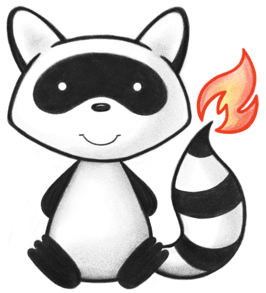
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 052 */ 053@ResourceDef(name="QuestionnaireResponse", profile="http://hl7.org/fhir/StructureDefinition/QuestionnaireResponse") 054public class QuestionnaireResponse extends DomainResource { 055 056 public enum QuestionnaireResponseStatus { 057 /** 058 * This QuestionnaireResponse has been partially filled out with answers but changes or additions are still expected to be made to it. 059 */ 060 INPROGRESS, 061 /** 062 * This QuestionnaireResponse has been filled out with answers and the current content is regarded as definitive. 063 */ 064 COMPLETED, 065 /** 066 * This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards. 067 */ 068 AMENDED, 069 /** 070 * This QuestionnaireResponse was entered in error and voided. 071 */ 072 ENTEREDINERROR, 073 /** 074 * This QuestionnaireResponse has been partially filled out with answers but has been abandoned. No subsequent changes can be made. 075 */ 076 STOPPED, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static QuestionnaireResponseStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("in-progress".equals(codeString)) 085 return INPROGRESS; 086 if ("completed".equals(codeString)) 087 return COMPLETED; 088 if ("amended".equals(codeString)) 089 return AMENDED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("stopped".equals(codeString)) 093 return STOPPED; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case INPROGRESS: return "in-progress"; 102 case COMPLETED: return "completed"; 103 case AMENDED: return "amended"; 104 case ENTEREDINERROR: return "entered-in-error"; 105 case STOPPED: return "stopped"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case INPROGRESS: return "http://hl7.org/fhir/questionnaire-answers-status"; 113 case COMPLETED: return "http://hl7.org/fhir/questionnaire-answers-status"; 114 case AMENDED: return "http://hl7.org/fhir/questionnaire-answers-status"; 115 case ENTEREDINERROR: return "http://hl7.org/fhir/questionnaire-answers-status"; 116 case STOPPED: return "http://hl7.org/fhir/questionnaire-answers-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case INPROGRESS: return "This QuestionnaireResponse has been partially filled out with answers but changes or additions are still expected to be made to it."; 124 case COMPLETED: return "This QuestionnaireResponse has been filled out with answers and the current content is regarded as definitive."; 125 case AMENDED: return "This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards."; 126 case ENTEREDINERROR: return "This QuestionnaireResponse was entered in error and voided."; 127 case STOPPED: return "This QuestionnaireResponse has been partially filled out with answers but has been abandoned. No subsequent changes can be made."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case INPROGRESS: return "In Progress"; 135 case COMPLETED: return "Completed"; 136 case AMENDED: return "Amended"; 137 case ENTEREDINERROR: return "Entered in Error"; 138 case STOPPED: return "Stopped"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class QuestionnaireResponseStatusEnumFactory implements EnumFactory<QuestionnaireResponseStatus> { 146 public QuestionnaireResponseStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("in-progress".equals(codeString)) 151 return QuestionnaireResponseStatus.INPROGRESS; 152 if ("completed".equals(codeString)) 153 return QuestionnaireResponseStatus.COMPLETED; 154 if ("amended".equals(codeString)) 155 return QuestionnaireResponseStatus.AMENDED; 156 if ("entered-in-error".equals(codeString)) 157 return QuestionnaireResponseStatus.ENTEREDINERROR; 158 if ("stopped".equals(codeString)) 159 return QuestionnaireResponseStatus.STOPPED; 160 throw new IllegalArgumentException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 161 } 162 public Enumeration<QuestionnaireResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 170 if ("in-progress".equals(codeString)) 171 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.INPROGRESS, code); 172 if ("completed".equals(codeString)) 173 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.COMPLETED, code); 174 if ("amended".equals(codeString)) 175 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.AMENDED, code); 176 if ("entered-in-error".equals(codeString)) 177 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.ENTEREDINERROR, code); 178 if ("stopped".equals(codeString)) 179 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.STOPPED, code); 180 throw new FHIRException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 181 } 182 public String toCode(QuestionnaireResponseStatus code) { 183 if (code == QuestionnaireResponseStatus.NULL) 184 return null; 185 if (code == QuestionnaireResponseStatus.INPROGRESS) 186 return "in-progress"; 187 if (code == QuestionnaireResponseStatus.COMPLETED) 188 return "completed"; 189 if (code == QuestionnaireResponseStatus.AMENDED) 190 return "amended"; 191 if (code == QuestionnaireResponseStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 if (code == QuestionnaireResponseStatus.STOPPED) 194 return "stopped"; 195 return "?"; 196 } 197 public String toSystem(QuestionnaireResponseStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class QuestionnaireResponseItemComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 206 */ 207 @Child(name = "linkId", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 208 @Description(shortDefinition="Pointer to specific item from Questionnaire", formalDefinition="The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource." ) 209 protected StringType linkId; 210 211 /** 212 * A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item. 213 */ 214 @Child(name = "definition", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 215 @Description(shortDefinition="ElementDefinition - details for the item", formalDefinition="A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item." ) 216 protected UriType definition; 217 218 /** 219 * Text that is displayed above the contents of the group or as the text of the question being answered. 220 */ 221 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Name for group or question text", formalDefinition="Text that is displayed above the contents of the group or as the text of the question being answered." ) 223 protected StringType text; 224 225 /** 226 * The respondent's answer(s) to the question. 227 */ 228 @Child(name = "answer", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 229 @Description(shortDefinition="The response(s) to the question", formalDefinition="The respondent's answer(s) to the question." ) 230 protected List<QuestionnaireResponseItemAnswerComponent> answer; 231 232 /** 233 * Sub-questions, sub-groups or display items nested beneath a group. 234 */ 235 @Child(name = "item", type = {QuestionnaireResponseItemComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 236 @Description(shortDefinition="Child items of group item", formalDefinition="Sub-questions, sub-groups or display items nested beneath a group." ) 237 protected List<QuestionnaireResponseItemComponent> item; 238 239 private static final long serialVersionUID = -1395483402L; 240 241 /** 242 * Constructor 243 */ 244 public QuestionnaireResponseItemComponent() { 245 super(); 246 } 247 248 /** 249 * Constructor 250 */ 251 public QuestionnaireResponseItemComponent(String linkId) { 252 super(); 253 this.setLinkId(linkId); 254 } 255 256 /** 257 * @return {@link #linkId} (The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 258 */ 259 public StringType getLinkIdElement() { 260 if (this.linkId == null) 261 if (Configuration.errorOnAutoCreate()) 262 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.linkId"); 263 else if (Configuration.doAutoCreate()) 264 this.linkId = new StringType(); // bb 265 return this.linkId; 266 } 267 268 public boolean hasLinkIdElement() { 269 return this.linkId != null && !this.linkId.isEmpty(); 270 } 271 272 public boolean hasLinkId() { 273 return this.linkId != null && !this.linkId.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #linkId} (The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 278 */ 279 public QuestionnaireResponseItemComponent setLinkIdElement(StringType value) { 280 this.linkId = value; 281 return this; 282 } 283 284 /** 285 * @return The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 286 */ 287 public String getLinkId() { 288 return this.linkId == null ? null : this.linkId.getValue(); 289 } 290 291 /** 292 * @param value The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 293 */ 294 public QuestionnaireResponseItemComponent setLinkId(String value) { 295 if (this.linkId == null) 296 this.linkId = new StringType(); 297 this.linkId.setValue(value); 298 return this; 299 } 300 301 /** 302 * @return {@link #definition} (A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 303 */ 304 public UriType getDefinitionElement() { 305 if (this.definition == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.definition"); 308 else if (Configuration.doAutoCreate()) 309 this.definition = new UriType(); // bb 310 return this.definition; 311 } 312 313 public boolean hasDefinitionElement() { 314 return this.definition != null && !this.definition.isEmpty(); 315 } 316 317 public boolean hasDefinition() { 318 return this.definition != null && !this.definition.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #definition} (A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 323 */ 324 public QuestionnaireResponseItemComponent setDefinitionElement(UriType value) { 325 this.definition = value; 326 return this; 327 } 328 329 /** 330 * @return A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item. 331 */ 332 public String getDefinition() { 333 return this.definition == null ? null : this.definition.getValue(); 334 } 335 336 /** 337 * @param value A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item. 338 */ 339 public QuestionnaireResponseItemComponent setDefinition(String value) { 340 if (Utilities.noString(value)) 341 this.definition = null; 342 else { 343 if (this.definition == null) 344 this.definition = new UriType(); 345 this.definition.setValue(value); 346 } 347 return this; 348 } 349 350 /** 351 * @return {@link #text} (Text that is displayed above the contents of the group or as the text of the question being answered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 352 */ 353 public StringType getTextElement() { 354 if (this.text == null) 355 if (Configuration.errorOnAutoCreate()) 356 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.text"); 357 else if (Configuration.doAutoCreate()) 358 this.text = new StringType(); // bb 359 return this.text; 360 } 361 362 public boolean hasTextElement() { 363 return this.text != null && !this.text.isEmpty(); 364 } 365 366 public boolean hasText() { 367 return this.text != null && !this.text.isEmpty(); 368 } 369 370 /** 371 * @param value {@link #text} (Text that is displayed above the contents of the group or as the text of the question being answered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 372 */ 373 public QuestionnaireResponseItemComponent setTextElement(StringType value) { 374 this.text = value; 375 return this; 376 } 377 378 /** 379 * @return Text that is displayed above the contents of the group or as the text of the question being answered. 380 */ 381 public String getText() { 382 return this.text == null ? null : this.text.getValue(); 383 } 384 385 /** 386 * @param value Text that is displayed above the contents of the group or as the text of the question being answered. 387 */ 388 public QuestionnaireResponseItemComponent setText(String value) { 389 if (Utilities.noString(value)) 390 this.text = null; 391 else { 392 if (this.text == null) 393 this.text = new StringType(); 394 this.text.setValue(value); 395 } 396 return this; 397 } 398 399 /** 400 * @return {@link #answer} (The respondent's answer(s) to the question.) 401 */ 402 public List<QuestionnaireResponseItemAnswerComponent> getAnswer() { 403 if (this.answer == null) 404 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 405 return this.answer; 406 } 407 408 /** 409 * @return Returns a reference to <code>this</code> for easy method chaining 410 */ 411 public QuestionnaireResponseItemComponent setAnswer(List<QuestionnaireResponseItemAnswerComponent> theAnswer) { 412 this.answer = theAnswer; 413 return this; 414 } 415 416 public boolean hasAnswer() { 417 if (this.answer == null) 418 return false; 419 for (QuestionnaireResponseItemAnswerComponent item : this.answer) 420 if (!item.isEmpty()) 421 return true; 422 return false; 423 } 424 425 public QuestionnaireResponseItemAnswerComponent addAnswer() { //3 426 QuestionnaireResponseItemAnswerComponent t = new QuestionnaireResponseItemAnswerComponent(); 427 if (this.answer == null) 428 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 429 this.answer.add(t); 430 return t; 431 } 432 433 public QuestionnaireResponseItemComponent addAnswer(QuestionnaireResponseItemAnswerComponent t) { //3 434 if (t == null) 435 return this; 436 if (this.answer == null) 437 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 438 this.answer.add(t); 439 return this; 440 } 441 442 /** 443 * @return The first repetition of repeating field {@link #answer}, creating it if it does not already exist {3} 444 */ 445 public QuestionnaireResponseItemAnswerComponent getAnswerFirstRep() { 446 if (getAnswer().isEmpty()) { 447 addAnswer(); 448 } 449 return getAnswer().get(0); 450 } 451 452 /** 453 * @return {@link #item} (Sub-questions, sub-groups or display items nested beneath a group.) 454 */ 455 public List<QuestionnaireResponseItemComponent> getItem() { 456 if (this.item == null) 457 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 458 return this.item; 459 } 460 461 /** 462 * @return Returns a reference to <code>this</code> for easy method chaining 463 */ 464 public QuestionnaireResponseItemComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 465 this.item = theItem; 466 return this; 467 } 468 469 public boolean hasItem() { 470 if (this.item == null) 471 return false; 472 for (QuestionnaireResponseItemComponent item : this.item) 473 if (!item.isEmpty()) 474 return true; 475 return false; 476 } 477 478 public QuestionnaireResponseItemComponent addItem() { //3 479 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 480 if (this.item == null) 481 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 482 this.item.add(t); 483 return t; 484 } 485 486 public QuestionnaireResponseItemComponent addItem(QuestionnaireResponseItemComponent t) { //3 487 if (t == null) 488 return this; 489 if (this.item == null) 490 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 491 this.item.add(t); 492 return this; 493 } 494 495 /** 496 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 497 */ 498 public QuestionnaireResponseItemComponent getItemFirstRep() { 499 if (getItem().isEmpty()) { 500 addItem(); 501 } 502 return getItem().get(0); 503 } 504 505 protected void listChildren(List<Property> children) { 506 super.listChildren(children); 507 children.add(new Property("linkId", "string", "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, linkId)); 508 children.add(new Property("definition", "uri", "A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.", 0, 1, definition)); 509 children.add(new Property("text", "string", "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, text)); 510 children.add(new Property("answer", "", "The respondent's answer(s) to the question.", 0, java.lang.Integer.MAX_VALUE, answer)); 511 children.add(new Property("item", "@QuestionnaireResponse.item", "Sub-questions, sub-groups or display items nested beneath a group.", 0, java.lang.Integer.MAX_VALUE, item)); 512 } 513 514 @Override 515 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 516 switch (_hash) { 517 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, linkId); 518 case -1014418093: /*definition*/ return new Property("definition", "uri", "A reference to an [ElementDefinition](elementdefinition.html) that provides the details for the item.", 0, 1, definition); 519 case 3556653: /*text*/ return new Property("text", "string", "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, text); 520 case -1412808770: /*answer*/ return new Property("answer", "", "The respondent's answer(s) to the question.", 0, java.lang.Integer.MAX_VALUE, answer); 521 case 3242771: /*item*/ return new Property("item", "@QuestionnaireResponse.item", "Sub-questions, sub-groups or display items nested beneath a group.", 0, java.lang.Integer.MAX_VALUE, item); 522 default: return super.getNamedProperty(_hash, _name, _checkValid); 523 } 524 525 } 526 527 @Override 528 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 529 switch (hash) { 530 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 531 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // UriType 532 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 533 case -1412808770: /*answer*/ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // QuestionnaireResponseItemAnswerComponent 534 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 535 default: return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case -1102667083: // linkId 544 this.linkId = TypeConvertor.castToString(value); // StringType 545 return value; 546 case -1014418093: // definition 547 this.definition = TypeConvertor.castToUri(value); // UriType 548 return value; 549 case 3556653: // text 550 this.text = TypeConvertor.castToString(value); // StringType 551 return value; 552 case -1412808770: // answer 553 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); // QuestionnaireResponseItemAnswerComponent 554 return value; 555 case 3242771: // item 556 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 557 return value; 558 default: return super.setProperty(hash, name, value); 559 } 560 561 } 562 563 @Override 564 public Base setProperty(String name, Base value) throws FHIRException { 565 if (name.equals("linkId")) { 566 this.linkId = TypeConvertor.castToString(value); // StringType 567 } else if (name.equals("definition")) { 568 this.definition = TypeConvertor.castToUri(value); // UriType 569 } else if (name.equals("text")) { 570 this.text = TypeConvertor.castToString(value); // StringType 571 } else if (name.equals("answer")) { 572 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); 573 } else if (name.equals("item")) { 574 this.getItem().add((QuestionnaireResponseItemComponent) value); 575 } else 576 return super.setProperty(name, value); 577 return value; 578 } 579 580 @Override 581 public void removeChild(String name, Base value) throws FHIRException { 582 if (name.equals("linkId")) { 583 this.linkId = null; 584 } else if (name.equals("definition")) { 585 this.definition = null; 586 } else if (name.equals("text")) { 587 this.text = null; 588 } else if (name.equals("answer")) { 589 this.getAnswer().remove((QuestionnaireResponseItemAnswerComponent) value); 590 } else if (name.equals("item")) { 591 this.getItem().remove((QuestionnaireResponseItemComponent) value); 592 } else 593 super.removeChild(name, value); 594 595 } 596 597 @Override 598 public Base makeProperty(int hash, String name) throws FHIRException { 599 switch (hash) { 600 case -1102667083: return getLinkIdElement(); 601 case -1014418093: return getDefinitionElement(); 602 case 3556653: return getTextElement(); 603 case -1412808770: return addAnswer(); 604 case 3242771: return addItem(); 605 default: return super.makeProperty(hash, name); 606 } 607 608 } 609 610 @Override 611 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 612 switch (hash) { 613 case -1102667083: /*linkId*/ return new String[] {"string"}; 614 case -1014418093: /*definition*/ return new String[] {"uri"}; 615 case 3556653: /*text*/ return new String[] {"string"}; 616 case -1412808770: /*answer*/ return new String[] {}; 617 case 3242771: /*item*/ return new String[] {"@QuestionnaireResponse.item"}; 618 default: return super.getTypesForProperty(hash, name); 619 } 620 621 } 622 623 @Override 624 public Base addChild(String name) throws FHIRException { 625 if (name.equals("linkId")) { 626 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.item.linkId"); 627 } 628 else if (name.equals("definition")) { 629 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.item.definition"); 630 } 631 else if (name.equals("text")) { 632 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.item.text"); 633 } 634 else if (name.equals("answer")) { 635 return addAnswer(); 636 } 637 else if (name.equals("item")) { 638 return addItem(); 639 } 640 else 641 return super.addChild(name); 642 } 643 644 public QuestionnaireResponseItemComponent copy() { 645 QuestionnaireResponseItemComponent dst = new QuestionnaireResponseItemComponent(); 646 copyValues(dst); 647 return dst; 648 } 649 650 public void copyValues(QuestionnaireResponseItemComponent dst) { 651 super.copyValues(dst); 652 dst.linkId = linkId == null ? null : linkId.copy(); 653 dst.definition = definition == null ? null : definition.copy(); 654 dst.text = text == null ? null : text.copy(); 655 if (answer != null) { 656 dst.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 657 for (QuestionnaireResponseItemAnswerComponent i : answer) 658 dst.answer.add(i.copy()); 659 }; 660 if (item != null) { 661 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 662 for (QuestionnaireResponseItemComponent i : item) 663 dst.item.add(i.copy()); 664 }; 665 } 666 667 @Override 668 public boolean equalsDeep(Base other_) { 669 if (!super.equalsDeep(other_)) 670 return false; 671 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 672 return false; 673 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 674 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) && compareDeep(text, o.text, true) 675 && compareDeep(answer, o.answer, true) && compareDeep(item, o.item, true); 676 } 677 678 @Override 679 public boolean equalsShallow(Base other_) { 680 if (!super.equalsShallow(other_)) 681 return false; 682 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 683 return false; 684 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 685 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) && compareValues(text, o.text, true) 686 ; 687 } 688 689 public boolean isEmpty() { 690 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, text 691 , answer, item); 692 } 693 694 public String fhirType() { 695 return "QuestionnaireResponse.item"; 696 697 } 698 699 } 700 701 @Block() 702 public static class QuestionnaireResponseItemAnswerComponent extends BackboneElement implements IBaseBackboneElement { 703 /** 704 * The answer (or one of the answers) provided by the respondent to the question. 705 */ 706 @Child(name = "value", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, Quantity.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 707 @Description(shortDefinition="Single-valued answer to the question", formalDefinition="The answer (or one of the answers) provided by the respondent to the question." ) 708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 709 protected DataType value; 710 711 /** 712 * Nested groups and/or questions found within this particular answer. 713 */ 714 @Child(name = "item", type = {QuestionnaireResponseItemComponent.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 715 @Description(shortDefinition="Child items of question", formalDefinition="Nested groups and/or questions found within this particular answer." ) 716 protected List<QuestionnaireResponseItemComponent> item; 717 718 private static final long serialVersionUID = 1790747618L; 719 720 /** 721 * Constructor 722 */ 723 public QuestionnaireResponseItemAnswerComponent() { 724 super(); 725 } 726 727 /** 728 * Constructor 729 */ 730 public QuestionnaireResponseItemAnswerComponent(DataType value) { 731 super(); 732 this.setValue(value); 733 } 734 735 /** 736 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 737 */ 738 public DataType getValue() { 739 return this.value; 740 } 741 742 /** 743 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 744 */ 745 public BooleanType getValueBooleanType() throws FHIRException { 746 if (this.value == null) 747 this.value = new BooleanType(); 748 if (!(this.value instanceof BooleanType)) 749 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 750 return (BooleanType) this.value; 751 } 752 753 public boolean hasValueBooleanType() { 754 return this.value instanceof BooleanType; 755 } 756 757 /** 758 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 759 */ 760 public DecimalType getValueDecimalType() throws FHIRException { 761 if (this.value == null) 762 this.value = new DecimalType(); 763 if (!(this.value instanceof DecimalType)) 764 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 765 return (DecimalType) this.value; 766 } 767 768 public boolean hasValueDecimalType() { 769 return this.value instanceof DecimalType; 770 } 771 772 /** 773 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 774 */ 775 public IntegerType getValueIntegerType() throws FHIRException { 776 if (this.value == null) 777 this.value = new IntegerType(); 778 if (!(this.value instanceof IntegerType)) 779 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 780 return (IntegerType) this.value; 781 } 782 783 public boolean hasValueIntegerType() { 784 return this.value instanceof IntegerType; 785 } 786 787 /** 788 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 789 */ 790 public DateType getValueDateType() throws FHIRException { 791 if (this.value == null) 792 this.value = new DateType(); 793 if (!(this.value instanceof DateType)) 794 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 795 return (DateType) this.value; 796 } 797 798 public boolean hasValueDateType() { 799 return this.value instanceof DateType; 800 } 801 802 /** 803 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 804 */ 805 public DateTimeType getValueDateTimeType() throws FHIRException { 806 if (this.value == null) 807 this.value = new DateTimeType(); 808 if (!(this.value instanceof DateTimeType)) 809 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 810 return (DateTimeType) this.value; 811 } 812 813 public boolean hasValueDateTimeType() { 814 return this.value instanceof DateTimeType; 815 } 816 817 /** 818 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 819 */ 820 public TimeType getValueTimeType() throws FHIRException { 821 if (this.value == null) 822 this.value = new TimeType(); 823 if (!(this.value instanceof TimeType)) 824 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 825 return (TimeType) this.value; 826 } 827 828 public boolean hasValueTimeType() { 829 return this.value instanceof TimeType; 830 } 831 832 /** 833 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 834 */ 835 public StringType getValueStringType() throws FHIRException { 836 if (this.value == null) 837 this.value = new StringType(); 838 if (!(this.value instanceof StringType)) 839 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 840 return (StringType) this.value; 841 } 842 843 public boolean hasValueStringType() { 844 return this.value instanceof StringType; 845 } 846 847 /** 848 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 849 */ 850 public UriType getValueUriType() throws FHIRException { 851 if (this.value == null) 852 this.value = new UriType(); 853 if (!(this.value instanceof UriType)) 854 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 855 return (UriType) this.value; 856 } 857 858 public boolean hasValueUriType() { 859 return this.value instanceof UriType; 860 } 861 862 /** 863 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 864 */ 865 public Attachment getValueAttachment() throws FHIRException { 866 if (this.value == null) 867 this.value = new Attachment(); 868 if (!(this.value instanceof Attachment)) 869 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 870 return (Attachment) this.value; 871 } 872 873 public boolean hasValueAttachment() { 874 return this.value instanceof Attachment; 875 } 876 877 /** 878 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 879 */ 880 public Coding getValueCoding() throws FHIRException { 881 if (this.value == null) 882 this.value = new Coding(); 883 if (!(this.value instanceof Coding)) 884 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 885 return (Coding) this.value; 886 } 887 888 public boolean hasValueCoding() { 889 return this.value instanceof Coding; 890 } 891 892 /** 893 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 894 */ 895 public Quantity getValueQuantity() throws FHIRException { 896 if (this.value == null) 897 this.value = new Quantity(); 898 if (!(this.value instanceof Quantity)) 899 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 900 return (Quantity) this.value; 901 } 902 903 public boolean hasValueQuantity() { 904 return this.value instanceof Quantity; 905 } 906 907 /** 908 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 909 */ 910 public Reference getValueReference() throws FHIRException { 911 if (this.value == null) 912 this.value = new Reference(); 913 if (!(this.value instanceof Reference)) 914 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 915 return (Reference) this.value; 916 } 917 918 public boolean hasValueReference() { 919 return this.value instanceof Reference; 920 } 921 922 public boolean hasValue() { 923 return this.value != null && !this.value.isEmpty(); 924 } 925 926 /** 927 * @param value {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 928 */ 929 public QuestionnaireResponseItemAnswerComponent setValue(DataType value) { 930 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof UriType || value instanceof Attachment || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 931 throw new FHIRException("Not the right type for QuestionnaireResponse.item.answer.value[x]: "+value.fhirType()); 932 this.value = value; 933 return this; 934 } 935 936 /** 937 * @return {@link #item} (Nested groups and/or questions found within this particular answer.) 938 */ 939 public List<QuestionnaireResponseItemComponent> getItem() { 940 if (this.item == null) 941 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 942 return this.item; 943 } 944 945 /** 946 * @return Returns a reference to <code>this</code> for easy method chaining 947 */ 948 public QuestionnaireResponseItemAnswerComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 949 this.item = theItem; 950 return this; 951 } 952 953 public boolean hasItem() { 954 if (this.item == null) 955 return false; 956 for (QuestionnaireResponseItemComponent item : this.item) 957 if (!item.isEmpty()) 958 return true; 959 return false; 960 } 961 962 public QuestionnaireResponseItemComponent addItem() { //3 963 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 964 if (this.item == null) 965 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 966 this.item.add(t); 967 return t; 968 } 969 970 public QuestionnaireResponseItemAnswerComponent addItem(QuestionnaireResponseItemComponent t) { //3 971 if (t == null) 972 return this; 973 if (this.item == null) 974 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 975 this.item.add(t); 976 return this; 977 } 978 979 /** 980 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 981 */ 982 public QuestionnaireResponseItemComponent getItemFirstRep() { 983 if (getItem().isEmpty()) { 984 addItem(); 985 } 986 return getItem().get(0); 987 } 988 989 protected void listChildren(List<Property> children) { 990 super.listChildren(children); 991 children.add(new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value)); 992 children.add(new Property("item", "@QuestionnaireResponse.item", "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item)); 993 } 994 995 @Override 996 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 997 switch (_hash) { 998 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 999 case 111972721: /*value*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1000 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1001 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1002 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1003 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1004 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1005 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1006 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1007 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1008 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1009 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1010 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1011 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1012 case 3242771: /*item*/ return new Property("item", "@QuestionnaireResponse.item", "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item); 1013 default: return super.getNamedProperty(_hash, _name, _checkValid); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1020 switch (hash) { 1021 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1022 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1023 default: return super.getProperty(hash, name, checkValid); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1030 switch (hash) { 1031 case 111972721: // value 1032 this.value = TypeConvertor.castToType(value); // DataType 1033 return value; 1034 case 3242771: // item 1035 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1036 return value; 1037 default: return super.setProperty(hash, name, value); 1038 } 1039 1040 } 1041 1042 @Override 1043 public Base setProperty(String name, Base value) throws FHIRException { 1044 if (name.equals("value[x]")) { 1045 this.value = TypeConvertor.castToType(value); // DataType 1046 } else if (name.equals("item")) { 1047 this.getItem().add((QuestionnaireResponseItemComponent) value); 1048 } else 1049 return super.setProperty(name, value); 1050 return value; 1051 } 1052 1053 @Override 1054 public void removeChild(String name, Base value) throws FHIRException { 1055 if (name.equals("value[x]")) { 1056 this.value = null; 1057 } else if (name.equals("item")) { 1058 this.getItem().remove((QuestionnaireResponseItemComponent) value); 1059 } else 1060 super.removeChild(name, value); 1061 1062 } 1063 1064 @Override 1065 public Base makeProperty(int hash, String name) throws FHIRException { 1066 switch (hash) { 1067 case -1410166417: return getValue(); 1068 case 111972721: return getValue(); 1069 case 3242771: return addItem(); 1070 default: return super.makeProperty(hash, name); 1071 } 1072 1073 } 1074 1075 @Override 1076 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1077 switch (hash) { 1078 case 111972721: /*value*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", "Attachment", "Coding", "Quantity", "Reference"}; 1079 case 3242771: /*item*/ return new String[] {"@QuestionnaireResponse.item"}; 1080 default: return super.getTypesForProperty(hash, name); 1081 } 1082 1083 } 1084 1085 @Override 1086 public Base addChild(String name) throws FHIRException { 1087 if (name.equals("valueBoolean")) { 1088 this.value = new BooleanType(); 1089 return this.value; 1090 } 1091 else if (name.equals("valueDecimal")) { 1092 this.value = new DecimalType(); 1093 return this.value; 1094 } 1095 else if (name.equals("valueInteger")) { 1096 this.value = new IntegerType(); 1097 return this.value; 1098 } 1099 else if (name.equals("valueDate")) { 1100 this.value = new DateType(); 1101 return this.value; 1102 } 1103 else if (name.equals("valueDateTime")) { 1104 this.value = new DateTimeType(); 1105 return this.value; 1106 } 1107 else if (name.equals("valueTime")) { 1108 this.value = new TimeType(); 1109 return this.value; 1110 } 1111 else if (name.equals("valueString")) { 1112 this.value = new StringType(); 1113 return this.value; 1114 } 1115 else if (name.equals("valueUri")) { 1116 this.value = new UriType(); 1117 return this.value; 1118 } 1119 else if (name.equals("valueAttachment")) { 1120 this.value = new Attachment(); 1121 return this.value; 1122 } 1123 else if (name.equals("valueCoding")) { 1124 this.value = new Coding(); 1125 return this.value; 1126 } 1127 else if (name.equals("valueQuantity")) { 1128 this.value = new Quantity(); 1129 return this.value; 1130 } 1131 else if (name.equals("valueReference")) { 1132 this.value = new Reference(); 1133 return this.value; 1134 } 1135 else if (name.equals("item")) { 1136 return addItem(); 1137 } 1138 else 1139 return super.addChild(name); 1140 } 1141 1142 public QuestionnaireResponseItemAnswerComponent copy() { 1143 QuestionnaireResponseItemAnswerComponent dst = new QuestionnaireResponseItemAnswerComponent(); 1144 copyValues(dst); 1145 return dst; 1146 } 1147 1148 public void copyValues(QuestionnaireResponseItemAnswerComponent dst) { 1149 super.copyValues(dst); 1150 dst.value = value == null ? null : value.copy(); 1151 if (item != null) { 1152 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1153 for (QuestionnaireResponseItemComponent i : item) 1154 dst.item.add(i.copy()); 1155 }; 1156 } 1157 1158 @Override 1159 public boolean equalsDeep(Base other_) { 1160 if (!super.equalsDeep(other_)) 1161 return false; 1162 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1163 return false; 1164 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1165 return compareDeep(value, o.value, true) && compareDeep(item, o.item, true); 1166 } 1167 1168 @Override 1169 public boolean equalsShallow(Base other_) { 1170 if (!super.equalsShallow(other_)) 1171 return false; 1172 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1173 return false; 1174 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1175 return true; 1176 } 1177 1178 public boolean isEmpty() { 1179 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, item); 1180 } 1181 1182 public String fhirType() { 1183 return "QuestionnaireResponse.item.answer"; 1184 1185 } 1186 1187 } 1188 1189 /** 1190 * Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server. 1191 */ 1192 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1193 @Description(shortDefinition="Business identifier for this set of answers", formalDefinition="Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server." ) 1194 protected List<Identifier> identifier; 1195 1196 /** 1197 * A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression. 1198 */ 1199 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1200 @Description(shortDefinition="Request fulfilled by this QuestionnaireResponse", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression." ) 1201 protected List<Reference> basedOn; 1202 1203 /** 1204 * A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of. 1205 */ 1206 @Child(name = "partOf", type = {Observation.class, Procedure.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1207 @Description(shortDefinition="Part of referenced event", formalDefinition="A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of." ) 1208 protected List<Reference> partOf; 1209 1210 /** 1211 * The Questionnaire that defines and organizes the questions for which answers are being provided. 1212 */ 1213 @Child(name = "questionnaire", type = {CanonicalType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1214 @Description(shortDefinition="Canonical URL of Questionnaire being answered", formalDefinition="The Questionnaire that defines and organizes the questions for which answers are being provided." ) 1215 protected CanonicalType questionnaire; 1216 1217 /** 1218 * The current state of the questionnaire response. 1219 */ 1220 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 1221 @Description(shortDefinition="in-progress | completed | amended | entered-in-error | stopped", formalDefinition="The current state of the questionnaire response." ) 1222 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers-status") 1223 protected Enumeration<QuestionnaireResponseStatus> status; 1224 1225 /** 1226 * The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information. 1227 */ 1228 @Child(name = "subject", type = {Reference.class}, order=5, min=0, max=1, modifier=false, summary=true) 1229 @Description(shortDefinition="The subject of the questions", formalDefinition="The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information." ) 1230 protected Reference subject; 1231 1232 /** 1233 * The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated. 1234 */ 1235 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 1236 @Description(shortDefinition="Encounter the questionnaire response is part of", formalDefinition="The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated." ) 1237 protected Reference encounter; 1238 1239 /** 1240 * The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status. 1241 */ 1242 @Child(name = "authored", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1243 @Description(shortDefinition="Date the answers were gathered", formalDefinition="The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status." ) 1244 protected DateTimeType authored; 1245 1246 /** 1247 * The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system. 1248 */ 1249 @Child(name = "author", type = {Device.class, Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=true) 1250 @Description(shortDefinition="The individual or device that received and recorded the answers", formalDefinition="The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system." ) 1251 protected Reference author; 1252 1253 /** 1254 * The individual or device that answered the questions about the subject. 1255 */ 1256 @Child(name = "source", type = {Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=true) 1257 @Description(shortDefinition="The individual or device that answered the questions", formalDefinition="The individual or device that answered the questions about the subject." ) 1258 protected Reference source; 1259 1260 /** 1261 * A group or question item from the original questionnaire for which answers are provided. 1262 */ 1263 @Child(name = "item", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1264 @Description(shortDefinition="Groups and questions", formalDefinition="A group or question item from the original questionnaire for which answers are provided." ) 1265 protected List<QuestionnaireResponseItemComponent> item; 1266 1267 private static final long serialVersionUID = 1660467089L; 1268 1269 /** 1270 * Constructor 1271 */ 1272 public QuestionnaireResponse() { 1273 super(); 1274 } 1275 1276 /** 1277 * Constructor 1278 */ 1279 public QuestionnaireResponse(String questionnaire, QuestionnaireResponseStatus status) { 1280 super(); 1281 this.setQuestionnaire(questionnaire); 1282 this.setStatus(status); 1283 } 1284 1285 /** 1286 * @return {@link #identifier} (Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 1287 */ 1288 public List<Identifier> getIdentifier() { 1289 if (this.identifier == null) 1290 this.identifier = new ArrayList<Identifier>(); 1291 return this.identifier; 1292 } 1293 1294 /** 1295 * @return Returns a reference to <code>this</code> for easy method chaining 1296 */ 1297 public QuestionnaireResponse setIdentifier(List<Identifier> theIdentifier) { 1298 this.identifier = theIdentifier; 1299 return this; 1300 } 1301 1302 public boolean hasIdentifier() { 1303 if (this.identifier == null) 1304 return false; 1305 for (Identifier item : this.identifier) 1306 if (!item.isEmpty()) 1307 return true; 1308 return false; 1309 } 1310 1311 public Identifier addIdentifier() { //3 1312 Identifier t = new Identifier(); 1313 if (this.identifier == null) 1314 this.identifier = new ArrayList<Identifier>(); 1315 this.identifier.add(t); 1316 return t; 1317 } 1318 1319 public QuestionnaireResponse addIdentifier(Identifier t) { //3 1320 if (t == null) 1321 return this; 1322 if (this.identifier == null) 1323 this.identifier = new ArrayList<Identifier>(); 1324 this.identifier.add(t); 1325 return this; 1326 } 1327 1328 /** 1329 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1330 */ 1331 public Identifier getIdentifierFirstRep() { 1332 if (getIdentifier().isEmpty()) { 1333 addIdentifier(); 1334 } 1335 return getIdentifier().get(0); 1336 } 1337 1338 /** 1339 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.) 1340 */ 1341 public List<Reference> getBasedOn() { 1342 if (this.basedOn == null) 1343 this.basedOn = new ArrayList<Reference>(); 1344 return this.basedOn; 1345 } 1346 1347 /** 1348 * @return Returns a reference to <code>this</code> for easy method chaining 1349 */ 1350 public QuestionnaireResponse setBasedOn(List<Reference> theBasedOn) { 1351 this.basedOn = theBasedOn; 1352 return this; 1353 } 1354 1355 public boolean hasBasedOn() { 1356 if (this.basedOn == null) 1357 return false; 1358 for (Reference item : this.basedOn) 1359 if (!item.isEmpty()) 1360 return true; 1361 return false; 1362 } 1363 1364 public Reference addBasedOn() { //3 1365 Reference t = new Reference(); 1366 if (this.basedOn == null) 1367 this.basedOn = new ArrayList<Reference>(); 1368 this.basedOn.add(t); 1369 return t; 1370 } 1371 1372 public QuestionnaireResponse addBasedOn(Reference t) { //3 1373 if (t == null) 1374 return this; 1375 if (this.basedOn == null) 1376 this.basedOn = new ArrayList<Reference>(); 1377 this.basedOn.add(t); 1378 return this; 1379 } 1380 1381 /** 1382 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1383 */ 1384 public Reference getBasedOnFirstRep() { 1385 if (getBasedOn().isEmpty()) { 1386 addBasedOn(); 1387 } 1388 return getBasedOn().get(0); 1389 } 1390 1391 /** 1392 * @return {@link #partOf} (A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.) 1393 */ 1394 public List<Reference> getPartOf() { 1395 if (this.partOf == null) 1396 this.partOf = new ArrayList<Reference>(); 1397 return this.partOf; 1398 } 1399 1400 /** 1401 * @return Returns a reference to <code>this</code> for easy method chaining 1402 */ 1403 public QuestionnaireResponse setPartOf(List<Reference> thePartOf) { 1404 this.partOf = thePartOf; 1405 return this; 1406 } 1407 1408 public boolean hasPartOf() { 1409 if (this.partOf == null) 1410 return false; 1411 for (Reference item : this.partOf) 1412 if (!item.isEmpty()) 1413 return true; 1414 return false; 1415 } 1416 1417 public Reference addPartOf() { //3 1418 Reference t = new Reference(); 1419 if (this.partOf == null) 1420 this.partOf = new ArrayList<Reference>(); 1421 this.partOf.add(t); 1422 return t; 1423 } 1424 1425 public QuestionnaireResponse addPartOf(Reference t) { //3 1426 if (t == null) 1427 return this; 1428 if (this.partOf == null) 1429 this.partOf = new ArrayList<Reference>(); 1430 this.partOf.add(t); 1431 return this; 1432 } 1433 1434 /** 1435 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 1436 */ 1437 public Reference getPartOfFirstRep() { 1438 if (getPartOf().isEmpty()) { 1439 addPartOf(); 1440 } 1441 return getPartOf().get(0); 1442 } 1443 1444 /** 1445 * @return {@link #questionnaire} (The Questionnaire that defines and organizes the questions for which answers are being provided.). This is the underlying object with id, value and extensions. The accessor "getQuestionnaire" gives direct access to the value 1446 */ 1447 public CanonicalType getQuestionnaireElement() { 1448 if (this.questionnaire == null) 1449 if (Configuration.errorOnAutoCreate()) 1450 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1451 else if (Configuration.doAutoCreate()) 1452 this.questionnaire = new CanonicalType(); // bb 1453 return this.questionnaire; 1454 } 1455 1456 public boolean hasQuestionnaireElement() { 1457 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1458 } 1459 1460 public boolean hasQuestionnaire() { 1461 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1462 } 1463 1464 /** 1465 * @param value {@link #questionnaire} (The Questionnaire that defines and organizes the questions for which answers are being provided.). This is the underlying object with id, value and extensions. The accessor "getQuestionnaire" gives direct access to the value 1466 */ 1467 public QuestionnaireResponse setQuestionnaireElement(CanonicalType value) { 1468 this.questionnaire = value; 1469 return this; 1470 } 1471 1472 /** 1473 * @return The Questionnaire that defines and organizes the questions for which answers are being provided. 1474 */ 1475 public String getQuestionnaire() { 1476 return this.questionnaire == null ? null : this.questionnaire.getValue(); 1477 } 1478 1479 /** 1480 * @param value The Questionnaire that defines and organizes the questions for which answers are being provided. 1481 */ 1482 public QuestionnaireResponse setQuestionnaire(String value) { 1483 if (this.questionnaire == null) 1484 this.questionnaire = new CanonicalType(); 1485 this.questionnaire.setValue(value); 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #status} (The current state of the questionnaire response.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1491 */ 1492 public Enumeration<QuestionnaireResponseStatus> getStatusElement() { 1493 if (this.status == null) 1494 if (Configuration.errorOnAutoCreate()) 1495 throw new Error("Attempt to auto-create QuestionnaireResponse.status"); 1496 else if (Configuration.doAutoCreate()) 1497 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); // bb 1498 return this.status; 1499 } 1500 1501 public boolean hasStatusElement() { 1502 return this.status != null && !this.status.isEmpty(); 1503 } 1504 1505 public boolean hasStatus() { 1506 return this.status != null && !this.status.isEmpty(); 1507 } 1508 1509 /** 1510 * @param value {@link #status} (The current state of the questionnaire response.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1511 */ 1512 public QuestionnaireResponse setStatusElement(Enumeration<QuestionnaireResponseStatus> value) { 1513 this.status = value; 1514 return this; 1515 } 1516 1517 /** 1518 * @return The current state of the questionnaire response. 1519 */ 1520 public QuestionnaireResponseStatus getStatus() { 1521 return this.status == null ? null : this.status.getValue(); 1522 } 1523 1524 /** 1525 * @param value The current state of the questionnaire response. 1526 */ 1527 public QuestionnaireResponse setStatus(QuestionnaireResponseStatus value) { 1528 if (this.status == null) 1529 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); 1530 this.status.setValue(value); 1531 return this; 1532 } 1533 1534 /** 1535 * @return {@link #subject} (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1536 */ 1537 public Reference getSubject() { 1538 if (this.subject == null) 1539 if (Configuration.errorOnAutoCreate()) 1540 throw new Error("Attempt to auto-create QuestionnaireResponse.subject"); 1541 else if (Configuration.doAutoCreate()) 1542 this.subject = new Reference(); // cc 1543 return this.subject; 1544 } 1545 1546 public boolean hasSubject() { 1547 return this.subject != null && !this.subject.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #subject} (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1552 */ 1553 public QuestionnaireResponse setSubject(Reference value) { 1554 this.subject = value; 1555 return this; 1556 } 1557 1558 /** 1559 * @return {@link #encounter} (The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.) 1560 */ 1561 public Reference getEncounter() { 1562 if (this.encounter == null) 1563 if (Configuration.errorOnAutoCreate()) 1564 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1565 else if (Configuration.doAutoCreate()) 1566 this.encounter = new Reference(); // cc 1567 return this.encounter; 1568 } 1569 1570 public boolean hasEncounter() { 1571 return this.encounter != null && !this.encounter.isEmpty(); 1572 } 1573 1574 /** 1575 * @param value {@link #encounter} (The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.) 1576 */ 1577 public QuestionnaireResponse setEncounter(Reference value) { 1578 this.encounter = value; 1579 return this; 1580 } 1581 1582 /** 1583 * @return {@link #authored} (The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.). This is the underlying object with id, value and extensions. The accessor "getAuthored" gives direct access to the value 1584 */ 1585 public DateTimeType getAuthoredElement() { 1586 if (this.authored == null) 1587 if (Configuration.errorOnAutoCreate()) 1588 throw new Error("Attempt to auto-create QuestionnaireResponse.authored"); 1589 else if (Configuration.doAutoCreate()) 1590 this.authored = new DateTimeType(); // bb 1591 return this.authored; 1592 } 1593 1594 public boolean hasAuthoredElement() { 1595 return this.authored != null && !this.authored.isEmpty(); 1596 } 1597 1598 public boolean hasAuthored() { 1599 return this.authored != null && !this.authored.isEmpty(); 1600 } 1601 1602 /** 1603 * @param value {@link #authored} (The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.). This is the underlying object with id, value and extensions. The accessor "getAuthored" gives direct access to the value 1604 */ 1605 public QuestionnaireResponse setAuthoredElement(DateTimeType value) { 1606 this.authored = value; 1607 return this; 1608 } 1609 1610 /** 1611 * @return The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status. 1612 */ 1613 public Date getAuthored() { 1614 return this.authored == null ? null : this.authored.getValue(); 1615 } 1616 1617 /** 1618 * @param value The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status. 1619 */ 1620 public QuestionnaireResponse setAuthored(Date value) { 1621 if (value == null) 1622 this.authored = null; 1623 else { 1624 if (this.authored == null) 1625 this.authored = new DateTimeType(); 1626 this.authored.setValue(value); 1627 } 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #author} (The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1633 */ 1634 public Reference getAuthor() { 1635 if (this.author == null) 1636 if (Configuration.errorOnAutoCreate()) 1637 throw new Error("Attempt to auto-create QuestionnaireResponse.author"); 1638 else if (Configuration.doAutoCreate()) 1639 this.author = new Reference(); // cc 1640 return this.author; 1641 } 1642 1643 public boolean hasAuthor() { 1644 return this.author != null && !this.author.isEmpty(); 1645 } 1646 1647 /** 1648 * @param value {@link #author} (The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1649 */ 1650 public QuestionnaireResponse setAuthor(Reference value) { 1651 this.author = value; 1652 return this; 1653 } 1654 1655 /** 1656 * @return {@link #source} (The individual or device that answered the questions about the subject.) 1657 */ 1658 public Reference getSource() { 1659 if (this.source == null) 1660 if (Configuration.errorOnAutoCreate()) 1661 throw new Error("Attempt to auto-create QuestionnaireResponse.source"); 1662 else if (Configuration.doAutoCreate()) 1663 this.source = new Reference(); // cc 1664 return this.source; 1665 } 1666 1667 public boolean hasSource() { 1668 return this.source != null && !this.source.isEmpty(); 1669 } 1670 1671 /** 1672 * @param value {@link #source} (The individual or device that answered the questions about the subject.) 1673 */ 1674 public QuestionnaireResponse setSource(Reference value) { 1675 this.source = value; 1676 return this; 1677 } 1678 1679 /** 1680 * @return {@link #item} (A group or question item from the original questionnaire for which answers are provided.) 1681 */ 1682 public List<QuestionnaireResponseItemComponent> getItem() { 1683 if (this.item == null) 1684 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1685 return this.item; 1686 } 1687 1688 /** 1689 * @return Returns a reference to <code>this</code> for easy method chaining 1690 */ 1691 public QuestionnaireResponse setItem(List<QuestionnaireResponseItemComponent> theItem) { 1692 this.item = theItem; 1693 return this; 1694 } 1695 1696 public boolean hasItem() { 1697 if (this.item == null) 1698 return false; 1699 for (QuestionnaireResponseItemComponent item : this.item) 1700 if (!item.isEmpty()) 1701 return true; 1702 return false; 1703 } 1704 1705 public QuestionnaireResponseItemComponent addItem() { //3 1706 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 1707 if (this.item == null) 1708 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1709 this.item.add(t); 1710 return t; 1711 } 1712 1713 public QuestionnaireResponse addItem(QuestionnaireResponseItemComponent t) { //3 1714 if (t == null) 1715 return this; 1716 if (this.item == null) 1717 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1718 this.item.add(t); 1719 return this; 1720 } 1721 1722 /** 1723 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 1724 */ 1725 public QuestionnaireResponseItemComponent getItemFirstRep() { 1726 if (getItem().isEmpty()) { 1727 addItem(); 1728 } 1729 return getItem().get(0); 1730 } 1731 1732 protected void listChildren(List<Property> children) { 1733 super.listChildren(children); 1734 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1735 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1736 children.add(new Property("partOf", "Reference(Observation|Procedure)", "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1737 children.add(new Property("questionnaire", "canonical(Questionnaire)", "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, questionnaire)); 1738 children.add(new Property("status", "code", "The current state of the questionnaire response.", 0, 1, status)); 1739 children.add(new Property("subject", "Reference(Any)", "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 0, 1, subject)); 1740 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 1741 children.add(new Property("authored", "dateTime", "The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.", 0, 1, authored)); 1742 children.add(new Property("author", "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", "The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 0, 1, author)); 1743 children.add(new Property("source", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The individual or device that answered the questions about the subject.", 0, 1, source)); 1744 children.add(new Property("item", "", "A group or question item from the original questionnaire for which answers are provided.", 0, java.lang.Integer.MAX_VALUE, item)); 1745 } 1746 1747 @Override 1748 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1749 switch (_hash) { 1750 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this questionnaire response by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1751 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this questionnaire response. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1752 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Observation|Procedure)", "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 0, java.lang.Integer.MAX_VALUE, partOf); 1753 case -1017049693: /*questionnaire*/ return new Property("questionnaire", "canonical(Questionnaire)", "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, questionnaire); 1754 case -892481550: /*status*/ return new Property("status", "code", "The current state of the questionnaire response.", 0, 1, status); 1755 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 0, 1, subject); 1756 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 1757 case 1433073514: /*authored*/ return new Property("authored", "dateTime", "The date and/or time that this questionnaire response was last modified by the user - e.g. changing answers or revising status.", 0, 1, authored); 1758 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", "The individual or device that received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 0, 1, author); 1759 case -896505829: /*source*/ return new Property("source", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The individual or device that answered the questions about the subject.", 0, 1, source); 1760 case 3242771: /*item*/ return new Property("item", "", "A group or question item from the original questionnaire for which answers are provided.", 0, java.lang.Integer.MAX_VALUE, item); 1761 default: return super.getNamedProperty(_hash, _name, _checkValid); 1762 } 1763 1764 } 1765 1766 @Override 1767 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1768 switch (hash) { 1769 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1770 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1771 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1772 case -1017049693: /*questionnaire*/ return this.questionnaire == null ? new Base[0] : new Base[] {this.questionnaire}; // CanonicalType 1773 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<QuestionnaireResponseStatus> 1774 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1775 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1776 case 1433073514: /*authored*/ return this.authored == null ? new Base[0] : new Base[] {this.authored}; // DateTimeType 1777 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1778 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1779 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1780 default: return super.getProperty(hash, name, checkValid); 1781 } 1782 1783 } 1784 1785 @Override 1786 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1787 switch (hash) { 1788 case -1618432855: // identifier 1789 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1790 return value; 1791 case -332612366: // basedOn 1792 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1793 return value; 1794 case -995410646: // partOf 1795 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1796 return value; 1797 case -1017049693: // questionnaire 1798 this.questionnaire = TypeConvertor.castToCanonical(value); // CanonicalType 1799 return value; 1800 case -892481550: // status 1801 value = new QuestionnaireResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1802 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 1803 return value; 1804 case -1867885268: // subject 1805 this.subject = TypeConvertor.castToReference(value); // Reference 1806 return value; 1807 case 1524132147: // encounter 1808 this.encounter = TypeConvertor.castToReference(value); // Reference 1809 return value; 1810 case 1433073514: // authored 1811 this.authored = TypeConvertor.castToDateTime(value); // DateTimeType 1812 return value; 1813 case -1406328437: // author 1814 this.author = TypeConvertor.castToReference(value); // Reference 1815 return value; 1816 case -896505829: // source 1817 this.source = TypeConvertor.castToReference(value); // Reference 1818 return value; 1819 case 3242771: // item 1820 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1821 return value; 1822 default: return super.setProperty(hash, name, value); 1823 } 1824 1825 } 1826 1827 @Override 1828 public Base setProperty(String name, Base value) throws FHIRException { 1829 if (name.equals("identifier")) { 1830 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1831 } else if (name.equals("basedOn")) { 1832 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1833 } else if (name.equals("partOf")) { 1834 this.getPartOf().add(TypeConvertor.castToReference(value)); 1835 } else if (name.equals("questionnaire")) { 1836 this.questionnaire = TypeConvertor.castToCanonical(value); // CanonicalType 1837 } else if (name.equals("status")) { 1838 value = new QuestionnaireResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1839 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 1840 } else if (name.equals("subject")) { 1841 this.subject = TypeConvertor.castToReference(value); // Reference 1842 } else if (name.equals("encounter")) { 1843 this.encounter = TypeConvertor.castToReference(value); // Reference 1844 } else if (name.equals("authored")) { 1845 this.authored = TypeConvertor.castToDateTime(value); // DateTimeType 1846 } else if (name.equals("author")) { 1847 this.author = TypeConvertor.castToReference(value); // Reference 1848 } else if (name.equals("source")) { 1849 this.source = TypeConvertor.castToReference(value); // Reference 1850 } else if (name.equals("item")) { 1851 this.getItem().add((QuestionnaireResponseItemComponent) value); 1852 } else 1853 return super.setProperty(name, value); 1854 return value; 1855 } 1856 1857 @Override 1858 public void removeChild(String name, Base value) throws FHIRException { 1859 if (name.equals("identifier")) { 1860 this.getIdentifier().remove(value); 1861 } else if (name.equals("basedOn")) { 1862 this.getBasedOn().remove(value); 1863 } else if (name.equals("partOf")) { 1864 this.getPartOf().remove(value); 1865 } else if (name.equals("questionnaire")) { 1866 this.questionnaire = null; 1867 } else if (name.equals("status")) { 1868 value = new QuestionnaireResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1869 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 1870 } else if (name.equals("subject")) { 1871 this.subject = null; 1872 } else if (name.equals("encounter")) { 1873 this.encounter = null; 1874 } else if (name.equals("authored")) { 1875 this.authored = null; 1876 } else if (name.equals("author")) { 1877 this.author = null; 1878 } else if (name.equals("source")) { 1879 this.source = null; 1880 } else if (name.equals("item")) { 1881 this.getItem().remove((QuestionnaireResponseItemComponent) value); 1882 } else 1883 super.removeChild(name, value); 1884 1885 } 1886 1887 @Override 1888 public Base makeProperty(int hash, String name) throws FHIRException { 1889 switch (hash) { 1890 case -1618432855: return addIdentifier(); 1891 case -332612366: return addBasedOn(); 1892 case -995410646: return addPartOf(); 1893 case -1017049693: return getQuestionnaireElement(); 1894 case -892481550: return getStatusElement(); 1895 case -1867885268: return getSubject(); 1896 case 1524132147: return getEncounter(); 1897 case 1433073514: return getAuthoredElement(); 1898 case -1406328437: return getAuthor(); 1899 case -896505829: return getSource(); 1900 case 3242771: return addItem(); 1901 default: return super.makeProperty(hash, name); 1902 } 1903 1904 } 1905 1906 @Override 1907 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1908 switch (hash) { 1909 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1910 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1911 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1912 case -1017049693: /*questionnaire*/ return new String[] {"canonical"}; 1913 case -892481550: /*status*/ return new String[] {"code"}; 1914 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1915 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1916 case 1433073514: /*authored*/ return new String[] {"dateTime"}; 1917 case -1406328437: /*author*/ return new String[] {"Reference"}; 1918 case -896505829: /*source*/ return new String[] {"Reference"}; 1919 case 3242771: /*item*/ return new String[] {}; 1920 default: return super.getTypesForProperty(hash, name); 1921 } 1922 1923 } 1924 1925 @Override 1926 public Base addChild(String name) throws FHIRException { 1927 if (name.equals("identifier")) { 1928 return addIdentifier(); 1929 } 1930 else if (name.equals("basedOn")) { 1931 return addBasedOn(); 1932 } 1933 else if (name.equals("partOf")) { 1934 return addPartOf(); 1935 } 1936 else if (name.equals("questionnaire")) { 1937 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.questionnaire"); 1938 } 1939 else if (name.equals("status")) { 1940 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.status"); 1941 } 1942 else if (name.equals("subject")) { 1943 this.subject = new Reference(); 1944 return this.subject; 1945 } 1946 else if (name.equals("encounter")) { 1947 this.encounter = new Reference(); 1948 return this.encounter; 1949 } 1950 else if (name.equals("authored")) { 1951 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.authored"); 1952 } 1953 else if (name.equals("author")) { 1954 this.author = new Reference(); 1955 return this.author; 1956 } 1957 else if (name.equals("source")) { 1958 this.source = new Reference(); 1959 return this.source; 1960 } 1961 else if (name.equals("item")) { 1962 return addItem(); 1963 } 1964 else 1965 return super.addChild(name); 1966 } 1967 1968 public String fhirType() { 1969 return "QuestionnaireResponse"; 1970 1971 } 1972 1973 public QuestionnaireResponse copy() { 1974 QuestionnaireResponse dst = new QuestionnaireResponse(); 1975 copyValues(dst); 1976 return dst; 1977 } 1978 1979 public void copyValues(QuestionnaireResponse dst) { 1980 super.copyValues(dst); 1981 if (identifier != null) { 1982 dst.identifier = new ArrayList<Identifier>(); 1983 for (Identifier i : identifier) 1984 dst.identifier.add(i.copy()); 1985 }; 1986 if (basedOn != null) { 1987 dst.basedOn = new ArrayList<Reference>(); 1988 for (Reference i : basedOn) 1989 dst.basedOn.add(i.copy()); 1990 }; 1991 if (partOf != null) { 1992 dst.partOf = new ArrayList<Reference>(); 1993 for (Reference i : partOf) 1994 dst.partOf.add(i.copy()); 1995 }; 1996 dst.questionnaire = questionnaire == null ? null : questionnaire.copy(); 1997 dst.status = status == null ? null : status.copy(); 1998 dst.subject = subject == null ? null : subject.copy(); 1999 dst.encounter = encounter == null ? null : encounter.copy(); 2000 dst.authored = authored == null ? null : authored.copy(); 2001 dst.author = author == null ? null : author.copy(); 2002 dst.source = source == null ? null : source.copy(); 2003 if (item != null) { 2004 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2005 for (QuestionnaireResponseItemComponent i : item) 2006 dst.item.add(i.copy()); 2007 }; 2008 } 2009 2010 protected QuestionnaireResponse typedCopy() { 2011 return copy(); 2012 } 2013 2014 @Override 2015 public boolean equalsDeep(Base other_) { 2016 if (!super.equalsDeep(other_)) 2017 return false; 2018 if (!(other_ instanceof QuestionnaireResponse)) 2019 return false; 2020 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2021 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 2022 && compareDeep(questionnaire, o.questionnaire, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 2023 && compareDeep(encounter, o.encounter, true) && compareDeep(authored, o.authored, true) && compareDeep(author, o.author, true) 2024 && compareDeep(source, o.source, true) && compareDeep(item, o.item, true); 2025 } 2026 2027 @Override 2028 public boolean equalsShallow(Base other_) { 2029 if (!super.equalsShallow(other_)) 2030 return false; 2031 if (!(other_ instanceof QuestionnaireResponse)) 2032 return false; 2033 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2034 return compareValues(questionnaire, o.questionnaire, true) && compareValues(status, o.status, true) 2035 && compareValues(authored, o.authored, true); 2036 } 2037 2038 public boolean isEmpty() { 2039 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 2040 , questionnaire, status, subject, encounter, authored, author, source, item 2041 ); 2042 } 2043 2044 @Override 2045 public ResourceType getResourceType() { 2046 return ResourceType.QuestionnaireResponse; 2047 } 2048 2049 /** 2050 * Search parameter: <b>author</b> 2051 * <p> 2052 * Description: <b>The author of the questionnaire response</b><br> 2053 * Type: <b>reference</b><br> 2054 * Path: <b>QuestionnaireResponse.author</b><br> 2055 * </p> 2056 */ 2057 @SearchParamDefinition(name="author", path="QuestionnaireResponse.author", description="The author of the questionnaire response", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2058 public static final String SP_AUTHOR = "author"; 2059 /** 2060 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2061 * <p> 2062 * Description: <b>The author of the questionnaire response</b><br> 2063 * Type: <b>reference</b><br> 2064 * Path: <b>QuestionnaireResponse.author</b><br> 2065 * </p> 2066 */ 2067 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2068 2069/** 2070 * Constant for fluent queries to be used to add include statements. Specifies 2071 * the path value of "<b>QuestionnaireResponse:author</b>". 2072 */ 2073 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:author").toLocked(); 2074 2075 /** 2076 * Search parameter: <b>authored</b> 2077 * <p> 2078 * Description: <b>When the questionnaire response was last changed</b><br> 2079 * Type: <b>date</b><br> 2080 * Path: <b>QuestionnaireResponse.authored</b><br> 2081 * </p> 2082 */ 2083 @SearchParamDefinition(name="authored", path="QuestionnaireResponse.authored", description="When the questionnaire response was last changed", type="date" ) 2084 public static final String SP_AUTHORED = "authored"; 2085 /** 2086 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2087 * <p> 2088 * Description: <b>When the questionnaire response was last changed</b><br> 2089 * Type: <b>date</b><br> 2090 * Path: <b>QuestionnaireResponse.authored</b><br> 2091 * </p> 2092 */ 2093 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 2094 2095 /** 2096 * Search parameter: <b>based-on</b> 2097 * <p> 2098 * Description: <b>Plan/proposal/order fulfilled by this questionnaire response</b><br> 2099 * Type: <b>reference</b><br> 2100 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2101 * </p> 2102 */ 2103 @SearchParamDefinition(name="based-on", path="QuestionnaireResponse.basedOn", description="Plan/proposal/order fulfilled by this questionnaire response", type="reference", target={CarePlan.class, ServiceRequest.class } ) 2104 public static final String SP_BASED_ON = "based-on"; 2105 /** 2106 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2107 * <p> 2108 * Description: <b>Plan/proposal/order fulfilled by this questionnaire response</b><br> 2109 * Type: <b>reference</b><br> 2110 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2111 * </p> 2112 */ 2113 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2114 2115/** 2116 * Constant for fluent queries to be used to add include statements. Specifies 2117 * the path value of "<b>QuestionnaireResponse:based-on</b>". 2118 */ 2119 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:based-on").toLocked(); 2120 2121 /** 2122 * Search parameter: <b>item-subject</b> 2123 * <p> 2124 * Description: <b>Allows searching for QuestionnaireResponses by item value where the item has isSubject=true</b><br> 2125 * Type: <b>reference</b><br> 2126 * Path: <b>QuestionnaireResponse.item.where(extension('http://hl7.org/fhir/StructureDefinition/questionnaireresponse-isSubject').exists()).answer.value.ofType(Reference)</b><br> 2127 * </p> 2128 */ 2129 @SearchParamDefinition(name="item-subject", path="QuestionnaireResponse.item.where(extension('http://hl7.org/fhir/StructureDefinition/questionnaireresponse-isSubject').exists()).answer.value.ofType(Reference)", description="Allows searching for QuestionnaireResponses by item value where the item has isSubject=true", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2130 public static final String SP_ITEM_SUBJECT = "item-subject"; 2131 /** 2132 * <b>Fluent Client</b> search parameter constant for <b>item-subject</b> 2133 * <p> 2134 * Description: <b>Allows searching for QuestionnaireResponses by item value where the item has isSubject=true</b><br> 2135 * Type: <b>reference</b><br> 2136 * Path: <b>QuestionnaireResponse.item.where(extension('http://hl7.org/fhir/StructureDefinition/questionnaireresponse-isSubject').exists()).answer.value.ofType(Reference)</b><br> 2137 * </p> 2138 */ 2139 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_SUBJECT); 2140 2141/** 2142 * Constant for fluent queries to be used to add include statements. Specifies 2143 * the path value of "<b>QuestionnaireResponse:item-subject</b>". 2144 */ 2145 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_SUBJECT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:item-subject").toLocked(); 2146 2147 /** 2148 * Search parameter: <b>part-of</b> 2149 * <p> 2150 * Description: <b>Procedure or observation this questionnaire response was performed as a part of</b><br> 2151 * Type: <b>reference</b><br> 2152 * Path: <b>QuestionnaireResponse.partOf</b><br> 2153 * </p> 2154 */ 2155 @SearchParamDefinition(name="part-of", path="QuestionnaireResponse.partOf", description="Procedure or observation this questionnaire response was performed as a part of", type="reference", target={Observation.class, Procedure.class } ) 2156 public static final String SP_PART_OF = "part-of"; 2157 /** 2158 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2159 * <p> 2160 * Description: <b>Procedure or observation this questionnaire response was performed as a part of</b><br> 2161 * Type: <b>reference</b><br> 2162 * Path: <b>QuestionnaireResponse.partOf</b><br> 2163 * </p> 2164 */ 2165 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2166 2167/** 2168 * Constant for fluent queries to be used to add include statements. Specifies 2169 * the path value of "<b>QuestionnaireResponse:part-of</b>". 2170 */ 2171 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:part-of").toLocked(); 2172 2173 /** 2174 * Search parameter: <b>questionnaire</b> 2175 * <p> 2176 * Description: <b>The questionnaire the answers are provided for</b><br> 2177 * Type: <b>reference</b><br> 2178 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2179 * </p> 2180 */ 2181 @SearchParamDefinition(name="questionnaire", path="QuestionnaireResponse.questionnaire", description="The questionnaire the answers are provided for", type="reference", target={Questionnaire.class } ) 2182 public static final String SP_QUESTIONNAIRE = "questionnaire"; 2183 /** 2184 * <b>Fluent Client</b> search parameter constant for <b>questionnaire</b> 2185 * <p> 2186 * Description: <b>The questionnaire the answers are provided for</b><br> 2187 * Type: <b>reference</b><br> 2188 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2189 * </p> 2190 */ 2191 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam QUESTIONNAIRE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_QUESTIONNAIRE); 2192 2193/** 2194 * Constant for fluent queries to be used to add include statements. Specifies 2195 * the path value of "<b>QuestionnaireResponse:questionnaire</b>". 2196 */ 2197 public static final ca.uhn.fhir.model.api.Include INCLUDE_QUESTIONNAIRE = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:questionnaire").toLocked(); 2198 2199 /** 2200 * Search parameter: <b>source</b> 2201 * <p> 2202 * Description: <b>The individual providing the information reflected in the questionnaire respose</b><br> 2203 * Type: <b>reference</b><br> 2204 * Path: <b>QuestionnaireResponse.source</b><br> 2205 * </p> 2206 */ 2207 @SearchParamDefinition(name="source", path="QuestionnaireResponse.source", description="The individual providing the information reflected in the questionnaire respose", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2208 public static final String SP_SOURCE = "source"; 2209 /** 2210 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2211 * <p> 2212 * Description: <b>The individual providing the information reflected in the questionnaire respose</b><br> 2213 * Type: <b>reference</b><br> 2214 * Path: <b>QuestionnaireResponse.source</b><br> 2215 * </p> 2216 */ 2217 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 2218 2219/** 2220 * Constant for fluent queries to be used to add include statements. Specifies 2221 * the path value of "<b>QuestionnaireResponse:source</b>". 2222 */ 2223 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:source").toLocked(); 2224 2225 /** 2226 * Search parameter: <b>status</b> 2227 * <p> 2228 * Description: <b>The status of the questionnaire response</b><br> 2229 * Type: <b>token</b><br> 2230 * Path: <b>QuestionnaireResponse.status</b><br> 2231 * </p> 2232 */ 2233 @SearchParamDefinition(name="status", path="QuestionnaireResponse.status", description="The status of the questionnaire response", type="token" ) 2234 public static final String SP_STATUS = "status"; 2235 /** 2236 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2237 * <p> 2238 * Description: <b>The status of the questionnaire response</b><br> 2239 * Type: <b>token</b><br> 2240 * Path: <b>QuestionnaireResponse.status</b><br> 2241 * </p> 2242 */ 2243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2244 2245 /** 2246 * Search parameter: <b>subject</b> 2247 * <p> 2248 * Description: <b>The subject of the questionnaire response</b><br> 2249 * Type: <b>reference</b><br> 2250 * Path: <b>QuestionnaireResponse.subject</b><br> 2251 * </p> 2252 */ 2253 @SearchParamDefinition(name="subject", path="QuestionnaireResponse.subject", description="The subject of the questionnaire response", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2254 public static final String SP_SUBJECT = "subject"; 2255 /** 2256 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2257 * <p> 2258 * Description: <b>The subject of the questionnaire response</b><br> 2259 * Type: <b>reference</b><br> 2260 * Path: <b>QuestionnaireResponse.subject</b><br> 2261 * </p> 2262 */ 2263 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2264 2265/** 2266 * Constant for fluent queries to be used to add include statements. Specifies 2267 * the path value of "<b>QuestionnaireResponse:subject</b>". 2268 */ 2269 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:subject").toLocked(); 2270 2271 /** 2272 * Search parameter: <b>encounter</b> 2273 * <p> 2274 * Description: <b>Multiple Resources: 2275 2276* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2277* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2278* [ChargeItem](chargeitem.html): Encounter associated with event 2279* [Claim](claim.html): Encounters associated with a billed line item 2280* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2281* [Communication](communication.html): The Encounter during which this Communication was created 2282* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2283* [Composition](composition.html): Context of the Composition 2284* [Condition](condition.html): The Encounter during which this Condition was created 2285* [DeviceRequest](devicerequest.html): Encounter during which request was created 2286* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2287* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2288* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2289* [Flag](flag.html): Alert relevant during encounter 2290* [ImagingStudy](imagingstudy.html): The context of the study 2291* [List](list.html): Context in which list created 2292* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2293* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2294* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2295* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2296* [Observation](observation.html): Encounter related to the observation 2297* [Procedure](procedure.html): The Encounter during which this Procedure was created 2298* [Provenance](provenance.html): Encounter related to the Provenance 2299* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2300* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2301* [RiskAssessment](riskassessment.html): Where was assessment performed? 2302* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2303* [Task](task.html): Search by encounter 2304* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2305</b><br> 2306 * Type: <b>reference</b><br> 2307 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2308 * </p> 2309 */ 2310 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2311 public static final String SP_ENCOUNTER = "encounter"; 2312 /** 2313 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2314 * <p> 2315 * Description: <b>Multiple Resources: 2316 2317* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2318* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2319* [ChargeItem](chargeitem.html): Encounter associated with event 2320* [Claim](claim.html): Encounters associated with a billed line item 2321* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2322* [Communication](communication.html): The Encounter during which this Communication was created 2323* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2324* [Composition](composition.html): Context of the Composition 2325* [Condition](condition.html): The Encounter during which this Condition was created 2326* [DeviceRequest](devicerequest.html): Encounter during which request was created 2327* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2328* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2329* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2330* [Flag](flag.html): Alert relevant during encounter 2331* [ImagingStudy](imagingstudy.html): The context of the study 2332* [List](list.html): Context in which list created 2333* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2334* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2335* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2336* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2337* [Observation](observation.html): Encounter related to the observation 2338* [Procedure](procedure.html): The Encounter during which this Procedure was created 2339* [Provenance](provenance.html): Encounter related to the Provenance 2340* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2341* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2342* [RiskAssessment](riskassessment.html): Where was assessment performed? 2343* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2344* [Task](task.html): Search by encounter 2345* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2346</b><br> 2347 * Type: <b>reference</b><br> 2348 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2349 * </p> 2350 */ 2351 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2352 2353/** 2354 * Constant for fluent queries to be used to add include statements. Specifies 2355 * the path value of "<b>QuestionnaireResponse:encounter</b>". 2356 */ 2357 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:encounter").toLocked(); 2358 2359 /** 2360 * Search parameter: <b>identifier</b> 2361 * <p> 2362 * Description: <b>Multiple Resources: 2363 2364* [Account](account.html): Account number 2365* [AdverseEvent](adverseevent.html): Business identifier for the event 2366* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2367* [Appointment](appointment.html): An Identifier of the Appointment 2368* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2369* [Basic](basic.html): Business identifier 2370* [BodyStructure](bodystructure.html): Bodystructure identifier 2371* [CarePlan](careplan.html): External Ids for this plan 2372* [CareTeam](careteam.html): External Ids for this team 2373* [ChargeItem](chargeitem.html): Business Identifier for item 2374* [Claim](claim.html): The primary identifier of the financial resource 2375* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2376* [ClinicalImpression](clinicalimpression.html): Business identifier 2377* [Communication](communication.html): Unique identifier 2378* [CommunicationRequest](communicationrequest.html): Unique identifier 2379* [Composition](composition.html): Version-independent identifier for the Composition 2380* [Condition](condition.html): A unique identifier of the condition record 2381* [Consent](consent.html): Identifier for this record (external references) 2382* [Contract](contract.html): The identity of the contract 2383* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2384* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2385* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2386* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2387* [DeviceRequest](devicerequest.html): Business identifier for request/order 2388* [DeviceUsage](deviceusage.html): Search by identifier 2389* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2390* [DocumentReference](documentreference.html): Identifier of the attachment binary 2391* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2392* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2393* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2394* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2395* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2396* [Flag](flag.html): Business identifier 2397* [Goal](goal.html): External Ids for this goal 2398* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2399* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2400* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2401* [Immunization](immunization.html): Business identifier 2402* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2403* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2404* [Invoice](invoice.html): Business Identifier for item 2405* [List](list.html): Business identifier 2406* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2407* [Medication](medication.html): Returns medications with this external identifier 2408* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2409* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2410* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2411* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2412* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2413* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2414* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2415* [Observation](observation.html): The unique id for a particular observation 2416* [Person](person.html): A person Identifier 2417* [Procedure](procedure.html): A unique identifier for a procedure 2418* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2419* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2420* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2421* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2422* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2423* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2424* [Specimen](specimen.html): The unique identifier associated with the specimen 2425* [SupplyDelivery](supplydelivery.html): External identifier 2426* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2427* [Task](task.html): Search for a task instance by its business identifier 2428* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2429</b><br> 2430 * Type: <b>token</b><br> 2431 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2432 * </p> 2433 */ 2434 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2435 public static final String SP_IDENTIFIER = "identifier"; 2436 /** 2437 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2438 * <p> 2439 * Description: <b>Multiple Resources: 2440 2441* [Account](account.html): Account number 2442* [AdverseEvent](adverseevent.html): Business identifier for the event 2443* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2444* [Appointment](appointment.html): An Identifier of the Appointment 2445* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2446* [Basic](basic.html): Business identifier 2447* [BodyStructure](bodystructure.html): Bodystructure identifier 2448* [CarePlan](careplan.html): External Ids for this plan 2449* [CareTeam](careteam.html): External Ids for this team 2450* [ChargeItem](chargeitem.html): Business Identifier for item 2451* [Claim](claim.html): The primary identifier of the financial resource 2452* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2453* [ClinicalImpression](clinicalimpression.html): Business identifier 2454* [Communication](communication.html): Unique identifier 2455* [CommunicationRequest](communicationrequest.html): Unique identifier 2456* [Composition](composition.html): Version-independent identifier for the Composition 2457* [Condition](condition.html): A unique identifier of the condition record 2458* [Consent](consent.html): Identifier for this record (external references) 2459* [Contract](contract.html): The identity of the contract 2460* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2461* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2462* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2463* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2464* [DeviceRequest](devicerequest.html): Business identifier for request/order 2465* [DeviceUsage](deviceusage.html): Search by identifier 2466* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2467* [DocumentReference](documentreference.html): Identifier of the attachment binary 2468* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2469* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2470* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2471* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2472* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2473* [Flag](flag.html): Business identifier 2474* [Goal](goal.html): External Ids for this goal 2475* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2476* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2477* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2478* [Immunization](immunization.html): Business identifier 2479* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2480* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2481* [Invoice](invoice.html): Business Identifier for item 2482* [List](list.html): Business identifier 2483* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2484* [Medication](medication.html): Returns medications with this external identifier 2485* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2486* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2487* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2488* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2489* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2490* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2491* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2492* [Observation](observation.html): The unique id for a particular observation 2493* [Person](person.html): A person Identifier 2494* [Procedure](procedure.html): A unique identifier for a procedure 2495* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2496* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2497* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2498* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2499* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2500* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2501* [Specimen](specimen.html): The unique identifier associated with the specimen 2502* [SupplyDelivery](supplydelivery.html): External identifier 2503* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2504* [Task](task.html): Search for a task instance by its business identifier 2505* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2506</b><br> 2507 * Type: <b>token</b><br> 2508 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2509 * </p> 2510 */ 2511 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2512 2513 /** 2514 * Search parameter: <b>patient</b> 2515 * <p> 2516 * Description: <b>Multiple Resources: 2517 2518* [Account](account.html): The entity that caused the expenses 2519* [AdverseEvent](adverseevent.html): Subject impacted by event 2520* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2521* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2522* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2523* [AuditEvent](auditevent.html): Where the activity involved patient data 2524* [Basic](basic.html): Identifies the focus of this resource 2525* [BodyStructure](bodystructure.html): Who this is about 2526* [CarePlan](careplan.html): Who the care plan is for 2527* [CareTeam](careteam.html): Who care team is for 2528* [ChargeItem](chargeitem.html): Individual service was done for/to 2529* [Claim](claim.html): Patient receiving the products or services 2530* [ClaimResponse](claimresponse.html): The subject of care 2531* [ClinicalImpression](clinicalimpression.html): Patient assessed 2532* [Communication](communication.html): Focus of message 2533* [CommunicationRequest](communicationrequest.html): Focus of message 2534* [Composition](composition.html): Who and/or what the composition is about 2535* [Condition](condition.html): Who has the condition? 2536* [Consent](consent.html): Who the consent applies to 2537* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2538* [Coverage](coverage.html): Retrieve coverages for a patient 2539* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2540* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2541* [DetectedIssue](detectedissue.html): Associated patient 2542* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2543* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2544* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2545* [DocumentReference](documentreference.html): Who/what is the subject of the document 2546* [Encounter](encounter.html): The patient present at the encounter 2547* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2548* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2549* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2550* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2551* [Flag](flag.html): The identity of a subject to list flags for 2552* [Goal](goal.html): Who this goal is intended for 2553* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2554* [ImagingSelection](imagingselection.html): Who the study is about 2555* [ImagingStudy](imagingstudy.html): Who the study is about 2556* [Immunization](immunization.html): The patient for the vaccination record 2557* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2558* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2559* [Invoice](invoice.html): Recipient(s) of goods and services 2560* [List](list.html): If all resources have the same subject 2561* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2562* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2563* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2564* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2565* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2566* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2567* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2568* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2569* [Observation](observation.html): The subject that the observation is about (if patient) 2570* [Person](person.html): The Person links to this Patient 2571* [Procedure](procedure.html): Search by subject - a patient 2572* [Provenance](provenance.html): Where the activity involved patient data 2573* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2574* [RelatedPerson](relatedperson.html): The patient this related person is related to 2575* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2576* [ResearchSubject](researchsubject.html): Who or what is part of study 2577* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2578* [ServiceRequest](servicerequest.html): Search by subject - a patient 2579* [Specimen](specimen.html): The patient the specimen comes from 2580* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2581* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2582* [Task](task.html): Search by patient 2583* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2584</b><br> 2585 * Type: <b>reference</b><br> 2586 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2587 * </p> 2588 */ 2589 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2590 public static final String SP_PATIENT = "patient"; 2591 /** 2592 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2593 * <p> 2594 * Description: <b>Multiple Resources: 2595 2596* [Account](account.html): The entity that caused the expenses 2597* [AdverseEvent](adverseevent.html): Subject impacted by event 2598* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2599* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2600* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2601* [AuditEvent](auditevent.html): Where the activity involved patient data 2602* [Basic](basic.html): Identifies the focus of this resource 2603* [BodyStructure](bodystructure.html): Who this is about 2604* [CarePlan](careplan.html): Who the care plan is for 2605* [CareTeam](careteam.html): Who care team is for 2606* [ChargeItem](chargeitem.html): Individual service was done for/to 2607* [Claim](claim.html): Patient receiving the products or services 2608* [ClaimResponse](claimresponse.html): The subject of care 2609* [ClinicalImpression](clinicalimpression.html): Patient assessed 2610* [Communication](communication.html): Focus of message 2611* [CommunicationRequest](communicationrequest.html): Focus of message 2612* [Composition](composition.html): Who and/or what the composition is about 2613* [Condition](condition.html): Who has the condition? 2614* [Consent](consent.html): Who the consent applies to 2615* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2616* [Coverage](coverage.html): Retrieve coverages for a patient 2617* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2618* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2619* [DetectedIssue](detectedissue.html): Associated patient 2620* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2621* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2622* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2623* [DocumentReference](documentreference.html): Who/what is the subject of the document 2624* [Encounter](encounter.html): The patient present at the encounter 2625* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2626* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2627* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2628* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2629* [Flag](flag.html): The identity of a subject to list flags for 2630* [Goal](goal.html): Who this goal is intended for 2631* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2632* [ImagingSelection](imagingselection.html): Who the study is about 2633* [ImagingStudy](imagingstudy.html): Who the study is about 2634* [Immunization](immunization.html): The patient for the vaccination record 2635* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2636* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2637* [Invoice](invoice.html): Recipient(s) of goods and services 2638* [List](list.html): If all resources have the same subject 2639* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2640* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2641* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2642* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2643* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2644* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2645* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2646* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2647* [Observation](observation.html): The subject that the observation is about (if patient) 2648* [Person](person.html): The Person links to this Patient 2649* [Procedure](procedure.html): Search by subject - a patient 2650* [Provenance](provenance.html): Where the activity involved patient data 2651* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2652* [RelatedPerson](relatedperson.html): The patient this related person is related to 2653* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2654* [ResearchSubject](researchsubject.html): Who or what is part of study 2655* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2656* [ServiceRequest](servicerequest.html): Search by subject - a patient 2657* [Specimen](specimen.html): The patient the specimen comes from 2658* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2659* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2660* [Task](task.html): Search by patient 2661* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2662</b><br> 2663 * Type: <b>reference</b><br> 2664 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2665 * </p> 2666 */ 2667 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2668 2669/** 2670 * Constant for fluent queries to be used to add include statements. Specifies 2671 * the path value of "<b>QuestionnaireResponse:patient</b>". 2672 */ 2673 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:patient").toLocked(); 2674 2675 2676} 2677