(Base other_) { 358 if (!super.equalsDeep(other_)) 359 return false; 360 if (!(other_ instanceof ProductShelfLife)) 361 return false; 362 ProductShelfLife o = (ProductShelfLife) other_; 363 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) && compareDeep(specialPrecautionsForStorage, o.specialPrecautionsForStorage, true) 364 ; 365 } 366 367 @Override 368 public boolean equalsShallow(Base other_) { 369 if (!super.equalsShallow(other_)) 370 return false; 371 if (!(other_ instanceof ProductShelfLife)) 372 return false; 373 ProductShelfLife o = (ProductShelfLife) other_; 374 return true; 375 } 376 377 public boolean isEmpty() { 378 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, specialPrecautionsForStorage 379 ); 380 } 381 382 383} 384