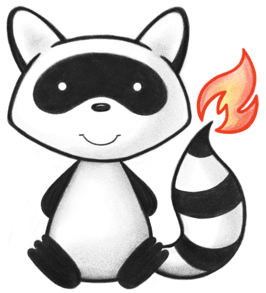
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 052 */ 053@ResourceDef(name="Provenance", profile="http://hl7.org/fhir/StructureDefinition/Provenance") 054public class Provenance extends DomainResource { 055 056 public enum ProvenanceEntityRole { 057 /** 058 * An entity that is used by the activity to produce a new version of that entity. 059 */ 060 REVISION, 061 /** 062 * An entity that is copied in full or part by an agent that is not the author of the entity. 063 */ 064 QUOTATION, 065 /** 066 * An entity that is used as input to the activity that produced the target. 067 */ 068 SOURCE, 069 /** 070 * The record resulting from this event adheres to the protocol, guideline, order set or other definition represented by this entity. 071 */ 072 INSTANTIATES, 073 /** 074 * An entity that is removed from accessibility, usually through the DELETE operator. 075 */ 076 REMOVAL, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static ProvenanceEntityRole fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("revision".equals(codeString)) 085 return REVISION; 086 if ("quotation".equals(codeString)) 087 return QUOTATION; 088 if ("source".equals(codeString)) 089 return SOURCE; 090 if ("instantiates".equals(codeString)) 091 return INSTANTIATES; 092 if ("removal".equals(codeString)) 093 return REMOVAL; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown ProvenanceEntityRole code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case REVISION: return "revision"; 102 case QUOTATION: return "quotation"; 103 case SOURCE: return "source"; 104 case INSTANTIATES: return "instantiates"; 105 case REMOVAL: return "removal"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case REVISION: return "http://hl7.org/fhir/provenance-entity-role"; 113 case QUOTATION: return "http://hl7.org/fhir/provenance-entity-role"; 114 case SOURCE: return "http://hl7.org/fhir/provenance-entity-role"; 115 case INSTANTIATES: return "http://hl7.org/fhir/provenance-entity-role"; 116 case REMOVAL: return "http://hl7.org/fhir/provenance-entity-role"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case REVISION: return "An entity that is used by the activity to produce a new version of that entity."; 124 case QUOTATION: return "An entity that is copied in full or part by an agent that is not the author of the entity."; 125 case SOURCE: return "An entity that is used as input to the activity that produced the target."; 126 case INSTANTIATES: return "The record resulting from this event adheres to the protocol, guideline, order set or other definition represented by this entity."; 127 case REMOVAL: return "An entity that is removed from accessibility, usually through the DELETE operator."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case REVISION: return "Revision"; 135 case QUOTATION: return "Quotation"; 136 case SOURCE: return "Source"; 137 case INSTANTIATES: return "Instantiates"; 138 case REMOVAL: return "Removal"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class ProvenanceEntityRoleEnumFactory implements EnumFactory<ProvenanceEntityRole> { 146 public ProvenanceEntityRole fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("revision".equals(codeString)) 151 return ProvenanceEntityRole.REVISION; 152 if ("quotation".equals(codeString)) 153 return ProvenanceEntityRole.QUOTATION; 154 if ("source".equals(codeString)) 155 return ProvenanceEntityRole.SOURCE; 156 if ("instantiates".equals(codeString)) 157 return ProvenanceEntityRole.INSTANTIATES; 158 if ("removal".equals(codeString)) 159 return ProvenanceEntityRole.REMOVAL; 160 throw new IllegalArgumentException("Unknown ProvenanceEntityRole code '"+codeString+"'"); 161 } 162 public Enumeration<ProvenanceEntityRole> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.NULL, code); 170 if ("revision".equals(codeString)) 171 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.REVISION, code); 172 if ("quotation".equals(codeString)) 173 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.QUOTATION, code); 174 if ("source".equals(codeString)) 175 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.SOURCE, code); 176 if ("instantiates".equals(codeString)) 177 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.INSTANTIATES, code); 178 if ("removal".equals(codeString)) 179 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.REMOVAL, code); 180 throw new FHIRException("Unknown ProvenanceEntityRole code '"+codeString+"'"); 181 } 182 public String toCode(ProvenanceEntityRole code) { 183 if (code == ProvenanceEntityRole.NULL) 184 return null; 185 if (code == ProvenanceEntityRole.REVISION) 186 return "revision"; 187 if (code == ProvenanceEntityRole.QUOTATION) 188 return "quotation"; 189 if (code == ProvenanceEntityRole.SOURCE) 190 return "source"; 191 if (code == ProvenanceEntityRole.INSTANTIATES) 192 return "instantiates"; 193 if (code == ProvenanceEntityRole.REMOVAL) 194 return "removal"; 195 return "?"; 196 } 197 public String toSystem(ProvenanceEntityRole code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class ProvenanceAgentComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * The Functional Role of the agent with respect to the activity. 206 */ 207 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 208 @Description(shortDefinition="How the agent participated", formalDefinition="The Functional Role of the agent with respect to the activity." ) 209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 210 protected CodeableConcept type; 211 212 /** 213 * The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity. 214 */ 215 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 216 @Description(shortDefinition="What the agents role was", formalDefinition="The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity." ) 217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 218 protected List<CodeableConcept> role; 219 220 /** 221 * Indicates who or what performed in the event. 222 */ 223 @Child(name = "who", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class}, order=3, min=1, max=1, modifier=false, summary=true) 224 @Description(shortDefinition="The agent that participated in the event", formalDefinition="Indicates who or what performed in the event." ) 225 protected Reference who; 226 227 /** 228 * The agent that delegated authority to perform the activity performed by the agent.who element. 229 */ 230 @Child(name = "onBehalfOf", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class}, order=4, min=0, max=1, modifier=false, summary=false) 231 @Description(shortDefinition="The agent that delegated", formalDefinition="The agent that delegated authority to perform the activity performed by the agent.who element." ) 232 protected Reference onBehalfOf; 233 234 private static final long serialVersionUID = 642650054L; 235 236 /** 237 * Constructor 238 */ 239 public ProvenanceAgentComponent() { 240 super(); 241 } 242 243 /** 244 * Constructor 245 */ 246 public ProvenanceAgentComponent(Reference who) { 247 super(); 248 this.setWho(who); 249 } 250 251 /** 252 * @return {@link #type} (The Functional Role of the agent with respect to the activity.) 253 */ 254 public CodeableConcept getType() { 255 if (this.type == null) 256 if (Configuration.errorOnAutoCreate()) 257 throw new Error("Attempt to auto-create ProvenanceAgentComponent.type"); 258 else if (Configuration.doAutoCreate()) 259 this.type = new CodeableConcept(); // cc 260 return this.type; 261 } 262 263 public boolean hasType() { 264 return this.type != null && !this.type.isEmpty(); 265 } 266 267 /** 268 * @param value {@link #type} (The Functional Role of the agent with respect to the activity.) 269 */ 270 public ProvenanceAgentComponent setType(CodeableConcept value) { 271 this.type = value; 272 return this; 273 } 274 275 /** 276 * @return {@link #role} (The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity.) 277 */ 278 public List<CodeableConcept> getRole() { 279 if (this.role == null) 280 this.role = new ArrayList<CodeableConcept>(); 281 return this.role; 282 } 283 284 /** 285 * @return Returns a reference to <code>this</code> for easy method chaining 286 */ 287 public ProvenanceAgentComponent setRole(List<CodeableConcept> theRole) { 288 this.role = theRole; 289 return this; 290 } 291 292 public boolean hasRole() { 293 if (this.role == null) 294 return false; 295 for (CodeableConcept item : this.role) 296 if (!item.isEmpty()) 297 return true; 298 return false; 299 } 300 301 public CodeableConcept addRole() { //3 302 CodeableConcept t = new CodeableConcept(); 303 if (this.role == null) 304 this.role = new ArrayList<CodeableConcept>(); 305 this.role.add(t); 306 return t; 307 } 308 309 public ProvenanceAgentComponent addRole(CodeableConcept t) { //3 310 if (t == null) 311 return this; 312 if (this.role == null) 313 this.role = new ArrayList<CodeableConcept>(); 314 this.role.add(t); 315 return this; 316 } 317 318 /** 319 * @return The first repetition of repeating field {@link #role}, creating it if it does not already exist {3} 320 */ 321 public CodeableConcept getRoleFirstRep() { 322 if (getRole().isEmpty()) { 323 addRole(); 324 } 325 return getRole().get(0); 326 } 327 328 /** 329 * @return {@link #who} (Indicates who or what performed in the event.) 330 */ 331 public Reference getWho() { 332 if (this.who == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create ProvenanceAgentComponent.who"); 335 else if (Configuration.doAutoCreate()) 336 this.who = new Reference(); // cc 337 return this.who; 338 } 339 340 public boolean hasWho() { 341 return this.who != null && !this.who.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #who} (Indicates who or what performed in the event.) 346 */ 347 public ProvenanceAgentComponent setWho(Reference value) { 348 this.who = value; 349 return this; 350 } 351 352 /** 353 * @return {@link #onBehalfOf} (The agent that delegated authority to perform the activity performed by the agent.who element.) 354 */ 355 public Reference getOnBehalfOf() { 356 if (this.onBehalfOf == null) 357 if (Configuration.errorOnAutoCreate()) 358 throw new Error("Attempt to auto-create ProvenanceAgentComponent.onBehalfOf"); 359 else if (Configuration.doAutoCreate()) 360 this.onBehalfOf = new Reference(); // cc 361 return this.onBehalfOf; 362 } 363 364 public boolean hasOnBehalfOf() { 365 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 366 } 367 368 /** 369 * @param value {@link #onBehalfOf} (The agent that delegated authority to perform the activity performed by the agent.who element.) 370 */ 371 public ProvenanceAgentComponent setOnBehalfOf(Reference value) { 372 this.onBehalfOf = value; 373 return this; 374 } 375 376 protected void listChildren(List<Property> children) { 377 super.listChildren(children); 378 children.add(new Property("type", "CodeableConcept", "The Functional Role of the agent with respect to the activity.", 0, 1, type)); 379 children.add(new Property("role", "CodeableConcept", "The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity.", 0, java.lang.Integer.MAX_VALUE, role)); 380 children.add(new Property("who", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Indicates who or what performed in the event.", 0, 1, who)); 381 children.add(new Property("onBehalfOf", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient)", "The agent that delegated authority to perform the activity performed by the agent.who element.", 0, 1, onBehalfOf)); 382 } 383 384 @Override 385 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 386 switch (_hash) { 387 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The Functional Role of the agent with respect to the activity.", 0, 1, type); 388 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity.", 0, java.lang.Integer.MAX_VALUE, role); 389 case 117694: /*who*/ return new Property("who", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Indicates who or what performed in the event.", 0, 1, who); 390 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient)", "The agent that delegated authority to perform the activity performed by the agent.who element.", 0, 1, onBehalfOf); 391 default: return super.getNamedProperty(_hash, _name, _checkValid); 392 } 393 394 } 395 396 @Override 397 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 398 switch (hash) { 399 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 400 case 3506294: /*role*/ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 401 case 117694: /*who*/ return this.who == null ? new Base[0] : new Base[] {this.who}; // Reference 402 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 403 default: return super.getProperty(hash, name, checkValid); 404 } 405 406 } 407 408 @Override 409 public Base setProperty(int hash, String name, Base value) throws FHIRException { 410 switch (hash) { 411 case 3575610: // type 412 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 413 return value; 414 case 3506294: // role 415 this.getRole().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 416 return value; 417 case 117694: // who 418 this.who = TypeConvertor.castToReference(value); // Reference 419 return value; 420 case -14402964: // onBehalfOf 421 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 422 return value; 423 default: return super.setProperty(hash, name, value); 424 } 425 426 } 427 428 @Override 429 public Base setProperty(String name, Base value) throws FHIRException { 430 if (name.equals("type")) { 431 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 432 } else if (name.equals("role")) { 433 this.getRole().add(TypeConvertor.castToCodeableConcept(value)); 434 } else if (name.equals("who")) { 435 this.who = TypeConvertor.castToReference(value); // Reference 436 } else if (name.equals("onBehalfOf")) { 437 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 438 } else 439 return super.setProperty(name, value); 440 return value; 441 } 442 443 @Override 444 public void removeChild(String name, Base value) throws FHIRException { 445 if (name.equals("type")) { 446 this.type = null; 447 } else if (name.equals("role")) { 448 this.getRole().remove(value); 449 } else if (name.equals("who")) { 450 this.who = null; 451 } else if (name.equals("onBehalfOf")) { 452 this.onBehalfOf = null; 453 } else 454 super.removeChild(name, value); 455 456 } 457 458 @Override 459 public Base makeProperty(int hash, String name) throws FHIRException { 460 switch (hash) { 461 case 3575610: return getType(); 462 case 3506294: return addRole(); 463 case 117694: return getWho(); 464 case -14402964: return getOnBehalfOf(); 465 default: return super.makeProperty(hash, name); 466 } 467 468 } 469 470 @Override 471 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 472 switch (hash) { 473 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 474 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 475 case 117694: /*who*/ return new String[] {"Reference"}; 476 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 477 default: return super.getTypesForProperty(hash, name); 478 } 479 480 } 481 482 @Override 483 public Base addChild(String name) throws FHIRException { 484 if (name.equals("type")) { 485 this.type = new CodeableConcept(); 486 return this.type; 487 } 488 else if (name.equals("role")) { 489 return addRole(); 490 } 491 else if (name.equals("who")) { 492 this.who = new Reference(); 493 return this.who; 494 } 495 else if (name.equals("onBehalfOf")) { 496 this.onBehalfOf = new Reference(); 497 return this.onBehalfOf; 498 } 499 else 500 return super.addChild(name); 501 } 502 503 public ProvenanceAgentComponent copy() { 504 ProvenanceAgentComponent dst = new ProvenanceAgentComponent(); 505 copyValues(dst); 506 return dst; 507 } 508 509 public void copyValues(ProvenanceAgentComponent dst) { 510 super.copyValues(dst); 511 dst.type = type == null ? null : type.copy(); 512 if (role != null) { 513 dst.role = new ArrayList<CodeableConcept>(); 514 for (CodeableConcept i : role) 515 dst.role.add(i.copy()); 516 }; 517 dst.who = who == null ? null : who.copy(); 518 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 519 } 520 521 @Override 522 public boolean equalsDeep(Base other_) { 523 if (!super.equalsDeep(other_)) 524 return false; 525 if (!(other_ instanceof ProvenanceAgentComponent)) 526 return false; 527 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other_; 528 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true) && compareDeep(who, o.who, true) 529 && compareDeep(onBehalfOf, o.onBehalfOf, true); 530 } 531 532 @Override 533 public boolean equalsShallow(Base other_) { 534 if (!super.equalsShallow(other_)) 535 return false; 536 if (!(other_ instanceof ProvenanceAgentComponent)) 537 return false; 538 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other_; 539 return true; 540 } 541 542 public boolean isEmpty() { 543 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role, who, onBehalfOf 544 ); 545 } 546 547 public String fhirType() { 548 return "Provenance.agent"; 549 550 } 551 552 } 553 554 @Block() 555 public static class ProvenanceEntityComponent extends BackboneElement implements IBaseBackboneElement { 556 /** 557 * How the entity was used during the activity. 558 */ 559 @Child(name = "role", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 560 @Description(shortDefinition="revision | quotation | source | instantiates | removal", formalDefinition="How the entity was used during the activity." ) 561 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provenance-entity-role") 562 protected Enumeration<ProvenanceEntityRole> role; 563 564 /** 565 * Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative. 566 */ 567 @Child(name = "what", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 568 @Description(shortDefinition="Identity of entity", formalDefinition="Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative." ) 569 protected Reference what; 570 571 /** 572 * The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which used the entity. 573 */ 574 @Child(name = "agent", type = {ProvenanceAgentComponent.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 575 @Description(shortDefinition="Entity is attributed to this agent", formalDefinition="The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which used the entity." ) 576 protected List<ProvenanceAgentComponent> agent; 577 578 private static final long serialVersionUID = 211110220L; 579 580 /** 581 * Constructor 582 */ 583 public ProvenanceEntityComponent() { 584 super(); 585 } 586 587 /** 588 * Constructor 589 */ 590 public ProvenanceEntityComponent(ProvenanceEntityRole role, Reference what) { 591 super(); 592 this.setRole(role); 593 this.setWhat(what); 594 } 595 596 /** 597 * @return {@link #role} (How the entity was used during the activity.). This is the underlying object with id, value and extensions. The accessor "getRole" gives direct access to the value 598 */ 599 public Enumeration<ProvenanceEntityRole> getRoleElement() { 600 if (this.role == null) 601 if (Configuration.errorOnAutoCreate()) 602 throw new Error("Attempt to auto-create ProvenanceEntityComponent.role"); 603 else if (Configuration.doAutoCreate()) 604 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); // bb 605 return this.role; 606 } 607 608 public boolean hasRoleElement() { 609 return this.role != null && !this.role.isEmpty(); 610 } 611 612 public boolean hasRole() { 613 return this.role != null && !this.role.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #role} (How the entity was used during the activity.). This is the underlying object with id, value and extensions. The accessor "getRole" gives direct access to the value 618 */ 619 public ProvenanceEntityComponent setRoleElement(Enumeration<ProvenanceEntityRole> value) { 620 this.role = value; 621 return this; 622 } 623 624 /** 625 * @return How the entity was used during the activity. 626 */ 627 public ProvenanceEntityRole getRole() { 628 return this.role == null ? null : this.role.getValue(); 629 } 630 631 /** 632 * @param value How the entity was used during the activity. 633 */ 634 public ProvenanceEntityComponent setRole(ProvenanceEntityRole value) { 635 if (this.role == null) 636 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); 637 this.role.setValue(value); 638 return this; 639 } 640 641 /** 642 * @return {@link #what} (Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.) 643 */ 644 public Reference getWhat() { 645 if (this.what == null) 646 if (Configuration.errorOnAutoCreate()) 647 throw new Error("Attempt to auto-create ProvenanceEntityComponent.what"); 648 else if (Configuration.doAutoCreate()) 649 this.what = new Reference(); // cc 650 return this.what; 651 } 652 653 public boolean hasWhat() { 654 return this.what != null && !this.what.isEmpty(); 655 } 656 657 /** 658 * @param value {@link #what} (Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.) 659 */ 660 public ProvenanceEntityComponent setWhat(Reference value) { 661 this.what = value; 662 return this; 663 } 664 665 /** 666 * @return {@link #agent} (The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which used the entity.) 667 */ 668 public List<ProvenanceAgentComponent> getAgent() { 669 if (this.agent == null) 670 this.agent = new ArrayList<ProvenanceAgentComponent>(); 671 return this.agent; 672 } 673 674 /** 675 * @return Returns a reference to <code>this</code> for easy method chaining 676 */ 677 public ProvenanceEntityComponent setAgent(List<ProvenanceAgentComponent> theAgent) { 678 this.agent = theAgent; 679 return this; 680 } 681 682 public boolean hasAgent() { 683 if (this.agent == null) 684 return false; 685 for (ProvenanceAgentComponent item : this.agent) 686 if (!item.isEmpty()) 687 return true; 688 return false; 689 } 690 691 public ProvenanceAgentComponent addAgent() { //3 692 ProvenanceAgentComponent t = new ProvenanceAgentComponent(); 693 if (this.agent == null) 694 this.agent = new ArrayList<ProvenanceAgentComponent>(); 695 this.agent.add(t); 696 return t; 697 } 698 699 public ProvenanceEntityComponent addAgent(ProvenanceAgentComponent t) { //3 700 if (t == null) 701 return this; 702 if (this.agent == null) 703 this.agent = new ArrayList<ProvenanceAgentComponent>(); 704 this.agent.add(t); 705 return this; 706 } 707 708 /** 709 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist {3} 710 */ 711 public ProvenanceAgentComponent getAgentFirstRep() { 712 if (getAgent().isEmpty()) { 713 addAgent(); 714 } 715 return getAgent().get(0); 716 } 717 718 protected void listChildren(List<Property> children) { 719 super.listChildren(children); 720 children.add(new Property("role", "code", "How the entity was used during the activity.", 0, 1, role)); 721 children.add(new Property("what", "Reference(Any)", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what)); 722 children.add(new Property("agent", "@Provenance.agent", "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which used the entity.", 0, java.lang.Integer.MAX_VALUE, agent)); 723 } 724 725 @Override 726 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 727 switch (_hash) { 728 case 3506294: /*role*/ return new Property("role", "code", "How the entity was used during the activity.", 0, 1, role); 729 case 3648196: /*what*/ return new Property("what", "Reference(Any)", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what); 730 case 92750597: /*agent*/ return new Property("agent", "@Provenance.agent", "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which used the entity.", 0, java.lang.Integer.MAX_VALUE, agent); 731 default: return super.getNamedProperty(_hash, _name, _checkValid); 732 } 733 734 } 735 736 @Override 737 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 738 switch (hash) { 739 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // Enumeration<ProvenanceEntityRole> 740 case 3648196: /*what*/ return this.what == null ? new Base[0] : new Base[] {this.what}; // Reference 741 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // ProvenanceAgentComponent 742 default: return super.getProperty(hash, name, checkValid); 743 } 744 745 } 746 747 @Override 748 public Base setProperty(int hash, String name, Base value) throws FHIRException { 749 switch (hash) { 750 case 3506294: // role 751 value = new ProvenanceEntityRoleEnumFactory().fromType(TypeConvertor.castToCode(value)); 752 this.role = (Enumeration) value; // Enumeration<ProvenanceEntityRole> 753 return value; 754 case 3648196: // what 755 this.what = TypeConvertor.castToReference(value); // Reference 756 return value; 757 case 92750597: // agent 758 this.getAgent().add((ProvenanceAgentComponent) value); // ProvenanceAgentComponent 759 return value; 760 default: return super.setProperty(hash, name, value); 761 } 762 763 } 764 765 @Override 766 public Base setProperty(String name, Base value) throws FHIRException { 767 if (name.equals("role")) { 768 value = new ProvenanceEntityRoleEnumFactory().fromType(TypeConvertor.castToCode(value)); 769 this.role = (Enumeration) value; // Enumeration<ProvenanceEntityRole> 770 } else if (name.equals("what")) { 771 this.what = TypeConvertor.castToReference(value); // Reference 772 } else if (name.equals("agent")) { 773 this.getAgent().add((ProvenanceAgentComponent) value); 774 } else 775 return super.setProperty(name, value); 776 return value; 777 } 778 779 @Override 780 public void removeChild(String name, Base value) throws FHIRException { 781 if (name.equals("role")) { 782 value = new ProvenanceEntityRoleEnumFactory().fromType(TypeConvertor.castToCode(value)); 783 this.role = (Enumeration) value; // Enumeration<ProvenanceEntityRole> 784 } else if (name.equals("what")) { 785 this.what = null; 786 } else if (name.equals("agent")) { 787 this.getAgent().remove((ProvenanceAgentComponent) value); 788 } else 789 super.removeChild(name, value); 790 791 } 792 793 @Override 794 public Base makeProperty(int hash, String name) throws FHIRException { 795 switch (hash) { 796 case 3506294: return getRoleElement(); 797 case 3648196: return getWhat(); 798 case 92750597: return addAgent(); 799 default: return super.makeProperty(hash, name); 800 } 801 802 } 803 804 @Override 805 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 806 switch (hash) { 807 case 3506294: /*role*/ return new String[] {"code"}; 808 case 3648196: /*what*/ return new String[] {"Reference"}; 809 case 92750597: /*agent*/ return new String[] {"@Provenance.agent"}; 810 default: return super.getTypesForProperty(hash, name); 811 } 812 813 } 814 815 @Override 816 public Base addChild(String name) throws FHIRException { 817 if (name.equals("role")) { 818 throw new FHIRException("Cannot call addChild on a singleton property Provenance.entity.role"); 819 } 820 else if (name.equals("what")) { 821 this.what = new Reference(); 822 return this.what; 823 } 824 else if (name.equals("agent")) { 825 return addAgent(); 826 } 827 else 828 return super.addChild(name); 829 } 830 831 public ProvenanceEntityComponent copy() { 832 ProvenanceEntityComponent dst = new ProvenanceEntityComponent(); 833 copyValues(dst); 834 return dst; 835 } 836 837 public void copyValues(ProvenanceEntityComponent dst) { 838 super.copyValues(dst); 839 dst.role = role == null ? null : role.copy(); 840 dst.what = what == null ? null : what.copy(); 841 if (agent != null) { 842 dst.agent = new ArrayList<ProvenanceAgentComponent>(); 843 for (ProvenanceAgentComponent i : agent) 844 dst.agent.add(i.copy()); 845 }; 846 } 847 848 @Override 849 public boolean equalsDeep(Base other_) { 850 if (!super.equalsDeep(other_)) 851 return false; 852 if (!(other_ instanceof ProvenanceEntityComponent)) 853 return false; 854 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other_; 855 return compareDeep(role, o.role, true) && compareDeep(what, o.what, true) && compareDeep(agent, o.agent, true) 856 ; 857 } 858 859 @Override 860 public boolean equalsShallow(Base other_) { 861 if (!super.equalsShallow(other_)) 862 return false; 863 if (!(other_ instanceof ProvenanceEntityComponent)) 864 return false; 865 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other_; 866 return compareValues(role, o.role, true); 867 } 868 869 public boolean isEmpty() { 870 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, what, agent); 871 } 872 873 public String fhirType() { 874 return "Provenance.entity"; 875 876 } 877 878 } 879 880 /** 881 * The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity. 882 */ 883 @Child(name = "target", type = {Reference.class}, order=0, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 884 @Description(shortDefinition="Target Reference(s) (usually version specific)", formalDefinition="The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity." ) 885 protected List<Reference> target; 886 887 /** 888 * The period during which the activity occurred. 889 */ 890 @Child(name = "occurred", type = {Period.class, DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 891 @Description(shortDefinition="When the activity occurred", formalDefinition="The period during which the activity occurred." ) 892 protected DataType occurred; 893 894 /** 895 * The instant of time at which the activity was recorded. 896 */ 897 @Child(name = "recorded", type = {InstantType.class}, order=2, min=0, max=1, modifier=false, summary=true) 898 @Description(shortDefinition="When the activity was recorded / updated", formalDefinition="The instant of time at which the activity was recorded." ) 899 protected InstantType recorded; 900 901 /** 902 * Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc. 903 */ 904 @Child(name = "policy", type = {UriType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 905 @Description(shortDefinition="Policy or plan the activity was defined by", formalDefinition="Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc." ) 906 protected List<UriType> policy; 907 908 /** 909 * Where the activity occurred, if relevant. 910 */ 911 @Child(name = "location", type = {Location.class}, order=4, min=0, max=1, modifier=false, summary=false) 912 @Description(shortDefinition="Where the activity occurred, if relevant", formalDefinition="Where the activity occurred, if relevant." ) 913 protected Reference location; 914 915 /** 916 * The authorization (e.g., PurposeOfUse) that was used during the event being recorded. 917 */ 918 @Child(name = "authorization", type = {CodeableReference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 919 @Description(shortDefinition="Authorization (purposeOfUse) related to the event", formalDefinition="The authorization (e.g., PurposeOfUse) that was used during the event being recorded." ) 920 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 921 protected List<CodeableReference> authorization; 922 923 /** 924 * An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities. 925 */ 926 @Child(name = "activity", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 927 @Description(shortDefinition="Activity that occurred", formalDefinition="An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities." ) 928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provenance-activity-type") 929 protected CodeableConcept activity; 930 931 /** 932 * Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon. 933 */ 934 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, Task.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 935 @Description(shortDefinition="Workflow authorization within which this event occurred", formalDefinition="Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon." ) 936 protected List<Reference> basedOn; 937 938 /** 939 * The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity. 940 */ 941 @Child(name = "patient", type = {Patient.class}, order=8, min=0, max=1, modifier=false, summary=false) 942 @Description(shortDefinition="The patient is the subject of the data created/updated (.target) by the activity", formalDefinition="The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity." ) 943 protected Reference patient; 944 945 /** 946 * This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests). 947 */ 948 @Child(name = "encounter", type = {Encounter.class}, order=9, min=0, max=1, modifier=false, summary=false) 949 @Description(shortDefinition="Encounter within which this event occurred or which the event is tightly associated", formalDefinition="This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests)." ) 950 protected Reference encounter; 951 952 /** 953 * An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place. 954 */ 955 @Child(name = "agent", type = {}, order=10, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 956 @Description(shortDefinition="Actor involved", formalDefinition="An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place." ) 957 protected List<ProvenanceAgentComponent> agent; 958 959 /** 960 * An entity used in this activity. 961 */ 962 @Child(name = "entity", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 963 @Description(shortDefinition="An entity used in this activity", formalDefinition="An entity used in this activity." ) 964 protected List<ProvenanceEntityComponent> entity; 965 966 /** 967 * A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated. 968 */ 969 @Child(name = "signature", type = {Signature.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 970 @Description(shortDefinition="Signature on target", formalDefinition="A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated." ) 971 protected List<Signature> signature; 972 973 private static final long serialVersionUID = 2001521682L; 974 975 /** 976 * Constructor 977 */ 978 public Provenance() { 979 super(); 980 } 981 982 /** 983 * Constructor 984 */ 985 public Provenance(Reference target, ProvenanceAgentComponent agent) { 986 super(); 987 this.addTarget(target); 988 this.addAgent(agent); 989 } 990 991 /** 992 * @return {@link #target} (The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.) 993 */ 994 public List<Reference> getTarget() { 995 if (this.target == null) 996 this.target = new ArrayList<Reference>(); 997 return this.target; 998 } 999 1000 /** 1001 * @return Returns a reference to <code>this</code> for easy method chaining 1002 */ 1003 public Provenance setTarget(List<Reference> theTarget) { 1004 this.target = theTarget; 1005 return this; 1006 } 1007 1008 public boolean hasTarget() { 1009 if (this.target == null) 1010 return false; 1011 for (Reference item : this.target) 1012 if (!item.isEmpty()) 1013 return true; 1014 return false; 1015 } 1016 1017 public Reference addTarget() { //3 1018 Reference t = new Reference(); 1019 if (this.target == null) 1020 this.target = new ArrayList<Reference>(); 1021 this.target.add(t); 1022 return t; 1023 } 1024 1025 public Provenance addTarget(Reference t) { //3 1026 if (t == null) 1027 return this; 1028 if (this.target == null) 1029 this.target = new ArrayList<Reference>(); 1030 this.target.add(t); 1031 return this; 1032 } 1033 1034 /** 1035 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 1036 */ 1037 public Reference getTargetFirstRep() { 1038 if (getTarget().isEmpty()) { 1039 addTarget(); 1040 } 1041 return getTarget().get(0); 1042 } 1043 1044 /** 1045 * @return {@link #occurred} (The period during which the activity occurred.) 1046 */ 1047 public DataType getOccurred() { 1048 return this.occurred; 1049 } 1050 1051 /** 1052 * @return {@link #occurred} (The period during which the activity occurred.) 1053 */ 1054 public Period getOccurredPeriod() throws FHIRException { 1055 if (this.occurred == null) 1056 this.occurred = new Period(); 1057 if (!(this.occurred instanceof Period)) 1058 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurred.getClass().getName()+" was encountered"); 1059 return (Period) this.occurred; 1060 } 1061 1062 public boolean hasOccurredPeriod() { 1063 return this != null && this.occurred instanceof Period; 1064 } 1065 1066 /** 1067 * @return {@link #occurred} (The period during which the activity occurred.) 1068 */ 1069 public DateTimeType getOccurredDateTimeType() throws FHIRException { 1070 if (this.occurred == null) 1071 this.occurred = new DateTimeType(); 1072 if (!(this.occurred instanceof DateTimeType)) 1073 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurred.getClass().getName()+" was encountered"); 1074 return (DateTimeType) this.occurred; 1075 } 1076 1077 public boolean hasOccurredDateTimeType() { 1078 return this != null && this.occurred instanceof DateTimeType; 1079 } 1080 1081 public boolean hasOccurred() { 1082 return this.occurred != null && !this.occurred.isEmpty(); 1083 } 1084 1085 /** 1086 * @param value {@link #occurred} (The period during which the activity occurred.) 1087 */ 1088 public Provenance setOccurred(DataType value) { 1089 if (value != null && !(value instanceof Period || value instanceof DateTimeType)) 1090 throw new FHIRException("Not the right type for Provenance.occurred[x]: "+value.fhirType()); 1091 this.occurred = value; 1092 return this; 1093 } 1094 1095 /** 1096 * @return {@link #recorded} (The instant of time at which the activity was recorded.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1097 */ 1098 public InstantType getRecordedElement() { 1099 if (this.recorded == null) 1100 if (Configuration.errorOnAutoCreate()) 1101 throw new Error("Attempt to auto-create Provenance.recorded"); 1102 else if (Configuration.doAutoCreate()) 1103 this.recorded = new InstantType(); // bb 1104 return this.recorded; 1105 } 1106 1107 public boolean hasRecordedElement() { 1108 return this.recorded != null && !this.recorded.isEmpty(); 1109 } 1110 1111 public boolean hasRecorded() { 1112 return this.recorded != null && !this.recorded.isEmpty(); 1113 } 1114 1115 /** 1116 * @param value {@link #recorded} (The instant of time at which the activity was recorded.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1117 */ 1118 public Provenance setRecordedElement(InstantType value) { 1119 this.recorded = value; 1120 return this; 1121 } 1122 1123 /** 1124 * @return The instant of time at which the activity was recorded. 1125 */ 1126 public Date getRecorded() { 1127 return this.recorded == null ? null : this.recorded.getValue(); 1128 } 1129 1130 /** 1131 * @param value The instant of time at which the activity was recorded. 1132 */ 1133 public Provenance setRecorded(Date value) { 1134 if (value == null) 1135 this.recorded = null; 1136 else { 1137 if (this.recorded == null) 1138 this.recorded = new InstantType(); 1139 this.recorded.setValue(value); 1140 } 1141 return this; 1142 } 1143 1144 /** 1145 * @return {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1146 */ 1147 public List<UriType> getPolicy() { 1148 if (this.policy == null) 1149 this.policy = new ArrayList<UriType>(); 1150 return this.policy; 1151 } 1152 1153 /** 1154 * @return Returns a reference to <code>this</code> for easy method chaining 1155 */ 1156 public Provenance setPolicy(List<UriType> thePolicy) { 1157 this.policy = thePolicy; 1158 return this; 1159 } 1160 1161 public boolean hasPolicy() { 1162 if (this.policy == null) 1163 return false; 1164 for (UriType item : this.policy) 1165 if (!item.isEmpty()) 1166 return true; 1167 return false; 1168 } 1169 1170 /** 1171 * @return {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1172 */ 1173 public UriType addPolicyElement() {//2 1174 UriType t = new UriType(); 1175 if (this.policy == null) 1176 this.policy = new ArrayList<UriType>(); 1177 this.policy.add(t); 1178 return t; 1179 } 1180 1181 /** 1182 * @param value {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1183 */ 1184 public Provenance addPolicy(String value) { //1 1185 UriType t = new UriType(); 1186 t.setValue(value); 1187 if (this.policy == null) 1188 this.policy = new ArrayList<UriType>(); 1189 this.policy.add(t); 1190 return this; 1191 } 1192 1193 /** 1194 * @param value {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1195 */ 1196 public boolean hasPolicy(String value) { 1197 if (this.policy == null) 1198 return false; 1199 for (UriType v : this.policy) 1200 if (v.getValue().equals(value)) // uri 1201 return true; 1202 return false; 1203 } 1204 1205 /** 1206 * @return {@link #location} (Where the activity occurred, if relevant.) 1207 */ 1208 public Reference getLocation() { 1209 if (this.location == null) 1210 if (Configuration.errorOnAutoCreate()) 1211 throw new Error("Attempt to auto-create Provenance.location"); 1212 else if (Configuration.doAutoCreate()) 1213 this.location = new Reference(); // cc 1214 return this.location; 1215 } 1216 1217 public boolean hasLocation() { 1218 return this.location != null && !this.location.isEmpty(); 1219 } 1220 1221 /** 1222 * @param value {@link #location} (Where the activity occurred, if relevant.) 1223 */ 1224 public Provenance setLocation(Reference value) { 1225 this.location = value; 1226 return this; 1227 } 1228 1229 /** 1230 * @return {@link #authorization} (The authorization (e.g., PurposeOfUse) that was used during the event being recorded.) 1231 */ 1232 public List<CodeableReference> getAuthorization() { 1233 if (this.authorization == null) 1234 this.authorization = new ArrayList<CodeableReference>(); 1235 return this.authorization; 1236 } 1237 1238 /** 1239 * @return Returns a reference to <code>this</code> for easy method chaining 1240 */ 1241 public Provenance setAuthorization(List<CodeableReference> theAuthorization) { 1242 this.authorization = theAuthorization; 1243 return this; 1244 } 1245 1246 public boolean hasAuthorization() { 1247 if (this.authorization == null) 1248 return false; 1249 for (CodeableReference item : this.authorization) 1250 if (!item.isEmpty()) 1251 return true; 1252 return false; 1253 } 1254 1255 public CodeableReference addAuthorization() { //3 1256 CodeableReference t = new CodeableReference(); 1257 if (this.authorization == null) 1258 this.authorization = new ArrayList<CodeableReference>(); 1259 this.authorization.add(t); 1260 return t; 1261 } 1262 1263 public Provenance addAuthorization(CodeableReference t) { //3 1264 if (t == null) 1265 return this; 1266 if (this.authorization == null) 1267 this.authorization = new ArrayList<CodeableReference>(); 1268 this.authorization.add(t); 1269 return this; 1270 } 1271 1272 /** 1273 * @return The first repetition of repeating field {@link #authorization}, creating it if it does not already exist {3} 1274 */ 1275 public CodeableReference getAuthorizationFirstRep() { 1276 if (getAuthorization().isEmpty()) { 1277 addAuthorization(); 1278 } 1279 return getAuthorization().get(0); 1280 } 1281 1282 /** 1283 * @return {@link #activity} (An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.) 1284 */ 1285 public CodeableConcept getActivity() { 1286 if (this.activity == null) 1287 if (Configuration.errorOnAutoCreate()) 1288 throw new Error("Attempt to auto-create Provenance.activity"); 1289 else if (Configuration.doAutoCreate()) 1290 this.activity = new CodeableConcept(); // cc 1291 return this.activity; 1292 } 1293 1294 public boolean hasActivity() { 1295 return this.activity != null && !this.activity.isEmpty(); 1296 } 1297 1298 /** 1299 * @param value {@link #activity} (An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.) 1300 */ 1301 public Provenance setActivity(CodeableConcept value) { 1302 this.activity = value; 1303 return this; 1304 } 1305 1306 /** 1307 * @return {@link #basedOn} (Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon.) 1308 */ 1309 public List<Reference> getBasedOn() { 1310 if (this.basedOn == null) 1311 this.basedOn = new ArrayList<Reference>(); 1312 return this.basedOn; 1313 } 1314 1315 /** 1316 * @return Returns a reference to <code>this</code> for easy method chaining 1317 */ 1318 public Provenance setBasedOn(List<Reference> theBasedOn) { 1319 this.basedOn = theBasedOn; 1320 return this; 1321 } 1322 1323 public boolean hasBasedOn() { 1324 if (this.basedOn == null) 1325 return false; 1326 for (Reference item : this.basedOn) 1327 if (!item.isEmpty()) 1328 return true; 1329 return false; 1330 } 1331 1332 public Reference addBasedOn() { //3 1333 Reference t = new Reference(); 1334 if (this.basedOn == null) 1335 this.basedOn = new ArrayList<Reference>(); 1336 this.basedOn.add(t); 1337 return t; 1338 } 1339 1340 public Provenance addBasedOn(Reference t) { //3 1341 if (t == null) 1342 return this; 1343 if (this.basedOn == null) 1344 this.basedOn = new ArrayList<Reference>(); 1345 this.basedOn.add(t); 1346 return this; 1347 } 1348 1349 /** 1350 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1351 */ 1352 public Reference getBasedOnFirstRep() { 1353 if (getBasedOn().isEmpty()) { 1354 addBasedOn(); 1355 } 1356 return getBasedOn().get(0); 1357 } 1358 1359 /** 1360 * @return {@link #patient} (The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.) 1361 */ 1362 public Reference getPatient() { 1363 if (this.patient == null) 1364 if (Configuration.errorOnAutoCreate()) 1365 throw new Error("Attempt to auto-create Provenance.patient"); 1366 else if (Configuration.doAutoCreate()) 1367 this.patient = new Reference(); // cc 1368 return this.patient; 1369 } 1370 1371 public boolean hasPatient() { 1372 return this.patient != null && !this.patient.isEmpty(); 1373 } 1374 1375 /** 1376 * @param value {@link #patient} (The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.) 1377 */ 1378 public Provenance setPatient(Reference value) { 1379 this.patient = value; 1380 return this; 1381 } 1382 1383 /** 1384 * @return {@link #encounter} (This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).) 1385 */ 1386 public Reference getEncounter() { 1387 if (this.encounter == null) 1388 if (Configuration.errorOnAutoCreate()) 1389 throw new Error("Attempt to auto-create Provenance.encounter"); 1390 else if (Configuration.doAutoCreate()) 1391 this.encounter = new Reference(); // cc 1392 return this.encounter; 1393 } 1394 1395 public boolean hasEncounter() { 1396 return this.encounter != null && !this.encounter.isEmpty(); 1397 } 1398 1399 /** 1400 * @param value {@link #encounter} (This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).) 1401 */ 1402 public Provenance setEncounter(Reference value) { 1403 this.encounter = value; 1404 return this; 1405 } 1406 1407 /** 1408 * @return {@link #agent} (An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.) 1409 */ 1410 public List<ProvenanceAgentComponent> getAgent() { 1411 if (this.agent == null) 1412 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1413 return this.agent; 1414 } 1415 1416 /** 1417 * @return Returns a reference to <code>this</code> for easy method chaining 1418 */ 1419 public Provenance setAgent(List<ProvenanceAgentComponent> theAgent) { 1420 this.agent = theAgent; 1421 return this; 1422 } 1423 1424 public boolean hasAgent() { 1425 if (this.agent == null) 1426 return false; 1427 for (ProvenanceAgentComponent item : this.agent) 1428 if (!item.isEmpty()) 1429 return true; 1430 return false; 1431 } 1432 1433 public ProvenanceAgentComponent addAgent() { //3 1434 ProvenanceAgentComponent t = new ProvenanceAgentComponent(); 1435 if (this.agent == null) 1436 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1437 this.agent.add(t); 1438 return t; 1439 } 1440 1441 public Provenance addAgent(ProvenanceAgentComponent t) { //3 1442 if (t == null) 1443 return this; 1444 if (this.agent == null) 1445 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1446 this.agent.add(t); 1447 return this; 1448 } 1449 1450 /** 1451 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist {3} 1452 */ 1453 public ProvenanceAgentComponent getAgentFirstRep() { 1454 if (getAgent().isEmpty()) { 1455 addAgent(); 1456 } 1457 return getAgent().get(0); 1458 } 1459 1460 /** 1461 * @return {@link #entity} (An entity used in this activity.) 1462 */ 1463 public List<ProvenanceEntityComponent> getEntity() { 1464 if (this.entity == null) 1465 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1466 return this.entity; 1467 } 1468 1469 /** 1470 * @return Returns a reference to <code>this</code> for easy method chaining 1471 */ 1472 public Provenance setEntity(List<ProvenanceEntityComponent> theEntity) { 1473 this.entity = theEntity; 1474 return this; 1475 } 1476 1477 public boolean hasEntity() { 1478 if (this.entity == null) 1479 return false; 1480 for (ProvenanceEntityComponent item : this.entity) 1481 if (!item.isEmpty()) 1482 return true; 1483 return false; 1484 } 1485 1486 public ProvenanceEntityComponent addEntity() { //3 1487 ProvenanceEntityComponent t = new ProvenanceEntityComponent(); 1488 if (this.entity == null) 1489 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1490 this.entity.add(t); 1491 return t; 1492 } 1493 1494 public Provenance addEntity(ProvenanceEntityComponent t) { //3 1495 if (t == null) 1496 return this; 1497 if (this.entity == null) 1498 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1499 this.entity.add(t); 1500 return this; 1501 } 1502 1503 /** 1504 * @return The first repetition of repeating field {@link #entity}, creating it if it does not already exist {3} 1505 */ 1506 public ProvenanceEntityComponent getEntityFirstRep() { 1507 if (getEntity().isEmpty()) { 1508 addEntity(); 1509 } 1510 return getEntity().get(0); 1511 } 1512 1513 /** 1514 * @return {@link #signature} (A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.) 1515 */ 1516 public List<Signature> getSignature() { 1517 if (this.signature == null) 1518 this.signature = new ArrayList<Signature>(); 1519 return this.signature; 1520 } 1521 1522 /** 1523 * @return Returns a reference to <code>this</code> for easy method chaining 1524 */ 1525 public Provenance setSignature(List<Signature> theSignature) { 1526 this.signature = theSignature; 1527 return this; 1528 } 1529 1530 public boolean hasSignature() { 1531 if (this.signature == null) 1532 return false; 1533 for (Signature item : this.signature) 1534 if (!item.isEmpty()) 1535 return true; 1536 return false; 1537 } 1538 1539 public Signature addSignature() { //3 1540 Signature t = new Signature(); 1541 if (this.signature == null) 1542 this.signature = new ArrayList<Signature>(); 1543 this.signature.add(t); 1544 return t; 1545 } 1546 1547 public Provenance addSignature(Signature t) { //3 1548 if (t == null) 1549 return this; 1550 if (this.signature == null) 1551 this.signature = new ArrayList<Signature>(); 1552 this.signature.add(t); 1553 return this; 1554 } 1555 1556 /** 1557 * @return The first repetition of repeating field {@link #signature}, creating it if it does not already exist {3} 1558 */ 1559 public Signature getSignatureFirstRep() { 1560 if (getSignature().isEmpty()) { 1561 addSignature(); 1562 } 1563 return getSignature().get(0); 1564 } 1565 1566 protected void listChildren(List<Property> children) { 1567 super.listChildren(children); 1568 children.add(new Property("target", "Reference(Any)", "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.", 0, java.lang.Integer.MAX_VALUE, target)); 1569 children.add(new Property("occurred[x]", "Period|dateTime", "The period during which the activity occurred.", 0, 1, occurred)); 1570 children.add(new Property("recorded", "instant", "The instant of time at which the activity was recorded.", 0, 1, recorded)); 1571 children.add(new Property("policy", "uri", "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.", 0, java.lang.Integer.MAX_VALUE, policy)); 1572 children.add(new Property("location", "Reference(Location)", "Where the activity occurred, if relevant.", 0, 1, location)); 1573 children.add(new Property("authorization", "CodeableReference", "The authorization (e.g., PurposeOfUse) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, authorization)); 1574 children.add(new Property("activity", "CodeableConcept", "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.", 0, 1, activity)); 1575 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest|Task)", "Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1576 children.add(new Property("patient", "Reference(Patient)", "The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.", 0, 1, patient)); 1577 children.add(new Property("encounter", "Reference(Encounter)", "This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).", 0, 1, encounter)); 1578 children.add(new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent)); 1579 children.add(new Property("entity", "", "An entity used in this activity.", 0, java.lang.Integer.MAX_VALUE, entity)); 1580 children.add(new Property("signature", "Signature", "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.", 0, java.lang.Integer.MAX_VALUE, signature)); 1581 } 1582 1583 @Override 1584 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1585 switch (_hash) { 1586 case -880905839: /*target*/ return new Property("target", "Reference(Any)", "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.", 0, java.lang.Integer.MAX_VALUE, target); 1587 case 784181563: /*occurred[x]*/ return new Property("occurred[x]", "Period|dateTime", "The period during which the activity occurred.", 0, 1, occurred); 1588 case 792816933: /*occurred*/ return new Property("occurred[x]", "Period|dateTime", "The period during which the activity occurred.", 0, 1, occurred); 1589 case 894082886: /*occurredPeriod*/ return new Property("occurred[x]", "Period", "The period during which the activity occurred.", 0, 1, occurred); 1590 case 1579027424: /*occurredDateTime*/ return new Property("occurred[x]", "dateTime", "The period during which the activity occurred.", 0, 1, occurred); 1591 case -799233872: /*recorded*/ return new Property("recorded", "instant", "The instant of time at which the activity was recorded.", 0, 1, recorded); 1592 case -982670030: /*policy*/ return new Property("policy", "uri", "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.", 0, java.lang.Integer.MAX_VALUE, policy); 1593 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Where the activity occurred, if relevant.", 0, 1, location); 1594 case -1385570183: /*authorization*/ return new Property("authorization", "CodeableReference", "The authorization (e.g., PurposeOfUse) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, authorization); 1595 case -1655966961: /*activity*/ return new Property("activity", "CodeableConcept", "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.", 0, 1, activity); 1596 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest|Task)", "Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1597 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.", 0, 1, patient); 1598 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).", 0, 1, encounter); 1599 case 92750597: /*agent*/ return new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent); 1600 case -1298275357: /*entity*/ return new Property("entity", "", "An entity used in this activity.", 0, java.lang.Integer.MAX_VALUE, entity); 1601 case 1073584312: /*signature*/ return new Property("signature", "Signature", "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.", 0, java.lang.Integer.MAX_VALUE, signature); 1602 default: return super.getNamedProperty(_hash, _name, _checkValid); 1603 } 1604 1605 } 1606 1607 @Override 1608 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1609 switch (hash) { 1610 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Reference 1611 case 792816933: /*occurred*/ return this.occurred == null ? new Base[0] : new Base[] {this.occurred}; // DataType 1612 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // InstantType 1613 case -982670030: /*policy*/ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // UriType 1614 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1615 case -1385570183: /*authorization*/ return this.authorization == null ? new Base[0] : this.authorization.toArray(new Base[this.authorization.size()]); // CodeableReference 1616 case -1655966961: /*activity*/ return this.activity == null ? new Base[0] : new Base[] {this.activity}; // CodeableConcept 1617 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1618 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1619 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1620 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // ProvenanceAgentComponent 1621 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : this.entity.toArray(new Base[this.entity.size()]); // ProvenanceEntityComponent 1622 case 1073584312: /*signature*/ return this.signature == null ? new Base[0] : this.signature.toArray(new Base[this.signature.size()]); // Signature 1623 default: return super.getProperty(hash, name, checkValid); 1624 } 1625 1626 } 1627 1628 @Override 1629 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1630 switch (hash) { 1631 case -880905839: // target 1632 this.getTarget().add(TypeConvertor.castToReference(value)); // Reference 1633 return value; 1634 case 792816933: // occurred 1635 this.occurred = TypeConvertor.castToType(value); // DataType 1636 return value; 1637 case -799233872: // recorded 1638 this.recorded = TypeConvertor.castToInstant(value); // InstantType 1639 return value; 1640 case -982670030: // policy 1641 this.getPolicy().add(TypeConvertor.castToUri(value)); // UriType 1642 return value; 1643 case 1901043637: // location 1644 this.location = TypeConvertor.castToReference(value); // Reference 1645 return value; 1646 case -1385570183: // authorization 1647 this.getAuthorization().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1648 return value; 1649 case -1655966961: // activity 1650 this.activity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1651 return value; 1652 case -332612366: // basedOn 1653 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1654 return value; 1655 case -791418107: // patient 1656 this.patient = TypeConvertor.castToReference(value); // Reference 1657 return value; 1658 case 1524132147: // encounter 1659 this.encounter = TypeConvertor.castToReference(value); // Reference 1660 return value; 1661 case 92750597: // agent 1662 this.getAgent().add((ProvenanceAgentComponent) value); // ProvenanceAgentComponent 1663 return value; 1664 case -1298275357: // entity 1665 this.getEntity().add((ProvenanceEntityComponent) value); // ProvenanceEntityComponent 1666 return value; 1667 case 1073584312: // signature 1668 this.getSignature().add(TypeConvertor.castToSignature(value)); // Signature 1669 return value; 1670 default: return super.setProperty(hash, name, value); 1671 } 1672 1673 } 1674 1675 @Override 1676 public Base setProperty(String name, Base value) throws FHIRException { 1677 if (name.equals("target")) { 1678 this.getTarget().add(TypeConvertor.castToReference(value)); 1679 } else if (name.equals("occurred[x]")) { 1680 this.occurred = TypeConvertor.castToType(value); // DataType 1681 } else if (name.equals("recorded")) { 1682 this.recorded = TypeConvertor.castToInstant(value); // InstantType 1683 } else if (name.equals("policy")) { 1684 this.getPolicy().add(TypeConvertor.castToUri(value)); 1685 } else if (name.equals("location")) { 1686 this.location = TypeConvertor.castToReference(value); // Reference 1687 } else if (name.equals("authorization")) { 1688 this.getAuthorization().add(TypeConvertor.castToCodeableReference(value)); 1689 } else if (name.equals("activity")) { 1690 this.activity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1691 } else if (name.equals("basedOn")) { 1692 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1693 } else if (name.equals("patient")) { 1694 this.patient = TypeConvertor.castToReference(value); // Reference 1695 } else if (name.equals("encounter")) { 1696 this.encounter = TypeConvertor.castToReference(value); // Reference 1697 } else if (name.equals("agent")) { 1698 this.getAgent().add((ProvenanceAgentComponent) value); 1699 } else if (name.equals("entity")) { 1700 this.getEntity().add((ProvenanceEntityComponent) value); 1701 } else if (name.equals("signature")) { 1702 this.getSignature().add(TypeConvertor.castToSignature(value)); 1703 } else 1704 return super.setProperty(name, value); 1705 return value; 1706 } 1707 1708 @Override 1709 public void removeChild(String name, Base value) throws FHIRException { 1710 if (name.equals("target")) { 1711 this.getTarget().remove(value); 1712 } else if (name.equals("occurred[x]")) { 1713 this.occurred = null; 1714 } else if (name.equals("recorded")) { 1715 this.recorded = null; 1716 } else if (name.equals("policy")) { 1717 this.getPolicy().remove(value); 1718 } else if (name.equals("location")) { 1719 this.location = null; 1720 } else if (name.equals("authorization")) { 1721 this.getAuthorization().remove(value); 1722 } else if (name.equals("activity")) { 1723 this.activity = null; 1724 } else if (name.equals("basedOn")) { 1725 this.getBasedOn().remove(value); 1726 } else if (name.equals("patient")) { 1727 this.patient = null; 1728 } else if (name.equals("encounter")) { 1729 this.encounter = null; 1730 } else if (name.equals("agent")) { 1731 this.getAgent().remove((ProvenanceAgentComponent) value); 1732 } else if (name.equals("entity")) { 1733 this.getEntity().remove((ProvenanceEntityComponent) value); 1734 } else if (name.equals("signature")) { 1735 this.getSignature().remove(value); 1736 } else 1737 super.removeChild(name, value); 1738 1739 } 1740 1741 @Override 1742 public Base makeProperty(int hash, String name) throws FHIRException { 1743 switch (hash) { 1744 case -880905839: return addTarget(); 1745 case 784181563: return getOccurred(); 1746 case 792816933: return getOccurred(); 1747 case -799233872: return getRecordedElement(); 1748 case -982670030: return addPolicyElement(); 1749 case 1901043637: return getLocation(); 1750 case -1385570183: return addAuthorization(); 1751 case -1655966961: return getActivity(); 1752 case -332612366: return addBasedOn(); 1753 case -791418107: return getPatient(); 1754 case 1524132147: return getEncounter(); 1755 case 92750597: return addAgent(); 1756 case -1298275357: return addEntity(); 1757 case 1073584312: return addSignature(); 1758 default: return super.makeProperty(hash, name); 1759 } 1760 1761 } 1762 1763 @Override 1764 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1765 switch (hash) { 1766 case -880905839: /*target*/ return new String[] {"Reference"}; 1767 case 792816933: /*occurred*/ return new String[] {"Period", "dateTime"}; 1768 case -799233872: /*recorded*/ return new String[] {"instant"}; 1769 case -982670030: /*policy*/ return new String[] {"uri"}; 1770 case 1901043637: /*location*/ return new String[] {"Reference"}; 1771 case -1385570183: /*authorization*/ return new String[] {"CodeableReference"}; 1772 case -1655966961: /*activity*/ return new String[] {"CodeableConcept"}; 1773 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1774 case -791418107: /*patient*/ return new String[] {"Reference"}; 1775 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1776 case 92750597: /*agent*/ return new String[] {}; 1777 case -1298275357: /*entity*/ return new String[] {}; 1778 case 1073584312: /*signature*/ return new String[] {"Signature"}; 1779 default: return super.getTypesForProperty(hash, name); 1780 } 1781 1782 } 1783 1784 @Override 1785 public Base addChild(String name) throws FHIRException { 1786 if (name.equals("target")) { 1787 return addTarget(); 1788 } 1789 else if (name.equals("occurredPeriod")) { 1790 this.occurred = new Period(); 1791 return this.occurred; 1792 } 1793 else if (name.equals("occurredDateTime")) { 1794 this.occurred = new DateTimeType(); 1795 return this.occurred; 1796 } 1797 else if (name.equals("recorded")) { 1798 throw new FHIRException("Cannot call addChild on a singleton property Provenance.recorded"); 1799 } 1800 else if (name.equals("policy")) { 1801 throw new FHIRException("Cannot call addChild on a singleton property Provenance.policy"); 1802 } 1803 else if (name.equals("location")) { 1804 this.location = new Reference(); 1805 return this.location; 1806 } 1807 else if (name.equals("authorization")) { 1808 return addAuthorization(); 1809 } 1810 else if (name.equals("activity")) { 1811 this.activity = new CodeableConcept(); 1812 return this.activity; 1813 } 1814 else if (name.equals("basedOn")) { 1815 return addBasedOn(); 1816 } 1817 else if (name.equals("patient")) { 1818 this.patient = new Reference(); 1819 return this.patient; 1820 } 1821 else if (name.equals("encounter")) { 1822 this.encounter = new Reference(); 1823 return this.encounter; 1824 } 1825 else if (name.equals("agent")) { 1826 return addAgent(); 1827 } 1828 else if (name.equals("entity")) { 1829 return addEntity(); 1830 } 1831 else if (name.equals("signature")) { 1832 return addSignature(); 1833 } 1834 else 1835 return super.addChild(name); 1836 } 1837 1838 public String fhirType() { 1839 return "Provenance"; 1840 1841 } 1842 1843 public Provenance copy() { 1844 Provenance dst = new Provenance(); 1845 copyValues(dst); 1846 return dst; 1847 } 1848 1849 public void copyValues(Provenance dst) { 1850 super.copyValues(dst); 1851 if (target != null) { 1852 dst.target = new ArrayList<Reference>(); 1853 for (Reference i : target) 1854 dst.target.add(i.copy()); 1855 }; 1856 dst.occurred = occurred == null ? null : occurred.copy(); 1857 dst.recorded = recorded == null ? null : recorded.copy(); 1858 if (policy != null) { 1859 dst.policy = new ArrayList<UriType>(); 1860 for (UriType i : policy) 1861 dst.policy.add(i.copy()); 1862 }; 1863 dst.location = location == null ? null : location.copy(); 1864 if (authorization != null) { 1865 dst.authorization = new ArrayList<CodeableReference>(); 1866 for (CodeableReference i : authorization) 1867 dst.authorization.add(i.copy()); 1868 }; 1869 dst.activity = activity == null ? null : activity.copy(); 1870 if (basedOn != null) { 1871 dst.basedOn = new ArrayList<Reference>(); 1872 for (Reference i : basedOn) 1873 dst.basedOn.add(i.copy()); 1874 }; 1875 dst.patient = patient == null ? null : patient.copy(); 1876 dst.encounter = encounter == null ? null : encounter.copy(); 1877 if (agent != null) { 1878 dst.agent = new ArrayList<ProvenanceAgentComponent>(); 1879 for (ProvenanceAgentComponent i : agent) 1880 dst.agent.add(i.copy()); 1881 }; 1882 if (entity != null) { 1883 dst.entity = new ArrayList<ProvenanceEntityComponent>(); 1884 for (ProvenanceEntityComponent i : entity) 1885 dst.entity.add(i.copy()); 1886 }; 1887 if (signature != null) { 1888 dst.signature = new ArrayList<Signature>(); 1889 for (Signature i : signature) 1890 dst.signature.add(i.copy()); 1891 }; 1892 } 1893 1894 protected Provenance typedCopy() { 1895 return copy(); 1896 } 1897 1898 @Override 1899 public boolean equalsDeep(Base other_) { 1900 if (!super.equalsDeep(other_)) 1901 return false; 1902 if (!(other_ instanceof Provenance)) 1903 return false; 1904 Provenance o = (Provenance) other_; 1905 return compareDeep(target, o.target, true) && compareDeep(occurred, o.occurred, true) && compareDeep(recorded, o.recorded, true) 1906 && compareDeep(policy, o.policy, true) && compareDeep(location, o.location, true) && compareDeep(authorization, o.authorization, true) 1907 && compareDeep(activity, o.activity, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(patient, o.patient, true) 1908 && compareDeep(encounter, o.encounter, true) && compareDeep(agent, o.agent, true) && compareDeep(entity, o.entity, true) 1909 && compareDeep(signature, o.signature, true); 1910 } 1911 1912 @Override 1913 public boolean equalsShallow(Base other_) { 1914 if (!super.equalsShallow(other_)) 1915 return false; 1916 if (!(other_ instanceof Provenance)) 1917 return false; 1918 Provenance o = (Provenance) other_; 1919 return compareValues(recorded, o.recorded, true) && compareValues(policy, o.policy, true); 1920 } 1921 1922 public boolean isEmpty() { 1923 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, occurred, recorded 1924 , policy, location, authorization, activity, basedOn, patient, encounter, agent 1925 , entity, signature); 1926 } 1927 1928 @Override 1929 public ResourceType getResourceType() { 1930 return ResourceType.Provenance; 1931 } 1932 1933 /** 1934 * Search parameter: <b>activity</b> 1935 * <p> 1936 * Description: <b>Activity that occurred</b><br> 1937 * Type: <b>token</b><br> 1938 * Path: <b>Provenance.activity</b><br> 1939 * </p> 1940 */ 1941 @SearchParamDefinition(name="activity", path="Provenance.activity", description="Activity that occurred", type="token" ) 1942 public static final String SP_ACTIVITY = "activity"; 1943 /** 1944 * <b>Fluent Client</b> search parameter constant for <b>activity</b> 1945 * <p> 1946 * Description: <b>Activity that occurred</b><br> 1947 * Type: <b>token</b><br> 1948 * Path: <b>Provenance.activity</b><br> 1949 * </p> 1950 */ 1951 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVITY); 1952 1953 /** 1954 * Search parameter: <b>agent-role</b> 1955 * <p> 1956 * Description: <b>What the agents role was</b><br> 1957 * Type: <b>token</b><br> 1958 * Path: <b>Provenance.agent.role</b><br> 1959 * </p> 1960 */ 1961 @SearchParamDefinition(name="agent-role", path="Provenance.agent.role", description="What the agents role was", type="token" ) 1962 public static final String SP_AGENT_ROLE = "agent-role"; 1963 /** 1964 * <b>Fluent Client</b> search parameter constant for <b>agent-role</b> 1965 * <p> 1966 * Description: <b>What the agents role was</b><br> 1967 * Type: <b>token</b><br> 1968 * Path: <b>Provenance.agent.role</b><br> 1969 * </p> 1970 */ 1971 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_AGENT_ROLE); 1972 1973 /** 1974 * Search parameter: <b>agent-type</b> 1975 * <p> 1976 * Description: <b>How the agent participated</b><br> 1977 * Type: <b>token</b><br> 1978 * Path: <b>Provenance.agent.type</b><br> 1979 * </p> 1980 */ 1981 @SearchParamDefinition(name="agent-type", path="Provenance.agent.type", description="How the agent participated", type="token" ) 1982 public static final String SP_AGENT_TYPE = "agent-type"; 1983 /** 1984 * <b>Fluent Client</b> search parameter constant for <b>agent-type</b> 1985 * <p> 1986 * Description: <b>How the agent participated</b><br> 1987 * Type: <b>token</b><br> 1988 * Path: <b>Provenance.agent.type</b><br> 1989 * </p> 1990 */ 1991 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_AGENT_TYPE); 1992 1993 /** 1994 * Search parameter: <b>agent</b> 1995 * <p> 1996 * Description: <b>Who participated</b><br> 1997 * Type: <b>reference</b><br> 1998 * Path: <b>Provenance.agent.who</b><br> 1999 * </p> 2000 */ 2001 @SearchParamDefinition(name="agent", path="Provenance.agent.who", description="Who participated", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2002 public static final String SP_AGENT = "agent"; 2003 /** 2004 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 2005 * <p> 2006 * Description: <b>Who participated</b><br> 2007 * Type: <b>reference</b><br> 2008 * Path: <b>Provenance.agent.who</b><br> 2009 * </p> 2010 */ 2011 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AGENT); 2012 2013/** 2014 * Constant for fluent queries to be used to add include statements. Specifies 2015 * the path value of "<b>Provenance:agent</b>". 2016 */ 2017 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include("Provenance:agent").toLocked(); 2018 2019 /** 2020 * Search parameter: <b>based-on</b> 2021 * <p> 2022 * Description: <b>Reference to the service request.</b><br> 2023 * Type: <b>reference</b><br> 2024 * Path: <b>Provenance.basedOn</b><br> 2025 * </p> 2026 */ 2027 @SearchParamDefinition(name="based-on", path="Provenance.basedOn", description="Reference to the service request.", type="reference", target={CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, Task.class } ) 2028 public static final String SP_BASED_ON = "based-on"; 2029 /** 2030 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2031 * <p> 2032 * Description: <b>Reference to the service request.</b><br> 2033 * Type: <b>reference</b><br> 2034 * Path: <b>Provenance.basedOn</b><br> 2035 * </p> 2036 */ 2037 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2038 2039/** 2040 * Constant for fluent queries to be used to add include statements. Specifies 2041 * the path value of "<b>Provenance:based-on</b>". 2042 */ 2043 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Provenance:based-on").toLocked(); 2044 2045 /** 2046 * Search parameter: <b>entity</b> 2047 * <p> 2048 * Description: <b>Identity of entity</b><br> 2049 * Type: <b>reference</b><br> 2050 * Path: <b>Provenance.entity.what</b><br> 2051 * </p> 2052 */ 2053 @SearchParamDefinition(name="entity", path="Provenance.entity.what", description="Identity of entity", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2054 public static final String SP_ENTITY = "entity"; 2055 /** 2056 * <b>Fluent Client</b> search parameter constant for <b>entity</b> 2057 * <p> 2058 * Description: <b>Identity of entity</b><br> 2059 * Type: <b>reference</b><br> 2060 * Path: <b>Provenance.entity.what</b><br> 2061 * </p> 2062 */ 2063 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTITY); 2064 2065/** 2066 * Constant for fluent queries to be used to add include statements. Specifies 2067 * the path value of "<b>Provenance:entity</b>". 2068 */ 2069 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTITY = new ca.uhn.fhir.model.api.Include("Provenance:entity").toLocked(); 2070 2071 /** 2072 * Search parameter: <b>location</b> 2073 * <p> 2074 * Description: <b>Where the activity occurred, if relevant</b><br> 2075 * Type: <b>reference</b><br> 2076 * Path: <b>Provenance.location</b><br> 2077 * </p> 2078 */ 2079 @SearchParamDefinition(name="location", path="Provenance.location", description="Where the activity occurred, if relevant", type="reference", target={Location.class } ) 2080 public static final String SP_LOCATION = "location"; 2081 /** 2082 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2083 * <p> 2084 * Description: <b>Where the activity occurred, if relevant</b><br> 2085 * Type: <b>reference</b><br> 2086 * Path: <b>Provenance.location</b><br> 2087 * </p> 2088 */ 2089 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2090 2091/** 2092 * Constant for fluent queries to be used to add include statements. Specifies 2093 * the path value of "<b>Provenance:location</b>". 2094 */ 2095 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Provenance:location").toLocked(); 2096 2097 /** 2098 * Search parameter: <b>recorded</b> 2099 * <p> 2100 * Description: <b>When the activity was recorded / updated</b><br> 2101 * Type: <b>date</b><br> 2102 * Path: <b>Provenance.recorded</b><br> 2103 * </p> 2104 */ 2105 @SearchParamDefinition(name="recorded", path="Provenance.recorded", description="When the activity was recorded / updated", type="date" ) 2106 public static final String SP_RECORDED = "recorded"; 2107 /** 2108 * <b>Fluent Client</b> search parameter constant for <b>recorded</b> 2109 * <p> 2110 * Description: <b>When the activity was recorded / updated</b><br> 2111 * Type: <b>date</b><br> 2112 * Path: <b>Provenance.recorded</b><br> 2113 * </p> 2114 */ 2115 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECORDED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_RECORDED); 2116 2117 /** 2118 * Search parameter: <b>signature-type</b> 2119 * <p> 2120 * Description: <b>Indication of the reason the entity signed the object(s)</b><br> 2121 * Type: <b>token</b><br> 2122 * Path: <b>Provenance.signature.type</b><br> 2123 * </p> 2124 */ 2125 @SearchParamDefinition(name="signature-type", path="Provenance.signature.type", description="Indication of the reason the entity signed the object(s)", type="token" ) 2126 public static final String SP_SIGNATURE_TYPE = "signature-type"; 2127 /** 2128 * <b>Fluent Client</b> search parameter constant for <b>signature-type</b> 2129 * <p> 2130 * Description: <b>Indication of the reason the entity signed the object(s)</b><br> 2131 * Type: <b>token</b><br> 2132 * Path: <b>Provenance.signature.type</b><br> 2133 * </p> 2134 */ 2135 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SIGNATURE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SIGNATURE_TYPE); 2136 2137 /** 2138 * Search parameter: <b>target</b> 2139 * <p> 2140 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2141 * Type: <b>reference</b><br> 2142 * Path: <b>Provenance.target</b><br> 2143 * </p> 2144 */ 2145 @SearchParamDefinition(name="target", path="Provenance.target", description="Target Reference(s) (usually version specific)", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2146 public static final String SP_TARGET = "target"; 2147 /** 2148 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2149 * <p> 2150 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2151 * Type: <b>reference</b><br> 2152 * Path: <b>Provenance.target</b><br> 2153 * </p> 2154 */ 2155 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET); 2156 2157/** 2158 * Constant for fluent queries to be used to add include statements. Specifies 2159 * the path value of "<b>Provenance:target</b>". 2160 */ 2161 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include("Provenance:target").toLocked(); 2162 2163 /** 2164 * Search parameter: <b>when</b> 2165 * <p> 2166 * Description: <b>When the activity occurred</b><br> 2167 * Type: <b>date</b><br> 2168 * Path: <b>(Provenance.occurred.ofType(dateTime))</b><br> 2169 * </p> 2170 */ 2171 @SearchParamDefinition(name="when", path="(Provenance.occurred.ofType(dateTime))", description="When the activity occurred", type="date" ) 2172 public static final String SP_WHEN = "when"; 2173 /** 2174 * <b>Fluent Client</b> search parameter constant for <b>when</b> 2175 * <p> 2176 * Description: <b>When the activity occurred</b><br> 2177 * Type: <b>date</b><br> 2178 * Path: <b>(Provenance.occurred.ofType(dateTime))</b><br> 2179 * </p> 2180 */ 2181 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHEN = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_WHEN); 2182 2183 /** 2184 * Search parameter: <b>encounter</b> 2185 * <p> 2186 * Description: <b>Multiple Resources: 2187 2188* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2189* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2190* [ChargeItem](chargeitem.html): Encounter associated with event 2191* [Claim](claim.html): Encounters associated with a billed line item 2192* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2193* [Communication](communication.html): The Encounter during which this Communication was created 2194* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2195* [Composition](composition.html): Context of the Composition 2196* [Condition](condition.html): The Encounter during which this Condition was created 2197* [DeviceRequest](devicerequest.html): Encounter during which request was created 2198* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2199* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2200* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2201* [Flag](flag.html): Alert relevant during encounter 2202* [ImagingStudy](imagingstudy.html): The context of the study 2203* [List](list.html): Context in which list created 2204* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2205* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2206* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2207* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2208* [Observation](observation.html): Encounter related to the observation 2209* [Procedure](procedure.html): The Encounter during which this Procedure was created 2210* [Provenance](provenance.html): Encounter related to the Provenance 2211* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2212* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2213* [RiskAssessment](riskassessment.html): Where was assessment performed? 2214* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2215* [Task](task.html): Search by encounter 2216* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2217</b><br> 2218 * Type: <b>reference</b><br> 2219 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2220 * </p> 2221 */ 2222 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", target={Encounter.class } ) 2223 public static final String SP_ENCOUNTER = "encounter"; 2224 /** 2225 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2226 * <p> 2227 * Description: <b>Multiple Resources: 2228 2229* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2230* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2231* [ChargeItem](chargeitem.html): Encounter associated with event 2232* [Claim](claim.html): Encounters associated with a billed line item 2233* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2234* [Communication](communication.html): The Encounter during which this Communication was created 2235* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2236* [Composition](composition.html): Context of the Composition 2237* [Condition](condition.html): The Encounter during which this Condition was created 2238* [DeviceRequest](devicerequest.html): Encounter during which request was created 2239* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2240* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2241* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2242* [Flag](flag.html): Alert relevant during encounter 2243* [ImagingStudy](imagingstudy.html): The context of the study 2244* [List](list.html): Context in which list created 2245* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2246* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2247* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2248* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2249* [Observation](observation.html): Encounter related to the observation 2250* [Procedure](procedure.html): The Encounter during which this Procedure was created 2251* [Provenance](provenance.html): Encounter related to the Provenance 2252* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2253* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2254* [RiskAssessment](riskassessment.html): Where was assessment performed? 2255* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2256* [Task](task.html): Search by encounter 2257* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2258</b><br> 2259 * Type: <b>reference</b><br> 2260 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2261 * </p> 2262 */ 2263 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2264 2265/** 2266 * Constant for fluent queries to be used to add include statements. Specifies 2267 * the path value of "<b>Provenance:encounter</b>". 2268 */ 2269 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Provenance:encounter").toLocked(); 2270 2271 /** 2272 * Search parameter: <b>patient</b> 2273 * <p> 2274 * Description: <b>Multiple Resources: 2275 2276* [Account](account.html): The entity that caused the expenses 2277* [AdverseEvent](adverseevent.html): Subject impacted by event 2278* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2279* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2280* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2281* [AuditEvent](auditevent.html): Where the activity involved patient data 2282* [Basic](basic.html): Identifies the focus of this resource 2283* [BodyStructure](bodystructure.html): Who this is about 2284* [CarePlan](careplan.html): Who the care plan is for 2285* [CareTeam](careteam.html): Who care team is for 2286* [ChargeItem](chargeitem.html): Individual service was done for/to 2287* [Claim](claim.html): Patient receiving the products or services 2288* [ClaimResponse](claimresponse.html): The subject of care 2289* [ClinicalImpression](clinicalimpression.html): Patient assessed 2290* [Communication](communication.html): Focus of message 2291* [CommunicationRequest](communicationrequest.html): Focus of message 2292* [Composition](composition.html): Who and/or what the composition is about 2293* [Condition](condition.html): Who has the condition? 2294* [Consent](consent.html): Who the consent applies to 2295* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2296* [Coverage](coverage.html): Retrieve coverages for a patient 2297* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2298* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2299* [DetectedIssue](detectedissue.html): Associated patient 2300* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2301* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2302* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2303* [DocumentReference](documentreference.html): Who/what is the subject of the document 2304* [Encounter](encounter.html): The patient present at the encounter 2305* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2306* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2307* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2308* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2309* [Flag](flag.html): The identity of a subject to list flags for 2310* [Goal](goal.html): Who this goal is intended for 2311* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2312* [ImagingSelection](imagingselection.html): Who the study is about 2313* [ImagingStudy](imagingstudy.html): Who the study is about 2314* [Immunization](immunization.html): The patient for the vaccination record 2315* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2316* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2317* [Invoice](invoice.html): Recipient(s) of goods and services 2318* [List](list.html): If all resources have the same subject 2319* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2320* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2321* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2322* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2323* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2324* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2325* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2326* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2327* [Observation](observation.html): The subject that the observation is about (if patient) 2328* [Person](person.html): The Person links to this Patient 2329* [Procedure](procedure.html): Search by subject - a patient 2330* [Provenance](provenance.html): Where the activity involved patient data 2331* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2332* [RelatedPerson](relatedperson.html): The patient this related person is related to 2333* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2334* [ResearchSubject](researchsubject.html): Who or what is part of study 2335* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2336* [ServiceRequest](servicerequest.html): Search by subject - a patient 2337* [Specimen](specimen.html): The patient the specimen comes from 2338* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2339* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2340* [Task](task.html): Search by patient 2341* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2342</b><br> 2343 * Type: <b>reference</b><br> 2344 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2345 * </p> 2346 */ 2347 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2348 public static final String SP_PATIENT = "patient"; 2349 /** 2350 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2351 * <p> 2352 * Description: <b>Multiple Resources: 2353 2354* [Account](account.html): The entity that caused the expenses 2355* [AdverseEvent](adverseevent.html): Subject impacted by event 2356* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2357* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2358* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2359* [AuditEvent](auditevent.html): Where the activity involved patient data 2360* [Basic](basic.html): Identifies the focus of this resource 2361* [BodyStructure](bodystructure.html): Who this is about 2362* [CarePlan](careplan.html): Who the care plan is for 2363* [CareTeam](careteam.html): Who care team is for 2364* [ChargeItem](chargeitem.html): Individual service was done for/to 2365* [Claim](claim.html): Patient receiving the products or services 2366* [ClaimResponse](claimresponse.html): The subject of care 2367* [ClinicalImpression](clinicalimpression.html): Patient assessed 2368* [Communication](communication.html): Focus of message 2369* [CommunicationRequest](communicationrequest.html): Focus of message 2370* [Composition](composition.html): Who and/or what the composition is about 2371* [Condition](condition.html): Who has the condition? 2372* [Consent](consent.html): Who the consent applies to 2373* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2374* [Coverage](coverage.html): Retrieve coverages for a patient 2375* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2376* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2377* [DetectedIssue](detectedissue.html): Associated patient 2378* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2379* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2380* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2381* [DocumentReference](documentreference.html): Who/what is the subject of the document 2382* [Encounter](encounter.html): The patient present at the encounter 2383* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2384* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2385* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2386* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2387* [Flag](flag.html): The identity of a subject to list flags for 2388* [Goal](goal.html): Who this goal is intended for 2389* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2390* [ImagingSelection](imagingselection.html): Who the study is about 2391* [ImagingStudy](imagingstudy.html): Who the study is about 2392* [Immunization](immunization.html): The patient for the vaccination record 2393* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2394* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2395* [Invoice](invoice.html): Recipient(s) of goods and services 2396* [List](list.html): If all resources have the same subject 2397* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2398* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2399* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2400* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2401* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2402* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2403* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2404* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2405* [Observation](observation.html): The subject that the observation is about (if patient) 2406* [Person](person.html): The Person links to this Patient 2407* [Procedure](procedure.html): Search by subject - a patient 2408* [Provenance](provenance.html): Where the activity involved patient data 2409* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2410* [RelatedPerson](relatedperson.html): The patient this related person is related to 2411* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2412* [ResearchSubject](researchsubject.html): Who or what is part of study 2413* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2414* [ServiceRequest](servicerequest.html): Search by subject - a patient 2415* [Specimen](specimen.html): The patient the specimen comes from 2416* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2417* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2418* [Task](task.html): Search by patient 2419* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2420</b><br> 2421 * Type: <b>reference</b><br> 2422 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2423 * </p> 2424 */ 2425 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2426 2427/** 2428 * Constant for fluent queries to be used to add include statements. Specifies 2429 * the path value of "<b>Provenance:patient</b>". 2430 */ 2431 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Provenance:patient").toLocked(); 2432 2433 2434} 2435