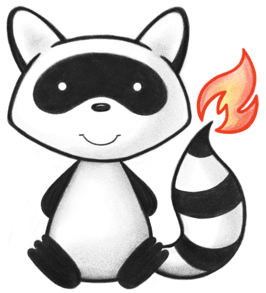
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 052 */ 053@ResourceDef(name="QuestionnaireResponse", profile="http://hl7.org/fhir/StructureDefinition/QuestionnaireResponse") 054public class QuestionnaireResponse extends DomainResource { 055 056 public enum QuestionnaireResponseStatus { 057 /** 058 * This QuestionnaireResponse has been partially filled out with answers but changes or additions are still expected to be made to it. 059 */ 060 INPROGRESS, 061 /** 062 * This QuestionnaireResponse has been filled out with answers and the current content is regarded as definitive. 063 */ 064 COMPLETED, 065 /** 066 * This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards. 067 */ 068 AMENDED, 069 /** 070 * This QuestionnaireResponse was entered in error and voided. 071 */ 072 ENTEREDINERROR, 073 /** 074 * This QuestionnaireResponse has been partially filled out with answers but has been abandoned. No subsequent changes can be made. 075 */ 076 STOPPED, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static QuestionnaireResponseStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("in-progress".equals(codeString)) 085 return INPROGRESS; 086 if ("completed".equals(codeString)) 087 return COMPLETED; 088 if ("amended".equals(codeString)) 089 return AMENDED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("stopped".equals(codeString)) 093 return STOPPED; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case INPROGRESS: return "in-progress"; 102 case COMPLETED: return "completed"; 103 case AMENDED: return "amended"; 104 case ENTEREDINERROR: return "entered-in-error"; 105 case STOPPED: return "stopped"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case INPROGRESS: return "http://hl7.org/fhir/questionnaire-answers-status"; 113 case COMPLETED: return "http://hl7.org/fhir/questionnaire-answers-status"; 114 case AMENDED: return "http://hl7.org/fhir/questionnaire-answers-status"; 115 case ENTEREDINERROR: return "http://hl7.org/fhir/questionnaire-answers-status"; 116 case STOPPED: return "http://hl7.org/fhir/questionnaire-answers-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case INPROGRESS: return "This QuestionnaireResponse has been partially filled out with answers but changes or additions are still expected to be made to it."; 124 case COMPLETED: return "This QuestionnaireResponse has been filled out with answers and the current content is regarded as definitive."; 125 case AMENDED: return "This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards."; 126 case ENTEREDINERROR: return "This QuestionnaireResponse was entered in error and voided."; 127 case STOPPED: return "This QuestionnaireResponse has been partially filled out with answers but has been abandoned. No subsequent changes can be made."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case INPROGRESS: return "In Progress"; 135 case COMPLETED: return "Completed"; 136 case AMENDED: return "Amended"; 137 case ENTEREDINERROR: return "Entered in Error"; 138 case STOPPED: return "Stopped"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class QuestionnaireResponseStatusEnumFactory implements EnumFactory<QuestionnaireResponseStatus> { 146 public QuestionnaireResponseStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("in-progress".equals(codeString)) 151 return QuestionnaireResponseStatus.INPROGRESS; 152 if ("completed".equals(codeString)) 153 return QuestionnaireResponseStatus.COMPLETED; 154 if ("amended".equals(codeString)) 155 return QuestionnaireResponseStatus.AMENDED; 156 if ("entered-in-error".equals(codeString)) 157 return QuestionnaireResponseStatus.ENTEREDINERROR; 158 if ("stopped".equals(codeString)) 159 return QuestionnaireResponseStatus.STOPPED; 160 throw new IllegalArgumentException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 161 } 162 public Enumeration<QuestionnaireResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 170 if ("in-progress".equals(codeString)) 171 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.INPROGRESS, code); 172 if ("completed".equals(codeString)) 173 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.COMPLETED, code); 174 if ("amended".equals(codeString)) 175 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.AMENDED, code); 176 if ("entered-in-error".equals(codeString)) 177 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.ENTEREDINERROR, code); 178 if ("stopped".equals(codeString)) 179 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.STOPPED, code); 180 throw new FHIRException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 181 } 182 public String toCode(QuestionnaireResponseStatus code) { 183 if (code == QuestionnaireResponseStatus.INPROGRESS) 184 return "in-progress"; 185 if (code == QuestionnaireResponseStatus.COMPLETED) 186 return "completed"; 187 if (code == QuestionnaireResponseStatus.AMENDED) 188 return "amended"; 189 if (code == QuestionnaireResponseStatus.ENTEREDINERROR) 190 return "entered-in-error"; 191 if (code == QuestionnaireResponseStatus.STOPPED) 192 return "stopped"; 193 return "?"; 194 } 195 public String toSystem(QuestionnaireResponseStatus code) { 196 return code.getSystem(); 197 } 198 } 199 200 @Block() 201 public static class QuestionnaireResponseItemComponent extends BackboneElement implements IBaseBackboneElement { 202 /** 203 * The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 204 */ 205 @Child(name = "linkId", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 206 @Description(shortDefinition="Pointer to specific item from Questionnaire", formalDefinition="The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource." ) 207 protected StringType linkId; 208 209 /** 210 * A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item. 211 */ 212 @Child(name = "definition", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 213 @Description(shortDefinition="ElementDefinition - details for the item", formalDefinition="A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item." ) 214 protected UriType definition; 215 216 /** 217 * Text that is displayed above the contents of the group or as the text of the question being answered. 218 */ 219 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 220 @Description(shortDefinition="Name for group or question text", formalDefinition="Text that is displayed above the contents of the group or as the text of the question being answered." ) 221 protected StringType text; 222 223 /** 224 * The respondent's answer(s) to the question. 225 */ 226 @Child(name = "answer", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 227 @Description(shortDefinition="The response(s) to the question", formalDefinition="The respondent's answer(s) to the question." ) 228 protected List<QuestionnaireResponseItemAnswerComponent> answer; 229 230 /** 231 * Sub-questions, sub-groups or display items nested beneath a group. 232 */ 233 @Child(name = "item", type = {QuestionnaireResponseItemComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 234 @Description(shortDefinition="Child items of group item", formalDefinition="Sub-questions, sub-groups or display items nested beneath a group." ) 235 protected List<QuestionnaireResponseItemComponent> item; 236 237 private static final long serialVersionUID = -1395483402L; 238 239 /** 240 * Constructor 241 */ 242 public QuestionnaireResponseItemComponent() { 243 super(); 244 } 245 246 /** 247 * Constructor 248 */ 249 public QuestionnaireResponseItemComponent(String linkId) { 250 super(); 251 this.setLinkId(linkId); 252 } 253 254 /** 255 * @return {@link #linkId} (The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 256 */ 257 public StringType getLinkIdElement() { 258 if (this.linkId == null) 259 if (Configuration.errorOnAutoCreate()) 260 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.linkId"); 261 else if (Configuration.doAutoCreate()) 262 this.linkId = new StringType(); // bb 263 return this.linkId; 264 } 265 266 public boolean hasLinkIdElement() { 267 return this.linkId != null && !this.linkId.isEmpty(); 268 } 269 270 public boolean hasLinkId() { 271 return this.linkId != null && !this.linkId.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #linkId} (The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 276 */ 277 public QuestionnaireResponseItemComponent setLinkIdElement(StringType value) { 278 this.linkId = value; 279 return this; 280 } 281 282 /** 283 * @return The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 284 */ 285 public String getLinkId() { 286 return this.linkId == null ? null : this.linkId.getValue(); 287 } 288 289 /** 290 * @param value The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 291 */ 292 public QuestionnaireResponseItemComponent setLinkId(String value) { 293 if (this.linkId == null) 294 this.linkId = new StringType(); 295 this.linkId.setValue(value); 296 return this; 297 } 298 299 /** 300 * @return {@link #definition} (A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 301 */ 302 public UriType getDefinitionElement() { 303 if (this.definition == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.definition"); 306 else if (Configuration.doAutoCreate()) 307 this.definition = new UriType(); // bb 308 return this.definition; 309 } 310 311 public boolean hasDefinitionElement() { 312 return this.definition != null && !this.definition.isEmpty(); 313 } 314 315 public boolean hasDefinition() { 316 return this.definition != null && !this.definition.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #definition} (A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 321 */ 322 public QuestionnaireResponseItemComponent setDefinitionElement(UriType value) { 323 this.definition = value; 324 return this; 325 } 326 327 /** 328 * @return A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item. 329 */ 330 public String getDefinition() { 331 return this.definition == null ? null : this.definition.getValue(); 332 } 333 334 /** 335 * @param value A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item. 336 */ 337 public QuestionnaireResponseItemComponent setDefinition(String value) { 338 if (Utilities.noString(value)) 339 this.definition = null; 340 else { 341 if (this.definition == null) 342 this.definition = new UriType(); 343 this.definition.setValue(value); 344 } 345 return this; 346 } 347 348 /** 349 * @return {@link #text} (Text that is displayed above the contents of the group or as the text of the question being answered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 350 */ 351 public StringType getTextElement() { 352 if (this.text == null) 353 if (Configuration.errorOnAutoCreate()) 354 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.text"); 355 else if (Configuration.doAutoCreate()) 356 this.text = new StringType(); // bb 357 return this.text; 358 } 359 360 public boolean hasTextElement() { 361 return this.text != null && !this.text.isEmpty(); 362 } 363 364 public boolean hasText() { 365 return this.text != null && !this.text.isEmpty(); 366 } 367 368 /** 369 * @param value {@link #text} (Text that is displayed above the contents of the group or as the text of the question being answered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 370 */ 371 public QuestionnaireResponseItemComponent setTextElement(StringType value) { 372 this.text = value; 373 return this; 374 } 375 376 /** 377 * @return Text that is displayed above the contents of the group or as the text of the question being answered. 378 */ 379 public String getText() { 380 return this.text == null ? null : this.text.getValue(); 381 } 382 383 /** 384 * @param value Text that is displayed above the contents of the group or as the text of the question being answered. 385 */ 386 public QuestionnaireResponseItemComponent setText(String value) { 387 if (Utilities.noString(value)) 388 this.text = null; 389 else { 390 if (this.text == null) 391 this.text = new StringType(); 392 this.text.setValue(value); 393 } 394 return this; 395 } 396 397 /** 398 * @return {@link #answer} (The respondent's answer(s) to the question.) 399 */ 400 public List<QuestionnaireResponseItemAnswerComponent> getAnswer() { 401 if (this.answer == null) 402 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 403 return this.answer; 404 } 405 406 /** 407 * @return Returns a reference to <code>this</code> for easy method chaining 408 */ 409 public QuestionnaireResponseItemComponent setAnswer(List<QuestionnaireResponseItemAnswerComponent> theAnswer) { 410 this.answer = theAnswer; 411 return this; 412 } 413 414 public boolean hasAnswer() { 415 if (this.answer == null) 416 return false; 417 for (QuestionnaireResponseItemAnswerComponent item : this.answer) 418 if (!item.isEmpty()) 419 return true; 420 return false; 421 } 422 423 public QuestionnaireResponseItemAnswerComponent addAnswer() { //3 424 QuestionnaireResponseItemAnswerComponent t = new QuestionnaireResponseItemAnswerComponent(); 425 if (this.answer == null) 426 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 427 this.answer.add(t); 428 return t; 429 } 430 431 public QuestionnaireResponseItemComponent addAnswer(QuestionnaireResponseItemAnswerComponent t) { //3 432 if (t == null) 433 return this; 434 if (this.answer == null) 435 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 436 this.answer.add(t); 437 return this; 438 } 439 440 /** 441 * @return The first repetition of repeating field {@link #answer}, creating it if it does not already exist {3} 442 */ 443 public QuestionnaireResponseItemAnswerComponent getAnswerFirstRep() { 444 if (getAnswer().isEmpty()) { 445 addAnswer(); 446 } 447 return getAnswer().get(0); 448 } 449 450 /** 451 * @return {@link #item} (Sub-questions, sub-groups or display items nested beneath a group.) 452 */ 453 public List<QuestionnaireResponseItemComponent> getItem() { 454 if (this.item == null) 455 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 456 return this.item; 457 } 458 459 /** 460 * @return Returns a reference to <code>this</code> for easy method chaining 461 */ 462 public QuestionnaireResponseItemComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 463 this.item = theItem; 464 return this; 465 } 466 467 public boolean hasItem() { 468 if (this.item == null) 469 return false; 470 for (QuestionnaireResponseItemComponent item : this.item) 471 if (!item.isEmpty()) 472 return true; 473 return false; 474 } 475 476 public QuestionnaireResponseItemComponent addItem() { //3 477 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 478 if (this.item == null) 479 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 480 this.item.add(t); 481 return t; 482 } 483 484 public QuestionnaireResponseItemComponent addItem(QuestionnaireResponseItemComponent t) { //3 485 if (t == null) 486 return this; 487 if (this.item == null) 488 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 489 this.item.add(t); 490 return this; 491 } 492 493 /** 494 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 495 */ 496 public QuestionnaireResponseItemComponent getItemFirstRep() { 497 if (getItem().isEmpty()) { 498 addItem(); 499 } 500 return getItem().get(0); 501 } 502 503 protected void listChildren(List<Property> children) { 504 super.listChildren(children); 505 children.add(new Property("linkId", "string", "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, linkId)); 506 children.add(new Property("definition", "uri", "A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.", 0, 1, definition)); 507 children.add(new Property("text", "string", "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, text)); 508 children.add(new Property("answer", "", "The respondent's answer(s) to the question.", 0, java.lang.Integer.MAX_VALUE, answer)); 509 children.add(new Property("item", "@QuestionnaireResponse.item", "Sub-questions, sub-groups or display items nested beneath a group.", 0, java.lang.Integer.MAX_VALUE, item)); 510 } 511 512 @Override 513 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 514 switch (_hash) { 515 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, linkId); 516 case -1014418093: /*definition*/ return new Property("definition", "uri", "A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.", 0, 1, definition); 517 case 3556653: /*text*/ return new Property("text", "string", "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, text); 518 case -1412808770: /*answer*/ return new Property("answer", "", "The respondent's answer(s) to the question.", 0, java.lang.Integer.MAX_VALUE, answer); 519 case 3242771: /*item*/ return new Property("item", "@QuestionnaireResponse.item", "Sub-questions, sub-groups or display items nested beneath a group.", 0, java.lang.Integer.MAX_VALUE, item); 520 default: return super.getNamedProperty(_hash, _name, _checkValid); 521 } 522 523 } 524 525 @Override 526 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 527 switch (hash) { 528 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 529 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // UriType 530 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 531 case -1412808770: /*answer*/ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // QuestionnaireResponseItemAnswerComponent 532 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 533 default: return super.getProperty(hash, name, checkValid); 534 } 535 536 } 537 538 @Override 539 public Base setProperty(int hash, String name, Base value) throws FHIRException { 540 switch (hash) { 541 case -1102667083: // linkId 542 this.linkId = TypeConvertor.castToString(value); // StringType 543 return value; 544 case -1014418093: // definition 545 this.definition = TypeConvertor.castToUri(value); // UriType 546 return value; 547 case 3556653: // text 548 this.text = TypeConvertor.castToString(value); // StringType 549 return value; 550 case -1412808770: // answer 551 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); // QuestionnaireResponseItemAnswerComponent 552 return value; 553 case 3242771: // item 554 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 555 return value; 556 default: return super.setProperty(hash, name, value); 557 } 558 559 } 560 561 @Override 562 public Base setProperty(String name, Base value) throws FHIRException { 563 if (name.equals("linkId")) { 564 this.linkId = TypeConvertor.castToString(value); // StringType 565 } else if (name.equals("definition")) { 566 this.definition = TypeConvertor.castToUri(value); // UriType 567 } else if (name.equals("text")) { 568 this.text = TypeConvertor.castToString(value); // StringType 569 } else if (name.equals("answer")) { 570 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); 571 } else if (name.equals("item")) { 572 this.getItem().add((QuestionnaireResponseItemComponent) value); 573 } else 574 return super.setProperty(name, value); 575 return value; 576 } 577 578 @Override 579 public Base makeProperty(int hash, String name) throws FHIRException { 580 switch (hash) { 581 case -1102667083: return getLinkIdElement(); 582 case -1014418093: return getDefinitionElement(); 583 case 3556653: return getTextElement(); 584 case -1412808770: return addAnswer(); 585 case 3242771: return addItem(); 586 default: return super.makeProperty(hash, name); 587 } 588 589 } 590 591 @Override 592 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 593 switch (hash) { 594 case -1102667083: /*linkId*/ return new String[] {"string"}; 595 case -1014418093: /*definition*/ return new String[] {"uri"}; 596 case 3556653: /*text*/ return new String[] {"string"}; 597 case -1412808770: /*answer*/ return new String[] {}; 598 case 3242771: /*item*/ return new String[] {"@QuestionnaireResponse.item"}; 599 default: return super.getTypesForProperty(hash, name); 600 } 601 602 } 603 604 @Override 605 public Base addChild(String name) throws FHIRException { 606 if (name.equals("linkId")) { 607 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.item.linkId"); 608 } 609 else if (name.equals("definition")) { 610 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.item.definition"); 611 } 612 else if (name.equals("text")) { 613 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.item.text"); 614 } 615 else if (name.equals("answer")) { 616 return addAnswer(); 617 } 618 else if (name.equals("item")) { 619 return addItem(); 620 } 621 else 622 return super.addChild(name); 623 } 624 625 public QuestionnaireResponseItemComponent copy() { 626 QuestionnaireResponseItemComponent dst = new QuestionnaireResponseItemComponent(); 627 copyValues(dst); 628 return dst; 629 } 630 631 public void copyValues(QuestionnaireResponseItemComponent dst) { 632 super.copyValues(dst); 633 dst.linkId = linkId == null ? null : linkId.copy(); 634 dst.definition = definition == null ? null : definition.copy(); 635 dst.text = text == null ? null : text.copy(); 636 if (answer != null) { 637 dst.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 638 for (QuestionnaireResponseItemAnswerComponent i : answer) 639 dst.answer.add(i.copy()); 640 }; 641 if (item != null) { 642 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 643 for (QuestionnaireResponseItemComponent i : item) 644 dst.item.add(i.copy()); 645 }; 646 } 647 648 @Override 649 public boolean equalsDeep(Base other_) { 650 if (!super.equalsDeep(other_)) 651 return false; 652 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 653 return false; 654 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 655 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) && compareDeep(text, o.text, true) 656 && compareDeep(answer, o.answer, true) && compareDeep(item, o.item, true); 657 } 658 659 @Override 660 public boolean equalsShallow(Base other_) { 661 if (!super.equalsShallow(other_)) 662 return false; 663 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 664 return false; 665 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 666 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) && compareValues(text, o.text, true) 667 ; 668 } 669 670 public boolean isEmpty() { 671 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, text 672 , answer, item); 673 } 674 675 public String fhirType() { 676 return "QuestionnaireResponse.item"; 677 678 } 679 680 } 681 682 @Block() 683 public static class QuestionnaireResponseItemAnswerComponent extends BackboneElement implements IBaseBackboneElement { 684 /** 685 * The answer (or one of the answers) provided by the respondent to the question. 686 */ 687 @Child(name = "value", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, Quantity.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 688 @Description(shortDefinition="Single-valued answer to the question", formalDefinition="The answer (or one of the answers) provided by the respondent to the question." ) 689 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 690 protected DataType value; 691 692 /** 693 * Nested groups and/or questions found within this particular answer. 694 */ 695 @Child(name = "item", type = {QuestionnaireResponseItemComponent.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 696 @Description(shortDefinition="Child items of question", formalDefinition="Nested groups and/or questions found within this particular answer." ) 697 protected List<QuestionnaireResponseItemComponent> item; 698 699 private static final long serialVersionUID = 1790747618L; 700 701 /** 702 * Constructor 703 */ 704 public QuestionnaireResponseItemAnswerComponent() { 705 super(); 706 } 707 708 /** 709 * Constructor 710 */ 711 public QuestionnaireResponseItemAnswerComponent(DataType value) { 712 super(); 713 this.setValue(value); 714 } 715 716 /** 717 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 718 */ 719 public DataType getValue() { 720 return this.value; 721 } 722 723 /** 724 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 725 */ 726 public BooleanType getValueBooleanType() throws FHIRException { 727 if (this.value == null) 728 this.value = new BooleanType(); 729 if (!(this.value instanceof BooleanType)) 730 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 731 return (BooleanType) this.value; 732 } 733 734 public boolean hasValueBooleanType() { 735 return this != null && this.value instanceof BooleanType; 736 } 737 738 /** 739 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 740 */ 741 public DecimalType getValueDecimalType() throws FHIRException { 742 if (this.value == null) 743 this.value = new DecimalType(); 744 if (!(this.value instanceof DecimalType)) 745 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 746 return (DecimalType) this.value; 747 } 748 749 public boolean hasValueDecimalType() { 750 return this != null && this.value instanceof DecimalType; 751 } 752 753 /** 754 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 755 */ 756 public IntegerType getValueIntegerType() throws FHIRException { 757 if (this.value == null) 758 this.value = new IntegerType(); 759 if (!(this.value instanceof IntegerType)) 760 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 761 return (IntegerType) this.value; 762 } 763 764 public boolean hasValueIntegerType() { 765 return this != null && this.value instanceof IntegerType; 766 } 767 768 /** 769 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 770 */ 771 public DateType getValueDateType() throws FHIRException { 772 if (this.value == null) 773 this.value = new DateType(); 774 if (!(this.value instanceof DateType)) 775 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 776 return (DateType) this.value; 777 } 778 779 public boolean hasValueDateType() { 780 return this != null && this.value instanceof DateType; 781 } 782 783 /** 784 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 785 */ 786 public DateTimeType getValueDateTimeType() throws FHIRException { 787 if (this.value == null) 788 this.value = new DateTimeType(); 789 if (!(this.value instanceof DateTimeType)) 790 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 791 return (DateTimeType) this.value; 792 } 793 794 public boolean hasValueDateTimeType() { 795 return this != null && this.value instanceof DateTimeType; 796 } 797 798 /** 799 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 800 */ 801 public TimeType getValueTimeType() throws FHIRException { 802 if (this.value == null) 803 this.value = new TimeType(); 804 if (!(this.value instanceof TimeType)) 805 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 806 return (TimeType) this.value; 807 } 808 809 public boolean hasValueTimeType() { 810 return this != null && this.value instanceof TimeType; 811 } 812 813 /** 814 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 815 */ 816 public StringType getValueStringType() throws FHIRException { 817 if (this.value == null) 818 this.value = new StringType(); 819 if (!(this.value instanceof StringType)) 820 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 821 return (StringType) this.value; 822 } 823 824 public boolean hasValueStringType() { 825 return this != null && this.value instanceof StringType; 826 } 827 828 /** 829 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 830 */ 831 public UriType getValueUriType() throws FHIRException { 832 if (this.value == null) 833 this.value = new UriType(); 834 if (!(this.value instanceof UriType)) 835 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 836 return (UriType) this.value; 837 } 838 839 public boolean hasValueUriType() { 840 return this != null && this.value instanceof UriType; 841 } 842 843 /** 844 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 845 */ 846 public Attachment getValueAttachment() throws FHIRException { 847 if (this.value == null) 848 this.value = new Attachment(); 849 if (!(this.value instanceof Attachment)) 850 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 851 return (Attachment) this.value; 852 } 853 854 public boolean hasValueAttachment() { 855 return this != null && this.value instanceof Attachment; 856 } 857 858 /** 859 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 860 */ 861 public Coding getValueCoding() throws FHIRException { 862 if (this.value == null) 863 this.value = new Coding(); 864 if (!(this.value instanceof Coding)) 865 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 866 return (Coding) this.value; 867 } 868 869 public boolean hasValueCoding() { 870 return this != null && this.value instanceof Coding; 871 } 872 873 /** 874 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 875 */ 876 public Quantity getValueQuantity() throws FHIRException { 877 if (this.value == null) 878 this.value = new Quantity(); 879 if (!(this.value instanceof Quantity)) 880 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 881 return (Quantity) this.value; 882 } 883 884 public boolean hasValueQuantity() { 885 return this != null && this.value instanceof Quantity; 886 } 887 888 /** 889 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 890 */ 891 public Reference getValueReference() throws FHIRException { 892 if (this.value == null) 893 this.value = new Reference(); 894 if (!(this.value instanceof Reference)) 895 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 896 return (Reference) this.value; 897 } 898 899 public boolean hasValueReference() { 900 return this != null && this.value instanceof Reference; 901 } 902 903 public boolean hasValue() { 904 return this.value != null && !this.value.isEmpty(); 905 } 906 907 /** 908 * @param value {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 909 */ 910 public QuestionnaireResponseItemAnswerComponent setValue(DataType value) { 911 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof UriType || value instanceof Attachment || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 912 throw new FHIRException("Not the right type for QuestionnaireResponse.item.answer.value[x]: "+value.fhirType()); 913 this.value = value; 914 return this; 915 } 916 917 /** 918 * @return {@link #item} (Nested groups and/or questions found within this particular answer.) 919 */ 920 public List<QuestionnaireResponseItemComponent> getItem() { 921 if (this.item == null) 922 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 923 return this.item; 924 } 925 926 /** 927 * @return Returns a reference to <code>this</code> for easy method chaining 928 */ 929 public QuestionnaireResponseItemAnswerComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 930 this.item = theItem; 931 return this; 932 } 933 934 public boolean hasItem() { 935 if (this.item == null) 936 return false; 937 for (QuestionnaireResponseItemComponent item : this.item) 938 if (!item.isEmpty()) 939 return true; 940 return false; 941 } 942 943 public QuestionnaireResponseItemComponent addItem() { //3 944 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 945 if (this.item == null) 946 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 947 this.item.add(t); 948 return t; 949 } 950 951 public QuestionnaireResponseItemAnswerComponent addItem(QuestionnaireResponseItemComponent t) { //3 952 if (t == null) 953 return this; 954 if (this.item == null) 955 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 956 this.item.add(t); 957 return this; 958 } 959 960 /** 961 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 962 */ 963 public QuestionnaireResponseItemComponent getItemFirstRep() { 964 if (getItem().isEmpty()) { 965 addItem(); 966 } 967 return getItem().get(0); 968 } 969 970 protected void listChildren(List<Property> children) { 971 super.listChildren(children); 972 children.add(new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value)); 973 children.add(new Property("item", "@QuestionnaireResponse.item", "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item)); 974 } 975 976 @Override 977 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 978 switch (_hash) { 979 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 980 case 111972721: /*value*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 981 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 982 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 983 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 984 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 985 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 986 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 987 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 988 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 989 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 990 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 991 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 992 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 993 case 3242771: /*item*/ return new Property("item", "@QuestionnaireResponse.item", "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item); 994 default: return super.getNamedProperty(_hash, _name, _checkValid); 995 } 996 997 } 998 999 @Override 1000 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1001 switch (hash) { 1002 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1003 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1004 default: return super.getProperty(hash, name, checkValid); 1005 } 1006 1007 } 1008 1009 @Override 1010 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1011 switch (hash) { 1012 case 111972721: // value 1013 this.value = TypeConvertor.castToType(value); // DataType 1014 return value; 1015 case 3242771: // item 1016 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1017 return value; 1018 default: return super.setProperty(hash, name, value); 1019 } 1020 1021 } 1022 1023 @Override 1024 public Base setProperty(String name, Base value) throws FHIRException { 1025 if (name.equals("value[x]")) { 1026 this.value = TypeConvertor.castToType(value); // DataType 1027 } else if (name.equals("item")) { 1028 this.getItem().add((QuestionnaireResponseItemComponent) value); 1029 } else 1030 return super.setProperty(name, value); 1031 return value; 1032 } 1033 1034 @Override 1035 public Base makeProperty(int hash, String name) throws FHIRException { 1036 switch (hash) { 1037 case -1410166417: return getValue(); 1038 case 111972721: return getValue(); 1039 case 3242771: return addItem(); 1040 default: return super.makeProperty(hash, name); 1041 } 1042 1043 } 1044 1045 @Override 1046 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1047 switch (hash) { 1048 case 111972721: /*value*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", "Attachment", "Coding", "Quantity", "Reference"}; 1049 case 3242771: /*item*/ return new String[] {"@QuestionnaireResponse.item"}; 1050 default: return super.getTypesForProperty(hash, name); 1051 } 1052 1053 } 1054 1055 @Override 1056 public Base addChild(String name) throws FHIRException { 1057 if (name.equals("valueBoolean")) { 1058 this.value = new BooleanType(); 1059 return this.value; 1060 } 1061 else if (name.equals("valueDecimal")) { 1062 this.value = new DecimalType(); 1063 return this.value; 1064 } 1065 else if (name.equals("valueInteger")) { 1066 this.value = new IntegerType(); 1067 return this.value; 1068 } 1069 else if (name.equals("valueDate")) { 1070 this.value = new DateType(); 1071 return this.value; 1072 } 1073 else if (name.equals("valueDateTime")) { 1074 this.value = new DateTimeType(); 1075 return this.value; 1076 } 1077 else if (name.equals("valueTime")) { 1078 this.value = new TimeType(); 1079 return this.value; 1080 } 1081 else if (name.equals("valueString")) { 1082 this.value = new StringType(); 1083 return this.value; 1084 } 1085 else if (name.equals("valueUri")) { 1086 this.value = new UriType(); 1087 return this.value; 1088 } 1089 else if (name.equals("valueAttachment")) { 1090 this.value = new Attachment(); 1091 return this.value; 1092 } 1093 else if (name.equals("valueCoding")) { 1094 this.value = new Coding(); 1095 return this.value; 1096 } 1097 else if (name.equals("valueQuantity")) { 1098 this.value = new Quantity(); 1099 return this.value; 1100 } 1101 else if (name.equals("valueReference")) { 1102 this.value = new Reference(); 1103 return this.value; 1104 } 1105 else if (name.equals("item")) { 1106 return addItem(); 1107 } 1108 else 1109 return super.addChild(name); 1110 } 1111 1112 public QuestionnaireResponseItemAnswerComponent copy() { 1113 QuestionnaireResponseItemAnswerComponent dst = new QuestionnaireResponseItemAnswerComponent(); 1114 copyValues(dst); 1115 return dst; 1116 } 1117 1118 public void copyValues(QuestionnaireResponseItemAnswerComponent dst) { 1119 super.copyValues(dst); 1120 dst.value = value == null ? null : value.copy(); 1121 if (item != null) { 1122 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1123 for (QuestionnaireResponseItemComponent i : item) 1124 dst.item.add(i.copy()); 1125 }; 1126 } 1127 1128 @Override 1129 public boolean equalsDeep(Base other_) { 1130 if (!super.equalsDeep(other_)) 1131 return false; 1132 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1133 return false; 1134 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1135 return compareDeep(value, o.value, true) && compareDeep(item, o.item, true); 1136 } 1137 1138 @Override 1139 public boolean equalsShallow(Base other_) { 1140 if (!super.equalsShallow(other_)) 1141 return false; 1142 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1143 return false; 1144 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1145 return true; 1146 } 1147 1148 public boolean isEmpty() { 1149 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, item); 1150 } 1151 1152 public String fhirType() { 1153 return "QuestionnaireResponse.item.answer"; 1154 1155 } 1156 1157 } 1158 1159 /** 1160 * Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server. 1161 */ 1162 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1163 @Description(shortDefinition="Business identifier for this set of answers", formalDefinition="Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server." ) 1164 protected List<Identifier> identifier; 1165 1166 /** 1167 * A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression. 1168 */ 1169 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1170 @Description(shortDefinition="Request fulfilled by this QuestionnaireResponse", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression." ) 1171 protected List<Reference> basedOn; 1172 1173 /** 1174 * A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of. 1175 */ 1176 @Child(name = "partOf", type = {Observation.class, Procedure.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1177 @Description(shortDefinition="Part of referenced event", formalDefinition="A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of." ) 1178 protected List<Reference> partOf; 1179 1180 /** 1181 * The Questionnaire that defines and organizes the questions for which answers are being provided. 1182 */ 1183 @Child(name = "questionnaire", type = {CanonicalType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1184 @Description(shortDefinition="Canonical URL of Questionnaire being answered", formalDefinition="The Questionnaire that defines and organizes the questions for which answers are being provided." ) 1185 protected CanonicalType questionnaire; 1186 1187 /** 1188 * The current state of the questionnaire response. 1189 */ 1190 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 1191 @Description(shortDefinition="in-progress | completed | amended | entered-in-error | stopped", formalDefinition="The current state of the questionnaire response." ) 1192 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers-status") 1193 protected Enumeration<QuestionnaireResponseStatus> status; 1194 1195 /** 1196 * The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information. 1197 */ 1198 @Child(name = "subject", type = {Reference.class}, order=5, min=0, max=1, modifier=false, summary=true) 1199 @Description(shortDefinition="The subject of the questions", formalDefinition="The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information." ) 1200 protected Reference subject; 1201 1202 /** 1203 * The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated. 1204 */ 1205 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 1206 @Description(shortDefinition="Encounter the questionnaire response is part of", formalDefinition="The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated." ) 1207 protected Reference encounter; 1208 1209 /** 1210 * The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status. 1211 */ 1212 @Child(name = "authored", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1213 @Description(shortDefinition="Date the answers were gathered", formalDefinition="The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status." ) 1214 protected DateTimeType authored; 1215 1216 /** 1217 * The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system. 1218 */ 1219 @Child(name = "author", type = {Device.class, Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=true) 1220 @Description(shortDefinition="The individual or device that received and recorded the answers", formalDefinition="The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system." ) 1221 protected Reference author; 1222 1223 /** 1224 * The individual or device that answered the questions about the subject. 1225 */ 1226 @Child(name = "source", type = {Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=true) 1227 @Description(shortDefinition="The individual or device that answered the questions", formalDefinition="The individual or device that answered the questions about the subject." ) 1228 protected Reference source; 1229 1230 /** 1231 * A group or question item from the original questionnaire for which answers are provided. 1232 */ 1233 @Child(name = "item", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1234 @Description(shortDefinition="Groups and questions", formalDefinition="A group or question item from the original questionnaire for which answers are provided." ) 1235 protected List<QuestionnaireResponseItemComponent> item; 1236 1237 private static final long serialVersionUID = 1660467089L; 1238 1239 /** 1240 * Constructor 1241 */ 1242 public QuestionnaireResponse() { 1243 super(); 1244 } 1245 1246 /** 1247 * Constructor 1248 */ 1249 public QuestionnaireResponse(String questionnaire, QuestionnaireResponseStatus status) { 1250 super(); 1251 this.setQuestionnaire(questionnaire); 1252 this.setStatus(status); 1253 } 1254 1255 /** 1256 * @return {@link #identifier} (Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 1257 */ 1258 public List<Identifier> getIdentifier() { 1259 if (this.identifier == null) 1260 this.identifier = new ArrayList<Identifier>(); 1261 return this.identifier; 1262 } 1263 1264 /** 1265 * @return Returns a reference to <code>this</code> for easy method chaining 1266 */ 1267 public QuestionnaireResponse setIdentifier(List<Identifier> theIdentifier) { 1268 this.identifier = theIdentifier; 1269 return this; 1270 } 1271 1272 public boolean hasIdentifier() { 1273 if (this.identifier == null) 1274 return false; 1275 for (Identifier item : this.identifier) 1276 if (!item.isEmpty()) 1277 return true; 1278 return false; 1279 } 1280 1281 public Identifier addIdentifier() { //3 1282 Identifier t = new Identifier(); 1283 if (this.identifier == null) 1284 this.identifier = new ArrayList<Identifier>(); 1285 this.identifier.add(t); 1286 return t; 1287 } 1288 1289 public QuestionnaireResponse addIdentifier(Identifier t) { //3 1290 if (t == null) 1291 return this; 1292 if (this.identifier == null) 1293 this.identifier = new ArrayList<Identifier>(); 1294 this.identifier.add(t); 1295 return this; 1296 } 1297 1298 /** 1299 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1300 */ 1301 public Identifier getIdentifierFirstRep() { 1302 if (getIdentifier().isEmpty()) { 1303 addIdentifier(); 1304 } 1305 return getIdentifier().get(0); 1306 } 1307 1308 /** 1309 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.) 1310 */ 1311 public List<Reference> getBasedOn() { 1312 if (this.basedOn == null) 1313 this.basedOn = new ArrayList<Reference>(); 1314 return this.basedOn; 1315 } 1316 1317 /** 1318 * @return Returns a reference to <code>this</code> for easy method chaining 1319 */ 1320 public QuestionnaireResponse setBasedOn(List<Reference> theBasedOn) { 1321 this.basedOn = theBasedOn; 1322 return this; 1323 } 1324 1325 public boolean hasBasedOn() { 1326 if (this.basedOn == null) 1327 return false; 1328 for (Reference item : this.basedOn) 1329 if (!item.isEmpty()) 1330 return true; 1331 return false; 1332 } 1333 1334 public Reference addBasedOn() { //3 1335 Reference t = new Reference(); 1336 if (this.basedOn == null) 1337 this.basedOn = new ArrayList<Reference>(); 1338 this.basedOn.add(t); 1339 return t; 1340 } 1341 1342 public QuestionnaireResponse addBasedOn(Reference t) { //3 1343 if (t == null) 1344 return this; 1345 if (this.basedOn == null) 1346 this.basedOn = new ArrayList<Reference>(); 1347 this.basedOn.add(t); 1348 return this; 1349 } 1350 1351 /** 1352 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1353 */ 1354 public Reference getBasedOnFirstRep() { 1355 if (getBasedOn().isEmpty()) { 1356 addBasedOn(); 1357 } 1358 return getBasedOn().get(0); 1359 } 1360 1361 /** 1362 * @return {@link #partOf} (A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.) 1363 */ 1364 public List<Reference> getPartOf() { 1365 if (this.partOf == null) 1366 this.partOf = new ArrayList<Reference>(); 1367 return this.partOf; 1368 } 1369 1370 /** 1371 * @return Returns a reference to <code>this</code> for easy method chaining 1372 */ 1373 public QuestionnaireResponse setPartOf(List<Reference> thePartOf) { 1374 this.partOf = thePartOf; 1375 return this; 1376 } 1377 1378 public boolean hasPartOf() { 1379 if (this.partOf == null) 1380 return false; 1381 for (Reference item : this.partOf) 1382 if (!item.isEmpty()) 1383 return true; 1384 return false; 1385 } 1386 1387 public Reference addPartOf() { //3 1388 Reference t = new Reference(); 1389 if (this.partOf == null) 1390 this.partOf = new ArrayList<Reference>(); 1391 this.partOf.add(t); 1392 return t; 1393 } 1394 1395 public QuestionnaireResponse addPartOf(Reference t) { //3 1396 if (t == null) 1397 return this; 1398 if (this.partOf == null) 1399 this.partOf = new ArrayList<Reference>(); 1400 this.partOf.add(t); 1401 return this; 1402 } 1403 1404 /** 1405 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 1406 */ 1407 public Reference getPartOfFirstRep() { 1408 if (getPartOf().isEmpty()) { 1409 addPartOf(); 1410 } 1411 return getPartOf().get(0); 1412 } 1413 1414 /** 1415 * @return {@link #questionnaire} (The Questionnaire that defines and organizes the questions for which answers are being provided.). This is the underlying object with id, value and extensions. The accessor "getQuestionnaire" gives direct access to the value 1416 */ 1417 public CanonicalType getQuestionnaireElement() { 1418 if (this.questionnaire == null) 1419 if (Configuration.errorOnAutoCreate()) 1420 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1421 else if (Configuration.doAutoCreate()) 1422 this.questionnaire = new CanonicalType(); // bb 1423 return this.questionnaire; 1424 } 1425 1426 public boolean hasQuestionnaireElement() { 1427 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1428 } 1429 1430 public boolean hasQuestionnaire() { 1431 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1432 } 1433 1434 /** 1435 * @param value {@link #questionnaire} (The Questionnaire that defines and organizes the questions for which answers are being provided.). This is the underlying object with id, value and extensions. The accessor "getQuestionnaire" gives direct access to the value 1436 */ 1437 public QuestionnaireResponse setQuestionnaireElement(CanonicalType value) { 1438 this.questionnaire = value; 1439 return this; 1440 } 1441 1442 /** 1443 * @return The Questionnaire that defines and organizes the questions for which answers are being provided. 1444 */ 1445 public String getQuestionnaire() { 1446 return this.questionnaire == null ? null : this.questionnaire.getValue(); 1447 } 1448 1449 /** 1450 * @param value The Questionnaire that defines and organizes the questions for which answers are being provided. 1451 */ 1452 public QuestionnaireResponse setQuestionnaire(String value) { 1453 if (this.questionnaire == null) 1454 this.questionnaire = new CanonicalType(); 1455 this.questionnaire.setValue(value); 1456 return this; 1457 } 1458 1459 /** 1460 * @return {@link #status} (The current state of the questionnaire response.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1461 */ 1462 public Enumeration<QuestionnaireResponseStatus> getStatusElement() { 1463 if (this.status == null) 1464 if (Configuration.errorOnAutoCreate()) 1465 throw new Error("Attempt to auto-create QuestionnaireResponse.status"); 1466 else if (Configuration.doAutoCreate()) 1467 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); // bb 1468 return this.status; 1469 } 1470 1471 public boolean hasStatusElement() { 1472 return this.status != null && !this.status.isEmpty(); 1473 } 1474 1475 public boolean hasStatus() { 1476 return this.status != null && !this.status.isEmpty(); 1477 } 1478 1479 /** 1480 * @param value {@link #status} (The current state of the questionnaire response.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1481 */ 1482 public QuestionnaireResponse setStatusElement(Enumeration<QuestionnaireResponseStatus> value) { 1483 this.status = value; 1484 return this; 1485 } 1486 1487 /** 1488 * @return The current state of the questionnaire response. 1489 */ 1490 public QuestionnaireResponseStatus getStatus() { 1491 return this.status == null ? null : this.status.getValue(); 1492 } 1493 1494 /** 1495 * @param value The current state of the questionnaire response. 1496 */ 1497 public QuestionnaireResponse setStatus(QuestionnaireResponseStatus value) { 1498 if (this.status == null) 1499 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); 1500 this.status.setValue(value); 1501 return this; 1502 } 1503 1504 /** 1505 * @return {@link #subject} (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1506 */ 1507 public Reference getSubject() { 1508 if (this.subject == null) 1509 if (Configuration.errorOnAutoCreate()) 1510 throw new Error("Attempt to auto-create QuestionnaireResponse.subject"); 1511 else if (Configuration.doAutoCreate()) 1512 this.subject = new Reference(); // cc 1513 return this.subject; 1514 } 1515 1516 public boolean hasSubject() { 1517 return this.subject != null && !this.subject.isEmpty(); 1518 } 1519 1520 /** 1521 * @param value {@link #subject} (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1522 */ 1523 public QuestionnaireResponse setSubject(Reference value) { 1524 this.subject = value; 1525 return this; 1526 } 1527 1528 /** 1529 * @return {@link #encounter} (The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.) 1530 */ 1531 public Reference getEncounter() { 1532 if (this.encounter == null) 1533 if (Configuration.errorOnAutoCreate()) 1534 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1535 else if (Configuration.doAutoCreate()) 1536 this.encounter = new Reference(); // cc 1537 return this.encounter; 1538 } 1539 1540 public boolean hasEncounter() { 1541 return this.encounter != null && !this.encounter.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #encounter} (The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.) 1546 */ 1547 public QuestionnaireResponse setEncounter(Reference value) { 1548 this.encounter = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return {@link #authored} (The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.). This is the underlying object with id, value and extensions. The accessor "getAuthored" gives direct access to the value 1554 */ 1555 public DateTimeType getAuthoredElement() { 1556 if (this.authored == null) 1557 if (Configuration.errorOnAutoCreate()) 1558 throw new Error("Attempt to auto-create QuestionnaireResponse.authored"); 1559 else if (Configuration.doAutoCreate()) 1560 this.authored = new DateTimeType(); // bb 1561 return this.authored; 1562 } 1563 1564 public boolean hasAuthoredElement() { 1565 return this.authored != null && !this.authored.isEmpty(); 1566 } 1567 1568 public boolean hasAuthored() { 1569 return this.authored != null && !this.authored.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #authored} (The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.). This is the underlying object with id, value and extensions. The accessor "getAuthored" gives direct access to the value 1574 */ 1575 public QuestionnaireResponse setAuthoredElement(DateTimeType value) { 1576 this.authored = value; 1577 return this; 1578 } 1579 1580 /** 1581 * @return The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status. 1582 */ 1583 public Date getAuthored() { 1584 return this.authored == null ? null : this.authored.getValue(); 1585 } 1586 1587 /** 1588 * @param value The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status. 1589 */ 1590 public QuestionnaireResponse setAuthored(Date value) { 1591 if (value == null) 1592 this.authored = null; 1593 else { 1594 if (this.authored == null) 1595 this.authored = new DateTimeType(); 1596 this.authored.setValue(value); 1597 } 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #author} (The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1603 */ 1604 public Reference getAuthor() { 1605 if (this.author == null) 1606 if (Configuration.errorOnAutoCreate()) 1607 throw new Error("Attempt to auto-create QuestionnaireResponse.author"); 1608 else if (Configuration.doAutoCreate()) 1609 this.author = new Reference(); // cc 1610 return this.author; 1611 } 1612 1613 public boolean hasAuthor() { 1614 return this.author != null && !this.author.isEmpty(); 1615 } 1616 1617 /** 1618 * @param value {@link #author} (The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1619 */ 1620 public QuestionnaireResponse setAuthor(Reference value) { 1621 this.author = value; 1622 return this; 1623 } 1624 1625 /** 1626 * @return {@link #source} (The individual or device that answered the questions about the subject.) 1627 */ 1628 public Reference getSource() { 1629 if (this.source == null) 1630 if (Configuration.errorOnAutoCreate()) 1631 throw new Error("Attempt to auto-create QuestionnaireResponse.source"); 1632 else if (Configuration.doAutoCreate()) 1633 this.source = new Reference(); // cc 1634 return this.source; 1635 } 1636 1637 public boolean hasSource() { 1638 return this.source != null && !this.source.isEmpty(); 1639 } 1640 1641 /** 1642 * @param value {@link #source} (The individual or device that answered the questions about the subject.) 1643 */ 1644 public QuestionnaireResponse setSource(Reference value) { 1645 this.source = value; 1646 return this; 1647 } 1648 1649 /** 1650 * @return {@link #item} (A group or question item from the original questionnaire for which answers are provided.) 1651 */ 1652 public List<QuestionnaireResponseItemComponent> getItem() { 1653 if (this.item == null) 1654 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1655 return this.item; 1656 } 1657 1658 /** 1659 * @return Returns a reference to <code>this</code> for easy method chaining 1660 */ 1661 public QuestionnaireResponse setItem(List<QuestionnaireResponseItemComponent> theItem) { 1662 this.item = theItem; 1663 return this; 1664 } 1665 1666 public boolean hasItem() { 1667 if (this.item == null) 1668 return false; 1669 for (QuestionnaireResponseItemComponent item : this.item) 1670 if (!item.isEmpty()) 1671 return true; 1672 return false; 1673 } 1674 1675 public QuestionnaireResponseItemComponent addItem() { //3 1676 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 1677 if (this.item == null) 1678 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1679 this.item.add(t); 1680 return t; 1681 } 1682 1683 public QuestionnaireResponse addItem(QuestionnaireResponseItemComponent t) { //3 1684 if (t == null) 1685 return this; 1686 if (this.item == null) 1687 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1688 this.item.add(t); 1689 return this; 1690 } 1691 1692 /** 1693 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 1694 */ 1695 public QuestionnaireResponseItemComponent getItemFirstRep() { 1696 if (getItem().isEmpty()) { 1697 addItem(); 1698 } 1699 return getItem().get(0); 1700 } 1701 1702 protected void listChildren(List<Property> children) { 1703 super.listChildren(children); 1704 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1705 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1706 children.add(new Property("partOf", "Reference(Observation|Procedure)", "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1707 children.add(new Property("questionnaire", "canonical(Questionnaire)", "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, questionnaire)); 1708 children.add(new Property("status", "code", "The current state of the questionnaire response.", 0, 1, status)); 1709 children.add(new Property("subject", "Reference(Any)", "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 0, 1, subject)); 1710 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 1711 children.add(new Property("authored", "dateTime", "The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.", 0, 1, authored)); 1712 children.add(new Property("author", "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", "The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 0, 1, author)); 1713 children.add(new Property("source", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The individual or device that answered the questions about the subject.", 0, 1, source)); 1714 children.add(new Property("item", "", "A group or question item from the original questionnaire for which answers are provided.", 0, java.lang.Integer.MAX_VALUE, item)); 1715 } 1716 1717 @Override 1718 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1719 switch (_hash) { 1720 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1721 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1722 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Observation|Procedure)", "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 0, java.lang.Integer.MAX_VALUE, partOf); 1723 case -1017049693: /*questionnaire*/ return new Property("questionnaire", "canonical(Questionnaire)", "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, questionnaire); 1724 case -892481550: /*status*/ return new Property("status", "code", "The current state of the questionnaire response.", 0, 1, status); 1725 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 0, 1, subject); 1726 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 1727 case 1433073514: /*authored*/ return new Property("authored", "dateTime", "The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.", 0, 1, authored); 1728 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", "The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 0, 1, author); 1729 case -896505829: /*source*/ return new Property("source", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The individual or device that answered the questions about the subject.", 0, 1, source); 1730 case 3242771: /*item*/ return new Property("item", "", "A group or question item from the original questionnaire for which answers are provided.", 0, java.lang.Integer.MAX_VALUE, item); 1731 default: return super.getNamedProperty(_hash, _name, _checkValid); 1732 } 1733 1734 } 1735 1736 @Override 1737 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1738 switch (hash) { 1739 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1740 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1741 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1742 case -1017049693: /*questionnaire*/ return this.questionnaire == null ? new Base[0] : new Base[] {this.questionnaire}; // CanonicalType 1743 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<QuestionnaireResponseStatus> 1744 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1745 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1746 case 1433073514: /*authored*/ return this.authored == null ? new Base[0] : new Base[] {this.authored}; // DateTimeType 1747 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1748 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1749 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1750 default: return super.getProperty(hash, name, checkValid); 1751 } 1752 1753 } 1754 1755 @Override 1756 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1757 switch (hash) { 1758 case -1618432855: // identifier 1759 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1760 return value; 1761 case -332612366: // basedOn 1762 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1763 return value; 1764 case -995410646: // partOf 1765 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1766 return value; 1767 case -1017049693: // questionnaire 1768 this.questionnaire = TypeConvertor.castToCanonical(value); // CanonicalType 1769 return value; 1770 case -892481550: // status 1771 value = new QuestionnaireResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1772 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 1773 return value; 1774 case -1867885268: // subject 1775 this.subject = TypeConvertor.castToReference(value); // Reference 1776 return value; 1777 case 1524132147: // encounter 1778 this.encounter = TypeConvertor.castToReference(value); // Reference 1779 return value; 1780 case 1433073514: // authored 1781 this.authored = TypeConvertor.castToDateTime(value); // DateTimeType 1782 return value; 1783 case -1406328437: // author 1784 this.author = TypeConvertor.castToReference(value); // Reference 1785 return value; 1786 case -896505829: // source 1787 this.source = TypeConvertor.castToReference(value); // Reference 1788 return value; 1789 case 3242771: // item 1790 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1791 return value; 1792 default: return super.setProperty(hash, name, value); 1793 } 1794 1795 } 1796 1797 @Override 1798 public Base setProperty(String name, Base value) throws FHIRException { 1799 if (name.equals("identifier")) { 1800 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1801 } else if (name.equals("basedOn")) { 1802 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1803 } else if (name.equals("partOf")) { 1804 this.getPartOf().add(TypeConvertor.castToReference(value)); 1805 } else if (name.equals("questionnaire")) { 1806 this.questionnaire = TypeConvertor.castToCanonical(value); // CanonicalType 1807 } else if (name.equals("status")) { 1808 value = new QuestionnaireResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1809 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 1810 } else if (name.equals("subject")) { 1811 this.subject = TypeConvertor.castToReference(value); // Reference 1812 } else if (name.equals("encounter")) { 1813 this.encounter = TypeConvertor.castToReference(value); // Reference 1814 } else if (name.equals("authored")) { 1815 this.authored = TypeConvertor.castToDateTime(value); // DateTimeType 1816 } else if (name.equals("author")) { 1817 this.author = TypeConvertor.castToReference(value); // Reference 1818 } else if (name.equals("source")) { 1819 this.source = TypeConvertor.castToReference(value); // Reference 1820 } else if (name.equals("item")) { 1821 this.getItem().add((QuestionnaireResponseItemComponent) value); 1822 } else 1823 return super.setProperty(name, value); 1824 return value; 1825 } 1826 1827 @Override 1828 public Base makeProperty(int hash, String name) throws FHIRException { 1829 switch (hash) { 1830 case -1618432855: return addIdentifier(); 1831 case -332612366: return addBasedOn(); 1832 case -995410646: return addPartOf(); 1833 case -1017049693: return getQuestionnaireElement(); 1834 case -892481550: return getStatusElement(); 1835 case -1867885268: return getSubject(); 1836 case 1524132147: return getEncounter(); 1837 case 1433073514: return getAuthoredElement(); 1838 case -1406328437: return getAuthor(); 1839 case -896505829: return getSource(); 1840 case 3242771: return addItem(); 1841 default: return super.makeProperty(hash, name); 1842 } 1843 1844 } 1845 1846 @Override 1847 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1848 switch (hash) { 1849 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1850 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1851 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1852 case -1017049693: /*questionnaire*/ return new String[] {"canonical"}; 1853 case -892481550: /*status*/ return new String[] {"code"}; 1854 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1855 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1856 case 1433073514: /*authored*/ return new String[] {"dateTime"}; 1857 case -1406328437: /*author*/ return new String[] {"Reference"}; 1858 case -896505829: /*source*/ return new String[] {"Reference"}; 1859 case 3242771: /*item*/ return new String[] {}; 1860 default: return super.getTypesForProperty(hash, name); 1861 } 1862 1863 } 1864 1865 @Override 1866 public Base addChild(String name) throws FHIRException { 1867 if (name.equals("identifier")) { 1868 return addIdentifier(); 1869 } 1870 else if (name.equals("basedOn")) { 1871 return addBasedOn(); 1872 } 1873 else if (name.equals("partOf")) { 1874 return addPartOf(); 1875 } 1876 else if (name.equals("questionnaire")) { 1877 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.questionnaire"); 1878 } 1879 else if (name.equals("status")) { 1880 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.status"); 1881 } 1882 else if (name.equals("subject")) { 1883 this.subject = new Reference(); 1884 return this.subject; 1885 } 1886 else if (name.equals("encounter")) { 1887 this.encounter = new Reference(); 1888 return this.encounter; 1889 } 1890 else if (name.equals("authored")) { 1891 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.authored"); 1892 } 1893 else if (name.equals("author")) { 1894 this.author = new Reference(); 1895 return this.author; 1896 } 1897 else if (name.equals("source")) { 1898 this.source = new Reference(); 1899 return this.source; 1900 } 1901 else if (name.equals("item")) { 1902 return addItem(); 1903 } 1904 else 1905 return super.addChild(name); 1906 } 1907 1908 public String fhirType() { 1909 return "QuestionnaireResponse"; 1910 1911 } 1912 1913 public QuestionnaireResponse copy() { 1914 QuestionnaireResponse dst = new QuestionnaireResponse(); 1915 copyValues(dst); 1916 return dst; 1917 } 1918 1919 public void copyValues(QuestionnaireResponse dst) { 1920 super.copyValues(dst); 1921 if (identifier != null) { 1922 dst.identifier = new ArrayList<Identifier>(); 1923 for (Identifier i : identifier) 1924 dst.identifier.add(i.copy()); 1925 }; 1926 if (basedOn != null) { 1927 dst.basedOn = new ArrayList<Reference>(); 1928 for (Reference i : basedOn) 1929 dst.basedOn.add(i.copy()); 1930 }; 1931 if (partOf != null) { 1932 dst.partOf = new ArrayList<Reference>(); 1933 for (Reference i : partOf) 1934 dst.partOf.add(i.copy()); 1935 }; 1936 dst.questionnaire = questionnaire == null ? null : questionnaire.copy(); 1937 dst.status = status == null ? null : status.copy(); 1938 dst.subject = subject == null ? null : subject.copy(); 1939 dst.encounter = encounter == null ? null : encounter.copy(); 1940 dst.authored = authored == null ? null : authored.copy(); 1941 dst.author = author == null ? null : author.copy(); 1942 dst.source = source == null ? null : source.copy(); 1943 if (item != null) { 1944 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1945 for (QuestionnaireResponseItemComponent i : item) 1946 dst.item.add(i.copy()); 1947 }; 1948 } 1949 1950 protected QuestionnaireResponse typedCopy() { 1951 return copy(); 1952 } 1953 1954 @Override 1955 public boolean equalsDeep(Base other_) { 1956 if (!super.equalsDeep(other_)) 1957 return false; 1958 if (!(other_ instanceof QuestionnaireResponse)) 1959 return false; 1960 QuestionnaireResponse o = (QuestionnaireResponse) other_; 1961 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1962 && compareDeep(questionnaire, o.questionnaire, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 1963 && compareDeep(encounter, o.encounter, true) && compareDeep(authored, o.authored, true) && compareDeep(author, o.author, true) 1964 && compareDeep(source, o.source, true) && compareDeep(item, o.item, true); 1965 } 1966 1967 @Override 1968 public boolean equalsShallow(Base other_) { 1969 if (!super.equalsShallow(other_)) 1970 return false; 1971 if (!(other_ instanceof QuestionnaireResponse)) 1972 return false; 1973 QuestionnaireResponse o = (QuestionnaireResponse) other_; 1974 return compareValues(questionnaire, o.questionnaire, true) && compareValues(status, o.status, true) 1975 && compareValues(authored, o.authored, true); 1976 } 1977 1978 public boolean isEmpty() { 1979 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1980 , questionnaire, status, subject, encounter, authored, author, source, item 1981 ); 1982 } 1983 1984 @Override 1985 public ResourceType getResourceType() { 1986 return ResourceType.QuestionnaireResponse; 1987 } 1988 1989 /** 1990 * Search parameter: <b>author</b> 1991 * <p> 1992 * Description: <b>The author of the questionnaire response</b><br> 1993 * Type: <b>reference</b><br> 1994 * Path: <b>QuestionnaireResponse.author</b><br> 1995 * </p> 1996 */ 1997 @SearchParamDefinition(name="author", path="QuestionnaireResponse.author", description="The author of the questionnaire response", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1998 public static final String SP_AUTHOR = "author"; 1999 /** 2000 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2001 * <p> 2002 * Description: <b>The author of the questionnaire response</b><br> 2003 * Type: <b>reference</b><br> 2004 * Path: <b>QuestionnaireResponse.author</b><br> 2005 * </p> 2006 */ 2007 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2008 2009/** 2010 * Constant for fluent queries to be used to add include statements. Specifies 2011 * the path value of "<b>QuestionnaireResponse:author</b>". 2012 */ 2013 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:author").toLocked(); 2014 2015 /** 2016 * Search parameter: <b>authored</b> 2017 * <p> 2018 * Description: <b>When the questionnaire response was last changed</b><br> 2019 * Type: <b>date</b><br> 2020 * Path: <b>QuestionnaireResponse.authored</b><br> 2021 * </p> 2022 */ 2023 @SearchParamDefinition(name="authored", path="QuestionnaireResponse.authored", description="When the questionnaire response was last changed", type="date" ) 2024 public static final String SP_AUTHORED = "authored"; 2025 /** 2026 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2027 * <p> 2028 * Description: <b>When the questionnaire response was last changed</b><br> 2029 * Type: <b>date</b><br> 2030 * Path: <b>QuestionnaireResponse.authored</b><br> 2031 * </p> 2032 */ 2033 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 2034 2035 /** 2036 * Search parameter: <b>based-on</b> 2037 * <p> 2038 * Description: <b>Plan/proposal/order fulfilled by this questionnaire response</b><br> 2039 * Type: <b>reference</b><br> 2040 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2041 * </p> 2042 */ 2043 @SearchParamDefinition(name="based-on", path="QuestionnaireResponse.basedOn", description="Plan/proposal/order fulfilled by this questionnaire response", type="reference", target={CarePlan.class, ServiceRequest.class } ) 2044 public static final String SP_BASED_ON = "based-on"; 2045 /** 2046 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2047 * <p> 2048 * Description: <b>Plan/proposal/order fulfilled by this questionnaire response</b><br> 2049 * Type: <b>reference</b><br> 2050 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2051 * </p> 2052 */ 2053 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2054 2055/** 2056 * Constant for fluent queries to be used to add include statements. Specifies 2057 * the path value of "<b>QuestionnaireResponse:based-on</b>". 2058 */ 2059 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:based-on").toLocked(); 2060 2061 /** 2062 * Search parameter: <b>item-subject</b> 2063 * <p> 2064 * Description: <b>Allows searching for QuestionnaireResponses by item value where the item has isSubject=true</b><br> 2065 * Type: <b>reference</b><br> 2066 * Path: <b>QuestionnaireResponse.item.where(extension('http://hl7.org/fhir/StructureDefinition/questionnaireresponse-isSubject').exists()).answer.value.ofType(Reference)</b><br> 2067 * </p> 2068 */ 2069 @SearchParamDefinition(name="item-subject", path="QuestionnaireResponse.item.where(extension('http://hl7.org/fhir/StructureDefinition/questionnaireresponse-isSubject').exists()).answer.value.ofType(Reference)", description="Allows searching for QuestionnaireResponses by item value where the item has isSubject=true", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2070 public static final String SP_ITEM_SUBJECT = "item-subject"; 2071 /** 2072 * <b>Fluent Client</b> search parameter constant for <b>item-subject</b> 2073 * <p> 2074 * Description: <b>Allows searching for QuestionnaireResponses by item value where the item has isSubject=true</b><br> 2075 * Type: <b>reference</b><br> 2076 * Path: <b>QuestionnaireResponse.item.where(extension('http://hl7.org/fhir/StructureDefinition/questionnaireresponse-isSubject').exists()).answer.value.ofType(Reference)</b><br> 2077 * </p> 2078 */ 2079 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_SUBJECT); 2080 2081/** 2082 * Constant for fluent queries to be used to add include statements. Specifies 2083 * the path value of "<b>QuestionnaireResponse:item-subject</b>". 2084 */ 2085 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_SUBJECT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:item-subject").toLocked(); 2086 2087 /** 2088 * Search parameter: <b>part-of</b> 2089 * <p> 2090 * Description: <b>Procedure or observation this questionnaire response was performed as a part of</b><br> 2091 * Type: <b>reference</b><br> 2092 * Path: <b>QuestionnaireResponse.partOf</b><br> 2093 * </p> 2094 */ 2095 @SearchParamDefinition(name="part-of", path="QuestionnaireResponse.partOf", description="Procedure or observation this questionnaire response was performed as a part of", type="reference", target={Observation.class, Procedure.class } ) 2096 public static final String SP_PART_OF = "part-of"; 2097 /** 2098 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2099 * <p> 2100 * Description: <b>Procedure or observation this questionnaire response was performed as a part of</b><br> 2101 * Type: <b>reference</b><br> 2102 * Path: <b>QuestionnaireResponse.partOf</b><br> 2103 * </p> 2104 */ 2105 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2106 2107/** 2108 * Constant for fluent queries to be used to add include statements. Specifies 2109 * the path value of "<b>QuestionnaireResponse:part-of</b>". 2110 */ 2111 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:part-of").toLocked(); 2112 2113 /** 2114 * Search parameter: <b>questionnaire</b> 2115 * <p> 2116 * Description: <b>The questionnaire the answers are provided for</b><br> 2117 * Type: <b>reference</b><br> 2118 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2119 * </p> 2120 */ 2121 @SearchParamDefinition(name="questionnaire", path="QuestionnaireResponse.questionnaire", description="The questionnaire the answers are provided for", type="reference", target={Questionnaire.class } ) 2122 public static final String SP_QUESTIONNAIRE = "questionnaire"; 2123 /** 2124 * <b>Fluent Client</b> search parameter constant for <b>questionnaire</b> 2125 * <p> 2126 * Description: <b>The questionnaire the answers are provided for</b><br> 2127 * Type: <b>reference</b><br> 2128 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2129 * </p> 2130 */ 2131 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam QUESTIONNAIRE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_QUESTIONNAIRE); 2132 2133/** 2134 * Constant for fluent queries to be used to add include statements. Specifies 2135 * the path value of "<b>QuestionnaireResponse:questionnaire</b>". 2136 */ 2137 public static final ca.uhn.fhir.model.api.Include INCLUDE_QUESTIONNAIRE = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:questionnaire").toLocked(); 2138 2139 /** 2140 * Search parameter: <b>source</b> 2141 * <p> 2142 * Description: <b>The individual providing the information reflected in the questionnaire respose</b><br> 2143 * Type: <b>reference</b><br> 2144 * Path: <b>QuestionnaireResponse.source</b><br> 2145 * </p> 2146 */ 2147 @SearchParamDefinition(name="source", path="QuestionnaireResponse.source", description="The individual providing the information reflected in the questionnaire respose", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2148 public static final String SP_SOURCE = "source"; 2149 /** 2150 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2151 * <p> 2152 * Description: <b>The individual providing the information reflected in the questionnaire respose</b><br> 2153 * Type: <b>reference</b><br> 2154 * Path: <b>QuestionnaireResponse.source</b><br> 2155 * </p> 2156 */ 2157 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 2158 2159/** 2160 * Constant for fluent queries to be used to add include statements. Specifies 2161 * the path value of "<b>QuestionnaireResponse:source</b>". 2162 */ 2163 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:source").toLocked(); 2164 2165 /** 2166 * Search parameter: <b>status</b> 2167 * <p> 2168 * Description: <b>The status of the questionnaire response</b><br> 2169 * Type: <b>token</b><br> 2170 * Path: <b>QuestionnaireResponse.status</b><br> 2171 * </p> 2172 */ 2173 @SearchParamDefinition(name="status", path="QuestionnaireResponse.status", description="The status of the questionnaire response", type="token" ) 2174 public static final String SP_STATUS = "status"; 2175 /** 2176 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2177 * <p> 2178 * Description: <b>The status of the questionnaire response</b><br> 2179 * Type: <b>token</b><br> 2180 * Path: <b>QuestionnaireResponse.status</b><br> 2181 * </p> 2182 */ 2183 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2184 2185 /** 2186 * Search parameter: <b>subject</b> 2187 * <p> 2188 * Description: <b>The subject of the questionnaire response</b><br> 2189 * Type: <b>reference</b><br> 2190 * Path: <b>QuestionnaireResponse.subject</b><br> 2191 * </p> 2192 */ 2193 @SearchParamDefinition(name="subject", path="QuestionnaireResponse.subject", description="The subject of the questionnaire response", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2194 public static final String SP_SUBJECT = "subject"; 2195 /** 2196 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2197 * <p> 2198 * Description: <b>The subject of the questionnaire response</b><br> 2199 * Type: <b>reference</b><br> 2200 * Path: <b>QuestionnaireResponse.subject</b><br> 2201 * </p> 2202 */ 2203 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2204 2205/** 2206 * Constant for fluent queries to be used to add include statements. Specifies 2207 * the path value of "<b>QuestionnaireResponse:subject</b>". 2208 */ 2209 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:subject").toLocked(); 2210 2211 /** 2212 * Search parameter: <b>encounter</b> 2213 * <p> 2214 * Description: <b>Multiple Resources: 2215 2216* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2217* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2218* [ChargeItem](chargeitem.html): Encounter associated with event 2219* [Claim](claim.html): Encounters associated with a billed line item 2220* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2221* [Communication](communication.html): The Encounter during which this Communication was created 2222* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2223* [Composition](composition.html): Context of the Composition 2224* [Condition](condition.html): The Encounter during which this Condition was created 2225* [DeviceRequest](devicerequest.html): Encounter during which request was created 2226* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2227* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2228* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2229* [Flag](flag.html): Alert relevant during encounter 2230* [ImagingStudy](imagingstudy.html): The context of the study 2231* [List](list.html): Context in which list created 2232* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2233* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2234* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2235* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2236* [Observation](observation.html): Encounter related to the observation 2237* [Procedure](procedure.html): The Encounter during which this Procedure was created 2238* [Provenance](provenance.html): Encounter related to the Provenance 2239* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2240* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2241* [RiskAssessment](riskassessment.html): Where was assessment performed? 2242* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2243* [Task](task.html): Search by encounter 2244* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2245</b><br> 2246 * Type: <b>reference</b><br> 2247 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2248 * </p> 2249 */ 2250 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2251 public static final String SP_ENCOUNTER = "encounter"; 2252 /** 2253 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2254 * <p> 2255 * Description: <b>Multiple Resources: 2256 2257* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2258* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2259* [ChargeItem](chargeitem.html): Encounter associated with event 2260* [Claim](claim.html): Encounters associated with a billed line item 2261* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2262* [Communication](communication.html): The Encounter during which this Communication was created 2263* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2264* [Composition](composition.html): Context of the Composition 2265* [Condition](condition.html): The Encounter during which this Condition was created 2266* [DeviceRequest](devicerequest.html): Encounter during which request was created 2267* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2268* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2269* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2270* [Flag](flag.html): Alert relevant during encounter 2271* [ImagingStudy](imagingstudy.html): The context of the study 2272* [List](list.html): Context in which list created 2273* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2274* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2275* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2276* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2277* [Observation](observation.html): Encounter related to the observation 2278* [Procedure](procedure.html): The Encounter during which this Procedure was created 2279* [Provenance](provenance.html): Encounter related to the Provenance 2280* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2281* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2282* [RiskAssessment](riskassessment.html): Where was assessment performed? 2283* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2284* [Task](task.html): Search by encounter 2285* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2286</b><br> 2287 * Type: <b>reference</b><br> 2288 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2289 * </p> 2290 */ 2291 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2292 2293/** 2294 * Constant for fluent queries to be used to add include statements. Specifies 2295 * the path value of "<b>QuestionnaireResponse:encounter</b>". 2296 */ 2297 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:encounter").toLocked(); 2298 2299 /** 2300 * Search parameter: <b>identifier</b> 2301 * <p> 2302 * Description: <b>Multiple Resources: 2303 2304* [Account](account.html): Account number 2305* [AdverseEvent](adverseevent.html): Business identifier for the event 2306* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2307* [Appointment](appointment.html): An Identifier of the Appointment 2308* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2309* [Basic](basic.html): Business identifier 2310* [BodyStructure](bodystructure.html): Bodystructure identifier 2311* [CarePlan](careplan.html): External Ids for this plan 2312* [CareTeam](careteam.html): External Ids for this team 2313* [ChargeItem](chargeitem.html): Business Identifier for item 2314* [Claim](claim.html): The primary identifier of the financial resource 2315* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2316* [ClinicalImpression](clinicalimpression.html): Business identifier 2317* [Communication](communication.html): Unique identifier 2318* [CommunicationRequest](communicationrequest.html): Unique identifier 2319* [Composition](composition.html): Version-independent identifier for the Composition 2320* [Condition](condition.html): A unique identifier of the condition record 2321* [Consent](consent.html): Identifier for this record (external references) 2322* [Contract](contract.html): The identity of the contract 2323* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2324* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2325* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2326* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2327* [DeviceRequest](devicerequest.html): Business identifier for request/order 2328* [DeviceUsage](deviceusage.html): Search by identifier 2329* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2330* [DocumentReference](documentreference.html): Identifier of the attachment binary 2331* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2332* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2333* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2334* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2335* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2336* [Flag](flag.html): Business identifier 2337* [Goal](goal.html): External Ids for this goal 2338* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2339* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2340* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2341* [Immunization](immunization.html): Business identifier 2342* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2343* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2344* [Invoice](invoice.html): Business Identifier for item 2345* [List](list.html): Business identifier 2346* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2347* [Medication](medication.html): Returns medications with this external identifier 2348* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2349* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2350* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2351* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2352* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2353* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2354* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2355* [Observation](observation.html): The unique id for a particular observation 2356* [Person](person.html): A person Identifier 2357* [Procedure](procedure.html): A unique identifier for a procedure 2358* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2359* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2360* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2361* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2362* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2363* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2364* [Specimen](specimen.html): The unique identifier associated with the specimen 2365* [SupplyDelivery](supplydelivery.html): External identifier 2366* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2367* [Task](task.html): Search for a task instance by its business identifier 2368* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2369</b><br> 2370 * Type: <b>token</b><br> 2371 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2372 * </p> 2373 */ 2374 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2375 public static final String SP_IDENTIFIER = "identifier"; 2376 /** 2377 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2378 * <p> 2379 * Description: <b>Multiple Resources: 2380 2381* [Account](account.html): Account number 2382* [AdverseEvent](adverseevent.html): Business identifier for the event 2383* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2384* [Appointment](appointment.html): An Identifier of the Appointment 2385* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2386* [Basic](basic.html): Business identifier 2387* [BodyStructure](bodystructure.html): Bodystructure identifier 2388* [CarePlan](careplan.html): External Ids for this plan 2389* [CareTeam](careteam.html): External Ids for this team 2390* [ChargeItem](chargeitem.html): Business Identifier for item 2391* [Claim](claim.html): The primary identifier of the financial resource 2392* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2393* [ClinicalImpression](clinicalimpression.html): Business identifier 2394* [Communication](communication.html): Unique identifier 2395* [CommunicationRequest](communicationrequest.html): Unique identifier 2396* [Composition](composition.html): Version-independent identifier for the Composition 2397* [Condition](condition.html): A unique identifier of the condition record 2398* [Consent](consent.html): Identifier for this record (external references) 2399* [Contract](contract.html): The identity of the contract 2400* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2401* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2402* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2403* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2404* [DeviceRequest](devicerequest.html): Business identifier for request/order 2405* [DeviceUsage](deviceusage.html): Search by identifier 2406* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2407* [DocumentReference](documentreference.html): Identifier of the attachment binary 2408* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2409* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2410* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2411* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2412* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2413* [Flag](flag.html): Business identifier 2414* [Goal](goal.html): External Ids for this goal 2415* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2416* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2417* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2418* [Immunization](immunization.html): Business identifier 2419* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2420* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2421* [Invoice](invoice.html): Business Identifier for item 2422* [List](list.html): Business identifier 2423* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2424* [Medication](medication.html): Returns medications with this external identifier 2425* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2426* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2427* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2428* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2429* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2430* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2431* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2432* [Observation](observation.html): The unique id for a particular observation 2433* [Person](person.html): A person Identifier 2434* [Procedure](procedure.html): A unique identifier for a procedure 2435* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2436* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2437* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2438* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2439* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2440* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2441* [Specimen](specimen.html): The unique identifier associated with the specimen 2442* [SupplyDelivery](supplydelivery.html): External identifier 2443* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2444* [Task](task.html): Search for a task instance by its business identifier 2445* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2446</b><br> 2447 * Type: <b>token</b><br> 2448 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2449 * </p> 2450 */ 2451 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2452 2453 /** 2454 * Search parameter: <b>patient</b> 2455 * <p> 2456 * Description: <b>Multiple Resources: 2457 2458* [Account](account.html): The entity that caused the expenses 2459* [AdverseEvent](adverseevent.html): Subject impacted by event 2460* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2461* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2462* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2463* [AuditEvent](auditevent.html): Where the activity involved patient data 2464* [Basic](basic.html): Identifies the focus of this resource 2465* [BodyStructure](bodystructure.html): Who this is about 2466* [CarePlan](careplan.html): Who the care plan is for 2467* [CareTeam](careteam.html): Who care team is for 2468* [ChargeItem](chargeitem.html): Individual service was done for/to 2469* [Claim](claim.html): Patient receiving the products or services 2470* [ClaimResponse](claimresponse.html): The subject of care 2471* [ClinicalImpression](clinicalimpression.html): Patient assessed 2472* [Communication](communication.html): Focus of message 2473* [CommunicationRequest](communicationrequest.html): Focus of message 2474* [Composition](composition.html): Who and/or what the composition is about 2475* [Condition](condition.html): Who has the condition? 2476* [Consent](consent.html): Who the consent applies to 2477* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2478* [Coverage](coverage.html): Retrieve coverages for a patient 2479* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2480* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2481* [DetectedIssue](detectedissue.html): Associated patient 2482* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2483* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2484* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2485* [DocumentReference](documentreference.html): Who/what is the subject of the document 2486* [Encounter](encounter.html): The patient present at the encounter 2487* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2488* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2489* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2490* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2491* [Flag](flag.html): The identity of a subject to list flags for 2492* [Goal](goal.html): Who this goal is intended for 2493* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2494* [ImagingSelection](imagingselection.html): Who the study is about 2495* [ImagingStudy](imagingstudy.html): Who the study is about 2496* [Immunization](immunization.html): The patient for the vaccination record 2497* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2498* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2499* [Invoice](invoice.html): Recipient(s) of goods and services 2500* [List](list.html): If all resources have the same subject 2501* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2502* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2503* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2504* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2505* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2506* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2507* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2508* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2509* [Observation](observation.html): The subject that the observation is about (if patient) 2510* [Person](person.html): The Person links to this Patient 2511* [Procedure](procedure.html): Search by subject - a patient 2512* [Provenance](provenance.html): Where the activity involved patient data 2513* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2514* [RelatedPerson](relatedperson.html): The patient this related person is related to 2515* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2516* [ResearchSubject](researchsubject.html): Who or what is part of study 2517* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2518* [ServiceRequest](servicerequest.html): Search by subject - a patient 2519* [Specimen](specimen.html): The patient the specimen comes from 2520* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2521* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2522* [Task](task.html): Search by patient 2523* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2524</b><br> 2525 * Type: <b>reference</b><br> 2526 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2527 * </p> 2528 */ 2529 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2530 public static final String SP_PATIENT = "patient"; 2531 /** 2532 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2533 * <p> 2534 * Description: <b>Multiple Resources: 2535 2536* [Account](account.html): The entity that caused the expenses 2537* [AdverseEvent](adverseevent.html): Subject impacted by event 2538* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2539* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2540* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2541* [AuditEvent](auditevent.html): Where the activity involved patient data 2542* [Basic](basic.html): Identifies the focus of this resource 2543* [BodyStructure](bodystructure.html): Who this is about 2544* [CarePlan](careplan.html): Who the care plan is for 2545* [CareTeam](careteam.html): Who care team is for 2546* [ChargeItem](chargeitem.html): Individual service was done for/to 2547* [Claim](claim.html): Patient receiving the products or services 2548* [ClaimResponse](claimresponse.html): The subject of care 2549* [ClinicalImpression](clinicalimpression.html): Patient assessed 2550* [Communication](communication.html): Focus of message 2551* [CommunicationRequest](communicationrequest.html): Focus of message 2552* [Composition](composition.html): Who and/or what the composition is about 2553* [Condition](condition.html): Who has the condition? 2554* [Consent](consent.html): Who the consent applies to 2555* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2556* [Coverage](coverage.html): Retrieve coverages for a patient 2557* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2558* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2559* [DetectedIssue](detectedissue.html): Associated patient 2560* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2561* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2562* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2563* [DocumentReference](documentreference.html): Who/what is the subject of the document 2564* [Encounter](encounter.html): The patient present at the encounter 2565* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2566* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2567* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2568* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2569* [Flag](flag.html): The identity of a subject to list flags for 2570* [Goal](goal.html): Who this goal is intended for 2571* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2572* [ImagingSelection](imagingselection.html): Who the study is about 2573* [ImagingStudy](imagingstudy.html): Who the study is about 2574* [Immunization](immunization.html): The patient for the vaccination record 2575* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2576* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2577* [Invoice](invoice.html): Recipient(s) of goods and services 2578* [List](list.html): If all resources have the same subject 2579* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2580* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2581* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2582* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2583* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2584* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2585* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2586* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2587* [Observation](observation.html): The subject that the observation is about (if patient) 2588* [Person](person.html): The Person links to this Patient 2589* [Procedure](procedure.html): Search by subject - a patient 2590* [Provenance](provenance.html): Where the activity involved patient data 2591* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2592* [RelatedPerson](relatedperson.html): The patient this related person is related to 2593* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2594* [ResearchSubject](researchsubject.html): Who or what is part of study 2595* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2596* [ServiceRequest](servicerequest.html): Search by subject - a patient 2597* [Specimen](specimen.html): The patient the specimen comes from 2598* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2599* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2600* [Task](task.html): Search by patient 2601* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2602</b><br> 2603 * Type: <b>reference</b><br> 2604 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2605 * </p> 2606 */ 2607 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2608 2609/** 2610 * Constant for fluent queries to be used to add include statements. Specifies 2611 * the path value of "<b>QuestionnaireResponse:patient</b>". 2612 */ 2613 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:patient").toLocked(); 2614 2615 2616} 2617