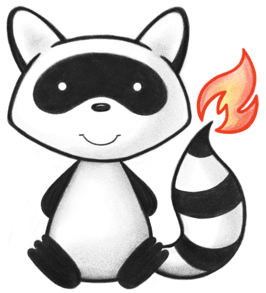
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047/** 048 * RatioRange Type: A range of ratios expressed as a low and high numerator and a denominator. 049 */ 050@DatatypeDef(name="RatioRange") 051public class RatioRange extends DataType implements ICompositeType { 052 053 /** 054 * The value of the low limit numerator. 055 */ 056 @Child(name = "lowNumerator", type = {Quantity.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="Low Numerator limit", formalDefinition="The value of the low limit numerator." ) 058 protected Quantity lowNumerator; 059 060 /** 061 * The value of the high limit numerator. 062 */ 063 @Child(name = "highNumerator", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="High Numerator limit", formalDefinition="The value of the high limit numerator." ) 065 protected Quantity highNumerator; 066 067 /** 068 * The value of the denominator. 069 */ 070 @Child(name = "denominator", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=true) 071 @Description(shortDefinition="Denominator value", formalDefinition="The value of the denominator." ) 072 protected Quantity denominator; 073 074 private static final long serialVersionUID = -1691080287L; 075 076 /** 077 * Constructor 078 */ 079 public RatioRange() { 080 super(); 081 } 082 083 /** 084 * @return {@link #lowNumerator} (The value of the low limit numerator.) 085 */ 086 public Quantity getLowNumerator() { 087 if (this.lowNumerator == null) 088 if (Configuration.errorOnAutoCreate()) 089 throw new Error("Attempt to auto-create RatioRange.lowNumerator"); 090 else if (Configuration.doAutoCreate()) 091 this.lowNumerator = new Quantity(); // cc 092 return this.lowNumerator; 093 } 094 095 public boolean hasLowNumerator() { 096 return this.lowNumerator != null && !this.lowNumerator.isEmpty(); 097 } 098 099 /** 100 * @param value {@link #lowNumerator} (The value of the low limit numerator.) 101 */ 102 public RatioRange setLowNumerator(Quantity value) { 103 this.lowNumerator = value; 104 return this; 105 } 106 107 /** 108 * @return {@link #highNumerator} (The value of the high limit numerator.) 109 */ 110 public Quantity getHighNumerator() { 111 if (this.highNumerator == null) 112 if (Configuration.errorOnAutoCreate()) 113 throw new Error("Attempt to auto-create RatioRange.highNumerator"); 114 else if (Configuration.doAutoCreate()) 115 this.highNumerator = new Quantity(); // cc 116 return this.highNumerator; 117 } 118 119 public boolean hasHighNumerator() { 120 return this.highNumerator != null && !this.highNumerator.isEmpty(); 121 } 122 123 /** 124 * @param value {@link #highNumerator} (The value of the high limit numerator.) 125 */ 126 public RatioRange setHighNumerator(Quantity value) { 127 this.highNumerator = value; 128 return this; 129 } 130 131 /** 132 * @return {@link #denominator} (The value of the denominator.) 133 */ 134 public Quantity getDenominator() { 135 if (this.denominator == null) 136 if (Configuration.errorOnAutoCreate()) 137 throw new Error("Attempt to auto-create RatioRange.denominator"); 138 else if (Configuration.doAutoCreate()) 139 this.denominator = new Quantity(); // cc 140 return this.denominator; 141 } 142 143 public boolean hasDenominator() { 144 return this.denominator != null && !this.denominator.isEmpty(); 145 } 146 147 /** 148 * @param value {@link #denominator} (The value of the denominator.) 149 */ 150 public RatioRange setDenominator(Quantity value) { 151 this.denominator = value; 152 return this; 153 } 154 155 protected void listChildren(List<Property> children) { 156 super.listChildren(children); 157 children.add(new Property("lowNumerator", "Quantity", "The value of the low limit numerator.", 0, 1, lowNumerator)); 158 children.add(new Property("highNumerator", "Quantity", "The value of the high limit numerator.", 0, 1, highNumerator)); 159 children.add(new Property("denominator", "Quantity", "The value of the denominator.", 0, 1, denominator)); 160 } 161 162 @Override 163 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 164 switch (_hash) { 165 case 1003958677: /*lowNumerator*/ return new Property("lowNumerator", "Quantity", "The value of the low limit numerator.", 0, 1, lowNumerator); 166 case 311013127: /*highNumerator*/ return new Property("highNumerator", "Quantity", "The value of the high limit numerator.", 0, 1, highNumerator); 167 case -1983274394: /*denominator*/ return new Property("denominator", "Quantity", "The value of the denominator.", 0, 1, denominator); 168 default: return super.getNamedProperty(_hash, _name, _checkValid); 169 } 170 171 } 172 173 @Override 174 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 175 switch (hash) { 176 case 1003958677: /*lowNumerator*/ return this.lowNumerator == null ? new Base[0] : new Base[] {this.lowNumerator}; // Quantity 177 case 311013127: /*highNumerator*/ return this.highNumerator == null ? new Base[0] : new Base[] {this.highNumerator}; // Quantity 178 case -1983274394: /*denominator*/ return this.denominator == null ? new Base[0] : new Base[] {this.denominator}; // Quantity 179 default: return super.getProperty(hash, name, checkValid); 180 } 181 182 } 183 184 @Override 185 public Base setProperty(int hash, String name, Base value) throws FHIRException { 186 switch (hash) { 187 case 1003958677: // lowNumerator 188 this.lowNumerator = TypeConvertor.castToQuantity(value); // Quantity 189 return value; 190 case 311013127: // highNumerator 191 this.highNumerator = TypeConvertor.castToQuantity(value); // Quantity 192 return value; 193 case -1983274394: // denominator 194 this.denominator = TypeConvertor.castToQuantity(value); // Quantity 195 return value; 196 default: return super.setProperty(hash, name, value); 197 } 198 199 } 200 201 @Override 202 public Base setProperty(String name, Base value) throws FHIRException { 203 if (name.equals("lowNumerator")) { 204 this.lowNumerator = TypeConvertor.castToQuantity(value); // Quantity 205 } else if (name.equals("highNumerator")) { 206 this.highNumerator = TypeConvertor.castToQuantity(value); // Quantity 207 } else if (name.equals("denominator")) { 208 this.denominator = TypeConvertor.castToQuantity(value); // Quantity 209 } else 210 return super.setProperty(name, value); 211 return value; 212 } 213 214 @Override 215 public void removeChild(String name, Base value) throws FHIRException { 216 if (name.equals("lowNumerator")) { 217 this.lowNumerator = null; 218 } else if (name.equals("highNumerator")) { 219 this.highNumerator = null; 220 } else if (name.equals("denominator")) { 221 this.denominator = null; 222 } else 223 super.removeChild(name, value); 224 225 } 226 227 @Override 228 public Base makeProperty(int hash, String name) throws FHIRException { 229 switch (hash) { 230 case 1003958677: return getLowNumerator(); 231 case 311013127: return getHighNumerator(); 232 case -1983274394: return getDenominator(); 233 default: return super.makeProperty(hash, name); 234 } 235 236 } 237 238 @Override 239 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 240 switch (hash) { 241 case 1003958677: /*lowNumerator*/ return new String[] {"Quantity"}; 242 case 311013127: /*highNumerator*/ return new String[] {"Quantity"}; 243 case -1983274394: /*denominator*/ return new String[] {"Quantity"}; 244 default: return super.getTypesForProperty(hash, name); 245 } 246 247 } 248 249 @Override 250 public Base addChild(String name) throws FHIRException { 251 if (name.equals("lowNumerator")) { 252 this.lowNumerator = new Quantity(); 253 return this.lowNumerator; 254 } 255 else if (name.equals("highNumerator")) { 256 this.highNumerator = new Quantity(); 257 return this.highNumerator; 258 } 259 else if (name.equals("denominator")) { 260 this.denominator = new Quantity(); 261 return this.denominator; 262 } 263 else 264 return super.addChild(name); 265 } 266 267 public String fhirType() { 268 return "RatioRange"; 269 270 } 271 272 public RatioRange copy() { 273 RatioRange dst = new RatioRange(); 274 copyValues(dst); 275 return dst; 276 } 277 278 public void copyValues(RatioRange dst) { 279 super.copyValues(dst); 280 dst.lowNumerator = lowNumerator == null ? null : lowNumerator.copy(); 281 dst.highNumerator = highNumerator == null ? null : highNumerator.copy(); 282 dst.denominator = denominator == null ? null : denominator.copy(); 283 } 284 285 protected RatioRange typedCopy() { 286 return copy(); 287 } 288 289 @Override 290 public boolean equalsDeep(Base other_) { 291 if (!super.equalsDeep(other_)) 292 return false; 293 if (!(other_ instanceof RatioRange)) 294 return false; 295 RatioRange o = (RatioRange) other_; 296 return compareDeep(lowNumerator, o.lowNumerator, true) && compareDeep(highNumerator, o.highNumerator, true) 297 && compareDeep(denominator, o.denominator, true); 298 } 299 300 @Override 301 public boolean equalsShallow(Base other_) { 302 if (!super.equalsShallow(other_)) 303 return false; 304 if (!(other_ instanceof RatioRange)) 305 return false; 306 RatioRange o = (RatioRange) other_; 307 return true; 308 } 309 310 public boolean isEmpty() { 311 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lowNumerator, highNumerator 312 , denominator); 313 } 314 315 316} 317