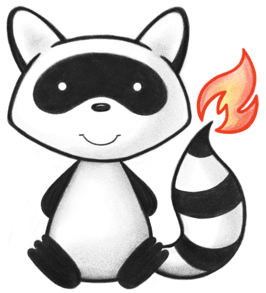
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 052 */ 053@ResourceDef(name="Questionnaire", profile="http://hl7.org/fhir/StructureDefinition/Questionnaire") 054public class Questionnaire extends MetadataResource { 055 056 public enum EnableWhenBehavior { 057 /** 058 * Enable the question when all the enableWhen criteria are satisfied. 059 */ 060 ALL, 061 /** 062 * Enable the question when any of the enableWhen criteria are satisfied. 063 */ 064 ANY, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static EnableWhenBehavior fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("all".equals(codeString)) 073 return ALL; 074 if ("any".equals(codeString)) 075 return ANY; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown EnableWhenBehavior code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case ALL: return "all"; 084 case ANY: return "any"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case ALL: return "http://hl7.org/fhir/questionnaire-enable-behavior"; 092 case ANY: return "http://hl7.org/fhir/questionnaire-enable-behavior"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case ALL: return "Enable the question when all the enableWhen criteria are satisfied."; 100 case ANY: return "Enable the question when any of the enableWhen criteria are satisfied."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case ALL: return "All"; 108 case ANY: return "Any"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class EnableWhenBehaviorEnumFactory implements EnumFactory<EnableWhenBehavior> { 116 public EnableWhenBehavior fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("all".equals(codeString)) 121 return EnableWhenBehavior.ALL; 122 if ("any".equals(codeString)) 123 return EnableWhenBehavior.ANY; 124 throw new IllegalArgumentException("Unknown EnableWhenBehavior code '"+codeString+"'"); 125 } 126 public Enumeration<EnableWhenBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.NULL, code); 134 if ("all".equals(codeString)) 135 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.ALL, code); 136 if ("any".equals(codeString)) 137 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.ANY, code); 138 throw new FHIRException("Unknown EnableWhenBehavior code '"+codeString+"'"); 139 } 140 public String toCode(EnableWhenBehavior code) { 141 if (code == EnableWhenBehavior.NULL) 142 return null; 143 if (code == EnableWhenBehavior.ALL) 144 return "all"; 145 if (code == EnableWhenBehavior.ANY) 146 return "any"; 147 return "?"; 148 } 149 public String toSystem(EnableWhenBehavior code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum QuestionnaireAnswerConstraint { 155 /** 156 * Only values listed as answerOption or in the expansion of the answerValueSet are permitted 157 */ 158 OPTIONSONLY, 159 /** 160 * In addition to the values listed as answerOption or in the expansion of the answerValueSet, any other values that correspond to the specified item.type are permitted 161 */ 162 OPTIONSORTYPE, 163 /** 164 * In addition to the values listed as answerOption or in the expansion of the answerValueSet, free-text strings are permitted. Answers will have a type of 'string'. 165 */ 166 OPTIONSORSTRING, 167 /** 168 * added to help the parsers with the generic types 169 */ 170 NULL; 171 public static QuestionnaireAnswerConstraint fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("optionsOnly".equals(codeString)) 175 return OPTIONSONLY; 176 if ("optionsOrType".equals(codeString)) 177 return OPTIONSORTYPE; 178 if ("optionsOrString".equals(codeString)) 179 return OPTIONSORSTRING; 180 if (Configuration.isAcceptInvalidEnums()) 181 return null; 182 else 183 throw new FHIRException("Unknown QuestionnaireAnswerConstraint code '"+codeString+"'"); 184 } 185 public String toCode() { 186 switch (this) { 187 case OPTIONSONLY: return "optionsOnly"; 188 case OPTIONSORTYPE: return "optionsOrType"; 189 case OPTIONSORSTRING: return "optionsOrString"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case OPTIONSONLY: return "http://hl7.org/fhir/questionnaire-answer-constraint"; 197 case OPTIONSORTYPE: return "http://hl7.org/fhir/questionnaire-answer-constraint"; 198 case OPTIONSORSTRING: return "http://hl7.org/fhir/questionnaire-answer-constraint"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getDefinition() { 204 switch (this) { 205 case OPTIONSONLY: return "Only values listed as answerOption or in the expansion of the answerValueSet are permitted"; 206 case OPTIONSORTYPE: return "In addition to the values listed as answerOption or in the expansion of the answerValueSet, any other values that correspond to the specified item.type are permitted"; 207 case OPTIONSORSTRING: return "In addition to the values listed as answerOption or in the expansion of the answerValueSet, free-text strings are permitted. Answers will have a type of 'string'."; 208 case NULL: return null; 209 default: return "?"; 210 } 211 } 212 public String getDisplay() { 213 switch (this) { 214 case OPTIONSONLY: return "Options only"; 215 case OPTIONSORTYPE: return "Options or 'type'"; 216 case OPTIONSORSTRING: return "Options or string"; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 } 222 223 public static class QuestionnaireAnswerConstraintEnumFactory implements EnumFactory<QuestionnaireAnswerConstraint> { 224 public QuestionnaireAnswerConstraint fromCode(String codeString) throws IllegalArgumentException { 225 if (codeString == null || "".equals(codeString)) 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("optionsOnly".equals(codeString)) 229 return QuestionnaireAnswerConstraint.OPTIONSONLY; 230 if ("optionsOrType".equals(codeString)) 231 return QuestionnaireAnswerConstraint.OPTIONSORTYPE; 232 if ("optionsOrString".equals(codeString)) 233 return QuestionnaireAnswerConstraint.OPTIONSORSTRING; 234 throw new IllegalArgumentException("Unknown QuestionnaireAnswerConstraint code '"+codeString+"'"); 235 } 236 public Enumeration<QuestionnaireAnswerConstraint> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<QuestionnaireAnswerConstraint>(this, QuestionnaireAnswerConstraint.NULL, code); 241 String codeString = ((PrimitiveType) code).asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<QuestionnaireAnswerConstraint>(this, QuestionnaireAnswerConstraint.NULL, code); 244 if ("optionsOnly".equals(codeString)) 245 return new Enumeration<QuestionnaireAnswerConstraint>(this, QuestionnaireAnswerConstraint.OPTIONSONLY, code); 246 if ("optionsOrType".equals(codeString)) 247 return new Enumeration<QuestionnaireAnswerConstraint>(this, QuestionnaireAnswerConstraint.OPTIONSORTYPE, code); 248 if ("optionsOrString".equals(codeString)) 249 return new Enumeration<QuestionnaireAnswerConstraint>(this, QuestionnaireAnswerConstraint.OPTIONSORSTRING, code); 250 throw new FHIRException("Unknown QuestionnaireAnswerConstraint code '"+codeString+"'"); 251 } 252 public String toCode(QuestionnaireAnswerConstraint code) { 253 if (code == QuestionnaireAnswerConstraint.NULL) 254 return null; 255 if (code == QuestionnaireAnswerConstraint.OPTIONSONLY) 256 return "optionsOnly"; 257 if (code == QuestionnaireAnswerConstraint.OPTIONSORTYPE) 258 return "optionsOrType"; 259 if (code == QuestionnaireAnswerConstraint.OPTIONSORSTRING) 260 return "optionsOrString"; 261 return "?"; 262 } 263 public String toSystem(QuestionnaireAnswerConstraint code) { 264 return code.getSystem(); 265 } 266 } 267 268 public enum QuestionnaireItemDisabledDisplay { 269 /** 270 * The item (and its children) should not be visible to the user at all. 271 */ 272 HIDDEN, 273 /** 274 * The item (and possibly its children) should not be selectable or editable but should still be visible - to allow the user to see what questions *could* have been completed had other answers caused the item to be enabled. 275 */ 276 PROTECTED, 277 /** 278 * added to help the parsers with the generic types 279 */ 280 NULL; 281 public static QuestionnaireItemDisabledDisplay fromCode(String codeString) throws FHIRException { 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("hidden".equals(codeString)) 285 return HIDDEN; 286 if ("protected".equals(codeString)) 287 return PROTECTED; 288 if (Configuration.isAcceptInvalidEnums()) 289 return null; 290 else 291 throw new FHIRException("Unknown QuestionnaireItemDisabledDisplay code '"+codeString+"'"); 292 } 293 public String toCode() { 294 switch (this) { 295 case HIDDEN: return "hidden"; 296 case PROTECTED: return "protected"; 297 case NULL: return null; 298 default: return "?"; 299 } 300 } 301 public String getSystem() { 302 switch (this) { 303 case HIDDEN: return "http://hl7.org/fhir/questionnaire-disabled-display"; 304 case PROTECTED: return "http://hl7.org/fhir/questionnaire-disabled-display"; 305 case NULL: return null; 306 default: return "?"; 307 } 308 } 309 public String getDefinition() { 310 switch (this) { 311 case HIDDEN: return "The item (and its children) should not be visible to the user at all."; 312 case PROTECTED: return "The item (and possibly its children) should not be selectable or editable but should still be visible - to allow the user to see what questions *could* have been completed had other answers caused the item to be enabled."; 313 case NULL: return null; 314 default: return "?"; 315 } 316 } 317 public String getDisplay() { 318 switch (this) { 319 case HIDDEN: return "Hidden"; 320 case PROTECTED: return "Protected"; 321 case NULL: return null; 322 default: return "?"; 323 } 324 } 325 } 326 327 public static class QuestionnaireItemDisabledDisplayEnumFactory implements EnumFactory<QuestionnaireItemDisabledDisplay> { 328 public QuestionnaireItemDisabledDisplay fromCode(String codeString) throws IllegalArgumentException { 329 if (codeString == null || "".equals(codeString)) 330 if (codeString == null || "".equals(codeString)) 331 return null; 332 if ("hidden".equals(codeString)) 333 return QuestionnaireItemDisabledDisplay.HIDDEN; 334 if ("protected".equals(codeString)) 335 return QuestionnaireItemDisabledDisplay.PROTECTED; 336 throw new IllegalArgumentException("Unknown QuestionnaireItemDisabledDisplay code '"+codeString+"'"); 337 } 338 public Enumeration<QuestionnaireItemDisabledDisplay> fromType(PrimitiveType<?> code) throws FHIRException { 339 if (code == null) 340 return null; 341 if (code.isEmpty()) 342 return new Enumeration<QuestionnaireItemDisabledDisplay>(this, QuestionnaireItemDisabledDisplay.NULL, code); 343 String codeString = ((PrimitiveType) code).asStringValue(); 344 if (codeString == null || "".equals(codeString)) 345 return new Enumeration<QuestionnaireItemDisabledDisplay>(this, QuestionnaireItemDisabledDisplay.NULL, code); 346 if ("hidden".equals(codeString)) 347 return new Enumeration<QuestionnaireItemDisabledDisplay>(this, QuestionnaireItemDisabledDisplay.HIDDEN, code); 348 if ("protected".equals(codeString)) 349 return new Enumeration<QuestionnaireItemDisabledDisplay>(this, QuestionnaireItemDisabledDisplay.PROTECTED, code); 350 throw new FHIRException("Unknown QuestionnaireItemDisabledDisplay code '"+codeString+"'"); 351 } 352 public String toCode(QuestionnaireItemDisabledDisplay code) { 353 if (code == QuestionnaireItemDisabledDisplay.NULL) 354 return null; 355 if (code == QuestionnaireItemDisabledDisplay.HIDDEN) 356 return "hidden"; 357 if (code == QuestionnaireItemDisabledDisplay.PROTECTED) 358 return "protected"; 359 return "?"; 360 } 361 public String toSystem(QuestionnaireItemDisabledDisplay code) { 362 return code.getSystem(); 363 } 364 } 365 366 public enum QuestionnaireItemOperator { 367 /** 368 * True if the determination of 'whether an answer exists for the question' is equal to the enableWhen answer (which must be a boolean). 369 */ 370 EXISTS, 371 /** 372 * True if at least one answer has a value that is equal to the enableWhen answer. 373 */ 374 EQUAL, 375 /** 376 * True if no answer has a value that is equal to the enableWhen answer. 377 */ 378 NOT_EQUAL, 379 /** 380 * True if at least one answer has a value that is greater than the enableWhen answer. 381 */ 382 GREATER_THAN, 383 /** 384 * True if at least one answer has a value that is less than the enableWhen answer. 385 */ 386 LESS_THAN, 387 /** 388 * True if at least one answer has a value that is greater or equal to the enableWhen answer. 389 */ 390 GREATER_OR_EQUAL, 391 /** 392 * True if at least one answer has a value that is less or equal to the enableWhen answer. 393 */ 394 LESS_OR_EQUAL, 395 /** 396 * added to help the parsers with the generic types 397 */ 398 NULL; 399 public static QuestionnaireItemOperator fromCode(String codeString) throws FHIRException { 400 if (codeString == null || "".equals(codeString)) 401 return null; 402 if ("exists".equals(codeString)) 403 return EXISTS; 404 if ("=".equals(codeString)) 405 return EQUAL; 406 if ("!=".equals(codeString)) 407 return NOT_EQUAL; 408 if (">".equals(codeString)) 409 return GREATER_THAN; 410 if ("<".equals(codeString)) 411 return LESS_THAN; 412 if (">=".equals(codeString)) 413 return GREATER_OR_EQUAL; 414 if ("<=".equals(codeString)) 415 return LESS_OR_EQUAL; 416 if (Configuration.isAcceptInvalidEnums()) 417 return null; 418 else 419 throw new FHIRException("Unknown QuestionnaireItemOperator code '"+codeString+"'"); 420 } 421 public String toCode() { 422 switch (this) { 423 case EXISTS: return "exists"; 424 case EQUAL: return "="; 425 case NOT_EQUAL: return "!="; 426 case GREATER_THAN: return ">"; 427 case LESS_THAN: return "<"; 428 case GREATER_OR_EQUAL: return ">="; 429 case LESS_OR_EQUAL: return "<="; 430 case NULL: return null; 431 default: return "?"; 432 } 433 } 434 public String getSystem() { 435 switch (this) { 436 case EXISTS: return "http://hl7.org/fhir/questionnaire-enable-operator"; 437 case EQUAL: return "http://hl7.org/fhir/questionnaire-enable-operator"; 438 case NOT_EQUAL: return "http://hl7.org/fhir/questionnaire-enable-operator"; 439 case GREATER_THAN: return "http://hl7.org/fhir/questionnaire-enable-operator"; 440 case LESS_THAN: return "http://hl7.org/fhir/questionnaire-enable-operator"; 441 case GREATER_OR_EQUAL: return "http://hl7.org/fhir/questionnaire-enable-operator"; 442 case LESS_OR_EQUAL: return "http://hl7.org/fhir/questionnaire-enable-operator"; 443 case NULL: return null; 444 default: return "?"; 445 } 446 } 447 public String getDefinition() { 448 switch (this) { 449 case EXISTS: return "True if the determination of 'whether an answer exists for the question' is equal to the enableWhen answer (which must be a boolean)."; 450 case EQUAL: return "True if at least one answer has a value that is equal to the enableWhen answer."; 451 case NOT_EQUAL: return "True if no answer has a value that is equal to the enableWhen answer."; 452 case GREATER_THAN: return "True if at least one answer has a value that is greater than the enableWhen answer."; 453 case LESS_THAN: return "True if at least one answer has a value that is less than the enableWhen answer."; 454 case GREATER_OR_EQUAL: return "True if at least one answer has a value that is greater or equal to the enableWhen answer."; 455 case LESS_OR_EQUAL: return "True if at least one answer has a value that is less or equal to the enableWhen answer."; 456 case NULL: return null; 457 default: return "?"; 458 } 459 } 460 public String getDisplay() { 461 switch (this) { 462 case EXISTS: return "Exists"; 463 case EQUAL: return "Equals"; 464 case NOT_EQUAL: return "Not Equals"; 465 case GREATER_THAN: return "Greater Than"; 466 case LESS_THAN: return "Less Than"; 467 case GREATER_OR_EQUAL: return "Greater or Equals"; 468 case LESS_OR_EQUAL: return "Less or Equals"; 469 case NULL: return null; 470 default: return "?"; 471 } 472 } 473 } 474 475 public static class QuestionnaireItemOperatorEnumFactory implements EnumFactory<QuestionnaireItemOperator> { 476 public QuestionnaireItemOperator fromCode(String codeString) throws IllegalArgumentException { 477 if (codeString == null || "".equals(codeString)) 478 if (codeString == null || "".equals(codeString)) 479 return null; 480 if ("exists".equals(codeString)) 481 return QuestionnaireItemOperator.EXISTS; 482 if ("=".equals(codeString)) 483 return QuestionnaireItemOperator.EQUAL; 484 if ("!=".equals(codeString)) 485 return QuestionnaireItemOperator.NOT_EQUAL; 486 if (">".equals(codeString)) 487 return QuestionnaireItemOperator.GREATER_THAN; 488 if ("<".equals(codeString)) 489 return QuestionnaireItemOperator.LESS_THAN; 490 if (">=".equals(codeString)) 491 return QuestionnaireItemOperator.GREATER_OR_EQUAL; 492 if ("<=".equals(codeString)) 493 return QuestionnaireItemOperator.LESS_OR_EQUAL; 494 throw new IllegalArgumentException("Unknown QuestionnaireItemOperator code '"+codeString+"'"); 495 } 496 public Enumeration<QuestionnaireItemOperator> fromType(PrimitiveType<?> code) throws FHIRException { 497 if (code == null) 498 return null; 499 if (code.isEmpty()) 500 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.NULL, code); 501 String codeString = ((PrimitiveType) code).asStringValue(); 502 if (codeString == null || "".equals(codeString)) 503 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.NULL, code); 504 if ("exists".equals(codeString)) 505 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.EXISTS, code); 506 if ("=".equals(codeString)) 507 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.EQUAL, code); 508 if ("!=".equals(codeString)) 509 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.NOT_EQUAL, code); 510 if (">".equals(codeString)) 511 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.GREATER_THAN, code); 512 if ("<".equals(codeString)) 513 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.LESS_THAN, code); 514 if (">=".equals(codeString)) 515 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.GREATER_OR_EQUAL, code); 516 if ("<=".equals(codeString)) 517 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.LESS_OR_EQUAL, code); 518 throw new FHIRException("Unknown QuestionnaireItemOperator code '"+codeString+"'"); 519 } 520 public String toCode(QuestionnaireItemOperator code) { 521 if (code == QuestionnaireItemOperator.NULL) 522 return null; 523 if (code == QuestionnaireItemOperator.EXISTS) 524 return "exists"; 525 if (code == QuestionnaireItemOperator.EQUAL) 526 return "="; 527 if (code == QuestionnaireItemOperator.NOT_EQUAL) 528 return "!="; 529 if (code == QuestionnaireItemOperator.GREATER_THAN) 530 return ">"; 531 if (code == QuestionnaireItemOperator.LESS_THAN) 532 return "<"; 533 if (code == QuestionnaireItemOperator.GREATER_OR_EQUAL) 534 return ">="; 535 if (code == QuestionnaireItemOperator.LESS_OR_EQUAL) 536 return "<="; 537 return "?"; 538 } 539 public String toSystem(QuestionnaireItemOperator code) { 540 return code.getSystem(); 541 } 542 } 543 544 public enum QuestionnaireItemType { 545 /** 546 * An item with no direct answer but should have at least one child item. 547 */ 548 GROUP, 549 /** 550 * Text for display that will not capture an answer or have child items. 551 */ 552 DISPLAY, 553 /** 554 * An item that defines a specific answer to be captured, and which may have child items. (the answer provided in the QuestionnaireResponse should be of the defined datatype). 555 */ 556 QUESTION, 557 /** 558 * Question with a yes/no answer (valueBoolean). 559 */ 560 BOOLEAN, 561 /** 562 * Question with is a real number answer (valueDecimal). There is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to computably convey the unit of measure associated with the answer for use when performing data extraction to an element of type Quantity. 563 */ 564 DECIMAL, 565 /** 566 * Question with an integer answer (valueInteger). There is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to computably convey the unit of measure associated with the answer for use when performing data extraction to an element of type Quantity. 567 */ 568 INTEGER, 569 /** 570 * Question with a date answer (valueDate). 571 */ 572 DATE, 573 /** 574 * Question with a date and time answer (valueDateTime). 575 */ 576 DATETIME, 577 /** 578 * Question with a time (hour:minute:second) answer independent of date. (valueTime). 579 */ 580 TIME, 581 /** 582 * Question with a short (few words to short sentence) free-text entry answer (valueString). Strings SHOULD NOT contain carriage return or newline characters. If multi-line answers are needed, use the 'text' type. 583 */ 584 STRING, 585 /** 586 * Question with a long (potentially multi-paragraph) free-text entry answer (valueString). 587 */ 588 TEXT, 589 /** 590 * Question with a URL (website, FTP site, etc.) answer (valueUri). 591 */ 592 URL, 593 /** 594 * Question with a Coding - generally drawn from a list of possible answers (valueCoding) 595 */ 596 CODING, 597 /** 598 * Question with binary content such as an image, PDF, etc. as an answer (valueAttachment). 599 */ 600 ATTACHMENT, 601 /** 602 * Question with a reference to another resource (practitioner, organization, etc.) as an answer (valueReference). 603 */ 604 REFERENCE, 605 /** 606 * Question with a combination of a numeric value and unit as an answer. (valueSimpleQuantity) There are two extensions ('http://hl7.org/fhir/StructureDefinition/questionnaire-unitOption' and 'http://hl7.org/fhir/StructureDefinition/questionnaire-unitValueSet') that can be used to define what unit should be selected for the Quantity.code and Quantity.system. 607 */ 608 QUANTITY, 609 /** 610 * added to help the parsers with the generic types 611 */ 612 NULL; 613 public static QuestionnaireItemType fromCode(String codeString) throws FHIRException { 614 if (codeString == null || "".equals(codeString)) 615 return null; 616 if ("group".equals(codeString)) 617 return GROUP; 618 if ("display".equals(codeString)) 619 return DISPLAY; 620 if ("question".equals(codeString)) 621 return QUESTION; 622 if ("boolean".equals(codeString)) 623 return BOOLEAN; 624 if ("decimal".equals(codeString)) 625 return DECIMAL; 626 if ("integer".equals(codeString)) 627 return INTEGER; 628 if ("date".equals(codeString)) 629 return DATE; 630 if ("dateTime".equals(codeString)) 631 return DATETIME; 632 if ("time".equals(codeString)) 633 return TIME; 634 if ("string".equals(codeString)) 635 return STRING; 636 if ("text".equals(codeString)) 637 return TEXT; 638 if ("url".equals(codeString)) 639 return URL; 640 if ("coding".equals(codeString)) 641 return CODING; 642 if ("attachment".equals(codeString)) 643 return ATTACHMENT; 644 if ("reference".equals(codeString)) 645 return REFERENCE; 646 if ("quantity".equals(codeString)) 647 return QUANTITY; 648 if (Configuration.isAcceptInvalidEnums()) 649 return null; 650 else 651 throw new FHIRException("Unknown QuestionnaireItemType code '"+codeString+"'"); 652 } 653 public String toCode() { 654 switch (this) { 655 case GROUP: return "group"; 656 case DISPLAY: return "display"; 657 case QUESTION: return "question"; 658 case BOOLEAN: return "boolean"; 659 case DECIMAL: return "decimal"; 660 case INTEGER: return "integer"; 661 case DATE: return "date"; 662 case DATETIME: return "dateTime"; 663 case TIME: return "time"; 664 case STRING: return "string"; 665 case TEXT: return "text"; 666 case URL: return "url"; 667 case CODING: return "coding"; 668 case ATTACHMENT: return "attachment"; 669 case REFERENCE: return "reference"; 670 case QUANTITY: return "quantity"; 671 case NULL: return null; 672 default: return "?"; 673 } 674 } 675 public String getSystem() { 676 switch (this) { 677 case GROUP: return "http://hl7.org/fhir/item-type"; 678 case DISPLAY: return "http://hl7.org/fhir/item-type"; 679 case QUESTION: return "http://hl7.org/fhir/item-type"; 680 case BOOLEAN: return "http://hl7.org/fhir/item-type"; 681 case DECIMAL: return "http://hl7.org/fhir/item-type"; 682 case INTEGER: return "http://hl7.org/fhir/item-type"; 683 case DATE: return "http://hl7.org/fhir/item-type"; 684 case DATETIME: return "http://hl7.org/fhir/item-type"; 685 case TIME: return "http://hl7.org/fhir/item-type"; 686 case STRING: return "http://hl7.org/fhir/item-type"; 687 case TEXT: return "http://hl7.org/fhir/item-type"; 688 case URL: return "http://hl7.org/fhir/item-type"; 689 case CODING: return "http://hl7.org/fhir/item-type"; 690 case ATTACHMENT: return "http://hl7.org/fhir/item-type"; 691 case REFERENCE: return "http://hl7.org/fhir/item-type"; 692 case QUANTITY: return "http://hl7.org/fhir/item-type"; 693 case NULL: return null; 694 default: return "?"; 695 } 696 } 697 public String getDefinition() { 698 switch (this) { 699 case GROUP: return "An item with no direct answer but should have at least one child item."; 700 case DISPLAY: return "Text for display that will not capture an answer or have child items."; 701 case QUESTION: return "An item that defines a specific answer to be captured, and which may have child items. (the answer provided in the QuestionnaireResponse should be of the defined datatype)."; 702 case BOOLEAN: return "Question with a yes/no answer (valueBoolean)."; 703 case DECIMAL: return "Question with is a real number answer (valueDecimal). There is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to computably convey the unit of measure associated with the answer for use when performing data extraction to an element of type Quantity."; 704 case INTEGER: return "Question with an integer answer (valueInteger). There is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to computably convey the unit of measure associated with the answer for use when performing data extraction to an element of type Quantity."; 705 case DATE: return "Question with a date answer (valueDate)."; 706 case DATETIME: return "Question with a date and time answer (valueDateTime)."; 707 case TIME: return "Question with a time (hour:minute:second) answer independent of date. (valueTime)."; 708 case STRING: return "Question with a short (few words to short sentence) free-text entry answer (valueString). Strings SHOULD NOT contain carriage return or newline characters. If multi-line answers are needed, use the 'text' type."; 709 case TEXT: return "Question with a long (potentially multi-paragraph) free-text entry answer (valueString)."; 710 case URL: return "Question with a URL (website, FTP site, etc.) answer (valueUri)."; 711 case CODING: return "Question with a Coding - generally drawn from a list of possible answers (valueCoding)"; 712 case ATTACHMENT: return "Question with binary content such as an image, PDF, etc. as an answer (valueAttachment)."; 713 case REFERENCE: return "Question with a reference to another resource (practitioner, organization, etc.) as an answer (valueReference)."; 714 case QUANTITY: return "Question with a combination of a numeric value and unit as an answer. (valueSimpleQuantity) There are two extensions ('http://hl7.org/fhir/StructureDefinition/questionnaire-unitOption' and 'http://hl7.org/fhir/StructureDefinition/questionnaire-unitValueSet') that can be used to define what unit should be selected for the Quantity.code and Quantity.system."; 715 case NULL: return null; 716 default: return "?"; 717 } 718 } 719 public String getDisplay() { 720 switch (this) { 721 case GROUP: return "Group"; 722 case DISPLAY: return "Display"; 723 case QUESTION: return "Question"; 724 case BOOLEAN: return "Boolean"; 725 case DECIMAL: return "Decimal"; 726 case INTEGER: return "Integer"; 727 case DATE: return "Date"; 728 case DATETIME: return "Date Time"; 729 case TIME: return "Time"; 730 case STRING: return "String"; 731 case TEXT: return "Text"; 732 case URL: return "Url"; 733 case CODING: return "Coding"; 734 case ATTACHMENT: return "Attachment"; 735 case REFERENCE: return "Reference"; 736 case QUANTITY: return "Quantity"; 737 case NULL: return null; 738 default: return "?"; 739 } 740 } 741 } 742 743 public static class QuestionnaireItemTypeEnumFactory implements EnumFactory<QuestionnaireItemType> { 744 public QuestionnaireItemType fromCode(String codeString) throws IllegalArgumentException { 745 if (codeString == null || "".equals(codeString)) 746 if (codeString == null || "".equals(codeString)) 747 return null; 748 if ("group".equals(codeString)) 749 return QuestionnaireItemType.GROUP; 750 if ("display".equals(codeString)) 751 return QuestionnaireItemType.DISPLAY; 752 if ("question".equals(codeString)) 753 return QuestionnaireItemType.QUESTION; 754 if ("boolean".equals(codeString)) 755 return QuestionnaireItemType.BOOLEAN; 756 if ("decimal".equals(codeString)) 757 return QuestionnaireItemType.DECIMAL; 758 if ("integer".equals(codeString)) 759 return QuestionnaireItemType.INTEGER; 760 if ("date".equals(codeString)) 761 return QuestionnaireItemType.DATE; 762 if ("dateTime".equals(codeString)) 763 return QuestionnaireItemType.DATETIME; 764 if ("time".equals(codeString)) 765 return QuestionnaireItemType.TIME; 766 if ("string".equals(codeString)) 767 return QuestionnaireItemType.STRING; 768 if ("text".equals(codeString)) 769 return QuestionnaireItemType.TEXT; 770 if ("url".equals(codeString)) 771 return QuestionnaireItemType.URL; 772 if ("coding".equals(codeString)) 773 return QuestionnaireItemType.CODING; 774 if ("attachment".equals(codeString)) 775 return QuestionnaireItemType.ATTACHMENT; 776 if ("reference".equals(codeString)) 777 return QuestionnaireItemType.REFERENCE; 778 if ("quantity".equals(codeString)) 779 return QuestionnaireItemType.QUANTITY; 780 throw new IllegalArgumentException("Unknown QuestionnaireItemType code '"+codeString+"'"); 781 } 782 public Enumeration<QuestionnaireItemType> fromType(PrimitiveType<?> code) throws FHIRException { 783 if (code == null) 784 return null; 785 if (code.isEmpty()) 786 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.NULL, code); 787 String codeString = ((PrimitiveType) code).asStringValue(); 788 if (codeString == null || "".equals(codeString)) 789 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.NULL, code); 790 if ("group".equals(codeString)) 791 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.GROUP, code); 792 if ("display".equals(codeString)) 793 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DISPLAY, code); 794 if ("question".equals(codeString)) 795 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.QUESTION, code); 796 if ("boolean".equals(codeString)) 797 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.BOOLEAN, code); 798 if ("decimal".equals(codeString)) 799 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DECIMAL, code); 800 if ("integer".equals(codeString)) 801 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.INTEGER, code); 802 if ("date".equals(codeString)) 803 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DATE, code); 804 if ("dateTime".equals(codeString)) 805 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DATETIME, code); 806 if ("time".equals(codeString)) 807 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.TIME, code); 808 if ("string".equals(codeString)) 809 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.STRING, code); 810 if ("text".equals(codeString)) 811 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.TEXT, code); 812 if ("url".equals(codeString)) 813 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.URL, code); 814 if ("coding".equals(codeString)) 815 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.CODING, code); 816 if ("attachment".equals(codeString)) 817 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.ATTACHMENT, code); 818 if ("reference".equals(codeString)) 819 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.REFERENCE, code); 820 if ("quantity".equals(codeString)) 821 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.QUANTITY, code); 822 throw new FHIRException("Unknown QuestionnaireItemType code '"+codeString+"'"); 823 } 824 public String toCode(QuestionnaireItemType code) { 825 if (code == QuestionnaireItemType.NULL) 826 return null; 827 if (code == QuestionnaireItemType.GROUP) 828 return "group"; 829 if (code == QuestionnaireItemType.DISPLAY) 830 return "display"; 831 if (code == QuestionnaireItemType.QUESTION) 832 return "question"; 833 if (code == QuestionnaireItemType.BOOLEAN) 834 return "boolean"; 835 if (code == QuestionnaireItemType.DECIMAL) 836 return "decimal"; 837 if (code == QuestionnaireItemType.INTEGER) 838 return "integer"; 839 if (code == QuestionnaireItemType.DATE) 840 return "date"; 841 if (code == QuestionnaireItemType.DATETIME) 842 return "dateTime"; 843 if (code == QuestionnaireItemType.TIME) 844 return "time"; 845 if (code == QuestionnaireItemType.STRING) 846 return "string"; 847 if (code == QuestionnaireItemType.TEXT) 848 return "text"; 849 if (code == QuestionnaireItemType.URL) 850 return "url"; 851 if (code == QuestionnaireItemType.CODING) 852 return "coding"; 853 if (code == QuestionnaireItemType.ATTACHMENT) 854 return "attachment"; 855 if (code == QuestionnaireItemType.REFERENCE) 856 return "reference"; 857 if (code == QuestionnaireItemType.QUANTITY) 858 return "quantity"; 859 return "?"; 860 } 861 public String toSystem(QuestionnaireItemType code) { 862 return code.getSystem(); 863 } 864 } 865 866 @Block() 867 public static class QuestionnaireItemComponent extends BackboneElement implements IBaseBackboneElement { 868 /** 869 * An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource. 870 */ 871 @Child(name = "linkId", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 872 @Description(shortDefinition="Unique id for item in questionnaire", formalDefinition="An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource." ) 873 protected StringType linkId; 874 875 /** 876 * This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below. 877 */ 878 @Child(name = "definition", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 879 @Description(shortDefinition="ElementDefinition - details for the item", formalDefinition="This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below." ) 880 protected UriType definition; 881 882 /** 883 * A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers). 884 */ 885 @Child(name = "code", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 886 @Description(shortDefinition="Corresponding concept for this item in a terminology", formalDefinition="A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers)." ) 887 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-questions") 888 protected List<Coding> code; 889 890 /** 891 * A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire. 892 */ 893 @Child(name = "prefix", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 894 @Description(shortDefinition="E.g. \"1(a)\", \"2.5.3\"", formalDefinition="A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire." ) 895 protected StringType prefix; 896 897 /** 898 * The name of a section, the text of a question or text content for a display item. 899 */ 900 @Child(name = "text", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 901 @Description(shortDefinition="Primary text for the item", formalDefinition="The name of a section, the text of a question or text content for a display item." ) 902 protected StringType text; 903 904 /** 905 * The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.). 906 */ 907 @Child(name = "type", type = {CodeType.class}, order=6, min=1, max=1, modifier=false, summary=false) 908 @Description(shortDefinition="group | display | boolean | decimal | integer | date | dateTime +", formalDefinition="The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.)." ) 909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/item-type") 910 protected Enumeration<QuestionnaireItemType> type; 911 912 /** 913 * A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true. 914 */ 915 @Child(name = "enableWhen", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=false) 916 @Description(shortDefinition="Only allow data when", formalDefinition="A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true." ) 917 protected List<QuestionnaireItemEnableWhenComponent> enableWhen; 918 919 /** 920 * Controls how multiple enableWhen values are interpreted - whether all or any must be true. 921 */ 922 @Child(name = "enableBehavior", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=false) 923 @Description(shortDefinition="all | any", formalDefinition="Controls how multiple enableWhen values are interpreted - whether all or any must be true." ) 924 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-enable-behavior") 925 protected Enumeration<EnableWhenBehavior> enableBehavior; 926 927 /** 928 * Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed. 929 */ 930 @Child(name = "disabledDisplay", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 931 @Description(shortDefinition="hidden | protected", formalDefinition="Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed." ) 932 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-disabled-display") 933 protected Enumeration<QuestionnaireItemDisabledDisplay> disabledDisplay; 934 935 /** 936 * An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire. 937 */ 938 @Child(name = "required", type = {BooleanType.class}, order=10, min=0, max=1, modifier=false, summary=false) 939 @Description(shortDefinition="Whether the item must be included in data results", formalDefinition="An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire." ) 940 protected BooleanType required; 941 942 /** 943 * An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items). 944 */ 945 @Child(name = "repeats", type = {BooleanType.class}, order=11, min=0, max=1, modifier=false, summary=false) 946 @Description(shortDefinition="Whether the item may repeat", formalDefinition="An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items)." ) 947 protected BooleanType repeats; 948 949 /** 950 * An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire. 951 */ 952 @Child(name = "readOnly", type = {BooleanType.class}, order=12, min=0, max=1, modifier=false, summary=false) 953 @Description(shortDefinition="Don't allow human editing", formalDefinition="An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire." ) 954 protected BooleanType readOnly; 955 956 /** 957 * The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse. 958 */ 959 @Child(name = "maxLength", type = {IntegerType.class}, order=13, min=0, max=1, modifier=false, summary=false) 960 @Description(shortDefinition="No more than these many characters", formalDefinition="The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse." ) 961 protected IntegerType maxLength; 962 963 /** 964 * For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected. 965 */ 966 @Child(name = "answerConstraint", type = {CodeType.class}, order=14, min=0, max=1, modifier=false, summary=false) 967 @Description(shortDefinition="optionsOnly | optionsOrType | optionsOrString", formalDefinition="For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected." ) 968 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answer-constraint") 969 protected Enumeration<QuestionnaireAnswerConstraint> answerConstraint; 970 971 /** 972 * A reference to a value set containing a list of values representing permitted answers for a question. 973 */ 974 @Child(name = "answerValueSet", type = {CanonicalType.class}, order=15, min=0, max=1, modifier=false, summary=false) 975 @Description(shortDefinition="ValueSet containing permitted answers", formalDefinition="A reference to a value set containing a list of values representing permitted answers for a question." ) 976 protected CanonicalType answerValueSet; 977 978 /** 979 * One of the permitted answers for the question. 980 */ 981 @Child(name = "answerOption", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 982 @Description(shortDefinition="Permitted answer", formalDefinition="One of the permitted answers for the question." ) 983 protected List<QuestionnaireItemAnswerOptionComponent> answerOption; 984 985 /** 986 * One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input. 987 */ 988 @Child(name = "initial", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 989 @Description(shortDefinition="Initial value(s) when item is first rendered", formalDefinition="One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input." ) 990 protected List<QuestionnaireItemInitialComponent> initial; 991 992 /** 993 * Text, questions and other groups to be nested beneath a question or group. 994 */ 995 @Child(name = "item", type = {QuestionnaireItemComponent.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 996 @Description(shortDefinition="Nested questionnaire items", formalDefinition="Text, questions and other groups to be nested beneath a question or group." ) 997 protected List<QuestionnaireItemComponent> item; 998 999 private static final long serialVersionUID = -1760914161L; 1000 1001 /** 1002 * Constructor 1003 */ 1004 public QuestionnaireItemComponent() { 1005 super(); 1006 } 1007 1008 /** 1009 * Constructor 1010 */ 1011 public QuestionnaireItemComponent(String linkId, QuestionnaireItemType type) { 1012 super(); 1013 this.setLinkId(linkId); 1014 this.setType(type); 1015 } 1016 1017 /** 1018 * @return {@link #linkId} (An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1019 */ 1020 public StringType getLinkIdElement() { 1021 if (this.linkId == null) 1022 if (Configuration.errorOnAutoCreate()) 1023 throw new Error("Attempt to auto-create QuestionnaireItemComponent.linkId"); 1024 else if (Configuration.doAutoCreate()) 1025 this.linkId = new StringType(); // bb 1026 return this.linkId; 1027 } 1028 1029 public boolean hasLinkIdElement() { 1030 return this.linkId != null && !this.linkId.isEmpty(); 1031 } 1032 1033 public boolean hasLinkId() { 1034 return this.linkId != null && !this.linkId.isEmpty(); 1035 } 1036 1037 /** 1038 * @param value {@link #linkId} (An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1039 */ 1040 public QuestionnaireItemComponent setLinkIdElement(StringType value) { 1041 this.linkId = value; 1042 return this; 1043 } 1044 1045 /** 1046 * @return An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource. 1047 */ 1048 public String getLinkId() { 1049 return this.linkId == null ? null : this.linkId.getValue(); 1050 } 1051 1052 /** 1053 * @param value An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource. 1054 */ 1055 public QuestionnaireItemComponent setLinkId(String value) { 1056 if (this.linkId == null) 1057 this.linkId = new StringType(); 1058 this.linkId.setValue(value); 1059 return this; 1060 } 1061 1062 /** 1063 * @return {@link #definition} (This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1064 */ 1065 public UriType getDefinitionElement() { 1066 if (this.definition == null) 1067 if (Configuration.errorOnAutoCreate()) 1068 throw new Error("Attempt to auto-create QuestionnaireItemComponent.definition"); 1069 else if (Configuration.doAutoCreate()) 1070 this.definition = new UriType(); // bb 1071 return this.definition; 1072 } 1073 1074 public boolean hasDefinitionElement() { 1075 return this.definition != null && !this.definition.isEmpty(); 1076 } 1077 1078 public boolean hasDefinition() { 1079 return this.definition != null && !this.definition.isEmpty(); 1080 } 1081 1082 /** 1083 * @param value {@link #definition} (This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1084 */ 1085 public QuestionnaireItemComponent setDefinitionElement(UriType value) { 1086 this.definition = value; 1087 return this; 1088 } 1089 1090 /** 1091 * @return This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below. 1092 */ 1093 public String getDefinition() { 1094 return this.definition == null ? null : this.definition.getValue(); 1095 } 1096 1097 /** 1098 * @param value This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below. 1099 */ 1100 public QuestionnaireItemComponent setDefinition(String value) { 1101 if (Utilities.noString(value)) 1102 this.definition = null; 1103 else { 1104 if (this.definition == null) 1105 this.definition = new UriType(); 1106 this.definition.setValue(value); 1107 } 1108 return this; 1109 } 1110 1111 /** 1112 * @return {@link #code} (A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).) 1113 */ 1114 public List<Coding> getCode() { 1115 if (this.code == null) 1116 this.code = new ArrayList<Coding>(); 1117 return this.code; 1118 } 1119 1120 /** 1121 * @return Returns a reference to <code>this</code> for easy method chaining 1122 */ 1123 public QuestionnaireItemComponent setCode(List<Coding> theCode) { 1124 this.code = theCode; 1125 return this; 1126 } 1127 1128 public boolean hasCode() { 1129 if (this.code == null) 1130 return false; 1131 for (Coding item : this.code) 1132 if (!item.isEmpty()) 1133 return true; 1134 return false; 1135 } 1136 1137 public Coding addCode() { //3 1138 Coding t = new Coding(); 1139 if (this.code == null) 1140 this.code = new ArrayList<Coding>(); 1141 this.code.add(t); 1142 return t; 1143 } 1144 1145 public QuestionnaireItemComponent addCode(Coding t) { //3 1146 if (t == null) 1147 return this; 1148 if (this.code == null) 1149 this.code = new ArrayList<Coding>(); 1150 this.code.add(t); 1151 return this; 1152 } 1153 1154 /** 1155 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 1156 */ 1157 public Coding getCodeFirstRep() { 1158 if (getCode().isEmpty()) { 1159 addCode(); 1160 } 1161 return getCode().get(0); 1162 } 1163 1164 /** 1165 * @return {@link #prefix} (A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 1166 */ 1167 public StringType getPrefixElement() { 1168 if (this.prefix == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create QuestionnaireItemComponent.prefix"); 1171 else if (Configuration.doAutoCreate()) 1172 this.prefix = new StringType(); // bb 1173 return this.prefix; 1174 } 1175 1176 public boolean hasPrefixElement() { 1177 return this.prefix != null && !this.prefix.isEmpty(); 1178 } 1179 1180 public boolean hasPrefix() { 1181 return this.prefix != null && !this.prefix.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #prefix} (A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 1186 */ 1187 public QuestionnaireItemComponent setPrefixElement(StringType value) { 1188 this.prefix = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire. 1194 */ 1195 public String getPrefix() { 1196 return this.prefix == null ? null : this.prefix.getValue(); 1197 } 1198 1199 /** 1200 * @param value A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire. 1201 */ 1202 public QuestionnaireItemComponent setPrefix(String value) { 1203 if (Utilities.noString(value)) 1204 this.prefix = null; 1205 else { 1206 if (this.prefix == null) 1207 this.prefix = new StringType(); 1208 this.prefix.setValue(value); 1209 } 1210 return this; 1211 } 1212 1213 /** 1214 * @return {@link #text} (The name of a section, the text of a question or text content for a display item.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 1215 */ 1216 public StringType getTextElement() { 1217 if (this.text == null) 1218 if (Configuration.errorOnAutoCreate()) 1219 throw new Error("Attempt to auto-create QuestionnaireItemComponent.text"); 1220 else if (Configuration.doAutoCreate()) 1221 this.text = new StringType(); // bb 1222 return this.text; 1223 } 1224 1225 public boolean hasTextElement() { 1226 return this.text != null && !this.text.isEmpty(); 1227 } 1228 1229 public boolean hasText() { 1230 return this.text != null && !this.text.isEmpty(); 1231 } 1232 1233 /** 1234 * @param value {@link #text} (The name of a section, the text of a question or text content for a display item.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 1235 */ 1236 public QuestionnaireItemComponent setTextElement(StringType value) { 1237 this.text = value; 1238 return this; 1239 } 1240 1241 /** 1242 * @return The name of a section, the text of a question or text content for a display item. 1243 */ 1244 public String getText() { 1245 return this.text == null ? null : this.text.getValue(); 1246 } 1247 1248 /** 1249 * @param value The name of a section, the text of a question or text content for a display item. 1250 */ 1251 public QuestionnaireItemComponent setText(String value) { 1252 if (Utilities.noString(value)) 1253 this.text = null; 1254 else { 1255 if (this.text == null) 1256 this.text = new StringType(); 1257 this.text.setValue(value); 1258 } 1259 return this; 1260 } 1261 1262 /** 1263 * @return {@link #type} (The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1264 */ 1265 public Enumeration<QuestionnaireItemType> getTypeElement() { 1266 if (this.type == null) 1267 if (Configuration.errorOnAutoCreate()) 1268 throw new Error("Attempt to auto-create QuestionnaireItemComponent.type"); 1269 else if (Configuration.doAutoCreate()) 1270 this.type = new Enumeration<QuestionnaireItemType>(new QuestionnaireItemTypeEnumFactory()); // bb 1271 return this.type; 1272 } 1273 1274 public boolean hasTypeElement() { 1275 return this.type != null && !this.type.isEmpty(); 1276 } 1277 1278 public boolean hasType() { 1279 return this.type != null && !this.type.isEmpty(); 1280 } 1281 1282 /** 1283 * @param value {@link #type} (The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1284 */ 1285 public QuestionnaireItemComponent setTypeElement(Enumeration<QuestionnaireItemType> value) { 1286 this.type = value; 1287 return this; 1288 } 1289 1290 /** 1291 * @return The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.). 1292 */ 1293 public QuestionnaireItemType getType() { 1294 return this.type == null ? null : this.type.getValue(); 1295 } 1296 1297 /** 1298 * @param value The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.). 1299 */ 1300 public QuestionnaireItemComponent setType(QuestionnaireItemType value) { 1301 if (this.type == null) 1302 this.type = new Enumeration<QuestionnaireItemType>(new QuestionnaireItemTypeEnumFactory()); 1303 this.type.setValue(value); 1304 return this; 1305 } 1306 1307 /** 1308 * @return {@link #enableWhen} (A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.) 1309 */ 1310 public List<QuestionnaireItemEnableWhenComponent> getEnableWhen() { 1311 if (this.enableWhen == null) 1312 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 1313 return this.enableWhen; 1314 } 1315 1316 /** 1317 * @return Returns a reference to <code>this</code> for easy method chaining 1318 */ 1319 public QuestionnaireItemComponent setEnableWhen(List<QuestionnaireItemEnableWhenComponent> theEnableWhen) { 1320 this.enableWhen = theEnableWhen; 1321 return this; 1322 } 1323 1324 public boolean hasEnableWhen() { 1325 if (this.enableWhen == null) 1326 return false; 1327 for (QuestionnaireItemEnableWhenComponent item : this.enableWhen) 1328 if (!item.isEmpty()) 1329 return true; 1330 return false; 1331 } 1332 1333 public QuestionnaireItemEnableWhenComponent addEnableWhen() { //3 1334 QuestionnaireItemEnableWhenComponent t = new QuestionnaireItemEnableWhenComponent(); 1335 if (this.enableWhen == null) 1336 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 1337 this.enableWhen.add(t); 1338 return t; 1339 } 1340 1341 public QuestionnaireItemComponent addEnableWhen(QuestionnaireItemEnableWhenComponent t) { //3 1342 if (t == null) 1343 return this; 1344 if (this.enableWhen == null) 1345 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 1346 this.enableWhen.add(t); 1347 return this; 1348 } 1349 1350 /** 1351 * @return The first repetition of repeating field {@link #enableWhen}, creating it if it does not already exist {3} 1352 */ 1353 public QuestionnaireItemEnableWhenComponent getEnableWhenFirstRep() { 1354 if (getEnableWhen().isEmpty()) { 1355 addEnableWhen(); 1356 } 1357 return getEnableWhen().get(0); 1358 } 1359 1360 /** 1361 * @return {@link #enableBehavior} (Controls how multiple enableWhen values are interpreted - whether all or any must be true.). This is the underlying object with id, value and extensions. The accessor "getEnableBehavior" gives direct access to the value 1362 */ 1363 public Enumeration<EnableWhenBehavior> getEnableBehaviorElement() { 1364 if (this.enableBehavior == null) 1365 if (Configuration.errorOnAutoCreate()) 1366 throw new Error("Attempt to auto-create QuestionnaireItemComponent.enableBehavior"); 1367 else if (Configuration.doAutoCreate()) 1368 this.enableBehavior = new Enumeration<EnableWhenBehavior>(new EnableWhenBehaviorEnumFactory()); // bb 1369 return this.enableBehavior; 1370 } 1371 1372 public boolean hasEnableBehaviorElement() { 1373 return this.enableBehavior != null && !this.enableBehavior.isEmpty(); 1374 } 1375 1376 public boolean hasEnableBehavior() { 1377 return this.enableBehavior != null && !this.enableBehavior.isEmpty(); 1378 } 1379 1380 /** 1381 * @param value {@link #enableBehavior} (Controls how multiple enableWhen values are interpreted - whether all or any must be true.). This is the underlying object with id, value and extensions. The accessor "getEnableBehavior" gives direct access to the value 1382 */ 1383 public QuestionnaireItemComponent setEnableBehaviorElement(Enumeration<EnableWhenBehavior> value) { 1384 this.enableBehavior = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return Controls how multiple enableWhen values are interpreted - whether all or any must be true. 1390 */ 1391 public EnableWhenBehavior getEnableBehavior() { 1392 return this.enableBehavior == null ? null : this.enableBehavior.getValue(); 1393 } 1394 1395 /** 1396 * @param value Controls how multiple enableWhen values are interpreted - whether all or any must be true. 1397 */ 1398 public QuestionnaireItemComponent setEnableBehavior(EnableWhenBehavior value) { 1399 if (value == null) 1400 this.enableBehavior = null; 1401 else { 1402 if (this.enableBehavior == null) 1403 this.enableBehavior = new Enumeration<EnableWhenBehavior>(new EnableWhenBehaviorEnumFactory()); 1404 this.enableBehavior.setValue(value); 1405 } 1406 return this; 1407 } 1408 1409 /** 1410 * @return {@link #disabledDisplay} (Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed.). This is the underlying object with id, value and extensions. The accessor "getDisabledDisplay" gives direct access to the value 1411 */ 1412 public Enumeration<QuestionnaireItemDisabledDisplay> getDisabledDisplayElement() { 1413 if (this.disabledDisplay == null) 1414 if (Configuration.errorOnAutoCreate()) 1415 throw new Error("Attempt to auto-create QuestionnaireItemComponent.disabledDisplay"); 1416 else if (Configuration.doAutoCreate()) 1417 this.disabledDisplay = new Enumeration<QuestionnaireItemDisabledDisplay>(new QuestionnaireItemDisabledDisplayEnumFactory()); // bb 1418 return this.disabledDisplay; 1419 } 1420 1421 public boolean hasDisabledDisplayElement() { 1422 return this.disabledDisplay != null && !this.disabledDisplay.isEmpty(); 1423 } 1424 1425 public boolean hasDisabledDisplay() { 1426 return this.disabledDisplay != null && !this.disabledDisplay.isEmpty(); 1427 } 1428 1429 /** 1430 * @param value {@link #disabledDisplay} (Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed.). This is the underlying object with id, value and extensions. The accessor "getDisabledDisplay" gives direct access to the value 1431 */ 1432 public QuestionnaireItemComponent setDisabledDisplayElement(Enumeration<QuestionnaireItemDisabledDisplay> value) { 1433 this.disabledDisplay = value; 1434 return this; 1435 } 1436 1437 /** 1438 * @return Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed. 1439 */ 1440 public QuestionnaireItemDisabledDisplay getDisabledDisplay() { 1441 return this.disabledDisplay == null ? null : this.disabledDisplay.getValue(); 1442 } 1443 1444 /** 1445 * @param value Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed. 1446 */ 1447 public QuestionnaireItemComponent setDisabledDisplay(QuestionnaireItemDisabledDisplay value) { 1448 if (value == null) 1449 this.disabledDisplay = null; 1450 else { 1451 if (this.disabledDisplay == null) 1452 this.disabledDisplay = new Enumeration<QuestionnaireItemDisabledDisplay>(new QuestionnaireItemDisabledDisplayEnumFactory()); 1453 this.disabledDisplay.setValue(value); 1454 } 1455 return this; 1456 } 1457 1458 /** 1459 * @return {@link #required} (An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 1460 */ 1461 public BooleanType getRequiredElement() { 1462 if (this.required == null) 1463 if (Configuration.errorOnAutoCreate()) 1464 throw new Error("Attempt to auto-create QuestionnaireItemComponent.required"); 1465 else if (Configuration.doAutoCreate()) 1466 this.required = new BooleanType(); // bb 1467 return this.required; 1468 } 1469 1470 public boolean hasRequiredElement() { 1471 return this.required != null && !this.required.isEmpty(); 1472 } 1473 1474 public boolean hasRequired() { 1475 return this.required != null && !this.required.isEmpty(); 1476 } 1477 1478 /** 1479 * @param value {@link #required} (An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 1480 */ 1481 public QuestionnaireItemComponent setRequiredElement(BooleanType value) { 1482 this.required = value; 1483 return this; 1484 } 1485 1486 /** 1487 * @return An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire. 1488 */ 1489 public boolean getRequired() { 1490 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 1491 } 1492 1493 /** 1494 * @param value An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire. 1495 */ 1496 public QuestionnaireItemComponent setRequired(boolean value) { 1497 if (this.required == null) 1498 this.required = new BooleanType(); 1499 this.required.setValue(value); 1500 return this; 1501 } 1502 1503 /** 1504 * @return {@link #repeats} (An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items).). This is the underlying object with id, value and extensions. The accessor "getRepeats" gives direct access to the value 1505 */ 1506 public BooleanType getRepeatsElement() { 1507 if (this.repeats == null) 1508 if (Configuration.errorOnAutoCreate()) 1509 throw new Error("Attempt to auto-create QuestionnaireItemComponent.repeats"); 1510 else if (Configuration.doAutoCreate()) 1511 this.repeats = new BooleanType(); // bb 1512 return this.repeats; 1513 } 1514 1515 public boolean hasRepeatsElement() { 1516 return this.repeats != null && !this.repeats.isEmpty(); 1517 } 1518 1519 public boolean hasRepeats() { 1520 return this.repeats != null && !this.repeats.isEmpty(); 1521 } 1522 1523 /** 1524 * @param value {@link #repeats} (An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items).). This is the underlying object with id, value and extensions. The accessor "getRepeats" gives direct access to the value 1525 */ 1526 public QuestionnaireItemComponent setRepeatsElement(BooleanType value) { 1527 this.repeats = value; 1528 return this; 1529 } 1530 1531 /** 1532 * @return An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items). 1533 */ 1534 public boolean getRepeats() { 1535 return this.repeats == null || this.repeats.isEmpty() ? false : this.repeats.getValue(); 1536 } 1537 1538 /** 1539 * @param value An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items). 1540 */ 1541 public QuestionnaireItemComponent setRepeats(boolean value) { 1542 if (this.repeats == null) 1543 this.repeats = new BooleanType(); 1544 this.repeats.setValue(value); 1545 return this; 1546 } 1547 1548 /** 1549 * @return {@link #readOnly} (An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.). This is the underlying object with id, value and extensions. The accessor "getReadOnly" gives direct access to the value 1550 */ 1551 public BooleanType getReadOnlyElement() { 1552 if (this.readOnly == null) 1553 if (Configuration.errorOnAutoCreate()) 1554 throw new Error("Attempt to auto-create QuestionnaireItemComponent.readOnly"); 1555 else if (Configuration.doAutoCreate()) 1556 this.readOnly = new BooleanType(); // bb 1557 return this.readOnly; 1558 } 1559 1560 public boolean hasReadOnlyElement() { 1561 return this.readOnly != null && !this.readOnly.isEmpty(); 1562 } 1563 1564 public boolean hasReadOnly() { 1565 return this.readOnly != null && !this.readOnly.isEmpty(); 1566 } 1567 1568 /** 1569 * @param value {@link #readOnly} (An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.). This is the underlying object with id, value and extensions. The accessor "getReadOnly" gives direct access to the value 1570 */ 1571 public QuestionnaireItemComponent setReadOnlyElement(BooleanType value) { 1572 this.readOnly = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire. 1578 */ 1579 public boolean getReadOnly() { 1580 return this.readOnly == null || this.readOnly.isEmpty() ? false : this.readOnly.getValue(); 1581 } 1582 1583 /** 1584 * @param value An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire. 1585 */ 1586 public QuestionnaireItemComponent setReadOnly(boolean value) { 1587 if (this.readOnly == null) 1588 this.readOnly = new BooleanType(); 1589 this.readOnly.setValue(value); 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #maxLength} (The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse.). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 1595 */ 1596 public IntegerType getMaxLengthElement() { 1597 if (this.maxLength == null) 1598 if (Configuration.errorOnAutoCreate()) 1599 throw new Error("Attempt to auto-create QuestionnaireItemComponent.maxLength"); 1600 else if (Configuration.doAutoCreate()) 1601 this.maxLength = new IntegerType(); // bb 1602 return this.maxLength; 1603 } 1604 1605 public boolean hasMaxLengthElement() { 1606 return this.maxLength != null && !this.maxLength.isEmpty(); 1607 } 1608 1609 public boolean hasMaxLength() { 1610 return this.maxLength != null && !this.maxLength.isEmpty(); 1611 } 1612 1613 /** 1614 * @param value {@link #maxLength} (The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse.). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 1615 */ 1616 public QuestionnaireItemComponent setMaxLengthElement(IntegerType value) { 1617 this.maxLength = value; 1618 return this; 1619 } 1620 1621 /** 1622 * @return The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse. 1623 */ 1624 public int getMaxLength() { 1625 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 1626 } 1627 1628 /** 1629 * @param value The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse. 1630 */ 1631 public QuestionnaireItemComponent setMaxLength(int value) { 1632 if (this.maxLength == null) 1633 this.maxLength = new IntegerType(); 1634 this.maxLength.setValue(value); 1635 return this; 1636 } 1637 1638 /** 1639 * @return {@link #answerConstraint} (For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected.). This is the underlying object with id, value and extensions. The accessor "getAnswerConstraint" gives direct access to the value 1640 */ 1641 public Enumeration<QuestionnaireAnswerConstraint> getAnswerConstraintElement() { 1642 if (this.answerConstraint == null) 1643 if (Configuration.errorOnAutoCreate()) 1644 throw new Error("Attempt to auto-create QuestionnaireItemComponent.answerConstraint"); 1645 else if (Configuration.doAutoCreate()) 1646 this.answerConstraint = new Enumeration<QuestionnaireAnswerConstraint>(new QuestionnaireAnswerConstraintEnumFactory()); // bb 1647 return this.answerConstraint; 1648 } 1649 1650 public boolean hasAnswerConstraintElement() { 1651 return this.answerConstraint != null && !this.answerConstraint.isEmpty(); 1652 } 1653 1654 public boolean hasAnswerConstraint() { 1655 return this.answerConstraint != null && !this.answerConstraint.isEmpty(); 1656 } 1657 1658 /** 1659 * @param value {@link #answerConstraint} (For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected.). This is the underlying object with id, value and extensions. The accessor "getAnswerConstraint" gives direct access to the value 1660 */ 1661 public QuestionnaireItemComponent setAnswerConstraintElement(Enumeration<QuestionnaireAnswerConstraint> value) { 1662 this.answerConstraint = value; 1663 return this; 1664 } 1665 1666 /** 1667 * @return For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected. 1668 */ 1669 public QuestionnaireAnswerConstraint getAnswerConstraint() { 1670 return this.answerConstraint == null ? null : this.answerConstraint.getValue(); 1671 } 1672 1673 /** 1674 * @param value For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected. 1675 */ 1676 public QuestionnaireItemComponent setAnswerConstraint(QuestionnaireAnswerConstraint value) { 1677 if (value == null) 1678 this.answerConstraint = null; 1679 else { 1680 if (this.answerConstraint == null) 1681 this.answerConstraint = new Enumeration<QuestionnaireAnswerConstraint>(new QuestionnaireAnswerConstraintEnumFactory()); 1682 this.answerConstraint.setValue(value); 1683 } 1684 return this; 1685 } 1686 1687 /** 1688 * @return {@link #answerValueSet} (A reference to a value set containing a list of values representing permitted answers for a question.). This is the underlying object with id, value and extensions. The accessor "getAnswerValueSet" gives direct access to the value 1689 */ 1690 public CanonicalType getAnswerValueSetElement() { 1691 if (this.answerValueSet == null) 1692 if (Configuration.errorOnAutoCreate()) 1693 throw new Error("Attempt to auto-create QuestionnaireItemComponent.answerValueSet"); 1694 else if (Configuration.doAutoCreate()) 1695 this.answerValueSet = new CanonicalType(); // bb 1696 return this.answerValueSet; 1697 } 1698 1699 public boolean hasAnswerValueSetElement() { 1700 return this.answerValueSet != null && !this.answerValueSet.isEmpty(); 1701 } 1702 1703 public boolean hasAnswerValueSet() { 1704 return this.answerValueSet != null && !this.answerValueSet.isEmpty(); 1705 } 1706 1707 /** 1708 * @param value {@link #answerValueSet} (A reference to a value set containing a list of values representing permitted answers for a question.). This is the underlying object with id, value and extensions. The accessor "getAnswerValueSet" gives direct access to the value 1709 */ 1710 public QuestionnaireItemComponent setAnswerValueSetElement(CanonicalType value) { 1711 this.answerValueSet = value; 1712 return this; 1713 } 1714 1715 /** 1716 * @return A reference to a value set containing a list of values representing permitted answers for a question. 1717 */ 1718 public String getAnswerValueSet() { 1719 return this.answerValueSet == null ? null : this.answerValueSet.getValue(); 1720 } 1721 1722 /** 1723 * @param value A reference to a value set containing a list of values representing permitted answers for a question. 1724 */ 1725 public QuestionnaireItemComponent setAnswerValueSet(String value) { 1726 if (Utilities.noString(value)) 1727 this.answerValueSet = null; 1728 else { 1729 if (this.answerValueSet == null) 1730 this.answerValueSet = new CanonicalType(); 1731 this.answerValueSet.setValue(value); 1732 } 1733 return this; 1734 } 1735 1736 /** 1737 * @return {@link #answerOption} (One of the permitted answers for the question.) 1738 */ 1739 public List<QuestionnaireItemAnswerOptionComponent> getAnswerOption() { 1740 if (this.answerOption == null) 1741 this.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 1742 return this.answerOption; 1743 } 1744 1745 /** 1746 * @return Returns a reference to <code>this</code> for easy method chaining 1747 */ 1748 public QuestionnaireItemComponent setAnswerOption(List<QuestionnaireItemAnswerOptionComponent> theAnswerOption) { 1749 this.answerOption = theAnswerOption; 1750 return this; 1751 } 1752 1753 public boolean hasAnswerOption() { 1754 if (this.answerOption == null) 1755 return false; 1756 for (QuestionnaireItemAnswerOptionComponent item : this.answerOption) 1757 if (!item.isEmpty()) 1758 return true; 1759 return false; 1760 } 1761 1762 public QuestionnaireItemAnswerOptionComponent addAnswerOption() { //3 1763 QuestionnaireItemAnswerOptionComponent t = new QuestionnaireItemAnswerOptionComponent(); 1764 if (this.answerOption == null) 1765 this.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 1766 this.answerOption.add(t); 1767 return t; 1768 } 1769 1770 public QuestionnaireItemComponent addAnswerOption(QuestionnaireItemAnswerOptionComponent t) { //3 1771 if (t == null) 1772 return this; 1773 if (this.answerOption == null) 1774 this.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 1775 this.answerOption.add(t); 1776 return this; 1777 } 1778 1779 /** 1780 * @return The first repetition of repeating field {@link #answerOption}, creating it if it does not already exist {3} 1781 */ 1782 public QuestionnaireItemAnswerOptionComponent getAnswerOptionFirstRep() { 1783 if (getAnswerOption().isEmpty()) { 1784 addAnswerOption(); 1785 } 1786 return getAnswerOption().get(0); 1787 } 1788 1789 /** 1790 * @return {@link #initial} (One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input.) 1791 */ 1792 public List<QuestionnaireItemInitialComponent> getInitial() { 1793 if (this.initial == null) 1794 this.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 1795 return this.initial; 1796 } 1797 1798 /** 1799 * @return Returns a reference to <code>this</code> for easy method chaining 1800 */ 1801 public QuestionnaireItemComponent setInitial(List<QuestionnaireItemInitialComponent> theInitial) { 1802 this.initial = theInitial; 1803 return this; 1804 } 1805 1806 public boolean hasInitial() { 1807 if (this.initial == null) 1808 return false; 1809 for (QuestionnaireItemInitialComponent item : this.initial) 1810 if (!item.isEmpty()) 1811 return true; 1812 return false; 1813 } 1814 1815 public QuestionnaireItemInitialComponent addInitial() { //3 1816 QuestionnaireItemInitialComponent t = new QuestionnaireItemInitialComponent(); 1817 if (this.initial == null) 1818 this.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 1819 this.initial.add(t); 1820 return t; 1821 } 1822 1823 public QuestionnaireItemComponent addInitial(QuestionnaireItemInitialComponent t) { //3 1824 if (t == null) 1825 return this; 1826 if (this.initial == null) 1827 this.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 1828 this.initial.add(t); 1829 return this; 1830 } 1831 1832 /** 1833 * @return The first repetition of repeating field {@link #initial}, creating it if it does not already exist {3} 1834 */ 1835 public QuestionnaireItemInitialComponent getInitialFirstRep() { 1836 if (getInitial().isEmpty()) { 1837 addInitial(); 1838 } 1839 return getInitial().get(0); 1840 } 1841 1842 /** 1843 * @return {@link #item} (Text, questions and other groups to be nested beneath a question or group.) 1844 */ 1845 public List<QuestionnaireItemComponent> getItem() { 1846 if (this.item == null) 1847 this.item = new ArrayList<QuestionnaireItemComponent>(); 1848 return this.item; 1849 } 1850 1851 /** 1852 * @return Returns a reference to <code>this</code> for easy method chaining 1853 */ 1854 public QuestionnaireItemComponent setItem(List<QuestionnaireItemComponent> theItem) { 1855 this.item = theItem; 1856 return this; 1857 } 1858 1859 public boolean hasItem() { 1860 if (this.item == null) 1861 return false; 1862 for (QuestionnaireItemComponent item : this.item) 1863 if (!item.isEmpty()) 1864 return true; 1865 return false; 1866 } 1867 1868 public QuestionnaireItemComponent addItem() { //3 1869 QuestionnaireItemComponent t = new QuestionnaireItemComponent(); 1870 if (this.item == null) 1871 this.item = new ArrayList<QuestionnaireItemComponent>(); 1872 this.item.add(t); 1873 return t; 1874 } 1875 1876 public QuestionnaireItemComponent addItem(QuestionnaireItemComponent t) { //3 1877 if (t == null) 1878 return this; 1879 if (this.item == null) 1880 this.item = new ArrayList<QuestionnaireItemComponent>(); 1881 this.item.add(t); 1882 return this; 1883 } 1884 1885 /** 1886 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 1887 */ 1888 public QuestionnaireItemComponent getItemFirstRep() { 1889 if (getItem().isEmpty()) { 1890 addItem(); 1891 } 1892 return getItem().get(0); 1893 } 1894 1895 protected void listChildren(List<Property> children) { 1896 super.listChildren(children); 1897 children.add(new Property("linkId", "string", "An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.", 0, 1, linkId)); 1898 children.add(new Property("definition", "uri", "This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below.", 0, 1, definition)); 1899 children.add(new Property("code", "Coding", "A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).", 0, java.lang.Integer.MAX_VALUE, code)); 1900 children.add(new Property("prefix", "string", "A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.", 0, 1, prefix)); 1901 children.add(new Property("text", "string", "The name of a section, the text of a question or text content for a display item.", 0, 1, text)); 1902 children.add(new Property("type", "code", "The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.).", 0, 1, type)); 1903 children.add(new Property("enableWhen", "", "A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.", 0, java.lang.Integer.MAX_VALUE, enableWhen)); 1904 children.add(new Property("enableBehavior", "code", "Controls how multiple enableWhen values are interpreted - whether all or any must be true.", 0, 1, enableBehavior)); 1905 children.add(new Property("disabledDisplay", "code", "Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed.", 0, 1, disabledDisplay)); 1906 children.add(new Property("required", "boolean", "An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.", 0, 1, required)); 1907 children.add(new Property("repeats", "boolean", "An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items).", 0, 1, repeats)); 1908 children.add(new Property("readOnly", "boolean", "An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.", 0, 1, readOnly)); 1909 children.add(new Property("maxLength", "integer", "The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse.", 0, 1, maxLength)); 1910 children.add(new Property("answerConstraint", "code", "For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected.", 0, 1, answerConstraint)); 1911 children.add(new Property("answerValueSet", "canonical(ValueSet)", "A reference to a value set containing a list of values representing permitted answers for a question.", 0, 1, answerValueSet)); 1912 children.add(new Property("answerOption", "", "One of the permitted answers for the question.", 0, java.lang.Integer.MAX_VALUE, answerOption)); 1913 children.add(new Property("initial", "", "One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input.", 0, java.lang.Integer.MAX_VALUE, initial)); 1914 children.add(new Property("item", "@Questionnaire.item", "Text, questions and other groups to be nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item)); 1915 } 1916 1917 @Override 1918 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1919 switch (_hash) { 1920 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.", 0, 1, linkId); 1921 case -1014418093: /*definition*/ return new Property("definition", "uri", "This element is a URI that refers to an [ElementDefinition](elementdefinition.html) or to an [ObservationDefinition](observationdefinition.html) that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in [Comments](questionnaire.html#definition), below.", 0, 1, definition); 1922 case 3059181: /*code*/ return new Property("code", "Coding", "A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).", 0, java.lang.Integer.MAX_VALUE, code); 1923 case -980110702: /*prefix*/ return new Property("prefix", "string", "A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.", 0, 1, prefix); 1924 case 3556653: /*text*/ return new Property("text", "string", "The name of a section, the text of a question or text content for a display item.", 0, 1, text); 1925 case 3575610: /*type*/ return new Property("type", "code", "The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, Coding, etc.).", 0, 1, type); 1926 case 1893321565: /*enableWhen*/ return new Property("enableWhen", "", "A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.", 0, java.lang.Integer.MAX_VALUE, enableWhen); 1927 case 1854802165: /*enableBehavior*/ return new Property("enableBehavior", "code", "Controls how multiple enableWhen values are interpreted - whether all or any must be true.", 0, 1, enableBehavior); 1928 case -1254886746: /*disabledDisplay*/ return new Property("disabledDisplay", "code", "Indicates if and how items that are disabled (because enableWhen evaluates to 'false') should be displayed.", 0, 1, disabledDisplay); 1929 case -393139297: /*required*/ return new Property("required", "boolean", "An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.", 0, 1, required); 1930 case 1094288952: /*repeats*/ return new Property("repeats", "boolean", "An indication, if true, that a QuestionnaireResponse for this item may include multiple answers associated with a single instance of this item (for question-type items) or multiple repetitions of the item (for group-type items).", 0, 1, repeats); 1931 case -867683742: /*readOnly*/ return new Property("readOnly", "boolean", "An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.", 0, 1, readOnly); 1932 case -791400086: /*maxLength*/ return new Property("maxLength", "integer", "The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse.", 0, 1, maxLength); 1933 case 746396731: /*answerConstraint*/ return new Property("answerConstraint", "code", "For items that have a defined set of allowed answers (via answerOption or answerValueSet), indicates whether values *other* than those specified can be selected.", 0, 1, answerConstraint); 1934 case -743278833: /*answerValueSet*/ return new Property("answerValueSet", "canonical(ValueSet)", "A reference to a value set containing a list of values representing permitted answers for a question.", 0, 1, answerValueSet); 1935 case -1527878189: /*answerOption*/ return new Property("answerOption", "", "One of the permitted answers for the question.", 0, java.lang.Integer.MAX_VALUE, answerOption); 1936 case 1948342084: /*initial*/ return new Property("initial", "", "One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input.", 0, java.lang.Integer.MAX_VALUE, initial); 1937 case 3242771: /*item*/ return new Property("item", "@Questionnaire.item", "Text, questions and other groups to be nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item); 1938 default: return super.getNamedProperty(_hash, _name, _checkValid); 1939 } 1940 1941 } 1942 1943 @Override 1944 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1945 switch (hash) { 1946 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 1947 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // UriType 1948 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 1949 case -980110702: /*prefix*/ return this.prefix == null ? new Base[0] : new Base[] {this.prefix}; // StringType 1950 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 1951 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<QuestionnaireItemType> 1952 case 1893321565: /*enableWhen*/ return this.enableWhen == null ? new Base[0] : this.enableWhen.toArray(new Base[this.enableWhen.size()]); // QuestionnaireItemEnableWhenComponent 1953 case 1854802165: /*enableBehavior*/ return this.enableBehavior == null ? new Base[0] : new Base[] {this.enableBehavior}; // Enumeration<EnableWhenBehavior> 1954 case -1254886746: /*disabledDisplay*/ return this.disabledDisplay == null ? new Base[0] : new Base[] {this.disabledDisplay}; // Enumeration<QuestionnaireItemDisabledDisplay> 1955 case -393139297: /*required*/ return this.required == null ? new Base[0] : new Base[] {this.required}; // BooleanType 1956 case 1094288952: /*repeats*/ return this.repeats == null ? new Base[0] : new Base[] {this.repeats}; // BooleanType 1957 case -867683742: /*readOnly*/ return this.readOnly == null ? new Base[0] : new Base[] {this.readOnly}; // BooleanType 1958 case -791400086: /*maxLength*/ return this.maxLength == null ? new Base[0] : new Base[] {this.maxLength}; // IntegerType 1959 case 746396731: /*answerConstraint*/ return this.answerConstraint == null ? new Base[0] : new Base[] {this.answerConstraint}; // Enumeration<QuestionnaireAnswerConstraint> 1960 case -743278833: /*answerValueSet*/ return this.answerValueSet == null ? new Base[0] : new Base[] {this.answerValueSet}; // CanonicalType 1961 case -1527878189: /*answerOption*/ return this.answerOption == null ? new Base[0] : this.answerOption.toArray(new Base[this.answerOption.size()]); // QuestionnaireItemAnswerOptionComponent 1962 case 1948342084: /*initial*/ return this.initial == null ? new Base[0] : this.initial.toArray(new Base[this.initial.size()]); // QuestionnaireItemInitialComponent 1963 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireItemComponent 1964 default: return super.getProperty(hash, name, checkValid); 1965 } 1966 1967 } 1968 1969 @Override 1970 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1971 switch (hash) { 1972 case -1102667083: // linkId 1973 this.linkId = TypeConvertor.castToString(value); // StringType 1974 return value; 1975 case -1014418093: // definition 1976 this.definition = TypeConvertor.castToUri(value); // UriType 1977 return value; 1978 case 3059181: // code 1979 this.getCode().add(TypeConvertor.castToCoding(value)); // Coding 1980 return value; 1981 case -980110702: // prefix 1982 this.prefix = TypeConvertor.castToString(value); // StringType 1983 return value; 1984 case 3556653: // text 1985 this.text = TypeConvertor.castToString(value); // StringType 1986 return value; 1987 case 3575610: // type 1988 value = new QuestionnaireItemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1989 this.type = (Enumeration) value; // Enumeration<QuestionnaireItemType> 1990 return value; 1991 case 1893321565: // enableWhen 1992 this.getEnableWhen().add((QuestionnaireItemEnableWhenComponent) value); // QuestionnaireItemEnableWhenComponent 1993 return value; 1994 case 1854802165: // enableBehavior 1995 value = new EnableWhenBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1996 this.enableBehavior = (Enumeration) value; // Enumeration<EnableWhenBehavior> 1997 return value; 1998 case -1254886746: // disabledDisplay 1999 value = new QuestionnaireItemDisabledDisplayEnumFactory().fromType(TypeConvertor.castToCode(value)); 2000 this.disabledDisplay = (Enumeration) value; // Enumeration<QuestionnaireItemDisabledDisplay> 2001 return value; 2002 case -393139297: // required 2003 this.required = TypeConvertor.castToBoolean(value); // BooleanType 2004 return value; 2005 case 1094288952: // repeats 2006 this.repeats = TypeConvertor.castToBoolean(value); // BooleanType 2007 return value; 2008 case -867683742: // readOnly 2009 this.readOnly = TypeConvertor.castToBoolean(value); // BooleanType 2010 return value; 2011 case -791400086: // maxLength 2012 this.maxLength = TypeConvertor.castToInteger(value); // IntegerType 2013 return value; 2014 case 746396731: // answerConstraint 2015 value = new QuestionnaireAnswerConstraintEnumFactory().fromType(TypeConvertor.castToCode(value)); 2016 this.answerConstraint = (Enumeration) value; // Enumeration<QuestionnaireAnswerConstraint> 2017 return value; 2018 case -743278833: // answerValueSet 2019 this.answerValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 2020 return value; 2021 case -1527878189: // answerOption 2022 this.getAnswerOption().add((QuestionnaireItemAnswerOptionComponent) value); // QuestionnaireItemAnswerOptionComponent 2023 return value; 2024 case 1948342084: // initial 2025 this.getInitial().add((QuestionnaireItemInitialComponent) value); // QuestionnaireItemInitialComponent 2026 return value; 2027 case 3242771: // item 2028 this.getItem().add((QuestionnaireItemComponent) value); // QuestionnaireItemComponent 2029 return value; 2030 default: return super.setProperty(hash, name, value); 2031 } 2032 2033 } 2034 2035 @Override 2036 public Base setProperty(String name, Base value) throws FHIRException { 2037 if (name.equals("linkId")) { 2038 this.linkId = TypeConvertor.castToString(value); // StringType 2039 } else if (name.equals("definition")) { 2040 this.definition = TypeConvertor.castToUri(value); // UriType 2041 } else if (name.equals("code")) { 2042 this.getCode().add(TypeConvertor.castToCoding(value)); 2043 } else if (name.equals("prefix")) { 2044 this.prefix = TypeConvertor.castToString(value); // StringType 2045 } else if (name.equals("text")) { 2046 this.text = TypeConvertor.castToString(value); // StringType 2047 } else if (name.equals("type")) { 2048 value = new QuestionnaireItemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2049 this.type = (Enumeration) value; // Enumeration<QuestionnaireItemType> 2050 } else if (name.equals("enableWhen")) { 2051 this.getEnableWhen().add((QuestionnaireItemEnableWhenComponent) value); 2052 } else if (name.equals("enableBehavior")) { 2053 value = new EnableWhenBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2054 this.enableBehavior = (Enumeration) value; // Enumeration<EnableWhenBehavior> 2055 } else if (name.equals("disabledDisplay")) { 2056 value = new QuestionnaireItemDisabledDisplayEnumFactory().fromType(TypeConvertor.castToCode(value)); 2057 this.disabledDisplay = (Enumeration) value; // Enumeration<QuestionnaireItemDisabledDisplay> 2058 } else if (name.equals("required")) { 2059 this.required = TypeConvertor.castToBoolean(value); // BooleanType 2060 } else if (name.equals("repeats")) { 2061 this.repeats = TypeConvertor.castToBoolean(value); // BooleanType 2062 } else if (name.equals("readOnly")) { 2063 this.readOnly = TypeConvertor.castToBoolean(value); // BooleanType 2064 } else if (name.equals("maxLength")) { 2065 this.maxLength = TypeConvertor.castToInteger(value); // IntegerType 2066 } else if (name.equals("answerConstraint")) { 2067 value = new QuestionnaireAnswerConstraintEnumFactory().fromType(TypeConvertor.castToCode(value)); 2068 this.answerConstraint = (Enumeration) value; // Enumeration<QuestionnaireAnswerConstraint> 2069 } else if (name.equals("answerValueSet")) { 2070 this.answerValueSet = TypeConvertor.castToCanonical(value); // CanonicalType 2071 } else if (name.equals("answerOption")) { 2072 this.getAnswerOption().add((QuestionnaireItemAnswerOptionComponent) value); 2073 } else if (name.equals("initial")) { 2074 this.getInitial().add((QuestionnaireItemInitialComponent) value); 2075 } else if (name.equals("item")) { 2076 this.getItem().add((QuestionnaireItemComponent) value); 2077 } else 2078 return super.setProperty(name, value); 2079 return value; 2080 } 2081 2082 @Override 2083 public void removeChild(String name, Base value) throws FHIRException { 2084 if (name.equals("linkId")) { 2085 this.linkId = null; 2086 } else if (name.equals("definition")) { 2087 this.definition = null; 2088 } else if (name.equals("code")) { 2089 this.getCode().remove(value); 2090 } else if (name.equals("prefix")) { 2091 this.prefix = null; 2092 } else if (name.equals("text")) { 2093 this.text = null; 2094 } else if (name.equals("type")) { 2095 value = new QuestionnaireItemTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2096 this.type = (Enumeration) value; // Enumeration<QuestionnaireItemType> 2097 } else if (name.equals("enableWhen")) { 2098 this.getEnableWhen().remove((QuestionnaireItemEnableWhenComponent) value); 2099 } else if (name.equals("enableBehavior")) { 2100 value = new EnableWhenBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2101 this.enableBehavior = (Enumeration) value; // Enumeration<EnableWhenBehavior> 2102 } else if (name.equals("disabledDisplay")) { 2103 value = new QuestionnaireItemDisabledDisplayEnumFactory().fromType(TypeConvertor.castToCode(value)); 2104 this.disabledDisplay = (Enumeration) value; // Enumeration<QuestionnaireItemDisabledDisplay> 2105 } else if (name.equals("required")) { 2106 this.required = null; 2107 } else if (name.equals("repeats")) { 2108 this.repeats = null; 2109 } else if (name.equals("readOnly")) { 2110 this.readOnly = null; 2111 } else if (name.equals("maxLength")) { 2112 this.maxLength = null; 2113 } else if (name.equals("answerConstraint")) { 2114 value = new QuestionnaireAnswerConstraintEnumFactory().fromType(TypeConvertor.castToCode(value)); 2115 this.answerConstraint = (Enumeration) value; // Enumeration<QuestionnaireAnswerConstraint> 2116 } else if (name.equals("answerValueSet")) { 2117 this.answerValueSet = null; 2118 } else if (name.equals("answerOption")) { 2119 this.getAnswerOption().remove((QuestionnaireItemAnswerOptionComponent) value); 2120 } else if (name.equals("initial")) { 2121 this.getInitial().remove((QuestionnaireItemInitialComponent) value); 2122 } else if (name.equals("item")) { 2123 this.getItem().remove((QuestionnaireItemComponent) value); 2124 } else 2125 super.removeChild(name, value); 2126 2127 } 2128 2129 @Override 2130 public Base makeProperty(int hash, String name) throws FHIRException { 2131 switch (hash) { 2132 case -1102667083: return getLinkIdElement(); 2133 case -1014418093: return getDefinitionElement(); 2134 case 3059181: return addCode(); 2135 case -980110702: return getPrefixElement(); 2136 case 3556653: return getTextElement(); 2137 case 3575610: return getTypeElement(); 2138 case 1893321565: return addEnableWhen(); 2139 case 1854802165: return getEnableBehaviorElement(); 2140 case -1254886746: return getDisabledDisplayElement(); 2141 case -393139297: return getRequiredElement(); 2142 case 1094288952: return getRepeatsElement(); 2143 case -867683742: return getReadOnlyElement(); 2144 case -791400086: return getMaxLengthElement(); 2145 case 746396731: return getAnswerConstraintElement(); 2146 case -743278833: return getAnswerValueSetElement(); 2147 case -1527878189: return addAnswerOption(); 2148 case 1948342084: return addInitial(); 2149 case 3242771: return addItem(); 2150 default: return super.makeProperty(hash, name); 2151 } 2152 2153 } 2154 2155 @Override 2156 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2157 switch (hash) { 2158 case -1102667083: /*linkId*/ return new String[] {"string"}; 2159 case -1014418093: /*definition*/ return new String[] {"uri"}; 2160 case 3059181: /*code*/ return new String[] {"Coding"}; 2161 case -980110702: /*prefix*/ return new String[] {"string"}; 2162 case 3556653: /*text*/ return new String[] {"string"}; 2163 case 3575610: /*type*/ return new String[] {"code"}; 2164 case 1893321565: /*enableWhen*/ return new String[] {}; 2165 case 1854802165: /*enableBehavior*/ return new String[] {"code"}; 2166 case -1254886746: /*disabledDisplay*/ return new String[] {"code"}; 2167 case -393139297: /*required*/ return new String[] {"boolean"}; 2168 case 1094288952: /*repeats*/ return new String[] {"boolean"}; 2169 case -867683742: /*readOnly*/ return new String[] {"boolean"}; 2170 case -791400086: /*maxLength*/ return new String[] {"integer"}; 2171 case 746396731: /*answerConstraint*/ return new String[] {"code"}; 2172 case -743278833: /*answerValueSet*/ return new String[] {"canonical"}; 2173 case -1527878189: /*answerOption*/ return new String[] {}; 2174 case 1948342084: /*initial*/ return new String[] {}; 2175 case 3242771: /*item*/ return new String[] {"@Questionnaire.item"}; 2176 default: return super.getTypesForProperty(hash, name); 2177 } 2178 2179 } 2180 2181 @Override 2182 public Base addChild(String name) throws FHIRException { 2183 if (name.equals("linkId")) { 2184 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.linkId"); 2185 } 2186 else if (name.equals("definition")) { 2187 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.definition"); 2188 } 2189 else if (name.equals("code")) { 2190 return addCode(); 2191 } 2192 else if (name.equals("prefix")) { 2193 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.prefix"); 2194 } 2195 else if (name.equals("text")) { 2196 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.text"); 2197 } 2198 else if (name.equals("type")) { 2199 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.type"); 2200 } 2201 else if (name.equals("enableWhen")) { 2202 return addEnableWhen(); 2203 } 2204 else if (name.equals("enableBehavior")) { 2205 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.enableBehavior"); 2206 } 2207 else if (name.equals("disabledDisplay")) { 2208 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.disabledDisplay"); 2209 } 2210 else if (name.equals("required")) { 2211 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.required"); 2212 } 2213 else if (name.equals("repeats")) { 2214 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.repeats"); 2215 } 2216 else if (name.equals("readOnly")) { 2217 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.readOnly"); 2218 } 2219 else if (name.equals("maxLength")) { 2220 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.maxLength"); 2221 } 2222 else if (name.equals("answerConstraint")) { 2223 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.answerConstraint"); 2224 } 2225 else if (name.equals("answerValueSet")) { 2226 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.answerValueSet"); 2227 } 2228 else if (name.equals("answerOption")) { 2229 return addAnswerOption(); 2230 } 2231 else if (name.equals("initial")) { 2232 return addInitial(); 2233 } 2234 else if (name.equals("item")) { 2235 return addItem(); 2236 } 2237 else 2238 return super.addChild(name); 2239 } 2240 2241 public QuestionnaireItemComponent copy() { 2242 QuestionnaireItemComponent dst = new QuestionnaireItemComponent(); 2243 copyValues(dst); 2244 return dst; 2245 } 2246 2247 public void copyValues(QuestionnaireItemComponent dst) { 2248 super.copyValues(dst); 2249 dst.linkId = linkId == null ? null : linkId.copy(); 2250 dst.definition = definition == null ? null : definition.copy(); 2251 if (code != null) { 2252 dst.code = new ArrayList<Coding>(); 2253 for (Coding i : code) 2254 dst.code.add(i.copy()); 2255 }; 2256 dst.prefix = prefix == null ? null : prefix.copy(); 2257 dst.text = text == null ? null : text.copy(); 2258 dst.type = type == null ? null : type.copy(); 2259 if (enableWhen != null) { 2260 dst.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 2261 for (QuestionnaireItemEnableWhenComponent i : enableWhen) 2262 dst.enableWhen.add(i.copy()); 2263 }; 2264 dst.enableBehavior = enableBehavior == null ? null : enableBehavior.copy(); 2265 dst.disabledDisplay = disabledDisplay == null ? null : disabledDisplay.copy(); 2266 dst.required = required == null ? null : required.copy(); 2267 dst.repeats = repeats == null ? null : repeats.copy(); 2268 dst.readOnly = readOnly == null ? null : readOnly.copy(); 2269 dst.maxLength = maxLength == null ? null : maxLength.copy(); 2270 dst.answerConstraint = answerConstraint == null ? null : answerConstraint.copy(); 2271 dst.answerValueSet = answerValueSet == null ? null : answerValueSet.copy(); 2272 if (answerOption != null) { 2273 dst.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 2274 for (QuestionnaireItemAnswerOptionComponent i : answerOption) 2275 dst.answerOption.add(i.copy()); 2276 }; 2277 if (initial != null) { 2278 dst.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 2279 for (QuestionnaireItemInitialComponent i : initial) 2280 dst.initial.add(i.copy()); 2281 }; 2282 if (item != null) { 2283 dst.item = new ArrayList<QuestionnaireItemComponent>(); 2284 for (QuestionnaireItemComponent i : item) 2285 dst.item.add(i.copy()); 2286 }; 2287 } 2288 2289 @Override 2290 public boolean equalsDeep(Base other_) { 2291 if (!super.equalsDeep(other_)) 2292 return false; 2293 if (!(other_ instanceof QuestionnaireItemComponent)) 2294 return false; 2295 QuestionnaireItemComponent o = (QuestionnaireItemComponent) other_; 2296 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) && compareDeep(code, o.code, true) 2297 && compareDeep(prefix, o.prefix, true) && compareDeep(text, o.text, true) && compareDeep(type, o.type, true) 2298 && compareDeep(enableWhen, o.enableWhen, true) && compareDeep(enableBehavior, o.enableBehavior, true) 2299 && compareDeep(disabledDisplay, o.disabledDisplay, true) && compareDeep(required, o.required, true) 2300 && compareDeep(repeats, o.repeats, true) && compareDeep(readOnly, o.readOnly, true) && compareDeep(maxLength, o.maxLength, true) 2301 && compareDeep(answerConstraint, o.answerConstraint, true) && compareDeep(answerValueSet, o.answerValueSet, true) 2302 && compareDeep(answerOption, o.answerOption, true) && compareDeep(initial, o.initial, true) && compareDeep(item, o.item, true) 2303 ; 2304 } 2305 2306 @Override 2307 public boolean equalsShallow(Base other_) { 2308 if (!super.equalsShallow(other_)) 2309 return false; 2310 if (!(other_ instanceof QuestionnaireItemComponent)) 2311 return false; 2312 QuestionnaireItemComponent o = (QuestionnaireItemComponent) other_; 2313 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) && compareValues(prefix, o.prefix, true) 2314 && compareValues(text, o.text, true) && compareValues(type, o.type, true) && compareValues(enableBehavior, o.enableBehavior, true) 2315 && compareValues(disabledDisplay, o.disabledDisplay, true) && compareValues(required, o.required, true) 2316 && compareValues(repeats, o.repeats, true) && compareValues(readOnly, o.readOnly, true) && compareValues(maxLength, o.maxLength, true) 2317 && compareValues(answerConstraint, o.answerConstraint, true) && compareValues(answerValueSet, o.answerValueSet, true) 2318 ; 2319 } 2320 2321 public boolean isEmpty() { 2322 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, code 2323 , prefix, text, type, enableWhen, enableBehavior, disabledDisplay, required, repeats 2324 , readOnly, maxLength, answerConstraint, answerValueSet, answerOption, initial, item 2325 ); 2326 } 2327 2328 public String fhirType() { 2329 return "Questionnaire.item"; 2330 2331 } 2332 2333// added from java-adornments.txt: 2334public QuestionnaireItemComponent getQuestion(String linkId) { 2335 if (linkId == null) 2336 return null; 2337 for (QuestionnaireItemComponent i : getItem()) { 2338 if (i.getLinkId().equals(linkId)) 2339 return i; 2340 QuestionnaireItemComponent t = i.getQuestion(linkId); 2341 if (t != null) 2342 return t; 2343 } 2344 return null; 2345 } 2346 2347 public QuestionnaireItemComponent getCommonGroup(QuestionnaireItemComponent q1, QuestionnaireItemComponent q2) { 2348 if (q1 == null || q2 == null) 2349 return null; 2350 for (QuestionnaireItemComponent i : getItem()) { 2351 QuestionnaireItemComponent t = i.getCommonGroup(q1, q2); 2352 if (t != null) 2353 return t; 2354 } 2355 if (containsQuestion(q1) && containsQuestion(q2)) 2356 return this; 2357 return null; 2358 } 2359 2360 public boolean containsQuestion(QuestionnaireItemComponent q) { 2361 if (q == this) 2362 return true; 2363 for (QuestionnaireItemComponent i : getItem()) { 2364 if (i.containsQuestion(q)) 2365 return true; 2366 } 2367 return false; 2368 } 2369// end addition 2370 } 2371 2372 @Block() 2373 public static class QuestionnaireItemEnableWhenComponent extends BackboneElement implements IBaseBackboneElement { 2374 /** 2375 * The linkId for the question whose answer (or lack of answer) governs whether this item is enabled. 2376 */ 2377 @Child(name = "question", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2378 @Description(shortDefinition="The linkId of question that determines whether item is enabled/disabled", formalDefinition="The linkId for the question whose answer (or lack of answer) governs whether this item is enabled." ) 2379 protected StringType question; 2380 2381 /** 2382 * Specifies the criteria by which the question is enabled. 2383 */ 2384 @Child(name = "operator", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2385 @Description(shortDefinition="exists | = | != | > | < | >= | <=", formalDefinition="Specifies the criteria by which the question is enabled." ) 2386 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-enable-operator") 2387 protected Enumeration<QuestionnaireItemOperator> operator; 2388 2389 /** 2390 * A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension. 2391 */ 2392 @Child(name = "answer", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, Coding.class, Quantity.class, Reference.class}, order=3, min=1, max=1, modifier=false, summary=false) 2393 @Description(shortDefinition="Value for question comparison based on operator", formalDefinition="A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension." ) 2394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 2395 protected DataType answer; 2396 2397 private static final long serialVersionUID = 1909865374L; 2398 2399 /** 2400 * Constructor 2401 */ 2402 public QuestionnaireItemEnableWhenComponent() { 2403 super(); 2404 } 2405 2406 /** 2407 * Constructor 2408 */ 2409 public QuestionnaireItemEnableWhenComponent(String question, QuestionnaireItemOperator operator, DataType answer) { 2410 super(); 2411 this.setQuestion(question); 2412 this.setOperator(operator); 2413 this.setAnswer(answer); 2414 } 2415 2416 /** 2417 * @return {@link #question} (The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.). This is the underlying object with id, value and extensions. The accessor "getQuestion" gives direct access to the value 2418 */ 2419 public StringType getQuestionElement() { 2420 if (this.question == null) 2421 if (Configuration.errorOnAutoCreate()) 2422 throw new Error("Attempt to auto-create QuestionnaireItemEnableWhenComponent.question"); 2423 else if (Configuration.doAutoCreate()) 2424 this.question = new StringType(); // bb 2425 return this.question; 2426 } 2427 2428 public boolean hasQuestionElement() { 2429 return this.question != null && !this.question.isEmpty(); 2430 } 2431 2432 public boolean hasQuestion() { 2433 return this.question != null && !this.question.isEmpty(); 2434 } 2435 2436 /** 2437 * @param value {@link #question} (The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.). This is the underlying object with id, value and extensions. The accessor "getQuestion" gives direct access to the value 2438 */ 2439 public QuestionnaireItemEnableWhenComponent setQuestionElement(StringType value) { 2440 this.question = value; 2441 return this; 2442 } 2443 2444 /** 2445 * @return The linkId for the question whose answer (or lack of answer) governs whether this item is enabled. 2446 */ 2447 public String getQuestion() { 2448 return this.question == null ? null : this.question.getValue(); 2449 } 2450 2451 /** 2452 * @param value The linkId for the question whose answer (or lack of answer) governs whether this item is enabled. 2453 */ 2454 public QuestionnaireItemEnableWhenComponent setQuestion(String value) { 2455 if (this.question == null) 2456 this.question = new StringType(); 2457 this.question.setValue(value); 2458 return this; 2459 } 2460 2461 /** 2462 * @return {@link #operator} (Specifies the criteria by which the question is enabled.). This is the underlying object with id, value and extensions. The accessor "getOperator" gives direct access to the value 2463 */ 2464 public Enumeration<QuestionnaireItemOperator> getOperatorElement() { 2465 if (this.operator == null) 2466 if (Configuration.errorOnAutoCreate()) 2467 throw new Error("Attempt to auto-create QuestionnaireItemEnableWhenComponent.operator"); 2468 else if (Configuration.doAutoCreate()) 2469 this.operator = new Enumeration<QuestionnaireItemOperator>(new QuestionnaireItemOperatorEnumFactory()); // bb 2470 return this.operator; 2471 } 2472 2473 public boolean hasOperatorElement() { 2474 return this.operator != null && !this.operator.isEmpty(); 2475 } 2476 2477 public boolean hasOperator() { 2478 return this.operator != null && !this.operator.isEmpty(); 2479 } 2480 2481 /** 2482 * @param value {@link #operator} (Specifies the criteria by which the question is enabled.). This is the underlying object with id, value and extensions. The accessor "getOperator" gives direct access to the value 2483 */ 2484 public QuestionnaireItemEnableWhenComponent setOperatorElement(Enumeration<QuestionnaireItemOperator> value) { 2485 this.operator = value; 2486 return this; 2487 } 2488 2489 /** 2490 * @return Specifies the criteria by which the question is enabled. 2491 */ 2492 public QuestionnaireItemOperator getOperator() { 2493 return this.operator == null ? null : this.operator.getValue(); 2494 } 2495 2496 /** 2497 * @param value Specifies the criteria by which the question is enabled. 2498 */ 2499 public QuestionnaireItemEnableWhenComponent setOperator(QuestionnaireItemOperator value) { 2500 if (this.operator == null) 2501 this.operator = new Enumeration<QuestionnaireItemOperator>(new QuestionnaireItemOperatorEnumFactory()); 2502 this.operator.setValue(value); 2503 return this; 2504 } 2505 2506 /** 2507 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2508 */ 2509 public DataType getAnswer() { 2510 return this.answer; 2511 } 2512 2513 /** 2514 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2515 */ 2516 public BooleanType getAnswerBooleanType() throws FHIRException { 2517 if (this.answer == null) 2518 this.answer = new BooleanType(); 2519 if (!(this.answer instanceof BooleanType)) 2520 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2521 return (BooleanType) this.answer; 2522 } 2523 2524 public boolean hasAnswerBooleanType() { 2525 return this.answer instanceof BooleanType; 2526 } 2527 2528 /** 2529 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2530 */ 2531 public DecimalType getAnswerDecimalType() throws FHIRException { 2532 if (this.answer == null) 2533 this.answer = new DecimalType(); 2534 if (!(this.answer instanceof DecimalType)) 2535 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2536 return (DecimalType) this.answer; 2537 } 2538 2539 public boolean hasAnswerDecimalType() { 2540 return this.answer instanceof DecimalType; 2541 } 2542 2543 /** 2544 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2545 */ 2546 public IntegerType getAnswerIntegerType() throws FHIRException { 2547 if (this.answer == null) 2548 this.answer = new IntegerType(); 2549 if (!(this.answer instanceof IntegerType)) 2550 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2551 return (IntegerType) this.answer; 2552 } 2553 2554 public boolean hasAnswerIntegerType() { 2555 return this.answer instanceof IntegerType; 2556 } 2557 2558 /** 2559 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2560 */ 2561 public DateType getAnswerDateType() throws FHIRException { 2562 if (this.answer == null) 2563 this.answer = new DateType(); 2564 if (!(this.answer instanceof DateType)) 2565 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2566 return (DateType) this.answer; 2567 } 2568 2569 public boolean hasAnswerDateType() { 2570 return this.answer instanceof DateType; 2571 } 2572 2573 /** 2574 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2575 */ 2576 public DateTimeType getAnswerDateTimeType() throws FHIRException { 2577 if (this.answer == null) 2578 this.answer = new DateTimeType(); 2579 if (!(this.answer instanceof DateTimeType)) 2580 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2581 return (DateTimeType) this.answer; 2582 } 2583 2584 public boolean hasAnswerDateTimeType() { 2585 return this.answer instanceof DateTimeType; 2586 } 2587 2588 /** 2589 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2590 */ 2591 public TimeType getAnswerTimeType() throws FHIRException { 2592 if (this.answer == null) 2593 this.answer = new TimeType(); 2594 if (!(this.answer instanceof TimeType)) 2595 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2596 return (TimeType) this.answer; 2597 } 2598 2599 public boolean hasAnswerTimeType() { 2600 return this.answer instanceof TimeType; 2601 } 2602 2603 /** 2604 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2605 */ 2606 public StringType getAnswerStringType() throws FHIRException { 2607 if (this.answer == null) 2608 this.answer = new StringType(); 2609 if (!(this.answer instanceof StringType)) 2610 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2611 return (StringType) this.answer; 2612 } 2613 2614 public boolean hasAnswerStringType() { 2615 return this.answer instanceof StringType; 2616 } 2617 2618 /** 2619 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2620 */ 2621 public Coding getAnswerCoding() throws FHIRException { 2622 if (this.answer == null) 2623 this.answer = new Coding(); 2624 if (!(this.answer instanceof Coding)) 2625 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.answer.getClass().getName()+" was encountered"); 2626 return (Coding) this.answer; 2627 } 2628 2629 public boolean hasAnswerCoding() { 2630 return this.answer instanceof Coding; 2631 } 2632 2633 /** 2634 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2635 */ 2636 public Quantity getAnswerQuantity() throws FHIRException { 2637 if (this.answer == null) 2638 this.answer = new Quantity(); 2639 if (!(this.answer instanceof Quantity)) 2640 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.answer.getClass().getName()+" was encountered"); 2641 return (Quantity) this.answer; 2642 } 2643 2644 public boolean hasAnswerQuantity() { 2645 return this.answer instanceof Quantity; 2646 } 2647 2648 /** 2649 * @return {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2650 */ 2651 public Reference getAnswerReference() throws FHIRException { 2652 if (this.answer == null) 2653 this.answer = new Reference(); 2654 if (!(this.answer instanceof Reference)) 2655 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.answer.getClass().getName()+" was encountered"); 2656 return (Reference) this.answer; 2657 } 2658 2659 public boolean hasAnswerReference() { 2660 return this.answer instanceof Reference; 2661 } 2662 2663 public boolean hasAnswer() { 2664 return this.answer != null && !this.answer.isEmpty(); 2665 } 2666 2667 /** 2668 * @param value {@link #answer} (A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.) 2669 */ 2670 public QuestionnaireItemEnableWhenComponent setAnswer(DataType value) { 2671 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 2672 throw new FHIRException("Not the right type for Questionnaire.item.enableWhen.answer[x]: "+value.fhirType()); 2673 this.answer = value; 2674 return this; 2675 } 2676 2677 protected void listChildren(List<Property> children) { 2678 super.listChildren(children); 2679 children.add(new Property("question", "string", "The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.", 0, 1, question)); 2680 children.add(new Property("operator", "code", "Specifies the criteria by which the question is enabled.", 0, 1, operator)); 2681 children.add(new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer)); 2682 } 2683 2684 @Override 2685 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2686 switch (_hash) { 2687 case -1165870106: /*question*/ return new Property("question", "string", "The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.", 0, 1, question); 2688 case -500553564: /*operator*/ return new Property("operator", "code", "Specifies the criteria by which the question is enabled.", 0, 1, operator); 2689 case 1693524994: /*answer[x]*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2690 case -1412808770: /*answer*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2691 case 1194603146: /*answerBoolean*/ return new Property("answer[x]", "boolean", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2692 case -1622812237: /*answerDecimal*/ return new Property("answer[x]", "decimal", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2693 case -1207023712: /*answerInteger*/ return new Property("answer[x]", "integer", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2694 case 958960780: /*answerDate*/ return new Property("answer[x]", "date", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2695 case -1835321991: /*answerDateTime*/ return new Property("answer[x]", "dateTime", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2696 case 959444907: /*answerTime*/ return new Property("answer[x]", "time", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2697 case -1409727121: /*answerString*/ return new Property("answer[x]", "string", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2698 case -1872828216: /*answerCoding*/ return new Property("answer[x]", "Coding", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2699 case -618108311: /*answerQuantity*/ return new Property("answer[x]", "Quantity", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2700 case -1726221011: /*answerReference*/ return new Property("answer[x]", "Reference(Any)", "A value that the referenced question is tested using the specified operator in order for the item to be enabled. If there are multiple answers, a match on any of the answers suffices. If different behavior is desired (all must match, at least 2 must match, etc.), consider using the enableWhenExpression extension.", 0, 1, answer); 2701 default: return super.getNamedProperty(_hash, _name, _checkValid); 2702 } 2703 2704 } 2705 2706 @Override 2707 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2708 switch (hash) { 2709 case -1165870106: /*question*/ return this.question == null ? new Base[0] : new Base[] {this.question}; // StringType 2710 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : new Base[] {this.operator}; // Enumeration<QuestionnaireItemOperator> 2711 case -1412808770: /*answer*/ return this.answer == null ? new Base[0] : new Base[] {this.answer}; // DataType 2712 default: return super.getProperty(hash, name, checkValid); 2713 } 2714 2715 } 2716 2717 @Override 2718 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2719 switch (hash) { 2720 case -1165870106: // question 2721 this.question = TypeConvertor.castToString(value); // StringType 2722 return value; 2723 case -500553564: // operator 2724 value = new QuestionnaireItemOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2725 this.operator = (Enumeration) value; // Enumeration<QuestionnaireItemOperator> 2726 return value; 2727 case -1412808770: // answer 2728 this.answer = TypeConvertor.castToType(value); // DataType 2729 return value; 2730 default: return super.setProperty(hash, name, value); 2731 } 2732 2733 } 2734 2735 @Override 2736 public Base setProperty(String name, Base value) throws FHIRException { 2737 if (name.equals("question")) { 2738 this.question = TypeConvertor.castToString(value); // StringType 2739 } else if (name.equals("operator")) { 2740 value = new QuestionnaireItemOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2741 this.operator = (Enumeration) value; // Enumeration<QuestionnaireItemOperator> 2742 } else if (name.equals("answer[x]")) { 2743 this.answer = TypeConvertor.castToType(value); // DataType 2744 } else 2745 return super.setProperty(name, value); 2746 return value; 2747 } 2748 2749 @Override 2750 public void removeChild(String name, Base value) throws FHIRException { 2751 if (name.equals("question")) { 2752 this.question = null; 2753 } else if (name.equals("operator")) { 2754 value = new QuestionnaireItemOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2755 this.operator = (Enumeration) value; // Enumeration<QuestionnaireItemOperator> 2756 } else if (name.equals("answer[x]")) { 2757 this.answer = null; 2758 } else 2759 super.removeChild(name, value); 2760 2761 } 2762 2763 @Override 2764 public Base makeProperty(int hash, String name) throws FHIRException { 2765 switch (hash) { 2766 case -1165870106: return getQuestionElement(); 2767 case -500553564: return getOperatorElement(); 2768 case 1693524994: return getAnswer(); 2769 case -1412808770: return getAnswer(); 2770 default: return super.makeProperty(hash, name); 2771 } 2772 2773 } 2774 2775 @Override 2776 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2777 switch (hash) { 2778 case -1165870106: /*question*/ return new String[] {"string"}; 2779 case -500553564: /*operator*/ return new String[] {"code"}; 2780 case -1412808770: /*answer*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "Coding", "Quantity", "Reference"}; 2781 default: return super.getTypesForProperty(hash, name); 2782 } 2783 2784 } 2785 2786 @Override 2787 public Base addChild(String name) throws FHIRException { 2788 if (name.equals("question")) { 2789 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.enableWhen.question"); 2790 } 2791 else if (name.equals("operator")) { 2792 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.enableWhen.operator"); 2793 } 2794 else if (name.equals("answerBoolean")) { 2795 this.answer = new BooleanType(); 2796 return this.answer; 2797 } 2798 else if (name.equals("answerDecimal")) { 2799 this.answer = new DecimalType(); 2800 return this.answer; 2801 } 2802 else if (name.equals("answerInteger")) { 2803 this.answer = new IntegerType(); 2804 return this.answer; 2805 } 2806 else if (name.equals("answerDate")) { 2807 this.answer = new DateType(); 2808 return this.answer; 2809 } 2810 else if (name.equals("answerDateTime")) { 2811 this.answer = new DateTimeType(); 2812 return this.answer; 2813 } 2814 else if (name.equals("answerTime")) { 2815 this.answer = new TimeType(); 2816 return this.answer; 2817 } 2818 else if (name.equals("answerString")) { 2819 this.answer = new StringType(); 2820 return this.answer; 2821 } 2822 else if (name.equals("answerCoding")) { 2823 this.answer = new Coding(); 2824 return this.answer; 2825 } 2826 else if (name.equals("answerQuantity")) { 2827 this.answer = new Quantity(); 2828 return this.answer; 2829 } 2830 else if (name.equals("answerReference")) { 2831 this.answer = new Reference(); 2832 return this.answer; 2833 } 2834 else 2835 return super.addChild(name); 2836 } 2837 2838 public QuestionnaireItemEnableWhenComponent copy() { 2839 QuestionnaireItemEnableWhenComponent dst = new QuestionnaireItemEnableWhenComponent(); 2840 copyValues(dst); 2841 return dst; 2842 } 2843 2844 public void copyValues(QuestionnaireItemEnableWhenComponent dst) { 2845 super.copyValues(dst); 2846 dst.question = question == null ? null : question.copy(); 2847 dst.operator = operator == null ? null : operator.copy(); 2848 dst.answer = answer == null ? null : answer.copy(); 2849 } 2850 2851 @Override 2852 public boolean equalsDeep(Base other_) { 2853 if (!super.equalsDeep(other_)) 2854 return false; 2855 if (!(other_ instanceof QuestionnaireItemEnableWhenComponent)) 2856 return false; 2857 QuestionnaireItemEnableWhenComponent o = (QuestionnaireItemEnableWhenComponent) other_; 2858 return compareDeep(question, o.question, true) && compareDeep(operator, o.operator, true) && compareDeep(answer, o.answer, true) 2859 ; 2860 } 2861 2862 @Override 2863 public boolean equalsShallow(Base other_) { 2864 if (!super.equalsShallow(other_)) 2865 return false; 2866 if (!(other_ instanceof QuestionnaireItemEnableWhenComponent)) 2867 return false; 2868 QuestionnaireItemEnableWhenComponent o = (QuestionnaireItemEnableWhenComponent) other_; 2869 return compareValues(question, o.question, true) && compareValues(operator, o.operator, true); 2870 } 2871 2872 public boolean isEmpty() { 2873 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(question, operator, answer 2874 ); 2875 } 2876 2877 public String fhirType() { 2878 return "Questionnaire.item.enableWhen"; 2879 2880 } 2881 2882 } 2883 2884 @Block() 2885 public static class QuestionnaireItemAnswerOptionComponent extends BackboneElement implements IBaseBackboneElement { 2886 /** 2887 * A potential answer that's allowed as the answer to this question. 2888 */ 2889 @Child(name = "value", type = {IntegerType.class, DateType.class, TimeType.class, StringType.class, Coding.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 2890 @Description(shortDefinition="Answer value", formalDefinition="A potential answer that's allowed as the answer to this question." ) 2891 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 2892 protected DataType value; 2893 2894 /** 2895 * Indicates whether the answer value is selected when the list of possible answers is initially shown. 2896 */ 2897 @Child(name = "initialSelected", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2898 @Description(shortDefinition="Whether option is selected by default", formalDefinition="Indicates whether the answer value is selected when the list of possible answers is initially shown." ) 2899 protected BooleanType initialSelected; 2900 2901 private static final long serialVersionUID = -504460934L; 2902 2903 /** 2904 * Constructor 2905 */ 2906 public QuestionnaireItemAnswerOptionComponent() { 2907 super(); 2908 } 2909 2910 /** 2911 * Constructor 2912 */ 2913 public QuestionnaireItemAnswerOptionComponent(DataType value) { 2914 super(); 2915 this.setValue(value); 2916 } 2917 2918 /** 2919 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2920 */ 2921 public DataType getValue() { 2922 return this.value; 2923 } 2924 2925 /** 2926 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2927 */ 2928 public IntegerType getValueIntegerType() throws FHIRException { 2929 if (this.value == null) 2930 this.value = new IntegerType(); 2931 if (!(this.value instanceof IntegerType)) 2932 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2933 return (IntegerType) this.value; 2934 } 2935 2936 public boolean hasValueIntegerType() { 2937 return this.value instanceof IntegerType; 2938 } 2939 2940 /** 2941 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2942 */ 2943 public DateType getValueDateType() throws FHIRException { 2944 if (this.value == null) 2945 this.value = new DateType(); 2946 if (!(this.value instanceof DateType)) 2947 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 2948 return (DateType) this.value; 2949 } 2950 2951 public boolean hasValueDateType() { 2952 return this.value instanceof DateType; 2953 } 2954 2955 /** 2956 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2957 */ 2958 public TimeType getValueTimeType() throws FHIRException { 2959 if (this.value == null) 2960 this.value = new TimeType(); 2961 if (!(this.value instanceof TimeType)) 2962 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2963 return (TimeType) this.value; 2964 } 2965 2966 public boolean hasValueTimeType() { 2967 return this.value instanceof TimeType; 2968 } 2969 2970 /** 2971 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2972 */ 2973 public StringType getValueStringType() throws FHIRException { 2974 if (this.value == null) 2975 this.value = new StringType(); 2976 if (!(this.value instanceof StringType)) 2977 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2978 return (StringType) this.value; 2979 } 2980 2981 public boolean hasValueStringType() { 2982 return this.value instanceof StringType; 2983 } 2984 2985 /** 2986 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2987 */ 2988 public Coding getValueCoding() throws FHIRException { 2989 if (this.value == null) 2990 this.value = new Coding(); 2991 if (!(this.value instanceof Coding)) 2992 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 2993 return (Coding) this.value; 2994 } 2995 2996 public boolean hasValueCoding() { 2997 return this.value instanceof Coding; 2998 } 2999 3000 /** 3001 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 3002 */ 3003 public Reference getValueReference() throws FHIRException { 3004 if (this.value == null) 3005 this.value = new Reference(); 3006 if (!(this.value instanceof Reference)) 3007 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 3008 return (Reference) this.value; 3009 } 3010 3011 public boolean hasValueReference() { 3012 return this.value instanceof Reference; 3013 } 3014 3015 public boolean hasValue() { 3016 return this.value != null && !this.value.isEmpty(); 3017 } 3018 3019 /** 3020 * @param value {@link #value} (A potential answer that's allowed as the answer to this question.) 3021 */ 3022 public QuestionnaireItemAnswerOptionComponent setValue(DataType value) { 3023 if (value != null && !(value instanceof IntegerType || value instanceof DateType || value instanceof TimeType || value instanceof StringType || value instanceof Coding || value instanceof Reference)) 3024 throw new FHIRException("Not the right type for Questionnaire.item.answerOption.value[x]: "+value.fhirType()); 3025 this.value = value; 3026 return this; 3027 } 3028 3029 /** 3030 * @return {@link #initialSelected} (Indicates whether the answer value is selected when the list of possible answers is initially shown.). This is the underlying object with id, value and extensions. The accessor "getInitialSelected" gives direct access to the value 3031 */ 3032 public BooleanType getInitialSelectedElement() { 3033 if (this.initialSelected == null) 3034 if (Configuration.errorOnAutoCreate()) 3035 throw new Error("Attempt to auto-create QuestionnaireItemAnswerOptionComponent.initialSelected"); 3036 else if (Configuration.doAutoCreate()) 3037 this.initialSelected = new BooleanType(); // bb 3038 return this.initialSelected; 3039 } 3040 3041 public boolean hasInitialSelectedElement() { 3042 return this.initialSelected != null && !this.initialSelected.isEmpty(); 3043 } 3044 3045 public boolean hasInitialSelected() { 3046 return this.initialSelected != null && !this.initialSelected.isEmpty(); 3047 } 3048 3049 /** 3050 * @param value {@link #initialSelected} (Indicates whether the answer value is selected when the list of possible answers is initially shown.). This is the underlying object with id, value and extensions. The accessor "getInitialSelected" gives direct access to the value 3051 */ 3052 public QuestionnaireItemAnswerOptionComponent setInitialSelectedElement(BooleanType value) { 3053 this.initialSelected = value; 3054 return this; 3055 } 3056 3057 /** 3058 * @return Indicates whether the answer value is selected when the list of possible answers is initially shown. 3059 */ 3060 public boolean getInitialSelected() { 3061 return this.initialSelected == null || this.initialSelected.isEmpty() ? false : this.initialSelected.getValue(); 3062 } 3063 3064 /** 3065 * @param value Indicates whether the answer value is selected when the list of possible answers is initially shown. 3066 */ 3067 public QuestionnaireItemAnswerOptionComponent setInitialSelected(boolean value) { 3068 if (this.initialSelected == null) 3069 this.initialSelected = new BooleanType(); 3070 this.initialSelected.setValue(value); 3071 return this; 3072 } 3073 3074 protected void listChildren(List<Property> children) { 3075 super.listChildren(children); 3076 children.add(new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", "A potential answer that's allowed as the answer to this question.", 0, 1, value)); 3077 children.add(new Property("initialSelected", "boolean", "Indicates whether the answer value is selected when the list of possible answers is initially shown.", 0, 1, initialSelected)); 3078 } 3079 3080 @Override 3081 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3082 switch (_hash) { 3083 case -1410166417: /*value[x]*/ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3084 case 111972721: /*value*/ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3085 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3086 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3087 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3088 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3089 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3090 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3091 case -1310184961: /*initialSelected*/ return new Property("initialSelected", "boolean", "Indicates whether the answer value is selected when the list of possible answers is initially shown.", 0, 1, initialSelected); 3092 default: return super.getNamedProperty(_hash, _name, _checkValid); 3093 } 3094 3095 } 3096 3097 @Override 3098 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3099 switch (hash) { 3100 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3101 case -1310184961: /*initialSelected*/ return this.initialSelected == null ? new Base[0] : new Base[] {this.initialSelected}; // BooleanType 3102 default: return super.getProperty(hash, name, checkValid); 3103 } 3104 3105 } 3106 3107 @Override 3108 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3109 switch (hash) { 3110 case 111972721: // value 3111 this.value = TypeConvertor.castToType(value); // DataType 3112 return value; 3113 case -1310184961: // initialSelected 3114 this.initialSelected = TypeConvertor.castToBoolean(value); // BooleanType 3115 return value; 3116 default: return super.setProperty(hash, name, value); 3117 } 3118 3119 } 3120 3121 @Override 3122 public Base setProperty(String name, Base value) throws FHIRException { 3123 if (name.equals("value[x]")) { 3124 this.value = TypeConvertor.castToType(value); // DataType 3125 } else if (name.equals("initialSelected")) { 3126 this.initialSelected = TypeConvertor.castToBoolean(value); // BooleanType 3127 } else 3128 return super.setProperty(name, value); 3129 return value; 3130 } 3131 3132 @Override 3133 public void removeChild(String name, Base value) throws FHIRException { 3134 if (name.equals("value[x]")) { 3135 this.value = null; 3136 } else if (name.equals("initialSelected")) { 3137 this.initialSelected = null; 3138 } else 3139 super.removeChild(name, value); 3140 3141 } 3142 3143 @Override 3144 public Base makeProperty(int hash, String name) throws FHIRException { 3145 switch (hash) { 3146 case -1410166417: return getValue(); 3147 case 111972721: return getValue(); 3148 case -1310184961: return getInitialSelectedElement(); 3149 default: return super.makeProperty(hash, name); 3150 } 3151 3152 } 3153 3154 @Override 3155 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3156 switch (hash) { 3157 case 111972721: /*value*/ return new String[] {"integer", "date", "time", "string", "Coding", "Reference"}; 3158 case -1310184961: /*initialSelected*/ return new String[] {"boolean"}; 3159 default: return super.getTypesForProperty(hash, name); 3160 } 3161 3162 } 3163 3164 @Override 3165 public Base addChild(String name) throws FHIRException { 3166 if (name.equals("valueInteger")) { 3167 this.value = new IntegerType(); 3168 return this.value; 3169 } 3170 else if (name.equals("valueDate")) { 3171 this.value = new DateType(); 3172 return this.value; 3173 } 3174 else if (name.equals("valueTime")) { 3175 this.value = new TimeType(); 3176 return this.value; 3177 } 3178 else if (name.equals("valueString")) { 3179 this.value = new StringType(); 3180 return this.value; 3181 } 3182 else if (name.equals("valueCoding")) { 3183 this.value = new Coding(); 3184 return this.value; 3185 } 3186 else if (name.equals("valueReference")) { 3187 this.value = new Reference(); 3188 return this.value; 3189 } 3190 else if (name.equals("initialSelected")) { 3191 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.item.answerOption.initialSelected"); 3192 } 3193 else 3194 return super.addChild(name); 3195 } 3196 3197 public QuestionnaireItemAnswerOptionComponent copy() { 3198 QuestionnaireItemAnswerOptionComponent dst = new QuestionnaireItemAnswerOptionComponent(); 3199 copyValues(dst); 3200 return dst; 3201 } 3202 3203 public void copyValues(QuestionnaireItemAnswerOptionComponent dst) { 3204 super.copyValues(dst); 3205 dst.value = value == null ? null : value.copy(); 3206 dst.initialSelected = initialSelected == null ? null : initialSelected.copy(); 3207 } 3208 3209 @Override 3210 public boolean equalsDeep(Base other_) { 3211 if (!super.equalsDeep(other_)) 3212 return false; 3213 if (!(other_ instanceof QuestionnaireItemAnswerOptionComponent)) 3214 return false; 3215 QuestionnaireItemAnswerOptionComponent o = (QuestionnaireItemAnswerOptionComponent) other_; 3216 return compareDeep(value, o.value, true) && compareDeep(initialSelected, o.initialSelected, true) 3217 ; 3218 } 3219 3220 @Override 3221 public boolean equalsShallow(Base other_) { 3222 if (!super.equalsShallow(other_)) 3223 return false; 3224 if (!(other_ instanceof QuestionnaireItemAnswerOptionComponent)) 3225 return false; 3226 QuestionnaireItemAnswerOptionComponent o = (QuestionnaireItemAnswerOptionComponent) other_; 3227 return compareValues(initialSelected, o.initialSelected, true); 3228 } 3229 3230 public boolean isEmpty() { 3231 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, initialSelected); 3232 } 3233 3234 public String fhirType() { 3235 return "Questionnaire.item.answerOption"; 3236 3237 } 3238 3239 } 3240 3241 @Block() 3242 public static class QuestionnaireItemInitialComponent extends BackboneElement implements IBaseBackboneElement { 3243 /** 3244 * The actual value to for an initial answer. 3245 */ 3246 @Child(name = "value", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, Quantity.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 3247 @Description(shortDefinition="Actual value for initializing the question", formalDefinition="The actual value to for an initial answer." ) 3248 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 3249 protected DataType value; 3250 3251 private static final long serialVersionUID = -1135414639L; 3252 3253 /** 3254 * Constructor 3255 */ 3256 public QuestionnaireItemInitialComponent() { 3257 super(); 3258 } 3259 3260 /** 3261 * Constructor 3262 */ 3263 public QuestionnaireItemInitialComponent(DataType value) { 3264 super(); 3265 this.setValue(value); 3266 } 3267 3268 /** 3269 * @return {@link #value} (The actual value to for an initial answer.) 3270 */ 3271 public DataType getValue() { 3272 return this.value; 3273 } 3274 3275 /** 3276 * @return {@link #value} (The actual value to for an initial answer.) 3277 */ 3278 public BooleanType getValueBooleanType() throws FHIRException { 3279 if (this.value == null) 3280 this.value = new BooleanType(); 3281 if (!(this.value instanceof BooleanType)) 3282 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 3283 return (BooleanType) this.value; 3284 } 3285 3286 public boolean hasValueBooleanType() { 3287 return this.value instanceof BooleanType; 3288 } 3289 3290 /** 3291 * @return {@link #value} (The actual value to for an initial answer.) 3292 */ 3293 public DecimalType getValueDecimalType() throws FHIRException { 3294 if (this.value == null) 3295 this.value = new DecimalType(); 3296 if (!(this.value instanceof DecimalType)) 3297 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 3298 return (DecimalType) this.value; 3299 } 3300 3301 public boolean hasValueDecimalType() { 3302 return this.value instanceof DecimalType; 3303 } 3304 3305 /** 3306 * @return {@link #value} (The actual value to for an initial answer.) 3307 */ 3308 public IntegerType getValueIntegerType() throws FHIRException { 3309 if (this.value == null) 3310 this.value = new IntegerType(); 3311 if (!(this.value instanceof IntegerType)) 3312 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 3313 return (IntegerType) this.value; 3314 } 3315 3316 public boolean hasValueIntegerType() { 3317 return this.value instanceof IntegerType; 3318 } 3319 3320 /** 3321 * @return {@link #value} (The actual value to for an initial answer.) 3322 */ 3323 public DateType getValueDateType() throws FHIRException { 3324 if (this.value == null) 3325 this.value = new DateType(); 3326 if (!(this.value instanceof DateType)) 3327 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 3328 return (DateType) this.value; 3329 } 3330 3331 public boolean hasValueDateType() { 3332 return this.value instanceof DateType; 3333 } 3334 3335 /** 3336 * @return {@link #value} (The actual value to for an initial answer.) 3337 */ 3338 public DateTimeType getValueDateTimeType() throws FHIRException { 3339 if (this.value == null) 3340 this.value = new DateTimeType(); 3341 if (!(this.value instanceof DateTimeType)) 3342 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3343 return (DateTimeType) this.value; 3344 } 3345 3346 public boolean hasValueDateTimeType() { 3347 return this.value instanceof DateTimeType; 3348 } 3349 3350 /** 3351 * @return {@link #value} (The actual value to for an initial answer.) 3352 */ 3353 public TimeType getValueTimeType() throws FHIRException { 3354 if (this.value == null) 3355 this.value = new TimeType(); 3356 if (!(this.value instanceof TimeType)) 3357 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3358 return (TimeType) this.value; 3359 } 3360 3361 public boolean hasValueTimeType() { 3362 return this.value instanceof TimeType; 3363 } 3364 3365 /** 3366 * @return {@link #value} (The actual value to for an initial answer.) 3367 */ 3368 public StringType getValueStringType() throws FHIRException { 3369 if (this.value == null) 3370 this.value = new StringType(); 3371 if (!(this.value instanceof StringType)) 3372 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 3373 return (StringType) this.value; 3374 } 3375 3376 public boolean hasValueStringType() { 3377 return this.value instanceof StringType; 3378 } 3379 3380 /** 3381 * @return {@link #value} (The actual value to for an initial answer.) 3382 */ 3383 public UriType getValueUriType() throws FHIRException { 3384 if (this.value == null) 3385 this.value = new UriType(); 3386 if (!(this.value instanceof UriType)) 3387 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 3388 return (UriType) this.value; 3389 } 3390 3391 public boolean hasValueUriType() { 3392 return this.value instanceof UriType; 3393 } 3394 3395 /** 3396 * @return {@link #value} (The actual value to for an initial answer.) 3397 */ 3398 public Attachment getValueAttachment() throws FHIRException { 3399 if (this.value == null) 3400 this.value = new Attachment(); 3401 if (!(this.value instanceof Attachment)) 3402 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 3403 return (Attachment) this.value; 3404 } 3405 3406 public boolean hasValueAttachment() { 3407 return this.value instanceof Attachment; 3408 } 3409 3410 /** 3411 * @return {@link #value} (The actual value to for an initial answer.) 3412 */ 3413 public Coding getValueCoding() throws FHIRException { 3414 if (this.value == null) 3415 this.value = new Coding(); 3416 if (!(this.value instanceof Coding)) 3417 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 3418 return (Coding) this.value; 3419 } 3420 3421 public boolean hasValueCoding() { 3422 return this.value instanceof Coding; 3423 } 3424 3425 /** 3426 * @return {@link #value} (The actual value to for an initial answer.) 3427 */ 3428 public Quantity getValueQuantity() throws FHIRException { 3429 if (this.value == null) 3430 this.value = new Quantity(); 3431 if (!(this.value instanceof Quantity)) 3432 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3433 return (Quantity) this.value; 3434 } 3435 3436 public boolean hasValueQuantity() { 3437 return this.value instanceof Quantity; 3438 } 3439 3440 /** 3441 * @return {@link #value} (The actual value to for an initial answer.) 3442 */ 3443 public Reference getValueReference() throws FHIRException { 3444 if (this.value == null) 3445 this.value = new Reference(); 3446 if (!(this.value instanceof Reference)) 3447 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 3448 return (Reference) this.value; 3449 } 3450 3451 public boolean hasValueReference() { 3452 return this.value instanceof Reference; 3453 } 3454 3455 public boolean hasValue() { 3456 return this.value != null && !this.value.isEmpty(); 3457 } 3458 3459 /** 3460 * @param value {@link #value} (The actual value to for an initial answer.) 3461 */ 3462 public QuestionnaireItemInitialComponent setValue(DataType value) { 3463 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof UriType || value instanceof Attachment || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 3464 throw new FHIRException("Not the right type for Questionnaire.item.initial.value[x]: "+value.fhirType()); 3465 this.value = value; 3466 return this; 3467 } 3468 3469 protected void listChildren(List<Property> children) { 3470 super.listChildren(children); 3471 children.add(new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The actual value to for an initial answer.", 0, 1, value)); 3472 } 3473 3474 @Override 3475 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3476 switch (_hash) { 3477 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The actual value to for an initial answer.", 0, 1, value); 3478 case 111972721: /*value*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The actual value to for an initial answer.", 0, 1, value); 3479 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The actual value to for an initial answer.", 0, 1, value); 3480 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The actual value to for an initial answer.", 0, 1, value); 3481 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The actual value to for an initial answer.", 0, 1, value); 3482 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The actual value to for an initial answer.", 0, 1, value); 3483 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The actual value to for an initial answer.", 0, 1, value); 3484 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The actual value to for an initial answer.", 0, 1, value); 3485 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The actual value to for an initial answer.", 0, 1, value); 3486 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The actual value to for an initial answer.", 0, 1, value); 3487 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The actual value to for an initial answer.", 0, 1, value); 3488 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The actual value to for an initial answer.", 0, 1, value); 3489 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The actual value to for an initial answer.", 0, 1, value); 3490 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "The actual value to for an initial answer.", 0, 1, value); 3491 default: return super.getNamedProperty(_hash, _name, _checkValid); 3492 } 3493 3494 } 3495 3496 @Override 3497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3498 switch (hash) { 3499 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3500 default: return super.getProperty(hash, name, checkValid); 3501 } 3502 3503 } 3504 3505 @Override 3506 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3507 switch (hash) { 3508 case 111972721: // value 3509 this.value = TypeConvertor.castToType(value); // DataType 3510 return value; 3511 default: return super.setProperty(hash, name, value); 3512 } 3513 3514 } 3515 3516 @Override 3517 public Base setProperty(String name, Base value) throws FHIRException { 3518 if (name.equals("value[x]")) { 3519 this.value = TypeConvertor.castToType(value); // DataType 3520 } else 3521 return super.setProperty(name, value); 3522 return value; 3523 } 3524 3525 @Override 3526 public void removeChild(String name, Base value) throws FHIRException { 3527 if (name.equals("value[x]")) { 3528 this.value = null; 3529 } else 3530 super.removeChild(name, value); 3531 3532 } 3533 3534 @Override 3535 public Base makeProperty(int hash, String name) throws FHIRException { 3536 switch (hash) { 3537 case -1410166417: return getValue(); 3538 case 111972721: return getValue(); 3539 default: return super.makeProperty(hash, name); 3540 } 3541 3542 } 3543 3544 @Override 3545 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3546 switch (hash) { 3547 case 111972721: /*value*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", "Attachment", "Coding", "Quantity", "Reference"}; 3548 default: return super.getTypesForProperty(hash, name); 3549 } 3550 3551 } 3552 3553 @Override 3554 public Base addChild(String name) throws FHIRException { 3555 if (name.equals("valueBoolean")) { 3556 this.value = new BooleanType(); 3557 return this.value; 3558 } 3559 else if (name.equals("valueDecimal")) { 3560 this.value = new DecimalType(); 3561 return this.value; 3562 } 3563 else if (name.equals("valueInteger")) { 3564 this.value = new IntegerType(); 3565 return this.value; 3566 } 3567 else if (name.equals("valueDate")) { 3568 this.value = new DateType(); 3569 return this.value; 3570 } 3571 else if (name.equals("valueDateTime")) { 3572 this.value = new DateTimeType(); 3573 return this.value; 3574 } 3575 else if (name.equals("valueTime")) { 3576 this.value = new TimeType(); 3577 return this.value; 3578 } 3579 else if (name.equals("valueString")) { 3580 this.value = new StringType(); 3581 return this.value; 3582 } 3583 else if (name.equals("valueUri")) { 3584 this.value = new UriType(); 3585 return this.value; 3586 } 3587 else if (name.equals("valueAttachment")) { 3588 this.value = new Attachment(); 3589 return this.value; 3590 } 3591 else if (name.equals("valueCoding")) { 3592 this.value = new Coding(); 3593 return this.value; 3594 } 3595 else if (name.equals("valueQuantity")) { 3596 this.value = new Quantity(); 3597 return this.value; 3598 } 3599 else if (name.equals("valueReference")) { 3600 this.value = new Reference(); 3601 return this.value; 3602 } 3603 else 3604 return super.addChild(name); 3605 } 3606 3607 public QuestionnaireItemInitialComponent copy() { 3608 QuestionnaireItemInitialComponent dst = new QuestionnaireItemInitialComponent(); 3609 copyValues(dst); 3610 return dst; 3611 } 3612 3613 public void copyValues(QuestionnaireItemInitialComponent dst) { 3614 super.copyValues(dst); 3615 dst.value = value == null ? null : value.copy(); 3616 } 3617 3618 @Override 3619 public boolean equalsDeep(Base other_) { 3620 if (!super.equalsDeep(other_)) 3621 return false; 3622 if (!(other_ instanceof QuestionnaireItemInitialComponent)) 3623 return false; 3624 QuestionnaireItemInitialComponent o = (QuestionnaireItemInitialComponent) other_; 3625 return compareDeep(value, o.value, true); 3626 } 3627 3628 @Override 3629 public boolean equalsShallow(Base other_) { 3630 if (!super.equalsShallow(other_)) 3631 return false; 3632 if (!(other_ instanceof QuestionnaireItemInitialComponent)) 3633 return false; 3634 QuestionnaireItemInitialComponent o = (QuestionnaireItemInitialComponent) other_; 3635 return true; 3636 } 3637 3638 public boolean isEmpty() { 3639 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 3640 } 3641 3642 public String fhirType() { 3643 return "Questionnaire.item.initial"; 3644 3645 } 3646 3647 } 3648 3649 /** 3650 * An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers. 3651 */ 3652 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 3653 @Description(shortDefinition="Canonical identifier for this questionnaire, represented as an absolute URI (globally unique)", formalDefinition="An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers." ) 3654 protected UriType url; 3655 3656 /** 3657 * A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance. 3658 */ 3659 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3660 @Description(shortDefinition="Business identifier for questionnaire", formalDefinition="A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 3661 protected List<Identifier> identifier; 3662 3663 /** 3664 * The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3665 */ 3666 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3667 @Description(shortDefinition="Business version of the questionnaire", formalDefinition="The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 3668 protected StringType version; 3669 3670 /** 3671 * Indicates the mechanism used to compare versions to determine which is more current. 3672 */ 3673 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 3674 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 3675 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 3676 protected DataType versionAlgorithm; 3677 3678 /** 3679 * A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3680 */ 3681 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3682 @Description(shortDefinition="Name for this questionnaire (computer friendly)", formalDefinition="A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 3683 protected StringType name; 3684 3685 /** 3686 * A short, descriptive, user-friendly title for the questionnaire. 3687 */ 3688 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3689 @Description(shortDefinition="Name for this questionnaire (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the questionnaire." ) 3690 protected StringType title; 3691 3692 /** 3693 * The URL of a Questionnaire that this Questionnaire is based on. 3694 */ 3695 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3696 @Description(shortDefinition="Based on Questionnaire", formalDefinition="The URL of a Questionnaire that this Questionnaire is based on." ) 3697 protected List<CanonicalType> derivedFrom; 3698 3699 /** 3700 * The current state of this questionnaire. 3701 */ 3702 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 3703 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of this questionnaire." ) 3704 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 3705 protected Enumeration<PublicationStatus> status; 3706 3707 /** 3708 * A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 3709 */ 3710 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 3711 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage." ) 3712 protected BooleanType experimental; 3713 3714 /** 3715 * The types of subjects that can be the subject of responses created for the questionnaire. 3716 */ 3717 @Child(name = "subjectType", type = {CodeType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3718 @Description(shortDefinition="Resource that can be subject of QuestionnaireResponse", formalDefinition="The types of subjects that can be the subject of responses created for the questionnaire." ) 3719 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 3720 protected List<CodeType> subjectType; 3721 3722 /** 3723 * The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes. 3724 */ 3725 @Child(name = "date", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3726 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes." ) 3727 protected DateTimeType date; 3728 3729 /** 3730 * The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire. 3731 */ 3732 @Child(name = "publisher", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 3733 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire." ) 3734 protected StringType publisher; 3735 3736 /** 3737 * Contact details to assist a user in finding and communicating with the publisher. 3738 */ 3739 @Child(name = "contact", type = {ContactDetail.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3740 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 3741 protected List<ContactDetail> contact; 3742 3743 /** 3744 * A free text natural language description of the questionnaire from a consumer's perspective. 3745 */ 3746 @Child(name = "description", type = {MarkdownType.class}, order=13, min=0, max=1, modifier=false, summary=true) 3747 @Description(shortDefinition="Natural language description of the questionnaire", formalDefinition="A free text natural language description of the questionnaire from a consumer's perspective." ) 3748 protected MarkdownType description; 3749 3750 /** 3751 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate questionnaires. 3752 */ 3753 @Child(name = "useContext", type = {UsageContext.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3754 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate questionnaires." ) 3755 protected List<UsageContext> useContext; 3756 3757 /** 3758 * A legal or geographic region in which the questionnaire is intended to be used. 3759 */ 3760 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3761 @Description(shortDefinition="Intended jurisdiction for questionnaire (if applicable)", formalDefinition="A legal or geographic region in which the questionnaire is intended to be used." ) 3762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 3763 protected List<CodeableConcept> jurisdiction; 3764 3765 /** 3766 * Explanation of why this questionnaire is needed and why it has been designed as it has. 3767 */ 3768 @Child(name = "purpose", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 3769 @Description(shortDefinition="Why this questionnaire is defined", formalDefinition="Explanation of why this questionnaire is needed and why it has been designed as it has." ) 3770 protected MarkdownType purpose; 3771 3772 /** 3773 * A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire. 3774 */ 3775 @Child(name = "copyright", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 3776 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire." ) 3777 protected MarkdownType copyright; 3778 3779 /** 3780 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3781 */ 3782 @Child(name = "copyrightLabel", type = {StringType.class}, order=18, min=0, max=1, modifier=false, summary=false) 3783 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 3784 protected StringType copyrightLabel; 3785 3786 /** 3787 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3788 */ 3789 @Child(name = "approvalDate", type = {DateType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3790 @Description(shortDefinition="When the questionnaire was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 3791 protected DateType approvalDate; 3792 3793 /** 3794 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3795 */ 3796 @Child(name = "lastReviewDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=false) 3797 @Description(shortDefinition="When the questionnaire was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 3798 protected DateType lastReviewDate; 3799 3800 /** 3801 * The period during which the questionnaire content was or is planned to be in active use. 3802 */ 3803 @Child(name = "effectivePeriod", type = {Period.class}, order=21, min=0, max=1, modifier=false, summary=true) 3804 @Description(shortDefinition="When the questionnaire is expected to be used", formalDefinition="The period during which the questionnaire content was or is planned to be in active use." ) 3805 protected Period effectivePeriod; 3806 3807 /** 3808 * An identifier for this collection of questions in a particular terminology such as LOINC. 3809 */ 3810 @Child(name = "code", type = {Coding.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3811 @Description(shortDefinition="Concept that represents the overall questionnaire", formalDefinition="An identifier for this collection of questions in a particular terminology such as LOINC." ) 3812 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-questions") 3813 protected List<Coding> code; 3814 3815 /** 3816 * A particular question, question grouping or display text that is part of the questionnaire. 3817 */ 3818 @Child(name = "item", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3819 @Description(shortDefinition="Questions and sections within the Questionnaire", formalDefinition="A particular question, question grouping or display text that is part of the questionnaire." ) 3820 protected List<QuestionnaireItemComponent> item; 3821 3822 private static final long serialVersionUID = -863684953L; 3823 3824 /** 3825 * Constructor 3826 */ 3827 public Questionnaire() { 3828 super(); 3829 } 3830 3831 /** 3832 * Constructor 3833 */ 3834 public Questionnaire(PublicationStatus status) { 3835 super(); 3836 this.setStatus(status); 3837 } 3838 3839 /** 3840 * @return {@link #url} (An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3841 */ 3842 public UriType getUrlElement() { 3843 if (this.url == null) 3844 if (Configuration.errorOnAutoCreate()) 3845 throw new Error("Attempt to auto-create Questionnaire.url"); 3846 else if (Configuration.doAutoCreate()) 3847 this.url = new UriType(); // bb 3848 return this.url; 3849 } 3850 3851 public boolean hasUrlElement() { 3852 return this.url != null && !this.url.isEmpty(); 3853 } 3854 3855 public boolean hasUrl() { 3856 return this.url != null && !this.url.isEmpty(); 3857 } 3858 3859 /** 3860 * @param value {@link #url} (An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3861 */ 3862 public Questionnaire setUrlElement(UriType value) { 3863 this.url = value; 3864 return this; 3865 } 3866 3867 /** 3868 * @return An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers. 3869 */ 3870 public String getUrl() { 3871 return this.url == null ? null : this.url.getValue(); 3872 } 3873 3874 /** 3875 * @param value An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers. 3876 */ 3877 public Questionnaire setUrl(String value) { 3878 if (Utilities.noString(value)) 3879 this.url = null; 3880 else { 3881 if (this.url == null) 3882 this.url = new UriType(); 3883 this.url.setValue(value); 3884 } 3885 return this; 3886 } 3887 3888 /** 3889 * @return {@link #identifier} (A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.) 3890 */ 3891 public List<Identifier> getIdentifier() { 3892 if (this.identifier == null) 3893 this.identifier = new ArrayList<Identifier>(); 3894 return this.identifier; 3895 } 3896 3897 /** 3898 * @return Returns a reference to <code>this</code> for easy method chaining 3899 */ 3900 public Questionnaire setIdentifier(List<Identifier> theIdentifier) { 3901 this.identifier = theIdentifier; 3902 return this; 3903 } 3904 3905 public boolean hasIdentifier() { 3906 if (this.identifier == null) 3907 return false; 3908 for (Identifier item : this.identifier) 3909 if (!item.isEmpty()) 3910 return true; 3911 return false; 3912 } 3913 3914 public Identifier addIdentifier() { //3 3915 Identifier t = new Identifier(); 3916 if (this.identifier == null) 3917 this.identifier = new ArrayList<Identifier>(); 3918 this.identifier.add(t); 3919 return t; 3920 } 3921 3922 public Questionnaire addIdentifier(Identifier t) { //3 3923 if (t == null) 3924 return this; 3925 if (this.identifier == null) 3926 this.identifier = new ArrayList<Identifier>(); 3927 this.identifier.add(t); 3928 return this; 3929 } 3930 3931 /** 3932 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3933 */ 3934 public Identifier getIdentifierFirstRep() { 3935 if (getIdentifier().isEmpty()) { 3936 addIdentifier(); 3937 } 3938 return getIdentifier().get(0); 3939 } 3940 3941 /** 3942 * @return {@link #version} (The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3943 */ 3944 public StringType getVersionElement() { 3945 if (this.version == null) 3946 if (Configuration.errorOnAutoCreate()) 3947 throw new Error("Attempt to auto-create Questionnaire.version"); 3948 else if (Configuration.doAutoCreate()) 3949 this.version = new StringType(); // bb 3950 return this.version; 3951 } 3952 3953 public boolean hasVersionElement() { 3954 return this.version != null && !this.version.isEmpty(); 3955 } 3956 3957 public boolean hasVersion() { 3958 return this.version != null && !this.version.isEmpty(); 3959 } 3960 3961 /** 3962 * @param value {@link #version} (The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3963 */ 3964 public Questionnaire setVersionElement(StringType value) { 3965 this.version = value; 3966 return this; 3967 } 3968 3969 /** 3970 * @return The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3971 */ 3972 public String getVersion() { 3973 return this.version == null ? null : this.version.getValue(); 3974 } 3975 3976 /** 3977 * @param value The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3978 */ 3979 public Questionnaire setVersion(String value) { 3980 if (Utilities.noString(value)) 3981 this.version = null; 3982 else { 3983 if (this.version == null) 3984 this.version = new StringType(); 3985 this.version.setValue(value); 3986 } 3987 return this; 3988 } 3989 3990 /** 3991 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3992 */ 3993 public DataType getVersionAlgorithm() { 3994 return this.versionAlgorithm; 3995 } 3996 3997 /** 3998 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3999 */ 4000 public StringType getVersionAlgorithmStringType() throws FHIRException { 4001 if (this.versionAlgorithm == null) 4002 this.versionAlgorithm = new StringType(); 4003 if (!(this.versionAlgorithm instanceof StringType)) 4004 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4005 return (StringType) this.versionAlgorithm; 4006 } 4007 4008 public boolean hasVersionAlgorithmStringType() { 4009 return this.versionAlgorithm instanceof StringType; 4010 } 4011 4012 /** 4013 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4014 */ 4015 public Coding getVersionAlgorithmCoding() throws FHIRException { 4016 if (this.versionAlgorithm == null) 4017 this.versionAlgorithm = new Coding(); 4018 if (!(this.versionAlgorithm instanceof Coding)) 4019 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4020 return (Coding) this.versionAlgorithm; 4021 } 4022 4023 public boolean hasVersionAlgorithmCoding() { 4024 return this.versionAlgorithm instanceof Coding; 4025 } 4026 4027 public boolean hasVersionAlgorithm() { 4028 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 4029 } 4030 4031 /** 4032 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4033 */ 4034 public Questionnaire setVersionAlgorithm(DataType value) { 4035 if (value != null && !(value instanceof StringType || value instanceof Coding)) 4036 throw new FHIRException("Not the right type for Questionnaire.versionAlgorithm[x]: "+value.fhirType()); 4037 this.versionAlgorithm = value; 4038 return this; 4039 } 4040 4041 /** 4042 * @return {@link #name} (A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4043 */ 4044 public StringType getNameElement() { 4045 if (this.name == null) 4046 if (Configuration.errorOnAutoCreate()) 4047 throw new Error("Attempt to auto-create Questionnaire.name"); 4048 else if (Configuration.doAutoCreate()) 4049 this.name = new StringType(); // bb 4050 return this.name; 4051 } 4052 4053 public boolean hasNameElement() { 4054 return this.name != null && !this.name.isEmpty(); 4055 } 4056 4057 public boolean hasName() { 4058 return this.name != null && !this.name.isEmpty(); 4059 } 4060 4061 /** 4062 * @param value {@link #name} (A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4063 */ 4064 public Questionnaire setNameElement(StringType value) { 4065 this.name = value; 4066 return this; 4067 } 4068 4069 /** 4070 * @return A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation. 4071 */ 4072 public String getName() { 4073 return this.name == null ? null : this.name.getValue(); 4074 } 4075 4076 /** 4077 * @param value A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation. 4078 */ 4079 public Questionnaire setName(String value) { 4080 if (Utilities.noString(value)) 4081 this.name = null; 4082 else { 4083 if (this.name == null) 4084 this.name = new StringType(); 4085 this.name.setValue(value); 4086 } 4087 return this; 4088 } 4089 4090 /** 4091 * @return {@link #title} (A short, descriptive, user-friendly title for the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4092 */ 4093 public StringType getTitleElement() { 4094 if (this.title == null) 4095 if (Configuration.errorOnAutoCreate()) 4096 throw new Error("Attempt to auto-create Questionnaire.title"); 4097 else if (Configuration.doAutoCreate()) 4098 this.title = new StringType(); // bb 4099 return this.title; 4100 } 4101 4102 public boolean hasTitleElement() { 4103 return this.title != null && !this.title.isEmpty(); 4104 } 4105 4106 public boolean hasTitle() { 4107 return this.title != null && !this.title.isEmpty(); 4108 } 4109 4110 /** 4111 * @param value {@link #title} (A short, descriptive, user-friendly title for the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4112 */ 4113 public Questionnaire setTitleElement(StringType value) { 4114 this.title = value; 4115 return this; 4116 } 4117 4118 /** 4119 * @return A short, descriptive, user-friendly title for the questionnaire. 4120 */ 4121 public String getTitle() { 4122 return this.title == null ? null : this.title.getValue(); 4123 } 4124 4125 /** 4126 * @param value A short, descriptive, user-friendly title for the questionnaire. 4127 */ 4128 public Questionnaire setTitle(String value) { 4129 if (Utilities.noString(value)) 4130 this.title = null; 4131 else { 4132 if (this.title == null) 4133 this.title = new StringType(); 4134 this.title.setValue(value); 4135 } 4136 return this; 4137 } 4138 4139 /** 4140 * @return {@link #derivedFrom} (The URL of a Questionnaire that this Questionnaire is based on.) 4141 */ 4142 public List<CanonicalType> getDerivedFrom() { 4143 if (this.derivedFrom == null) 4144 this.derivedFrom = new ArrayList<CanonicalType>(); 4145 return this.derivedFrom; 4146 } 4147 4148 /** 4149 * @return Returns a reference to <code>this</code> for easy method chaining 4150 */ 4151 public Questionnaire setDerivedFrom(List<CanonicalType> theDerivedFrom) { 4152 this.derivedFrom = theDerivedFrom; 4153 return this; 4154 } 4155 4156 public boolean hasDerivedFrom() { 4157 if (this.derivedFrom == null) 4158 return false; 4159 for (CanonicalType item : this.derivedFrom) 4160 if (!item.isEmpty()) 4161 return true; 4162 return false; 4163 } 4164 4165 /** 4166 * @return {@link #derivedFrom} (The URL of a Questionnaire that this Questionnaire is based on.) 4167 */ 4168 public CanonicalType addDerivedFromElement() {//2 4169 CanonicalType t = new CanonicalType(); 4170 if (this.derivedFrom == null) 4171 this.derivedFrom = new ArrayList<CanonicalType>(); 4172 this.derivedFrom.add(t); 4173 return t; 4174 } 4175 4176 /** 4177 * @param value {@link #derivedFrom} (The URL of a Questionnaire that this Questionnaire is based on.) 4178 */ 4179 public Questionnaire addDerivedFrom(String value) { //1 4180 CanonicalType t = new CanonicalType(); 4181 t.setValue(value); 4182 if (this.derivedFrom == null) 4183 this.derivedFrom = new ArrayList<CanonicalType>(); 4184 this.derivedFrom.add(t); 4185 return this; 4186 } 4187 4188 /** 4189 * @param value {@link #derivedFrom} (The URL of a Questionnaire that this Questionnaire is based on.) 4190 */ 4191 public boolean hasDerivedFrom(String value) { 4192 if (this.derivedFrom == null) 4193 return false; 4194 for (CanonicalType v : this.derivedFrom) 4195 if (v.getValue().equals(value)) // canonical 4196 return true; 4197 return false; 4198 } 4199 4200 /** 4201 * @return {@link #status} (The current state of this questionnaire.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4202 */ 4203 public Enumeration<PublicationStatus> getStatusElement() { 4204 if (this.status == null) 4205 if (Configuration.errorOnAutoCreate()) 4206 throw new Error("Attempt to auto-create Questionnaire.status"); 4207 else if (Configuration.doAutoCreate()) 4208 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 4209 return this.status; 4210 } 4211 4212 public boolean hasStatusElement() { 4213 return this.status != null && !this.status.isEmpty(); 4214 } 4215 4216 public boolean hasStatus() { 4217 return this.status != null && !this.status.isEmpty(); 4218 } 4219 4220 /** 4221 * @param value {@link #status} (The current state of this questionnaire.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4222 */ 4223 public Questionnaire setStatusElement(Enumeration<PublicationStatus> value) { 4224 this.status = value; 4225 return this; 4226 } 4227 4228 /** 4229 * @return The current state of this questionnaire. 4230 */ 4231 public PublicationStatus getStatus() { 4232 return this.status == null ? null : this.status.getValue(); 4233 } 4234 4235 /** 4236 * @param value The current state of this questionnaire. 4237 */ 4238 public Questionnaire setStatus(PublicationStatus value) { 4239 if (this.status == null) 4240 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 4241 this.status.setValue(value); 4242 return this; 4243 } 4244 4245 /** 4246 * @return {@link #experimental} (A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4247 */ 4248 public BooleanType getExperimentalElement() { 4249 if (this.experimental == null) 4250 if (Configuration.errorOnAutoCreate()) 4251 throw new Error("Attempt to auto-create Questionnaire.experimental"); 4252 else if (Configuration.doAutoCreate()) 4253 this.experimental = new BooleanType(); // bb 4254 return this.experimental; 4255 } 4256 4257 public boolean hasExperimentalElement() { 4258 return this.experimental != null && !this.experimental.isEmpty(); 4259 } 4260 4261 public boolean hasExperimental() { 4262 return this.experimental != null && !this.experimental.isEmpty(); 4263 } 4264 4265 /** 4266 * @param value {@link #experimental} (A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4267 */ 4268 public Questionnaire setExperimentalElement(BooleanType value) { 4269 this.experimental = value; 4270 return this; 4271 } 4272 4273 /** 4274 * @return A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 4275 */ 4276 public boolean getExperimental() { 4277 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4278 } 4279 4280 /** 4281 * @param value A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 4282 */ 4283 public Questionnaire setExperimental(boolean value) { 4284 if (this.experimental == null) 4285 this.experimental = new BooleanType(); 4286 this.experimental.setValue(value); 4287 return this; 4288 } 4289 4290 /** 4291 * @return {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 4292 */ 4293 public List<CodeType> getSubjectType() { 4294 if (this.subjectType == null) 4295 this.subjectType = new ArrayList<CodeType>(); 4296 return this.subjectType; 4297 } 4298 4299 /** 4300 * @return Returns a reference to <code>this</code> for easy method chaining 4301 */ 4302 public Questionnaire setSubjectType(List<CodeType> theSubjectType) { 4303 this.subjectType = theSubjectType; 4304 return this; 4305 } 4306 4307 public boolean hasSubjectType() { 4308 if (this.subjectType == null) 4309 return false; 4310 for (CodeType item : this.subjectType) 4311 if (!item.isEmpty()) 4312 return true; 4313 return false; 4314 } 4315 4316 /** 4317 * @return {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 4318 */ 4319 public CodeType addSubjectTypeElement() {//2 4320 CodeType t = new CodeType(); 4321 if (this.subjectType == null) 4322 this.subjectType = new ArrayList<CodeType>(); 4323 this.subjectType.add(t); 4324 return t; 4325 } 4326 4327 /** 4328 * @param value {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 4329 */ 4330 public Questionnaire addSubjectType(String value) { //1 4331 CodeType t = new CodeType(); 4332 t.setValue(value); 4333 if (this.subjectType == null) 4334 this.subjectType = new ArrayList<CodeType>(); 4335 this.subjectType.add(t); 4336 return this; 4337 } 4338 4339 /** 4340 * @param value {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 4341 */ 4342 public boolean hasSubjectType(String value) { 4343 if (this.subjectType == null) 4344 return false; 4345 for (CodeType v : this.subjectType) 4346 if (v.getValue().equals(value)) // code 4347 return true; 4348 return false; 4349 } 4350 4351 /** 4352 * @return {@link #date} (The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4353 */ 4354 public DateTimeType getDateElement() { 4355 if (this.date == null) 4356 if (Configuration.errorOnAutoCreate()) 4357 throw new Error("Attempt to auto-create Questionnaire.date"); 4358 else if (Configuration.doAutoCreate()) 4359 this.date = new DateTimeType(); // bb 4360 return this.date; 4361 } 4362 4363 public boolean hasDateElement() { 4364 return this.date != null && !this.date.isEmpty(); 4365 } 4366 4367 public boolean hasDate() { 4368 return this.date != null && !this.date.isEmpty(); 4369 } 4370 4371 /** 4372 * @param value {@link #date} (The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4373 */ 4374 public Questionnaire setDateElement(DateTimeType value) { 4375 this.date = value; 4376 return this; 4377 } 4378 4379 /** 4380 * @return The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes. 4381 */ 4382 public Date getDate() { 4383 return this.date == null ? null : this.date.getValue(); 4384 } 4385 4386 /** 4387 * @param value The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes. 4388 */ 4389 public Questionnaire setDate(Date value) { 4390 if (value == null) 4391 this.date = null; 4392 else { 4393 if (this.date == null) 4394 this.date = new DateTimeType(); 4395 this.date.setValue(value); 4396 } 4397 return this; 4398 } 4399 4400 /** 4401 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4402 */ 4403 public StringType getPublisherElement() { 4404 if (this.publisher == null) 4405 if (Configuration.errorOnAutoCreate()) 4406 throw new Error("Attempt to auto-create Questionnaire.publisher"); 4407 else if (Configuration.doAutoCreate()) 4408 this.publisher = new StringType(); // bb 4409 return this.publisher; 4410 } 4411 4412 public boolean hasPublisherElement() { 4413 return this.publisher != null && !this.publisher.isEmpty(); 4414 } 4415 4416 public boolean hasPublisher() { 4417 return this.publisher != null && !this.publisher.isEmpty(); 4418 } 4419 4420 /** 4421 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4422 */ 4423 public Questionnaire setPublisherElement(StringType value) { 4424 this.publisher = value; 4425 return this; 4426 } 4427 4428 /** 4429 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire. 4430 */ 4431 public String getPublisher() { 4432 return this.publisher == null ? null : this.publisher.getValue(); 4433 } 4434 4435 /** 4436 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire. 4437 */ 4438 public Questionnaire setPublisher(String value) { 4439 if (Utilities.noString(value)) 4440 this.publisher = null; 4441 else { 4442 if (this.publisher == null) 4443 this.publisher = new StringType(); 4444 this.publisher.setValue(value); 4445 } 4446 return this; 4447 } 4448 4449 /** 4450 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 4451 */ 4452 public List<ContactDetail> getContact() { 4453 if (this.contact == null) 4454 this.contact = new ArrayList<ContactDetail>(); 4455 return this.contact; 4456 } 4457 4458 /** 4459 * @return Returns a reference to <code>this</code> for easy method chaining 4460 */ 4461 public Questionnaire setContact(List<ContactDetail> theContact) { 4462 this.contact = theContact; 4463 return this; 4464 } 4465 4466 public boolean hasContact() { 4467 if (this.contact == null) 4468 return false; 4469 for (ContactDetail item : this.contact) 4470 if (!item.isEmpty()) 4471 return true; 4472 return false; 4473 } 4474 4475 public ContactDetail addContact() { //3 4476 ContactDetail t = new ContactDetail(); 4477 if (this.contact == null) 4478 this.contact = new ArrayList<ContactDetail>(); 4479 this.contact.add(t); 4480 return t; 4481 } 4482 4483 public Questionnaire addContact(ContactDetail t) { //3 4484 if (t == null) 4485 return this; 4486 if (this.contact == null) 4487 this.contact = new ArrayList<ContactDetail>(); 4488 this.contact.add(t); 4489 return this; 4490 } 4491 4492 /** 4493 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 4494 */ 4495 public ContactDetail getContactFirstRep() { 4496 if (getContact().isEmpty()) { 4497 addContact(); 4498 } 4499 return getContact().get(0); 4500 } 4501 4502 /** 4503 * @return {@link #description} (A free text natural language description of the questionnaire from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4504 */ 4505 public MarkdownType getDescriptionElement() { 4506 if (this.description == null) 4507 if (Configuration.errorOnAutoCreate()) 4508 throw new Error("Attempt to auto-create Questionnaire.description"); 4509 else if (Configuration.doAutoCreate()) 4510 this.description = new MarkdownType(); // bb 4511 return this.description; 4512 } 4513 4514 public boolean hasDescriptionElement() { 4515 return this.description != null && !this.description.isEmpty(); 4516 } 4517 4518 public boolean hasDescription() { 4519 return this.description != null && !this.description.isEmpty(); 4520 } 4521 4522 /** 4523 * @param value {@link #description} (A free text natural language description of the questionnaire from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4524 */ 4525 public Questionnaire setDescriptionElement(MarkdownType value) { 4526 this.description = value; 4527 return this; 4528 } 4529 4530 /** 4531 * @return A free text natural language description of the questionnaire from a consumer's perspective. 4532 */ 4533 public String getDescription() { 4534 return this.description == null ? null : this.description.getValue(); 4535 } 4536 4537 /** 4538 * @param value A free text natural language description of the questionnaire from a consumer's perspective. 4539 */ 4540 public Questionnaire setDescription(String value) { 4541 if (Utilities.noString(value)) 4542 this.description = null; 4543 else { 4544 if (this.description == null) 4545 this.description = new MarkdownType(); 4546 this.description.setValue(value); 4547 } 4548 return this; 4549 } 4550 4551 /** 4552 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate questionnaires.) 4553 */ 4554 public List<UsageContext> getUseContext() { 4555 if (this.useContext == null) 4556 this.useContext = new ArrayList<UsageContext>(); 4557 return this.useContext; 4558 } 4559 4560 /** 4561 * @return Returns a reference to <code>this</code> for easy method chaining 4562 */ 4563 public Questionnaire setUseContext(List<UsageContext> theUseContext) { 4564 this.useContext = theUseContext; 4565 return this; 4566 } 4567 4568 public boolean hasUseContext() { 4569 if (this.useContext == null) 4570 return false; 4571 for (UsageContext item : this.useContext) 4572 if (!item.isEmpty()) 4573 return true; 4574 return false; 4575 } 4576 4577 public UsageContext addUseContext() { //3 4578 UsageContext t = new UsageContext(); 4579 if (this.useContext == null) 4580 this.useContext = new ArrayList<UsageContext>(); 4581 this.useContext.add(t); 4582 return t; 4583 } 4584 4585 public Questionnaire addUseContext(UsageContext t) { //3 4586 if (t == null) 4587 return this; 4588 if (this.useContext == null) 4589 this.useContext = new ArrayList<UsageContext>(); 4590 this.useContext.add(t); 4591 return this; 4592 } 4593 4594 /** 4595 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 4596 */ 4597 public UsageContext getUseContextFirstRep() { 4598 if (getUseContext().isEmpty()) { 4599 addUseContext(); 4600 } 4601 return getUseContext().get(0); 4602 } 4603 4604 /** 4605 * @return {@link #jurisdiction} (A legal or geographic region in which the questionnaire is intended to be used.) 4606 */ 4607 public List<CodeableConcept> getJurisdiction() { 4608 if (this.jurisdiction == null) 4609 this.jurisdiction = new ArrayList<CodeableConcept>(); 4610 return this.jurisdiction; 4611 } 4612 4613 /** 4614 * @return Returns a reference to <code>this</code> for easy method chaining 4615 */ 4616 public Questionnaire setJurisdiction(List<CodeableConcept> theJurisdiction) { 4617 this.jurisdiction = theJurisdiction; 4618 return this; 4619 } 4620 4621 public boolean hasJurisdiction() { 4622 if (this.jurisdiction == null) 4623 return false; 4624 for (CodeableConcept item : this.jurisdiction) 4625 if (!item.isEmpty()) 4626 return true; 4627 return false; 4628 } 4629 4630 public CodeableConcept addJurisdiction() { //3 4631 CodeableConcept t = new CodeableConcept(); 4632 if (this.jurisdiction == null) 4633 this.jurisdiction = new ArrayList<CodeableConcept>(); 4634 this.jurisdiction.add(t); 4635 return t; 4636 } 4637 4638 public Questionnaire addJurisdiction(CodeableConcept t) { //3 4639 if (t == null) 4640 return this; 4641 if (this.jurisdiction == null) 4642 this.jurisdiction = new ArrayList<CodeableConcept>(); 4643 this.jurisdiction.add(t); 4644 return this; 4645 } 4646 4647 /** 4648 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 4649 */ 4650 public CodeableConcept getJurisdictionFirstRep() { 4651 if (getJurisdiction().isEmpty()) { 4652 addJurisdiction(); 4653 } 4654 return getJurisdiction().get(0); 4655 } 4656 4657 /** 4658 * @return {@link #purpose} (Explanation of why this questionnaire is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4659 */ 4660 public MarkdownType getPurposeElement() { 4661 if (this.purpose == null) 4662 if (Configuration.errorOnAutoCreate()) 4663 throw new Error("Attempt to auto-create Questionnaire.purpose"); 4664 else if (Configuration.doAutoCreate()) 4665 this.purpose = new MarkdownType(); // bb 4666 return this.purpose; 4667 } 4668 4669 public boolean hasPurposeElement() { 4670 return this.purpose != null && !this.purpose.isEmpty(); 4671 } 4672 4673 public boolean hasPurpose() { 4674 return this.purpose != null && !this.purpose.isEmpty(); 4675 } 4676 4677 /** 4678 * @param value {@link #purpose} (Explanation of why this questionnaire is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4679 */ 4680 public Questionnaire setPurposeElement(MarkdownType value) { 4681 this.purpose = value; 4682 return this; 4683 } 4684 4685 /** 4686 * @return Explanation of why this questionnaire is needed and why it has been designed as it has. 4687 */ 4688 public String getPurpose() { 4689 return this.purpose == null ? null : this.purpose.getValue(); 4690 } 4691 4692 /** 4693 * @param value Explanation of why this questionnaire is needed and why it has been designed as it has. 4694 */ 4695 public Questionnaire setPurpose(String value) { 4696 if (Utilities.noString(value)) 4697 this.purpose = null; 4698 else { 4699 if (this.purpose == null) 4700 this.purpose = new MarkdownType(); 4701 this.purpose.setValue(value); 4702 } 4703 return this; 4704 } 4705 4706 /** 4707 * @return {@link #copyright} (A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4708 */ 4709 public MarkdownType getCopyrightElement() { 4710 if (this.copyright == null) 4711 if (Configuration.errorOnAutoCreate()) 4712 throw new Error("Attempt to auto-create Questionnaire.copyright"); 4713 else if (Configuration.doAutoCreate()) 4714 this.copyright = new MarkdownType(); // bb 4715 return this.copyright; 4716 } 4717 4718 public boolean hasCopyrightElement() { 4719 return this.copyright != null && !this.copyright.isEmpty(); 4720 } 4721 4722 public boolean hasCopyright() { 4723 return this.copyright != null && !this.copyright.isEmpty(); 4724 } 4725 4726 /** 4727 * @param value {@link #copyright} (A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4728 */ 4729 public Questionnaire setCopyrightElement(MarkdownType value) { 4730 this.copyright = value; 4731 return this; 4732 } 4733 4734 /** 4735 * @return A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire. 4736 */ 4737 public String getCopyright() { 4738 return this.copyright == null ? null : this.copyright.getValue(); 4739 } 4740 4741 /** 4742 * @param value A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire. 4743 */ 4744 public Questionnaire setCopyright(String value) { 4745 if (Utilities.noString(value)) 4746 this.copyright = null; 4747 else { 4748 if (this.copyright == null) 4749 this.copyright = new MarkdownType(); 4750 this.copyright.setValue(value); 4751 } 4752 return this; 4753 } 4754 4755 /** 4756 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4757 */ 4758 public StringType getCopyrightLabelElement() { 4759 if (this.copyrightLabel == null) 4760 if (Configuration.errorOnAutoCreate()) 4761 throw new Error("Attempt to auto-create Questionnaire.copyrightLabel"); 4762 else if (Configuration.doAutoCreate()) 4763 this.copyrightLabel = new StringType(); // bb 4764 return this.copyrightLabel; 4765 } 4766 4767 public boolean hasCopyrightLabelElement() { 4768 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4769 } 4770 4771 public boolean hasCopyrightLabel() { 4772 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4773 } 4774 4775 /** 4776 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4777 */ 4778 public Questionnaire setCopyrightLabelElement(StringType value) { 4779 this.copyrightLabel = value; 4780 return this; 4781 } 4782 4783 /** 4784 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4785 */ 4786 public String getCopyrightLabel() { 4787 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 4788 } 4789 4790 /** 4791 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4792 */ 4793 public Questionnaire setCopyrightLabel(String value) { 4794 if (Utilities.noString(value)) 4795 this.copyrightLabel = null; 4796 else { 4797 if (this.copyrightLabel == null) 4798 this.copyrightLabel = new StringType(); 4799 this.copyrightLabel.setValue(value); 4800 } 4801 return this; 4802 } 4803 4804 /** 4805 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4806 */ 4807 public DateType getApprovalDateElement() { 4808 if (this.approvalDate == null) 4809 if (Configuration.errorOnAutoCreate()) 4810 throw new Error("Attempt to auto-create Questionnaire.approvalDate"); 4811 else if (Configuration.doAutoCreate()) 4812 this.approvalDate = new DateType(); // bb 4813 return this.approvalDate; 4814 } 4815 4816 public boolean hasApprovalDateElement() { 4817 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4818 } 4819 4820 public boolean hasApprovalDate() { 4821 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4822 } 4823 4824 /** 4825 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4826 */ 4827 public Questionnaire setApprovalDateElement(DateType value) { 4828 this.approvalDate = value; 4829 return this; 4830 } 4831 4832 /** 4833 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4834 */ 4835 public Date getApprovalDate() { 4836 return this.approvalDate == null ? null : this.approvalDate.getValue(); 4837 } 4838 4839 /** 4840 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4841 */ 4842 public Questionnaire setApprovalDate(Date value) { 4843 if (value == null) 4844 this.approvalDate = null; 4845 else { 4846 if (this.approvalDate == null) 4847 this.approvalDate = new DateType(); 4848 this.approvalDate.setValue(value); 4849 } 4850 return this; 4851 } 4852 4853 /** 4854 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4855 */ 4856 public DateType getLastReviewDateElement() { 4857 if (this.lastReviewDate == null) 4858 if (Configuration.errorOnAutoCreate()) 4859 throw new Error("Attempt to auto-create Questionnaire.lastReviewDate"); 4860 else if (Configuration.doAutoCreate()) 4861 this.lastReviewDate = new DateType(); // bb 4862 return this.lastReviewDate; 4863 } 4864 4865 public boolean hasLastReviewDateElement() { 4866 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4867 } 4868 4869 public boolean hasLastReviewDate() { 4870 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4871 } 4872 4873 /** 4874 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4875 */ 4876 public Questionnaire setLastReviewDateElement(DateType value) { 4877 this.lastReviewDate = value; 4878 return this; 4879 } 4880 4881 /** 4882 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4883 */ 4884 public Date getLastReviewDate() { 4885 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 4886 } 4887 4888 /** 4889 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4890 */ 4891 public Questionnaire setLastReviewDate(Date value) { 4892 if (value == null) 4893 this.lastReviewDate = null; 4894 else { 4895 if (this.lastReviewDate == null) 4896 this.lastReviewDate = new DateType(); 4897 this.lastReviewDate.setValue(value); 4898 } 4899 return this; 4900 } 4901 4902 /** 4903 * @return {@link #effectivePeriod} (The period during which the questionnaire content was or is planned to be in active use.) 4904 */ 4905 public Period getEffectivePeriod() { 4906 if (this.effectivePeriod == null) 4907 if (Configuration.errorOnAutoCreate()) 4908 throw new Error("Attempt to auto-create Questionnaire.effectivePeriod"); 4909 else if (Configuration.doAutoCreate()) 4910 this.effectivePeriod = new Period(); // cc 4911 return this.effectivePeriod; 4912 } 4913 4914 public boolean hasEffectivePeriod() { 4915 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 4916 } 4917 4918 /** 4919 * @param value {@link #effectivePeriod} (The period during which the questionnaire content was or is planned to be in active use.) 4920 */ 4921 public Questionnaire setEffectivePeriod(Period value) { 4922 this.effectivePeriod = value; 4923 return this; 4924 } 4925 4926 /** 4927 * @return {@link #code} (An identifier for this collection of questions in a particular terminology such as LOINC.) 4928 */ 4929 public List<Coding> getCode() { 4930 if (this.code == null) 4931 this.code = new ArrayList<Coding>(); 4932 return this.code; 4933 } 4934 4935 /** 4936 * @return Returns a reference to <code>this</code> for easy method chaining 4937 */ 4938 public Questionnaire setCode(List<Coding> theCode) { 4939 this.code = theCode; 4940 return this; 4941 } 4942 4943 public boolean hasCode() { 4944 if (this.code == null) 4945 return false; 4946 for (Coding item : this.code) 4947 if (!item.isEmpty()) 4948 return true; 4949 return false; 4950 } 4951 4952 public Coding addCode() { //3 4953 Coding t = new Coding(); 4954 if (this.code == null) 4955 this.code = new ArrayList<Coding>(); 4956 this.code.add(t); 4957 return t; 4958 } 4959 4960 public Questionnaire addCode(Coding t) { //3 4961 if (t == null) 4962 return this; 4963 if (this.code == null) 4964 this.code = new ArrayList<Coding>(); 4965 this.code.add(t); 4966 return this; 4967 } 4968 4969 /** 4970 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 4971 */ 4972 public Coding getCodeFirstRep() { 4973 if (getCode().isEmpty()) { 4974 addCode(); 4975 } 4976 return getCode().get(0); 4977 } 4978 4979 /** 4980 * @return {@link #item} (A particular question, question grouping or display text that is part of the questionnaire.) 4981 */ 4982 public List<QuestionnaireItemComponent> getItem() { 4983 if (this.item == null) 4984 this.item = new ArrayList<QuestionnaireItemComponent>(); 4985 return this.item; 4986 } 4987 4988 /** 4989 * @return Returns a reference to <code>this</code> for easy method chaining 4990 */ 4991 public Questionnaire setItem(List<QuestionnaireItemComponent> theItem) { 4992 this.item = theItem; 4993 return this; 4994 } 4995 4996 public boolean hasItem() { 4997 if (this.item == null) 4998 return false; 4999 for (QuestionnaireItemComponent item : this.item) 5000 if (!item.isEmpty()) 5001 return true; 5002 return false; 5003 } 5004 5005 public QuestionnaireItemComponent addItem() { //3 5006 QuestionnaireItemComponent t = new QuestionnaireItemComponent(); 5007 if (this.item == null) 5008 this.item = new ArrayList<QuestionnaireItemComponent>(); 5009 this.item.add(t); 5010 return t; 5011 } 5012 5013 public Questionnaire addItem(QuestionnaireItemComponent t) { //3 5014 if (t == null) 5015 return this; 5016 if (this.item == null) 5017 this.item = new ArrayList<QuestionnaireItemComponent>(); 5018 this.item.add(t); 5019 return this; 5020 } 5021 5022 /** 5023 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 5024 */ 5025 public QuestionnaireItemComponent getItemFirstRep() { 5026 if (getItem().isEmpty()) { 5027 addItem(); 5028 } 5029 return getItem().get(0); 5030 } 5031 5032 /** 5033 * not supported on this implementation 5034 */ 5035 @Override 5036 public int getTopicMax() { 5037 return 0; 5038 } 5039 /** 5040 * @return {@link #topic} (Descriptive topics related to the content of the questionnaire. Topics provide a high-level categorization as well as keywords for the questionnaire that can be useful for filtering and searching.) 5041 */ 5042 public List<CodeableConcept> getTopic() { 5043 return new ArrayList<>(); 5044 } 5045 /** 5046 * @return Returns a reference to <code>this</code> for easy method chaining 5047 */ 5048 public Questionnaire setTopic(List<CodeableConcept> theTopic) { 5049 throw new Error("The resource type \"Questionnaire\" does not implement the property \"topic\""); 5050 } 5051 public boolean hasTopic() { 5052 return false; 5053 } 5054 5055 public CodeableConcept addTopic() { //3 5056 throw new Error("The resource type \"Questionnaire\" does not implement the property \"topic\""); 5057 } 5058 public Questionnaire addTopic(CodeableConcept t) { //3 5059 throw new Error("The resource type \"Questionnaire\" does not implement the property \"topic\""); 5060 } 5061 /** 5062 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 5063 */ 5064 public CodeableConcept getTopicFirstRep() { 5065 throw new Error("The resource type \"Questionnaire\" does not implement the property \"topic\""); 5066 } 5067 /** 5068 * not supported on this implementation 5069 */ 5070 @Override 5071 public int getAuthorMax() { 5072 return 0; 5073 } 5074 /** 5075 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the questionnaire.) 5076 */ 5077 public List<ContactDetail> getAuthor() { 5078 return new ArrayList<>(); 5079 } 5080 /** 5081 * @return Returns a reference to <code>this</code> for easy method chaining 5082 */ 5083 public Questionnaire setAuthor(List<ContactDetail> theAuthor) { 5084 throw new Error("The resource type \"Questionnaire\" does not implement the property \"author\""); 5085 } 5086 public boolean hasAuthor() { 5087 return false; 5088 } 5089 5090 public ContactDetail addAuthor() { //3 5091 throw new Error("The resource type \"Questionnaire\" does not implement the property \"author\""); 5092 } 5093 public Questionnaire addAuthor(ContactDetail t) { //3 5094 throw new Error("The resource type \"Questionnaire\" does not implement the property \"author\""); 5095 } 5096 /** 5097 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {2} 5098 */ 5099 public ContactDetail getAuthorFirstRep() { 5100 throw new Error("The resource type \"Questionnaire\" does not implement the property \"author\""); 5101 } 5102 /** 5103 * not supported on this implementation 5104 */ 5105 @Override 5106 public int getEditorMax() { 5107 return 0; 5108 } 5109 /** 5110 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the questionnaire.) 5111 */ 5112 public List<ContactDetail> getEditor() { 5113 return new ArrayList<>(); 5114 } 5115 /** 5116 * @return Returns a reference to <code>this</code> for easy method chaining 5117 */ 5118 public Questionnaire setEditor(List<ContactDetail> theEditor) { 5119 throw new Error("The resource type \"Questionnaire\" does not implement the property \"editor\""); 5120 } 5121 public boolean hasEditor() { 5122 return false; 5123 } 5124 5125 public ContactDetail addEditor() { //3 5126 throw new Error("The resource type \"Questionnaire\" does not implement the property \"editor\""); 5127 } 5128 public Questionnaire addEditor(ContactDetail t) { //3 5129 throw new Error("The resource type \"Questionnaire\" does not implement the property \"editor\""); 5130 } 5131 /** 5132 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {2} 5133 */ 5134 public ContactDetail getEditorFirstRep() { 5135 throw new Error("The resource type \"Questionnaire\" does not implement the property \"editor\""); 5136 } 5137 /** 5138 * not supported on this implementation 5139 */ 5140 @Override 5141 public int getReviewerMax() { 5142 return 0; 5143 } 5144 /** 5145 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the questionnaire.) 5146 */ 5147 public List<ContactDetail> getReviewer() { 5148 return new ArrayList<>(); 5149 } 5150 /** 5151 * @return Returns a reference to <code>this</code> for easy method chaining 5152 */ 5153 public Questionnaire setReviewer(List<ContactDetail> theReviewer) { 5154 throw new Error("The resource type \"Questionnaire\" does not implement the property \"reviewer\""); 5155 } 5156 public boolean hasReviewer() { 5157 return false; 5158 } 5159 5160 public ContactDetail addReviewer() { //3 5161 throw new Error("The resource type \"Questionnaire\" does not implement the property \"reviewer\""); 5162 } 5163 public Questionnaire addReviewer(ContactDetail t) { //3 5164 throw new Error("The resource type \"Questionnaire\" does not implement the property \"reviewer\""); 5165 } 5166 /** 5167 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {2} 5168 */ 5169 public ContactDetail getReviewerFirstRep() { 5170 throw new Error("The resource type \"Questionnaire\" does not implement the property \"reviewer\""); 5171 } 5172 /** 5173 * not supported on this implementation 5174 */ 5175 @Override 5176 public int getEndorserMax() { 5177 return 0; 5178 } 5179 /** 5180 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the questionnaire for use in some setting.) 5181 */ 5182 public List<ContactDetail> getEndorser() { 5183 return new ArrayList<>(); 5184 } 5185 /** 5186 * @return Returns a reference to <code>this</code> for easy method chaining 5187 */ 5188 public Questionnaire setEndorser(List<ContactDetail> theEndorser) { 5189 throw new Error("The resource type \"Questionnaire\" does not implement the property \"endorser\""); 5190 } 5191 public boolean hasEndorser() { 5192 return false; 5193 } 5194 5195 public ContactDetail addEndorser() { //3 5196 throw new Error("The resource type \"Questionnaire\" does not implement the property \"endorser\""); 5197 } 5198 public Questionnaire addEndorser(ContactDetail t) { //3 5199 throw new Error("The resource type \"Questionnaire\" does not implement the property \"endorser\""); 5200 } 5201 /** 5202 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {2} 5203 */ 5204 public ContactDetail getEndorserFirstRep() { 5205 throw new Error("The resource type \"Questionnaire\" does not implement the property \"endorser\""); 5206 } 5207 /** 5208 * not supported on this implementation 5209 */ 5210 @Override 5211 public int getRelatedArtifactMax() { 5212 return 0; 5213 } 5214 /** 5215 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 5216 */ 5217 public List<RelatedArtifact> getRelatedArtifact() { 5218 return new ArrayList<>(); 5219 } 5220 /** 5221 * @return Returns a reference to <code>this</code> for easy method chaining 5222 */ 5223 public Questionnaire setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 5224 throw new Error("The resource type \"Questionnaire\" does not implement the property \"relatedArtifact\""); 5225 } 5226 public boolean hasRelatedArtifact() { 5227 return false; 5228 } 5229 5230 public RelatedArtifact addRelatedArtifact() { //3 5231 throw new Error("The resource type \"Questionnaire\" does not implement the property \"relatedArtifact\""); 5232 } 5233 public Questionnaire addRelatedArtifact(RelatedArtifact t) { //3 5234 throw new Error("The resource type \"Questionnaire\" does not implement the property \"relatedArtifact\""); 5235 } 5236 /** 5237 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {2} 5238 */ 5239 public RelatedArtifact getRelatedArtifactFirstRep() { 5240 throw new Error("The resource type \"Questionnaire\" does not implement the property \"relatedArtifact\""); 5241 } 5242 protected void listChildren(List<Property> children) { 5243 super.listChildren(children); 5244 children.add(new Property("url", "uri", "An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers.", 0, 1, url)); 5245 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 5246 children.add(new Property("version", "string", "The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 5247 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 5248 children.add(new Property("name", "string", "A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 5249 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the questionnaire.", 0, 1, title)); 5250 children.add(new Property("derivedFrom", "canonical(Questionnaire)", "The URL of a Questionnaire that this Questionnaire is based on.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 5251 children.add(new Property("status", "code", "The current state of this questionnaire.", 0, 1, status)); 5252 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.", 0, 1, experimental)); 5253 children.add(new Property("subjectType", "code", "The types of subjects that can be the subject of responses created for the questionnaire.", 0, java.lang.Integer.MAX_VALUE, subjectType)); 5254 children.add(new Property("date", "dateTime", "The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.", 0, 1, date)); 5255 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire.", 0, 1, publisher)); 5256 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 5257 children.add(new Property("description", "markdown", "A free text natural language description of the questionnaire from a consumer's perspective.", 0, 1, description)); 5258 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate questionnaires.", 0, java.lang.Integer.MAX_VALUE, useContext)); 5259 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the questionnaire is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 5260 children.add(new Property("purpose", "markdown", "Explanation of why this questionnaire is needed and why it has been designed as it has.", 0, 1, purpose)); 5261 children.add(new Property("copyright", "markdown", "A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.", 0, 1, copyright)); 5262 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 5263 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 5264 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 5265 children.add(new Property("effectivePeriod", "Period", "The period during which the questionnaire content was or is planned to be in active use.", 0, 1, effectivePeriod)); 5266 children.add(new Property("code", "Coding", "An identifier for this collection of questions in a particular terminology such as LOINC.", 0, java.lang.Integer.MAX_VALUE, code)); 5267 children.add(new Property("item", "", "A particular question, question grouping or display text that is part of the questionnaire.", 0, java.lang.Integer.MAX_VALUE, item)); 5268 } 5269 5270 @Override 5271 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5272 switch (_hash) { 5273 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers.", 0, 1, url); 5274 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 5275 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 5276 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5277 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5278 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5279 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5280 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 5281 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the questionnaire.", 0, 1, title); 5282 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(Questionnaire)", "The URL of a Questionnaire that this Questionnaire is based on.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 5283 case -892481550: /*status*/ return new Property("status", "code", "The current state of this questionnaire.", 0, 1, status); 5284 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.", 0, 1, experimental); 5285 case -603200890: /*subjectType*/ return new Property("subjectType", "code", "The types of subjects that can be the subject of responses created for the questionnaire.", 0, java.lang.Integer.MAX_VALUE, subjectType); 5286 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the questionnaire was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.", 0, 1, date); 5287 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the questionnaire.", 0, 1, publisher); 5288 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 5289 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the questionnaire from a consumer's perspective.", 0, 1, description); 5290 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate questionnaires.", 0, java.lang.Integer.MAX_VALUE, useContext); 5291 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the questionnaire is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 5292 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this questionnaire is needed and why it has been designed as it has.", 0, 1, purpose); 5293 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.", 0, 1, copyright); 5294 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 5295 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 5296 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 5297 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the questionnaire content was or is planned to be in active use.", 0, 1, effectivePeriod); 5298 case 3059181: /*code*/ return new Property("code", "Coding", "An identifier for this collection of questions in a particular terminology such as LOINC.", 0, java.lang.Integer.MAX_VALUE, code); 5299 case 3242771: /*item*/ return new Property("item", "", "A particular question, question grouping or display text that is part of the questionnaire.", 0, java.lang.Integer.MAX_VALUE, item); 5300 default: return super.getNamedProperty(_hash, _name, _checkValid); 5301 } 5302 5303 } 5304 5305 @Override 5306 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5307 switch (hash) { 5308 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 5309 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5310 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 5311 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 5312 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 5313 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 5314 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // CanonicalType 5315 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 5316 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 5317 case -603200890: /*subjectType*/ return this.subjectType == null ? new Base[0] : this.subjectType.toArray(new Base[this.subjectType.size()]); // CodeType 5318 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 5319 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 5320 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5321 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 5322 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5323 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5324 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 5325 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 5326 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 5327 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 5328 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 5329 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 5330 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 5331 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireItemComponent 5332 default: return super.getProperty(hash, name, checkValid); 5333 } 5334 5335 } 5336 5337 @Override 5338 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5339 switch (hash) { 5340 case 116079: // url 5341 this.url = TypeConvertor.castToUri(value); // UriType 5342 return value; 5343 case -1618432855: // identifier 5344 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 5345 return value; 5346 case 351608024: // version 5347 this.version = TypeConvertor.castToString(value); // StringType 5348 return value; 5349 case 1508158071: // versionAlgorithm 5350 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5351 return value; 5352 case 3373707: // name 5353 this.name = TypeConvertor.castToString(value); // StringType 5354 return value; 5355 case 110371416: // title 5356 this.title = TypeConvertor.castToString(value); // StringType 5357 return value; 5358 case 1077922663: // derivedFrom 5359 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); // CanonicalType 5360 return value; 5361 case -892481550: // status 5362 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5363 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5364 return value; 5365 case -404562712: // experimental 5366 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 5367 return value; 5368 case -603200890: // subjectType 5369 this.getSubjectType().add(TypeConvertor.castToCode(value)); // CodeType 5370 return value; 5371 case 3076014: // date 5372 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 5373 return value; 5374 case 1447404028: // publisher 5375 this.publisher = TypeConvertor.castToString(value); // StringType 5376 return value; 5377 case 951526432: // contact 5378 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5379 return value; 5380 case -1724546052: // description 5381 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 5382 return value; 5383 case -669707736: // useContext 5384 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5385 return value; 5386 case -507075711: // jurisdiction 5387 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5388 return value; 5389 case -220463842: // purpose 5390 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 5391 return value; 5392 case 1522889671: // copyright 5393 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5394 return value; 5395 case 765157229: // copyrightLabel 5396 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 5397 return value; 5398 case 223539345: // approvalDate 5399 this.approvalDate = TypeConvertor.castToDate(value); // DateType 5400 return value; 5401 case -1687512484: // lastReviewDate 5402 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 5403 return value; 5404 case -403934648: // effectivePeriod 5405 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 5406 return value; 5407 case 3059181: // code 5408 this.getCode().add(TypeConvertor.castToCoding(value)); // Coding 5409 return value; 5410 case 3242771: // item 5411 this.getItem().add((QuestionnaireItemComponent) value); // QuestionnaireItemComponent 5412 return value; 5413 default: return super.setProperty(hash, name, value); 5414 } 5415 5416 } 5417 5418 @Override 5419 public Base setProperty(String name, Base value) throws FHIRException { 5420 if (name.equals("url")) { 5421 this.url = TypeConvertor.castToUri(value); // UriType 5422 } else if (name.equals("identifier")) { 5423 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5424 } else if (name.equals("version")) { 5425 this.version = TypeConvertor.castToString(value); // StringType 5426 } else if (name.equals("versionAlgorithm[x]")) { 5427 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5428 } else if (name.equals("name")) { 5429 this.name = TypeConvertor.castToString(value); // StringType 5430 } else if (name.equals("title")) { 5431 this.title = TypeConvertor.castToString(value); // StringType 5432 } else if (name.equals("derivedFrom")) { 5433 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); 5434 } else if (name.equals("status")) { 5435 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5436 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5437 } else if (name.equals("experimental")) { 5438 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 5439 } else if (name.equals("subjectType")) { 5440 this.getSubjectType().add(TypeConvertor.castToCode(value)); 5441 } else if (name.equals("date")) { 5442 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 5443 } else if (name.equals("publisher")) { 5444 this.publisher = TypeConvertor.castToString(value); // StringType 5445 } else if (name.equals("contact")) { 5446 this.getContact().add(TypeConvertor.castToContactDetail(value)); 5447 } else if (name.equals("description")) { 5448 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 5449 } else if (name.equals("useContext")) { 5450 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 5451 } else if (name.equals("jurisdiction")) { 5452 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 5453 } else if (name.equals("purpose")) { 5454 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 5455 } else if (name.equals("copyright")) { 5456 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5457 } else if (name.equals("copyrightLabel")) { 5458 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 5459 } else if (name.equals("approvalDate")) { 5460 this.approvalDate = TypeConvertor.castToDate(value); // DateType 5461 } else if (name.equals("lastReviewDate")) { 5462 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 5463 } else if (name.equals("effectivePeriod")) { 5464 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 5465 } else if (name.equals("code")) { 5466 this.getCode().add(TypeConvertor.castToCoding(value)); 5467 } else if (name.equals("item")) { 5468 this.getItem().add((QuestionnaireItemComponent) value); 5469 } else 5470 return super.setProperty(name, value); 5471 return value; 5472 } 5473 5474 @Override 5475 public void removeChild(String name, Base value) throws FHIRException { 5476 if (name.equals("url")) { 5477 this.url = null; 5478 } else if (name.equals("identifier")) { 5479 this.getIdentifier().remove(value); 5480 } else if (name.equals("version")) { 5481 this.version = null; 5482 } else if (name.equals("versionAlgorithm[x]")) { 5483 this.versionAlgorithm = null; 5484 } else if (name.equals("name")) { 5485 this.name = null; 5486 } else if (name.equals("title")) { 5487 this.title = null; 5488 } else if (name.equals("derivedFrom")) { 5489 this.getDerivedFrom().remove(value); 5490 } else if (name.equals("status")) { 5491 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5492 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5493 } else if (name.equals("experimental")) { 5494 this.experimental = null; 5495 } else if (name.equals("subjectType")) { 5496 this.getSubjectType().remove(value); 5497 } else if (name.equals("date")) { 5498 this.date = null; 5499 } else if (name.equals("publisher")) { 5500 this.publisher = null; 5501 } else if (name.equals("contact")) { 5502 this.getContact().remove(value); 5503 } else if (name.equals("description")) { 5504 this.description = null; 5505 } else if (name.equals("useContext")) { 5506 this.getUseContext().remove(value); 5507 } else if (name.equals("jurisdiction")) { 5508 this.getJurisdiction().remove(value); 5509 } else if (name.equals("purpose")) { 5510 this.purpose = null; 5511 } else if (name.equals("copyright")) { 5512 this.copyright = null; 5513 } else if (name.equals("copyrightLabel")) { 5514 this.copyrightLabel = null; 5515 } else if (name.equals("approvalDate")) { 5516 this.approvalDate = null; 5517 } else if (name.equals("lastReviewDate")) { 5518 this.lastReviewDate = null; 5519 } else if (name.equals("effectivePeriod")) { 5520 this.effectivePeriod = null; 5521 } else if (name.equals("code")) { 5522 this.getCode().remove(value); 5523 } else if (name.equals("item")) { 5524 this.getItem().remove((QuestionnaireItemComponent) value); 5525 } else 5526 super.removeChild(name, value); 5527 5528 } 5529 5530 @Override 5531 public Base makeProperty(int hash, String name) throws FHIRException { 5532 switch (hash) { 5533 case 116079: return getUrlElement(); 5534 case -1618432855: return addIdentifier(); 5535 case 351608024: return getVersionElement(); 5536 case -115699031: return getVersionAlgorithm(); 5537 case 1508158071: return getVersionAlgorithm(); 5538 case 3373707: return getNameElement(); 5539 case 110371416: return getTitleElement(); 5540 case 1077922663: return addDerivedFromElement(); 5541 case -892481550: return getStatusElement(); 5542 case -404562712: return getExperimentalElement(); 5543 case -603200890: return addSubjectTypeElement(); 5544 case 3076014: return getDateElement(); 5545 case 1447404028: return getPublisherElement(); 5546 case 951526432: return addContact(); 5547 case -1724546052: return getDescriptionElement(); 5548 case -669707736: return addUseContext(); 5549 case -507075711: return addJurisdiction(); 5550 case -220463842: return getPurposeElement(); 5551 case 1522889671: return getCopyrightElement(); 5552 case 765157229: return getCopyrightLabelElement(); 5553 case 223539345: return getApprovalDateElement(); 5554 case -1687512484: return getLastReviewDateElement(); 5555 case -403934648: return getEffectivePeriod(); 5556 case 3059181: return addCode(); 5557 case 3242771: return addItem(); 5558 default: return super.makeProperty(hash, name); 5559 } 5560 5561 } 5562 5563 @Override 5564 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5565 switch (hash) { 5566 case 116079: /*url*/ return new String[] {"uri"}; 5567 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5568 case 351608024: /*version*/ return new String[] {"string"}; 5569 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 5570 case 3373707: /*name*/ return new String[] {"string"}; 5571 case 110371416: /*title*/ return new String[] {"string"}; 5572 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 5573 case -892481550: /*status*/ return new String[] {"code"}; 5574 case -404562712: /*experimental*/ return new String[] {"boolean"}; 5575 case -603200890: /*subjectType*/ return new String[] {"code"}; 5576 case 3076014: /*date*/ return new String[] {"dateTime"}; 5577 case 1447404028: /*publisher*/ return new String[] {"string"}; 5578 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 5579 case -1724546052: /*description*/ return new String[] {"markdown"}; 5580 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5581 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 5582 case -220463842: /*purpose*/ return new String[] {"markdown"}; 5583 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5584 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 5585 case 223539345: /*approvalDate*/ return new String[] {"date"}; 5586 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 5587 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 5588 case 3059181: /*code*/ return new String[] {"Coding"}; 5589 case 3242771: /*item*/ return new String[] {}; 5590 default: return super.getTypesForProperty(hash, name); 5591 } 5592 5593 } 5594 5595 @Override 5596 public Base addChild(String name) throws FHIRException { 5597 if (name.equals("url")) { 5598 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.url"); 5599 } 5600 else if (name.equals("identifier")) { 5601 return addIdentifier(); 5602 } 5603 else if (name.equals("version")) { 5604 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.version"); 5605 } 5606 else if (name.equals("versionAlgorithmString")) { 5607 this.versionAlgorithm = new StringType(); 5608 return this.versionAlgorithm; 5609 } 5610 else if (name.equals("versionAlgorithmCoding")) { 5611 this.versionAlgorithm = new Coding(); 5612 return this.versionAlgorithm; 5613 } 5614 else if (name.equals("name")) { 5615 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.name"); 5616 } 5617 else if (name.equals("title")) { 5618 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.title"); 5619 } 5620 else if (name.equals("derivedFrom")) { 5621 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.derivedFrom"); 5622 } 5623 else if (name.equals("status")) { 5624 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.status"); 5625 } 5626 else if (name.equals("experimental")) { 5627 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.experimental"); 5628 } 5629 else if (name.equals("subjectType")) { 5630 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.subjectType"); 5631 } 5632 else if (name.equals("date")) { 5633 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.date"); 5634 } 5635 else if (name.equals("publisher")) { 5636 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.publisher"); 5637 } 5638 else if (name.equals("contact")) { 5639 return addContact(); 5640 } 5641 else if (name.equals("description")) { 5642 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.description"); 5643 } 5644 else if (name.equals("useContext")) { 5645 return addUseContext(); 5646 } 5647 else if (name.equals("jurisdiction")) { 5648 return addJurisdiction(); 5649 } 5650 else if (name.equals("purpose")) { 5651 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.purpose"); 5652 } 5653 else if (name.equals("copyright")) { 5654 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.copyright"); 5655 } 5656 else if (name.equals("copyrightLabel")) { 5657 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.copyrightLabel"); 5658 } 5659 else if (name.equals("approvalDate")) { 5660 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.approvalDate"); 5661 } 5662 else if (name.equals("lastReviewDate")) { 5663 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.lastReviewDate"); 5664 } 5665 else if (name.equals("effectivePeriod")) { 5666 this.effectivePeriod = new Period(); 5667 return this.effectivePeriod; 5668 } 5669 else if (name.equals("code")) { 5670 return addCode(); 5671 } 5672 else if (name.equals("item")) { 5673 return addItem(); 5674 } 5675 else 5676 return super.addChild(name); 5677 } 5678 5679 public String fhirType() { 5680 return "Questionnaire"; 5681 5682 } 5683 5684 public Questionnaire copy() { 5685 Questionnaire dst = new Questionnaire(); 5686 copyValues(dst); 5687 return dst; 5688 } 5689 5690 public void copyValues(Questionnaire dst) { 5691 super.copyValues(dst); 5692 dst.url = url == null ? null : url.copy(); 5693 if (identifier != null) { 5694 dst.identifier = new ArrayList<Identifier>(); 5695 for (Identifier i : identifier) 5696 dst.identifier.add(i.copy()); 5697 }; 5698 dst.version = version == null ? null : version.copy(); 5699 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 5700 dst.name = name == null ? null : name.copy(); 5701 dst.title = title == null ? null : title.copy(); 5702 if (derivedFrom != null) { 5703 dst.derivedFrom = new ArrayList<CanonicalType>(); 5704 for (CanonicalType i : derivedFrom) 5705 dst.derivedFrom.add(i.copy()); 5706 }; 5707 dst.status = status == null ? null : status.copy(); 5708 dst.experimental = experimental == null ? null : experimental.copy(); 5709 if (subjectType != null) { 5710 dst.subjectType = new ArrayList<CodeType>(); 5711 for (CodeType i : subjectType) 5712 dst.subjectType.add(i.copy()); 5713 }; 5714 dst.date = date == null ? null : date.copy(); 5715 dst.publisher = publisher == null ? null : publisher.copy(); 5716 if (contact != null) { 5717 dst.contact = new ArrayList<ContactDetail>(); 5718 for (ContactDetail i : contact) 5719 dst.contact.add(i.copy()); 5720 }; 5721 dst.description = description == null ? null : description.copy(); 5722 if (useContext != null) { 5723 dst.useContext = new ArrayList<UsageContext>(); 5724 for (UsageContext i : useContext) 5725 dst.useContext.add(i.copy()); 5726 }; 5727 if (jurisdiction != null) { 5728 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5729 for (CodeableConcept i : jurisdiction) 5730 dst.jurisdiction.add(i.copy()); 5731 }; 5732 dst.purpose = purpose == null ? null : purpose.copy(); 5733 dst.copyright = copyright == null ? null : copyright.copy(); 5734 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 5735 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5736 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5737 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5738 if (code != null) { 5739 dst.code = new ArrayList<Coding>(); 5740 for (Coding i : code) 5741 dst.code.add(i.copy()); 5742 }; 5743 if (item != null) { 5744 dst.item = new ArrayList<QuestionnaireItemComponent>(); 5745 for (QuestionnaireItemComponent i : item) 5746 dst.item.add(i.copy()); 5747 }; 5748 } 5749 5750 protected Questionnaire typedCopy() { 5751 return copy(); 5752 } 5753 5754 @Override 5755 public boolean equalsDeep(Base other_) { 5756 if (!super.equalsDeep(other_)) 5757 return false; 5758 if (!(other_ instanceof Questionnaire)) 5759 return false; 5760 Questionnaire o = (Questionnaire) other_; 5761 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 5762 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 5763 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 5764 && compareDeep(subjectType, o.subjectType, true) && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) 5765 && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 5766 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 5767 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 5768 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 5769 && compareDeep(code, o.code, true) && compareDeep(item, o.item, true); 5770 } 5771 5772 @Override 5773 public boolean equalsShallow(Base other_) { 5774 if (!super.equalsShallow(other_)) 5775 return false; 5776 if (!(other_ instanceof Questionnaire)) 5777 return false; 5778 Questionnaire o = (Questionnaire) other_; 5779 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 5780 && compareValues(title, o.title, true) && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(status, o.status, true) 5781 && compareValues(experimental, o.experimental, true) && compareValues(subjectType, o.subjectType, true) 5782 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 5783 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 5784 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 5785 ; 5786 } 5787 5788 public boolean isEmpty() { 5789 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 5790 , versionAlgorithm, name, title, derivedFrom, status, experimental, subjectType 5791 , date, publisher, contact, description, useContext, jurisdiction, purpose, copyright 5792 , copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, code, item); 5793 } 5794 5795 @Override 5796 public ResourceType getResourceType() { 5797 return ResourceType.Questionnaire; 5798 } 5799 5800 /** 5801 * Search parameter: <b>context-quantity</b> 5802 * <p> 5803 * Description: <b>Multiple Resources: 5804 5805* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5806* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5807* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5808* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5809* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5810* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5811* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5812* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5813* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5814* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5815* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5816* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5817* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5818* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5819* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5820* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5821* [Library](library.html): A quantity- or range-valued use context assigned to the library 5822* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5823* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5824* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5825* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5826* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5827* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5828* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5829* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5830* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5831* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5832* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5833* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5834* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5835</b><br> 5836 * Type: <b>quantity</b><br> 5837 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5838 * </p> 5839 */ 5840 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 5841 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5842 /** 5843 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5844 * <p> 5845 * Description: <b>Multiple Resources: 5846 5847* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5848* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5849* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5850* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5851* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5852* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5853* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5854* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5855* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5856* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5857* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5858* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5859* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5860* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5861* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5862* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5863* [Library](library.html): A quantity- or range-valued use context assigned to the library 5864* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5865* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5866* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5867* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5868* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5869* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5870* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5871* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5872* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5873* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5874* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5875* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5876* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5877</b><br> 5878 * Type: <b>quantity</b><br> 5879 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5880 * </p> 5881 */ 5882 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 5883 5884 /** 5885 * Search parameter: <b>context-type-quantity</b> 5886 * <p> 5887 * Description: <b>Multiple Resources: 5888 5889* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5890* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5891* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5892* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5893* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5894* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5895* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5896* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5897* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5898* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5899* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5900* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5901* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5902* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5903* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5904* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5905* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5906* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5907* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5908* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5909* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5910* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5911* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5912* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5913* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5914* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5915* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5916* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5917* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5918* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5919</b><br> 5920 * Type: <b>composite</b><br> 5921 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5922 * </p> 5923 */ 5924 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 5925 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5926 /** 5927 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 5928 * <p> 5929 * Description: <b>Multiple Resources: 5930 5931* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5932* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5933* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5934* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5935* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5936* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5937* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5938* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5939* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5940* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5941* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5942* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5943* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5944* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5945* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5946* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5947* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5948* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5949* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5950* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5951* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5952* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5953* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5954* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5955* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5956* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5957* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5958* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5959* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5960* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5961</b><br> 5962 * Type: <b>composite</b><br> 5963 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5964 * </p> 5965 */ 5966 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 5967 5968 /** 5969 * Search parameter: <b>context-type-value</b> 5970 * <p> 5971 * Description: <b>Multiple Resources: 5972 5973* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5974* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5975* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5976* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5977* [Citation](citation.html): A use context type and value assigned to the citation 5978* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5979* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5980* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5981* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5982* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5983* [Evidence](evidence.html): A use context type and value assigned to the evidence 5984* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5985* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5986* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5987* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5988* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5989* [Library](library.html): A use context type and value assigned to the library 5990* [Measure](measure.html): A use context type and value assigned to the measure 5991* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5992* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5993* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5994* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5995* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5996* [Requirements](requirements.html): A use context type and value assigned to the requirements 5997* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5998* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5999* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 6000* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 6001* [TestScript](testscript.html): A use context type and value assigned to the test script 6002* [ValueSet](valueset.html): A use context type and value assigned to the value set 6003</b><br> 6004 * Type: <b>composite</b><br> 6005 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6006 * </p> 6007 */ 6008 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 6009 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 6010 /** 6011 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 6012 * <p> 6013 * Description: <b>Multiple Resources: 6014 6015* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 6016* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 6017* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 6018* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 6019* [Citation](citation.html): A use context type and value assigned to the citation 6020* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 6021* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 6022* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 6023* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 6024* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 6025* [Evidence](evidence.html): A use context type and value assigned to the evidence 6026* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 6027* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 6028* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 6029* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 6030* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 6031* [Library](library.html): A use context type and value assigned to the library 6032* [Measure](measure.html): A use context type and value assigned to the measure 6033* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 6034* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 6035* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 6036* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 6037* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 6038* [Requirements](requirements.html): A use context type and value assigned to the requirements 6039* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 6040* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 6041* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 6042* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 6043* [TestScript](testscript.html): A use context type and value assigned to the test script 6044* [ValueSet](valueset.html): A use context type and value assigned to the value set 6045</b><br> 6046 * Type: <b>composite</b><br> 6047 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6048 * </p> 6049 */ 6050 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 6051 6052 /** 6053 * Search parameter: <b>context-type</b> 6054 * <p> 6055 * Description: <b>Multiple Resources: 6056 6057* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 6058* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 6059* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 6060* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 6061* [Citation](citation.html): A type of use context assigned to the citation 6062* [CodeSystem](codesystem.html): A type of use context assigned to the code system 6063* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 6064* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 6065* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 6066* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 6067* [Evidence](evidence.html): A type of use context assigned to the evidence 6068* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 6069* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 6070* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 6071* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 6072* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 6073* [Library](library.html): A type of use context assigned to the library 6074* [Measure](measure.html): A type of use context assigned to the measure 6075* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 6076* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 6077* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 6078* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 6079* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 6080* [Requirements](requirements.html): A type of use context assigned to the requirements 6081* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 6082* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 6083* [StructureMap](structuremap.html): A type of use context assigned to the structure map 6084* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 6085* [TestScript](testscript.html): A type of use context assigned to the test script 6086* [ValueSet](valueset.html): A type of use context assigned to the value set 6087</b><br> 6088 * Type: <b>token</b><br> 6089 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 6090 * </p> 6091 */ 6092 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 6093 public static final String SP_CONTEXT_TYPE = "context-type"; 6094 /** 6095 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6096 * <p> 6097 * Description: <b>Multiple Resources: 6098 6099* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 6100* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 6101* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 6102* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 6103* [Citation](citation.html): A type of use context assigned to the citation 6104* [CodeSystem](codesystem.html): A type of use context assigned to the code system 6105* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 6106* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 6107* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 6108* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 6109* [Evidence](evidence.html): A type of use context assigned to the evidence 6110* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 6111* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 6112* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 6113* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 6114* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 6115* [Library](library.html): A type of use context assigned to the library 6116* [Measure](measure.html): A type of use context assigned to the measure 6117* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 6118* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 6119* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 6120* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 6121* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 6122* [Requirements](requirements.html): A type of use context assigned to the requirements 6123* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 6124* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 6125* [StructureMap](structuremap.html): A type of use context assigned to the structure map 6126* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 6127* [TestScript](testscript.html): A type of use context assigned to the test script 6128* [ValueSet](valueset.html): A type of use context assigned to the value set 6129</b><br> 6130 * Type: <b>token</b><br> 6131 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 6132 * </p> 6133 */ 6134 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 6135 6136 /** 6137 * Search parameter: <b>context</b> 6138 * <p> 6139 * Description: <b>Multiple Resources: 6140 6141* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 6142* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 6143* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 6144* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 6145* [Citation](citation.html): A use context assigned to the citation 6146* [CodeSystem](codesystem.html): A use context assigned to the code system 6147* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 6148* [ConceptMap](conceptmap.html): A use context assigned to the concept map 6149* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 6150* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 6151* [Evidence](evidence.html): A use context assigned to the evidence 6152* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 6153* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 6154* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 6155* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 6156* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 6157* [Library](library.html): A use context assigned to the library 6158* [Measure](measure.html): A use context assigned to the measure 6159* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 6160* [NamingSystem](namingsystem.html): A use context assigned to the naming system 6161* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 6162* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 6163* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 6164* [Requirements](requirements.html): A use context assigned to the requirements 6165* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 6166* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 6167* [StructureMap](structuremap.html): A use context assigned to the structure map 6168* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 6169* [TestScript](testscript.html): A use context assigned to the test script 6170* [ValueSet](valueset.html): A use context assigned to the value set 6171</b><br> 6172 * Type: <b>token</b><br> 6173 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 6174 * </p> 6175 */ 6176 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 6177 public static final String SP_CONTEXT = "context"; 6178 /** 6179 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6180 * <p> 6181 * Description: <b>Multiple Resources: 6182 6183* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 6184* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 6185* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 6186* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 6187* [Citation](citation.html): A use context assigned to the citation 6188* [CodeSystem](codesystem.html): A use context assigned to the code system 6189* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 6190* [ConceptMap](conceptmap.html): A use context assigned to the concept map 6191* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 6192* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 6193* [Evidence](evidence.html): A use context assigned to the evidence 6194* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 6195* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 6196* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 6197* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 6198* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 6199* [Library](library.html): A use context assigned to the library 6200* [Measure](measure.html): A use context assigned to the measure 6201* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 6202* [NamingSystem](namingsystem.html): A use context assigned to the naming system 6203* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 6204* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 6205* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 6206* [Requirements](requirements.html): A use context assigned to the requirements 6207* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 6208* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 6209* [StructureMap](structuremap.html): A use context assigned to the structure map 6210* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 6211* [TestScript](testscript.html): A use context assigned to the test script 6212* [ValueSet](valueset.html): A use context assigned to the value set 6213</b><br> 6214 * Type: <b>token</b><br> 6215 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 6216 * </p> 6217 */ 6218 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 6219 6220 /** 6221 * Search parameter: <b>date</b> 6222 * <p> 6223 * Description: <b>Multiple Resources: 6224 6225* [ActivityDefinition](activitydefinition.html): The activity definition publication date 6226* [ActorDefinition](actordefinition.html): The Actor Definition publication date 6227* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 6228* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 6229* [Citation](citation.html): The citation publication date 6230* [CodeSystem](codesystem.html): The code system publication date 6231* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 6232* [ConceptMap](conceptmap.html): The concept map publication date 6233* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 6234* [EventDefinition](eventdefinition.html): The event definition publication date 6235* [Evidence](evidence.html): The evidence publication date 6236* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 6237* [ExampleScenario](examplescenario.html): The example scenario publication date 6238* [GraphDefinition](graphdefinition.html): The graph definition publication date 6239* [ImplementationGuide](implementationguide.html): The implementation guide publication date 6240* [Library](library.html): The library publication date 6241* [Measure](measure.html): The measure publication date 6242* [MessageDefinition](messagedefinition.html): The message definition publication date 6243* [NamingSystem](namingsystem.html): The naming system publication date 6244* [OperationDefinition](operationdefinition.html): The operation definition publication date 6245* [PlanDefinition](plandefinition.html): The plan definition publication date 6246* [Questionnaire](questionnaire.html): The questionnaire publication date 6247* [Requirements](requirements.html): The requirements publication date 6248* [SearchParameter](searchparameter.html): The search parameter publication date 6249* [StructureDefinition](structuredefinition.html): The structure definition publication date 6250* [StructureMap](structuremap.html): The structure map publication date 6251* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 6252* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 6253* [TestScript](testscript.html): The test script publication date 6254* [ValueSet](valueset.html): The value set publication date 6255</b><br> 6256 * Type: <b>date</b><br> 6257 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 6258 * </p> 6259 */ 6260 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 6261 public static final String SP_DATE = "date"; 6262 /** 6263 * <b>Fluent Client</b> search parameter constant for <b>date</b> 6264 * <p> 6265 * Description: <b>Multiple Resources: 6266 6267* [ActivityDefinition](activitydefinition.html): The activity definition publication date 6268* [ActorDefinition](actordefinition.html): The Actor Definition publication date 6269* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 6270* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 6271* [Citation](citation.html): The citation publication date 6272* [CodeSystem](codesystem.html): The code system publication date 6273* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 6274* [ConceptMap](conceptmap.html): The concept map publication date 6275* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 6276* [EventDefinition](eventdefinition.html): The event definition publication date 6277* [Evidence](evidence.html): The evidence publication date 6278* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 6279* [ExampleScenario](examplescenario.html): The example scenario publication date 6280* [GraphDefinition](graphdefinition.html): The graph definition publication date 6281* [ImplementationGuide](implementationguide.html): The implementation guide publication date 6282* [Library](library.html): The library publication date 6283* [Measure](measure.html): The measure publication date 6284* [MessageDefinition](messagedefinition.html): The message definition publication date 6285* [NamingSystem](namingsystem.html): The naming system publication date 6286* [OperationDefinition](operationdefinition.html): The operation definition publication date 6287* [PlanDefinition](plandefinition.html): The plan definition publication date 6288* [Questionnaire](questionnaire.html): The questionnaire publication date 6289* [Requirements](requirements.html): The requirements publication date 6290* [SearchParameter](searchparameter.html): The search parameter publication date 6291* [StructureDefinition](structuredefinition.html): The structure definition publication date 6292* [StructureMap](structuremap.html): The structure map publication date 6293* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 6294* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 6295* [TestScript](testscript.html): The test script publication date 6296* [ValueSet](valueset.html): The value set publication date 6297</b><br> 6298 * Type: <b>date</b><br> 6299 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 6300 * </p> 6301 */ 6302 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 6303 6304 /** 6305 * Search parameter: <b>description</b> 6306 * <p> 6307 * Description: <b>Multiple Resources: 6308 6309* [ActivityDefinition](activitydefinition.html): The description of the activity definition 6310* [ActorDefinition](actordefinition.html): The description of the Actor Definition 6311* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 6312* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 6313* [Citation](citation.html): The description of the citation 6314* [CodeSystem](codesystem.html): The description of the code system 6315* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 6316* [ConceptMap](conceptmap.html): The description of the concept map 6317* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 6318* [EventDefinition](eventdefinition.html): The description of the event definition 6319* [Evidence](evidence.html): The description of the evidence 6320* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 6321* [GraphDefinition](graphdefinition.html): The description of the graph definition 6322* [ImplementationGuide](implementationguide.html): The description of the implementation guide 6323* [Library](library.html): The description of the library 6324* [Measure](measure.html): The description of the measure 6325* [MessageDefinition](messagedefinition.html): The description of the message definition 6326* [NamingSystem](namingsystem.html): The description of the naming system 6327* [OperationDefinition](operationdefinition.html): The description of the operation definition 6328* [PlanDefinition](plandefinition.html): The description of the plan definition 6329* [Questionnaire](questionnaire.html): The description of the questionnaire 6330* [Requirements](requirements.html): The description of the requirements 6331* [SearchParameter](searchparameter.html): The description of the search parameter 6332* [StructureDefinition](structuredefinition.html): The description of the structure definition 6333* [StructureMap](structuremap.html): The description of the structure map 6334* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 6335* [TestScript](testscript.html): The description of the test script 6336* [ValueSet](valueset.html): The description of the value set 6337</b><br> 6338 * Type: <b>string</b><br> 6339 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 6340 * </p> 6341 */ 6342 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 6343 public static final String SP_DESCRIPTION = "description"; 6344 /** 6345 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6346 * <p> 6347 * Description: <b>Multiple Resources: 6348 6349* [ActivityDefinition](activitydefinition.html): The description of the activity definition 6350* [ActorDefinition](actordefinition.html): The description of the Actor Definition 6351* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 6352* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 6353* [Citation](citation.html): The description of the citation 6354* [CodeSystem](codesystem.html): The description of the code system 6355* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 6356* [ConceptMap](conceptmap.html): The description of the concept map 6357* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 6358* [EventDefinition](eventdefinition.html): The description of the event definition 6359* [Evidence](evidence.html): The description of the evidence 6360* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 6361* [GraphDefinition](graphdefinition.html): The description of the graph definition 6362* [ImplementationGuide](implementationguide.html): The description of the implementation guide 6363* [Library](library.html): The description of the library 6364* [Measure](measure.html): The description of the measure 6365* [MessageDefinition](messagedefinition.html): The description of the message definition 6366* [NamingSystem](namingsystem.html): The description of the naming system 6367* [OperationDefinition](operationdefinition.html): The description of the operation definition 6368* [PlanDefinition](plandefinition.html): The description of the plan definition 6369* [Questionnaire](questionnaire.html): The description of the questionnaire 6370* [Requirements](requirements.html): The description of the requirements 6371* [SearchParameter](searchparameter.html): The description of the search parameter 6372* [StructureDefinition](structuredefinition.html): The description of the structure definition 6373* [StructureMap](structuremap.html): The description of the structure map 6374* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 6375* [TestScript](testscript.html): The description of the test script 6376* [ValueSet](valueset.html): The description of the value set 6377</b><br> 6378 * Type: <b>string</b><br> 6379 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 6380 * </p> 6381 */ 6382 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 6383 6384 /** 6385 * Search parameter: <b>identifier</b> 6386 * <p> 6387 * Description: <b>Multiple Resources: 6388 6389* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6390* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6391* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6392* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6393* [Citation](citation.html): External identifier for the citation 6394* [CodeSystem](codesystem.html): External identifier for the code system 6395* [ConceptMap](conceptmap.html): External identifier for the concept map 6396* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6397* [EventDefinition](eventdefinition.html): External identifier for the event definition 6398* [Evidence](evidence.html): External identifier for the evidence 6399* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6400* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6401* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6402* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6403* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6404* [Library](library.html): External identifier for the library 6405* [Measure](measure.html): External identifier for the measure 6406* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6407* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6408* [NamingSystem](namingsystem.html): External identifier for the naming system 6409* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6410* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6411* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6412* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6413* [Requirements](requirements.html): External identifier for the requirements 6414* [SearchParameter](searchparameter.html): External identifier for the search parameter 6415* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6416* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6417* [StructureMap](structuremap.html): External identifier for the structure map 6418* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6419* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6420* [TestPlan](testplan.html): An identifier for the test plan 6421* [TestScript](testscript.html): External identifier for the test script 6422* [ValueSet](valueset.html): External identifier for the value set 6423</b><br> 6424 * Type: <b>token</b><br> 6425 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6426 * </p> 6427 */ 6428 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 6429 public static final String SP_IDENTIFIER = "identifier"; 6430 /** 6431 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6432 * <p> 6433 * Description: <b>Multiple Resources: 6434 6435* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6436* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6437* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6438* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6439* [Citation](citation.html): External identifier for the citation 6440* [CodeSystem](codesystem.html): External identifier for the code system 6441* [ConceptMap](conceptmap.html): External identifier for the concept map 6442* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6443* [EventDefinition](eventdefinition.html): External identifier for the event definition 6444* [Evidence](evidence.html): External identifier for the evidence 6445* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6446* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6447* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6448* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6449* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6450* [Library](library.html): External identifier for the library 6451* [Measure](measure.html): External identifier for the measure 6452* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6453* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6454* [NamingSystem](namingsystem.html): External identifier for the naming system 6455* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6456* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6457* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6458* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6459* [Requirements](requirements.html): External identifier for the requirements 6460* [SearchParameter](searchparameter.html): External identifier for the search parameter 6461* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6462* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6463* [StructureMap](structuremap.html): External identifier for the structure map 6464* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6465* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6466* [TestPlan](testplan.html): An identifier for the test plan 6467* [TestScript](testscript.html): External identifier for the test script 6468* [ValueSet](valueset.html): External identifier for the value set 6469</b><br> 6470 * Type: <b>token</b><br> 6471 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6472 * </p> 6473 */ 6474 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6475 6476 /** 6477 * Search parameter: <b>jurisdiction</b> 6478 * <p> 6479 * Description: <b>Multiple Resources: 6480 6481* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 6482* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 6483* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 6484* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 6485* [Citation](citation.html): Intended jurisdiction for the citation 6486* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 6487* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 6488* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 6489* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 6490* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 6491* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 6492* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 6493* [Library](library.html): Intended jurisdiction for the library 6494* [Measure](measure.html): Intended jurisdiction for the measure 6495* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 6496* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 6497* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 6498* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 6499* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 6500* [Requirements](requirements.html): Intended jurisdiction for the requirements 6501* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 6502* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 6503* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 6504* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 6505* [TestScript](testscript.html): Intended jurisdiction for the test script 6506* [ValueSet](valueset.html): Intended jurisdiction for the value set 6507</b><br> 6508 * Type: <b>token</b><br> 6509 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 6510 * </p> 6511 */ 6512 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 6513 public static final String SP_JURISDICTION = "jurisdiction"; 6514 /** 6515 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6516 * <p> 6517 * Description: <b>Multiple Resources: 6518 6519* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 6520* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 6521* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 6522* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 6523* [Citation](citation.html): Intended jurisdiction for the citation 6524* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 6525* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 6526* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 6527* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 6528* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 6529* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 6530* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 6531* [Library](library.html): Intended jurisdiction for the library 6532* [Measure](measure.html): Intended jurisdiction for the measure 6533* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 6534* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 6535* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 6536* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 6537* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 6538* [Requirements](requirements.html): Intended jurisdiction for the requirements 6539* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 6540* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 6541* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 6542* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 6543* [TestScript](testscript.html): Intended jurisdiction for the test script 6544* [ValueSet](valueset.html): Intended jurisdiction for the value set 6545</b><br> 6546 * Type: <b>token</b><br> 6547 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 6548 * </p> 6549 */ 6550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 6551 6552 /** 6553 * Search parameter: <b>name</b> 6554 * <p> 6555 * Description: <b>Multiple Resources: 6556 6557* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6558* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6559* [Citation](citation.html): Computationally friendly name of the citation 6560* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6561* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6562* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6563* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6564* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6565* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6566* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6567* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6568* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6569* [Library](library.html): Computationally friendly name of the library 6570* [Measure](measure.html): Computationally friendly name of the measure 6571* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6572* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6573* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6574* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6575* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6576* [Requirements](requirements.html): Computationally friendly name of the requirements 6577* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6578* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6579* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6580* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6581* [TestScript](testscript.html): Computationally friendly name of the test script 6582* [ValueSet](valueset.html): Computationally friendly name of the value set 6583</b><br> 6584 * Type: <b>string</b><br> 6585 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6586 * </p> 6587 */ 6588 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 6589 public static final String SP_NAME = "name"; 6590 /** 6591 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6592 * <p> 6593 * Description: <b>Multiple Resources: 6594 6595* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6596* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6597* [Citation](citation.html): Computationally friendly name of the citation 6598* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6599* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6600* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6601* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6602* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6603* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6604* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6605* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6606* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6607* [Library](library.html): Computationally friendly name of the library 6608* [Measure](measure.html): Computationally friendly name of the measure 6609* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6610* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6611* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6612* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6613* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6614* [Requirements](requirements.html): Computationally friendly name of the requirements 6615* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6616* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6617* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6618* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6619* [TestScript](testscript.html): Computationally friendly name of the test script 6620* [ValueSet](valueset.html): Computationally friendly name of the value set 6621</b><br> 6622 * Type: <b>string</b><br> 6623 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6624 * </p> 6625 */ 6626 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 6627 6628 /** 6629 * Search parameter: <b>publisher</b> 6630 * <p> 6631 * Description: <b>Multiple Resources: 6632 6633* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6634* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6635* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6636* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6637* [Citation](citation.html): Name of the publisher of the citation 6638* [CodeSystem](codesystem.html): Name of the publisher of the code system 6639* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6640* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6641* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6642* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6643* [Evidence](evidence.html): Name of the publisher of the evidence 6644* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6645* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6646* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6647* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6648* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6649* [Library](library.html): Name of the publisher of the library 6650* [Measure](measure.html): Name of the publisher of the measure 6651* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6652* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6653* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6654* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6655* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6656* [Requirements](requirements.html): Name of the publisher of the requirements 6657* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6658* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6659* [StructureMap](structuremap.html): Name of the publisher of the structure map 6660* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6661* [TestScript](testscript.html): Name of the publisher of the test script 6662* [ValueSet](valueset.html): Name of the publisher of the value set 6663</b><br> 6664 * Type: <b>string</b><br> 6665 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6666 * </p> 6667 */ 6668 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 6669 public static final String SP_PUBLISHER = "publisher"; 6670 /** 6671 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6672 * <p> 6673 * Description: <b>Multiple Resources: 6674 6675* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6676* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6677* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6678* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6679* [Citation](citation.html): Name of the publisher of the citation 6680* [CodeSystem](codesystem.html): Name of the publisher of the code system 6681* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6682* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6683* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6684* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6685* [Evidence](evidence.html): Name of the publisher of the evidence 6686* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6687* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6688* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6689* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6690* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6691* [Library](library.html): Name of the publisher of the library 6692* [Measure](measure.html): Name of the publisher of the measure 6693* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6694* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6695* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6696* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6697* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6698* [Requirements](requirements.html): Name of the publisher of the requirements 6699* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6700* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6701* [StructureMap](structuremap.html): Name of the publisher of the structure map 6702* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6703* [TestScript](testscript.html): Name of the publisher of the test script 6704* [ValueSet](valueset.html): Name of the publisher of the value set 6705</b><br> 6706 * Type: <b>string</b><br> 6707 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6708 * </p> 6709 */ 6710 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 6711 6712 /** 6713 * Search parameter: <b>status</b> 6714 * <p> 6715 * Description: <b>Multiple Resources: 6716 6717* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6718* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6719* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6720* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6721* [Citation](citation.html): The current status of the citation 6722* [CodeSystem](codesystem.html): The current status of the code system 6723* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6724* [ConceptMap](conceptmap.html): The current status of the concept map 6725* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6726* [EventDefinition](eventdefinition.html): The current status of the event definition 6727* [Evidence](evidence.html): The current status of the evidence 6728* [EvidenceReport](evidencereport.html): The current status of the evidence report 6729* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6730* [ExampleScenario](examplescenario.html): The current status of the example scenario 6731* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6732* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6733* [Library](library.html): The current status of the library 6734* [Measure](measure.html): The current status of the measure 6735* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6736* [MessageDefinition](messagedefinition.html): The current status of the message definition 6737* [NamingSystem](namingsystem.html): The current status of the naming system 6738* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6739* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6740* [PlanDefinition](plandefinition.html): The current status of the plan definition 6741* [Questionnaire](questionnaire.html): The current status of the questionnaire 6742* [Requirements](requirements.html): The current status of the requirements 6743* [SearchParameter](searchparameter.html): The current status of the search parameter 6744* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6745* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6746* [StructureMap](structuremap.html): The current status of the structure map 6747* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6748* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6749* [TestPlan](testplan.html): The current status of the test plan 6750* [TestScript](testscript.html): The current status of the test script 6751* [ValueSet](valueset.html): The current status of the value set 6752</b><br> 6753 * Type: <b>token</b><br> 6754 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6755 * </p> 6756 */ 6757 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 6758 public static final String SP_STATUS = "status"; 6759 /** 6760 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6761 * <p> 6762 * Description: <b>Multiple Resources: 6763 6764* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6765* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6766* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6767* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6768* [Citation](citation.html): The current status of the citation 6769* [CodeSystem](codesystem.html): The current status of the code system 6770* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6771* [ConceptMap](conceptmap.html): The current status of the concept map 6772* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6773* [EventDefinition](eventdefinition.html): The current status of the event definition 6774* [Evidence](evidence.html): The current status of the evidence 6775* [EvidenceReport](evidencereport.html): The current status of the evidence report 6776* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6777* [ExampleScenario](examplescenario.html): The current status of the example scenario 6778* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6779* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6780* [Library](library.html): The current status of the library 6781* [Measure](measure.html): The current status of the measure 6782* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6783* [MessageDefinition](messagedefinition.html): The current status of the message definition 6784* [NamingSystem](namingsystem.html): The current status of the naming system 6785* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6786* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6787* [PlanDefinition](plandefinition.html): The current status of the plan definition 6788* [Questionnaire](questionnaire.html): The current status of the questionnaire 6789* [Requirements](requirements.html): The current status of the requirements 6790* [SearchParameter](searchparameter.html): The current status of the search parameter 6791* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6792* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6793* [StructureMap](structuremap.html): The current status of the structure map 6794* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6795* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6796* [TestPlan](testplan.html): The current status of the test plan 6797* [TestScript](testscript.html): The current status of the test script 6798* [ValueSet](valueset.html): The current status of the value set 6799</b><br> 6800 * Type: <b>token</b><br> 6801 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6802 * </p> 6803 */ 6804 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6805 6806 /** 6807 * Search parameter: <b>title</b> 6808 * <p> 6809 * Description: <b>Multiple Resources: 6810 6811* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6812* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6813* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6814* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6815* [Citation](citation.html): The human-friendly name of the citation 6816* [CodeSystem](codesystem.html): The human-friendly name of the code system 6817* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6818* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6819* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6820* [Evidence](evidence.html): The human-friendly name of the evidence 6821* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6822* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6823* [Library](library.html): The human-friendly name of the library 6824* [Measure](measure.html): The human-friendly name of the measure 6825* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6826* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6827* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6828* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6829* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6830* [Requirements](requirements.html): The human-friendly name of the requirements 6831* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6832* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6833* [StructureMap](structuremap.html): The human-friendly name of the structure map 6834* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6835* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6836* [TestScript](testscript.html): The human-friendly name of the test script 6837* [ValueSet](valueset.html): The human-friendly name of the value set 6838</b><br> 6839 * Type: <b>string</b><br> 6840 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6841 * </p> 6842 */ 6843 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 6844 public static final String SP_TITLE = "title"; 6845 /** 6846 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6847 * <p> 6848 * Description: <b>Multiple Resources: 6849 6850* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6851* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6852* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6853* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6854* [Citation](citation.html): The human-friendly name of the citation 6855* [CodeSystem](codesystem.html): The human-friendly name of the code system 6856* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6857* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6858* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6859* [Evidence](evidence.html): The human-friendly name of the evidence 6860* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6861* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6862* [Library](library.html): The human-friendly name of the library 6863* [Measure](measure.html): The human-friendly name of the measure 6864* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6865* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6866* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6867* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6868* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6869* [Requirements](requirements.html): The human-friendly name of the requirements 6870* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6871* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6872* [StructureMap](structuremap.html): The human-friendly name of the structure map 6873* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6874* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6875* [TestScript](testscript.html): The human-friendly name of the test script 6876* [ValueSet](valueset.html): The human-friendly name of the value set 6877</b><br> 6878 * Type: <b>string</b><br> 6879 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6880 * </p> 6881 */ 6882 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 6883 6884 /** 6885 * Search parameter: <b>url</b> 6886 * <p> 6887 * Description: <b>Multiple Resources: 6888 6889* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6890* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6891* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6892* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6893* [Citation](citation.html): The uri that identifies the citation 6894* [CodeSystem](codesystem.html): The uri that identifies the code system 6895* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6896* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6897* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6898* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6899* [Evidence](evidence.html): The uri that identifies the evidence 6900* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6901* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6902* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6903* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6904* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6905* [Library](library.html): The uri that identifies the library 6906* [Measure](measure.html): The uri that identifies the measure 6907* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6908* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6909* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6910* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6911* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6912* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6913* [Requirements](requirements.html): The uri that identifies the requirements 6914* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6915* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6916* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6917* [StructureMap](structuremap.html): The uri that identifies the structure map 6918* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6919* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6920* [TestPlan](testplan.html): The uri that identifies the test plan 6921* [TestScript](testscript.html): The uri that identifies the test script 6922* [ValueSet](valueset.html): The uri that identifies the value set 6923</b><br> 6924 * Type: <b>uri</b><br> 6925 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6926 * </p> 6927 */ 6928 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 6929 public static final String SP_URL = "url"; 6930 /** 6931 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6932 * <p> 6933 * Description: <b>Multiple Resources: 6934 6935* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6936* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6937* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6938* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6939* [Citation](citation.html): The uri that identifies the citation 6940* [CodeSystem](codesystem.html): The uri that identifies the code system 6941* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6942* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6943* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6944* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6945* [Evidence](evidence.html): The uri that identifies the evidence 6946* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6947* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6948* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6949* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6950* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6951* [Library](library.html): The uri that identifies the library 6952* [Measure](measure.html): The uri that identifies the measure 6953* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6954* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6955* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6956* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6957* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6958* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6959* [Requirements](requirements.html): The uri that identifies the requirements 6960* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6961* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6962* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6963* [StructureMap](structuremap.html): The uri that identifies the structure map 6964* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6965* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6966* [TestPlan](testplan.html): The uri that identifies the test plan 6967* [TestScript](testscript.html): The uri that identifies the test script 6968* [ValueSet](valueset.html): The uri that identifies the value set 6969</b><br> 6970 * Type: <b>uri</b><br> 6971 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6972 * </p> 6973 */ 6974 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6975 6976 /** 6977 * Search parameter: <b>version</b> 6978 * <p> 6979 * Description: <b>Multiple Resources: 6980 6981* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6982* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6983* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6984* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6985* [Citation](citation.html): The business version of the citation 6986* [CodeSystem](codesystem.html): The business version of the code system 6987* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6988* [ConceptMap](conceptmap.html): The business version of the concept map 6989* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6990* [EventDefinition](eventdefinition.html): The business version of the event definition 6991* [Evidence](evidence.html): The business version of the evidence 6992* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6993* [ExampleScenario](examplescenario.html): The business version of the example scenario 6994* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6995* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6996* [Library](library.html): The business version of the library 6997* [Measure](measure.html): The business version of the measure 6998* [MessageDefinition](messagedefinition.html): The business version of the message definition 6999* [NamingSystem](namingsystem.html): The business version of the naming system 7000* [OperationDefinition](operationdefinition.html): The business version of the operation definition 7001* [PlanDefinition](plandefinition.html): The business version of the plan definition 7002* [Questionnaire](questionnaire.html): The business version of the questionnaire 7003* [Requirements](requirements.html): The business version of the requirements 7004* [SearchParameter](searchparameter.html): The business version of the search parameter 7005* [StructureDefinition](structuredefinition.html): The business version of the structure definition 7006* [StructureMap](structuremap.html): The business version of the structure map 7007* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 7008* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 7009* [TestScript](testscript.html): The business version of the test script 7010* [ValueSet](valueset.html): The business version of the value set 7011</b><br> 7012 * Type: <b>token</b><br> 7013 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 7014 * </p> 7015 */ 7016 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 7017 public static final String SP_VERSION = "version"; 7018 /** 7019 * <b>Fluent Client</b> search parameter constant for <b>version</b> 7020 * <p> 7021 * Description: <b>Multiple Resources: 7022 7023* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 7024* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 7025* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 7026* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 7027* [Citation](citation.html): The business version of the citation 7028* [CodeSystem](codesystem.html): The business version of the code system 7029* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 7030* [ConceptMap](conceptmap.html): The business version of the concept map 7031* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 7032* [EventDefinition](eventdefinition.html): The business version of the event definition 7033* [Evidence](evidence.html): The business version of the evidence 7034* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 7035* [ExampleScenario](examplescenario.html): The business version of the example scenario 7036* [GraphDefinition](graphdefinition.html): The business version of the graph definition 7037* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 7038* [Library](library.html): The business version of the library 7039* [Measure](measure.html): The business version of the measure 7040* [MessageDefinition](messagedefinition.html): The business version of the message definition 7041* [NamingSystem](namingsystem.html): The business version of the naming system 7042* [OperationDefinition](operationdefinition.html): The business version of the operation definition 7043* [PlanDefinition](plandefinition.html): The business version of the plan definition 7044* [Questionnaire](questionnaire.html): The business version of the questionnaire 7045* [Requirements](requirements.html): The business version of the requirements 7046* [SearchParameter](searchparameter.html): The business version of the search parameter 7047* [StructureDefinition](structuredefinition.html): The business version of the structure definition 7048* [StructureMap](structuremap.html): The business version of the structure map 7049* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 7050* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 7051* [TestScript](testscript.html): The business version of the test script 7052* [ValueSet](valueset.html): The business version of the value set 7053</b><br> 7054 * Type: <b>token</b><br> 7055 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 7056 * </p> 7057 */ 7058 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 7059 7060 /** 7061 * Search parameter: <b>effective</b> 7062 * <p> 7063 * Description: <b>Multiple Resources: 7064 7065* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 7066* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 7067* [Citation](citation.html): The time during which the citation is intended to be in use 7068* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 7069* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 7070* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 7071* [Library](library.html): The time during which the library is intended to be in use 7072* [Measure](measure.html): The time during which the measure is intended to be in use 7073* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 7074* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 7075* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 7076* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 7077</b><br> 7078 * Type: <b>date</b><br> 7079 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 7080 * </p> 7081 */ 7082 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 7083 public static final String SP_EFFECTIVE = "effective"; 7084 /** 7085 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 7086 * <p> 7087 * Description: <b>Multiple Resources: 7088 7089* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 7090* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 7091* [Citation](citation.html): The time during which the citation is intended to be in use 7092* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 7093* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 7094* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 7095* [Library](library.html): The time during which the library is intended to be in use 7096* [Measure](measure.html): The time during which the measure is intended to be in use 7097* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 7098* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 7099* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 7100* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 7101</b><br> 7102 * Type: <b>date</b><br> 7103 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 7104 * </p> 7105 */ 7106 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 7107 7108 /** 7109 * Search parameter: <b>combo-code</b> 7110 * <p> 7111 * Description: <b>A code that corresponds to one of its items in the questionnaire</b><br> 7112 * Type: <b>token</b><br> 7113 * Path: <b>Questionnaire.code | Questionnaire.item.code</b><br> 7114 * </p> 7115 */ 7116 @SearchParamDefinition(name="combo-code", path="Questionnaire.code | Questionnaire.item.code", description="A code that corresponds to one of its items in the questionnaire", type="token" ) 7117 public static final String SP_COMBO_CODE = "combo-code"; 7118 /** 7119 * <b>Fluent Client</b> search parameter constant for <b>combo-code</b> 7120 * <p> 7121 * Description: <b>A code that corresponds to one of its items in the questionnaire</b><br> 7122 * Type: <b>token</b><br> 7123 * Path: <b>Questionnaire.code | Questionnaire.item.code</b><br> 7124 * </p> 7125 */ 7126 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_CODE); 7127 7128 /** 7129 * Search parameter: <b>definition</b> 7130 * <p> 7131 * Description: <b>ElementDefinition - details for the item</b><br> 7132 * Type: <b>uri</b><br> 7133 * Path: <b>Questionnaire.item.definition</b><br> 7134 * </p> 7135 */ 7136 @SearchParamDefinition(name="definition", path="Questionnaire.item.definition", description="ElementDefinition - details for the item", type="uri" ) 7137 public static final String SP_DEFINITION = "definition"; 7138 /** 7139 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 7140 * <p> 7141 * Description: <b>ElementDefinition - details for the item</b><br> 7142 * Type: <b>uri</b><br> 7143 * Path: <b>Questionnaire.item.definition</b><br> 7144 * </p> 7145 */ 7146 public static final ca.uhn.fhir.rest.gclient.UriClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DEFINITION); 7147 7148 /** 7149 * Search parameter: <b>item-code</b> 7150 * <p> 7151 * Description: <b>A code that corresponds to one of the items in the questionnaire</b><br> 7152 * Type: <b>token</b><br> 7153 * Path: <b>Questionnaire.item.code</b><br> 7154 * </p> 7155 */ 7156 @SearchParamDefinition(name="item-code", path="Questionnaire.item.code", description="A code that corresponds to one of the items in the questionnaire", type="token" ) 7157 public static final String SP_ITEM_CODE = "item-code"; 7158 /** 7159 * <b>Fluent Client</b> search parameter constant for <b>item-code</b> 7160 * <p> 7161 * Description: <b>A code that corresponds to one of the items in the questionnaire</b><br> 7162 * Type: <b>token</b><br> 7163 * Path: <b>Questionnaire.item.code</b><br> 7164 * </p> 7165 */ 7166 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ITEM_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ITEM_CODE); 7167 7168 /** 7169 * Search parameter: <b>questionnaire-code</b> 7170 * <p> 7171 * Description: <b>A code that matches one of the Questionnaire.code codings</b><br> 7172 * Type: <b>token</b><br> 7173 * Path: <b>Questionnaire.code</b><br> 7174 * </p> 7175 */ 7176 @SearchParamDefinition(name="questionnaire-code", path="Questionnaire.code", description="A code that matches one of the Questionnaire.code codings", type="token" ) 7177 public static final String SP_QUESTIONNAIRE_CODE = "questionnaire-code"; 7178 /** 7179 * <b>Fluent Client</b> search parameter constant for <b>questionnaire-code</b> 7180 * <p> 7181 * Description: <b>A code that matches one of the Questionnaire.code codings</b><br> 7182 * Type: <b>token</b><br> 7183 * Path: <b>Questionnaire.code</b><br> 7184 * </p> 7185 */ 7186 public static final ca.uhn.fhir.rest.gclient.TokenClientParam QUESTIONNAIRE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_QUESTIONNAIRE_CODE); 7187 7188 /** 7189 * Search parameter: <b>subject-type</b> 7190 * <p> 7191 * Description: <b>Resource that can be subject of QuestionnaireResponse</b><br> 7192 * Type: <b>token</b><br> 7193 * Path: <b>Questionnaire.subjectType</b><br> 7194 * </p> 7195 */ 7196 @SearchParamDefinition(name="subject-type", path="Questionnaire.subjectType", description="Resource that can be subject of QuestionnaireResponse", type="token" ) 7197 public static final String SP_SUBJECT_TYPE = "subject-type"; 7198 /** 7199 * <b>Fluent Client</b> search parameter constant for <b>subject-type</b> 7200 * <p> 7201 * Description: <b>Resource that can be subject of QuestionnaireResponse</b><br> 7202 * Type: <b>token</b><br> 7203 * Path: <b>Questionnaire.subjectType</b><br> 7204 * </p> 7205 */ 7206 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBJECT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUBJECT_TYPE); 7207 7208// Manual code (from Configuration.txt): 7209public QuestionnaireItemComponent getQuestion(String linkId) { 7210 if (linkId == null) 7211 return null; 7212 for (QuestionnaireItemComponent i : getItem()) { 7213 if (i.getLinkId().equals(linkId)) 7214 return i; 7215 QuestionnaireItemComponent t = i.getQuestion(linkId); 7216 if (t != null) 7217 return t; 7218 } 7219 return null; 7220 } 7221 7222 public QuestionnaireItemComponent getCommonGroup(QuestionnaireItemComponent q1, QuestionnaireItemComponent q2) { 7223 for (QuestionnaireItemComponent i : getItem()) { 7224 QuestionnaireItemComponent t = i.getCommonGroup(q1, q2); 7225 if (t != null) 7226 return t; 7227 } 7228 return null; 7229 } 7230 7231// end addition 7232 7233 7234} 7235