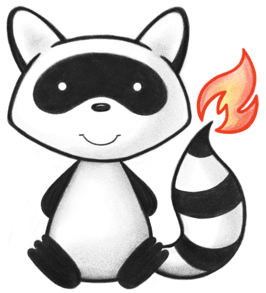
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Quantity Type: A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 051 */ 052@DatatypeDef(name="Quantity") 053public class Quantity extends DataType implements ICompositeType, ICoding { 054 055 /** 056 * The value of the measured amount. The value includes an implicit precision in the presentation of the value. 057 */ 058 @Child(name = "value", type = {DecimalType.class}, order=0, min=0, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Numerical value (with implicit precision)", formalDefinition="The value of the measured amount. The value includes an implicit precision in the presentation of the value." ) 060 protected DecimalType value; 061 062 /** 063 * How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value. 064 */ 065 @Child(name = "comparator", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 066 @Description(shortDefinition="< | <= | >= | > | ad - how to understand the value", formalDefinition="How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value." ) 067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/quantity-comparator") 068 protected Enumeration<QuantityComparator> comparator; 069 070 /** 071 * A human-readable form of the unit. 072 */ 073 @Child(name = "unit", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 074 @Description(shortDefinition="Unit representation", formalDefinition="A human-readable form of the unit." ) 075 protected StringType unit; 076 077 /** 078 * The identification of the system that provides the coded form of the unit. 079 */ 080 @Child(name = "system", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="System that defines coded unit form", formalDefinition="The identification of the system that provides the coded form of the unit." ) 082 protected UriType system; 083 084 /** 085 * A computer processable form of the unit in some unit representation system. 086 */ 087 @Child(name = "code", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 088 @Description(shortDefinition="Coded form of the unit", formalDefinition="A computer processable form of the unit in some unit representation system." ) 089 protected CodeType code; 090 091 private static final long serialVersionUID = 1069574054L; 092 093 /** 094 * Constructor 095 */ 096 public Quantity() { 097 super(); 098 } 099 100 /** 101 * Convenience constructor 102 * 103 * @param theValue The {@link #setValue(double) value} 104 */ 105 public Quantity(double theValue) { 106 setValue(theValue); 107 } 108 109 /** 110 * Convenience constructor 111 * 112 * @param theValue The {@link #setValue(long) value} 113 */ 114 public Quantity(long theValue) { 115 setValue(theValue); 116 } 117 118 /** 119 * Convenience constructor 120 * 121 * @param theComparator The {@link #setComparator(QuantityComparator) comparator} 122 * @param theValue The {@link #setValue(BigDecimal) value} 123 * @param theSystem The {@link #setSystem(String)} (the code system for the units} 124 * @param theCode The {@link #setCode(String)} (the code for the units} 125 * @param theUnit The {@link #setUnit(String)} (the human readable display name for the units} 126 */ 127 public Quantity(QuantityComparator theComparator, double theValue, String theSystem, String theCode, String theUnit) { 128 setValue(theValue); 129 setComparator(theComparator); 130 setSystem(theSystem); 131 setCode(theCode); 132 setUnit(theUnit); 133 } 134 135 /** 136 * Convenience constructor 137 * 138 * @param theComparator The {@link #setComparator(QuantityComparator) comparator} 139 * @param theValue The {@link #setValue(BigDecimal) value} 140 * @param theSystem The {@link #setSystem(String)} (the code system for the units} 141 * @param theCode The {@link #setCode(String)} (the code for the units} 142 * @param theUnit The {@link #setUnit(String)} (the human readable display name for the units} 143 */ 144 public Quantity(QuantityComparator theComparator, long theValue, String theSystem, String theCode, String theUnit) { 145 setValue(theValue); 146 setComparator(theComparator); 147 setSystem(theSystem); 148 setCode(theCode); 149 setUnit(theUnit); 150 } 151 /** 152 * @return {@link #value} (The value of the measured amount. The value includes an implicit precision in the presentation of the value.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 153 */ 154 public DecimalType getValueElement() { 155 if (this.value == null) 156 if (Configuration.errorOnAutoCreate()) 157 throw new Error("Attempt to auto-create Quantity.value"); 158 else if (Configuration.doAutoCreate()) 159 this.value = new DecimalType(); // bb 160 return this.value; 161 } 162 163 public boolean hasValueElement() { 164 return this.value != null && !this.value.isEmpty(); 165 } 166 167 public boolean hasValue() { 168 return this.value != null && !this.value.isEmpty(); 169 } 170 171 /** 172 * @param value {@link #value} (The value of the measured amount. The value includes an implicit precision in the presentation of the value.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 173 */ 174 public Quantity setValueElement(DecimalType value) { 175 this.value = value; 176 return this; 177 } 178 179 /** 180 * @return The value of the measured amount. The value includes an implicit precision in the presentation of the value. 181 */ 182 public BigDecimal getValue() { 183 return this.value == null ? null : this.value.getValue(); 184 } 185 186 /** 187 * @param value The value of the measured amount. The value includes an implicit precision in the presentation of the value. 188 */ 189 public Quantity setValue(BigDecimal value) { 190 if (value == null) 191 this.value = null; 192 else { 193 if (this.value == null) 194 this.value = new DecimalType(); 195 this.value.setValue(value); 196 } 197 return this; 198 } 199 200 /** 201 * @param value The value of the measured amount. The value includes an implicit precision in the presentation of the value. 202 */ 203 public Quantity setValue(long value) { 204 this.value = new DecimalType(); 205 this.value.setValue(value); 206 return this; 207 } 208 209 /** 210 * @param value The value of the measured amount. The value includes an implicit precision in the presentation of the value. 211 */ 212 public Quantity setValue(double value) { 213 this.value = new DecimalType(); 214 this.value.setValue(value); 215 return this; 216 } 217 218 /** 219 * @return {@link #comparator} (How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 220 */ 221 public Enumeration<QuantityComparator> getComparatorElement() { 222 if (this.comparator == null) 223 if (Configuration.errorOnAutoCreate()) 224 throw new Error("Attempt to auto-create Quantity.comparator"); 225 else if (Configuration.doAutoCreate()) 226 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); // bb 227 return this.comparator; 228 } 229 230 public boolean hasComparatorElement() { 231 return this.comparator != null && !this.comparator.isEmpty(); 232 } 233 234 public boolean hasComparator() { 235 return this.comparator != null && !this.comparator.isEmpty(); 236 } 237 238 /** 239 * @param value {@link #comparator} (How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 240 */ 241 public Quantity setComparatorElement(Enumeration<QuantityComparator> value) { 242 this.comparator = value; 243 return this; 244 } 245 246 /** 247 * @return How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value. 248 */ 249 public QuantityComparator getComparator() { 250 return this.comparator == null ? null : this.comparator.getValue(); 251 } 252 253 /** 254 * @param value How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value. 255 */ 256 public Quantity setComparator(QuantityComparator value) { 257 if (value == null) 258 this.comparator = null; 259 else { 260 if (this.comparator == null) 261 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); 262 this.comparator.setValue(value); 263 } 264 return this; 265 } 266 267 /** 268 * @return {@link #unit} (A human-readable form of the unit.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value 269 */ 270 public StringType getUnitElement() { 271 if (this.unit == null) 272 if (Configuration.errorOnAutoCreate()) 273 throw new Error("Attempt to auto-create Quantity.unit"); 274 else if (Configuration.doAutoCreate()) 275 this.unit = new StringType(); // bb 276 return this.unit; 277 } 278 279 public boolean hasUnitElement() { 280 return this.unit != null && !this.unit.isEmpty(); 281 } 282 283 public boolean hasUnit() { 284 return this.unit != null && !this.unit.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #unit} (A human-readable form of the unit.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value 289 */ 290 public Quantity setUnitElement(StringType value) { 291 this.unit = value; 292 return this; 293 } 294 295 /** 296 * @return A human-readable form of the unit. 297 */ 298 public String getUnit() { 299 return this.unit == null ? null : this.unit.getValue(); 300 } 301 302 /** 303 * @param value A human-readable form of the unit. 304 */ 305 public Quantity setUnit(String value) { 306 if (Utilities.noString(value)) 307 this.unit = null; 308 else { 309 if (this.unit == null) 310 this.unit = new StringType(); 311 this.unit.setValue(value); 312 } 313 return this; 314 } 315 316 /** 317 * @return {@link #system} (The identification of the system that provides the coded form of the unit.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 318 */ 319 public UriType getSystemElement() { 320 if (this.system == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create Quantity.system"); 323 else if (Configuration.doAutoCreate()) 324 this.system = new UriType(); // bb 325 return this.system; 326 } 327 328 public boolean hasSystemElement() { 329 return this.system != null && !this.system.isEmpty(); 330 } 331 332 public boolean hasSystem() { 333 return this.system != null && !this.system.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #system} (The identification of the system that provides the coded form of the unit.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 338 */ 339 public Quantity setSystemElement(UriType value) { 340 this.system = value; 341 return this; 342 } 343 344 /** 345 * @return The identification of the system that provides the coded form of the unit. 346 */ 347 public String getSystem() { 348 return this.system == null ? null : this.system.getValue(); 349 } 350 351 /** 352 * @param value The identification of the system that provides the coded form of the unit. 353 */ 354 public Quantity setSystem(String value) { 355 if (Utilities.noString(value)) 356 this.system = null; 357 else { 358 if (this.system == null) 359 this.system = new UriType(); 360 this.system.setValue(value); 361 } 362 return this; 363 } 364 365 /** 366 * @return {@link #code} (A computer processable form of the unit in some unit representation system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 367 */ 368 public CodeType getCodeElement() { 369 if (this.code == null) 370 if (Configuration.errorOnAutoCreate()) 371 throw new Error("Attempt to auto-create Quantity.code"); 372 else if (Configuration.doAutoCreate()) 373 this.code = new CodeType(); // bb 374 return this.code; 375 } 376 377 public boolean hasCodeElement() { 378 return this.code != null && !this.code.isEmpty(); 379 } 380 381 public boolean hasCode() { 382 return this.code != null && !this.code.isEmpty(); 383 } 384 385 /** 386 * @param value {@link #code} (A computer processable form of the unit in some unit representation system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 387 */ 388 public Quantity setCodeElement(CodeType value) { 389 this.code = value; 390 return this; 391 } 392 393 /** 394 * @return A computer processable form of the unit in some unit representation system. 395 */ 396 public String getCode() { 397 return this.code == null ? null : this.code.getValue(); 398 } 399 400 /** 401 * @param value A computer processable form of the unit in some unit representation system. 402 */ 403 public Quantity setCode(String value) { 404 if (Utilities.noString(value)) 405 this.code = null; 406 else { 407 if (this.code == null) 408 this.code = new CodeType(); 409 this.code.setValue(value); 410 } 411 return this; 412 } 413 414 protected void listChildren(List<Property> children) { 415 super.listChildren(children); 416 children.add(new Property("value", "decimal", "The value of the measured amount. The value includes an implicit precision in the presentation of the value.", 0, 1, value)); 417 children.add(new Property("comparator", "code", "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.", 0, 1, comparator)); 418 children.add(new Property("unit", "string", "A human-readable form of the unit.", 0, 1, unit)); 419 children.add(new Property("system", "uri", "The identification of the system that provides the coded form of the unit.", 0, 1, system)); 420 children.add(new Property("code", "code", "A computer processable form of the unit in some unit representation system.", 0, 1, code)); 421 } 422 423 @Override 424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 425 switch (_hash) { 426 case 111972721: /*value*/ return new Property("value", "decimal", "The value of the measured amount. The value includes an implicit precision in the presentation of the value.", 0, 1, value); 427 case -844673834: /*comparator*/ return new Property("comparator", "code", "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.", 0, 1, comparator); 428 case 3594628: /*unit*/ return new Property("unit", "string", "A human-readable form of the unit.", 0, 1, unit); 429 case -887328209: /*system*/ return new Property("system", "uri", "The identification of the system that provides the coded form of the unit.", 0, 1, system); 430 case 3059181: /*code*/ return new Property("code", "code", "A computer processable form of the unit in some unit representation system.", 0, 1, code); 431 default: return super.getNamedProperty(_hash, _name, _checkValid); 432 } 433 434 } 435 436 @Override 437 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 438 switch (hash) { 439 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DecimalType 440 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : new Base[] {this.comparator}; // Enumeration<QuantityComparator> 441 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // StringType 442 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 443 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 444 default: return super.getProperty(hash, name, checkValid); 445 } 446 447 } 448 449 @Override 450 public Base setProperty(int hash, String name, Base value) throws FHIRException { 451 switch (hash) { 452 case 111972721: // value 453 this.value = TypeConvertor.castToDecimal(value); // DecimalType 454 return value; 455 case -844673834: // comparator 456 value = new QuantityComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 457 this.comparator = (Enumeration) value; // Enumeration<QuantityComparator> 458 return value; 459 case 3594628: // unit 460 this.unit = TypeConvertor.castToString(value); // StringType 461 return value; 462 case -887328209: // system 463 this.system = TypeConvertor.castToUri(value); // UriType 464 return value; 465 case 3059181: // code 466 this.code = TypeConvertor.castToCode(value); // CodeType 467 return value; 468 default: return super.setProperty(hash, name, value); 469 } 470 471 } 472 473 @Override 474 public Base setProperty(String name, Base value) throws FHIRException { 475 if (name.equals("value")) { 476 this.value = TypeConvertor.castToDecimal(value); // DecimalType 477 } else if (name.equals("comparator")) { 478 value = new QuantityComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 479 this.comparator = (Enumeration) value; // Enumeration<QuantityComparator> 480 } else if (name.equals("unit")) { 481 this.unit = TypeConvertor.castToString(value); // StringType 482 } else if (name.equals("system")) { 483 this.system = TypeConvertor.castToUri(value); // UriType 484 } else if (name.equals("code")) { 485 this.code = TypeConvertor.castToCode(value); // CodeType 486 } else 487 return super.setProperty(name, value); 488 return value; 489 } 490 491 @Override 492 public void removeChild(String name, Base value) throws FHIRException { 493 if (name.equals("value")) { 494 this.value = null; 495 } else if (name.equals("comparator")) { 496 value = new QuantityComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 497 this.comparator = (Enumeration) value; // Enumeration<QuantityComparator> 498 } else if (name.equals("unit")) { 499 this.unit = null; 500 } else if (name.equals("system")) { 501 this.system = null; 502 } else if (name.equals("code")) { 503 this.code = null; 504 } else 505 super.removeChild(name, value); 506 507 } 508 509 @Override 510 public Base makeProperty(int hash, String name) throws FHIRException { 511 switch (hash) { 512 case 111972721: return getValueElement(); 513 case -844673834: return getComparatorElement(); 514 case 3594628: return getUnitElement(); 515 case -887328209: return getSystemElement(); 516 case 3059181: return getCodeElement(); 517 default: return super.makeProperty(hash, name); 518 } 519 520 } 521 522 @Override 523 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 524 switch (hash) { 525 case 111972721: /*value*/ return new String[] {"decimal"}; 526 case -844673834: /*comparator*/ return new String[] {"code"}; 527 case 3594628: /*unit*/ return new String[] {"string"}; 528 case -887328209: /*system*/ return new String[] {"uri"}; 529 case 3059181: /*code*/ return new String[] {"code"}; 530 default: return super.getTypesForProperty(hash, name); 531 } 532 533 } 534 535 @Override 536 public Base addChild(String name) throws FHIRException { 537 if (name.equals("value")) { 538 throw new FHIRException("Cannot call addChild on a singleton property Quantity.value"); 539 } 540 else if (name.equals("comparator")) { 541 throw new FHIRException("Cannot call addChild on a singleton property Quantity.comparator"); 542 } 543 else if (name.equals("unit")) { 544 throw new FHIRException("Cannot call addChild on a singleton property Quantity.unit"); 545 } 546 else if (name.equals("system")) { 547 throw new FHIRException("Cannot call addChild on a singleton property Quantity.system"); 548 } 549 else if (name.equals("code")) { 550 throw new FHIRException("Cannot call addChild on a singleton property Quantity.code"); 551 } 552 else 553 return super.addChild(name); 554 } 555 556 public String fhirType() { 557 return "Quantity"; 558 559 } 560 561 public Quantity copy() { 562 Quantity dst = new Quantity(); 563 copyValues(dst); 564 return dst; 565 } 566 567 public void copyValues(Quantity dst) { 568 super.copyValues(dst); 569 dst.value = value == null ? null : value.copy(); 570 dst.comparator = comparator == null ? null : comparator.copy(); 571 dst.unit = unit == null ? null : unit.copy(); 572 dst.system = system == null ? null : system.copy(); 573 dst.code = code == null ? null : code.copy(); 574 } 575 576 protected Quantity typedCopy() { 577 return copy(); 578 } 579 580 @Override 581 public boolean equalsDeep(Base other_) { 582 if (!super.equalsDeep(other_)) 583 return false; 584 if (!(other_ instanceof Quantity)) 585 return false; 586 Quantity o = (Quantity) other_; 587 return compareDeep(value, o.value, true) && compareDeep(comparator, o.comparator, true) && compareDeep(unit, o.unit, true) 588 && compareDeep(system, o.system, true) && compareDeep(code, o.code, true); 589 } 590 591 @Override 592 public boolean equalsShallow(Base other_) { 593 if (!super.equalsShallow(other_)) 594 return false; 595 if (!(other_ instanceof Quantity)) 596 return false; 597 Quantity o = (Quantity) other_; 598 return compareValues(value, o.value, true) && compareValues(comparator, o.comparator, true) && compareValues(unit, o.unit, true) 599 && compareValues(system, o.system, true) && compareValues(code, o.code, true); 600 } 601 602 public boolean isEmpty() { 603 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, comparator, unit 604 , system, code); 605 } 606 607// Manual code (from Configuration.txt): 608@Override 609 public String getVersion() { 610 return null; 611 } 612 613 @Override 614 public boolean hasVersion() { 615 return false; 616 } 617 618 @Override 619 public boolean supportsVersion() { 620 return false; 621 } 622 623 @Override 624 public String getDisplay() { 625 return null; 626 } 627 628 @Override 629 public boolean hasDisplay() { 630 return false; 631 } 632 633 @Override 634 public boolean supportsDisplay() { 635 return false; 636 } 637 638 public static Quantity fromUcum(String v, String code) { 639 Quantity res = new Quantity(); 640 res.setValue(new BigDecimal(v)); 641 res.setSystem("http://unitsofmeasure.org"); 642 res.setCode(code); 643 return res; 644 } 645// end addition 646 647} 648